Dataset Viewer
repo
stringclasses 15
values | pull_number
int64 147
18.3k
| instance_id
stringlengths 14
31
| issue_numbers
sequencelengths 1
3
| base_commit
stringlengths 40
40
| patch
stringlengths 289
585k
| test_patch
stringlengths 355
6.82M
| problem_statement
stringlengths 25
49.4k
| hints_text
stringlengths 0
58.9k
| created_at
timestamp[us, tz=UTC]date 2014-08-08 22:09:38
2024-06-28 03:13:12
| version
stringclasses 110
values | PASS_TO_PASS
sequencelengths 0
4.82k
| FAIL_TO_PASS
sequencelengths 1
1.06k
| language
stringclasses 4
values | image_urls
sequencelengths 0
4
| website links
sequencelengths 0
4
|
---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|
tailwindlabs/tailwindcss | 10,059 | tailwindlabs__tailwindcss-10059 | [
"10052"
] | 40c0cbe272dab4904cbe9e2aaa200ebdb44a25fd | diff --git a/src/lib/generateRules.js b/src/lib/generateRules.js
--- a/src/lib/generateRules.js
+++ b/src/lib/generateRules.js
@@ -733,6 +733,8 @@ function* resolveMatches(candidate, context, original = candidate) {
}
for (let match of matches) {
+ let isValid = true
+
match[1].raws.tailwind = { ...match[1].raws.tailwind, candidate }
// Apply final format selector
@@ -742,7 +744,7 @@ function* resolveMatches(candidate, context, original = candidate) {
container.walkRules((rule) => {
if (inKeyframes(rule)) return
- rule.selector = finalizeSelector(finalFormat, {
+ let selectorOptions = {
selector: rule.selector,
candidate: original,
base: candidate
@@ -751,11 +753,31 @@ function* resolveMatches(candidate, context, original = candidate) {
isArbitraryVariant: match[0].isArbitraryVariant,
context,
- })
+ }
+
+ try {
+ rule.selector = finalizeSelector(finalFormat, selectorOptions)
+ } catch {
+ // The selector we produced is invalid
+ // This could be because:
+ // - A bug exists
+ // - A plugin introduced an invalid variant selector (ex: `addVariant('foo', '&;foo')`)
+ // - The user used an invalid arbitrary variant (ex: `[&;foo]:underline`)
+ // Either way the build will fail because of this
+ // We would rather that the build pass "silently" given that this could
+ // happen because of picking up invalid things when scanning content
+ // So we'll throw out the candidate instead
+ isValid = false
+ return false
+ }
})
match[1] = container.nodes[0]
}
+ if (!isValid) {
+ continue
+ }
+
yield match
}
}
| diff --git a/tests/arbitrary-variants.test.js b/tests/arbitrary-variants.test.js
--- a/tests/arbitrary-variants.test.js
+++ b/tests/arbitrary-variants.test.js
@@ -1099,3 +1099,47 @@ it('Arbitrary variants are ordered alphabetically', () => {
`)
})
})
+
+it('Arbitrary variants support multiple attribute selectors', () => {
+ let config = {
+ content: [
+ {
+ raw: html` <div class="[[data-foo='bar'][data-baz]_&]:underline"></div> `,
+ },
+ ],
+ corePlugins: { preflight: false },
+ }
+
+ let input = css`
+ @tailwind utilities;
+ `
+
+ return run(input, config).then((result) => {
+ expect(result.css).toMatchFormattedCss(css`
+ [data-foo='bar'][data-baz] .\[\[data-foo\=\'bar\'\]\[data-baz\]_\&\]\:underline {
+ text-decoration-line: underline;
+ }
+ `)
+ })
+})
+
+it('Invalid arbitrary variants selectors should produce nothing instead of failing', () => {
+ let config = {
+ content: [
+ {
+ raw: html`
+ <div class="[&;foo]:underline"></div>
+ `,
+ },
+ ],
+ corePlugins: { preflight: false },
+ }
+
+ let input = css`
+ @tailwind utilities;
+ `
+
+ return run(input, config).then((result) => {
+ expect(result.css).toMatchFormattedCss(css``)
+ })
+})
| Fails to compile "expected opening square bracket" with two attribute selectors
<!-- Please provide all of the information requested below. We're a small team and without all of this information it's not possible for us to help and your bug report will be closed. -->
**What version of Tailwind CSS are you using?**
Latest Tailwind v3.2.4, reproducible in live playground below.
**Reproduction URL**
https://play.tailwindcss.com/w66a9h6SsL
**Describe your issue**
The Tailwind compiler fails with `Expected an opening square bracket.` when parsing a second attribute selector after one with a value.
The following code attempts to style the element when one of its parents has two attributes. Checking for the presence of two attributes works flawlessly. Checking for the presence of one and the value of the second works great as well. Swapping the order of the attributes breaks the compiler.
- ✅ `[data-beta][data-testid]`
- ✅ `[data-beta][data-testid='test']`
- ❌ `[data-testid='test'][data-beta]`
```html
<ul data-testid="test" data-beta>
<li class="[[data-beta][data-testid]_&]:bg-violet-500"> This works </li>
<li class="[[data-beta][data-testid='test']_&]:bg-violet-500"> This works </li>
<li class="[[data-testid='test'][data-beta]_&]:bg-violet-500"> This breaks </li>
</ul>
```
| 2022-12-12T15:55:19 | 3.2 | [] | [
"tests/arbitrary-variants.test.js"
] | TypeScript | [] | [
"https://play.tailwindcss.com/w66a9h6SsL"
] |
|
tailwindlabs/tailwindcss | 10,212 | tailwindlabs__tailwindcss-10212 | [
"10180"
] | 5594c3bec95e1db985e49f6836b51d7046d59579 | diff --git a/src/lib/defaultExtractor.js b/src/lib/defaultExtractor.js
--- a/src/lib/defaultExtractor.js
+++ b/src/lib/defaultExtractor.js
@@ -28,8 +28,14 @@ function* buildRegExps(context) {
: ''
let utility = regex.any([
- // Arbitrary properties
- /\[[^\s:'"`]+:[^\s]+\]/,
+ // Arbitrary properties (without square brackets)
+ /\[[^\s:'"`]+:[^\s\[\]]+\]/,
+
+ // Arbitrary properties with balanced square brackets
+ // This is a targeted fix to continue to allow theme()
+ // with square brackets to work in arbitrary properties
+ // while fixing a problem with the regex matching too much
+ /\[[^\s:'"`]+:[^\s]+?\[[^\s]+?\][^\s]+?\]/,
// Utilities
regex.pattern([
| diff --git a/tests/default-extractor.test.js b/tests/default-extractor.test.js
--- a/tests/default-extractor.test.js
+++ b/tests/default-extractor.test.js
@@ -488,3 +488,11 @@ test('ruby percent string array', () => {
expect(extractions).toContain(`text-[#bada55]`)
})
+
+test('arbitrary properties followed by square bracketed stuff', () => {
+ let extractions = defaultExtractor(
+ '<div class="h-16 items-end border border-white [display:inherit]">[foo]</div>'
+ )
+
+ expect(extractions).toContain(`[display:inherit]`)
+})
| Div content with brackets `[]` prevents arbitrary class from being generated
## This works: https://play.tailwindcss.com/0VLICQ8oUW
<img width="810" alt="image" src="https://user-images.githubusercontent.com/111561/209682078-7e43a107-52ff-4006-8c37-ec44414abe12.png">
## This does not work: https://play.tailwindcss.com/5KJf7xDKv6
<img width="821" alt="image" src="https://user-images.githubusercontent.com/111561/209682172-de1dcaaa-6e69-429b-907f-840691e35ceb.png">
| Hey @tordans I am trying to understand the problem statement. If you elaborate about the project and the problem it will be helpful for me cause I am new in this project and I am trying to contribute in this project.
I did check through your code and didnt find any issue just pust a space before ending "
| 2023-01-02T14:38:18 | 3.2 | [] | [
"tests/default-extractor.test.js"
] | TypeScript | [
"https://user-images.githubusercontent.com/111561/209682078-7e43a107-52ff-4006-8c37-ec44414abe12.png",
"https://user-images.githubusercontent.com/111561/209682172-de1dcaaa-6e69-429b-907f-840691e35ceb.png"
] | [
"https://play.tailwindcss.com/0VLICQ8oUW",
"https://play.tailwindcss.com/5KJf7xDKv6"
] |
tailwindlabs/tailwindcss | 10,214 | tailwindlabs__tailwindcss-10214 | [
"10125"
] | 2b885ef2525988758761cc3535383d8d1dc260bf | diff --git a/src/lib/generateRules.js b/src/lib/generateRules.js
--- a/src/lib/generateRules.js
+++ b/src/lib/generateRules.js
@@ -201,6 +201,7 @@ function applyVariant(variant, matches, context) {
}
if (context.variantMap.has(variant)) {
+ let isArbitraryVariant = isArbitraryValue(variant)
let variantFunctionTuples = context.variantMap.get(variant).slice()
let result = []
@@ -262,7 +263,10 @@ function applyVariant(variant, matches, context) {
clone.append(wrapper)
},
format(selectorFormat) {
- collectedFormats.push(selectorFormat)
+ collectedFormats.push({
+ format: selectorFormat,
+ isArbitraryVariant,
+ })
},
args,
})
@@ -288,7 +292,10 @@ function applyVariant(variant, matches, context) {
}
if (typeof ruleWithVariant === 'string') {
- collectedFormats.push(ruleWithVariant)
+ collectedFormats.push({
+ format: ruleWithVariant,
+ isArbitraryVariant,
+ })
}
if (ruleWithVariant === null) {
@@ -329,7 +336,10 @@ function applyVariant(variant, matches, context) {
// modified (by plugin): .foo .foo\\:markdown > p
// rebuiltBase (internal): .foo\\:markdown > p
// format: .foo &
- collectedFormats.push(modified.replace(rebuiltBase, '&'))
+ collectedFormats.push({
+ format: modified.replace(rebuiltBase, '&'),
+ isArbitraryVariant,
+ })
rule.selector = before
})
}
@@ -349,7 +359,6 @@ function applyVariant(variant, matches, context) {
Object.assign(args, context.variantOptions.get(variant))
),
collectedFormats: (meta.collectedFormats ?? []).concat(collectedFormats),
- isArbitraryVariant: isArbitraryValue(variant),
},
clone.nodes[0],
]
@@ -733,48 +742,15 @@ function* resolveMatches(candidate, context, original = candidate) {
}
for (let match of matches) {
- let isValid = true
-
match[1].raws.tailwind = { ...match[1].raws.tailwind, candidate }
// Apply final format selector
- if (match[0].collectedFormats) {
- let finalFormat = formatVariantSelector('&', ...match[0].collectedFormats)
- let container = postcss.root({ nodes: [match[1].clone()] })
- container.walkRules((rule) => {
- if (inKeyframes(rule)) return
-
- let selectorOptions = {
- selector: rule.selector,
- candidate: original,
- base: candidate
- .split(new RegExp(`\\${context?.tailwindConfig?.separator ?? ':'}(?![^[]*\\])`))
- .pop(),
- isArbitraryVariant: match[0].isArbitraryVariant,
-
- context,
- }
-
- try {
- rule.selector = finalizeSelector(finalFormat, selectorOptions)
- } catch {
- // The selector we produced is invalid
- // This could be because:
- // - A bug exists
- // - A plugin introduced an invalid variant selector (ex: `addVariant('foo', '&;foo')`)
- // - The user used an invalid arbitrary variant (ex: `[&;foo]:underline`)
- // Either way the build will fail because of this
- // We would rather that the build pass "silently" given that this could
- // happen because of picking up invalid things when scanning content
- // So we'll throw out the candidate instead
- isValid = false
- return false
- }
- })
- match[1] = container.nodes[0]
- }
+ match = applyFinalFormat(match, { context, candidate, original })
- if (!isValid) {
+ // Skip rules with invalid selectors
+ // This will cause the candidate to be added to the "not class"
+ // cache skipping it entirely for future builds
+ if (match === null) {
continue
}
@@ -783,6 +759,62 @@ function* resolveMatches(candidate, context, original = candidate) {
}
}
+function applyFinalFormat(match, { context, candidate, original }) {
+ if (!match[0].collectedFormats) {
+ return match
+ }
+
+ let isValid = true
+ let finalFormat
+
+ try {
+ finalFormat = formatVariantSelector(match[0].collectedFormats, {
+ context,
+ candidate,
+ })
+ } catch {
+ // The format selector we produced is invalid
+ // This could be because:
+ // - A bug exists
+ // - A plugin introduced an invalid variant selector (ex: `addVariant('foo', '&;foo')`)
+ // - The user used an invalid arbitrary variant (ex: `[&;foo]:underline`)
+ // Either way the build will fail because of this
+ // We would rather that the build pass "silently" given that this could
+ // happen because of picking up invalid things when scanning content
+ // So we'll throw out the candidate instead
+
+ return null
+ }
+
+ let container = postcss.root({ nodes: [match[1].clone()] })
+
+ container.walkRules((rule) => {
+ if (inKeyframes(rule)) {
+ return
+ }
+
+ try {
+ rule.selector = finalizeSelector(rule.selector, finalFormat, {
+ candidate: original,
+ context,
+ })
+ } catch {
+ // If this selector is invalid we also want to skip it
+ // But it's likely that being invalid here means there's a bug in a plugin rather than too loosely matching content
+ isValid = false
+ return false
+ }
+ })
+
+ if (!isValid) {
+ return null
+ }
+
+ match[1] = container.nodes[0]
+
+ return match
+}
+
function inKeyframes(rule) {
return rule.parent && rule.parent.type === 'atrule' && rule.parent.name === 'keyframes'
}
diff --git a/src/lib/setupContextUtils.js b/src/lib/setupContextUtils.js
--- a/src/lib/setupContextUtils.js
+++ b/src/lib/setupContextUtils.js
@@ -1080,20 +1080,38 @@ function registerPlugins(plugins, context) {
})
}
- let result = formatStrings.map((formatString) =>
- finalizeSelector(formatVariantSelector('&', ...formatString), {
- selector: `.${candidate}`,
- candidate,
- context,
- isArbitraryVariant: !(value in (options.values ?? {})),
- })
+ let isArbitraryVariant = !(value in (options.values ?? {}))
+
+ formatStrings = formatStrings.map((format) =>
+ format.map((str) => ({
+ format: str,
+ isArbitraryVariant,
+ }))
+ )
+
+ manualFormatStrings = manualFormatStrings.map((format) => ({
+ format,
+ isArbitraryVariant,
+ }))
+
+ let opts = {
+ candidate,
+ context,
+ }
+
+ let result = formatStrings.map((formats) =>
+ finalizeSelector(`.${candidate}`, formatVariantSelector(formats, opts), opts)
.replace(`.${candidate}`, '&')
.replace('{ & }', '')
.trim()
)
if (manualFormatStrings.length > 0) {
- result.push(formatVariantSelector('&', ...manualFormatStrings))
+ result.push(
+ formatVariantSelector(manualFormatStrings, opts)
+ .toString()
+ .replace(`.${candidate}`, '&')
+ )
}
return result
diff --git a/src/util/formatVariantSelector.js b/src/util/formatVariantSelector.js
--- a/src/util/formatVariantSelector.js
+++ b/src/util/formatVariantSelector.js
@@ -3,30 +3,57 @@ import unescape from 'postcss-selector-parser/dist/util/unesc'
import escapeClassName from '../util/escapeClassName'
import prefixSelector from '../util/prefixSelector'
+/** @typedef {import('postcss-selector-parser').Root} Root */
+/** @typedef {import('postcss-selector-parser').Selector} Selector */
+/** @typedef {import('postcss-selector-parser').Pseudo} Pseudo */
+/** @typedef {import('postcss-selector-parser').Node} Node */
+
+/** @typedef {{format: string, isArbitraryVariant: boolean}[]} RawFormats */
+/** @typedef {import('postcss-selector-parser').Root} ParsedFormats */
+/** @typedef {RawFormats | ParsedFormats} AcceptedFormats */
+
let MERGE = ':merge'
-let PARENT = '&'
-
-export let selectorFunctions = new Set([MERGE])
-
-export function formatVariantSelector(current, ...others) {
- for (let other of others) {
- let incomingValue = resolveFunctionArgument(other, MERGE)
- if (incomingValue !== null) {
- let existingValue = resolveFunctionArgument(current, MERGE, incomingValue)
- if (existingValue !== null) {
- let existingTarget = `${MERGE}(${incomingValue})`
- let splitIdx = other.indexOf(existingTarget)
- let addition = other.slice(splitIdx + existingTarget.length).split(' ')[0]
-
- current = current.replace(existingTarget, existingTarget + addition)
- continue
- }
+
+/**
+ * @param {RawFormats} formats
+ * @param {{context: any, candidate: string, base: string | null}} options
+ * @returns {ParsedFormats | null}
+ */
+export function formatVariantSelector(formats, { context, candidate }) {
+ let prefix = context?.tailwindConfig.prefix ?? ''
+
+ // Parse the format selector into an AST
+ let parsedFormats = formats.map((format) => {
+ let ast = selectorParser().astSync(format.format)
+
+ return {
+ ...format,
+ ast: format.isArbitraryVariant ? ast : prefixSelector(prefix, ast),
}
+ })
- current = other.replace(PARENT, current)
+ // We start with the candidate selector
+ let formatAst = selectorParser.root({
+ nodes: [
+ selectorParser.selector({
+ nodes: [selectorParser.className({ value: escapeClassName(candidate) })],
+ }),
+ ],
+ })
+
+ // And iteratively merge each format selector into the candidate selector
+ for (let { ast } of parsedFormats) {
+ // 1. Handle :merge() special pseudo-class
+ ;[formatAst, ast] = handleMergePseudo(formatAst, ast)
+
+ // 2. Merge the format selector into the current selector AST
+ ast.walkNesting((nesting) => nesting.replaceWith(...formatAst.nodes[0].nodes))
+
+ // 3. Keep going!
+ formatAst = ast
}
- return current
+ return formatAst
}
/**
@@ -35,11 +62,11 @@ export function formatVariantSelector(current, ...others) {
* Technically :is(), :not(), :has(), etc… can have combinators but those are nested
* inside the relevant node and won't be picked up so they're fine to ignore
*
- * @param {import('postcss-selector-parser').Node} node
- * @returns {import('postcss-selector-parser').Node[]}
+ * @param {Node} node
+ * @returns {Node[]}
**/
function simpleSelectorForNode(node) {
- /** @type {import('postcss-selector-parser').Node[]} */
+ /** @type {Node[]} */
let nodes = []
// Walk backwards until we hit a combinator node (or the start)
@@ -60,8 +87,8 @@ function simpleSelectorForNode(node) {
* Resorts the nodes in a selector to ensure they're in the correct order
* Tags go before classes, and pseudo classes go after classes
*
- * @param {import('postcss-selector-parser').Selector} sel
- * @returns {import('postcss-selector-parser').Selector}
+ * @param {Selector} sel
+ * @returns {Selector}
**/
function resortSelector(sel) {
sel.sort((a, b) => {
@@ -81,6 +108,18 @@ function resortSelector(sel) {
return sel
}
+/**
+ * Remove extraneous selectors that do not include the base class/candidate
+ *
+ * Example:
+ * Given the utility `.a, .b { color: red}`
+ * Given the candidate `sm:b`
+ *
+ * The final selector should be `.sm\:b` and not `.a, .sm\:b`
+ *
+ * @param {Selector} ast
+ * @param {string} base
+ */
function eliminateIrrelevantSelectors(sel, base) {
let hasClassesMatchingCandidate = false
@@ -104,41 +143,26 @@ function eliminateIrrelevantSelectors(sel, base) {
// TODO: Can we do this for :matches, :is, and :where?
}
-export function finalizeSelector(
- format,
- {
- selector,
- candidate,
- context,
- isArbitraryVariant,
-
- // Split by the separator, but ignore the separator inside square brackets:
- //
- // E.g.: dark:lg:hover:[paint-order:markers]
- // ┬ ┬ ┬ ┬
- // │ │ │ ╰── We will not split here
- // ╰──┴─────┴─────────────── We will split here
- //
- base = candidate
- .split(new RegExp(`\\${context?.tailwindConfig?.separator ?? ':'}(?![^[]*\\])`))
- .pop(),
- }
-) {
- let ast = selectorParser().astSync(selector)
-
- // We explicitly DO NOT prefix classes in arbitrary variants
- if (context?.tailwindConfig?.prefix && !isArbitraryVariant) {
- format = prefixSelector(context.tailwindConfig.prefix, format)
- }
-
- format = format.replace(PARENT, `.${escapeClassName(candidate)}`)
-
- let formatAst = selectorParser().astSync(format)
+/**
+ * @param {string} current
+ * @param {AcceptedFormats} formats
+ * @param {{context: any, candidate: string, base: string | null}} options
+ * @returns {string}
+ */
+export function finalizeSelector(current, formats, { context, candidate, base }) {
+ let separator = context?.tailwindConfig?.separator ?? ':'
+
+ // Split by the separator, but ignore the separator inside square brackets:
+ //
+ // E.g.: dark:lg:hover:[paint-order:markers]
+ // ┬ ┬ ┬ ┬
+ // │ │ │ ╰── We will not split here
+ // ╰──┴─────┴─────────────── We will split here
+ //
+ base = base ?? candidate.split(new RegExp(`\\${separator}(?![^[]*\\])`)).pop()
- // Remove extraneous selectors that do not include the base class/candidate being matched against
- // For example if we have a utility defined `.a, .b { color: red}`
- // And the formatted variant is sm:b then we want the final selector to be `.sm\:b` and not `.a, .sm\:b`
- ast.each((sel) => eliminateIrrelevantSelectors(sel, base))
+ // Parse the selector into an AST
+ let selector = selectorParser().astSync(current)
// Normalize escaped classes, e.g.:
//
@@ -151,18 +175,31 @@ export function finalizeSelector(
// base in selector: bg-\\[rgb\\(255\\,0\\,0\\)\\]
// escaped base: bg-\\[rgb\\(255\\2c 0\\2c 0\\)\\]
//
- ast.walkClasses((node) => {
+ selector.walkClasses((node) => {
if (node.raws && node.value.includes(base)) {
node.raws.value = escapeClassName(unescape(node.raws.value))
}
})
+ // Remove extraneous selectors that do not include the base candidate
+ selector.each((sel) => eliminateIrrelevantSelectors(sel, base))
+
+ // If there are no formats that means there were no variants added to the candidate
+ // so we can just return the selector as-is
+ let formatAst = Array.isArray(formats)
+ ? formatVariantSelector(formats, { context, candidate })
+ : formats
+
+ if (formatAst === null) {
+ return selector.toString()
+ }
+
let simpleStart = selectorParser.comment({ value: '/*__simple__*/' })
let simpleEnd = selectorParser.comment({ value: '/*__simple__*/' })
// We can safely replace the escaped base now, since the `base` section is
// now in a normalized escaped value.
- ast.walkClasses((node) => {
+ selector.walkClasses((node) => {
if (node.value !== base) {
return
}
@@ -200,47 +237,86 @@ export function finalizeSelector(
simpleEnd.remove()
})
- // This will make sure to move pseudo's to the correct spot (the end for
- // pseudo elements) because otherwise the selector will never work
- // anyway.
- //
- // E.g.:
- // - `before:hover:text-center` would result in `.before\:hover\:text-center:hover::before`
- // - `hover:before:text-center` would result in `.hover\:before\:text-center:hover::before`
- //
- // `::before:hover` doesn't work, which means that we can make it work for you by flipping the order.
- function collectPseudoElements(selector) {
- let nodes = []
-
- for (let node of selector.nodes) {
- if (isPseudoElement(node)) {
- nodes.push(node)
- selector.removeChild(node)
- }
-
- if (node?.nodes) {
- nodes.push(...collectPseudoElements(node))
- }
+ // Remove unnecessary pseudo selectors that we used as placeholders
+ selector.walkPseudos((p) => {
+ if (p.value === MERGE) {
+ p.replaceWith(p.nodes)
}
+ })
- return nodes
- }
-
- // Remove unnecessary pseudo selectors that we used as placeholders
- ast.each((selector) => {
- selector.walkPseudos((p) => {
- if (selectorFunctions.has(p.value)) {
- p.replaceWith(p.nodes)
- }
- })
-
- let pseudoElements = collectPseudoElements(selector)
+ // Move pseudo elements to the end of the selector (if necessary)
+ selector.each((sel) => {
+ let pseudoElements = collectPseudoElements(sel)
if (pseudoElements.length > 0) {
- selector.nodes.push(pseudoElements.sort(sortSelector))
+ sel.nodes.push(pseudoElements.sort(sortSelector))
+ }
+ })
+
+ return selector.toString()
+}
+
+/**
+ *
+ * @param {Selector} selector
+ * @param {Selector} format
+ */
+export function handleMergePseudo(selector, format) {
+ /** @type {{pseudo: Pseudo, value: string}[]} */
+ let merges = []
+
+ // Find all :merge() pseudo-classes in `selector`
+ selector.walkPseudos((pseudo) => {
+ if (pseudo.value === MERGE) {
+ merges.push({
+ pseudo,
+ value: pseudo.nodes[0].toString(),
+ })
}
})
- return ast.toString()
+ // Find all :merge() "attachments" in `format` and attach them to the matching selector in `selector`
+ format.walkPseudos((pseudo) => {
+ if (pseudo.value !== MERGE) {
+ return
+ }
+
+ let value = pseudo.nodes[0].toString()
+
+ // Does `selector` contain a :merge() pseudo-class with the same value?
+ let existing = merges.find((merge) => merge.value === value)
+
+ // Nope so there's nothing to do
+ if (!existing) {
+ return
+ }
+
+ // Everything after `:merge()` up to the next combinator is what is attached to the merged selector
+ let attachments = []
+ let next = pseudo.next()
+ while (next && next.type !== 'combinator') {
+ attachments.push(next)
+ next = next.next()
+ }
+
+ let combinator = next
+
+ existing.pseudo.parent.insertAfter(
+ existing.pseudo,
+ selectorParser.selector({ nodes: attachments.map((node) => node.clone()) })
+ )
+
+ pseudo.remove()
+ attachments.forEach((node) => node.remove())
+
+ // What about this case:
+ // :merge(.group):focus > &
+ // :merge(.group):hover &
+ if (combinator && combinator.type === 'combinator') {
+ combinator.remove()
+ }
+ })
+
+ return [selector, format]
}
// Note: As a rule, double colons (::) should be used instead of a single colon
@@ -263,6 +339,37 @@ let pseudoElementExceptions = [
'::-webkit-resizer',
]
+/**
+ * This will make sure to move pseudo's to the correct spot (the end for
+ * pseudo elements) because otherwise the selector will never work
+ * anyway.
+ *
+ * E.g.:
+ * - `before:hover:text-center` would result in `.before\:hover\:text-center:hover::before`
+ * - `hover:before:text-center` would result in `.hover\:before\:text-center:hover::before`
+ *
+ * `::before:hover` doesn't work, which means that we can make it work for you by flipping the order.
+ *
+ * @param {Selector} selector
+ **/
+function collectPseudoElements(selector) {
+ /** @type {Node[]} */
+ let nodes = []
+
+ for (let node of selector.nodes) {
+ if (isPseudoElement(node)) {
+ nodes.push(node)
+ selector.removeChild(node)
+ }
+
+ if (node?.nodes) {
+ nodes.push(...collectPseudoElements(node))
+ }
+ }
+
+ return nodes
+}
+
// This will make sure to move pseudo's to the correct spot (the end for
// pseudo elements) because otherwise the selector will never work
// anyway.
@@ -303,28 +410,3 @@ function isPseudoElement(node) {
return node.value.startsWith('::') || pseudoElementsBC.includes(node.value)
}
-
-function resolveFunctionArgument(haystack, needle, arg) {
- let startIdx = haystack.indexOf(arg ? `${needle}(${arg})` : needle)
- if (startIdx === -1) return null
-
- // Start inside the `(`
- startIdx += needle.length + 1
-
- let target = ''
- let count = 0
-
- for (let char of haystack.slice(startIdx)) {
- if (char !== '(' && char !== ')') {
- target += char
- } else if (char === '(') {
- target += char
- count++
- } else if (char === ')') {
- if (--count < 0) break // unbalanced
- target += char
- }
- }
-
- return target
-}
diff --git a/src/util/prefixSelector.js b/src/util/prefixSelector.js
--- a/src/util/prefixSelector.js
+++ b/src/util/prefixSelector.js
@@ -1,14 +1,32 @@
import parser from 'postcss-selector-parser'
+/**
+ * @template {string | import('postcss-selector-parser').Root} T
+ *
+ * Prefix all classes in the selector with the given prefix
+ *
+ * It can take either a string or a selector AST and will return the same type
+ *
+ * @param {string} prefix
+ * @param {T} selector
+ * @param {boolean} prependNegative
+ * @returns {T}
+ */
export default function (prefix, selector, prependNegative = false) {
- return parser((selectors) => {
- selectors.walkClasses((classSelector) => {
- let baseClass = classSelector.value
- let shouldPlaceNegativeBeforePrefix = prependNegative && baseClass.startsWith('-')
-
- classSelector.value = shouldPlaceNegativeBeforePrefix
- ? `-${prefix}${baseClass.slice(1)}`
- : `${prefix}${baseClass}`
- })
- }).processSync(selector)
+ if (prefix === '') {
+ return selector
+ }
+
+ let ast = typeof selector === 'string' ? parser().astSync(selector) : selector
+
+ ast.walkClasses((classSelector) => {
+ let baseClass = classSelector.value
+ let shouldPlaceNegativeBeforePrefix = prependNegative && baseClass.startsWith('-')
+
+ classSelector.value = shouldPlaceNegativeBeforePrefix
+ ? `-${prefix}${baseClass.slice(1)}`
+ : `${prefix}${baseClass}`
+ })
+
+ return typeof selector === 'string' ? ast.toString() : ast
}
| diff --git a/tests/arbitrary-variants.test.js b/tests/arbitrary-variants.test.js
--- a/tests/arbitrary-variants.test.js
+++ b/tests/arbitrary-variants.test.js
@@ -542,6 +542,14 @@ test('classes in arbitrary variants should not be prefixed', () => {
<div>should not be red</div>
<div class="foo">should be red</div>
</div>
+ <div class="hover:[&_.foo]:tw-text-red-400">
+ <div>should not be red</div>
+ <div class="foo">should be red</div>
+ </div>
+ <div class="[&_.foo]:hover:tw-text-red-400">
+ <div>should not be red</div>
+ <div class="foo">should be red</div>
+ </div>
`,
},
],
@@ -558,7 +566,14 @@ test('classes in arbitrary variants should not be prefixed', () => {
--tw-text-opacity: 1;
color: rgb(248 113 113 / var(--tw-text-opacity));
}
-
+ .hover\:\[\&_\.foo\]\:tw-text-red-400 .foo:hover {
+ --tw-text-opacity: 1;
+ color: rgb(248 113 113 / var(--tw-text-opacity));
+ }
+ .\[\&_\.foo\]\:hover\:tw-text-red-400:hover .foo {
+ --tw-text-opacity: 1;
+ color: rgb(248 113 113 / var(--tw-text-opacity));
+ }
.foo .\[\.foo_\&\]\:tw-text-red-400 {
--tw-text-opacity: 1;
color: rgb(248 113 113 / var(--tw-text-opacity));
diff --git a/tests/format-variant-selector.test.js b/tests/format-variant-selector.test.js
--- a/tests/format-variant-selector.test.js
+++ b/tests/format-variant-selector.test.js
@@ -1,23 +1,24 @@
-import { formatVariantSelector, finalizeSelector } from '../src/util/formatVariantSelector'
+import { finalizeSelector } from '../src/util/formatVariantSelector'
it('should be possible to add a simple variant to a simple selector', () => {
let selector = '.text-center'
let candidate = 'hover:text-center'
- let variants = ['&:hover']
+ let formats = [{ format: '&:hover', isArbitraryVariant: false }]
- expect(finalizeSelector(formatVariantSelector(...variants), { selector, candidate })).toEqual(
- '.hover\\:text-center:hover'
- )
+ expect(finalizeSelector(selector, formats, { candidate })).toEqual('.hover\\:text-center:hover')
})
it('should be possible to add a multiple simple variants to a simple selector', () => {
let selector = '.text-center'
let candidate = 'focus:hover:text-center'
- let variants = ['&:hover', '&:focus']
+ let formats = [
+ { format: '&:hover', isArbitraryVariant: false },
+ { format: '&:focus', isArbitraryVariant: false },
+ ]
- expect(finalizeSelector(formatVariantSelector(...variants), { selector, candidate })).toEqual(
+ expect(finalizeSelector(selector, formats, { candidate })).toEqual(
'.focus\\:hover\\:text-center:hover:focus'
)
})
@@ -26,9 +27,9 @@ it('should be possible to add a simple variant to a selector containing escaped
let selector = '.bg-\\[rgba\\(0\\,0\\,0\\)\\]'
let candidate = 'hover:bg-[rgba(0,0,0)]'
- let variants = ['&:hover']
+ let formats = [{ format: '&:hover', isArbitraryVariant: false }]
- expect(finalizeSelector(formatVariantSelector(...variants), { selector, candidate })).toEqual(
+ expect(finalizeSelector(selector, formats, { candidate })).toEqual(
'.hover\\:bg-\\[rgba\\(0\\2c 0\\2c 0\\)\\]:hover'
)
})
@@ -37,9 +38,9 @@ it('should be possible to add a simple variant to a selector containing escaped
let selector = '.bg-\\[rgba\\(0\\2c 0\\2c 0\\)\\]'
let candidate = 'hover:bg-[rgba(0,0,0)]'
- let variants = ['&:hover']
+ let formats = [{ format: '&:hover', isArbitraryVariant: false }]
- expect(finalizeSelector(formatVariantSelector(...variants), { selector, candidate })).toEqual(
+ expect(finalizeSelector(selector, formats, { candidate })).toEqual(
'.hover\\:bg-\\[rgba\\(0\\2c 0\\2c 0\\)\\]:hover'
)
})
@@ -48,9 +49,9 @@ it('should be possible to add a simple variant to a more complex selector', () =
let selector = '.space-x-4 > :not([hidden]) ~ :not([hidden])'
let candidate = 'hover:space-x-4'
- let variants = ['&:hover']
+ let formats = [{ format: '&:hover', isArbitraryVariant: false }]
- expect(finalizeSelector(formatVariantSelector(...variants), { selector, candidate })).toEqual(
+ expect(finalizeSelector(selector, formats, { candidate })).toEqual(
'.hover\\:space-x-4:hover > :not([hidden]) ~ :not([hidden])'
)
})
@@ -59,9 +60,13 @@ it('should be possible to add multiple simple variants to a more complex selecto
let selector = '.space-x-4 > :not([hidden]) ~ :not([hidden])'
let candidate = 'disabled:focus:hover:space-x-4'
- let variants = ['&:hover', '&:focus', '&:disabled']
+ let formats = [
+ { format: '&:hover', isArbitraryVariant: false },
+ { format: '&:focus', isArbitraryVariant: false },
+ { format: '&:disabled', isArbitraryVariant: false },
+ ]
- expect(finalizeSelector(formatVariantSelector(...variants), { selector, candidate })).toEqual(
+ expect(finalizeSelector(selector, formats, { candidate })).toEqual(
'.disabled\\:focus\\:hover\\:space-x-4:hover:focus:disabled > :not([hidden]) ~ :not([hidden])'
)
})
@@ -70,9 +75,9 @@ it('should be possible to add a single merge variant to a simple selector', () =
let selector = '.text-center'
let candidate = 'group-hover:text-center'
- let variants = [':merge(.group):hover &']
+ let formats = [{ format: ':merge(.group):hover &', isArbitraryVariant: false }]
- expect(finalizeSelector(formatVariantSelector(...variants), { selector, candidate })).toEqual(
+ expect(finalizeSelector(selector, formats, { candidate })).toEqual(
'.group:hover .group-hover\\:text-center'
)
})
@@ -81,9 +86,12 @@ it('should be possible to add multiple merge variants to a simple selector', ()
let selector = '.text-center'
let candidate = 'group-focus:group-hover:text-center'
- let variants = [':merge(.group):hover &', ':merge(.group):focus &']
+ let formats = [
+ { format: ':merge(.group):hover &', isArbitraryVariant: false },
+ { format: ':merge(.group):focus &', isArbitraryVariant: false },
+ ]
- expect(finalizeSelector(formatVariantSelector(...variants), { selector, candidate })).toEqual(
+ expect(finalizeSelector(selector, formats, { candidate })).toEqual(
'.group:focus:hover .group-focus\\:group-hover\\:text-center'
)
})
@@ -92,9 +100,9 @@ it('should be possible to add a single merge variant to a more complex selector'
let selector = '.space-x-4 ~ :not([hidden]) ~ :not([hidden])'
let candidate = 'group-hover:space-x-4'
- let variants = [':merge(.group):hover &']
+ let formats = [{ format: ':merge(.group):hover &', isArbitraryVariant: false }]
- expect(finalizeSelector(formatVariantSelector(...variants), { selector, candidate })).toEqual(
+ expect(finalizeSelector(selector, formats, { candidate })).toEqual(
'.group:hover .group-hover\\:space-x-4 ~ :not([hidden]) ~ :not([hidden])'
)
})
@@ -103,9 +111,12 @@ it('should be possible to add multiple merge variants to a more complex selector
let selector = '.space-x-4 ~ :not([hidden]) ~ :not([hidden])'
let candidate = 'group-focus:group-hover:space-x-4'
- let variants = [':merge(.group):hover &', ':merge(.group):focus &']
+ let formats = [
+ { format: ':merge(.group):hover &', isArbitraryVariant: false },
+ { format: ':merge(.group):focus &', isArbitraryVariant: false },
+ ]
- expect(finalizeSelector(formatVariantSelector(...variants), { selector, candidate })).toEqual(
+ expect(finalizeSelector(selector, formats, { candidate })).toEqual(
'.group:focus:hover .group-focus\\:group-hover\\:space-x-4 ~ :not([hidden]) ~ :not([hidden])'
)
})
@@ -114,9 +125,12 @@ it('should be possible to add multiple unique merge variants to a simple selecto
let selector = '.text-center'
let candidate = 'peer-focus:group-hover:text-center'
- let variants = [':merge(.group):hover &', ':merge(.peer):focus ~ &']
+ let formats = [
+ { format: ':merge(.group):hover &', isArbitraryVariant: false },
+ { format: ':merge(.peer):focus ~ &' },
+ ]
- expect(finalizeSelector(formatVariantSelector(...variants), { selector, candidate })).toEqual(
+ expect(finalizeSelector(selector, formats, { candidate })).toEqual(
'.peer:focus ~ .group:hover .peer-focus\\:group-hover\\:text-center'
)
})
@@ -125,37 +139,41 @@ it('should be possible to add multiple unique merge variants to a simple selecto
let selector = '.text-center'
let candidate = 'group-hover:peer-focus:text-center'
- let variants = [':merge(.peer):focus ~ &', ':merge(.group):hover &']
+ let formats = [
+ { format: ':merge(.peer):focus ~ &', isArbitraryVariant: false },
+ { format: ':merge(.group):hover &', isArbitraryVariant: false },
+ ]
- expect(finalizeSelector(formatVariantSelector(...variants), { selector, candidate })).toEqual(
+ expect(finalizeSelector(selector, formats, { candidate })).toEqual(
'.group:hover .peer:focus ~ .group-hover\\:peer-focus\\:text-center'
)
})
it('should be possible to use multiple :merge() calls with different "arguments"', () => {
- let result = '&'
- result = formatVariantSelector(result, ':merge(.group):hover &')
- expect(result).toEqual(':merge(.group):hover &')
-
- result = formatVariantSelector(result, ':merge(.peer):hover ~ &')
- expect(result).toEqual(':merge(.peer):hover ~ :merge(.group):hover &')
-
- result = formatVariantSelector(result, ':merge(.group):focus &')
- expect(result).toEqual(':merge(.peer):hover ~ :merge(.group):focus:hover &')
+ let selector = '.foo'
+ let candidate = 'peer-focus:group-focus:peer-hover:group-hover:foo'
+
+ let formats = [
+ { format: ':merge(.group):hover &', isArbitraryVariant: false },
+ { format: ':merge(.peer):hover ~ &', isArbitraryVariant: false },
+ { format: ':merge(.group):focus &', isArbitraryVariant: false },
+ { format: ':merge(.peer):focus ~ &', isArbitraryVariant: false },
+ ]
- result = formatVariantSelector(result, ':merge(.peer):focus ~ &')
- expect(result).toEqual(':merge(.peer):focus:hover ~ :merge(.group):focus:hover &')
+ expect(finalizeSelector(selector, formats, { candidate })).toEqual(
+ '.peer:focus:hover ~ .group:focus:hover .peer-focus\\:group-focus\\:peer-hover\\:group-hover\\:foo'
+ )
})
it('group hover and prose headings combination', () => {
let selector = '.text-center'
let candidate = 'group-hover:prose-headings:text-center'
- let variants = [
- ':where(&) :is(h1, h2, h3, h4)', // Prose Headings
- ':merge(.group):hover &', // Group Hover
+ let formats = [
+ { format: ':where(&) :is(h1, h2, h3, h4)', isArbitraryVariant: false }, // Prose Headings
+ { format: ':merge(.group):hover &', isArbitraryVariant: false }, // Group Hover
]
- expect(finalizeSelector(formatVariantSelector(...variants), { selector, candidate })).toEqual(
+ expect(finalizeSelector(selector, formats, { candidate })).toEqual(
'.group:hover :where(.group-hover\\:prose-headings\\:text-center) :is(h1, h2, h3, h4)'
)
})
@@ -163,12 +181,12 @@ it('group hover and prose headings combination', () => {
it('group hover and prose headings combination flipped', () => {
let selector = '.text-center'
let candidate = 'prose-headings:group-hover:text-center'
- let variants = [
- ':merge(.group):hover &', // Group Hover
- ':where(&) :is(h1, h2, h3, h4)', // Prose Headings
+ let formats = [
+ { format: ':merge(.group):hover &', isArbitraryVariant: false }, // Group Hover
+ { format: ':where(&) :is(h1, h2, h3, h4)', isArbitraryVariant: false }, // Prose Headings
]
- expect(finalizeSelector(formatVariantSelector(...variants), { selector, candidate })).toEqual(
+ expect(finalizeSelector(selector, formats, { candidate })).toEqual(
':where(.group:hover .prose-headings\\:group-hover\\:text-center) :is(h1, h2, h3, h4)'
)
})
@@ -176,28 +194,74 @@ it('group hover and prose headings combination flipped', () => {
it('should be possible to handle a complex utility', () => {
let selector = '.space-x-4 > :not([hidden]) ~ :not([hidden])'
let candidate = 'peer-disabled:peer-first-child:group-hover:group-focus:focus:hover:space-x-4'
- let variants = [
- '&:hover', // Hover
- '&:focus', // Focus
- ':merge(.group):focus &', // Group focus
- ':merge(.group):hover &', // Group hover
- ':merge(.peer):first-child ~ &', // Peer first-child
- ':merge(.peer):disabled ~ &', // Peer disabled
+ let formats = [
+ { format: '&:hover', isArbitraryVariant: false }, // Hover
+ { format: '&:focus', isArbitraryVariant: false }, // Focus
+ { format: ':merge(.group):focus &', isArbitraryVariant: false }, // Group focus
+ { format: ':merge(.group):hover &', isArbitraryVariant: false }, // Group hover
+ { format: ':merge(.peer):first-child ~ &', isArbitraryVariant: false }, // Peer first-child
+ { format: ':merge(.peer):disabled ~ &', isArbitraryVariant: false }, // Peer disabled
]
- expect(finalizeSelector(formatVariantSelector(...variants), { selector, candidate })).toEqual(
+ expect(finalizeSelector(selector, formats, { candidate })).toEqual(
'.peer:disabled:first-child ~ .group:hover:focus .peer-disabled\\:peer-first-child\\:group-hover\\:group-focus\\:focus\\:hover\\:space-x-4:hover:focus > :not([hidden]) ~ :not([hidden])'
)
})
+it('should match base utilities that are prefixed', () => {
+ let context = { tailwindConfig: { prefix: 'tw-' } }
+ let selector = '.tw-text-center'
+ let candidate = 'tw-text-center'
+ let formats = []
+
+ expect(finalizeSelector(selector, formats, { candidate, context })).toEqual('.tw-text-center')
+})
+
+it('should prefix classes from variants', () => {
+ let context = { tailwindConfig: { prefix: 'tw-' } }
+ let selector = '.tw-text-center'
+ let candidate = 'foo:tw-text-center'
+ let formats = [{ format: '.foo &', isArbitraryVariant: false }]
+
+ expect(finalizeSelector(selector, formats, { candidate, context })).toEqual(
+ '.tw-foo .foo\\:tw-text-center'
+ )
+})
+
+it('should not prefix classes from arbitrary variants', () => {
+ let context = { tailwindConfig: { prefix: 'tw-' } }
+ let selector = '.tw-text-center'
+ let candidate = '[.foo_&]:tw-text-center'
+ let formats = [{ format: '.foo &', isArbitraryVariant: true }]
+
+ expect(finalizeSelector(selector, formats, { candidate, context })).toEqual(
+ '.foo .\\[\\.foo_\\&\\]\\:tw-text-center'
+ )
+})
+
+it('Merged selectors with mixed combinators uses the first one', () => {
+ // This isn't explicitly specced behavior but it is how it works today
+
+ let selector = '.text-center'
+ let candidate = 'text-center'
+ let formats = [
+ { format: ':merge(.group):focus > &', isArbitraryVariant: true },
+ { format: ':merge(.group):hover &', isArbitraryVariant: true },
+ ]
+
+ expect(finalizeSelector(selector, formats, { candidate })).toEqual(
+ '.group:hover:focus > .text-center'
+ )
+})
+
describe('real examples', () => {
it('example a', () => {
let selector = '.placeholder-red-500::placeholder'
let candidate = 'hover:placeholder-red-500'
- let variants = ['&:hover']
+ let formats = [{ format: '&:hover', isArbitraryVariant: false }]
- expect(finalizeSelector(formatVariantSelector(...variants), { selector, candidate })).toEqual(
+ expect(finalizeSelector(selector, formats, { candidate })).toEqual(
'.hover\\:placeholder-red-500:hover::placeholder'
)
})
@@ -206,9 +270,12 @@ describe('real examples', () => {
let selector = '.space-x-4 > :not([hidden]) ~ :not([hidden])'
let candidate = 'group-hover:hover:space-x-4'
- let variants = ['&:hover', ':merge(.group):hover &']
+ let formats = [
+ { format: '&:hover', isArbitraryVariant: false },
+ { format: ':merge(.group):hover &', isArbitraryVariant: false },
+ ]
- expect(finalizeSelector(formatVariantSelector(...variants), { selector, candidate })).toEqual(
+ expect(finalizeSelector(selector, formats, { candidate })).toEqual(
'.group:hover .group-hover\\:hover\\:space-x-4:hover > :not([hidden]) ~ :not([hidden])'
)
})
@@ -217,9 +284,12 @@ describe('real examples', () => {
let selector = '.text-center'
let candidate = 'dark:group-hover:text-center'
- let variants = [':merge(.group):hover &', '.dark &']
+ let formats = [
+ { format: ':merge(.group):hover &', isArbitraryVariant: false },
+ { format: '.dark &', isArbitraryVariant: false },
+ ]
- expect(finalizeSelector(formatVariantSelector(...variants), { selector, candidate })).toEqual(
+ expect(finalizeSelector(selector, formats, { candidate })).toEqual(
'.dark .group:hover .dark\\:group-hover\\:text-center'
)
})
@@ -228,9 +298,12 @@ describe('real examples', () => {
let selector = '.text-center'
let candidate = 'group-hover:dark:text-center'
- let variants = ['.dark &', ':merge(.group):hover &']
+ let formats = [
+ { format: '.dark &' },
+ { format: ':merge(.group):hover &', isArbitraryVariant: false },
+ ]
- expect(finalizeSelector(formatVariantSelector(...variants), { selector, candidate })).toEqual(
+ expect(finalizeSelector(selector, formats, { candidate })).toEqual(
'.group:hover .dark .group-hover\\:dark\\:text-center'
)
})
@@ -240,9 +313,9 @@ describe('real examples', () => {
let selector = '.text-center'
let candidate = 'hover:prose-headings:text-center'
- let variants = [':where(&) :is(h1, h2, h3, h4)', '&:hover']
+ let formats = [{ format: ':where(&) :is(h1, h2, h3, h4)' }, { format: '&:hover' }]
- expect(finalizeSelector(formatVariantSelector(...variants), { selector, candidate })).toEqual(
+ expect(finalizeSelector(selector, formats, { candidate })).toEqual(
':where(.hover\\:prose-headings\\:text-center) :is(h1, h2, h3, h4):hover'
)
})
@@ -251,9 +324,9 @@ describe('real examples', () => {
let selector = '.text-center'
let candidate = 'prose-headings:hover:text-center'
- let variants = ['&:hover', ':where(&) :is(h1, h2, h3, h4)']
+ let formats = [{ format: '&:hover' }, { format: ':where(&) :is(h1, h2, h3, h4)' }]
- expect(finalizeSelector(formatVariantSelector(...variants), { selector, candidate })).toEqual(
+ expect(finalizeSelector(selector, formats, { candidate })).toEqual(
':where(.prose-headings\\:hover\\:text-center:hover) :is(h1, h2, h3, h4)'
)
})
@@ -274,8 +347,7 @@ describe('pseudo elements', () => {
${':where(&::before) :is(h1, h2, h3, h4)'} | ${':where(&) :is(h1, h2, h3, h4)::before'}
${':where(&::file-selector-button) :is(h1, h2, h3, h4)'} | ${':where(&::file-selector-button) :is(h1, h2, h3, h4)'}
`('should translate "$before" into "$after"', ({ before, after }) => {
- let result = finalizeSelector(formatVariantSelector('&', before), {
- selector: '.a',
+ let result = finalizeSelector('.a', [{ format: before, isArbitraryVariant: false }], {
candidate: 'a',
})
| `prefix` option + `modifier` + `arbitrary variant`: extra prefix is generated for classes inside variant
`prefix: "tw-",` + `hover:[&.header-is-scrolled_.menu-list_a]:tw-text-[#FF0000]`
generates:
```CSS
.hover\:\[\&\.header-is-scrolled_\.menu-list_a\]\:tw-text-\[\#FF0000\].tw-header-is-scrolled .tw-menu-list a:hover {
--tw-text-opacity: 1;
color: rgb(255 0 0 / var(--tw-text-opacity));
}
```
expected result:
```CSS
.hover\:\[\&\.header-is-scrolled_\.menu-list_a\]\:tw-text-\[\#FF0000\].header-is-scrolled .menu-list a:hover {
--tw-text-opacity: 1;
color: rgb(255 0 0 / var(--tw-text-opacity));
}
```
# [Tailwind Play Link](https://play.tailwindcss.com/kuo2fGhMiZ)
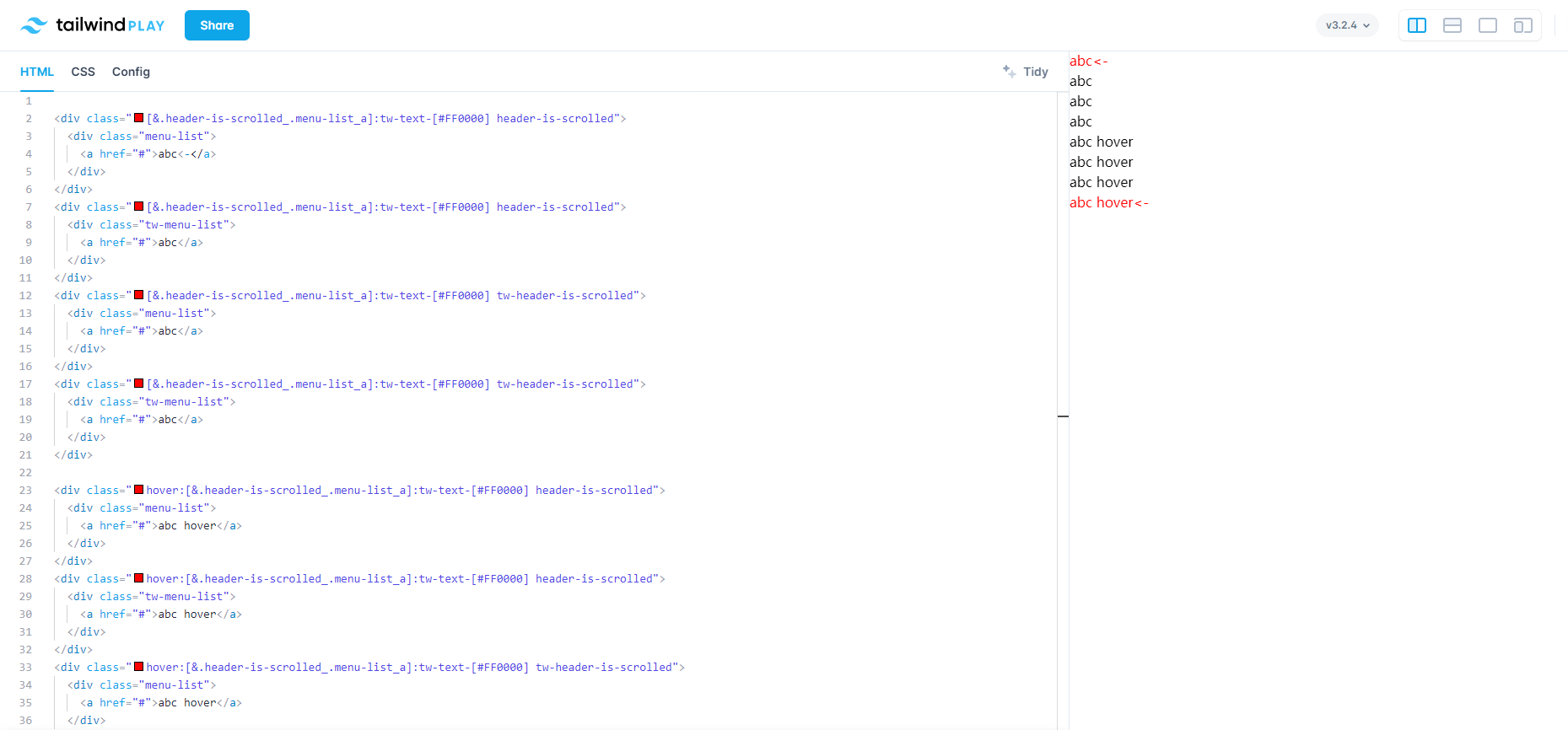
```HTML
<div class="[&.header-is-scrolled_.menu-list_a]:tw-text-[#FF0000] header-is-scrolled">
<div class="menu-list">
<a href="#">abc<-</a>
</div>
</div>
<div class="[&.header-is-scrolled_.menu-list_a]:tw-text-[#FF0000] header-is-scrolled">
<div class="tw-menu-list">
<a href="#">abc</a>
</div>
</div>
<div class="[&.header-is-scrolled_.menu-list_a]:tw-text-[#FF0000] tw-header-is-scrolled">
<div class="menu-list">
<a href="#">abc</a>
</div>
</div>
<div class="[&.header-is-scrolled_.menu-list_a]:tw-text-[#FF0000] tw-header-is-scrolled">
<div class="tw-menu-list">
<a href="#">abc</a>
</div>
</div>
<div class="hover:[&.header-is-scrolled_.menu-list_a]:tw-text-[#FF0000] header-is-scrolled">
<div class="menu-list">
<a href="#">abc</a>
</div>
</div>
<div class="hover:[&.header-is-scrolled_.menu-list_a]:tw-text-[#FF0000] header-is-scrolled">
<div class="tw-menu-list">
<a href="#">abc</a>
</div>
</div>
<div class="hover:[&.header-is-scrolled_.menu-list_a]:tw-text-[#FF0000] tw-header-is-scrolled">
<div class="menu-list">
<a href="#">abc</a>
</div>
</div>
<div class="hover:[&.header-is-scrolled_.menu-list_a]:tw-text-[#FF0000] tw-header-is-scrolled">
<div class="tw-menu-list">
<a href="#">abc<-</a>
</div>
</div>
```
| I think I'm having a similar issue with group
This is not working:
```html
<div class="tw-group is-published">
<div class="tw-hidden group-[.is-published]:block">
Published
</div>
</div>
```
But found this is working:
```html
<div class="tw-group tw-is-published">
<div class="tw-hidden group-[.is-published]:block">
Published
</div>
</div>
```
Is this the same issue? | 2023-01-02T20:13:22 | 3.2 | [] | [
"tests/format-variant-selector.test.js",
"tests/arbitrary-variants.test.js"
] | TypeScript | [] | [
"https://play.tailwindcss.com/kuo2fGhMiZ"
] |
tailwindlabs/tailwindcss | 10,276 | tailwindlabs__tailwindcss-10276 | [
"10266"
] | 7b3de616e9c26211c605d2378b9ceb4888a8cfa1 | diff --git a/src/corePlugins.js b/src/corePlugins.js
--- a/src/corePlugins.js
+++ b/src/corePlugins.js
@@ -150,9 +150,13 @@ export let variantPlugins = {
let variants = {
group: (_, { modifier }) =>
- modifier ? [`:merge(.group\\/${modifier})`, ' &'] : [`:merge(.group)`, ' &'],
+ modifier
+ ? [`:merge(.group\\/${escapeClassName(modifier)})`, ' &']
+ : [`:merge(.group)`, ' &'],
peer: (_, { modifier }) =>
- modifier ? [`:merge(.peer\\/${modifier})`, ' ~ &'] : [`:merge(.peer)`, ' ~ &'],
+ modifier
+ ? [`:merge(.peer\\/${escapeClassName(modifier)})`, ' ~ &']
+ : [`:merge(.peer)`, ' ~ &'],
}
for (let [name, fn] of Object.entries(variants)) {
diff --git a/src/lib/setupContextUtils.js b/src/lib/setupContextUtils.js
--- a/src/lib/setupContextUtils.js
+++ b/src/lib/setupContextUtils.js
@@ -54,32 +54,50 @@ function normalizeOptionTypes({ type = 'any', ...options }) {
}
function parseVariantFormatString(input) {
- if (input.includes('{')) {
- if (!isBalanced(input)) throw new Error(`Your { and } are unbalanced.`)
-
- return input
- .split(/{(.*)}/gim)
- .flatMap((line) => parseVariantFormatString(line))
- .filter(Boolean)
- }
-
- return [input.trim()]
-}
-
-function isBalanced(input) {
- let count = 0
-
- for (let char of input) {
- if (char === '{') {
- count++
+ /** @type {string[]} */
+ let parts = []
+
+ // When parsing whitespace around special characters are insignificant
+ // However, _inside_ of a variant they could be
+ // Because the selector could look like this
+ // @media { &[data-name="foo bar"] }
+ // This is why we do not skip whitespace
+
+ let current = ''
+ let depth = 0
+
+ for (let idx = 0; idx < input.length; idx++) {
+ let char = input[idx]
+
+ if (char === '\\') {
+ // Escaped characters are not special
+ current += '\\' + input[++idx]
+ } else if (char === '{') {
+ // Nested rule: start
+ ++depth
+ parts.push(current.trim())
+ current = ''
} else if (char === '}') {
- if (--count < 0) {
- return false // unbalanced
+ // Nested rule: end
+ if (--depth < 0) {
+ throw new Error(`Your { and } are unbalanced.`)
}
+
+ parts.push(current.trim())
+ current = ''
+ } else {
+ // Normal character
+ current += char
}
}
- return count === 0
+ if (current.length > 0) {
+ parts.push(current.trim())
+ }
+
+ parts = parts.filter((part) => part !== '')
+
+ return parts
}
function insertInto(list, value, { before = [] } = {}) {
| diff --git a/tests/basic-usage.test.js b/tests/basic-usage.test.js
--- a/tests/basic-usage.test.js
+++ b/tests/basic-usage.test.js
@@ -689,3 +689,27 @@ it('Ring color utilities are generated when using respectDefaultRingColorOpacity
`)
})
})
+
+it('should not crash when group names contain special characters', () => {
+ let config = {
+ future: { respectDefaultRingColorOpacity: true },
+ content: [
+ {
+ raw: '<div class="group/${id}"><div class="group-hover/${id}:visible"></div></div>',
+ },
+ ],
+ corePlugins: { preflight: false },
+ }
+
+ let input = css`
+ @tailwind utilities;
+ `
+
+ return run(input, config).then((result) => {
+ expect(result.css).toMatchFormattedCss(css`
+ .group\/\$\{id\}:hover .group-hover\/\$\{id\}\:visible {
+ visibility: visible;
+ }
+ `)
+ })
+})
| Dynamic nested groups names webpack error
**What version of Tailwind CSS are you using?**
I'm using the latest v3.2.4
**What build tool (or framework if it abstracts the build tool) are you using?**
I'm using Next.js (v13.1.1) with Webpack (v5.74.0)
**What version of Node.js are you using?**
v18.12.1
**What browser are you using?**
Chrome
**What operating system are you using?**
Windows
**Reproduction URL**
[CodeSandbox bug repoduction](https://codesandbox.io/p/sandbox/gifted-clarke-l6ite9?file=%2Fcomponents%2FContainer.tsx&selection=%5B%7B%22endColumn%22%3A1%2C%22endLineNumber%22%3A27%2C%22startColumn%22%3A1%2C%22startLineNumber%22%3A27%7D%5D)
**Describe your issue**
I tried to assign names to groups by props dynamically on runtime but it failed to compile with this message:
`./node_modules/next/dist/build/webpack/loaders/css-loader/src/index.js??ruleSet[1].rules[3].oneOf[11].use[1]!./node_modules/next/dist/build/webpack/loaders/postcss-loader/src/index.js??ruleSet[1].rules[3].oneOf[11].use[2]!./styles/globals.css
TypeError: Cannot read properties of undefined (reading '5')
at resolveMatches.next (<anonymous>)
at Function.from (<anonymous>)
at runMicrotasks (<anonymous>)`
When i concatenate the `id` prop to a group with `+` operator this error don't come up but nested group doesn't work. I tried every plugin out there but nothing worked naming groups dynamically by props.
| Hey! You can’t use concatenation or interpolation to create class names, as Tailwind generates all of your styles at build time and needs to be able to statically extract every class you’re using from your templates:
https://tailwindcss.com/docs/content-configuration#dynamic-class-names
It shouldn’t cause the build to fail or crash though, so will definitely look into that.
Here's a super minimal reproduction that triggers the error even in Play:
https://play.tailwindcss.com/s8ydiMPxcN
Hey! Thanks for that information, i do a little change and it's working now.
Going to keep this open so we can diagnose the crash 👍🏻 | 2023-01-09T16:08:52 | 3.2 | [] | [
"tests/basic-usage.test.js"
] | TypeScript | [] | [] |
tailwindlabs/tailwindcss | 10,288 | tailwindlabs__tailwindcss-10288 | [
"10267"
] | b05918ab75370bfbecb3d556fec8846cbd285f0d | diff --git a/src/lib/offsets.js b/src/lib/offsets.js
--- a/src/lib/offsets.js
+++ b/src/lib/offsets.js
@@ -13,6 +13,7 @@ import { remapBitfield } from './remap-bitfield.js'
* @property {function | undefined} sort The sort function
* @property {string|null} value The value we want to compare
* @property {string|null} modifier The modifier that was used (if any)
+ * @property {bigint} variant The variant bitmask
*/
/**
@@ -127,6 +128,8 @@ export class Offsets {
* @returns {RuleOffset}
*/
applyVariantOffset(rule, variant, options) {
+ options.variant = variant.variants
+
return {
...rule,
layer: 'variants',
@@ -211,6 +214,19 @@ export class Offsets {
for (let bOptions of b.options) {
if (aOptions.id !== bOptions.id) continue
if (!aOptions.sort || !bOptions.sort) continue
+
+ let maxFnVariant = max([aOptions.variant, bOptions.variant]) ?? 0n
+
+ // Create a mask of 0s from bits 1..N where N represents the mask of the Nth bit
+ let mask = ~(maxFnVariant | (maxFnVariant - 1n))
+ let aVariantsAfterFn = a.variants & mask
+ let bVariantsAfterFn = b.variants & mask
+
+ // If the variants the same, we _can_ sort them
+ if (aVariantsAfterFn !== bVariantsAfterFn) {
+ continue
+ }
+
let result = aOptions.sort(
{
value: aOptions.value,
| diff --git a/tests/arbitrary-variants.test.js b/tests/arbitrary-variants.test.js
--- a/tests/arbitrary-variants.test.js
+++ b/tests/arbitrary-variants.test.js
@@ -1158,3 +1158,201 @@ it('Invalid arbitrary variants selectors should produce nothing instead of faili
expect(result.css).toMatchFormattedCss(css``)
})
})
+
+it('should output responsive variants + stacked variants in the right order', () => {
+ let config = {
+ content: [
+ {
+ raw: html`
+ <div class="xl:p-1"></div>
+ <div class="md:[&_ul]:flex-row"></div>
+ <div class="[&_ul]:flex"></div>
+ <div class="[&_ul]:flex-col"></div>
+ `,
+ },
+ ],
+ corePlugins: { preflight: false },
+ }
+
+ let input = css`
+ @tailwind utilities;
+ `
+
+ return run(input, config).then((result) => {
+ expect(result.css).toMatchFormattedCss(css`
+ @media (min-width: 1280px) {
+ .xl\:p-1 {
+ padding: 0.25rem;
+ }
+ }
+ .\[\&_ul\]\:flex ul {
+ display: flex;
+ }
+ .\[\&_ul\]\:flex-col ul {
+ flex-direction: column;
+ }
+ @media (min-width: 768px) {
+ .md\:\[\&_ul\]\:flex-row ul {
+ flex-direction: row;
+ }
+ }
+ `)
+ })
+})
+
+it('should sort multiple variant fns with normal variants between them', () => {
+ /** @type {string[]} */
+ let lines = []
+
+ for (let a of [1, 2]) {
+ for (let b of [2, 1]) {
+ for (let c of [1, 2]) {
+ for (let d of [2, 1]) {
+ for (let e of [1, 2]) {
+ lines.push(`<div class="fred${a}:qux-[${b}]:baz${c}:bar-[${d}]:foo${e}:p-1"></div>`)
+ }
+ }
+ }
+ }
+ }
+
+ // Fisher-Yates shuffle
+ for (let i = lines.length - 1; i > 0; i--) {
+ let j = Math.floor(Math.random() * i)
+ ;[lines[i], lines[j]] = [lines[j], lines[i]]
+ }
+
+ let config = {
+ content: [
+ {
+ raw: lines.join('\n'),
+ },
+ ],
+ corePlugins: { preflight: false },
+ plugins: [
+ function ({ addVariant, matchVariant }) {
+ addVariant('foo1', '&[data-foo=1]')
+ addVariant('foo2', '&[data-foo=2]')
+
+ matchVariant('bar', (value) => `&[data-bar=${value}]`, {
+ sort: (a, b) => b.value - a.value,
+ })
+
+ addVariant('baz1', '&[data-baz=1]')
+ addVariant('baz2', '&[data-baz=2]')
+
+ matchVariant('qux', (value) => `&[data-qux=${value}]`, {
+ sort: (a, b) => b.value - a.value,
+ })
+
+ addVariant('fred1', '&[data-fred=1]')
+ addVariant('fred2', '&[data-fred=2]')
+ },
+ ],
+ }
+
+ let input = css`
+ @tailwind utilities;
+ `
+
+ return run(input, config).then((result) => {
+ expect(result.css).toMatchFormattedCss(css`
+ .fred1\:qux-\[2\]\:baz1\:bar-\[2\]\:foo1\:p-1[data-foo='1'][data-bar='2'][data-baz='1'][data-qux='2'][data-fred='1'] {
+ padding: 0.25rem;
+ }
+ .fred1\:qux-\[2\]\:baz1\:bar-\[2\]\:foo2\:p-1[data-foo='2'][data-bar='2'][data-baz='1'][data-qux='2'][data-fred='1'] {
+ padding: 0.25rem;
+ }
+ .fred1\:qux-\[2\]\:baz1\:bar-\[1\]\:foo1\:p-1[data-foo='1'][data-bar='1'][data-baz='1'][data-qux='2'][data-fred='1'] {
+ padding: 0.25rem;
+ }
+ .fred1\:qux-\[2\]\:baz1\:bar-\[1\]\:foo2\:p-1[data-foo='2'][data-bar='1'][data-baz='1'][data-qux='2'][data-fred='1'] {
+ padding: 0.25rem;
+ }
+ .fred1\:qux-\[2\]\:baz2\:bar-\[2\]\:foo1\:p-1[data-foo='1'][data-bar='2'][data-baz='2'][data-qux='2'][data-fred='1'] {
+ padding: 0.25rem;
+ }
+ .fred1\:qux-\[2\]\:baz2\:bar-\[2\]\:foo2\:p-1[data-foo='2'][data-bar='2'][data-baz='2'][data-qux='2'][data-fred='1'] {
+ padding: 0.25rem;
+ }
+ .fred1\:qux-\[2\]\:baz2\:bar-\[1\]\:foo1\:p-1[data-foo='1'][data-bar='1'][data-baz='2'][data-qux='2'][data-fred='1'] {
+ padding: 0.25rem;
+ }
+ .fred1\:qux-\[2\]\:baz2\:bar-\[1\]\:foo2\:p-1[data-foo='2'][data-bar='1'][data-baz='2'][data-qux='2'][data-fred='1'] {
+ padding: 0.25rem;
+ }
+ .fred1\:qux-\[1\]\:baz1\:bar-\[2\]\:foo1\:p-1[data-foo='1'][data-bar='2'][data-baz='1'][data-qux='1'][data-fred='1'] {
+ padding: 0.25rem;
+ }
+ .fred1\:qux-\[1\]\:baz1\:bar-\[2\]\:foo2\:p-1[data-foo='2'][data-bar='2'][data-baz='1'][data-qux='1'][data-fred='1'] {
+ padding: 0.25rem;
+ }
+ .fred1\:qux-\[1\]\:baz1\:bar-\[1\]\:foo1\:p-1[data-foo='1'][data-bar='1'][data-baz='1'][data-qux='1'][data-fred='1'] {
+ padding: 0.25rem;
+ }
+ .fred1\:qux-\[1\]\:baz1\:bar-\[1\]\:foo2\:p-1[data-foo='2'][data-bar='1'][data-baz='1'][data-qux='1'][data-fred='1'] {
+ padding: 0.25rem;
+ }
+ .fred1\:qux-\[1\]\:baz2\:bar-\[2\]\:foo1\:p-1[data-foo='1'][data-bar='2'][data-baz='2'][data-qux='1'][data-fred='1'] {
+ padding: 0.25rem;
+ }
+ .fred1\:qux-\[1\]\:baz2\:bar-\[2\]\:foo2\:p-1[data-foo='2'][data-bar='2'][data-baz='2'][data-qux='1'][data-fred='1'] {
+ padding: 0.25rem;
+ }
+ .fred1\:qux-\[1\]\:baz2\:bar-\[1\]\:foo1\:p-1[data-foo='1'][data-bar='1'][data-baz='2'][data-qux='1'][data-fred='1'] {
+ padding: 0.25rem;
+ }
+ .fred1\:qux-\[1\]\:baz2\:bar-\[1\]\:foo2\:p-1[data-foo='2'][data-bar='1'][data-baz='2'][data-qux='1'][data-fred='1'] {
+ padding: 0.25rem;
+ }
+ .fred2\:qux-\[2\]\:baz1\:bar-\[2\]\:foo1\:p-1[data-foo='1'][data-bar='2'][data-baz='1'][data-qux='2'][data-fred='2'] {
+ padding: 0.25rem;
+ }
+ .fred2\:qux-\[2\]\:baz1\:bar-\[2\]\:foo2\:p-1[data-foo='2'][data-bar='2'][data-baz='1'][data-qux='2'][data-fred='2'] {
+ padding: 0.25rem;
+ }
+ .fred2\:qux-\[2\]\:baz1\:bar-\[1\]\:foo1\:p-1[data-foo='1'][data-bar='1'][data-baz='1'][data-qux='2'][data-fred='2'] {
+ padding: 0.25rem;
+ }
+ .fred2\:qux-\[2\]\:baz1\:bar-\[1\]\:foo2\:p-1[data-foo='2'][data-bar='1'][data-baz='1'][data-qux='2'][data-fred='2'] {
+ padding: 0.25rem;
+ }
+ .fred2\:qux-\[2\]\:baz2\:bar-\[2\]\:foo1\:p-1[data-foo='1'][data-bar='2'][data-baz='2'][data-qux='2'][data-fred='2'] {
+ padding: 0.25rem;
+ }
+ .fred2\:qux-\[2\]\:baz2\:bar-\[2\]\:foo2\:p-1[data-foo='2'][data-bar='2'][data-baz='2'][data-qux='2'][data-fred='2'] {
+ padding: 0.25rem;
+ }
+ .fred2\:qux-\[2\]\:baz2\:bar-\[1\]\:foo1\:p-1[data-foo='1'][data-bar='1'][data-baz='2'][data-qux='2'][data-fred='2'] {
+ padding: 0.25rem;
+ }
+ .fred2\:qux-\[2\]\:baz2\:bar-\[1\]\:foo2\:p-1[data-foo='2'][data-bar='1'][data-baz='2'][data-qux='2'][data-fred='2'] {
+ padding: 0.25rem;
+ }
+ .fred2\:qux-\[1\]\:baz1\:bar-\[2\]\:foo1\:p-1[data-foo='1'][data-bar='2'][data-baz='1'][data-qux='1'][data-fred='2'] {
+ padding: 0.25rem;
+ }
+ .fred2\:qux-\[1\]\:baz1\:bar-\[2\]\:foo2\:p-1[data-foo='2'][data-bar='2'][data-baz='1'][data-qux='1'][data-fred='2'] {
+ padding: 0.25rem;
+ }
+ .fred2\:qux-\[1\]\:baz1\:bar-\[1\]\:foo1\:p-1[data-foo='1'][data-bar='1'][data-baz='1'][data-qux='1'][data-fred='2'] {
+ padding: 0.25rem;
+ }
+ .fred2\:qux-\[1\]\:baz1\:bar-\[1\]\:foo2\:p-1[data-foo='2'][data-bar='1'][data-baz='1'][data-qux='1'][data-fred='2'] {
+ padding: 0.25rem;
+ }
+ .fred2\:qux-\[1\]\:baz2\:bar-\[2\]\:foo1\:p-1[data-foo='1'][data-bar='2'][data-baz='2'][data-qux='1'][data-fred='2'] {
+ padding: 0.25rem;
+ }
+ .fred2\:qux-\[1\]\:baz2\:bar-\[2\]\:foo2\:p-1[data-foo='2'][data-bar='2'][data-baz='2'][data-qux='1'][data-fred='2'] {
+ padding: 0.25rem;
+ }
+ .fred2\:qux-\[1\]\:baz2\:bar-\[1\]\:foo1\:p-1[data-foo='1'][data-bar='1'][data-baz='2'][data-qux='1'][data-fred='2'] {
+ padding: 0.25rem;
+ }
+ .fred2\:qux-\[1\]\:baz2\:bar-\[1\]\:foo2\:p-1[data-foo='2'][data-bar='1'][data-baz='2'][data-qux='1'][data-fred='2'] {
+ padding: 0.25rem;
+ }
+ `)
+ })
+})
| Order of CSS selectors is not correct with v3.2.x in specific scenarios
**What version of Tailwind CSS are you using?**
v3.2.4
**What build tool (or framework if it abstracts the build tool) are you using?**
I use Vite. But I was able to reproduce the bug with `npx tailwindcss -i ./src/style.css -o ./dist/style.css`.
**What version of Node.js are you using?**
v16.16.0
**What browser are you using?**
Chrome
**What operating system are you using?**
Windows 10
**Reproduction URL**
https://replit.com/@rahulv3a/Tailwind-bug. Please click on `Run` to run the instance and `Show files` to view the code.
I couldn't replicate it on play.tailwindcss.com because the files are required to be in a specific folder structure to reproduce.
**Describe your issue**
I have a PHP project with hundreds of files. Markup of various components (like menus) is generated by a CMS. As adding classes is not an option, I need to use `[&_]` on the containers to style them. Some layouts break when I update from `v3.1.x` to `v3.2.x` because the CSS order of certain selectors in the CSS generated is wrong.
It occurs only when:
- `[&_]` classes are used with `v3.2.x`. Everything works flawlessly with `v3.1.x`.
- specific conditions are met. I'm still trying to figure them out. But I was able to create an isolated demo for you.
**Example**
In the following markup, the list items should be stacked in SM, and side-by-side from MD onwards. But they stay stacked in all viewports.
```html
<div class="[&_ul]:flex [&_ul]:flex-col md:[&_ul]:flex-row">
<ul>
<li>Item 1</li>
<li>Item 2</li>
<li>Item 3</li>
</ul>
</div>
```
Generated CSS:
```css
@media (min-width: 768px) {
.md\:\[\&_ul\]\:flex-row ul {
flex-direction: row;
}
}
.\[\&_ul\]\:flex ul {
display: flex;
}
.\[\&_ul\]\:flex-col ul {
flex-direction: column;
}
```
Expected CSS:
```css
.\[\&_ul\]\:flex ul {
display: flex;
}
.\[\&_ul\]\:flex-col ul {
flex-direction: column;
}
@media (min-width: 768px) {
.md\:\[\&_ul\]\:flex-row ul {
flex-direction: row;
}
}
```
**Demo**
I created an online demo https://replit.com/@rahulv3a/Tailwind-bug of the example mentioned above. It contains only the code required to reproduce the bug. Please click on `Run` to run the instance and `Show files` to view the code.
- Tailwind setup
- v3.2.4 is being used.
- `yarn tailwindcss -i ./src/style.css -o ./dist/style.css` is used to generate the CSS.
- `preflight` is disabled to keep things simple.
- Conditions
- tailwind.config.js
- `content` has `["./inc/**/*.php", "./templates/**/*.php"]`.
- The order of the paths is important to replicate the issue.
- inc/functions.php
- It contains two comments. In my project, `./inc` contains hundreds of functions that are documented in detail. The comments contain words like contents, lowercase, etc. Tailwind creates classes for them which is fine. But for some reason unknown, the comments that start with `//` interfere with the order of CSS selectors.
- template/01-required.php
- I don't know why but this code is required to replicate the issue.
- template/component.php
- This file contains the actual code mentioned in the example above.
**More examples**
Here are some more examples of the bug I experience in my project with `v3.2.x`:
- `<br>` stays hidden in all viewports.
```html
<h1 class="[&_br]:hidden [&_br]:xl:block">
Lorem<br />
Ipsum
</h1>
```
- `<img>` stays full width in all viewports.
```html
<figure class="[&_img]:w-full md:[&_img]:w-[300px] xl:[&_img]:w-[340px]">
<img src="./image.jpg" alt="some image" />
</figure>
```
I can create isolated demos for each of them if it helps you debug.
Please let me know if you need any other information.
| Thanks for reporting, I was able to distill the reproduction down to this minimal demo on Tailwind Play:
https://play.tailwindcss.com/3ujiz5LanM
Can see the sorting issue in the "Generated CSS" tab. Will look at this one next week!
Thanks for the reporting... | 2023-01-10T14:50:56 | 3.2 | [] | [
"tests/arbitrary-variants.test.js"
] | TypeScript | [] | [] |
tailwindlabs/tailwindcss | 10,400 | tailwindlabs__tailwindcss-10400 | [
"10353"
] | 667eac5e8845e3928055351e747606fa8864603f | diff --git a/src/corePlugins.js b/src/corePlugins.js
--- a/src/corePlugins.js
+++ b/src/corePlugins.js
@@ -168,7 +168,30 @@ export let variantPlugins = {
if (!result.includes('&')) result = '&' + result
let [a, b] = fn('', extra)
- return result.replace(/&(\S+)?/g, (_, pseudo = '') => a + pseudo + b)
+
+ let start = null
+ let end = null
+ let quotes = 0
+
+ for (let i = 0; i < result.length; ++i) {
+ let c = result[i]
+ if (c === '&') {
+ start = i
+ } else if (c === "'" || c === '"') {
+ quotes += 1
+ } else if (start !== null && c === ' ' && !quotes) {
+ end = i
+ }
+ }
+
+ if (start !== null && end === null) {
+ end = result.length
+ }
+
+ // Basically this but can handle quotes:
+ // result.replace(/&(\S+)?/g, (_, pseudo = '') => a + pseudo + b)
+
+ return result.slice(0, start) + a + result.slice(start + 1, end) + b + result.slice(end)
},
{ values: Object.fromEntries(pseudoVariants) }
)
diff --git a/src/lib/generateRules.js b/src/lib/generateRules.js
--- a/src/lib/generateRules.js
+++ b/src/lib/generateRules.js
@@ -152,10 +152,18 @@ function applyVariant(variant, matches, context) {
// Retrieve "modifier"
{
- let match = /(.*)\/(.*)$/g.exec(variant)
- if (match && !context.variantMap.has(variant)) {
- variant = match[1]
- args.modifier = match[2]
+ let [baseVariant, ...modifiers] = splitAtTopLevelOnly(variant, '/')
+
+ // This is a hack to support variants with `/` in them, like `ar-1/10/20:text-red-500`
+ // In this case 1/10 is a value but /20 is a modifier
+ if (modifiers.length > 1) {
+ baseVariant = baseVariant + '/' + modifiers.slice(0, -1).join('/')
+ modifiers = modifiers.slice(-1)
+ }
+
+ if (modifiers.length && !context.variantMap.has(variant)) {
+ variant = baseVariant
+ args.modifier = modifiers[0]
if (!flagEnabled(context.tailwindConfig, 'generalizedModifiers')) {
return []
| diff --git a/tests/basic-usage.test.js b/tests/basic-usage.test.js
--- a/tests/basic-usage.test.js
+++ b/tests/basic-usage.test.js
@@ -949,4 +949,66 @@ crosscheck(({ stable, oxide }) => {
`)
})
})
+
+ test('detects quoted arbitrary values containing a slash', async () => {
+ let config = {
+ content: [
+ {
+ raw: html`<div class="group-[[href^='/']]:hidden"></div>`,
+ },
+ ],
+ }
+
+ let input = css`
+ @tailwind utilities;
+ `
+
+ let result = await run(input, config)
+
+ oxide.expect(result.css).toMatchFormattedCss(css`
+ .group[href^='/'] .group-\[\[href\^\=\'\/\'\]\]\:hidden {
+ display: none;
+ }
+ `)
+
+ stable.expect(result.css).toMatchFormattedCss(css`
+ .hidden {
+ display: none;
+ }
+ .group[href^='/'] .group-\[\[href\^\=\'\/\'\]\]\:hidden {
+ display: none;
+ }
+ `)
+ })
+
+ test('handled quoted arbitrary values containing escaped spaces', async () => {
+ let config = {
+ content: [
+ {
+ raw: html`<div class="group-[[href^='_bar']]:hidden"></div>`,
+ },
+ ],
+ }
+
+ let input = css`
+ @tailwind utilities;
+ `
+
+ let result = await run(input, config)
+
+ oxide.expect(result.css).toMatchFormattedCss(css`
+ .group[href^=' bar'] .group-\[\[href\^\=\'_bar\'\]\]\:hidden {
+ display: none;
+ }
+ `)
+
+ stable.expect(result.css).toMatchFormattedCss(css`
+ .hidden {
+ display: none;
+ }
+ .group[href^=' bar'] .group-\[\[href\^\=\'_bar\'\]\]\:hidden {
+ display: none;
+ }
+ `)
+ })
})
diff --git a/tests/util/run.js b/tests/util/run.js
--- a/tests/util/run.js
+++ b/tests/util/run.js
@@ -60,6 +60,18 @@ let nullProxy = new Proxy(
}
)
+/**
+ * @typedef {object} CrossCheck
+ * @property {typeof import('@jest/globals')} oxide
+ * @property {typeof import('@jest/globals')} stable
+ * @property {object} engine
+ * @property {boolean} engine.oxide
+ * @property {boolean} engine.stable
+ */
+
+/**
+ * @param {(data: CrossCheck) => void} fn
+ */
export function crosscheck(fn) {
let engines =
env.ENGINE === 'oxide' ? [{ engine: 'Stable' }, { engine: 'Oxide' }] : [{ engine: 'Stable' }]
| double escape happening in attribute value selectors
<!-- Please provide all of the information requested below. We're a small team and without all of this information it's not possible for us to help and your bug report will be closed. -->
**What version of Tailwind CSS are you using?**
v3.2.4
**What build tool (or framework if it abstracts the build tool) are you using?**
postcss 8.4.19
**What version of Node.js are you using?**
v14.19.1
**What browser are you using?**
Chrome
**What operating system are you using?**
Kubuntu Linux
**Reproduction URL**
https://play.tailwindcss.com/sRVqoiJfLV
**Describe your issue**
in the attribute value of a attribute selector the value is being double escaped and there appears to be no way to un-escape it.
| 2023-01-23T16:11:12 | 3.2 | [] | [
"tests/basic-usage.test.js"
] | TypeScript | [] | [
"https://play.tailwindcss.com/sRVqoiJfLV"
] |
|
tailwindlabs/tailwindcss | 10,601 | tailwindlabs__tailwindcss-10601 | [
"10582"
] | 66c640b73599e36c6087644d3b2c231cc17b37ff | diff --git a/src/lib/generateRules.js b/src/lib/generateRules.js
--- a/src/lib/generateRules.js
+++ b/src/lib/generateRules.js
@@ -3,10 +3,14 @@ import selectorParser from 'postcss-selector-parser'
import parseObjectStyles from '../util/parseObjectStyles'
import isPlainObject from '../util/isPlainObject'
import prefixSelector from '../util/prefixSelector'
-import { updateAllClasses, filterSelectorsForClass, getMatchingTypes } from '../util/pluginUtils'
+import { updateAllClasses, getMatchingTypes } from '../util/pluginUtils'
import log from '../util/log'
import * as sharedState from './sharedState'
-import { formatVariantSelector, finalizeSelector } from '../util/formatVariantSelector'
+import {
+ formatVariantSelector,
+ finalizeSelector,
+ eliminateIrrelevantSelectors,
+} from '../util/formatVariantSelector'
import { asClass } from '../util/nameClass'
import { normalize } from '../util/dataTypes'
import { isValidVariantFormatString, parseVariant } from './setupContextUtils'
@@ -111,22 +115,28 @@ function applyImportant(matches, classCandidate) {
if (matches.length === 0) {
return matches
}
+
let result = []
for (let [meta, rule] of matches) {
let container = postcss.root({ nodes: [rule.clone()] })
+
container.walkRules((r) => {
- r.selector = updateAllClasses(
- filterSelectorsForClass(r.selector, classCandidate),
- (className) => {
- if (className === classCandidate) {
- return `!${className}`
- }
- return className
- }
+ let ast = selectorParser().astSync(r.selector)
+
+ // Remove extraneous selectors that do not include the base candidate
+ ast.each((sel) => eliminateIrrelevantSelectors(sel, classCandidate))
+
+ // Update all instances of the base candidate to include the important marker
+ updateAllClasses(ast, (className) =>
+ className === classCandidate ? `!${className}` : className
)
+
+ r.selector = ast.toString()
+
r.walkDecls((d) => (d.important = true))
})
+
result.push([{ ...meta, important: true }, container.nodes[0]])
}
diff --git a/src/util/formatVariantSelector.js b/src/util/formatVariantSelector.js
--- a/src/util/formatVariantSelector.js
+++ b/src/util/formatVariantSelector.js
@@ -120,7 +120,7 @@ function resortSelector(sel) {
* @param {Selector} ast
* @param {string} base
*/
-function eliminateIrrelevantSelectors(sel, base) {
+export function eliminateIrrelevantSelectors(sel, base) {
let hasClassesMatchingCandidate = false
sel.walk((child) => {
diff --git a/src/util/pluginUtils.js b/src/util/pluginUtils.js
--- a/src/util/pluginUtils.js
+++ b/src/util/pluginUtils.js
@@ -1,4 +1,3 @@
-import selectorParser from 'postcss-selector-parser'
import escapeCommas from './escapeCommas'
import { withAlphaValue } from './withAlphaVariable'
import {
@@ -21,37 +20,19 @@ import negateValue from './negateValue'
import { backgroundSize } from './validateFormalSyntax'
import { flagEnabled } from '../featureFlags.js'
+/**
+ * @param {import('postcss-selector-parser').Container} selectors
+ * @param {(className: string) => string} updateClass
+ * @returns {string}
+ */
export function updateAllClasses(selectors, updateClass) {
- let parser = selectorParser((selectors) => {
- selectors.walkClasses((sel) => {
- let updatedClass = updateClass(sel.value)
- sel.value = updatedClass
- if (sel.raws && sel.raws.value) {
- sel.raws.value = escapeCommas(sel.raws.value)
- }
- })
- })
-
- let result = parser.processSync(selectors)
+ selectors.walkClasses((sel) => {
+ sel.value = updateClass(sel.value)
- return result
-}
-
-export function filterSelectorsForClass(selectors, classCandidate) {
- let parser = selectorParser((selectors) => {
- selectors.each((sel) => {
- const containsClass = sel.nodes.some(
- (node) => node.type === 'class' && node.value === classCandidate
- )
- if (!containsClass) {
- sel.remove()
- }
- })
+ if (sel.raws && sel.raws.value) {
+ sel.raws.value = escapeCommas(sel.raws.value)
+ }
})
-
- let result = parser.processSync(selectors)
-
- return result
}
function resolveArbitraryValue(modifier, validate) {
| diff --git a/tests/important-modifier.test.js b/tests/important-modifier.test.js
--- a/tests/important-modifier.test.js
+++ b/tests/important-modifier.test.js
@@ -108,4 +108,46 @@ crosscheck(() => {
`)
})
})
+
+ test('the important modifier works on utilities using :where()', () => {
+ let config = {
+ content: [
+ {
+ raw: html` <div class="btn hover:btn !btn hover:focus:disabled:!btn"></div> `,
+ },
+ ],
+ corePlugins: { preflight: false },
+ plugins: [
+ function ({ addComponents }) {
+ addComponents({
+ ':where(.btn)': {
+ backgroundColor: '#00f',
+ },
+ })
+ },
+ ],
+ }
+
+ let input = css`
+ @tailwind components;
+ @tailwind utilities;
+ `
+
+ return run(input, config).then((result) => {
+ expect(result.css).toMatchFormattedCss(css`
+ :where(.\!btn) {
+ background-color: #00f !important;
+ }
+ :where(.btn) {
+ background-color: #00f;
+ }
+ :where(.hover\:btn:hover) {
+ background-color: #00f;
+ }
+ :where(.hover\:focus\:disabled\:\!btn:disabled:focus:hover) {
+ background-color: #00f !important;
+ }
+ `)
+ })
+ })
})
| Using `:where(.anything)` in a plugin and having `!anything` inside HTML, creates invalid CSS
<!-- Please provide all of the information requested below. We're a small team and without all of this information it's not possible for us to help and your bug report will be closed. -->
**What version of Tailwind CSS are you using?**
3.2.6
**What build tool (or framework if it abstracts the build tool) are you using?**
Tailwind Play (or any tool)
**Reproduction URL**
https://play.tailwindcss.com/Oy7NHRkftL?file=config
**Describe your issue**
If there is string with `!` prefix with the same class from a plugin that has a `:where()` selector, Tailwind creates invalid CSS
HTML:
```
!btn
```
tailwind.config.js:
```js
const plugin = require('tailwindcss/plugin')
module.exports = {
plugins: [
plugin(function({ addComponents }) {
addComponents({
'.btn': {
backgroundColor: 'red',
},
':where(.btn)': {
backgroundColor: 'blue',
},
})
})
]
}
```
Generated CSS:
```css
.\!btn {
background-color: red !important
}
{
background-color: blue !important
}
```
As you can see it can't apply important to the `:where()` selector and it generates an empty selector.
| Thanks for reporting! Here's a slightly simplified reproduction for our own reference:
https://play.tailwindcss.com/4MqOxcleAv?file=config | 2023-02-16T14:48:07 | 3.2 | [] | [
"tests/important-modifier.test.js"
] | TypeScript | [] | [
"https://play.tailwindcss.com/Oy7NHRkftL?file=config"
] |
tailwindlabs/tailwindcss | 10,604 | tailwindlabs__tailwindcss-10604 | [
"10591"
] | 17159ff6c28b2d9700fa489dddf4d6f8d8d6f376 | diff --git a/stubs/defaultConfig.stub.js b/stubs/defaultConfig.stub.js
--- a/stubs/defaultConfig.stub.js
+++ b/stubs/defaultConfig.stub.js
@@ -879,8 +879,8 @@ module.exports = {
none: 'none',
all: 'all',
DEFAULT:
- 'color, background-color, border-color, outline-color, text-decoration-color, fill, stroke, opacity, box-shadow, transform, filter, backdrop-filter',
- colors: 'color, background-color, border-color, outline-color, text-decoration-color, fill, stroke',
+ 'color, background-color, border-color, text-decoration-color, fill, stroke, opacity, box-shadow, transform, filter, backdrop-filter',
+ colors: 'color, background-color, border-color, text-decoration-color, fill, stroke',
opacity: 'opacity',
shadow: 'box-shadow',
transform: 'transform',
| diff --git a/tests/basic-usage.oxide.test.css b/tests/basic-usage.oxide.test.css
--- a/tests/basic-usage.oxide.test.css
+++ b/tests/basic-usage.oxide.test.css
@@ -1020,8 +1020,8 @@
backdrop-filter: none;
}
.transition {
- transition-property: color, background-color, border-color, outline-color, text-decoration-color,
- fill, stroke, opacity, box-shadow, transform, filter, backdrop-filter;
+ transition-property: color, background-color, border-color, text-decoration-color, fill, stroke,
+ opacity, box-shadow, transform, filter, backdrop-filter;
transition-duration: 0.15s;
transition-timing-function: cubic-bezier(0.4, 0, 0.2, 1);
}
diff --git a/tests/basic-usage.test.css b/tests/basic-usage.test.css
--- a/tests/basic-usage.test.css
+++ b/tests/basic-usage.test.css
@@ -1020,8 +1020,8 @@
backdrop-filter: none;
}
.transition {
- transition-property: color, background-color, border-color, outline-color, text-decoration-color,
- fill, stroke, opacity, box-shadow, transform, filter, backdrop-filter;
+ transition-property: color, background-color, border-color, text-decoration-color, fill, stroke,
+ opacity, box-shadow, transform, filter, backdrop-filter;
transition-duration: 0.15s;
transition-timing-function: cubic-bezier(0.4, 0, 0.2, 1);
}
diff --git a/tests/kitchen-sink.test.js b/tests/kitchen-sink.test.js
--- a/tests/kitchen-sink.test.js
+++ b/tests/kitchen-sink.test.js
@@ -698,9 +698,8 @@ crosscheck(() => {
}
@media (prefers-reduced-motion: no-preference) {
.motion-safe\:transition {
- transition-property: color, background-color, border-color, outline-color,
- text-decoration-color, fill, stroke, opacity, box-shadow, transform, filter,
- backdrop-filter;
+ transition-property: color, background-color, border-color, text-decoration-color, fill,
+ stroke, opacity, box-shadow, transform, filter, backdrop-filter;
transition-duration: 0.15s;
transition-timing-function: cubic-bezier(0.4, 0, 0.2, 1);
}
@@ -710,9 +709,8 @@ crosscheck(() => {
}
@media (prefers-reduced-motion: reduce) {
.motion-reduce\:transition {
- transition-property: color, background-color, border-color, outline-color,
- text-decoration-color, fill, stroke, opacity, box-shadow, transform, filter,
- backdrop-filter;
+ transition-property: color, background-color, border-color, text-decoration-color, fill,
+ stroke, opacity, box-shadow, transform, filter, backdrop-filter;
transition-duration: 0.15s;
transition-timing-function: cubic-bezier(0.4, 0, 0.2, 1);
}
@@ -759,9 +757,8 @@ crosscheck(() => {
}
@media (prefers-reduced-motion: no-preference) {
.md\:motion-safe\:hover\:transition:hover {
- transition-property: color, background-color, border-color, outline-color,
- text-decoration-color, fill, stroke, opacity, box-shadow, transform, filter,
- backdrop-filter;
+ transition-property: color, background-color, border-color, text-decoration-color,
+ fill, stroke, opacity, box-shadow, transform, filter, backdrop-filter;
transition-duration: 0.15s;
transition-timing-function: cubic-bezier(0.4, 0, 0.2, 1);
}
diff --git a/tests/raw-content.oxide.test.css b/tests/raw-content.oxide.test.css
--- a/tests/raw-content.oxide.test.css
+++ b/tests/raw-content.oxide.test.css
@@ -761,8 +761,8 @@
backdrop-filter: none;
}
.transition {
- transition-property: color, background-color, border-color, outline-color, text-decoration-color,
- fill, stroke, opacity, box-shadow, transform, filter, backdrop-filter;
+ transition-property: color, background-color, border-color, text-decoration-color, fill, stroke,
+ opacity, box-shadow, transform, filter, backdrop-filter;
transition-duration: 0.15s;
transition-timing-function: cubic-bezier(0.4, 0, 0.2, 1);
}
diff --git a/tests/raw-content.test.css b/tests/raw-content.test.css
--- a/tests/raw-content.test.css
+++ b/tests/raw-content.test.css
@@ -761,8 +761,8 @@
backdrop-filter: none;
}
.transition {
- transition-property: color, background-color, border-color, outline-color, text-decoration-color,
- fill, stroke, opacity, box-shadow, transform, filter, backdrop-filter;
+ transition-property: color, background-color, border-color, text-decoration-color, fill, stroke,
+ opacity, box-shadow, transform, filter, backdrop-filter;
transition-duration: 0.15s;
transition-timing-function: cubic-bezier(0.4, 0, 0.2, 1);
}
| Input outlines show during transition with Tailwind 3.2.6
### What version of @tailwindcss/forms are you using?
0.5.3
### What version of Node.js are you using?
18.13.0
### What browser are you using?
Chrome
### What operating system are you using?
Windows
### Reproduction repository
https://play.tailwindcss.com/irT3FCtMUB
### Describe your issue
Since https://github.com/tailwindlabs/tailwindcss/issues/10385 was merged inputs that use this plugin and the `transition` class receive an outline ring in addition to the box-shadow ring during their transition animation.
The way to fix this is to add `outline-none` to the input or apply that to all inputs in your style sheet.
`@tailwindcss/forms` sets the input's outline style to none, but only on the `:focus` state. For this to not be included in the transition it needs to be set as a base input style.
This caught me off guard when updating from Tailwind 3.2.4 to 3.2.6 and I thought it was a bug in that release. After figuring out what's going on it seems like something that should probably be fixed in this plugin since it's setting a default outline style.
| This issue is also visible on Heroicons in `Search all icons...` input
https://heroicons.com/
I too noticed flickering when `focus:outline-none` and `transition` are used at the same time.
Laravel Jetstream uses this combination.
There are two ways for a temporary fix:
- remove `outline-color` from `transition-property`
- use `outline-none` without `focus`
Thanks for reporting — working on a solution to this, will have a fix out today. | 2023-02-16T17:20:27 | 3.2 | [] | [
"tests/kitchen-sink.test.js"
] | TypeScript | [] | [
"https://play.tailwindcss.com/irT3FCtMUB"
] |
tailwindlabs/tailwindcss | 10,903 | tailwindlabs__tailwindcss-10903 | [
"10899"
] | a785c93b54c770b65364a8c781761dd97ac684d5 | diff --git a/src/lib/expandApplyAtRules.js b/src/lib/expandApplyAtRules.js
--- a/src/lib/expandApplyAtRules.js
+++ b/src/lib/expandApplyAtRules.js
@@ -4,6 +4,7 @@ import parser from 'postcss-selector-parser'
import { resolveMatches } from './generateRules'
import escapeClassName from '../util/escapeClassName'
import { applyImportantSelector } from '../util/applyImportantSelector'
+import { collectPseudoElements, sortSelector } from '../util/formatVariantSelector.js'
/** @typedef {Map<string, [any, import('postcss').Rule[]]>} ApplyCache */
@@ -562,6 +563,17 @@ function processApply(root, context, localCache) {
rule.walkDecls((d) => {
d.important = meta.important || important
})
+
+ // Move pseudo elements to the end of the selector (if necessary)
+ let selector = parser().astSync(rule.selector)
+ selector.each((sel) => {
+ let [pseudoElements] = collectPseudoElements(sel)
+ if (pseudoElements.length > 0) {
+ sel.nodes.push(...pseudoElements.sort(sortSelector))
+ }
+ })
+
+ rule.selector = selector.toString()
})
}
diff --git a/src/util/applyImportantSelector.js b/src/util/applyImportantSelector.js
--- a/src/util/applyImportantSelector.js
+++ b/src/util/applyImportantSelector.js
@@ -1,19 +1,31 @@
import { splitAtTopLevelOnly } from './splitAtTopLevelOnly'
+import parser from 'postcss-selector-parser'
+import { collectPseudoElements, sortSelector } from './formatVariantSelector.js'
export function applyImportantSelector(selector, important) {
- let matches = /^(.*?)(:before|:after|::[\w-]+)(\)*)$/g.exec(selector)
- if (!matches) return `${important} ${wrapWithIs(selector)}`
+ let sel = parser().astSync(selector)
- let [, before, pseudo, brackets] = matches
- return `${important} ${wrapWithIs(before + brackets)}${pseudo}`
-}
+ sel.each((sel) => {
+ // Wrap with :is if it's not already wrapped
+ let isWrapped =
+ sel.nodes[0].type === 'pseudo' &&
+ sel.nodes[0].value === ':is' &&
+ sel.nodes.every((node) => node.type !== 'combinator')
-function wrapWithIs(selector) {
- let parts = splitAtTopLevelOnly(selector, ' ')
+ if (!isWrapped) {
+ sel.nodes = [
+ parser.pseudo({
+ value: ':is',
+ nodes: [sel.clone()],
+ }),
+ ]
+ }
- if (parts.length === 1 && parts[0].startsWith(':is(') && parts[0].endsWith(')')) {
- return selector
- }
+ let [pseudoElements] = collectPseudoElements(sel)
+ if (pseudoElements.length > 0) {
+ sel.nodes.push(...pseudoElements.sort(sortSelector))
+ }
+ })
- return `:is(${selector})`
+ return `${important} ${sel.toString()}`
}
diff --git a/src/util/formatVariantSelector.js b/src/util/formatVariantSelector.js
--- a/src/util/formatVariantSelector.js
+++ b/src/util/formatVariantSelector.js
@@ -246,9 +246,9 @@ export function finalizeSelector(current, formats, { context, candidate, base })
// Move pseudo elements to the end of the selector (if necessary)
selector.each((sel) => {
- let pseudoElements = collectPseudoElements(sel)
+ let [pseudoElements] = collectPseudoElements(sel)
if (pseudoElements.length > 0) {
- sel.nodes.push(pseudoElements.sort(sortSelector))
+ sel.nodes.push(...pseudoElements.sort(sortSelector))
}
})
@@ -351,23 +351,45 @@ let pseudoElementExceptions = [
* `::before:hover` doesn't work, which means that we can make it work for you by flipping the order.
*
* @param {Selector} selector
+ * @param {boolean} force
**/
-function collectPseudoElements(selector) {
+export function collectPseudoElements(selector, force = false) {
/** @type {Node[]} */
let nodes = []
+ let seenPseudoElement = null
- for (let node of selector.nodes) {
- if (isPseudoElement(node)) {
+ for (let node of [...selector.nodes]) {
+ if (isPseudoElement(node, force)) {
nodes.push(node)
selector.removeChild(node)
+ seenPseudoElement = node.value
+ } else if (seenPseudoElement !== null) {
+ if (pseudoElementExceptions.includes(seenPseudoElement) && isPseudoClass(node, force)) {
+ nodes.push(node)
+ selector.removeChild(node)
+ } else {
+ seenPseudoElement = null
+ }
}
if (node?.nodes) {
- nodes.push(...collectPseudoElements(node))
+ let hasPseudoElementRestrictions =
+ node.type === 'pseudo' && (node.value === ':is' || node.value === ':has')
+
+ let [collected, seenPseudoElementInSelector] = collectPseudoElements(
+ node,
+ force || hasPseudoElementRestrictions
+ )
+
+ if (seenPseudoElementInSelector) {
+ seenPseudoElement = seenPseudoElementInSelector
+ }
+
+ nodes.push(...collected)
}
}
- return nodes
+ return [nodes, seenPseudoElement]
}
// This will make sure to move pseudo's to the correct spot (the end for
@@ -380,7 +402,7 @@ function collectPseudoElements(selector) {
//
// `::before:hover` doesn't work, which means that we can make it work
// for you by flipping the order.
-function sortSelector(a, z) {
+export function sortSelector(a, z) {
// Both nodes are non-pseudo's so we can safely ignore them and keep
// them in the same order.
if (a.type !== 'pseudo' && z.type !== 'pseudo') {
@@ -404,9 +426,13 @@ function sortSelector(a, z) {
return isPseudoElement(a) - isPseudoElement(z)
}
-function isPseudoElement(node) {
+function isPseudoElement(node, force = false) {
if (node.type !== 'pseudo') return false
- if (pseudoElementExceptions.includes(node.value)) return false
+ if (pseudoElementExceptions.includes(node.value) && !force) return false
return node.value.startsWith('::') || pseudoElementsBC.includes(node.value)
}
+
+function isPseudoClass(node, force) {
+ return node.type === 'pseudo' && !isPseudoElement(node, force)
+}
| diff --git a/tests/apply.test.js b/tests/apply.test.js
--- a/tests/apply.test.js
+++ b/tests/apply.test.js
@@ -2357,4 +2357,74 @@ crosscheck(({ stable, oxide }) => {
`)
})
})
+
+ it('pseudo elements inside apply are moved outside of :is() or :has()', () => {
+ let config = {
+ darkMode: 'class',
+ content: [
+ {
+ raw: html` <div class="foo bar baz qux steve bob"></div> `,
+ },
+ ],
+ }
+
+ let input = css`
+ .foo::before {
+ @apply dark:bg-black/100;
+ }
+
+ .bar::before {
+ @apply rtl:dark:bg-black/100;
+ }
+
+ .baz::before {
+ @apply rtl:dark:hover:bg-black/100;
+ }
+
+ .qux::file-selector-button {
+ @apply rtl:dark:hover:bg-black/100;
+ }
+
+ .steve::before {
+ @apply rtl:hover:dark:bg-black/100;
+ }
+
+ .bob::file-selector-button {
+ @apply rtl:hover:dark:bg-black/100;
+ }
+
+ .foo::before {
+ @apply [:has([dir="rtl"]_&)]:hover:bg-black/100;
+ }
+
+ .bar::file-selector-button {
+ @apply [:has([dir="rtl"]_&)]:hover:bg-black/100;
+ }
+ `
+
+ return run(input, config).then((result) => {
+ expect(result.css).toMatchFormattedCss(css`
+ :is(.dark .foo)::before,
+ :is([dir='rtl'] :is(.dark .bar))::before,
+ :is([dir='rtl'] :is(.dark .baz:hover))::before {
+ background-color: #000;
+ }
+ :is([dir='rtl'] :is(.dark .qux))::file-selector-button:hover {
+ background-color: #000;
+ }
+ :is([dir='rtl'] :is(.dark .steve):hover):before {
+ background-color: #000;
+ }
+ :is([dir='rtl'] :is(.dark .bob))::file-selector-button:hover {
+ background-color: #000;
+ }
+ :has([dir='rtl'] .foo:hover):before {
+ background-color: #000;
+ }
+ :has([dir='rtl'] .bar)::file-selector-button:hover {
+ background-color: #000;
+ }
+ `)
+ })
+ })
})
diff --git a/tests/important-selector.test.js b/tests/important-selector.test.js
--- a/tests/important-selector.test.js
+++ b/tests/important-selector.test.js
@@ -21,6 +21,7 @@ crosscheck(({ stable, oxide }) => {
<div class="group-hover:focus-within:text-left"></div>
<div class="rtl:active:text-center"></div>
<div class="dark:before:underline"></div>
+ <div class="hover:[&::file-selector-button]:rtl:dark:bg-black/100"></div>
`,
},
],
@@ -155,6 +156,12 @@ crosscheck(({ stable, oxide }) => {
text-align: right;
}
}
+ #app
+ :is(
+ [dir='rtl'] :is(.dark .hover\:\[\&\:\:file-selector-button\]\:rtl\:dark\:bg-black\/100)
+ )::file-selector-button:hover {
+ background-color: #000;
+ }
`)
})
})
| after update from 3.2.7 to 3.3.0 pseudo elements `after` and `before` doesn't get `dark: ...` anymore
<!-- Please provide all of the information requested below. We're a small team and without all of this information it's not possible for us to help and your bug report will be closed. -->
**What version of Tailwind CSS are you using?**
3.3.0
**What build tool (or framework if it abstracts the build tool) are you using?**
astro v2.1.8
**What version of Node.js are you using?**
19.8.1
**What browser are you using?**
Chrome 111
**What operating system are you using?**
Zorin OS 16.2
**Describe your issue**
after update every `after` and `before` pseudo element doesn't get any `dark` modifier value, for example if i write `... bg-gray-800 dark:bg-gray-100 ...` it get only `bg-gray-800` on version 3.2.7 it works as usual
| Hey, thank you for this bug report! 🙏
Can you please provide a minimal reproduction repo that shows the issue?
Trying to reproduce it and seems to work as expected: https://play.tailwindcss.com/d5HtkTSI6a
> Hey, thank you for this bug report! 🙏
>
> Can you please provide a minimal reproduction repo that shows the issue?
>
> Trying to reproduce it and seems to work as expected: https://play.tailwindcss.com/d5HtkTSI6a
I have reproduced a case here: https://play.tailwindcss.com/tsNrZ1Qtm5?file=css
> Hey, thank you for this bug report! pray
>
> Can you please provide a minimal reproduction repo that shows the issue?
>
> Trying to reproduce it and seems to work as expected: https://play.tailwindcss.com/d5HtkTSI6a
I tried to extract and abstract part of code of my use case: https://play.tailwindcss.com/ytDMhpXmfR?file=css | 2023-03-29T14:53:21 | 3.3 | [] | [
"tests/important-selector.test.js",
"tests/apply.test.js"
] | TypeScript | [] | [] |
tailwindlabs/tailwindcss | 10,943 | tailwindlabs__tailwindcss-10943 | [
"10937"
] | d7310491b5cd2c0fe96b398de8bbb6caf2bdee4d | diff --git a/src/util/formatVariantSelector.js b/src/util/formatVariantSelector.js
--- a/src/util/formatVariantSelector.js
+++ b/src/util/formatVariantSelector.js
@@ -337,6 +337,9 @@ let pseudoElementExceptions = [
'::-webkit-scrollbar-track-piece',
'::-webkit-scrollbar-corner',
'::-webkit-resizer',
+
+ // Old-style Angular Shadow DOM piercing pseudo element
+ '::ng-deep',
]
/**
| diff --git a/tests/apply.test.js b/tests/apply.test.js
--- a/tests/apply.test.js
+++ b/tests/apply.test.js
@@ -2427,4 +2427,34 @@ crosscheck(({ stable, oxide }) => {
`)
})
})
+
+ stable.test('::ng-deep pseudo element is left alone', () => {
+ let config = {
+ darkMode: 'class',
+ content: [
+ {
+ raw: html` <div class="foo bar"></div> `,
+ },
+ ],
+ }
+
+ let input = css`
+ ::ng-deep .foo .bar {
+ @apply font-bold;
+ }
+ `
+
+ return run(input, config).then((result) => {
+ expect(result.css).toMatchFormattedCss(css`
+ ::ng-deep .foo .bar {
+ font-weight: 700;
+ }
+ `)
+ })
+ })
+
+ // 1. `::ng-deep` is deprecated
+ // 2. It uses invalid selector syntax that Lightning CSS does not support
+ // It may be enough for Oxide to not support it at all
+ oxide.test.todo('::ng-deep pseudo element is left alone')
})
| @apply method is invalid after upgrade to 3.3.1
<!-- Please provide all of the information requested below. We're a small team and without all of this information it's not possible for us to help and your bug report will be closed. -->
**What version of Tailwind CSS are you using?**
v3.3.1
**What build tool (or framework if it abstracts the build tool) are you using?**
webpack 5.77.0
**What version of Node.js are you using?**
v16.16.0
**What browser are you using?**
Chrome
**What operating system are you using?**
macOS
**Reproduction URL**
https://github.com/Postcatlab/postcat/blob/3411792892633e5c53d4e86466a0fd52f4599f4a/src/browser/src/app/pages/workspace/overview/project-list/project-list.component.scss
**Describe your issue**
My previous version was 3.2.4 After upgrading to the latest version of 3.3.1, @apply is invalid.
* Angular 15.2.5
```scss
:host {
.operate-btn-list {
display: none;
}
.ant-card {
@include hover-item;
}
::ng-deep {
.ant-list-items {
.ant-list-item {
@apply px-[10px] rounded-[3px];
@include hover-item;
border: 1px solid transparent;
}
}
}
}
```
previous display
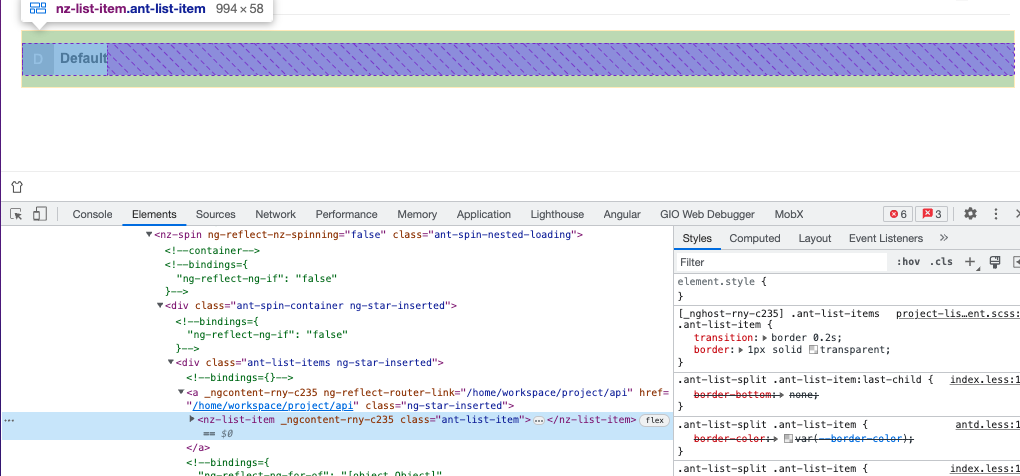
current display
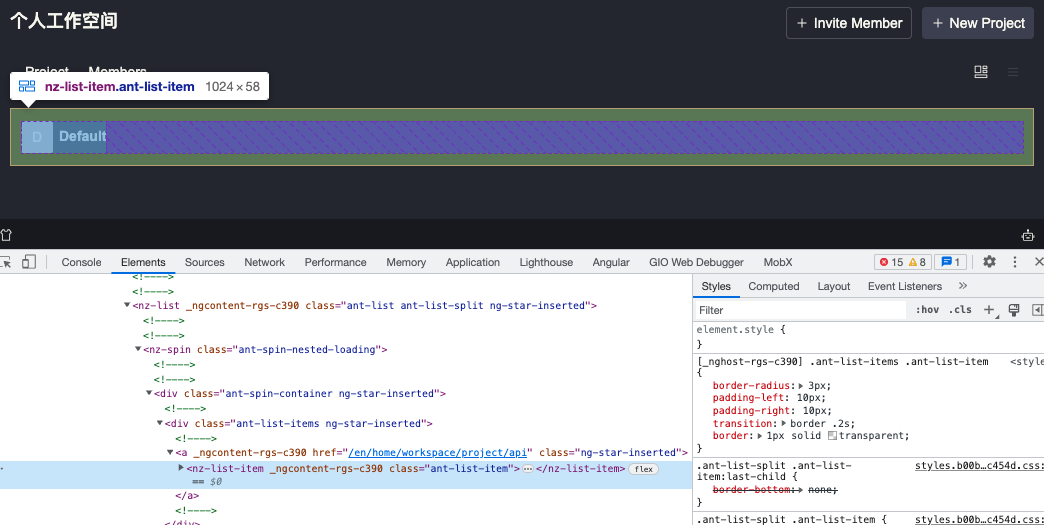
| 2023-04-04T16:29:09 | 3.3 | [] | [
"tests/apply.test.js"
] | TypeScript | [
"https://user-images.githubusercontent.com/15225835/229733712-d9d19b15-8461-4113-b2a5-7298d8cb71c4.png",
"https://user-images.githubusercontent.com/15225835/229733180-e01260c1-4772-45dd-848c-32ceb7696db1.png"
] | [] |
|
tailwindlabs/tailwindcss | 10,962 | tailwindlabs__tailwindcss-10962 | [
"10960"
] | 467a39e0d59a9fdc42deef3a9c7d51c44ace90e8 | diff --git a/jest/global-setup.js b/jest/global-setup.js
--- a/jest/global-setup.js
+++ b/jest/global-setup.js
@@ -1,6 +1,9 @@
let { execSync } = require('child_process')
+let state = { ran: false }
module.exports = function () {
+ if (state.ran) return
execSync('npm run build:rust', { stdio: 'ignore' })
execSync('npm run generate:plugin-list', { stdio: 'ignore' })
+ state.ran = true
}
diff --git a/src/lib/expandApplyAtRules.js b/src/lib/expandApplyAtRules.js
--- a/src/lib/expandApplyAtRules.js
+++ b/src/lib/expandApplyAtRules.js
@@ -4,7 +4,7 @@ import parser from 'postcss-selector-parser'
import { resolveMatches } from './generateRules'
import escapeClassName from '../util/escapeClassName'
import { applyImportantSelector } from '../util/applyImportantSelector'
-import { collectPseudoElements, sortSelector } from '../util/formatVariantSelector.js'
+import { movePseudos } from '../util/pseudoElements'
/** @typedef {Map<string, [any, import('postcss').Rule[]]>} ApplyCache */
@@ -566,13 +566,7 @@ function processApply(root, context, localCache) {
// Move pseudo elements to the end of the selector (if necessary)
let selector = parser().astSync(rule.selector)
- selector.each((sel) => {
- let [pseudoElements] = collectPseudoElements(sel)
- if (pseudoElements.length > 0) {
- sel.nodes.push(...pseudoElements.sort(sortSelector))
- }
- })
-
+ selector.each((sel) => movePseudos(sel))
rule.selector = selector.toString()
})
}
diff --git a/src/util/applyImportantSelector.js b/src/util/applyImportantSelector.js
--- a/src/util/applyImportantSelector.js
+++ b/src/util/applyImportantSelector.js
@@ -1,5 +1,5 @@
import parser from 'postcss-selector-parser'
-import { collectPseudoElements, sortSelector } from './formatVariantSelector.js'
+import { movePseudos } from './pseudoElements'
export function applyImportantSelector(selector, important) {
let sel = parser().astSync(selector)
@@ -20,10 +20,7 @@ export function applyImportantSelector(selector, important) {
]
}
- let [pseudoElements] = collectPseudoElements(sel)
- if (pseudoElements.length > 0) {
- sel.nodes.push(...pseudoElements.sort(sortSelector))
- }
+ movePseudos(sel)
})
return `${important} ${sel.toString()}`
diff --git a/src/util/formatVariantSelector.js b/src/util/formatVariantSelector.js
--- a/src/util/formatVariantSelector.js
+++ b/src/util/formatVariantSelector.js
@@ -2,6 +2,7 @@ import selectorParser from 'postcss-selector-parser'
import unescape from 'postcss-selector-parser/dist/util/unesc'
import escapeClassName from '../util/escapeClassName'
import prefixSelector from '../util/prefixSelector'
+import { movePseudos } from './pseudoElements'
/** @typedef {import('postcss-selector-parser').Root} Root */
/** @typedef {import('postcss-selector-parser').Selector} Selector */
@@ -245,12 +246,7 @@ export function finalizeSelector(current, formats, { context, candidate, base })
})
// Move pseudo elements to the end of the selector (if necessary)
- selector.each((sel) => {
- let [pseudoElements] = collectPseudoElements(sel)
- if (pseudoElements.length > 0) {
- sel.nodes.push(...pseudoElements.sort(sortSelector))
- }
- })
+ selector.each((sel) => movePseudos(sel))
return selector.toString()
}
@@ -318,124 +314,3 @@ export function handleMergePseudo(selector, format) {
return [selector, format]
}
-
-// Note: As a rule, double colons (::) should be used instead of a single colon
-// (:). This distinguishes pseudo-classes from pseudo-elements. However, since
-// this distinction was not present in older versions of the W3C spec, most
-// browsers support both syntaxes for the original pseudo-elements.
-let pseudoElementsBC = [':before', ':after', ':first-line', ':first-letter']
-
-// These pseudo-elements _can_ be combined with other pseudo selectors AND the order does matter.
-let pseudoElementExceptions = [
- '::file-selector-button',
-
- // Webkit scroll bar pseudo elements can be combined with user-action pseudo classes
- '::-webkit-scrollbar',
- '::-webkit-scrollbar-button',
- '::-webkit-scrollbar-thumb',
- '::-webkit-scrollbar-track',
- '::-webkit-scrollbar-track-piece',
- '::-webkit-scrollbar-corner',
- '::-webkit-resizer',
-
- // Old-style Angular Shadow DOM piercing pseudo element
- '::ng-deep',
-]
-
-/**
- * This will make sure to move pseudo's to the correct spot (the end for
- * pseudo elements) because otherwise the selector will never work
- * anyway.
- *
- * E.g.:
- * - `before:hover:text-center` would result in `.before\:hover\:text-center:hover::before`
- * - `hover:before:text-center` would result in `.hover\:before\:text-center:hover::before`
- *
- * `::before:hover` doesn't work, which means that we can make it work for you by flipping the order.
- *
- * @param {Selector} selector
- * @param {boolean} force
- **/
-export function collectPseudoElements(selector, force = false) {
- /** @type {Node[]} */
- let nodes = []
- let seenPseudoElement = null
-
- for (let node of [...selector.nodes]) {
- if (isPseudoElement(node, force)) {
- nodes.push(node)
- selector.removeChild(node)
- seenPseudoElement = node.value
- } else if (seenPseudoElement !== null) {
- if (pseudoElementExceptions.includes(seenPseudoElement) && isPseudoClass(node, force)) {
- nodes.push(node)
- selector.removeChild(node)
- } else {
- seenPseudoElement = null
- }
- }
-
- if (node?.nodes) {
- let hasPseudoElementRestrictions =
- node.type === 'pseudo' && (node.value === ':is' || node.value === ':has')
-
- let [collected, seenPseudoElementInSelector] = collectPseudoElements(
- node,
- force || hasPseudoElementRestrictions
- )
-
- if (seenPseudoElementInSelector) {
- seenPseudoElement = seenPseudoElementInSelector
- }
-
- nodes.push(...collected)
- }
- }
-
- return [nodes, seenPseudoElement]
-}
-
-// This will make sure to move pseudo's to the correct spot (the end for
-// pseudo elements) because otherwise the selector will never work
-// anyway.
-//
-// E.g.:
-// - `before:hover:text-center` would result in `.before\:hover\:text-center:hover::before`
-// - `hover:before:text-center` would result in `.hover\:before\:text-center:hover::before`
-//
-// `::before:hover` doesn't work, which means that we can make it work
-// for you by flipping the order.
-export function sortSelector(a, z) {
- // Both nodes are non-pseudo's so we can safely ignore them and keep
- // them in the same order.
- if (a.type !== 'pseudo' && z.type !== 'pseudo') {
- return 0
- }
-
- // If one of them is a combinator, we need to keep it in the same order
- // because that means it will start a new "section" in the selector.
- if ((a.type === 'combinator') ^ (z.type === 'combinator')) {
- return 0
- }
-
- // One of the items is a pseudo and the other one isn't. Let's move
- // the pseudo to the right.
- if ((a.type === 'pseudo') ^ (z.type === 'pseudo')) {
- return (a.type === 'pseudo') - (z.type === 'pseudo')
- }
-
- // Both are pseudo's, move the pseudo elements (except for
- // ::file-selector-button) to the right.
- return isPseudoElement(a) - isPseudoElement(z)
-}
-
-function isPseudoElement(node, force = false) {
- if (node.type !== 'pseudo') return false
- if (pseudoElementExceptions.includes(node.value) && !force) return false
-
- return node.value.startsWith('::') || pseudoElementsBC.includes(node.value)
-}
-
-function isPseudoClass(node, force) {
- return node.type === 'pseudo' && !isPseudoElement(node, force)
-}
diff --git a/src/util/pseudoElements.js b/src/util/pseudoElements.js
new file mode 100644
--- /dev/null
+++ b/src/util/pseudoElements.js
@@ -0,0 +1,170 @@
+/** @typedef {import('postcss-selector-parser').Root} Root */
+/** @typedef {import('postcss-selector-parser').Selector} Selector */
+/** @typedef {import('postcss-selector-parser').Pseudo} Pseudo */
+/** @typedef {import('postcss-selector-parser').Node} Node */
+
+// There are some pseudo-elements that may or may not be:
+
+// **Actionable**
+// Zero or more user-action pseudo-classes may be attached to the pseudo-element itself
+// structural-pseudo-classes are NOT allowed but we don't make
+// The spec is not clear on whether this is allowed or not — but in practice it is.
+
+// **Terminal**
+// It MUST be placed at the end of a selector
+//
+// This is the required in the spec. However, some pseudo elements are not "terminal" because
+// they represent a "boundary piercing" that is compiled out by a build step.
+
+// **Jumpable**
+// Any terminal element may "jump" over combinators when moving to the end of the selector
+//
+// This is a backwards-compat quirk of :before and :after variants.
+
+/** @typedef {'terminal' | 'actionable' | 'jumpable'} PseudoProperty */
+
+/** @type {Record<string, PseudoProperty[]>} */
+let elementProperties = {
+ '::after': ['terminal', 'jumpable'],
+ '::backdrop': ['terminal'],
+ '::before': ['terminal', 'jumpable'],
+ '::cue': ['terminal'],
+ '::cue-region': ['terminal'],
+ '::first-letter': ['terminal', 'jumpable'],
+ '::first-line': ['terminal', 'jumpable'],
+ '::grammar-error': ['terminal'],
+ '::marker': ['terminal'],
+ '::part': ['terminal', 'actionable'],
+ '::placeholder': ['terminal'],
+ '::selection': ['terminal'],
+ '::slotted': ['terminal'],
+ '::spelling-error': ['terminal'],
+ '::target-text': ['terminal'],
+
+ // other
+ '::file-selector-button': ['terminal', 'actionable'],
+ '::-webkit-progress-bar': ['terminal', 'actionable'],
+
+ // Webkit scroll bar pseudo elements can be combined with user-action pseudo classes
+ '::-webkit-scrollbar': ['terminal', 'actionable'],
+ '::-webkit-scrollbar-button': ['terminal', 'actionable'],
+ '::-webkit-scrollbar-thumb': ['terminal', 'actionable'],
+ '::-webkit-scrollbar-track': ['terminal', 'actionable'],
+ '::-webkit-scrollbar-track-piece': ['terminal', 'actionable'],
+ '::-webkit-scrollbar-corner': ['terminal', 'actionable'],
+ '::-webkit-resizer': ['terminal', 'actionable'],
+
+ // Note: As a rule, double colons (::) should be used instead of a single colon
+ // (:). This distinguishes pseudo-classes from pseudo-elements. However, since
+ // this distinction was not present in older versions of the W3C spec, most
+ // browsers support both syntaxes for the original pseudo-elements.
+ ':after': ['terminal', 'jumpable'],
+ ':before': ['terminal', 'jumpable'],
+ ':first-letter': ['terminal', 'jumpable'],
+ ':first-line': ['terminal', 'jumpable'],
+
+ // The default value is used when the pseudo-element is not recognized
+ // Because it's not recognized, we don't know if it's terminal or not
+ // So we assume it can't be moved AND can have user-action pseudo classes attached to it
+ __default__: ['actionable'],
+}
+
+/**
+ * @param {Selector} sel
+ * @returns {Selector}
+ */
+export function movePseudos(sel) {
+ let [pseudos] = movablePseudos(sel)
+
+ // Remove all pseudo elements from their respective selectors
+ pseudos.forEach(([sel, pseudo]) => sel.removeChild(pseudo))
+
+ // Re-add them to the end of the selector in the correct order.
+ // This moves terminal pseudo elements to the end of the
+ // selector otherwise the selector will not be valid.
+ //
+ // Examples:
+ // - `before:hover:text-center` would result in `.before\:hover\:text-center:hover::before`
+ // - `hover:before:text-center` would result in `.hover\:before\:text-center:hover::before`
+ //
+ // The selector `::before:hover` does not work but we
+ // can make it work for you by flipping the order.
+ sel.nodes.push(...pseudos.map(([, pseudo]) => pseudo))
+
+ return sel
+}
+
+/** @typedef {[sel: Selector, pseudo: Pseudo, attachedTo: Pseudo | null]} MovablePseudo */
+/** @typedef {[pseudos: MovablePseudo[], lastSeenElement: Pseudo | null]} MovablePseudosResult */
+
+/**
+ * @param {Selector} sel
+ * @returns {MovablePseudosResult}
+ */
+function movablePseudos(sel) {
+ /** @type {MovablePseudo[]} */
+ let buffer = []
+
+ /** @type {Pseudo | null} */
+ let lastSeenElement = null
+
+ for (let node of sel.nodes) {
+ if (node.type === 'combinator') {
+ buffer = buffer.filter(([, node]) => propertiesForPseudo(node).includes('jumpable'))
+ lastSeenElement = null
+ } else if (node.type === 'pseudo') {
+ if (isMovablePseudoElement(node)) {
+ lastSeenElement = node
+ buffer.push([sel, node, null])
+ } else if (lastSeenElement && isAttachablePseudoClass(node, lastSeenElement)) {
+ buffer.push([sel, node, lastSeenElement])
+ } else {
+ lastSeenElement = null
+ }
+
+ for (let sub of node.nodes ?? []) {
+ let [movable, lastSeenElementInSub] = movablePseudos(sub)
+ lastSeenElement = lastSeenElementInSub || lastSeenElement
+ buffer.push(...movable)
+ }
+ }
+ }
+
+ return [buffer, lastSeenElement]
+}
+
+/**
+ * @param {Node} node
+ * @returns {boolean}
+ */
+function isPseudoElement(node) {
+ return node.value.startsWith('::') || elementProperties[node.value] !== undefined
+}
+
+/**
+ * @param {Node} node
+ * @returns {boolean}
+ */
+function isMovablePseudoElement(node) {
+ return isPseudoElement(node) && propertiesForPseudo(node).includes('terminal')
+}
+
+/**
+ * @param {Node} node
+ * @param {Pseudo} pseudo
+ * @returns {boolean}
+ */
+function isAttachablePseudoClass(node, pseudo) {
+ if (node.type !== 'pseudo') return false
+ if (isPseudoElement(node)) return false
+
+ return propertiesForPseudo(pseudo).includes('actionable')
+}
+
+/**
+ * @param {Pseudo} pseudo
+ * @returns {PseudoProperty[]}
+ */
+function propertiesForPseudo(pseudo) {
+ return elementProperties[pseudo.value] ?? elementProperties.__default__
+}
| diff --git a/tests/apply.test.js b/tests/apply.test.js
--- a/tests/apply.test.js
+++ b/tests/apply.test.js
@@ -2428,7 +2428,7 @@ crosscheck(({ stable, oxide }) => {
})
})
- stable.test('::ng-deep pseudo element is left alone', () => {
+ stable.test('::ng-deep, ::deep, ::v-deep pseudo elements are left alone', () => {
let config = {
darkMode: 'class',
content: [
@@ -2442,6 +2442,12 @@ crosscheck(({ stable, oxide }) => {
::ng-deep .foo .bar {
@apply font-bold;
}
+ ::v-deep .foo .bar {
+ @apply font-bold;
+ }
+ ::deep .foo .bar {
+ @apply font-bold;
+ }
`
return run(input, config).then((result) => {
@@ -2449,12 +2455,19 @@ crosscheck(({ stable, oxide }) => {
::ng-deep .foo .bar {
font-weight: 700;
}
+ ::v-deep .foo .bar {
+ font-weight: 700;
+ }
+ ::deep .foo .bar {
+ font-weight: 700;
+ }
`)
})
})
// 1. `::ng-deep` is deprecated
- // 2. It uses invalid selector syntax that Lightning CSS does not support
+ // 2. `::deep` and `::v-deep` are non-standard
+ // 3. They all use invalid selector syntax that Lightning CSS does not support
// It may be enough for Oxide to not support it at all
- oxide.test.todo('::ng-deep pseudo element is left alone')
+ oxide.test.todo('::ng-deep, ::deep, ::v-deep pseudo elements are left alone')
})
diff --git a/tests/format-variant-selector.test.js b/tests/format-variant-selector.test.js
--- a/tests/format-variant-selector.test.js
+++ b/tests/format-variant-selector.test.js
@@ -350,6 +350,8 @@ crosscheck(() => {
${':where(&::file-selector-button) :is(h1, h2, h3, h4)'} | ${':where(&::file-selector-button) :is(h1, h2, h3, h4)'}
${'#app :is(.dark &::before)'} | ${'#app :is(.dark &)::before'}
${'#app :is(:is(.dark &)::before)'} | ${'#app :is(:is(.dark &))::before'}
+ ${'#app :is(.foo::file-selector-button)'} | ${'#app :is(.foo)::file-selector-button'}
+ ${'#app :is(.foo::-webkit-progress-bar)'} | ${'#app :is(.foo)::-webkit-progress-bar'}
`('should translate "$before" into "$after"', ({ before, after }) => {
let result = finalizeSelector('.a', [{ format: before, isArbitraryVariant: false }], {
candidate: 'a',
diff --git a/tests/parallel-variants.test.js b/tests/parallel-variants.test.js
--- a/tests/parallel-variants.test.js
+++ b/tests/parallel-variants.test.js
@@ -28,7 +28,7 @@ crosscheck(() => {
.test\:font-medium ::test {
font-weight: 500;
}
- .hover\:test\:font-black :hover::test {
+ .hover\:test\:font-black ::test:hover {
font-weight: 900;
}
.test\:font-bold::test {
@@ -37,7 +37,7 @@ crosscheck(() => {
.test\:font-medium::test {
font-weight: 500;
}
- .hover\:test\:font-black:hover::test {
+ .hover\:test\:font-black::test:hover {
font-weight: 900;
}
`)
@@ -77,10 +77,10 @@ crosscheck(() => {
.test\:font-medium::test {
font-weight: 500;
}
- .hover\:test\:font-black :hover::test {
+ .hover\:test\:font-black ::test:hover {
font-weight: 900;
}
- .hover\:test\:font-black:hover::test {
+ .hover\:test\:font-black::test:hover {
font-weight: 900;
}
`)
@@ -126,10 +126,10 @@ crosscheck(() => {
.test\:font-medium::test {
font-weight: 500;
}
- .hover\:test\:font-black :hover::test {
+ .hover\:test\:font-black ::test:hover {
font-weight: 900;
}
- .hover\:test\:font-black:hover::test {
+ .hover\:test\:font-black::test:hover {
font-weight: 900;
}
`)
| 3.3.1 not handling pseudo-elements properly
**What version of Tailwind CSS are you using?**
`3.3.1`
**What build tool (or framework if it abstracts the build tool) are you using?**
tailwindcss
**What version of Node.js are you using?**
`v18.14.0`
#### Steps to Repro
```bash
mkdir repro
cd repro
yarn global add [email protected]
tailwindcss init
```
Create `repro.css` with the following content:
```css
@config 'tailwind.config.js';
::deep [a] {
@apply bg-white;
}
```
Run `tailwindcss --input .\repro.css --output .\result.css`
```css
/* result.css / Incorrect */
[a]::deep {
--tw-bg-opacity: 1;
background-color: rgb(255 255 255 / var(--tw-bg-opacity))
}
```
You can fix this by downgrading tailwindcss to 3.3.0:
```bash
yarn global add [email protected]
tailwindcss --input .\repro.css --output .\result.css
````
And the CORRECT output
```css
/* Correct! */
::deep [a] {
--tw-bg-opacity: 1;
background-color: rgb(255 255 255 / var(--tw-bg-opacity))
}
```
| 2023-04-06T12:31:05 | 3.3 | [
"tests/format-variant-selector.test.js"
] | [
"tests/parallel-variants.test.js",
"tests/apply.test.js"
] | TypeScript | [] | [] |
|
tailwindlabs/tailwindcss | 11,111 | tailwindlabs__tailwindcss-11111 | [
"11107"
] | 18677447063f7d08252a3f34c34d7deb0bcd6995 | diff --git a/src/util/pseudoElements.js b/src/util/pseudoElements.js
--- a/src/util/pseudoElements.js
+++ b/src/util/pseudoElements.js
@@ -26,17 +26,17 @@
/** @type {Record<string, PseudoProperty[]>} */
let elementProperties = {
'::after': ['terminal', 'jumpable'],
- '::backdrop': ['terminal'],
+ '::backdrop': ['terminal', 'jumpable'],
'::before': ['terminal', 'jumpable'],
'::cue': ['terminal'],
'::cue-region': ['terminal'],
'::first-letter': ['terminal', 'jumpable'],
'::first-line': ['terminal', 'jumpable'],
'::grammar-error': ['terminal'],
- '::marker': ['terminal'],
+ '::marker': ['terminal', 'jumpable'],
'::part': ['terminal', 'actionable'],
- '::placeholder': ['terminal'],
- '::selection': ['terminal'],
+ '::placeholder': ['terminal', 'jumpable'],
+ '::selection': ['terminal', 'jumpable'],
'::slotted': ['terminal'],
'::spelling-error': ['terminal'],
'::target-text': ['terminal'],
| diff --git a/tests/format-variant-selector.test.js b/tests/format-variant-selector.test.js
--- a/tests/format-variant-selector.test.js
+++ b/tests/format-variant-selector.test.js
@@ -352,6 +352,10 @@ crosscheck(() => {
${'#app :is(:is(.dark &)::before)'} | ${'#app :is(:is(.dark &))::before'}
${'#app :is(.foo::file-selector-button)'} | ${'#app :is(.foo)::file-selector-button'}
${'#app :is(.foo::-webkit-progress-bar)'} | ${'#app :is(.foo)::-webkit-progress-bar'}
+ ${'.parent::marker li'} | ${'.parent li::marker'}
+ ${'.parent::selection li'} | ${'.parent li::selection'}
+ ${'.parent::placeholder input'} | ${'.parent input::placeholder'}
+ ${'.parent::backdrop dialog'} | ${'.parent dialog::backdrop'}
`('should translate "$before" into "$after"', ({ before, after }) => {
let result = finalizeSelector('.a', [{ format: before, isArbitraryVariant: false }], {
candidate: 'a',
| Unexpected behavior of pseudo-elements such as marker
**What version of Tailwind CSS are you using?**
For example: v3.3.2
**What build tool (or framework if it abstracts the build tool) are you using?**
vite 4.3.3, vue 3.2.47, postcss 8.4.23
**What version of Node.js are you using?**
v20.0.0
**What browser are you using?**
Chrome
**What operating system are you using?**
macOS
**Reproduction URL**
As v3.3.2 is currently unavailable in Tailwind Play, this is the Insiders version.
[https://play.tailwindcss.com/3SbW3c1AhG](https://play.tailwindcss.com/3SbW3c1AhG)
<img width="50" src="https://user-images.githubusercontent.com/36815907/234824052-0245f5dc-5045-4a89-b241-a21edf9218e5.png">
In v3.3.1, it works as expected.
[https://play.tailwindcss.com/1nJxOORQv7](https://play.tailwindcss.com/1nJxOORQv7)
<img width="50" src="https://user-images.githubusercontent.com/36815907/234824207-9d53f56f-edb1-4898-816d-162b81f4fc1a.png">
**Describe your issue**
In v3.3.2, some behaviors of pseudo-elements are not as expected, for example, the marker color cannot be changed correctly, while this works correctly in v3.3.1.
```html
<div class="[&>li]:marker:text-red-500">
<li>A</li>
<li>B</li>
<li>C</li>
</div>
<div class="prose prose-li:marker:text-purple-500">
<li>D</li>
<li>E</li>
<li>F</li>
</div>
```
| Hey, thank you for this bug report! 🙏
If you swap the variants around then it behaves as expected: https://play.tailwindcss.com/u1trU3XrlL however I do think we regressed on this.
---
@thecrypticace Pinging you because I know you worked on this recently to fix other bugs. We can also pair on this later today to see what's going on if you want. | 2023-04-27T13:34:47 | 3.3 | [] | [
"tests/format-variant-selector.test.js"
] | TypeScript | [
"https://user-images.githubusercontent.com/36815907/234824052-0245f5dc-5045-4a89-b241-a21edf9218e5.png",
"https://user-images.githubusercontent.com/36815907/234824207-9d53f56f-edb1-4898-816d-162b81f4fc1a.png"
] | [
"https://play.tailwindcss.com/3SbW3c1AhG](https://play.tailwindcss.com/3SbW3c1AhG",
"https://play.tailwindcss.com/1nJxOORQv7](https://play.tailwindcss.com/1nJxOORQv7"
] |
tailwindlabs/tailwindcss | 11,157 | tailwindlabs__tailwindcss-11157 | [
"11027"
] | cdca9cbcfe331b54ca4df80bc720f8cd78e303a0 | diff --git a/src/lib/evaluateTailwindFunctions.js b/src/lib/evaluateTailwindFunctions.js
--- a/src/lib/evaluateTailwindFunctions.js
+++ b/src/lib/evaluateTailwindFunctions.js
@@ -1,7 +1,7 @@
import dlv from 'dlv'
import didYouMean from 'didyoumean'
import transformThemeValue from '../util/transformThemeValue'
-import parseValue from 'postcss-value-parser'
+import parseValue from '../value-parser/index'
import { normalizeScreens } from '../util/normalizeScreens'
import buildMediaQuery from '../util/buildMediaQuery'
import { toPath } from '../util/toPath'
@@ -146,6 +146,9 @@ function resolveVNode(node, vNode, functions) {
}
function resolveFunctions(node, input, functions) {
+ let hasAnyFn = Object.keys(functions).some((fn) => input.includes(`${fn}(`))
+ if (!hasAnyFn) return input
+
return parseValue(input)
.walk((vNode) => {
resolveVNode(node, vNode, functions)
diff --git a/src/util/dataTypes.js b/src/util/dataTypes.js
--- a/src/util/dataTypes.js
+++ b/src/util/dataTypes.js
@@ -49,10 +49,22 @@ export function normalize(value, isRoot = true) {
value = value.trim()
}
- // Add spaces around operators inside math functions like calc() that do not follow an operator
- // or '('.
- value = value.replace(/(calc|min|max|clamp)\(.+\)/g, (match) => {
+ value = normalizeMathOperatorSpacing(value)
+
+ return value
+}
+
+/**
+ * Add spaces around operators inside math functions
+ * like calc() that do not follow an operator or '('.
+ *
+ * @param {string} value
+ * @returns {string}
+ */
+function normalizeMathOperatorSpacing(value) {
+ return value.replace(/(calc|min|max|clamp)\(.+\)/g, (match) => {
let vars = []
+
return match
.replace(/var\((--.+?)[,)]/g, (match, g1) => {
vars.push(g1)
@@ -61,8 +73,6 @@ export function normalize(value, isRoot = true) {
.replace(/(-?\d*\.?\d(?!\b-\d.+[,)](?![^+\-/*])\D)(?:%|[a-z]+)?|\))([+\-/*])/g, '$1 $2 ')
.replace(placeholderRe, () => vars.shift())
})
-
- return value
}
export function url(value) {
diff --git a/src/value-parser/index.d.ts b/src/value-parser/index.d.ts
new file mode 100644
--- /dev/null
+++ b/src/value-parser/index.d.ts
@@ -0,0 +1,177 @@
+declare namespace postcssValueParser {
+ interface BaseNode {
+ /**
+ * The offset, inclusive, inside the CSS value at which the node starts.
+ */
+ sourceIndex: number
+
+ /**
+ * The offset, exclusive, inside the CSS value at which the node ends.
+ */
+ sourceEndIndex: number
+
+ /**
+ * The node's characteristic value
+ */
+ value: string
+ }
+
+ interface ClosableNode {
+ /**
+ * Whether the parsed CSS value ended before the node was properly closed
+ */
+ unclosed?: true
+ }
+
+ interface AdjacentAwareNode {
+ /**
+ * The token at the start of the node
+ */
+ before: string
+
+ /**
+ * The token at the end of the node
+ */
+ after: string
+ }
+
+ interface CommentNode extends BaseNode, ClosableNode {
+ type: 'comment'
+ }
+
+ interface DivNode extends BaseNode, AdjacentAwareNode {
+ type: 'div'
+ }
+
+ interface FunctionNode extends BaseNode, ClosableNode, AdjacentAwareNode {
+ type: 'function'
+
+ /**
+ * Nodes inside the function
+ */
+ nodes: Node[]
+ }
+
+ interface SpaceNode extends BaseNode {
+ type: 'space'
+ }
+
+ interface StringNode extends BaseNode, ClosableNode {
+ type: 'string'
+
+ /**
+ * The quote type delimiting the string
+ */
+ quote: '"' | "'"
+ }
+
+ interface UnicodeRangeNode extends BaseNode {
+ type: 'unicode-range'
+ }
+
+ interface WordNode extends BaseNode {
+ type: 'word'
+ }
+
+ /**
+ * Any node parsed from a CSS value
+ */
+ type Node =
+ | CommentNode
+ | DivNode
+ | FunctionNode
+ | SpaceNode
+ | StringNode
+ | UnicodeRangeNode
+ | WordNode
+
+ interface CustomStringifierCallback {
+ /**
+ * @param node The node to stringify
+ * @returns The serialized CSS representation of the node
+ */
+ (nodes: Node): string | undefined
+ }
+
+ interface WalkCallback {
+ /**
+ * @param node The currently visited node
+ * @param index The index of the node in the series of parsed nodes
+ * @param nodes The series of parsed nodes
+ * @returns Returning `false` will prevent traversal of descendant nodes (only applies if `bubble` was set to `true` in the `walk()` call)
+ */
+ (node: Node, index: number, nodes: Node[]): void | boolean
+ }
+
+ /**
+ * A CSS dimension, decomposed into its numeric and unit parts
+ */
+ interface Dimension {
+ number: string
+ unit: string
+ }
+
+ /**
+ * A wrapper around a parsed CSS value that allows for inspecting and walking nodes
+ */
+ interface ParsedValue {
+ /**
+ * The series of parsed nodes
+ */
+ nodes: Node[]
+
+ /**
+ * Walk all parsed nodes, applying a callback
+ *
+ * @param callback A visitor callback that will be executed for each node
+ * @param bubble When set to `true`, walking will be done inside-out instead of outside-in
+ */
+ walk(callback: WalkCallback, bubble?: boolean): this
+ }
+
+ interface ValueParser {
+ /**
+ * Decompose a CSS dimension into its numeric and unit part
+ *
+ * @param value The dimension to decompose
+ * @returns An object representing `number` and `unit` part of the dimension or `false` if the decomposing fails
+ */
+ unit(value: string): Dimension | false
+
+ /**
+ * Serialize a series of nodes into a CSS value
+ *
+ * @param nodes The nodes to stringify
+ * @param custom A custom stringifier callback
+ * @returns The generated CSS value
+ */
+ stringify(nodes: Node | Node[], custom?: CustomStringifierCallback): string
+
+ /**
+ * Walk a series of nodes, applying a callback
+ *
+ * @param nodes The nodes to walk
+ * @param callback A visitor callback that will be executed for each node
+ * @param bubble When set to `true`, walking will be done inside-out instead of outside-in
+ */
+ walk(nodes: Node[], callback: WalkCallback, bubble?: boolean): void
+
+ /**
+ * Parse a CSS value into a series of nodes to operate on
+ *
+ * @param value The value to parse
+ */
+ new (value: string): ParsedValue
+
+ /**
+ * Parse a CSS value into a series of nodes to operate on
+ *
+ * @param value The value to parse
+ */
+ (value: string): ParsedValue
+ }
+}
+
+declare const postcssValueParser: postcssValueParser.ValueParser
+
+export = postcssValueParser
diff --git a/src/value-parser/index.js b/src/value-parser/index.js
new file mode 100644
--- /dev/null
+++ b/src/value-parser/index.js
@@ -0,0 +1,28 @@
+var parse = require('./parse')
+var walk = require('./walk')
+var stringify = require('./stringify')
+
+function ValueParser(value) {
+ if (this instanceof ValueParser) {
+ this.nodes = parse(value)
+ return this
+ }
+ return new ValueParser(value)
+}
+
+ValueParser.prototype.toString = function () {
+ return Array.isArray(this.nodes) ? stringify(this.nodes) : ''
+}
+
+ValueParser.prototype.walk = function (cb, bubble) {
+ walk(this.nodes, cb, bubble)
+ return this
+}
+
+ValueParser.unit = require('./unit')
+
+ValueParser.walk = walk
+
+ValueParser.stringify = stringify
+
+module.exports = ValueParser
diff --git a/src/value-parser/parse.js b/src/value-parser/parse.js
new file mode 100644
--- /dev/null
+++ b/src/value-parser/parse.js
@@ -0,0 +1,303 @@
+var openParentheses = '('.charCodeAt(0)
+var closeParentheses = ')'.charCodeAt(0)
+var singleQuote = "'".charCodeAt(0)
+var doubleQuote = '"'.charCodeAt(0)
+var backslash = '\\'.charCodeAt(0)
+var slash = '/'.charCodeAt(0)
+var comma = ','.charCodeAt(0)
+var colon = ':'.charCodeAt(0)
+var star = '*'.charCodeAt(0)
+var uLower = 'u'.charCodeAt(0)
+var uUpper = 'U'.charCodeAt(0)
+var plus = '+'.charCodeAt(0)
+var isUnicodeRange = /^[a-f0-9?-]+$/i
+
+module.exports = function (input) {
+ var tokens = []
+ var value = input
+
+ var next, quote, prev, token, escape, escapePos, whitespacePos, parenthesesOpenPos
+ var pos = 0
+ var code = value.charCodeAt(pos)
+ var max = value.length
+ var stack = [{ nodes: tokens }]
+ var balanced = 0
+ var parent
+
+ var name = ''
+ var before = ''
+ var after = ''
+
+ while (pos < max) {
+ // Whitespaces
+ if (code <= 32) {
+ next = pos
+ do {
+ next += 1
+ code = value.charCodeAt(next)
+ } while (code <= 32)
+ token = value.slice(pos, next)
+
+ prev = tokens[tokens.length - 1]
+ if (code === closeParentheses && balanced) {
+ after = token
+ } else if (prev && prev.type === 'div') {
+ prev.after = token
+ prev.sourceEndIndex += token.length
+ } else if (
+ code === comma ||
+ code === colon ||
+ (code === slash &&
+ value.charCodeAt(next + 1) !== star &&
+ (!parent || (parent && parent.type === 'function' && false)))
+ ) {
+ before = token
+ } else {
+ tokens.push({
+ type: 'space',
+ sourceIndex: pos,
+ sourceEndIndex: next,
+ value: token,
+ })
+ }
+
+ pos = next
+
+ // Quotes
+ } else if (code === singleQuote || code === doubleQuote) {
+ next = pos
+ quote = code === singleQuote ? "'" : '"'
+ token = {
+ type: 'string',
+ sourceIndex: pos,
+ quote: quote,
+ }
+ do {
+ escape = false
+ next = value.indexOf(quote, next + 1)
+ if (~next) {
+ escapePos = next
+ while (value.charCodeAt(escapePos - 1) === backslash) {
+ escapePos -= 1
+ escape = !escape
+ }
+ } else {
+ value += quote
+ next = value.length - 1
+ token.unclosed = true
+ }
+ } while (escape)
+ token.value = value.slice(pos + 1, next)
+ token.sourceEndIndex = token.unclosed ? next : next + 1
+ tokens.push(token)
+ pos = next + 1
+ code = value.charCodeAt(pos)
+
+ // Comments
+ } else if (code === slash && value.charCodeAt(pos + 1) === star) {
+ next = value.indexOf('*/', pos)
+
+ token = {
+ type: 'comment',
+ sourceIndex: pos,
+ sourceEndIndex: next + 2,
+ }
+
+ if (next === -1) {
+ token.unclosed = true
+ next = value.length
+ token.sourceEndIndex = next
+ }
+
+ token.value = value.slice(pos + 2, next)
+ tokens.push(token)
+
+ pos = next + 2
+ code = value.charCodeAt(pos)
+
+ // Operation within calc
+ } else if ((code === slash || code === star) && parent && parent.type === 'function' && true) {
+ token = value[pos]
+ tokens.push({
+ type: 'word',
+ sourceIndex: pos - before.length,
+ sourceEndIndex: pos + token.length,
+ value: token,
+ })
+ pos += 1
+ code = value.charCodeAt(pos)
+
+ // Dividers
+ } else if (code === slash || code === comma || code === colon) {
+ token = value[pos]
+
+ tokens.push({
+ type: 'div',
+ sourceIndex: pos - before.length,
+ sourceEndIndex: pos + token.length,
+ value: token,
+ before: before,
+ after: '',
+ })
+ before = ''
+
+ pos += 1
+ code = value.charCodeAt(pos)
+
+ // Open parentheses
+ } else if (openParentheses === code) {
+ // Whitespaces after open parentheses
+ next = pos
+ do {
+ next += 1
+ code = value.charCodeAt(next)
+ } while (code <= 32)
+ parenthesesOpenPos = pos
+ token = {
+ type: 'function',
+ sourceIndex: pos - name.length,
+ value: name,
+ before: value.slice(parenthesesOpenPos + 1, next),
+ }
+ pos = next
+
+ if (name === 'url' && code !== singleQuote && code !== doubleQuote) {
+ next -= 1
+ do {
+ escape = false
+ next = value.indexOf(')', next + 1)
+ if (~next) {
+ escapePos = next
+ while (value.charCodeAt(escapePos - 1) === backslash) {
+ escapePos -= 1
+ escape = !escape
+ }
+ } else {
+ value += ')'
+ next = value.length - 1
+ token.unclosed = true
+ }
+ } while (escape)
+ // Whitespaces before closed
+ whitespacePos = next
+ do {
+ whitespacePos -= 1
+ code = value.charCodeAt(whitespacePos)
+ } while (code <= 32)
+ if (parenthesesOpenPos < whitespacePos) {
+ if (pos !== whitespacePos + 1) {
+ token.nodes = [
+ {
+ type: 'word',
+ sourceIndex: pos,
+ sourceEndIndex: whitespacePos + 1,
+ value: value.slice(pos, whitespacePos + 1),
+ },
+ ]
+ } else {
+ token.nodes = []
+ }
+ if (token.unclosed && whitespacePos + 1 !== next) {
+ token.after = ''
+ token.nodes.push({
+ type: 'space',
+ sourceIndex: whitespacePos + 1,
+ sourceEndIndex: next,
+ value: value.slice(whitespacePos + 1, next),
+ })
+ } else {
+ token.after = value.slice(whitespacePos + 1, next)
+ token.sourceEndIndex = next
+ }
+ } else {
+ token.after = ''
+ token.nodes = []
+ }
+ pos = next + 1
+ token.sourceEndIndex = token.unclosed ? next : pos
+ code = value.charCodeAt(pos)
+ tokens.push(token)
+ } else {
+ balanced += 1
+ token.after = ''
+ token.sourceEndIndex = pos + 1
+ tokens.push(token)
+ stack.push(token)
+ tokens = token.nodes = []
+ parent = token
+ }
+ name = ''
+
+ // Close parentheses
+ } else if (closeParentheses === code && balanced) {
+ pos += 1
+ code = value.charCodeAt(pos)
+
+ parent.after = after
+ parent.sourceEndIndex += after.length
+ after = ''
+ balanced -= 1
+ stack[stack.length - 1].sourceEndIndex = pos
+ stack.pop()
+ parent = stack[balanced]
+ tokens = parent.nodes
+
+ // Words
+ } else {
+ next = pos
+ do {
+ if (code === backslash) {
+ next += 1
+ }
+ next += 1
+ code = value.charCodeAt(next)
+ } while (
+ next < max &&
+ !(
+ code <= 32 ||
+ code === singleQuote ||
+ code === doubleQuote ||
+ code === comma ||
+ code === colon ||
+ code === slash ||
+ code === openParentheses ||
+ (code === star && parent && parent.type === 'function' && true) ||
+ (code === slash && parent.type === 'function' && true) ||
+ (code === closeParentheses && balanced)
+ )
+ )
+ token = value.slice(pos, next)
+
+ if (openParentheses === code) {
+ name = token
+ } else if (
+ (uLower === token.charCodeAt(0) || uUpper === token.charCodeAt(0)) &&
+ plus === token.charCodeAt(1) &&
+ isUnicodeRange.test(token.slice(2))
+ ) {
+ tokens.push({
+ type: 'unicode-range',
+ sourceIndex: pos,
+ sourceEndIndex: next,
+ value: token,
+ })
+ } else {
+ tokens.push({
+ type: 'word',
+ sourceIndex: pos,
+ sourceEndIndex: next,
+ value: token,
+ })
+ }
+
+ pos = next
+ }
+ }
+
+ for (pos = stack.length - 1; pos; pos -= 1) {
+ stack[pos].unclosed = true
+ stack[pos].sourceEndIndex = value.length
+ }
+
+ return stack[0].nodes
+}
diff --git a/src/value-parser/stringify.js b/src/value-parser/stringify.js
new file mode 100644
--- /dev/null
+++ b/src/value-parser/stringify.js
@@ -0,0 +1,41 @@
+function stringifyNode(node, custom) {
+ var type = node.type
+ var value = node.value
+ var buf
+ var customResult
+
+ if (custom && (customResult = custom(node)) !== undefined) {
+ return customResult
+ } else if (type === 'word' || type === 'space') {
+ return value
+ } else if (type === 'string') {
+ buf = node.quote || ''
+ return buf + value + (node.unclosed ? '' : buf)
+ } else if (type === 'comment') {
+ return '/*' + value + (node.unclosed ? '' : '*/')
+ } else if (type === 'div') {
+ return (node.before || '') + value + (node.after || '')
+ } else if (Array.isArray(node.nodes)) {
+ buf = stringify(node.nodes, custom)
+ if (type !== 'function') {
+ return buf
+ }
+ return value + '(' + (node.before || '') + buf + (node.after || '') + (node.unclosed ? '' : ')')
+ }
+ return value
+}
+
+function stringify(nodes, custom) {
+ var result, i
+
+ if (Array.isArray(nodes)) {
+ result = ''
+ for (i = nodes.length - 1; ~i; i -= 1) {
+ result = stringifyNode(nodes[i], custom) + result
+ }
+ return result
+ }
+ return stringifyNode(nodes, custom)
+}
+
+module.exports = stringify
diff --git a/src/value-parser/unit.js b/src/value-parser/unit.js
new file mode 100644
--- /dev/null
+++ b/src/value-parser/unit.js
@@ -0,0 +1,118 @@
+var minus = '-'.charCodeAt(0)
+var plus = '+'.charCodeAt(0)
+var dot = '.'.charCodeAt(0)
+var exp = 'e'.charCodeAt(0)
+var EXP = 'E'.charCodeAt(0)
+
+// Check if three code points would start a number
+// https://www.w3.org/TR/css-syntax-3/#starts-with-a-number
+function likeNumber(value) {
+ var code = value.charCodeAt(0)
+ var nextCode
+
+ if (code === plus || code === minus) {
+ nextCode = value.charCodeAt(1)
+
+ if (nextCode >= 48 && nextCode <= 57) {
+ return true
+ }
+
+ var nextNextCode = value.charCodeAt(2)
+
+ if (nextCode === dot && nextNextCode >= 48 && nextNextCode <= 57) {
+ return true
+ }
+
+ return false
+ }
+
+ if (code === dot) {
+ nextCode = value.charCodeAt(1)
+
+ if (nextCode >= 48 && nextCode <= 57) {
+ return true
+ }
+
+ return false
+ }
+
+ if (code >= 48 && code <= 57) {
+ return true
+ }
+
+ return false
+}
+
+// Consume a number
+// https://www.w3.org/TR/css-syntax-3/#consume-number
+module.exports = function (value) {
+ var pos = 0
+ var length = value.length
+ var code
+ var nextCode
+ var nextNextCode
+
+ if (length === 0 || !likeNumber(value)) {
+ return false
+ }
+
+ code = value.charCodeAt(pos)
+
+ if (code === plus || code === minus) {
+ pos++
+ }
+
+ while (pos < length) {
+ code = value.charCodeAt(pos)
+
+ if (code < 48 || code > 57) {
+ break
+ }
+
+ pos += 1
+ }
+
+ code = value.charCodeAt(pos)
+ nextCode = value.charCodeAt(pos + 1)
+
+ if (code === dot && nextCode >= 48 && nextCode <= 57) {
+ pos += 2
+
+ while (pos < length) {
+ code = value.charCodeAt(pos)
+
+ if (code < 48 || code > 57) {
+ break
+ }
+
+ pos += 1
+ }
+ }
+
+ code = value.charCodeAt(pos)
+ nextCode = value.charCodeAt(pos + 1)
+ nextNextCode = value.charCodeAt(pos + 2)
+
+ if (
+ (code === exp || code === EXP) &&
+ ((nextCode >= 48 && nextCode <= 57) ||
+ ((nextCode === plus || nextCode === minus) && nextNextCode >= 48 && nextNextCode <= 57))
+ ) {
+ pos += nextCode === plus || nextCode === minus ? 3 : 2
+
+ while (pos < length) {
+ code = value.charCodeAt(pos)
+
+ if (code < 48 || code > 57) {
+ break
+ }
+
+ pos += 1
+ }
+ }
+
+ return {
+ number: value.slice(0, pos),
+ unit: value.slice(pos),
+ }
+}
diff --git a/src/value-parser/walk.js b/src/value-parser/walk.js
new file mode 100644
--- /dev/null
+++ b/src/value-parser/walk.js
@@ -0,0 +1,18 @@
+module.exports = function walk(nodes, cb, bubble) {
+ var i, max, node, result
+
+ for (i = 0, max = nodes.length; i < max; i += 1) {
+ node = nodes[i]
+ if (!bubble) {
+ result = cb(node, i, nodes)
+ }
+
+ if (result !== false && node.type === 'function' && Array.isArray(node.nodes)) {
+ walk(node.nodes, cb, bubble)
+ }
+
+ if (bubble) {
+ cb(node, i, nodes)
+ }
+ }
+}
| diff --git a/tests/evaluateTailwindFunctions.test.js b/tests/evaluateTailwindFunctions.test.js
--- a/tests/evaluateTailwindFunctions.test.js
+++ b/tests/evaluateTailwindFunctions.test.js
@@ -1383,5 +1383,36 @@ crosscheck(({ stable, oxide }) => {
// 4. But we've not received any further logs about it
expect().toHaveBeenWarnedWith(['invalid-theme-key-in-class'])
})
+
+ test('it works mayhaps', async () => {
+ let input = css`
+ .test {
+ /* prettier-ignore */
+ inset: calc(-1 * (2*theme("spacing.4")));
+ /* prettier-ignore */
+ padding: calc(-1 * (2* theme("spacing.4")));
+ }
+ `
+
+ let output = css`
+ .test {
+ /* prettier-ignore */
+ inset: calc(-1 * (2*1rem));
+ /* prettier-ignore */
+ padding: calc(-1 * (2* 1rem));
+ }
+ `
+
+ return run(input, {
+ theme: {
+ spacing: {
+ 4: '1rem',
+ },
+ },
+ }).then((result) => {
+ expect(result.css).toMatchCss(output)
+ expect(result.warnings().length).toBe(0)
+ })
+ })
})
})
diff --git a/tests/source-maps.test.js b/tests/source-maps.test.js
--- a/tests/source-maps.test.js
+++ b/tests/source-maps.test.js
@@ -260,145 +260,143 @@ crosscheck(({ stable, oxide }) => {
'2:6-20 -> 304:2-12',
'2:20 -> 305:0',
'2:6 -> 307:0',
- '2:20 -> 309:1',
- '2:6 -> 310:0',
- '2:6-20 -> 311:2-12',
- '2:20 -> 312:0',
- '2:6 -> 314:0',
- '2:20 -> 316:1',
- '2:6 -> 318:0',
- '2:6-20 -> 319:2-18',
- '2:20 -> 320:0',
- '2:6 -> 322:0',
- '2:20 -> 325:1',
- '2:6 -> 327:0',
- '2:6-20 -> 329:2-20',
- '2:6-20 -> 330:2-24',
- '2:20 -> 331:0',
- '2:6 -> 333:0',
- '2:20 -> 335:1',
- '2:6 -> 337:0',
- '2:6-20 -> 339:2-17',
- '2:20 -> 340:0',
+ '2:6-20 -> 308:2-12',
+ '2:20 -> 309:0',
+ '2:6 -> 311:0',
+ '2:20 -> 313:1',
+ '2:6 -> 315:0',
+ '2:6-20 -> 316:2-18',
+ '2:20 -> 317:0',
+ '2:6 -> 319:0',
+ '2:20 -> 322:1',
+ '2:6 -> 324:0',
+ '2:6-20 -> 326:2-20',
+ '2:6-20 -> 327:2-24',
+ '2:20 -> 328:0',
+ '2:6 -> 330:0',
+ '2:20 -> 332:1',
+ '2:6 -> 334:0',
+ '2:6-20 -> 336:2-17',
+ '2:20 -> 337:0',
+ '2:6 -> 339:0',
+ '2:20 -> 341:1',
'2:6 -> 342:0',
- '2:20 -> 344:1',
- '2:6 -> 345:0',
- '2:6-20 -> 346:2-17',
- '2:20 -> 347:0',
- '2:6 -> 349:0',
- '2:20 -> 353:1',
- '2:6 -> 355:0',
- '2:6-20 -> 363:2-24',
- '2:6-20 -> 364:2-32',
- '2:20 -> 365:0',
- '2:6 -> 367:0',
- '2:20 -> 369:1',
- '2:6 -> 371:0',
- '2:6-20 -> 373:2-17',
- '2:6-20 -> 374:2-14',
- '2:20 -> 375:0',
- '2:6-20 -> 377:0-72',
- '2:6 -> 378:0',
- '2:6-20 -> 379:2-15',
- '2:20 -> 380:0',
- '2:6 -> 382:0',
- '2:6-20 -> 383:2-26',
- '2:6-20 -> 384:2-26',
- '2:6-20 -> 385:2-21',
- '2:6-20 -> 386:2-21',
- '2:6-20 -> 387:2-16',
- '2:6-20 -> 388:2-16',
- '2:6-20 -> 389:2-16',
- '2:6-20 -> 390:2-17',
- '2:6-20 -> 391:2-17',
- '2:6-20 -> 392:2-15',
- '2:6-20 -> 393:2-15',
- '2:6-20 -> 394:2-20',
- '2:6-20 -> 395:2-40',
- '2:6-20 -> 396:2-32',
- '2:6-20 -> 397:2-31',
- '2:6-20 -> 398:2-30',
- '2:6-20 -> 399:2-17',
- '2:6-20 -> 400:2-22',
- '2:6-20 -> 401:2-24',
- '2:6-20 -> 402:2-25',
- '2:6-20 -> 403:2-26',
- '2:6-20 -> 404:2-20',
- '2:6-20 -> 405:2-29',
- '2:6-20 -> 406:2-30',
- '2:6-20 -> 407:2-40',
- '2:6-20 -> 408:2-36',
- '2:6-20 -> 409:2-29',
- '2:6-20 -> 410:2-24',
- '2:6-20 -> 411:2-32',
- '2:6-20 -> 412:2-14',
+ '2:6-20 -> 343:2-17',
+ '2:20 -> 344:0',
+ '2:6 -> 346:0',
+ '2:20 -> 350:1',
+ '2:6 -> 352:0',
+ '2:6-20 -> 360:2-24',
+ '2:6-20 -> 361:2-32',
+ '2:20 -> 362:0',
+ '2:6 -> 364:0',
+ '2:20 -> 366:1',
+ '2:6 -> 368:0',
+ '2:6-20 -> 370:2-17',
+ '2:6-20 -> 371:2-14',
+ '2:20 -> 372:0',
+ '2:6-20 -> 374:0-72',
+ '2:6 -> 375:0',
+ '2:6-20 -> 376:2-15',
+ '2:20 -> 377:0',
+ '2:6 -> 379:0',
+ '2:6-20 -> 380:2-26',
+ '2:6-20 -> 381:2-26',
+ '2:6-20 -> 382:2-21',
+ '2:6-20 -> 383:2-21',
+ '2:6-20 -> 384:2-16',
+ '2:6-20 -> 385:2-16',
+ '2:6-20 -> 386:2-16',
+ '2:6-20 -> 387:2-17',
+ '2:6-20 -> 388:2-17',
+ '2:6-20 -> 389:2-15',
+ '2:6-20 -> 390:2-15',
+ '2:6-20 -> 391:2-20',
+ '2:6-20 -> 392:2-40',
+ '2:6-20 -> 393:2-32',
+ '2:6-20 -> 394:2-31',
+ '2:6-20 -> 395:2-30',
+ '2:6-20 -> 396:2-17',
+ '2:6-20 -> 397:2-22',
+ '2:6-20 -> 398:2-24',
+ '2:6-20 -> 399:2-25',
+ '2:6-20 -> 400:2-26',
+ '2:6-20 -> 401:2-20',
+ '2:6-20 -> 402:2-29',
+ '2:6-20 -> 403:2-30',
+ '2:6-20 -> 404:2-40',
+ '2:6-20 -> 405:2-36',
+ '2:6-20 -> 406:2-29',
+ '2:6-20 -> 407:2-24',
+ '2:6-20 -> 408:2-32',
+ '2:6-20 -> 409:2-14',
+ '2:6-20 -> 410:2-20',
+ '2:6-20 -> 411:2-18',
+ '2:6-20 -> 412:2-19',
'2:6-20 -> 413:2-20',
- '2:6-20 -> 414:2-18',
- '2:6-20 -> 415:2-19',
- '2:6-20 -> 416:2-20',
- '2:6-20 -> 417:2-16',
- '2:6-20 -> 418:2-18',
- '2:6-20 -> 419:2-15',
- '2:6-20 -> 420:2-21',
- '2:6-20 -> 421:2-23',
+ '2:6-20 -> 414:2-16',
+ '2:6-20 -> 415:2-18',
+ '2:6-20 -> 416:2-15',
+ '2:6-20 -> 417:2-21',
+ '2:6-20 -> 418:2-23',
+ '2:6-20 -> 419:2-29',
+ '2:6-20 -> 420:2-27',
+ '2:6-20 -> 421:2-28',
'2:6-20 -> 422:2-29',
- '2:6-20 -> 423:2-27',
- '2:6-20 -> 424:2-28',
- '2:6-20 -> 425:2-29',
- '2:6-20 -> 426:2-25',
- '2:6-20 -> 427:2-26',
- '2:6-20 -> 428:2-27',
- '2:6 -> 429:2',
- '2:20 -> 430:0',
- '2:6 -> 432:0',
- '2:6-20 -> 433:2-26',
- '2:6-20 -> 434:2-26',
- '2:6-20 -> 435:2-21',
- '2:6-20 -> 436:2-21',
- '2:6-20 -> 437:2-16',
- '2:6-20 -> 438:2-16',
- '2:6-20 -> 439:2-16',
- '2:6-20 -> 440:2-17',
- '2:6-20 -> 441:2-17',
- '2:6-20 -> 442:2-15',
- '2:6-20 -> 443:2-15',
- '2:6-20 -> 444:2-20',
- '2:6-20 -> 445:2-40',
- '2:6-20 -> 446:2-32',
- '2:6-20 -> 447:2-31',
- '2:6-20 -> 448:2-30',
- '2:6-20 -> 449:2-17',
- '2:6-20 -> 450:2-22',
- '2:6-20 -> 451:2-24',
- '2:6-20 -> 452:2-25',
- '2:6-20 -> 453:2-26',
- '2:6-20 -> 454:2-20',
- '2:6-20 -> 455:2-29',
- '2:6-20 -> 456:2-30',
- '2:6-20 -> 457:2-40',
- '2:6-20 -> 458:2-36',
- '2:6-20 -> 459:2-29',
- '2:6-20 -> 460:2-24',
- '2:6-20 -> 461:2-32',
- '2:6-20 -> 462:2-14',
+ '2:6-20 -> 423:2-25',
+ '2:6-20 -> 424:2-26',
+ '2:6-20 -> 425:2-27',
+ '2:6 -> 426:2',
+ '2:20 -> 427:0',
+ '2:6 -> 429:0',
+ '2:6-20 -> 430:2-26',
+ '2:6-20 -> 431:2-26',
+ '2:6-20 -> 432:2-21',
+ '2:6-20 -> 433:2-21',
+ '2:6-20 -> 434:2-16',
+ '2:6-20 -> 435:2-16',
+ '2:6-20 -> 436:2-16',
+ '2:6-20 -> 437:2-17',
+ '2:6-20 -> 438:2-17',
+ '2:6-20 -> 439:2-15',
+ '2:6-20 -> 440:2-15',
+ '2:6-20 -> 441:2-20',
+ '2:6-20 -> 442:2-40',
+ '2:6-20 -> 443:2-32',
+ '2:6-20 -> 444:2-31',
+ '2:6-20 -> 445:2-30',
+ '2:6-20 -> 446:2-17',
+ '2:6-20 -> 447:2-22',
+ '2:6-20 -> 448:2-24',
+ '2:6-20 -> 449:2-25',
+ '2:6-20 -> 450:2-26',
+ '2:6-20 -> 451:2-20',
+ '2:6-20 -> 452:2-29',
+ '2:6-20 -> 453:2-30',
+ '2:6-20 -> 454:2-40',
+ '2:6-20 -> 455:2-36',
+ '2:6-20 -> 456:2-29',
+ '2:6-20 -> 457:2-24',
+ '2:6-20 -> 458:2-32',
+ '2:6-20 -> 459:2-14',
+ '2:6-20 -> 460:2-20',
+ '2:6-20 -> 461:2-18',
+ '2:6-20 -> 462:2-19',
'2:6-20 -> 463:2-20',
- '2:6-20 -> 464:2-18',
- '2:6-20 -> 465:2-19',
- '2:6-20 -> 466:2-20',
- '2:6-20 -> 467:2-16',
- '2:6-20 -> 468:2-18',
- '2:6-20 -> 469:2-15',
- '2:6-20 -> 470:2-21',
- '2:6-20 -> 471:2-23',
+ '2:6-20 -> 464:2-16',
+ '2:6-20 -> 465:2-18',
+ '2:6-20 -> 466:2-15',
+ '2:6-20 -> 467:2-21',
+ '2:6-20 -> 468:2-23',
+ '2:6-20 -> 469:2-29',
+ '2:6-20 -> 470:2-27',
+ '2:6-20 -> 471:2-28',
'2:6-20 -> 472:2-29',
- '2:6-20 -> 473:2-27',
- '2:6-20 -> 474:2-28',
- '2:6-20 -> 475:2-29',
- '2:6-20 -> 476:2-25',
- '2:6-20 -> 477:2-26',
- '2:6-20 -> 478:2-27',
- '2:6 -> 479:2',
- '2:20 -> 480:0',
+ '2:6-20 -> 473:2-25',
+ '2:6-20 -> 474:2-26',
+ '2:6-20 -> 475:2-27',
+ '2:6 -> 476:2',
+ '2:20 -> 477:0',
])
})
| Incorrect parsing of theme functions within calc operations without spaces
<!-- Please provide all of the information requested below. We're a small team and without all of this information it's not possible for us to help and your bug report will be closed. -->
**What version of Tailwind CSS are you using?**
v3.3.1
**What build tool (or framework if it abstracts the build tool) are you using?**
[play.tailwindcss.com](https://play.tailwindcss.com/) or Webpack
**What version of Node.js are you using?**
N/A
**What browser are you using?**
Chrome
**What operating system are you using?**
macOS
**Reproduction URL**
https://play.tailwindcss.com/sQrIVhIaM7?file=css
**Describe your issue**
Usage of the `theme` function isn’t getting replaced by the corresponding value when the function is in a `calc` expression without spaces. For example:
```css
.test {
/* Input: */
inset: calc(-1 * (2*theme("spacing.4")));
/* Expected: */
inset: calc(-1 * (2*1rem));
/* Actual: */
inset: calc(-1 * (2*theme("spacing.4")));
}
```
---
I believe this might be the same issue as https://github.com/TrySound/postcss-value-parser/issues/86, but thought I’d report it here as I’m not 100% sure and it definitely affects Tailwind.
This happened for us because we use Sass alongside Tailwind, via Webpack. sass-loader apparently outputs Sass differently in Webpack production and development mode (https://github.com/webpack-contrib/sass-loader/issues/1129), with spaces removed in production.
| 2023-05-04T13:50:14 | 3.3 | [
"tests/source-maps.test.js"
] | [
"tests/evaluateTailwindFunctions.test.js"
] | TypeScript | [] | [
"https://play.tailwindcss.com/",
"https://play.tailwindcss.com/sQrIVhIaM7?file=css"
] |
|
tailwindlabs/tailwindcss | 11,345 | tailwindlabs__tailwindcss-11345 | [
"11331"
] | eb8d9294c51c05713b93eb7478993b76be9d1bab | diff --git a/src/util/pseudoElements.js b/src/util/pseudoElements.js
--- a/src/util/pseudoElements.js
+++ b/src/util/pseudoElements.js
@@ -19,12 +19,13 @@
// **Jumpable**
// Any terminal element may "jump" over combinators when moving to the end of the selector
//
-// This is a backwards-compat quirk of :before and :after variants.
+// This is a backwards-compat quirk of pseudo element variants from earlier versions of Tailwind CSS.
/** @typedef {'terminal' | 'actionable' | 'jumpable'} PseudoProperty */
/** @type {Record<string, PseudoProperty[]>} */
let elementProperties = {
+ // Pseudo elements from the spec
'::after': ['terminal', 'jumpable'],
'::backdrop': ['terminal', 'jumpable'],
'::before': ['terminal', 'jumpable'],
@@ -41,18 +42,14 @@ let elementProperties = {
'::spelling-error': ['terminal'],
'::target-text': ['terminal'],
- // other
+ // Pseudo elements from the spec with special rules
'::file-selector-button': ['terminal', 'actionable'],
- '::-webkit-progress-bar': ['terminal', 'actionable'],
- // Webkit scroll bar pseudo elements can be combined with user-action pseudo classes
- '::-webkit-scrollbar': ['terminal', 'actionable'],
- '::-webkit-scrollbar-button': ['terminal', 'actionable'],
- '::-webkit-scrollbar-thumb': ['terminal', 'actionable'],
- '::-webkit-scrollbar-track': ['terminal', 'actionable'],
- '::-webkit-scrollbar-track-piece': ['terminal', 'actionable'],
- '::-webkit-scrollbar-corner': ['terminal', 'actionable'],
- '::-webkit-resizer': ['terminal', 'actionable'],
+ // Library-specific pseudo elements used by component libraries
+ // These are Shadow DOM-like
+ '::deep': ['actionable'],
+ '::v-deep': ['actionable'],
+ '::ng-deep': ['actionable'],
// Note: As a rule, double colons (::) should be used instead of a single colon
// (:). This distinguishes pseudo-classes from pseudo-elements. However, since
@@ -65,8 +62,8 @@ let elementProperties = {
// The default value is used when the pseudo-element is not recognized
// Because it's not recognized, we don't know if it's terminal or not
- // So we assume it can't be moved AND can have user-action pseudo classes attached to it
- __default__: ['actionable'],
+ // So we assume it can be moved AND can have user-action pseudo classes attached to it
+ __default__: ['terminal', 'actionable'],
}
/**
| diff --git a/tests/util/apply-important-selector.test.js b/tests/util/apply-important-selector.test.js
--- a/tests/util/apply-important-selector.test.js
+++ b/tests/util/apply-important-selector.test.js
@@ -16,6 +16,10 @@ it.each`
${':is(.foo) :is(.bar)'} | ${'#app :is(:is(.foo) :is(.bar))'}
${':is(.foo)::before'} | ${'#app :is(.foo)::before'}
${'.foo:before'} | ${'#app :is(.foo):before'}
+ ${'.foo::some-uknown-pseudo'} | ${'#app :is(.foo)::some-uknown-pseudo'}
+ ${'.foo::some-uknown-pseudo:hover'} | ${'#app :is(.foo)::some-uknown-pseudo:hover'}
+ ${'.foo:focus::some-uknown-pseudo:hover'} | ${'#app :is(.foo:focus)::some-uknown-pseudo:hover'}
+ ${'.foo:hover::some-uknown-pseudo:focus'} | ${'#app :is(.foo:hover)::some-uknown-pseudo:focus'}
`('should generate "$after" from "$before"', ({ before, after }) => {
expect(applyImportantSelector(before, '#app')).toEqual(after)
})
| Some arbitrary pseudo-elements are moved inside `:is()` when using `important` config option, breaking the selector
<blockquote><details><summary><b>Tailwind CSS/browser/device version information</b></summary></br >
**What version of Tailwind CSS are you using?**
v3.3.2
**What build tool (or framework if it abstracts the build tool) are you using?**
Tailwind Play
**What version of Node.js are you using?**
N/A
**What browser are you using?**
Chrome v114.0.5735.90
**What operating system are you using?**
macOS v13.1 (22C65)
</details></blockquote>
**Reproduction URL**
* ✅ Works when not using `important` (w/o `:is()`): https://play.tailwindcss.com/yOqpv2N25J
* ❌ Breaks when using `important` (w/ `:is()`): https://play.tailwindcss.com/gVUTg4DLNK
**Describe your issue**
This code:
**Config**
```js
module.exports = { important: 'body' }
```
**Markup**
```html
<progress value="5" max="10" class="
bg-gray-300
[&::-webkit-progress-bar]:bg-gray-300
[&::-moz-progress-bar]:bg-blue-500
[&::-webkit-progress-value]:bg-blue-500"
/>
```
**Generated CSS** (invalid)
```css
body :is(.\[\&\:\:-webkit-progress-value\]\:bg-blue-500::-webkit-progress-value) {
--tw-bg-opacity: 1;
background-color: rgb(59 130 246 / var(--tw-bg-opacity));
}
```
Instead of including the pseudo-element on the inside of `:is()`, it should remain on the outside, where it is valid.
For example, the rendered output of `[&::-moz-progress-bar]:[&:hover]:font-bold` should be…
```css
body :is(.\[\&\:\:-moz-progress-bar\]\:\[\&\:hover\]\:font-bold:hover)::-moz-progress-bar {
font-weight: 700;
}
```
but is currently…
```css
body :is(.\[\&\:\:-moz-progress-bar\]\:\[\&\:hover\]\:font-bold:hover::-moz-progress-bar) {
font-weight: 700;
}
```
This is unique to pseudo-elements and does not apply to pseudo-classes. Pseudo-classes should remain on the inside of `:is()`. This already works for most pseudo-elements in TailwindCSS, so maybe it's just keeping an index of all of them. Could TailwindCSS be set up such that any time a double `::` appears, it instructs the compiler to display it after the `:is()`?
I considered maybe it's the case that it keeps them all inside unless on a specific list since some devs write `:before` vs. `::before`, and trying to determine that intelligently for some like that is fine, but could the double `::`—when used—be set to always indicate a pseudo-element (vs. a pseudo-class)?
| I meet the same problem..Even using the @csstools/postcss-is-pseudo-class plugin can't convert successfully invalid:is() | 2023-06-02T18:11:03 | 3.3 | [] | [
"tests/util/apply-important-selector.test.js"
] | TypeScript | [] | [
"https://play.tailwindcss.com/gVUTg4DLNK",
"https://play.tailwindcss.com/yOqpv2N25J"
] |
End of preview. Expand
in Data Studio
This repository contains the data presented in OmniGIRL: A Multilingual and Multimodal Benchmark for GitHub Issue Resolution. OmniGIRL is a GitHub issue resolution benchmark that is multilingual, multimodal, and multi-domain. It includes 959 task instances collected from repositories across four programming languages (Python, JavaScript, TypeScript, and Java) and eight different domains.
- Downloads last month
- 78