repo
stringclasses 15
values | pull_number
int64 147
18.3k
| instance_id
stringlengths 14
31
| issue_numbers
sequencelengths 1
3
| base_commit
stringlengths 40
40
| patch
stringlengths 289
585k
| test_patch
stringlengths 355
6.82M
| problem_statement
stringlengths 25
49.4k
| hints_text
stringlengths 0
58.9k
| created_at
unknowndate 2014-08-08 22:09:38
2024-06-28 03:13:12
| version
stringclasses 110
values | PASS_TO_PASS
sequencelengths 0
4.82k
| FAIL_TO_PASS
sequencelengths 1
1.06k
| language
stringclasses 4
values | image_urls
sequencelengths 0
4
| website links
sequencelengths 0
4
|
---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|
statsmodels/statsmodels | 8,889 | statsmodels__statsmodels-8889 | [
"4751"
] | 77cb066320391ffed4196a32491ddca28e8c9122 | diff --git a/statsmodels/genmod/generalized_linear_model.py b/statsmodels/genmod/generalized_linear_model.py
--- a/statsmodels/genmod/generalized_linear_model.py
+++ b/statsmodels/genmod/generalized_linear_model.py
@@ -54,6 +54,7 @@
# need import in module instead of lazily to copy `__doc__`
from . import families
+
__all__ = ['GLM', 'PredictionResultsMean']
@@ -624,6 +625,90 @@ def information(self, params, scale=None):
scale = float_like(scale, "scale", optional=True)
return self.hessian(params, scale=scale, observed=False)
+ def _derivative_exog(self, params, exog=None, transform="dydx",
+ dummy_idx=None, count_idx=None,
+ offset=None, exposure=None):
+ """
+ Derivative of mean, expected endog with respect to the parameters
+ """
+ if exog is None:
+ exog = self.exog
+ if (offset is not None) or (exposure is not None):
+ raise NotImplementedError("offset and exposure not supported")
+
+ lin_pred = self.predict(params, exog, which="linear",
+ offset=offset, exposure=exposure)
+
+ k_extra = getattr(self, 'k_extra', 0)
+ params_exog = params if k_extra == 0 else params[:-k_extra]
+
+ margeff = (self.family.link.inverse_deriv(lin_pred)[:, None] *
+ params_exog)
+ if 'ex' in transform:
+ margeff *= exog
+ if 'ey' in transform:
+ mean = self.family.link.inverse(lin_pred)
+ margeff /= mean[:,None]
+
+ return self._derivative_exog_helper(margeff, params, exog,
+ dummy_idx, count_idx, transform)
+
+ def _derivative_exog_helper(self, margeff, params, exog, dummy_idx,
+ count_idx, transform):
+ """
+ Helper for _derivative_exog to wrap results appropriately
+ """
+ from statsmodels.discrete.discrete_margins import (
+ _get_count_effects,
+ _get_dummy_effects,
+ )
+
+ if count_idx is not None:
+ margeff = _get_count_effects(margeff, exog, count_idx, transform,
+ self, params)
+ if dummy_idx is not None:
+ margeff = _get_dummy_effects(margeff, exog, dummy_idx, transform,
+ self, params)
+
+ return margeff
+
+ def _derivative_predict(self, params, exog=None, transform='dydx',
+ offset=None, exposure=None):
+ """
+ Derivative of the expected endog with respect to the parameters.
+
+ Parameters
+ ----------
+ params : ndarray
+ parameter at which score is evaluated
+ exog : ndarray or None
+ Explanatory variables at which derivative are computed.
+ If None, then the estimation exog is used.
+ offset, exposure : None
+ Not yet implemented.
+
+ Returns
+ -------
+ The value of the derivative of the expected endog with respect
+ to the parameter vector.
+ """
+ # core part is same as derivative_mean_params
+ # additionally handles exog and transform
+ if exog is None:
+ exog = self.exog
+ if (offset is not None) or (exposure is not None) or (
+ getattr(self, 'offset', None) is not None):
+ raise NotImplementedError("offset and exposure not supported")
+
+ lin_pred = self.predict(params, exog=exog, which="linear")
+ idl = self.family.link.inverse_deriv(lin_pred)
+ dmat = exog * idl[:, None]
+ if 'ey' in transform:
+ mean = self.family.link.inverse(lin_pred)
+ dmat /= mean[:, None]
+
+ return dmat
+
def _deriv_mean_dparams(self, params):
"""
Derivative of the expected endog with respect to the parameters.
@@ -2210,6 +2295,92 @@ def get_distribution(self, exog=None, exposure=None,
mu, scale, var_weights=var_weights, **kwds)
return distr
+ def get_margeff(self, at='overall', method='dydx', atexog=None,
+ dummy=False, count=False):
+ """Get marginal effects of the fitted model.
+
+ Warning: offset, exposure and weights (var_weights and freq_weights)
+ are not supported by margeff.
+
+ Parameters
+ ----------
+ at : str, optional
+ Options are:
+
+ - 'overall', The average of the marginal effects at each
+ observation.
+ - 'mean', The marginal effects at the mean of each regressor.
+ - 'median', The marginal effects at the median of each regressor.
+ - 'zero', The marginal effects at zero for each regressor.
+ - 'all', The marginal effects at each observation. If `at` is all
+ only margeff will be available from the returned object.
+
+ Note that if `exog` is specified, then marginal effects for all
+ variables not specified by `exog` are calculated using the `at`
+ option.
+ method : str, optional
+ Options are:
+
+ - 'dydx' - dy/dx - No transformation is made and marginal effects
+ are returned. This is the default.
+ - 'eyex' - estimate elasticities of variables in `exog` --
+ d(lny)/d(lnx)
+ - 'dyex' - estimate semi-elasticity -- dy/d(lnx)
+ - 'eydx' - estimate semi-elasticity -- d(lny)/dx
+
+ Note that tranformations are done after each observation is
+ calculated. Semi-elasticities for binary variables are computed
+ using the midpoint method. 'dyex' and 'eyex' do not make sense
+ for discrete variables. For interpretations of these methods
+ see notes below.
+ atexog : array_like, optional
+ Optionally, you can provide the exogenous variables over which to
+ get the marginal effects. This should be a dictionary with the key
+ as the zero-indexed column number and the value of the dictionary.
+ Default is None for all independent variables less the constant.
+ dummy : bool, optional
+ If False, treats binary variables (if present) as continuous. This
+ is the default. Else if True, treats binary variables as
+ changing from 0 to 1. Note that any variable that is either 0 or 1
+ is treated as binary. Each binary variable is treated separately
+ for now.
+ count : bool, optional
+ If False, treats count variables (if present) as continuous. This
+ is the default. Else if True, the marginal effect is the
+ change in probabilities when each observation is increased by one.
+
+ Returns
+ -------
+ DiscreteMargins : marginal effects instance
+ Returns an object that holds the marginal effects, standard
+ errors, confidence intervals, etc. See
+ `statsmodels.discrete.discrete_margins.DiscreteMargins` for more
+ information.
+
+ Notes
+ -----
+ Interpretations of methods:
+
+ - 'dydx' - change in `endog` for a change in `exog`.
+ - 'eyex' - proportional change in `endog` for a proportional change
+ in `exog`.
+ - 'dyex' - change in `endog` for a proportional change in `exog`.
+ - 'eydx' - proportional change in `endog` for a change in `exog`.
+
+ When using after Poisson, returns the expected number of events per
+ period, assuming that the model is loglinear.
+
+ Status : unsupported features offset, exposure and weights. Default
+ handling of freq_weights for average effect "overall" might change.
+
+ """
+ if getattr(self.model, "offset", None) is not None:
+ raise NotImplementedError("Margins with offset are not available.")
+ if (np.any(self.model.var_weights != 1) or
+ np.any(self.model.freq_weights != 1)):
+ warnings.warn("weights are not taken into account by margeff")
+ from statsmodels.discrete.discrete_margins import DiscreteMargins
+ return DiscreteMargins(self, (at, method, atexog, dummy, count))
@Appender(base.LikelihoodModelResults.remove_data.__doc__)
def remove_data(self):
diff --git a/statsmodels/stats/_delta_method.py b/statsmodels/stats/_delta_method.py
--- a/statsmodels/stats/_delta_method.py
+++ b/statsmodels/stats/_delta_method.py
@@ -151,6 +151,9 @@ def var(self):
"""
g = self.grad()
var = (np.dot(g, self.cov_params) * g).sum(-1)
+
+ if var.ndim == 2:
+ var = var.T
return var
def se_vectorized(self):
| diff --git a/statsmodels/discrete/tests/test_sandwich_cov.py b/statsmodels/discrete/tests/test_sandwich_cov.py
--- a/statsmodels/discrete/tests/test_sandwich_cov.py
+++ b/statsmodels/discrete/tests/test_sandwich_cov.py
@@ -9,6 +9,8 @@
import os
import numpy as np
import pandas as pd
+import pytest
+
import statsmodels.discrete.discrete_model as smd
from statsmodels.genmod.generalized_linear_model import GLM
from statsmodels.genmod import families
@@ -499,6 +501,21 @@ def test_score_test(self):
res_lm2 = res2.score_test(exog_extra, cov_type='nonrobust')
assert_allclose(np.hstack(res_lm1), np.hstack(res_lm2), rtol=5e-7)
+ def test_margeff(self):
+ if (isinstance(self.res2.model, OLS) or
+ hasattr(self.res1.model, "offset")):
+ pytest.skip("not available yet")
+
+ marg1 = self.res1.get_margeff()
+ marg2 = self.res2.get_margeff()
+ assert_allclose(marg1.margeff, marg2.margeff, rtol=1e-10)
+ assert_allclose(marg1.margeff_se, marg2.margeff_se, rtol=1e-10)
+
+ marg1 = self.res1.get_margeff(count=True, dummy=True)
+ marg2 = self.res2.get_margeff(count=True, dummy=True)
+ assert_allclose(marg1.margeff, marg2.margeff, rtol=1e-10)
+ assert_allclose(marg1.margeff_se, marg2.margeff_se, rtol=1e-10)
+
class TestGLMPoisson(CheckDiscreteGLM):
| ENH: add GLM Margins and supporting helper functions
GEE has margins, although incomplete, see #3704
GLM has nothing related yet.
To compute hatmatrix or, more precisely, generalized leverage for generic MLE that have predict, I need one method d mu / d params, which is in discrete_models used for the margin computations.
GEE has `_derivative_exog` which is almost the same as `_derivative_predict` which is needed for margins but not yet in GEE.
GEE has model specific methods that compute with different argument, eg, `mean_deriv`, which should extend to GLM also.
I need `_derivative_predict` and similar derivatives in a generic interface, so I don't have to access the link directly.
To go the other way as a generic cross-model pattern see #3915
(e.g. I added the family to Poisson so I have access to the inverse link deriv)
naming: I think we need more explicit names to refere to the function and wrt which variable we take the derivative, e.g.
`deriv_predict_params` or `deriv_mean_params`
`deriv_mean_exog`
`deriv_mean_linpred`
`deriv_score_endog` (that's what I also need for generalized leverage)
or maybe another `d` in there
`deriv_mean_dlinpred`
(The new count models have many "which" in predict and we need derivatives to be able to get standard errors. It will be similar for other two part models like BetaRegression)
To get the equivalent of DFFITS, I also need` deriv_mean_params` or `derivative_predict`
e.g. currently I have it specific to the availability of `model.family.link`, i.e. GLM, and Poisson for now
```
def d_fittedvalues(self):
# in discrete we cannot reuse results.fittedvalues
fittedvalues = self.results.predict()
deriv = self.results.model.family.link.inverse_deriv(fittedvalues)
return deriv * self.d_linpred
```
| I just realized (again) that GLMResults still does not have `get_margeff`
We need it to e.g. compare logit and probit, Binomial with different link functions, params have different scaling and cannot be directly compared.
see also #8802 for another reason to look at margins in binomial
If we don't want to support weights in the first version #7341, then we could raise NotImplementedError for non-default weights.
| "2023-05-19T16:30:44Z" | 0.14 | [
"statsmodels/discrete/tests/test_sandwich_cov.py::TestNegbinCluExposureFit::test_basic",
"statsmodels/discrete/tests/test_sandwich_cov.py::TestNegbinCluExposureFit::test_waldtest",
"statsmodels/discrete/tests/test_sandwich_cov.py::TestNegbinCluExposureFit::test_ttest",
"statsmodels/discrete/tests/test_sandwich_cov.py::TestNegbinCluExposureFit::test_oth",
"statsmodels/discrete/tests/test_sandwich_cov.py::TestPoissonHC1Fit::test_waldtest",
"statsmodels/discrete/tests/test_sandwich_cov.py::TestPoissonHC1Fit::test_basic",
"statsmodels/discrete/tests/test_sandwich_cov.py::TestPoissonHC1Fit::test_ttest",
"statsmodels/discrete/tests/test_sandwich_cov.py::TestPoissonHC1Fit::test_oth",
"statsmodels/discrete/tests/test_sandwich_cov.py::TestGLMPoissonClu::test_basic",
"statsmodels/discrete/tests/test_sandwich_cov.py::TestGLMPoissonClu::test_ttest",
"statsmodels/discrete/tests/test_sandwich_cov.py::TestGLMPoissonClu::test_oth",
"statsmodels/discrete/tests/test_sandwich_cov.py::TestGLMPoissonClu::test_waldtest",
"statsmodels/discrete/tests/test_sandwich_cov.py::TestGLMLogitOffset::test_score_hessian",
"statsmodels/discrete/tests/test_sandwich_cov.py::TestGLMLogitOffset::test_basic",
"statsmodels/discrete/tests/test_sandwich_cov.py::TestGLMLogitOffset::test_score_test",
"statsmodels/discrete/tests/test_sandwich_cov.py::TestPoissonHC1FitExposure::test_ttest",
"statsmodels/discrete/tests/test_sandwich_cov.py::TestPoissonHC1FitExposure::test_basic",
"statsmodels/discrete/tests/test_sandwich_cov.py::TestPoissonHC1FitExposure::test_waldtest",
"statsmodels/discrete/tests/test_sandwich_cov.py::TestPoissonHC1FitExposure::test_oth",
"statsmodels/discrete/tests/test_sandwich_cov.py::TestGLMGaussClu::test_score_hessian",
"statsmodels/discrete/tests/test_sandwich_cov.py::TestGLMGaussClu::test_score_test",
"statsmodels/discrete/tests/test_sandwich_cov.py::TestGLMGaussClu::test_basic",
"statsmodels/discrete/tests/test_sandwich_cov.py::TestGLMGaussHACUniform::test_score_hessian",
"statsmodels/discrete/tests/test_sandwich_cov.py::TestGLMGaussHACUniform::test_score_test",
"statsmodels/discrete/tests/test_sandwich_cov.py::TestGLMGaussHACUniform::test_cov_options",
"statsmodels/discrete/tests/test_sandwich_cov.py::TestGLMGaussHACUniform::test_basic",
"statsmodels/discrete/tests/test_sandwich_cov.py::TestNegbinClu::test_oth",
"statsmodels/discrete/tests/test_sandwich_cov.py::TestNegbinClu::test_basic",
"statsmodels/discrete/tests/test_sandwich_cov.py::TestNegbinClu::test_waldtest",
"statsmodels/discrete/tests/test_sandwich_cov.py::TestNegbinClu::test_ttest",
"statsmodels/discrete/tests/test_sandwich_cov.py::TestNegbinCluExposure::test_waldtest",
"statsmodels/discrete/tests/test_sandwich_cov.py::TestNegbinCluExposure::test_ttest",
"statsmodels/discrete/tests/test_sandwich_cov.py::TestNegbinCluExposure::test_basic",
"statsmodels/discrete/tests/test_sandwich_cov.py::TestNegbinCluExposure::test_oth",
"statsmodels/discrete/tests/test_sandwich_cov.py::TestGLMGaussHACGroupsum::test_score_hessian",
"statsmodels/discrete/tests/test_sandwich_cov.py::TestGLMGaussHACGroupsum::test_kwd",
"statsmodels/discrete/tests/test_sandwich_cov.py::TestGLMGaussHACGroupsum::test_basic",
"statsmodels/discrete/tests/test_sandwich_cov.py::TestGLMGaussHACGroupsum::test_score_test",
"statsmodels/discrete/tests/test_sandwich_cov.py::TestNegbinCluFit::test_waldtest",
"statsmodels/discrete/tests/test_sandwich_cov.py::TestNegbinCluFit::test_basic",
"statsmodels/discrete/tests/test_sandwich_cov.py::TestNegbinCluFit::test_oth",
"statsmodels/discrete/tests/test_sandwich_cov.py::TestNegbinCluFit::test_ttest",
"statsmodels/discrete/tests/test_sandwich_cov.py::TestPoissonClu::test_basic",
"statsmodels/discrete/tests/test_sandwich_cov.py::TestPoissonClu::test_oth",
"statsmodels/discrete/tests/test_sandwich_cov.py::TestPoissonClu::test_waldtest",
"statsmodels/discrete/tests/test_sandwich_cov.py::TestPoissonClu::test_ttest",
"statsmodels/discrete/tests/test_sandwich_cov.py::TestGLMGaussHC::test_score_test",
"statsmodels/discrete/tests/test_sandwich_cov.py::TestGLMGaussHC::test_score_hessian",
"statsmodels/discrete/tests/test_sandwich_cov.py::TestGLMGaussHC::test_basic",
"statsmodels/discrete/tests/test_sandwich_cov.py::TestPoissonHC1Generic::test_basic",
"statsmodels/discrete/tests/test_sandwich_cov.py::TestPoissonHC1Generic::test_oth",
"statsmodels/discrete/tests/test_sandwich_cov.py::TestPoissonHC1Generic::test_waldtest",
"statsmodels/discrete/tests/test_sandwich_cov.py::TestPoissonHC1Generic::test_ttest",
"statsmodels/discrete/tests/test_sandwich_cov.py::TestGLMGaussHAC::test_basic",
"statsmodels/discrete/tests/test_sandwich_cov.py::TestGLMGaussHAC::test_score_test",
"statsmodels/discrete/tests/test_sandwich_cov.py::TestGLMGaussHAC::test_score_hessian",
"statsmodels/discrete/tests/test_sandwich_cov.py::TestGLMGaussHACPanel::test_score_test",
"statsmodels/discrete/tests/test_sandwich_cov.py::TestGLMGaussHACPanel::test_kwd",
"statsmodels/discrete/tests/test_sandwich_cov.py::TestGLMGaussHACPanel::test_basic",
"statsmodels/discrete/tests/test_sandwich_cov.py::TestGLMGaussHACPanel::test_score_hessian",
"statsmodels/discrete/tests/test_sandwich_cov.py::TestGLMPoissonCluFit::test_basic",
"statsmodels/discrete/tests/test_sandwich_cov.py::TestGLMPoissonCluFit::test_ttest",
"statsmodels/discrete/tests/test_sandwich_cov.py::TestGLMPoissonCluFit::test_waldtest",
"statsmodels/discrete/tests/test_sandwich_cov.py::TestGLMPoissonCluFit::test_oth",
"statsmodels/discrete/tests/test_sandwich_cov.py::TestGLMGaussHACUniform2::test_score_test",
"statsmodels/discrete/tests/test_sandwich_cov.py::TestGLMGaussHACUniform2::test_score_hessian",
"statsmodels/discrete/tests/test_sandwich_cov.py::TestGLMGaussHACUniform2::test_cov_options",
"statsmodels/discrete/tests/test_sandwich_cov.py::TestGLMGaussHACUniform2::test_basic",
"statsmodels/discrete/tests/test_sandwich_cov.py::TestGLMProbitOffset::test_score_test",
"statsmodels/discrete/tests/test_sandwich_cov.py::TestGLMProbitOffset::test_basic",
"statsmodels/discrete/tests/test_sandwich_cov.py::TestGLMProbitOffset::test_score_hessian",
"statsmodels/discrete/tests/test_sandwich_cov.py::TestPoissonCluGeneric::test_oth",
"statsmodels/discrete/tests/test_sandwich_cov.py::TestPoissonCluGeneric::test_basic",
"statsmodels/discrete/tests/test_sandwich_cov.py::TestPoissonCluGeneric::test_waldtest",
"statsmodels/discrete/tests/test_sandwich_cov.py::TestPoissonCluGeneric::test_ttest",
"statsmodels/discrete/tests/test_sandwich_cov.py::TestGLMPoissonCluGeneric::test_waldtest",
"statsmodels/discrete/tests/test_sandwich_cov.py::TestGLMPoissonCluGeneric::test_ttest",
"statsmodels/discrete/tests/test_sandwich_cov.py::TestGLMPoissonCluGeneric::test_basic",
"statsmodels/discrete/tests/test_sandwich_cov.py::TestGLMPoissonCluGeneric::test_oth",
"statsmodels/discrete/tests/test_sandwich_cov.py::TestGLMPoissonHC1Generic::test_ttest",
"statsmodels/discrete/tests/test_sandwich_cov.py::TestGLMPoissonHC1Generic::test_oth",
"statsmodels/discrete/tests/test_sandwich_cov.py::TestGLMPoissonHC1Generic::test_basic",
"statsmodels/discrete/tests/test_sandwich_cov.py::TestGLMPoissonHC1Generic::test_waldtest",
"statsmodels/discrete/tests/test_sandwich_cov.py::TestPoissonCluExposureGeneric::test_waldtest",
"statsmodels/discrete/tests/test_sandwich_cov.py::TestPoissonCluExposureGeneric::test_oth",
"statsmodels/discrete/tests/test_sandwich_cov.py::TestPoissonCluExposureGeneric::test_basic",
"statsmodels/discrete/tests/test_sandwich_cov.py::TestPoissonCluExposureGeneric::test_ttest",
"statsmodels/discrete/tests/test_sandwich_cov.py::TestGLMProbit::test_basic",
"statsmodels/discrete/tests/test_sandwich_cov.py::TestGLMProbit::test_score_hessian",
"statsmodels/discrete/tests/test_sandwich_cov.py::TestGLMProbit::test_score_test",
"statsmodels/discrete/tests/test_sandwich_cov.py::TestPoissonCluExposure::test_oth",
"statsmodels/discrete/tests/test_sandwich_cov.py::TestPoissonCluExposure::test_basic",
"statsmodels/discrete/tests/test_sandwich_cov.py::TestPoissonCluExposure::test_waldtest",
"statsmodels/discrete/tests/test_sandwich_cov.py::TestPoissonCluExposure::test_ttest",
"statsmodels/discrete/tests/test_sandwich_cov.py::TestGLMGaussHAC2::test_score_test",
"statsmodels/discrete/tests/test_sandwich_cov.py::TestGLMGaussHAC2::test_basic",
"statsmodels/discrete/tests/test_sandwich_cov.py::TestGLMGaussHAC2::test_score_hessian",
"statsmodels/discrete/tests/test_sandwich_cov.py::TestGLMPoisson::test_score_test",
"statsmodels/discrete/tests/test_sandwich_cov.py::TestGLMPoisson::test_score_hessian",
"statsmodels/discrete/tests/test_sandwich_cov.py::TestGLMPoisson::test_basic",
"statsmodels/discrete/tests/test_sandwich_cov.py::TestGLMGaussNonRobust::test_score_hessian",
"statsmodels/discrete/tests/test_sandwich_cov.py::TestGLMGaussNonRobust::test_basic",
"statsmodels/discrete/tests/test_sandwich_cov.py::TestGLMGaussNonRobust::test_score_test",
"statsmodels/discrete/tests/test_sandwich_cov.py::TestPoissonCluFit::test_oth",
"statsmodels/discrete/tests/test_sandwich_cov.py::TestPoissonCluFit::test_basic_inference",
"statsmodels/discrete/tests/test_sandwich_cov.py::TestPoissonCluFit::test_waldtest",
"statsmodels/discrete/tests/test_sandwich_cov.py::TestPoissonCluFit::test_ttest",
"statsmodels/discrete/tests/test_sandwich_cov.py::TestPoissonCluFit::test_basic",
"statsmodels/discrete/tests/test_sandwich_cov.py::TestGLMLogit::test_score_hessian",
"statsmodels/discrete/tests/test_sandwich_cov.py::TestGLMLogit::test_basic",
"statsmodels/discrete/tests/test_sandwich_cov.py::TestGLMLogit::test_score_test",
"statsmodels/discrete/tests/test_sandwich_cov.py::TestNegbinCluGeneric::test_waldtest",
"statsmodels/discrete/tests/test_sandwich_cov.py::TestNegbinCluGeneric::test_ttest",
"statsmodels/discrete/tests/test_sandwich_cov.py::TestNegbinCluGeneric::test_basic",
"statsmodels/discrete/tests/test_sandwich_cov.py::TestNegbinCluGeneric::test_oth",
"statsmodels/discrete/tests/test_sandwich_cov.py::TestGLMGaussHACPanelGroups::test_score_hessian",
"statsmodels/discrete/tests/test_sandwich_cov.py::TestGLMGaussHACPanelGroups::test_basic",
"statsmodels/discrete/tests/test_sandwich_cov.py::TestGLMGaussHACPanelGroups::test_score_test",
"statsmodels/discrete/tests/test_sandwich_cov.py::TestGLMPoissonHC1Fit::test_oth",
"statsmodels/discrete/tests/test_sandwich_cov.py::TestGLMPoissonHC1Fit::test_waldtest",
"statsmodels/discrete/tests/test_sandwich_cov.py::TestGLMPoissonHC1Fit::test_ttest",
"statsmodels/discrete/tests/test_sandwich_cov.py::TestGLMPoissonHC1Fit::test_basic"
] | [
"statsmodels/discrete/tests/test_sandwich_cov.py::TestGLMProbit::test_margeff",
"statsmodels/discrete/tests/test_sandwich_cov.py::TestGLMPoisson::test_margeff",
"statsmodels/discrete/tests/test_sandwich_cov.py::TestGLMLogit::test_margeff"
] | Python | [] | [] |
statsmodels/statsmodels | 8,907 | statsmodels__statsmodels-8907 | [
"8905"
] | 77cb066320391ffed4196a32491ddca28e8c9122 | diff --git a/statsmodels/base/model.py b/statsmodels/base/model.py
--- a/statsmodels/base/model.py
+++ b/statsmodels/base/model.py
@@ -1639,7 +1639,11 @@ def t_test(self, r_matrix, cov_p=None, use_t=None):
"""
from patsy import DesignInfo
use_t = bool_like(use_t, "use_t", strict=True, optional=True)
- names = self.model.data.cov_names
+ if self.params.ndim == 2:
+ names = ['y{}_{}'.format(i[0], i[1])
+ for i in self.model.data.cov_names]
+ else:
+ names = self.model.data.cov_names
LC = DesignInfo(names).linear_constraint(r_matrix)
r_matrix, q_matrix = LC.coefs, LC.constants
num_ttests = r_matrix.shape[0]
@@ -1649,7 +1653,7 @@ def t_test(self, r_matrix, cov_p=None, use_t=None):
not hasattr(self, 'cov_params_default')):
raise ValueError('Need covariance of parameters for computing '
'T statistics')
- params = self.params.ravel()
+ params = self.params.ravel(order="F")
if num_params != params.shape[0]:
raise ValueError('r_matrix and params are not aligned')
if q_matrix is None:
@@ -1853,8 +1857,12 @@ def wald_test(self, r_matrix, cov_p=None, invcov=None,
use_f = (hasattr(self, 'use_t') and self.use_t)
from patsy import DesignInfo
- names = self.model.data.cov_names
- params = self.params.ravel()
+ if self.params.ndim == 2:
+ names = ['y{}_{}'.format(i[0], i[1])
+ for i in self.model.data.cov_names]
+ else:
+ names = self.model.data.cov_names
+ params = self.params.ravel(order="F")
LC = DesignInfo(names).linear_constraint(r_matrix)
r_matrix, q_matrix = LC.coefs, LC.constants
| diff --git a/statsmodels/discrete/tests/test_discrete.py b/statsmodels/discrete/tests/test_discrete.py
--- a/statsmodels/discrete/tests/test_discrete.py
+++ b/statsmodels/discrete/tests/test_discrete.py
@@ -2586,16 +2586,15 @@ def test_cov_confint_pandas():
assert isinstance(ci.index, pd.MultiIndex)
-def test_t_test():
+def test_mlogit_t_test():
# GH669, check t_test works in multivariate model
- data = load_anes96()
+ data = sm.datasets.anes96.load()
exog = sm.add_constant(data.exog, prepend=False)
res1 = sm.MNLogit(data.endog, exog).fit(disp=0)
r = np.ones(res1.cov_params().shape[0])
t1 = res1.t_test(r)
f1 = res1.f_test(r)
- data = sm.datasets.anes96.load()
exog = sm.add_constant(data.exog, prepend=False)
endog, exog = np.asarray(data.endog), np.asarray(exog)
res2 = sm.MNLogit(endog, exog).fit(disp=0)
@@ -2604,3 +2603,20 @@ def test_t_test():
assert_allclose(t1.effect, t2.effect)
assert_allclose(f1.statistic, f2.statistic)
+
+ tt = res1.t_test(np.eye(np.size(res2.params)))
+ assert_allclose(tt.tvalue.reshape(6,6, order="F"), res1.tvalues.to_numpy())
+ tt = res2.t_test(np.eye(np.size(res2.params)))
+ assert_allclose(tt.tvalue.reshape(6,6, order="F"), res2.tvalues)
+
+ wt = res1.wald_test(np.eye(np.size(res2.params))[0], scalar=True)
+ assert_allclose(wt.pvalue, res1.pvalues.to_numpy()[0, 0])
+
+
+ tt = res1.t_test("y1_logpopul")
+ wt = res1.wald_test("y1_logpopul", scalar=True)
+ assert_allclose(tt.pvalue, wt.pvalue)
+
+ wt = res1.wald_test("y1_logpopul, y2_logpopul", scalar=True)
+ # regression test
+ assert_allclose(wt.statistic, 5.68660562, rtol=1e-8)
| BUG: base t_test incorrect ravel for MNLogit, cov_names are not strings
see #8904
It looks like t_test and wald test ravel params incorrectly, should be "F" order.
res.model.data.cov_names is raveled by columns ("F" order), i.e stacked by equation not by exog var
(pr #6012)
brief check `t_test(np.eye(...))` does not match res.tvalues.
another bug:
cov_names is a multi-index, i.e tuple and not a list of strings.
I don't see how this could be used in a string constraints. (all my tries errored)
another bug:
column labes in params, bse do not correspond to endog names/levels as used in summary and in cov_names
e.g. hanes test case: summary reports y-values from 1 to 6, (0 is reference), while params columns are indexed from 0 to 5.
```
res2.bse.columns, res2.params.columns
(RangeIndex(start=0, stop=6, step=1), RangeIndex(start=0, stop=6, step=1))
```
Also
Current hanes version is not a good test case, because `res1.params.shape` is square (6, 6).
indexing, or reshaping bugs will not raise if rows and columns are mixed up.
usage of t_test and f_test/wald_test:
currently we can only use contrast/restriction matrices directly.
However, params and cov_params don't match up.
We need example and test cases that wald test specific parameters, and helper functions to test manova type constraints L P M.
(I started something like that for my experimental code for MultivariateLS)
| multi-index to string names
Do we have a convention for combining equation names and exog names?
```
['y{}_{}'.format(i[0], i[1]) for i in res2.model.data.cov_names]
['y1_logpopul',
'y1_selfLR',
'y1_age',
'y1_educ',
'y1_income',
'y1_const',
'y2_logpopul',
'y2_selfLR',
'y2_age',
'y2_educ',
'y2_income',
```
using this in `base` t_test, then t_test works without exception, and correctly after a ravel with order "F"
```
tt = res2.t_test("y1_age")
tt, tt.effect, tt.sd, tt.tvalue
(<class 'statsmodels.stats.contrast.ContrastResults'>
Test for Constraints
==============================================================================
coef std err z P>|z| [0.025 0.975]
------------------------------------------------------------------------------
c0 -0.0249 0.007 -3.823 0.000 -0.038 -0.012
==============================================================================,
array([-0.024945]),
array([[0.00652486]]),
array([[-3.82307077]]))
col = 0 # different col indexing in params, ...
res2.params.loc["age", col], res2.bse.loc["age", col], res2.tvalues.loc["age", col]
(-0.024944995441998526, 0.006524858401442056, -3.8230707713879957)
```
for anes test case: we can drop logpopul so that params is not square array
(lopopul is mainly important for small popul for strongly republican (last level of endog PID)
```
c = ["y{}_logpopul".format(i) for i in range(1, 7)]
cs = ", ".join(c)
tt = res2.t_test(cs)
print(c)
print(tt.summary(xname=c))
['y1_logpopul', 'y2_logpopul', 'y3_logpopul', 'y4_logpopul', 'y5_logpopul', 'y6_logpopul']
Test for Constraints
===============================================================================
coef std err z P>|z| [0.025 0.975]
-------------------------------------------------------------------------------
y1_logpopul -0.0115 0.034 -0.336 0.736 -0.079 0.056
y2_logpopul -0.0888 0.039 -2.266 0.023 -0.166 -0.012
y3_logpopul -0.1060 0.057 -1.858 0.063 -0.218 0.006
y4_logpopul -0.0916 0.044 -2.091 0.037 -0.177 -0.006
y5_logpopul -0.0933 0.039 -2.371 0.018 -0.170 -0.016
y6_logpopul -0.1409 0.042 -3.343 0.001 -0.223 -0.058
===============================================================================
wt = res2.wald_test(cs, scalar=True)
wt
<class 'statsmodels.stats.contrast.ContrastResults'>
<Wald test (chi2): statistic=16.092245564731254, p-value=0.013267319745202254, df_denom=6>
```
However, `wald_test_terms` is still broken for MNLogit.
It would be convenient for row or column hypothesis, e.g.
`res2.wald_test_terms(skip_single=True, combine_terms=["logpopul"])`
I don't see an obvious quickfix.
wald_test_terms handles more cases and term definition in DesignInfo is only for exog and does not take multivariate endog into account.
It might be possible to add an option to skip all parts in wald_test_terms except the pattern matching.
new issue #8906 expand partial constraint matrices to full constraint matrices.
another test: test that all slope coefficients (params except const) are equal across equations/levels
(not quite right?, this ignores reference level 0)
test sequential pairs
```
c = ["y{}_{} - y{}_{}".format(j, i, j-1, i) for j in range(2, 7) for i in res2.model.exog_names[:-1]]
cs = ", ".join(c)
tt = res2.t_test(cs)
print(c)
print(tt.summary(xname=c))
['y2_logpopul - y1_logpopul', 'y2_selfLR - y1_selfLR', 'y2_age - y1_age', 'y2_educ - y1_educ', 'y2_income - y1_income',
'y3_logpopul - y2_logpopul', 'y3_selfLR - y2_selfLR', 'y3_age - y2_age', 'y3_educ - y2_educ', 'y3_income - y2_income',
'y4_logpopul - y3_logpopul', 'y4_selfLR - y3_selfLR', 'y4_age - y3_age', 'y4_educ - y3_educ', 'y4_income - y3_income',
'y5_logpopul - y4_logpopul', 'y5_selfLR - y4_selfLR', 'y5_age - y4_age', 'y5_educ - y4_educ', 'y5_income - y4_income',
'y6_logpopul - y5_logpopul', 'y6_selfLR - y5_selfLR', 'y6_age - y5_age', 'y6_educ - y5_educ', 'y6_income - y5_income']
Test for Constraints
=============================================================================================
coef std err z P>|z| [0.025 0.975]
---------------------------------------------------------------------------------------------
y2_logpopul - y1_logpopul -0.0772 0.039 -1.979 0.048 -0.154 -0.001
y2_selfLR - y1_selfLR 0.0940 0.105 0.892 0.372 -0.113 0.300
y2_age - y1_age 0.0020 0.008 0.253 0.800 -0.014 0.018
y2_educ - y1_educ 0.0986 0.086 1.152 0.249 -0.069 0.266
y2_income - y1_income 0.0427 0.022 1.928 0.054 -0.001 0.086
y3_logpopul - y2_logpopul -0.0172 0.060 -0.289 0.772 -0.134 0.099
y3_selfLR - y2_selfLR 0.1818 0.163 1.112 0.266 -0.139 0.502
y3_age - y2_age 0.0080 0.012 0.659 0.510 -0.016 0.032
y3_educ - y2_educ -0.1882 0.133 -1.420 0.156 -0.448 0.072
y3_income - y2_income 0.0097 0.036 0.270 0.787 -0.061 0.080
y4_logpopul - y3_logpopul 0.0144 0.061 0.234 0.815 -0.106 0.135
y4_selfLR - y3_selfLR 0.7053 0.172 4.095 0.000 0.368 1.043
y4_age - y3_age 0.0062 0.012 0.501 0.616 -0.018 0.030
y4_educ - y3_educ 0.2070 0.136 1.523 0.128 -0.059 0.473
y4_income - y3_income 0.0269 0.038 0.713 0.476 -0.047 0.101
y5_logpopul - y4_logpopul -0.0017 0.042 -0.042 0.967 -0.083 0.080
y5_selfLR - y4_selfLR 0.0682 0.120 0.568 0.570 -0.167 0.303
y5_age - y4_age -0.0092 0.008 -1.117 0.264 -0.025 0.007
y5_educ - y4_educ 0.0171 0.090 0.190 0.850 -0.160 0.194
y5_income - y4_income -0.0035 0.026 -0.134 0.893 -0.055 0.048
y6_logpopul - y5_logpopul -0.0476 0.036 -1.306 0.192 -0.119 0.024
y6_selfLR - y5_selfLR 0.7231 0.124 5.816 0.000 0.479 0.967
y6_age - y5_age 0.0085 0.007 1.148 0.251 -0.006 0.023
y6_educ - y5_educ 0.1050 0.080 1.311 0.190 -0.052 0.262
y6_income - y5_income 0.0279 0.024 1.188 0.235 -0.018 0.074
=============================================================================================
wt = res2.wald_test(cs, scalar=True)
wt
<class 'statsmodels.stats.contrast.ContrastResults'>
<Wald test (chi2): statistic=236.40573788169553, p-value=2.559570053943716e-36, df_denom=25>
```
Note, endog PID is ordinal left to right. So, we could also use the OrderedModel with it. But constant slopes is strongly rejected. | "2023-06-08T19:16:14Z" | 0.14 | [
"statsmodels/discrete/tests/test_discrete.py::TestSweepAlphaL1::test_sweep_alpha",
"statsmodels/discrete/tests/test_discrete.py::TestProbitPowell::test_pred_table",
"statsmodels/discrete/tests/test_discrete.py::TestProbitPowell::test_resid_generalized",
"statsmodels/discrete/tests/test_discrete.py::TestProbitPowell::test_fit_regularized_invalid_method",
"statsmodels/discrete/tests/test_discrete.py::TestProbitPowell::test_llr_pvalue",
"statsmodels/discrete/tests/test_discrete.py::TestProbitPowell::test_aic",
"statsmodels/discrete/tests/test_discrete.py::TestProbitPowell::test_bse",
"statsmodels/discrete/tests/test_discrete.py::TestProbitPowell::test_predict_xb",
"statsmodels/discrete/tests/test_discrete.py::TestProbitPowell::test_loglikeobs",
"statsmodels/discrete/tests/test_discrete.py::TestProbitPowell::test_pvalues",
"statsmodels/discrete/tests/test_discrete.py::TestProbitPowell::test_zstat",
"statsmodels/discrete/tests/test_discrete.py::TestProbitPowell::test_dof",
"statsmodels/discrete/tests/test_discrete.py::TestProbitPowell::test_bic",
"statsmodels/discrete/tests/test_discrete.py::TestProbitPowell::test_llnull",
"statsmodels/discrete/tests/test_discrete.py::TestProbitPowell::test_summary_latex",
"statsmodels/discrete/tests/test_discrete.py::TestProbitPowell::test_llf",
"statsmodels/discrete/tests/test_discrete.py::TestProbitPowell::test_llr",
"statsmodels/discrete/tests/test_discrete.py::TestProbitPowell::test_params",
"statsmodels/discrete/tests/test_discrete.py::TestProbitPowell::test_predict",
"statsmodels/discrete/tests/test_discrete.py::TestProbitPowell::test_resid_dev",
"statsmodels/discrete/tests/test_discrete.py::TestProbitPowell::test_conf_int",
"statsmodels/discrete/tests/test_discrete.py::TestProbitPowell::test_resid_response",
"statsmodels/discrete/tests/test_discrete.py::TestProbitPowell::test_distr",
"statsmodels/discrete/tests/test_discrete.py::TestProbitPowell::test_jac",
"statsmodels/discrete/tests/test_discrete.py::TestProbitPowell::test_cov_params",
"statsmodels/discrete/tests/test_discrete.py::TestProbitL1::test_cov_params",
"statsmodels/discrete/tests/test_discrete.py::TestProbitL1::test_aic",
"statsmodels/discrete/tests/test_discrete.py::TestProbitL1::test_params",
"statsmodels/discrete/tests/test_discrete.py::TestProbitL1::test_bic",
"statsmodels/discrete/tests/test_discrete.py::TestProbitL1::test_nnz_params",
"statsmodels/discrete/tests/test_discrete.py::TestProbitL1::test_conf_int",
"statsmodels/discrete/tests/test_discrete.py::TestProbitL1::test_bse",
"statsmodels/discrete/tests/test_discrete.py::TestNegativeBinomialNBP2Null::test_llnull",
"statsmodels/discrete/tests/test_discrete.py::TestNegativeBinomialNBP2Null::test_start_null",
"statsmodels/discrete/tests/test_discrete.py::TestProbitCG::test_bse",
"statsmodels/discrete/tests/test_discrete.py::TestProbitCG::test_bic",
"statsmodels/discrete/tests/test_discrete.py::TestProbitCG::test_dof",
"statsmodels/discrete/tests/test_discrete.py::TestProbitCG::test_resid_response",
"statsmodels/discrete/tests/test_discrete.py::TestProbitCG::test_params",
"statsmodels/discrete/tests/test_discrete.py::TestProbitCG::test_summary_latex",
"statsmodels/discrete/tests/test_discrete.py::TestProbitCG::test_fit_regularized_invalid_method",
"statsmodels/discrete/tests/test_discrete.py::TestProbitCG::test_conf_int",
"statsmodels/discrete/tests/test_discrete.py::TestProbitCG::test_resid_dev",
"statsmodels/discrete/tests/test_discrete.py::TestProbitCG::test_pvalues",
"statsmodels/discrete/tests/test_discrete.py::TestProbitCG::test_distr",
"statsmodels/discrete/tests/test_discrete.py::TestProbitCG::test_zstat",
"statsmodels/discrete/tests/test_discrete.py::TestProbitCG::test_predict_xb",
"statsmodels/discrete/tests/test_discrete.py::TestProbitCG::test_jac",
"statsmodels/discrete/tests/test_discrete.py::TestProbitCG::test_loglikeobs",
"statsmodels/discrete/tests/test_discrete.py::TestProbitCG::test_pred_table",
"statsmodels/discrete/tests/test_discrete.py::TestProbitCG::test_resid_generalized",
"statsmodels/discrete/tests/test_discrete.py::TestProbitCG::test_llr_pvalue",
"statsmodels/discrete/tests/test_discrete.py::TestProbitCG::test_llnull",
"statsmodels/discrete/tests/test_discrete.py::TestProbitCG::test_cov_params",
"statsmodels/discrete/tests/test_discrete.py::TestProbitCG::test_predict",
"statsmodels/discrete/tests/test_discrete.py::TestProbitCG::test_llr",
"statsmodels/discrete/tests/test_discrete.py::TestProbitCG::test_aic",
"statsmodels/discrete/tests/test_discrete.py::TestProbitCG::test_llf",
"statsmodels/discrete/tests/test_discrete.py::TestNegativeBinomialNB2BFGS::test_dof",
"statsmodels/discrete/tests/test_discrete.py::TestNegativeBinomialNB2BFGS::test_predict",
"statsmodels/discrete/tests/test_discrete.py::TestNegativeBinomialNB2BFGS::test_llr_pvalue",
"statsmodels/discrete/tests/test_discrete.py::TestNegativeBinomialNB2BFGS::test_llf",
"statsmodels/discrete/tests/test_discrete.py::TestNegativeBinomialNB2BFGS::test_jac",
"statsmodels/discrete/tests/test_discrete.py::TestNegativeBinomialNB2BFGS::test_alpha",
"statsmodels/discrete/tests/test_discrete.py::TestNegativeBinomialNB2BFGS::test_fit_regularized_invalid_method",
"statsmodels/discrete/tests/test_discrete.py::TestNegativeBinomialNB2BFGS::test_summary_latex",
"statsmodels/discrete/tests/test_discrete.py::TestNegativeBinomialNB2BFGS::test_loglikeobs",
"statsmodels/discrete/tests/test_discrete.py::TestNegativeBinomialNB2BFGS::test_fittedvalues",
"statsmodels/discrete/tests/test_discrete.py::TestNegativeBinomialNB2BFGS::test_aic",
"statsmodels/discrete/tests/test_discrete.py::TestNegativeBinomialNB2BFGS::test_bic",
"statsmodels/discrete/tests/test_discrete.py::TestNegativeBinomialNB2BFGS::test_llnull",
"statsmodels/discrete/tests/test_discrete.py::TestNegativeBinomialNB2BFGS::test_zstat",
"statsmodels/discrete/tests/test_discrete.py::TestNegativeBinomialNB2BFGS::test_params",
"statsmodels/discrete/tests/test_discrete.py::TestNegativeBinomialNB2BFGS::test_predict_xb",
"statsmodels/discrete/tests/test_discrete.py::TestNegativeBinomialNB2BFGS::test_bse",
"statsmodels/discrete/tests/test_discrete.py::TestNegativeBinomialNB2BFGS::test_llr",
"statsmodels/discrete/tests/test_discrete.py::TestNegativeBinomialNB2BFGS::test_conf_int",
"statsmodels/discrete/tests/test_discrete.py::TestNegativeBinomialNB2BFGS::test_distr",
"statsmodels/discrete/tests/test_discrete.py::TestPoissonNull::test_llnull",
"statsmodels/discrete/tests/test_discrete.py::TestNegativeBinomialPNB1Newton::test_jac",
"statsmodels/discrete/tests/test_discrete.py::TestNegativeBinomialPNB1Newton::test_bse",
"statsmodels/discrete/tests/test_discrete.py::TestNegativeBinomialPNB1Newton::test_aic",
"statsmodels/discrete/tests/test_discrete.py::TestNegativeBinomialPNB1Newton::test_predict_xb",
"statsmodels/discrete/tests/test_discrete.py::TestNegativeBinomialPNB1Newton::test_llnull",
"statsmodels/discrete/tests/test_discrete.py::TestNegativeBinomialPNB1Newton::test_zstat",
"statsmodels/discrete/tests/test_discrete.py::TestNegativeBinomialPNB1Newton::test_conf_int",
"statsmodels/discrete/tests/test_discrete.py::TestNegativeBinomialPNB1Newton::test_dof",
"statsmodels/discrete/tests/test_discrete.py::TestNegativeBinomialPNB1Newton::test_llr_pvalue",
"statsmodels/discrete/tests/test_discrete.py::TestNegativeBinomialPNB1Newton::test_params",
"statsmodels/discrete/tests/test_discrete.py::TestNegativeBinomialPNB1Newton::test_predict",
"statsmodels/discrete/tests/test_discrete.py::TestNegativeBinomialPNB1Newton::test_summary_latex",
"statsmodels/discrete/tests/test_discrete.py::TestNegativeBinomialPNB1Newton::test_llr",
"statsmodels/discrete/tests/test_discrete.py::TestNegativeBinomialPNB1Newton::test_lnalpha",
"statsmodels/discrete/tests/test_discrete.py::TestNegativeBinomialPNB1Newton::test_bic",
"statsmodels/discrete/tests/test_discrete.py::TestNegativeBinomialPNB1Newton::test_loglikeobs",
"statsmodels/discrete/tests/test_discrete.py::TestNegativeBinomialPNB1Newton::test_distr",
"statsmodels/discrete/tests/test_discrete.py::TestNegativeBinomialPNB1Newton::test_llf",
"statsmodels/discrete/tests/test_discrete.py::TestNegativeBinomialPNB1Newton::test_fit_regularized_invalid_method",
"statsmodels/discrete/tests/test_discrete.py::TestProbitMinimizeDefault::test_dof",
"statsmodels/discrete/tests/test_discrete.py::TestProbitMinimizeDefault::test_aic",
"statsmodels/discrete/tests/test_discrete.py::TestProbitMinimizeDefault::test_bic",
"statsmodels/discrete/tests/test_discrete.py::TestProbitMinimizeDefault::test_jac",
"statsmodels/discrete/tests/test_discrete.py::TestProbitMinimizeDefault::test_pvalues",
"statsmodels/discrete/tests/test_discrete.py::TestProbitMinimizeDefault::test_cov_params",
"statsmodels/discrete/tests/test_discrete.py::TestProbitMinimizeDefault::test_resid_dev",
"statsmodels/discrete/tests/test_discrete.py::TestProbitMinimizeDefault::test_pred_table",
"statsmodels/discrete/tests/test_discrete.py::TestProbitMinimizeDefault::test_llr_pvalue",
"statsmodels/discrete/tests/test_discrete.py::TestProbitMinimizeDefault::test_distr",
"statsmodels/discrete/tests/test_discrete.py::TestProbitMinimizeDefault::test_loglikeobs",
"statsmodels/discrete/tests/test_discrete.py::TestProbitMinimizeDefault::test_zstat",
"statsmodels/discrete/tests/test_discrete.py::TestProbitMinimizeDefault::test_llr",
"statsmodels/discrete/tests/test_discrete.py::TestProbitMinimizeDefault::test_bse",
"statsmodels/discrete/tests/test_discrete.py::TestProbitMinimizeDefault::test_llnull",
"statsmodels/discrete/tests/test_discrete.py::TestProbitMinimizeDefault::test_fit_regularized_invalid_method",
"statsmodels/discrete/tests/test_discrete.py::TestProbitMinimizeDefault::test_llf",
"statsmodels/discrete/tests/test_discrete.py::TestProbitMinimizeDefault::test_conf_int",
"statsmodels/discrete/tests/test_discrete.py::TestProbitMinimizeDefault::test_resid_response",
"statsmodels/discrete/tests/test_discrete.py::TestProbitMinimizeDefault::test_resid_generalized",
"statsmodels/discrete/tests/test_discrete.py::TestProbitMinimizeDefault::test_summary_latex",
"statsmodels/discrete/tests/test_discrete.py::TestProbitMinimizeDefault::test_predict_xb",
"statsmodels/discrete/tests/test_discrete.py::TestProbitMinimizeDefault::test_predict",
"statsmodels/discrete/tests/test_discrete.py::TestProbitMinimizeDefault::test_params",
"statsmodels/discrete/tests/test_discrete.py::TestProbitBasinhopping::test_distr",
"statsmodels/discrete/tests/test_discrete.py::TestProbitBasinhopping::test_resid_dev",
"statsmodels/discrete/tests/test_discrete.py::TestProbitBasinhopping::test_jac",
"statsmodels/discrete/tests/test_discrete.py::TestProbitBasinhopping::test_pvalues",
"statsmodels/discrete/tests/test_discrete.py::TestProbitBasinhopping::test_llnull",
"statsmodels/discrete/tests/test_discrete.py::TestProbitBasinhopping::test_llr_pvalue",
"statsmodels/discrete/tests/test_discrete.py::TestProbitBasinhopping::test_pred_table",
"statsmodels/discrete/tests/test_discrete.py::TestProbitBasinhopping::test_cov_params",
"statsmodels/discrete/tests/test_discrete.py::TestProbitBasinhopping::test_fit_regularized_invalid_method",
"statsmodels/discrete/tests/test_discrete.py::TestProbitBasinhopping::test_zstat",
"statsmodels/discrete/tests/test_discrete.py::TestProbitBasinhopping::test_loglikeobs",
"statsmodels/discrete/tests/test_discrete.py::TestProbitBasinhopping::test_predict_xb",
"statsmodels/discrete/tests/test_discrete.py::TestProbitBasinhopping::test_predict",
"statsmodels/discrete/tests/test_discrete.py::TestProbitBasinhopping::test_conf_int",
"statsmodels/discrete/tests/test_discrete.py::TestProbitBasinhopping::test_summary_latex",
"statsmodels/discrete/tests/test_discrete.py::TestProbitBasinhopping::test_bic",
"statsmodels/discrete/tests/test_discrete.py::TestProbitBasinhopping::test_resid_generalized",
"statsmodels/discrete/tests/test_discrete.py::TestProbitBasinhopping::test_llr",
"statsmodels/discrete/tests/test_discrete.py::TestProbitBasinhopping::test_aic",
"statsmodels/discrete/tests/test_discrete.py::TestProbitBasinhopping::test_llf",
"statsmodels/discrete/tests/test_discrete.py::TestProbitBasinhopping::test_resid_response",
"statsmodels/discrete/tests/test_discrete.py::TestProbitBasinhopping::test_bse",
"statsmodels/discrete/tests/test_discrete.py::TestProbitBasinhopping::test_dof",
"statsmodels/discrete/tests/test_discrete.py::TestProbitBasinhopping::test_params",
"statsmodels/discrete/tests/test_discrete.py::TestGeneralizedPoisson_underdispersion::test_mean_var",
"statsmodels/discrete/tests/test_discrete.py::TestGeneralizedPoisson_underdispersion::test_jac",
"statsmodels/discrete/tests/test_discrete.py::TestGeneralizedPoisson_underdispersion::test_newton",
"statsmodels/discrete/tests/test_discrete.py::TestGeneralizedPoisson_underdispersion::test_distr",
"statsmodels/discrete/tests/test_discrete.py::TestGeneralizedPoisson_underdispersion::test_predict_prob",
"statsmodels/discrete/tests/test_discrete.py::TestGeneralizedPoisson_underdispersion::test_basic",
"statsmodels/discrete/tests/test_discrete.py::TestNegativeBinomialL1Compatability::test_df",
"statsmodels/discrete/tests/test_discrete.py::TestNegativeBinomialL1Compatability::test_t_test",
"statsmodels/discrete/tests/test_discrete.py::TestNegativeBinomialL1Compatability::test_params",
"statsmodels/discrete/tests/test_discrete.py::TestNegativeBinomialL1Compatability::test_cov_params",
"statsmodels/discrete/tests/test_discrete.py::TestNegativeBinomialL1Compatability::test_bad_r_matrix",
"statsmodels/discrete/tests/test_discrete.py::TestNegativeBinomialL1Compatability::test_f_test",
"statsmodels/discrete/tests/test_discrete.py::TestProbitNCG::test_resid_generalized",
"statsmodels/discrete/tests/test_discrete.py::TestProbitNCG::test_pvalues",
"statsmodels/discrete/tests/test_discrete.py::TestProbitNCG::test_loglikeobs",
"statsmodels/discrete/tests/test_discrete.py::TestProbitNCG::test_llnull",
"statsmodels/discrete/tests/test_discrete.py::TestProbitNCG::test_llr_pvalue",
"statsmodels/discrete/tests/test_discrete.py::TestProbitNCG::test_dof",
"statsmodels/discrete/tests/test_discrete.py::TestProbitNCG::test_jac",
"statsmodels/discrete/tests/test_discrete.py::TestProbitNCG::test_fit_regularized_invalid_method",
"statsmodels/discrete/tests/test_discrete.py::TestProbitNCG::test_bic",
"statsmodels/discrete/tests/test_discrete.py::TestProbitNCG::test_resid_dev",
"statsmodels/discrete/tests/test_discrete.py::TestProbitNCG::test_distr",
"statsmodels/discrete/tests/test_discrete.py::TestProbitNCG::test_summary_latex",
"statsmodels/discrete/tests/test_discrete.py::TestProbitNCG::test_resid_response",
"statsmodels/discrete/tests/test_discrete.py::TestProbitNCG::test_predict_xb",
"statsmodels/discrete/tests/test_discrete.py::TestProbitNCG::test_cov_params",
"statsmodels/discrete/tests/test_discrete.py::TestProbitNCG::test_predict",
"statsmodels/discrete/tests/test_discrete.py::TestProbitNCG::test_conf_int",
"statsmodels/discrete/tests/test_discrete.py::TestProbitNCG::test_llf",
"statsmodels/discrete/tests/test_discrete.py::TestProbitNCG::test_aic",
"statsmodels/discrete/tests/test_discrete.py::TestProbitNCG::test_bse",
"statsmodels/discrete/tests/test_discrete.py::TestProbitNCG::test_zstat",
"statsmodels/discrete/tests/test_discrete.py::TestProbitNCG::test_llr",
"statsmodels/discrete/tests/test_discrete.py::TestProbitNCG::test_pred_table",
"statsmodels/discrete/tests/test_discrete.py::TestProbitNCG::test_params",
"statsmodels/discrete/tests/test_discrete.py::TestMNLogitNewtonBaseZero::test_pvalues",
"statsmodels/discrete/tests/test_discrete.py::TestMNLogitNewtonBaseZero::test_aic",
"statsmodels/discrete/tests/test_discrete.py::TestMNLogitNewtonBaseZero::test_j",
"statsmodels/discrete/tests/test_discrete.py::TestMNLogitNewtonBaseZero::test_loglikeobs",
"statsmodels/discrete/tests/test_discrete.py::TestMNLogitNewtonBaseZero::test_llr_pvalue",
"statsmodels/discrete/tests/test_discrete.py::TestMNLogitNewtonBaseZero::test_margeff_overall",
"statsmodels/discrete/tests/test_discrete.py::TestMNLogitNewtonBaseZero::test_llr",
"statsmodels/discrete/tests/test_discrete.py::TestMNLogitNewtonBaseZero::test_params",
"statsmodels/discrete/tests/test_discrete.py::TestMNLogitNewtonBaseZero::test_k",
"statsmodels/discrete/tests/test_discrete.py::TestMNLogitNewtonBaseZero::test_endog_names",
"statsmodels/discrete/tests/test_discrete.py::TestMNLogitNewtonBaseZero::test_bse",
"statsmodels/discrete/tests/test_discrete.py::TestMNLogitNewtonBaseZero::test_predict_xb",
"statsmodels/discrete/tests/test_discrete.py::TestMNLogitNewtonBaseZero::test_dof",
"statsmodels/discrete/tests/test_discrete.py::TestMNLogitNewtonBaseZero::test_jac",
"statsmodels/discrete/tests/test_discrete.py::TestMNLogitNewtonBaseZero::test_llnull",
"statsmodels/discrete/tests/test_discrete.py::TestMNLogitNewtonBaseZero::test_fit_regularized_invalid_method",
"statsmodels/discrete/tests/test_discrete.py::TestMNLogitNewtonBaseZero::test_margeff_mean",
"statsmodels/discrete/tests/test_discrete.py::TestMNLogitNewtonBaseZero::test_summary_latex",
"statsmodels/discrete/tests/test_discrete.py::TestMNLogitNewtonBaseZero::test_margeff_dummy",
"statsmodels/discrete/tests/test_discrete.py::TestMNLogitNewtonBaseZero::test_resid",
"statsmodels/discrete/tests/test_discrete.py::TestMNLogitNewtonBaseZero::test_conf_int",
"statsmodels/discrete/tests/test_discrete.py::TestMNLogitNewtonBaseZero::test_llf",
"statsmodels/discrete/tests/test_discrete.py::TestMNLogitNewtonBaseZero::test_bic",
"statsmodels/discrete/tests/test_discrete.py::TestMNLogitNewtonBaseZero::test_pred_table",
"statsmodels/discrete/tests/test_discrete.py::TestMNLogitNewtonBaseZero::test_zstat",
"statsmodels/discrete/tests/test_discrete.py::TestMNLogitNewtonBaseZero::test_predict",
"statsmodels/discrete/tests/test_discrete.py::TestNegativeBinomialNB2Null::test_llnull",
"statsmodels/discrete/tests/test_discrete.py::TestNegativeBinomialPL1Compatability::test_bad_r_matrix",
"statsmodels/discrete/tests/test_discrete.py::TestNegativeBinomialPL1Compatability::test_f_test",
"statsmodels/discrete/tests/test_discrete.py::TestNegativeBinomialPL1Compatability::test_cov_params",
"statsmodels/discrete/tests/test_discrete.py::TestNegativeBinomialPL1Compatability::test_params",
"statsmodels/discrete/tests/test_discrete.py::TestNegativeBinomialPL1Compatability::test_df",
"statsmodels/discrete/tests/test_discrete.py::TestNegativeBinomialPL1Compatability::test_t_test",
"statsmodels/discrete/tests/test_discrete.py::test_issue_341",
"statsmodels/discrete/tests/test_discrete.py::test_predict_with_exposure",
"statsmodels/discrete/tests/test_discrete.py::test_iscount",
"statsmodels/discrete/tests/test_discrete.py::test_issue_339",
"statsmodels/discrete/tests/test_discrete.py::test_poisson_predict",
"statsmodels/discrete/tests/test_discrete.py::test_formula_missing_exposure",
"statsmodels/discrete/tests/test_discrete.py::test_mnlogit_basinhopping",
"statsmodels/discrete/tests/test_discrete.py::test_mnlogit_factor_categorical",
"statsmodels/discrete/tests/test_discrete.py::test_binary_pred_table_zeros",
"statsmodels/discrete/tests/test_discrete.py::test_perfect_prediction",
"statsmodels/discrete/tests/test_discrete.py::test_isdummy",
"statsmodels/discrete/tests/test_discrete.py::test_negative_binomial_default_alpha_param",
"statsmodels/discrete/tests/test_discrete.py::test_mnlogit_factor",
"statsmodels/discrete/tests/test_discrete.py::test_poisson_newton",
"statsmodels/discrete/tests/test_discrete.py::test_non_binary",
"statsmodels/discrete/tests/test_discrete.py::test_optim_kwds_prelim",
"statsmodels/discrete/tests/test_discrete.py::test_unchanging_degrees_of_freedom",
"statsmodels/discrete/tests/test_discrete.py::test_cov_confint_pandas",
"statsmodels/discrete/tests/test_discrete.py::test_mnlogit_float_name",
"statsmodels/discrete/tests/test_discrete.py::test_null_options",
"statsmodels/discrete/tests/test_discrete.py::TestProbitL1Compatability::test_bad_r_matrix",
"statsmodels/discrete/tests/test_discrete.py::TestProbitL1Compatability::test_t_test",
"statsmodels/discrete/tests/test_discrete.py::TestProbitL1Compatability::test_f_test",
"statsmodels/discrete/tests/test_discrete.py::TestProbitL1Compatability::test_df",
"statsmodels/discrete/tests/test_discrete.py::TestProbitL1Compatability::test_cov_params",
"statsmodels/discrete/tests/test_discrete.py::TestProbitL1Compatability::test_params",
"statsmodels/discrete/tests/test_discrete.py::TestNegativeBinomialNB2Newton::test_fit_regularized_invalid_method",
"statsmodels/discrete/tests/test_discrete.py::TestNegativeBinomialNB2Newton::test_conf_int",
"statsmodels/discrete/tests/test_discrete.py::TestNegativeBinomialNB2Newton::test_llnull",
"statsmodels/discrete/tests/test_discrete.py::TestNegativeBinomialNB2Newton::test_bic",
"statsmodels/discrete/tests/test_discrete.py::TestNegativeBinomialNB2Newton::test_alpha",
"statsmodels/discrete/tests/test_discrete.py::TestNegativeBinomialNB2Newton::test_summary_latex",
"statsmodels/discrete/tests/test_discrete.py::TestNegativeBinomialNB2Newton::test_zstat",
"statsmodels/discrete/tests/test_discrete.py::TestNegativeBinomialNB2Newton::test_dof",
"statsmodels/discrete/tests/test_discrete.py::TestNegativeBinomialNB2Newton::test_params",
"statsmodels/discrete/tests/test_discrete.py::TestNegativeBinomialNB2Newton::test_fittedvalues",
"statsmodels/discrete/tests/test_discrete.py::TestNegativeBinomialNB2Newton::test_distr",
"statsmodels/discrete/tests/test_discrete.py::TestNegativeBinomialNB2Newton::test_llf",
"statsmodels/discrete/tests/test_discrete.py::TestNegativeBinomialNB2Newton::test_bse",
"statsmodels/discrete/tests/test_discrete.py::TestNegativeBinomialNB2Newton::test_jac",
"statsmodels/discrete/tests/test_discrete.py::TestNegativeBinomialNB2Newton::test_predict",
"statsmodels/discrete/tests/test_discrete.py::TestNegativeBinomialNB2Newton::test_loglikeobs",
"statsmodels/discrete/tests/test_discrete.py::TestNegativeBinomialNB2Newton::test_aic",
"statsmodels/discrete/tests/test_discrete.py::TestNegativeBinomialNB2Newton::test_llr",
"statsmodels/discrete/tests/test_discrete.py::TestNegativeBinomialNB2Newton::test_llr_pvalue",
"statsmodels/discrete/tests/test_discrete.py::TestNegativeBinomialNB2Newton::test_predict_xb",
"statsmodels/discrete/tests/test_discrete.py::TestL1AlphaZeroMNLogit::test_basic_results",
"statsmodels/discrete/tests/test_discrete.py::TestPoissonNewton::test_bic",
"statsmodels/discrete/tests/test_discrete.py::TestPoissonNewton::test_dof",
"statsmodels/discrete/tests/test_discrete.py::TestPoissonNewton::test_margeff_dummy_overall",
"statsmodels/discrete/tests/test_discrete.py::TestPoissonNewton::test_resid",
"statsmodels/discrete/tests/test_discrete.py::TestPoissonNewton::test_predict_xb",
"statsmodels/discrete/tests/test_discrete.py::TestPoissonNewton::test_margeff_overall",
"statsmodels/discrete/tests/test_discrete.py::TestPoissonNewton::test_pvalues",
"statsmodels/discrete/tests/test_discrete.py::TestPoissonNewton::test_params",
"statsmodels/discrete/tests/test_discrete.py::TestPoissonNewton::test_conf_int",
"statsmodels/discrete/tests/test_discrete.py::TestPoissonNewton::test_zstat",
"statsmodels/discrete/tests/test_discrete.py::TestPoissonNewton::test_summary_latex",
"statsmodels/discrete/tests/test_discrete.py::TestPoissonNewton::test_llr",
"statsmodels/discrete/tests/test_discrete.py::TestPoissonNewton::test_predict_prob",
"statsmodels/discrete/tests/test_discrete.py::TestPoissonNewton::test_llr_pvalue",
"statsmodels/discrete/tests/test_discrete.py::TestPoissonNewton::test_loglikeobs",
"statsmodels/discrete/tests/test_discrete.py::TestPoissonNewton::test_llf",
"statsmodels/discrete/tests/test_discrete.py::TestPoissonNewton::test_jac",
"statsmodels/discrete/tests/test_discrete.py::TestPoissonNewton::test_predict",
"statsmodels/discrete/tests/test_discrete.py::TestPoissonNewton::test_aic",
"statsmodels/discrete/tests/test_discrete.py::TestPoissonNewton::test_fit_regularized_invalid_method",
"statsmodels/discrete/tests/test_discrete.py::TestPoissonNewton::test_llnull",
"statsmodels/discrete/tests/test_discrete.py::TestPoissonNewton::test_distr",
"statsmodels/discrete/tests/test_discrete.py::TestPoissonNewton::test_bse",
"statsmodels/discrete/tests/test_discrete.py::TestProbitBFGS::test_cov_params",
"statsmodels/discrete/tests/test_discrete.py::TestProbitBFGS::test_pred_table",
"statsmodels/discrete/tests/test_discrete.py::TestProbitBFGS::test_predict",
"statsmodels/discrete/tests/test_discrete.py::TestProbitBFGS::test_bse",
"statsmodels/discrete/tests/test_discrete.py::TestProbitBFGS::test_resid_dev",
"statsmodels/discrete/tests/test_discrete.py::TestProbitBFGS::test_conf_int",
"statsmodels/discrete/tests/test_discrete.py::TestProbitBFGS::test_pvalues",
"statsmodels/discrete/tests/test_discrete.py::TestProbitBFGS::test_aic",
"statsmodels/discrete/tests/test_discrete.py::TestProbitBFGS::test_jac",
"statsmodels/discrete/tests/test_discrete.py::TestProbitBFGS::test_resid_generalized",
"statsmodels/discrete/tests/test_discrete.py::TestProbitBFGS::test_llnull",
"statsmodels/discrete/tests/test_discrete.py::TestProbitBFGS::test_llr",
"statsmodels/discrete/tests/test_discrete.py::TestProbitBFGS::test_fit_regularized_invalid_method",
"statsmodels/discrete/tests/test_discrete.py::TestProbitBFGS::test_dof",
"statsmodels/discrete/tests/test_discrete.py::TestProbitBFGS::test_params",
"statsmodels/discrete/tests/test_discrete.py::TestProbitBFGS::test_llf",
"statsmodels/discrete/tests/test_discrete.py::TestProbitBFGS::test_loglikeobs",
"statsmodels/discrete/tests/test_discrete.py::TestProbitBFGS::test_zstat",
"statsmodels/discrete/tests/test_discrete.py::TestProbitBFGS::test_summary_latex",
"statsmodels/discrete/tests/test_discrete.py::TestProbitBFGS::test_predict_xb",
"statsmodels/discrete/tests/test_discrete.py::TestProbitBFGS::test_distr",
"statsmodels/discrete/tests/test_discrete.py::TestProbitBFGS::test_resid_response",
"statsmodels/discrete/tests/test_discrete.py::TestProbitBFGS::test_llr_pvalue",
"statsmodels/discrete/tests/test_discrete.py::TestProbitBFGS::test_bic",
"statsmodels/discrete/tests/test_discrete.py::TestNegativeBinomialNB1BFGS::test_llr",
"statsmodels/discrete/tests/test_discrete.py::TestNegativeBinomialNB1BFGS::test_aic",
"statsmodels/discrete/tests/test_discrete.py::TestNegativeBinomialNB1BFGS::test_llr_pvalue",
"statsmodels/discrete/tests/test_discrete.py::TestNegativeBinomialNB1BFGS::test_params",
"statsmodels/discrete/tests/test_discrete.py::TestNegativeBinomialNB1BFGS::test_distr",
"statsmodels/discrete/tests/test_discrete.py::TestNegativeBinomialNB1BFGS::test_jac",
"statsmodels/discrete/tests/test_discrete.py::TestNegativeBinomialNB1BFGS::test_summary_latex",
"statsmodels/discrete/tests/test_discrete.py::TestNegativeBinomialNB1BFGS::test_loglikeobs",
"statsmodels/discrete/tests/test_discrete.py::TestNegativeBinomialNB1BFGS::test_bic",
"statsmodels/discrete/tests/test_discrete.py::TestNegativeBinomialNB1BFGS::test_llnull",
"statsmodels/discrete/tests/test_discrete.py::TestNegativeBinomialNB1BFGS::test_conf_int",
"statsmodels/discrete/tests/test_discrete.py::TestNegativeBinomialNB1BFGS::test_lnalpha",
"statsmodels/discrete/tests/test_discrete.py::TestNegativeBinomialNB1BFGS::test_dof",
"statsmodels/discrete/tests/test_discrete.py::TestNegativeBinomialNB1BFGS::test_bse",
"statsmodels/discrete/tests/test_discrete.py::TestNegativeBinomialNB1BFGS::test_fit_regularized_invalid_method",
"statsmodels/discrete/tests/test_discrete.py::TestNegativeBinomialNB1BFGS::test_zstat",
"statsmodels/discrete/tests/test_discrete.py::TestNegativeBinomialNB1BFGS::test_llf",
"statsmodels/discrete/tests/test_discrete.py::TestMNLogitLBFGSBaseZero::test_bic",
"statsmodels/discrete/tests/test_discrete.py::TestMNLogitLBFGSBaseZero::test_bse",
"statsmodels/discrete/tests/test_discrete.py::TestMNLogitLBFGSBaseZero::test_zstat",
"statsmodels/discrete/tests/test_discrete.py::TestMNLogitLBFGSBaseZero::test_predict_xb",
"statsmodels/discrete/tests/test_discrete.py::TestMNLogitLBFGSBaseZero::test_predict",
"statsmodels/discrete/tests/test_discrete.py::TestMNLogitLBFGSBaseZero::test_j",
"statsmodels/discrete/tests/test_discrete.py::TestMNLogitLBFGSBaseZero::test_summary_latex",
"statsmodels/discrete/tests/test_discrete.py::TestMNLogitLBFGSBaseZero::test_pred_table",
"statsmodels/discrete/tests/test_discrete.py::TestMNLogitLBFGSBaseZero::test_endog_names",
"statsmodels/discrete/tests/test_discrete.py::TestMNLogitLBFGSBaseZero::test_llr_pvalue",
"statsmodels/discrete/tests/test_discrete.py::TestMNLogitLBFGSBaseZero::test_fit_regularized_invalid_method",
"statsmodels/discrete/tests/test_discrete.py::TestMNLogitLBFGSBaseZero::test_k",
"statsmodels/discrete/tests/test_discrete.py::TestMNLogitLBFGSBaseZero::test_margeff_overall",
"statsmodels/discrete/tests/test_discrete.py::TestMNLogitLBFGSBaseZero::test_conf_int",
"statsmodels/discrete/tests/test_discrete.py::TestMNLogitLBFGSBaseZero::test_llf",
"statsmodels/discrete/tests/test_discrete.py::TestMNLogitLBFGSBaseZero::test_params",
"statsmodels/discrete/tests/test_discrete.py::TestMNLogitLBFGSBaseZero::test_margeff_mean",
"statsmodels/discrete/tests/test_discrete.py::TestMNLogitLBFGSBaseZero::test_dof",
"statsmodels/discrete/tests/test_discrete.py::TestMNLogitLBFGSBaseZero::test_loglikeobs",
"statsmodels/discrete/tests/test_discrete.py::TestMNLogitLBFGSBaseZero::test_llr",
"statsmodels/discrete/tests/test_discrete.py::TestMNLogitLBFGSBaseZero::test_jac",
"statsmodels/discrete/tests/test_discrete.py::TestMNLogitLBFGSBaseZero::test_aic",
"statsmodels/discrete/tests/test_discrete.py::TestMNLogitLBFGSBaseZero::test_margeff_dummy",
"statsmodels/discrete/tests/test_discrete.py::TestMNLogitLBFGSBaseZero::test_llnull",
"statsmodels/discrete/tests/test_discrete.py::TestMNLogitLBFGSBaseZero::test_pvalues",
"statsmodels/discrete/tests/test_discrete.py::TestMNLogitLBFGSBaseZero::test_resid",
"statsmodels/discrete/tests/test_discrete.py::TestGeneralizedPoisson_p1::test_hessian",
"statsmodels/discrete/tests/test_discrete.py::TestGeneralizedPoisson_p1::test_init_kwds",
"statsmodels/discrete/tests/test_discrete.py::TestGeneralizedPoisson_p1::test_t",
"statsmodels/discrete/tests/test_discrete.py::TestGeneralizedPoisson_p1::test_distr",
"statsmodels/discrete/tests/test_discrete.py::TestGeneralizedPoisson_p1::test_llf",
"statsmodels/discrete/tests/test_discrete.py::TestGeneralizedPoisson_p1::test_score",
"statsmodels/discrete/tests/test_discrete.py::TestGeneralizedPoisson_p1::test_fit_regularized",
"statsmodels/discrete/tests/test_discrete.py::TestNegativeBinomialNB1Newton::test_fit_regularized_invalid_method",
"statsmodels/discrete/tests/test_discrete.py::TestNegativeBinomialNB1Newton::test_jac",
"statsmodels/discrete/tests/test_discrete.py::TestNegativeBinomialNB1Newton::test_zstat",
"statsmodels/discrete/tests/test_discrete.py::TestNegativeBinomialNB1Newton::test_bic",
"statsmodels/discrete/tests/test_discrete.py::TestNegativeBinomialNB1Newton::test_llr",
"statsmodels/discrete/tests/test_discrete.py::TestNegativeBinomialNB1Newton::test_llf",
"statsmodels/discrete/tests/test_discrete.py::TestNegativeBinomialNB1Newton::test_bse",
"statsmodels/discrete/tests/test_discrete.py::TestNegativeBinomialNB1Newton::test_aic",
"statsmodels/discrete/tests/test_discrete.py::TestNegativeBinomialNB1Newton::test_params",
"statsmodels/discrete/tests/test_discrete.py::TestNegativeBinomialNB1Newton::test_distr",
"statsmodels/discrete/tests/test_discrete.py::TestNegativeBinomialNB1Newton::test_loglikeobs",
"statsmodels/discrete/tests/test_discrete.py::TestNegativeBinomialNB1Newton::test_dof",
"statsmodels/discrete/tests/test_discrete.py::TestNegativeBinomialNB1Newton::test_llnull",
"statsmodels/discrete/tests/test_discrete.py::TestNegativeBinomialNB1Newton::test_llr_pvalue",
"statsmodels/discrete/tests/test_discrete.py::TestNegativeBinomialNB1Newton::test_lnalpha",
"statsmodels/discrete/tests/test_discrete.py::TestNegativeBinomialNB1Newton::test_conf_int",
"statsmodels/discrete/tests/test_discrete.py::TestNegativeBinomialNB1Newton::test_summary_latex",
"statsmodels/discrete/tests/test_discrete.py::TestL1AlphaZeroProbit::test_basic_results",
"statsmodels/discrete/tests/test_discrete.py::TestL1AlphaZeroProbit::test_tests",
"statsmodels/discrete/tests/test_discrete.py::TestProbitMinimizeAdditionalOptions::test_pred_table",
"statsmodels/discrete/tests/test_discrete.py::TestProbitMinimizeAdditionalOptions::test_llr_pvalue",
"statsmodels/discrete/tests/test_discrete.py::TestProbitMinimizeAdditionalOptions::test_dof",
"statsmodels/discrete/tests/test_discrete.py::TestProbitMinimizeAdditionalOptions::test_predict",
"statsmodels/discrete/tests/test_discrete.py::TestProbitMinimizeAdditionalOptions::test_predict_xb",
"statsmodels/discrete/tests/test_discrete.py::TestProbitMinimizeAdditionalOptions::test_jac",
"statsmodels/discrete/tests/test_discrete.py::TestProbitMinimizeAdditionalOptions::test_resid_generalized",
"statsmodels/discrete/tests/test_discrete.py::TestProbitMinimizeAdditionalOptions::test_llf",
"statsmodels/discrete/tests/test_discrete.py::TestProbitMinimizeAdditionalOptions::test_loglikeobs",
"statsmodels/discrete/tests/test_discrete.py::TestProbitMinimizeAdditionalOptions::test_distr",
"statsmodels/discrete/tests/test_discrete.py::TestProbitMinimizeAdditionalOptions::test_conf_int",
"statsmodels/discrete/tests/test_discrete.py::TestProbitMinimizeAdditionalOptions::test_zstat",
"statsmodels/discrete/tests/test_discrete.py::TestProbitMinimizeAdditionalOptions::test_llr",
"statsmodels/discrete/tests/test_discrete.py::TestProbitMinimizeAdditionalOptions::test_aic",
"statsmodels/discrete/tests/test_discrete.py::TestProbitMinimizeAdditionalOptions::test_bic",
"statsmodels/discrete/tests/test_discrete.py::TestProbitMinimizeAdditionalOptions::test_params",
"statsmodels/discrete/tests/test_discrete.py::TestProbitMinimizeAdditionalOptions::test_llnull",
"statsmodels/discrete/tests/test_discrete.py::TestProbitMinimizeAdditionalOptions::test_cov_params",
"statsmodels/discrete/tests/test_discrete.py::TestProbitMinimizeAdditionalOptions::test_bse",
"statsmodels/discrete/tests/test_discrete.py::TestProbitMinimizeAdditionalOptions::test_resid_dev",
"statsmodels/discrete/tests/test_discrete.py::TestProbitMinimizeAdditionalOptions::test_summary_latex",
"statsmodels/discrete/tests/test_discrete.py::TestProbitMinimizeAdditionalOptions::test_fit_regularized_invalid_method",
"statsmodels/discrete/tests/test_discrete.py::TestProbitMinimizeAdditionalOptions::test_pvalues",
"statsmodels/discrete/tests/test_discrete.py::TestProbitMinimizeAdditionalOptions::test_resid_response",
"statsmodels/discrete/tests/test_discrete.py::TestNegativeBinomialPNB2BFGS::test_zstat",
"statsmodels/discrete/tests/test_discrete.py::TestNegativeBinomialPNB2BFGS::test_conf_int",
"statsmodels/discrete/tests/test_discrete.py::TestNegativeBinomialPNB2BFGS::test_jac",
"statsmodels/discrete/tests/test_discrete.py::TestNegativeBinomialPNB2BFGS::test_bic",
"statsmodels/discrete/tests/test_discrete.py::TestNegativeBinomialPNB2BFGS::test_aic",
"statsmodels/discrete/tests/test_discrete.py::TestNegativeBinomialPNB2BFGS::test_distr",
"statsmodels/discrete/tests/test_discrete.py::TestNegativeBinomialPNB2BFGS::test_llnull",
"statsmodels/discrete/tests/test_discrete.py::TestNegativeBinomialPNB2BFGS::test_llf",
"statsmodels/discrete/tests/test_discrete.py::TestNegativeBinomialPNB2BFGS::test_llr",
"statsmodels/discrete/tests/test_discrete.py::TestNegativeBinomialPNB2BFGS::test_bse",
"statsmodels/discrete/tests/test_discrete.py::TestNegativeBinomialPNB2BFGS::test_fit_regularized_invalid_method",
"statsmodels/discrete/tests/test_discrete.py::TestNegativeBinomialPNB2BFGS::test_loglikeobs",
"statsmodels/discrete/tests/test_discrete.py::TestNegativeBinomialPNB2BFGS::test_predict",
"statsmodels/discrete/tests/test_discrete.py::TestNegativeBinomialPNB2BFGS::test_fittedvalues",
"statsmodels/discrete/tests/test_discrete.py::TestNegativeBinomialPNB2BFGS::test_summary_latex",
"statsmodels/discrete/tests/test_discrete.py::TestNegativeBinomialPNB2BFGS::test_params",
"statsmodels/discrete/tests/test_discrete.py::TestNegativeBinomialPNB2BFGS::test_predict_xb",
"statsmodels/discrete/tests/test_discrete.py::TestNegativeBinomialPNB2BFGS::test_dof",
"statsmodels/discrete/tests/test_discrete.py::TestNegativeBinomialPNB2BFGS::test_llr_pvalue",
"statsmodels/discrete/tests/test_discrete.py::TestNegativeBinomialPNB2BFGS::test_alpha",
"statsmodels/discrete/tests/test_discrete.py::TestLogitNewtonPrepend::test_dummy_exog2",
"statsmodels/discrete/tests/test_discrete.py::TestLogitNewtonPrepend::test_dummy_dydxmean",
"statsmodels/discrete/tests/test_discrete.py::TestLogitNewtonPrepend::test_count_dummy_dydxoverall",
"statsmodels/discrete/tests/test_discrete.py::TestLogitNewtonPrepend::test_dummy_eydxmean",
"statsmodels/discrete/tests/test_discrete.py::TestLogitNewtonPrepend::test_nodummy_dyexmedian",
"statsmodels/discrete/tests/test_discrete.py::TestLogitNewtonPrepend::test_nodummy_eyexoverall",
"statsmodels/discrete/tests/test_discrete.py::TestLogitNewtonPrepend::test_dummy_dydxoverall",
"statsmodels/discrete/tests/test_discrete.py::TestLogitNewtonPrepend::test_nodummy_exog1",
"statsmodels/discrete/tests/test_discrete.py::TestLogitNewtonPrepend::test_count_dydxoverall",
"statsmodels/discrete/tests/test_discrete.py::TestLogitNewtonPrepend::test_count_dummy_dydxmean",
"statsmodels/discrete/tests/test_discrete.py::TestLogitNewtonPrepend::test_resid_pearson",
"statsmodels/discrete/tests/test_discrete.py::TestLogitNewtonPrepend::test_nodummy_eydxmedian",
"statsmodels/discrete/tests/test_discrete.py::TestLogitNewtonPrepend::test_dummy_eydxoverall",
"statsmodels/discrete/tests/test_discrete.py::TestLogitNewtonPrepend::test_nodummy_eyexmedian",
"statsmodels/discrete/tests/test_discrete.py::TestLogitNewtonPrepend::test_nodummy_eyexzero",
"statsmodels/discrete/tests/test_discrete.py::TestLogitNewtonPrepend::test_nodummy_dydxmedian",
"statsmodels/discrete/tests/test_discrete.py::TestLogitNewtonPrepend::test_nodummy_dyexoverall",
"statsmodels/discrete/tests/test_discrete.py::TestLogitNewtonPrepend::test_nodummy_eydxzero",
"statsmodels/discrete/tests/test_discrete.py::TestLogitNewtonPrepend::test_nodummy_exog2",
"statsmodels/discrete/tests/test_discrete.py::TestLogitNewtonPrepend::test_nodummy_eydxoverall",
"statsmodels/discrete/tests/test_discrete.py::TestLogitNewtonPrepend::test_nodummy_dyexmean",
"statsmodels/discrete/tests/test_discrete.py::TestLogitNewtonPrepend::test_nodummy_dydxzero",
"statsmodels/discrete/tests/test_discrete.py::TestLogitNewtonPrepend::test_nodummy_dydxmean",
"statsmodels/discrete/tests/test_discrete.py::TestLogitNewtonPrepend::test_nodummy_eydxmean",
"statsmodels/discrete/tests/test_discrete.py::TestLogitNewtonPrepend::test_dummy_exog1",
"statsmodels/discrete/tests/test_discrete.py::TestLogitNewtonPrepend::test_count_dydxmean",
"statsmodels/discrete/tests/test_discrete.py::TestLogitNewtonPrepend::test_nodummy_eyexmean",
"statsmodels/discrete/tests/test_discrete.py::TestLogitNewtonPrepend::test_nodummy_dyexzero",
"statsmodels/discrete/tests/test_discrete.py::TestLogitNewtonPrepend::test_nodummy_dydxoverall",
"statsmodels/discrete/tests/test_discrete.py::TestLogitL1Compatability::test_f_test",
"statsmodels/discrete/tests/test_discrete.py::TestLogitL1Compatability::test_df",
"statsmodels/discrete/tests/test_discrete.py::TestLogitL1Compatability::test_params",
"statsmodels/discrete/tests/test_discrete.py::TestLogitL1Compatability::test_bad_r_matrix",
"statsmodels/discrete/tests/test_discrete.py::TestLogitL1Compatability::test_t_test",
"statsmodels/discrete/tests/test_discrete.py::TestLogitL1Compatability::test_cov_params",
"statsmodels/discrete/tests/test_discrete.py::TestGeneralizedPoisson_p2::test_jac",
"statsmodels/discrete/tests/test_discrete.py::TestGeneralizedPoisson_p2::test_llf",
"statsmodels/discrete/tests/test_discrete.py::TestGeneralizedPoisson_p2::test_wald",
"statsmodels/discrete/tests/test_discrete.py::TestGeneralizedPoisson_p2::test_bse",
"statsmodels/discrete/tests/test_discrete.py::TestGeneralizedPoisson_p2::test_params",
"statsmodels/discrete/tests/test_discrete.py::TestGeneralizedPoisson_p2::test_conf_int",
"statsmodels/discrete/tests/test_discrete.py::TestGeneralizedPoisson_p2::test_aic",
"statsmodels/discrete/tests/test_discrete.py::TestGeneralizedPoisson_p2::test_t",
"statsmodels/discrete/tests/test_discrete.py::TestGeneralizedPoisson_p2::test_df",
"statsmodels/discrete/tests/test_discrete.py::TestGeneralizedPoisson_p2::test_alpha",
"statsmodels/discrete/tests/test_discrete.py::TestGeneralizedPoisson_p2::test_bic",
"statsmodels/discrete/tests/test_discrete.py::TestGeneralizedPoisson_p2::test_distr",
"statsmodels/discrete/tests/test_discrete.py::TestProbitNM::test_resid_dev",
"statsmodels/discrete/tests/test_discrete.py::TestProbitNM::test_distr",
"statsmodels/discrete/tests/test_discrete.py::TestProbitNM::test_pvalues",
"statsmodels/discrete/tests/test_discrete.py::TestProbitNM::test_params",
"statsmodels/discrete/tests/test_discrete.py::TestProbitNM::test_resid_generalized",
"statsmodels/discrete/tests/test_discrete.py::TestProbitNM::test_llr",
"statsmodels/discrete/tests/test_discrete.py::TestProbitNM::test_resid_response",
"statsmodels/discrete/tests/test_discrete.py::TestProbitNM::test_jac",
"statsmodels/discrete/tests/test_discrete.py::TestProbitNM::test_fit_regularized_invalid_method",
"statsmodels/discrete/tests/test_discrete.py::TestProbitNM::test_cov_params",
"statsmodels/discrete/tests/test_discrete.py::TestProbitNM::test_predict",
"statsmodels/discrete/tests/test_discrete.py::TestProbitNM::test_aic",
"statsmodels/discrete/tests/test_discrete.py::TestProbitNM::test_llr_pvalue",
"statsmodels/discrete/tests/test_discrete.py::TestProbitNM::test_conf_int",
"statsmodels/discrete/tests/test_discrete.py::TestProbitNM::test_dof",
"statsmodels/discrete/tests/test_discrete.py::TestProbitNM::test_zstat",
"statsmodels/discrete/tests/test_discrete.py::TestProbitNM::test_pred_table",
"statsmodels/discrete/tests/test_discrete.py::TestProbitNM::test_bic",
"statsmodels/discrete/tests/test_discrete.py::TestProbitNM::test_llf",
"statsmodels/discrete/tests/test_discrete.py::TestProbitNM::test_llnull",
"statsmodels/discrete/tests/test_discrete.py::TestProbitNM::test_bse",
"statsmodels/discrete/tests/test_discrete.py::TestProbitNM::test_summary_latex",
"statsmodels/discrete/tests/test_discrete.py::TestProbitNM::test_predict_xb",
"statsmodels/discrete/tests/test_discrete.py::TestProbitNM::test_loglikeobs",
"statsmodels/discrete/tests/test_discrete.py::TestLogitL1::test_nnz_params",
"statsmodels/discrete/tests/test_discrete.py::TestLogitL1::test_bic",
"statsmodels/discrete/tests/test_discrete.py::TestLogitL1::test_conf_int",
"statsmodels/discrete/tests/test_discrete.py::TestLogitL1::test_cov_params",
"statsmodels/discrete/tests/test_discrete.py::TestLogitL1::test_params",
"statsmodels/discrete/tests/test_discrete.py::TestLogitL1::test_bse",
"statsmodels/discrete/tests/test_discrete.py::TestLogitL1::test_aic",
"statsmodels/discrete/tests/test_discrete.py::TestGeneralizedPoisson_transparams::test_bse",
"statsmodels/discrete/tests/test_discrete.py::TestGeneralizedPoisson_transparams::test_df",
"statsmodels/discrete/tests/test_discrete.py::TestGeneralizedPoisson_transparams::test_params",
"statsmodels/discrete/tests/test_discrete.py::TestGeneralizedPoisson_transparams::test_conf_int",
"statsmodels/discrete/tests/test_discrete.py::TestGeneralizedPoisson_transparams::test_aic",
"statsmodels/discrete/tests/test_discrete.py::TestGeneralizedPoisson_transparams::test_llf",
"statsmodels/discrete/tests/test_discrete.py::TestGeneralizedPoisson_transparams::test_alpha",
"statsmodels/discrete/tests/test_discrete.py::TestGeneralizedPoisson_transparams::test_bic",
"statsmodels/discrete/tests/test_discrete.py::TestNegativeBinomialPNB2Newton::test_zstat",
"statsmodels/discrete/tests/test_discrete.py::TestNegativeBinomialPNB2Newton::test_bic",
"statsmodels/discrete/tests/test_discrete.py::TestNegativeBinomialPNB2Newton::test_fittedvalues",
"statsmodels/discrete/tests/test_discrete.py::TestNegativeBinomialPNB2Newton::test_aic",
"statsmodels/discrete/tests/test_discrete.py::TestNegativeBinomialPNB2Newton::test_llr_pvalue",
"statsmodels/discrete/tests/test_discrete.py::TestNegativeBinomialPNB2Newton::test_dof",
"statsmodels/discrete/tests/test_discrete.py::TestNegativeBinomialPNB2Newton::test_llr",
"statsmodels/discrete/tests/test_discrete.py::TestNegativeBinomialPNB2Newton::test_summary_latex",
"statsmodels/discrete/tests/test_discrete.py::TestNegativeBinomialPNB2Newton::test_loglikeobs",
"statsmodels/discrete/tests/test_discrete.py::TestNegativeBinomialPNB2Newton::test_jac",
"statsmodels/discrete/tests/test_discrete.py::TestNegativeBinomialPNB2Newton::test_llnull",
"statsmodels/discrete/tests/test_discrete.py::TestNegativeBinomialPNB2Newton::test_llf",
"statsmodels/discrete/tests/test_discrete.py::TestNegativeBinomialPNB2Newton::test_alpha",
"statsmodels/discrete/tests/test_discrete.py::TestNegativeBinomialPNB2Newton::test_conf_int",
"statsmodels/discrete/tests/test_discrete.py::TestNegativeBinomialPNB2Newton::test_distr",
"statsmodels/discrete/tests/test_discrete.py::TestNegativeBinomialPNB2Newton::test_bse",
"statsmodels/discrete/tests/test_discrete.py::TestNegativeBinomialPNB2Newton::test_params",
"statsmodels/discrete/tests/test_discrete.py::TestNegativeBinomialPNB2Newton::test_fit_regularized_invalid_method",
"statsmodels/discrete/tests/test_discrete.py::TestNegativeBinomialPNB2Newton::test_predict",
"statsmodels/discrete/tests/test_discrete.py::TestNegativeBinomialPNB2Newton::test_predict_xb",
"statsmodels/discrete/tests/test_discrete.py::TestLogitBFGS::test_fit_regularized_invalid_method",
"statsmodels/discrete/tests/test_discrete.py::TestLogitBFGS::test_dummy_eydxmean",
"statsmodels/discrete/tests/test_discrete.py::TestLogitBFGS::test_llr_pvalue",
"statsmodels/discrete/tests/test_discrete.py::TestLogitBFGS::test_nodummy_eydxoverall",
"statsmodels/discrete/tests/test_discrete.py::TestLogitBFGS::test_conf_int",
"statsmodels/discrete/tests/test_discrete.py::TestLogitBFGS::test_loglikeobs",
"statsmodels/discrete/tests/test_discrete.py::TestLogitBFGS::test_distr",
"statsmodels/discrete/tests/test_discrete.py::TestLogitBFGS::test_bse",
"statsmodels/discrete/tests/test_discrete.py::TestLogitBFGS::test_nodummy_dyexmean",
"statsmodels/discrete/tests/test_discrete.py::TestLogitBFGS::test_zstat",
"statsmodels/discrete/tests/test_discrete.py::TestLogitBFGS::test_cov_params",
"statsmodels/discrete/tests/test_discrete.py::TestLogitBFGS::test_llf",
"statsmodels/discrete/tests/test_discrete.py::TestLogitBFGS::test_nodummy_eyexmean",
"statsmodels/discrete/tests/test_discrete.py::TestLogitBFGS::test_aic",
"statsmodels/discrete/tests/test_discrete.py::TestLogitBFGS::test_nodummy_eydxzero",
"statsmodels/discrete/tests/test_discrete.py::TestLogitBFGS::test_dof",
"statsmodels/discrete/tests/test_discrete.py::TestLogitBFGS::test_dummy_dydxmean",
"statsmodels/discrete/tests/test_discrete.py::TestLogitBFGS::test_llnull",
"statsmodels/discrete/tests/test_discrete.py::TestLogitBFGS::test_nodummy_dydxmedian",
"statsmodels/discrete/tests/test_discrete.py::TestLogitBFGS::test_pvalues",
"statsmodels/discrete/tests/test_discrete.py::TestLogitBFGS::test_nodummy_dyexoverall",
"statsmodels/discrete/tests/test_discrete.py::TestLogitBFGS::test_pred_table",
"statsmodels/discrete/tests/test_discrete.py::TestLogitBFGS::test_resid_generalized",
"statsmodels/discrete/tests/test_discrete.py::TestLogitBFGS::test_nodummy_dydxmean",
"statsmodels/discrete/tests/test_discrete.py::TestLogitBFGS::test_llr",
"statsmodels/discrete/tests/test_discrete.py::TestLogitBFGS::test_params",
"statsmodels/discrete/tests/test_discrete.py::TestLogitBFGS::test_nodummy_eydxmedian",
"statsmodels/discrete/tests/test_discrete.py::TestLogitBFGS::test_nodummy_eyexzero",
"statsmodels/discrete/tests/test_discrete.py::TestLogitBFGS::test_nodummy_eydxmean",
"statsmodels/discrete/tests/test_discrete.py::TestLogitBFGS::test_nodummy_dyexmedian",
"statsmodels/discrete/tests/test_discrete.py::TestLogitBFGS::test_dummy_dydxoverall",
"statsmodels/discrete/tests/test_discrete.py::TestLogitBFGS::test_resid_response",
"statsmodels/discrete/tests/test_discrete.py::TestLogitBFGS::test_nodummy_dydxzero",
"statsmodels/discrete/tests/test_discrete.py::TestLogitBFGS::test_nodummy_eyexoverall",
"statsmodels/discrete/tests/test_discrete.py::TestLogitBFGS::test_count_dydxoverall",
"statsmodels/discrete/tests/test_discrete.py::TestLogitBFGS::test_count_dydxmean",
"statsmodels/discrete/tests/test_discrete.py::TestLogitBFGS::test_nodummy_dyexzero",
"statsmodels/discrete/tests/test_discrete.py::TestLogitBFGS::test_predict_xb",
"statsmodels/discrete/tests/test_discrete.py::TestLogitBFGS::test_nodummy_eyexmedian",
"statsmodels/discrete/tests/test_discrete.py::TestLogitBFGS::test_predict",
"statsmodels/discrete/tests/test_discrete.py::TestLogitBFGS::test_nodummy_dydxoverall",
"statsmodels/discrete/tests/test_discrete.py::TestLogitBFGS::test_summary_latex",
"statsmodels/discrete/tests/test_discrete.py::TestLogitBFGS::test_resid_dev",
"statsmodels/discrete/tests/test_discrete.py::TestLogitBFGS::test_count_dummy_dydxmean",
"statsmodels/discrete/tests/test_discrete.py::TestLogitBFGS::test_bic",
"statsmodels/discrete/tests/test_discrete.py::TestLogitBFGS::test_jac",
"statsmodels/discrete/tests/test_discrete.py::TestLogitBFGS::test_count_dummy_dydxoverall",
"statsmodels/discrete/tests/test_discrete.py::TestLogitBFGS::test_dummy_eydxoverall",
"statsmodels/discrete/tests/test_discrete.py::TestNegativeBinomialNB1Null::test_llnull",
"statsmodels/discrete/tests/test_discrete.py::TestMNLogitL1::test_conf_int",
"statsmodels/discrete/tests/test_discrete.py::TestMNLogitL1::test_bse",
"statsmodels/discrete/tests/test_discrete.py::TestMNLogitL1::test_bic",
"statsmodels/discrete/tests/test_discrete.py::TestMNLogitL1::test_params",
"statsmodels/discrete/tests/test_discrete.py::TestMNLogitL1::test_nnz_params",
"statsmodels/discrete/tests/test_discrete.py::TestMNLogitL1::test_aic",
"statsmodels/discrete/tests/test_discrete.py::TestNegativeBinomialNBP1Null::test_start_null",
"statsmodels/discrete/tests/test_discrete.py::TestNegativeBinomialNBP1Null::test_llnull",
"statsmodels/discrete/tests/test_discrete.py::TestNegativeBinomialGeoL1Compatability::test_t_test",
"statsmodels/discrete/tests/test_discrete.py::TestNegativeBinomialGeoL1Compatability::test_df",
"statsmodels/discrete/tests/test_discrete.py::TestNegativeBinomialGeoL1Compatability::test_bad_r_matrix",
"statsmodels/discrete/tests/test_discrete.py::TestNegativeBinomialGeoL1Compatability::test_cov_params",
"statsmodels/discrete/tests/test_discrete.py::TestNegativeBinomialGeoL1Compatability::test_params",
"statsmodels/discrete/tests/test_discrete.py::TestNegativeBinomialGeoL1Compatability::test_f_test",
"statsmodels/discrete/tests/test_discrete.py::TestGeneralizedPoissonNull::test_llnull",
"statsmodels/discrete/tests/test_discrete.py::TestPoissonL1Compatability::test_params",
"statsmodels/discrete/tests/test_discrete.py::TestPoissonL1Compatability::test_t_test",
"statsmodels/discrete/tests/test_discrete.py::TestPoissonL1Compatability::test_cov_params",
"statsmodels/discrete/tests/test_discrete.py::TestPoissonL1Compatability::test_df",
"statsmodels/discrete/tests/test_discrete.py::TestPoissonL1Compatability::test_bad_r_matrix",
"statsmodels/discrete/tests/test_discrete.py::TestPoissonL1Compatability::test_f_test",
"statsmodels/discrete/tests/test_discrete.py::TestMNLogitL1Compatability::test_params",
"statsmodels/discrete/tests/test_discrete.py::TestMNLogitL1Compatability::test_bad_r_matrix",
"statsmodels/discrete/tests/test_discrete.py::TestMNLogitL1Compatability::test_df",
"statsmodels/discrete/tests/test_discrete.py::TestMNLogitL1Compatability::test_t_test",
"statsmodels/discrete/tests/test_discrete.py::TestMNLogitL1Compatability::test_cov_params",
"statsmodels/discrete/tests/test_discrete.py::TestLogitNewton::test_nodummy_dyexoverall",
"statsmodels/discrete/tests/test_discrete.py::TestLogitNewton::test_distr",
"statsmodels/discrete/tests/test_discrete.py::TestLogitNewton::test_nodummy_dydxmedian",
"statsmodels/discrete/tests/test_discrete.py::TestLogitNewton::test_nodummy_eyexmean",
"statsmodels/discrete/tests/test_discrete.py::TestLogitNewton::test_llr",
"statsmodels/discrete/tests/test_discrete.py::TestLogitNewton::test_cov_params",
"statsmodels/discrete/tests/test_discrete.py::TestLogitNewton::test_pvalues",
"statsmodels/discrete/tests/test_discrete.py::TestLogitNewton::test_dummy_dydxmean",
"statsmodels/discrete/tests/test_discrete.py::TestLogitNewton::test_nodummy_dydxzero",
"statsmodels/discrete/tests/test_discrete.py::TestLogitNewton::test_predict",
"statsmodels/discrete/tests/test_discrete.py::TestLogitNewton::test_nodummy_dydxmean",
"statsmodels/discrete/tests/test_discrete.py::TestLogitNewton::test_loglikeobs",
"statsmodels/discrete/tests/test_discrete.py::TestLogitNewton::test_dummy_eydxmean",
"statsmodels/discrete/tests/test_discrete.py::TestLogitNewton::test_dummy_eydxoverall",
"statsmodels/discrete/tests/test_discrete.py::TestLogitNewton::test_nodummy_eydxzero",
"statsmodels/discrete/tests/test_discrete.py::TestLogitNewton::test_llnull",
"statsmodels/discrete/tests/test_discrete.py::TestLogitNewton::test_nodummy_exog2",
"statsmodels/discrete/tests/test_discrete.py::TestLogitNewton::test_nodummy_eydxmean",
"statsmodels/discrete/tests/test_discrete.py::TestLogitNewton::test_resid_generalized",
"statsmodels/discrete/tests/test_discrete.py::TestLogitNewton::test_dummy_exog2",
"statsmodels/discrete/tests/test_discrete.py::TestLogitNewton::test_resid_pearson",
"statsmodels/discrete/tests/test_discrete.py::TestLogitNewton::test_diagnostic",
"statsmodels/discrete/tests/test_discrete.py::TestLogitNewton::test_nodummy_dyexzero",
"statsmodels/discrete/tests/test_discrete.py::TestLogitNewton::test_aic",
"statsmodels/discrete/tests/test_discrete.py::TestLogitNewton::test_bic",
"statsmodels/discrete/tests/test_discrete.py::TestLogitNewton::test_predict_xb",
"statsmodels/discrete/tests/test_discrete.py::TestLogitNewton::test_nodummy_dyexmedian",
"statsmodels/discrete/tests/test_discrete.py::TestLogitNewton::test_dummy_exog1",
"statsmodels/discrete/tests/test_discrete.py::TestLogitNewton::test_params",
"statsmodels/discrete/tests/test_discrete.py::TestLogitNewton::test_zstat",
"statsmodels/discrete/tests/test_discrete.py::TestLogitNewton::test_resid_response",
"statsmodels/discrete/tests/test_discrete.py::TestLogitNewton::test_nodummy_eyexzero",
"statsmodels/discrete/tests/test_discrete.py::TestLogitNewton::test_count_dydxmean",
"statsmodels/discrete/tests/test_discrete.py::TestLogitNewton::test_nodummy_dydxoverall",
"statsmodels/discrete/tests/test_discrete.py::TestLogitNewton::test_dof",
"statsmodels/discrete/tests/test_discrete.py::TestLogitNewton::test_jac",
"statsmodels/discrete/tests/test_discrete.py::TestLogitNewton::test_llr_pvalue",
"statsmodels/discrete/tests/test_discrete.py::TestLogitNewton::test_count_dummy_dydxmean",
"statsmodels/discrete/tests/test_discrete.py::TestLogitNewton::test_count_dummy_dydxoverall",
"statsmodels/discrete/tests/test_discrete.py::TestLogitNewton::test_nodummy_dyexmean",
"statsmodels/discrete/tests/test_discrete.py::TestLogitNewton::test_count_dydxoverall",
"statsmodels/discrete/tests/test_discrete.py::TestLogitNewton::test_nodummy_eydxoverall",
"statsmodels/discrete/tests/test_discrete.py::TestLogitNewton::test_fit_regularized_invalid_method",
"statsmodels/discrete/tests/test_discrete.py::TestLogitNewton::test_nodummy_eyexmedian",
"statsmodels/discrete/tests/test_discrete.py::TestLogitNewton::test_llf",
"statsmodels/discrete/tests/test_discrete.py::TestLogitNewton::test_conf_int",
"statsmodels/discrete/tests/test_discrete.py::TestLogitNewton::test_summary_latex",
"statsmodels/discrete/tests/test_discrete.py::TestLogitNewton::test_nodummy_eyexoverall",
"statsmodels/discrete/tests/test_discrete.py::TestLogitNewton::test_dummy_dydxoverall",
"statsmodels/discrete/tests/test_discrete.py::TestLogitNewton::test_resid_dev",
"statsmodels/discrete/tests/test_discrete.py::TestLogitNewton::test_nodummy_eydxmedian",
"statsmodels/discrete/tests/test_discrete.py::TestLogitNewton::test_nodummy_exog1",
"statsmodels/discrete/tests/test_discrete.py::TestLogitNewton::test_pred_table",
"statsmodels/discrete/tests/test_discrete.py::TestLogitNewton::test_bse",
"statsmodels/discrete/tests/test_discrete.py::TestNegativeBinomialGeometricBFGS::test_params",
"statsmodels/discrete/tests/test_discrete.py::TestNegativeBinomialGeometricBFGS::test_fittedvalues",
"statsmodels/discrete/tests/test_discrete.py::TestNegativeBinomialGeometricBFGS::test_loglikeobs",
"statsmodels/discrete/tests/test_discrete.py::TestNegativeBinomialGeometricBFGS::test_bse",
"statsmodels/discrete/tests/test_discrete.py::TestNegativeBinomialGeometricBFGS::test_llr_pvalue",
"statsmodels/discrete/tests/test_discrete.py::TestNegativeBinomialGeometricBFGS::test_fit_regularized_invalid_method",
"statsmodels/discrete/tests/test_discrete.py::TestNegativeBinomialGeometricBFGS::test_jac",
"statsmodels/discrete/tests/test_discrete.py::TestNegativeBinomialGeometricBFGS::test_bic",
"statsmodels/discrete/tests/test_discrete.py::TestNegativeBinomialGeometricBFGS::test_llnull",
"statsmodels/discrete/tests/test_discrete.py::TestNegativeBinomialGeometricBFGS::test_conf_int",
"statsmodels/discrete/tests/test_discrete.py::TestNegativeBinomialGeometricBFGS::test_aic",
"statsmodels/discrete/tests/test_discrete.py::TestNegativeBinomialGeometricBFGS::test_predict_xb",
"statsmodels/discrete/tests/test_discrete.py::TestNegativeBinomialGeometricBFGS::test_dof",
"statsmodels/discrete/tests/test_discrete.py::TestNegativeBinomialGeometricBFGS::test_zstat",
"statsmodels/discrete/tests/test_discrete.py::TestNegativeBinomialGeometricBFGS::test_summary_latex",
"statsmodels/discrete/tests/test_discrete.py::TestNegativeBinomialGeometricBFGS::test_llr",
"statsmodels/discrete/tests/test_discrete.py::TestNegativeBinomialGeometricBFGS::test_predict",
"statsmodels/discrete/tests/test_discrete.py::TestNegativeBinomialGeometricBFGS::test_llf",
"statsmodels/discrete/tests/test_discrete.py::TestNegativeBinomialGeometricBFGS::test_distr",
"statsmodels/discrete/tests/test_discrete.py::TestNegativeBinomialPNB1BFGS::test_aic",
"statsmodels/discrete/tests/test_discrete.py::TestNegativeBinomialPNB1BFGS::test_distr",
"statsmodels/discrete/tests/test_discrete.py::TestNegativeBinomialPNB1BFGS::test_bic",
"statsmodels/discrete/tests/test_discrete.py::TestNegativeBinomialPNB1BFGS::test_dof",
"statsmodels/discrete/tests/test_discrete.py::TestNegativeBinomialPNB1BFGS::test_zstat",
"statsmodels/discrete/tests/test_discrete.py::TestNegativeBinomialPNB1BFGS::test_llf",
"statsmodels/discrete/tests/test_discrete.py::TestNegativeBinomialPNB1BFGS::test_init_kwds",
"statsmodels/discrete/tests/test_discrete.py::TestNegativeBinomialPNB1BFGS::test_lnalpha",
"statsmodels/discrete/tests/test_discrete.py::TestNegativeBinomialPNB1BFGS::test_llr_pvalue",
"statsmodels/discrete/tests/test_discrete.py::TestNegativeBinomialPNB1BFGS::test_params",
"statsmodels/discrete/tests/test_discrete.py::TestNegativeBinomialPNB1BFGS::test_llr",
"statsmodels/discrete/tests/test_discrete.py::TestNegativeBinomialPNB1BFGS::test_summary_latex",
"statsmodels/discrete/tests/test_discrete.py::TestNegativeBinomialPNB1BFGS::test_llnull",
"statsmodels/discrete/tests/test_discrete.py::TestNegativeBinomialPNB1BFGS::test_loglikeobs",
"statsmodels/discrete/tests/test_discrete.py::TestNegativeBinomialPNB1BFGS::test_conf_int",
"statsmodels/discrete/tests/test_discrete.py::TestNegativeBinomialPNB1BFGS::test_bse",
"statsmodels/discrete/tests/test_discrete.py::TestNegativeBinomialPNB1BFGS::test_fit_regularized_invalid_method",
"statsmodels/discrete/tests/test_discrete.py::TestNegativeBinomialPNB1BFGS::test_jac",
"statsmodels/discrete/tests/test_discrete.py::TestNegativeBinomialPNB1BFGS::test_predict_xb",
"statsmodels/discrete/tests/test_discrete.py::TestNegativeBinomialPNB1BFGS::test_predict",
"statsmodels/discrete/tests/test_discrete.py::TestNegativeBinomialPPredictProb::test_predict_prob_p2",
"statsmodels/discrete/tests/test_discrete.py::TestNegativeBinomialPPredictProb::test_predict_prob_p1",
"statsmodels/discrete/tests/test_discrete.py::TestProbitNewton::test_resid_response",
"statsmodels/discrete/tests/test_discrete.py::TestProbitNewton::test_aic",
"statsmodels/discrete/tests/test_discrete.py::TestProbitNewton::test_pvalues",
"statsmodels/discrete/tests/test_discrete.py::TestProbitNewton::test_llnull",
"statsmodels/discrete/tests/test_discrete.py::TestProbitNewton::test_summary_latex",
"statsmodels/discrete/tests/test_discrete.py::TestProbitNewton::test_bic",
"statsmodels/discrete/tests/test_discrete.py::TestProbitNewton::test_conf_int",
"statsmodels/discrete/tests/test_discrete.py::TestProbitNewton::test_loglikeobs",
"statsmodels/discrete/tests/test_discrete.py::TestProbitNewton::test_fit_regularized_invalid_method",
"statsmodels/discrete/tests/test_discrete.py::TestProbitNewton::test_cov_params",
"statsmodels/discrete/tests/test_discrete.py::TestProbitNewton::test_bse",
"statsmodels/discrete/tests/test_discrete.py::TestProbitNewton::test_dof",
"statsmodels/discrete/tests/test_discrete.py::TestProbitNewton::test_resid_generalized",
"statsmodels/discrete/tests/test_discrete.py::TestProbitNewton::test_zstat",
"statsmodels/discrete/tests/test_discrete.py::TestProbitNewton::test_llf",
"statsmodels/discrete/tests/test_discrete.py::TestProbitNewton::test_resid_dev",
"statsmodels/discrete/tests/test_discrete.py::TestProbitNewton::test_predict",
"statsmodels/discrete/tests/test_discrete.py::TestProbitNewton::test_params",
"statsmodels/discrete/tests/test_discrete.py::TestProbitNewton::test_distr",
"statsmodels/discrete/tests/test_discrete.py::TestProbitNewton::test_llr",
"statsmodels/discrete/tests/test_discrete.py::TestProbitNewton::test_pred_table",
"statsmodels/discrete/tests/test_discrete.py::TestProbitNewton::test_jac",
"statsmodels/discrete/tests/test_discrete.py::TestProbitNewton::test_init_kwargs",
"statsmodels/discrete/tests/test_discrete.py::TestProbitNewton::test_llr_pvalue",
"statsmodels/discrete/tests/test_discrete.py::TestProbitNewton::test_predict_xb",
"statsmodels/discrete/tests/test_discrete.py::TestProbitMinimizeDogleg::test_bse",
"statsmodels/discrete/tests/test_discrete.py::TestProbitMinimizeDogleg::test_conf_int",
"statsmodels/discrete/tests/test_discrete.py::TestProbitMinimizeDogleg::test_llf",
"statsmodels/discrete/tests/test_discrete.py::TestProbitMinimizeDogleg::test_predict_xb",
"statsmodels/discrete/tests/test_discrete.py::TestProbitMinimizeDogleg::test_params",
"statsmodels/discrete/tests/test_discrete.py::TestProbitMinimizeDogleg::test_llnull",
"statsmodels/discrete/tests/test_discrete.py::TestProbitMinimizeDogleg::test_llr",
"statsmodels/discrete/tests/test_discrete.py::TestProbitMinimizeDogleg::test_bic",
"statsmodels/discrete/tests/test_discrete.py::TestProbitMinimizeDogleg::test_loglikeobs",
"statsmodels/discrete/tests/test_discrete.py::TestProbitMinimizeDogleg::test_fit_regularized_invalid_method",
"statsmodels/discrete/tests/test_discrete.py::TestProbitMinimizeDogleg::test_resid_dev",
"statsmodels/discrete/tests/test_discrete.py::TestProbitMinimizeDogleg::test_pred_table",
"statsmodels/discrete/tests/test_discrete.py::TestProbitMinimizeDogleg::test_predict",
"statsmodels/discrete/tests/test_discrete.py::TestProbitMinimizeDogleg::test_llr_pvalue",
"statsmodels/discrete/tests/test_discrete.py::TestProbitMinimizeDogleg::test_jac",
"statsmodels/discrete/tests/test_discrete.py::TestProbitMinimizeDogleg::test_aic",
"statsmodels/discrete/tests/test_discrete.py::TestProbitMinimizeDogleg::test_summary_latex",
"statsmodels/discrete/tests/test_discrete.py::TestProbitMinimizeDogleg::test_dof",
"statsmodels/discrete/tests/test_discrete.py::TestProbitMinimizeDogleg::test_distr",
"statsmodels/discrete/tests/test_discrete.py::TestProbitMinimizeDogleg::test_zstat",
"statsmodels/discrete/tests/test_discrete.py::TestProbitMinimizeDogleg::test_pvalues",
"statsmodels/discrete/tests/test_discrete.py::TestProbitMinimizeDogleg::test_resid_generalized",
"statsmodels/discrete/tests/test_discrete.py::TestProbitMinimizeDogleg::test_resid_response",
"statsmodels/discrete/tests/test_discrete.py::TestProbitMinimizeDogleg::test_cov_params",
"statsmodels/discrete/tests/test_discrete.py::TestL1AlphaZeroLogit::test_tests",
"statsmodels/discrete/tests/test_discrete.py::TestL1AlphaZeroLogit::test_basic_results",
"statsmodels/discrete/tests/test_discrete.py::TestL1AlphaZeroLogit::test_converged"
] | [
"statsmodels/discrete/tests/test_discrete.py::test_mlogit_t_test"
] | Python | [] | [] |
statsmodels/statsmodels | 8,959 | statsmodels__statsmodels-8959 | [
"8936"
] | 3b61c469ed8d4a6752b5bf01390789512f81f0c6 | diff --git a/statsmodels/stats/weightstats.py b/statsmodels/stats/weightstats.py
--- a/statsmodels/stats/weightstats.py
+++ b/statsmodels/stats/weightstats.py
@@ -1510,10 +1510,10 @@ def ztest(
'larger' : H1: difference in means larger than value
'smaller' : H1: difference in means smaller than value
- usevar : str, 'pooled'
- Currently, only 'pooled' is implemented.
+ usevar : str, 'pooled' or 'unequal'
If ``pooled``, then the standard deviation of the samples is assumed to be
- the same. see CompareMeans.ztest_ind for different options.
+ the same. If ``unequal``, then the standard deviation of the sample is
+ assumed to be different.
ddof : int
Degrees of freedom use in the calculation of the variance of the mean
estimate. In the case of comparing means this is one, however it can
@@ -1528,35 +1528,38 @@ def ztest(
Notes
-----
- usevar not implemented, is always pooled in two sample case
- use CompareMeans instead.
+ usevar can be pooled or unequal in two sample case
"""
# TODO: this should delegate to CompareMeans like ttest_ind
# However that does not implement ddof
- # usevar is not used, always pooled
+ # usevar can be pooled or unequal
- if usevar != "pooled":
- raise NotImplementedError('only usevar="pooled" is implemented')
+ if usevar not in {"pooled", "unequal"}:
+ raise NotImplementedError('usevar can only be "pooled" or "unequal"')
x1 = np.asarray(x1)
nobs1 = x1.shape[0]
x1_mean = x1.mean(0)
x1_var = x1.var(0)
+
if x2 is not None:
x2 = np.asarray(x2)
nobs2 = x2.shape[0]
x2_mean = x2.mean(0)
x2_var = x2.var(0)
- var_pooled = nobs1 * x1_var + nobs2 * x2_var
- var_pooled /= nobs1 + nobs2 - 2 * ddof
- var_pooled *= 1.0 / nobs1 + 1.0 / nobs2
+ if usevar == "pooled":
+ var = nobs1 * x1_var + nobs2 * x2_var
+ var /= nobs1 + nobs2 - 2 * ddof
+ var *= 1.0 / nobs1 + 1.0 / nobs2
+ elif usevar == "unequal":
+ var = x1_var / (nobs1 - ddof) + x2_var / (nobs2 - ddof)
else:
- var_pooled = x1_var / (nobs1 - ddof)
+ var = x1_var / (nobs1 - ddof)
x2_mean = 0
- std_diff = np.sqrt(var_pooled)
+ std_diff = np.sqrt(var)
# stat = x1_mean - x2_mean - value
return _zstat_generic(x1_mean, x2_mean, std_diff, alternative, diff=value)
| diff --git a/statsmodels/stats/tests/test_weightstats.py b/statsmodels/stats/tests/test_weightstats.py
--- a/statsmodels/stats/tests/test_weightstats.py
+++ b/statsmodels/stats/tests/test_weightstats.py
@@ -677,6 +677,45 @@ def test_ztest_ztost():
ztest_larger_mu_1s.method = 'One-sample z-Test'
ztest_larger_mu_1s.data_name = 'x'
+# > zt = z.test(x, sigma.x=0.46436662631627995, y, sigma.y=0.7069805008424409)
+# > cat_items(zt, "ztest_unequal.")
+ztest_unequal = Holder()
+ztest_unequal.statistic = 6.12808151466544
+ztest_unequal.p_value = 8.89450168270109e-10
+ztest_unequal.conf_int = np.array([1.19415646579981, 2.31720717056382])
+ztest_unequal.estimate = np.array([7.01818181818182, 5.2625])
+ztest_unequal.null_value = 0
+ztest_unequal.alternative = 'two.sided'
+ztest_unequal.usevar = 'unequal'
+ztest_unequal.method = 'Two-sample z-Test'
+ztest_unequal.data_name = 'x and y'
+
+# > zt = z.test(x, sigma.x=0.46436662631627995, y, sigma.y=0.7069805008424409, alternative="less")
+# > cat_items(zt, "ztest_smaller_unequal.")
+ztest_smaller_unequal = Holder()
+ztest_smaller_unequal.statistic = 6.12808151466544
+ztest_smaller_unequal.p_value = 0.999999999555275
+ztest_smaller_unequal.conf_int = np.array([np.nan, 2.22692874913371])
+ztest_smaller_unequal.estimate = np.array([7.01818181818182, 5.2625])
+ztest_smaller_unequal.null_value = 0
+ztest_smaller_unequal.alternative = 'less'
+ztest_smaller_unequal.usevar = 'unequal'
+ztest_smaller_unequal.method = 'Two-sample z-Test'
+ztest_smaller_unequal.data_name = 'x and y'
+
+# > zt = z.test(x, sigma.x=0.46436662631627995, y, sigma.y=0.7069805008424409, alternative="greater")
+# > cat_items(zt, "ztest_larger_unequal.")
+ztest_larger_unequal = Holder()
+ztest_larger_unequal.statistic = 6.12808151466544
+ztest_larger_unequal.p_value = 4.44725034576265e-10
+ztest_larger_unequal.conf_int = np.array([1.28443488722992, np.nan])
+ztest_larger_unequal.estimate = np.array([7.01818181818182, 5.2625])
+ztest_larger_unequal.null_value = 0
+ztest_larger_unequal.alternative = 'greater'
+ztest_larger_unequal.usevar = 'unequal'
+ztest_larger_unequal.method = 'Two-sample z-Test'
+ztest_larger_unequal.data_name = 'x and y'
+
alternatives = {'less': 'smaller',
'greater': 'larger',
@@ -733,6 +772,14 @@ def test(self):
alternative=alternatives[tc.alternative])
assert_allclose(ci, tc_conf_int - tc.null_value, rtol=1e-10)
+ # unequal variances
+ for tc in [ztest_unequal, ztest_smaller_unequal, ztest_larger_unequal]:
+ zstat, pval = ztest(x1, x2, value=tc.null_value,
+ alternative=alternatives[tc.alternative],
+ usevar="unequal")
+ assert_allclose(zstat, tc.statistic, rtol=1e-10)
+ assert_allclose(pval, tc.p_value, rtol=1e-10, atol=1e-16)
+
# 1 sample test copy-paste
d1 = self.d1
for tc in [ztest_mu_1s, ztest_smaller_mu_1s, ztest_larger_mu_1s]:
| two-sample ztest may have bug
#### Describe the bug
The implementation of the two sample ztest does not appear to match documented algorithms
Specifically, the calculation of `var_pooled` below deviates slightly from how it is calculated elsewhere.
https://github.com/statsmodels/statsmodels/blob/114d812133c0b8360c6d2e6059414e0706007977/statsmodels/stats/weightstats.py#L1543-L1561
For reference consult the following documentation:
https://en.wikipedia.org/wiki/Student%27s_t-test#Independent_two-sample_t-test
The code most closely resembles the "Equal or unequal sample sizes, similar variances" equation, but on line 1552 should subtract `ddof` from the nobs e.g. `var_pooled = (nobs1 - ddof) * x1_var + (nobs2 - ddof) * x2_var`
However we should not assume that the variances are similar, and should be using the more general "Equal or unequal sample sizes, unequal variances" equation.
| Hi @gorj-tessella,
It seems there is a confusion between the z-test and the t-test. Your implementation aligns more with a t-test, considering the assumption of unequal variances. However, from my understanding, a z-test always assumes equal variances. Let me know if there is something I am missing.
unequal variance would also be useful in the z-test.
I don't remember why the option was not implemented.
However, the Satterthwaite degrees of freedom correction in the t-test will not apply and is irrelevant for the z-test.
I can implement the case of unequal variance. | "2023-07-20T14:02:19Z" | 0.14 | [
"statsmodels/stats/tests/test_weightstats.py::TestSim1n::test_mean",
"statsmodels/stats/tests/test_weightstats.py::TestSim1n::test_std",
"statsmodels/stats/tests/test_weightstats.py::TestSim1n::test_sem",
"statsmodels/stats/tests/test_weightstats.py::TestSim1n::test_quantiles",
"statsmodels/stats/tests/test_weightstats.py::TestSim1n::test_sum",
"statsmodels/stats/tests/test_weightstats.py::TestSim1n::test_var",
"statsmodels/stats/tests/test_weightstats.py::TestSim1t::test_sem",
"statsmodels/stats/tests/test_weightstats.py::TestSim1t::test_var",
"statsmodels/stats/tests/test_weightstats.py::TestSim1t::test_quantiles",
"statsmodels/stats/tests/test_weightstats.py::TestSim1t::test_mean",
"statsmodels/stats/tests/test_weightstats.py::TestSim1t::test_std",
"statsmodels/stats/tests/test_weightstats.py::TestSim1t::test_sum",
"statsmodels/stats/tests/test_weightstats.py::TestWeightstats2d_ddof::test_corr",
"statsmodels/stats/tests/test_weightstats.py::TestWeightstats2d_ddof::test_confint_mean",
"statsmodels/stats/tests/test_weightstats.py::TestWeightstats2d_ddof::test_basic",
"statsmodels/stats/tests/test_weightstats.py::TestWeightstats2d_ddof::test_ttest",
"statsmodels/stats/tests/test_weightstats.py::TestWeightstats2d_ddof::test_ttest_2sample",
"statsmodels/stats/tests/test_weightstats.py::test_ztest_ztost",
"statsmodels/stats/tests/test_weightstats.py::test_ttest_ind_with_uneq_var",
"statsmodels/stats/tests/test_weightstats.py::test_weightstats_2d_w1",
"statsmodels/stats/tests/test_weightstats.py::test_weightstats_len_1",
"statsmodels/stats/tests/test_weightstats.py::test_weightstats_2d_w2",
"statsmodels/stats/tests/test_weightstats.py::TestSim2::test_mean",
"statsmodels/stats/tests/test_weightstats.py::TestSim2::test_sem",
"statsmodels/stats/tests/test_weightstats.py::TestSim2::test_var",
"statsmodels/stats/tests/test_weightstats.py::TestSim2::test_std",
"statsmodels/stats/tests/test_weightstats.py::TestSim2::test_sum",
"statsmodels/stats/tests/test_weightstats.py::TestSim2::test_quantiles",
"statsmodels/stats/tests/test_weightstats.py::TestWeightstats2d_nobs::test_confint_mean",
"statsmodels/stats/tests/test_weightstats.py::TestWeightstats2d_nobs::test_ttest",
"statsmodels/stats/tests/test_weightstats.py::TestWeightstats2d_nobs::test_basic",
"statsmodels/stats/tests/test_weightstats.py::TestWeightstats2d_nobs::test_ttest_2sample",
"statsmodels/stats/tests/test_weightstats.py::TestWeightstats2d_nobs::test_corr",
"statsmodels/stats/tests/test_weightstats.py::TestSim1::test_std",
"statsmodels/stats/tests/test_weightstats.py::TestSim1::test_mean",
"statsmodels/stats/tests/test_weightstats.py::TestSim1::test_sum",
"statsmodels/stats/tests/test_weightstats.py::TestSim1::test_var",
"statsmodels/stats/tests/test_weightstats.py::TestSim1::test_quantiles",
"statsmodels/stats/tests/test_weightstats.py::TestSim1::test_sem",
"statsmodels/stats/tests/test_weightstats.py::TestWeightstats::test_comparemeans_convenient_interface",
"statsmodels/stats/tests/test_weightstats.py::TestWeightstats::test_weightstats_ddof_tests",
"statsmodels/stats/tests/test_weightstats.py::TestWeightstats::test_weightstats_3",
"statsmodels/stats/tests/test_weightstats.py::TestWeightstats::test_comparemeans_convenient_interface_1d",
"statsmodels/stats/tests/test_weightstats.py::TestWeightstats::test_weightstats_1",
"statsmodels/stats/tests/test_weightstats.py::TestWeightstats::test_weightstats_2",
"statsmodels/stats/tests/test_weightstats.py::TestWeightstats1d_ddof::test_ttest_2sample",
"statsmodels/stats/tests/test_weightstats.py::TestWeightstats1d_ddof::test_confint_mean",
"statsmodels/stats/tests/test_weightstats.py::TestWeightstats1d_ddof::test_basic",
"statsmodels/stats/tests/test_weightstats.py::TestWeightstats1d_ddof::test_ttest",
"statsmodels/stats/tests/test_weightstats.py::TestWeightstats2d::test_basic",
"statsmodels/stats/tests/test_weightstats.py::TestWeightstats2d::test_confint_mean",
"statsmodels/stats/tests/test_weightstats.py::TestWeightstats2d::test_ttest",
"statsmodels/stats/tests/test_weightstats.py::TestWeightstats2d::test_corr",
"statsmodels/stats/tests/test_weightstats.py::TestWeightstats2d::test_ttest_2sample"
] | [
"statsmodels/stats/tests/test_weightstats.py::TestZTest::test"
] | Python | [] | [] |
statsmodels/statsmodels | 9,016 | statsmodels__statsmodels-9016 | [
"9015"
] | 45a744de6c2d17bce6539d089d0f7426bc45630b | diff --git a/statsmodels/graphics/tsaplots.py b/statsmodels/graphics/tsaplots.py
--- a/statsmodels/graphics/tsaplots.py
+++ b/statsmodels/graphics/tsaplots.py
@@ -17,7 +17,7 @@ def _prepare_data_corr_plot(x, lags, zero):
if lags is None:
# GH 4663 - use a sensible default value
nobs = x.shape[0]
- lim = min(int(np.ceil(10 * np.log10(nobs))), nobs - 1)
+ lim = min(int(np.ceil(10 * np.log10(nobs))), nobs // 2)
lags = np.arange(not zero, lim + 1)
elif np.isscalar(lags):
lags = np.arange(not zero, int(lags) + 1) # +1 for zero lag
diff --git a/statsmodels/tsa/stattools.py b/statsmodels/tsa/stattools.py
--- a/statsmodels/tsa/stattools.py
+++ b/statsmodels/tsa/stattools.py
@@ -8,7 +8,7 @@
from statsmodels.compat.python import Literal, lzip
from statsmodels.compat.scipy import _next_regular
-from typing import Tuple
+from typing import Union, List
import warnings
import numpy as np
@@ -40,6 +40,8 @@
from statsmodels.tsa.adfvalues import mackinnoncrit, mackinnonp
from statsmodels.tsa.tsatools import add_trend, lagmat, lagmat2ds
+ArrayLike1D = Union[np.ndarray, pd.Series, List[float]]
+
__all__ = [
"acovf",
"acf",
@@ -709,8 +711,11 @@ def acf(
else:
return acf, qstat, pvalue
-
-def pacf_yw(x, nlags=None, method="adjusted"):
+def pacf_yw(
+ x: ArrayLike1D,
+ nlags: int | None = None,
+ method: Literal["adjusted", "mle"] = "adjusted",
+) -> np.ndarray:
"""
Partial autocorrelation estimated with non-recursive yule_walker.
@@ -747,8 +752,7 @@ def pacf_yw(x, nlags=None, method="adjusted"):
nlags = int_like(nlags, "nlags", optional=True)
nobs = x.shape[0]
if nlags is None:
- nlags = min(int(10 * np.log10(nobs)), nobs - 1)
-
+ nlags = max(min(int(10 * np.log10(nobs)), nobs - 1), 1)
method = string_like(method, "method", options=("adjusted", "mle"))
pacf = [1.0]
with warnings.catch_warnings():
@@ -758,7 +762,9 @@ def pacf_yw(x, nlags=None, method="adjusted"):
return np.array(pacf)
-def pacf_burg(x, nlags=None, demean=True):
+def pacf_burg(
+ x: ArrayLike1D, nlags: int | None = None, demean: bool = True
+) -> tuple[np.ndarray, np.ndarray]:
"""
Calculate Burg"s partial autocorrelation estimator.
@@ -800,6 +806,7 @@ def pacf_burg(x, nlags=None, demean=True):
x = x - x.mean()
nobs = x.shape[0]
p = nlags if nlags is not None else min(int(10 * np.log10(nobs)), nobs - 1)
+ p = max(p, 1)
if p > nobs - 1:
raise ValueError("nlags must be smaller than nobs - 1")
d = np.zeros(p + 1)
@@ -818,14 +825,19 @@ def pacf_burg(x, nlags=None, demean=True):
v[1:] = last_v[1:] - pacf[i] * last_u[:-1]
d[i + 1] = (1 - pacf[i] ** 2) * d[i] - v[i] ** 2 - u[-1] ** 2
pacf[i + 1] = 2 / d[i + 1] * v[i + 1 :].dot(u[i:-1])
- sigma2 = (1 - pacf ** 2) * d / (2.0 * (nobs - np.arange(0, p + 1)))
+ sigma2 = (1 - pacf**2) * d / (2.0 * (nobs - np.arange(0, p + 1)))
pacf[0] = 1 # Insert the 0 lag partial autocorrel
return pacf, sigma2
@deprecate_kwarg("unbiased", "adjusted")
-def pacf_ols(x, nlags=None, efficient=True, adjusted=False):
+def pacf_ols(
+ x: ArrayLike1D,
+ nlags: int | None = None,
+ efficient: bool = True,
+ adjusted: bool = False,
+) -> np.ndarray:
"""
Calculate partial autocorrelations via OLS.
@@ -885,8 +897,9 @@ def pacf_ols(x, nlags=None, efficient=True, adjusted=False):
adjusted = bool_like(adjusted, "adjusted")
nobs = x.shape[0]
if nlags is None:
- nlags = min(int(10 * np.log10(nobs)), nobs - 1)
-
+ nlags = max(min(int(10 * np.log10(nobs)), nobs // 2), 1)
+ if nlags > nobs//2:
+ raise ValueError(f"nlags must be smaller than nobs // 2 ({nobs//2})")
pacf = np.empty(nlags + 1)
pacf[0] = 1.0
if efficient:
@@ -903,14 +916,30 @@ def pacf_ols(x, nlags=None, efficient=True, adjusted=False):
params = lstsq(xlags[:, :k], x0, rcond=None)[0]
# Last coefficient corresponds to PACF value (see [1])
pacf[k] = np.squeeze(params[-1])
-
if adjusted:
pacf *= nobs / (nobs - np.arange(nlags + 1))
-
return pacf
-def pacf(x, nlags=None, method="ywadjusted", alpha=None):
+def pacf(
+ x: ArrayLike1D,
+ nlags: int | None = None,
+ method: Literal[
+ "yw",
+ "ywadjusted",
+ "ols",
+ "ols-inefficient",
+ "ols-adjusted",
+ "ywm",
+ "ywmle",
+ "ld",
+ "ldadjusted",
+ "ldb",
+ "ldbiased",
+ "burg",
+ ] = "ywadjusted",
+ alpha: float | None = None,
+) -> np.ndarray | tuple[np.ndarray, np.ndarray]:
"""
Partial autocorrelation estimate.
@@ -996,7 +1025,7 @@ def pacf(x, nlags=None, method="ywadjusted", alpha=None):
"ldb",
"ldbiased",
"ld_biased",
- "burg"
+ "burg",
)
x = array_like(x, "x", maxdim=2)
method = string_like(method, "method", options=methods)
@@ -1005,13 +1034,13 @@ def pacf(x, nlags=None, method="ywadjusted", alpha=None):
nobs = x.shape[0]
if nlags is None:
nlags = min(int(10 * np.log10(nobs)), nobs // 2 - 1)
- if nlags >= x.shape[0] // 2:
+ nlags = max(nlags, 1)
+ if nlags > x.shape[0] // 2:
raise ValueError(
"Can only compute partial correlations for lags up to 50% of the "
f"sample size. The requested nlags {nlags} must be < "
f"{x.shape[0] // 2}."
)
-
if method in ("ols", "ols-inefficient", "ols-adjusted"):
efficient = "inefficient" not in method
adjusted = "adjusted" in method
@@ -1031,7 +1060,6 @@ def pacf(x, nlags=None, method="ywadjusted", alpha=None):
acv = acovf(x, adjusted=False, fft=False)
ld_ = levinson_durbin(acv, nlags=nlags, isacov=True)
ret = ld_[2]
-
if alpha is not None:
varacf = 1.0 / len(x) # for all lags >=1
interval = stats.norm.ppf(1.0 - alpha / 2.0) * np.sqrt(varacf)
@@ -1538,7 +1566,7 @@ def grangercausalitytests(x, maxlag, addconst=True, verbose=None):
if x.shape[0] <= 3 * maxlag + int(addconst):
raise ValueError(
"Insufficient observations. Maximum allowable "
- "lag is {0}".format(int((x.shape[0] - int(addconst)) / 3) - 1)
+ "lag is {}".format(int((x.shape[0] - int(addconst)) / 3) - 1)
)
resli = {}
@@ -1945,7 +1973,7 @@ def kpss(
regression: Literal["c", "ct"] = "c",
nlags: Literal["auto", "legacy"] | int = "auto",
store: bool = False,
-) -> Tuple[float, float, int, dict[str, float]]:
+) -> tuple[float, float, int, dict[str, float]]:
"""
Kwiatkowski-Phillips-Schmidt-Shin test for stationarity.
@@ -2032,7 +2060,7 @@ def kpss(
# if m is not one, n != m * n
if nobs != x.size:
- raise ValueError("x of shape {0} not understood".format(x.shape))
+ raise ValueError(f"x of shape {x.shape} not understood")
if hypo == "ct":
# p. 162 Kwiatkowski et al. (1992): y_t = beta * t + r_t + e_t,
@@ -2106,8 +2134,8 @@ def kpss(
rstore.nobs = nobs
stationary_type = "level" if hypo == "c" else "trend"
- rstore.H0 = "The series is {0} stationary".format(stationary_type)
- rstore.HA = "The series is not {0} stationary".format(stationary_type)
+ rstore.H0 = f"The series is {stationary_type} stationary"
+ rstore.HA = f"The series is not {stationary_type} stationary"
return kpss_stat, p_value, crit_dict, rstore
else:
@@ -2200,7 +2228,7 @@ def range_unit_root_test(x, store=False):
# if m is not one, n != m * n
if nobs != x.size:
- raise ValueError("x of shape {0} not understood".format(x.shape))
+ raise ValueError(f"x of shape {x.shape} not understood")
# Table from [1] has been replicated using 200,000 samples
# Critical values for new n_obs values have been identified
| diff --git a/statsmodels/graphics/tests/test_tsaplots.py b/statsmodels/graphics/tests/test_tsaplots.py
--- a/statsmodels/graphics/tests/test_tsaplots.py
+++ b/statsmodels/graphics/tests/test_tsaplots.py
@@ -375,3 +375,14 @@ def test_predict_plot(use_pandas, model_and_args, alpha):
res = model(y, **kwargs).fit()
fig = plot_predict(res, start, end, alpha=alpha)
assert isinstance(fig, plt.Figure)
+
+
[email protected]
+def test_plot_pacf_small_sample():
+ idx = [pd.Timestamp.now() + pd.Timedelta(seconds=i) for i in range(10)]
+ df = pd.DataFrame(
+ index=idx,
+ columns=["a"],
+ data=list(range(10))
+ )
+ plot_pacf(df)
diff --git a/statsmodels/tsa/tests/test_stattools.py b/statsmodels/tsa/tests/test_stattools.py
--- a/statsmodels/tsa/tests/test_stattools.py
+++ b/statsmodels/tsa/tests/test_stattools.py
@@ -1533,7 +1533,7 @@ def test_acf_conservate_nanops(reset_randomstate):
def test_pacf_nlags_error(reset_randomstate):
- e = np.random.standard_normal(100)
+ e = np.random.standard_normal(99)
with pytest.raises(ValueError, match="Can only compute partial"):
pacf(e, 50)
@@ -1584,3 +1584,27 @@ def test_granger_causality_exception_maxlag(gc_data):
def test_granger_causality_verbose(gc_data):
with pytest.warns(FutureWarning, match="verbose"):
grangercausalitytests(gc_data, 3, verbose=True)
+
[email protected]("size",[3,5,7,9])
+def test_pacf_small_sample(size,reset_randomstate):
+ y = np.random.standard_normal(size)
+ a = pacf(y)
+ assert isinstance(a, np.ndarray)
+ a, b = pacf_burg(y)
+ assert isinstance(a, np.ndarray)
+ assert isinstance(b, np.ndarray)
+ a = pacf_ols(y)
+ assert isinstance(a, np.ndarray)
+ a = pacf_yw(y)
+ assert isinstance(a, np.ndarray)
+
+
+def test_pacf_1_obs(reset_randomstate):
+ y = np.random.standard_normal(1)
+ with pytest.raises(ValueError):
+ pacf(y)
+ with pytest.raises(ValueError):
+ pacf_burg(y)
+ with pytest.raises(ValueError):
+ pacf_ols(y)
+ pacf_yw(y)
| `plot_pacf` default `lags` raises with a small sample
#### Describe the bug
When the parameter `lags` is not specified in `statsmodels.graphics.tsaplots.plot_pacf`, the value which is computed by default causes the function to raise a `ValueError` due to `nlags` being more than 50% of the sample size.
The limitation of 50% of the sample size should be applied when computing default `nlags`.
#### Code Sample, a copy-pastable example if possible
```python
import pandas as pd
from statsmodels.graphics.tsaplots import plot_pacf
df = df = pd.DataFrame(
index=[pd.Timestamp.now() + pd.Timedelta(seconds=i) for i in range(10)],
columns=["a"],
data=list(range(10))
)
plot_pacf(df)
```
```traceback
File /tmp/venv/lib/python3.11/site-packages/statsmodels/graphics/tsaplots.py:353, in plot_pacf(x, ax, lags, alpha, method, use_vlines, title, zero, vlines_kwargs, **kwargs)
351 acf_x = pacf(x, nlags=nlags, alpha=alpha, method=method)
352 else:
--> 353 acf_x, confint = pacf(x, nlags=nlags, alpha=alpha, method=method)
355 _plot_corr(
356 ax,
357 title,
(...)
364 **kwargs,
365 )
367 return fig
File /tmp/venv/lib/python3.11/site-packages/statsmodels/tsa/stattools.py:1009, in pacf(x, nlags, method, alpha)
1007 nlags = min(int(10 * np.log10(nobs)), nobs // 2 - 1)
1008 if nlags >= x.shape[0] // 2:
-> 1009 raise ValueError(
1010 "Can only compute partial correlations for lags up to 50% of the "
1011 f"sample size. The requested nlags {nlags} must be < "
1012 f"{x.shape[0] // 2}."
1013 )
1015 if method in ("ols", "ols-inefficient", "ols-adjusted"):
1016 efficient = "inefficient" not in method
ValueError: Can only compute partial correlations for lags up to 50% of the sample size. The requested nlags 9 must be < 5.
```
<details>
**Note**: As you can see, there are many issues on our GitHub tracker, so it is very possible that your issue has been posted before. Please check first before submitting so that we do not have to handle and close duplicates.
**Note**: Please be sure you are using the latest released version of `statsmodels`, or a recent build of `main`. If your problem has been fixed in an unreleased version, you might be able to use `main` until a new release occurs.
**Note**: If you are using a released version, have you verified that the bug exists in the main branch of this repository? It helps the limited resources if we know problems exist in the current main branch so that they do not need to check whether the code sample produces a bug in the next release.
</details>
If the issue has not been resolved, please file it in the issue tracker.
#### Expected Output
The limitation of 50% of the sample size should be applied when computing default `nlags`.
See for example https://github.com/datamole-ai/edvart/pull/154 for a workaround.
#### Output of ``import statsmodels.api as sm; sm.show_versions()``
<details>
INSTALLED VERSIONS
------------------
Python: 3.11.3.final.0
OS: Linux 6.5.3-1-MANJARO #1 SMP PREEMPT_DYNAMIC Wed Sep 13 12:21:35 UTC 2023 x86_64
byteorder: little
LC_ALL: None
LANG: en_US.UTF-8
statsmodels
===========
Installed: 0.14.0 /tmp/venv/lib/python3.11/site-packages/statsmodels)
Required Dependencies
=====================
cython: Not installed
numpy: 1.24.4 (/tmp/venv/lib/python3.11/site-packages/numpy)
scipy: 1.10.1 (/tmp/venv/lib/python3.11/site-packages/scipy)
pandas: 1.5.3 (/tmp/venv/lib/python3.11/site-packages/pandas)
dateutil: 2.8.2 (/tmp/venv/lib/python3.11/site-packages/dateutil)
patsy: 0.5.3 (/tmp/venv/lib/python3.11/site-packages/patsy)
Optional Dependencies
=====================
matplotlib: 3.7.3 (/tmp/venv/lib/python3.11/site-packages/matplotlib)
backend: module://matplotlib_inline.backend_inline
cvxopt: Not installed
joblib: 1.3.2 (/tmp/venv/lib/python3.11/site-packages/joblib)
Developer Tools
================
IPython: 8.12.2 (/tmp/venv/lib/python3.11/site-packages/IPython)
jinja2: 3.1.2 (/tmp/venv/lib/python3.11/site-packages/jinja2)
sphinx: 7.1.2 (/tmp/venv/lib/python3.11/site-packages/sphinx)
pygments: 2.16.1 (/tmp/venv/lib/python3.11/site-packages/pygments)
pytest: 7.1.3 (/tmp/venv/lib/python3.11/site-packages/pytest)
virtualenv: Not installed
</details>
| "2023-10-02T10:18:54Z" | 0.14 | [
"statsmodels/tsa/tests/test_stattools.py::TestADFNoConstant::test_critvalues",
"statsmodels/tsa/tests/test_stattools.py::TestADFNoConstant::test_pvalue",
"statsmodels/tsa/tests/test_stattools.py::TestADFNoConstant::test_teststat",
"statsmodels/tsa/tests/test_stattools.py::test_adfuller_resid_variance_zero[x0]",
"statsmodels/tsa/tests/test_stattools.py::test_coint",
"statsmodels/tsa/tests/test_stattools.py::test_coint_perfect_collinearity",
"statsmodels/tsa/tests/test_stattools.py::test_coint_identical_series",
"statsmodels/tsa/tests/test_stattools.py::test_acovf_nlags[False-False-True-conservative]",
"statsmodels/tsa/tests/test_stattools.py::test_acovf_nlags[False-True-False-none]",
"statsmodels/tsa/tests/test_stattools.py::test_acovf_nlags[True-False-False-raise]",
"statsmodels/tsa/tests/test_stattools.py::test_acovf_nlags[False-False-False-drop]",
"statsmodels/tsa/tests/test_stattools.py::test_acovf_fft_vs_convolution[True-True]",
"statsmodels/tsa/tests/test_stattools.py::test_acovf_nlags[True-True-False-conservative]",
"statsmodels/tsa/tests/test_stattools.py::test_acovf_nlags[False-True-True-drop]",
"statsmodels/tsa/tests/test_stattools.py::test_acovf_nlags[True-True-True-raise]",
"statsmodels/tsa/tests/test_stattools.py::test_acovf_nlags[True-True-False-drop]",
"statsmodels/tsa/tests/test_stattools.py::test_acovf_nlags[True-True-True-conservative]",
"statsmodels/tsa/tests/test_stattools.py::test_pacf2acf_ar",
"statsmodels/tsa/tests/test_stattools.py::test_acovf_nlags[True-True-False-raise]",
"statsmodels/tsa/tests/test_stattools.py::test_levinson_durbin_acov",
"statsmodels/tsa/tests/test_stattools.py::test_acovf_nlags[True-True-True-drop]",
"statsmodels/tsa/tests/test_stattools.py::test_compare_acovf_vs_ccovf[False-True-False]",
"statsmodels/tsa/tests/test_stattools.py::test_acovf_nlags_missing[False-False-True-drop]",
"statsmodels/tsa/tests/test_stattools.py::test_compare_acovf_vs_ccovf[False-True-True]",
"statsmodels/tsa/tests/test_stattools.py::test_acovf_nlags[False-False-False-none]",
"statsmodels/tsa/tests/test_stattools.py::test_acovf_nlags[False-True-True-none]",
"statsmodels/tsa/tests/test_stattools.py::test_acovf_nlags_missing[False-False-True-conservative]",
"statsmodels/tsa/tests/test_stattools.py::test_acovf_nlags_missing[False-True-True-conservative]",
"statsmodels/tsa/tests/test_stattools.py::test_compare_acovf_vs_ccovf[True-False-False]",
"statsmodels/tsa/tests/test_stattools.py::test_pacf2acf_errors",
"statsmodels/tsa/tests/test_stattools.py::test_acovf_fft_vs_convolution[False-True]",
"statsmodels/tsa/tests/test_stattools.py::test_acovf_nlags[False-False-True-none]",
"statsmodels/tsa/tests/test_stattools.py::test_acovf_nlags[True-False-True-raise]",
"statsmodels/tsa/tests/test_stattools.py::test_acovf_nlags[False-False-True-raise]",
"statsmodels/tsa/tests/test_stattools.py::test_pacf_burg_error",
"statsmodels/tsa/tests/test_stattools.py::test_adfuller_short_series",
"statsmodels/tsa/tests/test_stattools.py::test_acovf_nlags[True-False-True-none]",
"statsmodels/tsa/tests/test_stattools.py::test_acovf_nlags_missing[True-True-True-conservative]",
"statsmodels/tsa/tests/test_stattools.py::test_acovf_nlags_missing[True-False-True-drop]",
"statsmodels/tsa/tests/test_stattools.py::test_innovations_algo_filter_kalman_filter",
"statsmodels/tsa/tests/test_stattools.py::test_arma_order_select_ic_failure",
"statsmodels/tsa/tests/test_stattools.py::test_acovf_error",
"statsmodels/tsa/tests/test_stattools.py::test_pandasacovf",
"statsmodels/tsa/tests/test_stattools.py::test_acovf_fft_vs_convolution[True-False]",
"statsmodels/tsa/tests/test_stattools.py::test_acovf_nlags_missing[True-False-False-conservative]",
"statsmodels/tsa/tests/test_stattools.py::test_acovf_fft_vs_convolution[False-False]",
"statsmodels/tsa/tests/test_stattools.py::test_compare_acovf_vs_ccovf[True-True-True]",
"statsmodels/tsa/tests/test_stattools.py::test_acovf_nlags[True-False-True-drop]",
"statsmodels/tsa/tests/test_stattools.py::test_acovf_nlags[False-True-False-raise]",
"statsmodels/tsa/tests/test_stattools.py::test_innovations_algo_brockwell_davis",
"statsmodels/tsa/tests/test_stattools.py::test_innovations_errors",
"statsmodels/tsa/tests/test_stattools.py::test_acovf_nlags_missing[True-False-False-drop]",
"statsmodels/tsa/tests/test_stattools.py::test_ccovf_fft_vs_convolution[True-True]",
"statsmodels/tsa/tests/test_stattools.py::test_acovf_nlags[True-False-True-conservative]",
"statsmodels/tsa/tests/test_stattools.py::test_acovf_nlags[False-True-False-drop]",
"statsmodels/tsa/tests/test_stattools.py::test_acovf_nlags[False-True-True-raise]",
"statsmodels/tsa/tests/test_stattools.py::test_acovf_nlags[False-False-False-conservative]",
"statsmodels/tsa/tests/test_stattools.py::test_innovations_algo_rtol",
"statsmodels/tsa/tests/test_stattools.py::test_acovf_nlags_missing[True-True-True-drop]",
"statsmodels/tsa/tests/test_stattools.py::test_acf_fft_dataframe",
"statsmodels/tsa/tests/test_stattools.py::test_compare_acovf_vs_ccovf[True-True-False]",
"statsmodels/tsa/tests/test_stattools.py::test_acovf_nlags[True-True-True-none]",
"statsmodels/tsa/tests/test_stattools.py::test_arma_order_select_ic",
"statsmodels/tsa/tests/test_stattools.py::test_innovations_filter_errors",
"statsmodels/tsa/tests/test_stattools.py::test_acovf_nlags_missing[True-True-False-conservative]",
"statsmodels/tsa/tests/test_stattools.py::test_ccovf_fft_vs_convolution[False-True]",
"statsmodels/tsa/tests/test_stattools.py::test_pacf2acf_levinson_durbin",
"statsmodels/tsa/tests/test_stattools.py::test_acovf_nlags_missing[False-True-False-conservative]",
"statsmodels/tsa/tests/test_stattools.py::test_acovf_nlags[False-False-True-drop]",
"statsmodels/tsa/tests/test_stattools.py::test_acovf_nlags[True-False-False-drop]",
"statsmodels/tsa/tests/test_stattools.py::test_ccovf_fft_vs_convolution[False-False]",
"statsmodels/tsa/tests/test_stattools.py::test_acovf_nlags_missing[False-False-False-conservative]",
"statsmodels/tsa/tests/test_stattools.py::test_acovf2d",
"statsmodels/tsa/tests/test_stattools.py::test_adfuller_maxlag_too_large",
"statsmodels/tsa/tests/test_stattools.py::test_innovations_filter_brockwell_davis",
"statsmodels/tsa/tests/test_stattools.py::test_acovf_nlags_missing[False-False-False-drop]",
"statsmodels/tsa/tests/test_stattools.py::test_acovf_nlags_missing[False-True-False-drop]",
"statsmodels/tsa/tests/test_stattools.py::test_acovf_nlags[False-False-False-raise]",
"statsmodels/tsa/tests/test_stattools.py::test_acovf_nlags[False-True-True-conservative]",
"statsmodels/tsa/tests/test_stattools.py::test_acovf_nlags[False-True-False-conservative]",
"statsmodels/tsa/tests/test_stattools.py::test_acovf_nlags[True-False-False-none]",
"statsmodels/tsa/tests/test_stattools.py::test_acovf_nlags_missing[True-True-False-drop]",
"statsmodels/tsa/tests/test_stattools.py::test_pacf_burg",
"statsmodels/tsa/tests/test_stattools.py::test_acovf_nlags_missing[False-True-True-drop]",
"statsmodels/tsa/tests/test_stattools.py::test_compare_acovf_vs_ccovf[True-False-True]",
"statsmodels/tsa/tests/test_stattools.py::test_acovf_nlags_missing[True-False-True-conservative]",
"statsmodels/tsa/tests/test_stattools.py::test_innovations_filter_pandas",
"statsmodels/tsa/tests/test_stattools.py::test_compare_acovf_vs_ccovf[False-False-False]",
"statsmodels/tsa/tests/test_stattools.py::test_compare_acovf_vs_ccovf[False-False-True]",
"statsmodels/tsa/tests/test_stattools.py::test_acovf_nlags[True-False-False-conservative]",
"statsmodels/tsa/tests/test_stattools.py::test_acovf_nlags[True-True-False-none]",
"statsmodels/tsa/tests/test_stattools.py::test_ccovf_fft_vs_convolution[True-False]",
"statsmodels/tsa/tests/test_stattools.py::test_pacf_small_sample[9]",
"statsmodels/tsa/tests/test_stattools.py::test_pacf_nlags_error",
"statsmodels/tsa/tests/test_stattools.py::test_granger_causality_exceptions[dataset2]",
"statsmodels/tsa/tests/test_stattools.py::test_granger_causality_exceptions[dataset0]",
"statsmodels/tsa/tests/test_stattools.py::test_granger_causality_exceptions[dataset3]",
"statsmodels/tsa/tests/test_stattools.py::test_granger_causality_verbose",
"statsmodels/tsa/tests/test_stattools.py::test_pacf_small_sample[7]",
"statsmodels/tsa/tests/test_stattools.py::test_pacf_small_sample[3]",
"statsmodels/tsa/tests/test_stattools.py::test_pacf_small_sample[5]",
"statsmodels/tsa/tests/test_stattools.py::test_granger_causality_exception_maxlag",
"statsmodels/tsa/tests/test_stattools.py::test_granger_causality_exceptions[dataset1]",
"statsmodels/tsa/tests/test_stattools.py::test_coint_auto_tstat",
"statsmodels/tsa/tests/test_stattools.py::test_acf_conservate_nanops",
"statsmodels/tsa/tests/test_stattools.py::TestADFConstantTrend2::test_pvalue",
"statsmodels/tsa/tests/test_stattools.py::TestADFConstantTrend2::test_critvalues",
"statsmodels/tsa/tests/test_stattools.py::TestADFConstantTrend2::test_teststat",
"statsmodels/tsa/tests/test_stattools.py::TestKPSS::test_teststat",
"statsmodels/tsa/tests/test_stattools.py::TestKPSS::test_unknown_lags",
"statsmodels/tsa/tests/test_stattools.py::TestKPSS::test_kpss_autolags_does_not_assign_lags_equal_to_nobs",
"statsmodels/tsa/tests/test_stattools.py::TestKPSS::test_lags",
"statsmodels/tsa/tests/test_stattools.py::TestKPSS::test_store",
"statsmodels/tsa/tests/test_stattools.py::TestKPSS::test_pval",
"statsmodels/tsa/tests/test_stattools.py::TestKPSS::test_legacy_lags",
"statsmodels/tsa/tests/test_stattools.py::TestKPSS::test_kpss_fails_on_nobs_check",
"statsmodels/tsa/tests/test_stattools.py::TestKPSS::test_none",
"statsmodels/tsa/tests/test_stattools.py::TestKPSS::test_fail_nonvector_input",
"statsmodels/tsa/tests/test_stattools.py::TestKPSS::test_fail_unclear_hypothesis",
"statsmodels/tsa/tests/test_stattools.py::TestZivotAndrews::test_autolag_case_sensitivity[aic]",
"statsmodels/tsa/tests/test_stattools.py::TestZivotAndrews::test_stkprc_case",
"statsmodels/tsa/tests/test_stattools.py::TestZivotAndrews::test_rand10000_case",
"statsmodels/tsa/tests/test_stattools.py::TestZivotAndrews::test_fail_trim_value",
"statsmodels/tsa/tests/test_stattools.py::TestZivotAndrews::test_fail_array_shape",
"statsmodels/tsa/tests/test_stattools.py::TestZivotAndrews::test_fail_regression_type",
"statsmodels/tsa/tests/test_stattools.py::TestZivotAndrews::test_fail_autolag_type",
"statsmodels/tsa/tests/test_stattools.py::TestZivotAndrews::test_rgnpq_case",
"statsmodels/tsa/tests/test_stattools.py::TestZivotAndrews::test_autolag_case_sensitivity[AIC]",
"statsmodels/tsa/tests/test_stattools.py::TestZivotAndrews::test_autolag_case_sensitivity[Aic]",
"statsmodels/tsa/tests/test_stattools.py::TestZivotAndrews::test_rgnp_case",
"statsmodels/tsa/tests/test_stattools.py::TestZivotAndrews::test_gnpdef_case",
"statsmodels/tsa/tests/test_stattools.py::TestADFConstant2::test_critvalues",
"statsmodels/tsa/tests/test_stattools.py::TestADFConstant2::test_pvalue",
"statsmodels/tsa/tests/test_stattools.py::TestADFConstant2::test_teststat",
"statsmodels/tsa/tests/test_stattools.py::TestCCF::test_confint",
"statsmodels/tsa/tests/test_stattools.py::TestCCF::test_ccf",
"statsmodels/tsa/tests/test_stattools.py::TestGrangerCausality::test_granger_fails_on_nobs_check",
"statsmodels/tsa/tests/test_stattools.py::TestGrangerCausality::test_granger_fails_on_finite_check",
"statsmodels/tsa/tests/test_stattools.py::TestGrangerCausality::test_granger_fails_on_zero_lag",
"statsmodels/tsa/tests/test_stattools.py::TestGrangerCausality::test_grangercausality_single",
"statsmodels/tsa/tests/test_stattools.py::TestGrangerCausality::test_grangercausality",
"statsmodels/tsa/tests/test_stattools.py::TestADFNoConstant2::test_pvalue",
"statsmodels/tsa/tests/test_stattools.py::TestADFNoConstant2::test_teststat",
"statsmodels/tsa/tests/test_stattools.py::TestADFNoConstant2::test_critvalues",
"statsmodels/tsa/tests/test_stattools.py::TestADFNoConstant2::test_store_str",
"statsmodels/tsa/tests/test_stattools.py::TestPACF::test_ols",
"statsmodels/tsa/tests/test_stattools.py::TestPACF::test_ld",
"statsmodels/tsa/tests/test_stattools.py::TestPACF::test_burg",
"statsmodels/tsa/tests/test_stattools.py::TestPACF::test_yw",
"statsmodels/tsa/tests/test_stattools.py::TestPACF::test_ols_inefficient",
"statsmodels/tsa/tests/test_stattools.py::TestPACF::test_yw_singular",
"statsmodels/tsa/tests/test_stattools.py::TestACFMissing::test_acf_none",
"statsmodels/tsa/tests/test_stattools.py::TestACFMissing::test_acf_drop",
"statsmodels/tsa/tests/test_stattools.py::TestACFMissing::test_qstat_none",
"statsmodels/tsa/tests/test_stattools.py::TestACFMissing::test_raise",
"statsmodels/tsa/tests/test_stattools.py::TestACFMissing::test_acf_conservative",
"statsmodels/tsa/tests/test_stattools.py::TestADFConstant::test_teststat",
"statsmodels/tsa/tests/test_stattools.py::TestADFConstant::test_pvalue",
"statsmodels/tsa/tests/test_stattools.py::TestADFConstant::test_critvalues",
"statsmodels/tsa/tests/test_stattools.py::TestACF_FFT::test_qstat",
"statsmodels/tsa/tests/test_stattools.py::TestACF_FFT::test_acf",
"statsmodels/tsa/tests/test_stattools.py::TestACF::test_qstat",
"statsmodels/tsa/tests/test_stattools.py::TestACF::test_acf",
"statsmodels/tsa/tests/test_stattools.py::TestACF::test_confint",
"statsmodels/tsa/tests/test_stattools.py::TestCoint_t::test_tstat",
"statsmodels/tsa/tests/test_stattools.py::TestRUR::test_pval",
"statsmodels/tsa/tests/test_stattools.py::TestRUR::test_fail_nonvector_input",
"statsmodels/tsa/tests/test_stattools.py::TestRUR::test_store",
"statsmodels/tsa/tests/test_stattools.py::TestRUR::test_teststat",
"statsmodels/tsa/tests/test_stattools.py::TestBreakvarHeteroskedasticityTest::test_alternative[two-sided-41-0.047619047619047616]",
"statsmodels/tsa/tests/test_stattools.py::TestBreakvarHeteroskedasticityTest::test_alternative[decreasing-0.024390243902439025-0.9761904761904762]",
"statsmodels/tsa/tests/test_stattools.py::TestBreakvarHeteroskedasticityTest::test_use_chi2",
"statsmodels/tsa/tests/test_stattools.py::TestBreakvarHeteroskedasticityTest::test_1d_input",
"statsmodels/tsa/tests/test_stattools.py::TestBreakvarHeteroskedasticityTest::test_2d_input_with_missing_values",
"statsmodels/tsa/tests/test_stattools.py::TestBreakvarHeteroskedasticityTest::test_alternative[increasing-41-0.023809523809523808]",
"statsmodels/tsa/tests/test_stattools.py::TestBreakvarHeteroskedasticityTest::test_subset_length[2-41-0.047619047619047616]",
"statsmodels/tsa/tests/test_stattools.py::TestBreakvarHeteroskedasticityTest::test_subset_length[0.5-10-0.09048484886749095]",
"statsmodels/tsa/tests/test_stattools.py::TestADFConstantTrend::test_critvalues",
"statsmodels/tsa/tests/test_stattools.py::TestADFConstantTrend::test_teststat",
"statsmodels/tsa/tests/test_stattools.py::TestADFConstantTrend::test_pvalue",
"statsmodels/graphics/tests/test_tsaplots.py::test_plot_acf_kwargs",
"statsmodels/graphics/tests/test_tsaplots.py::test_predict_plot[None-False-model_and_args0]",
"statsmodels/graphics/tests/test_tsaplots.py::test_plot_pacf",
"statsmodels/graphics/tests/test_tsaplots.py::test_plot_pacf_kwargs",
"statsmodels/graphics/tests/test_tsaplots.py::test_predict_plot[None-True-model_and_args1]",
"statsmodels/graphics/tests/test_tsaplots.py::test_predict_plot[0.1-True-model_and_args1]",
"statsmodels/graphics/tests/test_tsaplots.py::test_predict_plot[0.1-False-model_and_args0]",
"statsmodels/graphics/tests/test_tsaplots.py::test_plot_quarter",
"statsmodels/graphics/tests/test_tsaplots.py::test_plot_acf_missing",
"statsmodels/graphics/tests/test_tsaplots.py::test_plot_accf_grid",
"statsmodels/graphics/tests/test_tsaplots.py::test_plot_ccf",
"statsmodels/graphics/tests/test_tsaplots.py::test_predict_plot[None-True-model_and_args0]",
"statsmodels/graphics/tests/test_tsaplots.py::test_seasonal_plot",
"statsmodels/graphics/tests/test_tsaplots.py::test_plot_pacf_irregular",
"statsmodels/graphics/tests/test_tsaplots.py::test_predict_plot[0.1-True-model_and_args0]",
"statsmodels/graphics/tests/test_tsaplots.py::test_plot_acf",
"statsmodels/graphics/tests/test_tsaplots.py::test_predict_plot[None-False-model_and_args1]",
"statsmodels/graphics/tests/test_tsaplots.py::test_plot_acf_irregular",
"statsmodels/graphics/tests/test_tsaplots.py::test_predict_plot[0.1-False-model_and_args1]"
] | [
"statsmodels/tsa/tests/test_stattools.py::test_pacf_1_obs",
"statsmodels/graphics/tests/test_tsaplots.py::test_plot_pacf_small_sample"
] | Python | [] | [] |
|
statsmodels/statsmodels | 9,130 | statsmodels__statsmodels-9130 | [
"9100",
"9100"
] | a0eca865c65ef9336c6403f8ff4bc29a1d3ec26b | diff --git a/statsmodels/genmod/generalized_linear_model.py b/statsmodels/genmod/generalized_linear_model.py
--- a/statsmodels/genmod/generalized_linear_model.py
+++ b/statsmodels/genmod/generalized_linear_model.py
@@ -41,6 +41,7 @@
cached_data,
cached_value,
)
+from statsmodels.tools.data import _as_array_with_name
from statsmodels.tools.docstring import Docstring
from statsmodels.tools.sm_exceptions import (
DomainWarning,
@@ -307,15 +308,21 @@ def __init__(self, endog, exog, family=None, offset=None,
f"{type(family).__name__} family."),
DomainWarning)
+ self._exposure_name = None
+ self._offset_name = None
+ self._freq_weights_name = None
+ self._var_weights_name = None
+
if exposure is not None:
- exposure = np.log(exposure)
+ exposure_array, self._exposure_name = _as_array_with_name(exposure, "exposure")
+ exposure = np.log(exposure_array)
if offset is not None: # this should probably be done upstream
- offset = np.asarray(offset)
+ offset, self._offset_name = _as_array_with_name(offset, "offset")
if freq_weights is not None:
- freq_weights = np.asarray(freq_weights)
+ freq_weights, self._freq_weights_name = _as_array_with_name(freq_weights, "freq_weights")
if var_weights is not None:
- var_weights = np.asarray(var_weights)
+ var_weights, self._var_weights_name = _as_array_with_name(var_weights, "var_weights")
self.freq_weights = freq_weights
self.var_weights = var_weights
@@ -1558,6 +1565,39 @@ def fit_constrained(self, constraints, start_params=None, **fit_kwds):
res._results.results_constrained = res_constr
return res
+ @property
+ def offset_name(self):
+ """
+ Name of the offset variable if available. If offset is not a pd.Series,
+ defaults to 'offset'.
+ """
+ return self._offset_name
+
+ @property
+ def exposure_name(self):
+ """
+ Name of the exposure variable if available. If exposure is not a pd.Series,
+ defaults to 'exposure'.
+ """
+ return self._exposure_name
+
+ @property
+ def freq_weights_name(self):
+ """
+ Name of the freq weights variable if available. If freq_weights is not a
+ pd.Series, defaults to 'freq_weights'.
+ """
+ return self._freq_weights_name
+
+ @property
+ def var_weights_name(self):
+ """
+ Name of var weights variable if available. If var_weights is not a pd.Series,
+ defaults to 'var_weights'.
+
+ """
+ return self._var_weights_name
+
get_prediction_doc = Docstring(pred.get_prediction_glm.__doc__)
get_prediction_doc.remove_parameters("pred_kwds")
diff --git a/statsmodels/tools/data.py b/statsmodels/tools/data.py
--- a/statsmodels/tools/data.py
+++ b/statsmodels/tools/data.py
@@ -19,7 +19,8 @@ def _check_period_index(x, freq="M"):
if not inferred_freq.startswith(freq):
raise ValueError("Expected frequency {}. Got {}".format(freq,
inferred_freq))
-
+def is_series(obj):
+ return isinstance(obj, pd.Series)
def is_data_frame(obj):
return isinstance(obj, pd.DataFrame)
@@ -121,3 +122,24 @@ def _is_recarray(data):
return isinstance(data, np.core.recarray)
else:
return isinstance(data, np.rec.recarray)
+
+def _as_array_with_name(obj, default_name):
+ """
+ Call np.asarray() on obj and attempt to get the name if its a Series.
+
+ Parameters
+ ----------
+ obj: pd.Series
+ Series to convert to an array
+ default_name: str
+ The default name to return in case the object isn't a pd.Series or has
+ no name attribute.
+
+ Returns
+ -------
+ array_and_name: tuple[np.ndarray, str]
+ The data casted to np.ndarra and the series name or None
+ """
+ if is_series(obj):
+ return (np.asarray(obj), obj.name)
+ return (np.asarray(obj), default_name)
| diff --git a/statsmodels/genmod/tests/test_glm.py b/statsmodels/genmod/tests/test_glm.py
--- a/statsmodels/genmod/tests/test_glm.py
+++ b/statsmodels/genmod/tests/test_glm.py
@@ -2661,3 +2661,62 @@ def test_tweedie_score():
nhess = approx_hess_cs(pa, lambda x: model.loglike(x, scale=1))
ahess = model.hessian(pa, scale=1)
assert_allclose(nhess, ahess, atol=5e-8, rtol=5e-8)
+
+def test_names():
+ """Test the name properties if using a pandas series.
+
+ They should not be the defaults if the series has a name.
+
+ Don't care about the data here, only testing the name properties.
+ """
+ y = pd.Series([0, 1], name="endog_not_default")
+ x = pd.DataFrame({"a": [1, 1], "b": [1, 0]})
+ exposure = pd.Series([0, 0], name="exposure_not_default")
+ freq_weights = pd.Series([0, 0], name="freq_weights_not_default")
+ offset = pd.Series([0, 0], name="offset_not_default")
+ var_weights = pd.Series([0, 0], name="var_weights_not_default")
+
+ model = GLM(
+ endog=y,
+ exog=x,
+ exposure=exposure,
+ freq_weights=freq_weights,
+ offset=offset,
+ var_weights=var_weights,
+ family=sm.families.Tweedie(),
+ )
+ assert model.offset_name == "offset_not_default"
+ assert model.exposure_name == "exposure_not_default"
+ assert model.freq_weights_name == "freq_weights_not_default"
+ assert model.var_weights_name == "var_weights_not_default"
+ assert model.endog_names == "endog_not_default"
+ assert model.exog_names == ["a", "b"]
+
+
+def test_names_default():
+ """Test the name properties if using a numpy arrays.
+
+ Don't care about the data here, only testing the name properties.
+ """
+ y = np.array([0, 1])
+ x = np.array([[1, 1,], [1, 0]])
+ exposure = np.array([0, 0])
+ freq_weights = np.array([0, 0])
+ offset = np.array([0, 0])
+ var_weights = np.array([0, 0])
+
+ model = GLM(
+ endog=y,
+ exog=x,
+ exposure=exposure,
+ freq_weights=freq_weights,
+ offset=offset,
+ var_weights=var_weights,
+ family=sm.families.Tweedie(),
+ )
+ assert model.offset_name == "offset"
+ assert model.exposure_name == "exposure"
+ assert model.freq_weights_name == "freq_weights"
+ assert model.var_weights_name == "var_weights"
+ assert model.endog_names == "y"
+ assert model.exog_names == ["const", "x1"]
diff --git a/statsmodels/tools/tests/test_data.py b/statsmodels/tools/tests/test_data.py
--- a/statsmodels/tools/tests/test_data.py
+++ b/statsmodels/tools/tests/test_data.py
@@ -33,3 +33,16 @@ def test_patsy_577():
np.testing.assert_(data._is_using_patsy(endog, None))
exog = dmatrix("var2 - 1", df)
np.testing.assert_(data._is_using_patsy(endog, exog))
+
+
+def test_as_array_with_name_series():
+ s = pandas.Series([1], name="hello")
+ arr, name = data._as_array_with_name(s, "not_used")
+ np.testing.assert_array_equal(np.array([1]), arr)
+ assert name == "hello"
+
+
+def test_as_array_with_name_array():
+ arr, name = data._as_array_with_name(np.array([1]), "default")
+ np.testing.assert_array_equal(np.array([1]), arr)
+ assert name == "default"
| Save the offset name in `GLM` and results wrapper
#### Is your feature request related to a problem? Please describe
Post model training, it is helpful to know which variable was used as the offset. This aids in post model analysis and deployment.
The offset array is saved and can be accessed after saving the model, but the name of the offset variable is lost when it is a pandas series. The series is [converted to a np.array](https://github.com/statsmodels/statsmodels/blob/ab10165eb897729b50e703b4ea831ae712b53585/statsmodels/genmod/generalized_linear_model.py#L315-L316) which removed the name. Current state, it is difficult to tell which variable may have been used as an offset without tracking it outside the model.
Example use case: Sharing a saved model with a peer. They inspect it to determine what variable was used as the offset in training.
The same may apply to the `var_weights` and `freq_weights` for GLM.
#### Describe the solution you'd like
The model has access on `__init__` to the name of the offset if it is a pandas series. A way to save the offset array's name if it is a series would be wonderful.
Similar to how the endog and exog names can be used in the model summary.
Here's a few ideas I had for how to implement this. Happy to hear if there's a better option.
1. Add an `offset_name` property for GLM
- Similar to the base models [`endog_names`/`exog_names`](https://github.com/statsmodels/statsmodels/blob/ab10165eb897729b50e703b4ea831ae712b53585/statsmodels/base/model.py#L235-L247)
- Simple to implement
2. Add it to the `model.data` so it's handled by [`PandasData`](https://github.com/statsmodels/statsmodels/blob/ab10165eb897729b50e703b4ea831ae712b53585/statsmodels/base/data.py#L498)
- The name could be added back to the offset when making the results wrapper (at least I think that's how it works)
- I could use some guidance on how to implement this if it is the preferred approach
- I think it has something to do with the data attrs but it's a bit hard to track down
3. Do not convert to a numpy array if it is a series
- One could use `model.offset.name` to get at the variable name
- Doesn't line up with how the rest of the code works, it expects numpy arrays
- Likely not a good option
4. User adds `offset_name` attribute to the model class before saving it.
- Seems like a bad idea, would like support in statsmodels
#### Describe alternatives you have considered
Current workaround is saving the offset name in a separate file, which is not ideal.
#### Additional context
Happy to work on a PR for this.
Save the offset name in `GLM` and results wrapper
#### Is your feature request related to a problem? Please describe
Post model training, it is helpful to know which variable was used as the offset. This aids in post model analysis and deployment.
The offset array is saved and can be accessed after saving the model, but the name of the offset variable is lost when it is a pandas series. The series is [converted to a np.array](https://github.com/statsmodels/statsmodels/blob/ab10165eb897729b50e703b4ea831ae712b53585/statsmodels/genmod/generalized_linear_model.py#L315-L316) which removed the name. Current state, it is difficult to tell which variable may have been used as an offset without tracking it outside the model.
Example use case: Sharing a saved model with a peer. They inspect it to determine what variable was used as the offset in training.
The same may apply to the `var_weights` and `freq_weights` for GLM.
#### Describe the solution you'd like
The model has access on `__init__` to the name of the offset if it is a pandas series. A way to save the offset array's name if it is a series would be wonderful.
Similar to how the endog and exog names can be used in the model summary.
Here's a few ideas I had for how to implement this. Happy to hear if there's a better option.
1. Add an `offset_name` property for GLM
- Similar to the base models [`endog_names`/`exog_names`](https://github.com/statsmodels/statsmodels/blob/ab10165eb897729b50e703b4ea831ae712b53585/statsmodels/base/model.py#L235-L247)
- Simple to implement
2. Add it to the `model.data` so it's handled by [`PandasData`](https://github.com/statsmodels/statsmodels/blob/ab10165eb897729b50e703b4ea831ae712b53585/statsmodels/base/data.py#L498)
- The name could be added back to the offset when making the results wrapper (at least I think that's how it works)
- I could use some guidance on how to implement this if it is the preferred approach
- I think it has something to do with the data attrs but it's a bit hard to track down
3. Do not convert to a numpy array if it is a series
- One could use `model.offset.name` to get at the variable name
- Doesn't line up with how the rest of the code works, it expects numpy arrays
- Likely not a good option
4. User adds `offset_name` attribute to the model class before saving it.
- Seems like a bad idea, would like support in statsmodels
#### Describe alternatives you have considered
Current workaround is saving the offset name in a separate file, which is not ideal.
#### Additional context
Happy to work on a PR for this.
| I think currently `1.` is the only option. `2.` would be good but currently the extra arrays are not going through the endog/exog `model.data` handling (at least not in most cases.
We could add a helper function that can be added to the `__init__` as replacement for np.asarray which does asarray plus return additionally the name of the variable if it is available.
This could also be applied to other extra data like exposure and the various weights.
Current extra data like offset, exposure, weights are 1dim.
For flexibility the helper function could check for and distinguish 1dim and 2dim. In the later case, return individual column names instead of the Series name.
The same as in GLM also applies to discrete models and likely to some other models.
I think currently `1.` is the only option. `2.` would be good but currently the extra arrays are not going through the endog/exog `model.data` handling (at least not in most cases.
We could add a helper function that can be added to the `__init__` as replacement for np.asarray which does asarray plus return additionally the name of the variable if it is available.
This could also be applied to other extra data like exposure and the various weights.
Current extra data like offset, exposure, weights are 1dim.
For flexibility the helper function could check for and distinguish 1dim and 2dim. In the later case, return individual column names instead of the Series name.
The same as in GLM also applies to discrete models and likely to some other models.
| "2024-01-24T22:24:28Z" | 0.14 | [
"statsmodels/tools/tests/test_data.py::test_missing_data_pandas",
"statsmodels/tools/tests/test_data.py::test_patsy_577",
"statsmodels/tools/tests/test_data.py::test_dataframe",
"statsmodels/genmod/tests/test_glm.py::TestTweedieSpecialLog0::test_resid",
"statsmodels/genmod/tests/test_glm.py::TestTweedieSpecialLog0::test_mu",
"statsmodels/genmod/tests/test_glm.py::TestWtdTweediePower15::test_scale",
"statsmodels/genmod/tests/test_glm.py::TestWtdTweediePower15::test_tpvalues",
"statsmodels/genmod/tests/test_glm.py::TestWtdTweediePower15::test_loglike",
"statsmodels/genmod/tests/test_glm.py::TestWtdTweediePower15::test_standard_errors",
"statsmodels/genmod/tests/test_glm.py::TestWtdTweediePower15::test_null_deviance",
"statsmodels/genmod/tests/test_glm.py::TestWtdTweediePower15::test_deviance",
"statsmodels/genmod/tests/test_glm.py::TestWtdTweediePower15::test_params",
"statsmodels/genmod/tests/test_glm.py::TestWtdTweediePower15::test_bic",
"statsmodels/genmod/tests/test_glm.py::TestWtdTweediePower15::test_fittedvalues",
"statsmodels/genmod/tests/test_glm.py::TestWtdTweediePower15::test_aic",
"statsmodels/genmod/tests/test_glm.py::TestGlmGamma::test_fittedvalues",
"statsmodels/genmod/tests/test_glm.py::TestGlmGamma::test_loglike",
"statsmodels/genmod/tests/test_glm.py::TestGlmGamma::test_params",
"statsmodels/genmod/tests/test_glm.py::TestGlmGamma::test_get_distribution",
"statsmodels/genmod/tests/test_glm.py::TestGlmGamma::test_residuals",
"statsmodels/genmod/tests/test_glm.py::TestGlmGamma::test_null_deviance",
"statsmodels/genmod/tests/test_glm.py::TestGlmGamma::test_scale",
"statsmodels/genmod/tests/test_glm.py::TestGlmGamma::test_summary2",
"statsmodels/genmod/tests/test_glm.py::TestGlmGamma::test_prsquared",
"statsmodels/genmod/tests/test_glm.py::TestGlmGamma::test_standard_errors",
"statsmodels/genmod/tests/test_glm.py::TestGlmGamma::test_bic",
"statsmodels/genmod/tests/test_glm.py::TestGlmGamma::test_summary",
"statsmodels/genmod/tests/test_glm.py::TestGlmGamma::test_tpvalues",
"statsmodels/genmod/tests/test_glm.py::TestGlmGamma::test_pearson_chi2",
"statsmodels/genmod/tests/test_glm.py::TestGlmGamma::test_aic_R",
"statsmodels/genmod/tests/test_glm.py::TestGlmGamma::test_aic_Stata",
"statsmodels/genmod/tests/test_glm.py::TestGlmGamma::test_deviance",
"statsmodels/genmod/tests/test_glm.py::TestGlmGamma::test_degrees",
"statsmodels/genmod/tests/test_glm.py::TestTweedieLog1::test_df",
"statsmodels/genmod/tests/test_glm.py::TestTweedieLog1::test_summary",
"statsmodels/genmod/tests/test_glm.py::TestTweedieLog1::test_bse",
"statsmodels/genmod/tests/test_glm.py::TestTweedieLog1::test_fittedvalues",
"statsmodels/genmod/tests/test_glm.py::TestTweedieLog1::test_params",
"statsmodels/genmod/tests/test_glm.py::TestTweedieLog1::test_resid",
"statsmodels/genmod/tests/test_glm.py::TestTweedieLog1::test_deviance",
"statsmodels/genmod/tests/test_glm.py::TestGlmInvgauss::test_get_distribution",
"statsmodels/genmod/tests/test_glm.py::TestGlmInvgauss::test_pearson_chi2",
"statsmodels/genmod/tests/test_glm.py::TestGlmInvgauss::test_summary",
"statsmodels/genmod/tests/test_glm.py::TestGlmInvgauss::test_loglike",
"statsmodels/genmod/tests/test_glm.py::TestGlmInvgauss::test_scale",
"statsmodels/genmod/tests/test_glm.py::TestGlmInvgauss::test_degrees",
"statsmodels/genmod/tests/test_glm.py::TestGlmInvgauss::test_null_deviance",
"statsmodels/genmod/tests/test_glm.py::TestGlmInvgauss::test_aic_R",
"statsmodels/genmod/tests/test_glm.py::TestGlmInvgauss::test_standard_errors",
"statsmodels/genmod/tests/test_glm.py::TestGlmInvgauss::test_fittedvalues",
"statsmodels/genmod/tests/test_glm.py::TestGlmInvgauss::test_tpvalues",
"statsmodels/genmod/tests/test_glm.py::TestGlmInvgauss::test_aic_Stata",
"statsmodels/genmod/tests/test_glm.py::TestGlmInvgauss::test_prsquared",
"statsmodels/genmod/tests/test_glm.py::TestGlmInvgauss::test_summary2",
"statsmodels/genmod/tests/test_glm.py::TestGlmInvgauss::test_bic",
"statsmodels/genmod/tests/test_glm.py::TestGlmInvgauss::test_deviance",
"statsmodels/genmod/tests/test_glm.py::TestGlmInvgauss::test_params",
"statsmodels/genmod/tests/test_glm.py::TestGlmInvgauss::test_residuals",
"statsmodels/genmod/tests/test_glm.py::TestGaussianLog::test_loglike",
"statsmodels/genmod/tests/test_glm.py::TestGaussianLog::test_pearson_chi2",
"statsmodels/genmod/tests/test_glm.py::TestGaussianLog::test_null_deviance",
"statsmodels/genmod/tests/test_glm.py::TestGaussianLog::test_deviance",
"statsmodels/genmod/tests/test_glm.py::TestGaussianLog::test_scale",
"statsmodels/genmod/tests/test_glm.py::TestGaussianLog::test_summary",
"statsmodels/genmod/tests/test_glm.py::TestGaussianLog::test_fittedvalues",
"statsmodels/genmod/tests/test_glm.py::TestGaussianLog::test_tpvalues",
"statsmodels/genmod/tests/test_glm.py::TestGaussianLog::test_aic_R",
"statsmodels/genmod/tests/test_glm.py::TestGaussianLog::test_degrees",
"statsmodels/genmod/tests/test_glm.py::TestGaussianLog::test_prsquared",
"statsmodels/genmod/tests/test_glm.py::TestGaussianLog::test_bic",
"statsmodels/genmod/tests/test_glm.py::TestGaussianLog::test_standard_errors",
"statsmodels/genmod/tests/test_glm.py::TestGaussianLog::test_residuals",
"statsmodels/genmod/tests/test_glm.py::TestGaussianLog::test_get_distribution",
"statsmodels/genmod/tests/test_glm.py::TestGaussianLog::test_aic_Stata",
"statsmodels/genmod/tests/test_glm.py::TestGaussianLog::test_params",
"statsmodels/genmod/tests/test_glm.py::TestGaussianLog::test_summary2",
"statsmodels/genmod/tests/test_glm.py::TestConvergence::test_convergence_atol_rtol",
"statsmodels/genmod/tests/test_glm.py::TestConvergence::test_convergence_atol_only_params",
"statsmodels/genmod/tests/test_glm.py::TestConvergence::test_convergence_atol_rtol_params",
"statsmodels/genmod/tests/test_glm.py::TestConvergence::test_convergence_rtol_only_params",
"statsmodels/genmod/tests/test_glm.py::TestConvergence::test_convergence_atol_only",
"statsmodels/genmod/tests/test_glm.py::TestConvergence::test_convergence_rtol_only",
"statsmodels/genmod/tests/test_glm.py::TestRegularized::test_regularized",
"statsmodels/genmod/tests/test_glm.py::TestStartParams::test_aic_R",
"statsmodels/genmod/tests/test_glm.py::TestStartParams::test_residuals",
"statsmodels/genmod/tests/test_glm.py::TestStartParams::test_null_deviance",
"statsmodels/genmod/tests/test_glm.py::TestStartParams::test_deviance",
"statsmodels/genmod/tests/test_glm.py::TestStartParams::test_fittedvalues",
"statsmodels/genmod/tests/test_glm.py::TestStartParams::test_tpvalues",
"statsmodels/genmod/tests/test_glm.py::TestStartParams::test_degrees",
"statsmodels/genmod/tests/test_glm.py::TestStartParams::test_scale",
"statsmodels/genmod/tests/test_glm.py::TestStartParams::test_pearson_chi2",
"statsmodels/genmod/tests/test_glm.py::TestStartParams::test_get_distribution",
"statsmodels/genmod/tests/test_glm.py::TestStartParams::test_aic_Stata",
"statsmodels/genmod/tests/test_glm.py::TestStartParams::test_summary2",
"statsmodels/genmod/tests/test_glm.py::TestStartParams::test_params",
"statsmodels/genmod/tests/test_glm.py::TestStartParams::test_prsquared",
"statsmodels/genmod/tests/test_glm.py::TestStartParams::test_loglike",
"statsmodels/genmod/tests/test_glm.py::TestStartParams::test_summary",
"statsmodels/genmod/tests/test_glm.py::TestStartParams::test_bic",
"statsmodels/genmod/tests/test_glm.py::TestStartParams::test_standard_errors",
"statsmodels/genmod/tests/test_glm.py::TestWtdGlmPoisson::test_deviance",
"statsmodels/genmod/tests/test_glm.py::TestWtdGlmPoisson::test_fittedvalues",
"statsmodels/genmod/tests/test_glm.py::TestWtdGlmPoisson::test_bic",
"statsmodels/genmod/tests/test_glm.py::TestWtdGlmPoisson::test_scale",
"statsmodels/genmod/tests/test_glm.py::TestWtdGlmPoisson::test_params",
"statsmodels/genmod/tests/test_glm.py::TestWtdGlmPoisson::test_null_deviance",
"statsmodels/genmod/tests/test_glm.py::TestWtdGlmPoisson::test_tpvalues",
"statsmodels/genmod/tests/test_glm.py::TestWtdGlmPoisson::test_aic",
"statsmodels/genmod/tests/test_glm.py::TestWtdGlmPoisson::test_standard_errors",
"statsmodels/genmod/tests/test_glm.py::TestWtdGlmPoisson::test_loglike",
"statsmodels/genmod/tests/test_glm.py::TestWtdGlmGammaScale_dev::test_missing",
"statsmodels/genmod/tests/test_glm.py::TestWtdGlmGammaScale_dev::test_bic",
"statsmodels/genmod/tests/test_glm.py::TestWtdGlmGammaScale_dev::test_scale",
"statsmodels/genmod/tests/test_glm.py::TestWtdGlmGammaScale_dev::test_fittedvalues",
"statsmodels/genmod/tests/test_glm.py::TestWtdGlmGammaScale_dev::test_deviance",
"statsmodels/genmod/tests/test_glm.py::TestWtdGlmGammaScale_dev::test_null_deviance",
"statsmodels/genmod/tests/test_glm.py::TestWtdGlmGammaScale_dev::test_params",
"statsmodels/genmod/tests/test_glm.py::TestWtdGlmGammaScale_dev::test_aic",
"statsmodels/genmod/tests/test_glm.py::TestWtdGlmGammaScale_dev::test_standard_errors",
"statsmodels/genmod/tests/test_glm.py::TestWtdGlmGammaScale_dev::test_tpvalues",
"statsmodels/genmod/tests/test_glm.py::TestWtdGlmGammaScale_dev::test_loglike",
"statsmodels/genmod/tests/test_glm.py::TestGlmGaussianGradient::test_compare_OLS",
"statsmodels/genmod/tests/test_glm.py::TestGlmGaussianGradient::test_prsquared",
"statsmodels/genmod/tests/test_glm.py::TestGlmGaussianGradient::test_aic_R",
"statsmodels/genmod/tests/test_glm.py::TestGlmGaussianGradient::test_deviance",
"statsmodels/genmod/tests/test_glm.py::TestGlmGaussianGradient::test_degrees",
"statsmodels/genmod/tests/test_glm.py::TestGlmGaussianGradient::test_pearson_chi2",
"statsmodels/genmod/tests/test_glm.py::TestGlmGaussianGradient::test_residuals",
"statsmodels/genmod/tests/test_glm.py::TestGlmGaussianGradient::test_standard_errors",
"statsmodels/genmod/tests/test_glm.py::TestGlmGaussianGradient::test_tpvalues",
"statsmodels/genmod/tests/test_glm.py::TestGlmGaussianGradient::test_get_distribution",
"statsmodels/genmod/tests/test_glm.py::TestGlmGaussianGradient::test_summary",
"statsmodels/genmod/tests/test_glm.py::TestGlmGaussianGradient::test_fittedvalues",
"statsmodels/genmod/tests/test_glm.py::TestGlmGaussianGradient::test_null_deviance",
"statsmodels/genmod/tests/test_glm.py::TestGlmGaussianGradient::test_params",
"statsmodels/genmod/tests/test_glm.py::TestGlmGaussianGradient::test_bic",
"statsmodels/genmod/tests/test_glm.py::TestGlmGaussianGradient::test_aic_Stata",
"statsmodels/genmod/tests/test_glm.py::TestGlmGaussianGradient::test_scale",
"statsmodels/genmod/tests/test_glm.py::TestGlmGaussianGradient::test_loglike",
"statsmodels/genmod/tests/test_glm.py::TestGlmGaussianGradient::test_summary2",
"statsmodels/genmod/tests/test_glm.py::TestWtdTweedieLog::test_aic",
"statsmodels/genmod/tests/test_glm.py::TestWtdTweedieLog::test_bic",
"statsmodels/genmod/tests/test_glm.py::TestWtdTweedieLog::test_deviance",
"statsmodels/genmod/tests/test_glm.py::TestWtdTweedieLog::test_params",
"statsmodels/genmod/tests/test_glm.py::TestWtdTweedieLog::test_loglike",
"statsmodels/genmod/tests/test_glm.py::TestWtdTweedieLog::test_null_deviance",
"statsmodels/genmod/tests/test_glm.py::TestWtdTweedieLog::test_scale",
"statsmodels/genmod/tests/test_glm.py::TestWtdTweedieLog::test_tpvalues",
"statsmodels/genmod/tests/test_glm.py::TestWtdTweedieLog::test_fittedvalues",
"statsmodels/genmod/tests/test_glm.py::TestWtdTweedieLog::test_standard_errors",
"statsmodels/genmod/tests/test_glm.py::TestGlmBinomial::test_prsquared",
"statsmodels/genmod/tests/test_glm.py::TestGlmBinomial::test_invalid_endog_formula",
"statsmodels/genmod/tests/test_glm.py::TestGlmBinomial::test_pearson_chi2",
"statsmodels/genmod/tests/test_glm.py::TestGlmBinomial::test_summary2",
"statsmodels/genmod/tests/test_glm.py::TestGlmBinomial::test_aic_Stata",
"statsmodels/genmod/tests/test_glm.py::TestGlmBinomial::test_fittedvalues",
"statsmodels/genmod/tests/test_glm.py::TestGlmBinomial::test_params",
"statsmodels/genmod/tests/test_glm.py::TestGlmBinomial::test_degrees",
"statsmodels/genmod/tests/test_glm.py::TestGlmBinomial::test_get_distribution_binom_count",
"statsmodels/genmod/tests/test_glm.py::TestGlmBinomial::test_summary",
"statsmodels/genmod/tests/test_glm.py::TestGlmBinomial::test_loglike",
"statsmodels/genmod/tests/test_glm.py::TestGlmBinomial::test_bic",
"statsmodels/genmod/tests/test_glm.py::TestGlmBinomial::test_endog_dtype",
"statsmodels/genmod/tests/test_glm.py::TestGlmBinomial::test_standard_errors",
"statsmodels/genmod/tests/test_glm.py::TestGlmBinomial::test_deviance",
"statsmodels/genmod/tests/test_glm.py::TestGlmBinomial::test_invalid_endog",
"statsmodels/genmod/tests/test_glm.py::TestGlmBinomial::test_null_deviance",
"statsmodels/genmod/tests/test_glm.py::TestGlmBinomial::test_scale",
"statsmodels/genmod/tests/test_glm.py::TestGlmBinomial::test_get_distribution",
"statsmodels/genmod/tests/test_glm.py::TestGlmBinomial::test_tpvalues",
"statsmodels/genmod/tests/test_glm.py::TestGlmBinomial::test_residuals",
"statsmodels/genmod/tests/test_glm.py::TestGlmBinomial::test_aic_R",
"statsmodels/genmod/tests/test_glm.py::TestTweediePower2::test_fittedvalues",
"statsmodels/genmod/tests/test_glm.py::TestTweediePower2::test_df",
"statsmodels/genmod/tests/test_glm.py::TestTweediePower2::test_deviance",
"statsmodels/genmod/tests/test_glm.py::TestTweediePower2::test_bse",
"statsmodels/genmod/tests/test_glm.py::TestTweediePower2::test_resid",
"statsmodels/genmod/tests/test_glm.py::TestTweediePower2::test_params",
"statsmodels/genmod/tests/test_glm.py::TestTweediePower2::test_summary",
"statsmodels/genmod/tests/test_glm.py::TestGaussianInverse::test_deviance",
"statsmodels/genmod/tests/test_glm.py::TestGaussianInverse::test_aic_Stata",
"statsmodels/genmod/tests/test_glm.py::TestGaussianInverse::test_prsquared",
"statsmodels/genmod/tests/test_glm.py::TestGaussianInverse::test_params",
"statsmodels/genmod/tests/test_glm.py::TestGaussianInverse::test_degrees",
"statsmodels/genmod/tests/test_glm.py::TestGaussianInverse::test_fittedvalues",
"statsmodels/genmod/tests/test_glm.py::TestGaussianInverse::test_bic",
"statsmodels/genmod/tests/test_glm.py::TestGaussianInverse::test_null_deviance",
"statsmodels/genmod/tests/test_glm.py::TestGaussianInverse::test_summary2",
"statsmodels/genmod/tests/test_glm.py::TestGaussianInverse::test_summary",
"statsmodels/genmod/tests/test_glm.py::TestGaussianInverse::test_residuals",
"statsmodels/genmod/tests/test_glm.py::TestGaussianInverse::test_tpvalues",
"statsmodels/genmod/tests/test_glm.py::TestGaussianInverse::test_standard_errors",
"statsmodels/genmod/tests/test_glm.py::TestGaussianInverse::test_pearson_chi2",
"statsmodels/genmod/tests/test_glm.py::TestGaussianInverse::test_aic_R",
"statsmodels/genmod/tests/test_glm.py::TestGaussianInverse::test_scale",
"statsmodels/genmod/tests/test_glm.py::TestGaussianInverse::test_loglike",
"statsmodels/genmod/tests/test_glm.py::TestGaussianInverse::test_get_distribution",
"statsmodels/genmod/tests/test_glm.py::TestWtdGlmPoissonClu::test_standard_errors",
"statsmodels/genmod/tests/test_glm.py::TestWtdGlmPoissonClu::test_loglike",
"statsmodels/genmod/tests/test_glm.py::TestWtdGlmPoissonClu::test_tpvalues",
"statsmodels/genmod/tests/test_glm.py::TestWtdGlmPoissonClu::test_bic",
"statsmodels/genmod/tests/test_glm.py::TestWtdGlmPoissonClu::test_scale",
"statsmodels/genmod/tests/test_glm.py::TestWtdGlmPoissonClu::test_params",
"statsmodels/genmod/tests/test_glm.py::TestWtdGlmPoissonClu::test_null_deviance",
"statsmodels/genmod/tests/test_glm.py::TestWtdGlmPoissonClu::test_aic",
"statsmodels/genmod/tests/test_glm.py::TestWtdGlmPoissonClu::test_deviance",
"statsmodels/genmod/tests/test_glm.py::TestWtdGlmPoissonClu::test_fittedvalues",
"statsmodels/genmod/tests/test_glm.py::TestWtdGlmGamma::test_tpvalues",
"statsmodels/genmod/tests/test_glm.py::TestWtdGlmGamma::test_params",
"statsmodels/genmod/tests/test_glm.py::TestWtdGlmGamma::test_standard_errors",
"statsmodels/genmod/tests/test_glm.py::TestWtdGlmGamma::test_null_deviance",
"statsmodels/genmod/tests/test_glm.py::TestWtdGlmGamma::test_deviance",
"statsmodels/genmod/tests/test_glm.py::TestWtdGlmGamma::test_loglike",
"statsmodels/genmod/tests/test_glm.py::TestWtdGlmGamma::test_fittedvalues",
"statsmodels/genmod/tests/test_glm.py::TestWtdGlmGamma::test_scale",
"statsmodels/genmod/tests/test_glm.py::TestWtdGlmGamma::test_bic",
"statsmodels/genmod/tests/test_glm.py::TestWtdGlmGamma::test_aic",
"statsmodels/genmod/tests/test_glm.py::TestGlmInvgaussLog::test_tpvalues",
"statsmodels/genmod/tests/test_glm.py::TestGlmInvgaussLog::test_summary2",
"statsmodels/genmod/tests/test_glm.py::TestGlmInvgaussLog::test_degrees",
"statsmodels/genmod/tests/test_glm.py::TestGlmInvgaussLog::test_deviance",
"statsmodels/genmod/tests/test_glm.py::TestGlmInvgaussLog::test_aic_R",
"statsmodels/genmod/tests/test_glm.py::TestGlmInvgaussLog::test_residuals",
"statsmodels/genmod/tests/test_glm.py::TestGlmInvgaussLog::test_aic_Stata",
"statsmodels/genmod/tests/test_glm.py::TestGlmInvgaussLog::test_prsquared",
"statsmodels/genmod/tests/test_glm.py::TestGlmInvgaussLog::test_standard_errors",
"statsmodels/genmod/tests/test_glm.py::TestGlmInvgaussLog::test_params",
"statsmodels/genmod/tests/test_glm.py::TestGlmInvgaussLog::test_loglike",
"statsmodels/genmod/tests/test_glm.py::TestGlmInvgaussLog::test_get_distribution",
"statsmodels/genmod/tests/test_glm.py::TestGlmInvgaussLog::test_pearson_chi2",
"statsmodels/genmod/tests/test_glm.py::TestGlmInvgaussLog::test_summary",
"statsmodels/genmod/tests/test_glm.py::TestGlmInvgaussLog::test_scale",
"statsmodels/genmod/tests/test_glm.py::TestGlmInvgaussLog::test_null_deviance",
"statsmodels/genmod/tests/test_glm.py::TestGlmInvgaussLog::test_bic",
"statsmodels/genmod/tests/test_glm.py::TestGlmInvgaussLog::test_fittedvalues",
"statsmodels/genmod/tests/test_glm.py::TestTweedieSpecialLog1::test_resid",
"statsmodels/genmod/tests/test_glm.py::TestTweedieSpecialLog1::test_mu",
"statsmodels/genmod/tests/test_glm.py::TestWtdGlmGammaNewton::test_init_kwargs",
"statsmodels/genmod/tests/test_glm.py::TestWtdGlmGammaNewton::test_bic",
"statsmodels/genmod/tests/test_glm.py::TestWtdGlmGammaNewton::test_params",
"statsmodels/genmod/tests/test_glm.py::TestWtdGlmGammaNewton::test_standard_errors",
"statsmodels/genmod/tests/test_glm.py::TestWtdGlmGammaNewton::test_tpvalues",
"statsmodels/genmod/tests/test_glm.py::TestWtdGlmGammaNewton::test_deviance",
"statsmodels/genmod/tests/test_glm.py::TestWtdGlmGammaNewton::test_fittedvalues",
"statsmodels/genmod/tests/test_glm.py::TestWtdGlmGammaNewton::test_null_deviance",
"statsmodels/genmod/tests/test_glm.py::TestWtdGlmGammaNewton::test_scale",
"statsmodels/genmod/tests/test_glm.py::TestWtdGlmGammaNewton::test_aic",
"statsmodels/genmod/tests/test_glm.py::TestWtdGlmGammaNewton::test_loglike",
"statsmodels/genmod/tests/test_glm.py::TestGlmGaussian::test_deviance",
"statsmodels/genmod/tests/test_glm.py::TestGlmGaussian::test_fittedvalues",
"statsmodels/genmod/tests/test_glm.py::TestGlmGaussian::test_pearson_chi2",
"statsmodels/genmod/tests/test_glm.py::TestGlmGaussian::test_residuals",
"statsmodels/genmod/tests/test_glm.py::TestGlmGaussian::test_loglike",
"statsmodels/genmod/tests/test_glm.py::TestGlmGaussian::test_tpvalues",
"statsmodels/genmod/tests/test_glm.py::TestGlmGaussian::test_prsquared",
"statsmodels/genmod/tests/test_glm.py::TestGlmGaussian::test_aic_R",
"statsmodels/genmod/tests/test_glm.py::TestGlmGaussian::test_summary",
"statsmodels/genmod/tests/test_glm.py::TestGlmGaussian::test_get_distribution",
"statsmodels/genmod/tests/test_glm.py::TestGlmGaussian::test_aic_Stata",
"statsmodels/genmod/tests/test_glm.py::TestGlmGaussian::test_bic",
"statsmodels/genmod/tests/test_glm.py::TestGlmGaussian::test_standard_errors",
"statsmodels/genmod/tests/test_glm.py::TestGlmGaussian::test_params",
"statsmodels/genmod/tests/test_glm.py::TestGlmGaussian::test_degrees",
"statsmodels/genmod/tests/test_glm.py::TestGlmGaussian::test_scale",
"statsmodels/genmod/tests/test_glm.py::TestGlmGaussian::test_summary2",
"statsmodels/genmod/tests/test_glm.py::TestGlmGaussian::test_compare_OLS",
"statsmodels/genmod/tests/test_glm.py::TestGlmGaussian::test_null_deviance",
"statsmodels/genmod/tests/test_glm.py::TestGlmGammaIdentity::test_aic_Stata",
"statsmodels/genmod/tests/test_glm.py::TestGlmGammaIdentity::test_degrees",
"statsmodels/genmod/tests/test_glm.py::TestGlmGammaIdentity::test_prsquared",
"statsmodels/genmod/tests/test_glm.py::TestGlmGammaIdentity::test_null_deviance",
"statsmodels/genmod/tests/test_glm.py::TestGlmGammaIdentity::test_deviance",
"statsmodels/genmod/tests/test_glm.py::TestGlmGammaIdentity::test_residuals",
"statsmodels/genmod/tests/test_glm.py::TestGlmGammaIdentity::test_pearson_chi2",
"statsmodels/genmod/tests/test_glm.py::TestGlmGammaIdentity::test_scale",
"statsmodels/genmod/tests/test_glm.py::TestGlmGammaIdentity::test_summary",
"statsmodels/genmod/tests/test_glm.py::TestGlmGammaIdentity::test_fittedvalues",
"statsmodels/genmod/tests/test_glm.py::TestGlmGammaIdentity::test_params",
"statsmodels/genmod/tests/test_glm.py::TestGlmGammaIdentity::test_loglike",
"statsmodels/genmod/tests/test_glm.py::TestGlmGammaIdentity::test_tpvalues",
"statsmodels/genmod/tests/test_glm.py::TestGlmGammaIdentity::test_bic",
"statsmodels/genmod/tests/test_glm.py::TestGlmGammaIdentity::test_standard_errors",
"statsmodels/genmod/tests/test_glm.py::TestGlmGammaIdentity::test_get_distribution",
"statsmodels/genmod/tests/test_glm.py::TestGlmGammaIdentity::test_summary2",
"statsmodels/genmod/tests/test_glm.py::TestGlmGammaIdentity::test_aic_R",
"statsmodels/genmod/tests/test_glm.py::TestGlmPoisson::test_compare_discrete",
"statsmodels/genmod/tests/test_glm.py::TestGlmPoisson::test_bic",
"statsmodels/genmod/tests/test_glm.py::TestGlmPoisson::test_aic_Stata",
"statsmodels/genmod/tests/test_glm.py::TestGlmPoisson::test_degrees",
"statsmodels/genmod/tests/test_glm.py::TestGlmPoisson::test_summary",
"statsmodels/genmod/tests/test_glm.py::TestGlmPoisson::test_aic_R",
"statsmodels/genmod/tests/test_glm.py::TestGlmPoisson::test_prsquared",
"statsmodels/genmod/tests/test_glm.py::TestGlmPoisson::test_get_prediction",
"statsmodels/genmod/tests/test_glm.py::TestGlmPoisson::test_tpvalues",
"statsmodels/genmod/tests/test_glm.py::TestGlmPoisson::test_residuals",
"statsmodels/genmod/tests/test_glm.py::TestGlmPoisson::test_standard_errors",
"statsmodels/genmod/tests/test_glm.py::TestGlmPoisson::test_deviance",
"statsmodels/genmod/tests/test_glm.py::TestGlmPoisson::test_summary2",
"statsmodels/genmod/tests/test_glm.py::TestGlmPoisson::test_params",
"statsmodels/genmod/tests/test_glm.py::TestGlmPoisson::test_loglike",
"statsmodels/genmod/tests/test_glm.py::TestGlmPoisson::test_scale",
"statsmodels/genmod/tests/test_glm.py::TestGlmPoisson::test_fittedvalues",
"statsmodels/genmod/tests/test_glm.py::TestGlmPoisson::test_score_test",
"statsmodels/genmod/tests/test_glm.py::TestGlmPoisson::test_pearson_chi2",
"statsmodels/genmod/tests/test_glm.py::TestGlmPoisson::test_get_distribution",
"statsmodels/genmod/tests/test_glm.py::TestGlmPoisson::test_null_deviance",
"statsmodels/genmod/tests/test_glm.py::TestWtdGlmGammaScale_X2::test_deviance",
"statsmodels/genmod/tests/test_glm.py::TestWtdGlmGammaScale_X2::test_params",
"statsmodels/genmod/tests/test_glm.py::TestWtdGlmGammaScale_X2::test_tpvalues",
"statsmodels/genmod/tests/test_glm.py::TestWtdGlmGammaScale_X2::test_bic",
"statsmodels/genmod/tests/test_glm.py::TestWtdGlmGammaScale_X2::test_scale",
"statsmodels/genmod/tests/test_glm.py::TestWtdGlmGammaScale_X2::test_fittedvalues",
"statsmodels/genmod/tests/test_glm.py::TestWtdGlmGammaScale_X2::test_loglike",
"statsmodels/genmod/tests/test_glm.py::TestWtdGlmGammaScale_X2::test_standard_errors",
"statsmodels/genmod/tests/test_glm.py::TestWtdGlmGammaScale_X2::test_aic",
"statsmodels/genmod/tests/test_glm.py::TestWtdGlmGammaScale_X2::test_null_deviance",
"statsmodels/genmod/tests/test_glm.py::TestWtdGlmInverseGaussian::test_fittedvalues",
"statsmodels/genmod/tests/test_glm.py::TestWtdGlmInverseGaussian::test_scale",
"statsmodels/genmod/tests/test_glm.py::TestWtdGlmInverseGaussian::test_aic",
"statsmodels/genmod/tests/test_glm.py::TestWtdGlmInverseGaussian::test_params",
"statsmodels/genmod/tests/test_glm.py::TestWtdGlmInverseGaussian::test_standard_errors",
"statsmodels/genmod/tests/test_glm.py::TestWtdGlmInverseGaussian::test_deviance",
"statsmodels/genmod/tests/test_glm.py::TestWtdGlmInverseGaussian::test_bic",
"statsmodels/genmod/tests/test_glm.py::TestWtdGlmInverseGaussian::test_loglike",
"statsmodels/genmod/tests/test_glm.py::TestWtdGlmInverseGaussian::test_tpvalues",
"statsmodels/genmod/tests/test_glm.py::TestWtdGlmInverseGaussian::test_null_deviance",
"statsmodels/genmod/tests/test_glm.py::TestTweediePower15::test_fittedvalues",
"statsmodels/genmod/tests/test_glm.py::TestTweediePower15::test_params",
"statsmodels/genmod/tests/test_glm.py::TestTweediePower15::test_summary",
"statsmodels/genmod/tests/test_glm.py::TestTweediePower15::test_df",
"statsmodels/genmod/tests/test_glm.py::TestTweediePower15::test_bse",
"statsmodels/genmod/tests/test_glm.py::TestTweediePower15::test_resid",
"statsmodels/genmod/tests/test_glm.py::TestTweediePower15::test_deviance",
"statsmodels/genmod/tests/test_glm.py::test_score_test_ols",
"statsmodels/genmod/tests/test_glm.py::test_attribute_writable_resettable",
"statsmodels/genmod/tests/test_glm.py::test_perfect_pred",
"statsmodels/genmod/tests/test_glm.py::test_glm_irls_method",
"statsmodels/genmod/tests/test_glm.py::test_summary",
"statsmodels/genmod/tests/test_glm.py::test_plots",
"statsmodels/genmod/tests/test_glm.py::test_gradient_irls",
"statsmodels/genmod/tests/test_glm.py::test_gradient_irls_eim",
"statsmodels/genmod/tests/test_glm.py::test_glm_start_params",
"statsmodels/genmod/tests/test_glm.py::test_formula_missing_exposure",
"statsmodels/genmod/tests/test_glm.py::test_loglike_no_opt",
"statsmodels/genmod/tests/test_glm.py::test_wtd_patsy_missing",
"statsmodels/genmod/tests/test_glm.py::test_tweedie_elastic_net",
"statsmodels/genmod/tests/test_glm.py::testTweediePowerEstimate",
"statsmodels/genmod/tests/test_glm.py::test_tweedie_EQL",
"statsmodels/genmod/tests/test_glm.py::test_tweedie_EQL_upper_limit",
"statsmodels/genmod/tests/test_glm.py::test_glm_lasso_6431",
"statsmodels/genmod/tests/test_glm.py::test_tweedie_EQL_poisson_limit",
"statsmodels/genmod/tests/test_glm.py::test_int_exog[int32]",
"statsmodels/genmod/tests/test_glm.py::test_int_scale",
"statsmodels/genmod/tests/test_glm.py::test_glm_bic",
"statsmodels/genmod/tests/test_glm.py::test_output_exposure_null",
"statsmodels/genmod/tests/test_glm.py::test_poisson_deviance",
"statsmodels/genmod/tests/test_glm.py::test_int_exog[int16]",
"statsmodels/genmod/tests/test_glm.py::test_non_invertible_hessian_fails_summary",
"statsmodels/genmod/tests/test_glm.py::test_int_exog[int64]",
"statsmodels/genmod/tests/test_glm.py::test_glm_bic_warning",
"statsmodels/genmod/tests/test_glm.py::test_int_exog[int8]",
"statsmodels/genmod/tests/test_glm.py::test_qaic",
"statsmodels/genmod/tests/test_glm.py::test_tweedie_score",
"statsmodels/genmod/tests/test_glm.py::TestWtdGlmGaussian::test_tpvalues",
"statsmodels/genmod/tests/test_glm.py::TestWtdGlmGaussian::test_scale",
"statsmodels/genmod/tests/test_glm.py::TestWtdGlmGaussian::test_params",
"statsmodels/genmod/tests/test_glm.py::TestWtdGlmGaussian::test_aic",
"statsmodels/genmod/tests/test_glm.py::TestWtdGlmGaussian::test_deviance",
"statsmodels/genmod/tests/test_glm.py::TestWtdGlmGaussian::test_fittedvalues",
"statsmodels/genmod/tests/test_glm.py::TestWtdGlmGaussian::test_loglike",
"statsmodels/genmod/tests/test_glm.py::TestWtdGlmGaussian::test_standard_errors",
"statsmodels/genmod/tests/test_glm.py::TestWtdGlmGaussian::test_bic",
"statsmodels/genmod/tests/test_glm.py::TestWtdGlmGaussian::test_null_deviance",
"statsmodels/genmod/tests/test_glm.py::TestTweedieLog15Fair::test_resid",
"statsmodels/genmod/tests/test_glm.py::TestTweedieLog15Fair::test_df",
"statsmodels/genmod/tests/test_glm.py::TestTweedieLog15Fair::test_params",
"statsmodels/genmod/tests/test_glm.py::TestTweedieLog15Fair::test_summary",
"statsmodels/genmod/tests/test_glm.py::TestTweedieLog15Fair::test_bse",
"statsmodels/genmod/tests/test_glm.py::TestTweedieLog15Fair::test_fittedvalues",
"statsmodels/genmod/tests/test_glm.py::TestTweedieLog15Fair::test_deviance",
"statsmodels/genmod/tests/test_glm.py::TestGlmInvgaussIdentity::test_scale",
"statsmodels/genmod/tests/test_glm.py::TestGlmInvgaussIdentity::test_summary2",
"statsmodels/genmod/tests/test_glm.py::TestGlmInvgaussIdentity::test_fittedvalues",
"statsmodels/genmod/tests/test_glm.py::TestGlmInvgaussIdentity::test_deviance",
"statsmodels/genmod/tests/test_glm.py::TestGlmInvgaussIdentity::test_aic_R",
"statsmodels/genmod/tests/test_glm.py::TestGlmInvgaussIdentity::test_pearson_chi2",
"statsmodels/genmod/tests/test_glm.py::TestGlmInvgaussIdentity::test_tpvalues",
"statsmodels/genmod/tests/test_glm.py::TestGlmInvgaussIdentity::test_aic_Stata",
"statsmodels/genmod/tests/test_glm.py::TestGlmInvgaussIdentity::test_summary",
"statsmodels/genmod/tests/test_glm.py::TestGlmInvgaussIdentity::test_get_distribution",
"statsmodels/genmod/tests/test_glm.py::TestGlmInvgaussIdentity::test_degrees",
"statsmodels/genmod/tests/test_glm.py::TestGlmInvgaussIdentity::test_residuals",
"statsmodels/genmod/tests/test_glm.py::TestGlmInvgaussIdentity::test_params",
"statsmodels/genmod/tests/test_glm.py::TestGlmInvgaussIdentity::test_bic",
"statsmodels/genmod/tests/test_glm.py::TestGlmInvgaussIdentity::test_prsquared",
"statsmodels/genmod/tests/test_glm.py::TestGlmInvgaussIdentity::test_standard_errors",
"statsmodels/genmod/tests/test_glm.py::TestGlmInvgaussIdentity::test_null_deviance",
"statsmodels/genmod/tests/test_glm.py::TestGlmInvgaussIdentity::test_loglike",
"statsmodels/genmod/tests/test_glm.py::TestWtdGlmBinomial::test_aic",
"statsmodels/genmod/tests/test_glm.py::TestWtdGlmBinomial::test_standard_errors",
"statsmodels/genmod/tests/test_glm.py::TestWtdGlmBinomial::test_scale",
"statsmodels/genmod/tests/test_glm.py::TestWtdGlmBinomial::test_bic",
"statsmodels/genmod/tests/test_glm.py::TestWtdGlmBinomial::test_params",
"statsmodels/genmod/tests/test_glm.py::TestWtdGlmBinomial::test_loglike",
"statsmodels/genmod/tests/test_glm.py::TestWtdGlmBinomial::test_deviance",
"statsmodels/genmod/tests/test_glm.py::TestWtdGlmBinomial::test_null_deviance",
"statsmodels/genmod/tests/test_glm.py::TestWtdGlmBinomial::test_fittedvalues",
"statsmodels/genmod/tests/test_glm.py::TestWtdGlmBinomial::test_tpvalues",
"statsmodels/genmod/tests/test_glm.py::TestTweedieSpecialLog3::test_mu",
"statsmodels/genmod/tests/test_glm.py::TestTweedieSpecialLog3::test_resid",
"statsmodels/genmod/tests/test_glm.py::TestWtdGlmPoissonHC0::test_aic",
"statsmodels/genmod/tests/test_glm.py::TestWtdGlmPoissonHC0::test_params",
"statsmodels/genmod/tests/test_glm.py::TestWtdGlmPoissonHC0::test_fittedvalues",
"statsmodels/genmod/tests/test_glm.py::TestWtdGlmPoissonHC0::test_standard_errors",
"statsmodels/genmod/tests/test_glm.py::TestWtdGlmPoissonHC0::test_null_deviance",
"statsmodels/genmod/tests/test_glm.py::TestWtdGlmPoissonHC0::test_tpvalues",
"statsmodels/genmod/tests/test_glm.py::TestWtdGlmPoissonHC0::test_deviance",
"statsmodels/genmod/tests/test_glm.py::TestWtdGlmPoissonHC0::test_loglike",
"statsmodels/genmod/tests/test_glm.py::TestWtdGlmPoissonHC0::test_bic",
"statsmodels/genmod/tests/test_glm.py::TestWtdGlmPoissonHC0::test_scale",
"statsmodels/genmod/tests/test_glm.py::TestGlmGammaLog::test_get_distribution",
"statsmodels/genmod/tests/test_glm.py::TestGlmGammaLog::test_aic_Stata",
"statsmodels/genmod/tests/test_glm.py::TestGlmGammaLog::test_fittedvalues",
"statsmodels/genmod/tests/test_glm.py::TestGlmGammaLog::test_degrees",
"statsmodels/genmod/tests/test_glm.py::TestGlmGammaLog::test_residuals",
"statsmodels/genmod/tests/test_glm.py::TestGlmGammaLog::test_standard_errors",
"statsmodels/genmod/tests/test_glm.py::TestGlmGammaLog::test_loglike",
"statsmodels/genmod/tests/test_glm.py::TestGlmGammaLog::test_aic_R",
"statsmodels/genmod/tests/test_glm.py::TestGlmGammaLog::test_deviance",
"statsmodels/genmod/tests/test_glm.py::TestGlmGammaLog::test_scale",
"statsmodels/genmod/tests/test_glm.py::TestGlmGammaLog::test_params",
"statsmodels/genmod/tests/test_glm.py::TestGlmGammaLog::test_null_deviance",
"statsmodels/genmod/tests/test_glm.py::TestGlmGammaLog::test_bic",
"statsmodels/genmod/tests/test_glm.py::TestGlmGammaLog::test_prsquared",
"statsmodels/genmod/tests/test_glm.py::TestGlmGammaLog::test_pearson_chi2",
"statsmodels/genmod/tests/test_glm.py::TestGlmGammaLog::test_tpvalues",
"statsmodels/genmod/tests/test_glm.py::TestGlmGammaLog::test_summary",
"statsmodels/genmod/tests/test_glm.py::TestGlmGammaLog::test_summary2",
"statsmodels/genmod/tests/test_glm.py::TestTweedieSpecialLog2::test_mu",
"statsmodels/genmod/tests/test_glm.py::TestTweedieSpecialLog2::test_resid",
"statsmodels/genmod/tests/test_glm.py::TestWtdGlmPoissonNewton::test_fittedvalues",
"statsmodels/genmod/tests/test_glm.py::TestWtdGlmPoissonNewton::test_loglike",
"statsmodels/genmod/tests/test_glm.py::TestWtdGlmPoissonNewton::test_aic",
"statsmodels/genmod/tests/test_glm.py::TestWtdGlmPoissonNewton::test_standard_errors",
"statsmodels/genmod/tests/test_glm.py::TestWtdGlmPoissonNewton::test_null_deviance",
"statsmodels/genmod/tests/test_glm.py::TestWtdGlmPoissonNewton::test_params",
"statsmodels/genmod/tests/test_glm.py::TestWtdGlmPoissonNewton::test_bic",
"statsmodels/genmod/tests/test_glm.py::TestWtdGlmPoissonNewton::test_tpvalues",
"statsmodels/genmod/tests/test_glm.py::TestWtdGlmPoissonNewton::test_deviance",
"statsmodels/genmod/tests/test_glm.py::TestWtdGlmPoissonNewton::test_scale",
"statsmodels/genmod/tests/test_glm.py::TestWtdGlmNegativeBinomial::test_standard_errors",
"statsmodels/genmod/tests/test_glm.py::TestWtdGlmNegativeBinomial::test_null_deviance",
"statsmodels/genmod/tests/test_glm.py::TestWtdGlmNegativeBinomial::test_fittedvalues",
"statsmodels/genmod/tests/test_glm.py::TestWtdGlmNegativeBinomial::test_aic",
"statsmodels/genmod/tests/test_glm.py::TestWtdGlmNegativeBinomial::test_deviance",
"statsmodels/genmod/tests/test_glm.py::TestWtdGlmNegativeBinomial::test_loglike",
"statsmodels/genmod/tests/test_glm.py::TestWtdGlmNegativeBinomial::test_scale",
"statsmodels/genmod/tests/test_glm.py::TestWtdGlmNegativeBinomial::test_bic",
"statsmodels/genmod/tests/test_glm.py::TestWtdGlmNegativeBinomial::test_params",
"statsmodels/genmod/tests/test_glm.py::TestWtdGlmNegativeBinomial::test_tpvalues",
"statsmodels/genmod/tests/test_glm.py::TestGlmNegbinomial::test_residuals",
"statsmodels/genmod/tests/test_glm.py::TestGlmNegbinomial::test_summary",
"statsmodels/genmod/tests/test_glm.py::TestGlmNegbinomial::test_loglike",
"statsmodels/genmod/tests/test_glm.py::TestGlmNegbinomial::test_scale",
"statsmodels/genmod/tests/test_glm.py::TestGlmNegbinomial::test_aic_Stata",
"statsmodels/genmod/tests/test_glm.py::TestGlmNegbinomial::test_standard_errors",
"statsmodels/genmod/tests/test_glm.py::TestGlmNegbinomial::test_fittedvalues",
"statsmodels/genmod/tests/test_glm.py::TestGlmNegbinomial::test_prsquared",
"statsmodels/genmod/tests/test_glm.py::TestGlmNegbinomial::test_degrees",
"statsmodels/genmod/tests/test_glm.py::TestGlmNegbinomial::test_null_deviance",
"statsmodels/genmod/tests/test_glm.py::TestGlmNegbinomial::test_summary2",
"statsmodels/genmod/tests/test_glm.py::TestGlmNegbinomial::test_get_distribution",
"statsmodels/genmod/tests/test_glm.py::TestGlmNegbinomial::test_deviance",
"statsmodels/genmod/tests/test_glm.py::TestGlmNegbinomial::test_pearson_chi2",
"statsmodels/genmod/tests/test_glm.py::TestGlmNegbinomial::test_params",
"statsmodels/genmod/tests/test_glm.py::TestGlmNegbinomial::test_tpvalues",
"statsmodels/genmod/tests/test_glm.py::TestGlmNegbinomial::test_aic_R",
"statsmodels/genmod/tests/test_glm.py::TestGlmNegbinomial::test_bic",
"statsmodels/genmod/tests/test_glm.py::TestGlmPoissonOffset::test_tpvalues",
"statsmodels/genmod/tests/test_glm.py::TestGlmPoissonOffset::test_aic_Stata",
"statsmodels/genmod/tests/test_glm.py::TestGlmPoissonOffset::test_summary2",
"statsmodels/genmod/tests/test_glm.py::TestGlmPoissonOffset::test_get_distribution",
"statsmodels/genmod/tests/test_glm.py::TestGlmPoissonOffset::test_scale",
"statsmodels/genmod/tests/test_glm.py::TestGlmPoissonOffset::test_summary",
"statsmodels/genmod/tests/test_glm.py::TestGlmPoissonOffset::test_standard_errors",
"statsmodels/genmod/tests/test_glm.py::TestGlmPoissonOffset::test_bic",
"statsmodels/genmod/tests/test_glm.py::TestGlmPoissonOffset::test_residuals",
"statsmodels/genmod/tests/test_glm.py::TestGlmPoissonOffset::test_fittedvalues",
"statsmodels/genmod/tests/test_glm.py::TestGlmPoissonOffset::test_deviance",
"statsmodels/genmod/tests/test_glm.py::TestGlmPoissonOffset::test_degrees",
"statsmodels/genmod/tests/test_glm.py::TestGlmPoissonOffset::test_pearson_chi2",
"statsmodels/genmod/tests/test_glm.py::TestGlmPoissonOffset::test_missing",
"statsmodels/genmod/tests/test_glm.py::TestGlmPoissonOffset::test_loglike",
"statsmodels/genmod/tests/test_glm.py::TestGlmPoissonOffset::test_params",
"statsmodels/genmod/tests/test_glm.py::TestGlmPoissonOffset::test_predict",
"statsmodels/genmod/tests/test_glm.py::TestGlmPoissonOffset::test_offset_exposure",
"statsmodels/genmod/tests/test_glm.py::TestGlmPoissonOffset::test_prsquared",
"statsmodels/genmod/tests/test_glm.py::TestGlmPoissonOffset::test_null_deviance",
"statsmodels/genmod/tests/test_glm.py::TestGlmPoissonOffset::test_aic_R",
"statsmodels/genmod/tests/test_glm.py::TestGlmBernoulli::test_tpvalues",
"statsmodels/genmod/tests/test_glm.py::TestGlmBernoulli::test_params",
"statsmodels/genmod/tests/test_glm.py::TestGlmBernoulli::test_bic",
"statsmodels/genmod/tests/test_glm.py::TestGlmBernoulli::test_aic_Stata",
"statsmodels/genmod/tests/test_glm.py::TestGlmBernoulli::test_aic_R",
"statsmodels/genmod/tests/test_glm.py::TestGlmBernoulli::test_compare_discrete",
"statsmodels/genmod/tests/test_glm.py::TestGlmBernoulli::test_summary",
"statsmodels/genmod/tests/test_glm.py::TestGlmBernoulli::test_get_prediction",
"statsmodels/genmod/tests/test_glm.py::TestGlmBernoulli::test_get_distribution",
"statsmodels/genmod/tests/test_glm.py::TestGlmBernoulli::test_score_r",
"statsmodels/genmod/tests/test_glm.py::TestGlmBernoulli::test_degrees",
"statsmodels/genmod/tests/test_glm.py::TestGlmBernoulli::test_scale",
"statsmodels/genmod/tests/test_glm.py::TestGlmBernoulli::test_loglike",
"statsmodels/genmod/tests/test_glm.py::TestGlmBernoulli::test_score_test",
"statsmodels/genmod/tests/test_glm.py::TestGlmBernoulli::test_deviance",
"statsmodels/genmod/tests/test_glm.py::TestGlmBernoulli::test_residuals",
"statsmodels/genmod/tests/test_glm.py::TestGlmBernoulli::test_pearson_chi2",
"statsmodels/genmod/tests/test_glm.py::TestGlmBernoulli::test_fittedvalues",
"statsmodels/genmod/tests/test_glm.py::TestGlmBernoulli::test_prsquared",
"statsmodels/genmod/tests/test_glm.py::TestGlmBernoulli::test_null_deviance",
"statsmodels/genmod/tests/test_glm.py::TestGlmBernoulli::test_standard_errors",
"statsmodels/genmod/tests/test_glm.py::TestGlmBernoulli::test_summary2",
"statsmodels/genmod/tests/test_glm.py::TestWtdTweediePower2::test_loglike",
"statsmodels/genmod/tests/test_glm.py::TestWtdTweediePower2::test_params",
"statsmodels/genmod/tests/test_glm.py::TestWtdTweediePower2::test_fittedvalues",
"statsmodels/genmod/tests/test_glm.py::TestWtdTweediePower2::test_standard_errors",
"statsmodels/genmod/tests/test_glm.py::TestWtdTweediePower2::test_deviance",
"statsmodels/genmod/tests/test_glm.py::TestWtdTweediePower2::test_bic",
"statsmodels/genmod/tests/test_glm.py::TestWtdTweediePower2::test_tpvalues",
"statsmodels/genmod/tests/test_glm.py::TestWtdTweediePower2::test_aic",
"statsmodels/genmod/tests/test_glm.py::TestWtdTweediePower2::test_null_deviance",
"statsmodels/genmod/tests/test_glm.py::TestWtdTweediePower2::test_scale"
] | [
"statsmodels/tools/tests/test_data.py::test_as_array_with_name_array",
"statsmodels/tools/tests/test_data.py::test_as_array_with_name_series",
"statsmodels/genmod/tests/test_glm.py::test_names_default",
"statsmodels/genmod/tests/test_glm.py::test_names"
] | Python | [] | [] |
statsmodels/statsmodels | 9,165 | statsmodels__statsmodels-9165 | [
"9162"
] | 37bd2e421bad61bc54e8260b86a10a68d17892b3 | diff --git a/statsmodels/tsa/statespace/mlemodel.py b/statsmodels/tsa/statespace/mlemodel.py
--- a/statsmodels/tsa/statespace/mlemodel.py
+++ b/statsmodels/tsa/statespace/mlemodel.py
@@ -3644,6 +3644,10 @@ def simulate(self, nsimulations, measurement_shocks=None,
if iloc > self.nobs:
raise ValueError('Cannot anchor simulation outside of the sample.')
+ # GH 9162
+ from statsmodels.tsa.statespace import simulation_smoother
+ random_state = simulation_smoother.check_random_state(random_state)
+
# Setup the initial state
if initial_state is None:
initial_state_moments = (
@@ -3652,7 +3656,7 @@ def simulate(self, nsimulations, measurement_shocks=None,
_repetitions = 1 if repetitions is None else repetitions
- initial_state = np.random.multivariate_normal(
+ initial_state = random_state.multivariate_normal(
*initial_state_moments, size=_repetitions).T
scale = self.scale if self.filter_results.filter_concentrated else None
| diff --git a/statsmodels/tsa/arima/tests/test_model.py b/statsmodels/tsa/arima/tests/test_model.py
--- a/statsmodels/tsa/arima/tests/test_model.py
+++ b/statsmodels/tsa/arima/tests/test_model.py
@@ -413,3 +413,22 @@ def test_hannan_rissanen_with_fixed_params(ar_order, ma_order, fixed_params):
res = mod.fit(method='hannan_rissanen')
assert_allclose(res.params, desired_p.params)
+
+
[email protected](
+ "random_state_type", [7, np.random.RandomState, np.random.default_rng]
+)
+def test_reproducible_simulation(random_state_type):
+ x = np.random.randn(100)
+ res = ARIMA(x, order=(1, 0, 0)).fit()
+
+ def get_random_state(val):
+ if isinstance(random_state_type, int):
+ return 7
+ return random_state_type(7)
+
+ random_state = get_random_state(random_state_type)
+ sim1 = res.simulate(1, random_state=random_state)
+ random_state = get_random_state(random_state_type)
+ sim2 = res.simulate(1, random_state=random_state)
+ assert_allclose(sim1, sim2)
| `ARIMAResults.simulate` doesn't respect `random_state`
#### Describe the bug
The method `ARIMAResults.simulate` doesn't seem to respect the parameter `random_state`. It returns different results for a fixed random state parameter.
#### Code Sample
```python
from statsmodels.tsa.arima.model import ARIMA
import numpy as np
x = np.random.randn(100)
mod = ARIMA(x, order=(1, 0, 0))
res = mod.fit()
for i in range(5):
print(i, res.simulate(1, random_state=7))
```
#### Expected Output
For me, this prints a different number for each of the iterations. My expectation, however, is that the same number is generated on each invocation of `simulate` because `random_state` is fixed.
#### Output of ``import statsmodels.api as sm; sm.show_versions()``
<details>
INSTALLED VERSIONS
------------------
Python: 3.10.12.final.0
OS: Darwin 23.3.0 Darwin Kernel Version 23.3.0: Wed Dec 20 21:30:44 PST 2023; root:xnu-10002.81.5~7/RELEASE_ARM64_T6000 arm64
byteorder: little
LC_ALL: en_US.UTF-8
LANG: en_US.UTF-8
statsmodels
===========
Installed: 0.14.1 (/Users/adaitche/homebrew/lib/python3.10/site-packages/statsmodels)
Required Dependencies
=====================
cython: Not installed
numpy: 1.24.1 (/Users/adaitche/homebrew/lib/python3.10/site-packages/numpy)
scipy: 1.10.0 (/Users/adaitche/homebrew/lib/python3.10/site-packages/scipy)
pandas: 1.5.3 (/Users/adaitche/homebrew/lib/python3.10/site-packages/pandas)
dateutil: 2.8.2 (/Users/adaitche/homebrew/lib/python3.10/site-packages/dateutil)
patsy: 0.5.6 (/Users/adaitche/homebrew/lib/python3.10/site-packages/patsy)
Optional Dependencies
=====================
matplotlib: 3.6.3 (/Users/adaitche/homebrew/lib/python3.10/site-packages/matplotlib)
backend: MacOSX
cvxopt: Not installed
joblib: Not installed
Developer Tools
================
IPython: 8.9.0 (/Users/adaitche/homebrew/lib/python3.10/site-packages/IPython)
jinja2: 3.1.2 (/Users/adaitche/homebrew/lib/python3.10/site-packages/jinja2)
sphinx: Not installed
pygments: 2.14.0 (/Users/adaitche/homebrew/lib/python3.10/site-packages/pygments)
pytest: 7.4.3 (/Users/adaitche/homebrew/lib/python3.10/site-packages/pytest)
virtualenv: Not installed
</details>
| Thanks for reporting this. The problem appears to be that the `initial_state` is drawn without using the provided `random_state`: https://github.com/statsmodels/statsmodels/blob/main/statsmodels/tsa/statespace/mlemodel.py#L3648-L3656 | "2024-02-27T15:13:58Z" | 0.14 | [
"statsmodels/tsa/arima/tests/test_model.py::test_append_with_exog",
"statsmodels/tsa/arima/tests/test_model.py::test_hannan_rissanen_with_fixed_params[1-1-fixed_params1]",
"statsmodels/tsa/arima/tests/test_model.py::test_hannan_rissanen_with_fixed_params[2-3-fixed_params2]",
"statsmodels/tsa/arima/tests/test_model.py::test_hannan_rissanen_with_fixed_params[ar_order3-0-fixed_params3]",
"statsmodels/tsa/arima/tests/test_model.py::test_hannan_rissanen_with_fixed_params[1-1-fixed_params0]",
"statsmodels/tsa/arima/tests/test_model.py::test_forecast_with_exog",
"statsmodels/tsa/arima/tests/test_model.py::test_forecast",
"statsmodels/tsa/arima/tests/test_model.py::test_append_with_exog_pandas",
"statsmodels/tsa/arima/tests/test_model.py::test_clone",
"statsmodels/tsa/arima/tests/test_model.py::test_low_memory",
"statsmodels/tsa/arima/tests/test_model.py::test_default_trend",
"statsmodels/tsa/arima/tests/test_model.py::test_append",
"statsmodels/tsa/arima/tests/test_model.py::test_invalid",
"statsmodels/tsa/arima/tests/test_model.py::test_hannan_rissanen_with_fixed_params[ar_order4-ma_order4-fixed_params4]",
"statsmodels/tsa/arima/tests/test_model.py::test_append_with_exog_and_trend",
"statsmodels/tsa/arima/tests/test_model.py::test_burg",
"statsmodels/tsa/arima/tests/test_model.py::test_innovations",
"statsmodels/tsa/arima/tests/test_model.py::test_constant_integrated_model_error",
"statsmodels/tsa/arima/tests/test_model.py::test_innovations_mle",
"statsmodels/tsa/arima/tests/test_model.py::test_yule_walker",
"statsmodels/tsa/arima/tests/test_model.py::test_hannan_rissanen",
"statsmodels/tsa/arima/tests/test_model.py::test_cov_type_none",
"statsmodels/tsa/arima/tests/test_model.py::test_nonstationary_gls_error",
"statsmodels/tsa/arima/tests/test_model.py::test_statespace"
] | [
"statsmodels/tsa/arima/tests/test_model.py::test_reproducible_simulation[7]",
"statsmodels/tsa/arima/tests/test_model.py::test_reproducible_simulation[RandomState]",
"statsmodels/tsa/arima/tests/test_model.py::test_reproducible_simulation[default_rng]"
] | Python | [] | [] |
statsmodels/statsmodels | 9,227 | statsmodels__statsmodels-9227 | [
"9233"
] | bc1899510adacebf1ec351e92a78b7421c17dbb0 | diff --git a/statsmodels/robust/covariance.py b/statsmodels/robust/covariance.py
--- a/statsmodels/robust/covariance.py
+++ b/statsmodels/robust/covariance.py
@@ -28,9 +28,15 @@
from scipy import stats, linalg
from scipy.linalg.lapack import dtrtri
from .scale import mad
+import statsmodels.robust.norms as rnorms
+import statsmodels.robust.scale as rscale
+import statsmodels.robust.tools as rtools
from statsmodels.tools.testing import Holder
+from statsmodels.stats.covariance import corr_rank, corr_normal_scores
+
mad0 = lambda x: mad(x, center=0) # noqa: E731
+median = lambda x: np.median(x, axis=0) # noqa: E731
# from scikit-learn
@@ -311,6 +317,7 @@ def mahalanobis(data, cov=None, cov_inv=None, sqrt=False):
"""Mahalanobis distance squared
Note: this is without taking the square root.
+ assumes data is already centered.
Parameters
----------
@@ -405,13 +412,15 @@ def cov_gk(data, scale_func=mad):
def cov_ogk(data, maxiter=2, scale_func=mad, cov_func=cov_gk,
loc_func=lambda x: np.median(x, axis=0), reweight=0.9,
rescale=True, rescale_raw=True, ddof=1):
- """orthogonalized Gnanadesikan and Kettenring covariance estimator
+ """Orthogonalized Gnanadesikan and Kettenring covariance estimator.
Based on Maronna and Zamar 2002
Parameters
----------
- data : array_like, 2-D
+ data : array-like
+ Multivariate data set with observation in rows and variables in
+ columns.
maxiter : int
Number of iteration steps. According to Maronna and Zamar the
estimate doesn't improve much after the second iteration and the
@@ -590,12 +599,10 @@ def cov_tyler(data, start_cov=None, normalize=False, maxiter=100, eps=1e-13):
----------
.. [1] Tyler, David E. “A Distribution-Free M-Estimator of Multivariate
Scatter.” The Annals of Statistics 15, no. 1 (March 1, 1987): 234–51.
-
.. [2] Soloveychik, I., and A. Wiesel. 2014. Tyler's Covariance Matrix
Estimator in Elliptical Models With Convex Structure.
IEEE Transactions on Signal Processing 62 (20): 5251-59.
doi:10.1109/TSP.2014.2348951.
-
.. [3] Ollila, Esa, Daniel P. Palomar, and Frederic Pascal.
“Affine Equivariant Tyler’s M-Estimator Applied to Tail Parameter
Learning of Elliptical Distributions.” arXiv, May 7, 2023.
@@ -1083,7 +1090,7 @@ def _cov_iter(data, weights_func, weights_args=None, cov_init=None,
return res
-def _cov_starting(data, standardize=False, quantile=0.5):
+def _cov_starting(data, standardize=False, quantile=0.5, retransform=False):
"""compute some robust starting covariances
The returned covariance matrices are intended as starting values
@@ -1127,7 +1134,7 @@ def _cov_starting(data, standardize=False, quantile=0.5):
cov_all = []
d = mahalanobis(xs, cov=None, cov_inv=np.eye(k_vars))
- percentiles = [(k_vars+2) / nobs * 100 * 2, 25, 50]
+ percentiles = [(k_vars+2) / nobs * 100 * 2, 25, 50, 85]
cutoffs = np.percentile(d, percentiles)
for p, cutoff in zip(percentiles, cutoffs):
xsp = xs[d < cutoff]
@@ -1149,15 +1156,929 @@ def _cov_starting(data, standardize=False, quantile=0.5):
c03 = _cov_iter(xs, weights_quantile, weights_args=(quantile,),
rescale="med", cov_init=c02.cov, maxiter=100)
- if standardize:
+ if not standardize or not retransform:
cov_all.extend([c0, c01, c02, c03])
else:
# compensate for initial rescaling
+ # TODO: this does not return list of Holder anymore
s = np.outer(std, std)
cov_all.extend([r.cov * s for r in [c0, c01, c02, c03]])
c2 = cov_ogk(xs)
cov_all.append(c2)
+ c2raw = Holder(
+ cov=c2.cov_raw,
+ mean=c2.loc_raw * std + center,
+ method="ogk_raw",
+ )
+ cov_all.append(c2raw)
+
+ z_tanh = np.tanh(xs)
+ c_th = Holder(
+ cov=np.corrcoef(z_tanh.T), # not consistently scaled for cov
+ mean=center, # TODO: do we add inverted mean z_tanh ?
+ method="tanh",
+ )
+ cov_all.append(c_th)
+
+ x_spatial = xs / np.sqrt(np.sum(xs**2, axis=1))[:, None]
+ c_th = Holder(
+ cov=np.cov(x_spatial.T),
+ mean=center,
+ method="spatial",
+ )
+ cov_all.append(c_th)
+
+ c_th = Holder(
+ # not consistently scaled for cov
+ # cov=stats.spearmanr(xs)[0], # not correct shape if k=1 or 2
+ cov=corr_rank(xs), # always returns matrix, np.corrcoef result
+ mean=center,
+ method="spearman",
+ )
+ cov_all.append(c_th)
+
+ c_ns = Holder(
+ cov=corr_normal_scores(xs), # not consistently scaled for cov
+ mean=center, # TODO: do we add inverted mean z_tanh ?
+ method="normal-scores",
+ )
+ cov_all.append(c_ns)
+
# TODO: rescale back to original space using center and std
return cov_all
+
+
+# ####### Det, CovDet and helper functions, might be moved to separate module
+
+
+class _Standardize():
+ """Robust standardization of random variable
+
+ """
+
+ def __init__(self, x, func_center=None, func_scale=None):
+ # naming mean or center
+ # maybe also allow str func_scale for robust.scale, e.g. for not
+ # vectorized Qn
+ if func_center is None:
+ center = np.median(x, axis=0)
+ else:
+ center = func_center(x)
+ xdm = x - center
+ if func_scale is None:
+ scale = mad(xdm, center=0)
+ else:
+ # assumes vectorized
+ scale = func_scale(x)
+
+ self.x_stand = xdm / scale
+
+ self.center = center
+ self.scale = scale
+
+ def transform(self, x):
+ return (x - self.center) / self.scale
+
+ def untransform_mom(self, m, c):
+ mean = self.center + m
+ cov = c * np.outer(self.scale, self.scale)
+ return mean, cov
+
+
+def _orthogonalize_det(x, corr, loc_func, scale_func):
+ """Orthogonalize
+
+ This is a simplified version of the OGK method.
+ version from DetMCD works on zscored data
+ (does not return mean and cov of original data)
+ so we drop the compensation for scaling in zscoring
+
+ z is the data here, zscored with robust estimators,
+ e.g. median and Qn in DetMCD
+ """
+ evals, evecs = np.linalg.eigh(corr) # noqa: F841
+ z = x.dot(evecs)
+ transf0 = evecs
+
+ scale_z = scale_func(z) # scale of principal components
+ cov = (transf0 * scale_z**2).dot(transf0.T)
+ # extra step in DetMCD, sphering data with new cov to compute center
+ # I think this is equivalent to scaling z
+ # loc_z = loc_func(z / scale_z) * scale_z # center of principal components
+ # loc = (transf0 * scale_z).dot(loc_z)
+ transf1 = (transf0 * scale_z).dot(transf0.T)
+ # transf1inv = (transf0 * scale_z**(-1)).dot(transf0.T)
+
+ # loc = loc_func(x @ transf1inv) @ transf1
+ loc = loc_func((z / scale_z).dot(transf0.T)) @ transf1
+
+ return loc, cov
+
+
+def _get_detcov_startidx(z, h, options_start=None, methods_cov="all"):
+ """Starting sets for deterministic robust covariance estimators.
+
+ These are intended as starting sets for DetMCD, DetS and DetMM.
+ """
+
+ if options_start is None:
+ options_start = {}
+
+ loc_func = options_start.get("loc_func", median)
+ scale_func = options_start.get("scale_func", mad)
+ z = (z - loc_func(z)) / scale_func(z)
+
+ if np.squeeze(z).ndim == 1:
+ # only one random variable
+ z = np.squeeze(z)
+ nobs = z.shape[0]
+ idx_sel = np.argpartition(np.abs(z), h)[:h]
+ idx_all = [(idx_sel, "abs-resid")]
+ # next uses symmetric equal-tail trimming
+ idx_sorted = np.argsort(z)
+ h_tail = (nobs - h) // 2
+ idx_all.append((idx_sorted[h_tail : h_tail + h], "trimmed-tail"))
+ return idx_all
+
+ # continue if more than 1 random variable
+ cov_all = _cov_starting(z, standardize=False, quantile=0.5)
+
+ # orthogonalization step
+ idx_all = []
+ for c in cov_all:
+ if not hasattr(c, "method"):
+ continue
+ method = c.method
+ mean, cov = _orthogonalize_det(z, c.cov, loc_func, scale_func)
+ d = mahalanobis(z, mean, cov)
+ idx_sel = np.argpartition(d, h)[:h]
+ idx_all.append((idx_sel, method))
+
+ return idx_all
+
+
+class CovM:
+ """M-estimator for multivariate Mean and Scatter.
+
+ Interface incomplete and experimental.
+
+ Parameters
+ ----------
+ data : array-like
+ Multivariate data set with observation in rows and variables in
+ columns.
+ norm_mean : norm instance
+ If None, then TukeyBiweight norm is used.
+ (Currently no other norms are supported for calling the initial
+ S-estimator)
+ norm_scatter : None or norm instance
+ If norm_scatter is None, then the norm_mean will be used.
+ breakdown_point : float in (0, 0.5]
+ Breakdown point for first stage S-estimator.
+ scale_bias : None or float
+ Must currently be provided if norm_mean is not None.
+ method : str
+ Currently only S-estimator has automatic selection of scale function.
+ """
+
+ def __init__(self, data, norm_mean=None, norm_scatter=None,
+ scale_bias=None, method="S"):
+ # todo: method defines how norm_mean and norm_scatter are linked
+ # currently I try for S-estimator
+
+ if method.lower() not in ["s"]:
+ msg = f"method {method} option not recognize or implemented"
+ raise ValueError(msg)
+
+ self.data = np.asarray(data)
+ self.k_vars = k_vars = self.data.shape[1]
+
+ # Todo: check interface for scale bias
+ self.scale_bias = scale_bias
+ if norm_mean is None:
+ norm_mean = rnorms.TukeyBiweight()
+ c = rtools.tuning_s_cov(norm_mean, k_vars, breakdown_point=0.5)
+ norm_mean._set_tuning_param(c, inplace=True)
+ self.scale_bias = rtools.scale_bias_cov_biw(c, k_vars)[0]
+
+ self.norm_mean = norm_mean
+ if norm_scatter is None:
+ self.norm_scatter = self.norm_mean
+ else:
+ self.norm_scatter = norm_scatter
+
+ self.weights_mean = self.norm_mean.weights
+ self.weights_scatter = self.weights_mean
+ # self.weights_scatter = lambda d: self.norm_mean.rho(d) / d**2
+ # this is for S-estimator, M-scale
+ self.rho = self.norm_scatter.rho
+
+ def _fit_mean_shape(self, mean, shape, scale):
+ """Estimate mean and shape in iteration step.
+
+ This does only one step.
+
+ Parameters
+ ----------
+ mean : ndarray
+ Starting value for mean
+ shape : ndarray
+ Starting value for shape matrix.
+ scale : float
+ Starting value for scale.
+
+ Returns
+ -------
+ Holder instance with updated estimates.
+ """
+ d = mahalanobis(self.data - mean, shape, sqrt=True) / scale
+ weights_mean = self.weights_mean(d)
+ weights_cov = self.weights_scatter(d)
+
+ res = cov_weighted(
+ self.data,
+ weights=weights_mean,
+ center=None,
+ weights_cov=weights_cov,
+ weights_cov_denom="det",
+ ddof=1,
+ )
+ return res
+
+ def _fit_scale(self, maha, start_scale=None, maxiter=100, rtol=1e-5,
+ atol=1e-5):
+ """Estimate iterated M-scale.
+
+ Parameters
+ ----------
+ maha : ndarray
+ start_scale : None or float
+ Starting scale. If it is None, the mad of maha wi
+ maxiter : int
+ Maximum iterations to compute M-scale
+ rtol, atol : float
+ Relative and absolute convergence criteria for scale used with
+ allclose.
+
+ Returns
+ -------
+ float : scale estimate
+ """
+ if start_scale is None:
+ # TODO: this does not really make sense
+ # better scale to median of maha and chi or chi2
+ start_scale = mad(maha)
+
+ scale = rscale._scale_iter(
+ maha,
+ scale0=start_scale,
+ maxiter=maxiter,
+ rtol=rtol,
+ atol=atol,
+ meef_scale=self.rho,
+ scale_bias=self.scale_bias,
+ )
+ return scale
+
+ def fit(self, start_mean=None, start_shape=None, start_scale=None,
+ maxiter=100, update_scale=True):
+ """Estimate mean, shape and scale parameters with MM-estimator.
+
+ Parameters
+ ----------
+ start_mean : None or float
+ Starting value for mean, center.
+ If None, then median is used.
+ start_shape : None or 2-dim ndarray
+ Starting value of shape matrix, i.e. scatter matrix normalized
+ to det(scatter) = 1.
+ If None, then scaled covariance matrix of data is used.
+ start_scale : None or float.
+ Starting value of scale.
+ maxiter : int
+ Maximum number of iterations.
+ update_scale : bool
+ If update_scale is False, then
+
+ Returns
+ -------
+ results instance with mean, shape, scale, cov and other attributes.
+
+ Notes
+ -----
+ If start_scale is provided and update_scale is False, then this is
+ an M-estimator with a predetermined scale as used in the second
+ stage of an MM-estimator.
+
+ """
+
+ converged = False
+
+ if start_scale is not None:
+ scale_old = start_scale
+ else:
+ scale_old = 1
+ # will be reset if start_shape is also None.
+ if start_mean is not None:
+ mean_old = start_mean
+ else:
+ mean_old = np.median(self.data, axis=0)
+ if start_shape is not None:
+ shape_old = start_shape
+ else:
+ shape_old = np.cov(self.data.T)
+ scale = np.linalg.det(shape_old) ** (1 / self.k_vars)
+ shape_old /= scale
+ if start_scale is not None:
+ scale_old = scale
+
+ if update_scale is False:
+ scale = start_scale
+
+ for i in range(maxiter):
+ shape, mean = self._fit_mean_shape(mean_old, shape_old, scale_old)
+ d = mahalanobis(self.data - mean, shape, sqrt=True)
+ if update_scale:
+ scale = self._fit_scale(d, start_scale=scale_old, maxiter=10)
+
+ if (np.allclose(scale, scale_old, rtol=1e-5) and
+ np.allclose(mean, mean_old, rtol=1e-5) and
+ np.allclose(shape, shape_old, rtol=1e-5)
+ ): # noqa E124
+ converged = True
+ break
+ scale_old = scale
+ mean_old = mean
+ shape_old = shape
+
+ maha = mahalanobis(self.data - mean, shape / scale, sqrt=True)
+
+ res = Holder(
+ mean=mean,
+ shape=shape,
+ scale=scale,
+ cov=shape * scale**2,
+ converged=converged,
+ n_iter=i,
+ mahalanobis=maha,
+ )
+ return res
+
+
+class CovDetMCD:
+ """Minimum covariance determinant estimator with deterministic starts.
+
+ preliminary version
+
+ reproducability:
+
+ This uses deterministic starting sets and there is no randomness in the
+ estimator.
+ However, this will not be reprodusible across statsmodels versions
+ when the methods for starting sets or tuning parameters for the
+ optimization change.
+
+ Parameters
+ ----------
+ data : array-like
+ Multivariate data set with observation in rows and variables in
+ columns.
+
+ Notes
+ -----
+ The correction to the scale to take account of trimming in the reweighting
+ estimator is based on the chisquare tail probability.
+ This differs from CovMcd in R which uses the observed fraction of
+ observations above the metric trimming threshold.
+
+ References
+ ----------
+ ..[1] Hubert, Mia, Peter Rousseeuw, Dina Vanpaemel, and Tim Verdonck. 2015.
+ “The DetS and DetMM Estimators for Multivariate Location and Scatter.”
+ Computational Statistics & Data Analysis 81 (January): 64–75.
+ https://doi.org/10.1016/j.csda.2014.07.013.
+ ..[2] Hubert, Mia, Peter J. Rousseeuw, and Tim Verdonck. 2012. “A
+ Deterministic Algorithm for Robust Location and Scatter.” Journal of
+ Computational and Graphical Statistics 21 (3): 618–37.
+ https://doi.org/10.1080/10618600.2012.672100.
+ """
+
+ def __init__(self, data):
+ # no options yet, methods were written as functions
+ self.data = np.asarray(data)
+
+ def _cstep(self, x, mean, cov, h, maxiter=2, tol=1e-8):
+ """C-step for mcd iteration
+
+ x is data, perc is percentile h / nobs, don't need perc when we
+ use np.argpartition
+ requires starting mean and cov
+ """
+
+ converged = False
+
+ for _ in range(maxiter):
+ d = mahalanobis(x - mean, cov)
+ idx_sel = np.argpartition(d, h)[:h]
+ x_sel = x[idx_sel]
+ mean = x_sel.mean(0)
+ cov_new = np.cov(x_sel.T, ddof=1)
+
+ if ((cov - cov_new)**2).mean() < tol:
+ cov = cov_new
+ converged = True
+ break
+
+ cov = cov_new
+
+ return mean, cov, converged
+
+ def _fit_one(self, x, idx, h, maxiter=2, mean=None, cov=None):
+ """Compute mcd for one starting set of observations.
+
+ Parameters
+ ----------
+ x : ndarray
+ Data.
+ idx : ndarray
+ Indices or mask of observation in starting set, used as ``x[idx]``
+ h : int
+ Number of observations in evaluation set for cov.
+ maxiter : int
+ Maximum number of c-steps.
+
+ Returns
+ -------
+ mean : ndarray
+ Estimated mean.
+ cov : ndarray
+ Estimated covariance.
+ det : float
+ Determinant of estimated covariance matrix.
+
+ Notes
+ -----
+ This does not do any preprocessing of the data and returns the
+ empirical mean and covariance of evaluation set of the data ``x``.
+ """
+ if idx is not None:
+ x_sel = x[idx]
+ else:
+ x_sel = x
+ if mean is None:
+ mean = x_sel.mean(0)
+ if cov is None:
+ cov = np.cov(x_sel.T, ddof=1)
+
+ # updated with c-step
+ mean, cov, conv = self._cstep(x, mean, cov, h, maxiter=maxiter)
+ det = np.linalg.det(cov)
+
+ return mean, cov, det, conv
+
+ def fit(self, h, *, h_start=None, mean_func=None, scale_func=None,
+ maxiter=100, options_start=None, reweight=True,
+ trim_frac=0.975, maxiter_step=100):
+ """
+ Compute minimum covariance determinant estimate of mean and covariance.
+
+ x : array-like
+ Data with observation in rows and variables in columns.
+ h : int
+ Number of observations in evaluation set for minimimizing
+ determinant.
+ h_start : int
+ Number of observations used in starting mean and covariance.
+ mean_func, scale_func : callable or None.
+ Mean and scale function for initial standardization.
+ Current defaults, if they are None, are median and mad, but
+ default scale_func will likely change.
+ options_start : None or dict
+ Options for the starting estimators.
+ currently not used
+ TODO: which options? e.g. for OGK
+ reweight : bool
+ If reweight is true, then a reweighted estimator is returned. The
+ reweighting is based on a chisquare trimming of Mahalanobis
+ distances. The raw results are in the ``results_raw`` attribute.
+ trim_frac : float in (0, 1)
+ Trim fraction used if reweight is true. Used to compute quantile
+ of chisquare distribution with tail probability 1 - trim_frac.
+ maxiter_step : int
+ Number of iteration in the c-step.
+ In the current implementation a small maxiter in the c-step does
+ not find the optimal solution.
+
+ Returns
+ -------
+ Holder instance with results
+ """
+
+ x = self.data
+ nobs, k_vars = x.shape
+
+ if h is None:
+ h = (nobs + k_vars + 1) // 2 # check with literature
+ if mean_func is None:
+ mean_func = lambda x: np.median(x, axis=0) # noqa
+ if scale_func is None:
+ scale_func = mad
+ if options_start is None:
+ options_start = {}
+ if h_start is None:
+ nobs, k_vars = x.shape
+ h_start = max(nobs // 2 + 1, k_vars + 1)
+
+ m = mean_func(x)
+ s = scale_func(x)
+ z = (x - m) / s
+ # get initial mean, cov of standardized data, we only need ranking
+ # of obs
+ starts = _get_detcov_startidx(z, h_start, options_start)
+
+ fac_trunc = coef_normalize_cov_truncated(h / nobs, k_vars)
+
+ res = {}
+ for ii, ini in enumerate(starts):
+ idx_sel, method = ini
+ mean, cov, det, _ = self._fit_one(x, idx_sel, h,
+ maxiter=maxiter_step)
+ res[ii] = Holder(
+ mean=mean,
+ cov=cov * fac_trunc,
+ det_subset=det,
+ method=method,
+ )
+
+ det_all = np.array([i.det_subset for i in res.values()])
+ idx_best = np.argmin(det_all)
+ best = res[idx_best]
+ # mean = best.mean
+ # cov = best.cov
+
+ # need to c-step to convergence for best,
+ # is with best 2 in original DetMCD
+ if maxiter_step < maxiter:
+ mean, cov, det, conv = self._fit_one(x, None, h, maxiter=maxiter,
+ mean=best.mean, cov=best.cov)
+ best = Holder(
+ mean=mean,
+ cov=cov * fac_trunc,
+ det_subset=det,
+ method=method,
+ converged=conv,
+ )
+
+ # include extra info in returned Holder instance
+ best.det_all = det_all
+ best.idx_best = idx_best
+
+ best.tmean = m
+ best.tscale = s
+
+ if reweight:
+ cov, mean = _reweight(x, best.mean, best.cov, trim_frac=trim_frac,
+ ddof=1)
+ fac_trunc = coef_normalize_cov_truncated(trim_frac, k_vars)
+ best_w = Holder(
+ mean=mean,
+ cov=cov * fac_trunc,
+ # det_subset=det,
+ method=method,
+ results_raw=best,
+ )
+
+ return best_w
+ else:
+ return best # is Holder instance already
+
+
+class CovDetS:
+ """S-estimator for mean and covariance with deterministic starts.
+
+ Parameters
+ ----------
+ data : array-like
+ Multivariate data set with observation in rows and variables in
+ columns.
+ norm : norm instance
+ If None, then TukeyBiweight norm is used.
+ (Currently no other norms are supported for calling the initial
+ S-estimator)
+ breakdown_point : float in (0, 0.5]
+ Breakdown point for first stage S-estimator.
+
+ Notes
+ -----
+ Reproducability:
+
+ This uses deterministic starting sets and there is no randomness in the
+ estimator.
+ However, the estimates may not be reproducable across statsmodels versions
+ when the methods for starting sets or default tuning parameters for the
+ optimization change. With different starting sets, the estimate can
+ converge to a different local optimum.
+
+ References
+ ----------
+ ..[1] Hubert, Mia, Peter Rousseeuw, Dina Vanpaemel, and Tim Verdonck. 2015.
+ “The DetS and DetMM Estimators for Multivariate Location and Scatter.”
+ Computational Statistics & Data Analysis 81 (January): 64–75.
+ https://doi.org/10.1016/j.csda.2014.07.013.
+ ..[2] Hubert, Mia, Peter J. Rousseeuw, and Tim Verdonck. 2012. “A
+ Deterministic Algorithm for Robust Location and Scatter.” Journal of
+ Computational and Graphical Statistics 21 (3): 618–37.
+ https://doi.org/10.1080/10618600.2012.672100.
+ """
+
+ def __init__(self, data, norm=None, breakdown_point=0.5):
+ # no options yet, methods were written as functions
+ self.data = np.asarray(data)
+ self.nobs, k_vars = self.data.shape
+ self.k_vars = k_vars
+
+ # self.scale_bias = scale_bias
+ if norm is None:
+ norm = rnorms.TukeyBiweight()
+ c = rtools.tuning_s_cov(norm, k_vars, breakdown_point=0.5)
+ norm._set_tuning_param(c, inplace=True)
+ self.scale_bias = rtools.scale_bias_cov_biw(c, k_vars)[0]
+ else:
+ raise NotImplementedError("only Biweight norm is supported")
+
+ self.norm = norm
+
+ self.mod = CovM(data, norm_mean=norm, norm_scatter=norm,
+ scale_bias=self.scale_bias, method="S")
+
+ def _get_start_params(self, idx):
+ """Starting parameters from a subsample given by index
+
+ Parameters
+ ----------
+ idx : ndarray
+ Index used to select observations from the data. The index is used
+ for numpy arrays, so it can be either a boolean mask or integers.
+
+ Returns
+ -------
+ mean : ndarray
+ Mean of subsample
+ shape : ndarray
+ The shape matrix of the subsample which is the covariance
+ normalized so that determinant of shape is one.
+ scale : float
+ Scale of subsample, computed so that cov = shape * scale.
+ """
+ x_sel = self.data[idx]
+ k = x_sel.shape[1]
+
+ mean = x_sel.mean(0)
+ cov = np.cov(x_sel.T)
+
+ scale2 = np.linalg.det(cov) ** (1 / k)
+ shape = cov / scale2
+ scale = np.sqrt(scale2)
+ return mean, shape, scale
+
+ def _fit_one(self, mean=None, shape=None, scale=None, maxiter=100):
+ """Compute local M-estimator for one starting set of observations.
+
+ Parameters
+ ----------
+ x : ndarray
+ Data.
+ idx : ndarray
+ Indices or mask of observation in starting set, used as ``x[idx]``
+ h : int
+ Number of observations in evaluation set for cov.
+ maxiter : int
+ Maximum number of c-steps.
+
+ Returns
+ -------
+ mean : ndarray
+ Estimated mean.
+ cov : ndarray
+ Estimated covariance.
+ det : float
+ Determinant of estimated covariance matrix.
+
+ Notes
+ -----
+ This uses CovM to solve for the local optimum for given starting
+ values.
+ """
+
+ res = self.mod.fit(
+ start_mean=mean,
+ start_shape=shape,
+ start_scale=scale,
+ maxiter=maxiter,
+ update_scale=True
+ )
+
+ return res
+
+ def fit(self, *, h_start=None, mean_func=None, scale_func=None,
+ maxiter=100, options_start=None, maxiter_step=5):
+ """Compute S-estimator of mean and covariance.
+
+ Parameters
+ ----------
+ h_start : int
+ Number of observations used in starting mean and covariance.
+ mean_func, scale_func : callable or None.
+ Mean and scale function for initial standardization.
+ Current defaults, if they are None, are median and mad, but
+ default scale_func will likely change.
+ options_start : None or dict
+ Options for the starting estimators.
+ TODO: which options? e.g. for OGK
+
+ Returns
+ -------
+ Holder instance with results
+ """
+
+ x = self.data
+ nobs, k_vars = x.shape
+
+ if mean_func is None:
+ mean_func = lambda x: np.median(x, axis=0) # noqa
+ if scale_func is None:
+ scale_func = mad
+ if options_start is None:
+ options_start = {}
+ if h_start is None:
+ nobs, k_vars = x.shape
+ h_start = max(nobs // 2 + 1, k_vars + 1)
+
+ m = mean_func(x)
+ s = scale_func(x)
+ z = (x - m) / s
+ # get initial mean, cov of standardized data, we only need ranking
+ # of obs
+ starts = _get_detcov_startidx(z, h_start, options_start)
+
+ res = {}
+ for ii, ini in enumerate(starts):
+ idx_sel, method = ini
+ mean0, shape0, scale0 = self._get_start_params(idx_sel)
+ res_i = self._fit_one(
+ mean=mean0,
+ shape=shape0,
+ scale=scale0,
+ maxiter=maxiter_step,
+ )
+
+ res_i.method = method
+ res[ii] = res_i
+
+ scale_all = np.array([i.scale for i in res.values()])
+ idx_best = np.argmin(scale_all)
+ best = res[idx_best]
+ # mean = best.mean
+ # cov = best.cov
+
+ # need to c-step to convergence for best,
+ # is with best 2 in original DetMCD
+ if maxiter_step < maxiter:
+ best = self._fit_one(
+ mean=best.mean,
+ shape=best.shape,
+ scale=best.scale,
+ maxiter=maxiter,
+ )
+
+ # include extra info in returned Holder instance
+ best.scale_all = scale_all
+ best.idx_best = idx_best
+
+ best.tmean = m
+ best.tscale = s
+
+ return best # is Holder instance already
+
+
+class CovDetMM():
+ """MM estimator using DetS as first stage estimator.
+
+ Note: The tuning parameter for second stage M estimator is currently only
+ available for a small number of variables and only three values of
+ efficiency. For other cases, the user has to provide the norm instance
+ with desirec tuning parameter.
+
+ Parameters
+ ----------
+ data : array-like
+ Multivariate data set with observation in rows and variables in
+ columns.
+ norm : norm instance
+ If None, then TukeyBiweight norm is used.
+ (Currently no other norms are supported for calling the initial
+ S-estimator)
+ If ``norm`` is an instance of TukeyBiweight, then it will be used in
+ the second stage M-estimation. The ``efficiency`` argument is ignored
+ and the tuning parameter of the user provided instance is not changed.
+ breakdown_point : float in (0, 0.5]
+ Breakdown point for first stage S-estimator.
+ efficiency : float
+ Asymptotic efficiency of second stage M estimator.
+
+ Notes
+ -----
+ Current limitation is that only TukeyBiweight is supported.
+
+ The tuning parameter for second stage M estimator uses a table of values
+ for number of variables up to 15 and efficiency in
+ [0.75, 0.8, 0.85, 0.9, 0.95, 0.975, 0.99]. The tuning parameter for other
+ cases needs to be computed by numerical integration and rootfinding.
+ Alternatively, the user can provide a norm instance with desired tuning
+ parameter.
+
+ References
+ ----------
+ ..[1] Hubert, Mia, Peter Rousseeuw, Dina Vanpaemel, and Tim Verdonck. 2015.
+ “The DetS and DetMM Estimators for Multivariate Location and Scatter.”
+ Computational Statistics & Data Analysis 81 (January): 64–75.
+ https://doi.org/10.1016/j.csda.2014.07.013.
+ ..[2] Hubert, Mia, Peter J. Rousseeuw, and Tim Verdonck. 2012. “A
+ Deterministic Algorithm for Robust Location and Scatter.” Journal of
+ Computational and Graphical Statistics 21 (3): 618–37.
+ https://doi.org/10.1080/10618600.2012.672100.
+ ..[3] Lopuhaä, Hendrik P. 1989. “On the Relation between S-Estimators and
+ M-Estimators of Multivariate Location and Covariance.” The Annals of
+ Statistics 17 (4): 1662–83.
+ ..[4] Salibián-Barrera, Matías, Stefan Van Aelst, and Gert Willems. 2006.
+ “Principal Components Analysis Based on Multivariate MM Estimators with
+ Fast and Robust Bootstrap.” Journal of the American Statistical
+ Association 101 (475): 1198–1211.
+ ..[5] Tatsuoka, Kay S., and David E. Tyler. 2000. “On the Uniqueness of
+ S-Functionals and M-Functionals under Nonelliptical Distributions.” The
+ Annals of Statistics 28 (4): 1219–43.
+ """
+
+ def __init__(self, data, norm=None, breakdown_point=0.5, efficiency=0.95):
+ self.data = np.asarray(data)
+ self.nobs, k_vars = self.data.shape
+ self.k_vars = k_vars
+ self.breakdown_point = breakdown_point
+
+ # self.scale_bias = scale_bias
+ if norm is None:
+ norm = rnorms.TukeyBiweight()
+ c = rtools.tukeybiweight_mvmean_eff(k_vars, efficiency)
+ norm._set_tuning_param(c, inplace=True)
+ # scale_bias is not used for second stage MM norm
+ # self.scale_bias = rtools.scale_bias_cov_biw(c, k_vars)[0]
+ elif not isinstance(norm, rnorms.TukeyBiweight):
+ raise NotImplementedError("only Biweight norm is supported")
+ # We allow tukeybiweight norm instance with user provided c
+
+ self.norm = norm
+ # model for second stage M-estimator
+ self.mod = CovM(data, norm_mean=norm, norm_scatter=norm,
+ scale_bias=None, method="S")
+
+ def fit(self, maxiter=100):
+ """Estimate model parameters.
+
+ Parameters
+ ----------
+ maxiter : int
+ Maximum number of iterations in the second stage M-estimation.
+ fit args : dict
+ currently missing
+
+ Returns
+ -------
+ Instance of a results or holder class.
+
+ Notes
+ -----
+ This uses CovDetS for the first stage estimation and CovM with fixed
+ scale in the second stage MM-estimation.
+
+ TODO: fit options are missing.
+
+ """
+ # first stage estimate
+ mod_s = CovDetS(
+ self.data,
+ norm=None,
+ breakdown_point=self.breakdown_point
+ )
+ res_s = mod_s.fit()
+
+ res = self.mod.fit(
+ start_mean=res_s.mean,
+ start_shape=res_s.shape,
+ start_scale=res_s.scale,
+ maxiter=maxiter,
+ update_scale=False,
+ )
+
+ return res
diff --git a/statsmodels/robust/norms.py b/statsmodels/robust/norms.py
--- a/statsmodels/robust/norms.py
+++ b/statsmodels/robust/norms.py
@@ -1,6 +1,6 @@
import numpy as np
-# TODO: add plots to weighting functions for online docs.
+from . import tools as rtools
def _cabs(x):
@@ -41,6 +41,9 @@ class RobustNorm:
continuous = 1
+ def __repr__(self):
+ return self.__class__.__name__
+
def rho(self, z):
"""
The robust criterion estimator function.
@@ -199,12 +202,16 @@ class HuberT(RobustNorm):
def __init__(self, t=1.345):
self.t = t
- def _set_tuning_param(self, c):
+ def _set_tuning_param(self, c, inplace=False):
"""Set and change the tuning parameter of the Norm.
Warning: this needs to wipe cached attributes that depend on the param.
"""
- self.t = c
+ if inplace:
+ self.t = c
+ return self
+ else:
+ return self.__class__(t=c)
def max_rho(self):
return np.inf
@@ -322,6 +329,18 @@ class RamsayE(RobustNorm):
def __init__(self, a=.3):
self.a = a
+ def _set_tuning_param(self, c, inplace=False):
+ """Set and change the tuning parameter of the Norm.
+
+ Warning: this needs to wipe cached attributes that depend on the param.
+ """
+ # todo : change default to inplace=False, when tools are fixed
+ if inplace:
+ self.a = c
+ return self
+ else:
+ return self.__class__(a=c)
+
def max_rho(self):
return np.inf
@@ -419,12 +438,16 @@ class AndrewWave(RobustNorm):
def __init__(self, a=1.339):
self.a = a
- def _set_tuning_param(self, a):
+ def _set_tuning_param(self, c, inplace=False):
"""Set and change the tuning parameter of the Norm.
Warning: this needs to wipe cached attributes that depend on the param.
"""
- self.a = a
+ if inplace:
+ self.a = c
+ return self
+ else:
+ return self.__class__(a=c)
def max_rho(self):
return 2 * self.a**2
@@ -553,12 +576,16 @@ class TrimmedMean(RobustNorm):
def __init__(self, c=2.):
self.c = c
- def _set_tuning_param(self, c):
+ def _set_tuning_param(self, c, inplace=False):
"""Set and change the tuning parameter of the Norm.
Warning: this needs to wipe cached attributes that depend on the param.
"""
- self.c = c
+ if inplace:
+ self.c = c
+ return self
+ else:
+ return self.__class__(c=c)
def max_rho(self):
return self.rho(self.c)
@@ -674,14 +701,20 @@ def __init__(self, a=2., b=4., c=8.):
self.b = b
self.c = c
- def _set_tuning_param(self, c):
+ def _set_tuning_param(self, c, inplace=False):
"""Set and change the tuning parameter of the Norm.
Warning: this needs to wipe cached attributes that depend on the param.
"""
- self.c = c
- self.a = c / 4
- self.b = c / 2
+ a = c / 4
+ b = c / 2
+ if inplace:
+ self.c = c
+ self.a = a
+ self.b = b
+ return self
+ else:
+ return self.__class__(a=a, b=b, c=c)
def max_rho(self):
return self.rho(self.c)
@@ -862,12 +895,49 @@ class TukeyBiweight(RobustNorm):
def __init__(self, c=4.685):
self.c = c
- def _set_tuning_param(self, c):
+ def __repr__(self):
+ return f"{self.__class__.__name__}(c={self.c})"
+
+ @classmethod
+ def get_tuning(cls, bp=None, eff=None):
+ """Tuning parameter for given breakdown point or efficiency.
+
+ This currently only return values from a table.
+
+ Parameters
+ ----------
+ bp : float in [0.05, 0.5] or None
+ Required breakdown point
+ Either bp or eff has to be specified, but not both.
+ eff : float or None
+ Required asymptotic efficiency.
+ Either bp or eff has to be specified, but not both.
+
+ Returns
+ -------
+ float : tuning parameter.
+
+ """
+ if ((bp is None and eff is None) or
+ (bp is not None and eff is not None)):
+ raise ValueError("exactly one of bp and eff needs to be provided")
+
+ if bp is not None:
+ return rtools.tukeybiweight_bp[bp]
+ elif eff is not None:
+ return rtools.tukeybiweight_eff[eff]
+
+ def _set_tuning_param(self, c, inplace=False):
"""Set and change the tuning parameter of the Norm.
Warning: this needs to wipe cached attributes that depend on the param.
"""
- self.c = c
+ # todo : change default to inplace=False, when tools are fixed
+ if inplace:
+ self.c = c
+ return self
+ else:
+ return self.__class__(c=c)
def max_rho(self):
return self.rho(self.c)
@@ -891,7 +961,7 @@ def rho(self, z):
Returns
-------
rho : ndarray
- rho(z) = -(1 - (z/c)**2)**3 * c**2/6. for \|z\| <= R
+ rho(z) = -(1 - (z/c)**2)**3 * c**2/6 + c**2/6 for \|z\| <= R
rho(z) = 0 for \|z\| > R
"""
@@ -983,12 +1053,16 @@ def __init__(self, c=3.61752, k=4):
self.c = c
self.k = k
- def _set_tuning_param(self, c):
+ def _set_tuning_param(self, c, inplace=False):
"""Set and change the tuning parameter of the Norm.
Warning: this needs to wipe cached attributes that depend on the param.
"""
- self.c = c
+ if inplace:
+ self.c = c
+ return self
+ else:
+ return self.__class__(c=c, k=self.k)
def max_rho(self):
return self.rho(self.c)
@@ -1121,12 +1195,16 @@ def __init__(self, c=2.3849, df=4):
self.c = c
self.df = df
- def _set_tuning_param(self, c):
+ def _set_tuning_param(self, c, inplace=False):
"""Set and change the tuning parameter of the Norm.
Warning: this needs to wipe cached attributes that depend on the param.
"""
- self.c = c
+ if inplace:
+ self.c = c
+ return self
+ else:
+ return self.__class__(c=c, df=self.df)
def max_rho(self):
return np.inf
diff --git a/statsmodels/robust/resistant_linear_model.py b/statsmodels/robust/resistant_linear_model.py
new file mode 100644
--- /dev/null
+++ b/statsmodels/robust/resistant_linear_model.py
@@ -0,0 +1,248 @@
+"""
+Created on Apr. 19, 2024 12:17:03 p.m.
+
+Author: Josef Perktold
+License: BSD-3
+"""
+
+import numpy as np
+from statsmodels.base.model import Model
+from statsmodels.regression.linear_model import OLS
+from statsmodels.tools.testing import Holder
+
+from statsmodels.robust.robust_linear_model import RLM
+import statsmodels.robust.norms as rnorms
+import statsmodels.robust.scale as rscale
+from statsmodels.robust.covariance import _get_detcov_startidx
+
+
+class RLMDetS(Model):
+ """S-estimator for linear model with deterministic starts.
+
+
+ Notes
+ -----
+ This estimator combines the method of Fast-S regression (Saliban-Barrera
+ et al 2006) using starting sets similar to the deterministic estimation of
+ multivariate location and scatter DetS and DetMM of Hubert et al (2012).
+
+
+ References
+ ----------
+
+ .. [1] Hubert, Mia, Peter J. Rousseeuw, and Tim Verdonck. 2012. “A
+ Deterministic Algorithm for Robust Location and Scatter.” Journal of
+ Computational and Graphical Statistics 21 (3): 618–37.
+ https://doi.org/10.1080/10618600.2012.672100.
+
+ .. [2] Hubert, Mia, Peter Rousseeuw, Dina Vanpaemel, and Tim Verdonck.
+ 2015. “The DetS and DetMM Estimators for Multivariate Location and
+ Scatter.” Computational Statistics & Data Analysis 81 (January): 64–75.
+ https://doi.org/10.1016/j.csda.2014.07.013.
+
+ .. [3] Rousseeuw, Peter J., Stefan Van Aelst, Katrien Van Driessen, and
+ Jose Agulló. 2004. “Robust Multivariate Regression.”
+ Technometrics 46 (3): 293–305.
+
+ .. [4] Salibian-Barrera, Matías, and Víctor J. Yohai. 2006. “A Fast
+ Algorithm for S-Regression Estimates.” Journal of Computational and
+ Graphical Statistics 15 (2): 414–27.
+
+
+ """
+
+ def __init__(self, endog, exog, norm=None, breakdown_point=0.5,
+ col_indices=None, include_endog=False):
+ super().__init__(endog, exog)
+
+ if norm is None:
+ norm = rnorms.TukeyBiweight()
+
+ tune = norm.get_tuning(bp=breakdown_point)
+ c = tune[0]
+ scale_bias = tune[2]
+ norm = norm._set_tuning_param(c, inplace=False)
+ self.mscale = rscale.MScale(norm, scale_bias)
+
+ self.norm = norm
+ self.breakdown_point = breakdown_point
+
+ # TODO: detect constant
+ if col_indices is None:
+ exog_start = self.exog[:, 1:]
+ else:
+ exog_start = self.exog[:, col_indices]
+
+ # data for robust mahalanobis distance of starting sets
+ if include_endog:
+ self.data_start = np.column_stack((endog, exog_start))
+ else:
+ self.data_start = exog_start
+
+ def _get_start_params(self, h):
+ # I think we should use iterator with yield
+
+ if self.data_start.shape[1] == 0 and self.exog.shape[1] == 1:
+ quantiles = np.quantile(self.endog, [0.25, 0.5, 0.75])
+ start_params_all = [np.atleast_1d([q]) for q in quantiles]
+ return start_params_all
+
+ starts = _get_detcov_startidx(
+ self.data_start, h, options_start=None, methods_cov="all")
+
+ start_params_all = [
+ OLS(self.endog[idx], self.exog[idx]).fit().params
+ for (idx, method) in starts
+ ]
+ return start_params_all
+
+ def _fit_one(self, start_params, maxiter=100):
+ mod = RLM(self.endog, self.exog, M=self.norm)
+ res = mod.fit(start_params=start_params,
+ scale_est=self.mscale,
+ maxiter=maxiter)
+ return res
+
+ def fit(self, h, maxiter=100, maxiter_step=5, start_params_extra=None):
+
+ start_params_all = self._get_start_params(h)
+ if start_params_extra:
+ start_params_all.extend(start_params_extra)
+ res = {}
+ for ii, sp in enumerate(start_params_all):
+ res_ii = self._fit_one(sp, maxiter=maxiter_step)
+ res[ii] = Holder(
+ scale=res_ii.scale,
+ params=res_ii.params,
+ method=ii, # method # TODO need start set method
+ )
+
+ scale_all = np.array([i.scale for i in res.values()])
+ scale_sorted = np.argsort(scale_all)
+ best_idx = scale_sorted[0]
+
+ # TODO: iterate until convergence if start fits are not converged
+ res_best = self._fit_one(res[best_idx].params, maxiter=maxiter)
+
+ # TODO: add extra start and convergence info
+ res_best._results.results_iter = res
+ # results instance of _fit_once has RLM as `model`
+ res_best.model_dets = self
+ return res_best
+
+
+class RLMDetSMM(RLMDetS):
+ """MM-estimator with S-estimator starting values.
+
+ Parameters
+ ----------
+ endog : array-like, 1-dim
+ Dependent, endogenous variable.
+ exog array-like, 1-dim
+ Inependent, exogenous regressor variables.
+ norm : robust norm
+ Redescending robust norm used for S- and MM-estimation.
+ Default is TukeyBiweight.
+ efficiency : float in (0, 1)
+ Asymptotic efficiency of the MM-estimator (used in second stage).
+ breakdown_point : float in (0, 0.5)
+ Breakdown point of the preliminary S-estimator.
+ col_indices : None or array-like of ints
+ Index of columns of exog to use in the mahalanobis distance computation
+ for the starting sets of the S-estimator.
+ Default is all exog except first column (constant). Todo: will change
+ when we autodetect the constant column
+ include_endog : bool
+ If true, then the endog variable is combined with the exog variables
+ to compute the the mahalanobis distances for the starting sets of the
+ S-estimator.
+
+ """
+ def __init__(self, endog, exog, norm=None, efficiency=0.95,
+ breakdown_point=0.5, col_indices=None, include_endog=False):
+ super().__init__(
+ endog,
+ exog,
+ norm=norm,
+ breakdown_point=breakdown_point,
+ col_indices=col_indices,
+ include_endog=include_endog
+ )
+
+ self.efficiency = efficiency
+ if norm is None:
+ norm = rnorms.TukeyBiweight()
+
+ c = norm.get_tuning(eff=efficiency)[0]
+ norm = norm._set_tuning_param(c, inplace=False)
+ self.norm_mean = norm
+
+ def fit(self, h=None, scale_binding=False, start=None):
+ """Estimate the model
+
+ Parameters
+ ----------
+ h : int
+ The size of the initial sets for the S-estimator.
+ Default is .... (todo)
+ scale_binding : bool
+ If true, then the scale is fixed in the second stage M-estimation,
+ i.e. this is the MM-estimator.
+ If false, then the high breakdown point M-scale is used also in the
+ second stage M-estimation if that estimated scale is smaller than
+ the scale of the preliminary, first stage S-estimato.
+ start : tuple or None
+ If None, then the starting parameters and scale for the second
+ stage M-estimation are taken from the fist stage S-estimator.
+ Alternatively, the starting parameters and starting scale can be
+ provided by the user as tuple (start_params, start_scale). In this
+ case the first stage S-estimation in skipped.
+ maxiter, other optimization parameters are still missing (todo)
+
+ Returns
+ -------
+ results instance
+
+ Notes
+ -----
+ If scale_binding is false, then the estimator is a standard
+ MM-estimator with fixed scale in the second stage M-estimation.
+ If scale_binding is true, then the estimator will try to find an
+ estimate with lower M-scale using the same scale-norm rho as in the
+ first stage S-estimator. If the estimated scale, is not smaller than
+ then the scale estimated in the first stage S-estimator, then the
+ fixed scale MM-estimator is returned.
+
+
+ """
+ norm_m = self.norm_mean
+ if start is None:
+ res_s = super().fit(h)
+ start_params = np.asarray(res_s.params)
+ start_scale = res_s.scale
+ else:
+ start_params, start_scale = start
+ res_s = None
+
+ mod_m = RLM(self.endog, self.exog, M=norm_m)
+ res_mm = mod_m.fit(
+ start_params=start_params,
+ start_scale=start_scale,
+ update_scale=False
+ )
+
+ if not scale_binding:
+ # we can compute this first and skip MM if scale decrease
+ mod_sm = RLM(self.endog, self.exog, M=norm_m)
+ res_sm = mod_sm.fit(
+ start_params=start_params,
+ scale_est=self.mscale
+ )
+
+ if not scale_binding and res_sm.scale < res_mm.scale:
+ res = res_sm
+ else:
+ res = res_mm
+
+ res._results.results_dets = res_s
+ return res
diff --git a/statsmodels/robust/robust_linear_model.py b/statsmodels/robust/robust_linear_model.py
--- a/statsmodels/robust/robust_linear_model.py
+++ b/statsmodels/robust/robust_linear_model.py
@@ -263,6 +263,7 @@ def fit(self, maxiter=50, tol=1e-8, scale_est='mad', init=None, cov='H1',
wls_results = lm.WLS(self.endog, self.exog).fit()
else:
start_params = np.asarray(start_params, dtype=np.double).squeeze()
+ start_params = np.atleast_1d(start_params)
if (start_params.shape[0] != self.exog.shape[1] or
start_params.ndim != 1):
raise ValueError('start_params must by a 1-d array with {} '
@@ -276,6 +277,8 @@ def fit(self, maxiter=50, tol=1e-8, scale_est='mad', init=None, cov='H1',
self.scale = self._estimate_scale(wls_results.resid)
elif start_scale:
self.scale = start_scale
+ if not update_scale:
+ self.scale_est = scale_est = "fixed"
history = dict(params=[np.inf], scale=[])
if conv == 'coefs':
diff --git a/statsmodels/robust/scale.py b/statsmodels/robust/scale.py
--- a/statsmodels/robust/scale.py
+++ b/statsmodels/robust/scale.py
@@ -426,6 +426,9 @@ def __init__(self, chi_func, scale_bias):
self.chi_func = chi_func
self.scale_bias = scale_bias
+ def __repr__(self):
+ return repr(self.chi_func)
+
def __call__(self, data, **kwds):
return self.fit(data, **kwds)
diff --git a/statsmodels/robust/tools.py b/statsmodels/robust/tools.py
--- a/statsmodels/robust/tools.py
+++ b/statsmodels/robust/tools.py
@@ -4,7 +4,7 @@
Author: Josef Perktold
License: BSD-3
"""
-
+# flake8: noqa E731
import numpy as np
from scipy import stats, integrate, optimize
@@ -57,8 +57,8 @@ def _var_normal(norm):
"""
- num = stats.norm.expect(lambda x: norm.psi(x)**2)
- denom = stats.norm.expect(lambda x: norm.psi_deriv(x))**2
+ num = stats.norm.expect(lambda x: norm.psi(x)**2) #noqa E731
+ denom = stats.norm.expect(lambda x: norm.psi_deriv(x))**2 #noqa E731
return num / denom
@@ -100,7 +100,7 @@ def _var_normal_jump(norm):
"""
- num = stats.norm.expect(lambda x: norm.psi(x)**2)
+ num = stats.norm.expect(lambda x: norm.psi(x)**2) #noqa E731
def func(x):
# derivative normal pdf
@@ -142,7 +142,6 @@ def _get_tuning_param(norm, eff, kwd="c", kwargs=None, use_jump=False,
efficiency.
"""
- kwds = {} if kwargs is None else kwargs
if bracket is None:
bracket = [0.1, 10]
@@ -150,12 +149,12 @@ def _get_tuning_param(norm, eff, kwd="c", kwargs=None, use_jump=False,
def func(c):
# kwds.update({kwd: c})
# return _var_normal(norm(**kwds)) - 1 / eff
- norm._set_tuning_param(c)
+ norm._set_tuning_param(c, inplace=True)
return _var_normal(norm) - 1 / eff
else:
def func(c):
- norm._set_tuning_param(c)
- return _var_normal_jump(norm(**kwds) - 1 / eff)
+ norm._set_tuning_param(c, inplace=True)
+ return _var_normal_jump(norm) - 1 / eff
res = optimize.brentq(func, *bracket)
return res
@@ -215,14 +214,15 @@ def tuning_s_estimator_mean(norm, breakdown=None):
def func(c):
norm_ = norm
- norm_._set_tuning_param(c)
- bp = stats.norm.expect(lambda x : norm_.rho(x)) / norm_.max_rho()
+ norm_._set_tuning_param(c, inplace=True)
+ bp = (stats.norm.expect(lambda x : norm_.rho(x)) / #noqa E731
+ norm_.max_rho())
return bp
res = []
for bp in bps:
- c_bp = optimize.brentq(lambda c0: func(c0) - bp, 0.1, 10)
- norm._set_tuning_param(c_bp) # inplace modification
+ c_bp = optimize.brentq(lambda c0: func(c0) - bp, 0.1, 10) #noqa E731
+ norm._set_tuning_param(c_bp, inplace=True) # inplace modification
eff = 1 / _var_normal(norm)
b = stats.norm.expect(lambda x : norm.rho(x))
res.append([bp, eff, c_bp, b])
@@ -242,3 +242,321 @@ def func(c):
)
return res2
+
+
+def scale_bias_cov_biw(c, k_vars):
+ """Multivariate scale bias correction for TukeyBiweight norm.
+
+ This uses the chisquare distribution as reference distribution for the
+ squared Mahalanobis distance.
+ """
+ p = k_vars # alias for formula
+ chip, chip2, chip4, chip6 = stats.chi2.cdf(c**2, [p, p + 2, p + 4, p + 6])
+ b = p / 2 * chip2 - p * (p + 2) / (2 * c**2) * chip4
+ b += p * (p + 2) * (p + 4) / (6 * c**4) * chip6 + c**2 / 6 * (1 - chip)
+ return b, b / (c**2 / 6)
+
+
+def scale_bias_cov(norm, k_vars):
+ """Multivariate scale bias correction.
+
+
+ Parameter
+ ---------
+ norm : norm instance
+ The rho function of the norm is used in the moment condition for
+ estimating scale.
+ k_vars : int
+ Number of random variables in the multivariate data.
+
+ Returns
+ -------
+ scale_bias: float
+ breakdown_point : float
+ Breakdown point computed as scale bias divided by max rho.
+ """
+
+ rho = lambda x: (norm.rho(np.sqrt(x))) # noqa
+ scale_bias = stats.chi2.expect(rho, args=(k_vars,))
+ return scale_bias, scale_bias / norm.max_rho()
+
+
+def tuning_s_cov(norm, k_vars, breakdown_point=0.5, limits=()):
+ """Tuning parameter for multivariate S-estimator given breakdown point.
+ """
+ from .norms import TukeyBiweight # avoid circular import
+
+ if not limits:
+ limits = (0.5, 30)
+
+ if isinstance(norm, TukeyBiweight):
+ def func(c):
+ return scale_bias_cov_biw(c, k_vars)[1] - breakdown_point
+ else:
+ norm = norm._set_tuning_param(2., inplace=False) # create copy
+
+ def func(c):
+ norm._set_tuning_param(c, inplace=True)
+ return scale_bias_cov(norm, k_vars)[1] - breakdown_point
+
+ p_tune = optimize.brentq(func, limits[0], limits[1])
+ return p_tune
+
+
+def eff_mvmean(norm, k_vars):
+ """Efficiency for M-estimator of multivariate mean at normal distribution.
+
+ This also applies to estimators that are locally equivalent to an
+ M-estimator such as S- and MM-estimators.
+
+ Parameters
+ ----------
+ norm : instance of norm class
+ k_vars : int
+ Number of variables in multivariate random variable, i.e. dimension.
+
+ Returns
+ -------
+ eff : float
+ Asymptotic relative efficiency of mean at normal distribution.
+ alpha : float
+ Numerical integral. Efficiency is beta**2 / alpha
+ beta : float
+ Numerical integral.
+
+ Notes
+ -----
+ This implements equ. (5.3) p. 1671 in Lopuhaä 1989
+
+ References
+ ----------
+
+ .. [1] Lopuhaä, Hendrik P. 1989. “On the Relation between S-Estimators
+ and M-Estimators of Multivariate Location and Covariance.”
+ The Annals of Statistics 17 (4): 1662–83.
+
+ """
+ k = k_vars # shortcut
+ f_alpha = lambda d: norm.psi(d)**2 / k
+ f_beta = lambda d: ((1 - 1 / k) * norm.weights(d) +
+ 1 / k * norm.psi_deriv(d))
+ alpha = stats.chi(k).expect(f_alpha)
+ beta = stats.chi(k).expect(f_beta)
+ return beta**2 / alpha, alpha, beta
+
+
+def eff_mvshape(norm, k_vars):
+ """Efficiency of M-estimator of multivariate shape at normal distribution.
+
+ This also applies to estimators that are locally equivalent to an
+ M-estimator such as S- and MM-estimators.
+
+ Parameters
+ ----------
+ norm : instance of norm class
+ k_vars : int
+ Number of variables in multivariate random variable, i.e. dimension.
+
+ Returns
+ -------
+ eff : float
+ Asymptotic relative efficiency of mean at normal distribution.
+ alpha : float
+ Numerical integral. Efficiency is beta**2 / alpha
+ beta : float
+ Numerical integral.
+
+ Notes
+ -----
+ This implements sigma_1 in equ. (5.5) p. 1671 in Lopuhaä 1989.
+ Efficiency of shape is approximately 1 / sigma1.
+
+ References
+ ----------
+
+ .. [1] Lopuhaä, Hendrik P. 1989. “On the Relation between S-Estimators
+ and M-Estimators of Multivariate Location and Covariance.”
+ The Annals of Statistics 17 (4): 1662–83.
+
+ """
+
+ k = k_vars # shortcut
+ f_a = lambda d: k * (k + 2) * norm.psi(d)**2 * d**2
+ f_b = lambda d: norm.psi_deriv(d) * d**2 + (k + 1) * norm.psi(d) * d
+ a = stats.chi(k).expect(f_a)
+ b = stats.chi(k).expect(f_b)
+ return b**2 / a, a, b
+
+
+def tuning_m_cov_eff(norm, k_vars, efficiency=0.95, eff_mean=True, limits=()):
+ """Tuning parameter for multivariate M-estimator given efficiency.
+
+ This also applies to estimators that are locally equivalent to an
+ M-estimator such as S- and MM-estimators.
+
+ Parameters
+ ----------
+ norm : instance of norm class
+ k_vars : int
+ Number of variables in multivariate random variable, i.e. dimension.
+ efficiency : float < 1
+ Desired asymptotic relative efficiency of mean estimator.
+ Default is 0.95.
+ eff_mean : bool
+ If eff_mean is true (default), then tuning parameter is to achieve
+ efficiency of mean estimate.
+ If eff_mean is fale, then tuning parameter is to achieve efficiency
+ of shape estimate.
+ limits : tuple
+ Limits for rootfinding with scipy.optimize.brentq.
+ In some cases the interval limits for rootfinding can be too small
+ and not cover the root. Current default limits are (0.5, 30).
+
+ Returns
+ -------
+ float : Tuning parameter for the norm to achieve desired efficiency.
+ Asymptotic relative efficiency of mean at normal distribution.
+
+ Notes
+ -----
+ This uses numerical integration and rootfinding and will be
+ relatively slow.
+ """
+ if not limits:
+ limits = (0.5, 30)
+
+ # make copy of norm
+ norm = norm._set_tuning_param(1, inplace=False)
+
+ if eff_mean:
+ def func(c):
+ norm._set_tuning_param(c, inplace=True)
+ return eff_mvmean(norm, k_vars)[0] - efficiency
+ else:
+ def func(c):
+ norm._set_tuning_param(c, inplace=True)
+ return eff_mvshape(norm, k_vars)[0] - efficiency
+
+ p_tune = optimize.brentq(func, limits[0], limits[1])
+ return p_tune
+
+
+# ##### tables
+
+tukeybiweight_bp = {
+ # breakdown point : (tuning parameter, efficiency, scale bias)
+ 0.50: (1.547645, 0.286826, 0.199600),
+ 0.45: (1.756059, 0.369761, 0.231281),
+ 0.40: (1.987965, 0.461886, 0.263467),
+ 0.35: (2.251831, 0.560447, 0.295793),
+ 0.30: (2.560843, 0.661350, 0.327896),
+ 0.25: (2.937015, 0.759040, 0.359419),
+ 0.20: (3.420681, 0.846734, 0.390035),
+ 0.15: (4.096255, 0.917435, 0.419483),
+ 0.10: (5.182361, 0.966162, 0.447614),
+ 0.05: (7.545252, 0.992424, 0.474424),
+ }
+
+tukeybiweight_eff = {
+ # efficiency : (tuning parameter, breakdown point)
+ 0.65: (2.523102, 0.305646),
+ 0.70: (2.697221, 0.280593),
+ 0.75: (2.897166, 0.254790),
+ 0.80: (3.136909, 0.227597),
+ 0.85: (3.443690, 0.197957),
+ 0.90: (3.882662, 0.163779),
+ 0.95: (4.685065, 0.119414),
+ 0.97: (5.596823, 0.087088),
+ 0.98: (5.920719, 0.078604),
+ 0.99: (7.041392, 0.056969),
+ }
+
+# relative efficiency for M and MM estimator of multivariate location
+# Table 2 from Kudraszow and Maronna JMA 2011
+# k in [1, 2, 3, 4, 5, 10], eff in [0.8, 0.9, 0.95
+# TODO: need to replace with more larger table and more digits.
+# (4, 0.95): 5.76 -. 5.81 to better match R rrcov and numerical integration
+tukeybiweight_mvmean_eff_km = {
+ (1, 0.8): 3.14, (2, 0.8): 3.51, (3, 0.8): 3.82, (4, 0.8): 4.1,
+ (5, 0.8): 4.34, (10, 0.8): 5.39,
+ (1, 0.9): 3.88, (2, 0.9): 4.28, (3, 0.9): 4.62, (4, 0.9): 4.91,
+ (5, 0.9): 5.18, (10, 0.9): 6.38,
+ (1, 0.95): 4.68, (2, 0.95): 5.12, (3, 0.95): 5.48, (4, 0.95): 5.81,
+ (5, 0.95): 6.1, (10, 0.95): 7.67,
+ }
+
+_table_biweight_mvmean_eff = np.array([
+ [2.89717, 3.13691, 3.44369, 3.88266, 4.68506, 5.59682, 7.04139],
+ [3.26396, 3.51006, 3.82643, 4.2821, 5.12299, 6.0869, 7.62344],
+ [3.5721, 3.82354, 4.14794, 4.61754, 5.49025, 6.49697, 8.10889],
+ [3.84155, 4.09757, 4.42889, 4.91044, 5.81032, 6.85346, 8.52956],
+ [4.08323, 4.34327, 4.68065, 5.17267, 6.09627, 7.17117, 8.90335],
+ [4.30385, 4.56746, 4.91023, 5.41157, 6.35622, 7.45933, 9.24141],
+ [4.50783, 4.77466, 5.12228, 5.63199, 6.59558, 7.72408, 9.5512],
+ [4.69828, 4.96802, 5.32006, 5.83738, 6.81817, 7.96977, 9.83797],
+ [4.87744, 5.14986, 5.506, 6.03022, 7.02679, 8.19958, 10.10558],
+ [5.04704, 5.32191, 5.68171, 6.21243, 7.22354, 8.41594, 10.35696],
+ [5.20839, 5.48554, 5.84879, 6.38547, 7.41008, 8.62071, 10.59439],
+ [5.36254, 5.64181, 6.00827, 6.55051, 7.58772, 8.81538, 10.81968],
+ [5.51034, 5.7916, 6.16106, 6.7085, 7.75752, 9.00118, 11.03428],
+ [5.65249, 5.9356, 6.3079, 6.86021, 7.92034, 9.17908, 11.23939],
+ ])
+
+_table_biweight_mvshape_eff = np.array([
+ [3.57210, 3.82354, 4.14794, 4.61754, 5.49025, 6.49697, 8.10889],
+ [3.84155, 4.09757, 4.42889, 4.91044, 5.81032, 6.85346, 8.52956],
+ [4.08323, 4.34327, 4.68065, 5.17267, 6.09627, 7.17117, 8.90335],
+ [4.30385, 4.56746, 4.91023, 5.41157, 6.35622, 7.45933, 9.24141],
+ [4.50783, 4.77466, 5.12228, 5.63199, 6.59558, 7.72408, 9.55120],
+ [4.69828, 4.96802, 5.32006, 5.83738, 6.81817, 7.96977, 9.83797],
+ [4.87744, 5.14986, 5.50600, 6.03022, 7.02679, 8.19958, 10.10558],
+ [5.04704, 5.32191, 5.68171, 6.21243, 7.22354, 8.41594, 10.35696],
+ [5.20839, 5.48554, 5.84879, 6.38547, 7.41008, 8.62071, 10.59439],
+ [5.36254, 5.64181, 6.00827, 6.55051, 7.58772, 8.81538, 10.81968],
+ [5.51034, 5.79160, 6.16106, 6.70849, 7.75752, 9.00118, 11.03428],
+ [5.65249, 5.93560, 6.30790, 6.86021, 7.92034, 9.17908, 11.23939],
+ [5.78957, 6.07443, 6.44938, 7.00630, 8.07692, 9.34991, 11.43603],
+ [5.92206, 6.20858, 6.58604, 7.14731, 8.22785, 9.51437, 11.62502],
+ ])
+
+
+def _convert_to_dict_mvmean_effs(eff_mean=True):
+ effs_mvmean = [0.75, 0.8, 0.85, 0.9, 0.95, 0.975, 0.99]
+ ks = list(range(1, 15))
+ if eff_mean:
+ table = _table_biweight_mvmean_eff
+ else:
+ table = _table_biweight_mvshape_eff
+ tp = {}
+ for i, k in enumerate(ks):
+ for j, eff in enumerate(effs_mvmean):
+ tp[(k, eff)] = table[i, j]
+
+ return tp
+
+
+tukeybiweight_mvmean_eff_d = _convert_to_dict_mvmean_effs(eff_mean=True)
+tukeybiweight_mvshape_eff_d = _convert_to_dict_mvmean_effs(eff_mean=False)
+
+
+def tukeybiweight_mvmean_eff(k, eff, eff_mean=True):
+ """tuning parameter for biweight norm to achieve efficiency for mv-mean.
+
+ Uses values from precomputed table if available, otherwise computes it
+ numerically and adds it to the module global dict.
+
+ """
+ if eff_mean:
+ table_dict = tukeybiweight_mvmean_eff_d
+ else:
+ table_dict = tukeybiweight_mvshape_eff_d
+
+ try:
+ tp = table_dict[(k, eff)]
+ except KeyError:
+ # compute and cache
+ from .norms import TukeyBiweight # avoid circular import
+ norm = TukeyBiweight(c=1)
+ tp = tuning_m_cov_eff(norm, k, efficiency=eff, eff_mean=eff_mean)
+ table_dict[(k, eff)] = tp
+ return tp
diff --git a/statsmodels/stats/covariance.py b/statsmodels/stats/covariance.py
--- a/statsmodels/stats/covariance.py
+++ b/statsmodels/stats/covariance.py
@@ -149,6 +149,18 @@ def transform_corr_normal(corr, method, return_var=False, possdef=True):
return corr_n
+def corr_rank(data):
+ """Spearman rank correlation
+
+ simplified version of scipy.stats.spearmanr
+ """
+ x = np.asarray(data)
+ axisout = 0
+ ar = np.apply_along_axis(stats.rankdata, axisout, x)
+ corr = np.corrcoef(ar, rowvar=False)
+ return corr
+
+
def corr_normal_scores(data):
"""Gaussian rank (normal scores) correlation
| diff --git a/statsmodels/robust/tests/test_covariance.py b/statsmodels/robust/tests/test_covariance.py
--- a/statsmodels/robust/tests/test_covariance.py
+++ b/statsmodels/robust/tests/test_covariance.py
@@ -6,6 +6,7 @@
import pandas as pd
from statsmodels import robust
+import statsmodels.robust.norms as robnorms
import statsmodels.robust.covariance as robcov
import statsmodels.robust.scale as robscale
@@ -177,6 +178,121 @@ def test_tyler():
assert res1.n_iter == 55
+def test_cov_ms():
+ # use CovM as local CovS
+
+ # > CovSest(x) using package rrcov
+ # same result with > CovSest(x, method="sdet")
+ # but scale difers with method="biweight"
+ mean_r = np.array([1.53420879676, 1.82865741024, 1.65565146981])
+ cov_r = np.array([
+ [1.8090846049573, 0.0479283121828, 0.2446369025717],
+ [0.0479283121828, 1.8189886310494, 0.2513025527579],
+ [0.2446369025717, 0.2513025527579, 1.7287983150484],
+ ])
+
+ scale2_r = np.linalg.det(cov_r) ** (1/3)
+ shape_r = cov_r / scale2_r
+ scale_r = np.sqrt(scale2_r)
+
+ exog_df = dta_hbk[["X1", "X2", "X3"]]
+ mod = robcov.CovM(exog_df)
+ # with default start, default start could get wrong local optimum
+ res = mod.fit()
+ assert_allclose(res.mean, mean_r, rtol=1e-5)
+ assert_allclose(res.shape, shape_r, rtol=1e-5)
+ assert_allclose(res.cov, cov_r, rtol=1e-5)
+ assert_allclose(res.scale, scale_r, rtol=1e-5)
+
+ # with results start
+ res = mod.fit(start_mean=mean_r, start_shape=shape_r, start_scale=scale_r)
+ assert_allclose(res.mean, mean_r, rtol=1e-5)
+ assert_allclose(res.shape, shape_r, rtol=1e-5)
+ assert_allclose(res.cov, cov_r, rtol=1e-5)
+ assert_allclose(res.scale, scale_r, rtol=1e-5)
+
+ mod_s = robcov.CovDetS(exog_df)
+ res = mod_s.fit()
+ assert_allclose(res.mean, mean_r, rtol=1e-5)
+ assert_allclose(res.shape, shape_r, rtol=1e-5)
+ assert_allclose(res.cov, cov_r, rtol=1e-5)
+ assert_allclose(res.scale, scale_r, rtol=1e-5)
+
+
+def test_covdetmcd():
+
+ # results from rrcov
+ # > cdet = CovMcd(x = hbk, raw.only = TRUE, nsamp = "deterministic",
+ # use.correction=FALSE)
+ cov_dmcd_r = np.array("""
+ 2.2059619213639 0.0223939863695 0.7898958050933 0.4060613360808
+ 0.0223939863695 1.1384166802155 0.4315534571891 -0.2344041030201
+ 0.7898958050933 0.4315534571891 1.8930117467493 -0.3292893001459
+ 0.4060613360808 -0.2344041030201 -0.3292893001459 0.6179686100845
+ """.split(), float).reshape(4, 4)
+
+ mean_dmcd_r = np.array([1.7725, 2.2050, 1.5375, -0.0575])
+
+ mod = robcov.CovDetMCD(dta_hbk)
+ res = mod.fit(40, maxiter_step=100, reweight=False)
+ assert_allclose(res.mean, mean_dmcd_r, rtol=1e-5)
+ assert_allclose(res.cov, cov_dmcd_r, rtol=1e-5)
+
+ # with reweighting
+ # covMcd(x = hbk, nsamp = "deterministic", use.correction = FALSE)
+ # iBest: 5; C-step iterations: 7, 7, 7, 4, 6, 6
+ # Log(Det.): -2.42931967153
+
+ mean_dmcdw_r = np.array([1.5338983050847, 1.8322033898305, 1.6745762711864,
+ -0.0728813559322])
+ cov_dmcdw_r = np.array("""
+ 1.5677744869295 0.09285770205078 0.252076010128 0.13873444408300
+ 0.0928577020508 1.56769177397171 0.224929617385 -0.00516128856542
+ 0.2520760101278 0.22492961738467 1.483829106079 -0.20275013775619
+ 0.1387344440830 -0.00516128856542 -0.202750137756 0.43326701543885
+ """.split(), float).reshape(4, 4)
+
+ mod = robcov.CovDetMCD(dta_hbk)
+ res = mod.fit(40, maxiter_step=100) # default is reweight=True
+ assert_allclose(res.mean, mean_dmcdw_r, rtol=1e-5)
+ # R uses different trimming correction
+ # compare only shape (using trace for simplicity)
+ shape = res.cov / np.trace(res.cov)
+ shape_r = cov_dmcdw_r / np.trace(cov_dmcdw_r)
+ assert_allclose(shape, shape_r, rtol=1e-5)
+
+
+def test_covdetmm():
+
+ # results from rrcov
+ # CovMMest(x = hbk, eff.shape=FALSE,
+ # control=CovControlMMest(sest=CovControlSest(method="sdet")))
+ cov_dmm_r = np.array("""
+ 1.72174266670826 0.06925842715939 0.20781848922667 0.10749343153015
+ 0.06925842715939 1.74566218886362 0.22161135221404 -0.00517023660647
+ 0.20781848922667 0.22161135221404 1.63937749762534 -0.17217102475913
+ 0.10749343153015 -0.00517023660647 -0.17217102475913 0.48174480967136
+ """.split(), float).reshape(4, 4)
+
+ mean_dmm_r = np.array([1.5388643420460, 1.8027582110408, 1.6811517253521,
+ -0.0755069488908])
+
+ # using same c as rrcov
+ c = 5.81031555752526
+ mod = robcov.CovDetMM(dta_hbk, norm=robnorms.TukeyBiweight(c=c))
+ res = mod.fit()
+
+ assert_allclose(res.mean, mean_dmm_r, rtol=1e-5)
+ assert_allclose(res.cov, cov_dmm_r, rtol=1e-5, atol=1e-5)
+
+ # using c from table,
+ mod = robcov.CovDetMM(dta_hbk)
+ res = mod.fit()
+
+ assert_allclose(res.mean, mean_dmm_r, rtol=1e-3)
+ assert_allclose(res.cov, cov_dmm_r, rtol=1e-3, atol=1e-3)
+
+
def test_robcov_SMOKE():
# currently only smoke test or very loose comparisons to dgp
nobs, k_vars = 100, 3
diff --git a/statsmodels/robust/tests/test_tools.py b/statsmodels/robust/tests/test_tools.py
--- a/statsmodels/robust/tests/test_tools.py
+++ b/statsmodels/robust/tests/test_tools.py
@@ -49,24 +49,26 @@
@pytest.mark.parametrize("case", results_menenez)
def test_eff(case):
norm, res2 = case
+ use_jump = False
if norm.continuous == 2:
var_func = _var_normal
else:
var_func = _var_normal_jump
+ use_jump = True
res_eff = []
for c in res2:
- norm._set_tuning_param(c)
+ norm._set_tuning_param(c, inplace=True)
res_eff.append(1 / var_func(norm))
assert_allclose(res_eff, effs, atol=0.0005)
for c in res2:
# bp = stats.norm.expect(lambda x : norm.rho(x)) / norm.rho(norm.c)
- norm._set_tuning_param(c)
- eff = 1 / _var_normal(norm)
- tune = _get_tuning_param(norm, eff)
+ norm._set_tuning_param(c, inplace=True)
+ eff = 1 / var_func(norm)
+ tune = _get_tuning_param(norm, eff, use_jump=use_jump)
assert_allclose(tune, c, rtol=1e-6, atol=5e-4)
| BUG: RLM fit with start_params raises if only one parameter
if `start_params = np.array([5])` one element, 1-dim array, then the squeeze removes the 1-dim which then raises exception in shape check
```
statsmodels_gh\statsmodels\statsmodels\robust\robust_linear_model.py in fit(self, maxiter, tol, scale_est, init, cov, update_scale, conv, start_params, start_scale)
264 else:
265 start_params = np.asarray(start_params, dtype=np.double).squeeze()
--> 266 if (start_params.shape[0] != self.exog.shape[1] or
267 start_params.ndim != 1):
268 raise ValueError('start_params must by a 1-d array with {} '
IndexError: tuple index out of range
```
I ran into this for the constant only regression for resistant estimators in #9227
I don't know why there is the squeeze.
If we want or need to keep the squeeze, then a fix is to add
`start_params = np.atleast_1d(start_params),`
I will fix it this way in my PR, where I need it now
| "2024-04-22T15:56:21Z" | 0.14 | [
"statsmodels/robust/tests/test_covariance.py::TestOGKTau::test",
"statsmodels/robust/tests/test_covariance.py::TestOGKMad::test",
"statsmodels/robust/tests/test_covariance.py::test_mahalanobis",
"statsmodels/robust/tests/test_covariance.py::test_outliers_gy",
"statsmodels/robust/tests/test_covariance.py::test_robcov_SMOKE",
"statsmodels/robust/tests/test_covariance.py::test_tyler",
"statsmodels/robust/tests/test_tools.py::test_tuning_smoke[case3]",
"statsmodels/robust/tests/test_tools.py::test_tuning_smoke[case2]",
"statsmodels/robust/tests/test_tools.py::test_tuning_smoke[case1]",
"statsmodels/robust/tests/test_tools.py::test_tuning_smoke[case7]",
"statsmodels/robust/tests/test_tools.py::test_tuning_smoke[case5]",
"statsmodels/robust/tests/test_tools.py::test_tuning_smoke[case0]",
"statsmodels/robust/tests/test_tools.py::test_tuning_smoke[case4]",
"statsmodels/robust/tests/test_tools.py::test_tuning_biweight",
"statsmodels/robust/tests/test_tools.py::test_tuning_smoke[case6]",
"statsmodels/robust/tests/test_tools.py::test_hampel_eff"
] | [
"statsmodels/robust/tests/test_covariance.py::test_covdetmcd",
"statsmodels/robust/tests/test_covariance.py::test_cov_ms",
"statsmodels/robust/tests/test_covariance.py::test_covdetmm",
"statsmodels/robust/tests/test_tools.py::test_eff[case5]",
"statsmodels/robust/tests/test_tools.py::test_eff[case1]",
"statsmodels/robust/tests/test_tools.py::test_eff[case6]",
"statsmodels/robust/tests/test_tools.py::test_eff[case0]",
"statsmodels/robust/tests/test_tools.py::test_eff[case2]",
"statsmodels/robust/tests/test_tools.py::test_eff[case4]",
"statsmodels/robust/tests/test_tools.py::test_eff[case3]",
"statsmodels/robust/tests/test_tools.py::test_eff[case7]"
] | Python | [] | [] |
|
statsmodels/statsmodels | 9,240 | statsmodels__statsmodels-9240 | [
"9121"
] | c22837f0632ae8890f56886460c429ebf356bd9b | diff --git a/statsmodels/stats/diagnostic.py b/statsmodels/stats/diagnostic.py
--- a/statsmodels/stats/diagnostic.py
+++ b/statsmodels/stats/diagnostic.py
@@ -237,8 +237,9 @@ def compare_j(results_x, results_z, store=False):
return tstat, pval
+@deprecate_kwarg("cov_kwargs", "cov_kwds")
def compare_encompassing(results_x, results_z, cov_type="nonrobust",
- cov_kwargs=None):
+ cov_kwds=None):
r"""
Davidson-MacKinnon encompassing test for comparing non-nested models
@@ -253,7 +254,7 @@ def compare_encompassing(results_x, results_z, cov_type="nonrobust",
OLS covariance estimator. Specify one of "HC0", "HC1", "HC2", "HC3"
to use White's covariance estimator. All covariance types supported
by ``OLS.fit`` are accepted.
- cov_kwargs : dict, default None
+ cov_kwds : dict, default None
Dictionary of covariance options passed to ``OLS.fit``. See OLS.fit
for more details.
@@ -317,8 +318,8 @@ def _test_nested(endog, a, b, cov_est, cov_kwds):
df_num, df_denom = int(test.df_num), int(test.df_denom)
return stat, pvalue, df_num, df_denom
- x_nested = _test_nested(y, x, z, cov_type, cov_kwargs)
- z_nested = _test_nested(y, z, x, cov_type, cov_kwargs)
+ x_nested = _test_nested(y, x, z, cov_type, cov_kwds)
+ z_nested = _test_nested(y, z, x, cov_type, cov_kwds)
return pd.DataFrame([x_nested, z_nested],
index=["x", "z"],
columns=["stat", "pvalue", "df_num", "df_denom"])
@@ -479,9 +480,10 @@ def acorr_ljungbox(x, lags=None, boxpierce=False, model_df=0, period=None,
index=lags)
+@deprecate_kwarg("cov_kwargs", "cov_kwds")
@deprecate_kwarg("maxlag", "nlags")
def acorr_lm(resid, nlags=None, store=False, *, period=None,
- ddof=0, cov_type="nonrobust", cov_kwargs=None):
+ ddof=0, cov_type="nonrobust", cov_kwds=None):
"""
Lagrange Multiplier tests for autocorrelation.
@@ -510,7 +512,7 @@ def acorr_lm(resid, nlags=None, store=False, *, period=None,
OLS covariance estimator. Specify one of "HC0", "HC1", "HC2", "HC3"
to use White's covariance estimator. All covariance types supported
by ``OLS.fit`` are accepted.
- cov_kwargs : dict, default None
+ cov_kwds : dict, default None
Dictionary of covariance options passed to ``OLS.fit``. See OLS.fit for
more details.
@@ -545,8 +547,8 @@ def acorr_lm(resid, nlags=None, store=False, *, period=None,
"""
resid = array_like(resid, "resid", ndim=1)
cov_type = string_like(cov_type, "cov_type")
- cov_kwargs = {} if cov_kwargs is None else cov_kwargs
- cov_kwargs = dict_like(cov_kwargs, "cov_kwargs")
+ cov_kwds = {} if cov_kwds is None else cov_kwds
+ cov_kwds = dict_like(cov_kwds, "cov_kwds")
nobs = resid.shape[0]
if period is not None and nlags is None:
maxlag = min(nobs // 5, 2 * period)
@@ -563,7 +565,7 @@ def acorr_lm(resid, nlags=None, store=False, *, period=None,
usedlag = maxlag
resols = OLS(xshort, xdall[:, :usedlag + 1]).fit(cov_type=cov_type,
- cov_kwargs=cov_kwargs)
+ cov_kwds=cov_kwds)
fval = float(resols.fvalue)
fpval = float(resols.f_pvalue)
if cov_type == "nonrobust":
@@ -985,9 +987,10 @@ def het_goldfeldquandt(y, x, idx=None, split=None, drop=None,
return fval, fpval, ordering
+@deprecate_kwarg("cov_kwargs", "cov_kwds")
@deprecate_kwarg("result", "res")
def linear_reset(res, power=3, test_type="fitted", use_f=False,
- cov_type="nonrobust", cov_kwargs=None):
+ cov_type="nonrobust", cov_kwds=None):
r"""
Ramsey's RESET test for neglected nonlinearity
@@ -1015,7 +1018,7 @@ def linear_reset(res, power=3, test_type="fitted", use_f=False,
OLS covariance estimator. Specify one of "HC0", "HC1", "HC2", "HC3"
to use White's covariance estimator. All covariance types supported
by ``OLS.fit`` are accepted.
- cov_kwargs : dict, default None
+ cov_kwds : dict, default None
Dictionary of covariance options passed to ``OLS.fit``. See OLS.fit
for more details.
@@ -1055,7 +1058,7 @@ def linear_reset(res, power=3, test_type="fitted", use_f=False,
"non-constant column.")
test_type = string_like(test_type, "test_type",
options=("fitted", "exog", "princomp"))
- cov_kwargs = dict_like(cov_kwargs, "cov_kwargs", optional=True)
+ cov_kwds = dict_like(cov_kwds, "cov_kwds", optional=True)
use_f = bool_like(use_f, "use_f")
if isinstance(power, int):
if power < 2:
@@ -1093,8 +1096,8 @@ def linear_reset(res, power=3, test_type="fitted", use_f=False,
aug_exog = np.hstack([exog] + [aug ** p for p in power])
mod_class = res.model.__class__
mod = mod_class(res.model.data.endog, aug_exog)
- cov_kwargs = {} if cov_kwargs is None else cov_kwargs
- res = mod.fit(cov_type=cov_type, cov_kwargs=cov_kwargs)
+ cov_kwds = {} if cov_kwds is None else cov_kwds
+ res = mod.fit(cov_type=cov_type, cov_kwds=cov_kwds)
nrestr = aug_exog.shape[1] - exog.shape[1]
nparams = aug_exog.shape[1]
r_mat = np.eye(nrestr, nparams, k=nparams-nrestr)
| diff --git a/statsmodels/stats/tests/test_diagnostic.py b/statsmodels/stats/tests/test_diagnostic.py
--- a/statsmodels/stats/tests/test_diagnostic.py
+++ b/statsmodels/stats/tests/test_diagnostic.py
@@ -1805,8 +1805,8 @@ def test_encompasing_error(reset_randomstate):
@pytest.mark.parametrize(
"cov",
[
- dict(cov_type="nonrobust", cov_kwargs={}),
- dict(cov_type="HC0", cov_kwargs={}),
+ dict(cov_type="nonrobust", cov_kwds={}),
+ dict(cov_type="HC0", cov_kwds={}),
],
)
def test_reset_smoke(power, test_type, use_f, cov, reset_randomstate):
@@ -1826,8 +1826,8 @@ def test_reset_smoke(power, test_type, use_f, cov, reset_randomstate):
@pytest.mark.parametrize(
"cov",
[
- dict(cov_type="nonrobust", cov_kwargs={}),
- dict(cov_type="HC0", cov_kwargs={}),
+ dict(cov_type="nonrobust", cov_kwds={}),
+ dict(cov_type="HC0", cov_kwds={}),
],
)
def test_acorr_lm_smoke(store, ddof, cov, reset_randomstate):
@@ -1996,3 +1996,36 @@ def test_diagnostics_pandas(reset_randomstate):
res, order_by=np.arange(y.shape[0] - 1, 0 - 1, -1)
)
smsdia.spec_white(res.resid, x)
+
+
+def test_deprecated_argument():
+ x = np.random.randn(100)
+ y = 2 * x + np.random.randn(100)
+ result = OLS(y, add_constant(x)).fit(
+ cov_type="HAC", cov_kwds={"maxlags": 2}
+ )
+ with pytest.warns(FutureWarning, match="the "):
+ smsdia.linear_reset(
+ result,
+ power=2,
+ test_type="fitted",
+ cov_type="HAC",
+ cov_kwargs={"maxlags": 2},
+ )
+
+
+def test_diagnostics_hac(reset_randomstate):
+ x = np.random.randn(100)
+ y = 2 * x + np.random.randn(100)
+ result = OLS(y, add_constant(x)).fit(
+ cov_type="HAC", cov_kwds={"maxlags": 2}
+ )
+ reset_test = smsdia.linear_reset(
+ result,
+ power=2,
+ test_type="fitted",
+ cov_type="HAC",
+ cov_kwds={"maxlags": 2},
+ )
+ assert reset_test.statistic > 0
+ assert 0 <= reset_test.pvalue <= 1
diff --git a/statsmodels/tsa/tests/test_seasonal.py b/statsmodels/tsa/tests/test_seasonal.py
--- a/statsmodels/tsa/tests/test_seasonal.py
+++ b/statsmodels/tsa/tests/test_seasonal.py
@@ -315,17 +315,27 @@ def test_seasonal_decompose_multiple():
@pytest.mark.matplotlib
[email protected]('model', ['additive', 'multiplicative'])
[email protected]('freq', [4, 12])
[email protected]('two_sided', [True, False])
[email protected]('extrapolate_trend', [True, False])
-def test_seasonal_decompose_plot(model, freq, two_sided, extrapolate_trend):
[email protected]("model", ["additive", "multiplicative"])
[email protected]("freq", [4, 12])
[email protected]("two_sided", [True, False])
[email protected]("extrapolate_trend", [True, False])
+def test_seasonal_decompose_plot(
+ model, freq, two_sided, extrapolate_trend, close_figures
+):
x = np.array([-50, 175, 149, 214, 247, 237, 225, 329, 729, 809,
530, 489, 540, 457, 195, 176, 337, 239, 128, 102,
232, 429, 3, 98, 43, -141, -77, -13, 125, 361, -45, 184])
x -= x.min() + 1
x2 = np.r_[x[12:], x[:12]]
x = np.c_[x, x2]
- res = seasonal_decompose(x, period=freq, two_sided=two_sided,
- extrapolate_trend=extrapolate_trend)
- res.plot()
+ res = seasonal_decompose(
+ x,
+ period=freq,
+ two_sided=two_sided,
+ extrapolate_trend=extrapolate_trend
+ )
+ fig = res.plot()
+
+ import matplotlib.pyplot as plt
+
+ plt.close(fig)
| linear_reset can't be set with "HAC" and maxlags, KeyErrors
#### Describe the bug
Hello, thanks for your time. We've tried several things but it still doesn't work even with the newest version of Statsmodels. We think this might be a bug. Issue is: we were trying to use "statsmodels.stats.diagnostic.linear_reset" with HAC as coviance type and maxlags as the key word args, but we got KeyErrors.
#### Code Sample, a copy-pastable example if possible
```
# Import modules
import numpy as np
import statsmodels.api as sm
from statsmodels.stats.diagnostic import linear_reset
# Generate some random data
np.random.seed(42)
x = np.random.randn(100)
y = 2 * x + np.random.randn(100)
# Fit a linear regression model
model = sm.OLS(y, sm.add_constant(x))
# results = model.fit()
# Perform linear reset test
## opt 1
reset_test = linear_reset(results, power=2, test_type='fitted', cov_type='HAC', cov_kwargs={'maxlags': 2})
## opt 2
# test = linear_reset(results, cov_type='HAC', cov_kwds={'maxlags': 2})
## opt 3
# kwargs = {'maxlags': 2}
# test = linear_reset(results, cov_type='HAC', **kwargs)
## opt 4
# test = linear_reset(results, cov_type='HAC', maxlags=2)
```
```python
# Your code here that produces the bug
# This example should be self-contained, and so not rely on external data.
# It should run in a fresh ipython session, and so include all relevant imports.
```
<details>
---------------------------------------------------------------------------
KeyError Traceback (most recent call last)
File <command-2970740754718372>:19
16 model = sm.OLS(y, x).fit(cov_type='HAC', cov_kwds={'maxlags': 2})
18 # Perform linear reset test
---> 19 reset_test = linear_reset(model, power=2, test_type='fitted', cov_type='HAC', cov_kwargs={'maxlags': 2})
21 # # Perform the linear reset test with HAC covariance and maxlags=2
22 # test = linear_reset(results, cov_type='HAC', cov_kwds={'maxlags': 2})
23
(...)
33
34 # Print the test results
35 print(reset_test.summary())
File /databricks/python/lib/python3.9/site-packages/pandas/util/_decorators.py:207, in deprecate_kwarg.<locals>._deprecate_kwarg.<locals>.wrapper(*args, **kwargs)
205 else:
206 kwargs[new_arg_name] = new_arg_value
--> 207 return func(*args, **kwargs)
File /local_disk0/.ephemeral_nfs/cluster_libraries/python/lib/python3.9/site-packages/statsmodels/stats/diagnostic.py:1098, in linear_reset(res, power, test_type, use_f, cov_type, cov_kwargs)
1096 mod = mod_class(res.model.data.endog, aug_exog)
1097 cov_kwargs = {} if cov_kwargs is None else cov_kwargs
-> 1098 res = mod.fit(cov_type=cov_type, cov_kwargs=cov_kwargs)
1099 nrestr = aug_exog.shape[1] - exog.shape[1]
1100 nparams = aug_exog.shape[1]
File /local_disk0/.ephemeral_nfs/cluster_libraries/python/lib/python3.9/site-packages/statsmodels/regression/linear_model.py:373, in RegressionModel.fit(self, method, cov_type, cov_kwds, use_t, **kwargs)
370 self.df_resid = self.nobs - self.rank
372 if isinstance(self, OLS):
--> 373 lfit = OLSResults(
374 self, beta,
375 normalized_cov_params=self.normalized_cov_params,
376 cov_type=cov_type, cov_kwds=cov_kwds, use_t=use_t)
377 else:
378 lfit = RegressionResults(
379 self, beta,
380 normalized_cov_params=self.normalized_cov_params,
381 cov_type=cov_type, cov_kwds=cov_kwds, use_t=use_t,
382 **kwargs)
File /local_disk0/.ephemeral_nfs/cluster_libraries/python/lib/python3.9/site-packages/statsmodels/regression/linear_model.py:1653, in RegressionResults.__init__(self, model, params, normalized_cov_params, scale, cov_type, cov_kwds, use_t, **kwargs)
1651 use_t = use_t_2
1652 # TODO: warn or not?
-> 1653 self.get_robustcov_results(cov_type=cov_type, use_self=True,
1654 use_t=use_t, **cov_kwds)
1655 for key in kwargs:
1656 setattr(self, key, kwargs[key])
File /local_disk0/.ephemeral_nfs/cluster_libraries/python/lib/python3.9/site-packages/statsmodels/regression/linear_model.py:2570, in RegressionResults.get_robustcov_results(self, cov_type, use_t, **kwargs)
2567 res.cov_params_default = getattr(self, 'cov_' + cov_type.upper())
2568 elif cov_type.lower() == 'hac':
2569 # TODO: check if required, default in cov_hac_simple
-> 2570 maxlags = kwargs['maxlags']
2571 res.cov_kwds['maxlags'] = maxlags
2572 weights_func = kwargs.get('weights_func', sw.weights_bartlett)
KeyError: 'maxlags'
**Note**: As you can see, there are many issues on our GitHub tracker, so it is very possible that your issue has been posted before. Please check first before submitting so that we do not have to handle and close duplicates.
**Note**: Please be sure you are using the latest released version of `statsmodels`, or a recent build of `main`. If your problem has been fixed in an unreleased version, you might be able to use `main` until a new release occurs.
**Note**: If you are using a released version, have you verified that the bug exists in the main branch of this repository? It helps the limited resources if we know problems exist in the current main branch so that they do not need to check whether the code sample produces a bug in the next release.
</details>
If the issue has not been resolved, please file it in the issue tracker.
#### Expected Output
We expect that the code would produce the p-value from linear RESET test with "HAC" as the covariance estimator.
#### Output of ``import statsmodels.api as sm; sm.show_versions()``
<details>
[paste the output of ``import statsmodels.api as sm; sm.show_versions()`` here below this line]
0.14.1
</details>
| **update** this does not apply here, new issue #9122
robust cov_type is not implemented for, AFAIR, any of the diagnostic and specification tests.
Main reason: When I implemented those I only had references (and other packages for unit tests) for the standard version with basic OLS in the auxiliary regression.
At the time I was not sure about the theory and did not find explicit references for using `cov_type`s, although it's mentioned e.g. by Wooldridge that we can just use a specification robust cov_type in the auxiliary regression.
Also for some LM specification tests, we use uncentered rsquared as computational shortcut and not a standard wald test, i.e. it hardcodes the nonrobust hypothesis test.
For some specification/diagnostic tests we might be able to just forward the cov_type information to the auxiliary regression,
even if we cannot write unit test to verify against other packages.
In other cases it might be easier to use generic conditional moment test and newer score_test/lm_test which AFAIR already allow for cov_type (although currently with some restrictions).
I add elevated priority to issue to look at this.
I worked a lot on misspecification robust (HC, ...) CMT, score/lm test in the last years (but mostly for GLM and discrete), and should be able to get back to this.
Note: using wald test with augmented `exog` is easy to perform by just defining a new model.
e.g. one simple case for poisson https://gist.github.com/josef-pkt/1fdcc5c57cd63824fb3130d013e30103
Aside:
The older literature in econometrics was emphasizing auxiliary regression because it was relatively easy to use existing models from statistical packages for this. It also relied in many cases on an OPG version which is much easier to compute but has worse small sample properties (mainly relevant for nonlinear or non-gaussian models)
For statsmodels it's easier and provides more options to implement these tests based on generic theory and generic methods.
my mistake
the reset test in `diagnostic` was written by @bashtage and has cov_type keywords.
I found the bug
the reset test uses cov_kwargs, but the model.fit use cov_kwds
the correct code in the reset function should be
`res = mod.fit(cov_type=cov_type, cov_kwds=cov_kwargs)`
(I don't like the word kwargs, and prefer `xxx_kwds` for named options)
Is this problem been resolved yet? I'm using the latest version of statsmodels 0.14.2 and still have the exact same issue. | "2024-05-07T10:01:51Z" | 0.14 | [
"statsmodels/tsa/tests/test_seasonal.py::TestDecompose::test_ndarray",
"statsmodels/tsa/tests/test_seasonal.py::TestDecompose::test_raises",
"statsmodels/tsa/tests/test_seasonal.py::TestDecompose::test_pandas",
"statsmodels/tsa/tests/test_seasonal.py::TestDecompose::test_2d",
"statsmodels/tsa/tests/test_seasonal.py::TestDecompose::test_interpolate_trend",
"statsmodels/tsa/tests/test_seasonal.py::TestDecompose::test_pandas_nofreq",
"statsmodels/tsa/tests/test_seasonal.py::TestDecompose::test_filt",
"statsmodels/tsa/tests/test_seasonal.py::TestDecompose::test_one_sided_moving_average_in_stl_decompose",
"statsmodels/tsa/tests/test_seasonal.py::test_seasonal_decompose_smoke",
"statsmodels/tsa/tests/test_seasonal.py::test_seasonal_decompose_plot[False-True-12-multiplicative]",
"statsmodels/tsa/tests/test_seasonal.py::test_seasonal_decompose_plot[False-True-12-additive]",
"statsmodels/tsa/tests/test_seasonal.py::test_seasonal_decompose_plot[False-True-4-additive]",
"statsmodels/tsa/tests/test_seasonal.py::test_seasonal_decompose_plot[True-False-12-additive]",
"statsmodels/tsa/tests/test_seasonal.py::test_seasonal_decompose_too_short",
"statsmodels/tsa/tests/test_seasonal.py::test_seasonal_decompose_plot[True-False-4-multiplicative]",
"statsmodels/tsa/tests/test_seasonal.py::test_seasonal_decompose_plot[True-True-4-multiplicative]",
"statsmodels/tsa/tests/test_seasonal.py::test_seasonal_decompose_plot[True-True-4-additive]",
"statsmodels/tsa/tests/test_seasonal.py::test_seasonal_decompose_multiple",
"statsmodels/tsa/tests/test_seasonal.py::test_seasonal_decompose_plot[False-False-4-additive]",
"statsmodels/tsa/tests/test_seasonal.py::test_seasonal_decompose_plot[True-True-12-multiplicative]",
"statsmodels/tsa/tests/test_seasonal.py::test_seasonal_decompose_plot[False-True-4-multiplicative]",
"statsmodels/tsa/tests/test_seasonal.py::test_seasonal_decompose_plot[True-True-12-additive]",
"statsmodels/tsa/tests/test_seasonal.py::test_seasonal_decompose_plot[False-False-12-multiplicative]",
"statsmodels/tsa/tests/test_seasonal.py::test_seasonal_decompose_plot[False-False-4-multiplicative]",
"statsmodels/tsa/tests/test_seasonal.py::test_seasonal_decompose_plot[False-False-12-additive]",
"statsmodels/tsa/tests/test_seasonal.py::test_seasonal_decompose_plot[True-False-4-additive]",
"statsmodels/tsa/tests/test_seasonal.py::test_seasonal_decompose_plot[True-False-12-multiplicative]",
"statsmodels/stats/tests/test_diagnostic.py::TestDiagnosticGPandas::test_compare_nested[compare_cox]",
"statsmodels/stats/tests/test_diagnostic.py::TestDiagnosticGPandas::test_acorr_breusch_godfrey_multidim",
"statsmodels/stats/tests/test_diagnostic.py::TestDiagnosticGPandas::test_het_arch",
"statsmodels/stats/tests/test_diagnostic.py::TestDiagnosticGPandas::test_acorr_ljung_box_small_default",
"statsmodels/stats/tests/test_diagnostic.py::TestDiagnosticGPandas::test_compare_j",
"statsmodels/stats/tests/test_diagnostic.py::TestDiagnosticGPandas::test_hac",
"statsmodels/stats/tests/test_diagnostic.py::TestDiagnosticGPandas::test_het_white",
"statsmodels/stats/tests/test_diagnostic.py::TestDiagnosticGPandas::test_het_breusch_pagan_nonrobust",
"statsmodels/stats/tests/test_diagnostic.py::TestDiagnosticGPandas::test_basic",
"statsmodels/stats/tests/test_diagnostic.py::TestDiagnosticGPandas::test_acorr_breusch_godfrey_exogs",
"statsmodels/stats/tests/test_diagnostic.py::TestDiagnosticGPandas::test_harvey_collier",
"statsmodels/stats/tests/test_diagnostic.py::TestDiagnosticGPandas::test_influence",
"statsmodels/stats/tests/test_diagnostic.py::TestDiagnosticGPandas::test_compare_error[compare_cox]",
"statsmodels/stats/tests/test_diagnostic.py::TestDiagnosticGPandas::test_het_white_error",
"statsmodels/stats/tests/test_diagnostic.py::TestDiagnosticGPandas::test_compare_lr",
"statsmodels/stats/tests/test_diagnostic.py::TestDiagnosticGPandas::test_cusum_ols",
"statsmodels/stats/tests/test_diagnostic.py::TestDiagnosticGPandas::test_compare_cox",
"statsmodels/stats/tests/test_diagnostic.py::TestDiagnosticGPandas::test_het_goldfeldquandt",
"statsmodels/stats/tests/test_diagnostic.py::TestDiagnosticGPandas::test_rainbow",
"statsmodels/stats/tests/test_diagnostic.py::TestDiagnosticGPandas::test_het_arch2",
"statsmodels/stats/tests/test_diagnostic.py::TestDiagnosticGPandas::test_normality",
"statsmodels/stats/tests/test_diagnostic.py::TestDiagnosticGPandas::test_breaks_hansen",
"statsmodels/stats/tests/test_diagnostic.py::TestDiagnosticGPandas::test_acorr_ljung_box_big_default",
"statsmodels/stats/tests/test_diagnostic.py::TestDiagnosticGPandas::test_acorr_breusch_godfrey",
"statsmodels/stats/tests/test_diagnostic.py::TestDiagnosticGPandas::test_compare_nested[compare_j]",
"statsmodels/stats/tests/test_diagnostic.py::TestDiagnosticGPandas::test_het_breusch_pagan_1d_err",
"statsmodels/stats/tests/test_diagnostic.py::TestDiagnosticGPandas::test_het_breusch_pagan",
"statsmodels/stats/tests/test_diagnostic.py::TestDiagnosticGPandas::test_acorr_ljung_box_against_r",
"statsmodels/stats/tests/test_diagnostic.py::TestDiagnosticGPandas::test_acorr_ljung_box",
"statsmodels/stats/tests/test_diagnostic.py::TestDiagnosticGPandas::test_compare_error[compare_j]",
"statsmodels/stats/tests/test_diagnostic.py::TestDiagnosticGPandas::test_recursive_residuals",
"statsmodels/stats/tests/test_diagnostic.py::TestDiagnosticG::test_het_breusch_pagan",
"statsmodels/stats/tests/test_diagnostic.py::TestDiagnosticG::test_acorr_ljung_box",
"statsmodels/stats/tests/test_diagnostic.py::TestDiagnosticG::test_het_goldfeldquandt",
"statsmodels/stats/tests/test_diagnostic.py::TestDiagnosticG::test_het_breusch_pagan_1d_err",
"statsmodels/stats/tests/test_diagnostic.py::TestDiagnosticG::test_het_arch2",
"statsmodels/stats/tests/test_diagnostic.py::TestDiagnosticG::test_compare_cox",
"statsmodels/stats/tests/test_diagnostic.py::TestDiagnosticG::test_harvey_collier",
"statsmodels/stats/tests/test_diagnostic.py::TestDiagnosticG::test_rainbow",
"statsmodels/stats/tests/test_diagnostic.py::TestDiagnosticG::test_cusum_ols",
"statsmodels/stats/tests/test_diagnostic.py::TestDiagnosticG::test_acorr_ljung_box_small_default",
"statsmodels/stats/tests/test_diagnostic.py::TestDiagnosticG::test_recursive_residuals",
"statsmodels/stats/tests/test_diagnostic.py::TestDiagnosticG::test_het_arch",
"statsmodels/stats/tests/test_diagnostic.py::TestDiagnosticG::test_het_white",
"statsmodels/stats/tests/test_diagnostic.py::TestDiagnosticG::test_compare_j",
"statsmodels/stats/tests/test_diagnostic.py::TestDiagnosticG::test_compare_lr",
"statsmodels/stats/tests/test_diagnostic.py::TestDiagnosticG::test_compare_error[compare_j]",
"statsmodels/stats/tests/test_diagnostic.py::TestDiagnosticG::test_influence",
"statsmodels/stats/tests/test_diagnostic.py::TestDiagnosticG::test_acorr_ljung_box_big_default",
"statsmodels/stats/tests/test_diagnostic.py::TestDiagnosticG::test_compare_nested[compare_j]",
"statsmodels/stats/tests/test_diagnostic.py::TestDiagnosticG::test_normality",
"statsmodels/stats/tests/test_diagnostic.py::TestDiagnosticG::test_acorr_breusch_godfrey_multidim",
"statsmodels/stats/tests/test_diagnostic.py::TestDiagnosticG::test_acorr_ljung_box_against_r",
"statsmodels/stats/tests/test_diagnostic.py::TestDiagnosticG::test_breaks_hansen",
"statsmodels/stats/tests/test_diagnostic.py::TestDiagnosticG::test_het_white_error",
"statsmodels/stats/tests/test_diagnostic.py::TestDiagnosticG::test_basic",
"statsmodels/stats/tests/test_diagnostic.py::TestDiagnosticG::test_acorr_breusch_godfrey",
"statsmodels/stats/tests/test_diagnostic.py::TestDiagnosticG::test_acorr_breusch_godfrey_exogs",
"statsmodels/stats/tests/test_diagnostic.py::TestDiagnosticG::test_het_breusch_pagan_nonrobust",
"statsmodels/stats/tests/test_diagnostic.py::TestDiagnosticG::test_compare_nested[compare_cox]",
"statsmodels/stats/tests/test_diagnostic.py::TestDiagnosticG::test_hac",
"statsmodels/stats/tests/test_diagnostic.py::TestDiagnosticG::test_compare_error[compare_cox]",
"statsmodels/stats/tests/test_diagnostic.py::test_gq",
"statsmodels/stats/tests/test_diagnostic.py::test_linear_lm_direct",
"statsmodels/stats/tests/test_diagnostic.py::test_outlier_influence_funcs",
"statsmodels/stats/tests/test_diagnostic.py::test_rainbow_smoke_order_by[order_by1-0.5]",
"statsmodels/stats/tests/test_diagnostic.py::test_rainbow_smoke_order_by[order_by4-0.25]",
"statsmodels/stats/tests/test_diagnostic.py::test_encompasing_direct[nonrobust]",
"statsmodels/stats/tests/test_diagnostic.py::test_rainbow_smoke_order_by[order_by1-0.75]",
"statsmodels/stats/tests/test_diagnostic.py::test_rainbow_smoke_centered[None]",
"statsmodels/stats/tests/test_diagnostic.py::test_spec_white_error",
"statsmodels/stats/tests/test_diagnostic.py::test_rainbow_smoke_order_by[x0-0.25]",
"statsmodels/stats/tests/test_diagnostic.py::test_diagnostics_pandas",
"statsmodels/stats/tests/test_diagnostic.py::test_small_skip",
"statsmodels/stats/tests/test_diagnostic.py::test_spec_white",
"statsmodels/stats/tests/test_diagnostic.py::test_rainbow_smoke_order_by[None-0.75]",
"statsmodels/stats/tests/test_diagnostic.py::test_rainbow_smoke_order_by[order_by2-0.25]",
"statsmodels/stats/tests/test_diagnostic.py::test_rainbow_smoke_order_by[order_by4-0.5]",
"statsmodels/stats/tests/test_diagnostic.py::test_ljungbox_period",
"statsmodels/stats/tests/test_diagnostic.py::test_rainbow_smoke_order_by[order_by4-0.75]",
"statsmodels/stats/tests/test_diagnostic.py::test_rainbow_exception",
"statsmodels/stats/tests/test_diagnostic.py::test_encompasing_direct[HC0]",
"statsmodels/stats/tests/test_diagnostic.py::test_encompasing_error",
"statsmodels/stats/tests/test_diagnostic.py::test_rainbow_smoke_order_by[x0-0.75]",
"statsmodels/stats/tests/test_diagnostic.py::test_rainbow_smoke_centered[0.33]",
"statsmodels/stats/tests/test_diagnostic.py::test_outlier_test",
"statsmodels/stats/tests/test_diagnostic.py::test_rainbow_smoke_order_by[x0-0.5]",
"statsmodels/stats/tests/test_diagnostic.py::test_rainbow_smoke_order_by[None-0.5]",
"statsmodels/stats/tests/test_diagnostic.py::test_rainbow_smoke_order_by[order_by1-0.25]",
"statsmodels/stats/tests/test_diagnostic.py::test_acorr_lm_smoke_no_autolag",
"statsmodels/stats/tests/test_diagnostic.py::test_rainbow_smoke_order_by[None-0.25]",
"statsmodels/stats/tests/test_diagnostic.py::test_ljungbox_errors_warnings",
"statsmodels/stats/tests/test_diagnostic.py::test_rainbow_smoke_centered[300]",
"statsmodels/stats/tests/test_diagnostic.py::test_ljungbox_auto_lag_whitenoise",
"statsmodels/stats/tests/test_diagnostic.py::test_ljungbox_dof_adj",
"statsmodels/stats/tests/test_diagnostic.py::test_influence_dtype",
"statsmodels/stats/tests/test_diagnostic.py::test_rainbow_smoke_order_by[order_by2-0.5]",
"statsmodels/stats/tests/test_diagnostic.py::test_influence_wrapped",
"statsmodels/stats/tests/test_diagnostic.py::test_ljungbox_auto_lag_selection",
"statsmodels/stats/tests/test_diagnostic.py::test_rainbow_smoke_order_by[order_by2-0.75]"
] | [
"statsmodels/stats/tests/test_diagnostic.py::test_deprecated_argument",
"statsmodels/stats/tests/test_diagnostic.py::test_reset_smoke[cov0-False-princomp-2]",
"statsmodels/stats/tests/test_diagnostic.py::test_reset_smoke[cov1-False-princomp-3]",
"statsmodels/stats/tests/test_diagnostic.py::test_reset_smoke[cov0-True-fitted-3]",
"statsmodels/stats/tests/test_diagnostic.py::test_reset_smoke[cov0-True-exog-3]",
"statsmodels/stats/tests/test_diagnostic.py::test_acorr_lm_smoke[cov1-2-False]",
"statsmodels/stats/tests/test_diagnostic.py::test_reset_smoke[cov0-True-fitted-2]",
"statsmodels/stats/tests/test_diagnostic.py::test_reset_smoke[cov0-False-princomp-3]",
"statsmodels/stats/tests/test_diagnostic.py::test_reset_smoke[cov0-False-fitted-3]",
"statsmodels/stats/tests/test_diagnostic.py::test_reset_smoke[cov1-False-fitted-2]",
"statsmodels/stats/tests/test_diagnostic.py::test_reset_smoke[cov0-False-exog-3]",
"statsmodels/stats/tests/test_diagnostic.py::test_diagnostics_hac",
"statsmodels/stats/tests/test_diagnostic.py::test_reset_smoke[cov1-False-princomp-2]",
"statsmodels/stats/tests/test_diagnostic.py::test_reset_smoke[cov0-False-exog-2]",
"statsmodels/stats/tests/test_diagnostic.py::test_acorr_lm_smoke[cov0-0-True]",
"statsmodels/stats/tests/test_diagnostic.py::test_reset_smoke[cov0-True-princomp-2]",
"statsmodels/stats/tests/test_diagnostic.py::test_acorr_lm_smoke[cov1-2-True]",
"statsmodels/stats/tests/test_diagnostic.py::test_reset_smoke[cov1-True-fitted-3]",
"statsmodels/stats/tests/test_diagnostic.py::test_reset_smoke[cov1-False-exog-3]",
"statsmodels/stats/tests/test_diagnostic.py::test_reset_smoke[cov0-True-princomp-3]",
"statsmodels/stats/tests/test_diagnostic.py::test_reset_smoke[cov0-True-exog-2]",
"statsmodels/stats/tests/test_diagnostic.py::test_acorr_lm_smoke[cov1-0-False]",
"statsmodels/stats/tests/test_diagnostic.py::test_reset_smoke[cov1-True-exog-3]",
"statsmodels/stats/tests/test_diagnostic.py::test_reset_smoke[cov0-False-fitted-2]",
"statsmodels/stats/tests/test_diagnostic.py::test_reset_smoke[cov1-True-princomp-2]",
"statsmodels/stats/tests/test_diagnostic.py::test_reset_smoke[cov1-False-fitted-3]",
"statsmodels/stats/tests/test_diagnostic.py::test_reset_smoke[cov1-True-princomp-3]",
"statsmodels/stats/tests/test_diagnostic.py::test_reset_smoke[cov1-True-fitted-2]",
"statsmodels/stats/tests/test_diagnostic.py::test_acorr_lm_smoke[cov0-2-False]",
"statsmodels/stats/tests/test_diagnostic.py::test_reset_smoke[cov1-False-exog-2]",
"statsmodels/stats/tests/test_diagnostic.py::test_acorr_lm_smoke[cov0-2-True]",
"statsmodels/stats/tests/test_diagnostic.py::test_acorr_lm_smoke[cov1-0-True]",
"statsmodels/stats/tests/test_diagnostic.py::test_reset_smoke[cov1-True-exog-2]",
"statsmodels/stats/tests/test_diagnostic.py::test_acorr_lm_smoke[cov0-0-False]"
] | Python | [] | [] |
statsmodels/statsmodels | 9,249 | statsmodels__statsmodels-9249 | [
"9239"
] | 85fef91bdbde9c90be0055fb712459f6860861f8 | diff --git a/statsmodels/stats/proportion.py b/statsmodels/stats/proportion.py
--- a/statsmodels/stats/proportion.py
+++ b/statsmodels/stats/proportion.py
@@ -108,7 +108,7 @@ def _bisection_search_conservative(
return best_pt, best
-def proportion_confint(count, nobs, alpha:float=0.05, method="normal"):
+def proportion_confint(count, nobs, alpha:float=0.05, method="normal", alternative:str='two-sided'):
"""
Confidence interval for a binomial proportion
@@ -133,11 +133,16 @@ def proportion_confint(count, nobs, alpha:float=0.05, method="normal"):
- `jeffreys` : Jeffreys Bayesian Interval
- `binom_test` : Numerical inversion of binom_test
+ alternative : {"two-sided", "larger", "smaller"}
+ default: "two-sided"
+ specifies whether to calculate a two-sided or one-sided confidence interval.
+
Returns
-------
ci_low, ci_upp : {float, ndarray, Series DataFrame}
- lower and upper confidence level with coverage (approximately) 1-alpha.
+ larger and smaller confidence level with coverage (approximately) 1-alpha.
When a pandas object is returned, then the index is taken from `count`.
+ When side is not "two-sided", lower or upper bound is set to 0 or 1 respectively.
Notes
-----
@@ -188,21 +193,24 @@ def _check(x: np.ndarray, name: str) -> np.ndarray:
nobs_a = _check(np.asarray(nobs_a), "count")
q_ = count_a / nobs_a
- alpha_2 = 0.5 * alpha
+
+
+ if alternative == 'two-sided':
+ if method != "binom_test":
+ alpha = alpha / 2.0
+ elif alternative not in ['larger', 'smaller']:
+ raise NotImplementedError(f"alternative {alternative} is not available")
if method == "normal":
std_ = np.sqrt(q_ * (1 - q_) / nobs_a)
- dist = stats.norm.isf(alpha / 2.0) * std_
+ dist = stats.norm.isf(alpha) * std_
ci_low = q_ - dist
ci_upp = q_ + dist
- elif method == "binom_test":
- # inverting the binomial test
+ elif method == "binom_test" and alternative == 'two-sided':
def func_factory(count: int, nobs: int) -> Callable[[float], float]:
if hasattr(stats, "binomtest"):
-
def func(qi):
return stats.binomtest(count, nobs, p=qi).pvalue - alpha
-
else:
# Remove after min SciPy >= 1.7
def func(qi):
@@ -258,9 +266,9 @@ def func(qi):
ci_upp.flat[index] = 1 - ci_low.flat[index]
ci_low.flat[index] = 1 - temp
index = bcast.index
- elif method == "beta":
- ci_low = stats.beta.ppf(alpha_2, count_a, nobs_a - count_a + 1)
- ci_upp = stats.beta.isf(alpha_2, count_a + 1, nobs_a - count_a)
+ elif method == "beta" or (method == "binom_test" and alternative != 'two-sided'):
+ ci_low = stats.beta.ppf(alpha, count_a, nobs_a - count_a + 1)
+ ci_upp = stats.beta.isf(alpha, count_a + 1, nobs_a - count_a)
if np.ndim(ci_low) > 0:
ci_low.flat[q_.flat == 0] = 0
@@ -269,7 +277,7 @@ def func(qi):
ci_low = 0 if q_ == 0 else ci_low
ci_upp = 1 if q_ == 1 else ci_upp
elif method == "agresti_coull":
- crit = stats.norm.isf(alpha / 2.0)
+ crit = stats.norm.isf(alpha)
nobs_c = nobs_a + crit**2
q_c = (count_a + crit**2 / 2.0) / nobs_c
std_c = np.sqrt(q_c * (1.0 - q_c) / nobs_c)
@@ -277,7 +285,7 @@ def func(qi):
ci_low = q_c - dist
ci_upp = q_c + dist
elif method == "wilson":
- crit = stats.norm.isf(alpha / 2.0)
+ crit = stats.norm.isf(alpha)
crit2 = crit**2
denom = 1 + crit2 / nobs_a
center = (q_ + crit2 / (2 * nobs_a)) / denom
@@ -289,9 +297,8 @@ def func(qi):
ci_upp = center + dist
# method adjusted to be more forgiving of misspellings or incorrect option name
elif method[:4] == "jeff":
- ci_low, ci_upp = stats.beta.interval(
- 1 - alpha, count_a + 0.5, nobs_a - count_a + 0.5
- )
+ ci_low = stats.beta.ppf(alpha, count_a + 0.5, nobs_a - count_a + 0.5)
+ ci_upp = stats.beta.isf(alpha, count_a + 0.5, nobs_a - count_a + 0.5)
else:
raise NotImplementedError(f"method {method} is not available")
if method in ["normal", "agresti_coull"]:
@@ -301,6 +308,10 @@ def func(qi):
container = pd.Series if isinstance(count, pd.Series) else pd.DataFrame
ci_low = container(ci_low, index=count.index)
ci_upp = container(ci_upp, index=count.index)
+ if alternative == 'larger':
+ ci_low = 0
+ elif alternative == 'smaller':
+ ci_upp = 1
if is_scalar:
return float(ci_low), float(ci_upp)
return ci_low, ci_upp
| diff --git a/statsmodels/stats/tests/test_proportion.py b/statsmodels/stats/tests/test_proportion.py
--- a/statsmodels/stats/tests/test_proportion.py
+++ b/statsmodels/stats/tests/test_proportion.py
@@ -995,3 +995,67 @@ def test_ci_symmetry_array(count, method):
a = proportion_confint([count, count], n, method=method)
b = proportion_confint([n - count, n - count], n, method=method)
assert_allclose(np.array(a), 1.0 - np.array(b[::-1]))
+
+
[email protected]("count, nobs, alternative, expected", [
+ (1, 1, 'two-sided', [1.0, 1.0]),
+ (0, 1, 'two-sided', [0.0, 0.0]),
+ (1, 1, 'larger', [0.0, 1.0]),
+ (0, 1, 'larger', [0.0, 0.0]),
+ (1, 1, 'smaller', [1.0, 1.0]),
+ (0, 1, 'smaller', [0.0, 1.0]),
+])
+def test_proportion_confint_edge(count, nobs, alternative, expected):
+ # test against R 4.3.3 / DescTools / BinomCI and binom.test
+ ci_low, ci_upp = proportion_confint(count, nobs, alternative=alternative)
+ assert_allclose(np.array([ci_low, ci_upp]), np.array(expected))
+
+
[email protected]("count", [100])
[email protected]("nobs", [200])
[email protected]("method, alpha, alternative, expected", [
+ # two-sided
+ ("normal", 0.05, "two-sided", [0.4307048, 0.5692952]),
+ ("normal", 0.01, "two-sided", [0.4089307, 0.5910693]),
+ ("normal", 0.10, "two-sided", [0.4418456, 0.5581544]),
+ ("agresti_coull", 0.05, "two-sided", [0.4313609, 0.5686391]),
+ ("beta", 0.05, "two-sided", [0.4286584, 0.5713416]),
+ ("wilson", 0.05, "two-sided", [0.4313609, 0.5686391]),
+ ("jeffreys", 0.05, "two-sided", [0.4311217, 0.5688783]),
+ # larger
+ ("normal", 0.05, "larger", [0.0, 0.5581544]),
+ ("agresti_coull", 0.05, "larger", [0.0, 0.557765]),
+ ("beta", 0.05, "larger", [0.0, 0.560359]),
+ ("wilson", 0.05, "larger", [0.0, 0.557765]),
+ ("jeffreys", 0.05, "larger", [0.0, 0.5578862]),
+ # smaller
+ ("normal", 0.05, "smaller", [0.4418456, 1.0]),
+ ("agresti_coull", 0.05, "smaller", [0.442235, 1.0]),
+ ("beta", 0.05, "smaller", [0.439641, 1.0]),
+ ("wilson", 0.05, "smaller", [0.442235, 1.0]),
+ ("jeffreys", 0.05, "smaller", [0.4421138, 1.0]),
+])
+def test_proportion_confint(count, nobs, method, alpha, alternative, expected):
+ # test against R 4.3.3 / DescTools / BinomCI and binom.test
+ ci_low, ci_upp = proportion_confint(count, nobs, alpha=alpha, method=method, alternative=alternative)
+ assert_allclose(np.array([ci_low, ci_upp]), np.array(expected))
+
+
[email protected]("count", [100])
[email protected]("nobs", [200])
[email protected]("alpha, alternative, expected, rtol", [
+ # binom.exact with method="two.sided" returns values with a precision of up to 4 decimal places.
+ (0.01, "two-sided", [0.4094, 0.5906], 1e-4),
+ (0.05, "two-sided", [0.4299, 0.5701], 1e-4),
+ (0.10, "two-sided", [0.44, 0.56], 1e-4),
+ (0.01, "larger", [0.0, 0.5840449], 1e-7),
+ (0.05, "larger", [0.0, 0.560359], 1e-7),
+ (0.10, "larger", [0.0, 0.5476422], 1e-7),
+ (0.01, "smaller", [0.4159551, 1.0], 1e-7),
+ (0.05, "smaller", [0.439641, 1.0], 1e-7),
+ (0.10, "smaller", [0.4523578, 1.0], 1e-7),
+])
+def test_proportion_confint_binom_test(count, nobs, alpha, alternative, expected, rtol):
+ # test against R 4.3.3 / DescTools / exactci
+ ci_low, ci_upp = proportion_confint(count, nobs, alpha=alpha, method="binom_test", alternative=alternative)
+ assert_allclose(np.array([ci_low, ci_upp]), np.array(expected), rtol=rtol)
| ENH: proportion_confint only has two-sided confidence intervals, no "alternative" option
see
https://stats.stackexchange.com/questions/646445/multinomial-one-sided-confidence-intervals
same for multinomial_proportions_confint
I think for proportion_confint it should be just one interval at 2*alpha, except for "beta-test" which is minlike, and the one-sided will correspond to one side of equal-tail intervals.
I don't have a guess on one-sided interval for multinomial_proportions_confint. (too long ago). But it might be based on an equal-tail derivation.
| @josef-pkt
Hi, Josef.
I read the thread and agree that the one-sided interval is the same as one side of the two-sided interval for doubled alpha in a binomial distribution.
Can I take this issue on propotion_confint to add an option to explicitly get one-sided ci?
Yes, we want to add the `alternative` option and include one sided intervals.
Note, "binomtest" will have different one-sided intervals which should be easy to compute.
The will be the same as for the central, equal-tail binom test.
pull request would be welcome.
| "2024-05-19T14:51:31Z" | 0.14 | [
"statsmodels/stats/tests/test_proportion.py::TestProportion::test_number_pairs_1493",
"statsmodels/stats/tests/test_proportion.py::TestProportion::test_scalar",
"statsmodels/stats/tests/test_proportion.py::TestProportion::test_pairwiseproptest",
"statsmodels/stats/tests/test_proportion.py::TestProportion::test_proptest",
"statsmodels/stats/tests/test_proportion.py::TestProportion::test_default_values",
"statsmodels/stats/tests/test_proportion.py::test_confint_proportion[wilson-case4]",
"statsmodels/stats/tests/test_proportion.py::test_confint_proportion[beta-case3]",
"statsmodels/stats/tests/test_proportion.py::test_confint_proportion_ndim[normal]",
"statsmodels/stats/tests/test_proportion.py::test_confint_proportion[jeffreys-case0]",
"statsmodels/stats/tests/test_proportion.py::test_confint_proportion[beta-case6]",
"statsmodels/stats/tests/test_proportion.py::test_confint_proportion[wilson-case0]",
"statsmodels/stats/tests/test_proportion.py::test_confint_proportion[wilson-case6]",
"statsmodels/stats/tests/test_proportion.py::test_confint_proportion[normal-case5]",
"statsmodels/stats/tests/test_proportion.py::test_confint_proportion[normal-case1]",
"statsmodels/stats/tests/test_proportion.py::test_confint_proportion[jeffreys-case2]",
"statsmodels/stats/tests/test_proportion.py::test_confint_proportion[agresti_coull-case2]",
"statsmodels/stats/tests/test_proportion.py::test_confint_proportion[beta-case2]",
"statsmodels/stats/tests/test_proportion.py::test_confint_proportion[beta-case0]",
"statsmodels/stats/tests/test_proportion.py::test_confint_proportion[normal-case4]",
"statsmodels/stats/tests/test_proportion.py::test_confint_proportion[beta-case4]",
"statsmodels/stats/tests/test_proportion.py::test_confint_proportion[normal-case7]",
"statsmodels/stats/tests/test_proportion.py::test_multinomial_proportions_errors",
"statsmodels/stats/tests/test_proportion.py::test_confint_proportion[agresti_coull-case0]",
"statsmodels/stats/tests/test_proportion.py::test_confint_proportion[beta-case7]",
"statsmodels/stats/tests/test_proportion.py::test_confint_proportion_ndim[wilson]",
"statsmodels/stats/tests/test_proportion.py::test_confint_proportion[agresti_coull-case6]",
"statsmodels/stats/tests/test_proportion.py::test_confint_proportion[jeffreys-case1]",
"statsmodels/stats/tests/test_proportion.py::test_confint_proportion[normal-case2]",
"statsmodels/stats/tests/test_proportion.py::test_confint_proportion[beta-case1]",
"statsmodels/stats/tests/test_proportion.py::test_confint_proportion[agresti_coull-case1]",
"statsmodels/stats/tests/test_proportion.py::test_proportion_effect_size",
"statsmodels/stats/tests/test_proportion.py::test_confint_proportion[agresti_coull-case3]",
"statsmodels/stats/tests/test_proportion.py::test_confint_proportion[wilson-case2]",
"statsmodels/stats/tests/test_proportion.py::test_confint_proportion_ndim[beta]",
"statsmodels/stats/tests/test_proportion.py::test_samplesize_confidenceinterval_prop",
"statsmodels/stats/tests/test_proportion.py::test_confint_proportion[wilson-case5]",
"statsmodels/stats/tests/test_proportion.py::test_confint_proportion[normal-case6]",
"statsmodels/stats/tests/test_proportion.py::test_confint_multinomial_proportions_zeros",
"statsmodels/stats/tests/test_proportion.py::test_confint_proportion_ndim[agresti_coull]",
"statsmodels/stats/tests/test_proportion.py::test_confint_proportion[wilson-case1]",
"statsmodels/stats/tests/test_proportion.py::test_confint_proportion_ndim[jeffreys]",
"statsmodels/stats/tests/test_proportion.py::test_confint_proportion[beta-case5]",
"statsmodels/stats/tests/test_proportion.py::test_confint_proportion[wilson-case3]",
"statsmodels/stats/tests/test_proportion.py::test_confint_proportion[agresti_coull-case5]",
"statsmodels/stats/tests/test_proportion.py::test_confint_proportion[normal-case0]",
"statsmodels/stats/tests/test_proportion.py::test_confint_proportion[agresti_coull-case7]",
"statsmodels/stats/tests/test_proportion.py::test_confint_proportion[wilson-case7]",
"statsmodels/stats/tests/test_proportion.py::test_confint_proportion[normal-case3]",
"statsmodels/stats/tests/test_proportion.py::test_confint_multinomial_proportions",
"statsmodels/stats/tests/test_proportion.py::test_confint_proportion[agresti_coull-case4]",
"statsmodels/stats/tests/test_proportion.py::test_ci_symmetry_binom_test[False-count5-47]",
"statsmodels/stats/tests/test_proportion.py::test_ci_symmetry_binom_test[True-count12-47]",
"statsmodels/stats/tests/test_proportion.py::test_ci_symmetry_binom_test[False-count31-50]",
"statsmodels/stats/tests/test_proportion.py::test_ci_symmetry_binom_test[True-count27-50]",
"statsmodels/stats/tests/test_proportion.py::test_ci_symmetry_binom_test[True-count8-47]",
"statsmodels/stats/tests/test_proportion.py::test_ci_symmetry[True-normal-count13]",
"statsmodels/stats/tests/test_proportion.py::test_ci_symmetry[True-normal-count5]",
"statsmodels/stats/tests/test_proportion.py::test_ci_symmetry_binom_test[True-count15-47]",
"statsmodels/stats/tests/test_proportion.py::test_ci_symmetry[False-beta-count11]",
"statsmodels/stats/tests/test_proportion.py::test_ci_symmetry_array[wilson-count8]",
"statsmodels/stats/tests/test_proportion.py::test_ci_symmetry_array[wilson-count9]",
"statsmodels/stats/tests/test_proportion.py::test_ci_symmetry[False-wilson-count7]",
"statsmodels/stats/tests/test_proportion.py::test_ci_symmetry_array[binom_test-count6]",
"statsmodels/stats/tests/test_proportion.py::test_ci_symmetry[True-normal-count2]",
"statsmodels/stats/tests/test_proportion.py::test_ci_symmetry[True-jeffreys-count7]",
"statsmodels/stats/tests/test_proportion.py::test_ci_symmetry[False-wilson-count9]",
"statsmodels/stats/tests/test_proportion.py::test_ci_symmetry[False-agresti_coull-count8]",
"statsmodels/stats/tests/test_proportion.py::test_ci_symmetry[True-normal-count7]",
"statsmodels/stats/tests/test_proportion.py::test_ci_symmetry_array[binom_test-count11]",
"statsmodels/stats/tests/test_proportion.py::test_ci_symmetry_array[beta-count6]",
"statsmodels/stats/tests/test_proportion.py::test_ci_symmetry_array[jeffreys-count10]",
"statsmodels/stats/tests/test_proportion.py::test_ci_symmetry_binom_test[True-count32-50]",
"statsmodels/stats/tests/test_proportion.py::test_ci_symmetry_binom_test[False-count38-50]",
"statsmodels/stats/tests/test_proportion.py::test_ci_symmetry[True-jeffreys-count9]",
"statsmodels/stats/tests/test_proportion.py::test_ci_symmetry[False-agresti_coull-count2]",
"statsmodels/stats/tests/test_proportion.py::test_ci_symmetry_binom_test[False-count5-50]",
"statsmodels/stats/tests/test_proportion.py::test_ci_symmetry[True-agresti_coull-count1]",
"statsmodels/stats/tests/test_proportion.py::test_ci_symmetry_array[jeffreys-count0]",
"statsmodels/stats/tests/test_proportion.py::test_ci_symmetry_array[beta-count0]",
"statsmodels/stats/tests/test_proportion.py::test_ci_symmetry_binom_test[True-count41-50]",
"statsmodels/stats/tests/test_proportion.py::test_ci_symmetry[True-jeffreys-count4]",
"statsmodels/stats/tests/test_proportion.py::test_ci_symmetry_array[binom_test-count14]",
"statsmodels/stats/tests/test_proportion.py::test_power_ztost_prop_norm",
"statsmodels/stats/tests/test_proportion.py::test_ci_symmetry[True-agresti_coull-count2]",
"statsmodels/stats/tests/test_proportion.py::test_ci_symmetry_binom_test[False-count12-47]",
"statsmodels/stats/tests/test_proportion.py::test_ci_symmetry[True-binom_test-count3]",
"statsmodels/stats/tests/test_proportion.py::test_ci_symmetry[False-wilson-count8]",
"statsmodels/stats/tests/test_proportion.py::test_ci_symmetry[True-binom_test-count12]",
"statsmodels/stats/tests/test_proportion.py::test_ci_symmetry_array[wilson-count6]",
"statsmodels/stats/tests/test_proportion.py::test_ci_symmetry[True-agresti_coull-count7]",
"statsmodels/stats/tests/test_proportion.py::test_ci_symmetry[False-jeffreys-count11]",
"statsmodels/stats/tests/test_proportion.py::test_ci_symmetry_binom_test[False-count10-50]",
"statsmodels/stats/tests/test_proportion.py::test_ci_symmetry[False-binom_test-count14]",
"statsmodels/stats/tests/test_proportion.py::test_ci_symmetry[True-wilson-count6]",
"statsmodels/stats/tests/test_proportion.py::test_ci_symmetry[False-jeffreys-count15]",
"statsmodels/stats/tests/test_proportion.py::test_ci_symmetry_array[jeffreys-count9]",
"statsmodels/stats/tests/test_proportion.py::test_ci_symmetry[True-beta-count13]",
"statsmodels/stats/tests/test_proportion.py::test_ci_symmetry_array[jeffreys-count11]",
"statsmodels/stats/tests/test_proportion.py::test_ci_symmetry_binom_test[False-count6-50]",
"statsmodels/stats/tests/test_proportion.py::test_ci_symmetry_binom_test[False-count47-50]",
"statsmodels/stats/tests/test_proportion.py::test_ci_symmetry[True-beta-count0]",
"statsmodels/stats/tests/test_proportion.py::test_ci_symmetry_binom_test[False-count26-47]",
"statsmodels/stats/tests/test_proportion.py::test_ci_symmetry_binom_test[True-count45-47]",
"statsmodels/stats/tests/test_proportion.py::test_ci_symmetry_binom_test[False-count44-50]",
"statsmodels/stats/tests/test_proportion.py::test_ci_symmetry_binom_test[False-count42-50]",
"statsmodels/stats/tests/test_proportion.py::test_ci_symmetry[True-beta-count7]",
"statsmodels/stats/tests/test_proportion.py::test_ci_symmetry[True-beta-count8]",
"statsmodels/stats/tests/test_proportion.py::test_ci_symmetry[False-agresti_coull-count3]",
"statsmodels/stats/tests/test_proportion.py::test_ci_symmetry_binom_test[False-count24-50]",
"statsmodels/stats/tests/test_proportion.py::test_ci_symmetry[False-agresti_coull-count4]",
"statsmodels/stats/tests/test_proportion.py::test_ci_symmetry_binom_test[False-count35-47]",
"statsmodels/stats/tests/test_proportion.py::test_ci_symmetry[True-wilson-count4]",
"statsmodels/stats/tests/test_proportion.py::test_ci_symmetry_binom_test[False-count40-50]",
"statsmodels/stats/tests/test_proportion.py::test_ci_symmetry[False-jeffreys-count12]",
"statsmodels/stats/tests/test_proportion.py::test_test_2indep",
"statsmodels/stats/tests/test_proportion.py::test_ci_symmetry[False-normal-count11]",
"statsmodels/stats/tests/test_proportion.py::test_ci_symmetry[False-agresti_coull-count15]",
"statsmodels/stats/tests/test_proportion.py::test_ci_symmetry_array[normal-count12]",
"statsmodels/stats/tests/test_proportion.py::test_ci_symmetry[False-binom_test-count5]",
"statsmodels/stats/tests/test_proportion.py::test_ci_symmetry[False-jeffreys-count6]",
"statsmodels/stats/tests/test_proportion.py::test_ci_symmetry[False-normal-count15]",
"statsmodels/stats/tests/test_proportion.py::test_ci_symmetry_array[agresti_coull-count13]",
"statsmodels/stats/tests/test_proportion.py::test_ci_symmetry_array[normal-count0]",
"statsmodels/stats/tests/test_proportion.py::test_ci_symmetry_array[agresti_coull-count8]",
"statsmodels/stats/tests/test_proportion.py::test_ci_symmetry_binom_test[False-count13-50]",
"statsmodels/stats/tests/test_proportion.py::test_ci_symmetry[True-agresti_coull-count13]",
"statsmodels/stats/tests/test_proportion.py::test_ci_symmetry[False-agresti_coull-count9]",
"statsmodels/stats/tests/test_proportion.py::test_ci_symmetry_array[binom_test-count9]",
"statsmodels/stats/tests/test_proportion.py::test_ci_symmetry_binom_test[False-count28-50]",
"statsmodels/stats/tests/test_proportion.py::test_ci_symmetry_array[wilson-count10]",
"statsmodels/stats/tests/test_proportion.py::test_ci_symmetry[False-normal-count3]",
"statsmodels/stats/tests/test_proportion.py::test_ci_symmetry[True-beta-count9]",
"statsmodels/stats/tests/test_proportion.py::test_ci_symmetry_binom_test[True-count4-47]",
"statsmodels/stats/tests/test_proportion.py::test_ci_symmetry[True-jeffreys-count15]",
"statsmodels/stats/tests/test_proportion.py::test_ci_symmetry[True-binom_test-count5]",
"statsmodels/stats/tests/test_proportion.py::test_ci_symmetry_binom_test[True-count33-50]",
"statsmodels/stats/tests/test_proportion.py::test_ci_symmetry_binom_test[True-count3-50]",
"statsmodels/stats/tests/test_proportion.py::test_ci_symmetry[False-wilson-count11]",
"statsmodels/stats/tests/test_proportion.py::test_ci_symmetry_binom_test[True-count21-47]",
"statsmodels/stats/tests/test_proportion.py::test_ci_symmetry[True-normal-count3]",
"statsmodels/stats/tests/test_proportion.py::test_ci_symmetry[False-wilson-count4]",
"statsmodels/stats/tests/test_proportion.py::test_ci_symmetry[True-normal-count1]",
"statsmodels/stats/tests/test_proportion.py::test_ci_symmetry_binom_test[False-count22-47]",
"statsmodels/stats/tests/test_proportion.py::test_ci_symmetry_binom_test[True-count19-50]",
"statsmodels/stats/tests/test_proportion.py::test_ci_symmetry[False-normal-count12]",
"statsmodels/stats/tests/test_proportion.py::test_ztost",
"statsmodels/stats/tests/test_proportion.py::test_ci_symmetry[False-binom_test-count13]",
"statsmodels/stats/tests/test_proportion.py::test_ci_symmetry[True-beta-count14]",
"statsmodels/stats/tests/test_proportion.py::test_ci_symmetry[True-agresti_coull-count15]",
"statsmodels/stats/tests/test_proportion.py::test_ci_symmetry_binom_test[True-count23-47]",
"statsmodels/stats/tests/test_proportion.py::test_ci_symmetry[True-wilson-count5]",
"statsmodels/stats/tests/test_proportion.py::test_ci_symmetry_binom_test[True-count44-47]",
"statsmodels/stats/tests/test_proportion.py::test_ci_symmetry_array[agresti_coull-count14]",
"statsmodels/stats/tests/test_proportion.py::test_ci_symmetry[True-wilson-count7]",
"statsmodels/stats/tests/test_proportion.py::test_ci_symmetry_array[beta-count7]",
"statsmodels/stats/tests/test_proportion.py::test_ci_symmetry_binom_test[True-count17-50]",
"statsmodels/stats/tests/test_proportion.py::test_ci_symmetry_array[normal-count14]",
"statsmodels/stats/tests/test_proportion.py::test_ci_symmetry[False-jeffreys-count10]",
"statsmodels/stats/tests/test_proportion.py::test_ci_symmetry_binom_test[True-count43-47]",
"statsmodels/stats/tests/test_proportion.py::test_ci_symmetry[True-normal-count12]",
"statsmodels/stats/tests/test_proportion.py::test_ci_symmetry[False-binom_test-count12]",
"statsmodels/stats/tests/test_proportion.py::test_ci_symmetry_binom_test[True-count6-47]",
"statsmodels/stats/tests/test_proportion.py::test_ci_symmetry_binom_test[False-count28-47]",
"statsmodels/stats/tests/test_proportion.py::test_ci_symmetry_binom_test[True-count47-47]",
"statsmodels/stats/tests/test_proportion.py::test_ci_symmetry_binom_test[True-count41-47]",
"statsmodels/stats/tests/test_proportion.py::test_ci_symmetry_binom_test[True-count3-47]",
"statsmodels/stats/tests/test_proportion.py::test_ci_symmetry_binom_test[False-count41-50]",
"statsmodels/stats/tests/test_proportion.py::test_ci_symmetry[True-jeffreys-count11]",
"statsmodels/stats/tests/test_proportion.py::test_ci_symmetry_binom_test[False-count41-47]",
"statsmodels/stats/tests/test_proportion.py::test_ci_symmetry_binom_test[False-count46-50]",
"statsmodels/stats/tests/test_proportion.py::test_ci_symmetry[True-wilson-count8]",
"statsmodels/stats/tests/test_proportion.py::test_ci_symmetry_binom_test[True-count33-47]",
"statsmodels/stats/tests/test_proportion.py::test_ci_symmetry_binom_test[False-count34-47]",
"statsmodels/stats/tests/test_proportion.py::test_ci_symmetry_binom_test[False-count45-47]",
"statsmodels/stats/tests/test_proportion.py::test_ci_symmetry[False-beta-count4]",
"statsmodels/stats/tests/test_proportion.py::test_ci_symmetry_binom_test[True-count42-47]",
"statsmodels/stats/tests/test_proportion.py::test_ci_symmetry[False-jeffreys-count13]",
"statsmodels/stats/tests/test_proportion.py::test_ci_symmetry[False-normal-count9]",
"statsmodels/stats/tests/test_proportion.py::test_ci_symmetry_array[wilson-count14]",
"statsmodels/stats/tests/test_proportion.py::test_ci_symmetry[True-agresti_coull-count8]",
"statsmodels/stats/tests/test_proportion.py::test_ci_symmetry_binom_test[True-count22-47]",
"statsmodels/stats/tests/test_proportion.py::test_ci_symmetry_binom_test[True-count9-47]",
"statsmodels/stats/tests/test_proportion.py::test_ci_symmetry_array[binom_test-count3]",
"statsmodels/stats/tests/test_proportion.py::test_ci_symmetry_binom_test[False-count15-47]",
"statsmodels/stats/tests/test_proportion.py::test_ci_symmetry_array[binom_test-count0]",
"statsmodels/stats/tests/test_proportion.py::test_ci_symmetry_binom_test[True-count46-47]",
"statsmodels/stats/tests/test_proportion.py::test_ci_symmetry_array[normal-count4]",
"statsmodels/stats/tests/test_proportion.py::test_ci_symmetry[True-agresti_coull-count6]",
"statsmodels/stats/tests/test_proportion.py::test_ci_symmetry[True-binom_test-count13]",
"statsmodels/stats/tests/test_proportion.py::test_ci_symmetry[False-agresti_coull-count12]",
"statsmodels/stats/tests/test_proportion.py::test_score_confint_koopman_nam",
"statsmodels/stats/tests/test_proportion.py::test_ci_symmetry[True-agresti_coull-count12]",
"statsmodels/stats/tests/test_proportion.py::test_ci_symmetry_binom_test[False-count9-47]",
"statsmodels/stats/tests/test_proportion.py::test_ci_symmetry_binom_test[False-count6-47]",
"statsmodels/stats/tests/test_proportion.py::test_ci_symmetry[True-beta-count11]",
"statsmodels/stats/tests/test_proportion.py::test_ci_symmetry_array[wilson-count13]",
"statsmodels/stats/tests/test_proportion.py::test_ci_symmetry_binom_test[True-count8-50]",
"statsmodels/stats/tests/test_proportion.py::test_ci_symmetry_binom_test[False-count4-50]",
"statsmodels/stats/tests/test_proportion.py::test_ci_symmetry_binom_test[True-count11-47]",
"statsmodels/stats/tests/test_proportion.py::test_ci_symmetry_binom_test[False-count7-47]",
"statsmodels/stats/tests/test_proportion.py::test_ci_symmetry_binom_test[True-count31-47]",
"statsmodels/stats/tests/test_proportion.py::test_power_ztost_prop",
"statsmodels/stats/tests/test_proportion.py::test_ci_symmetry[True-agresti_coull-count4]",
"statsmodels/stats/tests/test_proportion.py::test_ci_symmetry_array[beta-count2]",
"statsmodels/stats/tests/test_proportion.py::test_ci_symmetry_binom_test[True-count37-47]",
"statsmodels/stats/tests/test_proportion.py::test_ci_symmetry_binom_test[True-count47-50]",
"statsmodels/stats/tests/test_proportion.py::test_ci_symmetry_binom_test[True-count7-47]",
"statsmodels/stats/tests/test_proportion.py::test_ci_symmetry_binom_test[True-count40-50]",
"statsmodels/stats/tests/test_proportion.py::test_ci_symmetry[False-agresti_coull-count0]",
"statsmodels/stats/tests/test_proportion.py::test_ci_symmetry_binom_test[False-count33-50]",
"statsmodels/stats/tests/test_proportion.py::test_ci_symmetry_array[jeffreys-count2]",
"statsmodels/stats/tests/test_proportion.py::test_ci_symmetry_binom_test[True-count25-50]",
"statsmodels/stats/tests/test_proportion.py::test_ci_symmetry_array[wilson-count0]",
"statsmodels/stats/tests/test_proportion.py::test_ci_symmetry[True-binom_test-count6]",
"statsmodels/stats/tests/test_proportion.py::test_ci_symmetry[False-beta-count10]",
"statsmodels/stats/tests/test_proportion.py::test_ci_symmetry[True-jeffreys-count2]",
"statsmodels/stats/tests/test_proportion.py::test_ci_symmetry_binom_test[False-count37-50]",
"statsmodels/stats/tests/test_proportion.py::test_ci_symmetry[False-beta-count1]",
"statsmodels/stats/tests/test_proportion.py::test_ci_symmetry_binom_test[False-count3-50]",
"statsmodels/stats/tests/test_proportion.py::test_score_test_2indep",
"statsmodels/stats/tests/test_proportion.py::test_ci_symmetry[True-agresti_coull-count10]",
"statsmodels/stats/tests/test_proportion.py::test_ci_symmetry_array[normal-count7]",
"statsmodels/stats/tests/test_proportion.py::test_ci_symmetry_binom_test[False-count36-47]",
"statsmodels/stats/tests/test_proportion.py::test_ci_symmetry_binom_test[False-count35-50]",
"statsmodels/stats/tests/test_proportion.py::test_ci_symmetry_binom_test[True-count38-47]",
"statsmodels/stats/tests/test_proportion.py::test_ci_symmetry_binom_test[True-count39-47]",
"statsmodels/stats/tests/test_proportion.py::test_ci_symmetry[True-normal-count6]",
"statsmodels/stats/tests/test_proportion.py::test_ci_symmetry_binom_test[True-count5-50]",
"statsmodels/stats/tests/test_proportion.py::test_ci_symmetry_array[wilson-count4]",
"statsmodels/stats/tests/test_proportion.py::test_ci_symmetry[False-jeffreys-count9]",
"statsmodels/stats/tests/test_proportion.py::test_ci_symmetry[False-binom_test-count4]",
"statsmodels/stats/tests/test_proportion.py::test_ci_symmetry_binom_test[True-count28-47]",
"statsmodels/stats/tests/test_proportion.py::test_ci_symmetry_array[jeffreys-count13]",
"statsmodels/stats/tests/test_proportion.py::test_ci_symmetry[False-jeffreys-count8]",
"statsmodels/stats/tests/test_proportion.py::test_ci_symmetry[False-normal-count2]",
"statsmodels/stats/tests/test_proportion.py::test_ci_symmetry[False-beta-count15]",
"statsmodels/stats/tests/test_proportion.py::test_ci_symmetry[False-beta-count9]",
"statsmodels/stats/tests/test_proportion.py::test_ci_symmetry_array[jeffreys-count8]",
"statsmodels/stats/tests/test_proportion.py::test_ci_symmetry_array[beta-count15]",
"statsmodels/stats/tests/test_proportion.py::test_ci_symmetry[False-agresti_coull-count13]",
"statsmodels/stats/tests/test_proportion.py::test_ci_symmetry_binom_test[False-count2-47]",
"statsmodels/stats/tests/test_proportion.py::test_ci_symmetry_binom_test[True-count37-50]",
"statsmodels/stats/tests/test_proportion.py::test_ci_symmetry_binom_test[False-count13-47]",
"statsmodels/stats/tests/test_proportion.py::test_ci_symmetry_binom_test[True-count20-47]",
"statsmodels/stats/tests/test_proportion.py::test_ci_symmetry_binom_test[True-count12-50]",
"statsmodels/stats/tests/test_proportion.py::test_ci_symmetry_binom_test[False-count38-47]",
"statsmodels/stats/tests/test_proportion.py::test_ci_symmetry_array[agresti_coull-count4]",
"statsmodels/stats/tests/test_proportion.py::test_ci_symmetry[True-jeffreys-count13]",
"statsmodels/stats/tests/test_proportion.py::test_proportion_ztests",
"statsmodels/stats/tests/test_proportion.py::test_ci_symmetry[True-wilson-count15]",
"statsmodels/stats/tests/test_proportion.py::test_ci_symmetry[False-binom_test-count10]",
"statsmodels/stats/tests/test_proportion.py::test_ci_symmetry[False-agresti_coull-count14]",
"statsmodels/stats/tests/test_proportion.py::test_ci_symmetry_binom_test[False-count21-50]",
"statsmodels/stats/tests/test_proportion.py::test_ci_symmetry_array[wilson-count5]",
"statsmodels/stats/tests/test_proportion.py::test_ci_symmetry_binom_test[True-count26-50]",
"statsmodels/stats/tests/test_proportion.py::test_ci_symmetry_binom_test[False-count32-50]",
"statsmodels/stats/tests/test_proportion.py::test_ci_symmetry_binom_test[False-count24-47]",
"statsmodels/stats/tests/test_proportion.py::test_ci_symmetry[False-normal-count5]",
"statsmodels/stats/tests/test_proportion.py::test_ci_symmetry_binom_test[False-count20-47]",
"statsmodels/stats/tests/test_proportion.py::test_int_check",
"statsmodels/stats/tests/test_proportion.py::test_ci_symmetry_array[beta-count13]",
"statsmodels/stats/tests/test_proportion.py::test_ci_symmetry[False-binom_test-count3]",
"statsmodels/stats/tests/test_proportion.py::test_ci_symmetry_binom_test[False-count22-50]",
"statsmodels/stats/tests/test_proportion.py::test_power_binom_tost",
"statsmodels/stats/tests/test_proportion.py::test_ci_symmetry_binom_test[True-count0-47]",
"statsmodels/stats/tests/test_proportion.py::test_ci_symmetry[False-jeffreys-count2]",
"statsmodels/stats/tests/test_proportion.py::test_ci_symmetry_array[binom_test-count12]",
"statsmodels/stats/tests/test_proportion.py::test_ci_symmetry_array[jeffreys-count3]",
"statsmodels/stats/tests/test_proportion.py::test_ci_symmetry_array[binom_test-count4]",
"statsmodels/stats/tests/test_proportion.py::test_ci_symmetry_array[beta-count5]",
"statsmodels/stats/tests/test_proportion.py::test_ci_symmetry_binom_test[False-count14-50]",
"statsmodels/stats/tests/test_proportion.py::test_ci_symmetry_binom_test[False-count16-50]",
"statsmodels/stats/tests/test_proportion.py::test_ci_symmetry_array[normal-count3]",
"statsmodels/stats/tests/test_proportion.py::test_ci_symmetry_binom_test[True-count43-50]",
"statsmodels/stats/tests/test_proportion.py::test_ci_symmetry_array[agresti_coull-count11]",
"statsmodels/stats/tests/test_proportion.py::test_ci_symmetry_binom_test[False-count17-47]",
"statsmodels/stats/tests/test_proportion.py::test_ci_symmetry[True-jeffreys-count3]",
"statsmodels/stats/tests/test_proportion.py::test_ci_symmetry_binom_test[False-count32-47]",
"statsmodels/stats/tests/test_proportion.py::test_ci_symmetry[False-beta-count6]",
"statsmodels/stats/tests/test_proportion.py::test_ci_symmetry_array[binom_test-count5]",
"statsmodels/stats/tests/test_proportion.py::test_ci_symmetry_binom_test[False-count9-50]",
"statsmodels/stats/tests/test_proportion.py::test_ci_symmetry_binom_test[False-count15-50]",
"statsmodels/stats/tests/test_proportion.py::test_ci_symmetry_array[normal-count2]",
"statsmodels/stats/tests/test_proportion.py::test_ci_symmetry[True-agresti_coull-count9]",
"statsmodels/stats/tests/test_proportion.py::test_ci_symmetry[True-jeffreys-count0]",
"statsmodels/stats/tests/test_proportion.py::test_ci_symmetry_binom_test[False-count19-50]",
"statsmodels/stats/tests/test_proportion.py::test_ci_symmetry_array[wilson-count2]",
"statsmodels/stats/tests/test_proportion.py::test_ci_symmetry_binom_test[True-count34-50]",
"statsmodels/stats/tests/test_proportion.py::test_ci_symmetry[True-jeffreys-count12]",
"statsmodels/stats/tests/test_proportion.py::test_ci_symmetry_binom_test[False-count47-47]",
"statsmodels/stats/tests/test_proportion.py::test_ci_symmetry_binom_test[False-count21-47]",
"statsmodels/stats/tests/test_proportion.py::test_ci_symmetry[False-jeffreys-count1]",
"statsmodels/stats/tests/test_proportion.py::test_ci_symmetry_array[beta-count3]",
"statsmodels/stats/tests/test_proportion.py::test_ci_symmetry_binom_test[True-count26-47]",
"statsmodels/stats/tests/test_proportion.py::test_ci_symmetry[True-normal-count0]",
"statsmodels/stats/tests/test_proportion.py::test_ci_symmetry[True-agresti_coull-count5]",
"statsmodels/stats/tests/test_proportion.py::test_ci_symmetry_binom_test[True-count39-50]",
"statsmodels/stats/tests/test_proportion.py::test_ci_symmetry_binom_test[True-count30-50]",
"statsmodels/stats/tests/test_proportion.py::test_equivalence_2indep",
"statsmodels/stats/tests/test_proportion.py::test_ci_symmetry_array[wilson-count7]",
"statsmodels/stats/tests/test_proportion.py::test_confint_2indep",
"statsmodels/stats/tests/test_proportion.py::test_ci_symmetry_array[jeffreys-count5]",
"statsmodels/stats/tests/test_proportion.py::test_confint_2indep_propcis",
"statsmodels/stats/tests/test_proportion.py::test_ci_symmetry_array[binom_test-count2]",
"statsmodels/stats/tests/test_proportion.py::test_ci_symmetry_binom_test[False-count27-50]",
"statsmodels/stats/tests/test_proportion.py::test_ci_symmetry_array[agresti_coull-count6]",
"statsmodels/stats/tests/test_proportion.py::test_ci_symmetry[True-wilson-count1]",
"statsmodels/stats/tests/test_proportion.py::test_power_2indep",
"statsmodels/stats/tests/test_proportion.py::test_ci_symmetry[False-wilson-count2]",
"statsmodels/stats/tests/test_proportion.py::test_ci_symmetry_array[normal-count8]",
"statsmodels/stats/tests/test_proportion.py::test_ci_symmetry_binom_test[False-count23-47]",
"statsmodels/stats/tests/test_proportion.py::test_ci_symmetry_binom_test[False-count8-50]",
"statsmodels/stats/tests/test_proportion.py::test_ci_symmetry_binom_test[True-count10-50]",
"statsmodels/stats/tests/test_proportion.py::test_ci_symmetry_binom_test[True-count1-50]",
"statsmodels/stats/tests/test_proportion.py::test_ci_symmetry_array[wilson-count12]",
"statsmodels/stats/tests/test_proportion.py::test_ci_symmetry[False-normal-count4]",
"statsmodels/stats/tests/test_proportion.py::test_ci_symmetry_binom_test[True-count14-50]",
"statsmodels/stats/tests/test_proportion.py::test_ci_symmetry[False-normal-count1]",
"statsmodels/stats/tests/test_proportion.py::test_ci_symmetry_binom_test[True-count24-47]",
"statsmodels/stats/tests/test_proportion.py::test_binom_test",
"statsmodels/stats/tests/test_proportion.py::test_ci_symmetry_binom_test[False-count2-50]",
"statsmodels/stats/tests/test_proportion.py::test_ci_symmetry_binom_test[False-count39-47]",
"statsmodels/stats/tests/test_proportion.py::test_ci_symmetry_array[agresti_coull-count10]",
"statsmodels/stats/tests/test_proportion.py::test_ci_symmetry[False-agresti_coull-count11]",
"statsmodels/stats/tests/test_proportion.py::test_ci_symmetry[True-wilson-count14]",
"statsmodels/stats/tests/test_proportion.py::test_ci_symmetry_array[binom_test-count1]",
"statsmodels/stats/tests/test_proportion.py::test_ci_symmetry[True-binom_test-count0]",
"statsmodels/stats/tests/test_proportion.py::test_ci_symmetry_binom_test[False-count11-47]",
"statsmodels/stats/tests/test_proportion.py::test_ci_symmetry_array[jeffreys-count7]",
"statsmodels/stats/tests/test_proportion.py::test_ci_symmetry_binom_test[False-count30-50]",
"statsmodels/stats/tests/test_proportion.py::test_ci_symmetry[True-wilson-count10]",
"statsmodels/stats/tests/test_proportion.py::test_ci_symmetry[False-wilson-count12]",
"statsmodels/stats/tests/test_proportion.py::test_ci_symmetry_array[normal-count15]",
"statsmodels/stats/tests/test_proportion.py::test_ci_symmetry[True-beta-count5]",
"statsmodels/stats/tests/test_proportion.py::test_ci_symmetry_binom_test[False-count43-50]",
"statsmodels/stats/tests/test_proportion.py::test_ci_symmetry[False-beta-count0]",
"statsmodels/stats/tests/test_proportion.py::test_ci_symmetry_binom_test[False-count36-50]",
"statsmodels/stats/tests/test_proportion.py::test_ci_symmetry[False-agresti_coull-count6]",
"statsmodels/stats/tests/test_proportion.py::test_ci_symmetry_binom_test[False-count18-47]",
"statsmodels/stats/tests/test_proportion.py::test_ci_symmetry_array[agresti_coull-count9]",
"statsmodels/stats/tests/test_proportion.py::test_ci_symmetry[False-normal-count13]",
"statsmodels/stats/tests/test_proportion.py::test_ci_symmetry[True-beta-count2]",
"statsmodels/stats/tests/test_proportion.py::test_ci_symmetry[False-binom_test-count15]",
"statsmodels/stats/tests/test_proportion.py::test_ci_symmetry_array[binom_test-count8]",
"statsmodels/stats/tests/test_proportion.py::test_ci_symmetry_binom_test[True-count21-50]",
"statsmodels/stats/tests/test_proportion.py::test_ci_symmetry_binom_test[True-count32-47]",
"statsmodels/stats/tests/test_proportion.py::test_ci_symmetry_binom_test[False-count27-47]",
"statsmodels/stats/tests/test_proportion.py::test_ci_symmetry_array[jeffreys-count15]",
"statsmodels/stats/tests/test_proportion.py::test_ci_symmetry_array[agresti_coull-count5]",
"statsmodels/stats/tests/test_proportion.py::test_ci_symmetry[True-wilson-count3]",
"statsmodels/stats/tests/test_proportion.py::test_ci_symmetry_binom_test[True-count29-47]",
"statsmodels/stats/tests/test_proportion.py::test_ci_symmetry[False-wilson-count15]",
"statsmodels/stats/tests/test_proportion.py::test_ci_symmetry[False-jeffreys-count5]",
"statsmodels/stats/tests/test_proportion.py::test_ci_symmetry_binom_test[True-count42-50]",
"statsmodels/stats/tests/test_proportion.py::test_ci_symmetry_binom_test[False-count43-47]",
"statsmodels/stats/tests/test_proportion.py::test_ci_symmetry_binom_test[False-count31-47]",
"statsmodels/stats/tests/test_proportion.py::test_ci_symmetry[True-beta-count1]",
"statsmodels/stats/tests/test_proportion.py::test_ci_symmetry[False-wilson-count10]",
"statsmodels/stats/tests/test_proportion.py::test_ci_symmetry_binom_test[True-count16-47]",
"statsmodels/stats/tests/test_proportion.py::test_ci_symmetry_array[beta-count12]",
"statsmodels/stats/tests/test_proportion.py::test_ci_symmetry_binom_test[True-count20-50]",
"statsmodels/stats/tests/test_proportion.py::test_ci_symmetry_binom_test[False-count16-47]",
"statsmodels/stats/tests/test_proportion.py::test_ci_symmetry[False-beta-count2]",
"statsmodels/stats/tests/test_proportion.py::test_ci_symmetry_array[binom_test-count13]",
"statsmodels/stats/tests/test_proportion.py::test_ci_symmetry_binom_test[True-count2-47]",
"statsmodels/stats/tests/test_proportion.py::test_ci_symmetry_binom_test[True-count19-47]",
"statsmodels/stats/tests/test_proportion.py::test_ci_symmetry_binom_test[True-count38-50]",
"statsmodels/stats/tests/test_proportion.py::test_ci_symmetry_array[beta-count9]",
"statsmodels/stats/tests/test_proportion.py::test_ci_symmetry[False-wilson-count13]",
"statsmodels/stats/tests/test_proportion.py::test_ci_symmetry_array[wilson-count15]",
"statsmodels/stats/tests/test_proportion.py::test_ci_symmetry[True-normal-count9]",
"statsmodels/stats/tests/test_proportion.py::test_ci_symmetry_binom_test[True-count18-47]",
"statsmodels/stats/tests/test_proportion.py::test_ci_symmetry[False-agresti_coull-count10]",
"statsmodels/stats/tests/test_proportion.py::test_ci_symmetry[True-agresti_coull-count0]",
"statsmodels/stats/tests/test_proportion.py::test_ci_symmetry_binom_test[True-count13-47]",
"statsmodels/stats/tests/test_proportion.py::test_ci_symmetry_binom_test[False-count1-50]",
"statsmodels/stats/tests/test_proportion.py::test_ci_symmetry_binom_test[False-count25-47]",
"statsmodels/stats/tests/test_proportion.py::test_ci_symmetry_binom_test[True-count18-50]",
"statsmodels/stats/tests/test_proportion.py::test_ci_symmetry[False-agresti_coull-count5]",
"statsmodels/stats/tests/test_proportion.py::test_ci_symmetry[False-binom_test-count1]",
"statsmodels/stats/tests/test_proportion.py::test_ci_symmetry_binom_test[False-count1-47]",
"statsmodels/stats/tests/test_proportion.py::test_ci_symmetry_binom_test[False-count45-50]",
"statsmodels/stats/tests/test_proportion.py::test_ci_symmetry[False-wilson-count3]",
"statsmodels/stats/tests/test_proportion.py::test_ci_symmetry_binom_test[False-count44-47]",
"statsmodels/stats/tests/test_proportion.py::test_ci_symmetry_binom_test[True-count46-50]",
"statsmodels/stats/tests/test_proportion.py::test_ci_symmetry[False-binom_test-count9]",
"statsmodels/stats/tests/test_proportion.py::test_ci_symmetry[True-agresti_coull-count11]",
"statsmodels/stats/tests/test_proportion.py::test_ci_symmetry[False-agresti_coull-count7]",
"statsmodels/stats/tests/test_proportion.py::test_ci_symmetry_binom_test[True-count13-50]",
"statsmodels/stats/tests/test_proportion.py::test_ci_symmetry_binom_test[False-count19-47]",
"statsmodels/stats/tests/test_proportion.py::test_ci_symmetry_array[beta-count10]",
"statsmodels/stats/tests/test_proportion.py::test_ci_symmetry_binom_test[False-count11-50]",
"statsmodels/stats/tests/test_proportion.py::test_ci_symmetry[True-wilson-count2]",
"statsmodels/stats/tests/test_proportion.py::test_ci_symmetry[False-beta-count12]",
"statsmodels/stats/tests/test_proportion.py::test_ci_symmetry_binom_test[True-count22-50]",
"statsmodels/stats/tests/test_proportion.py::test_ci_symmetry_binom_test[False-count0-50]",
"statsmodels/stats/tests/test_proportion.py::test_ci_symmetry[False-wilson-count5]",
"statsmodels/stats/tests/test_proportion.py::test_ci_symmetry_binom_test[False-count46-47]",
"statsmodels/stats/tests/test_proportion.py::test_ci_symmetry_binom_test[True-count6-50]",
"statsmodels/stats/tests/test_proportion.py::test_ci_symmetry[True-beta-count4]",
"statsmodels/stats/tests/test_proportion.py::test_ci_symmetry[True-normal-count11]",
"statsmodels/stats/tests/test_proportion.py::test_ci_symmetry_array[normal-count11]",
"statsmodels/stats/tests/test_proportion.py::test_ci_symmetry[False-normal-count7]",
"statsmodels/stats/tests/test_proportion.py::test_ci_symmetry[True-normal-count8]",
"statsmodels/stats/tests/test_proportion.py::test_ci_symmetry_binom_test[True-count29-50]",
"statsmodels/stats/tests/test_proportion.py::test_ci_symmetry[True-jeffreys-count5]",
"statsmodels/stats/tests/test_proportion.py::test_ci_symmetry_array[jeffreys-count1]",
"statsmodels/stats/tests/test_proportion.py::test_ci_symmetry_array[beta-count14]",
"statsmodels/stats/tests/test_proportion.py::test_ci_symmetry_array[jeffreys-count12]",
"statsmodels/stats/tests/test_proportion.py::test_ci_symmetry_binom_test[True-count11-50]",
"statsmodels/stats/tests/test_proportion.py::test_ci_symmetry_binom_test[True-count30-47]",
"statsmodels/stats/tests/test_proportion.py::test_ci_symmetry[True-jeffreys-count6]",
"statsmodels/stats/tests/test_proportion.py::test_ci_symmetry_array[jeffreys-count14]",
"statsmodels/stats/tests/test_proportion.py::test_ci_symmetry_binom_test[True-count16-50]",
"statsmodels/stats/tests/test_proportion.py::test_ci_symmetry_array[wilson-count1]",
"statsmodels/stats/tests/test_proportion.py::test_ci_symmetry[True-binom_test-count10]",
"statsmodels/stats/tests/test_proportion.py::test_ci_symmetry[False-binom_test-count6]",
"statsmodels/stats/tests/test_proportion.py::test_ci_symmetry[True-normal-count4]",
"statsmodels/stats/tests/test_proportion.py::test_ci_symmetry[False-beta-count13]",
"statsmodels/stats/tests/test_proportion.py::test_ci_symmetry[False-beta-count8]",
"statsmodels/stats/tests/test_proportion.py::test_binom_rejection_interval",
"statsmodels/stats/tests/test_proportion.py::test_ci_symmetry[True-agresti_coull-count14]",
"statsmodels/stats/tests/test_proportion.py::test_ci_symmetry_binom_test[True-count40-47]",
"statsmodels/stats/tests/test_proportion.py::test_ci_symmetry[True-normal-count10]",
"statsmodels/stats/tests/test_proportion.py::test_ci_symmetry_binom_test[False-count33-47]",
"statsmodels/stats/tests/test_proportion.py::test_ci_symmetry_binom_test[False-count10-47]",
"statsmodels/stats/tests/test_proportion.py::test_ci_symmetry[True-wilson-count9]",
"statsmodels/stats/tests/test_proportion.py::test_ci_symmetry_binom_test[False-count42-47]",
"statsmodels/stats/tests/test_proportion.py::test_ci_symmetry_binom_test[True-count35-50]",
"statsmodels/stats/tests/test_proportion.py::test_ci_symmetry_array[binom_test-count10]",
"statsmodels/stats/tests/test_proportion.py::test_ci_symmetry_binom_test[True-count25-47]",
"statsmodels/stats/tests/test_proportion.py::test_ci_symmetry_binom_test[True-count27-47]",
"statsmodels/stats/tests/test_proportion.py::test_ci_symmetry_binom_test[True-count14-47]",
"statsmodels/stats/tests/test_proportion.py::test_ci_symmetry[False-jeffreys-count7]",
"statsmodels/stats/tests/test_proportion.py::test_ci_symmetry_array[wilson-count11]",
"statsmodels/stats/tests/test_proportion.py::test_ci_symmetry_binom_test[True-count36-47]",
"statsmodels/stats/tests/test_proportion.py::test_ci_symmetry[True-beta-count12]",
"statsmodels/stats/tests/test_proportion.py::test_ci_symmetry[False-binom_test-count0]",
"statsmodels/stats/tests/test_proportion.py::test_ci_symmetry_array[jeffreys-count6]",
"statsmodels/stats/tests/test_proportion.py::test_ci_symmetry[True-binom_test-count14]",
"statsmodels/stats/tests/test_proportion.py::test_ci_symmetry_binom_test[False-count14-47]",
"statsmodels/stats/tests/test_proportion.py::test_ci_symmetry_binom_test[True-count35-47]",
"statsmodels/stats/tests/test_proportion.py::test_ci_symmetry[True-binom_test-count4]",
"statsmodels/stats/tests/test_proportion.py::test_ci_symmetry[True-wilson-count13]",
"statsmodels/stats/tests/test_proportion.py::test_ci_symmetry[False-normal-count0]",
"statsmodels/stats/tests/test_proportion.py::test_ci_symmetry_array[beta-count4]",
"statsmodels/stats/tests/test_proportion.py::test_ci_symmetry_array[wilson-count3]",
"statsmodels/stats/tests/test_proportion.py::test_ci_symmetry_binom_test[False-count18-50]",
"statsmodels/stats/tests/test_proportion.py::test_ci_symmetry[False-normal-count14]",
"statsmodels/stats/tests/test_proportion.py::test_ci_symmetry[True-binom_test-count2]",
"statsmodels/stats/tests/test_proportion.py::test_ci_symmetry[False-normal-count6]",
"statsmodels/stats/tests/test_proportion.py::test_ci_symmetry[True-binom_test-count1]",
"statsmodels/stats/tests/test_proportion.py::test_ci_symmetry_binom_test[False-count26-50]",
"statsmodels/stats/tests/test_proportion.py::test_ci_symmetry_array[jeffreys-count4]",
"statsmodels/stats/tests/test_proportion.py::test_ci_symmetry_array[normal-count9]",
"statsmodels/stats/tests/test_proportion.py::test_ci_symmetry_array[beta-count8]",
"statsmodels/stats/tests/test_proportion.py::test_ci_symmetry[True-binom_test-count15]",
"statsmodels/stats/tests/test_proportion.py::test_ci_symmetry[False-jeffreys-count4]",
"statsmodels/stats/tests/test_proportion.py::test_ci_symmetry_binom_test[False-count30-47]",
"statsmodels/stats/tests/test_proportion.py::test_ci_symmetry_binom_test[True-count34-47]",
"statsmodels/stats/tests/test_proportion.py::test_ci_symmetry[True-beta-count3]",
"statsmodels/stats/tests/test_proportion.py::test_ci_symmetry[False-wilson-count0]",
"statsmodels/stats/tests/test_proportion.py::test_ci_symmetry[True-beta-count6]",
"statsmodels/stats/tests/test_proportion.py::test_ci_symmetry[False-normal-count10]",
"statsmodels/stats/tests/test_proportion.py::test_ci_symmetry_binom_test[True-count2-50]",
"statsmodels/stats/tests/test_proportion.py::test_ci_symmetry_array[normal-count1]",
"statsmodels/stats/tests/test_proportion.py::test_ci_symmetry[True-binom_test-count11]",
"statsmodels/stats/tests/test_proportion.py::test_ci_symmetry[False-wilson-count1]",
"statsmodels/stats/tests/test_proportion.py::test_ci_symmetry_array[agresti_coull-count12]",
"statsmodels/stats/tests/test_proportion.py::test_ci_symmetry_binom_test[True-count10-47]",
"statsmodels/stats/tests/test_proportion.py::test_ci_symmetry[True-binom_test-count7]",
"statsmodels/stats/tests/test_proportion.py::test_ci_symmetry[False-agresti_coull-count1]",
"statsmodels/stats/tests/test_proportion.py::test_ci_symmetry[False-binom_test-count11]",
"statsmodels/stats/tests/test_proportion.py::test_ci_symmetry[True-wilson-count0]",
"statsmodels/stats/tests/test_proportion.py::test_ci_symmetry_binom_test[True-count0-50]",
"statsmodels/stats/tests/test_proportion.py::test_ci_symmetry[False-binom_test-count2]",
"statsmodels/stats/tests/test_proportion.py::test_ci_symmetry[False-beta-count7]",
"statsmodels/stats/tests/test_proportion.py::test_ci_symmetry[False-beta-count3]",
"statsmodels/stats/tests/test_proportion.py::test_ci_symmetry[True-jeffreys-count14]",
"statsmodels/stats/tests/test_proportion.py::test_ci_symmetry_binom_test[True-count36-50]",
"statsmodels/stats/tests/test_proportion.py::test_ci_symmetry[True-jeffreys-count1]",
"statsmodels/stats/tests/test_proportion.py::test_ci_symmetry_binom_test[False-count23-50]",
"statsmodels/stats/tests/test_proportion.py::test_ci_symmetry_array[agresti_coull-count7]",
"statsmodels/stats/tests/test_proportion.py::test_ci_symmetry_binom_test[False-count40-47]",
"statsmodels/stats/tests/test_proportion.py::test_ci_symmetry_binom_test[True-count5-47]",
"statsmodels/stats/tests/test_proportion.py::test_ci_symmetry[True-binom_test-count9]",
"statsmodels/stats/tests/test_proportion.py::test_ci_symmetry_binom_test[False-count4-47]",
"statsmodels/stats/tests/test_proportion.py::test_ci_symmetry_binom_test[False-count0-47]",
"statsmodels/stats/tests/test_proportion.py::test_ci_symmetry[False-beta-count5]",
"statsmodels/stats/tests/test_proportion.py::test_ci_symmetry_binom_test[False-count29-47]",
"statsmodels/stats/tests/test_proportion.py::test_ci_symmetry_binom_test[True-count9-50]",
"statsmodels/stats/tests/test_proportion.py::test_ci_symmetry[False-beta-count14]",
"statsmodels/stats/tests/test_proportion.py::test_ci_symmetry_array[normal-count10]",
"statsmodels/stats/tests/test_proportion.py::test_ci_symmetry_binom_test[False-count20-50]",
"statsmodels/stats/tests/test_proportion.py::test_ci_symmetry[False-jeffreys-count3]",
"statsmodels/stats/tests/test_proportion.py::test_ci_symmetry_binom_test[True-count7-50]",
"statsmodels/stats/tests/test_proportion.py::test_ci_symmetry_binom_test[False-count29-50]",
"statsmodels/stats/tests/test_proportion.py::test_ci_symmetry[True-jeffreys-count10]",
"statsmodels/stats/tests/test_proportion.py::test_ci_symmetry_binom_test[False-count37-47]",
"statsmodels/stats/tests/test_proportion.py::test_ci_symmetry[False-wilson-count6]",
"statsmodels/stats/tests/test_proportion.py::test_ci_symmetry_binom_test[False-count7-50]",
"statsmodels/stats/tests/test_proportion.py::test_ci_symmetry_array[normal-count5]",
"statsmodels/stats/tests/test_proportion.py::test_ci_symmetry[False-wilson-count14]",
"statsmodels/stats/tests/test_proportion.py::test_ci_symmetry_array[agresti_coull-count0]",
"statsmodels/stats/tests/test_proportion.py::test_ci_symmetry_binom_test[False-count3-47]",
"statsmodels/stats/tests/test_proportion.py::test_ci_symmetry[True-jeffreys-count8]",
"statsmodels/stats/tests/test_proportion.py::test_ci_symmetry[True-agresti_coull-count3]",
"statsmodels/stats/tests/test_proportion.py::test_ci_symmetry_array[agresti_coull-count2]",
"statsmodels/stats/tests/test_proportion.py::test_ci_symmetry[True-wilson-count11]",
"statsmodels/stats/tests/test_proportion.py::test_ci_symmetry_binom_test[False-count39-50]",
"statsmodels/stats/tests/test_proportion.py::test_ci_symmetry_binom_test[True-count45-50]",
"statsmodels/stats/tests/test_proportion.py::test_ci_symmetry_binom_test[True-count1-47]",
"statsmodels/stats/tests/test_proportion.py::test_ci_symmetry_array[binom_test-count7]",
"statsmodels/stats/tests/test_proportion.py::test_ci_symmetry[True-binom_test-count8]",
"statsmodels/stats/tests/test_proportion.py::test_ci_symmetry[True-normal-count14]",
"statsmodels/stats/tests/test_proportion.py::test_ci_symmetry[True-beta-count15]",
"statsmodels/stats/tests/test_proportion.py::test_ci_symmetry[False-binom_test-count8]",
"statsmodels/stats/tests/test_proportion.py::test_ci_symmetry_binom_test[False-count12-50]",
"statsmodels/stats/tests/test_proportion.py::test_ci_symmetry_binom_test[False-count34-50]",
"statsmodels/stats/tests/test_proportion.py::test_ci_symmetry_array[normal-count6]",
"statsmodels/stats/tests/test_proportion.py::test_ci_symmetry_array[normal-count13]",
"statsmodels/stats/tests/test_proportion.py::test_ci_symmetry_binom_test[True-count23-50]",
"statsmodels/stats/tests/test_proportion.py::test_ci_symmetry_binom_test[False-count25-50]",
"statsmodels/stats/tests/test_proportion.py::test_ci_symmetry_binom_test[True-count24-50]",
"statsmodels/stats/tests/test_proportion.py::test_ci_symmetry_binom_test[False-count17-50]",
"statsmodels/stats/tests/test_proportion.py::test_ci_symmetry[False-jeffreys-count0]",
"statsmodels/stats/tests/test_proportion.py::test_ci_symmetry_array[beta-count1]",
"statsmodels/stats/tests/test_proportion.py::test_ci_symmetry[False-jeffreys-count14]",
"statsmodels/stats/tests/test_proportion.py::test_ci_symmetry[True-wilson-count12]",
"statsmodels/stats/tests/test_proportion.py::test_ci_symmetry[False-normal-count8]",
"statsmodels/stats/tests/test_proportion.py::test_ci_symmetry_array[binom_test-count15]",
"statsmodels/stats/tests/test_proportion.py::test_ci_symmetry_binom_test[True-count15-50]",
"statsmodels/stats/tests/test_proportion.py::test_ci_symmetry[True-normal-count15]",
"statsmodels/stats/tests/test_proportion.py::test_ci_symmetry_binom_test[True-count28-50]",
"statsmodels/stats/tests/test_proportion.py::test_ci_symmetry_binom_test[True-count31-50]",
"statsmodels/stats/tests/test_proportion.py::test_ci_symmetry[True-beta-count10]",
"statsmodels/stats/tests/test_proportion.py::test_ci_symmetry_binom_test[True-count44-50]",
"statsmodels/stats/tests/test_proportion.py::test_ci_symmetry_array[beta-count11]",
"statsmodels/stats/tests/test_proportion.py::test_ci_symmetry_binom_test[False-count8-47]",
"statsmodels/stats/tests/test_proportion.py::test_binom_tost",
"statsmodels/stats/tests/test_proportion.py::test_ci_symmetry_array[agresti_coull-count15]",
"statsmodels/stats/tests/test_proportion.py::test_ci_symmetry_binom_test[True-count4-50]",
"statsmodels/stats/tests/test_proportion.py::test_ci_symmetry_array[agresti_coull-count1]",
"statsmodels/stats/tests/test_proportion.py::test_ci_symmetry_array[agresti_coull-count3]",
"statsmodels/stats/tests/test_proportion.py::test_ci_symmetry[False-binom_test-count7]",
"statsmodels/stats/tests/test_proportion.py::test_ci_symmetry_binom_test[True-count17-47]"
] | [
"statsmodels/stats/tests/test_proportion.py::test_proportion_confint[jeffreys-0.05-two-sided-expected6-200-100]",
"statsmodels/stats/tests/test_proportion.py::test_proportion_confint[beta-0.05-larger-expected9-200-100]",
"statsmodels/stats/tests/test_proportion.py::test_proportion_confint_binom_test[0.01-larger-expected3-1e-07-200-100]",
"statsmodels/stats/tests/test_proportion.py::test_proportion_confint[normal-0.05-two-sided-expected0-200-100]",
"statsmodels/stats/tests/test_proportion.py::test_proportion_confint[beta-0.05-two-sided-expected4-200-100]",
"statsmodels/stats/tests/test_proportion.py::test_proportion_confint_binom_test[0.05-larger-expected4-1e-07-200-100]",
"statsmodels/stats/tests/test_proportion.py::test_proportion_confint_edge[1-1-larger-expected2]",
"statsmodels/stats/tests/test_proportion.py::test_proportion_confint_binom_test[0.1-larger-expected5-1e-07-200-100]",
"statsmodels/stats/tests/test_proportion.py::test_proportion_confint_edge[0-1-two-sided-expected1]",
"statsmodels/stats/tests/test_proportion.py::test_proportion_confint[beta-0.05-smaller-expected14-200-100]",
"statsmodels/stats/tests/test_proportion.py::test_proportion_confint_binom_test[0.1-two-sided-expected2-0.0001-200-100]",
"statsmodels/stats/tests/test_proportion.py::test_proportion_confint[agresti_coull-0.05-larger-expected8-200-100]",
"statsmodels/stats/tests/test_proportion.py::test_proportion_confint_edge[0-1-smaller-expected5]",
"statsmodels/stats/tests/test_proportion.py::test_proportion_confint[wilson-0.05-smaller-expected15-200-100]",
"statsmodels/stats/tests/test_proportion.py::test_proportion_confint[jeffreys-0.05-smaller-expected16-200-100]",
"statsmodels/stats/tests/test_proportion.py::test_proportion_confint_edge[0-1-larger-expected3]",
"statsmodels/stats/tests/test_proportion.py::test_proportion_confint_binom_test[0.05-smaller-expected7-1e-07-200-100]",
"statsmodels/stats/tests/test_proportion.py::test_proportion_confint_edge[1-1-smaller-expected4]",
"statsmodels/stats/tests/test_proportion.py::test_proportion_confint[normal-0.05-larger-expected7-200-100]",
"statsmodels/stats/tests/test_proportion.py::test_proportion_confint[jeffreys-0.05-larger-expected11-200-100]",
"statsmodels/stats/tests/test_proportion.py::test_proportion_confint[normal-0.01-two-sided-expected1-200-100]",
"statsmodels/stats/tests/test_proportion.py::test_proportion_confint[wilson-0.05-two-sided-expected5-200-100]",
"statsmodels/stats/tests/test_proportion.py::test_proportion_confint_binom_test[0.1-smaller-expected8-1e-07-200-100]",
"statsmodels/stats/tests/test_proportion.py::test_proportion_confint_edge[1-1-two-sided-expected0]",
"statsmodels/stats/tests/test_proportion.py::test_proportion_confint[agresti_coull-0.05-smaller-expected13-200-100]",
"statsmodels/stats/tests/test_proportion.py::test_proportion_confint_binom_test[0.01-two-sided-expected0-0.0001-200-100]",
"statsmodels/stats/tests/test_proportion.py::test_proportion_confint_binom_test[0.01-smaller-expected6-1e-07-200-100]",
"statsmodels/stats/tests/test_proportion.py::test_proportion_confint[agresti_coull-0.05-two-sided-expected3-200-100]",
"statsmodels/stats/tests/test_proportion.py::test_proportion_confint[normal-0.05-smaller-expected12-200-100]",
"statsmodels/stats/tests/test_proportion.py::test_proportion_confint_binom_test[0.05-two-sided-expected1-0.0001-200-100]",
"statsmodels/stats/tests/test_proportion.py::test_proportion_confint[normal-0.1-two-sided-expected2-200-100]",
"statsmodels/stats/tests/test_proportion.py::test_proportion_confint[wilson-0.05-larger-expected10-200-100]"
] | Python | [] | [] |
statsmodels/statsmodels | 9,255 | statsmodels__statsmodels-9255 | [
"9254"
] | bf91082711ce95a5bbca557c4d9ab79afb990dbd | diff --git a/statsmodels/stats/rates.py b/statsmodels/stats/rates.py
--- a/statsmodels/stats/rates.py
+++ b/statsmodels/stats/rates.py
@@ -186,15 +186,15 @@ def test_poisson(count, nobs, value, method=None, alternative="two-sided",
return res
-def confint_poisson(count, exposure, method=None, alpha=0.05):
+def confint_poisson(count, exposure, method=None, alpha=0.05,
+ alternative="two-sided"):
"""Confidence interval for a Poisson mean or rate
The function is vectorized for all methods except "midp-c", which uses
an iterative method to invert the hypothesis test function.
All current methods are central, that is the probability of each tail is
- smaller or equal to alpha / 2. The one-sided interval limits can be
- obtained by doubling alpha.
+ smaller or equal to alpha / 2.
Parameters
----------
@@ -209,10 +209,16 @@ def confint_poisson(count, exposure, method=None, alpha=0.05):
alpha : float in (0, 1)
Significance level, nominal coverage of the confidence interval is
1 - alpha.
+ alternative : {"two-sider", "larger", "smaller")
+ default: "two-sided"
+ Specifies whether to calculate a two-sided or one-sided confidence
+ interval.
Returns
-------
tuple (low, upp) : confidence limits.
+ When alternative is not "two-sided", lower or upper bound is set to
+ 0 or inf respectively.
Notes
-----
@@ -270,7 +276,12 @@ def confint_poisson(count, exposure, method=None, alpha=0.05):
"""
n = exposure # short hand
rate = count / exposure
- alpha = alpha / 2 # two-sided
+
+ if alternative == 'two-sided':
+ alpha = alpha / 2
+ elif alternative not in ['larger', 'smaller']:
+ raise NotImplementedError(
+ f"alternative {alternative} is not available")
if method is None:
msg = "method needs to be specified, currently no default method"
@@ -365,6 +376,11 @@ def confint_poisson(count, exposure, method=None, alpha=0.05):
else:
raise ValueError("unknown method %s" % method)
+ if alternative == "larger":
+ ci = (0, ci[1])
+ elif alternative == "smaller":
+ ci = (ci[0], np.inf)
+
ci = (np.maximum(ci[0], 0), ci[1])
return ci
| diff --git a/statsmodels/stats/tests/test_rates_poisson.py b/statsmodels/stats/tests/test_rates_poisson.py
--- a/statsmodels/stats/tests/test_rates_poisson.py
+++ b/statsmodels/stats/tests/test_rates_poisson.py
@@ -1146,3 +1146,24 @@ def test_power_negbin():
assert_allclose(pow_p, pow1, atol=5e-2)
assert_allclose(pow_p, pow_, rtol=1e-13)
+
+
[email protected]('count', [0, 1, 10, 100])
[email protected]('exposure', [1, 10, 100, 1000])
[email protected]('alpha', [0.01, 0.05, 0.1])
[email protected]('method',
+ method_names_poisson_1samp['confint'])
[email protected]('alternative', ['larger', 'smaller'])
+def test_confint_poisson_alternative(count, exposure, method, alpha,
+ alternative):
+ # regression test
+ two_sided_ci = confint_poisson(count, exposure, method=method,
+ alpha=alpha * 2, alternative='two-sided')
+ one_sided_ci = confint_poisson(count, exposure, method=method,
+ alpha=alpha, alternative=alternative)
+ if alternative == 'larger':
+ two_sided_ci = (0, two_sided_ci[1])
+ assert_allclose(one_sided_ci, two_sided_ci, rtol=1e-12)
+ elif alternative == 'smaller':
+ two_sided_ci = (two_sided_ci[0], np.inf)
+ assert_allclose(one_sided_ci, two_sided_ci, rtol=1e-12)
| ENH: confint_poisson only has two-sided confidence intervals
https://www.statsmodels.org/dev/generated/statsmodels.stats.rates.confint_poisson.html
confint_poisson does not have an one-sided confidence interval option.
As mentioned in #9249, add a new option 'alternative' to confint_poisson.
Maybe same for multinomial_proportions_confint, later.
| for docstrings:
I will come up with a short explanation for the meaning and direction of alternative option for confidence intervals. Then, we can copy it to all confint functions that have the alternative keyword.
one sided confint for multinomial_proportions_confint will, most likely, not be obvious and we might have to check with the original articles.
For docstrings, should we create a new issue and PR like 'DOCS: XXX'? | "2024-05-26T00:37:06Z" | 0.14 | [
"statsmodels/stats/tests/test_rates_poisson.py::TestMethodsCompare2indep::test_test[diff-score]",
"statsmodels/stats/tests/test_rates_poisson.py::TestMethodsCompare2indep::test_test_vectorized[ratio-exact-cond]",
"statsmodels/stats/tests/test_rates_poisson.py::TestMethodsCompare2indep::test_confint[diff-wald]",
"statsmodels/stats/tests/test_rates_poisson.py::TestMethodsCompare2indep::test_test[ratio-etest-wald]",
"statsmodels/stats/tests/test_rates_poisson.py::TestMethodsCompare2indep::test_test[ratio-exact-cond]",
"statsmodels/stats/tests/test_rates_poisson.py::TestMethodsCompare2indep::test_test[ratio-etest-score]",
"statsmodels/stats/tests/test_rates_poisson.py::TestMethodsCompare2indep::test_test[diff-wald]",
"statsmodels/stats/tests/test_rates_poisson.py::TestMethodsCompare2indep::test_test[ratio-score-log]",
"statsmodels/stats/tests/test_rates_poisson.py::TestMethodsCompare2indep::test_test[ratio-score]",
"statsmodels/stats/tests/test_rates_poisson.py::TestMethodsCompare2indep::test_test_vectorized[ratio-score]",
"statsmodels/stats/tests/test_rates_poisson.py::TestMethodsCompare2indep::test_confint[ratio-sqrtcc]",
"statsmodels/stats/tests/test_rates_poisson.py::TestMethodsCompare2indep::test_confint[ratio-wald-log]",
"statsmodels/stats/tests/test_rates_poisson.py::TestMethodsCompare2indep::test_test_vectorized[diff-score]",
"statsmodels/stats/tests/test_rates_poisson.py::TestMethodsCompare2indep::test_confint[diff-score]",
"statsmodels/stats/tests/test_rates_poisson.py::TestMethodsCompare2indep::test_confint[ratio-score]",
"statsmodels/stats/tests/test_rates_poisson.py::TestMethodsCompare2indep::test_confint[diff-mover]",
"statsmodels/stats/tests/test_rates_poisson.py::TestMethodsCompare2indep::test_test_vectorized[diff-wald]",
"statsmodels/stats/tests/test_rates_poisson.py::TestMethodsCompare2indep::test_test[ratio-cond-midp]",
"statsmodels/stats/tests/test_rates_poisson.py::TestMethodsCompare2indep::test_test[ratio-wald-log]",
"statsmodels/stats/tests/test_rates_poisson.py::TestMethodsCompare2indep::test_confint[ratio-score-log]",
"statsmodels/stats/tests/test_rates_poisson.py::TestMethodsCompare2indep::test_test_vectorized[diff-waldccv]",
"statsmodels/stats/tests/test_rates_poisson.py::TestMethodsCompare2indep::test_test_vectorized[ratio-wald]",
"statsmodels/stats/tests/test_rates_poisson.py::TestMethodsCompare2indep::test_test[diff-etest-score]",
"statsmodels/stats/tests/test_rates_poisson.py::TestMethodsCompare2indep::test_test_vectorized[ratio-sqrt]",
"statsmodels/stats/tests/test_rates_poisson.py::TestMethodsCompare2indep::test_test_vectorized[ratio-cond-midp]",
"statsmodels/stats/tests/test_rates_poisson.py::TestMethodsCompare2indep::test_confint[ratio-mover]",
"statsmodels/stats/tests/test_rates_poisson.py::TestMethodsCompare2indep::test_test[ratio-wald]",
"statsmodels/stats/tests/test_rates_poisson.py::TestMethodsCompare2indep::test_test[ratio-sqrt]",
"statsmodels/stats/tests/test_rates_poisson.py::TestMethodsCompare2indep::test_confint[diff-waldccv]",
"statsmodels/stats/tests/test_rates_poisson.py::TestMethodsCompare2indep::test_test_vectorized[ratio-wald-log]",
"statsmodels/stats/tests/test_rates_poisson.py::TestMethodsCompare2indep::test_test_vectorized[ratio-score-log]",
"statsmodels/stats/tests/test_rates_poisson.py::TestMethodsCompare2indep::test_confint[ratio-waldcc]",
"statsmodels/stats/tests/test_rates_poisson.py::TestMethodsCompare2indep::test_test[diff-waldccv]",
"statsmodels/stats/tests/test_rates_poisson.py::TestMethodsCompare2indep::test_test[diff-etest-wald]",
"statsmodels/stats/tests/test_rates_poisson.py::TestMethodsCompar1samp::test_confint[sqrt-a]",
"statsmodels/stats/tests/test_rates_poisson.py::TestMethodsCompar1samp::test_confint[waldccv]",
"statsmodels/stats/tests/test_rates_poisson.py::TestMethodsCompar1samp::test_test[exact-c]",
"statsmodels/stats/tests/test_rates_poisson.py::TestMethodsCompar1samp::test_confint[sqrt-centcc]",
"statsmodels/stats/tests/test_rates_poisson.py::TestMethodsCompar1samp::test_test[sqrt-v]",
"statsmodels/stats/tests/test_rates_poisson.py::TestMethodsCompar1samp::test_test[sqrt-a]",
"statsmodels/stats/tests/test_rates_poisson.py::TestMethodsCompar1samp::test_test[score]",
"statsmodels/stats/tests/test_rates_poisson.py::TestMethodsCompar1samp::test_confint[sqrt-cent]",
"statsmodels/stats/tests/test_rates_poisson.py::TestMethodsCompar1samp::test_confint[sqrt]",
"statsmodels/stats/tests/test_rates_poisson.py::TestMethodsCompar1samp::test_test[midp-c]",
"statsmodels/stats/tests/test_rates_poisson.py::TestMethodsCompar1samp::test_test[wald]",
"statsmodels/stats/tests/test_rates_poisson.py::TestMethodsCompar1samp::test_confint[sqrt-v]",
"statsmodels/stats/tests/test_rates_poisson.py::TestMethodsCompar1samp::test_confint[jeff]",
"statsmodels/stats/tests/test_rates_poisson.py::TestMethodsCompar1samp::test_confint[exact-c]",
"statsmodels/stats/tests/test_rates_poisson.py::TestMethodsCompar1samp::test_confint[score]",
"statsmodels/stats/tests/test_rates_poisson.py::TestMethodsCompar1samp::test_confint[wald]",
"statsmodels/stats/tests/test_rates_poisson.py::TestMethodsCompar1samp::test_test[sqrt]",
"statsmodels/stats/tests/test_rates_poisson.py::TestMethodsCompar1samp::test_confint[midp-c]",
"statsmodels/stats/tests/test_rates_poisson.py::TestMethodsCompar1samp::test_test[waldccv]",
"statsmodels/stats/tests/test_rates_poisson.py::test_rate_poisson_r",
"statsmodels/stats/tests/test_rates_poisson.py::test_tol_int[case4]",
"statsmodels/stats/tests/test_rates_poisson.py::test_rate_poisson_consistency[sqrt-a]",
"statsmodels/stats/tests/test_rates_poisson.py::test_tol_int[case2]",
"statsmodels/stats/tests/test_rates_poisson.py::test_rate_poisson_consistency[midp-c]",
"statsmodels/stats/tests/test_rates_poisson.py::test_tol_int[case3]",
"statsmodels/stats/tests/test_rates_poisson.py::test_rate_poisson_consistency[waldccv]",
"statsmodels/stats/tests/test_rates_poisson.py::test_rate_poisson_consistency[wald]",
"statsmodels/stats/tests/test_rates_poisson.py::test_rate_poisson_consistency[score]",
"statsmodels/stats/tests/test_rates_poisson.py::test_rate_poisson_consistency[sqrt]",
"statsmodels/stats/tests/test_rates_poisson.py::test_tol_int[case1]",
"statsmodels/stats/tests/test_rates_poisson.py::test_rate_poisson_consistency[sqrt-v]",
"statsmodels/stats/tests/test_rates_poisson.py::test_rate_poisson_consistency[exact-c]",
"statsmodels/stats/tests/test_rates_poisson.py::test_tol_int[case0]",
"statsmodels/stats/tests/test_rates_poisson.py::test_alternative[case10]",
"statsmodels/stats/tests/test_rates_poisson.py::test_rate_poisson_ratio_consistency[wald-log]",
"statsmodels/stats/tests/test_rates_poisson.py::test_alternative[case19]",
"statsmodels/stats/tests/test_rates_poisson.py::test_alternative[case13]",
"statsmodels/stats/tests/test_rates_poisson.py::test_rate_poisson_diff_consistency[waldccv]",
"statsmodels/stats/tests/test_rates_poisson.py::test_twosample_poisson",
"statsmodels/stats/tests/test_rates_poisson.py::test_rate_poisson_diff_ratio_consistency[etest]",
"statsmodels/stats/tests/test_rates_poisson.py::test_confint_poisson_2indep",
"statsmodels/stats/tests/test_rates_poisson.py::test_alternative[case14]",
"statsmodels/stats/tests/test_rates_poisson.py::test_alternative[case6]",
"statsmodels/stats/tests/test_rates_poisson.py::test_alternative[case8]",
"statsmodels/stats/tests/test_rates_poisson.py::test_alternative[case3]",
"statsmodels/stats/tests/test_rates_poisson.py::test_alternative[case0]",
"statsmodels/stats/tests/test_rates_poisson.py::test_alternative[case11]",
"statsmodels/stats/tests/test_rates_poisson.py::test_alternative[case15]",
"statsmodels/stats/tests/test_rates_poisson.py::test_rate_poisson_diff_ratio_consistency[wald]",
"statsmodels/stats/tests/test_rates_poisson.py::test_alternative[case4]",
"statsmodels/stats/tests/test_rates_poisson.py::test_twosample_poisson_r",
"statsmodels/stats/tests/test_rates_poisson.py::test_alternative[case12]",
"statsmodels/stats/tests/test_rates_poisson.py::test_alternative[case16]",
"statsmodels/stats/tests/test_rates_poisson.py::test_alternative[case9]",
"statsmodels/stats/tests/test_rates_poisson.py::test_twosample_poisson_diff[case2]",
"statsmodels/stats/tests/test_rates_poisson.py::test_tost_poisson",
"statsmodels/stats/tests/test_rates_poisson.py::test_rate_poisson_diff_consistency[score]",
"statsmodels/stats/tests/test_rates_poisson.py::test_alternative[case2]",
"statsmodels/stats/tests/test_rates_poisson.py::test_twosample_poisson_diff[case3]",
"statsmodels/stats/tests/test_rates_poisson.py::test_rate_poisson_diff_ratio_consistency[etest-wald]",
"statsmodels/stats/tests/test_rates_poisson.py::test_twosample_poisson_diff[case1]",
"statsmodels/stats/tests/test_rates_poisson.py::test_alternative[case18]",
"statsmodels/stats/tests/test_rates_poisson.py::test_rate_poisson_diff_consistency[wald]",
"statsmodels/stats/tests/test_rates_poisson.py::test_alternative[case1]",
"statsmodels/stats/tests/test_rates_poisson.py::test_alternative[case20]",
"statsmodels/stats/tests/test_rates_poisson.py::test_twosample_poisson_diff[case0]",
"statsmodels/stats/tests/test_rates_poisson.py::test_rate_poisson_diff_ratio_consistency[score]",
"statsmodels/stats/tests/test_rates_poisson.py::test_rate_poisson_ratio_consistency[score-log]",
"statsmodels/stats/tests/test_rates_poisson.py::test_alternative[case5]",
"statsmodels/stats/tests/test_rates_poisson.py::test_alternative[case17]",
"statsmodels/stats/tests/test_rates_poisson.py::test_alternative[case7]",
"statsmodels/stats/tests/test_rates_poisson.py::test_y_grid_regression",
"statsmodels/stats/tests/test_rates_poisson.py::test_invalid_y_grid",
"statsmodels/stats/tests/test_rates_poisson.py::test_power_poisson_equal",
"statsmodels/stats/tests/test_rates_poisson.py::test_poisson_power_2ratio",
"statsmodels/stats/tests/test_rates_poisson.py::test_power_negbin"
] | [
"statsmodels/stats/tests/test_rates_poisson.py::test_confint_poisson_alternative[larger-score-0.01-1000-10]",
"statsmodels/stats/tests/test_rates_poisson.py::test_confint_poisson_alternative[larger-sqrt-0.1-1000-1]",
"statsmodels/stats/tests/test_rates_poisson.py::test_confint_poisson_alternative[smaller-sqrt-v-0.01-1000-1]",
"statsmodels/stats/tests/test_rates_poisson.py::test_confint_poisson_alternative[larger-jeff-0.05-10-0]",
"statsmodels/stats/tests/test_rates_poisson.py::test_confint_poisson_alternative[smaller-sqrt-cent-0.1-10-1]",
"statsmodels/stats/tests/test_rates_poisson.py::test_confint_poisson_alternative[larger-sqrt-0.01-1000-10]",
"statsmodels/stats/tests/test_rates_poisson.py::test_confint_poisson_alternative[larger-sqrt-a-0.01-100-1]",
"statsmodels/stats/tests/test_rates_poisson.py::test_confint_poisson_alternative[larger-midp-c-0.1-1-1]",
"statsmodels/stats/tests/test_rates_poisson.py::test_confint_poisson_alternative[smaller-exact-c-0.05-1-100]",
"statsmodels/stats/tests/test_rates_poisson.py::test_confint_poisson_alternative[smaller-sqrt-v-0.05-1-100]",
"statsmodels/stats/tests/test_rates_poisson.py::test_confint_poisson_alternative[larger-sqrt-cent-0.1-1000-1]",
"statsmodels/stats/tests/test_rates_poisson.py::test_confint_poisson_alternative[larger-sqrt-a-0.01-1-10]",
"statsmodels/stats/tests/test_rates_poisson.py::test_confint_poisson_alternative[smaller-sqrt-cent-0.1-100-10]",
"statsmodels/stats/tests/test_rates_poisson.py::test_confint_poisson_alternative[larger-midp-c-0.1-100-100]",
"statsmodels/stats/tests/test_rates_poisson.py::test_confint_poisson_alternative[larger-sqrt-centcc-0.05-100-10]",
"statsmodels/stats/tests/test_rates_poisson.py::test_confint_poisson_alternative[smaller-waldccv-0.1-100-10]",
"statsmodels/stats/tests/test_rates_poisson.py::test_confint_poisson_alternative[smaller-sqrt-v-0.1-1-10]",
"statsmodels/stats/tests/test_rates_poisson.py::test_confint_poisson_alternative[larger-sqrt-a-0.01-1000-10]",
"statsmodels/stats/tests/test_rates_poisson.py::test_confint_poisson_alternative[smaller-jeff-0.1-1-1]",
"statsmodels/stats/tests/test_rates_poisson.py::test_confint_poisson_alternative[larger-score-0.05-1000-100]",
"statsmodels/stats/tests/test_rates_poisson.py::test_confint_poisson_alternative[smaller-jeff-0.05-10-100]",
"statsmodels/stats/tests/test_rates_poisson.py::test_confint_poisson_alternative[larger-sqrt-cent-0.05-10-1]",
"statsmodels/stats/tests/test_rates_poisson.py::test_confint_poisson_alternative[larger-wald-0.1-10-100]",
"statsmodels/stats/tests/test_rates_poisson.py::test_confint_poisson_alternative[smaller-sqrt-v-0.1-10-1]",
"statsmodels/stats/tests/test_rates_poisson.py::test_confint_poisson_alternative[smaller-jeff-0.05-1-1]",
"statsmodels/stats/tests/test_rates_poisson.py::test_confint_poisson_alternative[larger-jeff-0.1-100-0]",
"statsmodels/stats/tests/test_rates_poisson.py::test_confint_poisson_alternative[larger-midp-c-0.05-100-100]",
"statsmodels/stats/tests/test_rates_poisson.py::test_confint_poisson_alternative[smaller-sqrt-a-0.05-1000-10]",
"statsmodels/stats/tests/test_rates_poisson.py::test_confint_poisson_alternative[smaller-sqrt-0.01-10-10]",
"statsmodels/stats/tests/test_rates_poisson.py::test_confint_poisson_alternative[smaller-sqrt-0.01-1-100]",
"statsmodels/stats/tests/test_rates_poisson.py::test_confint_poisson_alternative[larger-midp-c-0.01-10-1]",
"statsmodels/stats/tests/test_rates_poisson.py::test_confint_poisson_alternative[larger-sqrt-v-0.1-10-1]",
"statsmodels/stats/tests/test_rates_poisson.py::test_confint_poisson_alternative[smaller-jeff-0.05-100-1]",
"statsmodels/stats/tests/test_rates_poisson.py::test_confint_poisson_alternative[larger-sqrt-cent-0.05-100-0]",
"statsmodels/stats/tests/test_rates_poisson.py::test_confint_poisson_alternative[smaller-exact-c-0.05-10-10]",
"statsmodels/stats/tests/test_rates_poisson.py::test_confint_poisson_alternative[smaller-sqrt-v-0.05-1000-100]",
"statsmodels/stats/tests/test_rates_poisson.py::test_confint_poisson_alternative[smaller-sqrt-v-0.1-100-100]",
"statsmodels/stats/tests/test_rates_poisson.py::test_confint_poisson_alternative[larger-jeff-0.01-10-100]",
"statsmodels/stats/tests/test_rates_poisson.py::test_confint_poisson_alternative[smaller-wald-0.05-1-0]",
"statsmodels/stats/tests/test_rates_poisson.py::test_confint_poisson_alternative[larger-exact-c-0.1-100-10]",
"statsmodels/stats/tests/test_rates_poisson.py::test_confint_poisson_alternative[larger-sqrt-v-0.1-100-0]",
"statsmodels/stats/tests/test_rates_poisson.py::test_confint_poisson_alternative[larger-sqrt-v-0.01-100-100]",
"statsmodels/stats/tests/test_rates_poisson.py::test_confint_poisson_alternative[larger-sqrt-v-0.01-10-100]",
"statsmodels/stats/tests/test_rates_poisson.py::test_confint_poisson_alternative[smaller-sqrt-v-0.01-100-0]",
"statsmodels/stats/tests/test_rates_poisson.py::test_confint_poisson_alternative[larger-score-0.01-10-10]",
"statsmodels/stats/tests/test_rates_poisson.py::test_confint_poisson_alternative[smaller-wald-0.01-10-0]",
"statsmodels/stats/tests/test_rates_poisson.py::test_confint_poisson_alternative[larger-midp-c-0.01-1000-1]",
"statsmodels/stats/tests/test_rates_poisson.py::test_confint_poisson_alternative[larger-midp-c-0.1-100-10]",
"statsmodels/stats/tests/test_rates_poisson.py::test_confint_poisson_alternative[larger-sqrt-a-0.1-100-1]",
"statsmodels/stats/tests/test_rates_poisson.py::test_confint_poisson_alternative[larger-score-0.01-100-100]",
"statsmodels/stats/tests/test_rates_poisson.py::test_confint_poisson_alternative[larger-jeff-0.01-1000-0]",
"statsmodels/stats/tests/test_rates_poisson.py::test_confint_poisson_alternative[smaller-exact-c-0.1-1000-100]",
"statsmodels/stats/tests/test_rates_poisson.py::test_confint_poisson_alternative[smaller-sqrt-centcc-0.1-1-1]",
"statsmodels/stats/tests/test_rates_poisson.py::test_confint_poisson_alternative[smaller-exact-c-0.1-100-10]",
"statsmodels/stats/tests/test_rates_poisson.py::test_confint_poisson_alternative[larger-jeff-0.05-1000-1]",
"statsmodels/stats/tests/test_rates_poisson.py::test_confint_poisson_alternative[larger-sqrt-v-0.05-10-1]",
"statsmodels/stats/tests/test_rates_poisson.py::test_confint_poisson_alternative[smaller-exact-c-0.05-1-1]",
"statsmodels/stats/tests/test_rates_poisson.py::test_confint_poisson_alternative[larger-waldccv-0.1-10-100]",
"statsmodels/stats/tests/test_rates_poisson.py::test_confint_poisson_alternative[larger-sqrt-centcc-0.01-10-1]",
"statsmodels/stats/tests/test_rates_poisson.py::test_confint_poisson_alternative[larger-sqrt-a-0.01-1000-100]",
"statsmodels/stats/tests/test_rates_poisson.py::test_confint_poisson_alternative[smaller-exact-c-0.05-1000-0]",
"statsmodels/stats/tests/test_rates_poisson.py::test_confint_poisson_alternative[larger-wald-0.05-1000-10]",
"statsmodels/stats/tests/test_rates_poisson.py::test_confint_poisson_alternative[smaller-waldccv-0.05-10-0]",
"statsmodels/stats/tests/test_rates_poisson.py::test_confint_poisson_alternative[smaller-score-0.1-1-100]",
"statsmodels/stats/tests/test_rates_poisson.py::test_confint_poisson_alternative[smaller-sqrt-v-0.1-10-100]",
"statsmodels/stats/tests/test_rates_poisson.py::test_confint_poisson_alternative[smaller-sqrt-0.1-100-0]",
"statsmodels/stats/tests/test_rates_poisson.py::test_confint_poisson_alternative[smaller-sqrt-0.05-10-100]",
"statsmodels/stats/tests/test_rates_poisson.py::test_confint_poisson_alternative[larger-sqrt-0.1-1-1]",
"statsmodels/stats/tests/test_rates_poisson.py::test_confint_poisson_alternative[larger-jeff-0.01-1-10]",
"statsmodels/stats/tests/test_rates_poisson.py::test_confint_poisson_alternative[larger-sqrt-a-0.01-100-10]",
"statsmodels/stats/tests/test_rates_poisson.py::test_confint_poisson_alternative[smaller-wald-0.1-1-0]",
"statsmodels/stats/tests/test_rates_poisson.py::test_confint_poisson_alternative[larger-sqrt-v-0.05-10-100]",
"statsmodels/stats/tests/test_rates_poisson.py::test_confint_poisson_alternative[smaller-sqrt-a-0.1-1000-0]",
"statsmodels/stats/tests/test_rates_poisson.py::test_confint_poisson_alternative[smaller-waldccv-0.01-100-0]",
"statsmodels/stats/tests/test_rates_poisson.py::test_confint_poisson_alternative[larger-sqrt-centcc-0.01-1000-1]",
"statsmodels/stats/tests/test_rates_poisson.py::test_confint_poisson_alternative[larger-sqrt-centcc-0.1-10-0]",
"statsmodels/stats/tests/test_rates_poisson.py::test_confint_poisson_alternative[larger-exact-c-0.01-10-1]",
"statsmodels/stats/tests/test_rates_poisson.py::test_confint_poisson_alternative[larger-sqrt-v-0.05-1000-0]",
"statsmodels/stats/tests/test_rates_poisson.py::test_confint_poisson_alternative[smaller-sqrt-centcc-0.1-100-0]",
"statsmodels/stats/tests/test_rates_poisson.py::test_confint_poisson_alternative[larger-jeff-0.1-10-10]",
"statsmodels/stats/tests/test_rates_poisson.py::test_confint_poisson_alternative[smaller-exact-c-0.05-100-100]",
"statsmodels/stats/tests/test_rates_poisson.py::test_confint_poisson_alternative[larger-midp-c-0.1-1000-100]",
"statsmodels/stats/tests/test_rates_poisson.py::test_confint_poisson_alternative[larger-sqrt-cent-0.1-1-1]",
"statsmodels/stats/tests/test_rates_poisson.py::test_confint_poisson_alternative[larger-wald-0.05-1-1]",
"statsmodels/stats/tests/test_rates_poisson.py::test_confint_poisson_alternative[larger-jeff-0.01-1-1]",
"statsmodels/stats/tests/test_rates_poisson.py::test_confint_poisson_alternative[larger-exact-c-0.05-100-10]",
"statsmodels/stats/tests/test_rates_poisson.py::test_confint_poisson_alternative[larger-midp-c-0.1-10-0]",
"statsmodels/stats/tests/test_rates_poisson.py::test_confint_poisson_alternative[smaller-sqrt-centcc-0.01-1000-100]",
"statsmodels/stats/tests/test_rates_poisson.py::test_confint_poisson_alternative[larger-sqrt-0.1-1000-100]",
"statsmodels/stats/tests/test_rates_poisson.py::test_confint_poisson_alternative[smaller-sqrt-0.1-1000-10]",
"statsmodels/stats/tests/test_rates_poisson.py::test_confint_poisson_alternative[larger-waldccv-0.05-1-10]",
"statsmodels/stats/tests/test_rates_poisson.py::test_confint_poisson_alternative[larger-wald-0.01-100-100]",
"statsmodels/stats/tests/test_rates_poisson.py::test_confint_poisson_alternative[smaller-sqrt-v-0.05-1-0]",
"statsmodels/stats/tests/test_rates_poisson.py::test_confint_poisson_alternative[smaller-midp-c-0.01-1-10]",
"statsmodels/stats/tests/test_rates_poisson.py::test_confint_poisson_alternative[smaller-sqrt-a-0.05-1-10]",
"statsmodels/stats/tests/test_rates_poisson.py::test_confint_poisson_alternative[smaller-sqrt-v-0.01-1-1]",
"statsmodels/stats/tests/test_rates_poisson.py::test_confint_poisson_alternative[larger-sqrt-0.01-100-1]",
"statsmodels/stats/tests/test_rates_poisson.py::test_confint_poisson_alternative[larger-sqrt-v-0.05-1-1]",
"statsmodels/stats/tests/test_rates_poisson.py::test_confint_poisson_alternative[smaller-score-0.05-100-100]",
"statsmodels/stats/tests/test_rates_poisson.py::test_confint_poisson_alternative[larger-waldccv-0.1-1000-1]",
"statsmodels/stats/tests/test_rates_poisson.py::test_confint_poisson_alternative[smaller-sqrt-a-0.05-10-0]",
"statsmodels/stats/tests/test_rates_poisson.py::test_confint_poisson_alternative[larger-wald-0.05-100-1]",
"statsmodels/stats/tests/test_rates_poisson.py::test_confint_poisson_alternative[smaller-sqrt-0.01-1000-1]",
"statsmodels/stats/tests/test_rates_poisson.py::test_confint_poisson_alternative[smaller-sqrt-v-0.1-1-100]",
"statsmodels/stats/tests/test_rates_poisson.py::test_confint_poisson_alternative[larger-jeff-0.01-1000-100]",
"statsmodels/stats/tests/test_rates_poisson.py::test_confint_poisson_alternative[larger-sqrt-a-0.1-100-10]",
"statsmodels/stats/tests/test_rates_poisson.py::test_confint_poisson_alternative[smaller-sqrt-centcc-0.01-10-10]",
"statsmodels/stats/tests/test_rates_poisson.py::test_confint_poisson_alternative[larger-sqrt-centcc-0.05-10-0]",
"statsmodels/stats/tests/test_rates_poisson.py::test_confint_poisson_alternative[larger-jeff-0.1-1000-1]",
"statsmodels/stats/tests/test_rates_poisson.py::test_confint_poisson_alternative[smaller-sqrt-a-0.1-10-1]",
"statsmodels/stats/tests/test_rates_poisson.py::test_confint_poisson_alternative[smaller-score-0.1-10-100]",
"statsmodels/stats/tests/test_rates_poisson.py::test_confint_poisson_alternative[larger-sqrt-centcc-0.1-1-100]",
"statsmodels/stats/tests/test_rates_poisson.py::test_confint_poisson_alternative[larger-jeff-0.05-10-1]",
"statsmodels/stats/tests/test_rates_poisson.py::test_confint_poisson_alternative[larger-midp-c-0.05-1000-100]",
"statsmodels/stats/tests/test_rates_poisson.py::test_confint_poisson_alternative[larger-sqrt-a-0.1-1000-10]",
"statsmodels/stats/tests/test_rates_poisson.py::test_confint_poisson_alternative[larger-sqrt-centcc-0.01-1-0]",
"statsmodels/stats/tests/test_rates_poisson.py::test_confint_poisson_alternative[smaller-sqrt-a-0.05-1000-0]",
"statsmodels/stats/tests/test_rates_poisson.py::test_confint_poisson_alternative[larger-score-0.01-100-0]",
"statsmodels/stats/tests/test_rates_poisson.py::test_confint_poisson_alternative[larger-sqrt-centcc-0.1-1000-100]",
"statsmodels/stats/tests/test_rates_poisson.py::test_confint_poisson_alternative[smaller-exact-c-0.01-1000-0]",
"statsmodels/stats/tests/test_rates_poisson.py::test_confint_poisson_alternative[larger-score-0.01-1000-0]",
"statsmodels/stats/tests/test_rates_poisson.py::test_confint_poisson_alternative[larger-sqrt-cent-0.05-1000-100]",
"statsmodels/stats/tests/test_rates_poisson.py::test_confint_poisson_alternative[larger-sqrt-centcc-0.1-10-100]",
"statsmodels/stats/tests/test_rates_poisson.py::test_confint_poisson_alternative[larger-sqrt-centcc-0.1-100-1]",
"statsmodels/stats/tests/test_rates_poisson.py::test_confint_poisson_alternative[larger-jeff-0.1-100-1]",
"statsmodels/stats/tests/test_rates_poisson.py::test_confint_poisson_alternative[larger-sqrt-cent-0.1-10-0]",
"statsmodels/stats/tests/test_rates_poisson.py::test_confint_poisson_alternative[smaller-sqrt-v-0.05-10-0]",
"statsmodels/stats/tests/test_rates_poisson.py::test_confint_poisson_alternative[smaller-score-0.01-10-10]",
"statsmodels/stats/tests/test_rates_poisson.py::test_confint_poisson_alternative[larger-sqrt-centcc-0.01-1-100]",
"statsmodels/stats/tests/test_rates_poisson.py::test_confint_poisson_alternative[larger-sqrt-a-0.01-1-100]",
"statsmodels/stats/tests/test_rates_poisson.py::test_confint_poisson_alternative[smaller-sqrt-0.1-10-100]",
"statsmodels/stats/tests/test_rates_poisson.py::test_confint_poisson_alternative[smaller-sqrt-a-0.01-10-100]",
"statsmodels/stats/tests/test_rates_poisson.py::test_confint_poisson_alternative[larger-sqrt-0.01-1-0]",
"statsmodels/stats/tests/test_rates_poisson.py::test_confint_poisson_alternative[smaller-score-0.05-1-0]",
"statsmodels/stats/tests/test_rates_poisson.py::test_confint_poisson_alternative[larger-score-0.05-100-0]",
"statsmodels/stats/tests/test_rates_poisson.py::test_confint_poisson_alternative[larger-score-0.01-1-10]",
"statsmodels/stats/tests/test_rates_poisson.py::test_confint_poisson_alternative[smaller-sqrt-cent-0.01-1000-10]",
"statsmodels/stats/tests/test_rates_poisson.py::test_confint_poisson_alternative[larger-sqrt-0.05-10-100]",
"statsmodels/stats/tests/test_rates_poisson.py::test_confint_poisson_alternative[larger-midp-c-0.05-10-1]",
"statsmodels/stats/tests/test_rates_poisson.py::test_confint_poisson_alternative[smaller-jeff-0.05-10-1]",
"statsmodels/stats/tests/test_rates_poisson.py::test_confint_poisson_alternative[smaller-waldccv-0.1-10-10]",
"statsmodels/stats/tests/test_rates_poisson.py::test_confint_poisson_alternative[larger-score-0.01-10-0]",
"statsmodels/stats/tests/test_rates_poisson.py::test_confint_poisson_alternative[larger-sqrt-centcc-0.1-1000-1]",
"statsmodels/stats/tests/test_rates_poisson.py::test_confint_poisson_alternative[smaller-exact-c-0.1-1000-1]",
"statsmodels/stats/tests/test_rates_poisson.py::test_confint_poisson_alternative[larger-wald-0.1-1-100]",
"statsmodels/stats/tests/test_rates_poisson.py::test_confint_poisson_alternative[larger-waldccv-0.05-10-100]",
"statsmodels/stats/tests/test_rates_poisson.py::test_confint_poisson_alternative[smaller-sqrt-centcc-0.01-10-1]",
"statsmodels/stats/tests/test_rates_poisson.py::test_confint_poisson_alternative[larger-sqrt-v-0.05-100-100]",
"statsmodels/stats/tests/test_rates_poisson.py::test_confint_poisson_alternative[smaller-exact-c-0.05-10-100]",
"statsmodels/stats/tests/test_rates_poisson.py::test_confint_poisson_alternative[smaller-wald-0.05-100-1]",
"statsmodels/stats/tests/test_rates_poisson.py::test_confint_poisson_alternative[smaller-score-0.1-100-1]",
"statsmodels/stats/tests/test_rates_poisson.py::test_confint_poisson_alternative[larger-midp-c-0.05-10-10]",
"statsmodels/stats/tests/test_rates_poisson.py::test_confint_poisson_alternative[larger-sqrt-a-0.1-100-100]",
"statsmodels/stats/tests/test_rates_poisson.py::test_confint_poisson_alternative[smaller-waldccv-0.1-10-0]",
"statsmodels/stats/tests/test_rates_poisson.py::test_confint_poisson_alternative[smaller-sqrt-v-0.01-1-100]",
"statsmodels/stats/tests/test_rates_poisson.py::test_confint_poisson_alternative[larger-exact-c-0.01-100-10]",
"statsmodels/stats/tests/test_rates_poisson.py::test_confint_poisson_alternative[larger-sqrt-0.1-10-1]",
"statsmodels/stats/tests/test_rates_poisson.py::test_confint_poisson_alternative[smaller-sqrt-v-0.05-10-100]",
"statsmodels/stats/tests/test_rates_poisson.py::test_confint_poisson_alternative[larger-sqrt-centcc-0.01-1-10]",
"statsmodels/stats/tests/test_rates_poisson.py::test_confint_poisson_alternative[larger-waldccv-0.01-10-0]",
"statsmodels/stats/tests/test_rates_poisson.py::test_confint_poisson_alternative[larger-sqrt-0.01-1-10]",
"statsmodels/stats/tests/test_rates_poisson.py::test_confint_poisson_alternative[larger-sqrt-centcc-0.05-10-10]",
"statsmodels/stats/tests/test_rates_poisson.py::test_confint_poisson_alternative[smaller-sqrt-cent-0.01-100-1]",
"statsmodels/stats/tests/test_rates_poisson.py::test_confint_poisson_alternative[larger-sqrt-cent-0.05-1-0]",
"statsmodels/stats/tests/test_rates_poisson.py::test_confint_poisson_alternative[larger-score-0.01-1-100]",
"statsmodels/stats/tests/test_rates_poisson.py::test_confint_poisson_alternative[smaller-sqrt-centcc-0.01-1-100]",
"statsmodels/stats/tests/test_rates_poisson.py::test_confint_poisson_alternative[larger-sqrt-v-0.1-1-1]",
"statsmodels/stats/tests/test_rates_poisson.py::test_confint_poisson_alternative[larger-sqrt-v-0.01-10-1]",
"statsmodels/stats/tests/test_rates_poisson.py::test_confint_poisson_alternative[larger-sqrt-a-0.01-10-0]",
"statsmodels/stats/tests/test_rates_poisson.py::test_confint_poisson_alternative[smaller-sqrt-0.1-100-100]",
"statsmodels/stats/tests/test_rates_poisson.py::test_confint_poisson_alternative[smaller-sqrt-v-0.05-100-1]",
"statsmodels/stats/tests/test_rates_poisson.py::test_confint_poisson_alternative[smaller-midp-c-0.05-1-100]",
"statsmodels/stats/tests/test_rates_poisson.py::test_confint_poisson_alternative[smaller-waldccv-0.1-100-100]",
"statsmodels/stats/tests/test_rates_poisson.py::test_confint_poisson_alternative[smaller-score-0.1-1000-0]",
"statsmodels/stats/tests/test_rates_poisson.py::test_confint_poisson_alternative[larger-jeff-0.05-100-10]",
"statsmodels/stats/tests/test_rates_poisson.py::test_confint_poisson_alternative[larger-sqrt-centcc-0.01-1000-100]",
"statsmodels/stats/tests/test_rates_poisson.py::test_confint_poisson_alternative[larger-waldccv-0.1-10-1]",
"statsmodels/stats/tests/test_rates_poisson.py::test_confint_poisson_alternative[larger-midp-c-0.01-1-0]",
"statsmodels/stats/tests/test_rates_poisson.py::test_confint_poisson_alternative[larger-sqrt-0.01-10-0]",
"statsmodels/stats/tests/test_rates_poisson.py::test_confint_poisson_alternative[smaller-midp-c-0.1-1-0]",
"statsmodels/stats/tests/test_rates_poisson.py::test_confint_poisson_alternative[smaller-sqrt-0.05-1-1]",
"statsmodels/stats/tests/test_rates_poisson.py::test_confint_poisson_alternative[larger-sqrt-v-0.01-1000-100]",
"statsmodels/stats/tests/test_rates_poisson.py::test_confint_poisson_alternative[smaller-sqrt-centcc-0.1-1000-10]",
"statsmodels/stats/tests/test_rates_poisson.py::test_confint_poisson_alternative[larger-sqrt-v-0.1-100-1]",
"statsmodels/stats/tests/test_rates_poisson.py::test_confint_poisson_alternative[smaller-sqrt-cent-0.05-100-10]",
"statsmodels/stats/tests/test_rates_poisson.py::test_confint_poisson_alternative[larger-exact-c-0.1-1-1]",
"statsmodels/stats/tests/test_rates_poisson.py::test_confint_poisson_alternative[larger-exact-c-0.1-1000-100]",
"statsmodels/stats/tests/test_rates_poisson.py::test_confint_poisson_alternative[larger-wald-0.01-1-10]",
"statsmodels/stats/tests/test_rates_poisson.py::test_confint_poisson_alternative[larger-midp-c-0.01-1000-10]",
"statsmodels/stats/tests/test_rates_poisson.py::test_confint_poisson_alternative[smaller-wald-0.1-1000-1]",
"statsmodels/stats/tests/test_rates_poisson.py::test_confint_poisson_alternative[larger-sqrt-centcc-0.05-10-100]",
"statsmodels/stats/tests/test_rates_poisson.py::test_confint_poisson_alternative[larger-wald-0.01-10-1]",
"statsmodels/stats/tests/test_rates_poisson.py::test_confint_poisson_alternative[smaller-sqrt-cent-0.01-1-100]",
"statsmodels/stats/tests/test_rates_poisson.py::test_confint_poisson_alternative[smaller-midp-c-0.1-1-100]",
"statsmodels/stats/tests/test_rates_poisson.py::test_confint_poisson_alternative[smaller-sqrt-v-0.1-1000-1]",
"statsmodels/stats/tests/test_rates_poisson.py::test_confint_poisson_alternative[smaller-waldccv-0.01-1-100]",
"statsmodels/stats/tests/test_rates_poisson.py::test_confint_poisson_alternative[larger-jeff-0.01-10-10]",
"statsmodels/stats/tests/test_rates_poisson.py::test_confint_poisson_alternative[larger-jeff-0.01-10-0]",
"statsmodels/stats/tests/test_rates_poisson.py::test_confint_poisson_alternative[smaller-jeff-0.05-10-10]",
"statsmodels/stats/tests/test_rates_poisson.py::test_confint_poisson_alternative[smaller-sqrt-a-0.05-1-0]",
"statsmodels/stats/tests/test_rates_poisson.py::test_confint_poisson_alternative[smaller-sqrt-centcc-0.1-1000-100]",
"statsmodels/stats/tests/test_rates_poisson.py::test_confint_poisson_alternative[smaller-sqrt-cent-0.1-1000-1]",
"statsmodels/stats/tests/test_rates_poisson.py::test_confint_poisson_alternative[smaller-sqrt-v-0.05-10-1]",
"statsmodels/stats/tests/test_rates_poisson.py::test_confint_poisson_alternative[smaller-waldccv-0.05-10-10]",
"statsmodels/stats/tests/test_rates_poisson.py::test_confint_poisson_alternative[smaller-midp-c-0.05-10-1]",
"statsmodels/stats/tests/test_rates_poisson.py::test_confint_poisson_alternative[larger-sqrt-centcc-0.01-10-0]",
"statsmodels/stats/tests/test_rates_poisson.py::test_confint_poisson_alternative[smaller-sqrt-centcc-0.1-100-100]",
"statsmodels/stats/tests/test_rates_poisson.py::test_confint_poisson_alternative[smaller-jeff-0.1-10-1]",
"statsmodels/stats/tests/test_rates_poisson.py::test_confint_poisson_alternative[larger-sqrt-0.05-1000-100]",
"statsmodels/stats/tests/test_rates_poisson.py::test_confint_poisson_alternative[smaller-midp-c-0.05-1000-1]",
"statsmodels/stats/tests/test_rates_poisson.py::test_confint_poisson_alternative[smaller-wald-0.1-100-0]",
"statsmodels/stats/tests/test_rates_poisson.py::test_confint_poisson_alternative[larger-jeff-0.01-1000-1]",
"statsmodels/stats/tests/test_rates_poisson.py::test_confint_poisson_alternative[smaller-sqrt-centcc-0.1-1-10]",
"statsmodels/stats/tests/test_rates_poisson.py::test_confint_poisson_alternative[smaller-waldccv-0.01-1000-1]",
"statsmodels/stats/tests/test_rates_poisson.py::test_confint_poisson_alternative[larger-sqrt-0.01-1-100]",
"statsmodels/stats/tests/test_rates_poisson.py::test_confint_poisson_alternative[smaller-sqrt-v-0.1-1000-100]",
"statsmodels/stats/tests/test_rates_poisson.py::test_confint_poisson_alternative[larger-sqrt-centcc-0.1-1000-0]",
"statsmodels/stats/tests/test_rates_poisson.py::test_confint_poisson_alternative[smaller-midp-c-0.1-10-0]",
"statsmodels/stats/tests/test_rates_poisson.py::test_confint_poisson_alternative[larger-exact-c-0.1-1-100]",
"statsmodels/stats/tests/test_rates_poisson.py::test_confint_poisson_alternative[smaller-exact-c-0.01-1-1]",
"statsmodels/stats/tests/test_rates_poisson.py::test_confint_poisson_alternative[larger-waldccv-0.1-1000-10]",
"statsmodels/stats/tests/test_rates_poisson.py::test_confint_poisson_alternative[smaller-wald-0.1-100-10]",
"statsmodels/stats/tests/test_rates_poisson.py::test_confint_poisson_alternative[larger-midp-c-0.01-1-10]",
"statsmodels/stats/tests/test_rates_poisson.py::test_confint_poisson_alternative[larger-sqrt-cent-0.05-1-10]",
"statsmodels/stats/tests/test_rates_poisson.py::test_confint_poisson_alternative[larger-sqrt-v-0.05-10-10]",
"statsmodels/stats/tests/test_rates_poisson.py::test_confint_poisson_alternative[larger-sqrt-cent-0.01-100-100]",
"statsmodels/stats/tests/test_rates_poisson.py::test_confint_poisson_alternative[larger-sqrt-cent-0.01-1-0]",
"statsmodels/stats/tests/test_rates_poisson.py::test_confint_poisson_alternative[smaller-sqrt-cent-0.01-10-1]",
"statsmodels/stats/tests/test_rates_poisson.py::test_confint_poisson_alternative[larger-waldccv-0.01-100-10]",
"statsmodels/stats/tests/test_rates_poisson.py::test_confint_poisson_alternative[larger-exact-c-0.05-1000-100]",
"statsmodels/stats/tests/test_rates_poisson.py::test_confint_poisson_alternative[smaller-sqrt-0.1-100-10]",
"statsmodels/stats/tests/test_rates_poisson.py::test_confint_poisson_alternative[smaller-score-0.01-1000-0]",
"statsmodels/stats/tests/test_rates_poisson.py::test_confint_poisson_alternative[larger-sqrt-cent-0.01-1-1]",
"statsmodels/stats/tests/test_rates_poisson.py::test_confint_poisson_alternative[smaller-waldccv-0.1-1000-1]",
"statsmodels/stats/tests/test_rates_poisson.py::test_confint_poisson_alternative[larger-jeff-0.05-100-0]",
"statsmodels/stats/tests/test_rates_poisson.py::test_confint_poisson_alternative[smaller-jeff-0.1-10-0]",
"statsmodels/stats/tests/test_rates_poisson.py::test_confint_poisson_alternative[smaller-sqrt-centcc-0.05-1-100]",
"statsmodels/stats/tests/test_rates_poisson.py::test_confint_poisson_alternative[larger-wald-0.1-100-0]",
"statsmodels/stats/tests/test_rates_poisson.py::test_confint_poisson_alternative[larger-midp-c-0.1-10-10]",
"statsmodels/stats/tests/test_rates_poisson.py::test_confint_poisson_alternative[larger-score-0.1-10-10]",
"statsmodels/stats/tests/test_rates_poisson.py::test_confint_poisson_alternative[larger-wald-0.1-1-0]",
"statsmodels/stats/tests/test_rates_poisson.py::test_confint_poisson_alternative[larger-sqrt-a-0.1-100-0]",
"statsmodels/stats/tests/test_rates_poisson.py::test_confint_poisson_alternative[smaller-waldccv-0.05-1-1]",
"statsmodels/stats/tests/test_rates_poisson.py::test_confint_poisson_alternative[larger-sqrt-0.1-10-100]",
"statsmodels/stats/tests/test_rates_poisson.py::test_confint_poisson_alternative[larger-sqrt-v-0.05-1-10]",
"statsmodels/stats/tests/test_rates_poisson.py::test_confint_poisson_alternative[larger-sqrt-0.01-1000-100]",
"statsmodels/stats/tests/test_rates_poisson.py::test_confint_poisson_alternative[larger-sqrt-centcc-0.01-100-0]",
"statsmodels/stats/tests/test_rates_poisson.py::test_confint_poisson_alternative[larger-sqrt-cent-0.01-1000-1]",
"statsmodels/stats/tests/test_rates_poisson.py::test_confint_poisson_alternative[smaller-sqrt-centcc-0.01-1-1]",
"statsmodels/stats/tests/test_rates_poisson.py::test_confint_poisson_alternative[larger-sqrt-cent-0.01-1000-10]",
"statsmodels/stats/tests/test_rates_poisson.py::test_confint_poisson_alternative[smaller-waldccv-0.01-10-10]",
"statsmodels/stats/tests/test_rates_poisson.py::test_confint_poisson_alternative[smaller-sqrt-0.1-1-0]",
"statsmodels/stats/tests/test_rates_poisson.py::test_confint_poisson_alternative[smaller-midp-c-0.01-10-100]",
"statsmodels/stats/tests/test_rates_poisson.py::test_confint_poisson_alternative[smaller-score-0.1-100-100]",
"statsmodels/stats/tests/test_rates_poisson.py::test_confint_poisson_alternative[larger-exact-c-0.01-1-10]",
"statsmodels/stats/tests/test_rates_poisson.py::test_confint_poisson_alternative[smaller-score-0.1-100-10]",
"statsmodels/stats/tests/test_rates_poisson.py::test_confint_poisson_alternative[smaller-waldccv-0.01-1000-10]",
"statsmodels/stats/tests/test_rates_poisson.py::test_confint_poisson_alternative[smaller-sqrt-v-0.05-10-10]",
"statsmodels/stats/tests/test_rates_poisson.py::test_confint_poisson_alternative[smaller-sqrt-centcc-0.1-1000-1]",
"statsmodels/stats/tests/test_rates_poisson.py::test_confint_poisson_alternative[larger-sqrt-centcc-0.01-100-10]",
"statsmodels/stats/tests/test_rates_poisson.py::test_confint_poisson_alternative[larger-sqrt-cent-0.1-100-10]",
"statsmodels/stats/tests/test_rates_poisson.py::test_confint_poisson_alternative[smaller-midp-c-0.1-100-100]",
"statsmodels/stats/tests/test_rates_poisson.py::test_confint_poisson_alternative[smaller-waldccv-0.01-100-1]",
"statsmodels/stats/tests/test_rates_poisson.py::test_confint_poisson_alternative[larger-midp-c-0.01-10-100]",
"statsmodels/stats/tests/test_rates_poisson.py::test_confint_poisson_alternative[larger-exact-c-0.1-10-0]",
"statsmodels/stats/tests/test_rates_poisson.py::test_confint_poisson_alternative[smaller-midp-c-0.05-100-1]",
"statsmodels/stats/tests/test_rates_poisson.py::test_confint_poisson_alternative[smaller-exact-c-0.05-100-0]",
"statsmodels/stats/tests/test_rates_poisson.py::test_confint_poisson_alternative[larger-waldccv-0.05-10-1]",
"statsmodels/stats/tests/test_rates_poisson.py::test_confint_poisson_alternative[smaller-waldccv-0.1-10-1]",
"statsmodels/stats/tests/test_rates_poisson.py::test_confint_poisson_alternative[larger-exact-c-0.01-100-0]",
"statsmodels/stats/tests/test_rates_poisson.py::test_confint_poisson_alternative[larger-sqrt-cent-0.01-100-0]",
"statsmodels/stats/tests/test_rates_poisson.py::test_confint_poisson_alternative[smaller-sqrt-cent-0.1-10-100]",
"statsmodels/stats/tests/test_rates_poisson.py::test_confint_poisson_alternative[smaller-sqrt-0.01-100-1]",
"statsmodels/stats/tests/test_rates_poisson.py::test_confint_poisson_alternative[smaller-jeff-0.01-100-0]",
"statsmodels/stats/tests/test_rates_poisson.py::test_confint_poisson_alternative[smaller-jeff-0.05-1000-1]",
"statsmodels/stats/tests/test_rates_poisson.py::test_confint_poisson_alternative[larger-wald-0.01-10-0]",
"statsmodels/stats/tests/test_rates_poisson.py::test_confint_poisson_alternative[smaller-waldccv-0.1-1000-10]",
"statsmodels/stats/tests/test_rates_poisson.py::test_confint_poisson_alternative[smaller-sqrt-a-0.1-10-10]",
"statsmodels/stats/tests/test_rates_poisson.py::test_confint_poisson_alternative[smaller-waldccv-0.01-100-10]",
"statsmodels/stats/tests/test_rates_poisson.py::test_confint_poisson_alternative[smaller-sqrt-v-0.1-100-1]",
"statsmodels/stats/tests/test_rates_poisson.py::test_confint_poisson_alternative[smaller-score-0.05-10-1]",
"statsmodels/stats/tests/test_rates_poisson.py::test_confint_poisson_alternative[larger-sqrt-centcc-0.05-1000-10]",
"statsmodels/stats/tests/test_rates_poisson.py::test_confint_poisson_alternative[larger-sqrt-0.05-100-10]",
"statsmodels/stats/tests/test_rates_poisson.py::test_confint_poisson_alternative[smaller-exact-c-0.05-1-0]",
"statsmodels/stats/tests/test_rates_poisson.py::test_confint_poisson_alternative[larger-exact-c-0.01-1-100]",
"statsmodels/stats/tests/test_rates_poisson.py::test_confint_poisson_alternative[smaller-exact-c-0.1-10-10]",
"statsmodels/stats/tests/test_rates_poisson.py::test_confint_poisson_alternative[smaller-exact-c-0.1-1-100]",
"statsmodels/stats/tests/test_rates_poisson.py::test_confint_poisson_alternative[larger-waldccv-0.01-100-0]",
"statsmodels/stats/tests/test_rates_poisson.py::test_confint_poisson_alternative[smaller-sqrt-v-0.01-100-10]",
"statsmodels/stats/tests/test_rates_poisson.py::test_confint_poisson_alternative[larger-sqrt-v-0.1-1000-0]",
"statsmodels/stats/tests/test_rates_poisson.py::test_confint_poisson_alternative[larger-midp-c-0.05-100-1]",
"statsmodels/stats/tests/test_rates_poisson.py::test_confint_poisson_alternative[smaller-sqrt-a-0.1-1000-10]",
"statsmodels/stats/tests/test_rates_poisson.py::test_confint_poisson_alternative[smaller-midp-c-0.05-10-10]",
"statsmodels/stats/tests/test_rates_poisson.py::test_confint_poisson_alternative[larger-sqrt-0.05-1000-1]",
"statsmodels/stats/tests/test_rates_poisson.py::test_confint_poisson_alternative[larger-sqrt-cent-0.1-10-100]",
"statsmodels/stats/tests/test_rates_poisson.py::test_confint_poisson_alternative[larger-score-0.1-100-100]",
"statsmodels/stats/tests/test_rates_poisson.py::test_confint_poisson_alternative[larger-sqrt-0.1-10-0]",
"statsmodels/stats/tests/test_rates_poisson.py::test_confint_poisson_alternative[larger-sqrt-a-0.1-10-10]",
"statsmodels/stats/tests/test_rates_poisson.py::test_confint_poisson_alternative[larger-wald-0.05-1-100]",
"statsmodels/stats/tests/test_rates_poisson.py::test_confint_poisson_alternative[smaller-jeff-0.1-100-100]",
"statsmodels/stats/tests/test_rates_poisson.py::test_confint_poisson_alternative[larger-sqrt-centcc-0.01-1-1]",
"statsmodels/stats/tests/test_rates_poisson.py::test_confint_poisson_alternative[larger-sqrt-0.05-1-100]",
"statsmodels/stats/tests/test_rates_poisson.py::test_confint_poisson_alternative[smaller-sqrt-centcc-0.05-1000-0]",
"statsmodels/stats/tests/test_rates_poisson.py::test_confint_poisson_alternative[larger-exact-c-0.1-1-0]",
"statsmodels/stats/tests/test_rates_poisson.py::test_confint_poisson_alternative[smaller-sqrt-centcc-0.01-1000-0]",
"statsmodels/stats/tests/test_rates_poisson.py::test_confint_poisson_alternative[smaller-score-0.05-100-0]",
"statsmodels/stats/tests/test_rates_poisson.py::test_confint_poisson_alternative[smaller-jeff-0.1-1-0]",
"statsmodels/stats/tests/test_rates_poisson.py::test_confint_poisson_alternative[smaller-jeff-0.1-1000-100]",
"statsmodels/stats/tests/test_rates_poisson.py::test_confint_poisson_alternative[larger-exact-c-0.05-1000-1]",
"statsmodels/stats/tests/test_rates_poisson.py::test_confint_poisson_alternative[larger-midp-c-0.05-1-100]",
"statsmodels/stats/tests/test_rates_poisson.py::test_confint_poisson_alternative[smaller-exact-c-0.1-1-1]",
"statsmodels/stats/tests/test_rates_poisson.py::test_confint_poisson_alternative[smaller-sqrt-a-0.01-1000-0]",
"statsmodels/stats/tests/test_rates_poisson.py::test_confint_poisson_alternative[smaller-sqrt-0.1-1-10]",
"statsmodels/stats/tests/test_rates_poisson.py::test_confint_poisson_alternative[larger-midp-c-0.05-100-10]",
"statsmodels/stats/tests/test_rates_poisson.py::test_confint_poisson_alternative[larger-waldccv-0.01-1-0]",
"statsmodels/stats/tests/test_rates_poisson.py::test_confint_poisson_alternative[larger-sqrt-cent-0.05-1000-0]",
"statsmodels/stats/tests/test_rates_poisson.py::test_confint_poisson_alternative[larger-sqrt-a-0.01-10-100]",
"statsmodels/stats/tests/test_rates_poisson.py::test_confint_poisson_alternative[smaller-midp-c-0.01-1000-100]",
"statsmodels/stats/tests/test_rates_poisson.py::test_confint_poisson_alternative[larger-midp-c-0.1-1000-10]",
"statsmodels/stats/tests/test_rates_poisson.py::test_confint_poisson_alternative[smaller-midp-c-0.05-100-10]",
"statsmodels/stats/tests/test_rates_poisson.py::test_confint_poisson_alternative[larger-waldccv-0.1-1-1]",
"statsmodels/stats/tests/test_rates_poisson.py::test_confint_poisson_alternative[larger-score-0.05-1000-0]",
"statsmodels/stats/tests/test_rates_poisson.py::test_confint_poisson_alternative[larger-midp-c-0.1-1000-1]",
"statsmodels/stats/tests/test_rates_poisson.py::test_confint_poisson_alternative[smaller-wald-0.1-100-1]",
"statsmodels/stats/tests/test_rates_poisson.py::test_confint_poisson_alternative[larger-sqrt-v-0.1-1000-1]",
"statsmodels/stats/tests/test_rates_poisson.py::test_confint_poisson_alternative[smaller-score-0.1-1-10]",
"statsmodels/stats/tests/test_rates_poisson.py::test_confint_poisson_alternative[larger-midp-c-0.01-10-10]",
"statsmodels/stats/tests/test_rates_poisson.py::test_confint_poisson_alternative[smaller-sqrt-cent-0.1-1-100]",
"statsmodels/stats/tests/test_rates_poisson.py::test_confint_poisson_alternative[larger-exact-c-0.05-1000-0]",
"statsmodels/stats/tests/test_rates_poisson.py::test_confint_poisson_alternative[larger-sqrt-a-0.01-1000-0]",
"statsmodels/stats/tests/test_rates_poisson.py::test_confint_poisson_alternative[smaller-sqrt-cent-0.1-10-10]",
"statsmodels/stats/tests/test_rates_poisson.py::test_confint_poisson_alternative[smaller-sqrt-0.05-10-0]",
"statsmodels/stats/tests/test_rates_poisson.py::test_confint_poisson_alternative[larger-midp-c-0.01-100-1]",
"statsmodels/stats/tests/test_rates_poisson.py::test_confint_poisson_alternative[smaller-score-0.05-1000-10]",
"statsmodels/stats/tests/test_rates_poisson.py::test_confint_poisson_alternative[larger-midp-c-0.1-1-10]",
"statsmodels/stats/tests/test_rates_poisson.py::test_confint_poisson_alternative[larger-sqrt-a-0.05-100-1]",
"statsmodels/stats/tests/test_rates_poisson.py::test_confint_poisson_alternative[smaller-sqrt-cent-0.05-1000-1]",
"statsmodels/stats/tests/test_rates_poisson.py::test_confint_poisson_alternative[larger-sqrt-cent-0.01-10-0]",
"statsmodels/stats/tests/test_rates_poisson.py::test_confint_poisson_alternative[smaller-sqrt-a-0.01-1000-1]",
"statsmodels/stats/tests/test_rates_poisson.py::test_confint_poisson_alternative[larger-score-0.1-1-0]",
"statsmodels/stats/tests/test_rates_poisson.py::test_confint_poisson_alternative[larger-sqrt-a-0.05-1000-10]",
"statsmodels/stats/tests/test_rates_poisson.py::test_confint_poisson_alternative[smaller-midp-c-0.05-1-10]",
"statsmodels/stats/tests/test_rates_poisson.py::test_confint_poisson_alternative[smaller-sqrt-cent-0.01-1000-100]",
"statsmodels/stats/tests/test_rates_poisson.py::test_confint_poisson_alternative[larger-sqrt-v-0.01-1000-10]",
"statsmodels/stats/tests/test_rates_poisson.py::test_confint_poisson_alternative[larger-sqrt-cent-0.1-10-1]",
"statsmodels/stats/tests/test_rates_poisson.py::test_confint_poisson_alternative[larger-sqrt-0.1-100-100]",
"statsmodels/stats/tests/test_rates_poisson.py::test_confint_poisson_alternative[smaller-sqrt-0.01-1-0]",
"statsmodels/stats/tests/test_rates_poisson.py::test_confint_poisson_alternative[smaller-sqrt-centcc-0.01-1000-1]",
"statsmodels/stats/tests/test_rates_poisson.py::test_confint_poisson_alternative[larger-waldccv-0.01-1000-10]",
"statsmodels/stats/tests/test_rates_poisson.py::test_confint_poisson_alternative[smaller-score-0.01-10-100]",
"statsmodels/stats/tests/test_rates_poisson.py::test_confint_poisson_alternative[larger-sqrt-centcc-0.1-1-0]",
"statsmodels/stats/tests/test_rates_poisson.py::test_confint_poisson_alternative[smaller-wald-0.05-1000-10]",
"statsmodels/stats/tests/test_rates_poisson.py::test_confint_poisson_alternative[larger-sqrt-cent-0.1-100-100]",
"statsmodels/stats/tests/test_rates_poisson.py::test_confint_poisson_alternative[smaller-exact-c-0.01-1-0]",
"statsmodels/stats/tests/test_rates_poisson.py::test_confint_poisson_alternative[smaller-sqrt-0.05-1000-1]",
"statsmodels/stats/tests/test_rates_poisson.py::test_confint_poisson_alternative[smaller-waldccv-0.01-100-100]",
"statsmodels/stats/tests/test_rates_poisson.py::test_confint_poisson_alternative[larger-sqrt-0.01-10-1]",
"statsmodels/stats/tests/test_rates_poisson.py::test_confint_poisson_alternative[larger-sqrt-0.1-1-0]",
"statsmodels/stats/tests/test_rates_poisson.py::test_confint_poisson_alternative[smaller-sqrt-v-0.01-100-1]",
"statsmodels/stats/tests/test_rates_poisson.py::test_confint_poisson_alternative[smaller-midp-c-0.05-10-100]",
"statsmodels/stats/tests/test_rates_poisson.py::test_confint_poisson_alternative[larger-sqrt-centcc-0.1-100-0]",
"statsmodels/stats/tests/test_rates_poisson.py::test_confint_poisson_alternative[larger-waldccv-0.01-1000-0]",
"statsmodels/stats/tests/test_rates_poisson.py::test_confint_poisson_alternative[smaller-score-0.1-1-1]",
"statsmodels/stats/tests/test_rates_poisson.py::test_confint_poisson_alternative[larger-waldccv-0.05-100-100]",
"statsmodels/stats/tests/test_rates_poisson.py::test_confint_poisson_alternative[larger-jeff-0.05-1000-100]",
"statsmodels/stats/tests/test_rates_poisson.py::test_confint_poisson_alternative[larger-sqrt-0.01-10-100]",
"statsmodels/stats/tests/test_rates_poisson.py::test_confint_poisson_alternative[smaller-score-0.05-100-10]",
"statsmodels/stats/tests/test_rates_poisson.py::test_confint_poisson_alternative[smaller-sqrt-centcc-0.05-1-1]",
"statsmodels/stats/tests/test_rates_poisson.py::test_confint_poisson_alternative[smaller-wald-0.01-10-100]",
"statsmodels/stats/tests/test_rates_poisson.py::test_confint_poisson_alternative[larger-score-0.01-10-1]",
"statsmodels/stats/tests/test_rates_poisson.py::test_confint_poisson_alternative[larger-sqrt-0.05-10-10]",
"statsmodels/stats/tests/test_rates_poisson.py::test_confint_poisson_alternative[smaller-midp-c-0.05-1000-100]",
"statsmodels/stats/tests/test_rates_poisson.py::test_confint_poisson_alternative[larger-sqrt-0.1-100-10]",
"statsmodels/stats/tests/test_rates_poisson.py::test_confint_poisson_alternative[smaller-jeff-0.05-1000-10]",
"statsmodels/stats/tests/test_rates_poisson.py::test_confint_poisson_alternative[smaller-jeff-0.01-10-1]",
"statsmodels/stats/tests/test_rates_poisson.py::test_confint_poisson_alternative[smaller-score-0.01-1-10]",
"statsmodels/stats/tests/test_rates_poisson.py::test_confint_poisson_alternative[smaller-jeff-0.01-100-10]",
"statsmodels/stats/tests/test_rates_poisson.py::test_confint_poisson_alternative[smaller-sqrt-a-0.01-1-0]",
"statsmodels/stats/tests/test_rates_poisson.py::test_confint_poisson_alternative[larger-sqrt-v-0.05-1000-10]",
"statsmodels/stats/tests/test_rates_poisson.py::test_confint_poisson_alternative[larger-sqrt-0.01-1000-1]",
"statsmodels/stats/tests/test_rates_poisson.py::test_confint_poisson_alternative[smaller-waldccv-0.05-100-0]",
"statsmodels/stats/tests/test_rates_poisson.py::test_confint_poisson_alternative[larger-waldccv-0.1-1-0]",
"statsmodels/stats/tests/test_rates_poisson.py::test_confint_poisson_alternative[larger-score-0.05-10-1]",
"statsmodels/stats/tests/test_rates_poisson.py::test_confint_poisson_alternative[larger-exact-c-0.01-1-0]",
"statsmodels/stats/tests/test_rates_poisson.py::test_confint_poisson_alternative[larger-exact-c-0.1-100-100]",
"statsmodels/stats/tests/test_rates_poisson.py::test_confint_poisson_alternative[larger-sqrt-cent-0.01-100-1]",
"statsmodels/stats/tests/test_rates_poisson.py::test_confint_poisson_alternative[smaller-score-0.1-1-0]",
"statsmodels/stats/tests/test_rates_poisson.py::test_confint_poisson_alternative[larger-score-0.1-1000-0]",
"statsmodels/stats/tests/test_rates_poisson.py::test_confint_poisson_alternative[larger-wald-0.01-1-100]",
"statsmodels/stats/tests/test_rates_poisson.py::test_confint_poisson_alternative[smaller-sqrt-v-0.1-1-0]",
"statsmodels/stats/tests/test_rates_poisson.py::test_confint_poisson_alternative[smaller-midp-c-0.1-1000-100]",
"statsmodels/stats/tests/test_rates_poisson.py::test_confint_poisson_alternative[larger-midp-c-0.1-1000-0]",
"statsmodels/stats/tests/test_rates_poisson.py::test_confint_poisson_alternative[larger-sqrt-cent-0.05-10-100]",
"statsmodels/stats/tests/test_rates_poisson.py::test_confint_poisson_alternative[smaller-wald-0.01-100-1]",
"statsmodels/stats/tests/test_rates_poisson.py::test_confint_poisson_alternative[larger-midp-c-0.05-10-100]",
"statsmodels/stats/tests/test_rates_poisson.py::test_confint_poisson_alternative[larger-sqrt-centcc-0.1-1-10]",
"statsmodels/stats/tests/test_rates_poisson.py::test_confint_poisson_alternative[smaller-score-0.01-10-1]",
"statsmodels/stats/tests/test_rates_poisson.py::test_confint_poisson_alternative[smaller-sqrt-0.05-10-10]",
"statsmodels/stats/tests/test_rates_poisson.py::test_confint_poisson_alternative[larger-sqrt-cent-0.1-100-1]",
"statsmodels/stats/tests/test_rates_poisson.py::test_confint_poisson_alternative[smaller-score-0.01-1000-100]",
"statsmodels/stats/tests/test_rates_poisson.py::test_confint_poisson_alternative[larger-exact-c-0.05-1-0]",
"statsmodels/stats/tests/test_rates_poisson.py::test_confint_poisson_alternative[smaller-sqrt-cent-0.01-1000-1]",
"statsmodels/stats/tests/test_rates_poisson.py::test_confint_poisson_alternative[larger-midp-c-0.05-1000-0]",
"statsmodels/stats/tests/test_rates_poisson.py::test_confint_poisson_alternative[smaller-wald-0.05-10-1]",
"statsmodels/stats/tests/test_rates_poisson.py::test_confint_poisson_alternative[larger-sqrt-a-0.05-10-1]",
"statsmodels/stats/tests/test_rates_poisson.py::test_confint_poisson_alternative[smaller-exact-c-0.01-1000-100]",
"statsmodels/stats/tests/test_rates_poisson.py::test_confint_poisson_alternative[larger-sqrt-cent-0.01-10-100]",
"statsmodels/stats/tests/test_rates_poisson.py::test_confint_poisson_alternative[smaller-jeff-0.01-1-0]",
"statsmodels/stats/tests/test_rates_poisson.py::test_confint_poisson_alternative[larger-sqrt-a-0.05-100-0]",
"statsmodels/stats/tests/test_rates_poisson.py::test_confint_poisson_alternative[larger-score-0.05-1000-1]",
"statsmodels/stats/tests/test_rates_poisson.py::test_confint_poisson_alternative[smaller-midp-c-0.01-1-0]",
"statsmodels/stats/tests/test_rates_poisson.py::test_confint_poisson_alternative[larger-sqrt-v-0.01-100-0]",
"statsmodels/stats/tests/test_rates_poisson.py::test_confint_poisson_alternative[larger-score-0.05-1-1]",
"statsmodels/stats/tests/test_rates_poisson.py::test_confint_poisson_alternative[larger-sqrt-a-0.01-10-1]",
"statsmodels/stats/tests/test_rates_poisson.py::test_confint_poisson_alternative[smaller-sqrt-a-0.01-1000-10]",
"statsmodels/stats/tests/test_rates_poisson.py::test_confint_poisson_alternative[larger-wald-0.01-100-10]",
"statsmodels/stats/tests/test_rates_poisson.py::test_confint_poisson_alternative[larger-sqrt-centcc-0.05-100-0]",
"statsmodels/stats/tests/test_rates_poisson.py::test_confint_poisson_alternative[larger-midp-c-0.05-100-0]",
"statsmodels/stats/tests/test_rates_poisson.py::test_confint_poisson_alternative[larger-sqrt-a-0.05-1000-0]",
"statsmodels/stats/tests/test_rates_poisson.py::test_confint_poisson_alternative[smaller-sqrt-v-0.05-1-1]",
"statsmodels/stats/tests/test_rates_poisson.py::test_confint_poisson_alternative[larger-score-0.1-1-100]",
"statsmodels/stats/tests/test_rates_poisson.py::test_confint_poisson_alternative[smaller-sqrt-a-0.1-100-1]",
"statsmodels/stats/tests/test_rates_poisson.py::test_confint_poisson_alternative[smaller-sqrt-a-0.1-100-10]",
"statsmodels/stats/tests/test_rates_poisson.py::test_confint_poisson_alternative[smaller-jeff-0.01-100-1]",
"statsmodels/stats/tests/test_rates_poisson.py::test_confint_poisson_alternative[larger-jeff-0.05-1000-0]",
"statsmodels/stats/tests/test_rates_poisson.py::test_confint_poisson_alternative[larger-sqrt-a-0.1-1000-1]",
"statsmodels/stats/tests/test_rates_poisson.py::test_confint_poisson_alternative[larger-waldccv-0.05-10-0]",
"statsmodels/stats/tests/test_rates_poisson.py::test_confint_poisson_alternative[smaller-jeff-0.1-1000-10]",
"statsmodels/stats/tests/test_rates_poisson.py::test_confint_poisson_alternative[larger-sqrt-v-0.01-10-0]",
"statsmodels/stats/tests/test_rates_poisson.py::test_confint_poisson_alternative[larger-sqrt-v-0.01-1000-1]",
"statsmodels/stats/tests/test_rates_poisson.py::test_confint_poisson_alternative[smaller-wald-0.01-100-100]",
"statsmodels/stats/tests/test_rates_poisson.py::test_confint_poisson_alternative[larger-sqrt-v-0.05-100-1]",
"statsmodels/stats/tests/test_rates_poisson.py::test_confint_poisson_alternative[larger-midp-c-0.01-100-100]",
"statsmodels/stats/tests/test_rates_poisson.py::test_confint_poisson_alternative[smaller-waldccv-0.1-1000-0]",
"statsmodels/stats/tests/test_rates_poisson.py::test_confint_poisson_alternative[smaller-sqrt-a-0.01-10-10]",
"statsmodels/stats/tests/test_rates_poisson.py::test_confint_poisson_alternative[larger-sqrt-0.01-100-100]",
"statsmodels/stats/tests/test_rates_poisson.py::test_confint_poisson_alternative[smaller-sqrt-cent-0.05-100-0]",
"statsmodels/stats/tests/test_rates_poisson.py::test_confint_poisson_alternative[smaller-sqrt-a-0.1-1-1]",
"statsmodels/stats/tests/test_rates_poisson.py::test_confint_poisson_alternative[smaller-midp-c-0.01-100-100]",
"statsmodels/stats/tests/test_rates_poisson.py::test_confint_poisson_alternative[smaller-waldccv-0.01-10-0]",
"statsmodels/stats/tests/test_rates_poisson.py::test_confint_poisson_alternative[smaller-exact-c-0.01-1000-1]",
"statsmodels/stats/tests/test_rates_poisson.py::test_confint_poisson_alternative[smaller-jeff-0.1-100-1]",
"statsmodels/stats/tests/test_rates_poisson.py::test_confint_poisson_alternative[smaller-jeff-0.05-1-10]",
"statsmodels/stats/tests/test_rates_poisson.py::test_confint_poisson_alternative[larger-sqrt-centcc-0.01-1000-0]",
"statsmodels/stats/tests/test_rates_poisson.py::test_confint_poisson_alternative[larger-wald-0.1-1000-10]",
"statsmodels/stats/tests/test_rates_poisson.py::test_confint_poisson_alternative[smaller-wald-0.01-1-0]",
"statsmodels/stats/tests/test_rates_poisson.py::test_confint_poisson_alternative[larger-sqrt-v-0.05-100-10]",
"statsmodels/stats/tests/test_rates_poisson.py::test_confint_poisson_alternative[larger-jeff-0.1-1000-10]",
"statsmodels/stats/tests/test_rates_poisson.py::test_confint_poisson_alternative[smaller-midp-c-0.01-100-0]",
"statsmodels/stats/tests/test_rates_poisson.py::test_confint_poisson_alternative[smaller-midp-c-0.05-1000-10]",
"statsmodels/stats/tests/test_rates_poisson.py::test_confint_poisson_alternative[smaller-jeff-0.1-1000-0]",
"statsmodels/stats/tests/test_rates_poisson.py::test_confint_poisson_alternative[smaller-midp-c-0.1-1000-1]",
"statsmodels/stats/tests/test_rates_poisson.py::test_confint_poisson_alternative[smaller-exact-c-0.01-10-100]",
"statsmodels/stats/tests/test_rates_poisson.py::test_confint_poisson_alternative[smaller-sqrt-cent-0.1-100-100]",
"statsmodels/stats/tests/test_rates_poisson.py::test_confint_poisson_alternative[smaller-sqrt-centcc-0.05-1000-10]",
"statsmodels/stats/tests/test_rates_poisson.py::test_confint_poisson_alternative[larger-jeff-0.1-100-100]",
"statsmodels/stats/tests/test_rates_poisson.py::test_confint_poisson_alternative[smaller-jeff-0.05-10-0]",
"statsmodels/stats/tests/test_rates_poisson.py::test_confint_poisson_alternative[smaller-sqrt-a-0.1-1-100]",
"statsmodels/stats/tests/test_rates_poisson.py::test_confint_poisson_alternative[smaller-sqrt-centcc-0.05-100-1]",
"statsmodels/stats/tests/test_rates_poisson.py::test_confint_poisson_alternative[smaller-sqrt-cent-0.01-10-10]",
"statsmodels/stats/tests/test_rates_poisson.py::test_confint_poisson_alternative[smaller-waldccv-0.05-1-100]",
"statsmodels/stats/tests/test_rates_poisson.py::test_confint_poisson_alternative[larger-wald-0.05-10-100]",
"statsmodels/stats/tests/test_rates_poisson.py::test_confint_poisson_alternative[larger-sqrt-v-0.1-1000-10]",
"statsmodels/stats/tests/test_rates_poisson.py::test_confint_poisson_alternative[smaller-exact-c-0.05-1000-10]",
"statsmodels/stats/tests/test_rates_poisson.py::test_confint_poisson_alternative[larger-waldccv-0.05-1000-0]",
"statsmodels/stats/tests/test_rates_poisson.py::test_confint_poisson_alternative[smaller-sqrt-cent-0.05-10-10]",
"statsmodels/stats/tests/test_rates_poisson.py::test_confint_poisson_alternative[larger-score-0.05-10-10]",
"statsmodels/stats/tests/test_rates_poisson.py::test_confint_poisson_alternative[smaller-waldccv-0.1-100-1]",
"statsmodels/stats/tests/test_rates_poisson.py::test_confint_poisson_alternative[larger-score-0.1-1-1]",
"statsmodels/stats/tests/test_rates_poisson.py::test_confint_poisson_alternative[larger-jeff-0.01-10-1]",
"statsmodels/stats/tests/test_rates_poisson.py::test_confint_poisson_alternative[larger-exact-c-0.01-1000-100]",
"statsmodels/stats/tests/test_rates_poisson.py::test_confint_poisson_alternative[larger-score-0.1-1-10]",
"statsmodels/stats/tests/test_rates_poisson.py::test_confint_poisson_alternative[smaller-wald-0.05-100-100]",
"statsmodels/stats/tests/test_rates_poisson.py::test_confint_poisson_alternative[smaller-sqrt-a-0.01-1-10]",
"statsmodels/stats/tests/test_rates_poisson.py::test_confint_poisson_alternative[larger-midp-c-0.05-1000-10]",
"statsmodels/stats/tests/test_rates_poisson.py::test_confint_poisson_alternative[larger-sqrt-a-0.05-1-10]",
"statsmodels/stats/tests/test_rates_poisson.py::test_confint_poisson_alternative[smaller-sqrt-v-0.01-10-10]",
"statsmodels/stats/tests/test_rates_poisson.py::test_confint_poisson_alternative[smaller-sqrt-0.1-10-0]",
"statsmodels/stats/tests/test_rates_poisson.py::test_confint_poisson_alternative[larger-sqrt-centcc-0.05-1-100]",
"statsmodels/stats/tests/test_rates_poisson.py::test_confint_poisson_alternative[larger-sqrt-centcc-0.05-1000-1]",
"statsmodels/stats/tests/test_rates_poisson.py::test_confint_poisson_alternative[larger-sqrt-0.01-100-10]",
"statsmodels/stats/tests/test_rates_poisson.py::test_confint_poisson_alternative[smaller-sqrt-a-0.01-10-0]",
"statsmodels/stats/tests/test_rates_poisson.py::test_confint_poisson_alternative[larger-sqrt-centcc-0.05-1-0]",
"statsmodels/stats/tests/test_rates_poisson.py::test_confint_poisson_alternative[larger-jeff-0.1-1-0]",
"statsmodels/stats/tests/test_rates_poisson.py::test_confint_poisson_alternative[larger-sqrt-0.1-1-10]",
"statsmodels/stats/tests/test_rates_poisson.py::test_confint_poisson_alternative[larger-sqrt-v-0.1-1000-100]",
"statsmodels/stats/tests/test_rates_poisson.py::test_confint_poisson_alternative[larger-score-0.05-1-0]",
"statsmodels/stats/tests/test_rates_poisson.py::test_confint_poisson_alternative[smaller-score-0.01-100-10]",
"statsmodels/stats/tests/test_rates_poisson.py::test_confint_poisson_alternative[smaller-jeff-0.1-1000-1]",
"statsmodels/stats/tests/test_rates_poisson.py::test_confint_poisson_alternative[larger-sqrt-cent-0.05-100-100]",
"statsmodels/stats/tests/test_rates_poisson.py::test_confint_poisson_alternative[larger-sqrt-cent-0.01-1-10]",
"statsmodels/stats/tests/test_rates_poisson.py::test_confint_poisson_alternative[smaller-jeff-0.01-1-10]",
"statsmodels/stats/tests/test_rates_poisson.py::test_confint_poisson_alternative[smaller-waldccv-0.05-100-10]",
"statsmodels/stats/tests/test_rates_poisson.py::test_confint_poisson_alternative[smaller-wald-0.05-10-100]",
"statsmodels/stats/tests/test_rates_poisson.py::test_confint_poisson_alternative[larger-midp-c-0.1-100-1]",
"statsmodels/stats/tests/test_rates_poisson.py::test_confint_poisson_alternative[larger-waldccv-0.1-1000-0]",
"statsmodels/stats/tests/test_rates_poisson.py::test_confint_poisson_alternative[smaller-sqrt-0.01-10-100]",
"statsmodels/stats/tests/test_rates_poisson.py::test_confint_poisson_alternative[smaller-wald-0.05-1-10]",
"statsmodels/stats/tests/test_rates_poisson.py::test_confint_poisson_alternative[smaller-sqrt-0.1-1000-100]",
"statsmodels/stats/tests/test_rates_poisson.py::test_confint_poisson_alternative[smaller-score-0.05-10-0]",
"statsmodels/stats/tests/test_rates_poisson.py::test_confint_poisson_alternative[larger-sqrt-cent-0.1-1000-0]",
"statsmodels/stats/tests/test_rates_poisson.py::test_confint_poisson_alternative[larger-sqrt-v-0.1-10-100]",
"statsmodels/stats/tests/test_rates_poisson.py::test_confint_poisson_alternative[larger-jeff-0.05-10-100]",
"statsmodels/stats/tests/test_rates_poisson.py::test_confint_poisson_alternative[larger-midp-c-0.05-1-10]",
"statsmodels/stats/tests/test_rates_poisson.py::test_confint_poisson_alternative[smaller-sqrt-0.01-1000-10]",
"statsmodels/stats/tests/test_rates_poisson.py::test_confint_poisson_alternative[smaller-exact-c-0.1-10-100]",
"statsmodels/stats/tests/test_rates_poisson.py::test_confint_poisson_alternative[smaller-waldccv-0.05-1-10]",
"statsmodels/stats/tests/test_rates_poisson.py::test_confint_poisson_alternative[larger-sqrt-cent-0.05-100-10]",
"statsmodels/stats/tests/test_rates_poisson.py::test_confint_poisson_alternative[larger-sqrt-centcc-0.01-1000-10]",
"statsmodels/stats/tests/test_rates_poisson.py::test_confint_poisson_alternative[larger-wald-0.1-10-10]",
"statsmodels/stats/tests/test_rates_poisson.py::test_confint_poisson_alternative[larger-sqrt-v-0.1-10-0]",
"statsmodels/stats/tests/test_rates_poisson.py::test_confint_poisson_alternative[smaller-sqrt-0.01-100-100]",
"statsmodels/stats/tests/test_rates_poisson.py::test_confint_poisson_alternative[smaller-wald-0.05-100-10]",
"statsmodels/stats/tests/test_rates_poisson.py::test_confint_poisson_alternative[larger-wald-0.01-10-100]",
"statsmodels/stats/tests/test_rates_poisson.py::test_confint_poisson_alternative[smaller-midp-c-0.01-10-1]",
"statsmodels/stats/tests/test_rates_poisson.py::test_confint_poisson_alternative[larger-waldccv-0.05-1-100]",
"statsmodels/stats/tests/test_rates_poisson.py::test_confint_poisson_alternative[smaller-sqrt-0.1-1000-1]",
"statsmodels/stats/tests/test_rates_poisson.py::test_confint_poisson_alternative[larger-sqrt-centcc-0.05-1-10]",
"statsmodels/stats/tests/test_rates_poisson.py::test_confint_poisson_alternative[smaller-midp-c-0.1-100-0]",
"statsmodels/stats/tests/test_rates_poisson.py::test_confint_poisson_alternative[smaller-sqrt-cent-0.1-1-10]",
"statsmodels/stats/tests/test_rates_poisson.py::test_confint_poisson_alternative[smaller-sqrt-v-0.1-10-10]",
"statsmodels/stats/tests/test_rates_poisson.py::test_confint_poisson_alternative[larger-wald-0.1-100-1]",
"statsmodels/stats/tests/test_rates_poisson.py::test_confint_poisson_alternative[smaller-waldccv-0.01-1-10]",
"statsmodels/stats/tests/test_rates_poisson.py::test_confint_poisson_alternative[smaller-midp-c-0.01-1000-0]",
"statsmodels/stats/tests/test_rates_poisson.py::test_confint_poisson_alternative[smaller-sqrt-cent-0.05-1000-0]",
"statsmodels/stats/tests/test_rates_poisson.py::test_confint_poisson_alternative[smaller-sqrt-centcc-0.1-10-1]",
"statsmodels/stats/tests/test_rates_poisson.py::test_confint_poisson_alternative[larger-waldccv-0.01-1-1]",
"statsmodels/stats/tests/test_rates_poisson.py::test_confint_poisson_alternative[larger-score-0.01-10-100]",
"statsmodels/stats/tests/test_rates_poisson.py::test_confint_poisson_alternative[larger-sqrt-cent-0.01-1-100]",
"statsmodels/stats/tests/test_rates_poisson.py::test_confint_poisson_alternative[smaller-waldccv-0.01-10-100]",
"statsmodels/stats/tests/test_rates_poisson.py::test_confint_poisson_alternative[smaller-sqrt-centcc-0.05-10-10]",
"statsmodels/stats/tests/test_rates_poisson.py::test_confint_poisson_alternative[larger-sqrt-0.05-1000-10]",
"statsmodels/stats/tests/test_rates_poisson.py::test_confint_poisson_alternative[larger-exact-c-0.01-1000-10]",
"statsmodels/stats/tests/test_rates_poisson.py::test_confint_poisson_alternative[larger-jeff-0.05-10-10]",
"statsmodels/stats/tests/test_rates_poisson.py::test_confint_poisson_alternative[smaller-sqrt-cent-0.01-10-0]",
"statsmodels/stats/tests/test_rates_poisson.py::test_confint_poisson_alternative[smaller-midp-c-0.1-10-10]",
"statsmodels/stats/tests/test_rates_poisson.py::test_confint_poisson_alternative[larger-wald-0.1-1-1]",
"statsmodels/stats/tests/test_rates_poisson.py::test_confint_poisson_alternative[smaller-exact-c-0.05-100-10]",
"statsmodels/stats/tests/test_rates_poisson.py::test_confint_poisson_alternative[smaller-jeff-0.05-1000-0]",
"statsmodels/stats/tests/test_rates_poisson.py::test_confint_poisson_alternative[smaller-sqrt-0.1-1-1]",
"statsmodels/stats/tests/test_rates_poisson.py::test_confint_poisson_alternative[larger-sqrt-v-0.05-1000-1]",
"statsmodels/stats/tests/test_rates_poisson.py::test_confint_poisson_alternative[smaller-wald-0.05-1-100]",
"statsmodels/stats/tests/test_rates_poisson.py::test_confint_poisson_alternative[larger-sqrt-a-0.01-1-0]",
"statsmodels/stats/tests/test_rates_poisson.py::test_confint_poisson_alternative[larger-sqrt-cent-0.01-10-10]",
"statsmodels/stats/tests/test_rates_poisson.py::test_confint_poisson_alternative[smaller-sqrt-cent-0.1-100-1]",
"statsmodels/stats/tests/test_rates_poisson.py::test_confint_poisson_alternative[smaller-sqrt-centcc-0.1-1000-0]",
"statsmodels/stats/tests/test_rates_poisson.py::test_confint_poisson_alternative[smaller-score-0.01-100-1]",
"statsmodels/stats/tests/test_rates_poisson.py::test_confint_poisson_alternative[smaller-sqrt-cent-0.1-1000-100]",
"statsmodels/stats/tests/test_rates_poisson.py::test_confint_poisson_alternative[larger-sqrt-centcc-0.1-100-10]",
"statsmodels/stats/tests/test_rates_poisson.py::test_confint_poisson_alternative[smaller-sqrt-0.1-100-1]",
"statsmodels/stats/tests/test_rates_poisson.py::test_confint_poisson_alternative[larger-sqrt-0.05-1000-0]",
"statsmodels/stats/tests/test_rates_poisson.py::test_confint_poisson_alternative[larger-wald-0.1-100-100]",
"statsmodels/stats/tests/test_rates_poisson.py::test_confint_poisson_alternative[smaller-wald-0.01-1-10]",
"statsmodels/stats/tests/test_rates_poisson.py::test_confint_poisson_alternative[larger-jeff-0.1-1000-0]",
"statsmodels/stats/tests/test_rates_poisson.py::test_confint_poisson_alternative[smaller-midp-c-0.01-1000-1]",
"statsmodels/stats/tests/test_rates_poisson.py::test_confint_poisson_alternative[larger-sqrt-centcc-0.01-100-100]",
"statsmodels/stats/tests/test_rates_poisson.py::test_confint_poisson_alternative[larger-sqrt-a-0.1-1-100]",
"statsmodels/stats/tests/test_rates_poisson.py::test_confint_poisson_alternative[smaller-score-0.05-100-1]",
"statsmodels/stats/tests/test_rates_poisson.py::test_confint_poisson_alternative[smaller-midp-c-0.05-1-0]",
"statsmodels/stats/tests/test_rates_poisson.py::test_confint_poisson_alternative[larger-exact-c-0.05-10-1]",
"statsmodels/stats/tests/test_rates_poisson.py::test_confint_poisson_alternative[smaller-sqrt-a-0.01-100-10]",
"statsmodels/stats/tests/test_rates_poisson.py::test_confint_poisson_alternative[larger-waldccv-0.01-1000-100]",
"statsmodels/stats/tests/test_rates_poisson.py::test_confint_poisson_alternative[smaller-sqrt-0.05-100-1]",
"statsmodels/stats/tests/test_rates_poisson.py::test_confint_poisson_alternative[larger-jeff-0.01-1000-10]",
"statsmodels/stats/tests/test_rates_poisson.py::test_confint_poisson_alternative[larger-sqrt-a-0.05-1000-100]",
"statsmodels/stats/tests/test_rates_poisson.py::test_confint_poisson_alternative[larger-wald-0.05-100-10]",
"statsmodels/stats/tests/test_rates_poisson.py::test_confint_poisson_alternative[larger-sqrt-v-0.05-1000-100]",
"statsmodels/stats/tests/test_rates_poisson.py::test_confint_poisson_alternative[smaller-sqrt-a-0.1-1-0]",
"statsmodels/stats/tests/test_rates_poisson.py::test_confint_poisson_alternative[smaller-midp-c-0.01-1-1]",
"statsmodels/stats/tests/test_rates_poisson.py::test_confint_poisson_alternative[smaller-sqrt-0.05-100-0]",
"statsmodels/stats/tests/test_rates_poisson.py::test_confint_poisson_alternative[smaller-sqrt-v-0.1-100-10]",
"statsmodels/stats/tests/test_rates_poisson.py::test_confint_poisson_alternative[larger-sqrt-v-0.01-10-10]",
"statsmodels/stats/tests/test_rates_poisson.py::test_confint_poisson_alternative[larger-sqrt-v-0.05-10-0]",
"statsmodels/stats/tests/test_rates_poisson.py::test_confint_poisson_alternative[larger-exact-c-0.05-100-1]",
"statsmodels/stats/tests/test_rates_poisson.py::test_confint_poisson_alternative[larger-score-0.05-1000-10]",
"statsmodels/stats/tests/test_rates_poisson.py::test_confint_poisson_alternative[larger-sqrt-cent-0.1-1-10]",
"statsmodels/stats/tests/test_rates_poisson.py::test_confint_poisson_alternative[smaller-jeff-0.01-1000-10]",
"statsmodels/stats/tests/test_rates_poisson.py::test_confint_poisson_alternative[larger-midp-c-0.01-1-1]",
"statsmodels/stats/tests/test_rates_poisson.py::test_confint_poisson_alternative[larger-exact-c-0.05-100-100]",
"statsmodels/stats/tests/test_rates_poisson.py::test_confint_poisson_alternative[smaller-jeff-0.01-10-100]",
"statsmodels/stats/tests/test_rates_poisson.py::test_confint_poisson_alternative[smaller-wald-0.1-10-100]",
"statsmodels/stats/tests/test_rates_poisson.py::test_confint_poisson_alternative[smaller-midp-c-0.01-100-1]",
"statsmodels/stats/tests/test_rates_poisson.py::test_confint_poisson_alternative[larger-sqrt-cent-0.01-1000-0]",
"statsmodels/stats/tests/test_rates_poisson.py::test_confint_poisson_alternative[smaller-wald-0.05-1-1]",
"statsmodels/stats/tests/test_rates_poisson.py::test_confint_poisson_alternative[smaller-waldccv-0.05-1-0]",
"statsmodels/stats/tests/test_rates_poisson.py::test_confint_poisson_alternative[smaller-sqrt-centcc-0.01-1000-10]",
"statsmodels/stats/tests/test_rates_poisson.py::test_confint_poisson_alternative[smaller-sqrt-0.05-1-10]",
"statsmodels/stats/tests/test_rates_poisson.py::test_confint_poisson_alternative[larger-exact-c-0.1-1-10]",
"statsmodels/stats/tests/test_rates_poisson.py::test_confint_poisson_alternative[larger-waldccv-0.05-10-10]",
"statsmodels/stats/tests/test_rates_poisson.py::test_confint_poisson_alternative[smaller-sqrt-centcc-0.1-10-10]",
"statsmodels/stats/tests/test_rates_poisson.py::test_confint_poisson_alternative[larger-exact-c-0.01-1000-0]",
"statsmodels/stats/tests/test_rates_poisson.py::test_confint_poisson_alternative[smaller-exact-c-0.05-1000-100]",
"statsmodels/stats/tests/test_rates_poisson.py::test_confint_poisson_alternative[larger-score-0.1-1000-100]",
"statsmodels/stats/tests/test_rates_poisson.py::test_confint_poisson_alternative[larger-sqrt-a-0.1-1-10]",
"statsmodels/stats/tests/test_rates_poisson.py::test_confint_poisson_alternative[larger-sqrt-a-0.05-100-100]",
"statsmodels/stats/tests/test_rates_poisson.py::test_confint_poisson_alternative[larger-sqrt-0.05-1-10]",
"statsmodels/stats/tests/test_rates_poisson.py::test_confint_poisson_alternative[smaller-sqrt-v-0.01-1-0]",
"statsmodels/stats/tests/test_rates_poisson.py::test_confint_poisson_alternative[larger-waldccv-0.1-1-100]",
"statsmodels/stats/tests/test_rates_poisson.py::test_confint_poisson_alternative[larger-waldccv-0.05-1-1]",
"statsmodels/stats/tests/test_rates_poisson.py::test_confint_poisson_alternative[larger-sqrt-a-0.05-10-10]",
"statsmodels/stats/tests/test_rates_poisson.py::test_confint_poisson_alternative[larger-sqrt-0.01-1-1]",
"statsmodels/stats/tests/test_rates_poisson.py::test_confint_poisson_alternative[smaller-waldccv-0.05-1000-0]",
"statsmodels/stats/tests/test_rates_poisson.py::test_confint_poisson_alternative[smaller-score-0.05-1000-1]",
"statsmodels/stats/tests/test_rates_poisson.py::test_confint_poisson_alternative[larger-waldccv-0.1-100-10]",
"statsmodels/stats/tests/test_rates_poisson.py::test_confint_poisson_alternative[larger-wald-0.01-10-10]",
"statsmodels/stats/tests/test_rates_poisson.py::test_confint_poisson_alternative[smaller-sqrt-centcc-0.05-100-100]",
"statsmodels/stats/tests/test_rates_poisson.py::test_confint_poisson_alternative[larger-sqrt-a-0.1-1-1]",
"statsmodels/stats/tests/test_rates_poisson.py::test_confint_poisson_alternative[smaller-sqrt-cent-0.01-1-1]",
"statsmodels/stats/tests/test_rates_poisson.py::test_confint_poisson_alternative[larger-score-0.1-1000-1]",
"statsmodels/stats/tests/test_rates_poisson.py::test_confint_poisson_alternative[larger-score-0.01-1-1]",
"statsmodels/stats/tests/test_rates_poisson.py::test_confint_poisson_alternative[larger-score-0.05-100-1]",
"statsmodels/stats/tests/test_rates_poisson.py::test_confint_poisson_alternative[smaller-exact-c-0.05-10-1]",
"statsmodels/stats/tests/test_rates_poisson.py::test_confint_poisson_alternative[larger-exact-c-0.01-10-10]",
"statsmodels/stats/tests/test_rates_poisson.py::test_confint_poisson_alternative[smaller-sqrt-centcc-0.01-100-100]",
"statsmodels/stats/tests/test_rates_poisson.py::test_confint_poisson_alternative[larger-waldccv-0.1-100-1]",
"statsmodels/stats/tests/test_rates_poisson.py::test_confint_poisson_alternative[larger-sqrt-cent-0.05-1-1]",
"statsmodels/stats/tests/test_rates_poisson.py::test_confint_poisson_alternative[smaller-waldccv-0.05-10-1]",
"statsmodels/stats/tests/test_rates_poisson.py::test_confint_poisson_alternative[smaller-sqrt-v-0.01-1000-0]",
"statsmodels/stats/tests/test_rates_poisson.py::test_confint_poisson_alternative[smaller-score-0.01-1000-10]",
"statsmodels/stats/tests/test_rates_poisson.py::test_confint_poisson_alternative[smaller-sqrt-0.01-1000-100]",
"statsmodels/stats/tests/test_rates_poisson.py::test_confint_poisson_alternative[smaller-exact-c-0.01-100-1]",
"statsmodels/stats/tests/test_rates_poisson.py::test_confint_poisson_alternative[smaller-score-0.01-100-100]",
"statsmodels/stats/tests/test_rates_poisson.py::test_confint_poisson_alternative[smaller-sqrt-a-0.1-10-0]",
"statsmodels/stats/tests/test_rates_poisson.py::test_confint_poisson_alternative[smaller-sqrt-cent-0.01-100-10]",
"statsmodels/stats/tests/test_rates_poisson.py::test_confint_poisson_alternative[smaller-waldccv-0.01-10-1]",
"statsmodels/stats/tests/test_rates_poisson.py::test_confint_poisson_alternative[larger-sqrt-a-0.01-1-1]",
"statsmodels/stats/tests/test_rates_poisson.py::test_confint_poisson_alternative[smaller-midp-c-0.1-1-10]",
"statsmodels/stats/tests/test_rates_poisson.py::test_confint_poisson_alternative[larger-score-0.1-100-0]",
"statsmodels/stats/tests/test_rates_poisson.py::test_confint_poisson_alternative[larger-sqrt-cent-0.01-100-10]",
"statsmodels/stats/tests/test_rates_poisson.py::test_confint_poisson_alternative[larger-midp-c-0.1-1-0]",
"statsmodels/stats/tests/test_rates_poisson.py::test_confint_poisson_alternative[larger-wald-0.01-100-1]",
"statsmodels/stats/tests/test_rates_poisson.py::test_confint_poisson_alternative[larger-wald-0.05-10-1]",
"statsmodels/stats/tests/test_rates_poisson.py::test_confint_poisson_alternative[smaller-sqrt-0.05-1-100]",
"statsmodels/stats/tests/test_rates_poisson.py::test_confint_poisson_alternative[larger-wald-0.05-10-10]",
"statsmodels/stats/tests/test_rates_poisson.py::test_confint_poisson_alternative[larger-sqrt-v-0.01-1000-0]",
"statsmodels/stats/tests/test_rates_poisson.py::test_confint_poisson_alternative[smaller-sqrt-v-0.1-1000-0]",
"statsmodels/stats/tests/test_rates_poisson.py::test_confint_poisson_alternative[larger-exact-c-0.01-100-100]",
"statsmodels/stats/tests/test_rates_poisson.py::test_confint_poisson_alternative[larger-sqrt-a-0.05-1000-1]",
"statsmodels/stats/tests/test_rates_poisson.py::test_confint_poisson_alternative[smaller-sqrt-a-0.05-1-100]",
"statsmodels/stats/tests/test_rates_poisson.py::test_confint_poisson_alternative[larger-sqrt-a-0.05-10-0]",
"statsmodels/stats/tests/test_rates_poisson.py::test_confint_poisson_alternative[larger-midp-c-0.01-1000-0]",
"statsmodels/stats/tests/test_rates_poisson.py::test_confint_poisson_alternative[larger-wald-0.01-1000-10]",
"statsmodels/stats/tests/test_rates_poisson.py::test_confint_poisson_alternative[smaller-waldccv-0.01-1000-100]",
"statsmodels/stats/tests/test_rates_poisson.py::test_confint_poisson_alternative[smaller-jeff-0.01-10-10]",
"statsmodels/stats/tests/test_rates_poisson.py::test_confint_poisson_alternative[larger-score-0.05-10-0]",
"statsmodels/stats/tests/test_rates_poisson.py::test_confint_poisson_alternative[smaller-score-0.05-1-10]",
"statsmodels/stats/tests/test_rates_poisson.py::test_confint_poisson_alternative[larger-waldccv-0.1-1-10]",
"statsmodels/stats/tests/test_rates_poisson.py::test_confint_poisson_alternative[larger-sqrt-a-0.05-1-0]",
"statsmodels/stats/tests/test_rates_poisson.py::test_confint_poisson_alternative[smaller-sqrt-v-0.01-10-0]",
"statsmodels/stats/tests/test_rates_poisson.py::test_confint_poisson_alternative[larger-sqrt-centcc-0.05-1-1]",
"statsmodels/stats/tests/test_rates_poisson.py::test_confint_poisson_alternative[smaller-sqrt-0.1-10-10]",
"statsmodels/stats/tests/test_rates_poisson.py::test_confint_poisson_alternative[larger-sqrt-v-0.1-1-100]",
"statsmodels/stats/tests/test_rates_poisson.py::test_confint_poisson_alternative[smaller-sqrt-centcc-0.05-10-1]",
"statsmodels/stats/tests/test_rates_poisson.py::test_confint_poisson_alternative[larger-wald-0.05-1-10]",
"statsmodels/stats/tests/test_rates_poisson.py::test_confint_poisson_alternative[larger-midp-c-0.01-1-100]",
"statsmodels/stats/tests/test_rates_poisson.py::test_confint_poisson_alternative[smaller-waldccv-0.05-100-100]",
"statsmodels/stats/tests/test_rates_poisson.py::test_confint_poisson_alternative[smaller-waldccv-0.05-1000-10]",
"statsmodels/stats/tests/test_rates_poisson.py::test_confint_poisson_alternative[larger-wald-0.05-10-0]",
"statsmodels/stats/tests/test_rates_poisson.py::test_confint_poisson_alternative[smaller-sqrt-a-0.05-10-10]",
"statsmodels/stats/tests/test_rates_poisson.py::test_confint_poisson_alternative[smaller-sqrt-cent-0.05-1000-10]",
"statsmodels/stats/tests/test_rates_poisson.py::test_confint_poisson_alternative[smaller-sqrt-v-0.01-10-1]",
"statsmodels/stats/tests/test_rates_poisson.py::test_confint_poisson_alternative[smaller-wald-0.01-1000-10]",
"statsmodels/stats/tests/test_rates_poisson.py::test_confint_poisson_alternative[smaller-waldccv-0.1-10-100]",
"statsmodels/stats/tests/test_rates_poisson.py::test_confint_poisson_alternative[smaller-score-0.01-1-1]",
"statsmodels/stats/tests/test_rates_poisson.py::test_confint_poisson_alternative[larger-score-0.1-10-0]",
"statsmodels/stats/tests/test_rates_poisson.py::test_confint_poisson_alternative[larger-sqrt-v-0.05-1-0]",
"statsmodels/stats/tests/test_rates_poisson.py::test_confint_poisson_alternative[larger-wald-0.1-1000-1]",
"statsmodels/stats/tests/test_rates_poisson.py::test_confint_poisson_alternative[smaller-jeff-0.05-1-100]",
"statsmodels/stats/tests/test_rates_poisson.py::test_confint_poisson_alternative[smaller-wald-0.1-1-10]",
"statsmodels/stats/tests/test_rates_poisson.py::test_confint_poisson_alternative[smaller-sqrt-v-0.05-100-0]",
"statsmodels/stats/tests/test_rates_poisson.py::test_confint_poisson_alternative[larger-jeff-0.1-1-1]",
"statsmodels/stats/tests/test_rates_poisson.py::test_confint_poisson_alternative[smaller-waldccv-0.05-1000-100]",
"statsmodels/stats/tests/test_rates_poisson.py::test_confint_poisson_alternative[smaller-jeff-0.1-10-10]",
"statsmodels/stats/tests/test_rates_poisson.py::test_confint_poisson_alternative[larger-sqrt-0.1-1000-10]",
"statsmodels/stats/tests/test_rates_poisson.py::test_confint_poisson_alternative[smaller-jeff-0.01-1-100]",
"statsmodels/stats/tests/test_rates_poisson.py::test_confint_poisson_alternative[larger-wald-0.05-1000-0]",
"statsmodels/stats/tests/test_rates_poisson.py::test_confint_poisson_alternative[smaller-midp-c-0.1-1-1]",
"statsmodels/stats/tests/test_rates_poisson.py::test_confint_poisson_alternative[smaller-sqrt-0.01-1-1]",
"statsmodels/stats/tests/test_rates_poisson.py::test_confint_poisson_alternative[larger-sqrt-cent-0.1-1-100]",
"statsmodels/stats/tests/test_rates_poisson.py::test_confint_poisson_alternative[smaller-sqrt-a-0.05-1-1]",
"statsmodels/stats/tests/test_rates_poisson.py::test_confint_poisson_alternative[larger-score-0.1-10-100]",
"statsmodels/stats/tests/test_rates_poisson.py::test_confint_poisson_alternative[smaller-score-0.05-10-100]",
"statsmodels/stats/tests/test_rates_poisson.py::test_confint_poisson_alternative[larger-sqrt-centcc-0.1-100-100]",
"statsmodels/stats/tests/test_rates_poisson.py::test_confint_poisson_alternative[larger-exact-c-0.01-100-1]",
"statsmodels/stats/tests/test_rates_poisson.py::test_confint_poisson_alternative[smaller-exact-c-0.01-100-10]",
"statsmodels/stats/tests/test_rates_poisson.py::test_confint_poisson_alternative[smaller-sqrt-a-0.01-1-100]",
"statsmodels/stats/tests/test_rates_poisson.py::test_confint_poisson_alternative[larger-exact-c-0.05-10-0]",
"statsmodels/stats/tests/test_rates_poisson.py::test_confint_poisson_alternative[larger-jeff-0.05-1-1]",
"statsmodels/stats/tests/test_rates_poisson.py::test_confint_poisson_alternative[smaller-sqrt-cent-0.1-1-0]",
"statsmodels/stats/tests/test_rates_poisson.py::test_confint_poisson_alternative[smaller-wald-0.1-100-100]",
"statsmodels/stats/tests/test_rates_poisson.py::test_confint_poisson_alternative[larger-wald-0.1-1000-0]",
"statsmodels/stats/tests/test_rates_poisson.py::test_confint_poisson_alternative[larger-sqrt-v-0.01-100-1]",
"statsmodels/stats/tests/test_rates_poisson.py::test_confint_poisson_alternative[larger-score-0.1-100-10]",
"statsmodels/stats/tests/test_rates_poisson.py::test_confint_poisson_alternative[smaller-score-0.05-1000-100]",
"statsmodels/stats/tests/test_rates_poisson.py::test_confint_poisson_alternative[smaller-score-0.05-1-100]",
"statsmodels/stats/tests/test_rates_poisson.py::test_confint_poisson_alternative[smaller-exact-c-0.01-100-100]",
"statsmodels/stats/tests/test_rates_poisson.py::test_confint_poisson_alternative[smaller-sqrt-a-0.05-1000-1]",
"statsmodels/stats/tests/test_rates_poisson.py::test_confint_poisson_alternative[smaller-midp-c-0.05-1000-0]",
"statsmodels/stats/tests/test_rates_poisson.py::test_confint_poisson_alternative[smaller-score-0.1-100-0]",
"statsmodels/stats/tests/test_rates_poisson.py::test_confint_poisson_alternative[larger-sqrt-a-0.1-10-0]",
"statsmodels/stats/tests/test_rates_poisson.py::test_confint_poisson_alternative[larger-wald-0.1-10-1]",
"statsmodels/stats/tests/test_rates_poisson.py::test_confint_poisson_alternative[smaller-wald-0.1-1-1]",
"statsmodels/stats/tests/test_rates_poisson.py::test_confint_poisson_alternative[smaller-midp-c-0.05-1-1]",
"statsmodels/stats/tests/test_rates_poisson.py::test_confint_poisson_alternative[smaller-sqrt-centcc-0.01-10-0]",
"statsmodels/stats/tests/test_rates_poisson.py::test_confint_poisson_alternative[smaller-sqrt-cent-0.1-1000-0]",
"statsmodels/stats/tests/test_rates_poisson.py::test_confint_poisson_alternative[larger-wald-0.1-10-0]",
"statsmodels/stats/tests/test_rates_poisson.py::test_confint_poisson_alternative[smaller-sqrt-cent-0.01-100-100]",
"statsmodels/stats/tests/test_rates_poisson.py::test_confint_poisson_alternative[larger-sqrt-cent-0.05-100-1]",
"statsmodels/stats/tests/test_rates_poisson.py::test_confint_poisson_alternative[smaller-score-0.01-1-0]",
"statsmodels/stats/tests/test_rates_poisson.py::test_confint_poisson_alternative[larger-exact-c-0.1-1000-10]",
"statsmodels/stats/tests/test_rates_poisson.py::test_confint_poisson_alternative[smaller-sqrt-0.01-1000-0]",
"statsmodels/stats/tests/test_rates_poisson.py::test_confint_poisson_alternative[larger-midp-c-0.05-1-1]",
"statsmodels/stats/tests/test_rates_poisson.py::test_confint_poisson_alternative[smaller-sqrt-cent-0.05-100-100]",
"statsmodels/stats/tests/test_rates_poisson.py::test_confint_poisson_alternative[larger-score-0.05-100-10]",
"statsmodels/stats/tests/test_rates_poisson.py::test_confint_poisson_alternative[larger-sqrt-centcc-0.05-1000-100]",
"statsmodels/stats/tests/test_rates_poisson.py::test_confint_poisson_alternative[smaller-jeff-0.01-1000-1]",
"statsmodels/stats/tests/test_rates_poisson.py::test_confint_poisson_alternative[larger-midp-c-0.01-100-0]",
"statsmodels/stats/tests/test_rates_poisson.py::test_confint_poisson_alternative[larger-sqrt-centcc-0.1-1-1]",
"statsmodels/stats/tests/test_rates_poisson.py::test_confint_poisson_alternative[larger-sqrt-0.1-1-100]",
"statsmodels/stats/tests/test_rates_poisson.py::test_confint_poisson_alternative[larger-exact-c-0.05-10-10]",
"statsmodels/stats/tests/test_rates_poisson.py::test_confint_poisson_alternative[smaller-sqrt-a-0.05-100-10]",
"statsmodels/stats/tests/test_rates_poisson.py::test_confint_poisson_alternative[smaller-midp-c-0.01-100-10]",
"statsmodels/stats/tests/test_rates_poisson.py::test_confint_poisson_alternative[smaller-exact-c-0.1-100-100]",
"statsmodels/stats/tests/test_rates_poisson.py::test_confint_poisson_alternative[larger-sqrt-a-0.1-10-1]",
"statsmodels/stats/tests/test_rates_poisson.py::test_confint_poisson_alternative[larger-sqrt-v-0.05-100-0]",
"statsmodels/stats/tests/test_rates_poisson.py::test_confint_poisson_alternative[smaller-sqrt-0.01-1-10]",
"statsmodels/stats/tests/test_rates_poisson.py::test_confint_poisson_alternative[larger-sqrt-cent-0.1-1-0]",
"statsmodels/stats/tests/test_rates_poisson.py::test_confint_poisson_alternative[smaller-exact-c-0.01-1000-10]",
"statsmodels/stats/tests/test_rates_poisson.py::test_confint_poisson_alternative[larger-waldccv-0.05-1000-100]",
"statsmodels/stats/tests/test_rates_poisson.py::test_confint_poisson_alternative[smaller-waldccv-0.1-100-0]",
"statsmodels/stats/tests/test_rates_poisson.py::test_confint_poisson_alternative[larger-score-0.1-10-1]",
"statsmodels/stats/tests/test_rates_poisson.py::test_confint_poisson_alternative[larger-sqrt-v-0.1-10-10]",
"statsmodels/stats/tests/test_rates_poisson.py::test_confint_poisson_alternative[smaller-sqrt-cent-0.05-10-0]",
"statsmodels/stats/tests/test_rates_poisson.py::test_confint_poisson_alternative[larger-sqrt-centcc-0.05-1000-0]",
"statsmodels/stats/tests/test_rates_poisson.py::test_confint_poisson_alternative[smaller-sqrt-v-0.1-100-0]",
"statsmodels/stats/tests/test_rates_poisson.py::test_confint_poisson_alternative[larger-waldccv-0.01-10-1]",
"statsmodels/stats/tests/test_rates_poisson.py::test_confint_poisson_alternative[smaller-wald-0.1-10-10]",
"statsmodels/stats/tests/test_rates_poisson.py::test_confint_poisson_alternative[larger-sqrt-v-0.01-1-1]",
"statsmodels/stats/tests/test_rates_poisson.py::test_confint_poisson_alternative[larger-waldccv-0.01-1000-1]",
"statsmodels/stats/tests/test_rates_poisson.py::test_confint_poisson_alternative[smaller-sqrt-v-0.01-1000-10]",
"statsmodels/stats/tests/test_rates_poisson.py::test_confint_poisson_alternative[smaller-midp-c-0.01-10-10]",
"statsmodels/stats/tests/test_rates_poisson.py::test_confint_poisson_alternative[smaller-jeff-0.01-1-1]",
"statsmodels/stats/tests/test_rates_poisson.py::test_confint_poisson_alternative[smaller-jeff-0.05-100-0]",
"statsmodels/stats/tests/test_rates_poisson.py::test_confint_poisson_alternative[smaller-sqrt-cent-0.05-1000-100]",
"statsmodels/stats/tests/test_rates_poisson.py::test_confint_poisson_alternative[larger-sqrt-0.01-100-0]",
"statsmodels/stats/tests/test_rates_poisson.py::test_confint_poisson_alternative[smaller-sqrt-centcc-0.1-1-100]",
"statsmodels/stats/tests/test_rates_poisson.py::test_confint_poisson_alternative[smaller-jeff-0.01-10-0]",
"statsmodels/stats/tests/test_rates_poisson.py::test_confint_poisson_alternative[smaller-wald-0.05-10-0]",
"statsmodels/stats/tests/test_rates_poisson.py::test_confint_poisson_alternative[smaller-sqrt-0.05-100-100]",
"statsmodels/stats/tests/test_rates_poisson.py::test_confint_poisson_alternative[larger-wald-0.1-1-10]",
"statsmodels/stats/tests/test_rates_poisson.py::test_confint_poisson_alternative[larger-midp-c-0.1-1-100]",
"statsmodels/stats/tests/test_rates_poisson.py::test_confint_poisson_alternative[smaller-score-0.05-1000-0]",
"statsmodels/stats/tests/test_rates_poisson.py::test_confint_poisson_alternative[smaller-exact-c-0.05-1000-1]",
"statsmodels/stats/tests/test_rates_poisson.py::test_confint_poisson_alternative[smaller-sqrt-v-0.05-1000-0]",
"statsmodels/stats/tests/test_rates_poisson.py::test_confint_poisson_alternative[larger-wald-0.1-1000-100]",
"statsmodels/stats/tests/test_rates_poisson.py::test_confint_poisson_alternative[smaller-sqrt-centcc-0.05-10-0]",
"statsmodels/stats/tests/test_rates_poisson.py::test_confint_poisson_alternative[larger-sqrt-0.1-100-1]",
"statsmodels/stats/tests/test_rates_poisson.py::test_confint_poisson_alternative[smaller-exact-c-0.01-10-10]",
"statsmodels/stats/tests/test_rates_poisson.py::test_confint_poisson_alternative[larger-jeff-0.05-100-1]",
"statsmodels/stats/tests/test_rates_poisson.py::test_confint_poisson_alternative[larger-exact-c-0.05-1-1]",
"statsmodels/stats/tests/test_rates_poisson.py::test_confint_poisson_alternative[smaller-sqrt-centcc-0.01-100-10]",
"statsmodels/stats/tests/test_rates_poisson.py::test_confint_poisson_alternative[larger-waldccv-0.05-1000-10]",
"statsmodels/stats/tests/test_rates_poisson.py::test_confint_poisson_alternative[larger-waldccv-0.01-10-100]",
"statsmodels/stats/tests/test_rates_poisson.py::test_confint_poisson_alternative[larger-wald-0.01-1-0]",
"statsmodels/stats/tests/test_rates_poisson.py::test_confint_poisson_alternative[larger-sqrt-v-0.01-1-0]",
"statsmodels/stats/tests/test_rates_poisson.py::test_confint_poisson_alternative[smaller-midp-c-0.05-100-100]",
"statsmodels/stats/tests/test_rates_poisson.py::test_confint_poisson_alternative[smaller-jeff-0.05-1000-100]",
"statsmodels/stats/tests/test_rates_poisson.py::test_confint_poisson_alternative[smaller-sqrt-v-0.05-1000-10]",
"statsmodels/stats/tests/test_rates_poisson.py::test_confint_poisson_alternative[smaller-jeff-0.1-1-10]",
"statsmodels/stats/tests/test_rates_poisson.py::test_confint_poisson_alternative[smaller-sqrt-0.05-1000-0]",
"statsmodels/stats/tests/test_rates_poisson.py::test_confint_poisson_alternative[smaller-sqrt-v-0.1-10-0]",
"statsmodels/stats/tests/test_rates_poisson.py::test_confint_poisson_alternative[smaller-score-0.1-10-10]",
"statsmodels/stats/tests/test_rates_poisson.py::test_confint_poisson_alternative[smaller-sqrt-cent-0.05-10-1]",
"statsmodels/stats/tests/test_rates_poisson.py::test_confint_poisson_alternative[smaller-score-0.01-100-0]",
"statsmodels/stats/tests/test_rates_poisson.py::test_confint_poisson_alternative[larger-score-0.01-1-0]",
"statsmodels/stats/tests/test_rates_poisson.py::test_confint_poisson_alternative[larger-wald-0.1-100-10]",
"statsmodels/stats/tests/test_rates_poisson.py::test_confint_poisson_alternative[smaller-jeff-0.01-1000-100]",
"statsmodels/stats/tests/test_rates_poisson.py::test_confint_poisson_alternative[smaller-jeff-0.01-1000-0]",
"statsmodels/stats/tests/test_rates_poisson.py::test_confint_poisson_alternative[larger-score-0.01-100-10]",
"statsmodels/stats/tests/test_rates_poisson.py::test_confint_poisson_alternative[larger-jeff-0.05-100-100]",
"statsmodels/stats/tests/test_rates_poisson.py::test_confint_poisson_alternative[larger-jeff-0.05-1000-10]",
"statsmodels/stats/tests/test_rates_poisson.py::test_confint_poisson_alternative[smaller-wald-0.1-1000-10]",
"statsmodels/stats/tests/test_rates_poisson.py::test_confint_poisson_alternative[smaller-wald-0.01-10-1]",
"statsmodels/stats/tests/test_rates_poisson.py::test_confint_poisson_alternative[larger-sqrt-v-0.1-100-100]",
"statsmodels/stats/tests/test_rates_poisson.py::test_confint_poisson_alternative[smaller-sqrt-centcc-0.05-1-0]",
"statsmodels/stats/tests/test_rates_poisson.py::test_confint_poisson_alternative[smaller-sqrt-v-0.1-1000-10]",
"statsmodels/stats/tests/test_rates_poisson.py::test_confint_poisson_alternative[larger-sqrt-0.05-10-1]",
"statsmodels/stats/tests/test_rates_poisson.py::test_confint_poisson_alternative[smaller-exact-c-0.1-1-10]",
"statsmodels/stats/tests/test_rates_poisson.py::test_confint_poisson_alternative[larger-sqrt-0.1-100-0]",
"statsmodels/stats/tests/test_rates_poisson.py::test_confint_poisson_alternative[larger-sqrt-cent-0.05-10-10]",
"statsmodels/stats/tests/test_rates_poisson.py::test_confint_poisson_alternative[larger-sqrt-centcc-0.05-100-1]",
"statsmodels/stats/tests/test_rates_poisson.py::test_confint_poisson_alternative[smaller-sqrt-a-0.05-1000-100]",
"statsmodels/stats/tests/test_rates_poisson.py::test_confint_poisson_alternative[smaller-sqrt-cent-0.01-10-100]",
"statsmodels/stats/tests/test_rates_poisson.py::test_confint_poisson_alternative[smaller-waldccv-0.01-1-1]",
"statsmodels/stats/tests/test_rates_poisson.py::test_confint_poisson_alternative[smaller-sqrt-0.05-1-0]",
"statsmodels/stats/tests/test_rates_poisson.py::test_confint_poisson_alternative[larger-waldccv-0.05-100-10]",
"statsmodels/stats/tests/test_rates_poisson.py::test_confint_poisson_alternative[smaller-midp-c-0.1-10-1]",
"statsmodels/stats/tests/test_rates_poisson.py::test_confint_poisson_alternative[larger-exact-c-0.05-100-0]",
"statsmodels/stats/tests/test_rates_poisson.py::test_confint_poisson_alternative[larger-sqrt-a-0.01-100-100]",
"statsmodels/stats/tests/test_rates_poisson.py::test_confint_poisson_alternative[smaller-exact-c-0.1-10-0]",
"statsmodels/stats/tests/test_rates_poisson.py::test_confint_poisson_alternative[larger-waldccv-0.05-100-1]",
"statsmodels/stats/tests/test_rates_poisson.py::test_confint_poisson_alternative[smaller-sqrt-cent-0.01-1-0]",
"statsmodels/stats/tests/test_rates_poisson.py::test_confint_poisson_alternative[larger-jeff-0.1-1-100]",
"statsmodels/stats/tests/test_rates_poisson.py::test_confint_poisson_alternative[larger-waldccv-0.01-100-100]",
"statsmodels/stats/tests/test_rates_poisson.py::test_confint_poisson_alternative[smaller-sqrt-centcc-0.1-1-0]",
"statsmodels/stats/tests/test_rates_poisson.py::test_confint_poisson_alternative[larger-exact-c-0.05-1000-10]",
"statsmodels/stats/tests/test_rates_poisson.py::test_confint_poisson_alternative[smaller-waldccv-0.05-10-100]",
"statsmodels/stats/tests/test_rates_poisson.py::test_confint_poisson_alternative[smaller-sqrt-v-0.05-100-100]",
"statsmodels/stats/tests/test_rates_poisson.py::test_confint_poisson_alternative[larger-waldccv-0.05-1-0]",
"statsmodels/stats/tests/test_rates_poisson.py::test_confint_poisson_alternative[larger-sqrt-v-0.01-1-10]",
"statsmodels/stats/tests/test_rates_poisson.py::test_confint_poisson_alternative[smaller-midp-c-0.01-10-0]",
"statsmodels/stats/tests/test_rates_poisson.py::test_confint_poisson_alternative[larger-sqrt-cent-0.1-100-0]",
"statsmodels/stats/tests/test_rates_poisson.py::test_confint_poisson_alternative[smaller-sqrt-centcc-0.1-100-1]",
"statsmodels/stats/tests/test_rates_poisson.py::test_confint_poisson_alternative[smaller-score-0.01-1-100]",
"statsmodels/stats/tests/test_rates_poisson.py::test_confint_poisson_alternative[smaller-sqrt-centcc-0.01-1-0]",
"statsmodels/stats/tests/test_rates_poisson.py::test_confint_poisson_alternative[larger-exact-c-0.1-1000-0]",
"statsmodels/stats/tests/test_rates_poisson.py::test_confint_poisson_alternative[larger-sqrt-a-0.05-1-100]",
"statsmodels/stats/tests/test_rates_poisson.py::test_confint_poisson_alternative[larger-jeff-0.05-1-0]",
"statsmodels/stats/tests/test_rates_poisson.py::test_confint_poisson_alternative[larger-wald-0.01-1000-1]",
"statsmodels/stats/tests/test_rates_poisson.py::test_confint_poisson_alternative[larger-sqrt-centcc-0.1-1000-10]",
"statsmodels/stats/tests/test_rates_poisson.py::test_confint_poisson_alternative[smaller-score-0.1-1000-10]",
"statsmodels/stats/tests/test_rates_poisson.py::test_confint_poisson_alternative[smaller-exact-c-0.01-1-100]",
"statsmodels/stats/tests/test_rates_poisson.py::test_confint_poisson_alternative[larger-waldccv-0.05-100-0]",
"statsmodels/stats/tests/test_rates_poisson.py::test_confint_poisson_alternative[smaller-wald-0.01-100-0]",
"statsmodels/stats/tests/test_rates_poisson.py::test_confint_poisson_alternative[smaller-sqrt-cent-0.05-1-0]",
"statsmodels/stats/tests/test_rates_poisson.py::test_confint_poisson_alternative[larger-waldccv-0.1-10-10]",
"statsmodels/stats/tests/test_rates_poisson.py::test_confint_poisson_alternative[smaller-waldccv-0.1-1-1]",
"statsmodels/stats/tests/test_rates_poisson.py::test_confint_poisson_alternative[larger-midp-c-0.05-10-0]",
"statsmodels/stats/tests/test_rates_poisson.py::test_confint_poisson_alternative[smaller-exact-c-0.05-10-0]",
"statsmodels/stats/tests/test_rates_poisson.py::test_confint_poisson_alternative[larger-exact-c-0.05-1-100]",
"statsmodels/stats/tests/test_rates_poisson.py::test_confint_poisson_alternative[larger-wald-0.01-1000-0]",
"statsmodels/stats/tests/test_rates_poisson.py::test_confint_poisson_alternative[larger-midp-c-0.01-1000-100]",
"statsmodels/stats/tests/test_rates_poisson.py::test_confint_poisson_alternative[larger-wald-0.01-100-0]",
"statsmodels/stats/tests/test_rates_poisson.py::test_confint_poisson_alternative[larger-waldccv-0.05-1000-1]",
"statsmodels/stats/tests/test_rates_poisson.py::test_confint_poisson_alternative[larger-wald-0.05-1-0]",
"statsmodels/stats/tests/test_rates_poisson.py::test_confint_poisson_alternative[larger-score-0.05-1-10]",
"statsmodels/stats/tests/test_rates_poisson.py::test_confint_poisson_alternative[smaller-midp-c-0.01-1-100]",
"statsmodels/stats/tests/test_rates_poisson.py::test_confint_poisson_alternative[smaller-sqrt-centcc-0.05-100-10]",
"statsmodels/stats/tests/test_rates_poisson.py::test_confint_poisson_alternative[larger-waldccv-0.1-100-100]",
"statsmodels/stats/tests/test_rates_poisson.py::test_confint_poisson_alternative[smaller-sqrt-v-0.01-10-100]",
"statsmodels/stats/tests/test_rates_poisson.py::test_confint_poisson_alternative[larger-sqrt-0.1-1000-0]",
"statsmodels/stats/tests/test_rates_poisson.py::test_confint_poisson_alternative[smaller-sqrt-0.01-100-10]",
"statsmodels/stats/tests/test_rates_poisson.py::test_confint_poisson_alternative[smaller-sqrt-centcc-0.1-10-100]",
"statsmodels/stats/tests/test_rates_poisson.py::test_confint_poisson_alternative[larger-score-0.1-1000-10]",
"statsmodels/stats/tests/test_rates_poisson.py::test_confint_poisson_alternative[larger-sqrt-cent-0.05-10-0]",
"statsmodels/stats/tests/test_rates_poisson.py::test_confint_poisson_alternative[smaller-wald-0.05-100-0]",
"statsmodels/stats/tests/test_rates_poisson.py::test_confint_poisson_alternative[larger-sqrt-a-0.1-1000-0]",
"statsmodels/stats/tests/test_rates_poisson.py::test_confint_poisson_alternative[smaller-sqrt-0.01-10-0]",
"statsmodels/stats/tests/test_rates_poisson.py::test_confint_poisson_alternative[smaller-jeff-0.1-100-10]",
"statsmodels/stats/tests/test_rates_poisson.py::test_confint_poisson_alternative[larger-sqrt-0.05-1-0]",
"statsmodels/stats/tests/test_rates_poisson.py::test_confint_poisson_alternative[smaller-wald-0.01-1-1]",
"statsmodels/stats/tests/test_rates_poisson.py::test_confint_poisson_alternative[smaller-sqrt-centcc-0.05-1000-1]",
"statsmodels/stats/tests/test_rates_poisson.py::test_confint_poisson_alternative[smaller-sqrt-centcc-0.05-100-0]",
"statsmodels/stats/tests/test_rates_poisson.py::test_confint_poisson_alternative[smaller-jeff-0.1-10-100]",
"statsmodels/stats/tests/test_rates_poisson.py::test_confint_poisson_alternative[larger-jeff-0.1-1-10]",
"statsmodels/stats/tests/test_rates_poisson.py::test_confint_poisson_alternative[larger-sqrt-cent-0.05-1-100]",
"statsmodels/stats/tests/test_rates_poisson.py::test_confint_poisson_alternative[larger-exact-c-0.01-1000-1]",
"statsmodels/stats/tests/test_rates_poisson.py::test_confint_poisson_alternative[larger-jeff-0.01-100-1]",
"statsmodels/stats/tests/test_rates_poisson.py::test_confint_poisson_alternative[smaller-score-0.05-10-10]",
"statsmodels/stats/tests/test_rates_poisson.py::test_confint_poisson_alternative[smaller-wald-0.05-10-10]",
"statsmodels/stats/tests/test_rates_poisson.py::test_confint_poisson_alternative[larger-jeff-0.01-1-100]",
"statsmodels/stats/tests/test_rates_poisson.py::test_confint_poisson_alternative[larger-jeff-0.1-100-10]",
"statsmodels/stats/tests/test_rates_poisson.py::test_confint_poisson_alternative[smaller-sqrt-v-0.01-100-100]",
"statsmodels/stats/tests/test_rates_poisson.py::test_confint_poisson_alternative[larger-midp-c-0.01-100-10]",
"statsmodels/stats/tests/test_rates_poisson.py::test_confint_poisson_alternative[larger-sqrt-0.05-100-100]",
"statsmodels/stats/tests/test_rates_poisson.py::test_confint_poisson_alternative[larger-exact-c-0.1-1000-1]",
"statsmodels/stats/tests/test_rates_poisson.py::test_confint_poisson_alternative[smaller-jeff-0.01-100-100]",
"statsmodels/stats/tests/test_rates_poisson.py::test_confint_poisson_alternative[smaller-sqrt-a-0.1-1000-100]",
"statsmodels/stats/tests/test_rates_poisson.py::test_confint_poisson_alternative[larger-sqrt-v-0.01-100-10]",
"statsmodels/stats/tests/test_rates_poisson.py::test_confint_poisson_alternative[smaller-sqrt-0.05-1000-10]",
"statsmodels/stats/tests/test_rates_poisson.py::test_confint_poisson_alternative[larger-exact-c-0.1-100-0]",
"statsmodels/stats/tests/test_rates_poisson.py::test_confint_poisson_alternative[smaller-sqrt-a-0.1-100-100]",
"statsmodels/stats/tests/test_rates_poisson.py::test_confint_poisson_alternative[smaller-score-0.1-10-0]",
"statsmodels/stats/tests/test_rates_poisson.py::test_confint_poisson_alternative[smaller-sqrt-v-0.01-1000-100]",
"statsmodels/stats/tests/test_rates_poisson.py::test_confint_poisson_alternative[smaller-exact-c-0.1-10-1]",
"statsmodels/stats/tests/test_rates_poisson.py::test_confint_poisson_alternative[larger-jeff-0.1-1000-100]",
"statsmodels/stats/tests/test_rates_poisson.py::test_confint_poisson_alternative[smaller-midp-c-0.1-100-1]",
"statsmodels/stats/tests/test_rates_poisson.py::test_confint_poisson_alternative[larger-exact-c-0.1-100-1]",
"statsmodels/stats/tests/test_rates_poisson.py::test_confint_poisson_alternative[smaller-sqrt-centcc-0.05-10-100]",
"statsmodels/stats/tests/test_rates_poisson.py::test_confint_poisson_alternative[smaller-wald-0.1-1000-100]",
"statsmodels/stats/tests/test_rates_poisson.py::test_confint_poisson_alternative[smaller-wald-0.01-10-10]",
"statsmodels/stats/tests/test_rates_poisson.py::test_confint_poisson_alternative[smaller-sqrt-centcc-0.1-10-0]",
"statsmodels/stats/tests/test_rates_poisson.py::test_confint_poisson_alternative[larger-wald-0.01-1000-100]",
"statsmodels/stats/tests/test_rates_poisson.py::test_confint_poisson_alternative[smaller-exact-c-0.1-1-0]",
"statsmodels/stats/tests/test_rates_poisson.py::test_confint_poisson_alternative[larger-sqrt-a-0.05-100-10]",
"statsmodels/stats/tests/test_rates_poisson.py::test_confint_poisson_alternative[larger-sqrt-a-0.01-100-0]",
"statsmodels/stats/tests/test_rates_poisson.py::test_confint_poisson_alternative[larger-score-0.01-100-1]",
"statsmodels/stats/tests/test_rates_poisson.py::test_confint_poisson_alternative[smaller-wald-0.05-1000-0]",
"statsmodels/stats/tests/test_rates_poisson.py::test_confint_poisson_alternative[larger-sqrt-0.05-10-0]",
"statsmodels/stats/tests/test_rates_poisson.py::test_confint_poisson_alternative[smaller-score-0.01-1000-1]",
"statsmodels/stats/tests/test_rates_poisson.py::test_confint_poisson_alternative[smaller-exact-c-0.01-1-10]",
"statsmodels/stats/tests/test_rates_poisson.py::test_confint_poisson_alternative[smaller-sqrt-centcc-0.05-1-10]",
"statsmodels/stats/tests/test_rates_poisson.py::test_confint_poisson_alternative[smaller-sqrt-0.05-1000-100]",
"statsmodels/stats/tests/test_rates_poisson.py::test_confint_poisson_alternative[larger-exact-c-0.01-10-0]",
"statsmodels/stats/tests/test_rates_poisson.py::test_confint_poisson_alternative[larger-waldccv-0.1-1000-100]",
"statsmodels/stats/tests/test_rates_poisson.py::test_confint_poisson_alternative[larger-waldccv-0.01-10-10]",
"statsmodels/stats/tests/test_rates_poisson.py::test_confint_poisson_alternative[smaller-sqrt-0.01-10-1]",
"statsmodels/stats/tests/test_rates_poisson.py::test_confint_poisson_alternative[larger-sqrt-a-0.01-1000-1]",
"statsmodels/stats/tests/test_rates_poisson.py::test_confint_poisson_alternative[larger-exact-c-0.1-10-1]",
"statsmodels/stats/tests/test_rates_poisson.py::test_confint_poisson_alternative[larger-sqrt-a-0.1-10-100]",
"statsmodels/stats/tests/test_rates_poisson.py::test_confint_poisson_alternative[smaller-sqrt-a-0.01-100-100]",
"statsmodels/stats/tests/test_rates_poisson.py::test_confint_poisson_alternative[larger-sqrt-centcc-0.01-100-1]",
"statsmodels/stats/tests/test_rates_poisson.py::test_confint_poisson_alternative[smaller-midp-c-0.1-10-100]",
"statsmodels/stats/tests/test_rates_poisson.py::test_confint_poisson_alternative[larger-score-0.01-1000-1]",
"statsmodels/stats/tests/test_rates_poisson.py::test_confint_poisson_alternative[smaller-sqrt-centcc-0.01-100-0]",
"statsmodels/stats/tests/test_rates_poisson.py::test_confint_poisson_alternative[larger-sqrt-0.05-100-0]",
"statsmodels/stats/tests/test_rates_poisson.py::test_confint_poisson_alternative[smaller-sqrt-cent-0.05-10-100]",
"statsmodels/stats/tests/test_rates_poisson.py::test_confint_poisson_alternative[smaller-sqrt-centcc-0.01-1-10]",
"statsmodels/stats/tests/test_rates_poisson.py::test_confint_poisson_alternative[larger-midp-c-0.05-1000-1]",
"statsmodels/stats/tests/test_rates_poisson.py::test_confint_poisson_alternative[larger-midp-c-0.1-10-1]",
"statsmodels/stats/tests/test_rates_poisson.py::test_confint_poisson_alternative[smaller-sqrt-a-0.01-1-1]",
"statsmodels/stats/tests/test_rates_poisson.py::test_confint_poisson_alternative[smaller-exact-c-0.05-1-10]",
"statsmodels/stats/tests/test_rates_poisson.py::test_confint_poisson_alternative[larger-score-0.01-1000-100]",
"statsmodels/stats/tests/test_rates_poisson.py::test_confint_poisson_alternative[smaller-waldccv-0.1-1-0]",
"statsmodels/stats/tests/test_rates_poisson.py::test_confint_poisson_alternative[smaller-score-0.1-1000-100]",
"statsmodels/stats/tests/test_rates_poisson.py::test_confint_poisson_alternative[smaller-sqrt-a-0.1-10-100]",
"statsmodels/stats/tests/test_rates_poisson.py::test_confint_poisson_alternative[smaller-sqrt-cent-0.1-1-1]",
"statsmodels/stats/tests/test_rates_poisson.py::test_confint_poisson_alternative[smaller-waldccv-0.1-1-100]",
"statsmodels/stats/tests/test_rates_poisson.py::test_confint_poisson_alternative[larger-jeff-0.1-10-1]",
"statsmodels/stats/tests/test_rates_poisson.py::test_confint_poisson_alternative[larger-sqrt-a-0.1-1-0]",
"statsmodels/stats/tests/test_rates_poisson.py::test_confint_poisson_alternative[smaller-exact-c-0.1-100-1]",
"statsmodels/stats/tests/test_rates_poisson.py::test_confint_poisson_alternative[larger-sqrt-v-0.01-1-100]",
"statsmodels/stats/tests/test_rates_poisson.py::test_confint_poisson_alternative[smaller-jeff-0.1-100-0]",
"statsmodels/stats/tests/test_rates_poisson.py::test_confint_poisson_alternative[larger-jeff-0.01-100-0]",
"statsmodels/stats/tests/test_rates_poisson.py::test_confint_poisson_alternative[larger-sqrt-v-0.1-1-10]",
"statsmodels/stats/tests/test_rates_poisson.py::test_confint_poisson_alternative[larger-sqrt-0.05-1-1]",
"statsmodels/stats/tests/test_rates_poisson.py::test_confint_poisson_alternative[smaller-score-0.1-10-1]",
"statsmodels/stats/tests/test_rates_poisson.py::test_confint_poisson_alternative[larger-sqrt-0.01-10-10]",
"statsmodels/stats/tests/test_rates_poisson.py::test_confint_poisson_alternative[smaller-midp-c-0.05-100-0]",
"statsmodels/stats/tests/test_rates_poisson.py::test_confint_poisson_alternative[larger-sqrt-centcc-0.05-10-1]",
"statsmodels/stats/tests/test_rates_poisson.py::test_confint_poisson_alternative[smaller-score-0.01-10-0]",
"statsmodels/stats/tests/test_rates_poisson.py::test_confint_poisson_alternative[larger-jeff-0.05-1-100]",
"statsmodels/stats/tests/test_rates_poisson.py::test_confint_poisson_alternative[larger-score-0.1-100-1]",
"statsmodels/stats/tests/test_rates_poisson.py::test_confint_poisson_alternative[smaller-jeff-0.05-1-0]",
"statsmodels/stats/tests/test_rates_poisson.py::test_confint_poisson_alternative[larger-sqrt-cent-0.05-1000-1]",
"statsmodels/stats/tests/test_rates_poisson.py::test_confint_poisson_alternative[larger-exact-c-0.1-10-100]",
"statsmodels/stats/tests/test_rates_poisson.py::test_confint_poisson_alternative[larger-exact-c-0.05-10-100]",
"statsmodels/stats/tests/test_rates_poisson.py::test_confint_poisson_alternative[smaller-sqrt-a-0.05-10-100]",
"statsmodels/stats/tests/test_rates_poisson.py::test_confint_poisson_alternative[smaller-exact-c-0.1-100-0]",
"statsmodels/stats/tests/test_rates_poisson.py::test_confint_poisson_alternative[smaller-midp-c-0.01-1000-10]",
"statsmodels/stats/tests/test_rates_poisson.py::test_confint_poisson_alternative[smaller-midp-c-0.1-1000-0]",
"statsmodels/stats/tests/test_rates_poisson.py::test_confint_poisson_alternative[smaller-sqrt-cent-0.01-1-10]",
"statsmodels/stats/tests/test_rates_poisson.py::test_confint_poisson_alternative[smaller-waldccv-0.05-1000-1]",
"statsmodels/stats/tests/test_rates_poisson.py::test_confint_poisson_alternative[larger-sqrt-a-0.01-10-10]",
"statsmodels/stats/tests/test_rates_poisson.py::test_confint_poisson_alternative[smaller-sqrt-a-0.01-1000-100]",
"statsmodels/stats/tests/test_rates_poisson.py::test_confint_poisson_alternative[smaller-sqrt-a-0.01-100-1]",
"statsmodels/stats/tests/test_rates_poisson.py::test_confint_poisson_alternative[smaller-wald-0.01-100-10]",
"statsmodels/stats/tests/test_rates_poisson.py::test_confint_poisson_alternative[smaller-midp-c-0.1-100-10]",
"statsmodels/stats/tests/test_rates_poisson.py::test_confint_poisson_alternative[smaller-sqrt-0.1-1000-0]",
"statsmodels/stats/tests/test_rates_poisson.py::test_confint_poisson_alternative[larger-sqrt-cent-0.01-10-1]",
"statsmodels/stats/tests/test_rates_poisson.py::test_confint_poisson_alternative[smaller-jeff-0.1-1-100]",
"statsmodels/stats/tests/test_rates_poisson.py::test_confint_poisson_alternative[larger-sqrt-a-0.1-1000-100]",
"statsmodels/stats/tests/test_rates_poisson.py::test_confint_poisson_alternative[smaller-waldccv-0.01-1000-0]",
"statsmodels/stats/tests/test_rates_poisson.py::test_confint_poisson_alternative[larger-wald-0.05-100-100]",
"statsmodels/stats/tests/test_rates_poisson.py::test_confint_poisson_alternative[smaller-sqrt-0.05-100-10]",
"statsmodels/stats/tests/test_rates_poisson.py::test_confint_poisson_alternative[larger-sqrt-centcc-0.1-10-10]",
"statsmodels/stats/tests/test_rates_poisson.py::test_confint_poisson_alternative[larger-sqrt-cent-0.1-1000-10]",
"statsmodels/stats/tests/test_rates_poisson.py::test_confint_poisson_alternative[larger-waldccv-0.01-1-100]",
"statsmodels/stats/tests/test_rates_poisson.py::test_confint_poisson_alternative[smaller-exact-c-0.05-100-1]",
"statsmodels/stats/tests/test_rates_poisson.py::test_confint_poisson_alternative[smaller-sqrt-cent-0.01-1000-0]",
"statsmodels/stats/tests/test_rates_poisson.py::test_confint_poisson_alternative[smaller-sqrt-cent-0.05-1-1]",
"statsmodels/stats/tests/test_rates_poisson.py::test_confint_poisson_alternative[smaller-score-0.05-1-1]",
"statsmodels/stats/tests/test_rates_poisson.py::test_confint_poisson_alternative[smaller-waldccv-0.1-1-10]",
"statsmodels/stats/tests/test_rates_poisson.py::test_confint_poisson_alternative[smaller-sqrt-centcc-0.05-1000-100]",
"statsmodels/stats/tests/test_rates_poisson.py::test_confint_poisson_alternative[smaller-sqrt-a-0.1-1-10]",
"statsmodels/stats/tests/test_rates_poisson.py::test_confint_poisson_alternative[smaller-sqrt-cent-0.05-1-100]",
"statsmodels/stats/tests/test_rates_poisson.py::test_confint_poisson_alternative[smaller-midp-c-0.1-1000-10]",
"statsmodels/stats/tests/test_rates_poisson.py::test_confint_poisson_alternative[smaller-midp-c-0.05-10-0]",
"statsmodels/stats/tests/test_rates_poisson.py::test_confint_poisson_alternative[larger-wald-0.01-1-1]",
"statsmodels/stats/tests/test_rates_poisson.py::test_confint_poisson_alternative[smaller-sqrt-v-0.1-1-1]",
"statsmodels/stats/tests/test_rates_poisson.py::test_confint_poisson_alternative[larger-jeff-0.1-10-100]",
"statsmodels/stats/tests/test_rates_poisson.py::test_confint_poisson_alternative[larger-midp-c-0.05-1-0]",
"statsmodels/stats/tests/test_rates_poisson.py::test_confint_poisson_alternative[larger-jeff-0.01-100-10]",
"statsmodels/stats/tests/test_rates_poisson.py::test_confint_poisson_alternative[larger-sqrt-centcc-0.01-10-100]",
"statsmodels/stats/tests/test_rates_poisson.py::test_confint_poisson_alternative[smaller-exact-c-0.1-1000-10]",
"statsmodels/stats/tests/test_rates_poisson.py::test_confint_poisson_alternative[larger-sqrt-centcc-0.1-10-1]",
"statsmodels/stats/tests/test_rates_poisson.py::test_confint_poisson_alternative[smaller-wald-0.01-1000-100]",
"statsmodels/stats/tests/test_rates_poisson.py::test_confint_poisson_alternative[smaller-wald-0.01-1000-0]",
"statsmodels/stats/tests/test_rates_poisson.py::test_confint_poisson_alternative[smaller-wald-0.1-1000-0]",
"statsmodels/stats/tests/test_rates_poisson.py::test_confint_poisson_alternative[larger-sqrt-cent-0.1-10-10]",
"statsmodels/stats/tests/test_rates_poisson.py::test_confint_poisson_alternative[larger-sqrt-v-0.05-1-100]",
"statsmodels/stats/tests/test_rates_poisson.py::test_confint_poisson_alternative[smaller-wald-0.1-1-100]",
"statsmodels/stats/tests/test_rates_poisson.py::test_confint_poisson_alternative[smaller-sqrt-a-0.05-100-0]",
"statsmodels/stats/tests/test_rates_poisson.py::test_confint_poisson_alternative[smaller-sqrt-cent-0.05-1-10]",
"statsmodels/stats/tests/test_rates_poisson.py::test_confint_poisson_alternative[larger-jeff-0.01-100-100]",
"statsmodels/stats/tests/test_rates_poisson.py::test_confint_poisson_alternative[smaller-sqrt-v-0.05-1-10]",
"statsmodels/stats/tests/test_rates_poisson.py::test_confint_poisson_alternative[larger-sqrt-0.05-100-1]",
"statsmodels/stats/tests/test_rates_poisson.py::test_confint_poisson_alternative[larger-jeff-0.1-10-0]",
"statsmodels/stats/tests/test_rates_poisson.py::test_confint_poisson_alternative[larger-sqrt-cent-0.01-1000-100]",
"statsmodels/stats/tests/test_rates_poisson.py::test_confint_poisson_alternative[smaller-sqrt-v-0.05-100-10]",
"statsmodels/stats/tests/test_rates_poisson.py::test_confint_poisson_alternative[smaller-sqrt-centcc-0.01-100-1]",
"statsmodels/stats/tests/test_rates_poisson.py::test_confint_poisson_alternative[larger-exact-c-0.01-1-1]",
"statsmodels/stats/tests/test_rates_poisson.py::test_confint_poisson_alternative[larger-waldccv-0.01-100-1]",
"statsmodels/stats/tests/test_rates_poisson.py::test_confint_poisson_alternative[smaller-exact-c-0.1-1000-0]",
"statsmodels/stats/tests/test_rates_poisson.py::test_confint_poisson_alternative[smaller-score-0.1-1000-1]",
"statsmodels/stats/tests/test_rates_poisson.py::test_confint_poisson_alternative[larger-midp-c-0.01-10-0]",
"statsmodels/stats/tests/test_rates_poisson.py::test_confint_poisson_alternative[larger-midp-c-0.1-100-0]",
"statsmodels/stats/tests/test_rates_poisson.py::test_confint_poisson_alternative[larger-sqrt-v-0.1-1-0]",
"statsmodels/stats/tests/test_rates_poisson.py::test_confint_poisson_alternative[larger-sqrt-cent-0.1-1000-100]",
"statsmodels/stats/tests/test_rates_poisson.py::test_confint_poisson_alternative[smaller-jeff-0.05-100-100]",
"statsmodels/stats/tests/test_rates_poisson.py::test_confint_poisson_alternative[smaller-sqrt-a-0.1-100-0]",
"statsmodels/stats/tests/test_rates_poisson.py::test_confint_poisson_alternative[smaller-wald-0.1-10-1]",
"statsmodels/stats/tests/test_rates_poisson.py::test_confint_poisson_alternative[smaller-waldccv-0.01-1-0]",
"statsmodels/stats/tests/test_rates_poisson.py::test_confint_poisson_alternative[smaller-sqrt-centcc-0.01-10-100]",
"statsmodels/stats/tests/test_rates_poisson.py::test_confint_poisson_alternative[larger-sqrt-cent-0.05-1000-10]",
"statsmodels/stats/tests/test_rates_poisson.py::test_confint_poisson_alternative[larger-sqrt-a-0.05-1-1]",
"statsmodels/stats/tests/test_rates_poisson.py::test_confint_poisson_alternative[smaller-jeff-0.05-100-10]",
"statsmodels/stats/tests/test_rates_poisson.py::test_confint_poisson_alternative[smaller-sqrt-cent-0.1-100-0]",
"statsmodels/stats/tests/test_rates_poisson.py::test_confint_poisson_alternative[smaller-sqrt-0.01-100-0]",
"statsmodels/stats/tests/test_rates_poisson.py::test_confint_poisson_alternative[smaller-sqrt-0.1-10-1]",
"statsmodels/stats/tests/test_rates_poisson.py::test_confint_poisson_alternative[smaller-sqrt-0.05-10-1]",
"statsmodels/stats/tests/test_rates_poisson.py::test_confint_poisson_alternative[smaller-sqrt-v-0.01-1-10]",
"statsmodels/stats/tests/test_rates_poisson.py::test_confint_poisson_alternative[smaller-exact-c-0.01-10-0]",
"statsmodels/stats/tests/test_rates_poisson.py::test_confint_poisson_alternative[smaller-sqrt-a-0.01-10-1]",
"statsmodels/stats/tests/test_rates_poisson.py::test_confint_poisson_alternative[larger-sqrt-v-0.1-100-10]",
"statsmodels/stats/tests/test_rates_poisson.py::test_confint_poisson_alternative[smaller-wald-0.01-1-100]",
"statsmodels/stats/tests/test_rates_poisson.py::test_confint_poisson_alternative[smaller-sqrt-centcc-0.1-100-10]",
"statsmodels/stats/tests/test_rates_poisson.py::test_confint_poisson_alternative[smaller-sqrt-0.1-1-100]",
"statsmodels/stats/tests/test_rates_poisson.py::test_confint_poisson_alternative[smaller-sqrt-a-0.05-10-1]",
"statsmodels/stats/tests/test_rates_poisson.py::test_confint_poisson_alternative[smaller-sqrt-cent-0.1-10-0]",
"statsmodels/stats/tests/test_rates_poisson.py::test_confint_poisson_alternative[larger-midp-c-0.1-10-100]",
"statsmodels/stats/tests/test_rates_poisson.py::test_confint_poisson_alternative[larger-wald-0.05-100-0]",
"statsmodels/stats/tests/test_rates_poisson.py::test_confint_poisson_alternative[larger-exact-c-0.1-10-10]",
"statsmodels/stats/tests/test_rates_poisson.py::test_confint_poisson_alternative[larger-waldccv-0.1-100-0]",
"statsmodels/stats/tests/test_rates_poisson.py::test_confint_poisson_alternative[larger-sqrt-0.1-10-10]",
"statsmodels/stats/tests/test_rates_poisson.py::test_confint_poisson_alternative[larger-waldccv-0.1-10-0]",
"statsmodels/stats/tests/test_rates_poisson.py::test_confint_poisson_alternative[smaller-wald-0.05-1000-100]",
"statsmodels/stats/tests/test_rates_poisson.py::test_confint_poisson_alternative[smaller-wald-0.01-1000-1]",
"statsmodels/stats/tests/test_rates_poisson.py::test_confint_poisson_alternative[smaller-sqrt-a-0.05-100-100]",
"statsmodels/stats/tests/test_rates_poisson.py::test_confint_poisson_alternative[smaller-sqrt-a-0.01-100-0]",
"statsmodels/stats/tests/test_rates_poisson.py::test_confint_poisson_alternative[smaller-sqrt-cent-0.05-100-1]",
"statsmodels/stats/tests/test_rates_poisson.py::test_confint_poisson_alternative[larger-exact-c-0.05-1-10]",
"statsmodels/stats/tests/test_rates_poisson.py::test_confint_poisson_alternative[larger-wald-0.05-1000-100]",
"statsmodels/stats/tests/test_rates_poisson.py::test_confint_poisson_alternative[smaller-sqrt-cent-0.01-100-0]",
"statsmodels/stats/tests/test_rates_poisson.py::test_confint_poisson_alternative[larger-waldccv-0.01-1-10]",
"statsmodels/stats/tests/test_rates_poisson.py::test_confint_poisson_alternative[smaller-sqrt-v-0.05-1000-1]",
"statsmodels/stats/tests/test_rates_poisson.py::test_confint_poisson_alternative[smaller-sqrt-a-0.05-100-1]",
"statsmodels/stats/tests/test_rates_poisson.py::test_confint_poisson_alternative[smaller-wald-0.05-1000-1]",
"statsmodels/stats/tests/test_rates_poisson.py::test_confint_poisson_alternative[larger-score-0.05-10-100]",
"statsmodels/stats/tests/test_rates_poisson.py::test_confint_poisson_alternative[smaller-wald-0.1-10-0]",
"statsmodels/stats/tests/test_rates_poisson.py::test_confint_poisson_alternative[smaller-exact-c-0.01-10-1]",
"statsmodels/stats/tests/test_rates_poisson.py::test_confint_poisson_alternative[smaller-waldccv-0.1-1000-100]",
"statsmodels/stats/tests/test_rates_poisson.py::test_confint_poisson_alternative[larger-sqrt-0.01-1000-0]",
"statsmodels/stats/tests/test_rates_poisson.py::test_confint_poisson_alternative[larger-sqrt-centcc-0.05-100-100]",
"statsmodels/stats/tests/test_rates_poisson.py::test_confint_poisson_alternative[larger-score-0.05-100-100]",
"statsmodels/stats/tests/test_rates_poisson.py::test_confint_poisson_alternative[larger-score-0.05-1-100]",
"statsmodels/stats/tests/test_rates_poisson.py::test_confint_poisson_alternative[smaller-waldccv-0.05-100-1]",
"statsmodels/stats/tests/test_rates_poisson.py::test_confint_poisson_alternative[smaller-sqrt-cent-0.1-1000-10]",
"statsmodels/stats/tests/test_rates_poisson.py::test_confint_poisson_alternative[larger-wald-0.05-1000-1]",
"statsmodels/stats/tests/test_rates_poisson.py::test_confint_poisson_alternative[larger-jeff-0.05-1-10]",
"statsmodels/stats/tests/test_rates_poisson.py::test_confint_poisson_alternative[larger-sqrt-centcc-0.01-10-10]",
"statsmodels/stats/tests/test_rates_poisson.py::test_confint_poisson_alternative[larger-exact-c-0.01-10-100]",
"statsmodels/stats/tests/test_rates_poisson.py::test_confint_poisson_alternative[larger-sqrt-a-0.05-10-100]",
"statsmodels/stats/tests/test_rates_poisson.py::test_confint_poisson_alternative[smaller-sqrt-a-0.1-1000-1]",
"statsmodels/stats/tests/test_rates_poisson.py::test_confint_poisson_alternative[larger-jeff-0.01-1-0]",
"statsmodels/stats/tests/test_rates_poisson.py::test_confint_poisson_alternative[smaller-exact-c-0.01-100-0]"
] | Python | [] | [] |
statsmodels/statsmodels | 9,280 | statsmodels__statsmodels-9280 | [
"9191"
] | 52edda81159e557dd845cc97a8a9324915c69792 | diff --git a/statsmodels/iolib/summary2.py b/statsmodels/iolib/summary2.py
--- a/statsmodels/iolib/summary2.py
+++ b/statsmodels/iolib/summary2.py
@@ -471,7 +471,8 @@ def _make_unique(list_of_names):
def summary_col(results, float_format='%.4f', model_names=(), stars=False,
info_dict=None, regressor_order=(), drop_omitted=False,
- include_r2=True):
+ include_r2=True, fixed_effects=None, fe_present='Yes',
+ fe_absent=''):
"""
Summarize multiple results instances side-by-side (coefs and SEs)
@@ -504,6 +505,14 @@ def summary_col(results, float_format='%.4f', model_names=(), stars=False,
If True, only regressors in regressor_order will be included.
include_r2 : bool, optional
Includes R2 and adjusted R2 in the summary table.
+ fixed_effects : list[str], optional
+ List of categorical variables for which to indicate presence of
+ fixed effects.
+ fe_present : str, optional
+ String to indicate the presence of fixed effects. Default is "Yes".
+ fe_absent : str, optional
+ String to indicate the absence of fixed effects. Default is empty
+ string.
"""
if not isinstance(results, list):
@@ -562,6 +571,19 @@ def merg(x, y):
idx.append(index[i + 1])
summ.index = idx
+ # add fixed effects info
+ if fixed_effects:
+ if not info_dict:
+ info_dict = {}
+
+ for fe in fixed_effects:
+ info_dict[fe + ' FE'] = (
+ lambda x, fe=fe, fe_present=fe_present, fe_absent=fe_absent:
+ fe_present
+ if any((f'C({fe})' in param) for param in x.params.index)
+ else fe_absent
+ )
+
# add infos about the models.
if info_dict:
cols = [_col_info(x, info_dict.get(x.model.__class__.__name__,
@@ -581,6 +603,21 @@ def merg(x, y):
summ = summ.fillna('')
+ # fixed effects processing
+ if fixed_effects:
+ index_series = pd.Series(summ.index, index=summ.index)
+ skip_flag = index_series.apply(
+ lambda x: any((f'C({fe})' in x) for fe in fixed_effects)
+ )
+ skip_next_flag = skip_flag.shift(fill_value=False)
+ final_skip = skip_flag | skip_next_flag
+ summ = summ[~final_skip]
+
+ r_squared_rows = summ.index[summ.index.str.contains('R-squared')]
+ r_squared_section = summ.loc[r_squared_rows]
+ summ = summ.drop(index=r_squared_rows)
+ summ = pd.concat([summ, r_squared_section])
+
smry = Summary()
smry._merge_latex = True
smry.add_df(summ, header=True, align='l')
| diff --git a/statsmodels/iolib/tests/test_summary2.py b/statsmodels/iolib/tests/test_summary2.py
--- a/statsmodels/iolib/tests/test_summary2.py
+++ b/statsmodels/iolib/tests/test_summary2.py
@@ -168,6 +168,72 @@ def test_OLSsummary(self):
result = string_to_find in actual
assert (result is True)
+ def test_summarycol_fixed_effects(self):
+ desired1 = r"""
+======================================
+ model_0 model_1 model_2
+--------------------------------------
+Intercept 1.35 1.32 1.48
+ (0.19) (0.42) (0.73)
+yrs_married -0.03 -0.02 -0.02
+ (0.00) (0.00) (0.00)
+educ -0.03 -0.02 -0.02
+ (0.01) (0.02) (0.02)
+occupation FE Yes Yes
+religious FE Yes Yes
+R-squared 0.01 0.02 0.03
+R-squared Adj. 0.01 0.02 0.02
+======================================
+Standard errors in parentheses.""" # noqa:W291
+
+ desired2 = r"""
+========================================
+ mod0 mod1 mod2
+----------------------------------------
+Intercept 1.35 1.32 1.48
+ (0.19) (0.42) (0.73)
+yrs_married -0.03 -0.02 -0.02
+ (0.00) (0.00) (0.00)
+educ -0.03 -0.02 -0.02
+ (0.01) (0.02) (0.02)
+C(religious)[T.2.0] -0.46 -0.86
+ (0.08) (0.87)
+C(religious)[T.3.0] -0.66 -0.71
+ (0.08) (1.13)
+C(religious)[T.4.0] -0.91 -0.92
+ (0.11) (1.03)
+occupation FE Yes Yes
+R-squared 0.01 0.02 0.03
+R-squared Adj. 0.01 0.02 0.02
+========================================
+Standard errors in parentheses.""" # noqa:W291
+
+ from statsmodels.datasets.fair import load_pandas
+ df_fair = load_pandas().data
+
+ res0 = OLS.from_formula("affairs ~ yrs_married + educ", df_fair).fit()
+ form1 = "affairs ~ yrs_married + educ + C(occupation) + C(religious)"
+ res1 = OLS.from_formula(form1, df_fair).fit()
+ form2 = "affairs ~ yrs_married + educ + C(occupation) : C(religious)"
+ res2 = OLS.from_formula(form2, df_fair).fit()
+
+ regressions = [res0, res1, res2]
+ summary1 = summary_col(
+ regressions,
+ model_names=[f'model_{i}' for i, _ in enumerate(regressions)],
+ fixed_effects=['occupation', "religious"],
+ float_format='%0.2f',)
+
+ assert_equal(summary1.as_text(), desired1)
+
+ summary2 = summary_col(
+ regressions,
+ model_names=[f'mod{i}' for i, _ in enumerate(regressions)],
+ fixed_effects=['occupation'],
+ float_format='%0.2f',)
+
+ assert_equal(summary2.as_text(), desired2)
+
def test_ols_summary_rsquared_label():
# Check that the "uncentered" label is correctly added after rsquared
| ENH: Add optional parameters for summary_col to indicate FEs
Researchers tend to show they used categorical variables without all the coefficients, saying whether they included a fixed effect. This PR adds that feature.
### Example
```python
import numpy as np
import pandas as pd
import seaborn as sns
import statsmodels.formula.api as smf
from statsmodels.iolib.summary2 import summary_col
# Load the diamonds dataset
diamonds = sns.load_dataset('diamonds')
# Adapted regressions for the diamonds dataset
regressions = [
(smf.ols('np.log(price) ~ carat', data=diamonds).fit(), 'log(Price) ~ Carat'),
(smf.ols('np.log(price) ~ np.log(carat)', data=diamonds).fit(), 'log(Price) ~ log(Carat)'),
(smf.ols('np.log(price) ~ C(cut)', data=diamonds).fit(), 'log(Price) ~ C(Cut)'),
(smf.ols('np.log(price) ~ C(clarity)', data=diamonds).fit(), 'log(Price) ~ C(Clarity)'),
(smf.ols('np.log(price) ~ carat + C(cut) + C(clarity)', data=diamonds).fit(), 'log(Price) ~ Carat + C(Cut) + C(Clarity)')
]
# Output summary of all regressions
summary = summary_col([reg[0] for reg in regressions],
model_names=[f'{i}. '+reg[1] for i, reg in enumerate(regressions, 1)]
)
summary
```
<img width="971" alt="Screenshot 2024-04-02 at 9 50 22 PM" src="https://github.com/statsmodels/statsmodels/assets/72167238/6b075616-c711-4fc2-a3fe-ccd0cf943ef2">
With more complicated regressions or broader categories, this can quickly overwhelm the summary.
Now, with amended function.
```
"""
fixed_effects : list[str], optional
List of categorical variables for which to indicate presence of fixed effects.
fe_present : str, optional
String to indicate the presence of fixed effects. Default is "Yes".
fe_absent : str, optional
String to indicate the absence of fixed effects. Default is empty string.
"""
summary = summary_col([reg[0] for reg in regressions],
model_names=[f'{i}. '+reg[1] for i, reg in enumerate(regressions, 1)],
fixed_effects=['cut', 'clarity'],
)
```
<img width="997" alt="Screenshot 2024-04-02 at 9 50 30 PM" src="https://github.com/statsmodels/statsmodels/assets/72167238/2ad0dec7-f678-418b-926f-de3708002966">
Much more concise.
| "2024-06-14T15:39:28Z" | 0.14 | [
"statsmodels/iolib/tests/test_summary2.py::TestSummaryLatex::test_summary_col_ordering_preserved",
"statsmodels/iolib/tests/test_summary2.py::TestSummaryLatex::test_summarycol",
"statsmodels/iolib/tests/test_summary2.py::TestSummaryLatex::test__repr_latex_",
"statsmodels/iolib/tests/test_summary2.py::TestSummaryLatex::test_OLSsummary",
"statsmodels/iolib/tests/test_summary2.py::TestSummaryLatex::test_summarycol_drop_omitted",
"statsmodels/iolib/tests/test_summary2.py::TestSummaryLatex::test_summarycol_float_format",
"statsmodels/iolib/tests/test_summary2.py::TestSummaryLabels::test_absence_of_r2",
"statsmodels/iolib/tests/test_summary2.py::TestSummaryLabels::test_summary_col_r2",
"statsmodels/iolib/tests/test_summary2.py::test_ols_summary_rsquared_label"
] | [
"statsmodels/iolib/tests/test_summary2.py::TestSummaryLatex::test_summarycol_fixed_effects"
] | Python | [] | [] |
|
redis/redis-py | 1,741 | redis__redis-py-1741 | [
"1254"
] | f82ab336c3e249ee871ee5e50e10c0de08c4f38a | diff --git a/redis/commands/core.py b/redis/commands/core.py
--- a/redis/commands/core.py
+++ b/redis/commands/core.py
@@ -637,6 +637,31 @@ def flushdb(self, asynchronous=False, **kwargs):
args.append(b"ASYNC")
return self.execute_command("FLUSHDB", *args, **kwargs)
+ def sync(self):
+ """
+ Initiates a replication stream from the master.
+
+ For more information check https://redis.io/commands/sync
+ """
+ from redis.client import NEVER_DECODE
+
+ options = {}
+ options[NEVER_DECODE] = []
+ return self.execute_command("SYNC", **options)
+
+ def psync(self, replicationid, offset):
+ """
+ Initiates a replication stream from the master.
+ Newer version for `sync`.
+
+ For more information check https://redis.io/commands/sync
+ """
+ from redis.client import NEVER_DECODE
+
+ options = {}
+ options[NEVER_DECODE] = []
+ return self.execute_command("PSYNC", replicationid, offset, **options)
+
def swapdb(self, first, second, **kwargs):
"""
Swap two databases
diff --git a/redis/connection.py b/redis/connection.py
--- a/redis/connection.py
+++ b/redis/connection.py
@@ -382,7 +382,7 @@ def __del__(self):
except Exception:
pass
- def on_connect(self, connection):
+ def on_connect(self, connection, **kwargs):
self._sock = connection._sock
self._socket_timeout = connection.socket_timeout
kwargs = {
| diff --git a/tests/test_commands.py b/tests/test_commands.py
--- a/tests/test_commands.py
+++ b/tests/test_commands.py
@@ -4151,6 +4151,18 @@ def test_replicaof(self, r):
assert r.replicaof("NO ONE")
assert r.replicaof("NO", "ONE")
+ @skip_if_server_version_lt("2.8.0")
+ def test_sync(self, r):
+ r2 = redis.Redis(port=6380, decode_responses=False)
+ res = r2.sync()
+ assert b"REDIS" in res
+
+ @skip_if_server_version_lt("2.8.0")
+ def test_psync(self, r):
+ r2 = redis.Redis(port=6380, decode_responses=False)
+ res = r2.psync(r2.client_id(), 1)
+ assert b"FULLRESYNC" in res
+
@pytest.mark.onlynoncluster
class TestBinarySave:
| running dump() fails when decode_responses=True
redis-py 3.3.6
python 3.6.9
Ubuntu 18.04
redis 4.0.9
I have a `Redis` instance that I created with `decode_responses=True`. When I run `.dump(key)` the decoder fails to decode the bytes response, which makes sense, because it isn't unicode.
```
File "/home/brian/.local/lib/python3.6/site-packages/redis/client.py", line 3677, in execute
return execute(conn, stack, raise_on_error)
File "/home/brian/.local/lib/python3.6/site-packages/redis/client.py", line 3570, in _execute_transaction
response = self.parse_response(connection, '_')
File "/home/brian/.local/lib/python3.6/site-packages/redis/client.py", line 3636, in parse_response
self, connection, command_name, **options)
File "/home/brian/.local/lib/python3.6/site-packages/redis/client.py", line 853, in parse_response
response = connection.read_response()
File "/home/brian/.local/lib/python3.6/site-packages/redis/connection.py", line 687, in read_response
response = self._parser.read_response()
File "/home/brian/.local/lib/python3.6/site-packages/redis/connection.py", line 442, in read_response
response = self._reader.gets()
UnicodeDecodeError: 'utf-8' codec can't decode byte 0xc3 in position 1: invalid continuation byte
```
| Hello,
I have an issue too on decode_responses. UTF8 decoding for sentinel part seems not working :
```
self.master = self.sentinel.master_for(redis_sentinels_master, socket_timeout=socket_db_timeout, db=db, encoding=u'utf-8', decode_responses=True)
```
```
print(self.master.get(myKey))
b'xyz'
```
I don't know why, but this seems to have broken for me as well. | "2021-11-23T09:17:35Z" | 4.1 | [
"tests/test_commands.py::TestResponseCallbacks::test_response_callbacks",
"tests/test_commands.py::TestResponseCallbacks::test_case_insensitive_command_names",
"tests/test_commands.py::TestRedisCommands::test_command_on_invalid_key_type",
"tests/test_commands.py::TestRedisCommands::test_acl_cat_no_category",
"tests/test_commands.py::TestRedisCommands::test_acl_cat_with_category",
"tests/test_commands.py::TestRedisCommands::test_acl_deluser",
"tests/test_commands.py::TestRedisCommands::test_acl_genpass",
"tests/test_commands.py::TestRedisCommands::test_acl_help",
"tests/test_commands.py::TestRedisCommands::test_acl_list",
"tests/test_commands.py::TestRedisCommands::test_acl_log",
"tests/test_commands.py::TestRedisCommands::test_acl_setuser_categories_without_prefix_fails",
"tests/test_commands.py::TestRedisCommands::test_acl_setuser_commands_without_prefix_fails",
"tests/test_commands.py::TestRedisCommands::test_acl_setuser_add_passwords_and_nopass_fails",
"tests/test_commands.py::TestRedisCommands::test_acl_users",
"tests/test_commands.py::TestRedisCommands::test_acl_whoami",
"tests/test_commands.py::TestRedisCommands::test_client_list",
"tests/test_commands.py::TestRedisCommands::test_client_info",
"tests/test_commands.py::TestRedisCommands::test_client_list_types_not_replica",
"tests/test_commands.py::TestRedisCommands::test_client_list_replica",
"tests/test_commands.py::TestRedisCommands::test_client_list_client_id",
"tests/test_commands.py::TestRedisCommands::test_client_id",
"tests/test_commands.py::TestRedisCommands::test_client_trackinginfo",
"tests/test_commands.py::TestRedisCommands::test_client_unblock",
"tests/test_commands.py::TestRedisCommands::test_client_getname",
"tests/test_commands.py::TestRedisCommands::test_client_setname",
"tests/test_commands.py::TestRedisCommands::test_client_kill",
"tests/test_commands.py::TestRedisCommands::test_client_kill_filter_invalid_params",
"tests/test_commands.py::TestRedisCommands::test_client_kill_filter_by_id",
"tests/test_commands.py::TestRedisCommands::test_client_kill_filter_by_addr",
"tests/test_commands.py::TestRedisCommands::test_client_list_after_client_setname",
"tests/test_commands.py::TestRedisCommands::test_client_kill_filter_by_laddr",
"tests/test_commands.py::TestRedisCommands::test_client_kill_filter_by_user",
"tests/test_commands.py::TestRedisCommands::test_client_pause",
"tests/test_commands.py::TestRedisCommands::test_client_unpause",
"tests/test_commands.py::TestRedisCommands::test_client_reply",
"tests/test_commands.py::TestRedisCommands::test_client_getredir",
"tests/test_commands.py::TestRedisCommands::test_config_get",
"tests/test_commands.py::TestRedisCommands::test_config_resetstat",
"tests/test_commands.py::TestRedisCommands::test_config_set",
"tests/test_commands.py::TestRedisCommands::test_dbsize",
"tests/test_commands.py::TestRedisCommands::test_echo",
"tests/test_commands.py::TestRedisCommands::test_info",
"tests/test_commands.py::TestRedisCommands::test_lastsave",
"tests/test_commands.py::TestRedisCommands::test_lolwut",
"tests/test_commands.py::TestRedisCommands::test_object",
"tests/test_commands.py::TestRedisCommands::test_ping",
"tests/test_commands.py::TestRedisCommands::test_quit",
"tests/test_commands.py::TestRedisCommands::test_role",
"tests/test_commands.py::TestRedisCommands::test_slowlog_get",
"tests/test_commands.py::TestRedisCommands::test_slowlog_get_limit",
"tests/test_commands.py::TestRedisCommands::test_slowlog_length",
"tests/test_commands.py::TestRedisCommands::test_time",
"tests/test_commands.py::TestRedisCommands::test_bgsave",
"tests/test_commands.py::TestRedisCommands::test_append",
"tests/test_commands.py::TestRedisCommands::test_bitcount",
"tests/test_commands.py::TestRedisCommands::test_bitop_not_empty_string",
"tests/test_commands.py::TestRedisCommands::test_bitop_not",
"tests/test_commands.py::TestRedisCommands::test_bitop_not_in_place",
"tests/test_commands.py::TestRedisCommands::test_bitop_single_string",
"tests/test_commands.py::TestRedisCommands::test_bitop_string_operands",
"tests/test_commands.py::TestRedisCommands::test_bitpos",
"tests/test_commands.py::TestRedisCommands::test_bitpos_wrong_arguments",
"tests/test_commands.py::TestRedisCommands::test_copy",
"tests/test_commands.py::TestRedisCommands::test_copy_and_replace",
"tests/test_commands.py::TestRedisCommands::test_copy_to_another_database",
"tests/test_commands.py::TestRedisCommands::test_decr",
"tests/test_commands.py::TestRedisCommands::test_decrby",
"tests/test_commands.py::TestRedisCommands::test_delete",
"tests/test_commands.py::TestRedisCommands::test_delete_with_multiple_keys",
"tests/test_commands.py::TestRedisCommands::test_delitem",
"tests/test_commands.py::TestRedisCommands::test_unlink",
"tests/test_commands.py::TestRedisCommands::test_unlink_with_multiple_keys",
"tests/test_commands.py::TestRedisCommands::test_dump_and_restore",
"tests/test_commands.py::TestRedisCommands::test_dump_and_restore_and_replace",
"tests/test_commands.py::TestRedisCommands::test_dump_and_restore_absttl",
"tests/test_commands.py::TestRedisCommands::test_exists",
"tests/test_commands.py::TestRedisCommands::test_exists_contains",
"tests/test_commands.py::TestRedisCommands::test_expire",
"tests/test_commands.py::TestRedisCommands::test_expireat_datetime",
"tests/test_commands.py::TestRedisCommands::test_expireat_no_key",
"tests/test_commands.py::TestRedisCommands::test_expireat_unixtime",
"tests/test_commands.py::TestRedisCommands::test_get_and_set",
"tests/test_commands.py::TestRedisCommands::test_getdel",
"tests/test_commands.py::TestRedisCommands::test_getex",
"tests/test_commands.py::TestRedisCommands::test_getitem_and_setitem",
"tests/test_commands.py::TestRedisCommands::test_getitem_raises_keyerror_for_missing_key",
"tests/test_commands.py::TestRedisCommands::test_getitem_does_not_raise_keyerror_for_empty_string",
"tests/test_commands.py::TestRedisCommands::test_get_set_bit",
"tests/test_commands.py::TestRedisCommands::test_getrange",
"tests/test_commands.py::TestRedisCommands::test_getset",
"tests/test_commands.py::TestRedisCommands::test_incr",
"tests/test_commands.py::TestRedisCommands::test_incrby",
"tests/test_commands.py::TestRedisCommands::test_incrbyfloat",
"tests/test_commands.py::TestRedisCommands::test_keys",
"tests/test_commands.py::TestRedisCommands::test_mget",
"tests/test_commands.py::TestRedisCommands::test_lmove",
"tests/test_commands.py::TestRedisCommands::test_blmove",
"tests/test_commands.py::TestRedisCommands::test_mset",
"tests/test_commands.py::TestRedisCommands::test_msetnx",
"tests/test_commands.py::TestRedisCommands::test_pexpire",
"tests/test_commands.py::TestRedisCommands::test_pexpireat_datetime",
"tests/test_commands.py::TestRedisCommands::test_pexpireat_no_key",
"tests/test_commands.py::TestRedisCommands::test_pexpireat_unixtime",
"tests/test_commands.py::TestRedisCommands::test_psetex",
"tests/test_commands.py::TestRedisCommands::test_psetex_timedelta",
"tests/test_commands.py::TestRedisCommands::test_pttl",
"tests/test_commands.py::TestRedisCommands::test_pttl_no_key",
"tests/test_commands.py::TestRedisCommands::test_hrandfield",
"tests/test_commands.py::TestRedisCommands::test_randomkey",
"tests/test_commands.py::TestRedisCommands::test_rename",
"tests/test_commands.py::TestRedisCommands::test_renamenx",
"tests/test_commands.py::TestRedisCommands::test_set_nx",
"tests/test_commands.py::TestRedisCommands::test_set_xx",
"tests/test_commands.py::TestRedisCommands::test_set_px",
"tests/test_commands.py::TestRedisCommands::test_set_px_timedelta",
"tests/test_commands.py::TestRedisCommands::test_set_ex",
"tests/test_commands.py::TestRedisCommands::test_set_ex_timedelta",
"tests/test_commands.py::TestRedisCommands::test_set_exat_timedelta",
"tests/test_commands.py::TestRedisCommands::test_set_pxat_timedelta",
"tests/test_commands.py::TestRedisCommands::test_set_multipleoptions",
"tests/test_commands.py::TestRedisCommands::test_set_keepttl",
"tests/test_commands.py::TestRedisCommands::test_set_get",
"tests/test_commands.py::TestRedisCommands::test_setex",
"tests/test_commands.py::TestRedisCommands::test_setnx",
"tests/test_commands.py::TestRedisCommands::test_setrange",
"tests/test_commands.py::TestRedisCommands::test_stralgo_negative",
"tests/test_commands.py::TestRedisCommands::test_strlen",
"tests/test_commands.py::TestRedisCommands::test_substr",
"tests/test_commands.py::TestRedisCommands::test_ttl",
"tests/test_commands.py::TestRedisCommands::test_ttl_nokey",
"tests/test_commands.py::TestRedisCommands::test_type",
"tests/test_commands.py::TestRedisCommands::test_blpop",
"tests/test_commands.py::TestRedisCommands::test_brpop",
"tests/test_commands.py::TestRedisCommands::test_brpoplpush",
"tests/test_commands.py::TestRedisCommands::test_brpoplpush_empty_string",
"tests/test_commands.py::TestRedisCommands::test_lindex",
"tests/test_commands.py::TestRedisCommands::test_linsert",
"tests/test_commands.py::TestRedisCommands::test_llen",
"tests/test_commands.py::TestRedisCommands::test_lpop",
"tests/test_commands.py::TestRedisCommands::test_lpop_count",
"tests/test_commands.py::TestRedisCommands::test_lpush",
"tests/test_commands.py::TestRedisCommands::test_lpushx",
"tests/test_commands.py::TestRedisCommands::test_lpushx_with_list",
"tests/test_commands.py::TestRedisCommands::test_lrange",
"tests/test_commands.py::TestRedisCommands::test_lrem",
"tests/test_commands.py::TestRedisCommands::test_lset",
"tests/test_commands.py::TestRedisCommands::test_ltrim",
"tests/test_commands.py::TestRedisCommands::test_rpop",
"tests/test_commands.py::TestRedisCommands::test_rpop_count",
"tests/test_commands.py::TestRedisCommands::test_rpoplpush",
"tests/test_commands.py::TestRedisCommands::test_rpush",
"tests/test_commands.py::TestRedisCommands::test_lpos",
"tests/test_commands.py::TestRedisCommands::test_rpushx",
"tests/test_commands.py::TestRedisCommands::test_scan",
"tests/test_commands.py::TestRedisCommands::test_scan_type",
"tests/test_commands.py::TestRedisCommands::test_scan_iter",
"tests/test_commands.py::TestRedisCommands::test_sscan",
"tests/test_commands.py::TestRedisCommands::test_sscan_iter",
"tests/test_commands.py::TestRedisCommands::test_hscan",
"tests/test_commands.py::TestRedisCommands::test_hscan_iter",
"tests/test_commands.py::TestRedisCommands::test_zscan",
"tests/test_commands.py::TestRedisCommands::test_zscan_iter",
"tests/test_commands.py::TestRedisCommands::test_sadd",
"tests/test_commands.py::TestRedisCommands::test_scard",
"tests/test_commands.py::TestRedisCommands::test_sdiff",
"tests/test_commands.py::TestRedisCommands::test_sdiffstore",
"tests/test_commands.py::TestRedisCommands::test_sinter",
"tests/test_commands.py::TestRedisCommands::test_sinterstore",
"tests/test_commands.py::TestRedisCommands::test_sismember",
"tests/test_commands.py::TestRedisCommands::test_smembers",
"tests/test_commands.py::TestRedisCommands::test_smismember",
"tests/test_commands.py::TestRedisCommands::test_smove",
"tests/test_commands.py::TestRedisCommands::test_spop",
"tests/test_commands.py::TestRedisCommands::test_spop_multi_value",
"tests/test_commands.py::TestRedisCommands::test_srandmember",
"tests/test_commands.py::TestRedisCommands::test_srandmember_multi_value",
"tests/test_commands.py::TestRedisCommands::test_srem",
"tests/test_commands.py::TestRedisCommands::test_sunion",
"tests/test_commands.py::TestRedisCommands::test_sunionstore",
"tests/test_commands.py::TestRedisCommands::test_debug_segfault",
"tests/test_commands.py::TestRedisCommands::test_script_debug",
"tests/test_commands.py::TestRedisCommands::test_zadd",
"tests/test_commands.py::TestRedisCommands::test_zadd_nx",
"tests/test_commands.py::TestRedisCommands::test_zadd_xx",
"tests/test_commands.py::TestRedisCommands::test_zadd_ch",
"tests/test_commands.py::TestRedisCommands::test_zadd_incr",
"tests/test_commands.py::TestRedisCommands::test_zadd_incr_with_xx",
"tests/test_commands.py::TestRedisCommands::test_zadd_gt_lt",
"tests/test_commands.py::TestRedisCommands::test_zcard",
"tests/test_commands.py::TestRedisCommands::test_zcount",
"tests/test_commands.py::TestRedisCommands::test_zdiff",
"tests/test_commands.py::TestRedisCommands::test_zdiffstore",
"tests/test_commands.py::TestRedisCommands::test_zincrby",
"tests/test_commands.py::TestRedisCommands::test_zlexcount",
"tests/test_commands.py::TestRedisCommands::test_zinter",
"tests/test_commands.py::TestRedisCommands::test_zinterstore_sum",
"tests/test_commands.py::TestRedisCommands::test_zinterstore_max",
"tests/test_commands.py::TestRedisCommands::test_zinterstore_min",
"tests/test_commands.py::TestRedisCommands::test_zinterstore_with_weight",
"tests/test_commands.py::TestRedisCommands::test_zpopmax",
"tests/test_commands.py::TestRedisCommands::test_zpopmin",
"tests/test_commands.py::TestRedisCommands::test_zrandemember",
"tests/test_commands.py::TestRedisCommands::test_bzpopmax",
"tests/test_commands.py::TestRedisCommands::test_bzpopmin",
"tests/test_commands.py::TestRedisCommands::test_zrange",
"tests/test_commands.py::TestRedisCommands::test_zrange_errors",
"tests/test_commands.py::TestRedisCommands::test_zrange_params",
"tests/test_commands.py::TestRedisCommands::test_zrangestore",
"tests/test_commands.py::TestRedisCommands::test_zrangebylex",
"tests/test_commands.py::TestRedisCommands::test_zrevrangebylex",
"tests/test_commands.py::TestRedisCommands::test_zrangebyscore",
"tests/test_commands.py::TestRedisCommands::test_zrank",
"tests/test_commands.py::TestRedisCommands::test_zrem",
"tests/test_commands.py::TestRedisCommands::test_zrem_multiple_keys",
"tests/test_commands.py::TestRedisCommands::test_zremrangebylex",
"tests/test_commands.py::TestRedisCommands::test_zremrangebyrank",
"tests/test_commands.py::TestRedisCommands::test_zremrangebyscore",
"tests/test_commands.py::TestRedisCommands::test_zrevrange",
"tests/test_commands.py::TestRedisCommands::test_zrevrangebyscore",
"tests/test_commands.py::TestRedisCommands::test_zrevrank",
"tests/test_commands.py::TestRedisCommands::test_zscore",
"tests/test_commands.py::TestRedisCommands::test_zunion",
"tests/test_commands.py::TestRedisCommands::test_zunionstore_sum",
"tests/test_commands.py::TestRedisCommands::test_zunionstore_max",
"tests/test_commands.py::TestRedisCommands::test_zunionstore_min",
"tests/test_commands.py::TestRedisCommands::test_zunionstore_with_weight",
"tests/test_commands.py::TestRedisCommands::test_zmscore",
"tests/test_commands.py::TestRedisCommands::test_pfadd",
"tests/test_commands.py::TestRedisCommands::test_pfcount",
"tests/test_commands.py::TestRedisCommands::test_pfmerge",
"tests/test_commands.py::TestRedisCommands::test_hget_and_hset",
"tests/test_commands.py::TestRedisCommands::test_hset_with_multi_key_values",
"tests/test_commands.py::TestRedisCommands::test_hset_without_data",
"tests/test_commands.py::TestRedisCommands::test_hdel",
"tests/test_commands.py::TestRedisCommands::test_hexists",
"tests/test_commands.py::TestRedisCommands::test_hgetall",
"tests/test_commands.py::TestRedisCommands::test_hincrby",
"tests/test_commands.py::TestRedisCommands::test_hincrbyfloat",
"tests/test_commands.py::TestRedisCommands::test_hkeys",
"tests/test_commands.py::TestRedisCommands::test_hlen",
"tests/test_commands.py::TestRedisCommands::test_hmget",
"tests/test_commands.py::TestRedisCommands::test_hmset",
"tests/test_commands.py::TestRedisCommands::test_hsetnx",
"tests/test_commands.py::TestRedisCommands::test_hvals",
"tests/test_commands.py::TestRedisCommands::test_hstrlen",
"tests/test_commands.py::TestRedisCommands::test_sort_basic",
"tests/test_commands.py::TestRedisCommands::test_sort_limited",
"tests/test_commands.py::TestRedisCommands::test_sort_by",
"tests/test_commands.py::TestRedisCommands::test_sort_get",
"tests/test_commands.py::TestRedisCommands::test_sort_get_multi",
"tests/test_commands.py::TestRedisCommands::test_sort_get_groups_two",
"tests/test_commands.py::TestRedisCommands::test_sort_groups_string_get",
"tests/test_commands.py::TestRedisCommands::test_sort_groups_just_one_get",
"tests/test_commands.py::TestRedisCommands::test_sort_groups_no_get",
"tests/test_commands.py::TestRedisCommands::test_sort_groups_three_gets",
"tests/test_commands.py::TestRedisCommands::test_sort_desc",
"tests/test_commands.py::TestRedisCommands::test_sort_alpha",
"tests/test_commands.py::TestRedisCommands::test_sort_store",
"tests/test_commands.py::TestRedisCommands::test_sort_all_options",
"tests/test_commands.py::TestRedisCommands::test_sort_issue_924",
"tests/test_commands.py::TestRedisCommands::test_cluster_addslots",
"tests/test_commands.py::TestRedisCommands::test_cluster_count_failure_reports",
"tests/test_commands.py::TestRedisCommands::test_cluster_countkeysinslot",
"tests/test_commands.py::TestRedisCommands::test_cluster_delslots",
"tests/test_commands.py::TestRedisCommands::test_cluster_failover",
"tests/test_commands.py::TestRedisCommands::test_cluster_forget",
"tests/test_commands.py::TestRedisCommands::test_cluster_info",
"tests/test_commands.py::TestRedisCommands::test_cluster_keyslot",
"tests/test_commands.py::TestRedisCommands::test_cluster_meet",
"tests/test_commands.py::TestRedisCommands::test_cluster_nodes",
"tests/test_commands.py::TestRedisCommands::test_cluster_replicate",
"tests/test_commands.py::TestRedisCommands::test_cluster_reset",
"tests/test_commands.py::TestRedisCommands::test_cluster_saveconfig",
"tests/test_commands.py::TestRedisCommands::test_cluster_setslot",
"tests/test_commands.py::TestRedisCommands::test_cluster_slaves",
"tests/test_commands.py::TestRedisCommands::test_readonly_invalid_cluster_state",
"tests/test_commands.py::TestRedisCommands::test_readonly",
"tests/test_commands.py::TestRedisCommands::test_geoadd",
"tests/test_commands.py::TestRedisCommands::test_geoadd_nx",
"tests/test_commands.py::TestRedisCommands::test_geoadd_xx",
"tests/test_commands.py::TestRedisCommands::test_geoadd_ch",
"tests/test_commands.py::TestRedisCommands::test_geoadd_invalid_params",
"tests/test_commands.py::TestRedisCommands::test_geodist",
"tests/test_commands.py::TestRedisCommands::test_geodist_units",
"tests/test_commands.py::TestRedisCommands::test_geodist_missing_one_member",
"tests/test_commands.py::TestRedisCommands::test_geodist_invalid_units",
"tests/test_commands.py::TestRedisCommands::test_geohash",
"tests/test_commands.py::TestRedisCommands::test_geopos",
"tests/test_commands.py::TestRedisCommands::test_geopos_no_value",
"tests/test_commands.py::TestRedisCommands::test_geosearch",
"tests/test_commands.py::TestRedisCommands::test_geosearch_member",
"tests/test_commands.py::TestRedisCommands::test_geosearch_sort",
"tests/test_commands.py::TestRedisCommands::test_geosearch_with",
"tests/test_commands.py::TestRedisCommands::test_geosearch_negative",
"tests/test_commands.py::TestRedisCommands::test_geosearchstore",
"tests/test_commands.py::TestRedisCommands::test_geosearchstore_dist",
"tests/test_commands.py::TestRedisCommands::test_georadius",
"tests/test_commands.py::TestRedisCommands::test_georadius_no_values",
"tests/test_commands.py::TestRedisCommands::test_georadius_units",
"tests/test_commands.py::TestRedisCommands::test_georadius_with",
"tests/test_commands.py::TestRedisCommands::test_georadius_count",
"tests/test_commands.py::TestRedisCommands::test_georadius_sort",
"tests/test_commands.py::TestRedisCommands::test_georadius_store",
"tests/test_commands.py::TestRedisCommands::test_georadius_store_dist",
"tests/test_commands.py::TestRedisCommands::test_georadiusmember",
"tests/test_commands.py::TestRedisCommands::test_georadiusmember_count",
"tests/test_commands.py::TestRedisCommands::test_xack",
"tests/test_commands.py::TestRedisCommands::test_xadd",
"tests/test_commands.py::TestRedisCommands::test_xadd_nomkstream",
"tests/test_commands.py::TestRedisCommands::test_xadd_minlen_and_limit",
"tests/test_commands.py::TestRedisCommands::test_xautoclaim",
"tests/test_commands.py::TestRedisCommands::test_xautoclaim_negative",
"tests/test_commands.py::TestRedisCommands::test_xclaim",
"tests/test_commands.py::TestRedisCommands::test_xdel",
"tests/test_commands.py::TestRedisCommands::test_xgroup_delconsumer",
"tests/test_commands.py::TestRedisCommands::test_xgroup_createconsumer",
"tests/test_commands.py::TestRedisCommands::test_xgroup_destroy",
"tests/test_commands.py::TestRedisCommands::test_xinfo_stream",
"tests/test_commands.py::TestRedisCommands::test_xinfo_stream_full",
"tests/test_commands.py::TestRedisCommands::test_xlen",
"tests/test_commands.py::TestRedisCommands::test_xpending",
"tests/test_commands.py::TestRedisCommands::test_xpending_range",
"tests/test_commands.py::TestRedisCommands::test_xpending_range_idle",
"tests/test_commands.py::TestRedisCommands::test_xpending_range_negative",
"tests/test_commands.py::TestRedisCommands::test_xrange",
"tests/test_commands.py::TestRedisCommands::test_xread",
"tests/test_commands.py::TestRedisCommands::test_xreadgroup",
"tests/test_commands.py::TestRedisCommands::test_xrevrange",
"tests/test_commands.py::TestRedisCommands::test_xtrim",
"tests/test_commands.py::TestRedisCommands::test_xtrim_minlen_and_length_args",
"tests/test_commands.py::TestRedisCommands::test_bitfield_operations",
"tests/test_commands.py::TestRedisCommands::test_memory_help",
"tests/test_commands.py::TestRedisCommands::test_memory_doctor",
"tests/test_commands.py::TestRedisCommands::test_memory_malloc_stats",
"tests/test_commands.py::TestRedisCommands::test_memory_stats",
"tests/test_commands.py::TestRedisCommands::test_memory_usage",
"tests/test_commands.py::TestRedisCommands::test_module_list",
"tests/test_commands.py::TestRedisCommands::test_command_count",
"tests/test_commands.py::TestRedisCommands::test_command_getkeys",
"tests/test_commands.py::TestRedisCommands::test_command",
"tests/test_commands.py::TestRedisCommands::test_module",
"tests/test_commands.py::TestRedisCommands::test_restore",
"tests/test_commands.py::TestRedisCommands::test_restore_idletime",
"tests/test_commands.py::TestRedisCommands::test_restore_frequency",
"tests/test_commands.py::TestRedisCommands::test_replicaof",
"tests/test_commands.py::TestBinarySave::test_binary_get_set",
"tests/test_commands.py::TestBinarySave::test_binary_lists",
"tests/test_commands.py::TestBinarySave::test_22_info",
"tests/test_commands.py::TestBinarySave::test_large_responses",
"tests/test_commands.py::TestBinarySave::test_floating_point_encoding"
] | [
"tests/test_commands.py::TestRedisCommands::test_sync",
"tests/test_commands.py::TestRedisCommands::test_psync"
] | Python | [] | [] |
redis/redis-py | 1,817 | redis__redis-py-1817 | [
"1764"
] | 18c6809b761bc6755349e1d7e08e74e857ec2c65 | diff --git a/redis/client.py b/redis/client.py
--- a/redis/client.py
+++ b/redis/client.py
@@ -868,6 +868,7 @@ def __init__(
errors=None,
decode_responses=False,
retry_on_timeout=False,
+ retry_on_error=[],
ssl=False,
ssl_keyfile=None,
ssl_certfile=None,
@@ -886,8 +887,10 @@ def __init__(
):
"""
Initialize a new Redis client.
- To specify a retry policy, first set `retry_on_timeout` to `True`
- then set `retry` to a valid `Retry` object
+ To specify a retry policy for specific errors, first set
+ `retry_on_error` to a list of the error/s to retry on, then set
+ `retry` to a valid `Retry` object.
+ To retry on TimeoutError, `retry_on_timeout` can also be set to `True`.
"""
if not connection_pool:
if charset is not None:
@@ -904,7 +907,8 @@ def __init__(
)
)
encoding_errors = errors
-
+ if retry_on_timeout is True:
+ retry_on_error.append(TimeoutError)
kwargs = {
"db": db,
"username": username,
@@ -913,7 +917,7 @@ def __init__(
"encoding": encoding,
"encoding_errors": encoding_errors,
"decode_responses": decode_responses,
- "retry_on_timeout": retry_on_timeout,
+ "retry_on_error": retry_on_error,
"retry": copy.deepcopy(retry),
"max_connections": max_connections,
"health_check_interval": health_check_interval,
@@ -1144,11 +1148,14 @@ def _send_command_parse_response(self, conn, command_name, *args, **options):
def _disconnect_raise(self, conn, error):
"""
Close the connection and raise an exception
- if retry_on_timeout is not set or the error
- is not a TimeoutError
+ if retry_on_error is not set or the error
+ is not one of the specified error types
"""
conn.disconnect()
- if not (conn.retry_on_timeout and isinstance(error, TimeoutError)):
+ if (
+ conn.retry_on_error is None
+ or isinstance(error, tuple(conn.retry_on_error)) is False
+ ):
raise error
# COMMAND EXECUTION AND PROTOCOL PARSING
diff --git a/redis/cluster.py b/redis/cluster.py
--- a/redis/cluster.py
+++ b/redis/cluster.py
@@ -996,9 +996,11 @@ def _execute_command(self, target_node, *args, **kwargs):
self.reinitialize_counter += 1
if self._should_reinitialized():
self.nodes_manager.initialize()
+ # Reset the counter
+ self.reinitialize_counter = 0
else:
self.nodes_manager.update_moved_exception(e)
- moved = True
+ moved = True
except TryAgainError:
log.exception("TryAgainError")
@@ -1320,6 +1322,7 @@ def initialize(self):
tmp_slots = {}
disagreements = []
startup_nodes_reachable = False
+ fully_covered = False
kwargs = self.connection_kwargs
for startup_node in self.startup_nodes.values():
try:
@@ -1431,6 +1434,12 @@ def initialize(self):
f'slots cache: {", ".join(disagreements)}'
)
+ fully_covered = self.check_slots_coverage(tmp_slots)
+ if fully_covered:
+ # Don't need to continue to the next startup node if all
+ # slots are covered
+ break
+
if not startup_nodes_reachable:
raise RedisClusterException(
"Redis Cluster cannot be connected. Please provide at least "
@@ -1440,7 +1449,6 @@ def initialize(self):
# Create Redis connections to all nodes
self.create_redis_connections(list(tmp_nodes_cache.values()))
- fully_covered = self.check_slots_coverage(tmp_slots)
# Check if the slots are not fully covered
if not fully_covered and self._require_full_coverage:
# Despite the requirement that the slots be covered, there
@@ -1478,6 +1486,8 @@ def initialize(self):
self.default_node = self.get_nodes_by_server_type(PRIMARY)[0]
# Populate the startup nodes with all discovered nodes
self.populate_startup_nodes(self.nodes_cache.values())
+ # If initialize was called after a MovedError, clear it
+ self._moved_exception = None
def close(self):
self.default_node = None
diff --git a/redis/connection.py b/redis/connection.py
--- a/redis/connection.py
+++ b/redis/connection.py
@@ -513,6 +513,7 @@ def __init__(
socket_keepalive_options=None,
socket_type=0,
retry_on_timeout=False,
+ retry_on_error=[],
encoding="utf-8",
encoding_errors="strict",
decode_responses=False,
@@ -526,8 +527,10 @@ def __init__(
):
"""
Initialize a new Connection.
- To specify a retry policy, first set `retry_on_timeout` to `True`
- then set `retry` to a valid `Retry` object
+ To specify a retry policy for specific errors, first set
+ `retry_on_error` to a list of the error/s to retry on, then set
+ `retry` to a valid `Retry` object.
+ To retry on TimeoutError, `retry_on_timeout` can also be set to `True`.
"""
self.pid = os.getpid()
self.host = host
@@ -543,11 +546,17 @@ def __init__(
self.socket_type = socket_type
self.retry_on_timeout = retry_on_timeout
if retry_on_timeout:
+ # Add TimeoutError to the errors list to retry on
+ retry_on_error.append(TimeoutError)
+ self.retry_on_error = retry_on_error
+ if retry_on_error:
if retry is None:
self.retry = Retry(NoBackoff(), 1)
else:
# deep-copy the Retry object as it is mutable
self.retry = copy.deepcopy(retry)
+ # Update the retry's supported errors with the specified errors
+ self.retry.update_supported_erros(retry_on_error)
else:
self.retry = Retry(NoBackoff(), 0)
self.health_check_interval = health_check_interval
@@ -969,6 +978,7 @@ def __init__(
encoding_errors="strict",
decode_responses=False,
retry_on_timeout=False,
+ retry_on_error=[],
parser_class=DefaultParser,
socket_read_size=65536,
health_check_interval=0,
@@ -978,8 +988,10 @@ def __init__(
):
"""
Initialize a new UnixDomainSocketConnection.
- To specify a retry policy, first set `retry_on_timeout` to `True`
- then set `retry` to a valid `Retry` object
+ To specify a retry policy for specific errors, first set
+ `retry_on_error` to a list of the error/s to retry on, then set
+ `retry` to a valid `Retry` object.
+ To retry on TimeoutError, `retry_on_timeout` can also be set to `True`.
"""
self.pid = os.getpid()
self.path = path
@@ -990,11 +1002,17 @@ def __init__(
self.socket_timeout = socket_timeout
self.retry_on_timeout = retry_on_timeout
if retry_on_timeout:
+ # Add TimeoutError to the errors list to retry on
+ retry_on_error.append(TimeoutError)
+ self.retry_on_error = retry_on_error
+ if self.retry_on_error:
if retry is None:
self.retry = Retry(NoBackoff(), 1)
else:
# deep-copy the Retry object as it is mutable
self.retry = copy.deepcopy(retry)
+ # Update the retry's supported errors with the specified errors
+ self.retry.update_supported_erros(retry_on_error)
else:
self.retry = Retry(NoBackoff(), 0)
self.health_check_interval = health_check_interval
@@ -1052,6 +1070,7 @@ def to_bool(value):
"socket_connect_timeout": float,
"socket_keepalive": to_bool,
"retry_on_timeout": to_bool,
+ "retry_on_error": list,
"max_connections": int,
"health_check_interval": int,
"ssl_check_hostname": to_bool,
diff --git a/redis/retry.py b/redis/retry.py
--- a/redis/retry.py
+++ b/redis/retry.py
@@ -19,6 +19,14 @@ def __init__(
self._retries = retries
self._supported_errors = supported_errors
+ def update_supported_erros(self, specified_errors: list):
+ """
+ Updates the supported errors with the specified error types
+ """
+ self._supported_errors = tuple(
+ set(self._supported_errors + tuple(specified_errors))
+ )
+
def call_with_retry(self, do, fail):
"""
Execute an operation that might fail and returns its result, or
| diff --git a/tests/test_retry.py b/tests/test_retry.py
--- a/tests/test_retry.py
+++ b/tests/test_retry.py
@@ -1,10 +1,20 @@
+from unittest.mock import patch
+
import pytest
from redis.backoff import NoBackoff
+from redis.client import Redis
from redis.connection import Connection, UnixDomainSocketConnection
-from redis.exceptions import ConnectionError
+from redis.exceptions import (
+ BusyLoadingError,
+ ConnectionError,
+ ReadOnlyError,
+ TimeoutError,
+)
from redis.retry import Retry
+from .conftest import _get_client
+
class BackoffMock:
def __init__(self):
@@ -39,6 +49,37 @@ def test_retry_on_timeout_retry(self, Class, retries):
assert isinstance(c.retry, Retry)
assert c.retry._retries == retries
+ @pytest.mark.parametrize("Class", [Connection, UnixDomainSocketConnection])
+ def test_retry_on_error(self, Class):
+ c = Class(retry_on_error=[ReadOnlyError])
+ assert c.retry_on_error == [ReadOnlyError]
+ assert isinstance(c.retry, Retry)
+ assert c.retry._retries == 1
+
+ @pytest.mark.parametrize("Class", [Connection, UnixDomainSocketConnection])
+ def test_retry_on_error_empty_value(self, Class):
+ c = Class(retry_on_error=[])
+ assert c.retry_on_error == []
+ assert isinstance(c.retry, Retry)
+ assert c.retry._retries == 0
+
+ @pytest.mark.parametrize("Class", [Connection, UnixDomainSocketConnection])
+ def test_retry_on_error_and_timeout(self, Class):
+ c = Class(
+ retry_on_error=[ReadOnlyError, BusyLoadingError], retry_on_timeout=True
+ )
+ assert c.retry_on_error == [ReadOnlyError, BusyLoadingError, TimeoutError]
+ assert isinstance(c.retry, Retry)
+ assert c.retry._retries == 1
+
+ @pytest.mark.parametrize("retries", range(10))
+ @pytest.mark.parametrize("Class", [Connection, UnixDomainSocketConnection])
+ def test_retry_on_error_retry(self, Class, retries):
+ c = Class(retry_on_error=[ReadOnlyError], retry=Retry(NoBackoff(), retries))
+ assert c.retry_on_error == [ReadOnlyError]
+ assert isinstance(c.retry, Retry)
+ assert c.retry._retries == retries
+
class TestRetry:
"Test that Retry calls backoff and retries the expected number of times"
@@ -65,3 +106,85 @@ def test_retry(self, retries):
assert self.actual_failures == 1 + retries
assert backoff.reset_calls == 1
assert backoff.calls == retries
+
+
[email protected]
+class TestRedisClientRetry:
+ "Test the standalone Redis client behavior with retries"
+
+ def test_client_retry_on_error_with_success(self, request):
+ with patch.object(Redis, "parse_response") as parse_response:
+
+ def mock_parse_response(connection, *args, **options):
+ def ok_response(connection, *args, **options):
+ return "MOCK_OK"
+
+ parse_response.side_effect = ok_response
+ raise ReadOnlyError()
+
+ parse_response.side_effect = mock_parse_response
+ r = _get_client(Redis, request, retry_on_error=[ReadOnlyError])
+ assert r.get("foo") == "MOCK_OK"
+ assert parse_response.call_count == 2
+
+ def test_client_retry_on_error_raise(self, request):
+ with patch.object(Redis, "parse_response") as parse_response:
+ parse_response.side_effect = BusyLoadingError()
+ retries = 3
+ r = _get_client(
+ Redis,
+ request,
+ retry_on_error=[ReadOnlyError, BusyLoadingError],
+ retry=Retry(NoBackoff(), retries),
+ )
+ with pytest.raises(BusyLoadingError):
+ try:
+ r.get("foo")
+ finally:
+ assert parse_response.call_count == retries + 1
+
+ def test_client_retry_on_error_different_error_raised(self, request):
+ with patch.object(Redis, "parse_response") as parse_response:
+ parse_response.side_effect = TimeoutError()
+ retries = 3
+ r = _get_client(
+ Redis,
+ request,
+ retry_on_error=[ReadOnlyError],
+ retry=Retry(NoBackoff(), retries),
+ )
+ with pytest.raises(TimeoutError):
+ try:
+ r.get("foo")
+ finally:
+ assert parse_response.call_count == 1
+
+ def test_client_retry_on_error_and_timeout(self, request):
+ with patch.object(Redis, "parse_response") as parse_response:
+ parse_response.side_effect = TimeoutError()
+ retries = 3
+ r = _get_client(
+ Redis,
+ request,
+ retry_on_error=[ReadOnlyError],
+ retry_on_timeout=True,
+ retry=Retry(NoBackoff(), retries),
+ )
+ with pytest.raises(TimeoutError):
+ try:
+ r.get("foo")
+ finally:
+ assert parse_response.call_count == retries + 1
+
+ def test_client_retry_on_timeout(self, request):
+ with patch.object(Redis, "parse_response") as parse_response:
+ parse_response.side_effect = TimeoutError()
+ retries = 3
+ r = _get_client(
+ Redis, request, retry_on_timeout=True, retry=Retry(NoBackoff(), retries)
+ )
+ with pytest.raises(TimeoutError):
+ try:
+ r.get("foo")
+ finally:
+ assert parse_response.call_count == retries + 1
| Getting ReadOnly error when writing data to AWS elastic cache while the cluster is vertically scaled
I have a python application that writes some data to AWS elastic cache cluster at a regular interval.
Here's an example script that simulates the functionality of my application. It also replicates the error that I have been facing.
```py
from datetime import datetime
import redis
import time
import sys
import random
class Config:
default_host = "localhost"
master_host = "xxx.xx.0001.xxxx.cache.amazonaws.com"
replica_host = "xxx.xx.0001.xxxx.cache.amazonaws.com"
redis_db = 8
socket_conn_timeout = 10
request_delay_sec = 0.1
def get_redis_client():
return redis.Redis(
host=Config.master_host,
db=Config.redis_db,
socket_connect_timeout=Config.socket_conn_timeout,
)
def get_random_key_value():
val = time.time()
key = "test_key_" + str(random.randint(0, 100))
return key, val
r = get_redis_client()
r.flushdb()
flag = False
while True:
try:
if flag:
print("beat:", time.time())
r.set(*get_random_key_value())
time.sleep(Config.request_delay_sec)
except redis.RedisError as re:
print(datetime.now(), "Error:", type(re), re)
flag = True
# sys.exit()
except KeyboardInterrupt:
print("Stopping loop execution")
sys.exit()
```
Here are the environment details of my application
- OS(Ubuntu 18.04)
- Python(v 3.7.0)
- Redis-py(v 3.5.3)
- AWS elastic cache(cluster mode disabled, 1 master node, 1 read replica)
When I scale my AWS elastic cache cluster vertically and the above script is running, I get the following error for few seconds while the cluster scaling-up is in process and then it goes away.
```bash
<class 'redis.exceptions.ReadOnlyError'> You can't write against a read only replica.
```
AWS docs also states that during vertical scaling process some inconsistencies may occur because of data syncing(https://docs.aws.amazon.com/AmazonElastiCache/latest/red-ug/redis-cluster-vertical-scaling.html)
why this error occurs during the scale up process? how can it be fixed?
I tried the same thing with a golang script and it works perfectly fine.
| @Akshit8 This looks like an issue in ElastiCache as opposed to redis-py. Would you mind having a look at redis-py 4? This a release back - and to be honest, I'd expect the same behaviour.
If you're feeling particularly testworthy I'd *love* a hand testing the cluster code we pulled in from @barshaul in redis-py 4.1.0rc1.
I'll try with redis-py 4.
The above version of the script in Golang works fine, could it be related DNS resolution?
I don't think so. Ultimately it's (Redis) ElastiCache returning that error. That phrase doesn't appear anywhere in the codebase, so we're surfacing the alert you receive.
Hey @Akshit8, I will try to reproduce it this week
Could you share the golang script you're using too?
@barshaul
```go
package main
import (
"context"
"fmt"
"log"
"os"
"time"
"github.com/go-redis/redis/v8"
)
const (
DEFAULT_HOST = "localhost"
MASTER_HOST = "xxx.ng.0001.xxx.cache.amazonaws.com"
REPLICA_HOST = "xxx.xx.ng.0001.xxx.cache.amazonaws.com"
REDIS_DB_KEY = 8
SOCKET_CONNECTION_TIMEOUT = 5
REQUEST_DELAY_SEC = 100
)
func getRedisClient() *redis.Client {
return redis.NewClient(&redis.Options{
Addr: fmt.Sprintf("%s:%d", DEFAULT_HOST, 6379),
DB: REDIS_DB_KEY,
DialTimeout: time.Duration(SOCKET_CONNECTION_TIMEOUT * time.Second),
})
}
func getRandomKeyValue() (string, string) {
key := fmt.Sprintf("TEST_KEY_%d", time.Now().Unix())
value := "THIS IS A TEST MESSAGE"
return key, value
}
func main() {
log.Println("starting execution")
ctx := context.Background()
flag := false
rc := getRedisClient()
err := rc.FlushDB(ctx).Err()
if err != nil {
log.Println("ERROR:", err)
os.Exit(1)
}
for {
key, value := getRandomKeyValue()
err := rc.Set(ctx, key, value, time.Hour).Err()
if flag {
fmt.Println("beat:", time.Now())
}
if err != nil {
flag = true
log.Println("ERROR:", err)
}
time.Sleep(time.Duration(REQUEST_DELAY_SEC * time.Millisecond))
}
}
``` | "2021-12-18T12:14:28Z" | 4.1 | [
"tests/test_retry.py::TestConnectionConstructorWithRetry::test_retry_on_timeout_boolean[Connection-False]",
"tests/test_retry.py::TestConnectionConstructorWithRetry::test_retry_on_timeout_boolean[Connection-True]",
"tests/test_retry.py::TestConnectionConstructorWithRetry::test_retry_on_timeout_boolean[UnixDomainSocketConnection-False]",
"tests/test_retry.py::TestConnectionConstructorWithRetry::test_retry_on_timeout_boolean[UnixDomainSocketConnection-True]",
"tests/test_retry.py::TestConnectionConstructorWithRetry::test_retry_on_timeout_retry[Connection-0]",
"tests/test_retry.py::TestConnectionConstructorWithRetry::test_retry_on_timeout_retry[Connection-1]",
"tests/test_retry.py::TestConnectionConstructorWithRetry::test_retry_on_timeout_retry[Connection-2]",
"tests/test_retry.py::TestConnectionConstructorWithRetry::test_retry_on_timeout_retry[Connection-3]",
"tests/test_retry.py::TestConnectionConstructorWithRetry::test_retry_on_timeout_retry[Connection-4]",
"tests/test_retry.py::TestConnectionConstructorWithRetry::test_retry_on_timeout_retry[Connection-5]",
"tests/test_retry.py::TestConnectionConstructorWithRetry::test_retry_on_timeout_retry[Connection-6]",
"tests/test_retry.py::TestConnectionConstructorWithRetry::test_retry_on_timeout_retry[Connection-7]",
"tests/test_retry.py::TestConnectionConstructorWithRetry::test_retry_on_timeout_retry[Connection-8]",
"tests/test_retry.py::TestConnectionConstructorWithRetry::test_retry_on_timeout_retry[Connection-9]",
"tests/test_retry.py::TestConnectionConstructorWithRetry::test_retry_on_timeout_retry[UnixDomainSocketConnection-0]",
"tests/test_retry.py::TestConnectionConstructorWithRetry::test_retry_on_timeout_retry[UnixDomainSocketConnection-1]",
"tests/test_retry.py::TestConnectionConstructorWithRetry::test_retry_on_timeout_retry[UnixDomainSocketConnection-2]",
"tests/test_retry.py::TestConnectionConstructorWithRetry::test_retry_on_timeout_retry[UnixDomainSocketConnection-3]",
"tests/test_retry.py::TestConnectionConstructorWithRetry::test_retry_on_timeout_retry[UnixDomainSocketConnection-4]",
"tests/test_retry.py::TestConnectionConstructorWithRetry::test_retry_on_timeout_retry[UnixDomainSocketConnection-5]",
"tests/test_retry.py::TestConnectionConstructorWithRetry::test_retry_on_timeout_retry[UnixDomainSocketConnection-6]",
"tests/test_retry.py::TestConnectionConstructorWithRetry::test_retry_on_timeout_retry[UnixDomainSocketConnection-7]",
"tests/test_retry.py::TestConnectionConstructorWithRetry::test_retry_on_timeout_retry[UnixDomainSocketConnection-8]",
"tests/test_retry.py::TestConnectionConstructorWithRetry::test_retry_on_timeout_retry[UnixDomainSocketConnection-9]",
"tests/test_retry.py::TestRetry::test_retry[0]",
"tests/test_retry.py::TestRetry::test_retry[1]",
"tests/test_retry.py::TestRetry::test_retry[2]",
"tests/test_retry.py::TestRetry::test_retry[3]",
"tests/test_retry.py::TestRetry::test_retry[4]",
"tests/test_retry.py::TestRetry::test_retry[5]",
"tests/test_retry.py::TestRetry::test_retry[6]",
"tests/test_retry.py::TestRetry::test_retry[7]",
"tests/test_retry.py::TestRetry::test_retry[8]",
"tests/test_retry.py::TestRetry::test_retry[9]",
"tests/test_retry.py::TestRedisClientRetry::test_client_retry_on_timeout"
] | [
"tests/test_retry.py::TestConnectionConstructorWithRetry::test_retry_on_error[Connection]",
"tests/test_retry.py::TestConnectionConstructorWithRetry::test_retry_on_error[UnixDomainSocketConnection]",
"tests/test_retry.py::TestConnectionConstructorWithRetry::test_retry_on_error_empty_value[Connection]",
"tests/test_retry.py::TestConnectionConstructorWithRetry::test_retry_on_error_empty_value[UnixDomainSocketConnection]",
"tests/test_retry.py::TestConnectionConstructorWithRetry::test_retry_on_error_and_timeout[Connection]",
"tests/test_retry.py::TestConnectionConstructorWithRetry::test_retry_on_error_and_timeout[UnixDomainSocketConnection]",
"tests/test_retry.py::TestConnectionConstructorWithRetry::test_retry_on_error_retry[Connection-0]",
"tests/test_retry.py::TestConnectionConstructorWithRetry::test_retry_on_error_retry[Connection-1]",
"tests/test_retry.py::TestConnectionConstructorWithRetry::test_retry_on_error_retry[Connection-2]",
"tests/test_retry.py::TestConnectionConstructorWithRetry::test_retry_on_error_retry[Connection-3]",
"tests/test_retry.py::TestConnectionConstructorWithRetry::test_retry_on_error_retry[Connection-4]",
"tests/test_retry.py::TestConnectionConstructorWithRetry::test_retry_on_error_retry[Connection-5]",
"tests/test_retry.py::TestConnectionConstructorWithRetry::test_retry_on_error_retry[Connection-6]",
"tests/test_retry.py::TestConnectionConstructorWithRetry::test_retry_on_error_retry[Connection-7]",
"tests/test_retry.py::TestConnectionConstructorWithRetry::test_retry_on_error_retry[Connection-8]",
"tests/test_retry.py::TestConnectionConstructorWithRetry::test_retry_on_error_retry[Connection-9]",
"tests/test_retry.py::TestConnectionConstructorWithRetry::test_retry_on_error_retry[UnixDomainSocketConnection-0]",
"tests/test_retry.py::TestConnectionConstructorWithRetry::test_retry_on_error_retry[UnixDomainSocketConnection-1]",
"tests/test_retry.py::TestConnectionConstructorWithRetry::test_retry_on_error_retry[UnixDomainSocketConnection-2]",
"tests/test_retry.py::TestConnectionConstructorWithRetry::test_retry_on_error_retry[UnixDomainSocketConnection-3]",
"tests/test_retry.py::TestConnectionConstructorWithRetry::test_retry_on_error_retry[UnixDomainSocketConnection-4]",
"tests/test_retry.py::TestConnectionConstructorWithRetry::test_retry_on_error_retry[UnixDomainSocketConnection-5]",
"tests/test_retry.py::TestConnectionConstructorWithRetry::test_retry_on_error_retry[UnixDomainSocketConnection-6]",
"tests/test_retry.py::TestConnectionConstructorWithRetry::test_retry_on_error_retry[UnixDomainSocketConnection-7]",
"tests/test_retry.py::TestConnectionConstructorWithRetry::test_retry_on_error_retry[UnixDomainSocketConnection-8]",
"tests/test_retry.py::TestConnectionConstructorWithRetry::test_retry_on_error_retry[UnixDomainSocketConnection-9]",
"tests/test_retry.py::TestRedisClientRetry::test_client_retry_on_error_with_success",
"tests/test_retry.py::TestRedisClientRetry::test_client_retry_on_error_raise",
"tests/test_retry.py::TestRedisClientRetry::test_client_retry_on_error_different_error_raised",
"tests/test_retry.py::TestRedisClientRetry::test_client_retry_on_error_and_timeout"
] | Python | [] | [] |
redis/redis-py | 1,858 | redis__redis-py-1858 | [
"1809"
] | 9442d34beb8254aebe87b9b48ef7e627e1079837 | diff --git a/redis/commands/core.py b/redis/commands/core.py
--- a/redis/commands/core.py
+++ b/redis/commands/core.py
@@ -2224,6 +2224,39 @@ def sort(
options = {"groups": len(get) if groups else None}
return self.execute_command("SORT", *pieces, **options)
+ def sort_ro(
+ self,
+ key: str,
+ start: Optional[int] = None,
+ num: Optional[int] = None,
+ by: Optional[str] = None,
+ get: Optional[List[str]] = None,
+ desc: bool = False,
+ alpha: bool = False,
+ ) -> list:
+ """
+ Returns the elements contained in the list, set or sorted set at key.
+ (read-only variant of the SORT command)
+
+ ``start`` and ``num`` allow for paging through the sorted data
+
+ ``by`` allows using an external key to weight and sort the items.
+ Use an "*" to indicate where in the key the item value is located
+
+ ``get`` allows for returning items from external keys rather than the
+ sorted data itself. Use an "*" to indicate where in the key
+ the item value is located
+
+ ``desc`` allows for reversing the sort
+
+ ``alpha`` allows for sorting lexicographically rather than numerically
+
+ For more information check https://redis.io/commands/sort_ro
+ """
+ return self.sort(
+ key, start=start, num=num, by=by, get=get, desc=desc, alpha=alpha
+ )
+
class ScanCommands:
"""
| diff --git a/tests/test_commands.py b/tests/test_commands.py
--- a/tests/test_commands.py
+++ b/tests/test_commands.py
@@ -2700,6 +2700,16 @@ def test_sort_all_options(self, r):
assert num == 4
assert r.lrange("sorted", 0, 10) == [b"vodka", b"milk", b"gin", b"apple juice"]
+ @skip_if_server_version_lt("7.0.0")
+ def test_sort_ro(self, r):
+ r["score:1"] = 8
+ r["score:2"] = 3
+ r["score:3"] = 5
+ r.rpush("a", "3", "2", "1")
+ assert r.sort_ro("a", by="score:*") == [b"2", b"3", b"1"]
+ r.rpush("b", "2", "3", "1")
+ assert r.sort_ro("b", desc=True) == [b"3", b"2", b"1"]
+
def test_sort_issue_924(self, r):
# Tests for issue https://github.com/andymccurdy/redis-py/issues/924
r.execute_command("SADD", "issue#924", 1)
| Add support for SORT_RO
| "2022-01-05T10:56:52Z" | 4.1 | [
"tests/test_commands.py::TestResponseCallbacks::test_response_callbacks",
"tests/test_commands.py::TestResponseCallbacks::test_case_insensitive_command_names",
"tests/test_commands.py::TestRedisCommands::test_command_on_invalid_key_type",
"tests/test_commands.py::TestRedisCommands::test_acl_cat_no_category",
"tests/test_commands.py::TestRedisCommands::test_acl_cat_with_category",
"tests/test_commands.py::TestRedisCommands::test_acl_deluser",
"tests/test_commands.py::TestRedisCommands::test_acl_genpass",
"tests/test_commands.py::TestRedisCommands::test_acl_help",
"tests/test_commands.py::TestRedisCommands::test_acl_list",
"tests/test_commands.py::TestRedisCommands::test_acl_log",
"tests/test_commands.py::TestRedisCommands::test_acl_setuser_categories_without_prefix_fails",
"tests/test_commands.py::TestRedisCommands::test_acl_setuser_commands_without_prefix_fails",
"tests/test_commands.py::TestRedisCommands::test_acl_setuser_add_passwords_and_nopass_fails",
"tests/test_commands.py::TestRedisCommands::test_acl_users",
"tests/test_commands.py::TestRedisCommands::test_acl_whoami",
"tests/test_commands.py::TestRedisCommands::test_client_list",
"tests/test_commands.py::TestRedisCommands::test_client_info",
"tests/test_commands.py::TestRedisCommands::test_client_list_types_not_replica",
"tests/test_commands.py::TestRedisCommands::test_client_list_replica",
"tests/test_commands.py::TestRedisCommands::test_client_list_client_id",
"tests/test_commands.py::TestRedisCommands::test_client_id",
"tests/test_commands.py::TestRedisCommands::test_client_trackinginfo",
"tests/test_commands.py::TestRedisCommands::test_client_tracking",
"tests/test_commands.py::TestRedisCommands::test_client_unblock",
"tests/test_commands.py::TestRedisCommands::test_client_getname",
"tests/test_commands.py::TestRedisCommands::test_client_setname",
"tests/test_commands.py::TestRedisCommands::test_client_kill",
"tests/test_commands.py::TestRedisCommands::test_client_kill_filter_invalid_params",
"tests/test_commands.py::TestRedisCommands::test_client_kill_filter_by_id",
"tests/test_commands.py::TestRedisCommands::test_client_kill_filter_by_addr",
"tests/test_commands.py::TestRedisCommands::test_client_list_after_client_setname",
"tests/test_commands.py::TestRedisCommands::test_client_kill_filter_by_laddr",
"tests/test_commands.py::TestRedisCommands::test_client_kill_filter_by_user",
"tests/test_commands.py::TestRedisCommands::test_client_pause",
"tests/test_commands.py::TestRedisCommands::test_client_pause_all",
"tests/test_commands.py::TestRedisCommands::test_client_unpause",
"tests/test_commands.py::TestRedisCommands::test_client_reply",
"tests/test_commands.py::TestRedisCommands::test_client_getredir",
"tests/test_commands.py::TestRedisCommands::test_config_get",
"tests/test_commands.py::TestRedisCommands::test_config_resetstat",
"tests/test_commands.py::TestRedisCommands::test_config_set",
"tests/test_commands.py::TestRedisCommands::test_dbsize",
"tests/test_commands.py::TestRedisCommands::test_echo",
"tests/test_commands.py::TestRedisCommands::test_info",
"tests/test_commands.py::TestRedisCommands::test_lastsave",
"tests/test_commands.py::TestRedisCommands::test_lolwut",
"tests/test_commands.py::TestRedisCommands::test_reset",
"tests/test_commands.py::TestRedisCommands::test_object",
"tests/test_commands.py::TestRedisCommands::test_ping",
"tests/test_commands.py::TestRedisCommands::test_quit",
"tests/test_commands.py::TestRedisCommands::test_role",
"tests/test_commands.py::TestRedisCommands::test_select",
"tests/test_commands.py::TestRedisCommands::test_slowlog_get",
"tests/test_commands.py::TestRedisCommands::test_slowlog_get_limit",
"tests/test_commands.py::TestRedisCommands::test_slowlog_length",
"tests/test_commands.py::TestRedisCommands::test_time",
"tests/test_commands.py::TestRedisCommands::test_bgsave",
"tests/test_commands.py::TestRedisCommands::test_append",
"tests/test_commands.py::TestRedisCommands::test_bitcount",
"tests/test_commands.py::TestRedisCommands::test_bitop_not_empty_string",
"tests/test_commands.py::TestRedisCommands::test_bitop_not",
"tests/test_commands.py::TestRedisCommands::test_bitop_not_in_place",
"tests/test_commands.py::TestRedisCommands::test_bitop_single_string",
"tests/test_commands.py::TestRedisCommands::test_bitop_string_operands",
"tests/test_commands.py::TestRedisCommands::test_bitpos",
"tests/test_commands.py::TestRedisCommands::test_bitpos_wrong_arguments",
"tests/test_commands.py::TestRedisCommands::test_copy",
"tests/test_commands.py::TestRedisCommands::test_copy_and_replace",
"tests/test_commands.py::TestRedisCommands::test_copy_to_another_database",
"tests/test_commands.py::TestRedisCommands::test_decr",
"tests/test_commands.py::TestRedisCommands::test_decrby",
"tests/test_commands.py::TestRedisCommands::test_delete",
"tests/test_commands.py::TestRedisCommands::test_delete_with_multiple_keys",
"tests/test_commands.py::TestRedisCommands::test_delitem",
"tests/test_commands.py::TestRedisCommands::test_unlink",
"tests/test_commands.py::TestRedisCommands::test_unlink_with_multiple_keys",
"tests/test_commands.py::TestRedisCommands::test_dump_and_restore",
"tests/test_commands.py::TestRedisCommands::test_dump_and_restore_and_replace",
"tests/test_commands.py::TestRedisCommands::test_dump_and_restore_absttl",
"tests/test_commands.py::TestRedisCommands::test_exists",
"tests/test_commands.py::TestRedisCommands::test_exists_contains",
"tests/test_commands.py::TestRedisCommands::test_expire",
"tests/test_commands.py::TestRedisCommands::test_expireat_datetime",
"tests/test_commands.py::TestRedisCommands::test_expireat_no_key",
"tests/test_commands.py::TestRedisCommands::test_expireat_unixtime",
"tests/test_commands.py::TestRedisCommands::test_get_and_set",
"tests/test_commands.py::TestRedisCommands::test_getdel",
"tests/test_commands.py::TestRedisCommands::test_getex",
"tests/test_commands.py::TestRedisCommands::test_getitem_and_setitem",
"tests/test_commands.py::TestRedisCommands::test_getitem_raises_keyerror_for_missing_key",
"tests/test_commands.py::TestRedisCommands::test_getitem_does_not_raise_keyerror_for_empty_string",
"tests/test_commands.py::TestRedisCommands::test_get_set_bit",
"tests/test_commands.py::TestRedisCommands::test_getrange",
"tests/test_commands.py::TestRedisCommands::test_getset",
"tests/test_commands.py::TestRedisCommands::test_incr",
"tests/test_commands.py::TestRedisCommands::test_incrby",
"tests/test_commands.py::TestRedisCommands::test_incrbyfloat",
"tests/test_commands.py::TestRedisCommands::test_keys",
"tests/test_commands.py::TestRedisCommands::test_mget",
"tests/test_commands.py::TestRedisCommands::test_lmove",
"tests/test_commands.py::TestRedisCommands::test_blmove",
"tests/test_commands.py::TestRedisCommands::test_mset",
"tests/test_commands.py::TestRedisCommands::test_msetnx",
"tests/test_commands.py::TestRedisCommands::test_pexpire",
"tests/test_commands.py::TestRedisCommands::test_pexpireat_datetime",
"tests/test_commands.py::TestRedisCommands::test_pexpireat_no_key",
"tests/test_commands.py::TestRedisCommands::test_pexpireat_unixtime",
"tests/test_commands.py::TestRedisCommands::test_psetex",
"tests/test_commands.py::TestRedisCommands::test_psetex_timedelta",
"tests/test_commands.py::TestRedisCommands::test_pttl",
"tests/test_commands.py::TestRedisCommands::test_pttl_no_key",
"tests/test_commands.py::TestRedisCommands::test_hrandfield",
"tests/test_commands.py::TestRedisCommands::test_randomkey",
"tests/test_commands.py::TestRedisCommands::test_rename",
"tests/test_commands.py::TestRedisCommands::test_renamenx",
"tests/test_commands.py::TestRedisCommands::test_set_nx",
"tests/test_commands.py::TestRedisCommands::test_set_xx",
"tests/test_commands.py::TestRedisCommands::test_set_px",
"tests/test_commands.py::TestRedisCommands::test_set_px_timedelta",
"tests/test_commands.py::TestRedisCommands::test_set_ex",
"tests/test_commands.py::TestRedisCommands::test_set_ex_timedelta",
"tests/test_commands.py::TestRedisCommands::test_set_exat_timedelta",
"tests/test_commands.py::TestRedisCommands::test_set_pxat_timedelta",
"tests/test_commands.py::TestRedisCommands::test_set_multipleoptions",
"tests/test_commands.py::TestRedisCommands::test_set_keepttl",
"tests/test_commands.py::TestRedisCommands::test_set_get",
"tests/test_commands.py::TestRedisCommands::test_setex",
"tests/test_commands.py::TestRedisCommands::test_setnx",
"tests/test_commands.py::TestRedisCommands::test_setrange",
"tests/test_commands.py::TestRedisCommands::test_strlen",
"tests/test_commands.py::TestRedisCommands::test_substr",
"tests/test_commands.py::TestRedisCommands::test_ttl",
"tests/test_commands.py::TestRedisCommands::test_ttl_nokey",
"tests/test_commands.py::TestRedisCommands::test_type",
"tests/test_commands.py::TestRedisCommands::test_blpop",
"tests/test_commands.py::TestRedisCommands::test_brpop",
"tests/test_commands.py::TestRedisCommands::test_brpoplpush",
"tests/test_commands.py::TestRedisCommands::test_brpoplpush_empty_string",
"tests/test_commands.py::TestRedisCommands::test_lindex",
"tests/test_commands.py::TestRedisCommands::test_linsert",
"tests/test_commands.py::TestRedisCommands::test_llen",
"tests/test_commands.py::TestRedisCommands::test_lpop",
"tests/test_commands.py::TestRedisCommands::test_lpop_count",
"tests/test_commands.py::TestRedisCommands::test_lpush",
"tests/test_commands.py::TestRedisCommands::test_lpushx",
"tests/test_commands.py::TestRedisCommands::test_lpushx_with_list",
"tests/test_commands.py::TestRedisCommands::test_lrange",
"tests/test_commands.py::TestRedisCommands::test_lrem",
"tests/test_commands.py::TestRedisCommands::test_lset",
"tests/test_commands.py::TestRedisCommands::test_ltrim",
"tests/test_commands.py::TestRedisCommands::test_rpop",
"tests/test_commands.py::TestRedisCommands::test_rpop_count",
"tests/test_commands.py::TestRedisCommands::test_rpoplpush",
"tests/test_commands.py::TestRedisCommands::test_rpush",
"tests/test_commands.py::TestRedisCommands::test_lpos",
"tests/test_commands.py::TestRedisCommands::test_rpushx",
"tests/test_commands.py::TestRedisCommands::test_scan",
"tests/test_commands.py::TestRedisCommands::test_scan_type",
"tests/test_commands.py::TestRedisCommands::test_scan_iter",
"tests/test_commands.py::TestRedisCommands::test_sscan",
"tests/test_commands.py::TestRedisCommands::test_sscan_iter",
"tests/test_commands.py::TestRedisCommands::test_hscan",
"tests/test_commands.py::TestRedisCommands::test_hscan_iter",
"tests/test_commands.py::TestRedisCommands::test_zscan",
"tests/test_commands.py::TestRedisCommands::test_zscan_iter",
"tests/test_commands.py::TestRedisCommands::test_sadd",
"tests/test_commands.py::TestRedisCommands::test_scard",
"tests/test_commands.py::TestRedisCommands::test_sdiff",
"tests/test_commands.py::TestRedisCommands::test_sdiffstore",
"tests/test_commands.py::TestRedisCommands::test_sinter",
"tests/test_commands.py::TestRedisCommands::test_sinterstore",
"tests/test_commands.py::TestRedisCommands::test_sismember",
"tests/test_commands.py::TestRedisCommands::test_smembers",
"tests/test_commands.py::TestRedisCommands::test_smismember",
"tests/test_commands.py::TestRedisCommands::test_smove",
"tests/test_commands.py::TestRedisCommands::test_spop",
"tests/test_commands.py::TestRedisCommands::test_spop_multi_value",
"tests/test_commands.py::TestRedisCommands::test_srandmember",
"tests/test_commands.py::TestRedisCommands::test_srandmember_multi_value",
"tests/test_commands.py::TestRedisCommands::test_srem",
"tests/test_commands.py::TestRedisCommands::test_sunion",
"tests/test_commands.py::TestRedisCommands::test_sunionstore",
"tests/test_commands.py::TestRedisCommands::test_debug_segfault",
"tests/test_commands.py::TestRedisCommands::test_script_debug",
"tests/test_commands.py::TestRedisCommands::test_zadd",
"tests/test_commands.py::TestRedisCommands::test_zadd_nx",
"tests/test_commands.py::TestRedisCommands::test_zadd_xx",
"tests/test_commands.py::TestRedisCommands::test_zadd_ch",
"tests/test_commands.py::TestRedisCommands::test_zadd_incr",
"tests/test_commands.py::TestRedisCommands::test_zadd_incr_with_xx",
"tests/test_commands.py::TestRedisCommands::test_zadd_gt_lt",
"tests/test_commands.py::TestRedisCommands::test_zcard",
"tests/test_commands.py::TestRedisCommands::test_zcount",
"tests/test_commands.py::TestRedisCommands::test_zdiff",
"tests/test_commands.py::TestRedisCommands::test_zdiffstore",
"tests/test_commands.py::TestRedisCommands::test_zincrby",
"tests/test_commands.py::TestRedisCommands::test_zlexcount",
"tests/test_commands.py::TestRedisCommands::test_zinter",
"tests/test_commands.py::TestRedisCommands::test_zinterstore_sum",
"tests/test_commands.py::TestRedisCommands::test_zinterstore_max",
"tests/test_commands.py::TestRedisCommands::test_zinterstore_min",
"tests/test_commands.py::TestRedisCommands::test_zinterstore_with_weight",
"tests/test_commands.py::TestRedisCommands::test_zpopmax",
"tests/test_commands.py::TestRedisCommands::test_zpopmin",
"tests/test_commands.py::TestRedisCommands::test_zrandemember",
"tests/test_commands.py::TestRedisCommands::test_bzpopmax",
"tests/test_commands.py::TestRedisCommands::test_bzpopmin",
"tests/test_commands.py::TestRedisCommands::test_zrange",
"tests/test_commands.py::TestRedisCommands::test_zrange_errors",
"tests/test_commands.py::TestRedisCommands::test_zrange_params",
"tests/test_commands.py::TestRedisCommands::test_zrangestore",
"tests/test_commands.py::TestRedisCommands::test_zrangebylex",
"tests/test_commands.py::TestRedisCommands::test_zrevrangebylex",
"tests/test_commands.py::TestRedisCommands::test_zrangebyscore",
"tests/test_commands.py::TestRedisCommands::test_zrank",
"tests/test_commands.py::TestRedisCommands::test_zrem",
"tests/test_commands.py::TestRedisCommands::test_zrem_multiple_keys",
"tests/test_commands.py::TestRedisCommands::test_zremrangebylex",
"tests/test_commands.py::TestRedisCommands::test_zremrangebyrank",
"tests/test_commands.py::TestRedisCommands::test_zremrangebyscore",
"tests/test_commands.py::TestRedisCommands::test_zrevrange",
"tests/test_commands.py::TestRedisCommands::test_zrevrangebyscore",
"tests/test_commands.py::TestRedisCommands::test_zrevrank",
"tests/test_commands.py::TestRedisCommands::test_zscore",
"tests/test_commands.py::TestRedisCommands::test_zunion",
"tests/test_commands.py::TestRedisCommands::test_zunionstore_sum",
"tests/test_commands.py::TestRedisCommands::test_zunionstore_max",
"tests/test_commands.py::TestRedisCommands::test_zunionstore_min",
"tests/test_commands.py::TestRedisCommands::test_zunionstore_with_weight",
"tests/test_commands.py::TestRedisCommands::test_zmscore",
"tests/test_commands.py::TestRedisCommands::test_pfadd",
"tests/test_commands.py::TestRedisCommands::test_pfcount",
"tests/test_commands.py::TestRedisCommands::test_pfmerge",
"tests/test_commands.py::TestRedisCommands::test_hget_and_hset",
"tests/test_commands.py::TestRedisCommands::test_hset_with_multi_key_values",
"tests/test_commands.py::TestRedisCommands::test_hset_without_data",
"tests/test_commands.py::TestRedisCommands::test_hdel",
"tests/test_commands.py::TestRedisCommands::test_hexists",
"tests/test_commands.py::TestRedisCommands::test_hgetall",
"tests/test_commands.py::TestRedisCommands::test_hincrby",
"tests/test_commands.py::TestRedisCommands::test_hincrbyfloat",
"tests/test_commands.py::TestRedisCommands::test_hkeys",
"tests/test_commands.py::TestRedisCommands::test_hlen",
"tests/test_commands.py::TestRedisCommands::test_hmget",
"tests/test_commands.py::TestRedisCommands::test_hmset",
"tests/test_commands.py::TestRedisCommands::test_hsetnx",
"tests/test_commands.py::TestRedisCommands::test_hvals",
"tests/test_commands.py::TestRedisCommands::test_hstrlen",
"tests/test_commands.py::TestRedisCommands::test_sort_basic",
"tests/test_commands.py::TestRedisCommands::test_sort_limited",
"tests/test_commands.py::TestRedisCommands::test_sort_by",
"tests/test_commands.py::TestRedisCommands::test_sort_get",
"tests/test_commands.py::TestRedisCommands::test_sort_get_multi",
"tests/test_commands.py::TestRedisCommands::test_sort_get_groups_two",
"tests/test_commands.py::TestRedisCommands::test_sort_groups_string_get",
"tests/test_commands.py::TestRedisCommands::test_sort_groups_just_one_get",
"tests/test_commands.py::TestRedisCommands::test_sort_groups_no_get",
"tests/test_commands.py::TestRedisCommands::test_sort_groups_three_gets",
"tests/test_commands.py::TestRedisCommands::test_sort_desc",
"tests/test_commands.py::TestRedisCommands::test_sort_alpha",
"tests/test_commands.py::TestRedisCommands::test_sort_store",
"tests/test_commands.py::TestRedisCommands::test_sort_all_options",
"tests/test_commands.py::TestRedisCommands::test_sort_issue_924",
"tests/test_commands.py::TestRedisCommands::test_cluster_addslots",
"tests/test_commands.py::TestRedisCommands::test_cluster_count_failure_reports",
"tests/test_commands.py::TestRedisCommands::test_cluster_countkeysinslot",
"tests/test_commands.py::TestRedisCommands::test_cluster_delslots",
"tests/test_commands.py::TestRedisCommands::test_cluster_failover",
"tests/test_commands.py::TestRedisCommands::test_cluster_forget",
"tests/test_commands.py::TestRedisCommands::test_cluster_info",
"tests/test_commands.py::TestRedisCommands::test_cluster_keyslot",
"tests/test_commands.py::TestRedisCommands::test_cluster_meet",
"tests/test_commands.py::TestRedisCommands::test_cluster_nodes",
"tests/test_commands.py::TestRedisCommands::test_cluster_replicate",
"tests/test_commands.py::TestRedisCommands::test_cluster_reset",
"tests/test_commands.py::TestRedisCommands::test_cluster_saveconfig",
"tests/test_commands.py::TestRedisCommands::test_cluster_setslot",
"tests/test_commands.py::TestRedisCommands::test_cluster_slaves",
"tests/test_commands.py::TestRedisCommands::test_readonly_invalid_cluster_state",
"tests/test_commands.py::TestRedisCommands::test_readonly",
"tests/test_commands.py::TestRedisCommands::test_geoadd",
"tests/test_commands.py::TestRedisCommands::test_geoadd_nx",
"tests/test_commands.py::TestRedisCommands::test_geoadd_xx",
"tests/test_commands.py::TestRedisCommands::test_geoadd_ch",
"tests/test_commands.py::TestRedisCommands::test_geoadd_invalid_params",
"tests/test_commands.py::TestRedisCommands::test_geodist",
"tests/test_commands.py::TestRedisCommands::test_geodist_units",
"tests/test_commands.py::TestRedisCommands::test_geodist_missing_one_member",
"tests/test_commands.py::TestRedisCommands::test_geodist_invalid_units",
"tests/test_commands.py::TestRedisCommands::test_geohash",
"tests/test_commands.py::TestRedisCommands::test_geopos",
"tests/test_commands.py::TestRedisCommands::test_geopos_no_value",
"tests/test_commands.py::TestRedisCommands::test_geosearch",
"tests/test_commands.py::TestRedisCommands::test_geosearch_member",
"tests/test_commands.py::TestRedisCommands::test_geosearch_sort",
"tests/test_commands.py::TestRedisCommands::test_geosearch_with",
"tests/test_commands.py::TestRedisCommands::test_geosearch_negative",
"tests/test_commands.py::TestRedisCommands::test_geosearchstore",
"tests/test_commands.py::TestRedisCommands::test_geosearchstore_dist",
"tests/test_commands.py::TestRedisCommands::test_georadius",
"tests/test_commands.py::TestRedisCommands::test_georadius_no_values",
"tests/test_commands.py::TestRedisCommands::test_georadius_units",
"tests/test_commands.py::TestRedisCommands::test_georadius_with",
"tests/test_commands.py::TestRedisCommands::test_georadius_count",
"tests/test_commands.py::TestRedisCommands::test_georadius_sort",
"tests/test_commands.py::TestRedisCommands::test_georadius_store",
"tests/test_commands.py::TestRedisCommands::test_georadius_store_dist",
"tests/test_commands.py::TestRedisCommands::test_georadiusmember",
"tests/test_commands.py::TestRedisCommands::test_georadiusmember_count",
"tests/test_commands.py::TestRedisCommands::test_xack",
"tests/test_commands.py::TestRedisCommands::test_xadd",
"tests/test_commands.py::TestRedisCommands::test_xadd_nomkstream",
"tests/test_commands.py::TestRedisCommands::test_xadd_minlen_and_limit",
"tests/test_commands.py::TestRedisCommands::test_xautoclaim",
"tests/test_commands.py::TestRedisCommands::test_xautoclaim_negative",
"tests/test_commands.py::TestRedisCommands::test_xclaim",
"tests/test_commands.py::TestRedisCommands::test_xdel",
"tests/test_commands.py::TestRedisCommands::test_xgroup_delconsumer",
"tests/test_commands.py::TestRedisCommands::test_xgroup_createconsumer",
"tests/test_commands.py::TestRedisCommands::test_xgroup_destroy",
"tests/test_commands.py::TestRedisCommands::test_xinfo_stream",
"tests/test_commands.py::TestRedisCommands::test_xinfo_stream_full",
"tests/test_commands.py::TestRedisCommands::test_xlen",
"tests/test_commands.py::TestRedisCommands::test_xpending",
"tests/test_commands.py::TestRedisCommands::test_xpending_range",
"tests/test_commands.py::TestRedisCommands::test_xpending_range_idle",
"tests/test_commands.py::TestRedisCommands::test_xpending_range_negative",
"tests/test_commands.py::TestRedisCommands::test_xrange",
"tests/test_commands.py::TestRedisCommands::test_xread",
"tests/test_commands.py::TestRedisCommands::test_xreadgroup",
"tests/test_commands.py::TestRedisCommands::test_xrevrange",
"tests/test_commands.py::TestRedisCommands::test_xtrim",
"tests/test_commands.py::TestRedisCommands::test_xtrim_minlen_and_length_args",
"tests/test_commands.py::TestRedisCommands::test_bitfield_operations",
"tests/test_commands.py::TestRedisCommands::test_memory_help",
"tests/test_commands.py::TestRedisCommands::test_memory_doctor",
"tests/test_commands.py::TestRedisCommands::test_memory_malloc_stats",
"tests/test_commands.py::TestRedisCommands::test_memory_stats",
"tests/test_commands.py::TestRedisCommands::test_memory_usage",
"tests/test_commands.py::TestRedisCommands::test_module_list",
"tests/test_commands.py::TestRedisCommands::test_command_count",
"tests/test_commands.py::TestRedisCommands::test_command_getkeys",
"tests/test_commands.py::TestRedisCommands::test_command",
"tests/test_commands.py::TestRedisCommands::test_module",
"tests/test_commands.py::TestRedisCommands::test_restore",
"tests/test_commands.py::TestRedisCommands::test_restore_idletime",
"tests/test_commands.py::TestRedisCommands::test_restore_frequency",
"tests/test_commands.py::TestRedisCommands::test_replicaof",
"tests/test_commands.py::TestRedisCommands::test_sync",
"tests/test_commands.py::TestRedisCommands::test_psync",
"tests/test_commands.py::TestBinarySave::test_binary_get_set",
"tests/test_commands.py::TestBinarySave::test_binary_lists",
"tests/test_commands.py::TestBinarySave::test_22_info",
"tests/test_commands.py::TestBinarySave::test_large_responses",
"tests/test_commands.py::TestBinarySave::test_floating_point_encoding"
] | [
"tests/test_commands.py::TestRedisCommands::test_sort_ro"
] | Python | [] | [] |
|
redis/redis-py | 1,860 | redis__redis-py-1860 | [
"1806"
] | c4e408880c0fbfe714cd9ad4e1e6b908f00be9b5 | diff --git a/redis/commands/core.py b/redis/commands/core.py
--- a/redis/commands/core.py
+++ b/redis/commands/core.py
@@ -1514,6 +1514,15 @@ def expireat(self, name: KeyT, when: AbsExpiryT) -> ResponseT:
when = int(time.mktime(when.timetuple()))
return self.execute_command("EXPIREAT", name, when)
+ def expiretime(self, key: str) -> int:
+ """
+ Returns the absolute Unix timestamp (since January 1, 1970) in seconds
+ at which the given key will expire.
+
+ For more information check https://redis.io/commands/expiretime
+ """
+ return self.execute_command("EXPIRETIME", key)
+
def get(self, name: KeyT) -> ResponseT:
"""
Return the value at key ``name``, or None if the key doesn't exist
| diff --git a/tests/test_commands.py b/tests/test_commands.py
--- a/tests/test_commands.py
+++ b/tests/test_commands.py
@@ -1075,6 +1075,12 @@ def test_expireat_unixtime(self, r):
assert r.expireat("a", expire_at_seconds) is True
assert 0 < r.ttl("a") <= 61
+ @skip_if_server_version_lt("7.0.0")
+ def test_expiretime(self, r):
+ r.set("a", "foo")
+ r.expireat("a", 33177117420)
+ assert r.expiretime("a") == 33177117420
+
def test_get_and_set(self, r):
# get and set can't be tested independently of each other
assert r.get("a") is None
| Add support for EXPIRETIME
| "2022-01-05T11:00:10Z" | 4.2 | [
"tests/test_commands.py::TestResponseCallbacks::test_response_callbacks",
"tests/test_commands.py::TestResponseCallbacks::test_case_insensitive_command_names",
"tests/test_commands.py::TestRedisCommands::test_auth",
"tests/test_commands.py::TestRedisCommands::test_command_on_invalid_key_type",
"tests/test_commands.py::TestRedisCommands::test_acl_cat_no_category",
"tests/test_commands.py::TestRedisCommands::test_acl_cat_with_category",
"tests/test_commands.py::TestRedisCommands::test_acl_genpass",
"tests/test_commands.py::TestRedisCommands::test_acl_help",
"tests/test_commands.py::TestRedisCommands::test_acl_list",
"tests/test_commands.py::TestRedisCommands::test_acl_log",
"tests/test_commands.py::TestRedisCommands::test_acl_setuser_categories_without_prefix_fails",
"tests/test_commands.py::TestRedisCommands::test_acl_setuser_commands_without_prefix_fails",
"tests/test_commands.py::TestRedisCommands::test_acl_setuser_add_passwords_and_nopass_fails",
"tests/test_commands.py::TestRedisCommands::test_acl_users",
"tests/test_commands.py::TestRedisCommands::test_acl_whoami",
"tests/test_commands.py::TestRedisCommands::test_client_list",
"tests/test_commands.py::TestRedisCommands::test_client_info",
"tests/test_commands.py::TestRedisCommands::test_client_list_types_not_replica",
"tests/test_commands.py::TestRedisCommands::test_client_list_replica",
"tests/test_commands.py::TestRedisCommands::test_client_list_client_id",
"tests/test_commands.py::TestRedisCommands::test_client_id",
"tests/test_commands.py::TestRedisCommands::test_client_trackinginfo",
"tests/test_commands.py::TestRedisCommands::test_client_tracking",
"tests/test_commands.py::TestRedisCommands::test_client_unblock",
"tests/test_commands.py::TestRedisCommands::test_client_getname",
"tests/test_commands.py::TestRedisCommands::test_client_setname",
"tests/test_commands.py::TestRedisCommands::test_client_kill",
"tests/test_commands.py::TestRedisCommands::test_client_kill_filter_invalid_params",
"tests/test_commands.py::TestRedisCommands::test_client_kill_filter_by_id",
"tests/test_commands.py::TestRedisCommands::test_client_kill_filter_by_addr",
"tests/test_commands.py::TestRedisCommands::test_client_list_after_client_setname",
"tests/test_commands.py::TestRedisCommands::test_client_kill_filter_by_laddr",
"tests/test_commands.py::TestRedisCommands::test_client_kill_filter_by_user",
"tests/test_commands.py::TestRedisCommands::test_client_pause",
"tests/test_commands.py::TestRedisCommands::test_client_pause_all",
"tests/test_commands.py::TestRedisCommands::test_client_unpause",
"tests/test_commands.py::TestRedisCommands::test_client_reply",
"tests/test_commands.py::TestRedisCommands::test_client_getredir",
"tests/test_commands.py::TestRedisCommands::test_hello_notI_implemented",
"tests/test_commands.py::TestRedisCommands::test_config_get",
"tests/test_commands.py::TestRedisCommands::test_config_resetstat",
"tests/test_commands.py::TestRedisCommands::test_config_set",
"tests/test_commands.py::TestRedisCommands::test_failover",
"tests/test_commands.py::TestRedisCommands::test_dbsize",
"tests/test_commands.py::TestRedisCommands::test_echo",
"tests/test_commands.py::TestRedisCommands::test_info",
"tests/test_commands.py::TestRedisCommands::test_lastsave",
"tests/test_commands.py::TestRedisCommands::test_lolwut",
"tests/test_commands.py::TestRedisCommands::test_reset",
"tests/test_commands.py::TestRedisCommands::test_object",
"tests/test_commands.py::TestRedisCommands::test_ping",
"tests/test_commands.py::TestRedisCommands::test_quit",
"tests/test_commands.py::TestRedisCommands::test_role",
"tests/test_commands.py::TestRedisCommands::test_select",
"tests/test_commands.py::TestRedisCommands::test_slowlog_get",
"tests/test_commands.py::TestRedisCommands::test_slowlog_get_limit",
"tests/test_commands.py::TestRedisCommands::test_slowlog_length",
"tests/test_commands.py::TestRedisCommands::test_time",
"tests/test_commands.py::TestRedisCommands::test_bgsave",
"tests/test_commands.py::TestRedisCommands::test_append",
"tests/test_commands.py::TestRedisCommands::test_bitcount",
"tests/test_commands.py::TestRedisCommands::test_bitop_not_empty_string",
"tests/test_commands.py::TestRedisCommands::test_bitop_not",
"tests/test_commands.py::TestRedisCommands::test_bitop_not_in_place",
"tests/test_commands.py::TestRedisCommands::test_bitop_single_string",
"tests/test_commands.py::TestRedisCommands::test_bitop_string_operands",
"tests/test_commands.py::TestRedisCommands::test_bitpos",
"tests/test_commands.py::TestRedisCommands::test_bitpos_wrong_arguments",
"tests/test_commands.py::TestRedisCommands::test_copy",
"tests/test_commands.py::TestRedisCommands::test_copy_and_replace",
"tests/test_commands.py::TestRedisCommands::test_copy_to_another_database",
"tests/test_commands.py::TestRedisCommands::test_decr",
"tests/test_commands.py::TestRedisCommands::test_decrby",
"tests/test_commands.py::TestRedisCommands::test_delete",
"tests/test_commands.py::TestRedisCommands::test_delete_with_multiple_keys",
"tests/test_commands.py::TestRedisCommands::test_delitem",
"tests/test_commands.py::TestRedisCommands::test_unlink",
"tests/test_commands.py::TestRedisCommands::test_unlink_with_multiple_keys",
"tests/test_commands.py::TestRedisCommands::test_dump_and_restore",
"tests/test_commands.py::TestRedisCommands::test_dump_and_restore_and_replace",
"tests/test_commands.py::TestRedisCommands::test_dump_and_restore_absttl",
"tests/test_commands.py::TestRedisCommands::test_exists",
"tests/test_commands.py::TestRedisCommands::test_exists_contains",
"tests/test_commands.py::TestRedisCommands::test_expire",
"tests/test_commands.py::TestRedisCommands::test_expireat_datetime",
"tests/test_commands.py::TestRedisCommands::test_expireat_no_key",
"tests/test_commands.py::TestRedisCommands::test_expireat_unixtime",
"tests/test_commands.py::TestRedisCommands::test_get_and_set",
"tests/test_commands.py::TestRedisCommands::test_getdel",
"tests/test_commands.py::TestRedisCommands::test_getex",
"tests/test_commands.py::TestRedisCommands::test_getitem_and_setitem",
"tests/test_commands.py::TestRedisCommands::test_getitem_raises_keyerror_for_missing_key",
"tests/test_commands.py::TestRedisCommands::test_getitem_does_not_raise_keyerror_for_empty_string",
"tests/test_commands.py::TestRedisCommands::test_get_set_bit",
"tests/test_commands.py::TestRedisCommands::test_getrange",
"tests/test_commands.py::TestRedisCommands::test_getset",
"tests/test_commands.py::TestRedisCommands::test_incr",
"tests/test_commands.py::TestRedisCommands::test_incrby",
"tests/test_commands.py::TestRedisCommands::test_incrbyfloat",
"tests/test_commands.py::TestRedisCommands::test_keys",
"tests/test_commands.py::TestRedisCommands::test_mget",
"tests/test_commands.py::TestRedisCommands::test_lmove",
"tests/test_commands.py::TestRedisCommands::test_blmove",
"tests/test_commands.py::TestRedisCommands::test_mset",
"tests/test_commands.py::TestRedisCommands::test_msetnx",
"tests/test_commands.py::TestRedisCommands::test_pexpire",
"tests/test_commands.py::TestRedisCommands::test_pexpireat_datetime",
"tests/test_commands.py::TestRedisCommands::test_pexpireat_no_key",
"tests/test_commands.py::TestRedisCommands::test_pexpireat_unixtime",
"tests/test_commands.py::TestRedisCommands::test_psetex",
"tests/test_commands.py::TestRedisCommands::test_psetex_timedelta",
"tests/test_commands.py::TestRedisCommands::test_pttl",
"tests/test_commands.py::TestRedisCommands::test_pttl_no_key",
"tests/test_commands.py::TestRedisCommands::test_hrandfield",
"tests/test_commands.py::TestRedisCommands::test_randomkey",
"tests/test_commands.py::TestRedisCommands::test_rename",
"tests/test_commands.py::TestRedisCommands::test_renamenx",
"tests/test_commands.py::TestRedisCommands::test_set_nx",
"tests/test_commands.py::TestRedisCommands::test_set_xx",
"tests/test_commands.py::TestRedisCommands::test_set_px",
"tests/test_commands.py::TestRedisCommands::test_set_px_timedelta",
"tests/test_commands.py::TestRedisCommands::test_set_ex",
"tests/test_commands.py::TestRedisCommands::test_set_ex_timedelta",
"tests/test_commands.py::TestRedisCommands::test_set_exat_timedelta",
"tests/test_commands.py::TestRedisCommands::test_set_pxat_timedelta",
"tests/test_commands.py::TestRedisCommands::test_set_multipleoptions",
"tests/test_commands.py::TestRedisCommands::test_set_keepttl",
"tests/test_commands.py::TestRedisCommands::test_set_get",
"tests/test_commands.py::TestRedisCommands::test_setex",
"tests/test_commands.py::TestRedisCommands::test_setnx",
"tests/test_commands.py::TestRedisCommands::test_setrange",
"tests/test_commands.py::TestRedisCommands::test_strlen",
"tests/test_commands.py::TestRedisCommands::test_substr",
"tests/test_commands.py::TestRedisCommands::test_ttl",
"tests/test_commands.py::TestRedisCommands::test_ttl_nokey",
"tests/test_commands.py::TestRedisCommands::test_type",
"tests/test_commands.py::TestRedisCommands::test_blpop",
"tests/test_commands.py::TestRedisCommands::test_brpop",
"tests/test_commands.py::TestRedisCommands::test_brpoplpush",
"tests/test_commands.py::TestRedisCommands::test_brpoplpush_empty_string",
"tests/test_commands.py::TestRedisCommands::test_lindex",
"tests/test_commands.py::TestRedisCommands::test_linsert",
"tests/test_commands.py::TestRedisCommands::test_llen",
"tests/test_commands.py::TestRedisCommands::test_lpop",
"tests/test_commands.py::TestRedisCommands::test_lpop_count",
"tests/test_commands.py::TestRedisCommands::test_lpush",
"tests/test_commands.py::TestRedisCommands::test_lpushx",
"tests/test_commands.py::TestRedisCommands::test_lpushx_with_list",
"tests/test_commands.py::TestRedisCommands::test_lrange",
"tests/test_commands.py::TestRedisCommands::test_lrem",
"tests/test_commands.py::TestRedisCommands::test_lset",
"tests/test_commands.py::TestRedisCommands::test_ltrim",
"tests/test_commands.py::TestRedisCommands::test_rpop",
"tests/test_commands.py::TestRedisCommands::test_rpop_count",
"tests/test_commands.py::TestRedisCommands::test_rpoplpush",
"tests/test_commands.py::TestRedisCommands::test_rpush",
"tests/test_commands.py::TestRedisCommands::test_lpos",
"tests/test_commands.py::TestRedisCommands::test_rpushx",
"tests/test_commands.py::TestRedisCommands::test_scan",
"tests/test_commands.py::TestRedisCommands::test_scan_type",
"tests/test_commands.py::TestRedisCommands::test_scan_iter",
"tests/test_commands.py::TestRedisCommands::test_sscan",
"tests/test_commands.py::TestRedisCommands::test_sscan_iter",
"tests/test_commands.py::TestRedisCommands::test_hscan",
"tests/test_commands.py::TestRedisCommands::test_hscan_iter",
"tests/test_commands.py::TestRedisCommands::test_zscan",
"tests/test_commands.py::TestRedisCommands::test_zscan_iter",
"tests/test_commands.py::TestRedisCommands::test_sadd",
"tests/test_commands.py::TestRedisCommands::test_scard",
"tests/test_commands.py::TestRedisCommands::test_sdiff",
"tests/test_commands.py::TestRedisCommands::test_sdiffstore",
"tests/test_commands.py::TestRedisCommands::test_sinter",
"tests/test_commands.py::TestRedisCommands::test_sinterstore",
"tests/test_commands.py::TestRedisCommands::test_sismember",
"tests/test_commands.py::TestRedisCommands::test_smembers",
"tests/test_commands.py::TestRedisCommands::test_smismember",
"tests/test_commands.py::TestRedisCommands::test_smove",
"tests/test_commands.py::TestRedisCommands::test_spop",
"tests/test_commands.py::TestRedisCommands::test_spop_multi_value",
"tests/test_commands.py::TestRedisCommands::test_srandmember",
"tests/test_commands.py::TestRedisCommands::test_srandmember_multi_value",
"tests/test_commands.py::TestRedisCommands::test_srem",
"tests/test_commands.py::TestRedisCommands::test_sunion",
"tests/test_commands.py::TestRedisCommands::test_sunionstore",
"tests/test_commands.py::TestRedisCommands::test_debug_segfault",
"tests/test_commands.py::TestRedisCommands::test_script_debug",
"tests/test_commands.py::TestRedisCommands::test_zadd",
"tests/test_commands.py::TestRedisCommands::test_zadd_nx",
"tests/test_commands.py::TestRedisCommands::test_zadd_xx",
"tests/test_commands.py::TestRedisCommands::test_zadd_ch",
"tests/test_commands.py::TestRedisCommands::test_zadd_incr",
"tests/test_commands.py::TestRedisCommands::test_zadd_incr_with_xx",
"tests/test_commands.py::TestRedisCommands::test_zadd_gt_lt",
"tests/test_commands.py::TestRedisCommands::test_zcard",
"tests/test_commands.py::TestRedisCommands::test_zcount",
"tests/test_commands.py::TestRedisCommands::test_zdiff",
"tests/test_commands.py::TestRedisCommands::test_zdiffstore",
"tests/test_commands.py::TestRedisCommands::test_zincrby",
"tests/test_commands.py::TestRedisCommands::test_zlexcount",
"tests/test_commands.py::TestRedisCommands::test_zinter",
"tests/test_commands.py::TestRedisCommands::test_zinterstore_sum",
"tests/test_commands.py::TestRedisCommands::test_zinterstore_max",
"tests/test_commands.py::TestRedisCommands::test_zinterstore_min",
"tests/test_commands.py::TestRedisCommands::test_zinterstore_with_weight",
"tests/test_commands.py::TestRedisCommands::test_zpopmax",
"tests/test_commands.py::TestRedisCommands::test_zpopmin",
"tests/test_commands.py::TestRedisCommands::test_zrandemember",
"tests/test_commands.py::TestRedisCommands::test_bzpopmax",
"tests/test_commands.py::TestRedisCommands::test_bzpopmin",
"tests/test_commands.py::TestRedisCommands::test_zrange",
"tests/test_commands.py::TestRedisCommands::test_zrange_errors",
"tests/test_commands.py::TestRedisCommands::test_zrange_params",
"tests/test_commands.py::TestRedisCommands::test_zrangestore",
"tests/test_commands.py::TestRedisCommands::test_zrangebylex",
"tests/test_commands.py::TestRedisCommands::test_zrevrangebylex",
"tests/test_commands.py::TestRedisCommands::test_zrangebyscore",
"tests/test_commands.py::TestRedisCommands::test_zrank",
"tests/test_commands.py::TestRedisCommands::test_zrem",
"tests/test_commands.py::TestRedisCommands::test_zrem_multiple_keys",
"tests/test_commands.py::TestRedisCommands::test_zremrangebylex",
"tests/test_commands.py::TestRedisCommands::test_zremrangebyrank",
"tests/test_commands.py::TestRedisCommands::test_zremrangebyscore",
"tests/test_commands.py::TestRedisCommands::test_zrevrange",
"tests/test_commands.py::TestRedisCommands::test_zrevrangebyscore",
"tests/test_commands.py::TestRedisCommands::test_zrevrank",
"tests/test_commands.py::TestRedisCommands::test_zscore",
"tests/test_commands.py::TestRedisCommands::test_zunion",
"tests/test_commands.py::TestRedisCommands::test_zunionstore_sum",
"tests/test_commands.py::TestRedisCommands::test_zunionstore_max",
"tests/test_commands.py::TestRedisCommands::test_zunionstore_min",
"tests/test_commands.py::TestRedisCommands::test_zunionstore_with_weight",
"tests/test_commands.py::TestRedisCommands::test_zmscore",
"tests/test_commands.py::TestRedisCommands::test_pfadd",
"tests/test_commands.py::TestRedisCommands::test_pfcount",
"tests/test_commands.py::TestRedisCommands::test_pfmerge",
"tests/test_commands.py::TestRedisCommands::test_hget_and_hset",
"tests/test_commands.py::TestRedisCommands::test_hset_with_multi_key_values",
"tests/test_commands.py::TestRedisCommands::test_hset_with_key_values_passed_as_list",
"tests/test_commands.py::TestRedisCommands::test_hset_without_data",
"tests/test_commands.py::TestRedisCommands::test_hdel",
"tests/test_commands.py::TestRedisCommands::test_hexists",
"tests/test_commands.py::TestRedisCommands::test_hgetall",
"tests/test_commands.py::TestRedisCommands::test_hincrby",
"tests/test_commands.py::TestRedisCommands::test_hincrbyfloat",
"tests/test_commands.py::TestRedisCommands::test_hkeys",
"tests/test_commands.py::TestRedisCommands::test_hlen",
"tests/test_commands.py::TestRedisCommands::test_hmget",
"tests/test_commands.py::TestRedisCommands::test_hmset",
"tests/test_commands.py::TestRedisCommands::test_hsetnx",
"tests/test_commands.py::TestRedisCommands::test_hvals",
"tests/test_commands.py::TestRedisCommands::test_hstrlen",
"tests/test_commands.py::TestRedisCommands::test_sort_basic",
"tests/test_commands.py::TestRedisCommands::test_sort_limited",
"tests/test_commands.py::TestRedisCommands::test_sort_by",
"tests/test_commands.py::TestRedisCommands::test_sort_get",
"tests/test_commands.py::TestRedisCommands::test_sort_get_multi",
"tests/test_commands.py::TestRedisCommands::test_sort_get_groups_two",
"tests/test_commands.py::TestRedisCommands::test_sort_groups_string_get",
"tests/test_commands.py::TestRedisCommands::test_sort_groups_just_one_get",
"tests/test_commands.py::TestRedisCommands::test_sort_groups_no_get",
"tests/test_commands.py::TestRedisCommands::test_sort_groups_three_gets",
"tests/test_commands.py::TestRedisCommands::test_sort_desc",
"tests/test_commands.py::TestRedisCommands::test_sort_alpha",
"tests/test_commands.py::TestRedisCommands::test_sort_store",
"tests/test_commands.py::TestRedisCommands::test_sort_all_options",
"tests/test_commands.py::TestRedisCommands::test_sort_issue_924",
"tests/test_commands.py::TestRedisCommands::test_cluster_addslots",
"tests/test_commands.py::TestRedisCommands::test_cluster_count_failure_reports",
"tests/test_commands.py::TestRedisCommands::test_cluster_countkeysinslot",
"tests/test_commands.py::TestRedisCommands::test_cluster_delslots",
"tests/test_commands.py::TestRedisCommands::test_cluster_failover",
"tests/test_commands.py::TestRedisCommands::test_cluster_forget",
"tests/test_commands.py::TestRedisCommands::test_cluster_info",
"tests/test_commands.py::TestRedisCommands::test_cluster_keyslot",
"tests/test_commands.py::TestRedisCommands::test_cluster_meet",
"tests/test_commands.py::TestRedisCommands::test_cluster_nodes",
"tests/test_commands.py::TestRedisCommands::test_cluster_replicate",
"tests/test_commands.py::TestRedisCommands::test_cluster_reset",
"tests/test_commands.py::TestRedisCommands::test_cluster_saveconfig",
"tests/test_commands.py::TestRedisCommands::test_cluster_setslot",
"tests/test_commands.py::TestRedisCommands::test_cluster_slaves",
"tests/test_commands.py::TestRedisCommands::test_readonly_invalid_cluster_state",
"tests/test_commands.py::TestRedisCommands::test_readonly",
"tests/test_commands.py::TestRedisCommands::test_geoadd",
"tests/test_commands.py::TestRedisCommands::test_geoadd_nx",
"tests/test_commands.py::TestRedisCommands::test_geoadd_xx",
"tests/test_commands.py::TestRedisCommands::test_geoadd_ch",
"tests/test_commands.py::TestRedisCommands::test_geoadd_invalid_params",
"tests/test_commands.py::TestRedisCommands::test_geodist",
"tests/test_commands.py::TestRedisCommands::test_geodist_units",
"tests/test_commands.py::TestRedisCommands::test_geodist_missing_one_member",
"tests/test_commands.py::TestRedisCommands::test_geodist_invalid_units",
"tests/test_commands.py::TestRedisCommands::test_geohash",
"tests/test_commands.py::TestRedisCommands::test_geopos",
"tests/test_commands.py::TestRedisCommands::test_geopos_no_value",
"tests/test_commands.py::TestRedisCommands::test_geosearch",
"tests/test_commands.py::TestRedisCommands::test_geosearch_member",
"tests/test_commands.py::TestRedisCommands::test_geosearch_sort",
"tests/test_commands.py::TestRedisCommands::test_geosearch_with",
"tests/test_commands.py::TestRedisCommands::test_geosearch_negative",
"tests/test_commands.py::TestRedisCommands::test_geosearchstore",
"tests/test_commands.py::TestRedisCommands::test_geosearchstore_dist",
"tests/test_commands.py::TestRedisCommands::test_georadius",
"tests/test_commands.py::TestRedisCommands::test_georadius_no_values",
"tests/test_commands.py::TestRedisCommands::test_georadius_units",
"tests/test_commands.py::TestRedisCommands::test_georadius_with",
"tests/test_commands.py::TestRedisCommands::test_georadius_count",
"tests/test_commands.py::TestRedisCommands::test_georadius_sort",
"tests/test_commands.py::TestRedisCommands::test_georadius_store",
"tests/test_commands.py::TestRedisCommands::test_georadius_store_dist",
"tests/test_commands.py::TestRedisCommands::test_georadiusmember",
"tests/test_commands.py::TestRedisCommands::test_georadiusmember_count",
"tests/test_commands.py::TestRedisCommands::test_xack",
"tests/test_commands.py::TestRedisCommands::test_xadd",
"tests/test_commands.py::TestRedisCommands::test_xadd_nomkstream",
"tests/test_commands.py::TestRedisCommands::test_xadd_minlen_and_limit",
"tests/test_commands.py::TestRedisCommands::test_xautoclaim",
"tests/test_commands.py::TestRedisCommands::test_xautoclaim_negative",
"tests/test_commands.py::TestRedisCommands::test_xclaim",
"tests/test_commands.py::TestRedisCommands::test_xdel",
"tests/test_commands.py::TestRedisCommands::test_xgroup_delconsumer",
"tests/test_commands.py::TestRedisCommands::test_xgroup_createconsumer",
"tests/test_commands.py::TestRedisCommands::test_xgroup_destroy",
"tests/test_commands.py::TestRedisCommands::test_xinfo_stream",
"tests/test_commands.py::TestRedisCommands::test_xinfo_stream_full",
"tests/test_commands.py::TestRedisCommands::test_xlen",
"tests/test_commands.py::TestRedisCommands::test_xpending",
"tests/test_commands.py::TestRedisCommands::test_xpending_range",
"tests/test_commands.py::TestRedisCommands::test_xpending_range_idle",
"tests/test_commands.py::TestRedisCommands::test_xpending_range_negative",
"tests/test_commands.py::TestRedisCommands::test_xrange",
"tests/test_commands.py::TestRedisCommands::test_xread",
"tests/test_commands.py::TestRedisCommands::test_xreadgroup",
"tests/test_commands.py::TestRedisCommands::test_xrevrange",
"tests/test_commands.py::TestRedisCommands::test_xtrim",
"tests/test_commands.py::TestRedisCommands::test_xtrim_minlen_and_length_args",
"tests/test_commands.py::TestRedisCommands::test_bitfield_operations",
"tests/test_commands.py::TestRedisCommands::test_memory_help",
"tests/test_commands.py::TestRedisCommands::test_memory_doctor",
"tests/test_commands.py::TestRedisCommands::test_memory_malloc_stats",
"tests/test_commands.py::TestRedisCommands::test_memory_stats",
"tests/test_commands.py::TestRedisCommands::test_memory_usage",
"tests/test_commands.py::TestRedisCommands::test_module_list",
"tests/test_commands.py::TestRedisCommands::test_command_count",
"tests/test_commands.py::TestRedisCommands::test_command_getkeys",
"tests/test_commands.py::TestRedisCommands::test_command",
"tests/test_commands.py::TestRedisCommands::test_module",
"tests/test_commands.py::TestRedisCommands::test_restore",
"tests/test_commands.py::TestRedisCommands::test_restore_idletime",
"tests/test_commands.py::TestRedisCommands::test_restore_frequency",
"tests/test_commands.py::TestRedisCommands::test_replicaof",
"tests/test_commands.py::TestRedisCommands::test_sync",
"tests/test_commands.py::TestRedisCommands::test_psync",
"tests/test_commands.py::TestBinarySave::test_binary_get_set",
"tests/test_commands.py::TestBinarySave::test_binary_lists",
"tests/test_commands.py::TestBinarySave::test_22_info",
"tests/test_commands.py::TestBinarySave::test_large_responses",
"tests/test_commands.py::TestBinarySave::test_floating_point_encoding"
] | [
"tests/test_commands.py::TestRedisCommands::test_expiretime"
] | Python | [] | [] |
|
redis/redis-py | 1,861 | redis__redis-py-1861 | [
"1807"
] | c4e408880c0fbfe714cd9ad4e1e6b908f00be9b5 | diff --git a/redis/commands/core.py b/redis/commands/core.py
--- a/redis/commands/core.py
+++ b/redis/commands/core.py
@@ -1782,6 +1782,15 @@ def pexpireat(self, name: KeyT, when: AbsExpiryT) -> ResponseT:
when = int(time.mktime(when.timetuple())) * 1000 + ms
return self.execute_command("PEXPIREAT", name, when)
+ def pexpiretime(self, key: str) -> int:
+ """
+ Returns the absolute Unix timestamp (since January 1, 1970) in milliseconds
+ at which the given key will expire.
+
+ For more information check https://redis.io/commands/pexpiretime
+ """
+ return self.execute_command("PEXPIRETIME", key)
+
def psetex(
self,
name: KeyT,
| diff --git a/tests/test_commands.py b/tests/test_commands.py
--- a/tests/test_commands.py
+++ b/tests/test_commands.py
@@ -1248,6 +1248,12 @@ def test_pexpireat_unixtime(self, r):
assert r.pexpireat("a", expire_at_seconds) is True
assert 0 < r.pttl("a") <= 61000
+ @skip_if_server_version_lt("7.0.0")
+ def test_pexpiretime(self, r):
+ r.set("a", "foo")
+ r.pexpireat("a", 33177117420000)
+ assert r.pexpiretime("a") == 33177117420000
+
@skip_if_server_version_lt("2.6.0")
def test_psetex(self, r):
assert r.psetex("a", 1000, "value")
| Add support for PEXPIRETIME
| "2022-01-05T11:01:42Z" | 4.2 | [
"tests/test_commands.py::TestResponseCallbacks::test_response_callbacks",
"tests/test_commands.py::TestResponseCallbacks::test_case_insensitive_command_names",
"tests/test_commands.py::TestRedisCommands::test_auth",
"tests/test_commands.py::TestRedisCommands::test_command_on_invalid_key_type",
"tests/test_commands.py::TestRedisCommands::test_acl_cat_no_category",
"tests/test_commands.py::TestRedisCommands::test_acl_cat_with_category",
"tests/test_commands.py::TestRedisCommands::test_acl_genpass",
"tests/test_commands.py::TestRedisCommands::test_acl_help",
"tests/test_commands.py::TestRedisCommands::test_acl_list",
"tests/test_commands.py::TestRedisCommands::test_acl_log",
"tests/test_commands.py::TestRedisCommands::test_acl_setuser_categories_without_prefix_fails",
"tests/test_commands.py::TestRedisCommands::test_acl_setuser_commands_without_prefix_fails",
"tests/test_commands.py::TestRedisCommands::test_acl_setuser_add_passwords_and_nopass_fails",
"tests/test_commands.py::TestRedisCommands::test_acl_users",
"tests/test_commands.py::TestRedisCommands::test_acl_whoami",
"tests/test_commands.py::TestRedisCommands::test_client_list",
"tests/test_commands.py::TestRedisCommands::test_client_info",
"tests/test_commands.py::TestRedisCommands::test_client_list_types_not_replica",
"tests/test_commands.py::TestRedisCommands::test_client_list_replica",
"tests/test_commands.py::TestRedisCommands::test_client_list_client_id",
"tests/test_commands.py::TestRedisCommands::test_client_id",
"tests/test_commands.py::TestRedisCommands::test_client_trackinginfo",
"tests/test_commands.py::TestRedisCommands::test_client_tracking",
"tests/test_commands.py::TestRedisCommands::test_client_unblock",
"tests/test_commands.py::TestRedisCommands::test_client_getname",
"tests/test_commands.py::TestRedisCommands::test_client_setname",
"tests/test_commands.py::TestRedisCommands::test_client_kill",
"tests/test_commands.py::TestRedisCommands::test_client_kill_filter_invalid_params",
"tests/test_commands.py::TestRedisCommands::test_client_kill_filter_by_id",
"tests/test_commands.py::TestRedisCommands::test_client_kill_filter_by_addr",
"tests/test_commands.py::TestRedisCommands::test_client_list_after_client_setname",
"tests/test_commands.py::TestRedisCommands::test_client_kill_filter_by_laddr",
"tests/test_commands.py::TestRedisCommands::test_client_kill_filter_by_user",
"tests/test_commands.py::TestRedisCommands::test_client_pause",
"tests/test_commands.py::TestRedisCommands::test_client_pause_all",
"tests/test_commands.py::TestRedisCommands::test_client_unpause",
"tests/test_commands.py::TestRedisCommands::test_client_reply",
"tests/test_commands.py::TestRedisCommands::test_client_getredir",
"tests/test_commands.py::TestRedisCommands::test_hello_notI_implemented",
"tests/test_commands.py::TestRedisCommands::test_config_get",
"tests/test_commands.py::TestRedisCommands::test_config_resetstat",
"tests/test_commands.py::TestRedisCommands::test_config_set",
"tests/test_commands.py::TestRedisCommands::test_failover",
"tests/test_commands.py::TestRedisCommands::test_dbsize",
"tests/test_commands.py::TestRedisCommands::test_echo",
"tests/test_commands.py::TestRedisCommands::test_info",
"tests/test_commands.py::TestRedisCommands::test_lastsave",
"tests/test_commands.py::TestRedisCommands::test_lolwut",
"tests/test_commands.py::TestRedisCommands::test_reset",
"tests/test_commands.py::TestRedisCommands::test_object",
"tests/test_commands.py::TestRedisCommands::test_ping",
"tests/test_commands.py::TestRedisCommands::test_quit",
"tests/test_commands.py::TestRedisCommands::test_role",
"tests/test_commands.py::TestRedisCommands::test_select",
"tests/test_commands.py::TestRedisCommands::test_slowlog_get",
"tests/test_commands.py::TestRedisCommands::test_slowlog_get_limit",
"tests/test_commands.py::TestRedisCommands::test_slowlog_length",
"tests/test_commands.py::TestRedisCommands::test_time",
"tests/test_commands.py::TestRedisCommands::test_bgsave",
"tests/test_commands.py::TestRedisCommands::test_append",
"tests/test_commands.py::TestRedisCommands::test_bitcount",
"tests/test_commands.py::TestRedisCommands::test_bitop_not_empty_string",
"tests/test_commands.py::TestRedisCommands::test_bitop_not",
"tests/test_commands.py::TestRedisCommands::test_bitop_not_in_place",
"tests/test_commands.py::TestRedisCommands::test_bitop_single_string",
"tests/test_commands.py::TestRedisCommands::test_bitop_string_operands",
"tests/test_commands.py::TestRedisCommands::test_bitpos",
"tests/test_commands.py::TestRedisCommands::test_bitpos_wrong_arguments",
"tests/test_commands.py::TestRedisCommands::test_copy",
"tests/test_commands.py::TestRedisCommands::test_copy_and_replace",
"tests/test_commands.py::TestRedisCommands::test_copy_to_another_database",
"tests/test_commands.py::TestRedisCommands::test_decr",
"tests/test_commands.py::TestRedisCommands::test_decrby",
"tests/test_commands.py::TestRedisCommands::test_delete",
"tests/test_commands.py::TestRedisCommands::test_delete_with_multiple_keys",
"tests/test_commands.py::TestRedisCommands::test_delitem",
"tests/test_commands.py::TestRedisCommands::test_unlink",
"tests/test_commands.py::TestRedisCommands::test_unlink_with_multiple_keys",
"tests/test_commands.py::TestRedisCommands::test_dump_and_restore",
"tests/test_commands.py::TestRedisCommands::test_dump_and_restore_and_replace",
"tests/test_commands.py::TestRedisCommands::test_dump_and_restore_absttl",
"tests/test_commands.py::TestRedisCommands::test_exists",
"tests/test_commands.py::TestRedisCommands::test_exists_contains",
"tests/test_commands.py::TestRedisCommands::test_expire",
"tests/test_commands.py::TestRedisCommands::test_expireat_datetime",
"tests/test_commands.py::TestRedisCommands::test_expireat_no_key",
"tests/test_commands.py::TestRedisCommands::test_expireat_unixtime",
"tests/test_commands.py::TestRedisCommands::test_get_and_set",
"tests/test_commands.py::TestRedisCommands::test_getdel",
"tests/test_commands.py::TestRedisCommands::test_getex",
"tests/test_commands.py::TestRedisCommands::test_getitem_and_setitem",
"tests/test_commands.py::TestRedisCommands::test_getitem_raises_keyerror_for_missing_key",
"tests/test_commands.py::TestRedisCommands::test_getitem_does_not_raise_keyerror_for_empty_string",
"tests/test_commands.py::TestRedisCommands::test_get_set_bit",
"tests/test_commands.py::TestRedisCommands::test_getrange",
"tests/test_commands.py::TestRedisCommands::test_getset",
"tests/test_commands.py::TestRedisCommands::test_incr",
"tests/test_commands.py::TestRedisCommands::test_incrby",
"tests/test_commands.py::TestRedisCommands::test_incrbyfloat",
"tests/test_commands.py::TestRedisCommands::test_keys",
"tests/test_commands.py::TestRedisCommands::test_mget",
"tests/test_commands.py::TestRedisCommands::test_lmove",
"tests/test_commands.py::TestRedisCommands::test_blmove",
"tests/test_commands.py::TestRedisCommands::test_mset",
"tests/test_commands.py::TestRedisCommands::test_msetnx",
"tests/test_commands.py::TestRedisCommands::test_pexpire",
"tests/test_commands.py::TestRedisCommands::test_pexpireat_datetime",
"tests/test_commands.py::TestRedisCommands::test_pexpireat_no_key",
"tests/test_commands.py::TestRedisCommands::test_pexpireat_unixtime",
"tests/test_commands.py::TestRedisCommands::test_psetex",
"tests/test_commands.py::TestRedisCommands::test_psetex_timedelta",
"tests/test_commands.py::TestRedisCommands::test_pttl",
"tests/test_commands.py::TestRedisCommands::test_pttl_no_key",
"tests/test_commands.py::TestRedisCommands::test_hrandfield",
"tests/test_commands.py::TestRedisCommands::test_randomkey",
"tests/test_commands.py::TestRedisCommands::test_rename",
"tests/test_commands.py::TestRedisCommands::test_renamenx",
"tests/test_commands.py::TestRedisCommands::test_set_nx",
"tests/test_commands.py::TestRedisCommands::test_set_xx",
"tests/test_commands.py::TestRedisCommands::test_set_px",
"tests/test_commands.py::TestRedisCommands::test_set_px_timedelta",
"tests/test_commands.py::TestRedisCommands::test_set_ex",
"tests/test_commands.py::TestRedisCommands::test_set_ex_timedelta",
"tests/test_commands.py::TestRedisCommands::test_set_exat_timedelta",
"tests/test_commands.py::TestRedisCommands::test_set_pxat_timedelta",
"tests/test_commands.py::TestRedisCommands::test_set_multipleoptions",
"tests/test_commands.py::TestRedisCommands::test_set_keepttl",
"tests/test_commands.py::TestRedisCommands::test_set_get",
"tests/test_commands.py::TestRedisCommands::test_setex",
"tests/test_commands.py::TestRedisCommands::test_setnx",
"tests/test_commands.py::TestRedisCommands::test_setrange",
"tests/test_commands.py::TestRedisCommands::test_strlen",
"tests/test_commands.py::TestRedisCommands::test_substr",
"tests/test_commands.py::TestRedisCommands::test_ttl",
"tests/test_commands.py::TestRedisCommands::test_ttl_nokey",
"tests/test_commands.py::TestRedisCommands::test_type",
"tests/test_commands.py::TestRedisCommands::test_blpop",
"tests/test_commands.py::TestRedisCommands::test_brpop",
"tests/test_commands.py::TestRedisCommands::test_brpoplpush",
"tests/test_commands.py::TestRedisCommands::test_brpoplpush_empty_string",
"tests/test_commands.py::TestRedisCommands::test_lindex",
"tests/test_commands.py::TestRedisCommands::test_linsert",
"tests/test_commands.py::TestRedisCommands::test_llen",
"tests/test_commands.py::TestRedisCommands::test_lpop",
"tests/test_commands.py::TestRedisCommands::test_lpop_count",
"tests/test_commands.py::TestRedisCommands::test_lpush",
"tests/test_commands.py::TestRedisCommands::test_lpushx",
"tests/test_commands.py::TestRedisCommands::test_lpushx_with_list",
"tests/test_commands.py::TestRedisCommands::test_lrange",
"tests/test_commands.py::TestRedisCommands::test_lrem",
"tests/test_commands.py::TestRedisCommands::test_lset",
"tests/test_commands.py::TestRedisCommands::test_ltrim",
"tests/test_commands.py::TestRedisCommands::test_rpop",
"tests/test_commands.py::TestRedisCommands::test_rpop_count",
"tests/test_commands.py::TestRedisCommands::test_rpoplpush",
"tests/test_commands.py::TestRedisCommands::test_rpush",
"tests/test_commands.py::TestRedisCommands::test_lpos",
"tests/test_commands.py::TestRedisCommands::test_rpushx",
"tests/test_commands.py::TestRedisCommands::test_scan",
"tests/test_commands.py::TestRedisCommands::test_scan_type",
"tests/test_commands.py::TestRedisCommands::test_scan_iter",
"tests/test_commands.py::TestRedisCommands::test_sscan",
"tests/test_commands.py::TestRedisCommands::test_sscan_iter",
"tests/test_commands.py::TestRedisCommands::test_hscan",
"tests/test_commands.py::TestRedisCommands::test_hscan_iter",
"tests/test_commands.py::TestRedisCommands::test_zscan",
"tests/test_commands.py::TestRedisCommands::test_zscan_iter",
"tests/test_commands.py::TestRedisCommands::test_sadd",
"tests/test_commands.py::TestRedisCommands::test_scard",
"tests/test_commands.py::TestRedisCommands::test_sdiff",
"tests/test_commands.py::TestRedisCommands::test_sdiffstore",
"tests/test_commands.py::TestRedisCommands::test_sinter",
"tests/test_commands.py::TestRedisCommands::test_sinterstore",
"tests/test_commands.py::TestRedisCommands::test_sismember",
"tests/test_commands.py::TestRedisCommands::test_smembers",
"tests/test_commands.py::TestRedisCommands::test_smismember",
"tests/test_commands.py::TestRedisCommands::test_smove",
"tests/test_commands.py::TestRedisCommands::test_spop",
"tests/test_commands.py::TestRedisCommands::test_spop_multi_value",
"tests/test_commands.py::TestRedisCommands::test_srandmember",
"tests/test_commands.py::TestRedisCommands::test_srandmember_multi_value",
"tests/test_commands.py::TestRedisCommands::test_srem",
"tests/test_commands.py::TestRedisCommands::test_sunion",
"tests/test_commands.py::TestRedisCommands::test_sunionstore",
"tests/test_commands.py::TestRedisCommands::test_debug_segfault",
"tests/test_commands.py::TestRedisCommands::test_script_debug",
"tests/test_commands.py::TestRedisCommands::test_zadd",
"tests/test_commands.py::TestRedisCommands::test_zadd_nx",
"tests/test_commands.py::TestRedisCommands::test_zadd_xx",
"tests/test_commands.py::TestRedisCommands::test_zadd_ch",
"tests/test_commands.py::TestRedisCommands::test_zadd_incr",
"tests/test_commands.py::TestRedisCommands::test_zadd_incr_with_xx",
"tests/test_commands.py::TestRedisCommands::test_zadd_gt_lt",
"tests/test_commands.py::TestRedisCommands::test_zcard",
"tests/test_commands.py::TestRedisCommands::test_zcount",
"tests/test_commands.py::TestRedisCommands::test_zdiff",
"tests/test_commands.py::TestRedisCommands::test_zdiffstore",
"tests/test_commands.py::TestRedisCommands::test_zincrby",
"tests/test_commands.py::TestRedisCommands::test_zlexcount",
"tests/test_commands.py::TestRedisCommands::test_zinter",
"tests/test_commands.py::TestRedisCommands::test_zinterstore_sum",
"tests/test_commands.py::TestRedisCommands::test_zinterstore_max",
"tests/test_commands.py::TestRedisCommands::test_zinterstore_min",
"tests/test_commands.py::TestRedisCommands::test_zinterstore_with_weight",
"tests/test_commands.py::TestRedisCommands::test_zpopmax",
"tests/test_commands.py::TestRedisCommands::test_zpopmin",
"tests/test_commands.py::TestRedisCommands::test_zrandemember",
"tests/test_commands.py::TestRedisCommands::test_bzpopmax",
"tests/test_commands.py::TestRedisCommands::test_bzpopmin",
"tests/test_commands.py::TestRedisCommands::test_zrange",
"tests/test_commands.py::TestRedisCommands::test_zrange_errors",
"tests/test_commands.py::TestRedisCommands::test_zrange_params",
"tests/test_commands.py::TestRedisCommands::test_zrangestore",
"tests/test_commands.py::TestRedisCommands::test_zrangebylex",
"tests/test_commands.py::TestRedisCommands::test_zrevrangebylex",
"tests/test_commands.py::TestRedisCommands::test_zrangebyscore",
"tests/test_commands.py::TestRedisCommands::test_zrank",
"tests/test_commands.py::TestRedisCommands::test_zrem",
"tests/test_commands.py::TestRedisCommands::test_zrem_multiple_keys",
"tests/test_commands.py::TestRedisCommands::test_zremrangebylex",
"tests/test_commands.py::TestRedisCommands::test_zremrangebyrank",
"tests/test_commands.py::TestRedisCommands::test_zremrangebyscore",
"tests/test_commands.py::TestRedisCommands::test_zrevrange",
"tests/test_commands.py::TestRedisCommands::test_zrevrangebyscore",
"tests/test_commands.py::TestRedisCommands::test_zrevrank",
"tests/test_commands.py::TestRedisCommands::test_zscore",
"tests/test_commands.py::TestRedisCommands::test_zunion",
"tests/test_commands.py::TestRedisCommands::test_zunionstore_sum",
"tests/test_commands.py::TestRedisCommands::test_zunionstore_max",
"tests/test_commands.py::TestRedisCommands::test_zunionstore_min",
"tests/test_commands.py::TestRedisCommands::test_zunionstore_with_weight",
"tests/test_commands.py::TestRedisCommands::test_zmscore",
"tests/test_commands.py::TestRedisCommands::test_pfadd",
"tests/test_commands.py::TestRedisCommands::test_pfcount",
"tests/test_commands.py::TestRedisCommands::test_pfmerge",
"tests/test_commands.py::TestRedisCommands::test_hget_and_hset",
"tests/test_commands.py::TestRedisCommands::test_hset_with_multi_key_values",
"tests/test_commands.py::TestRedisCommands::test_hset_with_key_values_passed_as_list",
"tests/test_commands.py::TestRedisCommands::test_hset_without_data",
"tests/test_commands.py::TestRedisCommands::test_hdel",
"tests/test_commands.py::TestRedisCommands::test_hexists",
"tests/test_commands.py::TestRedisCommands::test_hgetall",
"tests/test_commands.py::TestRedisCommands::test_hincrby",
"tests/test_commands.py::TestRedisCommands::test_hincrbyfloat",
"tests/test_commands.py::TestRedisCommands::test_hkeys",
"tests/test_commands.py::TestRedisCommands::test_hlen",
"tests/test_commands.py::TestRedisCommands::test_hmget",
"tests/test_commands.py::TestRedisCommands::test_hmset",
"tests/test_commands.py::TestRedisCommands::test_hsetnx",
"tests/test_commands.py::TestRedisCommands::test_hvals",
"tests/test_commands.py::TestRedisCommands::test_hstrlen",
"tests/test_commands.py::TestRedisCommands::test_sort_basic",
"tests/test_commands.py::TestRedisCommands::test_sort_limited",
"tests/test_commands.py::TestRedisCommands::test_sort_by",
"tests/test_commands.py::TestRedisCommands::test_sort_get",
"tests/test_commands.py::TestRedisCommands::test_sort_get_multi",
"tests/test_commands.py::TestRedisCommands::test_sort_get_groups_two",
"tests/test_commands.py::TestRedisCommands::test_sort_groups_string_get",
"tests/test_commands.py::TestRedisCommands::test_sort_groups_just_one_get",
"tests/test_commands.py::TestRedisCommands::test_sort_groups_no_get",
"tests/test_commands.py::TestRedisCommands::test_sort_groups_three_gets",
"tests/test_commands.py::TestRedisCommands::test_sort_desc",
"tests/test_commands.py::TestRedisCommands::test_sort_alpha",
"tests/test_commands.py::TestRedisCommands::test_sort_store",
"tests/test_commands.py::TestRedisCommands::test_sort_all_options",
"tests/test_commands.py::TestRedisCommands::test_sort_issue_924",
"tests/test_commands.py::TestRedisCommands::test_cluster_addslots",
"tests/test_commands.py::TestRedisCommands::test_cluster_count_failure_reports",
"tests/test_commands.py::TestRedisCommands::test_cluster_countkeysinslot",
"tests/test_commands.py::TestRedisCommands::test_cluster_delslots",
"tests/test_commands.py::TestRedisCommands::test_cluster_failover",
"tests/test_commands.py::TestRedisCommands::test_cluster_forget",
"tests/test_commands.py::TestRedisCommands::test_cluster_info",
"tests/test_commands.py::TestRedisCommands::test_cluster_keyslot",
"tests/test_commands.py::TestRedisCommands::test_cluster_meet",
"tests/test_commands.py::TestRedisCommands::test_cluster_nodes",
"tests/test_commands.py::TestRedisCommands::test_cluster_replicate",
"tests/test_commands.py::TestRedisCommands::test_cluster_reset",
"tests/test_commands.py::TestRedisCommands::test_cluster_saveconfig",
"tests/test_commands.py::TestRedisCommands::test_cluster_setslot",
"tests/test_commands.py::TestRedisCommands::test_cluster_slaves",
"tests/test_commands.py::TestRedisCommands::test_readonly_invalid_cluster_state",
"tests/test_commands.py::TestRedisCommands::test_readonly",
"tests/test_commands.py::TestRedisCommands::test_geoadd",
"tests/test_commands.py::TestRedisCommands::test_geoadd_nx",
"tests/test_commands.py::TestRedisCommands::test_geoadd_xx",
"tests/test_commands.py::TestRedisCommands::test_geoadd_ch",
"tests/test_commands.py::TestRedisCommands::test_geoadd_invalid_params",
"tests/test_commands.py::TestRedisCommands::test_geodist",
"tests/test_commands.py::TestRedisCommands::test_geodist_units",
"tests/test_commands.py::TestRedisCommands::test_geodist_missing_one_member",
"tests/test_commands.py::TestRedisCommands::test_geodist_invalid_units",
"tests/test_commands.py::TestRedisCommands::test_geohash",
"tests/test_commands.py::TestRedisCommands::test_geopos",
"tests/test_commands.py::TestRedisCommands::test_geopos_no_value",
"tests/test_commands.py::TestRedisCommands::test_geosearch",
"tests/test_commands.py::TestRedisCommands::test_geosearch_member",
"tests/test_commands.py::TestRedisCommands::test_geosearch_sort",
"tests/test_commands.py::TestRedisCommands::test_geosearch_with",
"tests/test_commands.py::TestRedisCommands::test_geosearch_negative",
"tests/test_commands.py::TestRedisCommands::test_geosearchstore",
"tests/test_commands.py::TestRedisCommands::test_geosearchstore_dist",
"tests/test_commands.py::TestRedisCommands::test_georadius",
"tests/test_commands.py::TestRedisCommands::test_georadius_no_values",
"tests/test_commands.py::TestRedisCommands::test_georadius_units",
"tests/test_commands.py::TestRedisCommands::test_georadius_with",
"tests/test_commands.py::TestRedisCommands::test_georadius_count",
"tests/test_commands.py::TestRedisCommands::test_georadius_sort",
"tests/test_commands.py::TestRedisCommands::test_georadius_store",
"tests/test_commands.py::TestRedisCommands::test_georadius_store_dist",
"tests/test_commands.py::TestRedisCommands::test_georadiusmember",
"tests/test_commands.py::TestRedisCommands::test_georadiusmember_count",
"tests/test_commands.py::TestRedisCommands::test_xack",
"tests/test_commands.py::TestRedisCommands::test_xadd",
"tests/test_commands.py::TestRedisCommands::test_xadd_nomkstream",
"tests/test_commands.py::TestRedisCommands::test_xadd_minlen_and_limit",
"tests/test_commands.py::TestRedisCommands::test_xautoclaim",
"tests/test_commands.py::TestRedisCommands::test_xautoclaim_negative",
"tests/test_commands.py::TestRedisCommands::test_xclaim",
"tests/test_commands.py::TestRedisCommands::test_xdel",
"tests/test_commands.py::TestRedisCommands::test_xgroup_delconsumer",
"tests/test_commands.py::TestRedisCommands::test_xgroup_createconsumer",
"tests/test_commands.py::TestRedisCommands::test_xgroup_destroy",
"tests/test_commands.py::TestRedisCommands::test_xinfo_stream",
"tests/test_commands.py::TestRedisCommands::test_xinfo_stream_full",
"tests/test_commands.py::TestRedisCommands::test_xlen",
"tests/test_commands.py::TestRedisCommands::test_xpending",
"tests/test_commands.py::TestRedisCommands::test_xpending_range",
"tests/test_commands.py::TestRedisCommands::test_xpending_range_idle",
"tests/test_commands.py::TestRedisCommands::test_xpending_range_negative",
"tests/test_commands.py::TestRedisCommands::test_xrange",
"tests/test_commands.py::TestRedisCommands::test_xread",
"tests/test_commands.py::TestRedisCommands::test_xreadgroup",
"tests/test_commands.py::TestRedisCommands::test_xrevrange",
"tests/test_commands.py::TestRedisCommands::test_xtrim",
"tests/test_commands.py::TestRedisCommands::test_xtrim_minlen_and_length_args",
"tests/test_commands.py::TestRedisCommands::test_bitfield_operations",
"tests/test_commands.py::TestRedisCommands::test_memory_help",
"tests/test_commands.py::TestRedisCommands::test_memory_doctor",
"tests/test_commands.py::TestRedisCommands::test_memory_malloc_stats",
"tests/test_commands.py::TestRedisCommands::test_memory_stats",
"tests/test_commands.py::TestRedisCommands::test_memory_usage",
"tests/test_commands.py::TestRedisCommands::test_module_list",
"tests/test_commands.py::TestRedisCommands::test_command_count",
"tests/test_commands.py::TestRedisCommands::test_command_getkeys",
"tests/test_commands.py::TestRedisCommands::test_command",
"tests/test_commands.py::TestRedisCommands::test_module",
"tests/test_commands.py::TestRedisCommands::test_restore",
"tests/test_commands.py::TestRedisCommands::test_restore_idletime",
"tests/test_commands.py::TestRedisCommands::test_restore_frequency",
"tests/test_commands.py::TestRedisCommands::test_replicaof",
"tests/test_commands.py::TestRedisCommands::test_sync",
"tests/test_commands.py::TestRedisCommands::test_psync",
"tests/test_commands.py::TestBinarySave::test_binary_get_set",
"tests/test_commands.py::TestBinarySave::test_binary_lists",
"tests/test_commands.py::TestBinarySave::test_22_info",
"tests/test_commands.py::TestBinarySave::test_large_responses",
"tests/test_commands.py::TestBinarySave::test_floating_point_encoding"
] | [
"tests/test_commands.py::TestRedisCommands::test_pexpiretime"
] | Python | [] | [] |
|
redis/redis-py | 1,895 | redis__redis-py-1895 | [
"1833"
] | 7d23974a62982d1b4b5586a648f8e7b17eccc6e5 | diff --git a/redis/connection.py b/redis/connection.py
--- a/redis/connection.py
+++ b/redis/connection.py
@@ -604,7 +604,9 @@ def connect(self):
if self._sock:
return
try:
- sock = self._connect()
+ sock = self.retry.call_with_retry(
+ lambda: self._connect(), lambda error: self.disconnect(error)
+ )
except socket.timeout:
raise TimeoutError("Timeout connecting to server")
except OSError as e:
@@ -721,7 +723,7 @@ def on_connect(self):
if str_if_bytes(self.read_response()) != "OK":
raise ConnectionError("Invalid Database")
- def disconnect(self):
+ def disconnect(self, *args):
"Disconnects from the Redis server"
self._parser.on_disconnect()
if self._sock is None:
diff --git a/redis/retry.py b/redis/retry.py
--- a/redis/retry.py
+++ b/redis/retry.py
@@ -1,3 +1,4 @@
+import socket
from time import sleep
from redis.exceptions import ConnectionError, TimeoutError
@@ -7,7 +8,10 @@ class Retry:
"""Retry a specific number of times after a failure"""
def __init__(
- self, backoff, retries, supported_errors=(ConnectionError, TimeoutError)
+ self,
+ backoff,
+ retries,
+ supported_errors=(ConnectionError, TimeoutError, socket.timeout),
):
"""
Initialize a `Retry` object with a `Backoff` object
| diff --git a/tests/test_connection.py b/tests/test_connection.py
--- a/tests/test_connection.py
+++ b/tests/test_connection.py
@@ -1,10 +1,14 @@
+import socket
import types
from unittest import mock
+from unittest.mock import patch
import pytest
+from redis.backoff import NoBackoff
from redis.connection import Connection
-from redis.exceptions import InvalidResponse
+from redis.exceptions import ConnectionError, InvalidResponse, TimeoutError
+from redis.retry import Retry
from redis.utils import HIREDIS_AVAILABLE
from .conftest import skip_if_server_version_lt
@@ -74,3 +78,47 @@ def test_disconnect__close_OSError(self):
mock_sock.shutdown.assert_called_once()
mock_sock.close.assert_called_once()
assert conn._sock is None
+
+ def clear(self, conn):
+ conn.retry_on_error.clear()
+
+ def test_retry_connect_on_timeout_error(self):
+ """Test that the _connect function is retried in case of a timeout"""
+ conn = Connection(retry_on_timeout=True, retry=Retry(NoBackoff(), 3))
+ origin_connect = conn._connect
+ conn._connect = mock.Mock()
+
+ def mock_connect():
+ # connect only on the last retry
+ if conn._connect.call_count <= 2:
+ raise socket.timeout
+ else:
+ return origin_connect()
+
+ conn._connect.side_effect = mock_connect
+ conn.connect()
+ assert conn._connect.call_count == 3
+ self.clear(conn)
+
+ def test_connect_without_retry_on_os_error(self):
+ """Test that the _connect function is not being retried in case of a OSError"""
+ with patch.object(Connection, "_connect") as _connect:
+ _connect.side_effect = OSError("")
+ conn = Connection(retry_on_timeout=True, retry=Retry(NoBackoff(), 2))
+ with pytest.raises(ConnectionError):
+ conn.connect()
+ assert _connect.call_count == 1
+ self.clear(conn)
+
+ def test_connect_timeout_error_without_retry(self):
+ """Test that the _connect function is not being retried if retry_on_timeout is
+ set to False"""
+ conn = Connection(retry_on_timeout=False)
+ conn._connect = mock.Mock()
+ conn._connect.side_effect = socket.timeout
+
+ with pytest.raises(TimeoutError) as e:
+ conn.connect()
+ assert conn._connect.call_count == 1
+ assert str(e.value) == "Timeout connecting to server"
+ self.clear(conn)
| Question: resolving hostname when retry on connection timeout
I wonder if Redis client resolve again the hostname if connection timeout occurred while setting `retry_on_timeout=True`.
I'm asking since sometime the mapping between hostname to ip can be invalid since the DNS changed the mapping.
| Yes,
By setting retry_on_timeout=True, when timeoutError is thrown, the client disconnects its current connection and creates a new one. Upon creating a new connection, the hostname is resolved again.
To retry on other errors too, you can use the new argument `retry_on_error` and pass other error types in a list.
@barshaul thanks for your replay.
Another question - how many retries and what is the delay between each retry?
If you don't pass a Retry instance using the client's 'retry' argument, it will create a default one for you. The default Retry object has 1 retries and NoBackoff() strategy.
Here's more info about the Retry / Backoff strategies:
- Backoff base class has four backoff strategies based on https://aws.amazon.com/blogs/architecture/exponential-backoff-and-jitter/. see ConstantBackoff, NoBackoff, ExponentialBackoff, FullJitterBackoff.
- Retry class is constructed from a backoff strategy and a maximum number of retries
Example how to create a client with retry object:
```
from redis.backoff import ExponentialBackoff
from redis.retry import Retry
from redis.client import Redis
from redis.exceptions import (
BusyLoadingError,
ConnectionError,
TimeoutError
)
retry = Retry(ExponentialBackoff(), 3) // Run 3 retries with exponential backoff strategy
r = Redis(host='localhost', port=6379, retry=retry, retry_on_error=[BusyLoadingError, ConnectionError, TimeoutError])
r_only_timeout = Redis(host='localhost', port=6379, retry=retry, retry_on_timeout=True)
```
@barshaul Thank you!
@barshaul I tried the solution you suggested and it's not working when trying to connect to the server.
by reading the code I saw that the retry mechanism only apply at the command execution level and not when trying to connect to the server.
any suggestion? | "2022-01-23T18:03:50Z" | 4.1 | [
"tests/test_connection.py::test_loading_external_modules",
"tests/test_connection.py::TestConnection::test_disconnect",
"tests/test_connection.py::TestConnection::test_disconnect__shutdown_OSError",
"tests/test_connection.py::TestConnection::test_disconnect__close_OSError",
"tests/test_connection.py::TestConnection::test_connect_without_retry_on_os_error",
"tests/test_connection.py::TestConnection::test_connect_timeout_error_without_retry"
] | [
"tests/test_connection.py::TestConnection::test_retry_connect_on_timeout_error"
] | Python | [] | [] |
redis/redis-py | 2,027 | redis__redis-py-2027 | [
"1939"
] | 8d949a3f39bc6fe17ee90009e78f17b32f69899a | diff --git a/redis/commands/core.py b/redis/commands/core.py
--- a/redis/commands/core.py
+++ b/redis/commands/core.py
@@ -1787,18 +1787,41 @@ def pexpire(self, name: KeyT, time: ExpiryT) -> ResponseT:
time = int(time.total_seconds() * 1000)
return self.execute_command("PEXPIRE", name, time)
- def pexpireat(self, name: KeyT, when: AbsExpiryT) -> ResponseT:
+ def pexpireat(
+ self,
+ name: KeyT,
+ when: AbsExpiryT,
+ nx: bool = False,
+ xx: bool = False,
+ gt: bool = False,
+ lt: bool = False,
+ ) -> ResponseT:
"""
- Set an expire flag on key ``name``. ``when`` can be represented
- as an integer representing unix time in milliseconds (unix time * 1000)
- or a Python datetime object.
+ Set an expire flag on key ``name`` with given ``option``. ``when``
+ can be represented as an integer representing unix time in
+ milliseconds (unix time * 1000) or a Python datetime object.
+
+ Valid options are:
+ NX -> Set expiry only when the key has no expiry
+ XX -> Set expiry only when the key has an existing expiry
+ GT -> Set expiry only when the new expiry is greater than current one
+ LT -> Set expiry only when the new expiry is less than current one
For more information check https://redis.io/commands/pexpireat
"""
if isinstance(when, datetime.datetime):
ms = int(when.microsecond / 1000)
when = int(time.mktime(when.timetuple())) * 1000 + ms
- return self.execute_command("PEXPIREAT", name, when)
+ exp_option = list()
+ if nx:
+ exp_option.append("NX")
+ if xx:
+ exp_option.append("XX")
+ if gt:
+ exp_option.append("GT")
+ if lt:
+ exp_option.append("LT")
+ return self.execute_command("PEXPIREAT", name, when, *exp_option)
def pexpiretime(self, key: str) -> int:
"""
| diff --git a/tests/test_commands.py b/tests/test_commands.py
--- a/tests/test_commands.py
+++ b/tests/test_commands.py
@@ -1254,6 +1254,41 @@ def test_pexpireat_unixtime(self, r):
assert r.pexpireat("a", expire_at_seconds) is True
assert 0 < r.pttl("a") <= 61000
+ @skip_if_server_version_lt("7.0.0")
+ def test_pexpireat_option_nx(self, r):
+ assert r.set("key", "val") is True
+ expire_at = redis_server_time(r) + datetime.timedelta(minutes=1)
+ assert r.pexpireat("key", expire_at, nx=True) is True
+ assert r.pexpireat("key", expire_at, nx=True) is False
+
+ @skip_if_server_version_lt("7.0.0")
+ def test_pexpireat_option_xx(self, r):
+ assert r.set("key", "val") is True
+ expire_at = redis_server_time(r) + datetime.timedelta(minutes=1)
+ assert r.pexpireat("key", expire_at, xx=True) is False
+ assert r.pexpireat("key", expire_at) is True
+ assert r.pexpireat("key", expire_at, xx=True) is True
+
+ @skip_if_server_version_lt("7.0.0")
+ def test_pexpireat_option_gt(self, r):
+ assert r.set("key", "val") is True
+ expire_at = redis_server_time(r) + datetime.timedelta(minutes=2)
+ assert r.pexpireat("key", expire_at) is True
+ expire_at = redis_server_time(r) + datetime.timedelta(minutes=1)
+ assert r.pexpireat("key", expire_at, gt=True) is False
+ expire_at = redis_server_time(r) + datetime.timedelta(minutes=3)
+ assert r.pexpireat("key", expire_at, gt=True) is True
+
+ @skip_if_server_version_lt("7.0.0")
+ def test_pexpireat_option_lt(self, r):
+ assert r.set("key", "val") is True
+ expire_at = redis_server_time(r) + datetime.timedelta(minutes=2)
+ assert r.pexpireat("key", expire_at) is True
+ expire_at = redis_server_time(r) + datetime.timedelta(minutes=3)
+ assert r.pexpireat("key", expire_at, lt=True) is False
+ expire_at = redis_server_time(r) + datetime.timedelta(minutes=1)
+ assert r.pexpireat("key", expire_at, lt=True) is True
+
@skip_if_server_version_lt("7.0.0")
def test_pexpiretime(self, r):
r.set("a", "foo")
| Add support for PEXPIREAT redis-7 options [NX|XX|GT|LT]
| "2022-02-27T21:12:34Z" | 4.2 | [
"tests/test_commands.py::TestResponseCallbacks::test_response_callbacks",
"tests/test_commands.py::TestResponseCallbacks::test_case_insensitive_command_names",
"tests/test_commands.py::TestRedisCommands::test_auth",
"tests/test_commands.py::TestRedisCommands::test_command_on_invalid_key_type",
"tests/test_commands.py::TestRedisCommands::test_acl_cat_no_category",
"tests/test_commands.py::TestRedisCommands::test_acl_cat_with_category",
"tests/test_commands.py::TestRedisCommands::test_acl_genpass",
"tests/test_commands.py::TestRedisCommands::test_acl_help",
"tests/test_commands.py::TestRedisCommands::test_acl_list",
"tests/test_commands.py::TestRedisCommands::test_acl_log",
"tests/test_commands.py::TestRedisCommands::test_acl_setuser_categories_without_prefix_fails",
"tests/test_commands.py::TestRedisCommands::test_acl_setuser_commands_without_prefix_fails",
"tests/test_commands.py::TestRedisCommands::test_acl_setuser_add_passwords_and_nopass_fails",
"tests/test_commands.py::TestRedisCommands::test_acl_users",
"tests/test_commands.py::TestRedisCommands::test_acl_whoami",
"tests/test_commands.py::TestRedisCommands::test_client_list",
"tests/test_commands.py::TestRedisCommands::test_client_info",
"tests/test_commands.py::TestRedisCommands::test_client_list_types_not_replica",
"tests/test_commands.py::TestRedisCommands::test_client_list_replica",
"tests/test_commands.py::TestRedisCommands::test_client_list_client_id",
"tests/test_commands.py::TestRedisCommands::test_client_id",
"tests/test_commands.py::TestRedisCommands::test_client_trackinginfo",
"tests/test_commands.py::TestRedisCommands::test_client_tracking",
"tests/test_commands.py::TestRedisCommands::test_client_unblock",
"tests/test_commands.py::TestRedisCommands::test_client_getname",
"tests/test_commands.py::TestRedisCommands::test_client_setname",
"tests/test_commands.py::TestRedisCommands::test_client_kill",
"tests/test_commands.py::TestRedisCommands::test_client_kill_filter_invalid_params",
"tests/test_commands.py::TestRedisCommands::test_client_kill_filter_by_id",
"tests/test_commands.py::TestRedisCommands::test_client_kill_filter_by_addr",
"tests/test_commands.py::TestRedisCommands::test_client_list_after_client_setname",
"tests/test_commands.py::TestRedisCommands::test_client_kill_filter_by_laddr",
"tests/test_commands.py::TestRedisCommands::test_client_kill_filter_by_user",
"tests/test_commands.py::TestRedisCommands::test_client_pause",
"tests/test_commands.py::TestRedisCommands::test_client_pause_all",
"tests/test_commands.py::TestRedisCommands::test_client_unpause",
"tests/test_commands.py::TestRedisCommands::test_client_reply",
"tests/test_commands.py::TestRedisCommands::test_client_getredir",
"tests/test_commands.py::TestRedisCommands::test_hello_notI_implemented",
"tests/test_commands.py::TestRedisCommands::test_config_get",
"tests/test_commands.py::TestRedisCommands::test_config_resetstat",
"tests/test_commands.py::TestRedisCommands::test_config_set",
"tests/test_commands.py::TestRedisCommands::test_failover",
"tests/test_commands.py::TestRedisCommands::test_dbsize",
"tests/test_commands.py::TestRedisCommands::test_echo",
"tests/test_commands.py::TestRedisCommands::test_info",
"tests/test_commands.py::TestRedisCommands::test_lastsave",
"tests/test_commands.py::TestRedisCommands::test_lolwut",
"tests/test_commands.py::TestRedisCommands::test_reset",
"tests/test_commands.py::TestRedisCommands::test_object",
"tests/test_commands.py::TestRedisCommands::test_ping",
"tests/test_commands.py::TestRedisCommands::test_quit",
"tests/test_commands.py::TestRedisCommands::test_role",
"tests/test_commands.py::TestRedisCommands::test_select",
"tests/test_commands.py::TestRedisCommands::test_slowlog_get",
"tests/test_commands.py::TestRedisCommands::test_slowlog_get_limit",
"tests/test_commands.py::TestRedisCommands::test_slowlog_length",
"tests/test_commands.py::TestRedisCommands::test_time",
"tests/test_commands.py::TestRedisCommands::test_bgsave",
"tests/test_commands.py::TestRedisCommands::test_append",
"tests/test_commands.py::TestRedisCommands::test_bitcount",
"tests/test_commands.py::TestRedisCommands::test_bitop_not_empty_string",
"tests/test_commands.py::TestRedisCommands::test_bitop_not",
"tests/test_commands.py::TestRedisCommands::test_bitop_not_in_place",
"tests/test_commands.py::TestRedisCommands::test_bitop_single_string",
"tests/test_commands.py::TestRedisCommands::test_bitop_string_operands",
"tests/test_commands.py::TestRedisCommands::test_bitpos",
"tests/test_commands.py::TestRedisCommands::test_bitpos_wrong_arguments",
"tests/test_commands.py::TestRedisCommands::test_copy",
"tests/test_commands.py::TestRedisCommands::test_copy_and_replace",
"tests/test_commands.py::TestRedisCommands::test_copy_to_another_database",
"tests/test_commands.py::TestRedisCommands::test_decr",
"tests/test_commands.py::TestRedisCommands::test_decrby",
"tests/test_commands.py::TestRedisCommands::test_delete",
"tests/test_commands.py::TestRedisCommands::test_delete_with_multiple_keys",
"tests/test_commands.py::TestRedisCommands::test_delitem",
"tests/test_commands.py::TestRedisCommands::test_unlink",
"tests/test_commands.py::TestRedisCommands::test_unlink_with_multiple_keys",
"tests/test_commands.py::TestRedisCommands::test_dump_and_restore",
"tests/test_commands.py::TestRedisCommands::test_dump_and_restore_and_replace",
"tests/test_commands.py::TestRedisCommands::test_dump_and_restore_absttl",
"tests/test_commands.py::TestRedisCommands::test_exists",
"tests/test_commands.py::TestRedisCommands::test_exists_contains",
"tests/test_commands.py::TestRedisCommands::test_expire",
"tests/test_commands.py::TestRedisCommands::test_expireat_datetime",
"tests/test_commands.py::TestRedisCommands::test_expireat_no_key",
"tests/test_commands.py::TestRedisCommands::test_expireat_unixtime",
"tests/test_commands.py::TestRedisCommands::test_expiretime",
"tests/test_commands.py::TestRedisCommands::test_get_and_set",
"tests/test_commands.py::TestRedisCommands::test_getdel",
"tests/test_commands.py::TestRedisCommands::test_getex",
"tests/test_commands.py::TestRedisCommands::test_getitem_and_setitem",
"tests/test_commands.py::TestRedisCommands::test_getitem_raises_keyerror_for_missing_key",
"tests/test_commands.py::TestRedisCommands::test_getitem_does_not_raise_keyerror_for_empty_string",
"tests/test_commands.py::TestRedisCommands::test_get_set_bit",
"tests/test_commands.py::TestRedisCommands::test_getrange",
"tests/test_commands.py::TestRedisCommands::test_getset",
"tests/test_commands.py::TestRedisCommands::test_incr",
"tests/test_commands.py::TestRedisCommands::test_incrby",
"tests/test_commands.py::TestRedisCommands::test_incrbyfloat",
"tests/test_commands.py::TestRedisCommands::test_keys",
"tests/test_commands.py::TestRedisCommands::test_mget",
"tests/test_commands.py::TestRedisCommands::test_lmove",
"tests/test_commands.py::TestRedisCommands::test_blmove",
"tests/test_commands.py::TestRedisCommands::test_mset",
"tests/test_commands.py::TestRedisCommands::test_msetnx",
"tests/test_commands.py::TestRedisCommands::test_pexpire",
"tests/test_commands.py::TestRedisCommands::test_pexpireat_datetime",
"tests/test_commands.py::TestRedisCommands::test_pexpireat_no_key",
"tests/test_commands.py::TestRedisCommands::test_pexpireat_unixtime",
"tests/test_commands.py::TestRedisCommands::test_pexpiretime",
"tests/test_commands.py::TestRedisCommands::test_psetex",
"tests/test_commands.py::TestRedisCommands::test_psetex_timedelta",
"tests/test_commands.py::TestRedisCommands::test_pttl",
"tests/test_commands.py::TestRedisCommands::test_pttl_no_key",
"tests/test_commands.py::TestRedisCommands::test_hrandfield",
"tests/test_commands.py::TestRedisCommands::test_randomkey",
"tests/test_commands.py::TestRedisCommands::test_rename",
"tests/test_commands.py::TestRedisCommands::test_renamenx",
"tests/test_commands.py::TestRedisCommands::test_set_nx",
"tests/test_commands.py::TestRedisCommands::test_set_xx",
"tests/test_commands.py::TestRedisCommands::test_set_px",
"tests/test_commands.py::TestRedisCommands::test_set_px_timedelta",
"tests/test_commands.py::TestRedisCommands::test_set_ex",
"tests/test_commands.py::TestRedisCommands::test_set_ex_timedelta",
"tests/test_commands.py::TestRedisCommands::test_set_exat_timedelta",
"tests/test_commands.py::TestRedisCommands::test_set_pxat_timedelta",
"tests/test_commands.py::TestRedisCommands::test_set_multipleoptions",
"tests/test_commands.py::TestRedisCommands::test_set_keepttl",
"tests/test_commands.py::TestRedisCommands::test_set_get",
"tests/test_commands.py::TestRedisCommands::test_setex",
"tests/test_commands.py::TestRedisCommands::test_setnx",
"tests/test_commands.py::TestRedisCommands::test_setrange",
"tests/test_commands.py::TestRedisCommands::test_strlen",
"tests/test_commands.py::TestRedisCommands::test_substr",
"tests/test_commands.py::TestRedisCommands::test_ttl",
"tests/test_commands.py::TestRedisCommands::test_ttl_nokey",
"tests/test_commands.py::TestRedisCommands::test_type",
"tests/test_commands.py::TestRedisCommands::test_blpop",
"tests/test_commands.py::TestRedisCommands::test_brpop",
"tests/test_commands.py::TestRedisCommands::test_brpoplpush",
"tests/test_commands.py::TestRedisCommands::test_brpoplpush_empty_string",
"tests/test_commands.py::TestRedisCommands::test_lindex",
"tests/test_commands.py::TestRedisCommands::test_linsert",
"tests/test_commands.py::TestRedisCommands::test_llen",
"tests/test_commands.py::TestRedisCommands::test_lpop",
"tests/test_commands.py::TestRedisCommands::test_lpop_count",
"tests/test_commands.py::TestRedisCommands::test_lpush",
"tests/test_commands.py::TestRedisCommands::test_lpushx",
"tests/test_commands.py::TestRedisCommands::test_lpushx_with_list",
"tests/test_commands.py::TestRedisCommands::test_lrange",
"tests/test_commands.py::TestRedisCommands::test_lrem",
"tests/test_commands.py::TestRedisCommands::test_lset",
"tests/test_commands.py::TestRedisCommands::test_ltrim",
"tests/test_commands.py::TestRedisCommands::test_rpop",
"tests/test_commands.py::TestRedisCommands::test_rpop_count",
"tests/test_commands.py::TestRedisCommands::test_rpoplpush",
"tests/test_commands.py::TestRedisCommands::test_rpush",
"tests/test_commands.py::TestRedisCommands::test_lpos",
"tests/test_commands.py::TestRedisCommands::test_rpushx",
"tests/test_commands.py::TestRedisCommands::test_scan",
"tests/test_commands.py::TestRedisCommands::test_scan_type",
"tests/test_commands.py::TestRedisCommands::test_scan_iter",
"tests/test_commands.py::TestRedisCommands::test_sscan",
"tests/test_commands.py::TestRedisCommands::test_sscan_iter",
"tests/test_commands.py::TestRedisCommands::test_hscan",
"tests/test_commands.py::TestRedisCommands::test_hscan_iter",
"tests/test_commands.py::TestRedisCommands::test_zscan",
"tests/test_commands.py::TestRedisCommands::test_zscan_iter",
"tests/test_commands.py::TestRedisCommands::test_sadd",
"tests/test_commands.py::TestRedisCommands::test_scard",
"tests/test_commands.py::TestRedisCommands::test_sdiff",
"tests/test_commands.py::TestRedisCommands::test_sdiffstore",
"tests/test_commands.py::TestRedisCommands::test_sinter",
"tests/test_commands.py::TestRedisCommands::test_sinterstore",
"tests/test_commands.py::TestRedisCommands::test_sismember",
"tests/test_commands.py::TestRedisCommands::test_smembers",
"tests/test_commands.py::TestRedisCommands::test_smismember",
"tests/test_commands.py::TestRedisCommands::test_smove",
"tests/test_commands.py::TestRedisCommands::test_spop",
"tests/test_commands.py::TestRedisCommands::test_spop_multi_value",
"tests/test_commands.py::TestRedisCommands::test_srandmember",
"tests/test_commands.py::TestRedisCommands::test_srandmember_multi_value",
"tests/test_commands.py::TestRedisCommands::test_srem",
"tests/test_commands.py::TestRedisCommands::test_sunion",
"tests/test_commands.py::TestRedisCommands::test_sunionstore",
"tests/test_commands.py::TestRedisCommands::test_debug_segfault",
"tests/test_commands.py::TestRedisCommands::test_script_debug",
"tests/test_commands.py::TestRedisCommands::test_zadd",
"tests/test_commands.py::TestRedisCommands::test_zadd_nx",
"tests/test_commands.py::TestRedisCommands::test_zadd_xx",
"tests/test_commands.py::TestRedisCommands::test_zadd_ch",
"tests/test_commands.py::TestRedisCommands::test_zadd_incr",
"tests/test_commands.py::TestRedisCommands::test_zadd_incr_with_xx",
"tests/test_commands.py::TestRedisCommands::test_zadd_gt_lt",
"tests/test_commands.py::TestRedisCommands::test_zcard",
"tests/test_commands.py::TestRedisCommands::test_zcount",
"tests/test_commands.py::TestRedisCommands::test_zdiff",
"tests/test_commands.py::TestRedisCommands::test_zdiffstore",
"tests/test_commands.py::TestRedisCommands::test_zincrby",
"tests/test_commands.py::TestRedisCommands::test_zlexcount",
"tests/test_commands.py::TestRedisCommands::test_zinter",
"tests/test_commands.py::TestRedisCommands::test_zinterstore_sum",
"tests/test_commands.py::TestRedisCommands::test_zinterstore_max",
"tests/test_commands.py::TestRedisCommands::test_zinterstore_min",
"tests/test_commands.py::TestRedisCommands::test_zinterstore_with_weight",
"tests/test_commands.py::TestRedisCommands::test_zpopmax",
"tests/test_commands.py::TestRedisCommands::test_zpopmin",
"tests/test_commands.py::TestRedisCommands::test_zrandemember",
"tests/test_commands.py::TestRedisCommands::test_bzpopmax",
"tests/test_commands.py::TestRedisCommands::test_bzpopmin",
"tests/test_commands.py::TestRedisCommands::test_zrange",
"tests/test_commands.py::TestRedisCommands::test_zrange_errors",
"tests/test_commands.py::TestRedisCommands::test_zrange_params",
"tests/test_commands.py::TestRedisCommands::test_zrangestore",
"tests/test_commands.py::TestRedisCommands::test_zrangebylex",
"tests/test_commands.py::TestRedisCommands::test_zrevrangebylex",
"tests/test_commands.py::TestRedisCommands::test_zrangebyscore",
"tests/test_commands.py::TestRedisCommands::test_zrank",
"tests/test_commands.py::TestRedisCommands::test_zrem",
"tests/test_commands.py::TestRedisCommands::test_zrem_multiple_keys",
"tests/test_commands.py::TestRedisCommands::test_zremrangebylex",
"tests/test_commands.py::TestRedisCommands::test_zremrangebyrank",
"tests/test_commands.py::TestRedisCommands::test_zremrangebyscore",
"tests/test_commands.py::TestRedisCommands::test_zrevrange",
"tests/test_commands.py::TestRedisCommands::test_zrevrangebyscore",
"tests/test_commands.py::TestRedisCommands::test_zrevrank",
"tests/test_commands.py::TestRedisCommands::test_zscore",
"tests/test_commands.py::TestRedisCommands::test_zunion",
"tests/test_commands.py::TestRedisCommands::test_zunionstore_sum",
"tests/test_commands.py::TestRedisCommands::test_zunionstore_max",
"tests/test_commands.py::TestRedisCommands::test_zunionstore_min",
"tests/test_commands.py::TestRedisCommands::test_zunionstore_with_weight",
"tests/test_commands.py::TestRedisCommands::test_zmscore",
"tests/test_commands.py::TestRedisCommands::test_pfadd",
"tests/test_commands.py::TestRedisCommands::test_pfcount",
"tests/test_commands.py::TestRedisCommands::test_pfmerge",
"tests/test_commands.py::TestRedisCommands::test_hget_and_hset",
"tests/test_commands.py::TestRedisCommands::test_hset_with_multi_key_values",
"tests/test_commands.py::TestRedisCommands::test_hset_with_key_values_passed_as_list",
"tests/test_commands.py::TestRedisCommands::test_hset_without_data",
"tests/test_commands.py::TestRedisCommands::test_hdel",
"tests/test_commands.py::TestRedisCommands::test_hexists",
"tests/test_commands.py::TestRedisCommands::test_hgetall",
"tests/test_commands.py::TestRedisCommands::test_hincrby",
"tests/test_commands.py::TestRedisCommands::test_hincrbyfloat",
"tests/test_commands.py::TestRedisCommands::test_hkeys",
"tests/test_commands.py::TestRedisCommands::test_hlen",
"tests/test_commands.py::TestRedisCommands::test_hmget",
"tests/test_commands.py::TestRedisCommands::test_hmset",
"tests/test_commands.py::TestRedisCommands::test_hsetnx",
"tests/test_commands.py::TestRedisCommands::test_hvals",
"tests/test_commands.py::TestRedisCommands::test_hstrlen",
"tests/test_commands.py::TestRedisCommands::test_sort_basic",
"tests/test_commands.py::TestRedisCommands::test_sort_limited",
"tests/test_commands.py::TestRedisCommands::test_sort_by",
"tests/test_commands.py::TestRedisCommands::test_sort_get",
"tests/test_commands.py::TestRedisCommands::test_sort_get_multi",
"tests/test_commands.py::TestRedisCommands::test_sort_get_groups_two",
"tests/test_commands.py::TestRedisCommands::test_sort_groups_string_get",
"tests/test_commands.py::TestRedisCommands::test_sort_groups_just_one_get",
"tests/test_commands.py::TestRedisCommands::test_sort_groups_no_get",
"tests/test_commands.py::TestRedisCommands::test_sort_groups_three_gets",
"tests/test_commands.py::TestRedisCommands::test_sort_desc",
"tests/test_commands.py::TestRedisCommands::test_sort_alpha",
"tests/test_commands.py::TestRedisCommands::test_sort_store",
"tests/test_commands.py::TestRedisCommands::test_sort_all_options",
"tests/test_commands.py::TestRedisCommands::test_sort_issue_924",
"tests/test_commands.py::TestRedisCommands::test_cluster_addslots",
"tests/test_commands.py::TestRedisCommands::test_cluster_count_failure_reports",
"tests/test_commands.py::TestRedisCommands::test_cluster_countkeysinslot",
"tests/test_commands.py::TestRedisCommands::test_cluster_delslots",
"tests/test_commands.py::TestRedisCommands::test_cluster_failover",
"tests/test_commands.py::TestRedisCommands::test_cluster_forget",
"tests/test_commands.py::TestRedisCommands::test_cluster_info",
"tests/test_commands.py::TestRedisCommands::test_cluster_keyslot",
"tests/test_commands.py::TestRedisCommands::test_cluster_meet",
"tests/test_commands.py::TestRedisCommands::test_cluster_nodes",
"tests/test_commands.py::TestRedisCommands::test_cluster_replicate",
"tests/test_commands.py::TestRedisCommands::test_cluster_reset",
"tests/test_commands.py::TestRedisCommands::test_cluster_saveconfig",
"tests/test_commands.py::TestRedisCommands::test_cluster_setslot",
"tests/test_commands.py::TestRedisCommands::test_cluster_slaves",
"tests/test_commands.py::TestRedisCommands::test_readonly_invalid_cluster_state",
"tests/test_commands.py::TestRedisCommands::test_readonly",
"tests/test_commands.py::TestRedisCommands::test_geoadd",
"tests/test_commands.py::TestRedisCommands::test_geoadd_nx",
"tests/test_commands.py::TestRedisCommands::test_geoadd_xx",
"tests/test_commands.py::TestRedisCommands::test_geoadd_ch",
"tests/test_commands.py::TestRedisCommands::test_geoadd_invalid_params",
"tests/test_commands.py::TestRedisCommands::test_geodist",
"tests/test_commands.py::TestRedisCommands::test_geodist_units",
"tests/test_commands.py::TestRedisCommands::test_geodist_missing_one_member",
"tests/test_commands.py::TestRedisCommands::test_geodist_invalid_units",
"tests/test_commands.py::TestRedisCommands::test_geohash",
"tests/test_commands.py::TestRedisCommands::test_geopos",
"tests/test_commands.py::TestRedisCommands::test_geopos_no_value",
"tests/test_commands.py::TestRedisCommands::test_geosearch",
"tests/test_commands.py::TestRedisCommands::test_geosearch_member",
"tests/test_commands.py::TestRedisCommands::test_geosearch_sort",
"tests/test_commands.py::TestRedisCommands::test_geosearch_with",
"tests/test_commands.py::TestRedisCommands::test_geosearch_negative",
"tests/test_commands.py::TestRedisCommands::test_geosearchstore",
"tests/test_commands.py::TestRedisCommands::test_geosearchstore_dist",
"tests/test_commands.py::TestRedisCommands::test_georadius",
"tests/test_commands.py::TestRedisCommands::test_georadius_no_values",
"tests/test_commands.py::TestRedisCommands::test_georadius_units",
"tests/test_commands.py::TestRedisCommands::test_georadius_with",
"tests/test_commands.py::TestRedisCommands::test_georadius_count",
"tests/test_commands.py::TestRedisCommands::test_georadius_sort",
"tests/test_commands.py::TestRedisCommands::test_georadius_store",
"tests/test_commands.py::TestRedisCommands::test_georadius_store_dist",
"tests/test_commands.py::TestRedisCommands::test_georadiusmember",
"tests/test_commands.py::TestRedisCommands::test_georadiusmember_count",
"tests/test_commands.py::TestRedisCommands::test_xack",
"tests/test_commands.py::TestRedisCommands::test_xadd",
"tests/test_commands.py::TestRedisCommands::test_xadd_nomkstream",
"tests/test_commands.py::TestRedisCommands::test_xadd_minlen_and_limit",
"tests/test_commands.py::TestRedisCommands::test_xautoclaim",
"tests/test_commands.py::TestRedisCommands::test_xautoclaim_negative",
"tests/test_commands.py::TestRedisCommands::test_xclaim",
"tests/test_commands.py::TestRedisCommands::test_xdel",
"tests/test_commands.py::TestRedisCommands::test_xgroup_delconsumer",
"tests/test_commands.py::TestRedisCommands::test_xgroup_createconsumer",
"tests/test_commands.py::TestRedisCommands::test_xgroup_destroy",
"tests/test_commands.py::TestRedisCommands::test_xinfo_stream",
"tests/test_commands.py::TestRedisCommands::test_xinfo_stream_full",
"tests/test_commands.py::TestRedisCommands::test_xlen",
"tests/test_commands.py::TestRedisCommands::test_xpending",
"tests/test_commands.py::TestRedisCommands::test_xpending_range",
"tests/test_commands.py::TestRedisCommands::test_xpending_range_idle",
"tests/test_commands.py::TestRedisCommands::test_xpending_range_negative",
"tests/test_commands.py::TestRedisCommands::test_xrange",
"tests/test_commands.py::TestRedisCommands::test_xread",
"tests/test_commands.py::TestRedisCommands::test_xreadgroup",
"tests/test_commands.py::TestRedisCommands::test_xrevrange",
"tests/test_commands.py::TestRedisCommands::test_xtrim",
"tests/test_commands.py::TestRedisCommands::test_xtrim_minlen_and_length_args",
"tests/test_commands.py::TestRedisCommands::test_bitfield_operations",
"tests/test_commands.py::TestRedisCommands::test_memory_help",
"tests/test_commands.py::TestRedisCommands::test_memory_doctor",
"tests/test_commands.py::TestRedisCommands::test_memory_malloc_stats",
"tests/test_commands.py::TestRedisCommands::test_memory_stats",
"tests/test_commands.py::TestRedisCommands::test_memory_usage",
"tests/test_commands.py::TestRedisCommands::test_module_list",
"tests/test_commands.py::TestRedisCommands::test_command_count",
"tests/test_commands.py::TestRedisCommands::test_command_docs",
"tests/test_commands.py::TestRedisCommands::test_command_getkeys",
"tests/test_commands.py::TestRedisCommands::test_command",
"tests/test_commands.py::TestRedisCommands::test_module",
"tests/test_commands.py::TestRedisCommands::test_restore",
"tests/test_commands.py::TestRedisCommands::test_restore_idletime",
"tests/test_commands.py::TestRedisCommands::test_restore_frequency",
"tests/test_commands.py::TestRedisCommands::test_replicaof",
"tests/test_commands.py::TestRedisCommands::test_sync",
"tests/test_commands.py::TestRedisCommands::test_psync",
"tests/test_commands.py::TestBinarySave::test_binary_get_set",
"tests/test_commands.py::TestBinarySave::test_binary_lists",
"tests/test_commands.py::TestBinarySave::test_22_info",
"tests/test_commands.py::TestBinarySave::test_large_responses",
"tests/test_commands.py::TestBinarySave::test_floating_point_encoding"
] | [
"tests/test_commands.py::TestRedisCommands::test_pexpireat_option_nx",
"tests/test_commands.py::TestRedisCommands::test_pexpireat_option_xx",
"tests/test_commands.py::TestRedisCommands::test_pexpireat_option_gt",
"tests/test_commands.py::TestRedisCommands::test_pexpireat_option_lt"
] | Python | [] | [] |
|
redis/redis-py | 2,068 | redis__redis-py-2068 | [
"1967"
] | 876cafc56ff465af5639a92ea18625f49de47563 | diff --git a/redis/commands/core.py b/redis/commands/core.py
--- a/redis/commands/core.py
+++ b/redis/commands/core.py
@@ -1363,6 +1363,7 @@ def bitcount(
key: KeyT,
start: Union[int, None] = None,
end: Union[int, None] = None,
+ mode: Optional[str] = None,
) -> ResponseT:
"""
Returns the count of set bits in the value of ``key``. Optional
@@ -1376,6 +1377,8 @@ def bitcount(
params.append(end)
elif (start is not None and end is None) or (end is not None and start is None):
raise DataError("Both start and end must be specified")
+ if mode is not None:
+ params.append(mode)
return self.execute_command("BITCOUNT", *params)
def bitfield(
@@ -1411,6 +1414,7 @@ def bitpos(
bit: int,
start: Union[int, None] = None,
end: Union[int, None] = None,
+ mode: Optional[str] = None,
) -> ResponseT:
"""
Return the position of the first bit set to 1 or 0 in a string.
@@ -1430,6 +1434,9 @@ def bitpos(
params.append(end)
elif start is None and end is not None:
raise DataError("start argument is not set, " "when end is specified")
+
+ if mode is not None:
+ params.append(mode)
return self.execute_command("BITPOS", *params)
def copy(
| diff --git a/tests/test_commands.py b/tests/test_commands.py
--- a/tests/test_commands.py
+++ b/tests/test_commands.py
@@ -854,6 +854,15 @@ def test_bitcount(self, r):
assert r.bitcount("a", -2, -1) == 2
assert r.bitcount("a", 1, 1) == 1
+ @skip_if_server_version_lt("7.0.0")
+ def test_bitcount_mode(self, r):
+ r.set("mykey", "foobar")
+ assert r.bitcount("mykey") == 26
+ assert r.bitcount("mykey", 1, 1, "byte") == 6
+ assert r.bitcount("mykey", 5, 30, "bit") == 17
+ with pytest.raises(redis.ResponseError):
+ assert r.bitcount("mykey", 5, 30, "but")
+
@pytest.mark.onlynoncluster
@skip_if_server_version_lt("2.6.0")
def test_bitop_not_empty_string(self, r):
@@ -926,6 +935,15 @@ def test_bitpos_wrong_arguments(self, r):
with pytest.raises(exceptions.RedisError):
r.bitpos(key, 7) == 12
+ @skip_if_server_version_lt("7.0.0")
+ def test_bitpos_mode(self, r):
+ r.set("mykey", b"\x00\xff\xf0")
+ assert r.bitpos("mykey", 1, 0) == 8
+ assert r.bitpos("mykey", 1, 2, -1, "byte") == 16
+ assert r.bitpos("mykey", 0, 7, 15, "bit") == 7
+ with pytest.raises(redis.ResponseError):
+ r.bitpos("mykey", 1, 7, 15, "bite")
+
@pytest.mark.onlynoncluster
@skip_if_server_version_lt("6.2.0")
def test_copy(self, r):
| Add support for missing command BITPOS - [BYTE|BIT] field
| "2022-03-28T12:36:25Z" | 4.2 | [
"tests/test_commands.py::TestResponseCallbacks::test_response_callbacks",
"tests/test_commands.py::TestResponseCallbacks::test_case_insensitive_command_names",
"tests/test_commands.py::TestRedisCommands::test_auth",
"tests/test_commands.py::TestRedisCommands::test_command_on_invalid_key_type",
"tests/test_commands.py::TestRedisCommands::test_acl_cat_no_category",
"tests/test_commands.py::TestRedisCommands::test_acl_cat_with_category",
"tests/test_commands.py::TestRedisCommands::test_acl_genpass",
"tests/test_commands.py::TestRedisCommands::test_acl_help",
"tests/test_commands.py::TestRedisCommands::test_acl_list",
"tests/test_commands.py::TestRedisCommands::test_acl_log",
"tests/test_commands.py::TestRedisCommands::test_acl_setuser_categories_without_prefix_fails",
"tests/test_commands.py::TestRedisCommands::test_acl_setuser_commands_without_prefix_fails",
"tests/test_commands.py::TestRedisCommands::test_acl_setuser_add_passwords_and_nopass_fails",
"tests/test_commands.py::TestRedisCommands::test_acl_users",
"tests/test_commands.py::TestRedisCommands::test_acl_whoami",
"tests/test_commands.py::TestRedisCommands::test_client_list",
"tests/test_commands.py::TestRedisCommands::test_client_info",
"tests/test_commands.py::TestRedisCommands::test_client_list_types_not_replica",
"tests/test_commands.py::TestRedisCommands::test_client_list_replica",
"tests/test_commands.py::TestRedisCommands::test_client_list_client_id",
"tests/test_commands.py::TestRedisCommands::test_client_id",
"tests/test_commands.py::TestRedisCommands::test_client_trackinginfo",
"tests/test_commands.py::TestRedisCommands::test_client_tracking",
"tests/test_commands.py::TestRedisCommands::test_client_unblock",
"tests/test_commands.py::TestRedisCommands::test_client_getname",
"tests/test_commands.py::TestRedisCommands::test_client_setname",
"tests/test_commands.py::TestRedisCommands::test_client_kill",
"tests/test_commands.py::TestRedisCommands::test_client_kill_filter_invalid_params",
"tests/test_commands.py::TestRedisCommands::test_client_kill_filter_by_id",
"tests/test_commands.py::TestRedisCommands::test_client_kill_filter_by_addr",
"tests/test_commands.py::TestRedisCommands::test_client_list_after_client_setname",
"tests/test_commands.py::TestRedisCommands::test_client_kill_filter_by_laddr",
"tests/test_commands.py::TestRedisCommands::test_client_kill_filter_by_user",
"tests/test_commands.py::TestRedisCommands::test_client_pause",
"tests/test_commands.py::TestRedisCommands::test_client_pause_all",
"tests/test_commands.py::TestRedisCommands::test_client_unpause",
"tests/test_commands.py::TestRedisCommands::test_client_reply",
"tests/test_commands.py::TestRedisCommands::test_client_getredir",
"tests/test_commands.py::TestRedisCommands::test_hello_notI_implemented",
"tests/test_commands.py::TestRedisCommands::test_config_get",
"tests/test_commands.py::TestRedisCommands::test_config_resetstat",
"tests/test_commands.py::TestRedisCommands::test_config_set",
"tests/test_commands.py::TestRedisCommands::test_failover",
"tests/test_commands.py::TestRedisCommands::test_dbsize",
"tests/test_commands.py::TestRedisCommands::test_echo",
"tests/test_commands.py::TestRedisCommands::test_info",
"tests/test_commands.py::TestRedisCommands::test_lastsave",
"tests/test_commands.py::TestRedisCommands::test_lolwut",
"tests/test_commands.py::TestRedisCommands::test_reset",
"tests/test_commands.py::TestRedisCommands::test_object",
"tests/test_commands.py::TestRedisCommands::test_ping",
"tests/test_commands.py::TestRedisCommands::test_quit",
"tests/test_commands.py::TestRedisCommands::test_role",
"tests/test_commands.py::TestRedisCommands::test_select",
"tests/test_commands.py::TestRedisCommands::test_slowlog_get",
"tests/test_commands.py::TestRedisCommands::test_slowlog_get_limit",
"tests/test_commands.py::TestRedisCommands::test_slowlog_length",
"tests/test_commands.py::TestRedisCommands::test_time",
"tests/test_commands.py::TestRedisCommands::test_bgsave",
"tests/test_commands.py::TestRedisCommands::test_append",
"tests/test_commands.py::TestRedisCommands::test_bitcount",
"tests/test_commands.py::TestRedisCommands::test_bitop_not_empty_string",
"tests/test_commands.py::TestRedisCommands::test_bitop_not",
"tests/test_commands.py::TestRedisCommands::test_bitop_not_in_place",
"tests/test_commands.py::TestRedisCommands::test_bitop_single_string",
"tests/test_commands.py::TestRedisCommands::test_bitop_string_operands",
"tests/test_commands.py::TestRedisCommands::test_bitpos",
"tests/test_commands.py::TestRedisCommands::test_bitpos_wrong_arguments",
"tests/test_commands.py::TestRedisCommands::test_copy",
"tests/test_commands.py::TestRedisCommands::test_copy_and_replace",
"tests/test_commands.py::TestRedisCommands::test_copy_to_another_database",
"tests/test_commands.py::TestRedisCommands::test_decr",
"tests/test_commands.py::TestRedisCommands::test_decrby",
"tests/test_commands.py::TestRedisCommands::test_delete",
"tests/test_commands.py::TestRedisCommands::test_delete_with_multiple_keys",
"tests/test_commands.py::TestRedisCommands::test_delitem",
"tests/test_commands.py::TestRedisCommands::test_unlink",
"tests/test_commands.py::TestRedisCommands::test_unlink_with_multiple_keys",
"tests/test_commands.py::TestRedisCommands::test_dump_and_restore",
"tests/test_commands.py::TestRedisCommands::test_dump_and_restore_and_replace",
"tests/test_commands.py::TestRedisCommands::test_dump_and_restore_absttl",
"tests/test_commands.py::TestRedisCommands::test_exists",
"tests/test_commands.py::TestRedisCommands::test_exists_contains",
"tests/test_commands.py::TestRedisCommands::test_expire",
"tests/test_commands.py::TestRedisCommands::test_expire_option_nx",
"tests/test_commands.py::TestRedisCommands::test_expire_option_gt",
"tests/test_commands.py::TestRedisCommands::test_expire_option_lt",
"tests/test_commands.py::TestRedisCommands::test_expireat_datetime",
"tests/test_commands.py::TestRedisCommands::test_expireat_no_key",
"tests/test_commands.py::TestRedisCommands::test_expireat_unixtime",
"tests/test_commands.py::TestRedisCommands::test_expiretime",
"tests/test_commands.py::TestRedisCommands::test_expireat_option_nx",
"tests/test_commands.py::TestRedisCommands::test_expireat_option_xx",
"tests/test_commands.py::TestRedisCommands::test_expireat_option_gt",
"tests/test_commands.py::TestRedisCommands::test_expireat_option_lt",
"tests/test_commands.py::TestRedisCommands::test_get_and_set",
"tests/test_commands.py::TestRedisCommands::test_getdel",
"tests/test_commands.py::TestRedisCommands::test_getex",
"tests/test_commands.py::TestRedisCommands::test_getitem_and_setitem",
"tests/test_commands.py::TestRedisCommands::test_getitem_raises_keyerror_for_missing_key",
"tests/test_commands.py::TestRedisCommands::test_getitem_does_not_raise_keyerror_for_empty_string",
"tests/test_commands.py::TestRedisCommands::test_get_set_bit",
"tests/test_commands.py::TestRedisCommands::test_getrange",
"tests/test_commands.py::TestRedisCommands::test_getset",
"tests/test_commands.py::TestRedisCommands::test_incr",
"tests/test_commands.py::TestRedisCommands::test_incrby",
"tests/test_commands.py::TestRedisCommands::test_incrbyfloat",
"tests/test_commands.py::TestRedisCommands::test_keys",
"tests/test_commands.py::TestRedisCommands::test_mget",
"tests/test_commands.py::TestRedisCommands::test_lmove",
"tests/test_commands.py::TestRedisCommands::test_blmove",
"tests/test_commands.py::TestRedisCommands::test_mset",
"tests/test_commands.py::TestRedisCommands::test_msetnx",
"tests/test_commands.py::TestRedisCommands::test_pexpire",
"tests/test_commands.py::TestRedisCommands::test_pexpire_option_nx",
"tests/test_commands.py::TestRedisCommands::test_pexpire_option_xx",
"tests/test_commands.py::TestRedisCommands::test_pexpire_option_gt",
"tests/test_commands.py::TestRedisCommands::test_pexpire_option_lt",
"tests/test_commands.py::TestRedisCommands::test_pexpireat_datetime",
"tests/test_commands.py::TestRedisCommands::test_pexpireat_no_key",
"tests/test_commands.py::TestRedisCommands::test_pexpireat_unixtime",
"tests/test_commands.py::TestRedisCommands::test_pexpireat_option_nx",
"tests/test_commands.py::TestRedisCommands::test_pexpireat_option_xx",
"tests/test_commands.py::TestRedisCommands::test_pexpireat_option_gt",
"tests/test_commands.py::TestRedisCommands::test_pexpireat_option_lt",
"tests/test_commands.py::TestRedisCommands::test_pexpiretime",
"tests/test_commands.py::TestRedisCommands::test_psetex",
"tests/test_commands.py::TestRedisCommands::test_psetex_timedelta",
"tests/test_commands.py::TestRedisCommands::test_pttl",
"tests/test_commands.py::TestRedisCommands::test_pttl_no_key",
"tests/test_commands.py::TestRedisCommands::test_hrandfield",
"tests/test_commands.py::TestRedisCommands::test_randomkey",
"tests/test_commands.py::TestRedisCommands::test_rename",
"tests/test_commands.py::TestRedisCommands::test_renamenx",
"tests/test_commands.py::TestRedisCommands::test_set_nx",
"tests/test_commands.py::TestRedisCommands::test_set_xx",
"tests/test_commands.py::TestRedisCommands::test_set_px",
"tests/test_commands.py::TestRedisCommands::test_set_px_timedelta",
"tests/test_commands.py::TestRedisCommands::test_set_ex",
"tests/test_commands.py::TestRedisCommands::test_set_ex_timedelta",
"tests/test_commands.py::TestRedisCommands::test_set_exat_timedelta",
"tests/test_commands.py::TestRedisCommands::test_set_pxat_timedelta",
"tests/test_commands.py::TestRedisCommands::test_set_multipleoptions",
"tests/test_commands.py::TestRedisCommands::test_set_keepttl",
"tests/test_commands.py::TestRedisCommands::test_set_get",
"tests/test_commands.py::TestRedisCommands::test_setex",
"tests/test_commands.py::TestRedisCommands::test_setnx",
"tests/test_commands.py::TestRedisCommands::test_setrange",
"tests/test_commands.py::TestRedisCommands::test_strlen",
"tests/test_commands.py::TestRedisCommands::test_substr",
"tests/test_commands.py::TestRedisCommands::test_ttl",
"tests/test_commands.py::TestRedisCommands::test_ttl_nokey",
"tests/test_commands.py::TestRedisCommands::test_type",
"tests/test_commands.py::TestRedisCommands::test_blpop",
"tests/test_commands.py::TestRedisCommands::test_brpop",
"tests/test_commands.py::TestRedisCommands::test_brpoplpush",
"tests/test_commands.py::TestRedisCommands::test_brpoplpush_empty_string",
"tests/test_commands.py::TestRedisCommands::test_lindex",
"tests/test_commands.py::TestRedisCommands::test_linsert",
"tests/test_commands.py::TestRedisCommands::test_llen",
"tests/test_commands.py::TestRedisCommands::test_lpop",
"tests/test_commands.py::TestRedisCommands::test_lpop_count",
"tests/test_commands.py::TestRedisCommands::test_lpush",
"tests/test_commands.py::TestRedisCommands::test_lpushx",
"tests/test_commands.py::TestRedisCommands::test_lpushx_with_list",
"tests/test_commands.py::TestRedisCommands::test_lrange",
"tests/test_commands.py::TestRedisCommands::test_lrem",
"tests/test_commands.py::TestRedisCommands::test_lset",
"tests/test_commands.py::TestRedisCommands::test_ltrim",
"tests/test_commands.py::TestRedisCommands::test_rpop",
"tests/test_commands.py::TestRedisCommands::test_rpop_count",
"tests/test_commands.py::TestRedisCommands::test_rpoplpush",
"tests/test_commands.py::TestRedisCommands::test_rpush",
"tests/test_commands.py::TestRedisCommands::test_lpos",
"tests/test_commands.py::TestRedisCommands::test_rpushx",
"tests/test_commands.py::TestRedisCommands::test_scan",
"tests/test_commands.py::TestRedisCommands::test_scan_type",
"tests/test_commands.py::TestRedisCommands::test_scan_iter",
"tests/test_commands.py::TestRedisCommands::test_sscan",
"tests/test_commands.py::TestRedisCommands::test_sscan_iter",
"tests/test_commands.py::TestRedisCommands::test_hscan",
"tests/test_commands.py::TestRedisCommands::test_hscan_iter",
"tests/test_commands.py::TestRedisCommands::test_zscan",
"tests/test_commands.py::TestRedisCommands::test_zscan_iter",
"tests/test_commands.py::TestRedisCommands::test_sadd",
"tests/test_commands.py::TestRedisCommands::test_scard",
"tests/test_commands.py::TestRedisCommands::test_sdiff",
"tests/test_commands.py::TestRedisCommands::test_sdiffstore",
"tests/test_commands.py::TestRedisCommands::test_sinter",
"tests/test_commands.py::TestRedisCommands::test_sintercard",
"tests/test_commands.py::TestRedisCommands::test_sinterstore",
"tests/test_commands.py::TestRedisCommands::test_sismember",
"tests/test_commands.py::TestRedisCommands::test_smembers",
"tests/test_commands.py::TestRedisCommands::test_smismember",
"tests/test_commands.py::TestRedisCommands::test_smove",
"tests/test_commands.py::TestRedisCommands::test_spop",
"tests/test_commands.py::TestRedisCommands::test_spop_multi_value",
"tests/test_commands.py::TestRedisCommands::test_srandmember",
"tests/test_commands.py::TestRedisCommands::test_srandmember_multi_value",
"tests/test_commands.py::TestRedisCommands::test_srem",
"tests/test_commands.py::TestRedisCommands::test_sunion",
"tests/test_commands.py::TestRedisCommands::test_sunionstore",
"tests/test_commands.py::TestRedisCommands::test_debug_segfault",
"tests/test_commands.py::TestRedisCommands::test_script_debug",
"tests/test_commands.py::TestRedisCommands::test_zadd",
"tests/test_commands.py::TestRedisCommands::test_zadd_nx",
"tests/test_commands.py::TestRedisCommands::test_zadd_xx",
"tests/test_commands.py::TestRedisCommands::test_zadd_ch",
"tests/test_commands.py::TestRedisCommands::test_zadd_incr",
"tests/test_commands.py::TestRedisCommands::test_zadd_incr_with_xx",
"tests/test_commands.py::TestRedisCommands::test_zadd_gt_lt",
"tests/test_commands.py::TestRedisCommands::test_zcard",
"tests/test_commands.py::TestRedisCommands::test_zcount",
"tests/test_commands.py::TestRedisCommands::test_zdiff",
"tests/test_commands.py::TestRedisCommands::test_zdiffstore",
"tests/test_commands.py::TestRedisCommands::test_zincrby",
"tests/test_commands.py::TestRedisCommands::test_zlexcount",
"tests/test_commands.py::TestRedisCommands::test_zinter",
"tests/test_commands.py::TestRedisCommands::test_zintercard",
"tests/test_commands.py::TestRedisCommands::test_zinterstore_sum",
"tests/test_commands.py::TestRedisCommands::test_zinterstore_max",
"tests/test_commands.py::TestRedisCommands::test_zinterstore_min",
"tests/test_commands.py::TestRedisCommands::test_zinterstore_with_weight",
"tests/test_commands.py::TestRedisCommands::test_zpopmax",
"tests/test_commands.py::TestRedisCommands::test_zpopmin",
"tests/test_commands.py::TestRedisCommands::test_zrandemember",
"tests/test_commands.py::TestRedisCommands::test_bzpopmax",
"tests/test_commands.py::TestRedisCommands::test_bzpopmin",
"tests/test_commands.py::TestRedisCommands::test_zrange",
"tests/test_commands.py::TestRedisCommands::test_zrange_errors",
"tests/test_commands.py::TestRedisCommands::test_zrange_params",
"tests/test_commands.py::TestRedisCommands::test_zrangestore",
"tests/test_commands.py::TestRedisCommands::test_zrangebylex",
"tests/test_commands.py::TestRedisCommands::test_zrevrangebylex",
"tests/test_commands.py::TestRedisCommands::test_zrangebyscore",
"tests/test_commands.py::TestRedisCommands::test_zrank",
"tests/test_commands.py::TestRedisCommands::test_zrem",
"tests/test_commands.py::TestRedisCommands::test_zrem_multiple_keys",
"tests/test_commands.py::TestRedisCommands::test_zremrangebylex",
"tests/test_commands.py::TestRedisCommands::test_zremrangebyrank",
"tests/test_commands.py::TestRedisCommands::test_zremrangebyscore",
"tests/test_commands.py::TestRedisCommands::test_zrevrange",
"tests/test_commands.py::TestRedisCommands::test_zrevrangebyscore",
"tests/test_commands.py::TestRedisCommands::test_zrevrank",
"tests/test_commands.py::TestRedisCommands::test_zscore",
"tests/test_commands.py::TestRedisCommands::test_zunion",
"tests/test_commands.py::TestRedisCommands::test_zunionstore_sum",
"tests/test_commands.py::TestRedisCommands::test_zunionstore_max",
"tests/test_commands.py::TestRedisCommands::test_zunionstore_min",
"tests/test_commands.py::TestRedisCommands::test_zunionstore_with_weight",
"tests/test_commands.py::TestRedisCommands::test_zmscore",
"tests/test_commands.py::TestRedisCommands::test_pfadd",
"tests/test_commands.py::TestRedisCommands::test_pfcount",
"tests/test_commands.py::TestRedisCommands::test_pfmerge",
"tests/test_commands.py::TestRedisCommands::test_hget_and_hset",
"tests/test_commands.py::TestRedisCommands::test_hset_with_multi_key_values",
"tests/test_commands.py::TestRedisCommands::test_hset_with_key_values_passed_as_list",
"tests/test_commands.py::TestRedisCommands::test_hset_without_data",
"tests/test_commands.py::TestRedisCommands::test_hdel",
"tests/test_commands.py::TestRedisCommands::test_hexists",
"tests/test_commands.py::TestRedisCommands::test_hgetall",
"tests/test_commands.py::TestRedisCommands::test_hincrby",
"tests/test_commands.py::TestRedisCommands::test_hincrbyfloat",
"tests/test_commands.py::TestRedisCommands::test_hkeys",
"tests/test_commands.py::TestRedisCommands::test_hlen",
"tests/test_commands.py::TestRedisCommands::test_hmget",
"tests/test_commands.py::TestRedisCommands::test_hmset",
"tests/test_commands.py::TestRedisCommands::test_hsetnx",
"tests/test_commands.py::TestRedisCommands::test_hvals",
"tests/test_commands.py::TestRedisCommands::test_hstrlen",
"tests/test_commands.py::TestRedisCommands::test_sort_basic",
"tests/test_commands.py::TestRedisCommands::test_sort_limited",
"tests/test_commands.py::TestRedisCommands::test_sort_by",
"tests/test_commands.py::TestRedisCommands::test_sort_get",
"tests/test_commands.py::TestRedisCommands::test_sort_get_multi",
"tests/test_commands.py::TestRedisCommands::test_sort_get_groups_two",
"tests/test_commands.py::TestRedisCommands::test_sort_groups_string_get",
"tests/test_commands.py::TestRedisCommands::test_sort_groups_just_one_get",
"tests/test_commands.py::TestRedisCommands::test_sort_groups_no_get",
"tests/test_commands.py::TestRedisCommands::test_sort_groups_three_gets",
"tests/test_commands.py::TestRedisCommands::test_sort_desc",
"tests/test_commands.py::TestRedisCommands::test_sort_alpha",
"tests/test_commands.py::TestRedisCommands::test_sort_store",
"tests/test_commands.py::TestRedisCommands::test_sort_all_options",
"tests/test_commands.py::TestRedisCommands::test_sort_ro",
"tests/test_commands.py::TestRedisCommands::test_sort_issue_924",
"tests/test_commands.py::TestRedisCommands::test_cluster_addslots",
"tests/test_commands.py::TestRedisCommands::test_cluster_count_failure_reports",
"tests/test_commands.py::TestRedisCommands::test_cluster_countkeysinslot",
"tests/test_commands.py::TestRedisCommands::test_cluster_delslots",
"tests/test_commands.py::TestRedisCommands::test_cluster_failover",
"tests/test_commands.py::TestRedisCommands::test_cluster_forget",
"tests/test_commands.py::TestRedisCommands::test_cluster_info",
"tests/test_commands.py::TestRedisCommands::test_cluster_keyslot",
"tests/test_commands.py::TestRedisCommands::test_cluster_meet",
"tests/test_commands.py::TestRedisCommands::test_cluster_nodes",
"tests/test_commands.py::TestRedisCommands::test_cluster_replicate",
"tests/test_commands.py::TestRedisCommands::test_cluster_reset",
"tests/test_commands.py::TestRedisCommands::test_cluster_saveconfig",
"tests/test_commands.py::TestRedisCommands::test_cluster_setslot",
"tests/test_commands.py::TestRedisCommands::test_cluster_slaves",
"tests/test_commands.py::TestRedisCommands::test_readonly_invalid_cluster_state",
"tests/test_commands.py::TestRedisCommands::test_readonly",
"tests/test_commands.py::TestRedisCommands::test_geoadd",
"tests/test_commands.py::TestRedisCommands::test_geoadd_nx",
"tests/test_commands.py::TestRedisCommands::test_geoadd_xx",
"tests/test_commands.py::TestRedisCommands::test_geoadd_ch",
"tests/test_commands.py::TestRedisCommands::test_geoadd_invalid_params",
"tests/test_commands.py::TestRedisCommands::test_geodist",
"tests/test_commands.py::TestRedisCommands::test_geodist_units",
"tests/test_commands.py::TestRedisCommands::test_geodist_missing_one_member",
"tests/test_commands.py::TestRedisCommands::test_geodist_invalid_units",
"tests/test_commands.py::TestRedisCommands::test_geohash",
"tests/test_commands.py::TestRedisCommands::test_geopos",
"tests/test_commands.py::TestRedisCommands::test_geopos_no_value",
"tests/test_commands.py::TestRedisCommands::test_geosearch",
"tests/test_commands.py::TestRedisCommands::test_geosearch_member",
"tests/test_commands.py::TestRedisCommands::test_geosearch_sort",
"tests/test_commands.py::TestRedisCommands::test_geosearch_with",
"tests/test_commands.py::TestRedisCommands::test_geosearch_negative",
"tests/test_commands.py::TestRedisCommands::test_geosearchstore",
"tests/test_commands.py::TestRedisCommands::test_geosearchstore_dist",
"tests/test_commands.py::TestRedisCommands::test_georadius",
"tests/test_commands.py::TestRedisCommands::test_georadius_no_values",
"tests/test_commands.py::TestRedisCommands::test_georadius_units",
"tests/test_commands.py::TestRedisCommands::test_georadius_with",
"tests/test_commands.py::TestRedisCommands::test_georadius_count",
"tests/test_commands.py::TestRedisCommands::test_georadius_sort",
"tests/test_commands.py::TestRedisCommands::test_georadius_store",
"tests/test_commands.py::TestRedisCommands::test_georadius_store_dist",
"tests/test_commands.py::TestRedisCommands::test_georadiusmember",
"tests/test_commands.py::TestRedisCommands::test_georadiusmember_count",
"tests/test_commands.py::TestRedisCommands::test_xack",
"tests/test_commands.py::TestRedisCommands::test_xadd",
"tests/test_commands.py::TestRedisCommands::test_xadd_nomkstream",
"tests/test_commands.py::TestRedisCommands::test_xadd_minlen_and_limit",
"tests/test_commands.py::TestRedisCommands::test_xautoclaim",
"tests/test_commands.py::TestRedisCommands::test_xautoclaim_negative",
"tests/test_commands.py::TestRedisCommands::test_xclaim",
"tests/test_commands.py::TestRedisCommands::test_xdel",
"tests/test_commands.py::TestRedisCommands::test_xgroup_delconsumer",
"tests/test_commands.py::TestRedisCommands::test_xgroup_createconsumer",
"tests/test_commands.py::TestRedisCommands::test_xgroup_destroy",
"tests/test_commands.py::TestRedisCommands::test_xinfo_stream",
"tests/test_commands.py::TestRedisCommands::test_xinfo_stream_full",
"tests/test_commands.py::TestRedisCommands::test_xlen",
"tests/test_commands.py::TestRedisCommands::test_xpending",
"tests/test_commands.py::TestRedisCommands::test_xpending_range",
"tests/test_commands.py::TestRedisCommands::test_xpending_range_idle",
"tests/test_commands.py::TestRedisCommands::test_xpending_range_negative",
"tests/test_commands.py::TestRedisCommands::test_xrange",
"tests/test_commands.py::TestRedisCommands::test_xread",
"tests/test_commands.py::TestRedisCommands::test_xreadgroup",
"tests/test_commands.py::TestRedisCommands::test_xrevrange",
"tests/test_commands.py::TestRedisCommands::test_xtrim",
"tests/test_commands.py::TestRedisCommands::test_xtrim_minlen_and_length_args",
"tests/test_commands.py::TestRedisCommands::test_bitfield_operations",
"tests/test_commands.py::TestRedisCommands::test_memory_help",
"tests/test_commands.py::TestRedisCommands::test_memory_doctor",
"tests/test_commands.py::TestRedisCommands::test_memory_malloc_stats",
"tests/test_commands.py::TestRedisCommands::test_memory_stats",
"tests/test_commands.py::TestRedisCommands::test_memory_usage",
"tests/test_commands.py::TestRedisCommands::test_module_list",
"tests/test_commands.py::TestRedisCommands::test_command_count",
"tests/test_commands.py::TestRedisCommands::test_command_docs",
"tests/test_commands.py::TestRedisCommands::test_command_getkeys",
"tests/test_commands.py::TestRedisCommands::test_command",
"tests/test_commands.py::TestRedisCommands::test_module",
"tests/test_commands.py::TestRedisCommands::test_restore",
"tests/test_commands.py::TestRedisCommands::test_restore_idletime",
"tests/test_commands.py::TestRedisCommands::test_restore_frequency",
"tests/test_commands.py::TestRedisCommands::test_replicaof",
"tests/test_commands.py::TestRedisCommands::test_sync",
"tests/test_commands.py::TestRedisCommands::test_psync",
"tests/test_commands.py::TestBinarySave::test_binary_get_set",
"tests/test_commands.py::TestBinarySave::test_binary_lists",
"tests/test_commands.py::TestBinarySave::test_22_info",
"tests/test_commands.py::TestBinarySave::test_large_responses",
"tests/test_commands.py::TestBinarySave::test_floating_point_encoding"
] | [
"tests/test_commands.py::TestRedisCommands::test_bitcount_mode",
"tests/test_commands.py::TestRedisCommands::test_bitpos_mode"
] | Python | [] | [] |
|
redis/redis-py | 2,142 | redis__redis-py-2142 | [
"2121"
] | 2da2ac3368bc88ad909d78fe73029bc9c627545d | diff --git a/redis/commands/core.py b/redis/commands/core.py
--- a/redis/commands/core.py
+++ b/redis/commands/core.py
@@ -760,13 +760,15 @@ def command_docs(self, *args):
"COMMAND DOCS is intentionally not implemented in the client."
)
- def config_get(self, pattern: PatternT = "*", **kwargs) -> ResponseT:
+ def config_get(
+ self, pattern: PatternT = "*", *args: List[PatternT], **kwargs
+ ) -> ResponseT:
"""
Return a dictionary of configuration based on the ``pattern``
For more information see https://redis.io/commands/config-get
"""
- return self.execute_command("CONFIG GET", pattern, **kwargs)
+ return self.execute_command("CONFIG GET", pattern, *args, **kwargs)
def config_set(self, name: KeyT, value: EncodableT, **kwargs) -> ResponseT:
"""Set config item ``name`` with ``value``
| diff --git a/tests/test_commands.py b/tests/test_commands.py
--- a/tests/test_commands.py
+++ b/tests/test_commands.py
@@ -669,6 +669,12 @@ def test_config_get(self, r):
# # assert 'maxmemory' in data
# assert data['maxmemory'].isdigit()
+ @skip_if_server_version_lt("7.0.0")
+ def test_config_get_multi_params(self, r: redis.Redis):
+ res = r.config_get("*max-*-entries*", "maxmemory")
+ assert "maxmemory" in res
+ assert "hash-max-listpack-entries" in res
+
@pytest.mark.onlynoncluster
@skip_if_redis_enterprise()
def test_config_resetstat(self, r):
| CONFIG GET - add the ability to pass multiple pattern parameters in one call
| "2022-04-26T13:38:36Z" | 4.2 | [
"tests/test_commands.py::TestResponseCallbacks::test_response_callbacks",
"tests/test_commands.py::TestResponseCallbacks::test_case_insensitive_command_names",
"tests/test_commands.py::TestRedisCommands::test_auth",
"tests/test_commands.py::TestRedisCommands::test_command_on_invalid_key_type",
"tests/test_commands.py::TestRedisCommands::test_acl_cat_no_category",
"tests/test_commands.py::TestRedisCommands::test_acl_cat_with_category",
"tests/test_commands.py::TestRedisCommands::test_acl_genpass",
"tests/test_commands.py::TestRedisCommands::test_acl_help",
"tests/test_commands.py::TestRedisCommands::test_acl_list",
"tests/test_commands.py::TestRedisCommands::test_acl_log",
"tests/test_commands.py::TestRedisCommands::test_acl_setuser_categories_without_prefix_fails",
"tests/test_commands.py::TestRedisCommands::test_acl_setuser_commands_without_prefix_fails",
"tests/test_commands.py::TestRedisCommands::test_acl_setuser_add_passwords_and_nopass_fails",
"tests/test_commands.py::TestRedisCommands::test_acl_users",
"tests/test_commands.py::TestRedisCommands::test_acl_whoami",
"tests/test_commands.py::TestRedisCommands::test_client_list",
"tests/test_commands.py::TestRedisCommands::test_client_info",
"tests/test_commands.py::TestRedisCommands::test_client_list_types_not_replica",
"tests/test_commands.py::TestRedisCommands::test_client_list_replica",
"tests/test_commands.py::TestRedisCommands::test_client_list_client_id",
"tests/test_commands.py::TestRedisCommands::test_client_id",
"tests/test_commands.py::TestRedisCommands::test_client_trackinginfo",
"tests/test_commands.py::TestRedisCommands::test_client_tracking",
"tests/test_commands.py::TestRedisCommands::test_client_unblock",
"tests/test_commands.py::TestRedisCommands::test_client_getname",
"tests/test_commands.py::TestRedisCommands::test_client_setname",
"tests/test_commands.py::TestRedisCommands::test_client_kill",
"tests/test_commands.py::TestRedisCommands::test_client_kill_filter_invalid_params",
"tests/test_commands.py::TestRedisCommands::test_client_kill_filter_by_id",
"tests/test_commands.py::TestRedisCommands::test_client_kill_filter_by_addr",
"tests/test_commands.py::TestRedisCommands::test_client_list_after_client_setname",
"tests/test_commands.py::TestRedisCommands::test_client_kill_filter_by_laddr",
"tests/test_commands.py::TestRedisCommands::test_client_kill_filter_by_user",
"tests/test_commands.py::TestRedisCommands::test_client_pause",
"tests/test_commands.py::TestRedisCommands::test_client_pause_all",
"tests/test_commands.py::TestRedisCommands::test_client_unpause",
"tests/test_commands.py::TestRedisCommands::test_client_reply",
"tests/test_commands.py::TestRedisCommands::test_client_getredir",
"tests/test_commands.py::TestRedisCommands::test_hello_notI_implemented",
"tests/test_commands.py::TestRedisCommands::test_config_get",
"tests/test_commands.py::TestRedisCommands::test_config_resetstat",
"tests/test_commands.py::TestRedisCommands::test_config_set",
"tests/test_commands.py::TestRedisCommands::test_failover",
"tests/test_commands.py::TestRedisCommands::test_dbsize",
"tests/test_commands.py::TestRedisCommands::test_echo",
"tests/test_commands.py::TestRedisCommands::test_info",
"tests/test_commands.py::TestRedisCommands::test_lastsave",
"tests/test_commands.py::TestRedisCommands::test_lolwut",
"tests/test_commands.py::TestRedisCommands::test_reset",
"tests/test_commands.py::TestRedisCommands::test_object",
"tests/test_commands.py::TestRedisCommands::test_ping",
"tests/test_commands.py::TestRedisCommands::test_quit",
"tests/test_commands.py::TestRedisCommands::test_role",
"tests/test_commands.py::TestRedisCommands::test_select",
"tests/test_commands.py::TestRedisCommands::test_slowlog_get",
"tests/test_commands.py::TestRedisCommands::test_slowlog_get_limit",
"tests/test_commands.py::TestRedisCommands::test_slowlog_length",
"tests/test_commands.py::TestRedisCommands::test_time",
"tests/test_commands.py::TestRedisCommands::test_bgsave",
"tests/test_commands.py::TestRedisCommands::test_append",
"tests/test_commands.py::TestRedisCommands::test_bitcount",
"tests/test_commands.py::TestRedisCommands::test_bitcount_mode",
"tests/test_commands.py::TestRedisCommands::test_bitop_not_empty_string",
"tests/test_commands.py::TestRedisCommands::test_bitop_not",
"tests/test_commands.py::TestRedisCommands::test_bitop_not_in_place",
"tests/test_commands.py::TestRedisCommands::test_bitop_single_string",
"tests/test_commands.py::TestRedisCommands::test_bitop_string_operands",
"tests/test_commands.py::TestRedisCommands::test_bitpos",
"tests/test_commands.py::TestRedisCommands::test_bitpos_wrong_arguments",
"tests/test_commands.py::TestRedisCommands::test_bitpos_mode",
"tests/test_commands.py::TestRedisCommands::test_copy",
"tests/test_commands.py::TestRedisCommands::test_copy_and_replace",
"tests/test_commands.py::TestRedisCommands::test_copy_to_another_database",
"tests/test_commands.py::TestRedisCommands::test_decr",
"tests/test_commands.py::TestRedisCommands::test_decrby",
"tests/test_commands.py::TestRedisCommands::test_delete",
"tests/test_commands.py::TestRedisCommands::test_delete_with_multiple_keys",
"tests/test_commands.py::TestRedisCommands::test_delitem",
"tests/test_commands.py::TestRedisCommands::test_unlink",
"tests/test_commands.py::TestRedisCommands::test_unlink_with_multiple_keys",
"tests/test_commands.py::TestRedisCommands::test_dump_and_restore",
"tests/test_commands.py::TestRedisCommands::test_dump_and_restore_and_replace",
"tests/test_commands.py::TestRedisCommands::test_dump_and_restore_absttl",
"tests/test_commands.py::TestRedisCommands::test_exists",
"tests/test_commands.py::TestRedisCommands::test_exists_contains",
"tests/test_commands.py::TestRedisCommands::test_expire",
"tests/test_commands.py::TestRedisCommands::test_expire_option_nx",
"tests/test_commands.py::TestRedisCommands::test_expire_option_gt",
"tests/test_commands.py::TestRedisCommands::test_expire_option_lt",
"tests/test_commands.py::TestRedisCommands::test_expireat_datetime",
"tests/test_commands.py::TestRedisCommands::test_expireat_no_key",
"tests/test_commands.py::TestRedisCommands::test_expireat_unixtime",
"tests/test_commands.py::TestRedisCommands::test_expiretime",
"tests/test_commands.py::TestRedisCommands::test_expireat_option_nx",
"tests/test_commands.py::TestRedisCommands::test_expireat_option_xx",
"tests/test_commands.py::TestRedisCommands::test_expireat_option_gt",
"tests/test_commands.py::TestRedisCommands::test_expireat_option_lt",
"tests/test_commands.py::TestRedisCommands::test_get_and_set",
"tests/test_commands.py::TestRedisCommands::test_getdel",
"tests/test_commands.py::TestRedisCommands::test_getex",
"tests/test_commands.py::TestRedisCommands::test_getitem_and_setitem",
"tests/test_commands.py::TestRedisCommands::test_getitem_raises_keyerror_for_missing_key",
"tests/test_commands.py::TestRedisCommands::test_getitem_does_not_raise_keyerror_for_empty_string",
"tests/test_commands.py::TestRedisCommands::test_get_set_bit",
"tests/test_commands.py::TestRedisCommands::test_getrange",
"tests/test_commands.py::TestRedisCommands::test_getset",
"tests/test_commands.py::TestRedisCommands::test_incr",
"tests/test_commands.py::TestRedisCommands::test_incrby",
"tests/test_commands.py::TestRedisCommands::test_incrbyfloat",
"tests/test_commands.py::TestRedisCommands::test_keys",
"tests/test_commands.py::TestRedisCommands::test_mget",
"tests/test_commands.py::TestRedisCommands::test_lmove",
"tests/test_commands.py::TestRedisCommands::test_blmove",
"tests/test_commands.py::TestRedisCommands::test_mset",
"tests/test_commands.py::TestRedisCommands::test_msetnx",
"tests/test_commands.py::TestRedisCommands::test_pexpire",
"tests/test_commands.py::TestRedisCommands::test_pexpire_option_nx",
"tests/test_commands.py::TestRedisCommands::test_pexpire_option_xx",
"tests/test_commands.py::TestRedisCommands::test_pexpire_option_gt",
"tests/test_commands.py::TestRedisCommands::test_pexpire_option_lt",
"tests/test_commands.py::TestRedisCommands::test_pexpireat_datetime",
"tests/test_commands.py::TestRedisCommands::test_pexpireat_no_key",
"tests/test_commands.py::TestRedisCommands::test_pexpireat_unixtime",
"tests/test_commands.py::TestRedisCommands::test_pexpireat_option_nx",
"tests/test_commands.py::TestRedisCommands::test_pexpireat_option_xx",
"tests/test_commands.py::TestRedisCommands::test_pexpireat_option_gt",
"tests/test_commands.py::TestRedisCommands::test_pexpireat_option_lt",
"tests/test_commands.py::TestRedisCommands::test_pexpiretime",
"tests/test_commands.py::TestRedisCommands::test_psetex",
"tests/test_commands.py::TestRedisCommands::test_psetex_timedelta",
"tests/test_commands.py::TestRedisCommands::test_pttl",
"tests/test_commands.py::TestRedisCommands::test_pttl_no_key",
"tests/test_commands.py::TestRedisCommands::test_hrandfield",
"tests/test_commands.py::TestRedisCommands::test_randomkey",
"tests/test_commands.py::TestRedisCommands::test_rename",
"tests/test_commands.py::TestRedisCommands::test_renamenx",
"tests/test_commands.py::TestRedisCommands::test_set_nx",
"tests/test_commands.py::TestRedisCommands::test_set_xx",
"tests/test_commands.py::TestRedisCommands::test_set_px",
"tests/test_commands.py::TestRedisCommands::test_set_px_timedelta",
"tests/test_commands.py::TestRedisCommands::test_set_ex",
"tests/test_commands.py::TestRedisCommands::test_set_ex_timedelta",
"tests/test_commands.py::TestRedisCommands::test_set_exat_timedelta",
"tests/test_commands.py::TestRedisCommands::test_set_pxat_timedelta",
"tests/test_commands.py::TestRedisCommands::test_set_multipleoptions",
"tests/test_commands.py::TestRedisCommands::test_set_keepttl",
"tests/test_commands.py::TestRedisCommands::test_set_get",
"tests/test_commands.py::TestRedisCommands::test_setex",
"tests/test_commands.py::TestRedisCommands::test_setnx",
"tests/test_commands.py::TestRedisCommands::test_setrange",
"tests/test_commands.py::TestRedisCommands::test_strlen",
"tests/test_commands.py::TestRedisCommands::test_substr",
"tests/test_commands.py::TestRedisCommands::test_ttl",
"tests/test_commands.py::TestRedisCommands::test_ttl_nokey",
"tests/test_commands.py::TestRedisCommands::test_type",
"tests/test_commands.py::TestRedisCommands::test_blpop",
"tests/test_commands.py::TestRedisCommands::test_brpop",
"tests/test_commands.py::TestRedisCommands::test_brpoplpush",
"tests/test_commands.py::TestRedisCommands::test_brpoplpush_empty_string",
"tests/test_commands.py::TestRedisCommands::test_lindex",
"tests/test_commands.py::TestRedisCommands::test_linsert",
"tests/test_commands.py::TestRedisCommands::test_llen",
"tests/test_commands.py::TestRedisCommands::test_lpop",
"tests/test_commands.py::TestRedisCommands::test_lpop_count",
"tests/test_commands.py::TestRedisCommands::test_lpush",
"tests/test_commands.py::TestRedisCommands::test_lpushx",
"tests/test_commands.py::TestRedisCommands::test_lpushx_with_list",
"tests/test_commands.py::TestRedisCommands::test_lrange",
"tests/test_commands.py::TestRedisCommands::test_lrem",
"tests/test_commands.py::TestRedisCommands::test_lset",
"tests/test_commands.py::TestRedisCommands::test_ltrim",
"tests/test_commands.py::TestRedisCommands::test_rpop",
"tests/test_commands.py::TestRedisCommands::test_rpop_count",
"tests/test_commands.py::TestRedisCommands::test_rpoplpush",
"tests/test_commands.py::TestRedisCommands::test_rpush",
"tests/test_commands.py::TestRedisCommands::test_lpos",
"tests/test_commands.py::TestRedisCommands::test_rpushx",
"tests/test_commands.py::TestRedisCommands::test_scan",
"tests/test_commands.py::TestRedisCommands::test_scan_type",
"tests/test_commands.py::TestRedisCommands::test_scan_iter",
"tests/test_commands.py::TestRedisCommands::test_sscan",
"tests/test_commands.py::TestRedisCommands::test_sscan_iter",
"tests/test_commands.py::TestRedisCommands::test_hscan",
"tests/test_commands.py::TestRedisCommands::test_hscan_iter",
"tests/test_commands.py::TestRedisCommands::test_zscan",
"tests/test_commands.py::TestRedisCommands::test_zscan_iter",
"tests/test_commands.py::TestRedisCommands::test_sadd",
"tests/test_commands.py::TestRedisCommands::test_scard",
"tests/test_commands.py::TestRedisCommands::test_sdiff",
"tests/test_commands.py::TestRedisCommands::test_sdiffstore",
"tests/test_commands.py::TestRedisCommands::test_sinter",
"tests/test_commands.py::TestRedisCommands::test_sintercard",
"tests/test_commands.py::TestRedisCommands::test_sinterstore",
"tests/test_commands.py::TestRedisCommands::test_sismember",
"tests/test_commands.py::TestRedisCommands::test_smembers",
"tests/test_commands.py::TestRedisCommands::test_smismember",
"tests/test_commands.py::TestRedisCommands::test_smove",
"tests/test_commands.py::TestRedisCommands::test_spop",
"tests/test_commands.py::TestRedisCommands::test_spop_multi_value",
"tests/test_commands.py::TestRedisCommands::test_srandmember",
"tests/test_commands.py::TestRedisCommands::test_srandmember_multi_value",
"tests/test_commands.py::TestRedisCommands::test_srem",
"tests/test_commands.py::TestRedisCommands::test_sunion",
"tests/test_commands.py::TestRedisCommands::test_sunionstore",
"tests/test_commands.py::TestRedisCommands::test_debug_segfault",
"tests/test_commands.py::TestRedisCommands::test_script_debug",
"tests/test_commands.py::TestRedisCommands::test_zadd",
"tests/test_commands.py::TestRedisCommands::test_zadd_nx",
"tests/test_commands.py::TestRedisCommands::test_zadd_xx",
"tests/test_commands.py::TestRedisCommands::test_zadd_ch",
"tests/test_commands.py::TestRedisCommands::test_zadd_incr",
"tests/test_commands.py::TestRedisCommands::test_zadd_incr_with_xx",
"tests/test_commands.py::TestRedisCommands::test_zadd_gt_lt",
"tests/test_commands.py::TestRedisCommands::test_zcard",
"tests/test_commands.py::TestRedisCommands::test_zcount",
"tests/test_commands.py::TestRedisCommands::test_zdiff",
"tests/test_commands.py::TestRedisCommands::test_zdiffstore",
"tests/test_commands.py::TestRedisCommands::test_zincrby",
"tests/test_commands.py::TestRedisCommands::test_zlexcount",
"tests/test_commands.py::TestRedisCommands::test_zinter",
"tests/test_commands.py::TestRedisCommands::test_zintercard",
"tests/test_commands.py::TestRedisCommands::test_zinterstore_sum",
"tests/test_commands.py::TestRedisCommands::test_zinterstore_max",
"tests/test_commands.py::TestRedisCommands::test_zinterstore_min",
"tests/test_commands.py::TestRedisCommands::test_zinterstore_with_weight",
"tests/test_commands.py::TestRedisCommands::test_zpopmax",
"tests/test_commands.py::TestRedisCommands::test_zpopmin",
"tests/test_commands.py::TestRedisCommands::test_zrandemember",
"tests/test_commands.py::TestRedisCommands::test_bzpopmax",
"tests/test_commands.py::TestRedisCommands::test_bzpopmin",
"tests/test_commands.py::TestRedisCommands::test_zrange",
"tests/test_commands.py::TestRedisCommands::test_zrange_errors",
"tests/test_commands.py::TestRedisCommands::test_zrange_params",
"tests/test_commands.py::TestRedisCommands::test_zrangestore",
"tests/test_commands.py::TestRedisCommands::test_zrangebylex",
"tests/test_commands.py::TestRedisCommands::test_zrevrangebylex",
"tests/test_commands.py::TestRedisCommands::test_zrangebyscore",
"tests/test_commands.py::TestRedisCommands::test_zrank",
"tests/test_commands.py::TestRedisCommands::test_zrem",
"tests/test_commands.py::TestRedisCommands::test_zrem_multiple_keys",
"tests/test_commands.py::TestRedisCommands::test_zremrangebylex",
"tests/test_commands.py::TestRedisCommands::test_zremrangebyrank",
"tests/test_commands.py::TestRedisCommands::test_zremrangebyscore",
"tests/test_commands.py::TestRedisCommands::test_zrevrange",
"tests/test_commands.py::TestRedisCommands::test_zrevrangebyscore",
"tests/test_commands.py::TestRedisCommands::test_zrevrank",
"tests/test_commands.py::TestRedisCommands::test_zscore",
"tests/test_commands.py::TestRedisCommands::test_zunion",
"tests/test_commands.py::TestRedisCommands::test_zunionstore_sum",
"tests/test_commands.py::TestRedisCommands::test_zunionstore_max",
"tests/test_commands.py::TestRedisCommands::test_zunionstore_min",
"tests/test_commands.py::TestRedisCommands::test_zunionstore_with_weight",
"tests/test_commands.py::TestRedisCommands::test_zmscore",
"tests/test_commands.py::TestRedisCommands::test_pfadd",
"tests/test_commands.py::TestRedisCommands::test_pfcount",
"tests/test_commands.py::TestRedisCommands::test_pfmerge",
"tests/test_commands.py::TestRedisCommands::test_hget_and_hset",
"tests/test_commands.py::TestRedisCommands::test_hset_with_multi_key_values",
"tests/test_commands.py::TestRedisCommands::test_hset_with_key_values_passed_as_list",
"tests/test_commands.py::TestRedisCommands::test_hset_without_data",
"tests/test_commands.py::TestRedisCommands::test_hdel",
"tests/test_commands.py::TestRedisCommands::test_hexists",
"tests/test_commands.py::TestRedisCommands::test_hgetall",
"tests/test_commands.py::TestRedisCommands::test_hincrby",
"tests/test_commands.py::TestRedisCommands::test_hincrbyfloat",
"tests/test_commands.py::TestRedisCommands::test_hkeys",
"tests/test_commands.py::TestRedisCommands::test_hlen",
"tests/test_commands.py::TestRedisCommands::test_hmget",
"tests/test_commands.py::TestRedisCommands::test_hmset",
"tests/test_commands.py::TestRedisCommands::test_hsetnx",
"tests/test_commands.py::TestRedisCommands::test_hvals",
"tests/test_commands.py::TestRedisCommands::test_hstrlen",
"tests/test_commands.py::TestRedisCommands::test_sort_basic",
"tests/test_commands.py::TestRedisCommands::test_sort_limited",
"tests/test_commands.py::TestRedisCommands::test_sort_by",
"tests/test_commands.py::TestRedisCommands::test_sort_get",
"tests/test_commands.py::TestRedisCommands::test_sort_get_multi",
"tests/test_commands.py::TestRedisCommands::test_sort_get_groups_two",
"tests/test_commands.py::TestRedisCommands::test_sort_groups_string_get",
"tests/test_commands.py::TestRedisCommands::test_sort_groups_just_one_get",
"tests/test_commands.py::TestRedisCommands::test_sort_groups_no_get",
"tests/test_commands.py::TestRedisCommands::test_sort_groups_three_gets",
"tests/test_commands.py::TestRedisCommands::test_sort_desc",
"tests/test_commands.py::TestRedisCommands::test_sort_alpha",
"tests/test_commands.py::TestRedisCommands::test_sort_store",
"tests/test_commands.py::TestRedisCommands::test_sort_all_options",
"tests/test_commands.py::TestRedisCommands::test_sort_ro",
"tests/test_commands.py::TestRedisCommands::test_sort_issue_924",
"tests/test_commands.py::TestRedisCommands::test_cluster_addslots",
"tests/test_commands.py::TestRedisCommands::test_cluster_count_failure_reports",
"tests/test_commands.py::TestRedisCommands::test_cluster_countkeysinslot",
"tests/test_commands.py::TestRedisCommands::test_cluster_delslots",
"tests/test_commands.py::TestRedisCommands::test_cluster_failover",
"tests/test_commands.py::TestRedisCommands::test_cluster_forget",
"tests/test_commands.py::TestRedisCommands::test_cluster_info",
"tests/test_commands.py::TestRedisCommands::test_cluster_keyslot",
"tests/test_commands.py::TestRedisCommands::test_cluster_meet",
"tests/test_commands.py::TestRedisCommands::test_cluster_nodes",
"tests/test_commands.py::TestRedisCommands::test_cluster_replicate",
"tests/test_commands.py::TestRedisCommands::test_cluster_reset",
"tests/test_commands.py::TestRedisCommands::test_cluster_saveconfig",
"tests/test_commands.py::TestRedisCommands::test_cluster_setslot",
"tests/test_commands.py::TestRedisCommands::test_cluster_slaves",
"tests/test_commands.py::TestRedisCommands::test_readonly_invalid_cluster_state",
"tests/test_commands.py::TestRedisCommands::test_readonly",
"tests/test_commands.py::TestRedisCommands::test_geoadd",
"tests/test_commands.py::TestRedisCommands::test_geoadd_nx",
"tests/test_commands.py::TestRedisCommands::test_geoadd_xx",
"tests/test_commands.py::TestRedisCommands::test_geoadd_ch",
"tests/test_commands.py::TestRedisCommands::test_geoadd_invalid_params",
"tests/test_commands.py::TestRedisCommands::test_geodist",
"tests/test_commands.py::TestRedisCommands::test_geodist_units",
"tests/test_commands.py::TestRedisCommands::test_geodist_missing_one_member",
"tests/test_commands.py::TestRedisCommands::test_geodist_invalid_units",
"tests/test_commands.py::TestRedisCommands::test_geohash",
"tests/test_commands.py::TestRedisCommands::test_geopos",
"tests/test_commands.py::TestRedisCommands::test_geopos_no_value",
"tests/test_commands.py::TestRedisCommands::test_geosearch",
"tests/test_commands.py::TestRedisCommands::test_geosearch_member",
"tests/test_commands.py::TestRedisCommands::test_geosearch_sort",
"tests/test_commands.py::TestRedisCommands::test_geosearch_with",
"tests/test_commands.py::TestRedisCommands::test_geosearch_negative",
"tests/test_commands.py::TestRedisCommands::test_geosearchstore",
"tests/test_commands.py::TestRedisCommands::test_geosearchstore_dist",
"tests/test_commands.py::TestRedisCommands::test_georadius",
"tests/test_commands.py::TestRedisCommands::test_georadius_no_values",
"tests/test_commands.py::TestRedisCommands::test_georadius_units",
"tests/test_commands.py::TestRedisCommands::test_georadius_with",
"tests/test_commands.py::TestRedisCommands::test_georadius_count",
"tests/test_commands.py::TestRedisCommands::test_georadius_sort",
"tests/test_commands.py::TestRedisCommands::test_georadius_store",
"tests/test_commands.py::TestRedisCommands::test_georadius_store_dist",
"tests/test_commands.py::TestRedisCommands::test_georadiusmember",
"tests/test_commands.py::TestRedisCommands::test_georadiusmember_count",
"tests/test_commands.py::TestRedisCommands::test_xack",
"tests/test_commands.py::TestRedisCommands::test_xadd",
"tests/test_commands.py::TestRedisCommands::test_xadd_nomkstream",
"tests/test_commands.py::TestRedisCommands::test_xadd_minlen_and_limit",
"tests/test_commands.py::TestRedisCommands::test_xautoclaim",
"tests/test_commands.py::TestRedisCommands::test_xautoclaim_negative",
"tests/test_commands.py::TestRedisCommands::test_xclaim",
"tests/test_commands.py::TestRedisCommands::test_xdel",
"tests/test_commands.py::TestRedisCommands::test_xgroup_delconsumer",
"tests/test_commands.py::TestRedisCommands::test_xgroup_createconsumer",
"tests/test_commands.py::TestRedisCommands::test_xgroup_destroy",
"tests/test_commands.py::TestRedisCommands::test_xinfo_stream",
"tests/test_commands.py::TestRedisCommands::test_xinfo_stream_full",
"tests/test_commands.py::TestRedisCommands::test_xlen",
"tests/test_commands.py::TestRedisCommands::test_xpending",
"tests/test_commands.py::TestRedisCommands::test_xpending_range",
"tests/test_commands.py::TestRedisCommands::test_xpending_range_idle",
"tests/test_commands.py::TestRedisCommands::test_xpending_range_negative",
"tests/test_commands.py::TestRedisCommands::test_xrange",
"tests/test_commands.py::TestRedisCommands::test_xread",
"tests/test_commands.py::TestRedisCommands::test_xreadgroup",
"tests/test_commands.py::TestRedisCommands::test_xrevrange",
"tests/test_commands.py::TestRedisCommands::test_xtrim",
"tests/test_commands.py::TestRedisCommands::test_xtrim_minlen_and_length_args",
"tests/test_commands.py::TestRedisCommands::test_bitfield_operations",
"tests/test_commands.py::TestRedisCommands::test_memory_help",
"tests/test_commands.py::TestRedisCommands::test_memory_doctor",
"tests/test_commands.py::TestRedisCommands::test_memory_malloc_stats",
"tests/test_commands.py::TestRedisCommands::test_memory_stats",
"tests/test_commands.py::TestRedisCommands::test_memory_usage",
"tests/test_commands.py::TestRedisCommands::test_module_list",
"tests/test_commands.py::TestRedisCommands::test_command_count",
"tests/test_commands.py::TestRedisCommands::test_command_docs",
"tests/test_commands.py::TestRedisCommands::test_command_getkeys",
"tests/test_commands.py::TestRedisCommands::test_command",
"tests/test_commands.py::TestRedisCommands::test_module",
"tests/test_commands.py::TestRedisCommands::test_restore",
"tests/test_commands.py::TestRedisCommands::test_restore_idletime",
"tests/test_commands.py::TestRedisCommands::test_restore_frequency",
"tests/test_commands.py::TestRedisCommands::test_replicaof",
"tests/test_commands.py::TestRedisCommands::test_sync",
"tests/test_commands.py::TestRedisCommands::test_psync",
"tests/test_commands.py::TestBinarySave::test_binary_get_set",
"tests/test_commands.py::TestBinarySave::test_binary_lists",
"tests/test_commands.py::TestBinarySave::test_22_info",
"tests/test_commands.py::TestBinarySave::test_large_responses",
"tests/test_commands.py::TestBinarySave::test_floating_point_encoding"
] | [
"tests/test_commands.py::TestRedisCommands::test_config_get_multi_params"
] | Python | [] | [] |
|
redis/redis-py | 2,143 | redis__redis-py-2143 | [
"2122"
] | 2d8b90139710240d172e16f1a60b2cd847a0802c | diff --git a/redis/commands/core.py b/redis/commands/core.py
--- a/redis/commands/core.py
+++ b/redis/commands/core.py
@@ -770,12 +770,18 @@ def config_get(
"""
return self.execute_command("CONFIG GET", pattern, *args, **kwargs)
- def config_set(self, name: KeyT, value: EncodableT, **kwargs) -> ResponseT:
+ def config_set(
+ self,
+ name: KeyT,
+ value: EncodableT,
+ *args: List[Union[KeyT, EncodableT]],
+ **kwargs,
+ ) -> ResponseT:
"""Set config item ``name`` with ``value``
For more information see https://redis.io/commands/config-set
"""
- return self.execute_command("CONFIG SET", name, value, **kwargs)
+ return self.execute_command("CONFIG SET", name, value, *args, **kwargs)
def config_resetstat(self, **kwargs) -> ResponseT:
"""
| diff --git a/tests/test_commands.py b/tests/test_commands.py
--- a/tests/test_commands.py
+++ b/tests/test_commands.py
@@ -692,6 +692,16 @@ def test_config_set(self, r):
assert r.config_set("timeout", 0)
assert r.config_get()["timeout"] == "0"
+ @skip_if_server_version_lt("7.0.0")
+ @skip_if_redis_enterprise()
+ def test_config_set_multi_params(self, r: redis.Redis):
+ r.config_set("timeout", 70, "maxmemory", 100)
+ assert r.config_get()["timeout"] == "70"
+ assert r.config_get()["maxmemory"] == "100"
+ assert r.config_set("timeout", 0, "maxmemory", 0)
+ assert r.config_get()["timeout"] == "0"
+ assert r.config_get()["maxmemory"] == "0"
+
@skip_if_server_version_lt("6.0.0")
@skip_if_redis_enterprise()
def test_failover(self, r):
| CONFIG SET - add the ability to set multiple parameters in one call
| "2022-04-26T14:41:23Z" | 4.2 | [
"tests/test_commands.py::TestResponseCallbacks::test_response_callbacks",
"tests/test_commands.py::TestResponseCallbacks::test_case_insensitive_command_names",
"tests/test_commands.py::TestRedisCommands::test_auth",
"tests/test_commands.py::TestRedisCommands::test_command_on_invalid_key_type",
"tests/test_commands.py::TestRedisCommands::test_acl_cat_no_category",
"tests/test_commands.py::TestRedisCommands::test_acl_cat_with_category",
"tests/test_commands.py::TestRedisCommands::test_acl_genpass",
"tests/test_commands.py::TestRedisCommands::test_acl_help",
"tests/test_commands.py::TestRedisCommands::test_acl_list",
"tests/test_commands.py::TestRedisCommands::test_acl_log",
"tests/test_commands.py::TestRedisCommands::test_acl_setuser_categories_without_prefix_fails",
"tests/test_commands.py::TestRedisCommands::test_acl_setuser_commands_without_prefix_fails",
"tests/test_commands.py::TestRedisCommands::test_acl_setuser_add_passwords_and_nopass_fails",
"tests/test_commands.py::TestRedisCommands::test_acl_users",
"tests/test_commands.py::TestRedisCommands::test_acl_whoami",
"tests/test_commands.py::TestRedisCommands::test_client_list",
"tests/test_commands.py::TestRedisCommands::test_client_info",
"tests/test_commands.py::TestRedisCommands::test_client_list_types_not_replica",
"tests/test_commands.py::TestRedisCommands::test_client_list_replica",
"tests/test_commands.py::TestRedisCommands::test_client_list_client_id",
"tests/test_commands.py::TestRedisCommands::test_client_id",
"tests/test_commands.py::TestRedisCommands::test_client_trackinginfo",
"tests/test_commands.py::TestRedisCommands::test_client_tracking",
"tests/test_commands.py::TestRedisCommands::test_client_unblock",
"tests/test_commands.py::TestRedisCommands::test_client_getname",
"tests/test_commands.py::TestRedisCommands::test_client_setname",
"tests/test_commands.py::TestRedisCommands::test_client_kill",
"tests/test_commands.py::TestRedisCommands::test_client_kill_filter_invalid_params",
"tests/test_commands.py::TestRedisCommands::test_client_kill_filter_by_id",
"tests/test_commands.py::TestRedisCommands::test_client_kill_filter_by_addr",
"tests/test_commands.py::TestRedisCommands::test_client_list_after_client_setname",
"tests/test_commands.py::TestRedisCommands::test_client_kill_filter_by_laddr",
"tests/test_commands.py::TestRedisCommands::test_client_kill_filter_by_user",
"tests/test_commands.py::TestRedisCommands::test_client_pause",
"tests/test_commands.py::TestRedisCommands::test_client_pause_all",
"tests/test_commands.py::TestRedisCommands::test_client_unpause",
"tests/test_commands.py::TestRedisCommands::test_client_reply",
"tests/test_commands.py::TestRedisCommands::test_client_getredir",
"tests/test_commands.py::TestRedisCommands::test_hello_notI_implemented",
"tests/test_commands.py::TestRedisCommands::test_config_get",
"tests/test_commands.py::TestRedisCommands::test_config_get_multi_params",
"tests/test_commands.py::TestRedisCommands::test_config_resetstat",
"tests/test_commands.py::TestRedisCommands::test_config_set",
"tests/test_commands.py::TestRedisCommands::test_failover",
"tests/test_commands.py::TestRedisCommands::test_dbsize",
"tests/test_commands.py::TestRedisCommands::test_echo",
"tests/test_commands.py::TestRedisCommands::test_info",
"tests/test_commands.py::TestRedisCommands::test_lastsave",
"tests/test_commands.py::TestRedisCommands::test_lolwut",
"tests/test_commands.py::TestRedisCommands::test_reset",
"tests/test_commands.py::TestRedisCommands::test_object",
"tests/test_commands.py::TestRedisCommands::test_ping",
"tests/test_commands.py::TestRedisCommands::test_quit",
"tests/test_commands.py::TestRedisCommands::test_role",
"tests/test_commands.py::TestRedisCommands::test_select",
"tests/test_commands.py::TestRedisCommands::test_slowlog_get",
"tests/test_commands.py::TestRedisCommands::test_slowlog_get_limit",
"tests/test_commands.py::TestRedisCommands::test_slowlog_length",
"tests/test_commands.py::TestRedisCommands::test_time",
"tests/test_commands.py::TestRedisCommands::test_bgsave",
"tests/test_commands.py::TestRedisCommands::test_append",
"tests/test_commands.py::TestRedisCommands::test_bitcount",
"tests/test_commands.py::TestRedisCommands::test_bitcount_mode",
"tests/test_commands.py::TestRedisCommands::test_bitop_not_empty_string",
"tests/test_commands.py::TestRedisCommands::test_bitop_not",
"tests/test_commands.py::TestRedisCommands::test_bitop_not_in_place",
"tests/test_commands.py::TestRedisCommands::test_bitop_single_string",
"tests/test_commands.py::TestRedisCommands::test_bitop_string_operands",
"tests/test_commands.py::TestRedisCommands::test_bitpos",
"tests/test_commands.py::TestRedisCommands::test_bitpos_wrong_arguments",
"tests/test_commands.py::TestRedisCommands::test_bitpos_mode",
"tests/test_commands.py::TestRedisCommands::test_copy",
"tests/test_commands.py::TestRedisCommands::test_copy_and_replace",
"tests/test_commands.py::TestRedisCommands::test_copy_to_another_database",
"tests/test_commands.py::TestRedisCommands::test_decr",
"tests/test_commands.py::TestRedisCommands::test_decrby",
"tests/test_commands.py::TestRedisCommands::test_delete",
"tests/test_commands.py::TestRedisCommands::test_delete_with_multiple_keys",
"tests/test_commands.py::TestRedisCommands::test_delitem",
"tests/test_commands.py::TestRedisCommands::test_unlink",
"tests/test_commands.py::TestRedisCommands::test_unlink_with_multiple_keys",
"tests/test_commands.py::TestRedisCommands::test_dump_and_restore",
"tests/test_commands.py::TestRedisCommands::test_dump_and_restore_and_replace",
"tests/test_commands.py::TestRedisCommands::test_dump_and_restore_absttl",
"tests/test_commands.py::TestRedisCommands::test_exists",
"tests/test_commands.py::TestRedisCommands::test_exists_contains",
"tests/test_commands.py::TestRedisCommands::test_expire",
"tests/test_commands.py::TestRedisCommands::test_expire_option_nx",
"tests/test_commands.py::TestRedisCommands::test_expire_option_gt",
"tests/test_commands.py::TestRedisCommands::test_expire_option_lt",
"tests/test_commands.py::TestRedisCommands::test_expireat_datetime",
"tests/test_commands.py::TestRedisCommands::test_expireat_no_key",
"tests/test_commands.py::TestRedisCommands::test_expireat_unixtime",
"tests/test_commands.py::TestRedisCommands::test_expiretime",
"tests/test_commands.py::TestRedisCommands::test_expireat_option_nx",
"tests/test_commands.py::TestRedisCommands::test_expireat_option_xx",
"tests/test_commands.py::TestRedisCommands::test_expireat_option_gt",
"tests/test_commands.py::TestRedisCommands::test_expireat_option_lt",
"tests/test_commands.py::TestRedisCommands::test_get_and_set",
"tests/test_commands.py::TestRedisCommands::test_getdel",
"tests/test_commands.py::TestRedisCommands::test_getex",
"tests/test_commands.py::TestRedisCommands::test_getitem_and_setitem",
"tests/test_commands.py::TestRedisCommands::test_getitem_raises_keyerror_for_missing_key",
"tests/test_commands.py::TestRedisCommands::test_getitem_does_not_raise_keyerror_for_empty_string",
"tests/test_commands.py::TestRedisCommands::test_get_set_bit",
"tests/test_commands.py::TestRedisCommands::test_getrange",
"tests/test_commands.py::TestRedisCommands::test_getset",
"tests/test_commands.py::TestRedisCommands::test_incr",
"tests/test_commands.py::TestRedisCommands::test_incrby",
"tests/test_commands.py::TestRedisCommands::test_incrbyfloat",
"tests/test_commands.py::TestRedisCommands::test_keys",
"tests/test_commands.py::TestRedisCommands::test_mget",
"tests/test_commands.py::TestRedisCommands::test_lmove",
"tests/test_commands.py::TestRedisCommands::test_blmove",
"tests/test_commands.py::TestRedisCommands::test_mset",
"tests/test_commands.py::TestRedisCommands::test_msetnx",
"tests/test_commands.py::TestRedisCommands::test_pexpire",
"tests/test_commands.py::TestRedisCommands::test_pexpire_option_nx",
"tests/test_commands.py::TestRedisCommands::test_pexpire_option_xx",
"tests/test_commands.py::TestRedisCommands::test_pexpire_option_gt",
"tests/test_commands.py::TestRedisCommands::test_pexpire_option_lt",
"tests/test_commands.py::TestRedisCommands::test_pexpireat_datetime",
"tests/test_commands.py::TestRedisCommands::test_pexpireat_no_key",
"tests/test_commands.py::TestRedisCommands::test_pexpireat_unixtime",
"tests/test_commands.py::TestRedisCommands::test_pexpireat_option_nx",
"tests/test_commands.py::TestRedisCommands::test_pexpireat_option_xx",
"tests/test_commands.py::TestRedisCommands::test_pexpireat_option_gt",
"tests/test_commands.py::TestRedisCommands::test_pexpireat_option_lt",
"tests/test_commands.py::TestRedisCommands::test_pexpiretime",
"tests/test_commands.py::TestRedisCommands::test_psetex",
"tests/test_commands.py::TestRedisCommands::test_psetex_timedelta",
"tests/test_commands.py::TestRedisCommands::test_pttl",
"tests/test_commands.py::TestRedisCommands::test_pttl_no_key",
"tests/test_commands.py::TestRedisCommands::test_hrandfield",
"tests/test_commands.py::TestRedisCommands::test_randomkey",
"tests/test_commands.py::TestRedisCommands::test_rename",
"tests/test_commands.py::TestRedisCommands::test_renamenx",
"tests/test_commands.py::TestRedisCommands::test_set_nx",
"tests/test_commands.py::TestRedisCommands::test_set_xx",
"tests/test_commands.py::TestRedisCommands::test_set_px",
"tests/test_commands.py::TestRedisCommands::test_set_px_timedelta",
"tests/test_commands.py::TestRedisCommands::test_set_ex",
"tests/test_commands.py::TestRedisCommands::test_set_ex_timedelta",
"tests/test_commands.py::TestRedisCommands::test_set_exat_timedelta",
"tests/test_commands.py::TestRedisCommands::test_set_pxat_timedelta",
"tests/test_commands.py::TestRedisCommands::test_set_multipleoptions",
"tests/test_commands.py::TestRedisCommands::test_set_keepttl",
"tests/test_commands.py::TestRedisCommands::test_set_get",
"tests/test_commands.py::TestRedisCommands::test_setex",
"tests/test_commands.py::TestRedisCommands::test_setnx",
"tests/test_commands.py::TestRedisCommands::test_setrange",
"tests/test_commands.py::TestRedisCommands::test_strlen",
"tests/test_commands.py::TestRedisCommands::test_substr",
"tests/test_commands.py::TestRedisCommands::test_ttl",
"tests/test_commands.py::TestRedisCommands::test_ttl_nokey",
"tests/test_commands.py::TestRedisCommands::test_type",
"tests/test_commands.py::TestRedisCommands::test_blpop",
"tests/test_commands.py::TestRedisCommands::test_brpop",
"tests/test_commands.py::TestRedisCommands::test_brpoplpush",
"tests/test_commands.py::TestRedisCommands::test_brpoplpush_empty_string",
"tests/test_commands.py::TestRedisCommands::test_lindex",
"tests/test_commands.py::TestRedisCommands::test_linsert",
"tests/test_commands.py::TestRedisCommands::test_llen",
"tests/test_commands.py::TestRedisCommands::test_lpop",
"tests/test_commands.py::TestRedisCommands::test_lpop_count",
"tests/test_commands.py::TestRedisCommands::test_lpush",
"tests/test_commands.py::TestRedisCommands::test_lpushx",
"tests/test_commands.py::TestRedisCommands::test_lpushx_with_list",
"tests/test_commands.py::TestRedisCommands::test_lrange",
"tests/test_commands.py::TestRedisCommands::test_lrem",
"tests/test_commands.py::TestRedisCommands::test_lset",
"tests/test_commands.py::TestRedisCommands::test_ltrim",
"tests/test_commands.py::TestRedisCommands::test_rpop",
"tests/test_commands.py::TestRedisCommands::test_rpop_count",
"tests/test_commands.py::TestRedisCommands::test_rpoplpush",
"tests/test_commands.py::TestRedisCommands::test_rpush",
"tests/test_commands.py::TestRedisCommands::test_lpos",
"tests/test_commands.py::TestRedisCommands::test_rpushx",
"tests/test_commands.py::TestRedisCommands::test_scan",
"tests/test_commands.py::TestRedisCommands::test_scan_type",
"tests/test_commands.py::TestRedisCommands::test_scan_iter",
"tests/test_commands.py::TestRedisCommands::test_sscan",
"tests/test_commands.py::TestRedisCommands::test_sscan_iter",
"tests/test_commands.py::TestRedisCommands::test_hscan",
"tests/test_commands.py::TestRedisCommands::test_hscan_iter",
"tests/test_commands.py::TestRedisCommands::test_zscan",
"tests/test_commands.py::TestRedisCommands::test_zscan_iter",
"tests/test_commands.py::TestRedisCommands::test_sadd",
"tests/test_commands.py::TestRedisCommands::test_scard",
"tests/test_commands.py::TestRedisCommands::test_sdiff",
"tests/test_commands.py::TestRedisCommands::test_sdiffstore",
"tests/test_commands.py::TestRedisCommands::test_sinter",
"tests/test_commands.py::TestRedisCommands::test_sintercard",
"tests/test_commands.py::TestRedisCommands::test_sinterstore",
"tests/test_commands.py::TestRedisCommands::test_sismember",
"tests/test_commands.py::TestRedisCommands::test_smembers",
"tests/test_commands.py::TestRedisCommands::test_smismember",
"tests/test_commands.py::TestRedisCommands::test_smove",
"tests/test_commands.py::TestRedisCommands::test_spop",
"tests/test_commands.py::TestRedisCommands::test_spop_multi_value",
"tests/test_commands.py::TestRedisCommands::test_srandmember",
"tests/test_commands.py::TestRedisCommands::test_srandmember_multi_value",
"tests/test_commands.py::TestRedisCommands::test_srem",
"tests/test_commands.py::TestRedisCommands::test_sunion",
"tests/test_commands.py::TestRedisCommands::test_sunionstore",
"tests/test_commands.py::TestRedisCommands::test_debug_segfault",
"tests/test_commands.py::TestRedisCommands::test_script_debug",
"tests/test_commands.py::TestRedisCommands::test_zadd",
"tests/test_commands.py::TestRedisCommands::test_zadd_nx",
"tests/test_commands.py::TestRedisCommands::test_zadd_xx",
"tests/test_commands.py::TestRedisCommands::test_zadd_ch",
"tests/test_commands.py::TestRedisCommands::test_zadd_incr",
"tests/test_commands.py::TestRedisCommands::test_zadd_incr_with_xx",
"tests/test_commands.py::TestRedisCommands::test_zadd_gt_lt",
"tests/test_commands.py::TestRedisCommands::test_zcard",
"tests/test_commands.py::TestRedisCommands::test_zcount",
"tests/test_commands.py::TestRedisCommands::test_zdiff",
"tests/test_commands.py::TestRedisCommands::test_zdiffstore",
"tests/test_commands.py::TestRedisCommands::test_zincrby",
"tests/test_commands.py::TestRedisCommands::test_zlexcount",
"tests/test_commands.py::TestRedisCommands::test_zinter",
"tests/test_commands.py::TestRedisCommands::test_zintercard",
"tests/test_commands.py::TestRedisCommands::test_zinterstore_sum",
"tests/test_commands.py::TestRedisCommands::test_zinterstore_max",
"tests/test_commands.py::TestRedisCommands::test_zinterstore_min",
"tests/test_commands.py::TestRedisCommands::test_zinterstore_with_weight",
"tests/test_commands.py::TestRedisCommands::test_zpopmax",
"tests/test_commands.py::TestRedisCommands::test_zpopmin",
"tests/test_commands.py::TestRedisCommands::test_zrandemember",
"tests/test_commands.py::TestRedisCommands::test_bzpopmax",
"tests/test_commands.py::TestRedisCommands::test_bzpopmin",
"tests/test_commands.py::TestRedisCommands::test_zrange",
"tests/test_commands.py::TestRedisCommands::test_zrange_errors",
"tests/test_commands.py::TestRedisCommands::test_zrange_params",
"tests/test_commands.py::TestRedisCommands::test_zrangestore",
"tests/test_commands.py::TestRedisCommands::test_zrangebylex",
"tests/test_commands.py::TestRedisCommands::test_zrevrangebylex",
"tests/test_commands.py::TestRedisCommands::test_zrangebyscore",
"tests/test_commands.py::TestRedisCommands::test_zrank",
"tests/test_commands.py::TestRedisCommands::test_zrem",
"tests/test_commands.py::TestRedisCommands::test_zrem_multiple_keys",
"tests/test_commands.py::TestRedisCommands::test_zremrangebylex",
"tests/test_commands.py::TestRedisCommands::test_zremrangebyrank",
"tests/test_commands.py::TestRedisCommands::test_zremrangebyscore",
"tests/test_commands.py::TestRedisCommands::test_zrevrange",
"tests/test_commands.py::TestRedisCommands::test_zrevrangebyscore",
"tests/test_commands.py::TestRedisCommands::test_zrevrank",
"tests/test_commands.py::TestRedisCommands::test_zscore",
"tests/test_commands.py::TestRedisCommands::test_zunion",
"tests/test_commands.py::TestRedisCommands::test_zunionstore_sum",
"tests/test_commands.py::TestRedisCommands::test_zunionstore_max",
"tests/test_commands.py::TestRedisCommands::test_zunionstore_min",
"tests/test_commands.py::TestRedisCommands::test_zunionstore_with_weight",
"tests/test_commands.py::TestRedisCommands::test_zmscore",
"tests/test_commands.py::TestRedisCommands::test_pfadd",
"tests/test_commands.py::TestRedisCommands::test_pfcount",
"tests/test_commands.py::TestRedisCommands::test_pfmerge",
"tests/test_commands.py::TestRedisCommands::test_hget_and_hset",
"tests/test_commands.py::TestRedisCommands::test_hset_with_multi_key_values",
"tests/test_commands.py::TestRedisCommands::test_hset_with_key_values_passed_as_list",
"tests/test_commands.py::TestRedisCommands::test_hset_without_data",
"tests/test_commands.py::TestRedisCommands::test_hdel",
"tests/test_commands.py::TestRedisCommands::test_hexists",
"tests/test_commands.py::TestRedisCommands::test_hgetall",
"tests/test_commands.py::TestRedisCommands::test_hincrby",
"tests/test_commands.py::TestRedisCommands::test_hincrbyfloat",
"tests/test_commands.py::TestRedisCommands::test_hkeys",
"tests/test_commands.py::TestRedisCommands::test_hlen",
"tests/test_commands.py::TestRedisCommands::test_hmget",
"tests/test_commands.py::TestRedisCommands::test_hmset",
"tests/test_commands.py::TestRedisCommands::test_hsetnx",
"tests/test_commands.py::TestRedisCommands::test_hvals",
"tests/test_commands.py::TestRedisCommands::test_hstrlen",
"tests/test_commands.py::TestRedisCommands::test_sort_basic",
"tests/test_commands.py::TestRedisCommands::test_sort_limited",
"tests/test_commands.py::TestRedisCommands::test_sort_by",
"tests/test_commands.py::TestRedisCommands::test_sort_get",
"tests/test_commands.py::TestRedisCommands::test_sort_get_multi",
"tests/test_commands.py::TestRedisCommands::test_sort_get_groups_two",
"tests/test_commands.py::TestRedisCommands::test_sort_groups_string_get",
"tests/test_commands.py::TestRedisCommands::test_sort_groups_just_one_get",
"tests/test_commands.py::TestRedisCommands::test_sort_groups_no_get",
"tests/test_commands.py::TestRedisCommands::test_sort_groups_three_gets",
"tests/test_commands.py::TestRedisCommands::test_sort_desc",
"tests/test_commands.py::TestRedisCommands::test_sort_alpha",
"tests/test_commands.py::TestRedisCommands::test_sort_store",
"tests/test_commands.py::TestRedisCommands::test_sort_all_options",
"tests/test_commands.py::TestRedisCommands::test_sort_ro",
"tests/test_commands.py::TestRedisCommands::test_sort_issue_924",
"tests/test_commands.py::TestRedisCommands::test_cluster_addslots",
"tests/test_commands.py::TestRedisCommands::test_cluster_count_failure_reports",
"tests/test_commands.py::TestRedisCommands::test_cluster_countkeysinslot",
"tests/test_commands.py::TestRedisCommands::test_cluster_delslots",
"tests/test_commands.py::TestRedisCommands::test_cluster_failover",
"tests/test_commands.py::TestRedisCommands::test_cluster_forget",
"tests/test_commands.py::TestRedisCommands::test_cluster_info",
"tests/test_commands.py::TestRedisCommands::test_cluster_keyslot",
"tests/test_commands.py::TestRedisCommands::test_cluster_meet",
"tests/test_commands.py::TestRedisCommands::test_cluster_nodes",
"tests/test_commands.py::TestRedisCommands::test_cluster_replicate",
"tests/test_commands.py::TestRedisCommands::test_cluster_reset",
"tests/test_commands.py::TestRedisCommands::test_cluster_saveconfig",
"tests/test_commands.py::TestRedisCommands::test_cluster_setslot",
"tests/test_commands.py::TestRedisCommands::test_cluster_slaves",
"tests/test_commands.py::TestRedisCommands::test_readonly_invalid_cluster_state",
"tests/test_commands.py::TestRedisCommands::test_readonly",
"tests/test_commands.py::TestRedisCommands::test_geoadd",
"tests/test_commands.py::TestRedisCommands::test_geoadd_nx",
"tests/test_commands.py::TestRedisCommands::test_geoadd_xx",
"tests/test_commands.py::TestRedisCommands::test_geoadd_ch",
"tests/test_commands.py::TestRedisCommands::test_geoadd_invalid_params",
"tests/test_commands.py::TestRedisCommands::test_geodist",
"tests/test_commands.py::TestRedisCommands::test_geodist_units",
"tests/test_commands.py::TestRedisCommands::test_geodist_missing_one_member",
"tests/test_commands.py::TestRedisCommands::test_geodist_invalid_units",
"tests/test_commands.py::TestRedisCommands::test_geohash",
"tests/test_commands.py::TestRedisCommands::test_geopos",
"tests/test_commands.py::TestRedisCommands::test_geopos_no_value",
"tests/test_commands.py::TestRedisCommands::test_geosearch",
"tests/test_commands.py::TestRedisCommands::test_geosearch_member",
"tests/test_commands.py::TestRedisCommands::test_geosearch_sort",
"tests/test_commands.py::TestRedisCommands::test_geosearch_with",
"tests/test_commands.py::TestRedisCommands::test_geosearch_negative",
"tests/test_commands.py::TestRedisCommands::test_geosearchstore",
"tests/test_commands.py::TestRedisCommands::test_geosearchstore_dist",
"tests/test_commands.py::TestRedisCommands::test_georadius",
"tests/test_commands.py::TestRedisCommands::test_georadius_no_values",
"tests/test_commands.py::TestRedisCommands::test_georadius_units",
"tests/test_commands.py::TestRedisCommands::test_georadius_with",
"tests/test_commands.py::TestRedisCommands::test_georadius_count",
"tests/test_commands.py::TestRedisCommands::test_georadius_sort",
"tests/test_commands.py::TestRedisCommands::test_georadius_store",
"tests/test_commands.py::TestRedisCommands::test_georadius_store_dist",
"tests/test_commands.py::TestRedisCommands::test_georadiusmember",
"tests/test_commands.py::TestRedisCommands::test_georadiusmember_count",
"tests/test_commands.py::TestRedisCommands::test_xack",
"tests/test_commands.py::TestRedisCommands::test_xadd",
"tests/test_commands.py::TestRedisCommands::test_xadd_nomkstream",
"tests/test_commands.py::TestRedisCommands::test_xadd_minlen_and_limit",
"tests/test_commands.py::TestRedisCommands::test_xautoclaim",
"tests/test_commands.py::TestRedisCommands::test_xautoclaim_negative",
"tests/test_commands.py::TestRedisCommands::test_xclaim",
"tests/test_commands.py::TestRedisCommands::test_xdel",
"tests/test_commands.py::TestRedisCommands::test_xgroup_delconsumer",
"tests/test_commands.py::TestRedisCommands::test_xgroup_createconsumer",
"tests/test_commands.py::TestRedisCommands::test_xgroup_destroy",
"tests/test_commands.py::TestRedisCommands::test_xinfo_stream",
"tests/test_commands.py::TestRedisCommands::test_xinfo_stream_full",
"tests/test_commands.py::TestRedisCommands::test_xlen",
"tests/test_commands.py::TestRedisCommands::test_xpending",
"tests/test_commands.py::TestRedisCommands::test_xpending_range",
"tests/test_commands.py::TestRedisCommands::test_xpending_range_idle",
"tests/test_commands.py::TestRedisCommands::test_xpending_range_negative",
"tests/test_commands.py::TestRedisCommands::test_xrange",
"tests/test_commands.py::TestRedisCommands::test_xread",
"tests/test_commands.py::TestRedisCommands::test_xreadgroup",
"tests/test_commands.py::TestRedisCommands::test_xrevrange",
"tests/test_commands.py::TestRedisCommands::test_xtrim",
"tests/test_commands.py::TestRedisCommands::test_xtrim_minlen_and_length_args",
"tests/test_commands.py::TestRedisCommands::test_bitfield_operations",
"tests/test_commands.py::TestRedisCommands::test_memory_help",
"tests/test_commands.py::TestRedisCommands::test_memory_doctor",
"tests/test_commands.py::TestRedisCommands::test_memory_malloc_stats",
"tests/test_commands.py::TestRedisCommands::test_memory_stats",
"tests/test_commands.py::TestRedisCommands::test_memory_usage",
"tests/test_commands.py::TestRedisCommands::test_module_list",
"tests/test_commands.py::TestRedisCommands::test_command_count",
"tests/test_commands.py::TestRedisCommands::test_command_docs",
"tests/test_commands.py::TestRedisCommands::test_command_getkeys",
"tests/test_commands.py::TestRedisCommands::test_command",
"tests/test_commands.py::TestRedisCommands::test_module",
"tests/test_commands.py::TestRedisCommands::test_restore",
"tests/test_commands.py::TestRedisCommands::test_restore_idletime",
"tests/test_commands.py::TestRedisCommands::test_restore_frequency",
"tests/test_commands.py::TestRedisCommands::test_replicaof",
"tests/test_commands.py::TestRedisCommands::test_sync",
"tests/test_commands.py::TestRedisCommands::test_psync",
"tests/test_commands.py::TestBinarySave::test_binary_get_set",
"tests/test_commands.py::TestBinarySave::test_binary_lists",
"tests/test_commands.py::TestBinarySave::test_22_info",
"tests/test_commands.py::TestBinarySave::test_large_responses",
"tests/test_commands.py::TestBinarySave::test_floating_point_encoding"
] | [
"tests/test_commands.py::TestRedisCommands::test_config_set_multi_params"
] | Python | [] | [] |
|
redis/redis-py | 2,145 | redis__redis-py-2145 | [
"2123"
] | c198612a3ed67d78d7964b0253e96b3fa1d7d707 | diff --git a/redis/commands/core.py b/redis/commands/core.py
--- a/redis/commands/core.py
+++ b/redis/commands/core.py
@@ -900,7 +900,9 @@ def select(self, index: int, **kwargs) -> ResponseT:
"""
return self.execute_command("SELECT", index, **kwargs)
- def info(self, section: Union[str, None] = None, **kwargs) -> ResponseT:
+ def info(
+ self, section: Union[str, None] = None, *args: List[str], **kwargs
+ ) -> ResponseT:
"""
Returns a dictionary containing information about the Redis server
@@ -915,7 +917,7 @@ def info(self, section: Union[str, None] = None, **kwargs) -> ResponseT:
if section is None:
return self.execute_command("INFO", **kwargs)
else:
- return self.execute_command("INFO", section, **kwargs)
+ return self.execute_command("INFO", section, *args, **kwargs)
def lastsave(self, **kwargs) -> ResponseT:
"""
| diff --git a/tests/test_commands.py b/tests/test_commands.py
--- a/tests/test_commands.py
+++ b/tests/test_commands.py
@@ -727,6 +727,14 @@ def test_info(self, r):
assert "arch_bits" in info.keys()
assert "redis_version" in info.keys()
+ @pytest.mark.onlynoncluster
+ @skip_if_server_version_lt("7.0.0")
+ def test_info_multi_sections(self, r):
+ res = r.info("clients", "server")
+ assert isinstance(res, dict)
+ assert "redis_version" in res
+ assert "connected_clients" in res
+
@pytest.mark.onlynoncluster
@skip_if_redis_enterprise()
def test_lastsave(self, r):
| INFO - add support for taking multiple section arguments
| "2022-04-27T10:16:56Z" | 4.2 | [
"tests/test_commands.py::TestResponseCallbacks::test_response_callbacks",
"tests/test_commands.py::TestResponseCallbacks::test_case_insensitive_command_names",
"tests/test_commands.py::TestRedisCommands::test_auth",
"tests/test_commands.py::TestRedisCommands::test_command_on_invalid_key_type",
"tests/test_commands.py::TestRedisCommands::test_acl_cat_no_category",
"tests/test_commands.py::TestRedisCommands::test_acl_cat_with_category",
"tests/test_commands.py::TestRedisCommands::test_acl_genpass",
"tests/test_commands.py::TestRedisCommands::test_acl_help",
"tests/test_commands.py::TestRedisCommands::test_acl_list",
"tests/test_commands.py::TestRedisCommands::test_acl_log",
"tests/test_commands.py::TestRedisCommands::test_acl_setuser_categories_without_prefix_fails",
"tests/test_commands.py::TestRedisCommands::test_acl_setuser_commands_without_prefix_fails",
"tests/test_commands.py::TestRedisCommands::test_acl_setuser_add_passwords_and_nopass_fails",
"tests/test_commands.py::TestRedisCommands::test_acl_users",
"tests/test_commands.py::TestRedisCommands::test_acl_whoami",
"tests/test_commands.py::TestRedisCommands::test_client_list",
"tests/test_commands.py::TestRedisCommands::test_client_info",
"tests/test_commands.py::TestRedisCommands::test_client_list_types_not_replica",
"tests/test_commands.py::TestRedisCommands::test_client_list_replica",
"tests/test_commands.py::TestRedisCommands::test_client_list_client_id",
"tests/test_commands.py::TestRedisCommands::test_client_id",
"tests/test_commands.py::TestRedisCommands::test_client_trackinginfo",
"tests/test_commands.py::TestRedisCommands::test_client_tracking",
"tests/test_commands.py::TestRedisCommands::test_client_unblock",
"tests/test_commands.py::TestRedisCommands::test_client_getname",
"tests/test_commands.py::TestRedisCommands::test_client_setname",
"tests/test_commands.py::TestRedisCommands::test_client_kill",
"tests/test_commands.py::TestRedisCommands::test_client_kill_filter_invalid_params",
"tests/test_commands.py::TestRedisCommands::test_client_kill_filter_by_id",
"tests/test_commands.py::TestRedisCommands::test_client_kill_filter_by_addr",
"tests/test_commands.py::TestRedisCommands::test_client_list_after_client_setname",
"tests/test_commands.py::TestRedisCommands::test_client_kill_filter_by_laddr",
"tests/test_commands.py::TestRedisCommands::test_client_kill_filter_by_user",
"tests/test_commands.py::TestRedisCommands::test_client_pause",
"tests/test_commands.py::TestRedisCommands::test_client_pause_all",
"tests/test_commands.py::TestRedisCommands::test_client_unpause",
"tests/test_commands.py::TestRedisCommands::test_client_reply",
"tests/test_commands.py::TestRedisCommands::test_client_getredir",
"tests/test_commands.py::TestRedisCommands::test_hello_notI_implemented",
"tests/test_commands.py::TestRedisCommands::test_config_get",
"tests/test_commands.py::TestRedisCommands::test_config_get_multi_params",
"tests/test_commands.py::TestRedisCommands::test_config_resetstat",
"tests/test_commands.py::TestRedisCommands::test_config_set",
"tests/test_commands.py::TestRedisCommands::test_config_set_multi_params",
"tests/test_commands.py::TestRedisCommands::test_failover",
"tests/test_commands.py::TestRedisCommands::test_dbsize",
"tests/test_commands.py::TestRedisCommands::test_echo",
"tests/test_commands.py::TestRedisCommands::test_info",
"tests/test_commands.py::TestRedisCommands::test_lastsave",
"tests/test_commands.py::TestRedisCommands::test_lolwut",
"tests/test_commands.py::TestRedisCommands::test_reset",
"tests/test_commands.py::TestRedisCommands::test_object",
"tests/test_commands.py::TestRedisCommands::test_ping",
"tests/test_commands.py::TestRedisCommands::test_quit",
"tests/test_commands.py::TestRedisCommands::test_role",
"tests/test_commands.py::TestRedisCommands::test_select",
"tests/test_commands.py::TestRedisCommands::test_slowlog_get",
"tests/test_commands.py::TestRedisCommands::test_slowlog_get_limit",
"tests/test_commands.py::TestRedisCommands::test_slowlog_length",
"tests/test_commands.py::TestRedisCommands::test_time",
"tests/test_commands.py::TestRedisCommands::test_bgsave",
"tests/test_commands.py::TestRedisCommands::test_append",
"tests/test_commands.py::TestRedisCommands::test_bitcount",
"tests/test_commands.py::TestRedisCommands::test_bitcount_mode",
"tests/test_commands.py::TestRedisCommands::test_bitop_not_empty_string",
"tests/test_commands.py::TestRedisCommands::test_bitop_not",
"tests/test_commands.py::TestRedisCommands::test_bitop_not_in_place",
"tests/test_commands.py::TestRedisCommands::test_bitop_single_string",
"tests/test_commands.py::TestRedisCommands::test_bitop_string_operands",
"tests/test_commands.py::TestRedisCommands::test_bitpos",
"tests/test_commands.py::TestRedisCommands::test_bitpos_wrong_arguments",
"tests/test_commands.py::TestRedisCommands::test_bitpos_mode",
"tests/test_commands.py::TestRedisCommands::test_copy",
"tests/test_commands.py::TestRedisCommands::test_copy_and_replace",
"tests/test_commands.py::TestRedisCommands::test_copy_to_another_database",
"tests/test_commands.py::TestRedisCommands::test_decr",
"tests/test_commands.py::TestRedisCommands::test_decrby",
"tests/test_commands.py::TestRedisCommands::test_delete",
"tests/test_commands.py::TestRedisCommands::test_delete_with_multiple_keys",
"tests/test_commands.py::TestRedisCommands::test_delitem",
"tests/test_commands.py::TestRedisCommands::test_unlink",
"tests/test_commands.py::TestRedisCommands::test_unlink_with_multiple_keys",
"tests/test_commands.py::TestRedisCommands::test_dump_and_restore",
"tests/test_commands.py::TestRedisCommands::test_dump_and_restore_and_replace",
"tests/test_commands.py::TestRedisCommands::test_dump_and_restore_absttl",
"tests/test_commands.py::TestRedisCommands::test_exists",
"tests/test_commands.py::TestRedisCommands::test_exists_contains",
"tests/test_commands.py::TestRedisCommands::test_expire",
"tests/test_commands.py::TestRedisCommands::test_expire_option_nx",
"tests/test_commands.py::TestRedisCommands::test_expire_option_gt",
"tests/test_commands.py::TestRedisCommands::test_expire_option_lt",
"tests/test_commands.py::TestRedisCommands::test_expireat_datetime",
"tests/test_commands.py::TestRedisCommands::test_expireat_no_key",
"tests/test_commands.py::TestRedisCommands::test_expireat_unixtime",
"tests/test_commands.py::TestRedisCommands::test_expiretime",
"tests/test_commands.py::TestRedisCommands::test_expireat_option_nx",
"tests/test_commands.py::TestRedisCommands::test_expireat_option_xx",
"tests/test_commands.py::TestRedisCommands::test_expireat_option_gt",
"tests/test_commands.py::TestRedisCommands::test_expireat_option_lt",
"tests/test_commands.py::TestRedisCommands::test_get_and_set",
"tests/test_commands.py::TestRedisCommands::test_getdel",
"tests/test_commands.py::TestRedisCommands::test_getex",
"tests/test_commands.py::TestRedisCommands::test_getitem_and_setitem",
"tests/test_commands.py::TestRedisCommands::test_getitem_raises_keyerror_for_missing_key",
"tests/test_commands.py::TestRedisCommands::test_getitem_does_not_raise_keyerror_for_empty_string",
"tests/test_commands.py::TestRedisCommands::test_get_set_bit",
"tests/test_commands.py::TestRedisCommands::test_getrange",
"tests/test_commands.py::TestRedisCommands::test_getset",
"tests/test_commands.py::TestRedisCommands::test_incr",
"tests/test_commands.py::TestRedisCommands::test_incrby",
"tests/test_commands.py::TestRedisCommands::test_incrbyfloat",
"tests/test_commands.py::TestRedisCommands::test_keys",
"tests/test_commands.py::TestRedisCommands::test_mget",
"tests/test_commands.py::TestRedisCommands::test_lmove",
"tests/test_commands.py::TestRedisCommands::test_blmove",
"tests/test_commands.py::TestRedisCommands::test_mset",
"tests/test_commands.py::TestRedisCommands::test_msetnx",
"tests/test_commands.py::TestRedisCommands::test_pexpire",
"tests/test_commands.py::TestRedisCommands::test_pexpire_option_nx",
"tests/test_commands.py::TestRedisCommands::test_pexpire_option_xx",
"tests/test_commands.py::TestRedisCommands::test_pexpire_option_gt",
"tests/test_commands.py::TestRedisCommands::test_pexpire_option_lt",
"tests/test_commands.py::TestRedisCommands::test_pexpireat_datetime",
"tests/test_commands.py::TestRedisCommands::test_pexpireat_no_key",
"tests/test_commands.py::TestRedisCommands::test_pexpireat_unixtime",
"tests/test_commands.py::TestRedisCommands::test_pexpireat_option_nx",
"tests/test_commands.py::TestRedisCommands::test_pexpireat_option_xx",
"tests/test_commands.py::TestRedisCommands::test_pexpireat_option_gt",
"tests/test_commands.py::TestRedisCommands::test_pexpireat_option_lt",
"tests/test_commands.py::TestRedisCommands::test_pexpiretime",
"tests/test_commands.py::TestRedisCommands::test_psetex",
"tests/test_commands.py::TestRedisCommands::test_psetex_timedelta",
"tests/test_commands.py::TestRedisCommands::test_pttl",
"tests/test_commands.py::TestRedisCommands::test_pttl_no_key",
"tests/test_commands.py::TestRedisCommands::test_hrandfield",
"tests/test_commands.py::TestRedisCommands::test_randomkey",
"tests/test_commands.py::TestRedisCommands::test_rename",
"tests/test_commands.py::TestRedisCommands::test_renamenx",
"tests/test_commands.py::TestRedisCommands::test_set_nx",
"tests/test_commands.py::TestRedisCommands::test_set_xx",
"tests/test_commands.py::TestRedisCommands::test_set_px",
"tests/test_commands.py::TestRedisCommands::test_set_px_timedelta",
"tests/test_commands.py::TestRedisCommands::test_set_ex",
"tests/test_commands.py::TestRedisCommands::test_set_ex_timedelta",
"tests/test_commands.py::TestRedisCommands::test_set_exat_timedelta",
"tests/test_commands.py::TestRedisCommands::test_set_pxat_timedelta",
"tests/test_commands.py::TestRedisCommands::test_set_multipleoptions",
"tests/test_commands.py::TestRedisCommands::test_set_keepttl",
"tests/test_commands.py::TestRedisCommands::test_set_get",
"tests/test_commands.py::TestRedisCommands::test_setex",
"tests/test_commands.py::TestRedisCommands::test_setnx",
"tests/test_commands.py::TestRedisCommands::test_setrange",
"tests/test_commands.py::TestRedisCommands::test_strlen",
"tests/test_commands.py::TestRedisCommands::test_substr",
"tests/test_commands.py::TestRedisCommands::test_ttl",
"tests/test_commands.py::TestRedisCommands::test_ttl_nokey",
"tests/test_commands.py::TestRedisCommands::test_type",
"tests/test_commands.py::TestRedisCommands::test_blpop",
"tests/test_commands.py::TestRedisCommands::test_brpop",
"tests/test_commands.py::TestRedisCommands::test_brpoplpush",
"tests/test_commands.py::TestRedisCommands::test_brpoplpush_empty_string",
"tests/test_commands.py::TestRedisCommands::test_lindex",
"tests/test_commands.py::TestRedisCommands::test_linsert",
"tests/test_commands.py::TestRedisCommands::test_llen",
"tests/test_commands.py::TestRedisCommands::test_lpop",
"tests/test_commands.py::TestRedisCommands::test_lpop_count",
"tests/test_commands.py::TestRedisCommands::test_lpush",
"tests/test_commands.py::TestRedisCommands::test_lpushx",
"tests/test_commands.py::TestRedisCommands::test_lpushx_with_list",
"tests/test_commands.py::TestRedisCommands::test_lrange",
"tests/test_commands.py::TestRedisCommands::test_lrem",
"tests/test_commands.py::TestRedisCommands::test_lset",
"tests/test_commands.py::TestRedisCommands::test_ltrim",
"tests/test_commands.py::TestRedisCommands::test_rpop",
"tests/test_commands.py::TestRedisCommands::test_rpop_count",
"tests/test_commands.py::TestRedisCommands::test_rpoplpush",
"tests/test_commands.py::TestRedisCommands::test_rpush",
"tests/test_commands.py::TestRedisCommands::test_lpos",
"tests/test_commands.py::TestRedisCommands::test_rpushx",
"tests/test_commands.py::TestRedisCommands::test_scan",
"tests/test_commands.py::TestRedisCommands::test_scan_type",
"tests/test_commands.py::TestRedisCommands::test_scan_iter",
"tests/test_commands.py::TestRedisCommands::test_sscan",
"tests/test_commands.py::TestRedisCommands::test_sscan_iter",
"tests/test_commands.py::TestRedisCommands::test_hscan",
"tests/test_commands.py::TestRedisCommands::test_hscan_iter",
"tests/test_commands.py::TestRedisCommands::test_zscan",
"tests/test_commands.py::TestRedisCommands::test_zscan_iter",
"tests/test_commands.py::TestRedisCommands::test_sadd",
"tests/test_commands.py::TestRedisCommands::test_scard",
"tests/test_commands.py::TestRedisCommands::test_sdiff",
"tests/test_commands.py::TestRedisCommands::test_sdiffstore",
"tests/test_commands.py::TestRedisCommands::test_sinter",
"tests/test_commands.py::TestRedisCommands::test_sintercard",
"tests/test_commands.py::TestRedisCommands::test_sinterstore",
"tests/test_commands.py::TestRedisCommands::test_sismember",
"tests/test_commands.py::TestRedisCommands::test_smembers",
"tests/test_commands.py::TestRedisCommands::test_smismember",
"tests/test_commands.py::TestRedisCommands::test_smove",
"tests/test_commands.py::TestRedisCommands::test_spop",
"tests/test_commands.py::TestRedisCommands::test_spop_multi_value",
"tests/test_commands.py::TestRedisCommands::test_srandmember",
"tests/test_commands.py::TestRedisCommands::test_srandmember_multi_value",
"tests/test_commands.py::TestRedisCommands::test_srem",
"tests/test_commands.py::TestRedisCommands::test_sunion",
"tests/test_commands.py::TestRedisCommands::test_sunionstore",
"tests/test_commands.py::TestRedisCommands::test_debug_segfault",
"tests/test_commands.py::TestRedisCommands::test_script_debug",
"tests/test_commands.py::TestRedisCommands::test_zadd",
"tests/test_commands.py::TestRedisCommands::test_zadd_nx",
"tests/test_commands.py::TestRedisCommands::test_zadd_xx",
"tests/test_commands.py::TestRedisCommands::test_zadd_ch",
"tests/test_commands.py::TestRedisCommands::test_zadd_incr",
"tests/test_commands.py::TestRedisCommands::test_zadd_incr_with_xx",
"tests/test_commands.py::TestRedisCommands::test_zadd_gt_lt",
"tests/test_commands.py::TestRedisCommands::test_zcard",
"tests/test_commands.py::TestRedisCommands::test_zcount",
"tests/test_commands.py::TestRedisCommands::test_zdiff",
"tests/test_commands.py::TestRedisCommands::test_zdiffstore",
"tests/test_commands.py::TestRedisCommands::test_zincrby",
"tests/test_commands.py::TestRedisCommands::test_zlexcount",
"tests/test_commands.py::TestRedisCommands::test_zinter",
"tests/test_commands.py::TestRedisCommands::test_zintercard",
"tests/test_commands.py::TestRedisCommands::test_zinterstore_sum",
"tests/test_commands.py::TestRedisCommands::test_zinterstore_max",
"tests/test_commands.py::TestRedisCommands::test_zinterstore_min",
"tests/test_commands.py::TestRedisCommands::test_zinterstore_with_weight",
"tests/test_commands.py::TestRedisCommands::test_zpopmax",
"tests/test_commands.py::TestRedisCommands::test_zpopmin",
"tests/test_commands.py::TestRedisCommands::test_zrandemember",
"tests/test_commands.py::TestRedisCommands::test_bzpopmax",
"tests/test_commands.py::TestRedisCommands::test_bzpopmin",
"tests/test_commands.py::TestRedisCommands::test_zrange",
"tests/test_commands.py::TestRedisCommands::test_zrange_errors",
"tests/test_commands.py::TestRedisCommands::test_zrange_params",
"tests/test_commands.py::TestRedisCommands::test_zrangestore",
"tests/test_commands.py::TestRedisCommands::test_zrangebylex",
"tests/test_commands.py::TestRedisCommands::test_zrevrangebylex",
"tests/test_commands.py::TestRedisCommands::test_zrangebyscore",
"tests/test_commands.py::TestRedisCommands::test_zrank",
"tests/test_commands.py::TestRedisCommands::test_zrem",
"tests/test_commands.py::TestRedisCommands::test_zrem_multiple_keys",
"tests/test_commands.py::TestRedisCommands::test_zremrangebylex",
"tests/test_commands.py::TestRedisCommands::test_zremrangebyrank",
"tests/test_commands.py::TestRedisCommands::test_zremrangebyscore",
"tests/test_commands.py::TestRedisCommands::test_zrevrange",
"tests/test_commands.py::TestRedisCommands::test_zrevrangebyscore",
"tests/test_commands.py::TestRedisCommands::test_zrevrank",
"tests/test_commands.py::TestRedisCommands::test_zscore",
"tests/test_commands.py::TestRedisCommands::test_zunion",
"tests/test_commands.py::TestRedisCommands::test_zunionstore_sum",
"tests/test_commands.py::TestRedisCommands::test_zunionstore_max",
"tests/test_commands.py::TestRedisCommands::test_zunionstore_min",
"tests/test_commands.py::TestRedisCommands::test_zunionstore_with_weight",
"tests/test_commands.py::TestRedisCommands::test_zmscore",
"tests/test_commands.py::TestRedisCommands::test_pfadd",
"tests/test_commands.py::TestRedisCommands::test_pfcount",
"tests/test_commands.py::TestRedisCommands::test_pfmerge",
"tests/test_commands.py::TestRedisCommands::test_hget_and_hset",
"tests/test_commands.py::TestRedisCommands::test_hset_with_multi_key_values",
"tests/test_commands.py::TestRedisCommands::test_hset_with_key_values_passed_as_list",
"tests/test_commands.py::TestRedisCommands::test_hset_without_data",
"tests/test_commands.py::TestRedisCommands::test_hdel",
"tests/test_commands.py::TestRedisCommands::test_hexists",
"tests/test_commands.py::TestRedisCommands::test_hgetall",
"tests/test_commands.py::TestRedisCommands::test_hincrby",
"tests/test_commands.py::TestRedisCommands::test_hincrbyfloat",
"tests/test_commands.py::TestRedisCommands::test_hkeys",
"tests/test_commands.py::TestRedisCommands::test_hlen",
"tests/test_commands.py::TestRedisCommands::test_hmget",
"tests/test_commands.py::TestRedisCommands::test_hmset",
"tests/test_commands.py::TestRedisCommands::test_hsetnx",
"tests/test_commands.py::TestRedisCommands::test_hvals",
"tests/test_commands.py::TestRedisCommands::test_hstrlen",
"tests/test_commands.py::TestRedisCommands::test_sort_basic",
"tests/test_commands.py::TestRedisCommands::test_sort_limited",
"tests/test_commands.py::TestRedisCommands::test_sort_by",
"tests/test_commands.py::TestRedisCommands::test_sort_get",
"tests/test_commands.py::TestRedisCommands::test_sort_get_multi",
"tests/test_commands.py::TestRedisCommands::test_sort_get_groups_two",
"tests/test_commands.py::TestRedisCommands::test_sort_groups_string_get",
"tests/test_commands.py::TestRedisCommands::test_sort_groups_just_one_get",
"tests/test_commands.py::TestRedisCommands::test_sort_groups_no_get",
"tests/test_commands.py::TestRedisCommands::test_sort_groups_three_gets",
"tests/test_commands.py::TestRedisCommands::test_sort_desc",
"tests/test_commands.py::TestRedisCommands::test_sort_alpha",
"tests/test_commands.py::TestRedisCommands::test_sort_store",
"tests/test_commands.py::TestRedisCommands::test_sort_all_options",
"tests/test_commands.py::TestRedisCommands::test_sort_ro",
"tests/test_commands.py::TestRedisCommands::test_sort_issue_924",
"tests/test_commands.py::TestRedisCommands::test_cluster_addslots",
"tests/test_commands.py::TestRedisCommands::test_cluster_count_failure_reports",
"tests/test_commands.py::TestRedisCommands::test_cluster_countkeysinslot",
"tests/test_commands.py::TestRedisCommands::test_cluster_delslots",
"tests/test_commands.py::TestRedisCommands::test_cluster_failover",
"tests/test_commands.py::TestRedisCommands::test_cluster_forget",
"tests/test_commands.py::TestRedisCommands::test_cluster_info",
"tests/test_commands.py::TestRedisCommands::test_cluster_keyslot",
"tests/test_commands.py::TestRedisCommands::test_cluster_meet",
"tests/test_commands.py::TestRedisCommands::test_cluster_nodes",
"tests/test_commands.py::TestRedisCommands::test_cluster_replicate",
"tests/test_commands.py::TestRedisCommands::test_cluster_reset",
"tests/test_commands.py::TestRedisCommands::test_cluster_saveconfig",
"tests/test_commands.py::TestRedisCommands::test_cluster_setslot",
"tests/test_commands.py::TestRedisCommands::test_cluster_slaves",
"tests/test_commands.py::TestRedisCommands::test_readonly_invalid_cluster_state",
"tests/test_commands.py::TestRedisCommands::test_readonly",
"tests/test_commands.py::TestRedisCommands::test_geoadd",
"tests/test_commands.py::TestRedisCommands::test_geoadd_nx",
"tests/test_commands.py::TestRedisCommands::test_geoadd_xx",
"tests/test_commands.py::TestRedisCommands::test_geoadd_ch",
"tests/test_commands.py::TestRedisCommands::test_geoadd_invalid_params",
"tests/test_commands.py::TestRedisCommands::test_geodist",
"tests/test_commands.py::TestRedisCommands::test_geodist_units",
"tests/test_commands.py::TestRedisCommands::test_geodist_missing_one_member",
"tests/test_commands.py::TestRedisCommands::test_geodist_invalid_units",
"tests/test_commands.py::TestRedisCommands::test_geohash",
"tests/test_commands.py::TestRedisCommands::test_geopos",
"tests/test_commands.py::TestRedisCommands::test_geopos_no_value",
"tests/test_commands.py::TestRedisCommands::test_geosearch",
"tests/test_commands.py::TestRedisCommands::test_geosearch_member",
"tests/test_commands.py::TestRedisCommands::test_geosearch_sort",
"tests/test_commands.py::TestRedisCommands::test_geosearch_with",
"tests/test_commands.py::TestRedisCommands::test_geosearch_negative",
"tests/test_commands.py::TestRedisCommands::test_geosearchstore",
"tests/test_commands.py::TestRedisCommands::test_geosearchstore_dist",
"tests/test_commands.py::TestRedisCommands::test_georadius",
"tests/test_commands.py::TestRedisCommands::test_georadius_no_values",
"tests/test_commands.py::TestRedisCommands::test_georadius_units",
"tests/test_commands.py::TestRedisCommands::test_georadius_with",
"tests/test_commands.py::TestRedisCommands::test_georadius_count",
"tests/test_commands.py::TestRedisCommands::test_georadius_sort",
"tests/test_commands.py::TestRedisCommands::test_georadius_store",
"tests/test_commands.py::TestRedisCommands::test_georadius_store_dist",
"tests/test_commands.py::TestRedisCommands::test_georadiusmember",
"tests/test_commands.py::TestRedisCommands::test_georadiusmember_count",
"tests/test_commands.py::TestRedisCommands::test_xack",
"tests/test_commands.py::TestRedisCommands::test_xadd",
"tests/test_commands.py::TestRedisCommands::test_xadd_nomkstream",
"tests/test_commands.py::TestRedisCommands::test_xadd_minlen_and_limit",
"tests/test_commands.py::TestRedisCommands::test_xautoclaim",
"tests/test_commands.py::TestRedisCommands::test_xautoclaim_negative",
"tests/test_commands.py::TestRedisCommands::test_xclaim",
"tests/test_commands.py::TestRedisCommands::test_xdel",
"tests/test_commands.py::TestRedisCommands::test_xgroup_delconsumer",
"tests/test_commands.py::TestRedisCommands::test_xgroup_createconsumer",
"tests/test_commands.py::TestRedisCommands::test_xgroup_destroy",
"tests/test_commands.py::TestRedisCommands::test_xinfo_stream",
"tests/test_commands.py::TestRedisCommands::test_xinfo_stream_full",
"tests/test_commands.py::TestRedisCommands::test_xlen",
"tests/test_commands.py::TestRedisCommands::test_xpending",
"tests/test_commands.py::TestRedisCommands::test_xpending_range",
"tests/test_commands.py::TestRedisCommands::test_xpending_range_idle",
"tests/test_commands.py::TestRedisCommands::test_xpending_range_negative",
"tests/test_commands.py::TestRedisCommands::test_xrange",
"tests/test_commands.py::TestRedisCommands::test_xread",
"tests/test_commands.py::TestRedisCommands::test_xreadgroup",
"tests/test_commands.py::TestRedisCommands::test_xrevrange",
"tests/test_commands.py::TestRedisCommands::test_xtrim",
"tests/test_commands.py::TestRedisCommands::test_xtrim_minlen_and_length_args",
"tests/test_commands.py::TestRedisCommands::test_bitfield_operations",
"tests/test_commands.py::TestRedisCommands::test_memory_help",
"tests/test_commands.py::TestRedisCommands::test_memory_doctor",
"tests/test_commands.py::TestRedisCommands::test_memory_malloc_stats",
"tests/test_commands.py::TestRedisCommands::test_memory_stats",
"tests/test_commands.py::TestRedisCommands::test_memory_usage",
"tests/test_commands.py::TestRedisCommands::test_module_list",
"tests/test_commands.py::TestRedisCommands::test_command_count",
"tests/test_commands.py::TestRedisCommands::test_command_docs",
"tests/test_commands.py::TestRedisCommands::test_command_getkeys",
"tests/test_commands.py::TestRedisCommands::test_command",
"tests/test_commands.py::TestRedisCommands::test_module",
"tests/test_commands.py::TestRedisCommands::test_restore",
"tests/test_commands.py::TestRedisCommands::test_restore_idletime",
"tests/test_commands.py::TestRedisCommands::test_restore_frequency",
"tests/test_commands.py::TestRedisCommands::test_replicaof",
"tests/test_commands.py::TestRedisCommands::test_sync",
"tests/test_commands.py::TestRedisCommands::test_psync",
"tests/test_commands.py::TestBinarySave::test_binary_get_set",
"tests/test_commands.py::TestBinarySave::test_binary_lists",
"tests/test_commands.py::TestBinarySave::test_22_info",
"tests/test_commands.py::TestBinarySave::test_large_responses",
"tests/test_commands.py::TestBinarySave::test_floating_point_encoding"
] | [
"tests/test_commands.py::TestRedisCommands::test_info_multi_sections"
] | Python | [] | [] |
|
redis/redis-py | 2,146 | redis__redis-py-2146 | [
"2125"
] | a696fe5e3155238fd8e9ec65224f94f6f25bb72b | diff --git a/redis/commands/core.py b/redis/commands/core.py
--- a/redis/commands/core.py
+++ b/redis/commands/core.py
@@ -5592,6 +5592,27 @@ def module_load(self, path, *args) -> ResponseT:
"""
return self.execute_command("MODULE LOAD", path, *args)
+ def module_loadex(
+ self,
+ path: str,
+ options: Optional[List[str]] = None,
+ args: Optional[List[str]] = None,
+ ) -> ResponseT:
+ """
+ Loads a module from a dynamic library at runtime with configuration directives.
+
+ For more information see https://redis.io/commands/module-loadex
+ """
+ pieces = []
+ if options is not None:
+ pieces.append("CONFIG")
+ pieces.extend(options)
+ if args is not None:
+ pieces.append("ARGS")
+ pieces.extend(args)
+
+ return self.execute_command("MODULE LOADEX", path, *pieces)
+
def module_unload(self, name) -> ResponseT:
"""
Unloads the module ``name``.
| diff --git a/tests/test_commands.py b/tests/test_commands.py
--- a/tests/test_commands.py
+++ b/tests/test_commands.py
@@ -4513,6 +4513,18 @@ def test_module(self, r):
r.module_load("/some/fake/path", "arg1", "arg2", "arg3", "arg4")
assert "Error loading the extension." in str(excinfo.value)
+ @pytest.mark.onlynoncluster
+ @skip_if_server_version_lt("7.0.0")
+ @skip_if_redis_enterprise()
+ def test_module_loadex(self, r: redis.Redis):
+ with pytest.raises(redis.exceptions.ModuleError) as excinfo:
+ r.module_loadex("/some/fake/path")
+ assert "Error loading the extension." in str(excinfo.value)
+
+ with pytest.raises(redis.exceptions.ModuleError) as excinfo:
+ r.module_loadex("/some/fake/path", ["name", "value"], ["arg1", "arg2"])
+ assert "Error loading the extension." in str(excinfo.value)
+
@skip_if_server_version_lt("2.6.0")
def test_restore(self, r):
| Add support for MODULE LOADEX
| "2022-04-27T10:57:48Z" | 4.2 | [
"tests/test_commands.py::TestResponseCallbacks::test_response_callbacks",
"tests/test_commands.py::TestResponseCallbacks::test_case_insensitive_command_names",
"tests/test_commands.py::TestRedisCommands::test_auth",
"tests/test_commands.py::TestRedisCommands::test_command_on_invalid_key_type",
"tests/test_commands.py::TestRedisCommands::test_acl_cat_no_category",
"tests/test_commands.py::TestRedisCommands::test_acl_cat_with_category",
"tests/test_commands.py::TestRedisCommands::test_acl_genpass",
"tests/test_commands.py::TestRedisCommands::test_acl_help",
"tests/test_commands.py::TestRedisCommands::test_acl_list",
"tests/test_commands.py::TestRedisCommands::test_acl_log",
"tests/test_commands.py::TestRedisCommands::test_acl_setuser_categories_without_prefix_fails",
"tests/test_commands.py::TestRedisCommands::test_acl_setuser_commands_without_prefix_fails",
"tests/test_commands.py::TestRedisCommands::test_acl_setuser_add_passwords_and_nopass_fails",
"tests/test_commands.py::TestRedisCommands::test_acl_users",
"tests/test_commands.py::TestRedisCommands::test_acl_whoami",
"tests/test_commands.py::TestRedisCommands::test_client_list",
"tests/test_commands.py::TestRedisCommands::test_client_info",
"tests/test_commands.py::TestRedisCommands::test_client_list_types_not_replica",
"tests/test_commands.py::TestRedisCommands::test_client_list_replica",
"tests/test_commands.py::TestRedisCommands::test_client_list_client_id",
"tests/test_commands.py::TestRedisCommands::test_client_id",
"tests/test_commands.py::TestRedisCommands::test_client_trackinginfo",
"tests/test_commands.py::TestRedisCommands::test_client_tracking",
"tests/test_commands.py::TestRedisCommands::test_client_unblock",
"tests/test_commands.py::TestRedisCommands::test_client_getname",
"tests/test_commands.py::TestRedisCommands::test_client_setname",
"tests/test_commands.py::TestRedisCommands::test_client_kill",
"tests/test_commands.py::TestRedisCommands::test_client_kill_filter_invalid_params",
"tests/test_commands.py::TestRedisCommands::test_client_kill_filter_by_id",
"tests/test_commands.py::TestRedisCommands::test_client_kill_filter_by_addr",
"tests/test_commands.py::TestRedisCommands::test_client_list_after_client_setname",
"tests/test_commands.py::TestRedisCommands::test_client_kill_filter_by_laddr",
"tests/test_commands.py::TestRedisCommands::test_client_kill_filter_by_user",
"tests/test_commands.py::TestRedisCommands::test_client_pause",
"tests/test_commands.py::TestRedisCommands::test_client_pause_all",
"tests/test_commands.py::TestRedisCommands::test_client_unpause",
"tests/test_commands.py::TestRedisCommands::test_client_reply",
"tests/test_commands.py::TestRedisCommands::test_client_getredir",
"tests/test_commands.py::TestRedisCommands::test_hello_notI_implemented",
"tests/test_commands.py::TestRedisCommands::test_config_get",
"tests/test_commands.py::TestRedisCommands::test_config_get_multi_params",
"tests/test_commands.py::TestRedisCommands::test_config_resetstat",
"tests/test_commands.py::TestRedisCommands::test_config_set",
"tests/test_commands.py::TestRedisCommands::test_config_set_multi_params",
"tests/test_commands.py::TestRedisCommands::test_failover",
"tests/test_commands.py::TestRedisCommands::test_dbsize",
"tests/test_commands.py::TestRedisCommands::test_echo",
"tests/test_commands.py::TestRedisCommands::test_info",
"tests/test_commands.py::TestRedisCommands::test_info_multi_sections",
"tests/test_commands.py::TestRedisCommands::test_lastsave",
"tests/test_commands.py::TestRedisCommands::test_lolwut",
"tests/test_commands.py::TestRedisCommands::test_reset",
"tests/test_commands.py::TestRedisCommands::test_object",
"tests/test_commands.py::TestRedisCommands::test_ping",
"tests/test_commands.py::TestRedisCommands::test_quit",
"tests/test_commands.py::TestRedisCommands::test_role",
"tests/test_commands.py::TestRedisCommands::test_select",
"tests/test_commands.py::TestRedisCommands::test_slowlog_get",
"tests/test_commands.py::TestRedisCommands::test_slowlog_get_limit",
"tests/test_commands.py::TestRedisCommands::test_slowlog_length",
"tests/test_commands.py::TestRedisCommands::test_time",
"tests/test_commands.py::TestRedisCommands::test_bgsave",
"tests/test_commands.py::TestRedisCommands::test_append",
"tests/test_commands.py::TestRedisCommands::test_bitcount",
"tests/test_commands.py::TestRedisCommands::test_bitcount_mode",
"tests/test_commands.py::TestRedisCommands::test_bitop_not_empty_string",
"tests/test_commands.py::TestRedisCommands::test_bitop_not",
"tests/test_commands.py::TestRedisCommands::test_bitop_not_in_place",
"tests/test_commands.py::TestRedisCommands::test_bitop_single_string",
"tests/test_commands.py::TestRedisCommands::test_bitop_string_operands",
"tests/test_commands.py::TestRedisCommands::test_bitpos",
"tests/test_commands.py::TestRedisCommands::test_bitpos_wrong_arguments",
"tests/test_commands.py::TestRedisCommands::test_bitpos_mode",
"tests/test_commands.py::TestRedisCommands::test_copy",
"tests/test_commands.py::TestRedisCommands::test_copy_and_replace",
"tests/test_commands.py::TestRedisCommands::test_copy_to_another_database",
"tests/test_commands.py::TestRedisCommands::test_decr",
"tests/test_commands.py::TestRedisCommands::test_decrby",
"tests/test_commands.py::TestRedisCommands::test_delete",
"tests/test_commands.py::TestRedisCommands::test_delete_with_multiple_keys",
"tests/test_commands.py::TestRedisCommands::test_delitem",
"tests/test_commands.py::TestRedisCommands::test_unlink",
"tests/test_commands.py::TestRedisCommands::test_unlink_with_multiple_keys",
"tests/test_commands.py::TestRedisCommands::test_dump_and_restore",
"tests/test_commands.py::TestRedisCommands::test_dump_and_restore_and_replace",
"tests/test_commands.py::TestRedisCommands::test_dump_and_restore_absttl",
"tests/test_commands.py::TestRedisCommands::test_exists",
"tests/test_commands.py::TestRedisCommands::test_exists_contains",
"tests/test_commands.py::TestRedisCommands::test_expire",
"tests/test_commands.py::TestRedisCommands::test_expire_option_nx",
"tests/test_commands.py::TestRedisCommands::test_expire_option_gt",
"tests/test_commands.py::TestRedisCommands::test_expire_option_lt",
"tests/test_commands.py::TestRedisCommands::test_expireat_datetime",
"tests/test_commands.py::TestRedisCommands::test_expireat_no_key",
"tests/test_commands.py::TestRedisCommands::test_expireat_unixtime",
"tests/test_commands.py::TestRedisCommands::test_expiretime",
"tests/test_commands.py::TestRedisCommands::test_expireat_option_nx",
"tests/test_commands.py::TestRedisCommands::test_expireat_option_xx",
"tests/test_commands.py::TestRedisCommands::test_expireat_option_gt",
"tests/test_commands.py::TestRedisCommands::test_expireat_option_lt",
"tests/test_commands.py::TestRedisCommands::test_get_and_set",
"tests/test_commands.py::TestRedisCommands::test_getdel",
"tests/test_commands.py::TestRedisCommands::test_getex",
"tests/test_commands.py::TestRedisCommands::test_getitem_and_setitem",
"tests/test_commands.py::TestRedisCommands::test_getitem_raises_keyerror_for_missing_key",
"tests/test_commands.py::TestRedisCommands::test_getitem_does_not_raise_keyerror_for_empty_string",
"tests/test_commands.py::TestRedisCommands::test_get_set_bit",
"tests/test_commands.py::TestRedisCommands::test_getrange",
"tests/test_commands.py::TestRedisCommands::test_getset",
"tests/test_commands.py::TestRedisCommands::test_incr",
"tests/test_commands.py::TestRedisCommands::test_incrby",
"tests/test_commands.py::TestRedisCommands::test_incrbyfloat",
"tests/test_commands.py::TestRedisCommands::test_keys",
"tests/test_commands.py::TestRedisCommands::test_mget",
"tests/test_commands.py::TestRedisCommands::test_lmove",
"tests/test_commands.py::TestRedisCommands::test_blmove",
"tests/test_commands.py::TestRedisCommands::test_mset",
"tests/test_commands.py::TestRedisCommands::test_msetnx",
"tests/test_commands.py::TestRedisCommands::test_pexpire",
"tests/test_commands.py::TestRedisCommands::test_pexpire_option_nx",
"tests/test_commands.py::TestRedisCommands::test_pexpire_option_xx",
"tests/test_commands.py::TestRedisCommands::test_pexpire_option_gt",
"tests/test_commands.py::TestRedisCommands::test_pexpire_option_lt",
"tests/test_commands.py::TestRedisCommands::test_pexpireat_datetime",
"tests/test_commands.py::TestRedisCommands::test_pexpireat_no_key",
"tests/test_commands.py::TestRedisCommands::test_pexpireat_unixtime",
"tests/test_commands.py::TestRedisCommands::test_pexpireat_option_nx",
"tests/test_commands.py::TestRedisCommands::test_pexpireat_option_xx",
"tests/test_commands.py::TestRedisCommands::test_pexpireat_option_gt",
"tests/test_commands.py::TestRedisCommands::test_pexpireat_option_lt",
"tests/test_commands.py::TestRedisCommands::test_pexpiretime",
"tests/test_commands.py::TestRedisCommands::test_psetex",
"tests/test_commands.py::TestRedisCommands::test_psetex_timedelta",
"tests/test_commands.py::TestRedisCommands::test_pttl",
"tests/test_commands.py::TestRedisCommands::test_pttl_no_key",
"tests/test_commands.py::TestRedisCommands::test_hrandfield",
"tests/test_commands.py::TestRedisCommands::test_randomkey",
"tests/test_commands.py::TestRedisCommands::test_rename",
"tests/test_commands.py::TestRedisCommands::test_renamenx",
"tests/test_commands.py::TestRedisCommands::test_set_nx",
"tests/test_commands.py::TestRedisCommands::test_set_xx",
"tests/test_commands.py::TestRedisCommands::test_set_px",
"tests/test_commands.py::TestRedisCommands::test_set_px_timedelta",
"tests/test_commands.py::TestRedisCommands::test_set_ex",
"tests/test_commands.py::TestRedisCommands::test_set_ex_timedelta",
"tests/test_commands.py::TestRedisCommands::test_set_exat_timedelta",
"tests/test_commands.py::TestRedisCommands::test_set_pxat_timedelta",
"tests/test_commands.py::TestRedisCommands::test_set_multipleoptions",
"tests/test_commands.py::TestRedisCommands::test_set_keepttl",
"tests/test_commands.py::TestRedisCommands::test_set_get",
"tests/test_commands.py::TestRedisCommands::test_setex",
"tests/test_commands.py::TestRedisCommands::test_setnx",
"tests/test_commands.py::TestRedisCommands::test_setrange",
"tests/test_commands.py::TestRedisCommands::test_strlen",
"tests/test_commands.py::TestRedisCommands::test_substr",
"tests/test_commands.py::TestRedisCommands::test_ttl",
"tests/test_commands.py::TestRedisCommands::test_ttl_nokey",
"tests/test_commands.py::TestRedisCommands::test_type",
"tests/test_commands.py::TestRedisCommands::test_blpop",
"tests/test_commands.py::TestRedisCommands::test_brpop",
"tests/test_commands.py::TestRedisCommands::test_brpoplpush",
"tests/test_commands.py::TestRedisCommands::test_brpoplpush_empty_string",
"tests/test_commands.py::TestRedisCommands::test_lindex",
"tests/test_commands.py::TestRedisCommands::test_linsert",
"tests/test_commands.py::TestRedisCommands::test_llen",
"tests/test_commands.py::TestRedisCommands::test_lpop",
"tests/test_commands.py::TestRedisCommands::test_lpop_count",
"tests/test_commands.py::TestRedisCommands::test_lpush",
"tests/test_commands.py::TestRedisCommands::test_lpushx",
"tests/test_commands.py::TestRedisCommands::test_lpushx_with_list",
"tests/test_commands.py::TestRedisCommands::test_lrange",
"tests/test_commands.py::TestRedisCommands::test_lrem",
"tests/test_commands.py::TestRedisCommands::test_lset",
"tests/test_commands.py::TestRedisCommands::test_ltrim",
"tests/test_commands.py::TestRedisCommands::test_rpop",
"tests/test_commands.py::TestRedisCommands::test_rpop_count",
"tests/test_commands.py::TestRedisCommands::test_rpoplpush",
"tests/test_commands.py::TestRedisCommands::test_rpush",
"tests/test_commands.py::TestRedisCommands::test_lpos",
"tests/test_commands.py::TestRedisCommands::test_rpushx",
"tests/test_commands.py::TestRedisCommands::test_scan",
"tests/test_commands.py::TestRedisCommands::test_scan_type",
"tests/test_commands.py::TestRedisCommands::test_scan_iter",
"tests/test_commands.py::TestRedisCommands::test_sscan",
"tests/test_commands.py::TestRedisCommands::test_sscan_iter",
"tests/test_commands.py::TestRedisCommands::test_hscan",
"tests/test_commands.py::TestRedisCommands::test_hscan_iter",
"tests/test_commands.py::TestRedisCommands::test_zscan",
"tests/test_commands.py::TestRedisCommands::test_zscan_iter",
"tests/test_commands.py::TestRedisCommands::test_sadd",
"tests/test_commands.py::TestRedisCommands::test_scard",
"tests/test_commands.py::TestRedisCommands::test_sdiff",
"tests/test_commands.py::TestRedisCommands::test_sdiffstore",
"tests/test_commands.py::TestRedisCommands::test_sinter",
"tests/test_commands.py::TestRedisCommands::test_sintercard",
"tests/test_commands.py::TestRedisCommands::test_sinterstore",
"tests/test_commands.py::TestRedisCommands::test_sismember",
"tests/test_commands.py::TestRedisCommands::test_smembers",
"tests/test_commands.py::TestRedisCommands::test_smismember",
"tests/test_commands.py::TestRedisCommands::test_smove",
"tests/test_commands.py::TestRedisCommands::test_spop",
"tests/test_commands.py::TestRedisCommands::test_spop_multi_value",
"tests/test_commands.py::TestRedisCommands::test_srandmember",
"tests/test_commands.py::TestRedisCommands::test_srandmember_multi_value",
"tests/test_commands.py::TestRedisCommands::test_srem",
"tests/test_commands.py::TestRedisCommands::test_sunion",
"tests/test_commands.py::TestRedisCommands::test_sunionstore",
"tests/test_commands.py::TestRedisCommands::test_debug_segfault",
"tests/test_commands.py::TestRedisCommands::test_script_debug",
"tests/test_commands.py::TestRedisCommands::test_zadd",
"tests/test_commands.py::TestRedisCommands::test_zadd_nx",
"tests/test_commands.py::TestRedisCommands::test_zadd_xx",
"tests/test_commands.py::TestRedisCommands::test_zadd_ch",
"tests/test_commands.py::TestRedisCommands::test_zadd_incr",
"tests/test_commands.py::TestRedisCommands::test_zadd_incr_with_xx",
"tests/test_commands.py::TestRedisCommands::test_zadd_gt_lt",
"tests/test_commands.py::TestRedisCommands::test_zcard",
"tests/test_commands.py::TestRedisCommands::test_zcount",
"tests/test_commands.py::TestRedisCommands::test_zdiff",
"tests/test_commands.py::TestRedisCommands::test_zdiffstore",
"tests/test_commands.py::TestRedisCommands::test_zincrby",
"tests/test_commands.py::TestRedisCommands::test_zlexcount",
"tests/test_commands.py::TestRedisCommands::test_zinter",
"tests/test_commands.py::TestRedisCommands::test_zintercard",
"tests/test_commands.py::TestRedisCommands::test_zinterstore_sum",
"tests/test_commands.py::TestRedisCommands::test_zinterstore_max",
"tests/test_commands.py::TestRedisCommands::test_zinterstore_min",
"tests/test_commands.py::TestRedisCommands::test_zinterstore_with_weight",
"tests/test_commands.py::TestRedisCommands::test_zpopmax",
"tests/test_commands.py::TestRedisCommands::test_zpopmin",
"tests/test_commands.py::TestRedisCommands::test_zrandemember",
"tests/test_commands.py::TestRedisCommands::test_bzpopmax",
"tests/test_commands.py::TestRedisCommands::test_bzpopmin",
"tests/test_commands.py::TestRedisCommands::test_zrange",
"tests/test_commands.py::TestRedisCommands::test_zrange_errors",
"tests/test_commands.py::TestRedisCommands::test_zrange_params",
"tests/test_commands.py::TestRedisCommands::test_zrangestore",
"tests/test_commands.py::TestRedisCommands::test_zrangebylex",
"tests/test_commands.py::TestRedisCommands::test_zrevrangebylex",
"tests/test_commands.py::TestRedisCommands::test_zrangebyscore",
"tests/test_commands.py::TestRedisCommands::test_zrank",
"tests/test_commands.py::TestRedisCommands::test_zrem",
"tests/test_commands.py::TestRedisCommands::test_zrem_multiple_keys",
"tests/test_commands.py::TestRedisCommands::test_zremrangebylex",
"tests/test_commands.py::TestRedisCommands::test_zremrangebyrank",
"tests/test_commands.py::TestRedisCommands::test_zremrangebyscore",
"tests/test_commands.py::TestRedisCommands::test_zrevrange",
"tests/test_commands.py::TestRedisCommands::test_zrevrangebyscore",
"tests/test_commands.py::TestRedisCommands::test_zrevrank",
"tests/test_commands.py::TestRedisCommands::test_zscore",
"tests/test_commands.py::TestRedisCommands::test_zunion",
"tests/test_commands.py::TestRedisCommands::test_zunionstore_sum",
"tests/test_commands.py::TestRedisCommands::test_zunionstore_max",
"tests/test_commands.py::TestRedisCommands::test_zunionstore_min",
"tests/test_commands.py::TestRedisCommands::test_zunionstore_with_weight",
"tests/test_commands.py::TestRedisCommands::test_zmscore",
"tests/test_commands.py::TestRedisCommands::test_pfadd",
"tests/test_commands.py::TestRedisCommands::test_pfcount",
"tests/test_commands.py::TestRedisCommands::test_pfmerge",
"tests/test_commands.py::TestRedisCommands::test_hget_and_hset",
"tests/test_commands.py::TestRedisCommands::test_hset_with_multi_key_values",
"tests/test_commands.py::TestRedisCommands::test_hset_with_key_values_passed_as_list",
"tests/test_commands.py::TestRedisCommands::test_hset_without_data",
"tests/test_commands.py::TestRedisCommands::test_hdel",
"tests/test_commands.py::TestRedisCommands::test_hexists",
"tests/test_commands.py::TestRedisCommands::test_hgetall",
"tests/test_commands.py::TestRedisCommands::test_hincrby",
"tests/test_commands.py::TestRedisCommands::test_hincrbyfloat",
"tests/test_commands.py::TestRedisCommands::test_hkeys",
"tests/test_commands.py::TestRedisCommands::test_hlen",
"tests/test_commands.py::TestRedisCommands::test_hmget",
"tests/test_commands.py::TestRedisCommands::test_hmset",
"tests/test_commands.py::TestRedisCommands::test_hsetnx",
"tests/test_commands.py::TestRedisCommands::test_hvals",
"tests/test_commands.py::TestRedisCommands::test_hstrlen",
"tests/test_commands.py::TestRedisCommands::test_sort_basic",
"tests/test_commands.py::TestRedisCommands::test_sort_limited",
"tests/test_commands.py::TestRedisCommands::test_sort_by",
"tests/test_commands.py::TestRedisCommands::test_sort_get",
"tests/test_commands.py::TestRedisCommands::test_sort_get_multi",
"tests/test_commands.py::TestRedisCommands::test_sort_get_groups_two",
"tests/test_commands.py::TestRedisCommands::test_sort_groups_string_get",
"tests/test_commands.py::TestRedisCommands::test_sort_groups_just_one_get",
"tests/test_commands.py::TestRedisCommands::test_sort_groups_no_get",
"tests/test_commands.py::TestRedisCommands::test_sort_groups_three_gets",
"tests/test_commands.py::TestRedisCommands::test_sort_desc",
"tests/test_commands.py::TestRedisCommands::test_sort_alpha",
"tests/test_commands.py::TestRedisCommands::test_sort_store",
"tests/test_commands.py::TestRedisCommands::test_sort_all_options",
"tests/test_commands.py::TestRedisCommands::test_sort_ro",
"tests/test_commands.py::TestRedisCommands::test_sort_issue_924",
"tests/test_commands.py::TestRedisCommands::test_cluster_addslots",
"tests/test_commands.py::TestRedisCommands::test_cluster_count_failure_reports",
"tests/test_commands.py::TestRedisCommands::test_cluster_countkeysinslot",
"tests/test_commands.py::TestRedisCommands::test_cluster_delslots",
"tests/test_commands.py::TestRedisCommands::test_cluster_failover",
"tests/test_commands.py::TestRedisCommands::test_cluster_forget",
"tests/test_commands.py::TestRedisCommands::test_cluster_info",
"tests/test_commands.py::TestRedisCommands::test_cluster_keyslot",
"tests/test_commands.py::TestRedisCommands::test_cluster_meet",
"tests/test_commands.py::TestRedisCommands::test_cluster_nodes",
"tests/test_commands.py::TestRedisCommands::test_cluster_replicate",
"tests/test_commands.py::TestRedisCommands::test_cluster_reset",
"tests/test_commands.py::TestRedisCommands::test_cluster_saveconfig",
"tests/test_commands.py::TestRedisCommands::test_cluster_setslot",
"tests/test_commands.py::TestRedisCommands::test_cluster_slaves",
"tests/test_commands.py::TestRedisCommands::test_readonly_invalid_cluster_state",
"tests/test_commands.py::TestRedisCommands::test_readonly",
"tests/test_commands.py::TestRedisCommands::test_geoadd",
"tests/test_commands.py::TestRedisCommands::test_geoadd_nx",
"tests/test_commands.py::TestRedisCommands::test_geoadd_xx",
"tests/test_commands.py::TestRedisCommands::test_geoadd_ch",
"tests/test_commands.py::TestRedisCommands::test_geoadd_invalid_params",
"tests/test_commands.py::TestRedisCommands::test_geodist",
"tests/test_commands.py::TestRedisCommands::test_geodist_units",
"tests/test_commands.py::TestRedisCommands::test_geodist_missing_one_member",
"tests/test_commands.py::TestRedisCommands::test_geodist_invalid_units",
"tests/test_commands.py::TestRedisCommands::test_geohash",
"tests/test_commands.py::TestRedisCommands::test_geopos",
"tests/test_commands.py::TestRedisCommands::test_geopos_no_value",
"tests/test_commands.py::TestRedisCommands::test_geosearch",
"tests/test_commands.py::TestRedisCommands::test_geosearch_member",
"tests/test_commands.py::TestRedisCommands::test_geosearch_sort",
"tests/test_commands.py::TestRedisCommands::test_geosearch_with",
"tests/test_commands.py::TestRedisCommands::test_geosearch_negative",
"tests/test_commands.py::TestRedisCommands::test_geosearchstore",
"tests/test_commands.py::TestRedisCommands::test_geosearchstore_dist",
"tests/test_commands.py::TestRedisCommands::test_georadius",
"tests/test_commands.py::TestRedisCommands::test_georadius_no_values",
"tests/test_commands.py::TestRedisCommands::test_georadius_units",
"tests/test_commands.py::TestRedisCommands::test_georadius_with",
"tests/test_commands.py::TestRedisCommands::test_georadius_count",
"tests/test_commands.py::TestRedisCommands::test_georadius_sort",
"tests/test_commands.py::TestRedisCommands::test_georadius_store",
"tests/test_commands.py::TestRedisCommands::test_georadius_store_dist",
"tests/test_commands.py::TestRedisCommands::test_georadiusmember",
"tests/test_commands.py::TestRedisCommands::test_georadiusmember_count",
"tests/test_commands.py::TestRedisCommands::test_xack",
"tests/test_commands.py::TestRedisCommands::test_xadd",
"tests/test_commands.py::TestRedisCommands::test_xadd_nomkstream",
"tests/test_commands.py::TestRedisCommands::test_xadd_minlen_and_limit",
"tests/test_commands.py::TestRedisCommands::test_xautoclaim",
"tests/test_commands.py::TestRedisCommands::test_xautoclaim_negative",
"tests/test_commands.py::TestRedisCommands::test_xclaim",
"tests/test_commands.py::TestRedisCommands::test_xdel",
"tests/test_commands.py::TestRedisCommands::test_xgroup_delconsumer",
"tests/test_commands.py::TestRedisCommands::test_xgroup_createconsumer",
"tests/test_commands.py::TestRedisCommands::test_xgroup_destroy",
"tests/test_commands.py::TestRedisCommands::test_xinfo_stream",
"tests/test_commands.py::TestRedisCommands::test_xinfo_stream_full",
"tests/test_commands.py::TestRedisCommands::test_xlen",
"tests/test_commands.py::TestRedisCommands::test_xpending",
"tests/test_commands.py::TestRedisCommands::test_xpending_range",
"tests/test_commands.py::TestRedisCommands::test_xpending_range_idle",
"tests/test_commands.py::TestRedisCommands::test_xpending_range_negative",
"tests/test_commands.py::TestRedisCommands::test_xrange",
"tests/test_commands.py::TestRedisCommands::test_xread",
"tests/test_commands.py::TestRedisCommands::test_xreadgroup",
"tests/test_commands.py::TestRedisCommands::test_xrevrange",
"tests/test_commands.py::TestRedisCommands::test_xtrim",
"tests/test_commands.py::TestRedisCommands::test_xtrim_minlen_and_length_args",
"tests/test_commands.py::TestRedisCommands::test_bitfield_operations",
"tests/test_commands.py::TestRedisCommands::test_memory_help",
"tests/test_commands.py::TestRedisCommands::test_memory_doctor",
"tests/test_commands.py::TestRedisCommands::test_memory_malloc_stats",
"tests/test_commands.py::TestRedisCommands::test_memory_stats",
"tests/test_commands.py::TestRedisCommands::test_memory_usage",
"tests/test_commands.py::TestRedisCommands::test_module_list",
"tests/test_commands.py::TestRedisCommands::test_command_count",
"tests/test_commands.py::TestRedisCommands::test_command_docs",
"tests/test_commands.py::TestRedisCommands::test_command_getkeys",
"tests/test_commands.py::TestRedisCommands::test_command",
"tests/test_commands.py::TestRedisCommands::test_module",
"tests/test_commands.py::TestRedisCommands::test_restore",
"tests/test_commands.py::TestRedisCommands::test_restore_idletime",
"tests/test_commands.py::TestRedisCommands::test_restore_frequency",
"tests/test_commands.py::TestRedisCommands::test_replicaof",
"tests/test_commands.py::TestRedisCommands::test_sync",
"tests/test_commands.py::TestRedisCommands::test_psync",
"tests/test_commands.py::TestBinarySave::test_binary_get_set",
"tests/test_commands.py::TestBinarySave::test_binary_lists",
"tests/test_commands.py::TestBinarySave::test_22_info",
"tests/test_commands.py::TestBinarySave::test_large_responses",
"tests/test_commands.py::TestBinarySave::test_floating_point_encoding"
] | [
"tests/test_commands.py::TestRedisCommands::test_module_loadex"
] | Python | [] | [] |
|
redis/redis-py | 2,147 | redis__redis-py-2147 | [
"2124"
] | 6ba46418236718d95f9dee09adaa8a42a4d5c23b | diff --git a/redis/commands/core.py b/redis/commands/core.py
--- a/redis/commands/core.py
+++ b/redis/commands/core.py
@@ -1044,6 +1044,15 @@ def memory_purge(self, **kwargs) -> ResponseT:
"""
return self.execute_command("MEMORY PURGE", **kwargs)
+ def latency_histogram(self, *args):
+ """
+ This function throws a NotImplementedError since it is intentionally
+ not supported.
+ """
+ raise NotImplementedError(
+ "LATENCY HISTOGRAM is intentionally not implemented in the client."
+ )
+
def ping(self, **kwargs) -> ResponseT:
"""
Ping the Redis server
| diff --git a/tests/test_commands.py b/tests/test_commands.py
--- a/tests/test_commands.py
+++ b/tests/test_commands.py
@@ -4420,6 +4420,11 @@ def test_memory_usage(self, r):
r.set("foo", "bar")
assert isinstance(r.memory_usage("foo"), int)
+ @skip_if_server_version_lt("7.0.0")
+ def test_latency_histogram_not_implemented(self, r: redis.Redis):
+ with pytest.raises(NotImplementedError):
+ r.latency_histogram()
+
@pytest.mark.onlynoncluster
@skip_if_server_version_lt("4.0.0")
@skip_if_redis_enterprise()
| Add support for LATENCY HISTOGRAM
| "2022-04-27T11:25:33Z" | 4.2 | [
"tests/test_commands.py::TestResponseCallbacks::test_response_callbacks",
"tests/test_commands.py::TestResponseCallbacks::test_case_insensitive_command_names",
"tests/test_commands.py::TestRedisCommands::test_auth",
"tests/test_commands.py::TestRedisCommands::test_command_on_invalid_key_type",
"tests/test_commands.py::TestRedisCommands::test_acl_cat_no_category",
"tests/test_commands.py::TestRedisCommands::test_acl_cat_with_category",
"tests/test_commands.py::TestRedisCommands::test_acl_genpass",
"tests/test_commands.py::TestRedisCommands::test_acl_help",
"tests/test_commands.py::TestRedisCommands::test_acl_list",
"tests/test_commands.py::TestRedisCommands::test_acl_log",
"tests/test_commands.py::TestRedisCommands::test_acl_setuser_categories_without_prefix_fails",
"tests/test_commands.py::TestRedisCommands::test_acl_setuser_commands_without_prefix_fails",
"tests/test_commands.py::TestRedisCommands::test_acl_setuser_add_passwords_and_nopass_fails",
"tests/test_commands.py::TestRedisCommands::test_acl_users",
"tests/test_commands.py::TestRedisCommands::test_acl_whoami",
"tests/test_commands.py::TestRedisCommands::test_client_list",
"tests/test_commands.py::TestRedisCommands::test_client_info",
"tests/test_commands.py::TestRedisCommands::test_client_list_types_not_replica",
"tests/test_commands.py::TestRedisCommands::test_client_list_replica",
"tests/test_commands.py::TestRedisCommands::test_client_list_client_id",
"tests/test_commands.py::TestRedisCommands::test_client_id",
"tests/test_commands.py::TestRedisCommands::test_client_trackinginfo",
"tests/test_commands.py::TestRedisCommands::test_client_tracking",
"tests/test_commands.py::TestRedisCommands::test_client_unblock",
"tests/test_commands.py::TestRedisCommands::test_client_getname",
"tests/test_commands.py::TestRedisCommands::test_client_setname",
"tests/test_commands.py::TestRedisCommands::test_client_kill",
"tests/test_commands.py::TestRedisCommands::test_client_kill_filter_invalid_params",
"tests/test_commands.py::TestRedisCommands::test_client_kill_filter_by_id",
"tests/test_commands.py::TestRedisCommands::test_client_kill_filter_by_addr",
"tests/test_commands.py::TestRedisCommands::test_client_list_after_client_setname",
"tests/test_commands.py::TestRedisCommands::test_client_kill_filter_by_laddr",
"tests/test_commands.py::TestRedisCommands::test_client_kill_filter_by_user",
"tests/test_commands.py::TestRedisCommands::test_client_pause",
"tests/test_commands.py::TestRedisCommands::test_client_pause_all",
"tests/test_commands.py::TestRedisCommands::test_client_unpause",
"tests/test_commands.py::TestRedisCommands::test_client_reply",
"tests/test_commands.py::TestRedisCommands::test_client_getredir",
"tests/test_commands.py::TestRedisCommands::test_hello_notI_implemented",
"tests/test_commands.py::TestRedisCommands::test_config_get",
"tests/test_commands.py::TestRedisCommands::test_config_resetstat",
"tests/test_commands.py::TestRedisCommands::test_config_set",
"tests/test_commands.py::TestRedisCommands::test_failover",
"tests/test_commands.py::TestRedisCommands::test_dbsize",
"tests/test_commands.py::TestRedisCommands::test_echo",
"tests/test_commands.py::TestRedisCommands::test_info",
"tests/test_commands.py::TestRedisCommands::test_lastsave",
"tests/test_commands.py::TestRedisCommands::test_lolwut",
"tests/test_commands.py::TestRedisCommands::test_reset",
"tests/test_commands.py::TestRedisCommands::test_object",
"tests/test_commands.py::TestRedisCommands::test_ping",
"tests/test_commands.py::TestRedisCommands::test_quit",
"tests/test_commands.py::TestRedisCommands::test_role",
"tests/test_commands.py::TestRedisCommands::test_select",
"tests/test_commands.py::TestRedisCommands::test_slowlog_get",
"tests/test_commands.py::TestRedisCommands::test_slowlog_get_limit",
"tests/test_commands.py::TestRedisCommands::test_slowlog_length",
"tests/test_commands.py::TestRedisCommands::test_time",
"tests/test_commands.py::TestRedisCommands::test_bgsave",
"tests/test_commands.py::TestRedisCommands::test_append",
"tests/test_commands.py::TestRedisCommands::test_bitcount",
"tests/test_commands.py::TestRedisCommands::test_bitcount_mode",
"tests/test_commands.py::TestRedisCommands::test_bitop_not_empty_string",
"tests/test_commands.py::TestRedisCommands::test_bitop_not",
"tests/test_commands.py::TestRedisCommands::test_bitop_not_in_place",
"tests/test_commands.py::TestRedisCommands::test_bitop_single_string",
"tests/test_commands.py::TestRedisCommands::test_bitop_string_operands",
"tests/test_commands.py::TestRedisCommands::test_bitpos",
"tests/test_commands.py::TestRedisCommands::test_bitpos_wrong_arguments",
"tests/test_commands.py::TestRedisCommands::test_bitpos_mode",
"tests/test_commands.py::TestRedisCommands::test_copy",
"tests/test_commands.py::TestRedisCommands::test_copy_and_replace",
"tests/test_commands.py::TestRedisCommands::test_copy_to_another_database",
"tests/test_commands.py::TestRedisCommands::test_decr",
"tests/test_commands.py::TestRedisCommands::test_decrby",
"tests/test_commands.py::TestRedisCommands::test_delete",
"tests/test_commands.py::TestRedisCommands::test_delete_with_multiple_keys",
"tests/test_commands.py::TestRedisCommands::test_delitem",
"tests/test_commands.py::TestRedisCommands::test_unlink",
"tests/test_commands.py::TestRedisCommands::test_unlink_with_multiple_keys",
"tests/test_commands.py::TestRedisCommands::test_dump_and_restore",
"tests/test_commands.py::TestRedisCommands::test_dump_and_restore_and_replace",
"tests/test_commands.py::TestRedisCommands::test_dump_and_restore_absttl",
"tests/test_commands.py::TestRedisCommands::test_exists",
"tests/test_commands.py::TestRedisCommands::test_exists_contains",
"tests/test_commands.py::TestRedisCommands::test_expire",
"tests/test_commands.py::TestRedisCommands::test_expire_option_nx",
"tests/test_commands.py::TestRedisCommands::test_expire_option_gt",
"tests/test_commands.py::TestRedisCommands::test_expire_option_lt",
"tests/test_commands.py::TestRedisCommands::test_expireat_datetime",
"tests/test_commands.py::TestRedisCommands::test_expireat_no_key",
"tests/test_commands.py::TestRedisCommands::test_expireat_unixtime",
"tests/test_commands.py::TestRedisCommands::test_expiretime",
"tests/test_commands.py::TestRedisCommands::test_expireat_option_nx",
"tests/test_commands.py::TestRedisCommands::test_expireat_option_xx",
"tests/test_commands.py::TestRedisCommands::test_expireat_option_gt",
"tests/test_commands.py::TestRedisCommands::test_expireat_option_lt",
"tests/test_commands.py::TestRedisCommands::test_get_and_set",
"tests/test_commands.py::TestRedisCommands::test_getdel",
"tests/test_commands.py::TestRedisCommands::test_getex",
"tests/test_commands.py::TestRedisCommands::test_getitem_and_setitem",
"tests/test_commands.py::TestRedisCommands::test_getitem_raises_keyerror_for_missing_key",
"tests/test_commands.py::TestRedisCommands::test_getitem_does_not_raise_keyerror_for_empty_string",
"tests/test_commands.py::TestRedisCommands::test_get_set_bit",
"tests/test_commands.py::TestRedisCommands::test_getrange",
"tests/test_commands.py::TestRedisCommands::test_getset",
"tests/test_commands.py::TestRedisCommands::test_incr",
"tests/test_commands.py::TestRedisCommands::test_incrby",
"tests/test_commands.py::TestRedisCommands::test_incrbyfloat",
"tests/test_commands.py::TestRedisCommands::test_keys",
"tests/test_commands.py::TestRedisCommands::test_mget",
"tests/test_commands.py::TestRedisCommands::test_lmove",
"tests/test_commands.py::TestRedisCommands::test_blmove",
"tests/test_commands.py::TestRedisCommands::test_mset",
"tests/test_commands.py::TestRedisCommands::test_msetnx",
"tests/test_commands.py::TestRedisCommands::test_pexpire",
"tests/test_commands.py::TestRedisCommands::test_pexpire_option_nx",
"tests/test_commands.py::TestRedisCommands::test_pexpire_option_xx",
"tests/test_commands.py::TestRedisCommands::test_pexpire_option_gt",
"tests/test_commands.py::TestRedisCommands::test_pexpire_option_lt",
"tests/test_commands.py::TestRedisCommands::test_pexpireat_datetime",
"tests/test_commands.py::TestRedisCommands::test_pexpireat_no_key",
"tests/test_commands.py::TestRedisCommands::test_pexpireat_unixtime",
"tests/test_commands.py::TestRedisCommands::test_pexpireat_option_nx",
"tests/test_commands.py::TestRedisCommands::test_pexpireat_option_xx",
"tests/test_commands.py::TestRedisCommands::test_pexpireat_option_gt",
"tests/test_commands.py::TestRedisCommands::test_pexpireat_option_lt",
"tests/test_commands.py::TestRedisCommands::test_pexpiretime",
"tests/test_commands.py::TestRedisCommands::test_psetex",
"tests/test_commands.py::TestRedisCommands::test_psetex_timedelta",
"tests/test_commands.py::TestRedisCommands::test_pttl",
"tests/test_commands.py::TestRedisCommands::test_pttl_no_key",
"tests/test_commands.py::TestRedisCommands::test_hrandfield",
"tests/test_commands.py::TestRedisCommands::test_randomkey",
"tests/test_commands.py::TestRedisCommands::test_rename",
"tests/test_commands.py::TestRedisCommands::test_renamenx",
"tests/test_commands.py::TestRedisCommands::test_set_nx",
"tests/test_commands.py::TestRedisCommands::test_set_xx",
"tests/test_commands.py::TestRedisCommands::test_set_px",
"tests/test_commands.py::TestRedisCommands::test_set_px_timedelta",
"tests/test_commands.py::TestRedisCommands::test_set_ex",
"tests/test_commands.py::TestRedisCommands::test_set_ex_timedelta",
"tests/test_commands.py::TestRedisCommands::test_set_exat_timedelta",
"tests/test_commands.py::TestRedisCommands::test_set_pxat_timedelta",
"tests/test_commands.py::TestRedisCommands::test_set_multipleoptions",
"tests/test_commands.py::TestRedisCommands::test_set_keepttl",
"tests/test_commands.py::TestRedisCommands::test_set_get",
"tests/test_commands.py::TestRedisCommands::test_setex",
"tests/test_commands.py::TestRedisCommands::test_setnx",
"tests/test_commands.py::TestRedisCommands::test_setrange",
"tests/test_commands.py::TestRedisCommands::test_strlen",
"tests/test_commands.py::TestRedisCommands::test_substr",
"tests/test_commands.py::TestRedisCommands::test_ttl",
"tests/test_commands.py::TestRedisCommands::test_ttl_nokey",
"tests/test_commands.py::TestRedisCommands::test_type",
"tests/test_commands.py::TestRedisCommands::test_blpop",
"tests/test_commands.py::TestRedisCommands::test_brpop",
"tests/test_commands.py::TestRedisCommands::test_brpoplpush",
"tests/test_commands.py::TestRedisCommands::test_brpoplpush_empty_string",
"tests/test_commands.py::TestRedisCommands::test_lindex",
"tests/test_commands.py::TestRedisCommands::test_linsert",
"tests/test_commands.py::TestRedisCommands::test_llen",
"tests/test_commands.py::TestRedisCommands::test_lpop",
"tests/test_commands.py::TestRedisCommands::test_lpop_count",
"tests/test_commands.py::TestRedisCommands::test_lpush",
"tests/test_commands.py::TestRedisCommands::test_lpushx",
"tests/test_commands.py::TestRedisCommands::test_lpushx_with_list",
"tests/test_commands.py::TestRedisCommands::test_lrange",
"tests/test_commands.py::TestRedisCommands::test_lrem",
"tests/test_commands.py::TestRedisCommands::test_lset",
"tests/test_commands.py::TestRedisCommands::test_ltrim",
"tests/test_commands.py::TestRedisCommands::test_rpop",
"tests/test_commands.py::TestRedisCommands::test_rpop_count",
"tests/test_commands.py::TestRedisCommands::test_rpoplpush",
"tests/test_commands.py::TestRedisCommands::test_rpush",
"tests/test_commands.py::TestRedisCommands::test_lpos",
"tests/test_commands.py::TestRedisCommands::test_rpushx",
"tests/test_commands.py::TestRedisCommands::test_scan",
"tests/test_commands.py::TestRedisCommands::test_scan_type",
"tests/test_commands.py::TestRedisCommands::test_scan_iter",
"tests/test_commands.py::TestRedisCommands::test_sscan",
"tests/test_commands.py::TestRedisCommands::test_sscan_iter",
"tests/test_commands.py::TestRedisCommands::test_hscan",
"tests/test_commands.py::TestRedisCommands::test_hscan_iter",
"tests/test_commands.py::TestRedisCommands::test_zscan",
"tests/test_commands.py::TestRedisCommands::test_zscan_iter",
"tests/test_commands.py::TestRedisCommands::test_sadd",
"tests/test_commands.py::TestRedisCommands::test_scard",
"tests/test_commands.py::TestRedisCommands::test_sdiff",
"tests/test_commands.py::TestRedisCommands::test_sdiffstore",
"tests/test_commands.py::TestRedisCommands::test_sinter",
"tests/test_commands.py::TestRedisCommands::test_sintercard",
"tests/test_commands.py::TestRedisCommands::test_sinterstore",
"tests/test_commands.py::TestRedisCommands::test_sismember",
"tests/test_commands.py::TestRedisCommands::test_smembers",
"tests/test_commands.py::TestRedisCommands::test_smismember",
"tests/test_commands.py::TestRedisCommands::test_smove",
"tests/test_commands.py::TestRedisCommands::test_spop",
"tests/test_commands.py::TestRedisCommands::test_spop_multi_value",
"tests/test_commands.py::TestRedisCommands::test_srandmember",
"tests/test_commands.py::TestRedisCommands::test_srandmember_multi_value",
"tests/test_commands.py::TestRedisCommands::test_srem",
"tests/test_commands.py::TestRedisCommands::test_sunion",
"tests/test_commands.py::TestRedisCommands::test_sunionstore",
"tests/test_commands.py::TestRedisCommands::test_debug_segfault",
"tests/test_commands.py::TestRedisCommands::test_script_debug",
"tests/test_commands.py::TestRedisCommands::test_zadd",
"tests/test_commands.py::TestRedisCommands::test_zadd_nx",
"tests/test_commands.py::TestRedisCommands::test_zadd_xx",
"tests/test_commands.py::TestRedisCommands::test_zadd_ch",
"tests/test_commands.py::TestRedisCommands::test_zadd_incr",
"tests/test_commands.py::TestRedisCommands::test_zadd_incr_with_xx",
"tests/test_commands.py::TestRedisCommands::test_zadd_gt_lt",
"tests/test_commands.py::TestRedisCommands::test_zcard",
"tests/test_commands.py::TestRedisCommands::test_zcount",
"tests/test_commands.py::TestRedisCommands::test_zdiff",
"tests/test_commands.py::TestRedisCommands::test_zdiffstore",
"tests/test_commands.py::TestRedisCommands::test_zincrby",
"tests/test_commands.py::TestRedisCommands::test_zlexcount",
"tests/test_commands.py::TestRedisCommands::test_zinter",
"tests/test_commands.py::TestRedisCommands::test_zintercard",
"tests/test_commands.py::TestRedisCommands::test_zinterstore_sum",
"tests/test_commands.py::TestRedisCommands::test_zinterstore_max",
"tests/test_commands.py::TestRedisCommands::test_zinterstore_min",
"tests/test_commands.py::TestRedisCommands::test_zinterstore_with_weight",
"tests/test_commands.py::TestRedisCommands::test_zpopmax",
"tests/test_commands.py::TestRedisCommands::test_zpopmin",
"tests/test_commands.py::TestRedisCommands::test_zrandemember",
"tests/test_commands.py::TestRedisCommands::test_bzpopmax",
"tests/test_commands.py::TestRedisCommands::test_bzpopmin",
"tests/test_commands.py::TestRedisCommands::test_zrange",
"tests/test_commands.py::TestRedisCommands::test_zrange_errors",
"tests/test_commands.py::TestRedisCommands::test_zrange_params",
"tests/test_commands.py::TestRedisCommands::test_zrangestore",
"tests/test_commands.py::TestRedisCommands::test_zrangebylex",
"tests/test_commands.py::TestRedisCommands::test_zrevrangebylex",
"tests/test_commands.py::TestRedisCommands::test_zrangebyscore",
"tests/test_commands.py::TestRedisCommands::test_zrank",
"tests/test_commands.py::TestRedisCommands::test_zrem",
"tests/test_commands.py::TestRedisCommands::test_zrem_multiple_keys",
"tests/test_commands.py::TestRedisCommands::test_zremrangebylex",
"tests/test_commands.py::TestRedisCommands::test_zremrangebyrank",
"tests/test_commands.py::TestRedisCommands::test_zremrangebyscore",
"tests/test_commands.py::TestRedisCommands::test_zrevrange",
"tests/test_commands.py::TestRedisCommands::test_zrevrangebyscore",
"tests/test_commands.py::TestRedisCommands::test_zrevrank",
"tests/test_commands.py::TestRedisCommands::test_zscore",
"tests/test_commands.py::TestRedisCommands::test_zunion",
"tests/test_commands.py::TestRedisCommands::test_zunionstore_sum",
"tests/test_commands.py::TestRedisCommands::test_zunionstore_max",
"tests/test_commands.py::TestRedisCommands::test_zunionstore_min",
"tests/test_commands.py::TestRedisCommands::test_zunionstore_with_weight",
"tests/test_commands.py::TestRedisCommands::test_zmscore",
"tests/test_commands.py::TestRedisCommands::test_pfadd",
"tests/test_commands.py::TestRedisCommands::test_pfcount",
"tests/test_commands.py::TestRedisCommands::test_pfmerge",
"tests/test_commands.py::TestRedisCommands::test_hget_and_hset",
"tests/test_commands.py::TestRedisCommands::test_hset_with_multi_key_values",
"tests/test_commands.py::TestRedisCommands::test_hset_with_key_values_passed_as_list",
"tests/test_commands.py::TestRedisCommands::test_hset_without_data",
"tests/test_commands.py::TestRedisCommands::test_hdel",
"tests/test_commands.py::TestRedisCommands::test_hexists",
"tests/test_commands.py::TestRedisCommands::test_hgetall",
"tests/test_commands.py::TestRedisCommands::test_hincrby",
"tests/test_commands.py::TestRedisCommands::test_hincrbyfloat",
"tests/test_commands.py::TestRedisCommands::test_hkeys",
"tests/test_commands.py::TestRedisCommands::test_hlen",
"tests/test_commands.py::TestRedisCommands::test_hmget",
"tests/test_commands.py::TestRedisCommands::test_hmset",
"tests/test_commands.py::TestRedisCommands::test_hsetnx",
"tests/test_commands.py::TestRedisCommands::test_hvals",
"tests/test_commands.py::TestRedisCommands::test_hstrlen",
"tests/test_commands.py::TestRedisCommands::test_sort_basic",
"tests/test_commands.py::TestRedisCommands::test_sort_limited",
"tests/test_commands.py::TestRedisCommands::test_sort_by",
"tests/test_commands.py::TestRedisCommands::test_sort_get",
"tests/test_commands.py::TestRedisCommands::test_sort_get_multi",
"tests/test_commands.py::TestRedisCommands::test_sort_get_groups_two",
"tests/test_commands.py::TestRedisCommands::test_sort_groups_string_get",
"tests/test_commands.py::TestRedisCommands::test_sort_groups_just_one_get",
"tests/test_commands.py::TestRedisCommands::test_sort_groups_no_get",
"tests/test_commands.py::TestRedisCommands::test_sort_groups_three_gets",
"tests/test_commands.py::TestRedisCommands::test_sort_desc",
"tests/test_commands.py::TestRedisCommands::test_sort_alpha",
"tests/test_commands.py::TestRedisCommands::test_sort_store",
"tests/test_commands.py::TestRedisCommands::test_sort_all_options",
"tests/test_commands.py::TestRedisCommands::test_sort_ro",
"tests/test_commands.py::TestRedisCommands::test_sort_issue_924",
"tests/test_commands.py::TestRedisCommands::test_cluster_addslots",
"tests/test_commands.py::TestRedisCommands::test_cluster_count_failure_reports",
"tests/test_commands.py::TestRedisCommands::test_cluster_countkeysinslot",
"tests/test_commands.py::TestRedisCommands::test_cluster_delslots",
"tests/test_commands.py::TestRedisCommands::test_cluster_failover",
"tests/test_commands.py::TestRedisCommands::test_cluster_forget",
"tests/test_commands.py::TestRedisCommands::test_cluster_info",
"tests/test_commands.py::TestRedisCommands::test_cluster_keyslot",
"tests/test_commands.py::TestRedisCommands::test_cluster_meet",
"tests/test_commands.py::TestRedisCommands::test_cluster_nodes",
"tests/test_commands.py::TestRedisCommands::test_cluster_replicate",
"tests/test_commands.py::TestRedisCommands::test_cluster_reset",
"tests/test_commands.py::TestRedisCommands::test_cluster_saveconfig",
"tests/test_commands.py::TestRedisCommands::test_cluster_setslot",
"tests/test_commands.py::TestRedisCommands::test_cluster_slaves",
"tests/test_commands.py::TestRedisCommands::test_readonly_invalid_cluster_state",
"tests/test_commands.py::TestRedisCommands::test_readonly",
"tests/test_commands.py::TestRedisCommands::test_geoadd",
"tests/test_commands.py::TestRedisCommands::test_geoadd_nx",
"tests/test_commands.py::TestRedisCommands::test_geoadd_xx",
"tests/test_commands.py::TestRedisCommands::test_geoadd_ch",
"tests/test_commands.py::TestRedisCommands::test_geoadd_invalid_params",
"tests/test_commands.py::TestRedisCommands::test_geodist",
"tests/test_commands.py::TestRedisCommands::test_geodist_units",
"tests/test_commands.py::TestRedisCommands::test_geodist_missing_one_member",
"tests/test_commands.py::TestRedisCommands::test_geodist_invalid_units",
"tests/test_commands.py::TestRedisCommands::test_geohash",
"tests/test_commands.py::TestRedisCommands::test_geopos",
"tests/test_commands.py::TestRedisCommands::test_geopos_no_value",
"tests/test_commands.py::TestRedisCommands::test_geosearch",
"tests/test_commands.py::TestRedisCommands::test_geosearch_member",
"tests/test_commands.py::TestRedisCommands::test_geosearch_sort",
"tests/test_commands.py::TestRedisCommands::test_geosearch_with",
"tests/test_commands.py::TestRedisCommands::test_geosearch_negative",
"tests/test_commands.py::TestRedisCommands::test_geosearchstore",
"tests/test_commands.py::TestRedisCommands::test_geosearchstore_dist",
"tests/test_commands.py::TestRedisCommands::test_georadius",
"tests/test_commands.py::TestRedisCommands::test_georadius_no_values",
"tests/test_commands.py::TestRedisCommands::test_georadius_units",
"tests/test_commands.py::TestRedisCommands::test_georadius_with",
"tests/test_commands.py::TestRedisCommands::test_georadius_count",
"tests/test_commands.py::TestRedisCommands::test_georadius_sort",
"tests/test_commands.py::TestRedisCommands::test_georadius_store",
"tests/test_commands.py::TestRedisCommands::test_georadius_store_dist",
"tests/test_commands.py::TestRedisCommands::test_georadiusmember",
"tests/test_commands.py::TestRedisCommands::test_georadiusmember_count",
"tests/test_commands.py::TestRedisCommands::test_xack",
"tests/test_commands.py::TestRedisCommands::test_xadd",
"tests/test_commands.py::TestRedisCommands::test_xadd_nomkstream",
"tests/test_commands.py::TestRedisCommands::test_xadd_minlen_and_limit",
"tests/test_commands.py::TestRedisCommands::test_xautoclaim",
"tests/test_commands.py::TestRedisCommands::test_xautoclaim_negative",
"tests/test_commands.py::TestRedisCommands::test_xclaim",
"tests/test_commands.py::TestRedisCommands::test_xdel",
"tests/test_commands.py::TestRedisCommands::test_xgroup_delconsumer",
"tests/test_commands.py::TestRedisCommands::test_xgroup_createconsumer",
"tests/test_commands.py::TestRedisCommands::test_xgroup_destroy",
"tests/test_commands.py::TestRedisCommands::test_xinfo_stream",
"tests/test_commands.py::TestRedisCommands::test_xinfo_stream_full",
"tests/test_commands.py::TestRedisCommands::test_xlen",
"tests/test_commands.py::TestRedisCommands::test_xpending",
"tests/test_commands.py::TestRedisCommands::test_xpending_range",
"tests/test_commands.py::TestRedisCommands::test_xpending_range_idle",
"tests/test_commands.py::TestRedisCommands::test_xpending_range_negative",
"tests/test_commands.py::TestRedisCommands::test_xrange",
"tests/test_commands.py::TestRedisCommands::test_xread",
"tests/test_commands.py::TestRedisCommands::test_xreadgroup",
"tests/test_commands.py::TestRedisCommands::test_xrevrange",
"tests/test_commands.py::TestRedisCommands::test_xtrim",
"tests/test_commands.py::TestRedisCommands::test_xtrim_minlen_and_length_args",
"tests/test_commands.py::TestRedisCommands::test_bitfield_operations",
"tests/test_commands.py::TestRedisCommands::test_memory_help",
"tests/test_commands.py::TestRedisCommands::test_memory_doctor",
"tests/test_commands.py::TestRedisCommands::test_memory_malloc_stats",
"tests/test_commands.py::TestRedisCommands::test_memory_stats",
"tests/test_commands.py::TestRedisCommands::test_memory_usage",
"tests/test_commands.py::TestRedisCommands::test_module_list",
"tests/test_commands.py::TestRedisCommands::test_command_count",
"tests/test_commands.py::TestRedisCommands::test_command_docs",
"tests/test_commands.py::TestRedisCommands::test_command_getkeys",
"tests/test_commands.py::TestRedisCommands::test_command",
"tests/test_commands.py::TestRedisCommands::test_module",
"tests/test_commands.py::TestRedisCommands::test_restore",
"tests/test_commands.py::TestRedisCommands::test_restore_idletime",
"tests/test_commands.py::TestRedisCommands::test_restore_frequency",
"tests/test_commands.py::TestRedisCommands::test_replicaof",
"tests/test_commands.py::TestRedisCommands::test_sync",
"tests/test_commands.py::TestRedisCommands::test_psync",
"tests/test_commands.py::TestBinarySave::test_binary_get_set",
"tests/test_commands.py::TestBinarySave::test_binary_lists",
"tests/test_commands.py::TestBinarySave::test_22_info",
"tests/test_commands.py::TestBinarySave::test_large_responses",
"tests/test_commands.py::TestBinarySave::test_floating_point_encoding"
] | [
"tests/test_commands.py::TestRedisCommands::test_latency_histogram_not_implemented"
] | Python | [] | [] |
|
redis/redis-py | 2,157 | redis__redis-py-2157 | [
"2136"
] | fdb9075745060e7a3633248fa6f419e895f010b7 | diff --git a/redis/commands/core.py b/redis/commands/core.py
--- a/redis/commands/core.py
+++ b/redis/commands/core.py
@@ -3505,6 +3505,7 @@ def xgroup_create(
groupname: GroupT,
id: StreamIdT = "$",
mkstream: bool = False,
+ entries_read: Optional[int] = None,
) -> ResponseT:
"""
Create a new consumer group associated with a stream.
@@ -3517,6 +3518,9 @@ def xgroup_create(
pieces: list[EncodableT] = ["XGROUP CREATE", name, groupname, id]
if mkstream:
pieces.append(b"MKSTREAM")
+ if entries_read is not None:
+ pieces.extend(["ENTRIESREAD", entries_read])
+
return self.execute_command(*pieces)
def xgroup_delconsumer(
@@ -3572,6 +3576,7 @@ def xgroup_setid(
name: KeyT,
groupname: GroupT,
id: StreamIdT,
+ entries_read: Optional[int] = None,
) -> ResponseT:
"""
Set the consumer group last delivered ID to something else.
@@ -3581,7 +3586,10 @@ def xgroup_setid(
For more information see https://redis.io/commands/xgroup-setid
"""
- return self.execute_command("XGROUP SETID", name, groupname, id)
+ pieces = [name, groupname, id]
+ if entries_read is not None:
+ pieces.extend(["ENTRIESREAD", entries_read])
+ return self.execute_command("XGROUP SETID", *pieces)
def xinfo_consumers(self, name: KeyT, groupname: GroupT) -> ResponseT:
"""
| diff --git a/tests/test_commands.py b/tests/test_commands.py
--- a/tests/test_commands.py
+++ b/tests/test_commands.py
@@ -3735,6 +3735,13 @@ def test_xadd_minlen_and_limit(self, r):
r.xadd(stream, {"foo": "bar"})
assert r.xadd(stream, {"foo": "bar"}, approximate=True, minid=m3)
+ @skip_if_server_version_lt("7.0.0")
+ def test_xadd_explicit_ms(self, r: redis.Redis):
+ stream = "stream"
+ message_id = r.xadd(stream, {"foo": "bar"}, "9999999999999999999-*")
+ ms = message_id[: message_id.index(b"-")]
+ assert ms == b"9999999999999999999"
+
@skip_if_server_version_lt("6.2.0")
def test_xautoclaim(self, r):
stream = "stream"
@@ -3820,7 +3827,7 @@ def test_xclaim(self, r):
== [message_id]
)
- @skip_if_server_version_lt("5.0.0")
+ @skip_if_server_version_lt("7.0.0")
def test_xclaim_trimmed(self, r):
# xclaim should not raise an exception if the item is not there
stream = "stream"
@@ -3841,9 +3848,8 @@ def test_xclaim_trimmed(self, r):
# xclaim them from consumer2
# the item that is still in the stream should be returned
item = r.xclaim(stream, group, "consumer2", 0, [sid1, sid2])
- assert len(item) == 2
- assert item[0] == (None, None)
- assert item[1][0] == sid2
+ assert len(item) == 1
+ assert item[0][0] == sid2
@skip_if_server_version_lt("5.0.0")
def test_xdel(self, r):
@@ -3860,7 +3866,7 @@ def test_xdel(self, r):
assert r.xdel(stream, m1) == 1
assert r.xdel(stream, m2, m3) == 2
- @skip_if_server_version_lt("5.0.0")
+ @skip_if_server_version_lt("7.0.0")
def test_xgroup_create(self, r):
# tests xgroup_create and xinfo_groups
stream = "stream"
@@ -3877,11 +3883,13 @@ def test_xgroup_create(self, r):
"consumers": 0,
"pending": 0,
"last-delivered-id": b"0-0",
+ "entries-read": None,
+ "lag": 1,
}
]
assert r.xinfo_groups(stream) == expected
- @skip_if_server_version_lt("5.0.0")
+ @skip_if_server_version_lt("7.0.0")
def test_xgroup_create_mkstream(self, r):
# tests xgroup_create and xinfo_groups
stream = "stream"
@@ -3901,6 +3909,30 @@ def test_xgroup_create_mkstream(self, r):
"consumers": 0,
"pending": 0,
"last-delivered-id": b"0-0",
+ "entries-read": None,
+ "lag": 0,
+ }
+ ]
+ assert r.xinfo_groups(stream) == expected
+
+ @skip_if_server_version_lt("7.0.0")
+ def test_xgroup_create_entriesread(self, r: redis.Redis):
+ stream = "stream"
+ group = "group"
+ r.xadd(stream, {"foo": "bar"})
+
+ # no group is setup yet, no info to obtain
+ assert r.xinfo_groups(stream) == []
+
+ assert r.xgroup_create(stream, group, 0, entries_read=7)
+ expected = [
+ {
+ "name": group.encode(),
+ "consumers": 0,
+ "pending": 0,
+ "last-delivered-id": b"0-0",
+ "entries-read": 7,
+ "lag": -6,
}
]
assert r.xinfo_groups(stream) == expected
@@ -3951,7 +3983,7 @@ def test_xgroup_destroy(self, r):
r.xgroup_create(stream, group, 0)
assert r.xgroup_destroy(stream, group)
- @skip_if_server_version_lt("5.0.0")
+ @skip_if_server_version_lt("7.0.0")
def test_xgroup_setid(self, r):
stream = "stream"
group = "group"
@@ -3959,13 +3991,15 @@ def test_xgroup_setid(self, r):
r.xgroup_create(stream, group, 0)
# advance the last_delivered_id to the message_id
- r.xgroup_setid(stream, group, message_id)
+ r.xgroup_setid(stream, group, message_id, entries_read=2)
expected = [
{
"name": group.encode(),
"consumers": 0,
"pending": 0,
"last-delivered-id": message_id,
+ "entries-read": 2,
+ "lag": -1,
}
]
assert r.xinfo_groups(stream) == expected
@@ -3995,7 +4029,7 @@ def test_xinfo_consumers(self, r):
assert isinstance(info[1].pop("idle"), int)
assert info == expected
- @skip_if_server_version_lt("5.0.0")
+ @skip_if_server_version_lt("7.0.0")
def test_xinfo_stream(self, r):
stream = "stream"
m1 = r.xadd(stream, {"foo": "bar"})
@@ -4005,6 +4039,9 @@ def test_xinfo_stream(self, r):
assert info["length"] == 2
assert info["first-entry"] == get_stream_message(r, stream, m1)
assert info["last-entry"] == get_stream_message(r, stream, m2)
+ assert info["max-deleted-entry-id"] == b"0-0"
+ assert info["entries-added"] == 2
+ assert info["recorded-first-entry-id"] == m1
@skip_if_server_version_lt("6.0.0")
def test_xinfo_stream_full(self, r):
| XINFO STREAM - add the `max-deleted-entry-id`, `entries-added`, `recorded-first-entry-id`, `entries-read` and `lag` fields
| "2022-05-01T13:21:17Z" | 4.2 | [
"tests/test_commands.py::TestResponseCallbacks::test_response_callbacks",
"tests/test_commands.py::TestResponseCallbacks::test_case_insensitive_command_names",
"tests/test_commands.py::TestRedisCommands::test_auth",
"tests/test_commands.py::TestRedisCommands::test_command_on_invalid_key_type",
"tests/test_commands.py::TestRedisCommands::test_acl_cat_no_category",
"tests/test_commands.py::TestRedisCommands::test_acl_cat_with_category",
"tests/test_commands.py::TestRedisCommands::test_acl_genpass",
"tests/test_commands.py::TestRedisCommands::test_acl_help",
"tests/test_commands.py::TestRedisCommands::test_acl_list",
"tests/test_commands.py::TestRedisCommands::test_acl_log",
"tests/test_commands.py::TestRedisCommands::test_acl_setuser_categories_without_prefix_fails",
"tests/test_commands.py::TestRedisCommands::test_acl_setuser_commands_without_prefix_fails",
"tests/test_commands.py::TestRedisCommands::test_acl_setuser_add_passwords_and_nopass_fails",
"tests/test_commands.py::TestRedisCommands::test_acl_users",
"tests/test_commands.py::TestRedisCommands::test_acl_whoami",
"tests/test_commands.py::TestRedisCommands::test_client_list",
"tests/test_commands.py::TestRedisCommands::test_client_info",
"tests/test_commands.py::TestRedisCommands::test_client_list_types_not_replica",
"tests/test_commands.py::TestRedisCommands::test_client_list_replica",
"tests/test_commands.py::TestRedisCommands::test_client_list_client_id",
"tests/test_commands.py::TestRedisCommands::test_client_id",
"tests/test_commands.py::TestRedisCommands::test_client_trackinginfo",
"tests/test_commands.py::TestRedisCommands::test_client_tracking",
"tests/test_commands.py::TestRedisCommands::test_client_unblock",
"tests/test_commands.py::TestRedisCommands::test_client_getname",
"tests/test_commands.py::TestRedisCommands::test_client_setname",
"tests/test_commands.py::TestRedisCommands::test_client_kill",
"tests/test_commands.py::TestRedisCommands::test_client_kill_filter_invalid_params",
"tests/test_commands.py::TestRedisCommands::test_client_kill_filter_by_id",
"tests/test_commands.py::TestRedisCommands::test_client_kill_filter_by_addr",
"tests/test_commands.py::TestRedisCommands::test_client_list_after_client_setname",
"tests/test_commands.py::TestRedisCommands::test_client_kill_filter_by_laddr",
"tests/test_commands.py::TestRedisCommands::test_client_kill_filter_by_user",
"tests/test_commands.py::TestRedisCommands::test_client_pause",
"tests/test_commands.py::TestRedisCommands::test_client_pause_all",
"tests/test_commands.py::TestRedisCommands::test_client_unpause",
"tests/test_commands.py::TestRedisCommands::test_client_reply",
"tests/test_commands.py::TestRedisCommands::test_client_getredir",
"tests/test_commands.py::TestRedisCommands::test_hello_notI_implemented",
"tests/test_commands.py::TestRedisCommands::test_config_get",
"tests/test_commands.py::TestRedisCommands::test_config_get_multi_params",
"tests/test_commands.py::TestRedisCommands::test_config_resetstat",
"tests/test_commands.py::TestRedisCommands::test_config_set",
"tests/test_commands.py::TestRedisCommands::test_config_set_multi_params",
"tests/test_commands.py::TestRedisCommands::test_failover",
"tests/test_commands.py::TestRedisCommands::test_dbsize",
"tests/test_commands.py::TestRedisCommands::test_echo",
"tests/test_commands.py::TestRedisCommands::test_info",
"tests/test_commands.py::TestRedisCommands::test_info_multi_sections",
"tests/test_commands.py::TestRedisCommands::test_lastsave",
"tests/test_commands.py::TestRedisCommands::test_lolwut",
"tests/test_commands.py::TestRedisCommands::test_reset",
"tests/test_commands.py::TestRedisCommands::test_object",
"tests/test_commands.py::TestRedisCommands::test_ping",
"tests/test_commands.py::TestRedisCommands::test_quit",
"tests/test_commands.py::TestRedisCommands::test_role",
"tests/test_commands.py::TestRedisCommands::test_select",
"tests/test_commands.py::TestRedisCommands::test_slowlog_get",
"tests/test_commands.py::TestRedisCommands::test_slowlog_get_limit",
"tests/test_commands.py::TestRedisCommands::test_slowlog_length",
"tests/test_commands.py::TestRedisCommands::test_time",
"tests/test_commands.py::TestRedisCommands::test_bgsave",
"tests/test_commands.py::TestRedisCommands::test_append",
"tests/test_commands.py::TestRedisCommands::test_bitcount",
"tests/test_commands.py::TestRedisCommands::test_bitcount_mode",
"tests/test_commands.py::TestRedisCommands::test_bitop_not_empty_string",
"tests/test_commands.py::TestRedisCommands::test_bitop_not",
"tests/test_commands.py::TestRedisCommands::test_bitop_not_in_place",
"tests/test_commands.py::TestRedisCommands::test_bitop_single_string",
"tests/test_commands.py::TestRedisCommands::test_bitop_string_operands",
"tests/test_commands.py::TestRedisCommands::test_bitpos",
"tests/test_commands.py::TestRedisCommands::test_bitpos_wrong_arguments",
"tests/test_commands.py::TestRedisCommands::test_bitpos_mode",
"tests/test_commands.py::TestRedisCommands::test_copy",
"tests/test_commands.py::TestRedisCommands::test_copy_and_replace",
"tests/test_commands.py::TestRedisCommands::test_copy_to_another_database",
"tests/test_commands.py::TestRedisCommands::test_decr",
"tests/test_commands.py::TestRedisCommands::test_decrby",
"tests/test_commands.py::TestRedisCommands::test_delete",
"tests/test_commands.py::TestRedisCommands::test_delete_with_multiple_keys",
"tests/test_commands.py::TestRedisCommands::test_delitem",
"tests/test_commands.py::TestRedisCommands::test_unlink",
"tests/test_commands.py::TestRedisCommands::test_unlink_with_multiple_keys",
"tests/test_commands.py::TestRedisCommands::test_dump_and_restore",
"tests/test_commands.py::TestRedisCommands::test_dump_and_restore_and_replace",
"tests/test_commands.py::TestRedisCommands::test_dump_and_restore_absttl",
"tests/test_commands.py::TestRedisCommands::test_exists",
"tests/test_commands.py::TestRedisCommands::test_exists_contains",
"tests/test_commands.py::TestRedisCommands::test_expire",
"tests/test_commands.py::TestRedisCommands::test_expire_option_nx",
"tests/test_commands.py::TestRedisCommands::test_expire_option_gt",
"tests/test_commands.py::TestRedisCommands::test_expire_option_lt",
"tests/test_commands.py::TestRedisCommands::test_expireat_datetime",
"tests/test_commands.py::TestRedisCommands::test_expireat_no_key",
"tests/test_commands.py::TestRedisCommands::test_expireat_unixtime",
"tests/test_commands.py::TestRedisCommands::test_expiretime",
"tests/test_commands.py::TestRedisCommands::test_expireat_option_nx",
"tests/test_commands.py::TestRedisCommands::test_expireat_option_xx",
"tests/test_commands.py::TestRedisCommands::test_expireat_option_gt",
"tests/test_commands.py::TestRedisCommands::test_expireat_option_lt",
"tests/test_commands.py::TestRedisCommands::test_get_and_set",
"tests/test_commands.py::TestRedisCommands::test_getdel",
"tests/test_commands.py::TestRedisCommands::test_getex",
"tests/test_commands.py::TestRedisCommands::test_getitem_and_setitem",
"tests/test_commands.py::TestRedisCommands::test_getitem_raises_keyerror_for_missing_key",
"tests/test_commands.py::TestRedisCommands::test_getitem_does_not_raise_keyerror_for_empty_string",
"tests/test_commands.py::TestRedisCommands::test_get_set_bit",
"tests/test_commands.py::TestRedisCommands::test_getrange",
"tests/test_commands.py::TestRedisCommands::test_getset",
"tests/test_commands.py::TestRedisCommands::test_incr",
"tests/test_commands.py::TestRedisCommands::test_incrby",
"tests/test_commands.py::TestRedisCommands::test_incrbyfloat",
"tests/test_commands.py::TestRedisCommands::test_keys",
"tests/test_commands.py::TestRedisCommands::test_mget",
"tests/test_commands.py::TestRedisCommands::test_lmove",
"tests/test_commands.py::TestRedisCommands::test_blmove",
"tests/test_commands.py::TestRedisCommands::test_mset",
"tests/test_commands.py::TestRedisCommands::test_msetnx",
"tests/test_commands.py::TestRedisCommands::test_pexpire",
"tests/test_commands.py::TestRedisCommands::test_pexpire_option_nx",
"tests/test_commands.py::TestRedisCommands::test_pexpire_option_xx",
"tests/test_commands.py::TestRedisCommands::test_pexpire_option_gt",
"tests/test_commands.py::TestRedisCommands::test_pexpire_option_lt",
"tests/test_commands.py::TestRedisCommands::test_pexpireat_datetime",
"tests/test_commands.py::TestRedisCommands::test_pexpireat_no_key",
"tests/test_commands.py::TestRedisCommands::test_pexpireat_unixtime",
"tests/test_commands.py::TestRedisCommands::test_pexpireat_option_nx",
"tests/test_commands.py::TestRedisCommands::test_pexpireat_option_xx",
"tests/test_commands.py::TestRedisCommands::test_pexpireat_option_gt",
"tests/test_commands.py::TestRedisCommands::test_pexpireat_option_lt",
"tests/test_commands.py::TestRedisCommands::test_pexpiretime",
"tests/test_commands.py::TestRedisCommands::test_psetex",
"tests/test_commands.py::TestRedisCommands::test_psetex_timedelta",
"tests/test_commands.py::TestRedisCommands::test_pttl",
"tests/test_commands.py::TestRedisCommands::test_pttl_no_key",
"tests/test_commands.py::TestRedisCommands::test_hrandfield",
"tests/test_commands.py::TestRedisCommands::test_randomkey",
"tests/test_commands.py::TestRedisCommands::test_rename",
"tests/test_commands.py::TestRedisCommands::test_renamenx",
"tests/test_commands.py::TestRedisCommands::test_set_nx",
"tests/test_commands.py::TestRedisCommands::test_set_xx",
"tests/test_commands.py::TestRedisCommands::test_set_px",
"tests/test_commands.py::TestRedisCommands::test_set_px_timedelta",
"tests/test_commands.py::TestRedisCommands::test_set_ex",
"tests/test_commands.py::TestRedisCommands::test_set_ex_timedelta",
"tests/test_commands.py::TestRedisCommands::test_set_exat_timedelta",
"tests/test_commands.py::TestRedisCommands::test_set_pxat_timedelta",
"tests/test_commands.py::TestRedisCommands::test_set_multipleoptions",
"tests/test_commands.py::TestRedisCommands::test_set_keepttl",
"tests/test_commands.py::TestRedisCommands::test_set_get",
"tests/test_commands.py::TestRedisCommands::test_setex",
"tests/test_commands.py::TestRedisCommands::test_setnx",
"tests/test_commands.py::TestRedisCommands::test_setrange",
"tests/test_commands.py::TestRedisCommands::test_strlen",
"tests/test_commands.py::TestRedisCommands::test_substr",
"tests/test_commands.py::TestRedisCommands::test_ttl",
"tests/test_commands.py::TestRedisCommands::test_ttl_nokey",
"tests/test_commands.py::TestRedisCommands::test_type",
"tests/test_commands.py::TestRedisCommands::test_blpop",
"tests/test_commands.py::TestRedisCommands::test_brpop",
"tests/test_commands.py::TestRedisCommands::test_brpoplpush",
"tests/test_commands.py::TestRedisCommands::test_brpoplpush_empty_string",
"tests/test_commands.py::TestRedisCommands::test_lindex",
"tests/test_commands.py::TestRedisCommands::test_linsert",
"tests/test_commands.py::TestRedisCommands::test_llen",
"tests/test_commands.py::TestRedisCommands::test_lpop",
"tests/test_commands.py::TestRedisCommands::test_lpop_count",
"tests/test_commands.py::TestRedisCommands::test_lpush",
"tests/test_commands.py::TestRedisCommands::test_lpushx",
"tests/test_commands.py::TestRedisCommands::test_lpushx_with_list",
"tests/test_commands.py::TestRedisCommands::test_lrange",
"tests/test_commands.py::TestRedisCommands::test_lrem",
"tests/test_commands.py::TestRedisCommands::test_lset",
"tests/test_commands.py::TestRedisCommands::test_ltrim",
"tests/test_commands.py::TestRedisCommands::test_rpop",
"tests/test_commands.py::TestRedisCommands::test_rpop_count",
"tests/test_commands.py::TestRedisCommands::test_rpoplpush",
"tests/test_commands.py::TestRedisCommands::test_rpush",
"tests/test_commands.py::TestRedisCommands::test_lpos",
"tests/test_commands.py::TestRedisCommands::test_rpushx",
"tests/test_commands.py::TestRedisCommands::test_scan",
"tests/test_commands.py::TestRedisCommands::test_scan_type",
"tests/test_commands.py::TestRedisCommands::test_scan_iter",
"tests/test_commands.py::TestRedisCommands::test_sscan",
"tests/test_commands.py::TestRedisCommands::test_sscan_iter",
"tests/test_commands.py::TestRedisCommands::test_hscan",
"tests/test_commands.py::TestRedisCommands::test_hscan_iter",
"tests/test_commands.py::TestRedisCommands::test_zscan",
"tests/test_commands.py::TestRedisCommands::test_zscan_iter",
"tests/test_commands.py::TestRedisCommands::test_sadd",
"tests/test_commands.py::TestRedisCommands::test_scard",
"tests/test_commands.py::TestRedisCommands::test_sdiff",
"tests/test_commands.py::TestRedisCommands::test_sdiffstore",
"tests/test_commands.py::TestRedisCommands::test_sinter",
"tests/test_commands.py::TestRedisCommands::test_sintercard",
"tests/test_commands.py::TestRedisCommands::test_sinterstore",
"tests/test_commands.py::TestRedisCommands::test_sismember",
"tests/test_commands.py::TestRedisCommands::test_smembers",
"tests/test_commands.py::TestRedisCommands::test_smismember",
"tests/test_commands.py::TestRedisCommands::test_smove",
"tests/test_commands.py::TestRedisCommands::test_spop",
"tests/test_commands.py::TestRedisCommands::test_spop_multi_value",
"tests/test_commands.py::TestRedisCommands::test_srandmember",
"tests/test_commands.py::TestRedisCommands::test_srandmember_multi_value",
"tests/test_commands.py::TestRedisCommands::test_srem",
"tests/test_commands.py::TestRedisCommands::test_sunion",
"tests/test_commands.py::TestRedisCommands::test_sunionstore",
"tests/test_commands.py::TestRedisCommands::test_debug_segfault",
"tests/test_commands.py::TestRedisCommands::test_script_debug",
"tests/test_commands.py::TestRedisCommands::test_zadd",
"tests/test_commands.py::TestRedisCommands::test_zadd_nx",
"tests/test_commands.py::TestRedisCommands::test_zadd_xx",
"tests/test_commands.py::TestRedisCommands::test_zadd_ch",
"tests/test_commands.py::TestRedisCommands::test_zadd_incr",
"tests/test_commands.py::TestRedisCommands::test_zadd_incr_with_xx",
"tests/test_commands.py::TestRedisCommands::test_zadd_gt_lt",
"tests/test_commands.py::TestRedisCommands::test_zcard",
"tests/test_commands.py::TestRedisCommands::test_zcount",
"tests/test_commands.py::TestRedisCommands::test_zdiff",
"tests/test_commands.py::TestRedisCommands::test_zdiffstore",
"tests/test_commands.py::TestRedisCommands::test_zincrby",
"tests/test_commands.py::TestRedisCommands::test_zlexcount",
"tests/test_commands.py::TestRedisCommands::test_zinter",
"tests/test_commands.py::TestRedisCommands::test_zintercard",
"tests/test_commands.py::TestRedisCommands::test_zinterstore_sum",
"tests/test_commands.py::TestRedisCommands::test_zinterstore_max",
"tests/test_commands.py::TestRedisCommands::test_zinterstore_min",
"tests/test_commands.py::TestRedisCommands::test_zinterstore_with_weight",
"tests/test_commands.py::TestRedisCommands::test_zpopmax",
"tests/test_commands.py::TestRedisCommands::test_zpopmin",
"tests/test_commands.py::TestRedisCommands::test_zrandemember",
"tests/test_commands.py::TestRedisCommands::test_bzpopmax",
"tests/test_commands.py::TestRedisCommands::test_bzpopmin",
"tests/test_commands.py::TestRedisCommands::test_zrange",
"tests/test_commands.py::TestRedisCommands::test_zrange_errors",
"tests/test_commands.py::TestRedisCommands::test_zrange_params",
"tests/test_commands.py::TestRedisCommands::test_zrangestore",
"tests/test_commands.py::TestRedisCommands::test_zrangebylex",
"tests/test_commands.py::TestRedisCommands::test_zrevrangebylex",
"tests/test_commands.py::TestRedisCommands::test_zrangebyscore",
"tests/test_commands.py::TestRedisCommands::test_zrank",
"tests/test_commands.py::TestRedisCommands::test_zrem",
"tests/test_commands.py::TestRedisCommands::test_zrem_multiple_keys",
"tests/test_commands.py::TestRedisCommands::test_zremrangebylex",
"tests/test_commands.py::TestRedisCommands::test_zremrangebyrank",
"tests/test_commands.py::TestRedisCommands::test_zremrangebyscore",
"tests/test_commands.py::TestRedisCommands::test_zrevrange",
"tests/test_commands.py::TestRedisCommands::test_zrevrangebyscore",
"tests/test_commands.py::TestRedisCommands::test_zrevrank",
"tests/test_commands.py::TestRedisCommands::test_zscore",
"tests/test_commands.py::TestRedisCommands::test_zunion",
"tests/test_commands.py::TestRedisCommands::test_zunionstore_sum",
"tests/test_commands.py::TestRedisCommands::test_zunionstore_max",
"tests/test_commands.py::TestRedisCommands::test_zunionstore_min",
"tests/test_commands.py::TestRedisCommands::test_zunionstore_with_weight",
"tests/test_commands.py::TestRedisCommands::test_zmscore",
"tests/test_commands.py::TestRedisCommands::test_pfadd",
"tests/test_commands.py::TestRedisCommands::test_pfcount",
"tests/test_commands.py::TestRedisCommands::test_pfmerge",
"tests/test_commands.py::TestRedisCommands::test_hget_and_hset",
"tests/test_commands.py::TestRedisCommands::test_hset_with_multi_key_values",
"tests/test_commands.py::TestRedisCommands::test_hset_with_key_values_passed_as_list",
"tests/test_commands.py::TestRedisCommands::test_hset_without_data",
"tests/test_commands.py::TestRedisCommands::test_hdel",
"tests/test_commands.py::TestRedisCommands::test_hexists",
"tests/test_commands.py::TestRedisCommands::test_hgetall",
"tests/test_commands.py::TestRedisCommands::test_hincrby",
"tests/test_commands.py::TestRedisCommands::test_hincrbyfloat",
"tests/test_commands.py::TestRedisCommands::test_hkeys",
"tests/test_commands.py::TestRedisCommands::test_hlen",
"tests/test_commands.py::TestRedisCommands::test_hmget",
"tests/test_commands.py::TestRedisCommands::test_hmset",
"tests/test_commands.py::TestRedisCommands::test_hsetnx",
"tests/test_commands.py::TestRedisCommands::test_hvals",
"tests/test_commands.py::TestRedisCommands::test_hstrlen",
"tests/test_commands.py::TestRedisCommands::test_sort_basic",
"tests/test_commands.py::TestRedisCommands::test_sort_limited",
"tests/test_commands.py::TestRedisCommands::test_sort_by",
"tests/test_commands.py::TestRedisCommands::test_sort_get",
"tests/test_commands.py::TestRedisCommands::test_sort_get_multi",
"tests/test_commands.py::TestRedisCommands::test_sort_get_groups_two",
"tests/test_commands.py::TestRedisCommands::test_sort_groups_string_get",
"tests/test_commands.py::TestRedisCommands::test_sort_groups_just_one_get",
"tests/test_commands.py::TestRedisCommands::test_sort_groups_no_get",
"tests/test_commands.py::TestRedisCommands::test_sort_groups_three_gets",
"tests/test_commands.py::TestRedisCommands::test_sort_desc",
"tests/test_commands.py::TestRedisCommands::test_sort_alpha",
"tests/test_commands.py::TestRedisCommands::test_sort_store",
"tests/test_commands.py::TestRedisCommands::test_sort_all_options",
"tests/test_commands.py::TestRedisCommands::test_sort_ro",
"tests/test_commands.py::TestRedisCommands::test_sort_issue_924",
"tests/test_commands.py::TestRedisCommands::test_cluster_addslots",
"tests/test_commands.py::TestRedisCommands::test_cluster_count_failure_reports",
"tests/test_commands.py::TestRedisCommands::test_cluster_countkeysinslot",
"tests/test_commands.py::TestRedisCommands::test_cluster_delslots",
"tests/test_commands.py::TestRedisCommands::test_cluster_failover",
"tests/test_commands.py::TestRedisCommands::test_cluster_forget",
"tests/test_commands.py::TestRedisCommands::test_cluster_info",
"tests/test_commands.py::TestRedisCommands::test_cluster_keyslot",
"tests/test_commands.py::TestRedisCommands::test_cluster_meet",
"tests/test_commands.py::TestRedisCommands::test_cluster_nodes",
"tests/test_commands.py::TestRedisCommands::test_cluster_replicate",
"tests/test_commands.py::TestRedisCommands::test_cluster_reset",
"tests/test_commands.py::TestRedisCommands::test_cluster_saveconfig",
"tests/test_commands.py::TestRedisCommands::test_cluster_setslot",
"tests/test_commands.py::TestRedisCommands::test_cluster_slaves",
"tests/test_commands.py::TestRedisCommands::test_readonly_invalid_cluster_state",
"tests/test_commands.py::TestRedisCommands::test_readonly",
"tests/test_commands.py::TestRedisCommands::test_geoadd",
"tests/test_commands.py::TestRedisCommands::test_geoadd_nx",
"tests/test_commands.py::TestRedisCommands::test_geoadd_xx",
"tests/test_commands.py::TestRedisCommands::test_geoadd_ch",
"tests/test_commands.py::TestRedisCommands::test_geoadd_invalid_params",
"tests/test_commands.py::TestRedisCommands::test_geodist",
"tests/test_commands.py::TestRedisCommands::test_geodist_units",
"tests/test_commands.py::TestRedisCommands::test_geodist_missing_one_member",
"tests/test_commands.py::TestRedisCommands::test_geodist_invalid_units",
"tests/test_commands.py::TestRedisCommands::test_geohash",
"tests/test_commands.py::TestRedisCommands::test_geopos",
"tests/test_commands.py::TestRedisCommands::test_geopos_no_value",
"tests/test_commands.py::TestRedisCommands::test_geosearch",
"tests/test_commands.py::TestRedisCommands::test_geosearch_member",
"tests/test_commands.py::TestRedisCommands::test_geosearch_sort",
"tests/test_commands.py::TestRedisCommands::test_geosearch_with",
"tests/test_commands.py::TestRedisCommands::test_geosearch_negative",
"tests/test_commands.py::TestRedisCommands::test_geosearchstore",
"tests/test_commands.py::TestRedisCommands::test_geosearchstore_dist",
"tests/test_commands.py::TestRedisCommands::test_georadius",
"tests/test_commands.py::TestRedisCommands::test_georadius_no_values",
"tests/test_commands.py::TestRedisCommands::test_georadius_units",
"tests/test_commands.py::TestRedisCommands::test_georadius_with",
"tests/test_commands.py::TestRedisCommands::test_georadius_count",
"tests/test_commands.py::TestRedisCommands::test_georadius_sort",
"tests/test_commands.py::TestRedisCommands::test_georadius_store",
"tests/test_commands.py::TestRedisCommands::test_georadius_store_dist",
"tests/test_commands.py::TestRedisCommands::test_georadiusmember",
"tests/test_commands.py::TestRedisCommands::test_georadiusmember_count",
"tests/test_commands.py::TestRedisCommands::test_xack",
"tests/test_commands.py::TestRedisCommands::test_xadd",
"tests/test_commands.py::TestRedisCommands::test_xadd_nomkstream",
"tests/test_commands.py::TestRedisCommands::test_xadd_minlen_and_limit",
"tests/test_commands.py::TestRedisCommands::test_xadd_explicit_ms",
"tests/test_commands.py::TestRedisCommands::test_xautoclaim",
"tests/test_commands.py::TestRedisCommands::test_xautoclaim_negative",
"tests/test_commands.py::TestRedisCommands::test_xclaim",
"tests/test_commands.py::TestRedisCommands::test_xclaim_trimmed",
"tests/test_commands.py::TestRedisCommands::test_xdel",
"tests/test_commands.py::TestRedisCommands::test_xgroup_create",
"tests/test_commands.py::TestRedisCommands::test_xgroup_create_mkstream",
"tests/test_commands.py::TestRedisCommands::test_xgroup_delconsumer",
"tests/test_commands.py::TestRedisCommands::test_xgroup_createconsumer",
"tests/test_commands.py::TestRedisCommands::test_xgroup_destroy",
"tests/test_commands.py::TestRedisCommands::test_xinfo_stream",
"tests/test_commands.py::TestRedisCommands::test_xinfo_stream_full",
"tests/test_commands.py::TestRedisCommands::test_xlen",
"tests/test_commands.py::TestRedisCommands::test_xpending",
"tests/test_commands.py::TestRedisCommands::test_xpending_range",
"tests/test_commands.py::TestRedisCommands::test_xpending_range_idle",
"tests/test_commands.py::TestRedisCommands::test_xpending_range_negative",
"tests/test_commands.py::TestRedisCommands::test_xrange",
"tests/test_commands.py::TestRedisCommands::test_xread",
"tests/test_commands.py::TestRedisCommands::test_xreadgroup",
"tests/test_commands.py::TestRedisCommands::test_xrevrange",
"tests/test_commands.py::TestRedisCommands::test_xtrim",
"tests/test_commands.py::TestRedisCommands::test_xtrim_minlen_and_length_args",
"tests/test_commands.py::TestRedisCommands::test_bitfield_operations",
"tests/test_commands.py::TestRedisCommands::test_memory_help",
"tests/test_commands.py::TestRedisCommands::test_memory_doctor",
"tests/test_commands.py::TestRedisCommands::test_memory_malloc_stats",
"tests/test_commands.py::TestRedisCommands::test_memory_stats",
"tests/test_commands.py::TestRedisCommands::test_memory_usage",
"tests/test_commands.py::TestRedisCommands::test_latency_histogram_not_implemented",
"tests/test_commands.py::TestRedisCommands::test_module_list",
"tests/test_commands.py::TestRedisCommands::test_command_count",
"tests/test_commands.py::TestRedisCommands::test_command_docs",
"tests/test_commands.py::TestRedisCommands::test_command_getkeys",
"tests/test_commands.py::TestRedisCommands::test_command",
"tests/test_commands.py::TestRedisCommands::test_module",
"tests/test_commands.py::TestRedisCommands::test_module_loadex",
"tests/test_commands.py::TestRedisCommands::test_restore",
"tests/test_commands.py::TestRedisCommands::test_restore_idletime",
"tests/test_commands.py::TestRedisCommands::test_restore_frequency",
"tests/test_commands.py::TestRedisCommands::test_replicaof",
"tests/test_commands.py::TestRedisCommands::test_sync",
"tests/test_commands.py::TestRedisCommands::test_psync",
"tests/test_commands.py::TestBinarySave::test_binary_get_set",
"tests/test_commands.py::TestBinarySave::test_binary_lists",
"tests/test_commands.py::TestBinarySave::test_22_info",
"tests/test_commands.py::TestBinarySave::test_large_responses",
"tests/test_commands.py::TestBinarySave::test_floating_point_encoding"
] | [
"tests/test_commands.py::TestRedisCommands::test_xgroup_create_entriesread",
"tests/test_commands.py::TestRedisCommands::test_xgroup_setid"
] | Python | [] | [] |
|
redis/redis-py | 2,329 | redis__redis-py-2329 | [
"2325"
] | 2cea637b2e936265d2001043d2b2d4e62559dc17 | diff --git a/redis/asyncio/connection.py b/redis/asyncio/connection.py
--- a/redis/asyncio/connection.py
+++ b/redis/asyncio/connection.py
@@ -87,6 +87,15 @@ class _Sentinel(enum.Enum):
"exports one or more module-side data "
"types, can't unload"
)
+# user send an AUTH cmd to a server without authorization configured
+NO_AUTH_SET_ERROR = {
+ # Redis >= 6.0
+ "AUTH <password> called without any password "
+ "configured for the default user. Are you sure "
+ "your configuration is correct?": AuthenticationError,
+ # Redis < 6.0
+ "Client sent AUTH, but no password is set": AuthenticationError,
+}
class _HiredisReaderArgs(TypedDict, total=False):
@@ -160,7 +169,9 @@ class BaseParser:
MODULE_EXPORTS_DATA_TYPES_ERROR: ModuleError,
NO_SUCH_MODULE_ERROR: ModuleError,
MODULE_UNLOAD_NOT_POSSIBLE_ERROR: ModuleError,
+ **NO_AUTH_SET_ERROR,
},
+ "WRONGPASS": AuthenticationError,
"EXECABORT": ExecAbortError,
"LOADING": BusyLoadingError,
"NOSCRIPT": NoScriptError,
diff --git a/redis/connection.py b/redis/connection.py
--- a/redis/connection.py
+++ b/redis/connection.py
@@ -80,6 +80,15 @@
"exports one or more module-side data "
"types, can't unload"
)
+# user send an AUTH cmd to a server without authorization configured
+NO_AUTH_SET_ERROR = {
+ # Redis >= 6.0
+ "AUTH <password> called without any password "
+ "configured for the default user. Are you sure "
+ "your configuration is correct?": AuthenticationError,
+ # Redis < 6.0
+ "Client sent AUTH, but no password is set": AuthenticationError,
+}
class Encoder:
@@ -127,7 +136,6 @@ class BaseParser:
EXCEPTION_CLASSES = {
"ERR": {
"max number of clients reached": ConnectionError,
- "Client sent AUTH, but no password is set": AuthenticationError,
"invalid password": AuthenticationError,
# some Redis server versions report invalid command syntax
# in lowercase
@@ -141,7 +149,9 @@ class BaseParser:
MODULE_EXPORTS_DATA_TYPES_ERROR: ModuleError,
NO_SUCH_MODULE_ERROR: ModuleError,
MODULE_UNLOAD_NOT_POSSIBLE_ERROR: ModuleError,
+ **NO_AUTH_SET_ERROR,
},
+ "WRONGPASS": AuthenticationError,
"EXECABORT": ExecAbortError,
"LOADING": BusyLoadingError,
"NOSCRIPT": NoScriptError,
| diff --git a/tests/test_commands.py b/tests/test_commands.py
--- a/tests/test_commands.py
+++ b/tests/test_commands.py
@@ -68,6 +68,14 @@ def test_case_insensitive_command_names(self, r):
class TestRedisCommands:
@skip_if_redis_enterprise()
def test_auth(self, r, request):
+ # sending an AUTH command before setting a user/password on the
+ # server should return an AuthenticationError
+ with pytest.raises(exceptions.AuthenticationError):
+ r.auth("some_password")
+
+ with pytest.raises(exceptions.AuthenticationError):
+ r.auth("some_password", "some_user")
+
# first, test for default user (`username` is supposed to be optional)
default_username = "default"
temp_pass = "temp_pass"
@@ -81,9 +89,19 @@ def test_auth(self, r, request):
def teardown():
try:
- r.auth(temp_pass)
- except exceptions.ResponseError:
- r.auth("default", "")
+ # this is needed because after an AuthenticationError the connection
+ # is closed, and if we send an AUTH command a new connection is
+ # created, but in this case we'd get an "Authentication required"
+ # error when switching to the db 9 because we're not authenticated yet
+ # setting the password on the connection itself triggers the
+ # authentication in the connection's `on_connect` method
+ r.connection.password = temp_pass
+ except AttributeError:
+ # connection field is not set in Redis Cluster, but that's ok
+ # because the problem discussed above does not apply to Redis Cluster
+ pass
+
+ r.auth(temp_pass)
r.config_set("requirepass", "")
r.acl_deluser(username)
@@ -95,7 +113,7 @@ def teardown():
assert r.auth(username=username, password="strong_password") is True
- with pytest.raises(exceptions.ResponseError):
+ with pytest.raises(exceptions.AuthenticationError):
r.auth(username=username, password="wrong_password")
def test_command_on_invalid_key_type(self, r):
diff --git a/tests/test_connection_pool.py b/tests/test_connection_pool.py
--- a/tests/test_connection_pool.py
+++ b/tests/test_connection_pool.py
@@ -545,22 +545,40 @@ def test_connect_from_url_unix(self):
)
@skip_if_redis_enterprise()
- def test_connect_no_auth_supplied_when_required(self, r):
+ def test_connect_no_auth_configured(self, r):
"""
- AuthenticationError should be raised when the server requires a
- password but one isn't supplied.
+ AuthenticationError should be raised when the server is not configured with auth
+ but credentials are supplied by the user.
"""
+ # Redis < 6
with pytest.raises(redis.AuthenticationError):
r.execute_command(
"DEBUG", "ERROR", "ERR Client sent AUTH, but no password is set"
)
+ # Redis >= 6
+ with pytest.raises(redis.AuthenticationError):
+ r.execute_command(
+ "DEBUG",
+ "ERROR",
+ "ERR AUTH <password> called without any password "
+ "configured for the default user. Are you sure "
+ "your configuration is correct?",
+ )
+
@skip_if_redis_enterprise()
- def test_connect_invalid_password_supplied(self, r):
- "AuthenticationError should be raised when sending the wrong password"
+ def test_connect_invalid_auth_credentials_supplied(self, r):
+ """
+ AuthenticationError should be raised when sending invalid username/password
+ """
+ # Redis < 6
with pytest.raises(redis.AuthenticationError):
r.execute_command("DEBUG", "ERROR", "ERR invalid password")
+ # Redis >= 6
+ with pytest.raises(redis.AuthenticationError):
+ r.execute_command("DEBUG", "ERROR", "WRONGPASS")
+
@pytest.mark.onlynoncluster
class TestMultiConnectionClient:
| WRONGPASS response doesn't raise AuthenticationError exception
**Version**: What redis-py and what redis version is the issue happening on?
redis-py 4.3.4
redis 6.3.6
**Platform**: What platform / version? (For example Python 3.5.1 on Windows 7 / Ubuntu 15.10 / Azure)
python 3.9 on macOS Monterey 12.0.1
**Description**: Description of your issue, stack traces from errors and code that reproduces the issue
I've expected that `WRONGPASS` response should raise `AuthenticationError` exception. But when I tried to catch it, it's just ResponseError.
```
if __name__ == '__main__':
redis = RedisClient(
host="localhost",
port=6379,
socket_timeout=REDIS_SOCKET_TIMEOUT_SECONDS,
retry_on_timeout=True,
username="admin",
# actual password is different
password="random"
)
try:
val = redis.get("test")
except AuthenticationError as e:
print("I can't reach that code")
val = 0
print(val)
```
When I debugged `connection.py` file, I guess that raising of `AuthenticationError` in case of `WRONGPASS` should occur [here](https://github.com/redis/redis-py/blob/e6cd4fdf3b159d7f118f154e28e884069da89d7e/redis/connection.py#L722):
```
if str_if_bytes(auth_response) != "OK":
raise AuthenticationError("Invalid Username or Password")
```
but this part of code can't be reached because of this [part](https://github.com/redis/redis-py/blob/e6cd4fdf3b159d7f118f154e28e884069da89d7e/redis/connection.py#L713):
```
auth_response = self.read_response()
```
It raises `ResponseError` which is not handled in any except block in function `on_connect`.
| "2022-08-09T17:15:44Z" | 4.4 | [
"tests/test_commands.py::TestResponseCallbacks::test_response_callbacks",
"tests/test_commands.py::TestResponseCallbacks::test_case_insensitive_command_names",
"tests/test_commands.py::TestRedisCommands::test_command_on_invalid_key_type",
"tests/test_commands.py::TestRedisCommands::test_acl_cat_no_category",
"tests/test_commands.py::TestRedisCommands::test_acl_cat_with_category",
"tests/test_commands.py::TestRedisCommands::test_acl_deluser",
"tests/test_commands.py::TestRedisCommands::test_acl_genpass",
"tests/test_commands.py::TestRedisCommands::test_acl_help",
"tests/test_commands.py::TestRedisCommands::test_acl_list",
"tests/test_commands.py::TestRedisCommands::test_acl_log",
"tests/test_commands.py::TestRedisCommands::test_acl_setuser_categories_without_prefix_fails",
"tests/test_commands.py::TestRedisCommands::test_acl_setuser_commands_without_prefix_fails",
"tests/test_commands.py::TestRedisCommands::test_acl_setuser_add_passwords_and_nopass_fails",
"tests/test_commands.py::TestRedisCommands::test_acl_users",
"tests/test_commands.py::TestRedisCommands::test_acl_whoami",
"tests/test_commands.py::TestRedisCommands::test_client_list",
"tests/test_commands.py::TestRedisCommands::test_client_info",
"tests/test_commands.py::TestRedisCommands::test_client_list_types_not_replica",
"tests/test_commands.py::TestRedisCommands::test_client_list_replica",
"tests/test_commands.py::TestRedisCommands::test_client_list_client_id",
"tests/test_commands.py::TestRedisCommands::test_client_id",
"tests/test_commands.py::TestRedisCommands::test_client_trackinginfo",
"tests/test_commands.py::TestRedisCommands::test_client_tracking",
"tests/test_commands.py::TestRedisCommands::test_client_unblock",
"tests/test_commands.py::TestRedisCommands::test_client_getname",
"tests/test_commands.py::TestRedisCommands::test_client_setname",
"tests/test_commands.py::TestRedisCommands::test_client_kill",
"tests/test_commands.py::TestRedisCommands::test_client_kill_filter_invalid_params",
"tests/test_commands.py::TestRedisCommands::test_client_kill_filter_by_id",
"tests/test_commands.py::TestRedisCommands::test_client_kill_filter_by_addr",
"tests/test_commands.py::TestRedisCommands::test_client_list_after_client_setname",
"tests/test_commands.py::TestRedisCommands::test_client_kill_filter_by_laddr",
"tests/test_commands.py::TestRedisCommands::test_client_kill_filter_by_user",
"tests/test_commands.py::TestRedisCommands::test_client_pause",
"tests/test_commands.py::TestRedisCommands::test_client_pause_all",
"tests/test_commands.py::TestRedisCommands::test_client_unpause",
"tests/test_commands.py::TestRedisCommands::test_client_no_evict",
"tests/test_commands.py::TestRedisCommands::test_client_reply",
"tests/test_commands.py::TestRedisCommands::test_client_getredir",
"tests/test_commands.py::TestRedisCommands::test_hello_notI_implemented",
"tests/test_commands.py::TestRedisCommands::test_config_get",
"tests/test_commands.py::TestRedisCommands::test_config_get_multi_params",
"tests/test_commands.py::TestRedisCommands::test_config_resetstat",
"tests/test_commands.py::TestRedisCommands::test_config_set",
"tests/test_commands.py::TestRedisCommands::test_config_set_multi_params",
"tests/test_commands.py::TestRedisCommands::test_failover",
"tests/test_commands.py::TestRedisCommands::test_dbsize",
"tests/test_commands.py::TestRedisCommands::test_echo",
"tests/test_commands.py::TestRedisCommands::test_info",
"tests/test_commands.py::TestRedisCommands::test_info_multi_sections",
"tests/test_commands.py::TestRedisCommands::test_lastsave",
"tests/test_commands.py::TestRedisCommands::test_lolwut",
"tests/test_commands.py::TestRedisCommands::test_reset",
"tests/test_commands.py::TestRedisCommands::test_object",
"tests/test_commands.py::TestRedisCommands::test_ping",
"tests/test_commands.py::TestRedisCommands::test_quit",
"tests/test_commands.py::TestRedisCommands::test_role",
"tests/test_commands.py::TestRedisCommands::test_select",
"tests/test_commands.py::TestRedisCommands::test_slowlog_get",
"tests/test_commands.py::TestRedisCommands::test_slowlog_get_limit",
"tests/test_commands.py::TestRedisCommands::test_slowlog_length",
"tests/test_commands.py::TestRedisCommands::test_time",
"tests/test_commands.py::TestRedisCommands::test_bgsave",
"tests/test_commands.py::TestRedisCommands::test_append",
"tests/test_commands.py::TestRedisCommands::test_bitcount",
"tests/test_commands.py::TestRedisCommands::test_bitcount_mode",
"tests/test_commands.py::TestRedisCommands::test_bitop_not_empty_string",
"tests/test_commands.py::TestRedisCommands::test_bitop_not",
"tests/test_commands.py::TestRedisCommands::test_bitop_not_in_place",
"tests/test_commands.py::TestRedisCommands::test_bitop_single_string",
"tests/test_commands.py::TestRedisCommands::test_bitop_string_operands",
"tests/test_commands.py::TestRedisCommands::test_bitpos",
"tests/test_commands.py::TestRedisCommands::test_bitpos_wrong_arguments",
"tests/test_commands.py::TestRedisCommands::test_bitpos_mode",
"tests/test_commands.py::TestRedisCommands::test_copy",
"tests/test_commands.py::TestRedisCommands::test_copy_and_replace",
"tests/test_commands.py::TestRedisCommands::test_copy_to_another_database",
"tests/test_commands.py::TestRedisCommands::test_decr",
"tests/test_commands.py::TestRedisCommands::test_decrby",
"tests/test_commands.py::TestRedisCommands::test_delete",
"tests/test_commands.py::TestRedisCommands::test_delete_with_multiple_keys",
"tests/test_commands.py::TestRedisCommands::test_delitem",
"tests/test_commands.py::TestRedisCommands::test_unlink",
"tests/test_commands.py::TestRedisCommands::test_unlink_with_multiple_keys",
"tests/test_commands.py::TestRedisCommands::test_lcs",
"tests/test_commands.py::TestRedisCommands::test_dump_and_restore",
"tests/test_commands.py::TestRedisCommands::test_dump_and_restore_and_replace",
"tests/test_commands.py::TestRedisCommands::test_dump_and_restore_absttl",
"tests/test_commands.py::TestRedisCommands::test_exists",
"tests/test_commands.py::TestRedisCommands::test_exists_contains",
"tests/test_commands.py::TestRedisCommands::test_expire",
"tests/test_commands.py::TestRedisCommands::test_expire_option_nx",
"tests/test_commands.py::TestRedisCommands::test_expire_option_xx",
"tests/test_commands.py::TestRedisCommands::test_expire_option_gt",
"tests/test_commands.py::TestRedisCommands::test_expire_option_lt",
"tests/test_commands.py::TestRedisCommands::test_expireat_datetime",
"tests/test_commands.py::TestRedisCommands::test_expireat_no_key",
"tests/test_commands.py::TestRedisCommands::test_expireat_unixtime",
"tests/test_commands.py::TestRedisCommands::test_expiretime",
"tests/test_commands.py::TestRedisCommands::test_expireat_option_nx",
"tests/test_commands.py::TestRedisCommands::test_expireat_option_xx",
"tests/test_commands.py::TestRedisCommands::test_expireat_option_gt",
"tests/test_commands.py::TestRedisCommands::test_expireat_option_lt",
"tests/test_commands.py::TestRedisCommands::test_get_and_set",
"tests/test_commands.py::TestRedisCommands::test_getdel",
"tests/test_commands.py::TestRedisCommands::test_getex",
"tests/test_commands.py::TestRedisCommands::test_getitem_and_setitem",
"tests/test_commands.py::TestRedisCommands::test_getitem_raises_keyerror_for_missing_key",
"tests/test_commands.py::TestRedisCommands::test_getitem_does_not_raise_keyerror_for_empty_string",
"tests/test_commands.py::TestRedisCommands::test_get_set_bit",
"tests/test_commands.py::TestRedisCommands::test_getrange",
"tests/test_commands.py::TestRedisCommands::test_getset",
"tests/test_commands.py::TestRedisCommands::test_incr",
"tests/test_commands.py::TestRedisCommands::test_incrby",
"tests/test_commands.py::TestRedisCommands::test_incrbyfloat",
"tests/test_commands.py::TestRedisCommands::test_keys",
"tests/test_commands.py::TestRedisCommands::test_mget",
"tests/test_commands.py::TestRedisCommands::test_lmove",
"tests/test_commands.py::TestRedisCommands::test_blmove",
"tests/test_commands.py::TestRedisCommands::test_mset",
"tests/test_commands.py::TestRedisCommands::test_msetnx",
"tests/test_commands.py::TestRedisCommands::test_pexpire",
"tests/test_commands.py::TestRedisCommands::test_pexpire_option_nx",
"tests/test_commands.py::TestRedisCommands::test_pexpire_option_xx",
"tests/test_commands.py::TestRedisCommands::test_pexpire_option_gt",
"tests/test_commands.py::TestRedisCommands::test_pexpire_option_lt",
"tests/test_commands.py::TestRedisCommands::test_pexpireat_datetime",
"tests/test_commands.py::TestRedisCommands::test_pexpireat_no_key",
"tests/test_commands.py::TestRedisCommands::test_pexpireat_unixtime",
"tests/test_commands.py::TestRedisCommands::test_pexpireat_option_nx",
"tests/test_commands.py::TestRedisCommands::test_pexpireat_option_xx",
"tests/test_commands.py::TestRedisCommands::test_pexpireat_option_gt",
"tests/test_commands.py::TestRedisCommands::test_pexpireat_option_lt",
"tests/test_commands.py::TestRedisCommands::test_pexpiretime",
"tests/test_commands.py::TestRedisCommands::test_psetex",
"tests/test_commands.py::TestRedisCommands::test_psetex_timedelta",
"tests/test_commands.py::TestRedisCommands::test_pttl",
"tests/test_commands.py::TestRedisCommands::test_pttl_no_key",
"tests/test_commands.py::TestRedisCommands::test_hrandfield",
"tests/test_commands.py::TestRedisCommands::test_randomkey",
"tests/test_commands.py::TestRedisCommands::test_rename",
"tests/test_commands.py::TestRedisCommands::test_renamenx",
"tests/test_commands.py::TestRedisCommands::test_set_nx",
"tests/test_commands.py::TestRedisCommands::test_set_xx",
"tests/test_commands.py::TestRedisCommands::test_set_px",
"tests/test_commands.py::TestRedisCommands::test_set_px_timedelta",
"tests/test_commands.py::TestRedisCommands::test_set_ex",
"tests/test_commands.py::TestRedisCommands::test_set_ex_timedelta",
"tests/test_commands.py::TestRedisCommands::test_set_exat_timedelta",
"tests/test_commands.py::TestRedisCommands::test_set_pxat_timedelta",
"tests/test_commands.py::TestRedisCommands::test_set_multipleoptions",
"tests/test_commands.py::TestRedisCommands::test_set_keepttl",
"tests/test_commands.py::TestRedisCommands::test_set_get",
"tests/test_commands.py::TestRedisCommands::test_setex",
"tests/test_commands.py::TestRedisCommands::test_setnx",
"tests/test_commands.py::TestRedisCommands::test_setrange",
"tests/test_commands.py::TestRedisCommands::test_strlen",
"tests/test_commands.py::TestRedisCommands::test_substr",
"tests/test_commands.py::TestRedisCommands::test_ttl",
"tests/test_commands.py::TestRedisCommands::test_ttl_nokey",
"tests/test_commands.py::TestRedisCommands::test_type",
"tests/test_commands.py::TestRedisCommands::test_blpop",
"tests/test_commands.py::TestRedisCommands::test_brpop",
"tests/test_commands.py::TestRedisCommands::test_brpoplpush",
"tests/test_commands.py::TestRedisCommands::test_brpoplpush_empty_string",
"tests/test_commands.py::TestRedisCommands::test_blmpop",
"tests/test_commands.py::TestRedisCommands::test_lmpop",
"tests/test_commands.py::TestRedisCommands::test_lindex",
"tests/test_commands.py::TestRedisCommands::test_linsert",
"tests/test_commands.py::TestRedisCommands::test_llen",
"tests/test_commands.py::TestRedisCommands::test_lpop",
"tests/test_commands.py::TestRedisCommands::test_lpop_count",
"tests/test_commands.py::TestRedisCommands::test_lpush",
"tests/test_commands.py::TestRedisCommands::test_lpushx",
"tests/test_commands.py::TestRedisCommands::test_lpushx_with_list",
"tests/test_commands.py::TestRedisCommands::test_lrange",
"tests/test_commands.py::TestRedisCommands::test_lrem",
"tests/test_commands.py::TestRedisCommands::test_lset",
"tests/test_commands.py::TestRedisCommands::test_ltrim",
"tests/test_commands.py::TestRedisCommands::test_rpop",
"tests/test_commands.py::TestRedisCommands::test_rpop_count",
"tests/test_commands.py::TestRedisCommands::test_rpoplpush",
"tests/test_commands.py::TestRedisCommands::test_rpush",
"tests/test_commands.py::TestRedisCommands::test_lpos",
"tests/test_commands.py::TestRedisCommands::test_rpushx",
"tests/test_commands.py::TestRedisCommands::test_scan",
"tests/test_commands.py::TestRedisCommands::test_scan_type",
"tests/test_commands.py::TestRedisCommands::test_scan_iter",
"tests/test_commands.py::TestRedisCommands::test_sscan",
"tests/test_commands.py::TestRedisCommands::test_sscan_iter",
"tests/test_commands.py::TestRedisCommands::test_hscan",
"tests/test_commands.py::TestRedisCommands::test_hscan_iter",
"tests/test_commands.py::TestRedisCommands::test_zscan",
"tests/test_commands.py::TestRedisCommands::test_zscan_iter",
"tests/test_commands.py::TestRedisCommands::test_sadd",
"tests/test_commands.py::TestRedisCommands::test_scard",
"tests/test_commands.py::TestRedisCommands::test_sdiff",
"tests/test_commands.py::TestRedisCommands::test_sdiffstore",
"tests/test_commands.py::TestRedisCommands::test_sinter",
"tests/test_commands.py::TestRedisCommands::test_sintercard",
"tests/test_commands.py::TestRedisCommands::test_sinterstore",
"tests/test_commands.py::TestRedisCommands::test_sismember",
"tests/test_commands.py::TestRedisCommands::test_smembers",
"tests/test_commands.py::TestRedisCommands::test_smismember",
"tests/test_commands.py::TestRedisCommands::test_smove",
"tests/test_commands.py::TestRedisCommands::test_spop",
"tests/test_commands.py::TestRedisCommands::test_spop_multi_value",
"tests/test_commands.py::TestRedisCommands::test_srandmember",
"tests/test_commands.py::TestRedisCommands::test_srandmember_multi_value",
"tests/test_commands.py::TestRedisCommands::test_srem",
"tests/test_commands.py::TestRedisCommands::test_sunion",
"tests/test_commands.py::TestRedisCommands::test_sunionstore",
"tests/test_commands.py::TestRedisCommands::test_debug_segfault",
"tests/test_commands.py::TestRedisCommands::test_script_debug",
"tests/test_commands.py::TestRedisCommands::test_zadd",
"tests/test_commands.py::TestRedisCommands::test_zadd_nx",
"tests/test_commands.py::TestRedisCommands::test_zadd_xx",
"tests/test_commands.py::TestRedisCommands::test_zadd_ch",
"tests/test_commands.py::TestRedisCommands::test_zadd_incr",
"tests/test_commands.py::TestRedisCommands::test_zadd_incr_with_xx",
"tests/test_commands.py::TestRedisCommands::test_zadd_gt_lt",
"tests/test_commands.py::TestRedisCommands::test_zcard",
"tests/test_commands.py::TestRedisCommands::test_zcount",
"tests/test_commands.py::TestRedisCommands::test_zdiff",
"tests/test_commands.py::TestRedisCommands::test_zdiffstore",
"tests/test_commands.py::TestRedisCommands::test_zincrby",
"tests/test_commands.py::TestRedisCommands::test_zlexcount",
"tests/test_commands.py::TestRedisCommands::test_zinter",
"tests/test_commands.py::TestRedisCommands::test_zintercard",
"tests/test_commands.py::TestRedisCommands::test_zinterstore_sum",
"tests/test_commands.py::TestRedisCommands::test_zinterstore_max",
"tests/test_commands.py::TestRedisCommands::test_zinterstore_min",
"tests/test_commands.py::TestRedisCommands::test_zinterstore_with_weight",
"tests/test_commands.py::TestRedisCommands::test_zpopmax",
"tests/test_commands.py::TestRedisCommands::test_zpopmin",
"tests/test_commands.py::TestRedisCommands::test_zrandemember",
"tests/test_commands.py::TestRedisCommands::test_bzpopmax",
"tests/test_commands.py::TestRedisCommands::test_bzpopmin",
"tests/test_commands.py::TestRedisCommands::test_zmpop",
"tests/test_commands.py::TestRedisCommands::test_bzmpop",
"tests/test_commands.py::TestRedisCommands::test_zrange",
"tests/test_commands.py::TestRedisCommands::test_zrange_errors",
"tests/test_commands.py::TestRedisCommands::test_zrange_params",
"tests/test_commands.py::TestRedisCommands::test_zrangestore",
"tests/test_commands.py::TestRedisCommands::test_zrangebylex",
"tests/test_commands.py::TestRedisCommands::test_zrevrangebylex",
"tests/test_commands.py::TestRedisCommands::test_zrangebyscore",
"tests/test_commands.py::TestRedisCommands::test_zrank",
"tests/test_commands.py::TestRedisCommands::test_zrem",
"tests/test_commands.py::TestRedisCommands::test_zrem_multiple_keys",
"tests/test_commands.py::TestRedisCommands::test_zremrangebylex",
"tests/test_commands.py::TestRedisCommands::test_zremrangebyrank",
"tests/test_commands.py::TestRedisCommands::test_zremrangebyscore",
"tests/test_commands.py::TestRedisCommands::test_zrevrange",
"tests/test_commands.py::TestRedisCommands::test_zrevrangebyscore",
"tests/test_commands.py::TestRedisCommands::test_zrevrank",
"tests/test_commands.py::TestRedisCommands::test_zscore",
"tests/test_commands.py::TestRedisCommands::test_zunion",
"tests/test_commands.py::TestRedisCommands::test_zunionstore_sum",
"tests/test_commands.py::TestRedisCommands::test_zunionstore_max",
"tests/test_commands.py::TestRedisCommands::test_zunionstore_min",
"tests/test_commands.py::TestRedisCommands::test_zunionstore_with_weight",
"tests/test_commands.py::TestRedisCommands::test_zmscore",
"tests/test_commands.py::TestRedisCommands::test_pfadd",
"tests/test_commands.py::TestRedisCommands::test_pfcount",
"tests/test_commands.py::TestRedisCommands::test_pfmerge",
"tests/test_commands.py::TestRedisCommands::test_hget_and_hset",
"tests/test_commands.py::TestRedisCommands::test_hset_with_multi_key_values",
"tests/test_commands.py::TestRedisCommands::test_hset_with_key_values_passed_as_list",
"tests/test_commands.py::TestRedisCommands::test_hset_without_data",
"tests/test_commands.py::TestRedisCommands::test_hdel",
"tests/test_commands.py::TestRedisCommands::test_hexists",
"tests/test_commands.py::TestRedisCommands::test_hgetall",
"tests/test_commands.py::TestRedisCommands::test_hincrby",
"tests/test_commands.py::TestRedisCommands::test_hincrbyfloat",
"tests/test_commands.py::TestRedisCommands::test_hkeys",
"tests/test_commands.py::TestRedisCommands::test_hlen",
"tests/test_commands.py::TestRedisCommands::test_hmget",
"tests/test_commands.py::TestRedisCommands::test_hmset",
"tests/test_commands.py::TestRedisCommands::test_hsetnx",
"tests/test_commands.py::TestRedisCommands::test_hvals",
"tests/test_commands.py::TestRedisCommands::test_hstrlen",
"tests/test_commands.py::TestRedisCommands::test_sort_basic",
"tests/test_commands.py::TestRedisCommands::test_sort_limited",
"tests/test_commands.py::TestRedisCommands::test_sort_by",
"tests/test_commands.py::TestRedisCommands::test_sort_get",
"tests/test_commands.py::TestRedisCommands::test_sort_get_multi",
"tests/test_commands.py::TestRedisCommands::test_sort_get_groups_two",
"tests/test_commands.py::TestRedisCommands::test_sort_groups_string_get",
"tests/test_commands.py::TestRedisCommands::test_sort_groups_just_one_get",
"tests/test_commands.py::TestRedisCommands::test_sort_groups_no_get",
"tests/test_commands.py::TestRedisCommands::test_sort_groups_three_gets",
"tests/test_commands.py::TestRedisCommands::test_sort_desc",
"tests/test_commands.py::TestRedisCommands::test_sort_alpha",
"tests/test_commands.py::TestRedisCommands::test_sort_store",
"tests/test_commands.py::TestRedisCommands::test_sort_all_options",
"tests/test_commands.py::TestRedisCommands::test_sort_ro",
"tests/test_commands.py::TestRedisCommands::test_sort_issue_924",
"tests/test_commands.py::TestRedisCommands::test_cluster_addslots",
"tests/test_commands.py::TestRedisCommands::test_cluster_count_failure_reports",
"tests/test_commands.py::TestRedisCommands::test_cluster_countkeysinslot",
"tests/test_commands.py::TestRedisCommands::test_cluster_delslots",
"tests/test_commands.py::TestRedisCommands::test_cluster_failover",
"tests/test_commands.py::TestRedisCommands::test_cluster_forget",
"tests/test_commands.py::TestRedisCommands::test_cluster_info",
"tests/test_commands.py::TestRedisCommands::test_cluster_keyslot",
"tests/test_commands.py::TestRedisCommands::test_cluster_meet",
"tests/test_commands.py::TestRedisCommands::test_cluster_nodes",
"tests/test_commands.py::TestRedisCommands::test_cluster_replicate",
"tests/test_commands.py::TestRedisCommands::test_cluster_reset",
"tests/test_commands.py::TestRedisCommands::test_cluster_saveconfig",
"tests/test_commands.py::TestRedisCommands::test_cluster_setslot",
"tests/test_commands.py::TestRedisCommands::test_cluster_slaves",
"tests/test_commands.py::TestRedisCommands::test_readonly_invalid_cluster_state",
"tests/test_commands.py::TestRedisCommands::test_readonly",
"tests/test_commands.py::TestRedisCommands::test_geoadd",
"tests/test_commands.py::TestRedisCommands::test_geoadd_nx",
"tests/test_commands.py::TestRedisCommands::test_geoadd_xx",
"tests/test_commands.py::TestRedisCommands::test_geoadd_ch",
"tests/test_commands.py::TestRedisCommands::test_geoadd_invalid_params",
"tests/test_commands.py::TestRedisCommands::test_geodist",
"tests/test_commands.py::TestRedisCommands::test_geodist_units",
"tests/test_commands.py::TestRedisCommands::test_geodist_missing_one_member",
"tests/test_commands.py::TestRedisCommands::test_geodist_invalid_units",
"tests/test_commands.py::TestRedisCommands::test_geohash",
"tests/test_commands.py::TestRedisCommands::test_geopos",
"tests/test_commands.py::TestRedisCommands::test_geopos_no_value",
"tests/test_commands.py::TestRedisCommands::test_geosearch",
"tests/test_commands.py::TestRedisCommands::test_geosearch_member",
"tests/test_commands.py::TestRedisCommands::test_geosearch_sort",
"tests/test_commands.py::TestRedisCommands::test_geosearch_with",
"tests/test_commands.py::TestRedisCommands::test_geosearch_negative",
"tests/test_commands.py::TestRedisCommands::test_geosearchstore",
"tests/test_commands.py::TestRedisCommands::test_geosearchstore_dist",
"tests/test_commands.py::TestRedisCommands::test_georadius",
"tests/test_commands.py::TestRedisCommands::test_georadius_no_values",
"tests/test_commands.py::TestRedisCommands::test_georadius_units",
"tests/test_commands.py::TestRedisCommands::test_georadius_with",
"tests/test_commands.py::TestRedisCommands::test_georadius_count",
"tests/test_commands.py::TestRedisCommands::test_georadius_sort",
"tests/test_commands.py::TestRedisCommands::test_georadius_store",
"tests/test_commands.py::TestRedisCommands::test_georadius_store_dist",
"tests/test_commands.py::TestRedisCommands::test_georadiusmember",
"tests/test_commands.py::TestRedisCommands::test_georadiusmember_count",
"tests/test_commands.py::TestRedisCommands::test_xack",
"tests/test_commands.py::TestRedisCommands::test_xadd",
"tests/test_commands.py::TestRedisCommands::test_xadd_nomkstream",
"tests/test_commands.py::TestRedisCommands::test_xadd_minlen_and_limit",
"tests/test_commands.py::TestRedisCommands::test_xadd_explicit_ms",
"tests/test_commands.py::TestRedisCommands::test_xautoclaim_negative",
"tests/test_commands.py::TestRedisCommands::test_xclaim",
"tests/test_commands.py::TestRedisCommands::test_xclaim_trimmed",
"tests/test_commands.py::TestRedisCommands::test_xdel",
"tests/test_commands.py::TestRedisCommands::test_xgroup_create",
"tests/test_commands.py::TestRedisCommands::test_xgroup_create_mkstream",
"tests/test_commands.py::TestRedisCommands::test_xgroup_create_entriesread",
"tests/test_commands.py::TestRedisCommands::test_xgroup_delconsumer",
"tests/test_commands.py::TestRedisCommands::test_xgroup_createconsumer",
"tests/test_commands.py::TestRedisCommands::test_xgroup_destroy",
"tests/test_commands.py::TestRedisCommands::test_xgroup_setid",
"tests/test_commands.py::TestRedisCommands::test_xinfo_stream",
"tests/test_commands.py::TestRedisCommands::test_xinfo_stream_full",
"tests/test_commands.py::TestRedisCommands::test_xlen",
"tests/test_commands.py::TestRedisCommands::test_xpending",
"tests/test_commands.py::TestRedisCommands::test_xpending_range",
"tests/test_commands.py::TestRedisCommands::test_xpending_range_idle",
"tests/test_commands.py::TestRedisCommands::test_xpending_range_negative",
"tests/test_commands.py::TestRedisCommands::test_xrange",
"tests/test_commands.py::TestRedisCommands::test_xread",
"tests/test_commands.py::TestRedisCommands::test_xreadgroup",
"tests/test_commands.py::TestRedisCommands::test_xrevrange",
"tests/test_commands.py::TestRedisCommands::test_xtrim",
"tests/test_commands.py::TestRedisCommands::test_xtrim_minlen_and_length_args",
"tests/test_commands.py::TestRedisCommands::test_bitfield_operations",
"tests/test_commands.py::TestRedisCommands::test_memory_help",
"tests/test_commands.py::TestRedisCommands::test_memory_doctor",
"tests/test_commands.py::TestRedisCommands::test_memory_malloc_stats",
"tests/test_commands.py::TestRedisCommands::test_memory_stats",
"tests/test_commands.py::TestRedisCommands::test_memory_usage",
"tests/test_commands.py::TestRedisCommands::test_latency_histogram_not_implemented",
"tests/test_commands.py::TestRedisCommands::test_module_list",
"tests/test_commands.py::TestRedisCommands::test_command_count",
"tests/test_commands.py::TestRedisCommands::test_command_docs",
"tests/test_commands.py::TestRedisCommands::test_command_list",
"tests/test_commands.py::TestRedisCommands::test_command_getkeys",
"tests/test_commands.py::TestRedisCommands::test_command",
"tests/test_commands.py::TestRedisCommands::test_command_getkeysandflags",
"tests/test_commands.py::TestRedisCommands::test_module",
"tests/test_commands.py::TestRedisCommands::test_module_loadex",
"tests/test_commands.py::TestRedisCommands::test_restore",
"tests/test_commands.py::TestRedisCommands::test_restore_idletime",
"tests/test_commands.py::TestRedisCommands::test_restore_frequency",
"tests/test_commands.py::TestRedisCommands::test_replicaof",
"tests/test_commands.py::TestRedisCommands::test_shutdown",
"tests/test_commands.py::TestRedisCommands::test_shutdown_with_params",
"tests/test_commands.py::TestRedisCommands::test_sync",
"tests/test_commands.py::TestRedisCommands::test_psync",
"tests/test_commands.py::TestBinarySave::test_binary_get_set",
"tests/test_commands.py::TestBinarySave::test_binary_lists",
"tests/test_commands.py::TestBinarySave::test_22_info",
"tests/test_commands.py::TestBinarySave::test_large_responses",
"tests/test_commands.py::TestBinarySave::test_floating_point_encoding",
"tests/test_connection_pool.py::TestConnectionPool::test_connection_creation",
"tests/test_connection_pool.py::TestConnectionPool::test_multiple_connections",
"tests/test_connection_pool.py::TestConnectionPool::test_max_connections",
"tests/test_connection_pool.py::TestConnectionPool::test_reuse_previously_released_connection",
"tests/test_connection_pool.py::TestConnectionPool::test_repr_contains_db_info_tcp",
"tests/test_connection_pool.py::TestConnectionPool::test_repr_contains_db_info_unix",
"tests/test_connection_pool.py::TestBlockingConnectionPool::test_connection_creation",
"tests/test_connection_pool.py::TestBlockingConnectionPool::test_multiple_connections",
"tests/test_connection_pool.py::TestBlockingConnectionPool::test_connection_pool_blocks_until_timeout",
"tests/test_connection_pool.py::TestBlockingConnectionPool::test_connection_pool_blocks_until_conn_available",
"tests/test_connection_pool.py::TestBlockingConnectionPool::test_reuse_previously_released_connection",
"tests/test_connection_pool.py::TestBlockingConnectionPool::test_repr_contains_db_info_tcp",
"tests/test_connection_pool.py::TestBlockingConnectionPool::test_repr_contains_db_info_unix",
"tests/test_connection_pool.py::TestConnectionPoolURLParsing::test_hostname",
"tests/test_connection_pool.py::TestConnectionPoolURLParsing::test_quoted_hostname",
"tests/test_connection_pool.py::TestConnectionPoolURLParsing::test_port",
"tests/test_connection_pool.py::TestConnectionPoolURLParsing::test_username",
"tests/test_connection_pool.py::TestConnectionPoolURLParsing::test_quoted_username",
"tests/test_connection_pool.py::TestConnectionPoolURLParsing::test_password",
"tests/test_connection_pool.py::TestConnectionPoolURLParsing::test_quoted_password",
"tests/test_connection_pool.py::TestConnectionPoolURLParsing::test_username_and_password",
"tests/test_connection_pool.py::TestConnectionPoolURLParsing::test_db_as_argument",
"tests/test_connection_pool.py::TestConnectionPoolURLParsing::test_db_in_path",
"tests/test_connection_pool.py::TestConnectionPoolURLParsing::test_db_in_querystring",
"tests/test_connection_pool.py::TestConnectionPoolURLParsing::test_extra_typed_querystring_options",
"tests/test_connection_pool.py::TestConnectionPoolURLParsing::test_boolean_parsing",
"tests/test_connection_pool.py::TestConnectionPoolURLParsing::test_client_name_in_querystring",
"tests/test_connection_pool.py::TestConnectionPoolURLParsing::test_invalid_extra_typed_querystring_options",
"tests/test_connection_pool.py::TestConnectionPoolURLParsing::test_extra_querystring_options",
"tests/test_connection_pool.py::TestConnectionPoolURLParsing::test_calling_from_subclass_returns_correct_instance",
"tests/test_connection_pool.py::TestConnectionPoolURLParsing::test_client_creates_connection_pool",
"tests/test_connection_pool.py::TestConnectionPoolURLParsing::test_invalid_scheme_raises_error",
"tests/test_connection_pool.py::TestConnectionPoolUnixSocketURLParsing::test_defaults",
"tests/test_connection_pool.py::TestConnectionPoolUnixSocketURLParsing::test_username",
"tests/test_connection_pool.py::TestConnectionPoolUnixSocketURLParsing::test_quoted_username",
"tests/test_connection_pool.py::TestConnectionPoolUnixSocketURLParsing::test_password",
"tests/test_connection_pool.py::TestConnectionPoolUnixSocketURLParsing::test_quoted_password",
"tests/test_connection_pool.py::TestConnectionPoolUnixSocketURLParsing::test_quoted_path",
"tests/test_connection_pool.py::TestConnectionPoolUnixSocketURLParsing::test_db_as_argument",
"tests/test_connection_pool.py::TestConnectionPoolUnixSocketURLParsing::test_db_in_querystring",
"tests/test_connection_pool.py::TestConnectionPoolUnixSocketURLParsing::test_client_name_in_querystring",
"tests/test_connection_pool.py::TestConnectionPoolUnixSocketURLParsing::test_extra_querystring_options",
"tests/test_connection_pool.py::TestConnectionPoolUnixSocketURLParsing::test_connection_class_override",
"tests/test_connection_pool.py::TestSSLConnectionURLParsing::test_host",
"tests/test_connection_pool.py::TestSSLConnectionURLParsing::test_connection_class_override",
"tests/test_connection_pool.py::TestSSLConnectionURLParsing::test_cert_reqs_options",
"tests/test_connection_pool.py::TestConnection::test_on_connect_error",
"tests/test_connection_pool.py::TestConnection::test_busy_loading_disconnects_socket",
"tests/test_connection_pool.py::TestConnection::test_busy_loading_from_pipeline_immediate_command",
"tests/test_connection_pool.py::TestConnection::test_busy_loading_from_pipeline",
"tests/test_connection_pool.py::TestConnection::test_read_only_error",
"tests/test_connection_pool.py::TestConnection::test_connect_from_url_tcp",
"tests/test_connection_pool.py::TestConnection::test_connect_from_url_unix",
"tests/test_connection_pool.py::TestMultiConnectionClient::test_multi_connection_command",
"tests/test_connection_pool.py::TestHealthCheck::test_health_check_runs",
"tests/test_connection_pool.py::TestHealthCheck::test_arbitrary_command_invokes_health_check",
"tests/test_connection_pool.py::TestHealthCheck::test_arbitrary_command_advances_next_health_check",
"tests/test_connection_pool.py::TestHealthCheck::test_health_check_not_invoked_within_interval",
"tests/test_connection_pool.py::TestHealthCheck::test_health_check_in_pipeline",
"tests/test_connection_pool.py::TestHealthCheck::test_health_check_in_transaction",
"tests/test_connection_pool.py::TestHealthCheck::test_health_check_in_watched_pipeline",
"tests/test_connection_pool.py::TestHealthCheck::test_health_check_in_pubsub_before_subscribe",
"tests/test_connection_pool.py::TestHealthCheck::test_health_check_in_pubsub_after_subscribed",
"tests/test_connection_pool.py::TestHealthCheck::test_health_check_in_pubsub_poll"
] | [
"tests/test_commands.py::TestRedisCommands::test_auth",
"tests/test_connection_pool.py::TestConnection::test_connect_no_auth_configured",
"tests/test_connection_pool.py::TestConnection::test_connect_invalid_auth_credentials_supplied"
] | Python | [] | [] |
|
redis/redis-py | 2,340 | redis__redis-py-2340 | [
"1966"
] | 771109e74feb3b8f630c047bf4f93da2800ec22e | diff --git a/redis/commands/core.py b/redis/commands/core.py
--- a/redis/commands/core.py
+++ b/redis/commands/core.py
@@ -1504,6 +1504,29 @@ def bitfield(
"""
return BitFieldOperation(self, key, default_overflow=default_overflow)
+ def bitfield_ro(
+ self: Union["Redis", "AsyncRedis"],
+ key: KeyT,
+ encoding: str,
+ offset: BitfieldOffsetT,
+ items: Optional[list] = None,
+ ) -> ResponseT:
+ """
+ Return an array of the specified bitfield values
+ where the first value is found using ``encoding`` and ``offset``
+ parameters and remaining values are result of corresponding
+ encoding/offset pairs in optional list ``items``
+ Read-only variant of the BITFIELD command.
+
+ For more information see https://redis.io/commands/bitfield_ro
+ """
+ params = [key, "GET", encoding, offset]
+
+ items = items or []
+ for encoding, offset in items:
+ params.extend(["GET", encoding, offset])
+ return self.execute_command("BITFIELD_RO", *params)
+
def bitop(self, operation: str, dest: KeyT, *keys: KeyT) -> ResponseT:
"""
Perform a bitwise operation using ``operation`` between ``keys`` and
| diff --git a/tests/test_asyncio/test_commands.py b/tests/test_asyncio/test_commands.py
--- a/tests/test_asyncio/test_commands.py
+++ b/tests/test_asyncio/test_commands.py
@@ -2953,6 +2953,19 @@ async def test_bitfield_operations(self, r: redis.Redis):
)
assert resp == [0, None, 255]
+ @skip_if_server_version_lt("6.0.0")
+ async def test_bitfield_ro(self, r: redis.Redis):
+ bf = r.bitfield("a")
+ resp = await bf.set("u8", 8, 255).execute()
+ assert resp == [0]
+
+ resp = await r.bitfield_ro("a", "u8", 0)
+ assert resp == [0]
+
+ items = [("u4", 8), ("u4", 12), ("u4", 13)]
+ resp = await r.bitfield_ro("a", "u8", 0, items)
+ assert resp == [0, 15, 15, 14]
+
@skip_if_server_version_lt("4.0.0")
async def test_memory_stats(self, r: redis.Redis):
# put a key into the current db to make sure that "db.<current-db>"
diff --git a/tests/test_commands.py b/tests/test_commands.py
--- a/tests/test_commands.py
+++ b/tests/test_commands.py
@@ -4438,6 +4438,19 @@ def test_bitfield_operations(self, r):
)
assert resp == [0, None, 255]
+ @skip_if_server_version_lt("6.0.0")
+ def test_bitfield_ro(self, r: redis.Redis):
+ bf = r.bitfield("a")
+ resp = bf.set("u8", 8, 255).execute()
+ assert resp == [0]
+
+ resp = r.bitfield_ro("a", "u8", 0)
+ assert resp == [0]
+
+ items = [("u4", 8), ("u4", 12), ("u4", 13)]
+ resp = r.bitfield_ro("a", "u8", 0, items)
+ assert resp == [0, 15, 15, 14]
+
@skip_if_server_version_lt("4.0.0")
def test_memory_help(self, r):
with pytest.raises(NotImplementedError):
| Add support for missing command BITFIELD_RO
| "2022-08-16T17:13:22Z" | 4.4 | [
"tests/test_asyncio/test_commands.py::TestResponseCallbacks::test_response_callbacks[single-python-parser]",
"tests/test_asyncio/test_commands.py::TestResponseCallbacks::test_response_callbacks[pool-python-parser]",
"tests/test_asyncio/test_commands.py::TestResponseCallbacks::test_response_callbacks[single-hiredis]",
"tests/test_asyncio/test_commands.py::TestResponseCallbacks::test_response_callbacks[pool-hiredis]",
"tests/test_asyncio/test_commands.py::TestResponseCallbacks::test_case_insensitive_command_names[single-python-parser]",
"tests/test_asyncio/test_commands.py::TestResponseCallbacks::test_case_insensitive_command_names[pool-python-parser]",
"tests/test_asyncio/test_commands.py::TestResponseCallbacks::test_case_insensitive_command_names[single-hiredis]",
"tests/test_asyncio/test_commands.py::TestResponseCallbacks::test_case_insensitive_command_names[pool-hiredis]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_command_on_invalid_key_type[single-python-parser]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_command_on_invalid_key_type[pool-python-parser]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_command_on_invalid_key_type[single-hiredis]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_command_on_invalid_key_type[pool-hiredis]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_acl_cat_no_category[single-python-parser]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_acl_cat_no_category[pool-python-parser]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_acl_cat_no_category[single-hiredis]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_acl_cat_no_category[pool-hiredis]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_acl_cat_with_category[single-python-parser]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_acl_cat_with_category[pool-python-parser]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_acl_cat_with_category[single-hiredis]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_acl_cat_with_category[pool-hiredis]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_acl_deluser[single-python-parser]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_acl_deluser[pool-python-parser]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_acl_deluser[single-hiredis]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_acl_deluser[pool-hiredis]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_acl_genpass[single-python-parser]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_acl_genpass[pool-python-parser]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_acl_genpass[single-hiredis]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_acl_genpass[pool-hiredis]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_acl_log[single-python-parser]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_acl_log[pool-python-parser]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_acl_log[single-hiredis]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_acl_log[pool-hiredis]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_acl_setuser_categories_without_prefix_fails[single-python-parser]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_acl_setuser_categories_without_prefix_fails[pool-python-parser]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_acl_setuser_categories_without_prefix_fails[single-hiredis]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_acl_setuser_categories_without_prefix_fails[pool-hiredis]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_acl_setuser_commands_without_prefix_fails[single-python-parser]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_acl_setuser_commands_without_prefix_fails[pool-python-parser]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_acl_setuser_commands_without_prefix_fails[single-hiredis]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_acl_setuser_commands_without_prefix_fails[pool-hiredis]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_acl_setuser_add_passwords_and_nopass_fails[single-python-parser]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_acl_setuser_add_passwords_and_nopass_fails[pool-python-parser]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_acl_setuser_add_passwords_and_nopass_fails[single-hiredis]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_acl_setuser_add_passwords_and_nopass_fails[pool-hiredis]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_acl_users[single-python-parser]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_acl_users[pool-python-parser]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_acl_users[single-hiredis]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_acl_users[pool-hiredis]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_acl_whoami[single-python-parser]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_acl_whoami[pool-python-parser]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_acl_whoami[single-hiredis]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_acl_whoami[pool-hiredis]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_client_list[single-python-parser]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_client_list[pool-python-parser]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_client_list[single-hiredis]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_client_list[pool-hiredis]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_client_list_type[single-python-parser]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_client_list_type[pool-python-parser]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_client_list_type[single-hiredis]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_client_list_type[pool-hiredis]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_client_id[single-python-parser]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_client_id[pool-python-parser]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_client_id[single-hiredis]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_client_id[pool-hiredis]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_client_unblock[single-python-parser]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_client_unblock[pool-python-parser]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_client_unblock[single-hiredis]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_client_unblock[pool-hiredis]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_client_getname[single-python-parser]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_client_getname[pool-python-parser]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_client_getname[single-hiredis]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_client_getname[pool-hiredis]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_client_setname[single-python-parser]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_client_setname[pool-python-parser]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_client_setname[single-hiredis]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_client_setname[pool-hiredis]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_client_kill[single-python-parser]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_client_kill[pool-python-parser]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_client_kill[single-hiredis]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_client_kill[pool-hiredis]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_client_kill_filter_invalid_params[single-python-parser]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_client_kill_filter_invalid_params[pool-python-parser]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_client_kill_filter_invalid_params[single-hiredis]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_client_kill_filter_invalid_params[pool-hiredis]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_client_kill_filter_by_id[single-python-parser]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_client_kill_filter_by_id[pool-python-parser]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_client_kill_filter_by_id[single-hiredis]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_client_kill_filter_by_id[pool-hiredis]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_client_kill_filter_by_addr[single-python-parser]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_client_kill_filter_by_addr[pool-python-parser]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_client_kill_filter_by_addr[single-hiredis]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_client_kill_filter_by_addr[pool-hiredis]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_client_list_after_client_setname[single-python-parser]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_client_list_after_client_setname[pool-python-parser]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_client_list_after_client_setname[single-hiredis]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_client_list_after_client_setname[pool-hiredis]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_client_pause[single-python-parser]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_client_pause[pool-python-parser]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_client_pause[single-hiredis]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_client_pause[pool-hiredis]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_config_get[single-python-parser]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_config_get[pool-python-parser]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_config_get[single-hiredis]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_config_get[pool-hiredis]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_config_resetstat[single-python-parser]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_config_resetstat[pool-python-parser]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_config_resetstat[single-hiredis]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_config_resetstat[pool-hiredis]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_config_set[single-python-parser]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_config_set[pool-python-parser]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_config_set[single-hiredis]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_config_set[pool-hiredis]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_dbsize[single-python-parser]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_dbsize[pool-python-parser]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_dbsize[single-hiredis]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_dbsize[pool-hiredis]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_echo[single-python-parser]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_echo[pool-python-parser]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_echo[single-hiredis]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_echo[pool-hiredis]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_info[single-python-parser]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_info[pool-python-parser]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_info[single-hiredis]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_info[pool-hiredis]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_lastsave[single-python-parser]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_lastsave[pool-python-parser]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_lastsave[single-hiredis]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_lastsave[pool-hiredis]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_object[single-python-parser]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_object[pool-python-parser]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_object[single-hiredis]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_object[pool-hiredis]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_ping[single-python-parser]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_ping[pool-python-parser]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_ping[single-hiredis]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_ping[pool-hiredis]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_slowlog_get[single-python-parser]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_slowlog_get[pool-python-parser]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_slowlog_get[single-hiredis]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_slowlog_get[pool-hiredis]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_slowlog_get_limit[single-python-parser]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_slowlog_get_limit[pool-python-parser]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_slowlog_get_limit[single-hiredis]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_slowlog_get_limit[pool-hiredis]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_slowlog_length[single-python-parser]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_slowlog_length[pool-python-parser]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_slowlog_length[single-hiredis]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_slowlog_length[pool-hiredis]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_time[single-python-parser]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_time[pool-python-parser]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_time[single-hiredis]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_time[pool-hiredis]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_append[single-python-parser]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_append[pool-python-parser]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_append[single-hiredis]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_append[pool-hiredis]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_bitcount[single-python-parser]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_bitcount[pool-python-parser]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_bitcount[single-hiredis]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_bitcount[pool-hiredis]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_bitop_not_empty_string[single-python-parser]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_bitop_not_empty_string[pool-python-parser]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_bitop_not_empty_string[single-hiredis]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_bitop_not_empty_string[pool-hiredis]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_bitop_not[single-python-parser]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_bitop_not[pool-python-parser]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_bitop_not[single-hiredis]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_bitop_not[pool-hiredis]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_bitop_not_in_place[single-python-parser]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_bitop_not_in_place[pool-python-parser]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_bitop_not_in_place[single-hiredis]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_bitop_not_in_place[pool-hiredis]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_bitop_single_string[single-python-parser]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_bitop_single_string[pool-python-parser]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_bitop_single_string[single-hiredis]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_bitop_single_string[pool-hiredis]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_bitop_string_operands[single-python-parser]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_bitop_string_operands[pool-python-parser]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_bitop_string_operands[single-hiredis]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_bitop_string_operands[pool-hiredis]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_bitpos[single-python-parser]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_bitpos[pool-python-parser]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_bitpos[single-hiredis]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_bitpos[pool-hiredis]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_bitpos_wrong_arguments[single-python-parser]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_bitpos_wrong_arguments[pool-python-parser]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_bitpos_wrong_arguments[single-hiredis]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_bitpos_wrong_arguments[pool-hiredis]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_decr[single-python-parser]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_decr[pool-python-parser]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_decr[single-hiredis]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_decr[pool-hiredis]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_decrby[single-python-parser]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_decrby[pool-python-parser]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_decrby[single-hiredis]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_decrby[pool-hiredis]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_delete[single-python-parser]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_delete[pool-python-parser]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_delete[single-hiredis]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_delete[pool-hiredis]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_delete_with_multiple_keys[single-python-parser]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_delete_with_multiple_keys[pool-python-parser]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_delete_with_multiple_keys[single-hiredis]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_delete_with_multiple_keys[pool-hiredis]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_delitem[single-python-parser]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_delitem[pool-python-parser]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_delitem[single-hiredis]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_delitem[pool-hiredis]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_unlink[single-python-parser]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_unlink[pool-python-parser]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_unlink[single-hiredis]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_unlink[pool-hiredis]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_unlink_with_multiple_keys[single-python-parser]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_unlink_with_multiple_keys[pool-python-parser]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_unlink_with_multiple_keys[single-hiredis]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_unlink_with_multiple_keys[pool-hiredis]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_dump_and_restore[single-python-parser]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_dump_and_restore[pool-python-parser]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_dump_and_restore[single-hiredis]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_dump_and_restore[pool-hiredis]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_dump_and_restore_and_replace[single-python-parser]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_dump_and_restore_and_replace[pool-python-parser]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_dump_and_restore_and_replace[single-hiredis]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_dump_and_restore_and_replace[pool-hiredis]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_dump_and_restore_absttl[single-python-parser]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_dump_and_restore_absttl[pool-python-parser]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_dump_and_restore_absttl[single-hiredis]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_dump_and_restore_absttl[pool-hiredis]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_exists[single-python-parser]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_exists[pool-python-parser]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_exists[single-hiredis]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_exists[pool-hiredis]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_exists_contains[single-python-parser]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_exists_contains[pool-python-parser]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_exists_contains[single-hiredis]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_exists_contains[pool-hiredis]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_expire[single-python-parser]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_expire[pool-python-parser]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_expire[single-hiredis]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_expire[pool-hiredis]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_expireat_datetime[single-python-parser]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_expireat_datetime[pool-python-parser]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_expireat_datetime[single-hiredis]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_expireat_datetime[pool-hiredis]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_expireat_no_key[single-python-parser]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_expireat_no_key[pool-python-parser]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_expireat_no_key[single-hiredis]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_expireat_no_key[pool-hiredis]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_expireat_unixtime[single-python-parser]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_expireat_unixtime[pool-python-parser]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_expireat_unixtime[single-hiredis]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_expireat_unixtime[pool-hiredis]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_get_and_set[single-python-parser]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_get_and_set[pool-python-parser]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_get_and_set[single-hiredis]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_get_and_set[pool-hiredis]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_get_set_bit[single-python-parser]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_get_set_bit[pool-python-parser]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_get_set_bit[single-hiredis]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_get_set_bit[pool-hiredis]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_getrange[single-python-parser]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_getrange[pool-python-parser]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_getrange[single-hiredis]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_getrange[pool-hiredis]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_getset[single-python-parser]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_getset[pool-python-parser]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_getset[single-hiredis]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_getset[pool-hiredis]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_incr[single-python-parser]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_incr[pool-python-parser]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_incr[single-hiredis]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_incr[pool-hiredis]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_incrby[single-python-parser]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_incrby[pool-python-parser]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_incrby[single-hiredis]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_incrby[pool-hiredis]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_incrbyfloat[single-python-parser]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_incrbyfloat[pool-python-parser]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_incrbyfloat[single-hiredis]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_incrbyfloat[pool-hiredis]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_keys[single-python-parser]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_keys[pool-python-parser]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_keys[single-hiredis]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_keys[pool-hiredis]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_mget[single-python-parser]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_mget[pool-python-parser]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_mget[single-hiredis]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_mget[pool-hiredis]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_mset[single-python-parser]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_mset[pool-python-parser]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_mset[single-hiredis]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_mset[pool-hiredis]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_msetnx[single-python-parser]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_msetnx[pool-python-parser]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_msetnx[single-hiredis]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_msetnx[pool-hiredis]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_pexpire[single-python-parser]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_pexpire[pool-python-parser]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_pexpire[single-hiredis]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_pexpire[pool-hiredis]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_pexpireat_datetime[single-python-parser]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_pexpireat_datetime[pool-python-parser]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_pexpireat_datetime[single-hiredis]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_pexpireat_datetime[pool-hiredis]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_pexpireat_no_key[single-python-parser]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_pexpireat_no_key[pool-python-parser]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_pexpireat_no_key[single-hiredis]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_pexpireat_no_key[pool-hiredis]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_pexpireat_unixtime[single-python-parser]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_pexpireat_unixtime[pool-python-parser]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_pexpireat_unixtime[single-hiredis]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_pexpireat_unixtime[pool-hiredis]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_psetex[single-python-parser]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_psetex[pool-python-parser]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_psetex[single-hiredis]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_psetex[pool-hiredis]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_psetex_timedelta[single-python-parser]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_psetex_timedelta[pool-python-parser]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_psetex_timedelta[single-hiredis]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_psetex_timedelta[pool-hiredis]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_pttl[single-python-parser]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_pttl[pool-python-parser]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_pttl[single-hiredis]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_pttl[pool-hiredis]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_pttl_no_key[single-python-parser]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_pttl_no_key[pool-python-parser]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_pttl_no_key[single-hiredis]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_pttl_no_key[pool-hiredis]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_randomkey[single-python-parser]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_randomkey[pool-python-parser]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_randomkey[single-hiredis]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_randomkey[pool-hiredis]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_rename[single-python-parser]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_rename[pool-python-parser]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_rename[single-hiredis]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_rename[pool-hiredis]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_renamenx[single-python-parser]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_renamenx[pool-python-parser]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_renamenx[single-hiredis]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_renamenx[pool-hiredis]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_set_nx[single-python-parser]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_set_nx[pool-python-parser]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_set_nx[single-hiredis]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_set_nx[pool-hiredis]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_set_xx[single-python-parser]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_set_xx[pool-python-parser]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_set_xx[single-hiredis]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_set_xx[pool-hiredis]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_set_px[single-python-parser]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_set_px[pool-python-parser]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_set_px[single-hiredis]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_set_px[pool-hiredis]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_set_px_timedelta[single-python-parser]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_set_px_timedelta[pool-python-parser]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_set_px_timedelta[single-hiredis]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_set_px_timedelta[pool-hiredis]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_set_ex[single-python-parser]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_set_ex[pool-python-parser]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_set_ex[single-hiredis]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_set_ex[pool-hiredis]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_set_ex_timedelta[single-python-parser]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_set_ex_timedelta[pool-python-parser]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_set_ex_timedelta[single-hiredis]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_set_ex_timedelta[pool-hiredis]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_set_multipleoptions[single-python-parser]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_set_multipleoptions[pool-python-parser]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_set_multipleoptions[single-hiredis]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_set_multipleoptions[pool-hiredis]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_set_keepttl[single-python-parser]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_set_keepttl[pool-python-parser]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_set_keepttl[single-hiredis]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_set_keepttl[pool-hiredis]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_setex[single-python-parser]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_setex[pool-python-parser]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_setex[single-hiredis]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_setex[pool-hiredis]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_setnx[single-python-parser]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_setnx[pool-python-parser]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_setnx[single-hiredis]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_setnx[pool-hiredis]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_setrange[single-python-parser]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_setrange[pool-python-parser]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_setrange[single-hiredis]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_setrange[pool-hiredis]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_strlen[single-python-parser]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_strlen[pool-python-parser]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_strlen[single-hiredis]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_strlen[pool-hiredis]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_substr[single-python-parser]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_substr[pool-python-parser]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_substr[single-hiredis]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_substr[pool-hiredis]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_ttl[single-python-parser]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_ttl[pool-python-parser]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_ttl[single-hiredis]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_ttl[pool-hiredis]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_ttl_nokey[single-python-parser]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_ttl_nokey[pool-python-parser]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_ttl_nokey[single-hiredis]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_ttl_nokey[pool-hiredis]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_type[single-python-parser]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_type[pool-python-parser]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_type[single-hiredis]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_type[pool-hiredis]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_blpop[single-python-parser]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_blpop[pool-python-parser]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_blpop[single-hiredis]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_blpop[pool-hiredis]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_brpop[single-python-parser]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_brpop[pool-python-parser]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_brpop[single-hiredis]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_brpop[pool-hiredis]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_brpoplpush[single-python-parser]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_brpoplpush[pool-python-parser]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_brpoplpush[single-hiredis]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_brpoplpush[pool-hiredis]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_brpoplpush_empty_string[single-python-parser]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_brpoplpush_empty_string[pool-python-parser]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_brpoplpush_empty_string[single-hiredis]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_brpoplpush_empty_string[pool-hiredis]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_lindex[single-python-parser]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_lindex[pool-python-parser]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_lindex[single-hiredis]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_lindex[pool-hiredis]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_linsert[single-python-parser]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_linsert[pool-python-parser]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_linsert[single-hiredis]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_linsert[pool-hiredis]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_llen[single-python-parser]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_llen[pool-python-parser]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_llen[single-hiredis]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_llen[pool-hiredis]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_lpop[single-python-parser]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_lpop[pool-python-parser]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_lpop[single-hiredis]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_lpop[pool-hiredis]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_lpush[single-python-parser]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_lpush[pool-python-parser]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_lpush[single-hiredis]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_lpush[pool-hiredis]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_lpushx[single-python-parser]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_lpushx[pool-python-parser]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_lpushx[single-hiredis]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_lpushx[pool-hiredis]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_lrange[single-python-parser]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_lrange[pool-python-parser]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_lrange[single-hiredis]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_lrange[pool-hiredis]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_lrem[single-python-parser]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_lrem[pool-python-parser]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_lrem[single-hiredis]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_lrem[pool-hiredis]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_lset[single-python-parser]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_lset[pool-python-parser]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_lset[single-hiredis]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_lset[pool-hiredis]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_ltrim[single-python-parser]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_ltrim[pool-python-parser]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_ltrim[single-hiredis]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_ltrim[pool-hiredis]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_rpop[single-python-parser]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_rpop[pool-python-parser]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_rpop[single-hiredis]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_rpop[pool-hiredis]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_rpoplpush[single-python-parser]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_rpoplpush[pool-python-parser]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_rpoplpush[single-hiredis]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_rpoplpush[pool-hiredis]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_rpush[single-python-parser]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_rpush[pool-python-parser]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_rpush[single-hiredis]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_rpush[pool-hiredis]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_lpos[single-python-parser]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_lpos[pool-python-parser]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_lpos[single-hiredis]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_lpos[pool-hiredis]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_rpushx[single-python-parser]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_rpushx[pool-python-parser]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_rpushx[single-hiredis]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_rpushx[pool-hiredis]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_scan[single-python-parser]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_scan[pool-python-parser]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_scan[single-hiredis]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_scan[pool-hiredis]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_scan_type[single-python-parser]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_scan_type[pool-python-parser]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_scan_type[single-hiredis]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_scan_type[pool-hiredis]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_scan_iter[single-python-parser]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_scan_iter[pool-python-parser]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_scan_iter[single-hiredis]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_scan_iter[pool-hiredis]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_sscan[single-python-parser]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_sscan[pool-python-parser]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_sscan[single-hiredis]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_sscan[pool-hiredis]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_sscan_iter[single-python-parser]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_sscan_iter[pool-python-parser]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_sscan_iter[single-hiredis]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_sscan_iter[pool-hiredis]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_hscan[single-python-parser]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_hscan[pool-python-parser]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_hscan[single-hiredis]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_hscan[pool-hiredis]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_hscan_iter[single-python-parser]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_hscan_iter[pool-python-parser]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_hscan_iter[single-hiredis]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_hscan_iter[pool-hiredis]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_zscan[single-python-parser]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_zscan[pool-python-parser]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_zscan[single-hiredis]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_zscan[pool-hiredis]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_zscan_iter[single-python-parser]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_zscan_iter[pool-python-parser]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_zscan_iter[single-hiredis]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_zscan_iter[pool-hiredis]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_sadd[single-python-parser]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_sadd[pool-python-parser]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_sadd[single-hiredis]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_sadd[pool-hiredis]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_scard[single-python-parser]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_scard[pool-python-parser]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_scard[single-hiredis]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_scard[pool-hiredis]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_sdiff[single-python-parser]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_sdiff[pool-python-parser]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_sdiff[single-hiredis]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_sdiff[pool-hiredis]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_sdiffstore[single-python-parser]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_sdiffstore[pool-python-parser]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_sdiffstore[single-hiredis]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_sdiffstore[pool-hiredis]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_sinter[single-python-parser]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_sinter[pool-python-parser]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_sinter[single-hiredis]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_sinter[pool-hiredis]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_sinterstore[single-python-parser]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_sinterstore[pool-python-parser]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_sinterstore[single-hiredis]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_sinterstore[pool-hiredis]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_sismember[single-python-parser]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_sismember[pool-python-parser]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_sismember[single-hiredis]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_sismember[pool-hiredis]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_smembers[single-python-parser]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_smembers[pool-python-parser]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_smembers[single-hiredis]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_smembers[pool-hiredis]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_smove[single-python-parser]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_smove[pool-python-parser]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_smove[single-hiredis]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_smove[pool-hiredis]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_spop[single-python-parser]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_spop[pool-python-parser]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_spop[single-hiredis]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_spop[pool-hiredis]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_spop_multi_value[single-python-parser]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_spop_multi_value[pool-python-parser]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_spop_multi_value[single-hiredis]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_spop_multi_value[pool-hiredis]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_srandmember[single-python-parser]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_srandmember[pool-python-parser]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_srandmember[single-hiredis]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_srandmember[pool-hiredis]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_srandmember_multi_value[single-python-parser]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_srandmember_multi_value[pool-python-parser]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_srandmember_multi_value[single-hiredis]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_srandmember_multi_value[pool-hiredis]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_srem[single-python-parser]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_srem[pool-python-parser]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_srem[single-hiredis]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_srem[pool-hiredis]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_sunion[single-python-parser]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_sunion[pool-python-parser]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_sunion[single-hiredis]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_sunion[pool-hiredis]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_sunionstore[single-python-parser]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_sunionstore[pool-python-parser]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_sunionstore[single-hiredis]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_sunionstore[pool-hiredis]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_zadd[single-python-parser]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_zadd[pool-python-parser]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_zadd[single-hiredis]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_zadd[pool-hiredis]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_zadd_nx[single-python-parser]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_zadd_nx[pool-python-parser]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_zadd_nx[single-hiredis]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_zadd_nx[pool-hiredis]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_zadd_xx[single-python-parser]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_zadd_xx[pool-python-parser]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_zadd_xx[single-hiredis]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_zadd_xx[pool-hiredis]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_zadd_ch[single-python-parser]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_zadd_ch[pool-python-parser]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_zadd_ch[single-hiredis]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_zadd_ch[pool-hiredis]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_zadd_incr[single-python-parser]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_zadd_incr[pool-python-parser]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_zadd_incr[single-hiredis]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_zadd_incr[pool-hiredis]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_zadd_incr_with_xx[single-python-parser]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_zadd_incr_with_xx[pool-python-parser]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_zadd_incr_with_xx[single-hiredis]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_zadd_incr_with_xx[pool-hiredis]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_zcard[single-python-parser]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_zcard[pool-python-parser]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_zcard[single-hiredis]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_zcard[pool-hiredis]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_zcount[single-python-parser]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_zcount[pool-python-parser]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_zcount[single-hiredis]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_zcount[pool-hiredis]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_zincrby[single-python-parser]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_zincrby[pool-python-parser]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_zincrby[single-hiredis]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_zincrby[pool-hiredis]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_zlexcount[single-python-parser]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_zlexcount[pool-python-parser]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_zlexcount[single-hiredis]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_zlexcount[pool-hiredis]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_zinterstore_sum[single-python-parser]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_zinterstore_sum[pool-python-parser]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_zinterstore_sum[single-hiredis]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_zinterstore_sum[pool-hiredis]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_zinterstore_max[single-python-parser]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_zinterstore_max[pool-python-parser]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_zinterstore_max[single-hiredis]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_zinterstore_max[pool-hiredis]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_zinterstore_min[single-python-parser]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_zinterstore_min[pool-python-parser]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_zinterstore_min[single-hiredis]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_zinterstore_min[pool-hiredis]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_zinterstore_with_weight[single-python-parser]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_zinterstore_with_weight[pool-python-parser]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_zinterstore_with_weight[single-hiredis]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_zinterstore_with_weight[pool-hiredis]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_zpopmax[single-python-parser]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_zpopmax[pool-python-parser]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_zpopmax[single-hiredis]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_zpopmax[pool-hiredis]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_zpopmin[single-python-parser]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_zpopmin[pool-python-parser]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_zpopmin[single-hiredis]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_zpopmin[pool-hiredis]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_bzpopmax[single-python-parser]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_bzpopmax[pool-python-parser]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_bzpopmax[single-hiredis]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_bzpopmax[pool-hiredis]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_bzpopmin[single-python-parser]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_bzpopmin[pool-python-parser]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_bzpopmin[single-hiredis]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_bzpopmin[pool-hiredis]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_zrange[single-python-parser]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_zrange[pool-python-parser]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_zrange[single-hiredis]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_zrange[pool-hiredis]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_zrangebylex[single-python-parser]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_zrangebylex[pool-python-parser]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_zrangebylex[single-hiredis]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_zrangebylex[pool-hiredis]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_zrevrangebylex[single-python-parser]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_zrevrangebylex[pool-python-parser]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_zrevrangebylex[single-hiredis]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_zrevrangebylex[pool-hiredis]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_zrangebyscore[single-python-parser]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_zrangebyscore[pool-python-parser]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_zrangebyscore[single-hiredis]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_zrangebyscore[pool-hiredis]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_zrank[single-python-parser]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_zrank[pool-python-parser]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_zrank[single-hiredis]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_zrank[pool-hiredis]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_zrem[single-python-parser]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_zrem[pool-python-parser]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_zrem[single-hiredis]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_zrem[pool-hiredis]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_zrem_multiple_keys[single-python-parser]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_zrem_multiple_keys[pool-python-parser]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_zrem_multiple_keys[single-hiredis]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_zrem_multiple_keys[pool-hiredis]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_zremrangebylex[single-python-parser]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_zremrangebylex[pool-python-parser]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_zremrangebylex[single-hiredis]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_zremrangebylex[pool-hiredis]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_zremrangebyrank[single-python-parser]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_zremrangebyrank[pool-python-parser]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_zremrangebyrank[single-hiredis]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_zremrangebyrank[pool-hiredis]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_zremrangebyscore[single-python-parser]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_zremrangebyscore[pool-python-parser]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_zremrangebyscore[single-hiredis]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_zremrangebyscore[pool-hiredis]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_zrevrange[single-python-parser]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_zrevrange[pool-python-parser]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_zrevrange[single-hiredis]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_zrevrange[pool-hiredis]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_zrevrangebyscore[single-python-parser]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_zrevrangebyscore[pool-python-parser]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_zrevrangebyscore[single-hiredis]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_zrevrangebyscore[pool-hiredis]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_zrevrank[single-python-parser]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_zrevrank[pool-python-parser]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_zrevrank[single-hiredis]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_zrevrank[pool-hiredis]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_zscore[single-python-parser]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_zscore[pool-python-parser]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_zscore[single-hiredis]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_zscore[pool-hiredis]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_zunionstore_sum[single-python-parser]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_zunionstore_sum[pool-python-parser]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_zunionstore_sum[single-hiredis]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_zunionstore_sum[pool-hiredis]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_zunionstore_max[single-python-parser]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_zunionstore_max[pool-python-parser]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_zunionstore_max[single-hiredis]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_zunionstore_max[pool-hiredis]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_zunionstore_min[single-python-parser]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_zunionstore_min[pool-python-parser]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_zunionstore_min[single-hiredis]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_zunionstore_min[pool-hiredis]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_zunionstore_with_weight[single-python-parser]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_zunionstore_with_weight[pool-python-parser]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_zunionstore_with_weight[single-hiredis]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_zunionstore_with_weight[pool-hiredis]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_pfadd[single-python-parser]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_pfadd[pool-python-parser]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_pfadd[single-hiredis]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_pfadd[pool-hiredis]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_pfcount[single-python-parser]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_pfcount[pool-python-parser]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_pfcount[single-hiredis]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_pfcount[pool-hiredis]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_pfmerge[single-python-parser]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_pfmerge[pool-python-parser]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_pfmerge[single-hiredis]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_pfmerge[pool-hiredis]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_hget_and_hset[single-python-parser]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_hget_and_hset[pool-python-parser]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_hget_and_hset[single-hiredis]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_hget_and_hset[pool-hiredis]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_hset_with_multi_key_values[single-python-parser]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_hset_with_multi_key_values[pool-python-parser]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_hset_with_multi_key_values[single-hiredis]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_hset_with_multi_key_values[pool-hiredis]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_hset_without_data[single-python-parser]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_hset_without_data[pool-python-parser]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_hset_without_data[single-hiredis]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_hset_without_data[pool-hiredis]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_hdel[single-python-parser]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_hdel[pool-python-parser]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_hdel[single-hiredis]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_hdel[pool-hiredis]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_hexists[single-python-parser]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_hexists[pool-python-parser]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_hexists[single-hiredis]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_hexists[pool-hiredis]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_hgetall[single-python-parser]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_hgetall[pool-python-parser]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_hgetall[single-hiredis]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_hgetall[pool-hiredis]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_hincrby[single-python-parser]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_hincrby[pool-python-parser]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_hincrby[single-hiredis]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_hincrby[pool-hiredis]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_hincrbyfloat[single-python-parser]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_hincrbyfloat[pool-python-parser]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_hincrbyfloat[single-hiredis]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_hincrbyfloat[pool-hiredis]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_hkeys[single-python-parser]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_hkeys[pool-python-parser]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_hkeys[single-hiredis]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_hkeys[pool-hiredis]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_hlen[single-python-parser]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_hlen[pool-python-parser]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_hlen[single-hiredis]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_hlen[pool-hiredis]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_hmget[single-python-parser]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_hmget[pool-python-parser]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_hmget[single-hiredis]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_hmget[pool-hiredis]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_hmset[single-python-parser]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_hmset[pool-python-parser]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_hmset[single-hiredis]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_hmset[pool-hiredis]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_hsetnx[single-python-parser]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_hsetnx[pool-python-parser]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_hsetnx[single-hiredis]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_hsetnx[pool-hiredis]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_hvals[single-python-parser]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_hvals[pool-python-parser]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_hvals[single-hiredis]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_hvals[pool-hiredis]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_hstrlen[single-python-parser]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_hstrlen[pool-python-parser]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_hstrlen[single-hiredis]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_hstrlen[pool-hiredis]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_sort_basic[single-python-parser]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_sort_basic[pool-python-parser]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_sort_basic[single-hiredis]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_sort_basic[pool-hiredis]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_sort_limited[single-python-parser]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_sort_limited[pool-python-parser]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_sort_limited[single-hiredis]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_sort_limited[pool-hiredis]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_sort_by[single-python-parser]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_sort_by[pool-python-parser]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_sort_by[single-hiredis]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_sort_by[pool-hiredis]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_sort_get[single-python-parser]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_sort_get[pool-python-parser]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_sort_get[single-hiredis]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_sort_get[pool-hiredis]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_sort_get_multi[single-python-parser]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_sort_get_multi[pool-python-parser]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_sort_get_multi[single-hiredis]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_sort_get_multi[pool-hiredis]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_sort_get_groups_two[single-python-parser]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_sort_get_groups_two[pool-python-parser]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_sort_get_groups_two[single-hiredis]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_sort_get_groups_two[pool-hiredis]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_sort_groups_string_get[single-python-parser]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_sort_groups_string_get[pool-python-parser]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_sort_groups_string_get[single-hiredis]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_sort_groups_string_get[pool-hiredis]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_sort_groups_just_one_get[single-python-parser]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_sort_groups_just_one_get[pool-python-parser]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_sort_groups_just_one_get[single-hiredis]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_sort_groups_just_one_get[pool-hiredis]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_sort_groups_no_get[single-python-parser]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_sort_groups_no_get[pool-python-parser]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_sort_groups_no_get[single-hiredis]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_sort_groups_no_get[pool-hiredis]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_sort_groups_three_gets[single-python-parser]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_sort_groups_three_gets[pool-python-parser]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_sort_groups_three_gets[single-hiredis]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_sort_groups_three_gets[pool-hiredis]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_sort_desc[single-python-parser]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_sort_desc[pool-python-parser]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_sort_desc[single-hiredis]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_sort_desc[pool-hiredis]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_sort_alpha[single-python-parser]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_sort_alpha[pool-python-parser]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_sort_alpha[single-hiredis]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_sort_alpha[pool-hiredis]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_sort_store[single-python-parser]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_sort_store[pool-python-parser]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_sort_store[single-hiredis]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_sort_store[pool-hiredis]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_sort_all_options[single-python-parser]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_sort_all_options[pool-python-parser]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_sort_all_options[single-hiredis]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_sort_all_options[pool-hiredis]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_sort_issue_924[single-python-parser]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_sort_issue_924[pool-python-parser]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_sort_issue_924[single-hiredis]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_sort_issue_924[pool-hiredis]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_cluster_addslots[single-python-parser]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_cluster_addslots[pool-python-parser]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_cluster_addslots[single-hiredis]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_cluster_addslots[pool-hiredis]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_cluster_count_failure_reports[single-python-parser]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_cluster_count_failure_reports[pool-python-parser]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_cluster_count_failure_reports[single-hiredis]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_cluster_count_failure_reports[pool-hiredis]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_cluster_countkeysinslot[single-python-parser]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_cluster_countkeysinslot[pool-python-parser]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_cluster_countkeysinslot[single-hiredis]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_cluster_countkeysinslot[pool-hiredis]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_cluster_delslots[single-python-parser]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_cluster_delslots[pool-python-parser]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_cluster_delslots[single-hiredis]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_cluster_delslots[pool-hiredis]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_cluster_failover[single-python-parser]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_cluster_failover[pool-python-parser]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_cluster_failover[single-hiredis]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_cluster_failover[pool-hiredis]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_cluster_forget[single-python-parser]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_cluster_forget[pool-python-parser]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_cluster_forget[single-hiredis]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_cluster_forget[pool-hiredis]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_cluster_info[single-python-parser]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_cluster_info[pool-python-parser]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_cluster_info[single-hiredis]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_cluster_info[pool-hiredis]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_cluster_keyslot[single-python-parser]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_cluster_keyslot[pool-python-parser]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_cluster_keyslot[single-hiredis]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_cluster_keyslot[pool-hiredis]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_cluster_meet[single-python-parser]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_cluster_meet[pool-python-parser]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_cluster_meet[single-hiredis]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_cluster_meet[pool-hiredis]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_cluster_nodes[single-python-parser]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_cluster_nodes[pool-python-parser]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_cluster_nodes[single-hiredis]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_cluster_nodes[pool-hiredis]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_cluster_replicate[single-python-parser]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_cluster_replicate[pool-python-parser]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_cluster_replicate[single-hiredis]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_cluster_replicate[pool-hiredis]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_cluster_reset[single-python-parser]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_cluster_reset[pool-python-parser]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_cluster_reset[single-hiredis]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_cluster_reset[pool-hiredis]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_cluster_saveconfig[single-python-parser]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_cluster_saveconfig[pool-python-parser]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_cluster_saveconfig[single-hiredis]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_cluster_saveconfig[pool-hiredis]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_cluster_setslot[single-python-parser]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_cluster_setslot[pool-python-parser]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_cluster_setslot[single-hiredis]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_cluster_setslot[pool-hiredis]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_cluster_slaves[single-python-parser]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_cluster_slaves[pool-python-parser]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_cluster_slaves[single-hiredis]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_cluster_slaves[pool-hiredis]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_readonly_invalid_cluster_state[single-python-parser]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_readonly_invalid_cluster_state[pool-python-parser]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_readonly_invalid_cluster_state[single-hiredis]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_readonly_invalid_cluster_state[pool-hiredis]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_readonly[single-python-parser]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_readonly[pool-python-parser]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_readonly[single-hiredis]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_readonly[pool-hiredis]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_geoadd[single-python-parser]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_geoadd[pool-python-parser]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_geoadd[single-hiredis]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_geoadd[pool-hiredis]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_geoadd_invalid_params[single-python-parser]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_geoadd_invalid_params[pool-python-parser]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_geoadd_invalid_params[single-hiredis]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_geoadd_invalid_params[pool-hiredis]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_geodist[single-python-parser]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_geodist[pool-python-parser]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_geodist[single-hiredis]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_geodist[pool-hiredis]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_geodist_units[single-python-parser]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_geodist_units[pool-python-parser]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_geodist_units[single-hiredis]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_geodist_units[pool-hiredis]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_geodist_missing_one_member[single-python-parser]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_geodist_missing_one_member[pool-python-parser]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_geodist_missing_one_member[single-hiredis]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_geodist_missing_one_member[pool-hiredis]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_geodist_invalid_units[single-python-parser]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_geodist_invalid_units[pool-python-parser]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_geodist_invalid_units[single-hiredis]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_geodist_invalid_units[pool-hiredis]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_geohash[single-python-parser]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_geohash[pool-python-parser]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_geohash[single-hiredis]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_geohash[pool-hiredis]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_geopos[single-python-parser]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_geopos[pool-python-parser]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_geopos[single-hiredis]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_geopos[pool-hiredis]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_geopos_no_value[single-python-parser]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_geopos_no_value[pool-python-parser]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_geopos_no_value[single-hiredis]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_geopos_no_value[pool-hiredis]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_georadius[single-python-parser]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_georadius[pool-python-parser]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_georadius[single-hiredis]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_georadius[pool-hiredis]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_georadius_no_values[single-python-parser]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_georadius_no_values[pool-python-parser]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_georadius_no_values[single-hiredis]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_georadius_no_values[pool-hiredis]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_georadius_units[single-python-parser]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_georadius_units[pool-python-parser]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_georadius_units[single-hiredis]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_georadius_units[pool-hiredis]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_georadius_with[single-python-parser]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_georadius_with[pool-python-parser]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_georadius_with[single-hiredis]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_georadius_with[pool-hiredis]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_georadius_count[single-python-parser]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_georadius_count[pool-python-parser]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_georadius_count[single-hiredis]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_georadius_count[pool-hiredis]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_georadius_sort[single-python-parser]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_georadius_sort[pool-python-parser]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_georadius_sort[single-hiredis]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_georadius_sort[pool-hiredis]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_georadius_store[single-python-parser]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_georadius_store[pool-python-parser]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_georadius_store[single-hiredis]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_georadius_store[pool-hiredis]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_georadius_store_dist[single-python-parser]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_georadius_store_dist[pool-python-parser]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_georadius_store_dist[single-hiredis]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_georadius_store_dist[pool-hiredis]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_georadiusmember[single-python-parser]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_georadiusmember[pool-python-parser]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_georadiusmember[single-hiredis]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_georadiusmember[pool-hiredis]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_xack[single-python-parser]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_xack[pool-python-parser]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_xack[single-hiredis]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_xack[pool-hiredis]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_xadd[single-python-parser]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_xadd[pool-python-parser]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_xadd[single-hiredis]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_xadd[pool-hiredis]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_xclaim[single-python-parser]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_xclaim[pool-python-parser]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_xclaim[single-hiredis]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_xclaim[pool-hiredis]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_xclaim_trimmed[single-python-parser]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_xclaim_trimmed[pool-python-parser]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_xclaim_trimmed[single-hiredis]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_xclaim_trimmed[pool-hiredis]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_xdel[single-python-parser]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_xdel[pool-python-parser]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_xdel[single-hiredis]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_xdel[pool-hiredis]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_xgroup_create[single-python-parser]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_xgroup_create[pool-python-parser]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_xgroup_create[single-hiredis]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_xgroup_create[pool-hiredis]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_xgroup_create_mkstream[single-python-parser]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_xgroup_create_mkstream[pool-python-parser]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_xgroup_create_mkstream[single-hiredis]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_xgroup_create_mkstream[pool-hiredis]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_xgroup_delconsumer[single-python-parser]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_xgroup_delconsumer[pool-python-parser]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_xgroup_delconsumer[single-hiredis]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_xgroup_delconsumer[pool-hiredis]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_xgroup_destroy[single-python-parser]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_xgroup_destroy[pool-python-parser]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_xgroup_destroy[single-hiredis]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_xgroup_destroy[pool-hiredis]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_xgroup_setid[single-python-parser]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_xgroup_setid[pool-python-parser]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_xgroup_setid[single-hiredis]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_xgroup_setid[pool-hiredis]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_xinfo_stream[single-python-parser]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_xinfo_stream[pool-python-parser]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_xinfo_stream[single-hiredis]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_xinfo_stream[pool-hiredis]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_xlen[single-python-parser]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_xlen[pool-python-parser]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_xlen[single-hiredis]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_xlen[pool-hiredis]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_xpending[single-python-parser]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_xpending[pool-python-parser]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_xpending[single-hiredis]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_xpending[pool-hiredis]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_xpending_range[single-python-parser]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_xpending_range[pool-python-parser]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_xpending_range[single-hiredis]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_xpending_range[pool-hiredis]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_xrange[single-python-parser]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_xrange[pool-python-parser]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_xrange[single-hiredis]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_xrange[pool-hiredis]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_xread[single-python-parser]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_xread[pool-python-parser]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_xread[single-hiredis]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_xread[pool-hiredis]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_xreadgroup[single-python-parser]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_xreadgroup[pool-python-parser]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_xreadgroup[single-hiredis]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_xreadgroup[pool-hiredis]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_xrevrange[single-python-parser]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_xrevrange[pool-python-parser]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_xrevrange[single-hiredis]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_xrevrange[pool-hiredis]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_xtrim[single-python-parser]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_xtrim[pool-python-parser]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_xtrim[single-hiredis]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_xtrim[pool-hiredis]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_bitfield_operations[single-python-parser]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_bitfield_operations[pool-python-parser]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_bitfield_operations[single-hiredis]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_bitfield_operations[pool-hiredis]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_memory_stats[single-python-parser]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_memory_stats[pool-python-parser]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_memory_stats[single-hiredis]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_memory_stats[pool-hiredis]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_memory_usage[single-python-parser]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_memory_usage[pool-python-parser]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_memory_usage[single-hiredis]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_memory_usage[pool-hiredis]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_module_list[single-python-parser]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_module_list[pool-python-parser]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_module_list[single-hiredis]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_module_list[pool-hiredis]",
"tests/test_asyncio/test_commands.py::TestBinarySave::test_binary_get_set[single-python-parser]",
"tests/test_asyncio/test_commands.py::TestBinarySave::test_binary_get_set[pool-python-parser]",
"tests/test_asyncio/test_commands.py::TestBinarySave::test_binary_get_set[single-hiredis]",
"tests/test_asyncio/test_commands.py::TestBinarySave::test_binary_get_set[pool-hiredis]",
"tests/test_asyncio/test_commands.py::TestBinarySave::test_binary_lists[single-python-parser]",
"tests/test_asyncio/test_commands.py::TestBinarySave::test_binary_lists[pool-python-parser]",
"tests/test_asyncio/test_commands.py::TestBinarySave::test_binary_lists[single-hiredis]",
"tests/test_asyncio/test_commands.py::TestBinarySave::test_binary_lists[pool-hiredis]",
"tests/test_asyncio/test_commands.py::TestBinarySave::test_22_info[single-python-parser]",
"tests/test_asyncio/test_commands.py::TestBinarySave::test_22_info[pool-python-parser]",
"tests/test_asyncio/test_commands.py::TestBinarySave::test_22_info[single-hiredis]",
"tests/test_asyncio/test_commands.py::TestBinarySave::test_22_info[pool-hiredis]",
"tests/test_asyncio/test_commands.py::TestBinarySave::test_large_responses[single-python-parser]",
"tests/test_asyncio/test_commands.py::TestBinarySave::test_large_responses[pool-python-parser]",
"tests/test_asyncio/test_commands.py::TestBinarySave::test_large_responses[single-hiredis]",
"tests/test_asyncio/test_commands.py::TestBinarySave::test_large_responses[pool-hiredis]",
"tests/test_asyncio/test_commands.py::TestBinarySave::test_floating_point_encoding[single-python-parser]",
"tests/test_asyncio/test_commands.py::TestBinarySave::test_floating_point_encoding[pool-python-parser]",
"tests/test_asyncio/test_commands.py::TestBinarySave::test_floating_point_encoding[single-hiredis]",
"tests/test_asyncio/test_commands.py::TestBinarySave::test_floating_point_encoding[pool-hiredis]",
"tests/test_commands.py::TestResponseCallbacks::test_response_callbacks",
"tests/test_commands.py::TestResponseCallbacks::test_case_insensitive_command_names",
"tests/test_commands.py::TestRedisCommands::test_auth",
"tests/test_commands.py::TestRedisCommands::test_command_on_invalid_key_type",
"tests/test_commands.py::TestRedisCommands::test_acl_cat_no_category",
"tests/test_commands.py::TestRedisCommands::test_acl_cat_with_category",
"tests/test_commands.py::TestRedisCommands::test_acl_deluser",
"tests/test_commands.py::TestRedisCommands::test_acl_genpass",
"tests/test_commands.py::TestRedisCommands::test_acl_help",
"tests/test_commands.py::TestRedisCommands::test_acl_list",
"tests/test_commands.py::TestRedisCommands::test_acl_log",
"tests/test_commands.py::TestRedisCommands::test_acl_setuser_categories_without_prefix_fails",
"tests/test_commands.py::TestRedisCommands::test_acl_setuser_commands_without_prefix_fails",
"tests/test_commands.py::TestRedisCommands::test_acl_setuser_add_passwords_and_nopass_fails",
"tests/test_commands.py::TestRedisCommands::test_acl_users",
"tests/test_commands.py::TestRedisCommands::test_acl_whoami",
"tests/test_commands.py::TestRedisCommands::test_client_list",
"tests/test_commands.py::TestRedisCommands::test_client_info",
"tests/test_commands.py::TestRedisCommands::test_client_list_types_not_replica",
"tests/test_commands.py::TestRedisCommands::test_client_list_replica",
"tests/test_commands.py::TestRedisCommands::test_client_list_client_id",
"tests/test_commands.py::TestRedisCommands::test_client_id",
"tests/test_commands.py::TestRedisCommands::test_client_trackinginfo",
"tests/test_commands.py::TestRedisCommands::test_client_tracking",
"tests/test_commands.py::TestRedisCommands::test_client_unblock",
"tests/test_commands.py::TestRedisCommands::test_client_getname",
"tests/test_commands.py::TestRedisCommands::test_client_setname",
"tests/test_commands.py::TestRedisCommands::test_client_kill",
"tests/test_commands.py::TestRedisCommands::test_client_kill_filter_invalid_params",
"tests/test_commands.py::TestRedisCommands::test_client_kill_filter_by_id",
"tests/test_commands.py::TestRedisCommands::test_client_kill_filter_by_addr",
"tests/test_commands.py::TestRedisCommands::test_client_list_after_client_setname",
"tests/test_commands.py::TestRedisCommands::test_client_kill_filter_by_laddr",
"tests/test_commands.py::TestRedisCommands::test_client_kill_filter_by_user",
"tests/test_commands.py::TestRedisCommands::test_client_pause",
"tests/test_commands.py::TestRedisCommands::test_client_pause_all",
"tests/test_commands.py::TestRedisCommands::test_client_unpause",
"tests/test_commands.py::TestRedisCommands::test_client_no_evict",
"tests/test_commands.py::TestRedisCommands::test_client_reply",
"tests/test_commands.py::TestRedisCommands::test_client_getredir",
"tests/test_commands.py::TestRedisCommands::test_hello_notI_implemented",
"tests/test_commands.py::TestRedisCommands::test_config_get",
"tests/test_commands.py::TestRedisCommands::test_config_get_multi_params",
"tests/test_commands.py::TestRedisCommands::test_config_resetstat",
"tests/test_commands.py::TestRedisCommands::test_config_set",
"tests/test_commands.py::TestRedisCommands::test_config_set_multi_params",
"tests/test_commands.py::TestRedisCommands::test_failover",
"tests/test_commands.py::TestRedisCommands::test_dbsize",
"tests/test_commands.py::TestRedisCommands::test_echo",
"tests/test_commands.py::TestRedisCommands::test_info",
"tests/test_commands.py::TestRedisCommands::test_info_multi_sections",
"tests/test_commands.py::TestRedisCommands::test_lastsave",
"tests/test_commands.py::TestRedisCommands::test_lolwut",
"tests/test_commands.py::TestRedisCommands::test_reset",
"tests/test_commands.py::TestRedisCommands::test_object",
"tests/test_commands.py::TestRedisCommands::test_ping",
"tests/test_commands.py::TestRedisCommands::test_quit",
"tests/test_commands.py::TestRedisCommands::test_role",
"tests/test_commands.py::TestRedisCommands::test_select",
"tests/test_commands.py::TestRedisCommands::test_slowlog_get",
"tests/test_commands.py::TestRedisCommands::test_slowlog_get_limit",
"tests/test_commands.py::TestRedisCommands::test_slowlog_length",
"tests/test_commands.py::TestRedisCommands::test_time",
"tests/test_commands.py::TestRedisCommands::test_bgsave",
"tests/test_commands.py::TestRedisCommands::test_append",
"tests/test_commands.py::TestRedisCommands::test_bitcount",
"tests/test_commands.py::TestRedisCommands::test_bitcount_mode",
"tests/test_commands.py::TestRedisCommands::test_bitop_not_empty_string",
"tests/test_commands.py::TestRedisCommands::test_bitop_not",
"tests/test_commands.py::TestRedisCommands::test_bitop_not_in_place",
"tests/test_commands.py::TestRedisCommands::test_bitop_single_string",
"tests/test_commands.py::TestRedisCommands::test_bitop_string_operands",
"tests/test_commands.py::TestRedisCommands::test_bitpos",
"tests/test_commands.py::TestRedisCommands::test_bitpos_wrong_arguments",
"tests/test_commands.py::TestRedisCommands::test_bitpos_mode",
"tests/test_commands.py::TestRedisCommands::test_copy",
"tests/test_commands.py::TestRedisCommands::test_copy_and_replace",
"tests/test_commands.py::TestRedisCommands::test_copy_to_another_database",
"tests/test_commands.py::TestRedisCommands::test_decr",
"tests/test_commands.py::TestRedisCommands::test_decrby",
"tests/test_commands.py::TestRedisCommands::test_delete",
"tests/test_commands.py::TestRedisCommands::test_delete_with_multiple_keys",
"tests/test_commands.py::TestRedisCommands::test_delitem",
"tests/test_commands.py::TestRedisCommands::test_unlink",
"tests/test_commands.py::TestRedisCommands::test_unlink_with_multiple_keys",
"tests/test_commands.py::TestRedisCommands::test_lcs",
"tests/test_commands.py::TestRedisCommands::test_dump_and_restore",
"tests/test_commands.py::TestRedisCommands::test_dump_and_restore_and_replace",
"tests/test_commands.py::TestRedisCommands::test_dump_and_restore_absttl",
"tests/test_commands.py::TestRedisCommands::test_exists",
"tests/test_commands.py::TestRedisCommands::test_exists_contains",
"tests/test_commands.py::TestRedisCommands::test_expire",
"tests/test_commands.py::TestRedisCommands::test_expire_option_nx",
"tests/test_commands.py::TestRedisCommands::test_expire_option_xx",
"tests/test_commands.py::TestRedisCommands::test_expire_option_gt",
"tests/test_commands.py::TestRedisCommands::test_expire_option_lt",
"tests/test_commands.py::TestRedisCommands::test_expireat_datetime",
"tests/test_commands.py::TestRedisCommands::test_expireat_no_key",
"tests/test_commands.py::TestRedisCommands::test_expireat_unixtime",
"tests/test_commands.py::TestRedisCommands::test_expiretime",
"tests/test_commands.py::TestRedisCommands::test_expireat_option_nx",
"tests/test_commands.py::TestRedisCommands::test_expireat_option_xx",
"tests/test_commands.py::TestRedisCommands::test_expireat_option_gt",
"tests/test_commands.py::TestRedisCommands::test_expireat_option_lt",
"tests/test_commands.py::TestRedisCommands::test_get_and_set",
"tests/test_commands.py::TestRedisCommands::test_getdel",
"tests/test_commands.py::TestRedisCommands::test_getex",
"tests/test_commands.py::TestRedisCommands::test_getitem_and_setitem",
"tests/test_commands.py::TestRedisCommands::test_getitem_raises_keyerror_for_missing_key",
"tests/test_commands.py::TestRedisCommands::test_getitem_does_not_raise_keyerror_for_empty_string",
"tests/test_commands.py::TestRedisCommands::test_get_set_bit",
"tests/test_commands.py::TestRedisCommands::test_getrange",
"tests/test_commands.py::TestRedisCommands::test_getset",
"tests/test_commands.py::TestRedisCommands::test_incr",
"tests/test_commands.py::TestRedisCommands::test_incrby",
"tests/test_commands.py::TestRedisCommands::test_incrbyfloat",
"tests/test_commands.py::TestRedisCommands::test_keys",
"tests/test_commands.py::TestRedisCommands::test_mget",
"tests/test_commands.py::TestRedisCommands::test_lmove",
"tests/test_commands.py::TestRedisCommands::test_blmove",
"tests/test_commands.py::TestRedisCommands::test_mset",
"tests/test_commands.py::TestRedisCommands::test_msetnx",
"tests/test_commands.py::TestRedisCommands::test_pexpire",
"tests/test_commands.py::TestRedisCommands::test_pexpire_option_nx",
"tests/test_commands.py::TestRedisCommands::test_pexpire_option_xx",
"tests/test_commands.py::TestRedisCommands::test_pexpire_option_gt",
"tests/test_commands.py::TestRedisCommands::test_pexpire_option_lt",
"tests/test_commands.py::TestRedisCommands::test_pexpireat_datetime",
"tests/test_commands.py::TestRedisCommands::test_pexpireat_no_key",
"tests/test_commands.py::TestRedisCommands::test_pexpireat_unixtime",
"tests/test_commands.py::TestRedisCommands::test_pexpireat_option_nx",
"tests/test_commands.py::TestRedisCommands::test_pexpireat_option_xx",
"tests/test_commands.py::TestRedisCommands::test_pexpireat_option_gt",
"tests/test_commands.py::TestRedisCommands::test_pexpireat_option_lt",
"tests/test_commands.py::TestRedisCommands::test_pexpiretime",
"tests/test_commands.py::TestRedisCommands::test_psetex",
"tests/test_commands.py::TestRedisCommands::test_psetex_timedelta",
"tests/test_commands.py::TestRedisCommands::test_pttl",
"tests/test_commands.py::TestRedisCommands::test_pttl_no_key",
"tests/test_commands.py::TestRedisCommands::test_hrandfield",
"tests/test_commands.py::TestRedisCommands::test_randomkey",
"tests/test_commands.py::TestRedisCommands::test_rename",
"tests/test_commands.py::TestRedisCommands::test_renamenx",
"tests/test_commands.py::TestRedisCommands::test_set_nx",
"tests/test_commands.py::TestRedisCommands::test_set_xx",
"tests/test_commands.py::TestRedisCommands::test_set_px",
"tests/test_commands.py::TestRedisCommands::test_set_px_timedelta",
"tests/test_commands.py::TestRedisCommands::test_set_ex",
"tests/test_commands.py::TestRedisCommands::test_set_ex_timedelta",
"tests/test_commands.py::TestRedisCommands::test_set_exat_timedelta",
"tests/test_commands.py::TestRedisCommands::test_set_pxat_timedelta",
"tests/test_commands.py::TestRedisCommands::test_set_multipleoptions",
"tests/test_commands.py::TestRedisCommands::test_set_keepttl",
"tests/test_commands.py::TestRedisCommands::test_set_get",
"tests/test_commands.py::TestRedisCommands::test_setex",
"tests/test_commands.py::TestRedisCommands::test_setnx",
"tests/test_commands.py::TestRedisCommands::test_setrange",
"tests/test_commands.py::TestRedisCommands::test_strlen",
"tests/test_commands.py::TestRedisCommands::test_substr",
"tests/test_commands.py::TestRedisCommands::test_ttl",
"tests/test_commands.py::TestRedisCommands::test_ttl_nokey",
"tests/test_commands.py::TestRedisCommands::test_type",
"tests/test_commands.py::TestRedisCommands::test_blpop",
"tests/test_commands.py::TestRedisCommands::test_brpop",
"tests/test_commands.py::TestRedisCommands::test_brpoplpush",
"tests/test_commands.py::TestRedisCommands::test_brpoplpush_empty_string",
"tests/test_commands.py::TestRedisCommands::test_blmpop",
"tests/test_commands.py::TestRedisCommands::test_lmpop",
"tests/test_commands.py::TestRedisCommands::test_lindex",
"tests/test_commands.py::TestRedisCommands::test_linsert",
"tests/test_commands.py::TestRedisCommands::test_llen",
"tests/test_commands.py::TestRedisCommands::test_lpop",
"tests/test_commands.py::TestRedisCommands::test_lpop_count",
"tests/test_commands.py::TestRedisCommands::test_lpush",
"tests/test_commands.py::TestRedisCommands::test_lpushx",
"tests/test_commands.py::TestRedisCommands::test_lpushx_with_list",
"tests/test_commands.py::TestRedisCommands::test_lrange",
"tests/test_commands.py::TestRedisCommands::test_lrem",
"tests/test_commands.py::TestRedisCommands::test_lset",
"tests/test_commands.py::TestRedisCommands::test_ltrim",
"tests/test_commands.py::TestRedisCommands::test_rpop",
"tests/test_commands.py::TestRedisCommands::test_rpop_count",
"tests/test_commands.py::TestRedisCommands::test_rpoplpush",
"tests/test_commands.py::TestRedisCommands::test_rpush",
"tests/test_commands.py::TestRedisCommands::test_lpos",
"tests/test_commands.py::TestRedisCommands::test_rpushx",
"tests/test_commands.py::TestRedisCommands::test_scan",
"tests/test_commands.py::TestRedisCommands::test_scan_type",
"tests/test_commands.py::TestRedisCommands::test_scan_iter",
"tests/test_commands.py::TestRedisCommands::test_sscan",
"tests/test_commands.py::TestRedisCommands::test_sscan_iter",
"tests/test_commands.py::TestRedisCommands::test_hscan",
"tests/test_commands.py::TestRedisCommands::test_hscan_iter",
"tests/test_commands.py::TestRedisCommands::test_zscan",
"tests/test_commands.py::TestRedisCommands::test_zscan_iter",
"tests/test_commands.py::TestRedisCommands::test_sadd",
"tests/test_commands.py::TestRedisCommands::test_scard",
"tests/test_commands.py::TestRedisCommands::test_sdiff",
"tests/test_commands.py::TestRedisCommands::test_sdiffstore",
"tests/test_commands.py::TestRedisCommands::test_sinter",
"tests/test_commands.py::TestRedisCommands::test_sintercard",
"tests/test_commands.py::TestRedisCommands::test_sinterstore",
"tests/test_commands.py::TestRedisCommands::test_sismember",
"tests/test_commands.py::TestRedisCommands::test_smembers",
"tests/test_commands.py::TestRedisCommands::test_smismember",
"tests/test_commands.py::TestRedisCommands::test_smove",
"tests/test_commands.py::TestRedisCommands::test_spop",
"tests/test_commands.py::TestRedisCommands::test_spop_multi_value",
"tests/test_commands.py::TestRedisCommands::test_srandmember",
"tests/test_commands.py::TestRedisCommands::test_srandmember_multi_value",
"tests/test_commands.py::TestRedisCommands::test_srem",
"tests/test_commands.py::TestRedisCommands::test_sunion",
"tests/test_commands.py::TestRedisCommands::test_sunionstore",
"tests/test_commands.py::TestRedisCommands::test_debug_segfault",
"tests/test_commands.py::TestRedisCommands::test_script_debug",
"tests/test_commands.py::TestRedisCommands::test_zadd",
"tests/test_commands.py::TestRedisCommands::test_zadd_nx",
"tests/test_commands.py::TestRedisCommands::test_zadd_xx",
"tests/test_commands.py::TestRedisCommands::test_zadd_ch",
"tests/test_commands.py::TestRedisCommands::test_zadd_incr",
"tests/test_commands.py::TestRedisCommands::test_zadd_incr_with_xx",
"tests/test_commands.py::TestRedisCommands::test_zadd_gt_lt",
"tests/test_commands.py::TestRedisCommands::test_zcard",
"tests/test_commands.py::TestRedisCommands::test_zcount",
"tests/test_commands.py::TestRedisCommands::test_zdiff",
"tests/test_commands.py::TestRedisCommands::test_zdiffstore",
"tests/test_commands.py::TestRedisCommands::test_zincrby",
"tests/test_commands.py::TestRedisCommands::test_zlexcount",
"tests/test_commands.py::TestRedisCommands::test_zinter",
"tests/test_commands.py::TestRedisCommands::test_zintercard",
"tests/test_commands.py::TestRedisCommands::test_zinterstore_sum",
"tests/test_commands.py::TestRedisCommands::test_zinterstore_max",
"tests/test_commands.py::TestRedisCommands::test_zinterstore_min",
"tests/test_commands.py::TestRedisCommands::test_zinterstore_with_weight",
"tests/test_commands.py::TestRedisCommands::test_zpopmax",
"tests/test_commands.py::TestRedisCommands::test_zpopmin",
"tests/test_commands.py::TestRedisCommands::test_zrandemember",
"tests/test_commands.py::TestRedisCommands::test_bzpopmax",
"tests/test_commands.py::TestRedisCommands::test_bzpopmin",
"tests/test_commands.py::TestRedisCommands::test_zmpop",
"tests/test_commands.py::TestRedisCommands::test_bzmpop",
"tests/test_commands.py::TestRedisCommands::test_zrange",
"tests/test_commands.py::TestRedisCommands::test_zrange_errors",
"tests/test_commands.py::TestRedisCommands::test_zrange_params",
"tests/test_commands.py::TestRedisCommands::test_zrangestore",
"tests/test_commands.py::TestRedisCommands::test_zrangebylex",
"tests/test_commands.py::TestRedisCommands::test_zrevrangebylex",
"tests/test_commands.py::TestRedisCommands::test_zrangebyscore",
"tests/test_commands.py::TestRedisCommands::test_zrank",
"tests/test_commands.py::TestRedisCommands::test_zrem",
"tests/test_commands.py::TestRedisCommands::test_zrem_multiple_keys",
"tests/test_commands.py::TestRedisCommands::test_zremrangebylex",
"tests/test_commands.py::TestRedisCommands::test_zremrangebyrank",
"tests/test_commands.py::TestRedisCommands::test_zremrangebyscore",
"tests/test_commands.py::TestRedisCommands::test_zrevrange",
"tests/test_commands.py::TestRedisCommands::test_zrevrangebyscore",
"tests/test_commands.py::TestRedisCommands::test_zrevrank",
"tests/test_commands.py::TestRedisCommands::test_zscore",
"tests/test_commands.py::TestRedisCommands::test_zunion",
"tests/test_commands.py::TestRedisCommands::test_zunionstore_sum",
"tests/test_commands.py::TestRedisCommands::test_zunionstore_max",
"tests/test_commands.py::TestRedisCommands::test_zunionstore_min",
"tests/test_commands.py::TestRedisCommands::test_zunionstore_with_weight",
"tests/test_commands.py::TestRedisCommands::test_zmscore",
"tests/test_commands.py::TestRedisCommands::test_pfadd",
"tests/test_commands.py::TestRedisCommands::test_pfcount",
"tests/test_commands.py::TestRedisCommands::test_pfmerge",
"tests/test_commands.py::TestRedisCommands::test_hget_and_hset",
"tests/test_commands.py::TestRedisCommands::test_hset_with_multi_key_values",
"tests/test_commands.py::TestRedisCommands::test_hset_with_key_values_passed_as_list",
"tests/test_commands.py::TestRedisCommands::test_hset_without_data",
"tests/test_commands.py::TestRedisCommands::test_hdel",
"tests/test_commands.py::TestRedisCommands::test_hexists",
"tests/test_commands.py::TestRedisCommands::test_hgetall",
"tests/test_commands.py::TestRedisCommands::test_hincrby",
"tests/test_commands.py::TestRedisCommands::test_hincrbyfloat",
"tests/test_commands.py::TestRedisCommands::test_hkeys",
"tests/test_commands.py::TestRedisCommands::test_hlen",
"tests/test_commands.py::TestRedisCommands::test_hmget",
"tests/test_commands.py::TestRedisCommands::test_hmset",
"tests/test_commands.py::TestRedisCommands::test_hsetnx",
"tests/test_commands.py::TestRedisCommands::test_hvals",
"tests/test_commands.py::TestRedisCommands::test_hstrlen",
"tests/test_commands.py::TestRedisCommands::test_sort_basic",
"tests/test_commands.py::TestRedisCommands::test_sort_limited",
"tests/test_commands.py::TestRedisCommands::test_sort_by",
"tests/test_commands.py::TestRedisCommands::test_sort_get",
"tests/test_commands.py::TestRedisCommands::test_sort_get_multi",
"tests/test_commands.py::TestRedisCommands::test_sort_get_groups_two",
"tests/test_commands.py::TestRedisCommands::test_sort_groups_string_get",
"tests/test_commands.py::TestRedisCommands::test_sort_groups_just_one_get",
"tests/test_commands.py::TestRedisCommands::test_sort_groups_no_get",
"tests/test_commands.py::TestRedisCommands::test_sort_groups_three_gets",
"tests/test_commands.py::TestRedisCommands::test_sort_desc",
"tests/test_commands.py::TestRedisCommands::test_sort_alpha",
"tests/test_commands.py::TestRedisCommands::test_sort_store",
"tests/test_commands.py::TestRedisCommands::test_sort_all_options",
"tests/test_commands.py::TestRedisCommands::test_sort_ro",
"tests/test_commands.py::TestRedisCommands::test_sort_issue_924",
"tests/test_commands.py::TestRedisCommands::test_cluster_addslots",
"tests/test_commands.py::TestRedisCommands::test_cluster_count_failure_reports",
"tests/test_commands.py::TestRedisCommands::test_cluster_countkeysinslot",
"tests/test_commands.py::TestRedisCommands::test_cluster_delslots",
"tests/test_commands.py::TestRedisCommands::test_cluster_failover",
"tests/test_commands.py::TestRedisCommands::test_cluster_forget",
"tests/test_commands.py::TestRedisCommands::test_cluster_info",
"tests/test_commands.py::TestRedisCommands::test_cluster_keyslot",
"tests/test_commands.py::TestRedisCommands::test_cluster_meet",
"tests/test_commands.py::TestRedisCommands::test_cluster_nodes",
"tests/test_commands.py::TestRedisCommands::test_cluster_replicate",
"tests/test_commands.py::TestRedisCommands::test_cluster_reset",
"tests/test_commands.py::TestRedisCommands::test_cluster_saveconfig",
"tests/test_commands.py::TestRedisCommands::test_cluster_setslot",
"tests/test_commands.py::TestRedisCommands::test_cluster_slaves",
"tests/test_commands.py::TestRedisCommands::test_readonly_invalid_cluster_state",
"tests/test_commands.py::TestRedisCommands::test_readonly",
"tests/test_commands.py::TestRedisCommands::test_geoadd",
"tests/test_commands.py::TestRedisCommands::test_geoadd_nx",
"tests/test_commands.py::TestRedisCommands::test_geoadd_xx",
"tests/test_commands.py::TestRedisCommands::test_geoadd_ch",
"tests/test_commands.py::TestRedisCommands::test_geoadd_invalid_params",
"tests/test_commands.py::TestRedisCommands::test_geodist",
"tests/test_commands.py::TestRedisCommands::test_geodist_units",
"tests/test_commands.py::TestRedisCommands::test_geodist_missing_one_member",
"tests/test_commands.py::TestRedisCommands::test_geodist_invalid_units",
"tests/test_commands.py::TestRedisCommands::test_geohash",
"tests/test_commands.py::TestRedisCommands::test_geopos",
"tests/test_commands.py::TestRedisCommands::test_geopos_no_value",
"tests/test_commands.py::TestRedisCommands::test_geosearch",
"tests/test_commands.py::TestRedisCommands::test_geosearch_member",
"tests/test_commands.py::TestRedisCommands::test_geosearch_sort",
"tests/test_commands.py::TestRedisCommands::test_geosearch_with",
"tests/test_commands.py::TestRedisCommands::test_geosearch_negative",
"tests/test_commands.py::TestRedisCommands::test_geosearchstore",
"tests/test_commands.py::TestRedisCommands::test_geosearchstore_dist",
"tests/test_commands.py::TestRedisCommands::test_georadius",
"tests/test_commands.py::TestRedisCommands::test_georadius_no_values",
"tests/test_commands.py::TestRedisCommands::test_georadius_units",
"tests/test_commands.py::TestRedisCommands::test_georadius_with",
"tests/test_commands.py::TestRedisCommands::test_georadius_count",
"tests/test_commands.py::TestRedisCommands::test_georadius_sort",
"tests/test_commands.py::TestRedisCommands::test_georadius_store",
"tests/test_commands.py::TestRedisCommands::test_georadius_store_dist",
"tests/test_commands.py::TestRedisCommands::test_georadiusmember",
"tests/test_commands.py::TestRedisCommands::test_georadiusmember_count",
"tests/test_commands.py::TestRedisCommands::test_xack",
"tests/test_commands.py::TestRedisCommands::test_xadd",
"tests/test_commands.py::TestRedisCommands::test_xadd_nomkstream",
"tests/test_commands.py::TestRedisCommands::test_xadd_minlen_and_limit",
"tests/test_commands.py::TestRedisCommands::test_xadd_explicit_ms",
"tests/test_commands.py::TestRedisCommands::test_xautoclaim_negative",
"tests/test_commands.py::TestRedisCommands::test_xclaim",
"tests/test_commands.py::TestRedisCommands::test_xclaim_trimmed",
"tests/test_commands.py::TestRedisCommands::test_xdel",
"tests/test_commands.py::TestRedisCommands::test_xgroup_create",
"tests/test_commands.py::TestRedisCommands::test_xgroup_create_mkstream",
"tests/test_commands.py::TestRedisCommands::test_xgroup_create_entriesread",
"tests/test_commands.py::TestRedisCommands::test_xgroup_delconsumer",
"tests/test_commands.py::TestRedisCommands::test_xgroup_createconsumer",
"tests/test_commands.py::TestRedisCommands::test_xgroup_destroy",
"tests/test_commands.py::TestRedisCommands::test_xgroup_setid",
"tests/test_commands.py::TestRedisCommands::test_xinfo_stream",
"tests/test_commands.py::TestRedisCommands::test_xinfo_stream_full",
"tests/test_commands.py::TestRedisCommands::test_xlen",
"tests/test_commands.py::TestRedisCommands::test_xpending",
"tests/test_commands.py::TestRedisCommands::test_xpending_range",
"tests/test_commands.py::TestRedisCommands::test_xpending_range_idle",
"tests/test_commands.py::TestRedisCommands::test_xpending_range_negative",
"tests/test_commands.py::TestRedisCommands::test_xrange",
"tests/test_commands.py::TestRedisCommands::test_xread",
"tests/test_commands.py::TestRedisCommands::test_xreadgroup",
"tests/test_commands.py::TestRedisCommands::test_xrevrange",
"tests/test_commands.py::TestRedisCommands::test_xtrim",
"tests/test_commands.py::TestRedisCommands::test_xtrim_minlen_and_length_args",
"tests/test_commands.py::TestRedisCommands::test_bitfield_operations",
"tests/test_commands.py::TestRedisCommands::test_memory_help",
"tests/test_commands.py::TestRedisCommands::test_memory_doctor",
"tests/test_commands.py::TestRedisCommands::test_memory_malloc_stats",
"tests/test_commands.py::TestRedisCommands::test_memory_stats",
"tests/test_commands.py::TestRedisCommands::test_memory_usage",
"tests/test_commands.py::TestRedisCommands::test_latency_histogram_not_implemented",
"tests/test_commands.py::TestRedisCommands::test_module_list",
"tests/test_commands.py::TestRedisCommands::test_command_count",
"tests/test_commands.py::TestRedisCommands::test_command_docs",
"tests/test_commands.py::TestRedisCommands::test_command_list",
"tests/test_commands.py::TestRedisCommands::test_command_getkeys",
"tests/test_commands.py::TestRedisCommands::test_command",
"tests/test_commands.py::TestRedisCommands::test_command_getkeysandflags",
"tests/test_commands.py::TestRedisCommands::test_module",
"tests/test_commands.py::TestRedisCommands::test_module_loadex",
"tests/test_commands.py::TestRedisCommands::test_restore",
"tests/test_commands.py::TestRedisCommands::test_restore_idletime",
"tests/test_commands.py::TestRedisCommands::test_restore_frequency",
"tests/test_commands.py::TestRedisCommands::test_replicaof",
"tests/test_commands.py::TestRedisCommands::test_shutdown",
"tests/test_commands.py::TestRedisCommands::test_shutdown_with_params",
"tests/test_commands.py::TestRedisCommands::test_sync",
"tests/test_commands.py::TestRedisCommands::test_psync",
"tests/test_commands.py::TestBinarySave::test_binary_get_set",
"tests/test_commands.py::TestBinarySave::test_binary_lists",
"tests/test_commands.py::TestBinarySave::test_22_info",
"tests/test_commands.py::TestBinarySave::test_large_responses",
"tests/test_commands.py::TestBinarySave::test_floating_point_encoding"
] | [
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_bitfield_ro[single-python-parser]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_bitfield_ro[pool-python-parser]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_bitfield_ro[single-hiredis]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_bitfield_ro[pool-hiredis]",
"tests/test_commands.py::TestRedisCommands::test_bitfield_ro"
] | Python | [] | [] |
|
redis/redis-py | 2,501 | redis__redis-py-2501 | [
"1977"
] | 6219574b042a6596b150ca8248441198f01f8c87 | diff --git a/redis/commands/core.py b/redis/commands/core.py
--- a/redis/commands/core.py
+++ b/redis/commands/core.py
@@ -980,6 +980,34 @@ def lastsave(self, **kwargs) -> ResponseT:
"""
return self.execute_command("LASTSAVE", **kwargs)
+ def latency_doctor(self):
+ """Raise a NotImplementedError, as the client will not support LATENCY DOCTOR.
+ This funcion is best used within the redis-cli.
+
+ For more information see https://redis.io/commands/latency-doctor
+ """
+ raise NotImplementedError(
+ """
+ LATENCY DOCTOR is intentionally not implemented in the client.
+
+ For more information see https://redis.io/commands/latency-doctor
+ """
+ )
+
+ def latency_graph(self):
+ """Raise a NotImplementedError, as the client will not support LATENCY GRAPH.
+ This funcion is best used within the redis-cli.
+
+ For more information see https://redis.io/commands/latency-graph.
+ """
+ raise NotImplementedError(
+ """
+ LATENCY GRAPH is intentionally not implemented in the client.
+
+ For more information see https://redis.io/commands/latency-graph
+ """
+ )
+
def lolwut(self, *version_numbers: Union[str, float], **kwargs) -> ResponseT:
"""
Get the Redis version and a piece of generative computer art
| diff --git a/tests/test_commands.py b/tests/test_commands.py
--- a/tests/test_commands.py
+++ b/tests/test_commands.py
@@ -4527,6 +4527,16 @@ def test_latency_histogram_not_implemented(self, r: redis.Redis):
with pytest.raises(NotImplementedError):
r.latency_histogram()
+ @skip_if_server_version_lt("7.0.0")
+ def test_latency_graph_not_implemented(self, r: redis.Redis):
+ with pytest.raises(NotImplementedError):
+ r.latency_graph()
+
+ @skip_if_server_version_lt("7.0.0")
+ def test_latency_doctor_not_implemented(self, r: redis.Redis):
+ with pytest.raises(NotImplementedError):
+ r.latency_doctor()
+
@pytest.mark.onlynoncluster
@skip_if_server_version_lt("4.0.0")
@skip_if_redis_enterprise()
| Add support for missing command LATENCY DOCTOR
| "2022-12-11T07:25:13Z" | 4.4 | [
"tests/test_commands.py::TestResponseCallbacks::test_response_callbacks",
"tests/test_commands.py::TestResponseCallbacks::test_case_insensitive_command_names",
"tests/test_commands.py::TestRedisCommands::test_auth",
"tests/test_commands.py::TestRedisCommands::test_command_on_invalid_key_type",
"tests/test_commands.py::TestRedisCommands::test_acl_cat_no_category",
"tests/test_commands.py::TestRedisCommands::test_acl_cat_with_category",
"tests/test_commands.py::TestRedisCommands::test_acl_deluser",
"tests/test_commands.py::TestRedisCommands::test_acl_genpass",
"tests/test_commands.py::TestRedisCommands::test_acl_help",
"tests/test_commands.py::TestRedisCommands::test_acl_list",
"tests/test_commands.py::TestRedisCommands::test_acl_log",
"tests/test_commands.py::TestRedisCommands::test_acl_setuser_categories_without_prefix_fails",
"tests/test_commands.py::TestRedisCommands::test_acl_setuser_commands_without_prefix_fails",
"tests/test_commands.py::TestRedisCommands::test_acl_setuser_add_passwords_and_nopass_fails",
"tests/test_commands.py::TestRedisCommands::test_acl_users",
"tests/test_commands.py::TestRedisCommands::test_acl_whoami",
"tests/test_commands.py::TestRedisCommands::test_client_list",
"tests/test_commands.py::TestRedisCommands::test_client_info",
"tests/test_commands.py::TestRedisCommands::test_client_list_types_not_replica",
"tests/test_commands.py::TestRedisCommands::test_client_list_replica",
"tests/test_commands.py::TestRedisCommands::test_client_list_client_id",
"tests/test_commands.py::TestRedisCommands::test_client_id",
"tests/test_commands.py::TestRedisCommands::test_client_trackinginfo",
"tests/test_commands.py::TestRedisCommands::test_client_tracking",
"tests/test_commands.py::TestRedisCommands::test_client_unblock",
"tests/test_commands.py::TestRedisCommands::test_client_getname",
"tests/test_commands.py::TestRedisCommands::test_client_setname",
"tests/test_commands.py::TestRedisCommands::test_client_kill",
"tests/test_commands.py::TestRedisCommands::test_client_kill_filter_invalid_params",
"tests/test_commands.py::TestRedisCommands::test_client_kill_filter_by_id",
"tests/test_commands.py::TestRedisCommands::test_client_kill_filter_by_addr",
"tests/test_commands.py::TestRedisCommands::test_client_list_after_client_setname",
"tests/test_commands.py::TestRedisCommands::test_client_kill_filter_by_laddr",
"tests/test_commands.py::TestRedisCommands::test_client_kill_filter_by_user",
"tests/test_commands.py::TestRedisCommands::test_client_pause",
"tests/test_commands.py::TestRedisCommands::test_client_pause_all",
"tests/test_commands.py::TestRedisCommands::test_client_unpause",
"tests/test_commands.py::TestRedisCommands::test_client_no_evict",
"tests/test_commands.py::TestRedisCommands::test_client_reply",
"tests/test_commands.py::TestRedisCommands::test_client_getredir",
"tests/test_commands.py::TestRedisCommands::test_hello_notI_implemented",
"tests/test_commands.py::TestRedisCommands::test_config_get",
"tests/test_commands.py::TestRedisCommands::test_config_get_multi_params",
"tests/test_commands.py::TestRedisCommands::test_config_resetstat",
"tests/test_commands.py::TestRedisCommands::test_config_set",
"tests/test_commands.py::TestRedisCommands::test_config_set_multi_params",
"tests/test_commands.py::TestRedisCommands::test_failover",
"tests/test_commands.py::TestRedisCommands::test_dbsize",
"tests/test_commands.py::TestRedisCommands::test_echo",
"tests/test_commands.py::TestRedisCommands::test_info",
"tests/test_commands.py::TestRedisCommands::test_info_multi_sections",
"tests/test_commands.py::TestRedisCommands::test_lastsave",
"tests/test_commands.py::TestRedisCommands::test_lolwut",
"tests/test_commands.py::TestRedisCommands::test_reset",
"tests/test_commands.py::TestRedisCommands::test_object",
"tests/test_commands.py::TestRedisCommands::test_ping",
"tests/test_commands.py::TestRedisCommands::test_quit",
"tests/test_commands.py::TestRedisCommands::test_role",
"tests/test_commands.py::TestRedisCommands::test_select",
"tests/test_commands.py::TestRedisCommands::test_slowlog_get",
"tests/test_commands.py::TestRedisCommands::test_slowlog_get_limit",
"tests/test_commands.py::TestRedisCommands::test_slowlog_length",
"tests/test_commands.py::TestRedisCommands::test_time",
"tests/test_commands.py::TestRedisCommands::test_bgsave",
"tests/test_commands.py::TestRedisCommands::test_never_decode_option",
"tests/test_commands.py::TestRedisCommands::test_empty_response_option",
"tests/test_commands.py::TestRedisCommands::test_append",
"tests/test_commands.py::TestRedisCommands::test_bitcount",
"tests/test_commands.py::TestRedisCommands::test_bitcount_mode",
"tests/test_commands.py::TestRedisCommands::test_bitop_not_empty_string",
"tests/test_commands.py::TestRedisCommands::test_bitop_not",
"tests/test_commands.py::TestRedisCommands::test_bitop_not_in_place",
"tests/test_commands.py::TestRedisCommands::test_bitop_single_string",
"tests/test_commands.py::TestRedisCommands::test_bitop_string_operands",
"tests/test_commands.py::TestRedisCommands::test_bitpos",
"tests/test_commands.py::TestRedisCommands::test_bitpos_wrong_arguments",
"tests/test_commands.py::TestRedisCommands::test_bitpos_mode",
"tests/test_commands.py::TestRedisCommands::test_copy",
"tests/test_commands.py::TestRedisCommands::test_copy_and_replace",
"tests/test_commands.py::TestRedisCommands::test_copy_to_another_database",
"tests/test_commands.py::TestRedisCommands::test_decr",
"tests/test_commands.py::TestRedisCommands::test_decrby",
"tests/test_commands.py::TestRedisCommands::test_delete",
"tests/test_commands.py::TestRedisCommands::test_delete_with_multiple_keys",
"tests/test_commands.py::TestRedisCommands::test_delitem",
"tests/test_commands.py::TestRedisCommands::test_unlink",
"tests/test_commands.py::TestRedisCommands::test_unlink_with_multiple_keys",
"tests/test_commands.py::TestRedisCommands::test_lcs",
"tests/test_commands.py::TestRedisCommands::test_dump_and_restore",
"tests/test_commands.py::TestRedisCommands::test_dump_and_restore_and_replace",
"tests/test_commands.py::TestRedisCommands::test_dump_and_restore_absttl",
"tests/test_commands.py::TestRedisCommands::test_exists",
"tests/test_commands.py::TestRedisCommands::test_exists_contains",
"tests/test_commands.py::TestRedisCommands::test_expire",
"tests/test_commands.py::TestRedisCommands::test_expire_option_nx",
"tests/test_commands.py::TestRedisCommands::test_expire_option_xx",
"tests/test_commands.py::TestRedisCommands::test_expire_option_gt",
"tests/test_commands.py::TestRedisCommands::test_expire_option_lt",
"tests/test_commands.py::TestRedisCommands::test_expireat_datetime",
"tests/test_commands.py::TestRedisCommands::test_expireat_no_key",
"tests/test_commands.py::TestRedisCommands::test_expireat_unixtime",
"tests/test_commands.py::TestRedisCommands::test_expiretime",
"tests/test_commands.py::TestRedisCommands::test_expireat_option_nx",
"tests/test_commands.py::TestRedisCommands::test_expireat_option_xx",
"tests/test_commands.py::TestRedisCommands::test_expireat_option_gt",
"tests/test_commands.py::TestRedisCommands::test_expireat_option_lt",
"tests/test_commands.py::TestRedisCommands::test_get_and_set",
"tests/test_commands.py::TestRedisCommands::test_getdel",
"tests/test_commands.py::TestRedisCommands::test_getex",
"tests/test_commands.py::TestRedisCommands::test_getitem_and_setitem",
"tests/test_commands.py::TestRedisCommands::test_getitem_raises_keyerror_for_missing_key",
"tests/test_commands.py::TestRedisCommands::test_getitem_does_not_raise_keyerror_for_empty_string",
"tests/test_commands.py::TestRedisCommands::test_get_set_bit",
"tests/test_commands.py::TestRedisCommands::test_getrange",
"tests/test_commands.py::TestRedisCommands::test_getset",
"tests/test_commands.py::TestRedisCommands::test_incr",
"tests/test_commands.py::TestRedisCommands::test_incrby",
"tests/test_commands.py::TestRedisCommands::test_incrbyfloat",
"tests/test_commands.py::TestRedisCommands::test_keys",
"tests/test_commands.py::TestRedisCommands::test_mget",
"tests/test_commands.py::TestRedisCommands::test_lmove",
"tests/test_commands.py::TestRedisCommands::test_blmove",
"tests/test_commands.py::TestRedisCommands::test_mset",
"tests/test_commands.py::TestRedisCommands::test_msetnx",
"tests/test_commands.py::TestRedisCommands::test_pexpire",
"tests/test_commands.py::TestRedisCommands::test_pexpire_option_nx",
"tests/test_commands.py::TestRedisCommands::test_pexpire_option_xx",
"tests/test_commands.py::TestRedisCommands::test_pexpire_option_gt",
"tests/test_commands.py::TestRedisCommands::test_pexpire_option_lt",
"tests/test_commands.py::TestRedisCommands::test_pexpireat_datetime",
"tests/test_commands.py::TestRedisCommands::test_pexpireat_no_key",
"tests/test_commands.py::TestRedisCommands::test_pexpireat_unixtime",
"tests/test_commands.py::TestRedisCommands::test_pexpireat_option_nx",
"tests/test_commands.py::TestRedisCommands::test_pexpireat_option_xx",
"tests/test_commands.py::TestRedisCommands::test_pexpireat_option_gt",
"tests/test_commands.py::TestRedisCommands::test_pexpireat_option_lt",
"tests/test_commands.py::TestRedisCommands::test_pexpiretime",
"tests/test_commands.py::TestRedisCommands::test_psetex",
"tests/test_commands.py::TestRedisCommands::test_psetex_timedelta",
"tests/test_commands.py::TestRedisCommands::test_pttl",
"tests/test_commands.py::TestRedisCommands::test_pttl_no_key",
"tests/test_commands.py::TestRedisCommands::test_hrandfield",
"tests/test_commands.py::TestRedisCommands::test_randomkey",
"tests/test_commands.py::TestRedisCommands::test_rename",
"tests/test_commands.py::TestRedisCommands::test_renamenx",
"tests/test_commands.py::TestRedisCommands::test_set_nx",
"tests/test_commands.py::TestRedisCommands::test_set_xx",
"tests/test_commands.py::TestRedisCommands::test_set_px",
"tests/test_commands.py::TestRedisCommands::test_set_px_timedelta",
"tests/test_commands.py::TestRedisCommands::test_set_ex",
"tests/test_commands.py::TestRedisCommands::test_set_ex_timedelta",
"tests/test_commands.py::TestRedisCommands::test_set_exat_timedelta",
"tests/test_commands.py::TestRedisCommands::test_set_pxat_timedelta",
"tests/test_commands.py::TestRedisCommands::test_set_multipleoptions",
"tests/test_commands.py::TestRedisCommands::test_set_keepttl",
"tests/test_commands.py::TestRedisCommands::test_set_get",
"tests/test_commands.py::TestRedisCommands::test_setex",
"tests/test_commands.py::TestRedisCommands::test_setnx",
"tests/test_commands.py::TestRedisCommands::test_setrange",
"tests/test_commands.py::TestRedisCommands::test_strlen",
"tests/test_commands.py::TestRedisCommands::test_substr",
"tests/test_commands.py::TestRedisCommands::test_ttl",
"tests/test_commands.py::TestRedisCommands::test_ttl_nokey",
"tests/test_commands.py::TestRedisCommands::test_type",
"tests/test_commands.py::TestRedisCommands::test_blpop",
"tests/test_commands.py::TestRedisCommands::test_brpop",
"tests/test_commands.py::TestRedisCommands::test_brpoplpush",
"tests/test_commands.py::TestRedisCommands::test_brpoplpush_empty_string",
"tests/test_commands.py::TestRedisCommands::test_blmpop",
"tests/test_commands.py::TestRedisCommands::test_lmpop",
"tests/test_commands.py::TestRedisCommands::test_lindex",
"tests/test_commands.py::TestRedisCommands::test_linsert",
"tests/test_commands.py::TestRedisCommands::test_llen",
"tests/test_commands.py::TestRedisCommands::test_lpop",
"tests/test_commands.py::TestRedisCommands::test_lpop_count",
"tests/test_commands.py::TestRedisCommands::test_lpush",
"tests/test_commands.py::TestRedisCommands::test_lpushx",
"tests/test_commands.py::TestRedisCommands::test_lpushx_with_list",
"tests/test_commands.py::TestRedisCommands::test_lrange",
"tests/test_commands.py::TestRedisCommands::test_lrem",
"tests/test_commands.py::TestRedisCommands::test_lset",
"tests/test_commands.py::TestRedisCommands::test_ltrim",
"tests/test_commands.py::TestRedisCommands::test_rpop",
"tests/test_commands.py::TestRedisCommands::test_rpop_count",
"tests/test_commands.py::TestRedisCommands::test_rpoplpush",
"tests/test_commands.py::TestRedisCommands::test_rpush",
"tests/test_commands.py::TestRedisCommands::test_lpos",
"tests/test_commands.py::TestRedisCommands::test_rpushx",
"tests/test_commands.py::TestRedisCommands::test_scan",
"tests/test_commands.py::TestRedisCommands::test_scan_type",
"tests/test_commands.py::TestRedisCommands::test_scan_iter",
"tests/test_commands.py::TestRedisCommands::test_sscan",
"tests/test_commands.py::TestRedisCommands::test_sscan_iter",
"tests/test_commands.py::TestRedisCommands::test_hscan",
"tests/test_commands.py::TestRedisCommands::test_hscan_iter",
"tests/test_commands.py::TestRedisCommands::test_zscan",
"tests/test_commands.py::TestRedisCommands::test_zscan_iter",
"tests/test_commands.py::TestRedisCommands::test_sadd",
"tests/test_commands.py::TestRedisCommands::test_scard",
"tests/test_commands.py::TestRedisCommands::test_sdiff",
"tests/test_commands.py::TestRedisCommands::test_sdiffstore",
"tests/test_commands.py::TestRedisCommands::test_sinter",
"tests/test_commands.py::TestRedisCommands::test_sintercard",
"tests/test_commands.py::TestRedisCommands::test_sinterstore",
"tests/test_commands.py::TestRedisCommands::test_sismember",
"tests/test_commands.py::TestRedisCommands::test_smembers",
"tests/test_commands.py::TestRedisCommands::test_smismember",
"tests/test_commands.py::TestRedisCommands::test_smove",
"tests/test_commands.py::TestRedisCommands::test_spop",
"tests/test_commands.py::TestRedisCommands::test_spop_multi_value",
"tests/test_commands.py::TestRedisCommands::test_srandmember",
"tests/test_commands.py::TestRedisCommands::test_srandmember_multi_value",
"tests/test_commands.py::TestRedisCommands::test_srem",
"tests/test_commands.py::TestRedisCommands::test_sunion",
"tests/test_commands.py::TestRedisCommands::test_sunionstore",
"tests/test_commands.py::TestRedisCommands::test_debug_segfault",
"tests/test_commands.py::TestRedisCommands::test_script_debug",
"tests/test_commands.py::TestRedisCommands::test_zadd",
"tests/test_commands.py::TestRedisCommands::test_zadd_nx",
"tests/test_commands.py::TestRedisCommands::test_zadd_xx",
"tests/test_commands.py::TestRedisCommands::test_zadd_ch",
"tests/test_commands.py::TestRedisCommands::test_zadd_incr",
"tests/test_commands.py::TestRedisCommands::test_zadd_incr_with_xx",
"tests/test_commands.py::TestRedisCommands::test_zadd_gt_lt",
"tests/test_commands.py::TestRedisCommands::test_zcard",
"tests/test_commands.py::TestRedisCommands::test_zcount",
"tests/test_commands.py::TestRedisCommands::test_zdiff",
"tests/test_commands.py::TestRedisCommands::test_zdiffstore",
"tests/test_commands.py::TestRedisCommands::test_zincrby",
"tests/test_commands.py::TestRedisCommands::test_zlexcount",
"tests/test_commands.py::TestRedisCommands::test_zinter",
"tests/test_commands.py::TestRedisCommands::test_zintercard",
"tests/test_commands.py::TestRedisCommands::test_zinterstore_sum",
"tests/test_commands.py::TestRedisCommands::test_zinterstore_max",
"tests/test_commands.py::TestRedisCommands::test_zinterstore_min",
"tests/test_commands.py::TestRedisCommands::test_zinterstore_with_weight",
"tests/test_commands.py::TestRedisCommands::test_zpopmax",
"tests/test_commands.py::TestRedisCommands::test_zpopmin",
"tests/test_commands.py::TestRedisCommands::test_zrandemember",
"tests/test_commands.py::TestRedisCommands::test_bzpopmax",
"tests/test_commands.py::TestRedisCommands::test_bzpopmin",
"tests/test_commands.py::TestRedisCommands::test_zmpop",
"tests/test_commands.py::TestRedisCommands::test_bzmpop",
"tests/test_commands.py::TestRedisCommands::test_zrange",
"tests/test_commands.py::TestRedisCommands::test_zrange_errors",
"tests/test_commands.py::TestRedisCommands::test_zrange_params",
"tests/test_commands.py::TestRedisCommands::test_zrangestore",
"tests/test_commands.py::TestRedisCommands::test_zrangebylex",
"tests/test_commands.py::TestRedisCommands::test_zrevrangebylex",
"tests/test_commands.py::TestRedisCommands::test_zrangebyscore",
"tests/test_commands.py::TestRedisCommands::test_zrank",
"tests/test_commands.py::TestRedisCommands::test_zrem",
"tests/test_commands.py::TestRedisCommands::test_zrem_multiple_keys",
"tests/test_commands.py::TestRedisCommands::test_zremrangebylex",
"tests/test_commands.py::TestRedisCommands::test_zremrangebyrank",
"tests/test_commands.py::TestRedisCommands::test_zremrangebyscore",
"tests/test_commands.py::TestRedisCommands::test_zrevrange",
"tests/test_commands.py::TestRedisCommands::test_zrevrangebyscore",
"tests/test_commands.py::TestRedisCommands::test_zrevrank",
"tests/test_commands.py::TestRedisCommands::test_zscore",
"tests/test_commands.py::TestRedisCommands::test_zunion",
"tests/test_commands.py::TestRedisCommands::test_zunionstore_sum",
"tests/test_commands.py::TestRedisCommands::test_zunionstore_max",
"tests/test_commands.py::TestRedisCommands::test_zunionstore_min",
"tests/test_commands.py::TestRedisCommands::test_zunionstore_with_weight",
"tests/test_commands.py::TestRedisCommands::test_zmscore",
"tests/test_commands.py::TestRedisCommands::test_pfadd",
"tests/test_commands.py::TestRedisCommands::test_pfcount",
"tests/test_commands.py::TestRedisCommands::test_pfmerge",
"tests/test_commands.py::TestRedisCommands::test_hget_and_hset",
"tests/test_commands.py::TestRedisCommands::test_hset_with_multi_key_values",
"tests/test_commands.py::TestRedisCommands::test_hset_with_key_values_passed_as_list",
"tests/test_commands.py::TestRedisCommands::test_hset_without_data",
"tests/test_commands.py::TestRedisCommands::test_hdel",
"tests/test_commands.py::TestRedisCommands::test_hexists",
"tests/test_commands.py::TestRedisCommands::test_hgetall",
"tests/test_commands.py::TestRedisCommands::test_hincrby",
"tests/test_commands.py::TestRedisCommands::test_hincrbyfloat",
"tests/test_commands.py::TestRedisCommands::test_hkeys",
"tests/test_commands.py::TestRedisCommands::test_hlen",
"tests/test_commands.py::TestRedisCommands::test_hmget",
"tests/test_commands.py::TestRedisCommands::test_hmset",
"tests/test_commands.py::TestRedisCommands::test_hsetnx",
"tests/test_commands.py::TestRedisCommands::test_hvals",
"tests/test_commands.py::TestRedisCommands::test_hstrlen",
"tests/test_commands.py::TestRedisCommands::test_sort_basic",
"tests/test_commands.py::TestRedisCommands::test_sort_limited",
"tests/test_commands.py::TestRedisCommands::test_sort_by",
"tests/test_commands.py::TestRedisCommands::test_sort_get",
"tests/test_commands.py::TestRedisCommands::test_sort_get_multi",
"tests/test_commands.py::TestRedisCommands::test_sort_get_groups_two",
"tests/test_commands.py::TestRedisCommands::test_sort_groups_string_get",
"tests/test_commands.py::TestRedisCommands::test_sort_groups_just_one_get",
"tests/test_commands.py::TestRedisCommands::test_sort_groups_no_get",
"tests/test_commands.py::TestRedisCommands::test_sort_groups_three_gets",
"tests/test_commands.py::TestRedisCommands::test_sort_desc",
"tests/test_commands.py::TestRedisCommands::test_sort_alpha",
"tests/test_commands.py::TestRedisCommands::test_sort_store",
"tests/test_commands.py::TestRedisCommands::test_sort_all_options",
"tests/test_commands.py::TestRedisCommands::test_sort_ro",
"tests/test_commands.py::TestRedisCommands::test_sort_issue_924",
"tests/test_commands.py::TestRedisCommands::test_cluster_addslots",
"tests/test_commands.py::TestRedisCommands::test_cluster_count_failure_reports",
"tests/test_commands.py::TestRedisCommands::test_cluster_countkeysinslot",
"tests/test_commands.py::TestRedisCommands::test_cluster_delslots",
"tests/test_commands.py::TestRedisCommands::test_cluster_failover",
"tests/test_commands.py::TestRedisCommands::test_cluster_forget",
"tests/test_commands.py::TestRedisCommands::test_cluster_info",
"tests/test_commands.py::TestRedisCommands::test_cluster_keyslot",
"tests/test_commands.py::TestRedisCommands::test_cluster_meet",
"tests/test_commands.py::TestRedisCommands::test_cluster_nodes",
"tests/test_commands.py::TestRedisCommands::test_cluster_replicate",
"tests/test_commands.py::TestRedisCommands::test_cluster_reset",
"tests/test_commands.py::TestRedisCommands::test_cluster_saveconfig",
"tests/test_commands.py::TestRedisCommands::test_cluster_setslot",
"tests/test_commands.py::TestRedisCommands::test_cluster_slaves",
"tests/test_commands.py::TestRedisCommands::test_readonly_invalid_cluster_state",
"tests/test_commands.py::TestRedisCommands::test_readonly",
"tests/test_commands.py::TestRedisCommands::test_geoadd",
"tests/test_commands.py::TestRedisCommands::test_geoadd_nx",
"tests/test_commands.py::TestRedisCommands::test_geoadd_xx",
"tests/test_commands.py::TestRedisCommands::test_geoadd_ch",
"tests/test_commands.py::TestRedisCommands::test_geoadd_invalid_params",
"tests/test_commands.py::TestRedisCommands::test_geodist",
"tests/test_commands.py::TestRedisCommands::test_geodist_units",
"tests/test_commands.py::TestRedisCommands::test_geodist_missing_one_member",
"tests/test_commands.py::TestRedisCommands::test_geodist_invalid_units",
"tests/test_commands.py::TestRedisCommands::test_geohash",
"tests/test_commands.py::TestRedisCommands::test_geopos",
"tests/test_commands.py::TestRedisCommands::test_geopos_no_value",
"tests/test_commands.py::TestRedisCommands::test_geosearch",
"tests/test_commands.py::TestRedisCommands::test_geosearch_member",
"tests/test_commands.py::TestRedisCommands::test_geosearch_sort",
"tests/test_commands.py::TestRedisCommands::test_geosearch_with",
"tests/test_commands.py::TestRedisCommands::test_geosearch_negative",
"tests/test_commands.py::TestRedisCommands::test_geosearchstore",
"tests/test_commands.py::TestRedisCommands::test_geosearchstore_dist",
"tests/test_commands.py::TestRedisCommands::test_georadius",
"tests/test_commands.py::TestRedisCommands::test_georadius_no_values",
"tests/test_commands.py::TestRedisCommands::test_georadius_units",
"tests/test_commands.py::TestRedisCommands::test_georadius_with",
"tests/test_commands.py::TestRedisCommands::test_georadius_count",
"tests/test_commands.py::TestRedisCommands::test_georadius_sort",
"tests/test_commands.py::TestRedisCommands::test_georadius_store",
"tests/test_commands.py::TestRedisCommands::test_georadius_store_dist",
"tests/test_commands.py::TestRedisCommands::test_georadiusmember",
"tests/test_commands.py::TestRedisCommands::test_georadiusmember_count",
"tests/test_commands.py::TestRedisCommands::test_xack",
"tests/test_commands.py::TestRedisCommands::test_xadd",
"tests/test_commands.py::TestRedisCommands::test_xadd_nomkstream",
"tests/test_commands.py::TestRedisCommands::test_xadd_minlen_and_limit",
"tests/test_commands.py::TestRedisCommands::test_xadd_explicit_ms",
"tests/test_commands.py::TestRedisCommands::test_xautoclaim_negative",
"tests/test_commands.py::TestRedisCommands::test_xclaim",
"tests/test_commands.py::TestRedisCommands::test_xclaim_trimmed",
"tests/test_commands.py::TestRedisCommands::test_xdel",
"tests/test_commands.py::TestRedisCommands::test_xgroup_create",
"tests/test_commands.py::TestRedisCommands::test_xgroup_create_mkstream",
"tests/test_commands.py::TestRedisCommands::test_xgroup_create_entriesread",
"tests/test_commands.py::TestRedisCommands::test_xgroup_delconsumer",
"tests/test_commands.py::TestRedisCommands::test_xgroup_createconsumer",
"tests/test_commands.py::TestRedisCommands::test_xgroup_destroy",
"tests/test_commands.py::TestRedisCommands::test_xgroup_setid",
"tests/test_commands.py::TestRedisCommands::test_xinfo_stream",
"tests/test_commands.py::TestRedisCommands::test_xinfo_stream_full",
"tests/test_commands.py::TestRedisCommands::test_xlen",
"tests/test_commands.py::TestRedisCommands::test_xpending",
"tests/test_commands.py::TestRedisCommands::test_xpending_range",
"tests/test_commands.py::TestRedisCommands::test_xpending_range_idle",
"tests/test_commands.py::TestRedisCommands::test_xpending_range_negative",
"tests/test_commands.py::TestRedisCommands::test_xrange",
"tests/test_commands.py::TestRedisCommands::test_xread",
"tests/test_commands.py::TestRedisCommands::test_xreadgroup",
"tests/test_commands.py::TestRedisCommands::test_xrevrange",
"tests/test_commands.py::TestRedisCommands::test_xtrim",
"tests/test_commands.py::TestRedisCommands::test_xtrim_minlen_and_length_args",
"tests/test_commands.py::TestRedisCommands::test_bitfield_operations",
"tests/test_commands.py::TestRedisCommands::test_bitfield_ro",
"tests/test_commands.py::TestRedisCommands::test_memory_help",
"tests/test_commands.py::TestRedisCommands::test_memory_doctor",
"tests/test_commands.py::TestRedisCommands::test_memory_malloc_stats",
"tests/test_commands.py::TestRedisCommands::test_memory_stats",
"tests/test_commands.py::TestRedisCommands::test_memory_usage",
"tests/test_commands.py::TestRedisCommands::test_latency_histogram_not_implemented",
"tests/test_commands.py::TestRedisCommands::test_module_list",
"tests/test_commands.py::TestRedisCommands::test_command_count",
"tests/test_commands.py::TestRedisCommands::test_command_docs",
"tests/test_commands.py::TestRedisCommands::test_command_list",
"tests/test_commands.py::TestRedisCommands::test_command_getkeys",
"tests/test_commands.py::TestRedisCommands::test_command",
"tests/test_commands.py::TestRedisCommands::test_command_getkeysandflags",
"tests/test_commands.py::TestRedisCommands::test_module",
"tests/test_commands.py::TestRedisCommands::test_module_loadex",
"tests/test_commands.py::TestRedisCommands::test_restore",
"tests/test_commands.py::TestRedisCommands::test_restore_idletime",
"tests/test_commands.py::TestRedisCommands::test_restore_frequency",
"tests/test_commands.py::TestRedisCommands::test_replicaof",
"tests/test_commands.py::TestRedisCommands::test_shutdown",
"tests/test_commands.py::TestRedisCommands::test_shutdown_with_params",
"tests/test_commands.py::TestRedisCommands::test_psync",
"tests/test_commands.py::TestBinarySave::test_binary_get_set",
"tests/test_commands.py::TestBinarySave::test_binary_lists",
"tests/test_commands.py::TestBinarySave::test_22_info",
"tests/test_commands.py::TestBinarySave::test_large_responses",
"tests/test_commands.py::TestBinarySave::test_floating_point_encoding"
] | [
"tests/test_commands.py::TestRedisCommands::test_latency_graph_not_implemented",
"tests/test_commands.py::TestRedisCommands::test_latency_doctor_not_implemented"
] | Python | [] | [] |
|
redis/redis-py | 2,503 | redis__redis-py-2503 | [
"1982"
] | 3a121bef7bbc5bb5f07b119b0eef2f7527a38eda | diff --git a/redis/cluster.py b/redis/cluster.py
--- a/redis/cluster.py
+++ b/redis/cluster.py
@@ -265,6 +265,9 @@ class AbstractRedisCluster:
"READWRITE",
"TIME",
"GRAPH.CONFIG",
+ "LATENCY HISTORY",
+ "LATENCY LATEST",
+ "LATENCY RESET",
],
DEFAULT_NODE,
),
diff --git a/redis/commands/core.py b/redis/commands/core.py
--- a/redis/commands/core.py
+++ b/redis/commands/core.py
@@ -1151,6 +1151,30 @@ def latency_histogram(self, *args):
"LATENCY HISTOGRAM is intentionally not implemented in the client."
)
+ def latency_history(self, event: str) -> ResponseT:
+ """
+ Returns the raw data of the ``event``'s latency spikes time series.
+
+ For more information see https://redis.io/commands/latency-history
+ """
+ return self.execute_command("LATENCY HISTORY", event)
+
+ def latency_latest(self) -> ResponseT:
+ """
+ Reports the latest latency events logged.
+
+ For more information see https://redis.io/commands/latency-latest
+ """
+ return self.execute_command("LATENCY LATEST")
+
+ def latency_reset(self, *events: str) -> ResponseT:
+ """
+ Resets the latency spikes time series of all, or only some, events.
+
+ For more information see https://redis.io/commands/latency-reset
+ """
+ return self.execute_command("LATENCY RESET", *events)
+
def ping(self, **kwargs) -> ResponseT:
"""
Ping the Redis server
| diff --git a/tests/test_commands.py b/tests/test_commands.py
--- a/tests/test_commands.py
+++ b/tests/test_commands.py
@@ -4527,16 +4527,23 @@ def test_latency_histogram_not_implemented(self, r: redis.Redis):
with pytest.raises(NotImplementedError):
r.latency_histogram()
- @skip_if_server_version_lt("7.0.0")
def test_latency_graph_not_implemented(self, r: redis.Redis):
with pytest.raises(NotImplementedError):
r.latency_graph()
- @skip_if_server_version_lt("7.0.0")
def test_latency_doctor_not_implemented(self, r: redis.Redis):
with pytest.raises(NotImplementedError):
r.latency_doctor()
+ def test_latency_history(self, r: redis.Redis):
+ assert r.latency_history("command") == []
+
+ def test_latency_latest(self, r: redis.Redis):
+ assert r.latency_latest() == []
+
+ def test_latency_reset(self, r: redis.Redis):
+ assert r.latency_reset() == 0
+
@pytest.mark.onlynoncluster
@skip_if_server_version_lt("4.0.0")
@skip_if_redis_enterprise()
| Add support for missing command LATENCY RESET
| "2022-12-12T12:53:19Z" | 4.4 | [
"tests/test_commands.py::TestResponseCallbacks::test_response_callbacks",
"tests/test_commands.py::TestResponseCallbacks::test_case_insensitive_command_names",
"tests/test_commands.py::TestRedisCommands::test_auth",
"tests/test_commands.py::TestRedisCommands::test_command_on_invalid_key_type",
"tests/test_commands.py::TestRedisCommands::test_acl_cat_no_category",
"tests/test_commands.py::TestRedisCommands::test_acl_cat_with_category",
"tests/test_commands.py::TestRedisCommands::test_acl_deluser",
"tests/test_commands.py::TestRedisCommands::test_acl_genpass",
"tests/test_commands.py::TestRedisCommands::test_acl_help",
"tests/test_commands.py::TestRedisCommands::test_acl_list",
"tests/test_commands.py::TestRedisCommands::test_acl_log",
"tests/test_commands.py::TestRedisCommands::test_acl_setuser_categories_without_prefix_fails",
"tests/test_commands.py::TestRedisCommands::test_acl_setuser_commands_without_prefix_fails",
"tests/test_commands.py::TestRedisCommands::test_acl_setuser_add_passwords_and_nopass_fails",
"tests/test_commands.py::TestRedisCommands::test_acl_users",
"tests/test_commands.py::TestRedisCommands::test_acl_whoami",
"tests/test_commands.py::TestRedisCommands::test_client_list",
"tests/test_commands.py::TestRedisCommands::test_client_info",
"tests/test_commands.py::TestRedisCommands::test_client_list_types_not_replica",
"tests/test_commands.py::TestRedisCommands::test_client_list_replica",
"tests/test_commands.py::TestRedisCommands::test_client_list_client_id",
"tests/test_commands.py::TestRedisCommands::test_client_id",
"tests/test_commands.py::TestRedisCommands::test_client_trackinginfo",
"tests/test_commands.py::TestRedisCommands::test_client_tracking",
"tests/test_commands.py::TestRedisCommands::test_client_unblock",
"tests/test_commands.py::TestRedisCommands::test_client_getname",
"tests/test_commands.py::TestRedisCommands::test_client_setname",
"tests/test_commands.py::TestRedisCommands::test_client_kill",
"tests/test_commands.py::TestRedisCommands::test_client_kill_filter_invalid_params",
"tests/test_commands.py::TestRedisCommands::test_client_kill_filter_by_id",
"tests/test_commands.py::TestRedisCommands::test_client_kill_filter_by_addr",
"tests/test_commands.py::TestRedisCommands::test_client_list_after_client_setname",
"tests/test_commands.py::TestRedisCommands::test_client_kill_filter_by_laddr",
"tests/test_commands.py::TestRedisCommands::test_client_kill_filter_by_user",
"tests/test_commands.py::TestRedisCommands::test_client_pause",
"tests/test_commands.py::TestRedisCommands::test_client_pause_all",
"tests/test_commands.py::TestRedisCommands::test_client_unpause",
"tests/test_commands.py::TestRedisCommands::test_client_no_evict",
"tests/test_commands.py::TestRedisCommands::test_client_reply",
"tests/test_commands.py::TestRedisCommands::test_client_getredir",
"tests/test_commands.py::TestRedisCommands::test_hello_notI_implemented",
"tests/test_commands.py::TestRedisCommands::test_config_get",
"tests/test_commands.py::TestRedisCommands::test_config_get_multi_params",
"tests/test_commands.py::TestRedisCommands::test_config_resetstat",
"tests/test_commands.py::TestRedisCommands::test_config_set",
"tests/test_commands.py::TestRedisCommands::test_config_set_multi_params",
"tests/test_commands.py::TestRedisCommands::test_failover",
"tests/test_commands.py::TestRedisCommands::test_dbsize",
"tests/test_commands.py::TestRedisCommands::test_echo",
"tests/test_commands.py::TestRedisCommands::test_info",
"tests/test_commands.py::TestRedisCommands::test_info_multi_sections",
"tests/test_commands.py::TestRedisCommands::test_lastsave",
"tests/test_commands.py::TestRedisCommands::test_lolwut",
"tests/test_commands.py::TestRedisCommands::test_reset",
"tests/test_commands.py::TestRedisCommands::test_object",
"tests/test_commands.py::TestRedisCommands::test_ping",
"tests/test_commands.py::TestRedisCommands::test_quit",
"tests/test_commands.py::TestRedisCommands::test_role",
"tests/test_commands.py::TestRedisCommands::test_select",
"tests/test_commands.py::TestRedisCommands::test_slowlog_get",
"tests/test_commands.py::TestRedisCommands::test_slowlog_get_limit",
"tests/test_commands.py::TestRedisCommands::test_slowlog_length",
"tests/test_commands.py::TestRedisCommands::test_time",
"tests/test_commands.py::TestRedisCommands::test_bgsave",
"tests/test_commands.py::TestRedisCommands::test_never_decode_option",
"tests/test_commands.py::TestRedisCommands::test_empty_response_option",
"tests/test_commands.py::TestRedisCommands::test_append",
"tests/test_commands.py::TestRedisCommands::test_bitcount",
"tests/test_commands.py::TestRedisCommands::test_bitcount_mode",
"tests/test_commands.py::TestRedisCommands::test_bitop_not_empty_string",
"tests/test_commands.py::TestRedisCommands::test_bitop_not",
"tests/test_commands.py::TestRedisCommands::test_bitop_not_in_place",
"tests/test_commands.py::TestRedisCommands::test_bitop_single_string",
"tests/test_commands.py::TestRedisCommands::test_bitop_string_operands",
"tests/test_commands.py::TestRedisCommands::test_bitpos",
"tests/test_commands.py::TestRedisCommands::test_bitpos_wrong_arguments",
"tests/test_commands.py::TestRedisCommands::test_bitpos_mode",
"tests/test_commands.py::TestRedisCommands::test_copy",
"tests/test_commands.py::TestRedisCommands::test_copy_and_replace",
"tests/test_commands.py::TestRedisCommands::test_copy_to_another_database",
"tests/test_commands.py::TestRedisCommands::test_decr",
"tests/test_commands.py::TestRedisCommands::test_decrby",
"tests/test_commands.py::TestRedisCommands::test_delete",
"tests/test_commands.py::TestRedisCommands::test_delete_with_multiple_keys",
"tests/test_commands.py::TestRedisCommands::test_delitem",
"tests/test_commands.py::TestRedisCommands::test_unlink",
"tests/test_commands.py::TestRedisCommands::test_unlink_with_multiple_keys",
"tests/test_commands.py::TestRedisCommands::test_lcs",
"tests/test_commands.py::TestRedisCommands::test_dump_and_restore",
"tests/test_commands.py::TestRedisCommands::test_dump_and_restore_and_replace",
"tests/test_commands.py::TestRedisCommands::test_dump_and_restore_absttl",
"tests/test_commands.py::TestRedisCommands::test_exists",
"tests/test_commands.py::TestRedisCommands::test_exists_contains",
"tests/test_commands.py::TestRedisCommands::test_expire",
"tests/test_commands.py::TestRedisCommands::test_expire_option_nx",
"tests/test_commands.py::TestRedisCommands::test_expire_option_xx",
"tests/test_commands.py::TestRedisCommands::test_expire_option_gt",
"tests/test_commands.py::TestRedisCommands::test_expire_option_lt",
"tests/test_commands.py::TestRedisCommands::test_expireat_datetime",
"tests/test_commands.py::TestRedisCommands::test_expireat_no_key",
"tests/test_commands.py::TestRedisCommands::test_expireat_unixtime",
"tests/test_commands.py::TestRedisCommands::test_expiretime",
"tests/test_commands.py::TestRedisCommands::test_expireat_option_nx",
"tests/test_commands.py::TestRedisCommands::test_expireat_option_xx",
"tests/test_commands.py::TestRedisCommands::test_expireat_option_gt",
"tests/test_commands.py::TestRedisCommands::test_expireat_option_lt",
"tests/test_commands.py::TestRedisCommands::test_get_and_set",
"tests/test_commands.py::TestRedisCommands::test_getdel",
"tests/test_commands.py::TestRedisCommands::test_getex",
"tests/test_commands.py::TestRedisCommands::test_getitem_and_setitem",
"tests/test_commands.py::TestRedisCommands::test_getitem_raises_keyerror_for_missing_key",
"tests/test_commands.py::TestRedisCommands::test_getitem_does_not_raise_keyerror_for_empty_string",
"tests/test_commands.py::TestRedisCommands::test_get_set_bit",
"tests/test_commands.py::TestRedisCommands::test_getrange",
"tests/test_commands.py::TestRedisCommands::test_getset",
"tests/test_commands.py::TestRedisCommands::test_incr",
"tests/test_commands.py::TestRedisCommands::test_incrby",
"tests/test_commands.py::TestRedisCommands::test_incrbyfloat",
"tests/test_commands.py::TestRedisCommands::test_keys",
"tests/test_commands.py::TestRedisCommands::test_mget",
"tests/test_commands.py::TestRedisCommands::test_lmove",
"tests/test_commands.py::TestRedisCommands::test_blmove",
"tests/test_commands.py::TestRedisCommands::test_mset",
"tests/test_commands.py::TestRedisCommands::test_msetnx",
"tests/test_commands.py::TestRedisCommands::test_pexpire",
"tests/test_commands.py::TestRedisCommands::test_pexpire_option_nx",
"tests/test_commands.py::TestRedisCommands::test_pexpire_option_xx",
"tests/test_commands.py::TestRedisCommands::test_pexpire_option_gt",
"tests/test_commands.py::TestRedisCommands::test_pexpire_option_lt",
"tests/test_commands.py::TestRedisCommands::test_pexpireat_datetime",
"tests/test_commands.py::TestRedisCommands::test_pexpireat_no_key",
"tests/test_commands.py::TestRedisCommands::test_pexpireat_unixtime",
"tests/test_commands.py::TestRedisCommands::test_pexpireat_option_nx",
"tests/test_commands.py::TestRedisCommands::test_pexpireat_option_xx",
"tests/test_commands.py::TestRedisCommands::test_pexpireat_option_gt",
"tests/test_commands.py::TestRedisCommands::test_pexpireat_option_lt",
"tests/test_commands.py::TestRedisCommands::test_pexpiretime",
"tests/test_commands.py::TestRedisCommands::test_psetex",
"tests/test_commands.py::TestRedisCommands::test_psetex_timedelta",
"tests/test_commands.py::TestRedisCommands::test_pttl",
"tests/test_commands.py::TestRedisCommands::test_pttl_no_key",
"tests/test_commands.py::TestRedisCommands::test_hrandfield",
"tests/test_commands.py::TestRedisCommands::test_randomkey",
"tests/test_commands.py::TestRedisCommands::test_rename",
"tests/test_commands.py::TestRedisCommands::test_renamenx",
"tests/test_commands.py::TestRedisCommands::test_set_nx",
"tests/test_commands.py::TestRedisCommands::test_set_xx",
"tests/test_commands.py::TestRedisCommands::test_set_px",
"tests/test_commands.py::TestRedisCommands::test_set_px_timedelta",
"tests/test_commands.py::TestRedisCommands::test_set_ex",
"tests/test_commands.py::TestRedisCommands::test_set_ex_timedelta",
"tests/test_commands.py::TestRedisCommands::test_set_exat_timedelta",
"tests/test_commands.py::TestRedisCommands::test_set_pxat_timedelta",
"tests/test_commands.py::TestRedisCommands::test_set_multipleoptions",
"tests/test_commands.py::TestRedisCommands::test_set_keepttl",
"tests/test_commands.py::TestRedisCommands::test_set_get",
"tests/test_commands.py::TestRedisCommands::test_setex",
"tests/test_commands.py::TestRedisCommands::test_setnx",
"tests/test_commands.py::TestRedisCommands::test_setrange",
"tests/test_commands.py::TestRedisCommands::test_strlen",
"tests/test_commands.py::TestRedisCommands::test_substr",
"tests/test_commands.py::TestRedisCommands::test_ttl",
"tests/test_commands.py::TestRedisCommands::test_ttl_nokey",
"tests/test_commands.py::TestRedisCommands::test_type",
"tests/test_commands.py::TestRedisCommands::test_blpop",
"tests/test_commands.py::TestRedisCommands::test_brpop",
"tests/test_commands.py::TestRedisCommands::test_brpoplpush",
"tests/test_commands.py::TestRedisCommands::test_brpoplpush_empty_string",
"tests/test_commands.py::TestRedisCommands::test_blmpop",
"tests/test_commands.py::TestRedisCommands::test_lmpop",
"tests/test_commands.py::TestRedisCommands::test_lindex",
"tests/test_commands.py::TestRedisCommands::test_linsert",
"tests/test_commands.py::TestRedisCommands::test_llen",
"tests/test_commands.py::TestRedisCommands::test_lpop",
"tests/test_commands.py::TestRedisCommands::test_lpop_count",
"tests/test_commands.py::TestRedisCommands::test_lpush",
"tests/test_commands.py::TestRedisCommands::test_lpushx",
"tests/test_commands.py::TestRedisCommands::test_lpushx_with_list",
"tests/test_commands.py::TestRedisCommands::test_lrange",
"tests/test_commands.py::TestRedisCommands::test_lrem",
"tests/test_commands.py::TestRedisCommands::test_lset",
"tests/test_commands.py::TestRedisCommands::test_ltrim",
"tests/test_commands.py::TestRedisCommands::test_rpop",
"tests/test_commands.py::TestRedisCommands::test_rpop_count",
"tests/test_commands.py::TestRedisCommands::test_rpoplpush",
"tests/test_commands.py::TestRedisCommands::test_rpush",
"tests/test_commands.py::TestRedisCommands::test_lpos",
"tests/test_commands.py::TestRedisCommands::test_rpushx",
"tests/test_commands.py::TestRedisCommands::test_scan",
"tests/test_commands.py::TestRedisCommands::test_scan_type",
"tests/test_commands.py::TestRedisCommands::test_scan_iter",
"tests/test_commands.py::TestRedisCommands::test_sscan",
"tests/test_commands.py::TestRedisCommands::test_sscan_iter",
"tests/test_commands.py::TestRedisCommands::test_hscan",
"tests/test_commands.py::TestRedisCommands::test_hscan_iter",
"tests/test_commands.py::TestRedisCommands::test_zscan",
"tests/test_commands.py::TestRedisCommands::test_zscan_iter",
"tests/test_commands.py::TestRedisCommands::test_sadd",
"tests/test_commands.py::TestRedisCommands::test_scard",
"tests/test_commands.py::TestRedisCommands::test_sdiff",
"tests/test_commands.py::TestRedisCommands::test_sdiffstore",
"tests/test_commands.py::TestRedisCommands::test_sinter",
"tests/test_commands.py::TestRedisCommands::test_sintercard",
"tests/test_commands.py::TestRedisCommands::test_sinterstore",
"tests/test_commands.py::TestRedisCommands::test_sismember",
"tests/test_commands.py::TestRedisCommands::test_smembers",
"tests/test_commands.py::TestRedisCommands::test_smismember",
"tests/test_commands.py::TestRedisCommands::test_smove",
"tests/test_commands.py::TestRedisCommands::test_spop",
"tests/test_commands.py::TestRedisCommands::test_spop_multi_value",
"tests/test_commands.py::TestRedisCommands::test_srandmember",
"tests/test_commands.py::TestRedisCommands::test_srandmember_multi_value",
"tests/test_commands.py::TestRedisCommands::test_srem",
"tests/test_commands.py::TestRedisCommands::test_sunion",
"tests/test_commands.py::TestRedisCommands::test_sunionstore",
"tests/test_commands.py::TestRedisCommands::test_debug_segfault",
"tests/test_commands.py::TestRedisCommands::test_script_debug",
"tests/test_commands.py::TestRedisCommands::test_zadd",
"tests/test_commands.py::TestRedisCommands::test_zadd_nx",
"tests/test_commands.py::TestRedisCommands::test_zadd_xx",
"tests/test_commands.py::TestRedisCommands::test_zadd_ch",
"tests/test_commands.py::TestRedisCommands::test_zadd_incr",
"tests/test_commands.py::TestRedisCommands::test_zadd_incr_with_xx",
"tests/test_commands.py::TestRedisCommands::test_zadd_gt_lt",
"tests/test_commands.py::TestRedisCommands::test_zcard",
"tests/test_commands.py::TestRedisCommands::test_zcount",
"tests/test_commands.py::TestRedisCommands::test_zdiff",
"tests/test_commands.py::TestRedisCommands::test_zdiffstore",
"tests/test_commands.py::TestRedisCommands::test_zincrby",
"tests/test_commands.py::TestRedisCommands::test_zlexcount",
"tests/test_commands.py::TestRedisCommands::test_zinter",
"tests/test_commands.py::TestRedisCommands::test_zintercard",
"tests/test_commands.py::TestRedisCommands::test_zinterstore_sum",
"tests/test_commands.py::TestRedisCommands::test_zinterstore_max",
"tests/test_commands.py::TestRedisCommands::test_zinterstore_min",
"tests/test_commands.py::TestRedisCommands::test_zinterstore_with_weight",
"tests/test_commands.py::TestRedisCommands::test_zpopmax",
"tests/test_commands.py::TestRedisCommands::test_zpopmin",
"tests/test_commands.py::TestRedisCommands::test_zrandemember",
"tests/test_commands.py::TestRedisCommands::test_bzpopmax",
"tests/test_commands.py::TestRedisCommands::test_bzpopmin",
"tests/test_commands.py::TestRedisCommands::test_zmpop",
"tests/test_commands.py::TestRedisCommands::test_bzmpop",
"tests/test_commands.py::TestRedisCommands::test_zrange",
"tests/test_commands.py::TestRedisCommands::test_zrange_errors",
"tests/test_commands.py::TestRedisCommands::test_zrange_params",
"tests/test_commands.py::TestRedisCommands::test_zrangestore",
"tests/test_commands.py::TestRedisCommands::test_zrangebylex",
"tests/test_commands.py::TestRedisCommands::test_zrevrangebylex",
"tests/test_commands.py::TestRedisCommands::test_zrangebyscore",
"tests/test_commands.py::TestRedisCommands::test_zrank",
"tests/test_commands.py::TestRedisCommands::test_zrem",
"tests/test_commands.py::TestRedisCommands::test_zrem_multiple_keys",
"tests/test_commands.py::TestRedisCommands::test_zremrangebylex",
"tests/test_commands.py::TestRedisCommands::test_zremrangebyrank",
"tests/test_commands.py::TestRedisCommands::test_zremrangebyscore",
"tests/test_commands.py::TestRedisCommands::test_zrevrange",
"tests/test_commands.py::TestRedisCommands::test_zrevrangebyscore",
"tests/test_commands.py::TestRedisCommands::test_zrevrank",
"tests/test_commands.py::TestRedisCommands::test_zscore",
"tests/test_commands.py::TestRedisCommands::test_zunion",
"tests/test_commands.py::TestRedisCommands::test_zunionstore_sum",
"tests/test_commands.py::TestRedisCommands::test_zunionstore_max",
"tests/test_commands.py::TestRedisCommands::test_zunionstore_min",
"tests/test_commands.py::TestRedisCommands::test_zunionstore_with_weight",
"tests/test_commands.py::TestRedisCommands::test_zmscore",
"tests/test_commands.py::TestRedisCommands::test_pfadd",
"tests/test_commands.py::TestRedisCommands::test_pfcount",
"tests/test_commands.py::TestRedisCommands::test_pfmerge",
"tests/test_commands.py::TestRedisCommands::test_hget_and_hset",
"tests/test_commands.py::TestRedisCommands::test_hset_with_multi_key_values",
"tests/test_commands.py::TestRedisCommands::test_hset_with_key_values_passed_as_list",
"tests/test_commands.py::TestRedisCommands::test_hset_without_data",
"tests/test_commands.py::TestRedisCommands::test_hdel",
"tests/test_commands.py::TestRedisCommands::test_hexists",
"tests/test_commands.py::TestRedisCommands::test_hgetall",
"tests/test_commands.py::TestRedisCommands::test_hincrby",
"tests/test_commands.py::TestRedisCommands::test_hincrbyfloat",
"tests/test_commands.py::TestRedisCommands::test_hkeys",
"tests/test_commands.py::TestRedisCommands::test_hlen",
"tests/test_commands.py::TestRedisCommands::test_hmget",
"tests/test_commands.py::TestRedisCommands::test_hmset",
"tests/test_commands.py::TestRedisCommands::test_hsetnx",
"tests/test_commands.py::TestRedisCommands::test_hvals",
"tests/test_commands.py::TestRedisCommands::test_hstrlen",
"tests/test_commands.py::TestRedisCommands::test_sort_basic",
"tests/test_commands.py::TestRedisCommands::test_sort_limited",
"tests/test_commands.py::TestRedisCommands::test_sort_by",
"tests/test_commands.py::TestRedisCommands::test_sort_get",
"tests/test_commands.py::TestRedisCommands::test_sort_get_multi",
"tests/test_commands.py::TestRedisCommands::test_sort_get_groups_two",
"tests/test_commands.py::TestRedisCommands::test_sort_groups_string_get",
"tests/test_commands.py::TestRedisCommands::test_sort_groups_just_one_get",
"tests/test_commands.py::TestRedisCommands::test_sort_groups_no_get",
"tests/test_commands.py::TestRedisCommands::test_sort_groups_three_gets",
"tests/test_commands.py::TestRedisCommands::test_sort_desc",
"tests/test_commands.py::TestRedisCommands::test_sort_alpha",
"tests/test_commands.py::TestRedisCommands::test_sort_store",
"tests/test_commands.py::TestRedisCommands::test_sort_all_options",
"tests/test_commands.py::TestRedisCommands::test_sort_ro",
"tests/test_commands.py::TestRedisCommands::test_sort_issue_924",
"tests/test_commands.py::TestRedisCommands::test_cluster_addslots",
"tests/test_commands.py::TestRedisCommands::test_cluster_count_failure_reports",
"tests/test_commands.py::TestRedisCommands::test_cluster_countkeysinslot",
"tests/test_commands.py::TestRedisCommands::test_cluster_delslots",
"tests/test_commands.py::TestRedisCommands::test_cluster_failover",
"tests/test_commands.py::TestRedisCommands::test_cluster_forget",
"tests/test_commands.py::TestRedisCommands::test_cluster_info",
"tests/test_commands.py::TestRedisCommands::test_cluster_keyslot",
"tests/test_commands.py::TestRedisCommands::test_cluster_meet",
"tests/test_commands.py::TestRedisCommands::test_cluster_nodes",
"tests/test_commands.py::TestRedisCommands::test_cluster_replicate",
"tests/test_commands.py::TestRedisCommands::test_cluster_reset",
"tests/test_commands.py::TestRedisCommands::test_cluster_saveconfig",
"tests/test_commands.py::TestRedisCommands::test_cluster_setslot",
"tests/test_commands.py::TestRedisCommands::test_cluster_slaves",
"tests/test_commands.py::TestRedisCommands::test_readonly_invalid_cluster_state",
"tests/test_commands.py::TestRedisCommands::test_readonly",
"tests/test_commands.py::TestRedisCommands::test_geoadd",
"tests/test_commands.py::TestRedisCommands::test_geoadd_nx",
"tests/test_commands.py::TestRedisCommands::test_geoadd_xx",
"tests/test_commands.py::TestRedisCommands::test_geoadd_ch",
"tests/test_commands.py::TestRedisCommands::test_geoadd_invalid_params",
"tests/test_commands.py::TestRedisCommands::test_geodist",
"tests/test_commands.py::TestRedisCommands::test_geodist_units",
"tests/test_commands.py::TestRedisCommands::test_geodist_missing_one_member",
"tests/test_commands.py::TestRedisCommands::test_geodist_invalid_units",
"tests/test_commands.py::TestRedisCommands::test_geohash",
"tests/test_commands.py::TestRedisCommands::test_geopos",
"tests/test_commands.py::TestRedisCommands::test_geopos_no_value",
"tests/test_commands.py::TestRedisCommands::test_geosearch",
"tests/test_commands.py::TestRedisCommands::test_geosearch_member",
"tests/test_commands.py::TestRedisCommands::test_geosearch_sort",
"tests/test_commands.py::TestRedisCommands::test_geosearch_with",
"tests/test_commands.py::TestRedisCommands::test_geosearch_negative",
"tests/test_commands.py::TestRedisCommands::test_geosearchstore",
"tests/test_commands.py::TestRedisCommands::test_geosearchstore_dist",
"tests/test_commands.py::TestRedisCommands::test_georadius",
"tests/test_commands.py::TestRedisCommands::test_georadius_no_values",
"tests/test_commands.py::TestRedisCommands::test_georadius_units",
"tests/test_commands.py::TestRedisCommands::test_georadius_with",
"tests/test_commands.py::TestRedisCommands::test_georadius_count",
"tests/test_commands.py::TestRedisCommands::test_georadius_sort",
"tests/test_commands.py::TestRedisCommands::test_georadius_store",
"tests/test_commands.py::TestRedisCommands::test_georadius_store_dist",
"tests/test_commands.py::TestRedisCommands::test_georadiusmember",
"tests/test_commands.py::TestRedisCommands::test_georadiusmember_count",
"tests/test_commands.py::TestRedisCommands::test_xack",
"tests/test_commands.py::TestRedisCommands::test_xadd",
"tests/test_commands.py::TestRedisCommands::test_xadd_nomkstream",
"tests/test_commands.py::TestRedisCommands::test_xadd_minlen_and_limit",
"tests/test_commands.py::TestRedisCommands::test_xadd_explicit_ms",
"tests/test_commands.py::TestRedisCommands::test_xautoclaim_negative",
"tests/test_commands.py::TestRedisCommands::test_xclaim",
"tests/test_commands.py::TestRedisCommands::test_xclaim_trimmed",
"tests/test_commands.py::TestRedisCommands::test_xdel",
"tests/test_commands.py::TestRedisCommands::test_xgroup_create",
"tests/test_commands.py::TestRedisCommands::test_xgroup_create_mkstream",
"tests/test_commands.py::TestRedisCommands::test_xgroup_create_entriesread",
"tests/test_commands.py::TestRedisCommands::test_xgroup_delconsumer",
"tests/test_commands.py::TestRedisCommands::test_xgroup_createconsumer",
"tests/test_commands.py::TestRedisCommands::test_xgroup_destroy",
"tests/test_commands.py::TestRedisCommands::test_xgroup_setid",
"tests/test_commands.py::TestRedisCommands::test_xinfo_stream",
"tests/test_commands.py::TestRedisCommands::test_xinfo_stream_full",
"tests/test_commands.py::TestRedisCommands::test_xlen",
"tests/test_commands.py::TestRedisCommands::test_xpending",
"tests/test_commands.py::TestRedisCommands::test_xpending_range",
"tests/test_commands.py::TestRedisCommands::test_xpending_range_idle",
"tests/test_commands.py::TestRedisCommands::test_xpending_range_negative",
"tests/test_commands.py::TestRedisCommands::test_xrange",
"tests/test_commands.py::TestRedisCommands::test_xread",
"tests/test_commands.py::TestRedisCommands::test_xreadgroup",
"tests/test_commands.py::TestRedisCommands::test_xrevrange",
"tests/test_commands.py::TestRedisCommands::test_xtrim",
"tests/test_commands.py::TestRedisCommands::test_xtrim_minlen_and_length_args",
"tests/test_commands.py::TestRedisCommands::test_bitfield_operations",
"tests/test_commands.py::TestRedisCommands::test_bitfield_ro",
"tests/test_commands.py::TestRedisCommands::test_memory_help",
"tests/test_commands.py::TestRedisCommands::test_memory_doctor",
"tests/test_commands.py::TestRedisCommands::test_memory_malloc_stats",
"tests/test_commands.py::TestRedisCommands::test_memory_stats",
"tests/test_commands.py::TestRedisCommands::test_memory_usage",
"tests/test_commands.py::TestRedisCommands::test_latency_histogram_not_implemented",
"tests/test_commands.py::TestRedisCommands::test_latency_graph_not_implemented",
"tests/test_commands.py::TestRedisCommands::test_latency_doctor_not_implemented",
"tests/test_commands.py::TestRedisCommands::test_module_list",
"tests/test_commands.py::TestRedisCommands::test_command_count",
"tests/test_commands.py::TestRedisCommands::test_command_docs",
"tests/test_commands.py::TestRedisCommands::test_command_list",
"tests/test_commands.py::TestRedisCommands::test_command_getkeys",
"tests/test_commands.py::TestRedisCommands::test_command",
"tests/test_commands.py::TestRedisCommands::test_command_getkeysandflags",
"tests/test_commands.py::TestRedisCommands::test_module",
"tests/test_commands.py::TestRedisCommands::test_module_loadex",
"tests/test_commands.py::TestRedisCommands::test_restore",
"tests/test_commands.py::TestRedisCommands::test_restore_idletime",
"tests/test_commands.py::TestRedisCommands::test_restore_frequency",
"tests/test_commands.py::TestRedisCommands::test_replicaof",
"tests/test_commands.py::TestRedisCommands::test_shutdown",
"tests/test_commands.py::TestRedisCommands::test_shutdown_with_params",
"tests/test_commands.py::TestRedisCommands::test_sync",
"tests/test_commands.py::TestRedisCommands::test_psync",
"tests/test_commands.py::TestBinarySave::test_binary_get_set",
"tests/test_commands.py::TestBinarySave::test_binary_lists",
"tests/test_commands.py::TestBinarySave::test_22_info",
"tests/test_commands.py::TestBinarySave::test_large_responses",
"tests/test_commands.py::TestBinarySave::test_floating_point_encoding"
] | [
"tests/test_commands.py::TestRedisCommands::test_latency_history",
"tests/test_commands.py::TestRedisCommands::test_latency_latest",
"tests/test_commands.py::TestRedisCommands::test_latency_reset"
] | Python | [] | [] |
|
redis/redis-py | 2,529 | redis__redis-py-2529 | [
"2178"
] | f06f3db647c81bc24fa9bdad33822ca6175c32eb | diff --git a/redis/commands/core.py b/redis/commands/core.py
--- a/redis/commands/core.py
+++ b/redis/commands/core.py
@@ -2255,6 +2255,8 @@ def set(
pieces.append(int(ex.total_seconds()))
elif isinstance(ex, int):
pieces.append(ex)
+ elif isinstance(ex, str) and ex.isdigit():
+ pieces.append(int(ex))
else:
raise DataError("ex must be datetime.timedelta or int")
if px is not None:
| diff --git a/tests/test_commands.py b/tests/test_commands.py
--- a/tests/test_commands.py
+++ b/tests/test_commands.py
@@ -1600,6 +1600,13 @@ def test_set_ex(self, r):
with pytest.raises(exceptions.DataError):
assert r.set("a", "1", ex=10.0)
+ @skip_if_server_version_lt("2.6.0")
+ def test_set_ex_str(self, r):
+ assert r.set("a", "1", ex="10")
+ assert 0 < r.ttl("a") <= 10
+ with pytest.raises(exceptions.DataError):
+ assert r.set("a", "1", ex="10.5")
+
@skip_if_server_version_lt("2.6.0")
def test_set_ex_timedelta(self, r):
expire_at = datetime.timedelta(seconds=60)
| `set` and `expire` api diffrent handle to 'expire time' param
expire: https://github.com/redis/redis-py/blob/42b937fa1f73f3e8251eba5ec8ead2fcbaec7c50/redis/commands/core.py#L1592
set: https://github.com/redis/redis-py/blob/42b937fa1f73f3e8251eba5ec8ead2fcbaec7c50/redis/commands/core.py#L2175
The `set` api `ex` should be a interger, but `expire`'s `time` can use a string(integer in string type) as input.
It make me confusing. Both can also accept string type is more convenient.
| "2022-12-22T13:09:56Z" | 4.4 | [
"tests/test_commands.py::TestResponseCallbacks::test_response_callbacks",
"tests/test_commands.py::TestResponseCallbacks::test_case_insensitive_command_names",
"tests/test_commands.py::TestRedisCommands::test_auth",
"tests/test_commands.py::TestRedisCommands::test_command_on_invalid_key_type",
"tests/test_commands.py::TestRedisCommands::test_acl_cat_no_category",
"tests/test_commands.py::TestRedisCommands::test_acl_cat_with_category",
"tests/test_commands.py::TestRedisCommands::test_acl_deluser",
"tests/test_commands.py::TestRedisCommands::test_acl_genpass",
"tests/test_commands.py::TestRedisCommands::test_acl_help",
"tests/test_commands.py::TestRedisCommands::test_acl_list",
"tests/test_commands.py::TestRedisCommands::test_acl_log",
"tests/test_commands.py::TestRedisCommands::test_acl_setuser_categories_without_prefix_fails",
"tests/test_commands.py::TestRedisCommands::test_acl_setuser_commands_without_prefix_fails",
"tests/test_commands.py::TestRedisCommands::test_acl_setuser_add_passwords_and_nopass_fails",
"tests/test_commands.py::TestRedisCommands::test_acl_users",
"tests/test_commands.py::TestRedisCommands::test_acl_whoami",
"tests/test_commands.py::TestRedisCommands::test_client_list",
"tests/test_commands.py::TestRedisCommands::test_client_info",
"tests/test_commands.py::TestRedisCommands::test_client_list_types_not_replica",
"tests/test_commands.py::TestRedisCommands::test_client_list_replica",
"tests/test_commands.py::TestRedisCommands::test_client_list_client_id",
"tests/test_commands.py::TestRedisCommands::test_client_id",
"tests/test_commands.py::TestRedisCommands::test_client_trackinginfo",
"tests/test_commands.py::TestRedisCommands::test_client_tracking",
"tests/test_commands.py::TestRedisCommands::test_client_unblock",
"tests/test_commands.py::TestRedisCommands::test_client_getname",
"tests/test_commands.py::TestRedisCommands::test_client_setname",
"tests/test_commands.py::TestRedisCommands::test_client_kill",
"tests/test_commands.py::TestRedisCommands::test_client_kill_filter_invalid_params",
"tests/test_commands.py::TestRedisCommands::test_client_kill_filter_by_id",
"tests/test_commands.py::TestRedisCommands::test_client_kill_filter_by_addr",
"tests/test_commands.py::TestRedisCommands::test_client_list_after_client_setname",
"tests/test_commands.py::TestRedisCommands::test_client_kill_filter_by_laddr",
"tests/test_commands.py::TestRedisCommands::test_client_kill_filter_by_user",
"tests/test_commands.py::TestRedisCommands::test_client_pause",
"tests/test_commands.py::TestRedisCommands::test_client_pause_all",
"tests/test_commands.py::TestRedisCommands::test_client_unpause",
"tests/test_commands.py::TestRedisCommands::test_client_no_evict",
"tests/test_commands.py::TestRedisCommands::test_client_reply",
"tests/test_commands.py::TestRedisCommands::test_client_getredir",
"tests/test_commands.py::TestRedisCommands::test_hello_notI_implemented",
"tests/test_commands.py::TestRedisCommands::test_config_get",
"tests/test_commands.py::TestRedisCommands::test_config_get_multi_params",
"tests/test_commands.py::TestRedisCommands::test_config_resetstat",
"tests/test_commands.py::TestRedisCommands::test_config_set",
"tests/test_commands.py::TestRedisCommands::test_config_set_multi_params",
"tests/test_commands.py::TestRedisCommands::test_failover",
"tests/test_commands.py::TestRedisCommands::test_dbsize",
"tests/test_commands.py::TestRedisCommands::test_echo",
"tests/test_commands.py::TestRedisCommands::test_info",
"tests/test_commands.py::TestRedisCommands::test_info_multi_sections",
"tests/test_commands.py::TestRedisCommands::test_lastsave",
"tests/test_commands.py::TestRedisCommands::test_lolwut",
"tests/test_commands.py::TestRedisCommands::test_reset",
"tests/test_commands.py::TestRedisCommands::test_object",
"tests/test_commands.py::TestRedisCommands::test_ping",
"tests/test_commands.py::TestRedisCommands::test_quit",
"tests/test_commands.py::TestRedisCommands::test_role",
"tests/test_commands.py::TestRedisCommands::test_select",
"tests/test_commands.py::TestRedisCommands::test_slowlog_get",
"tests/test_commands.py::TestRedisCommands::test_slowlog_get_limit",
"tests/test_commands.py::TestRedisCommands::test_slowlog_length",
"tests/test_commands.py::TestRedisCommands::test_time",
"tests/test_commands.py::TestRedisCommands::test_bgsave",
"tests/test_commands.py::TestRedisCommands::test_never_decode_option",
"tests/test_commands.py::TestRedisCommands::test_empty_response_option",
"tests/test_commands.py::TestRedisCommands::test_append",
"tests/test_commands.py::TestRedisCommands::test_bitcount",
"tests/test_commands.py::TestRedisCommands::test_bitcount_mode",
"tests/test_commands.py::TestRedisCommands::test_bitop_not_empty_string",
"tests/test_commands.py::TestRedisCommands::test_bitop_not",
"tests/test_commands.py::TestRedisCommands::test_bitop_not_in_place",
"tests/test_commands.py::TestRedisCommands::test_bitop_single_string",
"tests/test_commands.py::TestRedisCommands::test_bitop_string_operands",
"tests/test_commands.py::TestRedisCommands::test_bitpos",
"tests/test_commands.py::TestRedisCommands::test_bitpos_wrong_arguments",
"tests/test_commands.py::TestRedisCommands::test_bitpos_mode",
"tests/test_commands.py::TestRedisCommands::test_copy",
"tests/test_commands.py::TestRedisCommands::test_copy_and_replace",
"tests/test_commands.py::TestRedisCommands::test_copy_to_another_database",
"tests/test_commands.py::TestRedisCommands::test_decr",
"tests/test_commands.py::TestRedisCommands::test_decrby",
"tests/test_commands.py::TestRedisCommands::test_delete",
"tests/test_commands.py::TestRedisCommands::test_delete_with_multiple_keys",
"tests/test_commands.py::TestRedisCommands::test_delitem",
"tests/test_commands.py::TestRedisCommands::test_unlink",
"tests/test_commands.py::TestRedisCommands::test_unlink_with_multiple_keys",
"tests/test_commands.py::TestRedisCommands::test_lcs",
"tests/test_commands.py::TestRedisCommands::test_dump_and_restore",
"tests/test_commands.py::TestRedisCommands::test_dump_and_restore_and_replace",
"tests/test_commands.py::TestRedisCommands::test_dump_and_restore_absttl",
"tests/test_commands.py::TestRedisCommands::test_exists",
"tests/test_commands.py::TestRedisCommands::test_exists_contains",
"tests/test_commands.py::TestRedisCommands::test_expire",
"tests/test_commands.py::TestRedisCommands::test_expire_option_nx",
"tests/test_commands.py::TestRedisCommands::test_expire_option_xx",
"tests/test_commands.py::TestRedisCommands::test_expire_option_gt",
"tests/test_commands.py::TestRedisCommands::test_expire_option_lt",
"tests/test_commands.py::TestRedisCommands::test_expireat_datetime",
"tests/test_commands.py::TestRedisCommands::test_expireat_no_key",
"tests/test_commands.py::TestRedisCommands::test_expireat_unixtime",
"tests/test_commands.py::TestRedisCommands::test_expiretime",
"tests/test_commands.py::TestRedisCommands::test_expireat_option_nx",
"tests/test_commands.py::TestRedisCommands::test_expireat_option_xx",
"tests/test_commands.py::TestRedisCommands::test_expireat_option_gt",
"tests/test_commands.py::TestRedisCommands::test_expireat_option_lt",
"tests/test_commands.py::TestRedisCommands::test_get_and_set",
"tests/test_commands.py::TestRedisCommands::test_getdel",
"tests/test_commands.py::TestRedisCommands::test_getex",
"tests/test_commands.py::TestRedisCommands::test_getitem_and_setitem",
"tests/test_commands.py::TestRedisCommands::test_getitem_raises_keyerror_for_missing_key",
"tests/test_commands.py::TestRedisCommands::test_getitem_does_not_raise_keyerror_for_empty_string",
"tests/test_commands.py::TestRedisCommands::test_get_set_bit",
"tests/test_commands.py::TestRedisCommands::test_getrange",
"tests/test_commands.py::TestRedisCommands::test_getset",
"tests/test_commands.py::TestRedisCommands::test_incr",
"tests/test_commands.py::TestRedisCommands::test_incrby",
"tests/test_commands.py::TestRedisCommands::test_incrbyfloat",
"tests/test_commands.py::TestRedisCommands::test_keys",
"tests/test_commands.py::TestRedisCommands::test_mget",
"tests/test_commands.py::TestRedisCommands::test_lmove",
"tests/test_commands.py::TestRedisCommands::test_blmove",
"tests/test_commands.py::TestRedisCommands::test_mset",
"tests/test_commands.py::TestRedisCommands::test_msetnx",
"tests/test_commands.py::TestRedisCommands::test_pexpire",
"tests/test_commands.py::TestRedisCommands::test_pexpire_option_nx",
"tests/test_commands.py::TestRedisCommands::test_pexpire_option_xx",
"tests/test_commands.py::TestRedisCommands::test_pexpire_option_gt",
"tests/test_commands.py::TestRedisCommands::test_pexpire_option_lt",
"tests/test_commands.py::TestRedisCommands::test_pexpireat_datetime",
"tests/test_commands.py::TestRedisCommands::test_pexpireat_no_key",
"tests/test_commands.py::TestRedisCommands::test_pexpireat_unixtime",
"tests/test_commands.py::TestRedisCommands::test_pexpireat_option_nx",
"tests/test_commands.py::TestRedisCommands::test_pexpireat_option_xx",
"tests/test_commands.py::TestRedisCommands::test_pexpireat_option_gt",
"tests/test_commands.py::TestRedisCommands::test_pexpireat_option_lt",
"tests/test_commands.py::TestRedisCommands::test_pexpiretime",
"tests/test_commands.py::TestRedisCommands::test_psetex",
"tests/test_commands.py::TestRedisCommands::test_psetex_timedelta",
"tests/test_commands.py::TestRedisCommands::test_pttl",
"tests/test_commands.py::TestRedisCommands::test_pttl_no_key",
"tests/test_commands.py::TestRedisCommands::test_hrandfield",
"tests/test_commands.py::TestRedisCommands::test_randomkey",
"tests/test_commands.py::TestRedisCommands::test_rename",
"tests/test_commands.py::TestRedisCommands::test_renamenx",
"tests/test_commands.py::TestRedisCommands::test_set_nx",
"tests/test_commands.py::TestRedisCommands::test_set_xx",
"tests/test_commands.py::TestRedisCommands::test_set_px",
"tests/test_commands.py::TestRedisCommands::test_set_px_timedelta",
"tests/test_commands.py::TestRedisCommands::test_set_ex",
"tests/test_commands.py::TestRedisCommands::test_set_ex_timedelta",
"tests/test_commands.py::TestRedisCommands::test_set_exat_timedelta",
"tests/test_commands.py::TestRedisCommands::test_set_pxat_timedelta",
"tests/test_commands.py::TestRedisCommands::test_set_multipleoptions",
"tests/test_commands.py::TestRedisCommands::test_set_keepttl",
"tests/test_commands.py::TestRedisCommands::test_set_get",
"tests/test_commands.py::TestRedisCommands::test_setex",
"tests/test_commands.py::TestRedisCommands::test_setnx",
"tests/test_commands.py::TestRedisCommands::test_setrange",
"tests/test_commands.py::TestRedisCommands::test_strlen",
"tests/test_commands.py::TestRedisCommands::test_substr",
"tests/test_commands.py::TestRedisCommands::test_ttl",
"tests/test_commands.py::TestRedisCommands::test_ttl_nokey",
"tests/test_commands.py::TestRedisCommands::test_type",
"tests/test_commands.py::TestRedisCommands::test_blpop",
"tests/test_commands.py::TestRedisCommands::test_brpop",
"tests/test_commands.py::TestRedisCommands::test_brpoplpush",
"tests/test_commands.py::TestRedisCommands::test_brpoplpush_empty_string",
"tests/test_commands.py::TestRedisCommands::test_blmpop",
"tests/test_commands.py::TestRedisCommands::test_lmpop",
"tests/test_commands.py::TestRedisCommands::test_lindex",
"tests/test_commands.py::TestRedisCommands::test_linsert",
"tests/test_commands.py::TestRedisCommands::test_llen",
"tests/test_commands.py::TestRedisCommands::test_lpop",
"tests/test_commands.py::TestRedisCommands::test_lpop_count",
"tests/test_commands.py::TestRedisCommands::test_lpush",
"tests/test_commands.py::TestRedisCommands::test_lpushx",
"tests/test_commands.py::TestRedisCommands::test_lpushx_with_list",
"tests/test_commands.py::TestRedisCommands::test_lrange",
"tests/test_commands.py::TestRedisCommands::test_lrem",
"tests/test_commands.py::TestRedisCommands::test_lset",
"tests/test_commands.py::TestRedisCommands::test_ltrim",
"tests/test_commands.py::TestRedisCommands::test_rpop",
"tests/test_commands.py::TestRedisCommands::test_rpop_count",
"tests/test_commands.py::TestRedisCommands::test_rpoplpush",
"tests/test_commands.py::TestRedisCommands::test_rpush",
"tests/test_commands.py::TestRedisCommands::test_lpos",
"tests/test_commands.py::TestRedisCommands::test_rpushx",
"tests/test_commands.py::TestRedisCommands::test_scan",
"tests/test_commands.py::TestRedisCommands::test_scan_type",
"tests/test_commands.py::TestRedisCommands::test_scan_iter",
"tests/test_commands.py::TestRedisCommands::test_sscan",
"tests/test_commands.py::TestRedisCommands::test_sscan_iter",
"tests/test_commands.py::TestRedisCommands::test_hscan",
"tests/test_commands.py::TestRedisCommands::test_hscan_iter",
"tests/test_commands.py::TestRedisCommands::test_zscan",
"tests/test_commands.py::TestRedisCommands::test_zscan_iter",
"tests/test_commands.py::TestRedisCommands::test_sadd",
"tests/test_commands.py::TestRedisCommands::test_scard",
"tests/test_commands.py::TestRedisCommands::test_sdiff",
"tests/test_commands.py::TestRedisCommands::test_sdiffstore",
"tests/test_commands.py::TestRedisCommands::test_sinter",
"tests/test_commands.py::TestRedisCommands::test_sintercard",
"tests/test_commands.py::TestRedisCommands::test_sinterstore",
"tests/test_commands.py::TestRedisCommands::test_sismember",
"tests/test_commands.py::TestRedisCommands::test_smembers",
"tests/test_commands.py::TestRedisCommands::test_smismember",
"tests/test_commands.py::TestRedisCommands::test_smove",
"tests/test_commands.py::TestRedisCommands::test_spop",
"tests/test_commands.py::TestRedisCommands::test_spop_multi_value",
"tests/test_commands.py::TestRedisCommands::test_srandmember",
"tests/test_commands.py::TestRedisCommands::test_srandmember_multi_value",
"tests/test_commands.py::TestRedisCommands::test_srem",
"tests/test_commands.py::TestRedisCommands::test_sunion",
"tests/test_commands.py::TestRedisCommands::test_sunionstore",
"tests/test_commands.py::TestRedisCommands::test_debug_segfault",
"tests/test_commands.py::TestRedisCommands::test_script_debug",
"tests/test_commands.py::TestRedisCommands::test_zadd",
"tests/test_commands.py::TestRedisCommands::test_zadd_nx",
"tests/test_commands.py::TestRedisCommands::test_zadd_xx",
"tests/test_commands.py::TestRedisCommands::test_zadd_ch",
"tests/test_commands.py::TestRedisCommands::test_zadd_incr",
"tests/test_commands.py::TestRedisCommands::test_zadd_incr_with_xx",
"tests/test_commands.py::TestRedisCommands::test_zadd_gt_lt",
"tests/test_commands.py::TestRedisCommands::test_zcard",
"tests/test_commands.py::TestRedisCommands::test_zcount",
"tests/test_commands.py::TestRedisCommands::test_zdiff",
"tests/test_commands.py::TestRedisCommands::test_zdiffstore",
"tests/test_commands.py::TestRedisCommands::test_zincrby",
"tests/test_commands.py::TestRedisCommands::test_zlexcount",
"tests/test_commands.py::TestRedisCommands::test_zinter",
"tests/test_commands.py::TestRedisCommands::test_zintercard",
"tests/test_commands.py::TestRedisCommands::test_zinterstore_sum",
"tests/test_commands.py::TestRedisCommands::test_zinterstore_max",
"tests/test_commands.py::TestRedisCommands::test_zinterstore_min",
"tests/test_commands.py::TestRedisCommands::test_zinterstore_with_weight",
"tests/test_commands.py::TestRedisCommands::test_zpopmax",
"tests/test_commands.py::TestRedisCommands::test_zpopmin",
"tests/test_commands.py::TestRedisCommands::test_zrandemember",
"tests/test_commands.py::TestRedisCommands::test_bzpopmax",
"tests/test_commands.py::TestRedisCommands::test_bzpopmin",
"tests/test_commands.py::TestRedisCommands::test_zmpop",
"tests/test_commands.py::TestRedisCommands::test_bzmpop",
"tests/test_commands.py::TestRedisCommands::test_zrange",
"tests/test_commands.py::TestRedisCommands::test_zrange_errors",
"tests/test_commands.py::TestRedisCommands::test_zrange_params",
"tests/test_commands.py::TestRedisCommands::test_zrangestore",
"tests/test_commands.py::TestRedisCommands::test_zrangebylex",
"tests/test_commands.py::TestRedisCommands::test_zrevrangebylex",
"tests/test_commands.py::TestRedisCommands::test_zrangebyscore",
"tests/test_commands.py::TestRedisCommands::test_zrank",
"tests/test_commands.py::TestRedisCommands::test_zrem",
"tests/test_commands.py::TestRedisCommands::test_zrem_multiple_keys",
"tests/test_commands.py::TestRedisCommands::test_zremrangebylex",
"tests/test_commands.py::TestRedisCommands::test_zremrangebyrank",
"tests/test_commands.py::TestRedisCommands::test_zremrangebyscore",
"tests/test_commands.py::TestRedisCommands::test_zrevrange",
"tests/test_commands.py::TestRedisCommands::test_zrevrangebyscore",
"tests/test_commands.py::TestRedisCommands::test_zrevrank",
"tests/test_commands.py::TestRedisCommands::test_zscore",
"tests/test_commands.py::TestRedisCommands::test_zunion",
"tests/test_commands.py::TestRedisCommands::test_zunionstore_sum",
"tests/test_commands.py::TestRedisCommands::test_zunionstore_max",
"tests/test_commands.py::TestRedisCommands::test_zunionstore_min",
"tests/test_commands.py::TestRedisCommands::test_zunionstore_with_weight",
"tests/test_commands.py::TestRedisCommands::test_zmscore",
"tests/test_commands.py::TestRedisCommands::test_pfadd",
"tests/test_commands.py::TestRedisCommands::test_pfcount",
"tests/test_commands.py::TestRedisCommands::test_pfmerge",
"tests/test_commands.py::TestRedisCommands::test_hget_and_hset",
"tests/test_commands.py::TestRedisCommands::test_hset_with_multi_key_values",
"tests/test_commands.py::TestRedisCommands::test_hset_with_key_values_passed_as_list",
"tests/test_commands.py::TestRedisCommands::test_hset_without_data",
"tests/test_commands.py::TestRedisCommands::test_hdel",
"tests/test_commands.py::TestRedisCommands::test_hexists",
"tests/test_commands.py::TestRedisCommands::test_hgetall",
"tests/test_commands.py::TestRedisCommands::test_hincrby",
"tests/test_commands.py::TestRedisCommands::test_hincrbyfloat",
"tests/test_commands.py::TestRedisCommands::test_hkeys",
"tests/test_commands.py::TestRedisCommands::test_hlen",
"tests/test_commands.py::TestRedisCommands::test_hmget",
"tests/test_commands.py::TestRedisCommands::test_hmset",
"tests/test_commands.py::TestRedisCommands::test_hsetnx",
"tests/test_commands.py::TestRedisCommands::test_hvals",
"tests/test_commands.py::TestRedisCommands::test_hstrlen",
"tests/test_commands.py::TestRedisCommands::test_sort_basic",
"tests/test_commands.py::TestRedisCommands::test_sort_limited",
"tests/test_commands.py::TestRedisCommands::test_sort_by",
"tests/test_commands.py::TestRedisCommands::test_sort_get",
"tests/test_commands.py::TestRedisCommands::test_sort_get_multi",
"tests/test_commands.py::TestRedisCommands::test_sort_get_groups_two",
"tests/test_commands.py::TestRedisCommands::test_sort_groups_string_get",
"tests/test_commands.py::TestRedisCommands::test_sort_groups_just_one_get",
"tests/test_commands.py::TestRedisCommands::test_sort_groups_no_get",
"tests/test_commands.py::TestRedisCommands::test_sort_groups_three_gets",
"tests/test_commands.py::TestRedisCommands::test_sort_desc",
"tests/test_commands.py::TestRedisCommands::test_sort_alpha",
"tests/test_commands.py::TestRedisCommands::test_sort_store",
"tests/test_commands.py::TestRedisCommands::test_sort_all_options",
"tests/test_commands.py::TestRedisCommands::test_sort_ro",
"tests/test_commands.py::TestRedisCommands::test_sort_issue_924",
"tests/test_commands.py::TestRedisCommands::test_cluster_addslots",
"tests/test_commands.py::TestRedisCommands::test_cluster_count_failure_reports",
"tests/test_commands.py::TestRedisCommands::test_cluster_countkeysinslot",
"tests/test_commands.py::TestRedisCommands::test_cluster_delslots",
"tests/test_commands.py::TestRedisCommands::test_cluster_failover",
"tests/test_commands.py::TestRedisCommands::test_cluster_forget",
"tests/test_commands.py::TestRedisCommands::test_cluster_info",
"tests/test_commands.py::TestRedisCommands::test_cluster_keyslot",
"tests/test_commands.py::TestRedisCommands::test_cluster_meet",
"tests/test_commands.py::TestRedisCommands::test_cluster_nodes",
"tests/test_commands.py::TestRedisCommands::test_cluster_replicate",
"tests/test_commands.py::TestRedisCommands::test_cluster_reset",
"tests/test_commands.py::TestRedisCommands::test_cluster_saveconfig",
"tests/test_commands.py::TestRedisCommands::test_cluster_setslot",
"tests/test_commands.py::TestRedisCommands::test_cluster_slaves",
"tests/test_commands.py::TestRedisCommands::test_readonly_invalid_cluster_state",
"tests/test_commands.py::TestRedisCommands::test_readonly",
"tests/test_commands.py::TestRedisCommands::test_geoadd",
"tests/test_commands.py::TestRedisCommands::test_geoadd_nx",
"tests/test_commands.py::TestRedisCommands::test_geoadd_xx",
"tests/test_commands.py::TestRedisCommands::test_geoadd_ch",
"tests/test_commands.py::TestRedisCommands::test_geoadd_invalid_params",
"tests/test_commands.py::TestRedisCommands::test_geodist",
"tests/test_commands.py::TestRedisCommands::test_geodist_units",
"tests/test_commands.py::TestRedisCommands::test_geodist_missing_one_member",
"tests/test_commands.py::TestRedisCommands::test_geodist_invalid_units",
"tests/test_commands.py::TestRedisCommands::test_geohash",
"tests/test_commands.py::TestRedisCommands::test_geopos",
"tests/test_commands.py::TestRedisCommands::test_geopos_no_value",
"tests/test_commands.py::TestRedisCommands::test_geosearch",
"tests/test_commands.py::TestRedisCommands::test_geosearch_member",
"tests/test_commands.py::TestRedisCommands::test_geosearch_sort",
"tests/test_commands.py::TestRedisCommands::test_geosearch_with",
"tests/test_commands.py::TestRedisCommands::test_geosearch_negative",
"tests/test_commands.py::TestRedisCommands::test_geosearchstore",
"tests/test_commands.py::TestRedisCommands::test_geosearchstore_dist",
"tests/test_commands.py::TestRedisCommands::test_georadius",
"tests/test_commands.py::TestRedisCommands::test_georadius_no_values",
"tests/test_commands.py::TestRedisCommands::test_georadius_units",
"tests/test_commands.py::TestRedisCommands::test_georadius_with",
"tests/test_commands.py::TestRedisCommands::test_georadius_count",
"tests/test_commands.py::TestRedisCommands::test_georadius_sort",
"tests/test_commands.py::TestRedisCommands::test_georadius_store",
"tests/test_commands.py::TestRedisCommands::test_georadius_store_dist",
"tests/test_commands.py::TestRedisCommands::test_georadiusmember",
"tests/test_commands.py::TestRedisCommands::test_georadiusmember_count",
"tests/test_commands.py::TestRedisCommands::test_xack",
"tests/test_commands.py::TestRedisCommands::test_xadd",
"tests/test_commands.py::TestRedisCommands::test_xadd_nomkstream",
"tests/test_commands.py::TestRedisCommands::test_xadd_minlen_and_limit",
"tests/test_commands.py::TestRedisCommands::test_xadd_explicit_ms",
"tests/test_commands.py::TestRedisCommands::test_xautoclaim_negative",
"tests/test_commands.py::TestRedisCommands::test_xclaim",
"tests/test_commands.py::TestRedisCommands::test_xclaim_trimmed",
"tests/test_commands.py::TestRedisCommands::test_xdel",
"tests/test_commands.py::TestRedisCommands::test_xgroup_create",
"tests/test_commands.py::TestRedisCommands::test_xgroup_create_mkstream",
"tests/test_commands.py::TestRedisCommands::test_xgroup_create_entriesread",
"tests/test_commands.py::TestRedisCommands::test_xgroup_delconsumer",
"tests/test_commands.py::TestRedisCommands::test_xgroup_createconsumer",
"tests/test_commands.py::TestRedisCommands::test_xgroup_destroy",
"tests/test_commands.py::TestRedisCommands::test_xgroup_setid",
"tests/test_commands.py::TestRedisCommands::test_xinfo_stream",
"tests/test_commands.py::TestRedisCommands::test_xinfo_stream_full",
"tests/test_commands.py::TestRedisCommands::test_xlen",
"tests/test_commands.py::TestRedisCommands::test_xpending",
"tests/test_commands.py::TestRedisCommands::test_xpending_range",
"tests/test_commands.py::TestRedisCommands::test_xpending_range_idle",
"tests/test_commands.py::TestRedisCommands::test_xpending_range_negative",
"tests/test_commands.py::TestRedisCommands::test_xrange",
"tests/test_commands.py::TestRedisCommands::test_xread",
"tests/test_commands.py::TestRedisCommands::test_xreadgroup",
"tests/test_commands.py::TestRedisCommands::test_xrevrange",
"tests/test_commands.py::TestRedisCommands::test_xtrim",
"tests/test_commands.py::TestRedisCommands::test_xtrim_minlen_and_length_args",
"tests/test_commands.py::TestRedisCommands::test_bitfield_operations",
"tests/test_commands.py::TestRedisCommands::test_bitfield_ro",
"tests/test_commands.py::TestRedisCommands::test_memory_help",
"tests/test_commands.py::TestRedisCommands::test_memory_doctor",
"tests/test_commands.py::TestRedisCommands::test_memory_malloc_stats",
"tests/test_commands.py::TestRedisCommands::test_memory_stats",
"tests/test_commands.py::TestRedisCommands::test_memory_usage",
"tests/test_commands.py::TestRedisCommands::test_latency_histogram_not_implemented",
"tests/test_commands.py::TestRedisCommands::test_latency_graph_not_implemented",
"tests/test_commands.py::TestRedisCommands::test_latency_doctor_not_implemented",
"tests/test_commands.py::TestRedisCommands::test_latency_history",
"tests/test_commands.py::TestRedisCommands::test_latency_latest",
"tests/test_commands.py::TestRedisCommands::test_latency_reset",
"tests/test_commands.py::TestRedisCommands::test_module_list",
"tests/test_commands.py::TestRedisCommands::test_command_count",
"tests/test_commands.py::TestRedisCommands::test_command_docs",
"tests/test_commands.py::TestRedisCommands::test_command_list",
"tests/test_commands.py::TestRedisCommands::test_command_getkeys",
"tests/test_commands.py::TestRedisCommands::test_command",
"tests/test_commands.py::TestRedisCommands::test_command_getkeysandflags",
"tests/test_commands.py::TestRedisCommands::test_module",
"tests/test_commands.py::TestRedisCommands::test_module_loadex",
"tests/test_commands.py::TestRedisCommands::test_restore",
"tests/test_commands.py::TestRedisCommands::test_restore_idletime",
"tests/test_commands.py::TestRedisCommands::test_restore_frequency",
"tests/test_commands.py::TestRedisCommands::test_replicaof",
"tests/test_commands.py::TestRedisCommands::test_shutdown",
"tests/test_commands.py::TestRedisCommands::test_shutdown_with_params",
"tests/test_commands.py::TestRedisCommands::test_sync",
"tests/test_commands.py::TestRedisCommands::test_psync",
"tests/test_commands.py::TestBinarySave::test_binary_get_set",
"tests/test_commands.py::TestBinarySave::test_binary_lists",
"tests/test_commands.py::TestBinarySave::test_22_info",
"tests/test_commands.py::TestBinarySave::test_large_responses",
"tests/test_commands.py::TestBinarySave::test_floating_point_encoding"
] | [
"tests/test_commands.py::TestRedisCommands::test_set_ex_str"
] | Python | [] | [] |
|
redis/redis-py | 2,583 | redis__redis-py-2583 | [
"2581"
] | 5cb5712d283fa8fb300abc9d71a61c1a81de5643 | diff --git a/redis/connection.py b/redis/connection.py
--- a/redis/connection.py
+++ b/redis/connection.py
@@ -1153,6 +1153,7 @@ def __init__(
retry=None,
redis_connect_func=None,
credential_provider: Optional[CredentialProvider] = None,
+ command_packer=None,
):
"""
Initialize a new UnixDomainSocketConnection.
@@ -1202,6 +1203,7 @@ def __init__(
self.set_parser(parser_class)
self._connect_callbacks = []
self._buffer_cutoff = 6000
+ self._command_packer = self._construct_command_packer(command_packer)
def repr_pieces(self):
pieces = [("path", self.path), ("db", self.db)]
| diff --git a/tests/test_connection.py b/tests/test_connection.py
--- a/tests/test_connection.py
+++ b/tests/test_connection.py
@@ -7,7 +7,13 @@
import redis
from redis.backoff import NoBackoff
-from redis.connection import Connection, HiredisParser, PythonParser
+from redis.connection import (
+ Connection,
+ HiredisParser,
+ PythonParser,
+ SSLConnection,
+ UnixDomainSocketConnection,
+)
from redis.exceptions import ConnectionError, InvalidResponse, TimeoutError
from redis.retry import Retry
from redis.utils import HIREDIS_AVAILABLE
@@ -163,3 +169,39 @@ def test_connection_parse_response_resume(r: redis.Redis, parser_class):
pytest.fail("didn't receive a response")
assert response
assert i > 0
+
+
[email protected]
[email protected](
+ "Class",
+ [
+ Connection,
+ SSLConnection,
+ UnixDomainSocketConnection,
+ ],
+)
+def test_pack_command(Class):
+ """
+ This test verifies that the pack_command works
+ on all supported connections. #2581
+ """
+ cmd = (
+ "HSET",
+ "foo",
+ "key",
+ "value1",
+ b"key_b",
+ b"bytes str",
+ b"key_i",
+ 67,
+ "key_f",
+ 3.14159265359,
+ )
+ expected = (
+ b"*10\r\n$4\r\nHSET\r\n$3\r\nfoo\r\n$3\r\nkey\r\n$6\r\nvalue1\r\n"
+ b"$5\r\nkey_b\r\n$9\r\nbytes str\r\n$5\r\nkey_i\r\n$2\r\n67\r\n$5"
+ b"\r\nkey_f\r\n$13\r\n3.14159265359\r\n"
+ )
+
+ actual = Class().pack_command(*cmd)[0]
+ assert actual == expected, f"actual = {actual}, expected = {expected}"
| 'UnixDomainSocketConnection' object has no attribute '_command_packer'
**Version**: v4.5.0
**Platform**: Debian Bullseye using Python v3.9.2
**Description**:
The following code which used to work on up to and including v4.4.2 now crashes with the stack trace below on v4.5.0.
```python
redis_conn = redis.Redis(
unix_socket_path="/var/run/redis/redis-server.sock",
decode_responses=True)
redis_conn.ping()
```
```
Traceback (most recent call last):
File "<redacted>", line 142, in main
redis_conn.ping()
File "/usr/local/lib/python3.9/dist-packages/redis/commands/core.py", line 1194, in ping
return self.execute_command("PING", **kwargs)
File "/usr/local/lib/python3.9/dist-packages/redis/client.py", line 1258, in execute_command
return conn.retry.call_with_retry(
File "/usr/local/lib/python3.9/dist-packages/redis/retry.py", line 46, in call_with_retry
return do()
File "/usr/local/lib/python3.9/dist-packages/redis/client.py", line 1259, in <lambda>
lambda: self._send_command_parse_response(
File "/usr/local/lib/python3.9/dist-packages/redis/client.py", line 1234, in _send_command_parse_response
conn.send_command(*args)
File "/usr/local/lib/python3.9/dist-packages/redis/connection.py", line 916, in send_command
self._command_packer.pack(*args),
AttributeError: 'UnixDomainSocketConnection' object has no attribute '_command_packer'
```
| also seeing this in our apps, had to pin to 4.4.2 to prevent our django app from crashing anytime it attempted to use the cache
I guess this is the issue:
```python
class Connection:
def __init__(self, ...):
...
self._command_packer = self._construct_command_packer(command_packer)
class SSLConnection(Connection):
def __init__(self, ...):
...
super().__init__(...)
class UnixDomainSocketConnection(Connection):
def __init__(self, ...):
# does not call _construct_command_packer nor super().__init__()
```
Seems like the bug was introduced just before the 4.5.0 release: https://github.com/redis/redis-py/pull/2570
@prokazov Could you take a look please?
Strange that this does not get picked up by the tests.
Looks like there is no synchronous tests that run any command on SSLConnection/UnixDomainSocketConnection.
Actually `SSLConnection` does call `super().__init__` so that one should be fine.
The fact that `UnixDomainSocketConnection.__init__ ` does not call `super().__init__` is probably worth fixing. I see lots of copy&paste so seems doable? Perhaps introduce an `AbstractConnection` with abstract methods `_connect`, `repr_pieces` and `_error_message`?
`Connection` has `host` and `port` while `UnixDomainSocketConnection` has `path`.
`Connection` has in addition `socket_connect_timeout`, `socket_keepalive `, `socket_keepalive_options` and `socket_type`.
Lastly there is `if retry or retry_on_error:` vs. `if retry_on_error:`. This discrepancy is probably also a mistake https://github.com/redis/redis-py/pull/2377 @barshaul ?
The rest is identical. | "2023-02-08T04:03:44Z" | 4.5 | [
"tests/test_connection.py::test_loading_external_modules",
"tests/test_connection.py::TestConnection::test_disconnect",
"tests/test_connection.py::TestConnection::test_disconnect__shutdown_OSError",
"tests/test_connection.py::TestConnection::test_disconnect__close_OSError",
"tests/test_connection.py::TestConnection::test_retry_connect_on_timeout_error",
"tests/test_connection.py::TestConnection::test_connect_without_retry_on_os_error",
"tests/test_connection.py::TestConnection::test_connect_timeout_error_without_retry",
"tests/test_connection.py::test_connection_parse_response_resume[PythonParser]",
"tests/test_connection.py::test_connection_parse_response_resume[HiredisParser]",
"tests/test_connection.py::test_pack_command[Connection]",
"tests/test_connection.py::test_pack_command[SSLConnection]"
] | [
"tests/test_connection.py::test_pack_command[UnixDomainSocketConnection]"
] | Python | [] | [] |
redis/redis-py | 2,628 | redis__redis-py-2628 | [
"2609"
] | 318b114f4da9846a2a7c150e1fb702e9bebd9fdf | diff --git a/redis/client.py b/redis/client.py
--- a/redis/client.py
+++ b/redis/client.py
@@ -518,10 +518,13 @@ def parse_geosearch_generic(response, **options):
Parse the response of 'GEOSEARCH', GEORADIUS' and 'GEORADIUSBYMEMBER'
commands according to 'withdist', 'withhash' and 'withcoord' labels.
"""
- if options["store"] or options["store_dist"]:
- # `store` and `store_dist` cant be combined
- # with other command arguments.
- # relevant to 'GEORADIUS' and 'GEORADIUSBYMEMBER'
+ try:
+ if options["store"] or options["store_dist"]:
+ # `store` and `store_dist` cant be combined
+ # with other command arguments.
+ # relevant to 'GEORADIUS' and 'GEORADIUSBYMEMBER'
+ return response
+ except KeyError: # it means the command was sent via execute_command
return response
if type(response) != list:
| diff --git a/tests/test_commands.py b/tests/test_commands.py
--- a/tests/test_commands.py
+++ b/tests/test_commands.py
@@ -3508,6 +3508,12 @@ def test_geosearchstore_dist(self, r):
# instead of save the geo score, the distance is saved.
assert r.zscore("places_barcelona", "place1") == 88.05060698409301
+ @skip_if_server_version_lt("3.2.0")
+ def test_georadius_Issue2609(self, r):
+ # test for issue #2609 (Geo search functions don't work with execute_command)
+ r.geoadd(name="my-key", values=[1, 2, "data"])
+ assert r.execute_command("GEORADIUS", "my-key", 1, 2, 400, "m") == [b"data"]
+
@skip_if_server_version_lt("3.2.0")
def test_georadius(self, r):
values = (2.1909389952632, 41.433791470673, "place1") + (
| Geo search functions don't work with execute_command
**Version**: What redis-py and what redis version is the issue happening on?
* `redis-py==4.5.1`
* `redis` docker image `redis:5.0.6`
**Platform**: What platform / version? (For example Python 3.5.1 on Windows 7 / Ubuntu 15.10 / Azure)
Linux, `python:3.8-slim-bullseye` container.
**Description**: Description of your issue, stack traces from errors and code that reproduces the issue
Below is a minimal example that shows the issue
```python
import redis
c = redis.Redis(host="redis", single_connection_client=True)
c.geoadd(name="my-key", values=[1, 2, "data"])
# works fine
x = c.georadius("my-key", 1, 2, 400, unit="m", withcoord=True)
print(x)
# fails
y = c.execute_command("GEORADIUS", 'my-key', 1, 2, 400, "m")
print(y)
```
The stack trace printed is:
```
Traceback (most recent call last):
File "/opt/.pycharm_helpers/pydev/pydevconsole.py", line 364, in runcode
coro = func()
File "<input>", line 1, in <module>
File "/opt/.pycharm_helpers/pydev/_pydev_bundle/pydev_umd.py", line 198, in runfile
pydev_imports.execfile(filename, global_vars, local_vars) # execute the script
File "/opt/.pycharm_helpers/pydev/_pydev_imps/_pydev_execfile.py", line 18, in execfile
exec(compile(contents+"\n", file, 'exec'), glob, loc)
File "/opt/project/apps/park-out-events/api/parkoutevents/redis_check.py", line 48, in <module>
y = c.execute_command("GEORADIUS", 'poe-2023-03-11T23:38', 1, 2, 400, "m")
File "/usr/local/lib/python3.8/site-packages/redis/client.py", line 1238, in execute_command
return conn.retry.call_with_retry(
File "/usr/local/lib/python3.8/site-packages/redis/retry.py", line 46, in call_with_retry
return do()
File "/usr/local/lib/python3.8/site-packages/redis/client.py", line 1239, in <lambda>
lambda: self._send_command_parse_response(
File "/usr/local/lib/python3.8/site-packages/redis/client.py", line 1215, in _send_command_parse_response
return self.parse_response(conn, command_name, **options)
File "/usr/local/lib/python3.8/site-packages/redis/client.py", line 1260, in parse_response
return self.response_callbacks[command_name](response, **options)
File "/usr/local/lib/python3.8/site-packages/redis/client.py", line 518, in parse_geosearch_generic
if options["store"] or options["store_dist"]:
KeyError: 'store'
```
It looks like `parse_geosearch_generic` expects `store` and `store_dist` to be boolean args instead of flags in the CLI commands, like they are in the Python interface.
This also happens for `withdist`, `withcoord`, and `withhash` as the function expects them to be present in `**options`.
Digging a bit further, even if the call is made with these flags, they aren't passed through and available in the `options` argument for `parse_geosearch_generic`, e.g.
```python
r.execute_command("GEORADIUS", "barcelona", 2.187, 41.406, 1000, "m", "WITHDIST")
```
| "2023-03-19T16:01:21Z" | 4.5 | [
"tests/test_commands.py::TestResponseCallbacks::test_response_callbacks",
"tests/test_commands.py::TestResponseCallbacks::test_case_insensitive_command_names",
"tests/test_commands.py::TestRedisCommands::test_auth",
"tests/test_commands.py::TestRedisCommands::test_command_on_invalid_key_type",
"tests/test_commands.py::TestRedisCommands::test_acl_cat_no_category",
"tests/test_commands.py::TestRedisCommands::test_acl_cat_with_category",
"tests/test_commands.py::TestRedisCommands::test_acl_deluser",
"tests/test_commands.py::TestRedisCommands::test_acl_genpass",
"tests/test_commands.py::TestRedisCommands::test_acl_help",
"tests/test_commands.py::TestRedisCommands::test_acl_list",
"tests/test_commands.py::TestRedisCommands::test_acl_log",
"tests/test_commands.py::TestRedisCommands::test_acl_setuser_categories_without_prefix_fails",
"tests/test_commands.py::TestRedisCommands::test_acl_setuser_commands_without_prefix_fails",
"tests/test_commands.py::TestRedisCommands::test_acl_setuser_add_passwords_and_nopass_fails",
"tests/test_commands.py::TestRedisCommands::test_acl_users",
"tests/test_commands.py::TestRedisCommands::test_acl_whoami",
"tests/test_commands.py::TestRedisCommands::test_client_list",
"tests/test_commands.py::TestRedisCommands::test_client_info",
"tests/test_commands.py::TestRedisCommands::test_client_list_types_not_replica",
"tests/test_commands.py::TestRedisCommands::test_client_list_replica",
"tests/test_commands.py::TestRedisCommands::test_client_list_client_id",
"tests/test_commands.py::TestRedisCommands::test_client_id",
"tests/test_commands.py::TestRedisCommands::test_client_trackinginfo",
"tests/test_commands.py::TestRedisCommands::test_client_tracking",
"tests/test_commands.py::TestRedisCommands::test_client_unblock",
"tests/test_commands.py::TestRedisCommands::test_client_getname",
"tests/test_commands.py::TestRedisCommands::test_client_setname",
"tests/test_commands.py::TestRedisCommands::test_client_kill",
"tests/test_commands.py::TestRedisCommands::test_client_kill_filter_invalid_params",
"tests/test_commands.py::TestRedisCommands::test_client_kill_filter_by_id",
"tests/test_commands.py::TestRedisCommands::test_client_kill_filter_by_addr",
"tests/test_commands.py::TestRedisCommands::test_client_list_after_client_setname",
"tests/test_commands.py::TestRedisCommands::test_client_kill_filter_by_laddr",
"tests/test_commands.py::TestRedisCommands::test_client_kill_filter_by_user",
"tests/test_commands.py::TestRedisCommands::test_client_pause",
"tests/test_commands.py::TestRedisCommands::test_client_pause_all",
"tests/test_commands.py::TestRedisCommands::test_client_unpause",
"tests/test_commands.py::TestRedisCommands::test_client_no_evict",
"tests/test_commands.py::TestRedisCommands::test_client_reply",
"tests/test_commands.py::TestRedisCommands::test_client_getredir",
"tests/test_commands.py::TestRedisCommands::test_hello_notI_implemented",
"tests/test_commands.py::TestRedisCommands::test_config_get",
"tests/test_commands.py::TestRedisCommands::test_config_get_multi_params",
"tests/test_commands.py::TestRedisCommands::test_config_resetstat",
"tests/test_commands.py::TestRedisCommands::test_config_set",
"tests/test_commands.py::TestRedisCommands::test_config_set_multi_params",
"tests/test_commands.py::TestRedisCommands::test_failover",
"tests/test_commands.py::TestRedisCommands::test_dbsize",
"tests/test_commands.py::TestRedisCommands::test_echo",
"tests/test_commands.py::TestRedisCommands::test_info",
"tests/test_commands.py::TestRedisCommands::test_info_multi_sections",
"tests/test_commands.py::TestRedisCommands::test_lastsave",
"tests/test_commands.py::TestRedisCommands::test_lolwut",
"tests/test_commands.py::TestRedisCommands::test_reset",
"tests/test_commands.py::TestRedisCommands::test_object",
"tests/test_commands.py::TestRedisCommands::test_ping",
"tests/test_commands.py::TestRedisCommands::test_quit",
"tests/test_commands.py::TestRedisCommands::test_role",
"tests/test_commands.py::TestRedisCommands::test_select",
"tests/test_commands.py::TestRedisCommands::test_slowlog_get",
"tests/test_commands.py::TestRedisCommands::test_slowlog_get_limit",
"tests/test_commands.py::TestRedisCommands::test_slowlog_length",
"tests/test_commands.py::TestRedisCommands::test_time",
"tests/test_commands.py::TestRedisCommands::test_bgsave",
"tests/test_commands.py::TestRedisCommands::test_never_decode_option",
"tests/test_commands.py::TestRedisCommands::test_empty_response_option",
"tests/test_commands.py::TestRedisCommands::test_append",
"tests/test_commands.py::TestRedisCommands::test_bitcount",
"tests/test_commands.py::TestRedisCommands::test_bitcount_mode",
"tests/test_commands.py::TestRedisCommands::test_bitop_not_empty_string",
"tests/test_commands.py::TestRedisCommands::test_bitop_not",
"tests/test_commands.py::TestRedisCommands::test_bitop_not_in_place",
"tests/test_commands.py::TestRedisCommands::test_bitop_single_string",
"tests/test_commands.py::TestRedisCommands::test_bitop_string_operands",
"tests/test_commands.py::TestRedisCommands::test_bitpos",
"tests/test_commands.py::TestRedisCommands::test_bitpos_wrong_arguments",
"tests/test_commands.py::TestRedisCommands::test_bitpos_mode",
"tests/test_commands.py::TestRedisCommands::test_copy",
"tests/test_commands.py::TestRedisCommands::test_copy_and_replace",
"tests/test_commands.py::TestRedisCommands::test_copy_to_another_database",
"tests/test_commands.py::TestRedisCommands::test_decr",
"tests/test_commands.py::TestRedisCommands::test_decrby",
"tests/test_commands.py::TestRedisCommands::test_delete",
"tests/test_commands.py::TestRedisCommands::test_delete_with_multiple_keys",
"tests/test_commands.py::TestRedisCommands::test_delitem",
"tests/test_commands.py::TestRedisCommands::test_unlink",
"tests/test_commands.py::TestRedisCommands::test_unlink_with_multiple_keys",
"tests/test_commands.py::TestRedisCommands::test_lcs",
"tests/test_commands.py::TestRedisCommands::test_dump_and_restore",
"tests/test_commands.py::TestRedisCommands::test_dump_and_restore_and_replace",
"tests/test_commands.py::TestRedisCommands::test_dump_and_restore_absttl",
"tests/test_commands.py::TestRedisCommands::test_exists",
"tests/test_commands.py::TestRedisCommands::test_exists_contains",
"tests/test_commands.py::TestRedisCommands::test_expire",
"tests/test_commands.py::TestRedisCommands::test_expire_option_nx",
"tests/test_commands.py::TestRedisCommands::test_expire_option_xx",
"tests/test_commands.py::TestRedisCommands::test_expire_option_gt",
"tests/test_commands.py::TestRedisCommands::test_expire_option_lt",
"tests/test_commands.py::TestRedisCommands::test_expireat_datetime",
"tests/test_commands.py::TestRedisCommands::test_expireat_no_key",
"tests/test_commands.py::TestRedisCommands::test_expireat_unixtime",
"tests/test_commands.py::TestRedisCommands::test_expiretime",
"tests/test_commands.py::TestRedisCommands::test_expireat_option_nx",
"tests/test_commands.py::TestRedisCommands::test_expireat_option_xx",
"tests/test_commands.py::TestRedisCommands::test_expireat_option_gt",
"tests/test_commands.py::TestRedisCommands::test_expireat_option_lt",
"tests/test_commands.py::TestRedisCommands::test_get_and_set",
"tests/test_commands.py::TestRedisCommands::test_getdel",
"tests/test_commands.py::TestRedisCommands::test_getex",
"tests/test_commands.py::TestRedisCommands::test_getitem_and_setitem",
"tests/test_commands.py::TestRedisCommands::test_getitem_raises_keyerror_for_missing_key",
"tests/test_commands.py::TestRedisCommands::test_getitem_does_not_raise_keyerror_for_empty_string",
"tests/test_commands.py::TestRedisCommands::test_get_set_bit",
"tests/test_commands.py::TestRedisCommands::test_getrange",
"tests/test_commands.py::TestRedisCommands::test_getset",
"tests/test_commands.py::TestRedisCommands::test_incr",
"tests/test_commands.py::TestRedisCommands::test_incrby",
"tests/test_commands.py::TestRedisCommands::test_incrbyfloat",
"tests/test_commands.py::TestRedisCommands::test_keys",
"tests/test_commands.py::TestRedisCommands::test_mget",
"tests/test_commands.py::TestRedisCommands::test_lmove",
"tests/test_commands.py::TestRedisCommands::test_blmove",
"tests/test_commands.py::TestRedisCommands::test_mset",
"tests/test_commands.py::TestRedisCommands::test_msetnx",
"tests/test_commands.py::TestRedisCommands::test_pexpire",
"tests/test_commands.py::TestRedisCommands::test_pexpire_option_nx",
"tests/test_commands.py::TestRedisCommands::test_pexpire_option_xx",
"tests/test_commands.py::TestRedisCommands::test_pexpire_option_gt",
"tests/test_commands.py::TestRedisCommands::test_pexpire_option_lt",
"tests/test_commands.py::TestRedisCommands::test_pexpireat_datetime",
"tests/test_commands.py::TestRedisCommands::test_pexpireat_no_key",
"tests/test_commands.py::TestRedisCommands::test_pexpireat_unixtime",
"tests/test_commands.py::TestRedisCommands::test_pexpireat_option_nx",
"tests/test_commands.py::TestRedisCommands::test_pexpireat_option_xx",
"tests/test_commands.py::TestRedisCommands::test_pexpireat_option_gt",
"tests/test_commands.py::TestRedisCommands::test_pexpireat_option_lt",
"tests/test_commands.py::TestRedisCommands::test_pexpiretime",
"tests/test_commands.py::TestRedisCommands::test_psetex",
"tests/test_commands.py::TestRedisCommands::test_psetex_timedelta",
"tests/test_commands.py::TestRedisCommands::test_pttl",
"tests/test_commands.py::TestRedisCommands::test_pttl_no_key",
"tests/test_commands.py::TestRedisCommands::test_hrandfield",
"tests/test_commands.py::TestRedisCommands::test_randomkey",
"tests/test_commands.py::TestRedisCommands::test_rename",
"tests/test_commands.py::TestRedisCommands::test_renamenx",
"tests/test_commands.py::TestRedisCommands::test_set_nx",
"tests/test_commands.py::TestRedisCommands::test_set_xx",
"tests/test_commands.py::TestRedisCommands::test_set_px",
"tests/test_commands.py::TestRedisCommands::test_set_px_timedelta",
"tests/test_commands.py::TestRedisCommands::test_set_ex",
"tests/test_commands.py::TestRedisCommands::test_set_ex_str",
"tests/test_commands.py::TestRedisCommands::test_set_ex_timedelta",
"tests/test_commands.py::TestRedisCommands::test_set_exat_timedelta",
"tests/test_commands.py::TestRedisCommands::test_set_pxat_timedelta",
"tests/test_commands.py::TestRedisCommands::test_set_multipleoptions",
"tests/test_commands.py::TestRedisCommands::test_set_keepttl",
"tests/test_commands.py::TestRedisCommands::test_set_get",
"tests/test_commands.py::TestRedisCommands::test_setex",
"tests/test_commands.py::TestRedisCommands::test_setnx",
"tests/test_commands.py::TestRedisCommands::test_setrange",
"tests/test_commands.py::TestRedisCommands::test_strlen",
"tests/test_commands.py::TestRedisCommands::test_substr",
"tests/test_commands.py::TestRedisCommands::test_ttl",
"tests/test_commands.py::TestRedisCommands::test_ttl_nokey",
"tests/test_commands.py::TestRedisCommands::test_type",
"tests/test_commands.py::TestRedisCommands::test_blpop",
"tests/test_commands.py::TestRedisCommands::test_brpop",
"tests/test_commands.py::TestRedisCommands::test_brpoplpush",
"tests/test_commands.py::TestRedisCommands::test_brpoplpush_empty_string",
"tests/test_commands.py::TestRedisCommands::test_blmpop",
"tests/test_commands.py::TestRedisCommands::test_lmpop",
"tests/test_commands.py::TestRedisCommands::test_lindex",
"tests/test_commands.py::TestRedisCommands::test_linsert",
"tests/test_commands.py::TestRedisCommands::test_llen",
"tests/test_commands.py::TestRedisCommands::test_lpop",
"tests/test_commands.py::TestRedisCommands::test_lpop_count",
"tests/test_commands.py::TestRedisCommands::test_lpush",
"tests/test_commands.py::TestRedisCommands::test_lpushx",
"tests/test_commands.py::TestRedisCommands::test_lpushx_with_list",
"tests/test_commands.py::TestRedisCommands::test_lrange",
"tests/test_commands.py::TestRedisCommands::test_lrem",
"tests/test_commands.py::TestRedisCommands::test_lset",
"tests/test_commands.py::TestRedisCommands::test_ltrim",
"tests/test_commands.py::TestRedisCommands::test_rpop",
"tests/test_commands.py::TestRedisCommands::test_rpop_count",
"tests/test_commands.py::TestRedisCommands::test_rpoplpush",
"tests/test_commands.py::TestRedisCommands::test_rpush",
"tests/test_commands.py::TestRedisCommands::test_lpos",
"tests/test_commands.py::TestRedisCommands::test_rpushx",
"tests/test_commands.py::TestRedisCommands::test_scan",
"tests/test_commands.py::TestRedisCommands::test_scan_type",
"tests/test_commands.py::TestRedisCommands::test_scan_iter",
"tests/test_commands.py::TestRedisCommands::test_sscan",
"tests/test_commands.py::TestRedisCommands::test_sscan_iter",
"tests/test_commands.py::TestRedisCommands::test_hscan",
"tests/test_commands.py::TestRedisCommands::test_hscan_iter",
"tests/test_commands.py::TestRedisCommands::test_zscan",
"tests/test_commands.py::TestRedisCommands::test_zscan_iter",
"tests/test_commands.py::TestRedisCommands::test_sadd",
"tests/test_commands.py::TestRedisCommands::test_scard",
"tests/test_commands.py::TestRedisCommands::test_sdiff",
"tests/test_commands.py::TestRedisCommands::test_sdiffstore",
"tests/test_commands.py::TestRedisCommands::test_sinter",
"tests/test_commands.py::TestRedisCommands::test_sintercard",
"tests/test_commands.py::TestRedisCommands::test_sinterstore",
"tests/test_commands.py::TestRedisCommands::test_sismember",
"tests/test_commands.py::TestRedisCommands::test_smembers",
"tests/test_commands.py::TestRedisCommands::test_smismember",
"tests/test_commands.py::TestRedisCommands::test_smove",
"tests/test_commands.py::TestRedisCommands::test_spop",
"tests/test_commands.py::TestRedisCommands::test_spop_multi_value",
"tests/test_commands.py::TestRedisCommands::test_srandmember",
"tests/test_commands.py::TestRedisCommands::test_srandmember_multi_value",
"tests/test_commands.py::TestRedisCommands::test_srem",
"tests/test_commands.py::TestRedisCommands::test_sunion",
"tests/test_commands.py::TestRedisCommands::test_sunionstore",
"tests/test_commands.py::TestRedisCommands::test_debug_segfault",
"tests/test_commands.py::TestRedisCommands::test_script_debug",
"tests/test_commands.py::TestRedisCommands::test_zadd",
"tests/test_commands.py::TestRedisCommands::test_zadd_nx",
"tests/test_commands.py::TestRedisCommands::test_zadd_xx",
"tests/test_commands.py::TestRedisCommands::test_zadd_ch",
"tests/test_commands.py::TestRedisCommands::test_zadd_incr",
"tests/test_commands.py::TestRedisCommands::test_zadd_incr_with_xx",
"tests/test_commands.py::TestRedisCommands::test_zadd_gt_lt",
"tests/test_commands.py::TestRedisCommands::test_zcard",
"tests/test_commands.py::TestRedisCommands::test_zcount",
"tests/test_commands.py::TestRedisCommands::test_zdiff",
"tests/test_commands.py::TestRedisCommands::test_zdiffstore",
"tests/test_commands.py::TestRedisCommands::test_zincrby",
"tests/test_commands.py::TestRedisCommands::test_zlexcount",
"tests/test_commands.py::TestRedisCommands::test_zinter",
"tests/test_commands.py::TestRedisCommands::test_zintercard",
"tests/test_commands.py::TestRedisCommands::test_zinterstore_sum",
"tests/test_commands.py::TestRedisCommands::test_zinterstore_max",
"tests/test_commands.py::TestRedisCommands::test_zinterstore_min",
"tests/test_commands.py::TestRedisCommands::test_zinterstore_with_weight",
"tests/test_commands.py::TestRedisCommands::test_zpopmax",
"tests/test_commands.py::TestRedisCommands::test_zpopmin",
"tests/test_commands.py::TestRedisCommands::test_zrandemember",
"tests/test_commands.py::TestRedisCommands::test_bzpopmax",
"tests/test_commands.py::TestRedisCommands::test_bzpopmin",
"tests/test_commands.py::TestRedisCommands::test_zmpop",
"tests/test_commands.py::TestRedisCommands::test_bzmpop",
"tests/test_commands.py::TestRedisCommands::test_zrange",
"tests/test_commands.py::TestRedisCommands::test_zrange_errors",
"tests/test_commands.py::TestRedisCommands::test_zrange_params",
"tests/test_commands.py::TestRedisCommands::test_zrangestore",
"tests/test_commands.py::TestRedisCommands::test_zrangebylex",
"tests/test_commands.py::TestRedisCommands::test_zrevrangebylex",
"tests/test_commands.py::TestRedisCommands::test_zrangebyscore",
"tests/test_commands.py::TestRedisCommands::test_zrank",
"tests/test_commands.py::TestRedisCommands::test_zrem",
"tests/test_commands.py::TestRedisCommands::test_zrem_multiple_keys",
"tests/test_commands.py::TestRedisCommands::test_zremrangebylex",
"tests/test_commands.py::TestRedisCommands::test_zremrangebyrank",
"tests/test_commands.py::TestRedisCommands::test_zremrangebyscore",
"tests/test_commands.py::TestRedisCommands::test_zrevrange",
"tests/test_commands.py::TestRedisCommands::test_zrevrangebyscore",
"tests/test_commands.py::TestRedisCommands::test_zrevrank",
"tests/test_commands.py::TestRedisCommands::test_zscore",
"tests/test_commands.py::TestRedisCommands::test_zunion",
"tests/test_commands.py::TestRedisCommands::test_zunionstore_sum",
"tests/test_commands.py::TestRedisCommands::test_zunionstore_max",
"tests/test_commands.py::TestRedisCommands::test_zunionstore_min",
"tests/test_commands.py::TestRedisCommands::test_zunionstore_with_weight",
"tests/test_commands.py::TestRedisCommands::test_zmscore",
"tests/test_commands.py::TestRedisCommands::test_pfadd",
"tests/test_commands.py::TestRedisCommands::test_pfcount",
"tests/test_commands.py::TestRedisCommands::test_pfmerge",
"tests/test_commands.py::TestRedisCommands::test_hget_and_hset",
"tests/test_commands.py::TestRedisCommands::test_hset_with_multi_key_values",
"tests/test_commands.py::TestRedisCommands::test_hset_with_key_values_passed_as_list",
"tests/test_commands.py::TestRedisCommands::test_hset_without_data",
"tests/test_commands.py::TestRedisCommands::test_hdel",
"tests/test_commands.py::TestRedisCommands::test_hexists",
"tests/test_commands.py::TestRedisCommands::test_hgetall",
"tests/test_commands.py::TestRedisCommands::test_hincrby",
"tests/test_commands.py::TestRedisCommands::test_hincrbyfloat",
"tests/test_commands.py::TestRedisCommands::test_hkeys",
"tests/test_commands.py::TestRedisCommands::test_hlen",
"tests/test_commands.py::TestRedisCommands::test_hmget",
"tests/test_commands.py::TestRedisCommands::test_hmset",
"tests/test_commands.py::TestRedisCommands::test_hsetnx",
"tests/test_commands.py::TestRedisCommands::test_hvals",
"tests/test_commands.py::TestRedisCommands::test_hstrlen",
"tests/test_commands.py::TestRedisCommands::test_sort_basic",
"tests/test_commands.py::TestRedisCommands::test_sort_limited",
"tests/test_commands.py::TestRedisCommands::test_sort_by",
"tests/test_commands.py::TestRedisCommands::test_sort_get",
"tests/test_commands.py::TestRedisCommands::test_sort_get_multi",
"tests/test_commands.py::TestRedisCommands::test_sort_get_groups_two",
"tests/test_commands.py::TestRedisCommands::test_sort_groups_string_get",
"tests/test_commands.py::TestRedisCommands::test_sort_groups_just_one_get",
"tests/test_commands.py::TestRedisCommands::test_sort_groups_no_get",
"tests/test_commands.py::TestRedisCommands::test_sort_groups_three_gets",
"tests/test_commands.py::TestRedisCommands::test_sort_desc",
"tests/test_commands.py::TestRedisCommands::test_sort_alpha",
"tests/test_commands.py::TestRedisCommands::test_sort_store",
"tests/test_commands.py::TestRedisCommands::test_sort_all_options",
"tests/test_commands.py::TestRedisCommands::test_sort_ro",
"tests/test_commands.py::TestRedisCommands::test_sort_issue_924",
"tests/test_commands.py::TestRedisCommands::test_cluster_addslots",
"tests/test_commands.py::TestRedisCommands::test_cluster_count_failure_reports",
"tests/test_commands.py::TestRedisCommands::test_cluster_countkeysinslot",
"tests/test_commands.py::TestRedisCommands::test_cluster_delslots",
"tests/test_commands.py::TestRedisCommands::test_cluster_failover",
"tests/test_commands.py::TestRedisCommands::test_cluster_forget",
"tests/test_commands.py::TestRedisCommands::test_cluster_info",
"tests/test_commands.py::TestRedisCommands::test_cluster_keyslot",
"tests/test_commands.py::TestRedisCommands::test_cluster_meet",
"tests/test_commands.py::TestRedisCommands::test_cluster_nodes",
"tests/test_commands.py::TestRedisCommands::test_cluster_replicate",
"tests/test_commands.py::TestRedisCommands::test_cluster_reset",
"tests/test_commands.py::TestRedisCommands::test_cluster_saveconfig",
"tests/test_commands.py::TestRedisCommands::test_cluster_setslot",
"tests/test_commands.py::TestRedisCommands::test_cluster_slaves",
"tests/test_commands.py::TestRedisCommands::test_readonly_invalid_cluster_state",
"tests/test_commands.py::TestRedisCommands::test_readonly",
"tests/test_commands.py::TestRedisCommands::test_geoadd",
"tests/test_commands.py::TestRedisCommands::test_geoadd_nx",
"tests/test_commands.py::TestRedisCommands::test_geoadd_xx",
"tests/test_commands.py::TestRedisCommands::test_geoadd_ch",
"tests/test_commands.py::TestRedisCommands::test_geoadd_invalid_params",
"tests/test_commands.py::TestRedisCommands::test_geodist",
"tests/test_commands.py::TestRedisCommands::test_geodist_units",
"tests/test_commands.py::TestRedisCommands::test_geodist_missing_one_member",
"tests/test_commands.py::TestRedisCommands::test_geodist_invalid_units",
"tests/test_commands.py::TestRedisCommands::test_geohash",
"tests/test_commands.py::TestRedisCommands::test_geopos",
"tests/test_commands.py::TestRedisCommands::test_geopos_no_value",
"tests/test_commands.py::TestRedisCommands::test_geosearch",
"tests/test_commands.py::TestRedisCommands::test_geosearch_member",
"tests/test_commands.py::TestRedisCommands::test_geosearch_sort",
"tests/test_commands.py::TestRedisCommands::test_geosearch_with",
"tests/test_commands.py::TestRedisCommands::test_geosearch_negative",
"tests/test_commands.py::TestRedisCommands::test_geosearchstore",
"tests/test_commands.py::TestRedisCommands::test_geosearchstore_dist",
"tests/test_commands.py::TestRedisCommands::test_georadius",
"tests/test_commands.py::TestRedisCommands::test_georadius_no_values",
"tests/test_commands.py::TestRedisCommands::test_georadius_units",
"tests/test_commands.py::TestRedisCommands::test_georadius_with",
"tests/test_commands.py::TestRedisCommands::test_georadius_count",
"tests/test_commands.py::TestRedisCommands::test_georadius_sort",
"tests/test_commands.py::TestRedisCommands::test_georadius_store",
"tests/test_commands.py::TestRedisCommands::test_georadius_store_dist",
"tests/test_commands.py::TestRedisCommands::test_georadiusmember",
"tests/test_commands.py::TestRedisCommands::test_georadiusmember_count",
"tests/test_commands.py::TestRedisCommands::test_xack",
"tests/test_commands.py::TestRedisCommands::test_xadd",
"tests/test_commands.py::TestRedisCommands::test_xadd_nomkstream",
"tests/test_commands.py::TestRedisCommands::test_xadd_minlen_and_limit",
"tests/test_commands.py::TestRedisCommands::test_xadd_explicit_ms",
"tests/test_commands.py::TestRedisCommands::test_xautoclaim_negative",
"tests/test_commands.py::TestRedisCommands::test_xclaim",
"tests/test_commands.py::TestRedisCommands::test_xclaim_trimmed",
"tests/test_commands.py::TestRedisCommands::test_xdel",
"tests/test_commands.py::TestRedisCommands::test_xgroup_create",
"tests/test_commands.py::TestRedisCommands::test_xgroup_create_mkstream",
"tests/test_commands.py::TestRedisCommands::test_xgroup_create_entriesread",
"tests/test_commands.py::TestRedisCommands::test_xgroup_delconsumer",
"tests/test_commands.py::TestRedisCommands::test_xgroup_createconsumer",
"tests/test_commands.py::TestRedisCommands::test_xgroup_destroy",
"tests/test_commands.py::TestRedisCommands::test_xgroup_setid",
"tests/test_commands.py::TestRedisCommands::test_xinfo_stream",
"tests/test_commands.py::TestRedisCommands::test_xinfo_stream_full",
"tests/test_commands.py::TestRedisCommands::test_xlen",
"tests/test_commands.py::TestRedisCommands::test_xpending",
"tests/test_commands.py::TestRedisCommands::test_xpending_range",
"tests/test_commands.py::TestRedisCommands::test_xpending_range_idle",
"tests/test_commands.py::TestRedisCommands::test_xpending_range_negative",
"tests/test_commands.py::TestRedisCommands::test_xrange",
"tests/test_commands.py::TestRedisCommands::test_xread",
"tests/test_commands.py::TestRedisCommands::test_xreadgroup",
"tests/test_commands.py::TestRedisCommands::test_xrevrange",
"tests/test_commands.py::TestRedisCommands::test_xtrim",
"tests/test_commands.py::TestRedisCommands::test_xtrim_minlen_and_length_args",
"tests/test_commands.py::TestRedisCommands::test_bitfield_operations",
"tests/test_commands.py::TestRedisCommands::test_bitfield_ro",
"tests/test_commands.py::TestRedisCommands::test_memory_help",
"tests/test_commands.py::TestRedisCommands::test_memory_doctor",
"tests/test_commands.py::TestRedisCommands::test_memory_malloc_stats",
"tests/test_commands.py::TestRedisCommands::test_memory_stats",
"tests/test_commands.py::TestRedisCommands::test_memory_usage",
"tests/test_commands.py::TestRedisCommands::test_latency_histogram_not_implemented",
"tests/test_commands.py::TestRedisCommands::test_latency_graph_not_implemented",
"tests/test_commands.py::TestRedisCommands::test_latency_doctor_not_implemented",
"tests/test_commands.py::TestRedisCommands::test_latency_history",
"tests/test_commands.py::TestRedisCommands::test_latency_latest",
"tests/test_commands.py::TestRedisCommands::test_latency_reset",
"tests/test_commands.py::TestRedisCommands::test_module_list",
"tests/test_commands.py::TestRedisCommands::test_command_count",
"tests/test_commands.py::TestRedisCommands::test_command_docs",
"tests/test_commands.py::TestRedisCommands::test_command_list",
"tests/test_commands.py::TestRedisCommands::test_command_getkeys",
"tests/test_commands.py::TestRedisCommands::test_command",
"tests/test_commands.py::TestRedisCommands::test_command_getkeysandflags",
"tests/test_commands.py::TestRedisCommands::test_module",
"tests/test_commands.py::TestRedisCommands::test_module_loadex",
"tests/test_commands.py::TestRedisCommands::test_restore",
"tests/test_commands.py::TestRedisCommands::test_restore_idletime",
"tests/test_commands.py::TestRedisCommands::test_restore_frequency",
"tests/test_commands.py::TestRedisCommands::test_replicaof",
"tests/test_commands.py::TestRedisCommands::test_shutdown",
"tests/test_commands.py::TestRedisCommands::test_shutdown_with_params",
"tests/test_commands.py::TestRedisCommands::test_sync",
"tests/test_commands.py::TestRedisCommands::test_psync",
"tests/test_commands.py::TestBinarySave::test_binary_get_set",
"tests/test_commands.py::TestBinarySave::test_binary_lists",
"tests/test_commands.py::TestBinarySave::test_22_info",
"tests/test_commands.py::TestBinarySave::test_large_responses",
"tests/test_commands.py::TestBinarySave::test_floating_point_encoding"
] | [
"tests/test_commands.py::TestRedisCommands::test_georadius_Issue2609"
] | Python | [] | [] |
|
redis/redis-py | 2,732 | redis__redis-py-2732 | [
"2677"
] | bf528fc7f776ce8e926b2e9abfa4e2460d73baa4 | diff --git a/redis/client.py b/redis/client.py
--- a/redis/client.py
+++ b/redis/client.py
@@ -420,9 +420,13 @@ def parse_item(item):
# an O(N) complexity) instead of the command.
if isinstance(item[3], list):
result["command"] = space.join(item[3])
+ result["client_address"] = item[4]
+ result["client_name"] = item[5]
else:
result["complexity"] = item[3]
result["command"] = space.join(item[4])
+ result["client_address"] = item[5]
+ result["client_name"] = item[6]
return result
return [parse_item(item) for item in response]
| diff --git a/tests/test_commands.py b/tests/test_commands.py
--- a/tests/test_commands.py
+++ b/tests/test_commands.py
@@ -861,6 +861,8 @@ def test_slowlog_get(self, r, slowlog):
# make sure other attributes are typed correctly
assert isinstance(slowlog[0]["start_time"], int)
assert isinstance(slowlog[0]["duration"], int)
+ assert isinstance(slowlog[0]["client_address"], bytes)
+ assert isinstance(slowlog[0]["client_name"], bytes)
# Mock result if we didn't get slowlog complexity info.
if "complexity" not in slowlog[0]:
| slowlog_get() return value count less than Redis Doc mentioned and redis-cli returned.
Thanks for wanting to report an issue you've found in redis-py. Please delete this text and fill in the template below.
It is of course not always possible to reduce your code to a small test case, but it's highly appreciated to have as much data as possible. Thank you!
**Version**: What redis-py and what redis version is the issue happening on?
```
redis-py version: 4.5.3 (and 4.5.1 before I upgrade version that try to solve the problem)
redis version: Redis server v=7.0.8 sha=00000000:0 malloc=jemalloc-5.2.1 bits=64 build=12b7d21b977d6ccd
```
**Platform**: What platform / version? (For example Python 3.5.1 on Windows 7 / Ubuntu 15.10 / Azure)
```
Platform: Python 3.8.10 on Ubuntu 20.04-05 TLS
```
**Description**: Description of your issue, stack traces from errors and code that reproduces the issue
As Redis doc mentioned, 'slowlog get' command will return 6 values for each item:
```
Each entry from the slow log is comprised of the following six values:
1. A unique progressive identifier for every slow log entry.
2. The unix timestamp at which the logged command was processed.
3. The amount of time needed for its execution, in microseconds.
4. The array composing the arguments of the command.
5. Client IP address and port.
6. Client name if set via the [CLIENT SETNAME](https://redis.io/commands/client-setname) command.
Doc URL: https://redis.io/commands/slowlog-get/
```
Here is my redis-cli output sample:
```
1) 1) (integer) 19
2) (integer) 1680064492
3) (integer) 10187
4) 1) "LRANGE"
2) "mylist:{8M}}"
3) "0"
4) "599"
5) "192.168.8.1:48132"
6) ""
```
And this is redis-py output sample:
```
[{'id': 673, 'start_time': 1680018547, 'duration': 17716, 'command': b'CLUSTER NODES'}]
```
I have try both execute_command() and slowlog_get() to get result, but no luck.
My code snippet:
```
def get_slowlog(self, num=None):
redis_command='SLOWLOG GET'
try:
# result = self.r.execute_command(redis_command)
if num:
result = self.r.slowlog_get(num=num)
else:
result = self.r.slowlog_get()
```
Remark: where 'r' in the code is Redis client:
```
self.r = Redis(
host=host,
port=port,
db=0,
password=self.PASSWORD,
retry=self.RETRY,
# retry_on_error=self.RETRY_ON_ERROR,
socket_timeout=60,
socket_connect_timeout=10
)
```
| [This](https://github.com/redis/redis-py/blob/master/redis/client.py#L413) is where the response is parsed and needs to be updated to capture the missing fields. | "2023-04-30T15:57:15Z" | 4.5 | [
"tests/test_commands.py::TestResponseCallbacks::test_response_callbacks",
"tests/test_commands.py::TestResponseCallbacks::test_case_insensitive_command_names",
"tests/test_commands.py::TestRedisCommands::test_auth",
"tests/test_commands.py::TestRedisCommands::test_command_on_invalid_key_type",
"tests/test_commands.py::TestRedisCommands::test_acl_cat_no_category",
"tests/test_commands.py::TestRedisCommands::test_acl_cat_with_category",
"tests/test_commands.py::TestRedisCommands::test_acl_deluser",
"tests/test_commands.py::TestRedisCommands::test_acl_genpass",
"tests/test_commands.py::TestRedisCommands::test_acl_help",
"tests/test_commands.py::TestRedisCommands::test_acl_list",
"tests/test_commands.py::TestRedisCommands::test_acl_log",
"tests/test_commands.py::TestRedisCommands::test_acl_setuser_categories_without_prefix_fails",
"tests/test_commands.py::TestRedisCommands::test_acl_setuser_commands_without_prefix_fails",
"tests/test_commands.py::TestRedisCommands::test_acl_setuser_add_passwords_and_nopass_fails",
"tests/test_commands.py::TestRedisCommands::test_acl_users",
"tests/test_commands.py::TestRedisCommands::test_acl_whoami",
"tests/test_commands.py::TestRedisCommands::test_client_list",
"tests/test_commands.py::TestRedisCommands::test_client_info",
"tests/test_commands.py::TestRedisCommands::test_client_list_types_not_replica",
"tests/test_commands.py::TestRedisCommands::test_client_list_replica",
"tests/test_commands.py::TestRedisCommands::test_client_list_client_id",
"tests/test_commands.py::TestRedisCommands::test_client_id",
"tests/test_commands.py::TestRedisCommands::test_client_trackinginfo",
"tests/test_commands.py::TestRedisCommands::test_client_tracking",
"tests/test_commands.py::TestRedisCommands::test_client_unblock",
"tests/test_commands.py::TestRedisCommands::test_client_getname",
"tests/test_commands.py::TestRedisCommands::test_client_setname",
"tests/test_commands.py::TestRedisCommands::test_client_kill",
"tests/test_commands.py::TestRedisCommands::test_client_kill_filter_invalid_params",
"tests/test_commands.py::TestRedisCommands::test_client_kill_filter_by_id",
"tests/test_commands.py::TestRedisCommands::test_client_kill_filter_by_addr",
"tests/test_commands.py::TestRedisCommands::test_client_list_after_client_setname",
"tests/test_commands.py::TestRedisCommands::test_client_kill_filter_by_laddr",
"tests/test_commands.py::TestRedisCommands::test_client_kill_filter_by_user",
"tests/test_commands.py::TestRedisCommands::test_client_pause",
"tests/test_commands.py::TestRedisCommands::test_client_pause_all",
"tests/test_commands.py::TestRedisCommands::test_client_unpause",
"tests/test_commands.py::TestRedisCommands::test_client_no_evict",
"tests/test_commands.py::TestRedisCommands::test_client_reply",
"tests/test_commands.py::TestRedisCommands::test_client_getredir",
"tests/test_commands.py::TestRedisCommands::test_hello_notI_implemented",
"tests/test_commands.py::TestRedisCommands::test_config_get",
"tests/test_commands.py::TestRedisCommands::test_config_get_multi_params",
"tests/test_commands.py::TestRedisCommands::test_config_resetstat",
"tests/test_commands.py::TestRedisCommands::test_config_set",
"tests/test_commands.py::TestRedisCommands::test_config_set_multi_params",
"tests/test_commands.py::TestRedisCommands::test_failover",
"tests/test_commands.py::TestRedisCommands::test_dbsize",
"tests/test_commands.py::TestRedisCommands::test_echo",
"tests/test_commands.py::TestRedisCommands::test_info",
"tests/test_commands.py::TestRedisCommands::test_info_multi_sections",
"tests/test_commands.py::TestRedisCommands::test_lastsave",
"tests/test_commands.py::TestRedisCommands::test_lolwut",
"tests/test_commands.py::TestRedisCommands::test_reset",
"tests/test_commands.py::TestRedisCommands::test_object",
"tests/test_commands.py::TestRedisCommands::test_ping",
"tests/test_commands.py::TestRedisCommands::test_quit",
"tests/test_commands.py::TestRedisCommands::test_role",
"tests/test_commands.py::TestRedisCommands::test_select",
"tests/test_commands.py::TestRedisCommands::test_slowlog_get_limit",
"tests/test_commands.py::TestRedisCommands::test_slowlog_length",
"tests/test_commands.py::TestRedisCommands::test_time",
"tests/test_commands.py::TestRedisCommands::test_bgsave",
"tests/test_commands.py::TestRedisCommands::test_never_decode_option",
"tests/test_commands.py::TestRedisCommands::test_empty_response_option",
"tests/test_commands.py::TestRedisCommands::test_append",
"tests/test_commands.py::TestRedisCommands::test_bitcount",
"tests/test_commands.py::TestRedisCommands::test_bitcount_mode",
"tests/test_commands.py::TestRedisCommands::test_bitop_not_empty_string",
"tests/test_commands.py::TestRedisCommands::test_bitop_not",
"tests/test_commands.py::TestRedisCommands::test_bitop_not_in_place",
"tests/test_commands.py::TestRedisCommands::test_bitop_single_string",
"tests/test_commands.py::TestRedisCommands::test_bitop_string_operands",
"tests/test_commands.py::TestRedisCommands::test_bitpos",
"tests/test_commands.py::TestRedisCommands::test_bitpos_wrong_arguments",
"tests/test_commands.py::TestRedisCommands::test_bitpos_mode",
"tests/test_commands.py::TestRedisCommands::test_copy",
"tests/test_commands.py::TestRedisCommands::test_copy_and_replace",
"tests/test_commands.py::TestRedisCommands::test_copy_to_another_database",
"tests/test_commands.py::TestRedisCommands::test_decr",
"tests/test_commands.py::TestRedisCommands::test_decrby",
"tests/test_commands.py::TestRedisCommands::test_delete",
"tests/test_commands.py::TestRedisCommands::test_delete_with_multiple_keys",
"tests/test_commands.py::TestRedisCommands::test_delitem",
"tests/test_commands.py::TestRedisCommands::test_unlink",
"tests/test_commands.py::TestRedisCommands::test_unlink_with_multiple_keys",
"tests/test_commands.py::TestRedisCommands::test_lcs",
"tests/test_commands.py::TestRedisCommands::test_dump_and_restore",
"tests/test_commands.py::TestRedisCommands::test_dump_and_restore_and_replace",
"tests/test_commands.py::TestRedisCommands::test_dump_and_restore_absttl",
"tests/test_commands.py::TestRedisCommands::test_exists",
"tests/test_commands.py::TestRedisCommands::test_exists_contains",
"tests/test_commands.py::TestRedisCommands::test_expire",
"tests/test_commands.py::TestRedisCommands::test_expire_option_nx",
"tests/test_commands.py::TestRedisCommands::test_expire_option_xx",
"tests/test_commands.py::TestRedisCommands::test_expire_option_gt",
"tests/test_commands.py::TestRedisCommands::test_expire_option_lt",
"tests/test_commands.py::TestRedisCommands::test_expireat_datetime",
"tests/test_commands.py::TestRedisCommands::test_expireat_no_key",
"tests/test_commands.py::TestRedisCommands::test_expireat_unixtime",
"tests/test_commands.py::TestRedisCommands::test_expiretime",
"tests/test_commands.py::TestRedisCommands::test_expireat_option_nx",
"tests/test_commands.py::TestRedisCommands::test_expireat_option_xx",
"tests/test_commands.py::TestRedisCommands::test_expireat_option_gt",
"tests/test_commands.py::TestRedisCommands::test_expireat_option_lt",
"tests/test_commands.py::TestRedisCommands::test_get_and_set",
"tests/test_commands.py::TestRedisCommands::test_getdel",
"tests/test_commands.py::TestRedisCommands::test_getex",
"tests/test_commands.py::TestRedisCommands::test_getitem_and_setitem",
"tests/test_commands.py::TestRedisCommands::test_getitem_raises_keyerror_for_missing_key",
"tests/test_commands.py::TestRedisCommands::test_getitem_does_not_raise_keyerror_for_empty_string",
"tests/test_commands.py::TestRedisCommands::test_get_set_bit",
"tests/test_commands.py::TestRedisCommands::test_getrange",
"tests/test_commands.py::TestRedisCommands::test_getset",
"tests/test_commands.py::TestRedisCommands::test_incr",
"tests/test_commands.py::TestRedisCommands::test_incrby",
"tests/test_commands.py::TestRedisCommands::test_incrbyfloat",
"tests/test_commands.py::TestRedisCommands::test_keys",
"tests/test_commands.py::TestRedisCommands::test_mget",
"tests/test_commands.py::TestRedisCommands::test_lmove",
"tests/test_commands.py::TestRedisCommands::test_blmove",
"tests/test_commands.py::TestRedisCommands::test_mset",
"tests/test_commands.py::TestRedisCommands::test_msetnx",
"tests/test_commands.py::TestRedisCommands::test_pexpire",
"tests/test_commands.py::TestRedisCommands::test_pexpire_option_nx",
"tests/test_commands.py::TestRedisCommands::test_pexpire_option_xx",
"tests/test_commands.py::TestRedisCommands::test_pexpire_option_gt",
"tests/test_commands.py::TestRedisCommands::test_pexpire_option_lt",
"tests/test_commands.py::TestRedisCommands::test_pexpireat_datetime",
"tests/test_commands.py::TestRedisCommands::test_pexpireat_no_key",
"tests/test_commands.py::TestRedisCommands::test_pexpireat_unixtime",
"tests/test_commands.py::TestRedisCommands::test_pexpireat_option_nx",
"tests/test_commands.py::TestRedisCommands::test_pexpireat_option_xx",
"tests/test_commands.py::TestRedisCommands::test_pexpireat_option_gt",
"tests/test_commands.py::TestRedisCommands::test_pexpireat_option_lt",
"tests/test_commands.py::TestRedisCommands::test_pexpiretime",
"tests/test_commands.py::TestRedisCommands::test_psetex",
"tests/test_commands.py::TestRedisCommands::test_psetex_timedelta",
"tests/test_commands.py::TestRedisCommands::test_pttl",
"tests/test_commands.py::TestRedisCommands::test_pttl_no_key",
"tests/test_commands.py::TestRedisCommands::test_hrandfield",
"tests/test_commands.py::TestRedisCommands::test_randomkey",
"tests/test_commands.py::TestRedisCommands::test_rename",
"tests/test_commands.py::TestRedisCommands::test_renamenx",
"tests/test_commands.py::TestRedisCommands::test_set_nx",
"tests/test_commands.py::TestRedisCommands::test_set_xx",
"tests/test_commands.py::TestRedisCommands::test_set_px",
"tests/test_commands.py::TestRedisCommands::test_set_px_timedelta",
"tests/test_commands.py::TestRedisCommands::test_set_ex",
"tests/test_commands.py::TestRedisCommands::test_set_ex_str",
"tests/test_commands.py::TestRedisCommands::test_set_ex_timedelta",
"tests/test_commands.py::TestRedisCommands::test_set_exat_timedelta",
"tests/test_commands.py::TestRedisCommands::test_set_pxat_timedelta",
"tests/test_commands.py::TestRedisCommands::test_set_multipleoptions",
"tests/test_commands.py::TestRedisCommands::test_set_keepttl",
"tests/test_commands.py::TestRedisCommands::test_set_get",
"tests/test_commands.py::TestRedisCommands::test_setex",
"tests/test_commands.py::TestRedisCommands::test_setnx",
"tests/test_commands.py::TestRedisCommands::test_setrange",
"tests/test_commands.py::TestRedisCommands::test_strlen",
"tests/test_commands.py::TestRedisCommands::test_substr",
"tests/test_commands.py::TestRedisCommands::test_ttl",
"tests/test_commands.py::TestRedisCommands::test_ttl_nokey",
"tests/test_commands.py::TestRedisCommands::test_type",
"tests/test_commands.py::TestRedisCommands::test_blpop",
"tests/test_commands.py::TestRedisCommands::test_brpop",
"tests/test_commands.py::TestRedisCommands::test_brpoplpush",
"tests/test_commands.py::TestRedisCommands::test_brpoplpush_empty_string",
"tests/test_commands.py::TestRedisCommands::test_blmpop",
"tests/test_commands.py::TestRedisCommands::test_lmpop",
"tests/test_commands.py::TestRedisCommands::test_lindex",
"tests/test_commands.py::TestRedisCommands::test_linsert",
"tests/test_commands.py::TestRedisCommands::test_llen",
"tests/test_commands.py::TestRedisCommands::test_lpop",
"tests/test_commands.py::TestRedisCommands::test_lpop_count",
"tests/test_commands.py::TestRedisCommands::test_lpush",
"tests/test_commands.py::TestRedisCommands::test_lpushx",
"tests/test_commands.py::TestRedisCommands::test_lpushx_with_list",
"tests/test_commands.py::TestRedisCommands::test_lrange",
"tests/test_commands.py::TestRedisCommands::test_lrem",
"tests/test_commands.py::TestRedisCommands::test_lset",
"tests/test_commands.py::TestRedisCommands::test_ltrim",
"tests/test_commands.py::TestRedisCommands::test_rpop",
"tests/test_commands.py::TestRedisCommands::test_rpop_count",
"tests/test_commands.py::TestRedisCommands::test_rpoplpush",
"tests/test_commands.py::TestRedisCommands::test_rpush",
"tests/test_commands.py::TestRedisCommands::test_lpos",
"tests/test_commands.py::TestRedisCommands::test_rpushx",
"tests/test_commands.py::TestRedisCommands::test_scan",
"tests/test_commands.py::TestRedisCommands::test_scan_type",
"tests/test_commands.py::TestRedisCommands::test_scan_iter",
"tests/test_commands.py::TestRedisCommands::test_sscan",
"tests/test_commands.py::TestRedisCommands::test_sscan_iter",
"tests/test_commands.py::TestRedisCommands::test_hscan",
"tests/test_commands.py::TestRedisCommands::test_hscan_iter",
"tests/test_commands.py::TestRedisCommands::test_zscan",
"tests/test_commands.py::TestRedisCommands::test_zscan_iter",
"tests/test_commands.py::TestRedisCommands::test_sadd",
"tests/test_commands.py::TestRedisCommands::test_scard",
"tests/test_commands.py::TestRedisCommands::test_sdiff",
"tests/test_commands.py::TestRedisCommands::test_sdiffstore",
"tests/test_commands.py::TestRedisCommands::test_sinter",
"tests/test_commands.py::TestRedisCommands::test_sintercard",
"tests/test_commands.py::TestRedisCommands::test_sinterstore",
"tests/test_commands.py::TestRedisCommands::test_sismember",
"tests/test_commands.py::TestRedisCommands::test_smembers",
"tests/test_commands.py::TestRedisCommands::test_smismember",
"tests/test_commands.py::TestRedisCommands::test_smove",
"tests/test_commands.py::TestRedisCommands::test_spop",
"tests/test_commands.py::TestRedisCommands::test_spop_multi_value",
"tests/test_commands.py::TestRedisCommands::test_srandmember",
"tests/test_commands.py::TestRedisCommands::test_srandmember_multi_value",
"tests/test_commands.py::TestRedisCommands::test_srem",
"tests/test_commands.py::TestRedisCommands::test_sunion",
"tests/test_commands.py::TestRedisCommands::test_sunionstore",
"tests/test_commands.py::TestRedisCommands::test_debug_segfault",
"tests/test_commands.py::TestRedisCommands::test_script_debug",
"tests/test_commands.py::TestRedisCommands::test_zadd",
"tests/test_commands.py::TestRedisCommands::test_zadd_nx",
"tests/test_commands.py::TestRedisCommands::test_zadd_xx",
"tests/test_commands.py::TestRedisCommands::test_zadd_ch",
"tests/test_commands.py::TestRedisCommands::test_zadd_incr",
"tests/test_commands.py::TestRedisCommands::test_zadd_incr_with_xx",
"tests/test_commands.py::TestRedisCommands::test_zadd_gt_lt",
"tests/test_commands.py::TestRedisCommands::test_zcard",
"tests/test_commands.py::TestRedisCommands::test_zcount",
"tests/test_commands.py::TestRedisCommands::test_zdiff",
"tests/test_commands.py::TestRedisCommands::test_zdiffstore",
"tests/test_commands.py::TestRedisCommands::test_zincrby",
"tests/test_commands.py::TestRedisCommands::test_zlexcount",
"tests/test_commands.py::TestRedisCommands::test_zinter",
"tests/test_commands.py::TestRedisCommands::test_zintercard",
"tests/test_commands.py::TestRedisCommands::test_zinterstore_sum",
"tests/test_commands.py::TestRedisCommands::test_zinterstore_max",
"tests/test_commands.py::TestRedisCommands::test_zinterstore_min",
"tests/test_commands.py::TestRedisCommands::test_zinterstore_with_weight",
"tests/test_commands.py::TestRedisCommands::test_zpopmax",
"tests/test_commands.py::TestRedisCommands::test_zpopmin",
"tests/test_commands.py::TestRedisCommands::test_zrandemember",
"tests/test_commands.py::TestRedisCommands::test_bzpopmax",
"tests/test_commands.py::TestRedisCommands::test_bzpopmin",
"tests/test_commands.py::TestRedisCommands::test_zmpop",
"tests/test_commands.py::TestRedisCommands::test_bzmpop",
"tests/test_commands.py::TestRedisCommands::test_zrange",
"tests/test_commands.py::TestRedisCommands::test_zrange_errors",
"tests/test_commands.py::TestRedisCommands::test_zrange_params",
"tests/test_commands.py::TestRedisCommands::test_zrangestore",
"tests/test_commands.py::TestRedisCommands::test_zrangebylex",
"tests/test_commands.py::TestRedisCommands::test_zrevrangebylex",
"tests/test_commands.py::TestRedisCommands::test_zrangebyscore",
"tests/test_commands.py::TestRedisCommands::test_zrank",
"tests/test_commands.py::TestRedisCommands::test_zrem",
"tests/test_commands.py::TestRedisCommands::test_zrem_multiple_keys",
"tests/test_commands.py::TestRedisCommands::test_zremrangebylex",
"tests/test_commands.py::TestRedisCommands::test_zremrangebyrank",
"tests/test_commands.py::TestRedisCommands::test_zremrangebyscore",
"tests/test_commands.py::TestRedisCommands::test_zrevrange",
"tests/test_commands.py::TestRedisCommands::test_zrevrangebyscore",
"tests/test_commands.py::TestRedisCommands::test_zrevrank",
"tests/test_commands.py::TestRedisCommands::test_zscore",
"tests/test_commands.py::TestRedisCommands::test_zunion",
"tests/test_commands.py::TestRedisCommands::test_zunionstore_sum",
"tests/test_commands.py::TestRedisCommands::test_zunionstore_max",
"tests/test_commands.py::TestRedisCommands::test_zunionstore_min",
"tests/test_commands.py::TestRedisCommands::test_zunionstore_with_weight",
"tests/test_commands.py::TestRedisCommands::test_zmscore",
"tests/test_commands.py::TestRedisCommands::test_pfadd",
"tests/test_commands.py::TestRedisCommands::test_pfcount",
"tests/test_commands.py::TestRedisCommands::test_pfmerge",
"tests/test_commands.py::TestRedisCommands::test_hget_and_hset",
"tests/test_commands.py::TestRedisCommands::test_hset_with_multi_key_values",
"tests/test_commands.py::TestRedisCommands::test_hset_with_key_values_passed_as_list",
"tests/test_commands.py::TestRedisCommands::test_hset_without_data",
"tests/test_commands.py::TestRedisCommands::test_hdel",
"tests/test_commands.py::TestRedisCommands::test_hexists",
"tests/test_commands.py::TestRedisCommands::test_hgetall",
"tests/test_commands.py::TestRedisCommands::test_hincrby",
"tests/test_commands.py::TestRedisCommands::test_hincrbyfloat",
"tests/test_commands.py::TestRedisCommands::test_hkeys",
"tests/test_commands.py::TestRedisCommands::test_hlen",
"tests/test_commands.py::TestRedisCommands::test_hmget",
"tests/test_commands.py::TestRedisCommands::test_hmset",
"tests/test_commands.py::TestRedisCommands::test_hsetnx",
"tests/test_commands.py::TestRedisCommands::test_hvals",
"tests/test_commands.py::TestRedisCommands::test_hstrlen",
"tests/test_commands.py::TestRedisCommands::test_sort_basic",
"tests/test_commands.py::TestRedisCommands::test_sort_limited",
"tests/test_commands.py::TestRedisCommands::test_sort_by",
"tests/test_commands.py::TestRedisCommands::test_sort_get",
"tests/test_commands.py::TestRedisCommands::test_sort_get_multi",
"tests/test_commands.py::TestRedisCommands::test_sort_get_groups_two",
"tests/test_commands.py::TestRedisCommands::test_sort_groups_string_get",
"tests/test_commands.py::TestRedisCommands::test_sort_groups_just_one_get",
"tests/test_commands.py::TestRedisCommands::test_sort_groups_no_get",
"tests/test_commands.py::TestRedisCommands::test_sort_groups_three_gets",
"tests/test_commands.py::TestRedisCommands::test_sort_desc",
"tests/test_commands.py::TestRedisCommands::test_sort_alpha",
"tests/test_commands.py::TestRedisCommands::test_sort_store",
"tests/test_commands.py::TestRedisCommands::test_sort_all_options",
"tests/test_commands.py::TestRedisCommands::test_sort_ro",
"tests/test_commands.py::TestRedisCommands::test_sort_issue_924",
"tests/test_commands.py::TestRedisCommands::test_cluster_addslots",
"tests/test_commands.py::TestRedisCommands::test_cluster_count_failure_reports",
"tests/test_commands.py::TestRedisCommands::test_cluster_countkeysinslot",
"tests/test_commands.py::TestRedisCommands::test_cluster_delslots",
"tests/test_commands.py::TestRedisCommands::test_cluster_failover",
"tests/test_commands.py::TestRedisCommands::test_cluster_forget",
"tests/test_commands.py::TestRedisCommands::test_cluster_info",
"tests/test_commands.py::TestRedisCommands::test_cluster_keyslot",
"tests/test_commands.py::TestRedisCommands::test_cluster_meet",
"tests/test_commands.py::TestRedisCommands::test_cluster_nodes",
"tests/test_commands.py::TestRedisCommands::test_cluster_replicate",
"tests/test_commands.py::TestRedisCommands::test_cluster_reset",
"tests/test_commands.py::TestRedisCommands::test_cluster_saveconfig",
"tests/test_commands.py::TestRedisCommands::test_cluster_setslot",
"tests/test_commands.py::TestRedisCommands::test_cluster_slaves",
"tests/test_commands.py::TestRedisCommands::test_readonly_invalid_cluster_state",
"tests/test_commands.py::TestRedisCommands::test_readonly",
"tests/test_commands.py::TestRedisCommands::test_geoadd",
"tests/test_commands.py::TestRedisCommands::test_geoadd_nx",
"tests/test_commands.py::TestRedisCommands::test_geoadd_xx",
"tests/test_commands.py::TestRedisCommands::test_geoadd_ch",
"tests/test_commands.py::TestRedisCommands::test_geoadd_invalid_params",
"tests/test_commands.py::TestRedisCommands::test_geodist",
"tests/test_commands.py::TestRedisCommands::test_geodist_units",
"tests/test_commands.py::TestRedisCommands::test_geodist_missing_one_member",
"tests/test_commands.py::TestRedisCommands::test_geodist_invalid_units",
"tests/test_commands.py::TestRedisCommands::test_geohash",
"tests/test_commands.py::TestRedisCommands::test_geopos",
"tests/test_commands.py::TestRedisCommands::test_geopos_no_value",
"tests/test_commands.py::TestRedisCommands::test_geosearch",
"tests/test_commands.py::TestRedisCommands::test_geosearch_member",
"tests/test_commands.py::TestRedisCommands::test_geosearch_sort",
"tests/test_commands.py::TestRedisCommands::test_geosearch_with",
"tests/test_commands.py::TestRedisCommands::test_geosearch_negative",
"tests/test_commands.py::TestRedisCommands::test_geosearchstore",
"tests/test_commands.py::TestRedisCommands::test_geosearchstore_dist",
"tests/test_commands.py::TestRedisCommands::test_georadius_Issue2609",
"tests/test_commands.py::TestRedisCommands::test_georadius",
"tests/test_commands.py::TestRedisCommands::test_georadius_no_values",
"tests/test_commands.py::TestRedisCommands::test_georadius_units",
"tests/test_commands.py::TestRedisCommands::test_georadius_with",
"tests/test_commands.py::TestRedisCommands::test_georadius_count",
"tests/test_commands.py::TestRedisCommands::test_georadius_sort",
"tests/test_commands.py::TestRedisCommands::test_georadius_store",
"tests/test_commands.py::TestRedisCommands::test_georadius_store_dist",
"tests/test_commands.py::TestRedisCommands::test_georadiusmember",
"tests/test_commands.py::TestRedisCommands::test_georadiusmember_count",
"tests/test_commands.py::TestRedisCommands::test_xack",
"tests/test_commands.py::TestRedisCommands::test_xadd",
"tests/test_commands.py::TestRedisCommands::test_xadd_nomkstream",
"tests/test_commands.py::TestRedisCommands::test_xadd_minlen_and_limit",
"tests/test_commands.py::TestRedisCommands::test_xadd_explicit_ms",
"tests/test_commands.py::TestRedisCommands::test_xautoclaim_negative",
"tests/test_commands.py::TestRedisCommands::test_xclaim",
"tests/test_commands.py::TestRedisCommands::test_xclaim_trimmed",
"tests/test_commands.py::TestRedisCommands::test_xdel",
"tests/test_commands.py::TestRedisCommands::test_xgroup_create",
"tests/test_commands.py::TestRedisCommands::test_xgroup_create_mkstream",
"tests/test_commands.py::TestRedisCommands::test_xgroup_create_entriesread",
"tests/test_commands.py::TestRedisCommands::test_xgroup_delconsumer",
"tests/test_commands.py::TestRedisCommands::test_xgroup_createconsumer",
"tests/test_commands.py::TestRedisCommands::test_xgroup_destroy",
"tests/test_commands.py::TestRedisCommands::test_xgroup_setid",
"tests/test_commands.py::TestRedisCommands::test_xinfo_stream",
"tests/test_commands.py::TestRedisCommands::test_xinfo_stream_full",
"tests/test_commands.py::TestRedisCommands::test_xlen",
"tests/test_commands.py::TestRedisCommands::test_xpending",
"tests/test_commands.py::TestRedisCommands::test_xpending_range",
"tests/test_commands.py::TestRedisCommands::test_xpending_range_idle",
"tests/test_commands.py::TestRedisCommands::test_xpending_range_negative",
"tests/test_commands.py::TestRedisCommands::test_xrange",
"tests/test_commands.py::TestRedisCommands::test_xread",
"tests/test_commands.py::TestRedisCommands::test_xreadgroup",
"tests/test_commands.py::TestRedisCommands::test_xrevrange",
"tests/test_commands.py::TestRedisCommands::test_xtrim",
"tests/test_commands.py::TestRedisCommands::test_xtrim_minlen_and_length_args",
"tests/test_commands.py::TestRedisCommands::test_bitfield_operations",
"tests/test_commands.py::TestRedisCommands::test_bitfield_ro",
"tests/test_commands.py::TestRedisCommands::test_memory_help",
"tests/test_commands.py::TestRedisCommands::test_memory_doctor",
"tests/test_commands.py::TestRedisCommands::test_memory_malloc_stats",
"tests/test_commands.py::TestRedisCommands::test_memory_stats",
"tests/test_commands.py::TestRedisCommands::test_memory_usage",
"tests/test_commands.py::TestRedisCommands::test_latency_histogram_not_implemented",
"tests/test_commands.py::TestRedisCommands::test_latency_graph_not_implemented",
"tests/test_commands.py::TestRedisCommands::test_latency_doctor_not_implemented",
"tests/test_commands.py::TestRedisCommands::test_latency_history",
"tests/test_commands.py::TestRedisCommands::test_latency_latest",
"tests/test_commands.py::TestRedisCommands::test_latency_reset",
"tests/test_commands.py::TestRedisCommands::test_module_list",
"tests/test_commands.py::TestRedisCommands::test_command_count",
"tests/test_commands.py::TestRedisCommands::test_command_docs",
"tests/test_commands.py::TestRedisCommands::test_command_list",
"tests/test_commands.py::TestRedisCommands::test_command_getkeys",
"tests/test_commands.py::TestRedisCommands::test_command",
"tests/test_commands.py::TestRedisCommands::test_command_getkeysandflags",
"tests/test_commands.py::TestRedisCommands::test_module",
"tests/test_commands.py::TestRedisCommands::test_module_loadex",
"tests/test_commands.py::TestRedisCommands::test_restore",
"tests/test_commands.py::TestRedisCommands::test_restore_idletime",
"tests/test_commands.py::TestRedisCommands::test_restore_frequency",
"tests/test_commands.py::TestRedisCommands::test_replicaof",
"tests/test_commands.py::TestRedisCommands::test_shutdown",
"tests/test_commands.py::TestRedisCommands::test_shutdown_with_params",
"tests/test_commands.py::TestRedisCommands::test_sync",
"tests/test_commands.py::TestRedisCommands::test_psync",
"tests/test_commands.py::TestBinarySave::test_binary_get_set",
"tests/test_commands.py::TestBinarySave::test_binary_lists",
"tests/test_commands.py::TestBinarySave::test_22_info",
"tests/test_commands.py::TestBinarySave::test_large_responses",
"tests/test_commands.py::TestBinarySave::test_floating_point_encoding"
] | [
"tests/test_commands.py::TestRedisCommands::test_slowlog_get"
] | Python | [] | [] |
redis/redis-py | 2,745 | redis__redis-py-2745 | [
"2681"
] | 3748a8b36d5c765f5d21c6d20b041fa1876021ae | diff --git a/redis/commands/core.py b/redis/commands/core.py
--- a/redis/commands/core.py
+++ b/redis/commands/core.py
@@ -761,6 +761,17 @@ def client_no_evict(self, mode: str) -> Union[Awaitable[str], str]:
"""
return self.execute_command("CLIENT NO-EVICT", mode)
+ def client_no_touch(self, mode: str) -> Union[Awaitable[str], str]:
+ """
+ # The command controls whether commands sent by the client will alter
+ # the LRU/LFU of the keys they access.
+ # When turned on, the current client will not change LFU/LRU stats,
+ # unless it sends the TOUCH command.
+
+ For more information see https://redis.io/commands/client-no-touch
+ """
+ return self.execute_command("CLIENT NO-TOUCH", mode)
+
def command(self, **kwargs):
"""
Returns dict reply of details about all Redis commands.
| diff --git a/tests/test_asyncio/test_commands.py b/tests/test_asyncio/test_commands.py
--- a/tests/test_asyncio/test_commands.py
+++ b/tests/test_asyncio/test_commands.py
@@ -446,6 +446,14 @@ async def test_client_pause(self, r: redis.Redis):
with pytest.raises(exceptions.RedisError):
await r.client_pause(timeout="not an integer")
+ @skip_if_server_version_lt("7.2.0")
+ @pytest.mark.onlynoncluster
+ async def test_client_no_touch(self, r: redis.Redis):
+ assert await r.client_no_touch("ON") == b"OK"
+ assert await r.client_no_touch("OFF") == b"OK"
+ with pytest.raises(TypeError):
+ await r.client_no_touch()
+
async def test_config_get(self, r: redis.Redis):
data = await r.config_get()
assert "maxmemory" in data
diff --git a/tests/test_commands.py b/tests/test_commands.py
--- a/tests/test_commands.py
+++ b/tests/test_commands.py
@@ -693,6 +693,14 @@ def test_client_no_evict(self, r):
with pytest.raises(TypeError):
r.client_no_evict()
+ @pytest.mark.onlynoncluster
+ @skip_if_server_version_lt("7.2.0")
+ def test_client_no_touch(self, r):
+ assert r.client_no_touch("ON") == b"OK"
+ assert r.client_no_touch("OFF") == b"OK"
+ with pytest.raises(TypeError):
+ r.client_no_touch()
+
@pytest.mark.onlynoncluster
@skip_if_server_version_lt("3.2.0")
def test_client_reply(self, r, r_timeout):
| Add support for new redis command CLIENT NO-TOUCH
7.2.0 adds support for the new redis command CLIENT NO-TOUCH. We need to add support, documented [here](https://redis.io/commands/CLIENT-NO-TOUCH).
| "2023-05-04T15:05:26Z" | 4.5 | [
"tests/test_asyncio/test_commands.py::TestResponseCallbacks::test_response_callbacks[single-python-parser]",
"tests/test_asyncio/test_commands.py::TestResponseCallbacks::test_response_callbacks[pool-python-parser]",
"tests/test_asyncio/test_commands.py::TestResponseCallbacks::test_response_callbacks[single-hiredis]",
"tests/test_asyncio/test_commands.py::TestResponseCallbacks::test_response_callbacks[pool-hiredis]",
"tests/test_asyncio/test_commands.py::TestResponseCallbacks::test_case_insensitive_command_names[single-python-parser]",
"tests/test_asyncio/test_commands.py::TestResponseCallbacks::test_case_insensitive_command_names[pool-python-parser]",
"tests/test_asyncio/test_commands.py::TestResponseCallbacks::test_case_insensitive_command_names[single-hiredis]",
"tests/test_asyncio/test_commands.py::TestResponseCallbacks::test_case_insensitive_command_names[pool-hiredis]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_command_on_invalid_key_type[single-python-parser]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_command_on_invalid_key_type[pool-python-parser]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_command_on_invalid_key_type[single-hiredis]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_command_on_invalid_key_type[pool-hiredis]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_acl_cat_no_category[single-python-parser]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_acl_cat_no_category[pool-python-parser]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_acl_cat_no_category[single-hiredis]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_acl_cat_no_category[pool-hiredis]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_acl_cat_with_category[single-python-parser]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_acl_cat_with_category[pool-python-parser]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_acl_cat_with_category[single-hiredis]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_acl_cat_with_category[pool-hiredis]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_acl_deluser[single-python-parser]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_acl_deluser[pool-python-parser]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_acl_deluser[single-hiredis]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_acl_deluser[pool-hiredis]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_acl_genpass[single-python-parser]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_acl_genpass[pool-python-parser]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_acl_genpass[single-hiredis]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_acl_genpass[pool-hiredis]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_acl_log[single-python-parser]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_acl_log[pool-python-parser]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_acl_log[single-hiredis]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_acl_log[pool-hiredis]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_acl_setuser_categories_without_prefix_fails[single-python-parser]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_acl_setuser_categories_without_prefix_fails[pool-python-parser]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_acl_setuser_categories_without_prefix_fails[single-hiredis]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_acl_setuser_categories_without_prefix_fails[pool-hiredis]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_acl_setuser_commands_without_prefix_fails[single-python-parser]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_acl_setuser_commands_without_prefix_fails[pool-python-parser]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_acl_setuser_commands_without_prefix_fails[single-hiredis]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_acl_setuser_commands_without_prefix_fails[pool-hiredis]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_acl_setuser_add_passwords_and_nopass_fails[single-python-parser]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_acl_setuser_add_passwords_and_nopass_fails[pool-python-parser]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_acl_setuser_add_passwords_and_nopass_fails[single-hiredis]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_acl_setuser_add_passwords_and_nopass_fails[pool-hiredis]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_acl_users[single-python-parser]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_acl_users[pool-python-parser]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_acl_users[single-hiredis]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_acl_users[pool-hiredis]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_acl_whoami[single-python-parser]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_acl_whoami[pool-python-parser]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_acl_whoami[single-hiredis]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_acl_whoami[pool-hiredis]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_client_list[single-python-parser]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_client_list[pool-python-parser]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_client_list[single-hiredis]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_client_list[pool-hiredis]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_client_list_type[single-python-parser]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_client_list_type[pool-python-parser]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_client_list_type[single-hiredis]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_client_list_type[pool-hiredis]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_client_id[single-python-parser]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_client_id[pool-python-parser]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_client_id[single-hiredis]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_client_id[pool-hiredis]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_client_unblock[single-python-parser]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_client_unblock[pool-python-parser]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_client_unblock[single-hiredis]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_client_unblock[pool-hiredis]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_client_getname[single-python-parser]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_client_getname[pool-python-parser]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_client_getname[single-hiredis]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_client_getname[pool-hiredis]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_client_setname[single-python-parser]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_client_setname[pool-python-parser]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_client_setname[single-hiredis]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_client_setname[pool-hiredis]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_client_kill[single-python-parser]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_client_kill[pool-python-parser]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_client_kill[single-hiredis]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_client_kill[pool-hiredis]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_client_kill_filter_invalid_params[single-python-parser]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_client_kill_filter_invalid_params[pool-python-parser]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_client_kill_filter_invalid_params[single-hiredis]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_client_kill_filter_invalid_params[pool-hiredis]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_client_kill_filter_by_id[single-python-parser]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_client_kill_filter_by_id[pool-python-parser]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_client_kill_filter_by_id[single-hiredis]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_client_kill_filter_by_id[pool-hiredis]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_client_kill_filter_by_addr[single-python-parser]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_client_kill_filter_by_addr[pool-python-parser]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_client_kill_filter_by_addr[single-hiredis]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_client_kill_filter_by_addr[pool-hiredis]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_client_list_after_client_setname[single-python-parser]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_client_list_after_client_setname[pool-python-parser]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_client_list_after_client_setname[single-hiredis]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_client_list_after_client_setname[pool-hiredis]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_client_pause[single-python-parser]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_client_pause[pool-python-parser]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_client_pause[single-hiredis]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_client_pause[pool-hiredis]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_config_get[single-python-parser]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_config_get[pool-python-parser]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_config_get[single-hiredis]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_config_get[pool-hiredis]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_config_resetstat[single-python-parser]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_config_resetstat[pool-python-parser]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_config_resetstat[single-hiredis]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_config_resetstat[pool-hiredis]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_config_set[single-python-parser]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_config_set[pool-python-parser]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_config_set[single-hiredis]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_config_set[pool-hiredis]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_dbsize[single-python-parser]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_dbsize[pool-python-parser]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_dbsize[single-hiredis]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_dbsize[pool-hiredis]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_echo[single-python-parser]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_echo[pool-python-parser]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_echo[single-hiredis]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_echo[pool-hiredis]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_info[single-python-parser]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_info[pool-python-parser]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_info[single-hiredis]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_info[pool-hiredis]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_lastsave[single-python-parser]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_lastsave[pool-python-parser]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_lastsave[single-hiredis]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_lastsave[pool-hiredis]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_object[single-python-parser]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_object[pool-python-parser]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_object[single-hiredis]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_object[pool-hiredis]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_ping[single-python-parser]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_ping[pool-python-parser]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_ping[single-hiredis]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_ping[pool-hiredis]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_slowlog_get[single-python-parser]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_slowlog_get[pool-python-parser]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_slowlog_get[single-hiredis]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_slowlog_get[pool-hiredis]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_slowlog_get_limit[single-python-parser]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_slowlog_get_limit[pool-python-parser]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_slowlog_get_limit[single-hiredis]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_slowlog_get_limit[pool-hiredis]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_slowlog_length[single-python-parser]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_slowlog_length[pool-python-parser]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_slowlog_length[single-hiredis]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_slowlog_length[pool-hiredis]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_time[single-python-parser]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_time[pool-python-parser]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_time[single-hiredis]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_time[pool-hiredis]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_never_decode_option[single-python-parser]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_never_decode_option[pool-python-parser]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_never_decode_option[single-hiredis]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_never_decode_option[pool-hiredis]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_empty_response_option[single-python-parser]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_empty_response_option[pool-python-parser]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_empty_response_option[single-hiredis]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_empty_response_option[pool-hiredis]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_append[single-python-parser]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_append[pool-python-parser]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_append[single-hiredis]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_append[pool-hiredis]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_bitcount[single-python-parser]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_bitcount[pool-python-parser]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_bitcount[single-hiredis]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_bitcount[pool-hiredis]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_bitop_not_empty_string[single-python-parser]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_bitop_not_empty_string[pool-python-parser]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_bitop_not_empty_string[single-hiredis]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_bitop_not_empty_string[pool-hiredis]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_bitop_not[single-python-parser]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_bitop_not[pool-python-parser]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_bitop_not[single-hiredis]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_bitop_not[pool-hiredis]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_bitop_not_in_place[single-python-parser]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_bitop_not_in_place[pool-python-parser]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_bitop_not_in_place[single-hiredis]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_bitop_not_in_place[pool-hiredis]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_bitop_single_string[single-python-parser]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_bitop_single_string[pool-python-parser]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_bitop_single_string[single-hiredis]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_bitop_single_string[pool-hiredis]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_bitop_string_operands[single-python-parser]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_bitop_string_operands[pool-python-parser]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_bitop_string_operands[single-hiredis]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_bitop_string_operands[pool-hiredis]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_bitpos[single-python-parser]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_bitpos[pool-python-parser]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_bitpos[single-hiredis]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_bitpos[pool-hiredis]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_bitpos_wrong_arguments[single-python-parser]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_bitpos_wrong_arguments[pool-python-parser]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_bitpos_wrong_arguments[single-hiredis]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_bitpos_wrong_arguments[pool-hiredis]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_decr[single-python-parser]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_decr[pool-python-parser]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_decr[single-hiredis]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_decr[pool-hiredis]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_decrby[single-python-parser]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_decrby[pool-python-parser]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_decrby[single-hiredis]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_decrby[pool-hiredis]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_delete[single-python-parser]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_delete[pool-python-parser]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_delete[single-hiredis]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_delete[pool-hiredis]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_delete_with_multiple_keys[single-python-parser]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_delete_with_multiple_keys[pool-python-parser]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_delete_with_multiple_keys[single-hiredis]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_delete_with_multiple_keys[pool-hiredis]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_delitem[single-python-parser]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_delitem[pool-python-parser]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_delitem[single-hiredis]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_delitem[pool-hiredis]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_unlink[single-python-parser]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_unlink[pool-python-parser]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_unlink[single-hiredis]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_unlink[pool-hiredis]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_unlink_with_multiple_keys[single-python-parser]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_unlink_with_multiple_keys[pool-python-parser]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_unlink_with_multiple_keys[single-hiredis]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_unlink_with_multiple_keys[pool-hiredis]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_dump_and_restore[single-python-parser]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_dump_and_restore[pool-python-parser]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_dump_and_restore[single-hiredis]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_dump_and_restore[pool-hiredis]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_dump_and_restore_and_replace[single-python-parser]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_dump_and_restore_and_replace[pool-python-parser]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_dump_and_restore_and_replace[single-hiredis]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_dump_and_restore_and_replace[pool-hiredis]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_dump_and_restore_absttl[single-python-parser]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_dump_and_restore_absttl[pool-python-parser]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_dump_and_restore_absttl[single-hiredis]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_dump_and_restore_absttl[pool-hiredis]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_exists[single-python-parser]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_exists[pool-python-parser]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_exists[single-hiredis]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_exists[pool-hiredis]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_exists_contains[single-python-parser]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_exists_contains[pool-python-parser]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_exists_contains[single-hiredis]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_exists_contains[pool-hiredis]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_expire[single-python-parser]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_expire[pool-python-parser]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_expire[single-hiredis]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_expire[pool-hiredis]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_expireat_datetime[single-python-parser]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_expireat_datetime[pool-python-parser]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_expireat_datetime[single-hiredis]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_expireat_datetime[pool-hiredis]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_expireat_no_key[single-python-parser]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_expireat_no_key[pool-python-parser]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_expireat_no_key[single-hiredis]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_expireat_no_key[pool-hiredis]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_expireat_unixtime[single-python-parser]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_expireat_unixtime[pool-python-parser]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_expireat_unixtime[single-hiredis]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_expireat_unixtime[pool-hiredis]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_get_and_set[single-python-parser]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_get_and_set[pool-python-parser]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_get_and_set[single-hiredis]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_get_and_set[pool-hiredis]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_get_set_bit[single-python-parser]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_get_set_bit[pool-python-parser]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_get_set_bit[single-hiredis]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_get_set_bit[pool-hiredis]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_getrange[single-python-parser]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_getrange[pool-python-parser]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_getrange[single-hiredis]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_getrange[pool-hiredis]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_getset[single-python-parser]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_getset[pool-python-parser]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_getset[single-hiredis]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_getset[pool-hiredis]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_incr[single-python-parser]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_incr[pool-python-parser]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_incr[single-hiredis]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_incr[pool-hiredis]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_incrby[single-python-parser]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_incrby[pool-python-parser]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_incrby[single-hiredis]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_incrby[pool-hiredis]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_incrbyfloat[single-python-parser]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_incrbyfloat[pool-python-parser]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_incrbyfloat[single-hiredis]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_incrbyfloat[pool-hiredis]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_keys[single-python-parser]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_keys[pool-python-parser]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_keys[single-hiredis]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_keys[pool-hiredis]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_mget[single-python-parser]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_mget[pool-python-parser]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_mget[single-hiredis]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_mget[pool-hiredis]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_mset[single-python-parser]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_mset[pool-python-parser]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_mset[single-hiredis]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_mset[pool-hiredis]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_msetnx[single-python-parser]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_msetnx[pool-python-parser]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_msetnx[single-hiredis]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_msetnx[pool-hiredis]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_pexpire[single-python-parser]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_pexpire[pool-python-parser]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_pexpire[single-hiredis]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_pexpire[pool-hiredis]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_pexpireat_datetime[single-python-parser]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_pexpireat_datetime[pool-python-parser]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_pexpireat_datetime[single-hiredis]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_pexpireat_datetime[pool-hiredis]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_pexpireat_no_key[single-python-parser]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_pexpireat_no_key[pool-python-parser]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_pexpireat_no_key[single-hiredis]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_pexpireat_no_key[pool-hiredis]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_pexpireat_unixtime[single-python-parser]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_pexpireat_unixtime[pool-python-parser]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_pexpireat_unixtime[single-hiredis]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_pexpireat_unixtime[pool-hiredis]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_psetex[single-python-parser]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_psetex[pool-python-parser]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_psetex[single-hiredis]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_psetex[pool-hiredis]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_psetex_timedelta[single-python-parser]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_psetex_timedelta[pool-python-parser]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_psetex_timedelta[single-hiredis]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_psetex_timedelta[pool-hiredis]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_pttl[single-python-parser]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_pttl[pool-python-parser]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_pttl[single-hiredis]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_pttl[pool-hiredis]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_pttl_no_key[single-python-parser]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_pttl_no_key[pool-python-parser]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_pttl_no_key[single-hiredis]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_pttl_no_key[pool-hiredis]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_randomkey[single-python-parser]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_randomkey[pool-python-parser]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_randomkey[single-hiredis]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_randomkey[pool-hiredis]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_rename[single-python-parser]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_rename[pool-python-parser]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_rename[single-hiredis]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_rename[pool-hiredis]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_renamenx[single-python-parser]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_renamenx[pool-python-parser]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_renamenx[single-hiredis]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_renamenx[pool-hiredis]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_set_nx[single-python-parser]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_set_nx[pool-python-parser]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_set_nx[single-hiredis]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_set_nx[pool-hiredis]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_set_xx[single-python-parser]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_set_xx[pool-python-parser]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_set_xx[single-hiredis]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_set_xx[pool-hiredis]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_set_px[single-python-parser]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_set_px[pool-python-parser]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_set_px[single-hiredis]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_set_px[pool-hiredis]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_set_px_timedelta[single-python-parser]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_set_px_timedelta[pool-python-parser]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_set_px_timedelta[single-hiredis]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_set_px_timedelta[pool-hiredis]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_set_ex[single-python-parser]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_set_ex[pool-python-parser]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_set_ex[single-hiredis]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_set_ex[pool-hiredis]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_set_ex_timedelta[single-python-parser]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_set_ex_timedelta[pool-python-parser]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_set_ex_timedelta[single-hiredis]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_set_ex_timedelta[pool-hiredis]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_set_multipleoptions[single-python-parser]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_set_multipleoptions[pool-python-parser]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_set_multipleoptions[single-hiredis]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_set_multipleoptions[pool-hiredis]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_set_keepttl[single-python-parser]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_set_keepttl[pool-python-parser]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_set_keepttl[single-hiredis]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_set_keepttl[pool-hiredis]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_setex[single-python-parser]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_setex[pool-python-parser]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_setex[single-hiredis]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_setex[pool-hiredis]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_setnx[single-python-parser]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_setnx[pool-python-parser]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_setnx[single-hiredis]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_setnx[pool-hiredis]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_setrange[single-python-parser]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_setrange[pool-python-parser]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_setrange[single-hiredis]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_setrange[pool-hiredis]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_strlen[single-python-parser]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_strlen[pool-python-parser]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_strlen[single-hiredis]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_strlen[pool-hiredis]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_substr[single-python-parser]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_substr[pool-python-parser]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_substr[single-hiredis]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_substr[pool-hiredis]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_ttl[single-python-parser]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_ttl[pool-python-parser]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_ttl[single-hiredis]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_ttl[pool-hiredis]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_ttl_nokey[single-python-parser]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_ttl_nokey[pool-python-parser]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_ttl_nokey[single-hiredis]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_ttl_nokey[pool-hiredis]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_type[single-python-parser]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_type[pool-python-parser]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_type[single-hiredis]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_type[pool-hiredis]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_blpop[single-python-parser]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_blpop[pool-python-parser]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_blpop[single-hiredis]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_blpop[pool-hiredis]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_brpop[single-python-parser]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_brpop[pool-python-parser]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_brpop[single-hiredis]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_brpop[pool-hiredis]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_brpoplpush[single-python-parser]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_brpoplpush[pool-python-parser]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_brpoplpush[single-hiredis]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_brpoplpush[pool-hiredis]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_brpoplpush_empty_string[single-python-parser]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_brpoplpush_empty_string[pool-python-parser]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_brpoplpush_empty_string[single-hiredis]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_brpoplpush_empty_string[pool-hiredis]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_lindex[single-python-parser]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_lindex[pool-python-parser]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_lindex[single-hiredis]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_lindex[pool-hiredis]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_linsert[single-python-parser]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_linsert[pool-python-parser]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_linsert[single-hiredis]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_linsert[pool-hiredis]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_llen[single-python-parser]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_llen[pool-python-parser]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_llen[single-hiredis]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_llen[pool-hiredis]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_lpop[single-python-parser]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_lpop[pool-python-parser]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_lpop[single-hiredis]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_lpop[pool-hiredis]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_lpush[single-python-parser]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_lpush[pool-python-parser]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_lpush[single-hiredis]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_lpush[pool-hiredis]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_lpushx[single-python-parser]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_lpushx[pool-python-parser]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_lpushx[single-hiredis]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_lpushx[pool-hiredis]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_lrange[single-python-parser]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_lrange[pool-python-parser]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_lrange[single-hiredis]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_lrange[pool-hiredis]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_lrem[single-python-parser]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_lrem[pool-python-parser]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_lrem[single-hiredis]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_lrem[pool-hiredis]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_lset[single-python-parser]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_lset[pool-python-parser]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_lset[single-hiredis]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_lset[pool-hiredis]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_ltrim[single-python-parser]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_ltrim[pool-python-parser]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_ltrim[single-hiredis]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_ltrim[pool-hiredis]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_rpop[single-python-parser]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_rpop[pool-python-parser]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_rpop[single-hiredis]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_rpop[pool-hiredis]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_rpoplpush[single-python-parser]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_rpoplpush[pool-python-parser]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_rpoplpush[single-hiredis]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_rpoplpush[pool-hiredis]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_rpush[single-python-parser]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_rpush[pool-python-parser]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_rpush[single-hiredis]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_rpush[pool-hiredis]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_lpos[single-python-parser]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_lpos[pool-python-parser]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_lpos[single-hiredis]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_lpos[pool-hiredis]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_rpushx[single-python-parser]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_rpushx[pool-python-parser]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_rpushx[single-hiredis]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_rpushx[pool-hiredis]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_scan[single-python-parser]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_scan[pool-python-parser]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_scan[single-hiredis]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_scan[pool-hiredis]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_scan_type[single-python-parser]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_scan_type[pool-python-parser]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_scan_type[single-hiredis]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_scan_type[pool-hiredis]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_scan_iter[single-python-parser]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_scan_iter[pool-python-parser]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_scan_iter[single-hiredis]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_scan_iter[pool-hiredis]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_sscan[single-python-parser]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_sscan[pool-python-parser]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_sscan[single-hiredis]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_sscan[pool-hiredis]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_sscan_iter[single-python-parser]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_sscan_iter[pool-python-parser]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_sscan_iter[single-hiredis]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_sscan_iter[pool-hiredis]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_hscan[single-python-parser]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_hscan[pool-python-parser]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_hscan[single-hiredis]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_hscan[pool-hiredis]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_hscan_iter[single-python-parser]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_hscan_iter[pool-python-parser]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_hscan_iter[single-hiredis]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_hscan_iter[pool-hiredis]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_zscan[single-python-parser]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_zscan[pool-python-parser]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_zscan[single-hiredis]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_zscan[pool-hiredis]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_zscan_iter[single-python-parser]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_zscan_iter[pool-python-parser]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_zscan_iter[single-hiredis]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_zscan_iter[pool-hiredis]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_sadd[single-python-parser]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_sadd[pool-python-parser]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_sadd[single-hiredis]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_sadd[pool-hiredis]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_scard[single-python-parser]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_scard[pool-python-parser]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_scard[single-hiredis]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_scard[pool-hiredis]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_sdiff[single-python-parser]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_sdiff[pool-python-parser]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_sdiff[single-hiredis]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_sdiff[pool-hiredis]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_sdiffstore[single-python-parser]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_sdiffstore[pool-python-parser]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_sdiffstore[single-hiredis]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_sdiffstore[pool-hiredis]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_sinter[single-python-parser]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_sinter[pool-python-parser]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_sinter[single-hiredis]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_sinter[pool-hiredis]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_sinterstore[single-python-parser]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_sinterstore[pool-python-parser]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_sinterstore[single-hiredis]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_sinterstore[pool-hiredis]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_sismember[single-python-parser]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_sismember[pool-python-parser]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_sismember[single-hiredis]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_sismember[pool-hiredis]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_smembers[single-python-parser]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_smembers[pool-python-parser]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_smembers[single-hiredis]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_smembers[pool-hiredis]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_smove[single-python-parser]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_smove[pool-python-parser]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_smove[single-hiredis]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_smove[pool-hiredis]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_spop[single-python-parser]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_spop[pool-python-parser]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_spop[single-hiredis]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_spop[pool-hiredis]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_spop_multi_value[single-python-parser]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_spop_multi_value[pool-python-parser]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_spop_multi_value[single-hiredis]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_spop_multi_value[pool-hiredis]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_srandmember[single-python-parser]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_srandmember[pool-python-parser]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_srandmember[single-hiredis]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_srandmember[pool-hiredis]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_srandmember_multi_value[single-python-parser]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_srandmember_multi_value[pool-python-parser]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_srandmember_multi_value[single-hiredis]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_srandmember_multi_value[pool-hiredis]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_srem[single-python-parser]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_srem[pool-python-parser]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_srem[single-hiredis]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_srem[pool-hiredis]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_sunion[single-python-parser]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_sunion[pool-python-parser]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_sunion[single-hiredis]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_sunion[pool-hiredis]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_sunionstore[single-python-parser]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_sunionstore[pool-python-parser]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_sunionstore[single-hiredis]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_sunionstore[pool-hiredis]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_zadd[single-python-parser]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_zadd[pool-python-parser]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_zadd[single-hiredis]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_zadd[pool-hiredis]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_zadd_nx[single-python-parser]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_zadd_nx[pool-python-parser]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_zadd_nx[single-hiredis]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_zadd_nx[pool-hiredis]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_zadd_xx[single-python-parser]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_zadd_xx[pool-python-parser]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_zadd_xx[single-hiredis]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_zadd_xx[pool-hiredis]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_zadd_ch[single-python-parser]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_zadd_ch[pool-python-parser]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_zadd_ch[single-hiredis]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_zadd_ch[pool-hiredis]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_zadd_incr[single-python-parser]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_zadd_incr[pool-python-parser]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_zadd_incr[single-hiredis]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_zadd_incr[pool-hiredis]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_zadd_incr_with_xx[single-python-parser]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_zadd_incr_with_xx[pool-python-parser]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_zadd_incr_with_xx[single-hiredis]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_zadd_incr_with_xx[pool-hiredis]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_zcard[single-python-parser]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_zcard[pool-python-parser]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_zcard[single-hiredis]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_zcard[pool-hiredis]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_zcount[single-python-parser]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_zcount[pool-python-parser]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_zcount[single-hiredis]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_zcount[pool-hiredis]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_zincrby[single-python-parser]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_zincrby[pool-python-parser]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_zincrby[single-hiredis]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_zincrby[pool-hiredis]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_zlexcount[single-python-parser]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_zlexcount[pool-python-parser]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_zlexcount[single-hiredis]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_zlexcount[pool-hiredis]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_zinterstore_sum[single-python-parser]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_zinterstore_sum[pool-python-parser]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_zinterstore_sum[single-hiredis]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_zinterstore_sum[pool-hiredis]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_zinterstore_max[single-python-parser]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_zinterstore_max[pool-python-parser]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_zinterstore_max[single-hiredis]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_zinterstore_max[pool-hiredis]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_zinterstore_min[single-python-parser]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_zinterstore_min[pool-python-parser]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_zinterstore_min[single-hiredis]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_zinterstore_min[pool-hiredis]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_zinterstore_with_weight[single-python-parser]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_zinterstore_with_weight[pool-python-parser]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_zinterstore_with_weight[single-hiredis]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_zinterstore_with_weight[pool-hiredis]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_zpopmax[single-python-parser]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_zpopmax[pool-python-parser]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_zpopmax[single-hiredis]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_zpopmax[pool-hiredis]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_zpopmin[single-python-parser]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_zpopmin[pool-python-parser]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_zpopmin[single-hiredis]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_zpopmin[pool-hiredis]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_bzpopmax[single-python-parser]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_bzpopmax[pool-python-parser]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_bzpopmax[single-hiredis]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_bzpopmax[pool-hiredis]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_bzpopmin[single-python-parser]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_bzpopmin[pool-python-parser]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_bzpopmin[single-hiredis]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_bzpopmin[pool-hiredis]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_zrange[single-python-parser]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_zrange[pool-python-parser]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_zrange[single-hiredis]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_zrange[pool-hiredis]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_zrangebylex[single-python-parser]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_zrangebylex[pool-python-parser]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_zrangebylex[single-hiredis]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_zrangebylex[pool-hiredis]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_zrevrangebylex[single-python-parser]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_zrevrangebylex[pool-python-parser]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_zrevrangebylex[single-hiredis]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_zrevrangebylex[pool-hiredis]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_zrangebyscore[single-python-parser]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_zrangebyscore[pool-python-parser]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_zrangebyscore[single-hiredis]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_zrangebyscore[pool-hiredis]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_zrank[single-python-parser]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_zrank[pool-python-parser]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_zrank[single-hiredis]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_zrank[pool-hiredis]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_zrem[single-python-parser]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_zrem[pool-python-parser]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_zrem[single-hiredis]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_zrem[pool-hiredis]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_zrem_multiple_keys[single-python-parser]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_zrem_multiple_keys[pool-python-parser]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_zrem_multiple_keys[single-hiredis]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_zrem_multiple_keys[pool-hiredis]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_zremrangebylex[single-python-parser]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_zremrangebylex[pool-python-parser]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_zremrangebylex[single-hiredis]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_zremrangebylex[pool-hiredis]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_zremrangebyrank[single-python-parser]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_zremrangebyrank[pool-python-parser]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_zremrangebyrank[single-hiredis]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_zremrangebyrank[pool-hiredis]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_zremrangebyscore[single-python-parser]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_zremrangebyscore[pool-python-parser]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_zremrangebyscore[single-hiredis]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_zremrangebyscore[pool-hiredis]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_zrevrange[single-python-parser]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_zrevrange[pool-python-parser]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_zrevrange[single-hiredis]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_zrevrange[pool-hiredis]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_zrevrangebyscore[single-python-parser]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_zrevrangebyscore[pool-python-parser]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_zrevrangebyscore[single-hiredis]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_zrevrangebyscore[pool-hiredis]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_zrevrank[single-python-parser]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_zrevrank[pool-python-parser]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_zrevrank[single-hiredis]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_zrevrank[pool-hiredis]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_zscore[single-python-parser]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_zscore[pool-python-parser]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_zscore[single-hiredis]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_zscore[pool-hiredis]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_zunionstore_sum[single-python-parser]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_zunionstore_sum[pool-python-parser]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_zunionstore_sum[single-hiredis]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_zunionstore_sum[pool-hiredis]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_zunionstore_max[single-python-parser]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_zunionstore_max[pool-python-parser]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_zunionstore_max[single-hiredis]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_zunionstore_max[pool-hiredis]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_zunionstore_min[single-python-parser]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_zunionstore_min[pool-python-parser]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_zunionstore_min[single-hiredis]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_zunionstore_min[pool-hiredis]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_zunionstore_with_weight[single-python-parser]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_zunionstore_with_weight[pool-python-parser]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_zunionstore_with_weight[single-hiredis]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_zunionstore_with_weight[pool-hiredis]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_pfadd[single-python-parser]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_pfadd[pool-python-parser]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_pfadd[single-hiredis]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_pfadd[pool-hiredis]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_pfcount[single-python-parser]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_pfcount[pool-python-parser]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_pfcount[single-hiredis]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_pfcount[pool-hiredis]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_pfmerge[single-python-parser]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_pfmerge[pool-python-parser]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_pfmerge[single-hiredis]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_pfmerge[pool-hiredis]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_hget_and_hset[single-python-parser]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_hget_and_hset[pool-python-parser]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_hget_and_hset[single-hiredis]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_hget_and_hset[pool-hiredis]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_hset_with_multi_key_values[single-python-parser]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_hset_with_multi_key_values[pool-python-parser]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_hset_with_multi_key_values[single-hiredis]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_hset_with_multi_key_values[pool-hiredis]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_hset_without_data[single-python-parser]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_hset_without_data[pool-python-parser]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_hset_without_data[single-hiredis]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_hset_without_data[pool-hiredis]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_hdel[single-python-parser]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_hdel[pool-python-parser]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_hdel[single-hiredis]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_hdel[pool-hiredis]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_hexists[single-python-parser]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_hexists[pool-python-parser]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_hexists[single-hiredis]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_hexists[pool-hiredis]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_hgetall[single-python-parser]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_hgetall[pool-python-parser]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_hgetall[single-hiredis]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_hgetall[pool-hiredis]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_hincrby[single-python-parser]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_hincrby[pool-python-parser]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_hincrby[single-hiredis]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_hincrby[pool-hiredis]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_hincrbyfloat[single-python-parser]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_hincrbyfloat[pool-python-parser]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_hincrbyfloat[single-hiredis]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_hincrbyfloat[pool-hiredis]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_hkeys[single-python-parser]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_hkeys[pool-python-parser]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_hkeys[single-hiredis]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_hkeys[pool-hiredis]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_hlen[single-python-parser]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_hlen[pool-python-parser]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_hlen[single-hiredis]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_hlen[pool-hiredis]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_hmget[single-python-parser]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_hmget[pool-python-parser]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_hmget[single-hiredis]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_hmget[pool-hiredis]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_hmset[single-python-parser]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_hmset[pool-python-parser]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_hmset[single-hiredis]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_hmset[pool-hiredis]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_hsetnx[single-python-parser]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_hsetnx[pool-python-parser]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_hsetnx[single-hiredis]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_hsetnx[pool-hiredis]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_hvals[single-python-parser]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_hvals[pool-python-parser]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_hvals[single-hiredis]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_hvals[pool-hiredis]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_hstrlen[single-python-parser]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_hstrlen[pool-python-parser]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_hstrlen[single-hiredis]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_hstrlen[pool-hiredis]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_sort_basic[single-python-parser]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_sort_basic[pool-python-parser]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_sort_basic[single-hiredis]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_sort_basic[pool-hiredis]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_sort_limited[single-python-parser]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_sort_limited[pool-python-parser]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_sort_limited[single-hiredis]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_sort_limited[pool-hiredis]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_sort_by[single-python-parser]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_sort_by[pool-python-parser]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_sort_by[single-hiredis]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_sort_by[pool-hiredis]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_sort_get[single-python-parser]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_sort_get[pool-python-parser]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_sort_get[single-hiredis]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_sort_get[pool-hiredis]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_sort_get_multi[single-python-parser]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_sort_get_multi[pool-python-parser]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_sort_get_multi[single-hiredis]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_sort_get_multi[pool-hiredis]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_sort_get_groups_two[single-python-parser]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_sort_get_groups_two[pool-python-parser]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_sort_get_groups_two[single-hiredis]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_sort_get_groups_two[pool-hiredis]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_sort_groups_string_get[single-python-parser]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_sort_groups_string_get[pool-python-parser]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_sort_groups_string_get[single-hiredis]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_sort_groups_string_get[pool-hiredis]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_sort_groups_just_one_get[single-python-parser]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_sort_groups_just_one_get[pool-python-parser]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_sort_groups_just_one_get[single-hiredis]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_sort_groups_just_one_get[pool-hiredis]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_sort_groups_no_get[single-python-parser]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_sort_groups_no_get[pool-python-parser]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_sort_groups_no_get[single-hiredis]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_sort_groups_no_get[pool-hiredis]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_sort_groups_three_gets[single-python-parser]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_sort_groups_three_gets[pool-python-parser]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_sort_groups_three_gets[single-hiredis]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_sort_groups_three_gets[pool-hiredis]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_sort_desc[single-python-parser]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_sort_desc[pool-python-parser]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_sort_desc[single-hiredis]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_sort_desc[pool-hiredis]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_sort_alpha[single-python-parser]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_sort_alpha[pool-python-parser]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_sort_alpha[single-hiredis]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_sort_alpha[pool-hiredis]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_sort_store[single-python-parser]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_sort_store[pool-python-parser]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_sort_store[single-hiredis]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_sort_store[pool-hiredis]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_sort_all_options[single-python-parser]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_sort_all_options[pool-python-parser]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_sort_all_options[single-hiredis]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_sort_all_options[pool-hiredis]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_sort_issue_924[single-python-parser]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_sort_issue_924[pool-python-parser]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_sort_issue_924[single-hiredis]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_sort_issue_924[pool-hiredis]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_cluster_addslots[single-python-parser]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_cluster_addslots[pool-python-parser]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_cluster_addslots[single-hiredis]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_cluster_addslots[pool-hiredis]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_cluster_count_failure_reports[single-python-parser]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_cluster_count_failure_reports[pool-python-parser]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_cluster_count_failure_reports[single-hiredis]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_cluster_count_failure_reports[pool-hiredis]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_cluster_countkeysinslot[single-python-parser]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_cluster_countkeysinslot[pool-python-parser]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_cluster_countkeysinslot[single-hiredis]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_cluster_countkeysinslot[pool-hiredis]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_cluster_delslots[single-python-parser]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_cluster_delslots[pool-python-parser]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_cluster_delslots[single-hiredis]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_cluster_delslots[pool-hiredis]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_cluster_failover[single-python-parser]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_cluster_failover[pool-python-parser]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_cluster_failover[single-hiredis]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_cluster_failover[pool-hiredis]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_cluster_forget[single-python-parser]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_cluster_forget[pool-python-parser]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_cluster_forget[single-hiredis]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_cluster_forget[pool-hiredis]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_cluster_info[single-python-parser]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_cluster_info[pool-python-parser]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_cluster_info[single-hiredis]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_cluster_info[pool-hiredis]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_cluster_keyslot[single-python-parser]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_cluster_keyslot[pool-python-parser]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_cluster_keyslot[single-hiredis]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_cluster_keyslot[pool-hiredis]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_cluster_meet[single-python-parser]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_cluster_meet[pool-python-parser]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_cluster_meet[single-hiredis]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_cluster_meet[pool-hiredis]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_cluster_nodes[single-python-parser]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_cluster_nodes[pool-python-parser]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_cluster_nodes[single-hiredis]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_cluster_nodes[pool-hiredis]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_cluster_replicate[single-python-parser]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_cluster_replicate[pool-python-parser]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_cluster_replicate[single-hiredis]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_cluster_replicate[pool-hiredis]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_cluster_reset[single-python-parser]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_cluster_reset[pool-python-parser]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_cluster_reset[single-hiredis]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_cluster_reset[pool-hiredis]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_cluster_saveconfig[single-python-parser]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_cluster_saveconfig[pool-python-parser]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_cluster_saveconfig[single-hiredis]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_cluster_saveconfig[pool-hiredis]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_cluster_setslot[single-python-parser]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_cluster_setslot[pool-python-parser]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_cluster_setslot[single-hiredis]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_cluster_setslot[pool-hiredis]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_cluster_slaves[single-python-parser]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_cluster_slaves[pool-python-parser]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_cluster_slaves[single-hiredis]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_cluster_slaves[pool-hiredis]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_readonly_invalid_cluster_state[single-python-parser]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_readonly_invalid_cluster_state[pool-python-parser]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_readonly_invalid_cluster_state[single-hiredis]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_readonly_invalid_cluster_state[pool-hiredis]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_readonly[single-python-parser]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_readonly[pool-python-parser]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_readonly[single-hiredis]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_readonly[pool-hiredis]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_geoadd[single-python-parser]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_geoadd[pool-python-parser]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_geoadd[single-hiredis]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_geoadd[pool-hiredis]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_geoadd_invalid_params[single-python-parser]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_geoadd_invalid_params[pool-python-parser]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_geoadd_invalid_params[single-hiredis]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_geoadd_invalid_params[pool-hiredis]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_geodist[single-python-parser]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_geodist[pool-python-parser]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_geodist[single-hiredis]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_geodist[pool-hiredis]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_geodist_units[single-python-parser]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_geodist_units[pool-python-parser]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_geodist_units[single-hiredis]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_geodist_units[pool-hiredis]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_geodist_missing_one_member[single-python-parser]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_geodist_missing_one_member[pool-python-parser]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_geodist_missing_one_member[single-hiredis]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_geodist_missing_one_member[pool-hiredis]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_geodist_invalid_units[single-python-parser]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_geodist_invalid_units[pool-python-parser]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_geodist_invalid_units[single-hiredis]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_geodist_invalid_units[pool-hiredis]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_geohash[single-python-parser]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_geohash[pool-python-parser]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_geohash[single-hiredis]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_geohash[pool-hiredis]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_geopos[single-python-parser]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_geopos[pool-python-parser]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_geopos[single-hiredis]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_geopos[pool-hiredis]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_geopos_no_value[single-python-parser]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_geopos_no_value[pool-python-parser]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_geopos_no_value[single-hiredis]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_geopos_no_value[pool-hiredis]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_georadius[single-python-parser]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_georadius[pool-python-parser]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_georadius[single-hiredis]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_georadius[pool-hiredis]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_georadius_no_values[single-python-parser]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_georadius_no_values[pool-python-parser]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_georadius_no_values[single-hiredis]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_georadius_no_values[pool-hiredis]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_georadius_units[single-python-parser]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_georadius_units[pool-python-parser]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_georadius_units[single-hiredis]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_georadius_units[pool-hiredis]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_georadius_with[single-python-parser]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_georadius_with[pool-python-parser]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_georadius_with[single-hiredis]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_georadius_with[pool-hiredis]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_georadius_count[single-python-parser]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_georadius_count[pool-python-parser]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_georadius_count[single-hiredis]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_georadius_count[pool-hiredis]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_georadius_sort[single-python-parser]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_georadius_sort[pool-python-parser]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_georadius_sort[single-hiredis]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_georadius_sort[pool-hiredis]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_georadius_store[single-python-parser]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_georadius_store[pool-python-parser]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_georadius_store[single-hiredis]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_georadius_store[pool-hiredis]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_georadius_store_dist[single-python-parser]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_georadius_store_dist[pool-python-parser]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_georadius_store_dist[single-hiredis]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_georadius_store_dist[pool-hiredis]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_georadiusmember[single-python-parser]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_georadiusmember[pool-python-parser]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_georadiusmember[single-hiredis]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_georadiusmember[pool-hiredis]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_xack[single-python-parser]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_xack[pool-python-parser]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_xack[single-hiredis]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_xack[pool-hiredis]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_xadd[single-python-parser]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_xadd[pool-python-parser]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_xadd[single-hiredis]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_xadd[pool-hiredis]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_xclaim[single-python-parser]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_xclaim[pool-python-parser]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_xclaim[single-hiredis]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_xclaim[pool-hiredis]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_xclaim_trimmed[single-python-parser]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_xclaim_trimmed[pool-python-parser]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_xclaim_trimmed[single-hiredis]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_xclaim_trimmed[pool-hiredis]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_xdel[single-python-parser]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_xdel[pool-python-parser]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_xdel[single-hiredis]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_xdel[pool-hiredis]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_xgroup_create[single-python-parser]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_xgroup_create[pool-python-parser]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_xgroup_create[single-hiredis]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_xgroup_create[pool-hiredis]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_xgroup_create_mkstream[single-python-parser]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_xgroup_create_mkstream[pool-python-parser]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_xgroup_create_mkstream[single-hiredis]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_xgroup_create_mkstream[pool-hiredis]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_xgroup_delconsumer[single-python-parser]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_xgroup_delconsumer[pool-python-parser]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_xgroup_delconsumer[single-hiredis]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_xgroup_delconsumer[pool-hiredis]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_xgroup_destroy[single-python-parser]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_xgroup_destroy[pool-python-parser]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_xgroup_destroy[single-hiredis]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_xgroup_destroy[pool-hiredis]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_xgroup_setid[single-python-parser]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_xgroup_setid[pool-python-parser]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_xgroup_setid[single-hiredis]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_xgroup_setid[pool-hiredis]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_xinfo_stream[single-python-parser]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_xinfo_stream[pool-python-parser]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_xinfo_stream[single-hiredis]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_xinfo_stream[pool-hiredis]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_xlen[single-python-parser]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_xlen[pool-python-parser]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_xlen[single-hiredis]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_xlen[pool-hiredis]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_xpending[single-python-parser]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_xpending[pool-python-parser]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_xpending[single-hiredis]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_xpending[pool-hiredis]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_xpending_range[single-python-parser]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_xpending_range[pool-python-parser]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_xpending_range[single-hiredis]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_xpending_range[pool-hiredis]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_xrange[single-python-parser]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_xrange[pool-python-parser]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_xrange[single-hiredis]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_xrange[pool-hiredis]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_xread[single-python-parser]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_xread[pool-python-parser]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_xread[single-hiredis]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_xread[pool-hiredis]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_xreadgroup[single-python-parser]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_xreadgroup[pool-python-parser]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_xreadgroup[single-hiredis]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_xreadgroup[pool-hiredis]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_xrevrange[single-python-parser]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_xrevrange[pool-python-parser]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_xrevrange[single-hiredis]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_xrevrange[pool-hiredis]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_xtrim[single-python-parser]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_xtrim[pool-python-parser]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_xtrim[single-hiredis]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_xtrim[pool-hiredis]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_bitfield_operations[single-python-parser]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_bitfield_operations[pool-python-parser]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_bitfield_operations[single-hiredis]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_bitfield_operations[pool-hiredis]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_bitfield_ro[single-python-parser]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_bitfield_ro[pool-python-parser]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_bitfield_ro[single-hiredis]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_bitfield_ro[pool-hiredis]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_memory_stats[single-python-parser]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_memory_stats[pool-python-parser]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_memory_stats[single-hiredis]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_memory_stats[pool-hiredis]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_memory_usage[single-python-parser]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_memory_usage[pool-python-parser]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_memory_usage[single-hiredis]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_memory_usage[pool-hiredis]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_module_list[single-python-parser]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_module_list[pool-python-parser]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_module_list[single-hiredis]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_module_list[pool-hiredis]",
"tests/test_asyncio/test_commands.py::TestBinarySave::test_binary_get_set[single-python-parser]",
"tests/test_asyncio/test_commands.py::TestBinarySave::test_binary_get_set[pool-python-parser]",
"tests/test_asyncio/test_commands.py::TestBinarySave::test_binary_get_set[single-hiredis]",
"tests/test_asyncio/test_commands.py::TestBinarySave::test_binary_get_set[pool-hiredis]",
"tests/test_asyncio/test_commands.py::TestBinarySave::test_binary_lists[single-python-parser]",
"tests/test_asyncio/test_commands.py::TestBinarySave::test_binary_lists[pool-python-parser]",
"tests/test_asyncio/test_commands.py::TestBinarySave::test_binary_lists[single-hiredis]",
"tests/test_asyncio/test_commands.py::TestBinarySave::test_binary_lists[pool-hiredis]",
"tests/test_asyncio/test_commands.py::TestBinarySave::test_22_info[single-python-parser]",
"tests/test_asyncio/test_commands.py::TestBinarySave::test_22_info[pool-python-parser]",
"tests/test_asyncio/test_commands.py::TestBinarySave::test_22_info[single-hiredis]",
"tests/test_asyncio/test_commands.py::TestBinarySave::test_22_info[pool-hiredis]",
"tests/test_asyncio/test_commands.py::TestBinarySave::test_large_responses[single-python-parser]",
"tests/test_asyncio/test_commands.py::TestBinarySave::test_large_responses[pool-python-parser]",
"tests/test_asyncio/test_commands.py::TestBinarySave::test_large_responses[single-hiredis]",
"tests/test_asyncio/test_commands.py::TestBinarySave::test_large_responses[pool-hiredis]",
"tests/test_asyncio/test_commands.py::TestBinarySave::test_floating_point_encoding[single-python-parser]",
"tests/test_asyncio/test_commands.py::TestBinarySave::test_floating_point_encoding[pool-python-parser]",
"tests/test_asyncio/test_commands.py::TestBinarySave::test_floating_point_encoding[single-hiredis]",
"tests/test_asyncio/test_commands.py::TestBinarySave::test_floating_point_encoding[pool-hiredis]",
"tests/test_commands.py::TestResponseCallbacks::test_response_callbacks",
"tests/test_commands.py::TestResponseCallbacks::test_case_insensitive_command_names",
"tests/test_commands.py::TestRedisCommands::test_auth",
"tests/test_commands.py::TestRedisCommands::test_command_on_invalid_key_type",
"tests/test_commands.py::TestRedisCommands::test_acl_cat_no_category",
"tests/test_commands.py::TestRedisCommands::test_acl_cat_with_category",
"tests/test_commands.py::TestRedisCommands::test_acl_deluser",
"tests/test_commands.py::TestRedisCommands::test_acl_genpass",
"tests/test_commands.py::TestRedisCommands::test_acl_help",
"tests/test_commands.py::TestRedisCommands::test_acl_list",
"tests/test_commands.py::TestRedisCommands::test_acl_log",
"tests/test_commands.py::TestRedisCommands::test_acl_setuser_categories_without_prefix_fails",
"tests/test_commands.py::TestRedisCommands::test_acl_setuser_commands_without_prefix_fails",
"tests/test_commands.py::TestRedisCommands::test_acl_setuser_add_passwords_and_nopass_fails",
"tests/test_commands.py::TestRedisCommands::test_acl_users",
"tests/test_commands.py::TestRedisCommands::test_acl_whoami",
"tests/test_commands.py::TestRedisCommands::test_client_list",
"tests/test_commands.py::TestRedisCommands::test_client_info",
"tests/test_commands.py::TestRedisCommands::test_client_list_types_not_replica",
"tests/test_commands.py::TestRedisCommands::test_client_list_replica",
"tests/test_commands.py::TestRedisCommands::test_client_list_client_id",
"tests/test_commands.py::TestRedisCommands::test_client_id",
"tests/test_commands.py::TestRedisCommands::test_client_trackinginfo",
"tests/test_commands.py::TestRedisCommands::test_client_tracking",
"tests/test_commands.py::TestRedisCommands::test_client_unblock",
"tests/test_commands.py::TestRedisCommands::test_client_getname",
"tests/test_commands.py::TestRedisCommands::test_client_setname",
"tests/test_commands.py::TestRedisCommands::test_client_kill",
"tests/test_commands.py::TestRedisCommands::test_client_kill_filter_invalid_params",
"tests/test_commands.py::TestRedisCommands::test_client_kill_filter_by_id",
"tests/test_commands.py::TestRedisCommands::test_client_kill_filter_by_addr",
"tests/test_commands.py::TestRedisCommands::test_client_list_after_client_setname",
"tests/test_commands.py::TestRedisCommands::test_client_kill_filter_by_laddr",
"tests/test_commands.py::TestRedisCommands::test_client_kill_filter_by_user",
"tests/test_commands.py::TestRedisCommands::test_client_pause",
"tests/test_commands.py::TestRedisCommands::test_client_pause_all",
"tests/test_commands.py::TestRedisCommands::test_client_unpause",
"tests/test_commands.py::TestRedisCommands::test_client_no_evict",
"tests/test_commands.py::TestRedisCommands::test_client_reply",
"tests/test_commands.py::TestRedisCommands::test_client_getredir",
"tests/test_commands.py::TestRedisCommands::test_hello_notI_implemented",
"tests/test_commands.py::TestRedisCommands::test_config_get",
"tests/test_commands.py::TestRedisCommands::test_config_get_multi_params",
"tests/test_commands.py::TestRedisCommands::test_config_resetstat",
"tests/test_commands.py::TestRedisCommands::test_config_set",
"tests/test_commands.py::TestRedisCommands::test_config_set_multi_params",
"tests/test_commands.py::TestRedisCommands::test_failover",
"tests/test_commands.py::TestRedisCommands::test_dbsize",
"tests/test_commands.py::TestRedisCommands::test_echo",
"tests/test_commands.py::TestRedisCommands::test_info",
"tests/test_commands.py::TestRedisCommands::test_info_multi_sections",
"tests/test_commands.py::TestRedisCommands::test_lastsave",
"tests/test_commands.py::TestRedisCommands::test_lolwut",
"tests/test_commands.py::TestRedisCommands::test_reset",
"tests/test_commands.py::TestRedisCommands::test_object",
"tests/test_commands.py::TestRedisCommands::test_ping",
"tests/test_commands.py::TestRedisCommands::test_quit",
"tests/test_commands.py::TestRedisCommands::test_role",
"tests/test_commands.py::TestRedisCommands::test_select",
"tests/test_commands.py::TestRedisCommands::test_slowlog_get",
"tests/test_commands.py::TestRedisCommands::test_slowlog_get_limit",
"tests/test_commands.py::TestRedisCommands::test_slowlog_length",
"tests/test_commands.py::TestRedisCommands::test_time",
"tests/test_commands.py::TestRedisCommands::test_bgsave",
"tests/test_commands.py::TestRedisCommands::test_never_decode_option",
"tests/test_commands.py::TestRedisCommands::test_empty_response_option",
"tests/test_commands.py::TestRedisCommands::test_append",
"tests/test_commands.py::TestRedisCommands::test_bitcount",
"tests/test_commands.py::TestRedisCommands::test_bitcount_mode",
"tests/test_commands.py::TestRedisCommands::test_bitop_not_empty_string",
"tests/test_commands.py::TestRedisCommands::test_bitop_not",
"tests/test_commands.py::TestRedisCommands::test_bitop_not_in_place",
"tests/test_commands.py::TestRedisCommands::test_bitop_single_string",
"tests/test_commands.py::TestRedisCommands::test_bitop_string_operands",
"tests/test_commands.py::TestRedisCommands::test_bitpos",
"tests/test_commands.py::TestRedisCommands::test_bitpos_wrong_arguments",
"tests/test_commands.py::TestRedisCommands::test_bitpos_mode",
"tests/test_commands.py::TestRedisCommands::test_copy",
"tests/test_commands.py::TestRedisCommands::test_copy_and_replace",
"tests/test_commands.py::TestRedisCommands::test_copy_to_another_database",
"tests/test_commands.py::TestRedisCommands::test_decr",
"tests/test_commands.py::TestRedisCommands::test_decrby",
"tests/test_commands.py::TestRedisCommands::test_delete",
"tests/test_commands.py::TestRedisCommands::test_delete_with_multiple_keys",
"tests/test_commands.py::TestRedisCommands::test_delitem",
"tests/test_commands.py::TestRedisCommands::test_unlink",
"tests/test_commands.py::TestRedisCommands::test_unlink_with_multiple_keys",
"tests/test_commands.py::TestRedisCommands::test_lcs",
"tests/test_commands.py::TestRedisCommands::test_dump_and_restore",
"tests/test_commands.py::TestRedisCommands::test_dump_and_restore_and_replace",
"tests/test_commands.py::TestRedisCommands::test_dump_and_restore_absttl",
"tests/test_commands.py::TestRedisCommands::test_exists",
"tests/test_commands.py::TestRedisCommands::test_exists_contains",
"tests/test_commands.py::TestRedisCommands::test_expire",
"tests/test_commands.py::TestRedisCommands::test_expire_option_nx",
"tests/test_commands.py::TestRedisCommands::test_expire_option_xx",
"tests/test_commands.py::TestRedisCommands::test_expire_option_gt",
"tests/test_commands.py::TestRedisCommands::test_expire_option_lt",
"tests/test_commands.py::TestRedisCommands::test_expireat_datetime",
"tests/test_commands.py::TestRedisCommands::test_expireat_no_key",
"tests/test_commands.py::TestRedisCommands::test_expireat_unixtime",
"tests/test_commands.py::TestRedisCommands::test_expiretime",
"tests/test_commands.py::TestRedisCommands::test_expireat_option_nx",
"tests/test_commands.py::TestRedisCommands::test_expireat_option_xx",
"tests/test_commands.py::TestRedisCommands::test_expireat_option_gt",
"tests/test_commands.py::TestRedisCommands::test_expireat_option_lt",
"tests/test_commands.py::TestRedisCommands::test_get_and_set",
"tests/test_commands.py::TestRedisCommands::test_getdel",
"tests/test_commands.py::TestRedisCommands::test_getex",
"tests/test_commands.py::TestRedisCommands::test_getitem_and_setitem",
"tests/test_commands.py::TestRedisCommands::test_getitem_raises_keyerror_for_missing_key",
"tests/test_commands.py::TestRedisCommands::test_getitem_does_not_raise_keyerror_for_empty_string",
"tests/test_commands.py::TestRedisCommands::test_get_set_bit",
"tests/test_commands.py::TestRedisCommands::test_getrange",
"tests/test_commands.py::TestRedisCommands::test_getset",
"tests/test_commands.py::TestRedisCommands::test_incr",
"tests/test_commands.py::TestRedisCommands::test_incrby",
"tests/test_commands.py::TestRedisCommands::test_incrbyfloat",
"tests/test_commands.py::TestRedisCommands::test_keys",
"tests/test_commands.py::TestRedisCommands::test_mget",
"tests/test_commands.py::TestRedisCommands::test_lmove",
"tests/test_commands.py::TestRedisCommands::test_blmove",
"tests/test_commands.py::TestRedisCommands::test_mset",
"tests/test_commands.py::TestRedisCommands::test_msetnx",
"tests/test_commands.py::TestRedisCommands::test_pexpire",
"tests/test_commands.py::TestRedisCommands::test_pexpire_option_nx",
"tests/test_commands.py::TestRedisCommands::test_pexpire_option_xx",
"tests/test_commands.py::TestRedisCommands::test_pexpire_option_gt",
"tests/test_commands.py::TestRedisCommands::test_pexpire_option_lt",
"tests/test_commands.py::TestRedisCommands::test_pexpireat_datetime",
"tests/test_commands.py::TestRedisCommands::test_pexpireat_no_key",
"tests/test_commands.py::TestRedisCommands::test_pexpireat_unixtime",
"tests/test_commands.py::TestRedisCommands::test_pexpireat_option_nx",
"tests/test_commands.py::TestRedisCommands::test_pexpireat_option_xx",
"tests/test_commands.py::TestRedisCommands::test_pexpireat_option_gt",
"tests/test_commands.py::TestRedisCommands::test_pexpireat_option_lt",
"tests/test_commands.py::TestRedisCommands::test_pexpiretime",
"tests/test_commands.py::TestRedisCommands::test_psetex",
"tests/test_commands.py::TestRedisCommands::test_psetex_timedelta",
"tests/test_commands.py::TestRedisCommands::test_pttl",
"tests/test_commands.py::TestRedisCommands::test_pttl_no_key",
"tests/test_commands.py::TestRedisCommands::test_hrandfield",
"tests/test_commands.py::TestRedisCommands::test_randomkey",
"tests/test_commands.py::TestRedisCommands::test_rename",
"tests/test_commands.py::TestRedisCommands::test_renamenx",
"tests/test_commands.py::TestRedisCommands::test_set_nx",
"tests/test_commands.py::TestRedisCommands::test_set_xx",
"tests/test_commands.py::TestRedisCommands::test_set_px",
"tests/test_commands.py::TestRedisCommands::test_set_px_timedelta",
"tests/test_commands.py::TestRedisCommands::test_set_ex",
"tests/test_commands.py::TestRedisCommands::test_set_ex_str",
"tests/test_commands.py::TestRedisCommands::test_set_ex_timedelta",
"tests/test_commands.py::TestRedisCommands::test_set_exat_timedelta",
"tests/test_commands.py::TestRedisCommands::test_set_pxat_timedelta",
"tests/test_commands.py::TestRedisCommands::test_set_multipleoptions",
"tests/test_commands.py::TestRedisCommands::test_set_keepttl",
"tests/test_commands.py::TestRedisCommands::test_set_get",
"tests/test_commands.py::TestRedisCommands::test_setex",
"tests/test_commands.py::TestRedisCommands::test_setnx",
"tests/test_commands.py::TestRedisCommands::test_setrange",
"tests/test_commands.py::TestRedisCommands::test_strlen",
"tests/test_commands.py::TestRedisCommands::test_substr",
"tests/test_commands.py::TestRedisCommands::test_ttl",
"tests/test_commands.py::TestRedisCommands::test_ttl_nokey",
"tests/test_commands.py::TestRedisCommands::test_type",
"tests/test_commands.py::TestRedisCommands::test_blpop",
"tests/test_commands.py::TestRedisCommands::test_brpop",
"tests/test_commands.py::TestRedisCommands::test_brpoplpush",
"tests/test_commands.py::TestRedisCommands::test_brpoplpush_empty_string",
"tests/test_commands.py::TestRedisCommands::test_blmpop",
"tests/test_commands.py::TestRedisCommands::test_lmpop",
"tests/test_commands.py::TestRedisCommands::test_lindex",
"tests/test_commands.py::TestRedisCommands::test_linsert",
"tests/test_commands.py::TestRedisCommands::test_llen",
"tests/test_commands.py::TestRedisCommands::test_lpop",
"tests/test_commands.py::TestRedisCommands::test_lpop_count",
"tests/test_commands.py::TestRedisCommands::test_lpush",
"tests/test_commands.py::TestRedisCommands::test_lpushx",
"tests/test_commands.py::TestRedisCommands::test_lpushx_with_list",
"tests/test_commands.py::TestRedisCommands::test_lrange",
"tests/test_commands.py::TestRedisCommands::test_lrem",
"tests/test_commands.py::TestRedisCommands::test_lset",
"tests/test_commands.py::TestRedisCommands::test_ltrim",
"tests/test_commands.py::TestRedisCommands::test_rpop",
"tests/test_commands.py::TestRedisCommands::test_rpop_count",
"tests/test_commands.py::TestRedisCommands::test_rpoplpush",
"tests/test_commands.py::TestRedisCommands::test_rpush",
"tests/test_commands.py::TestRedisCommands::test_lpos",
"tests/test_commands.py::TestRedisCommands::test_rpushx",
"tests/test_commands.py::TestRedisCommands::test_scan",
"tests/test_commands.py::TestRedisCommands::test_scan_type",
"tests/test_commands.py::TestRedisCommands::test_scan_iter",
"tests/test_commands.py::TestRedisCommands::test_sscan",
"tests/test_commands.py::TestRedisCommands::test_sscan_iter",
"tests/test_commands.py::TestRedisCommands::test_hscan",
"tests/test_commands.py::TestRedisCommands::test_hscan_iter",
"tests/test_commands.py::TestRedisCommands::test_zscan",
"tests/test_commands.py::TestRedisCommands::test_zscan_iter",
"tests/test_commands.py::TestRedisCommands::test_sadd",
"tests/test_commands.py::TestRedisCommands::test_scard",
"tests/test_commands.py::TestRedisCommands::test_sdiff",
"tests/test_commands.py::TestRedisCommands::test_sdiffstore",
"tests/test_commands.py::TestRedisCommands::test_sinter",
"tests/test_commands.py::TestRedisCommands::test_sintercard",
"tests/test_commands.py::TestRedisCommands::test_sinterstore",
"tests/test_commands.py::TestRedisCommands::test_sismember",
"tests/test_commands.py::TestRedisCommands::test_smembers",
"tests/test_commands.py::TestRedisCommands::test_smismember",
"tests/test_commands.py::TestRedisCommands::test_smove",
"tests/test_commands.py::TestRedisCommands::test_spop",
"tests/test_commands.py::TestRedisCommands::test_spop_multi_value",
"tests/test_commands.py::TestRedisCommands::test_srandmember",
"tests/test_commands.py::TestRedisCommands::test_srandmember_multi_value",
"tests/test_commands.py::TestRedisCommands::test_srem",
"tests/test_commands.py::TestRedisCommands::test_sunion",
"tests/test_commands.py::TestRedisCommands::test_sunionstore",
"tests/test_commands.py::TestRedisCommands::test_debug_segfault",
"tests/test_commands.py::TestRedisCommands::test_script_debug",
"tests/test_commands.py::TestRedisCommands::test_zadd",
"tests/test_commands.py::TestRedisCommands::test_zadd_nx",
"tests/test_commands.py::TestRedisCommands::test_zadd_xx",
"tests/test_commands.py::TestRedisCommands::test_zadd_ch",
"tests/test_commands.py::TestRedisCommands::test_zadd_incr",
"tests/test_commands.py::TestRedisCommands::test_zadd_incr_with_xx",
"tests/test_commands.py::TestRedisCommands::test_zadd_gt_lt",
"tests/test_commands.py::TestRedisCommands::test_zcard",
"tests/test_commands.py::TestRedisCommands::test_zcount",
"tests/test_commands.py::TestRedisCommands::test_zdiff",
"tests/test_commands.py::TestRedisCommands::test_zdiffstore",
"tests/test_commands.py::TestRedisCommands::test_zincrby",
"tests/test_commands.py::TestRedisCommands::test_zlexcount",
"tests/test_commands.py::TestRedisCommands::test_zinter",
"tests/test_commands.py::TestRedisCommands::test_zintercard",
"tests/test_commands.py::TestRedisCommands::test_zinterstore_sum",
"tests/test_commands.py::TestRedisCommands::test_zinterstore_max",
"tests/test_commands.py::TestRedisCommands::test_zinterstore_min",
"tests/test_commands.py::TestRedisCommands::test_zinterstore_with_weight",
"tests/test_commands.py::TestRedisCommands::test_zpopmax",
"tests/test_commands.py::TestRedisCommands::test_zpopmin",
"tests/test_commands.py::TestRedisCommands::test_zrandemember",
"tests/test_commands.py::TestRedisCommands::test_bzpopmax",
"tests/test_commands.py::TestRedisCommands::test_bzpopmin",
"tests/test_commands.py::TestRedisCommands::test_zmpop",
"tests/test_commands.py::TestRedisCommands::test_bzmpop",
"tests/test_commands.py::TestRedisCommands::test_zrange",
"tests/test_commands.py::TestRedisCommands::test_zrange_errors",
"tests/test_commands.py::TestRedisCommands::test_zrange_params",
"tests/test_commands.py::TestRedisCommands::test_zrangestore",
"tests/test_commands.py::TestRedisCommands::test_zrangebylex",
"tests/test_commands.py::TestRedisCommands::test_zrevrangebylex",
"tests/test_commands.py::TestRedisCommands::test_zrangebyscore",
"tests/test_commands.py::TestRedisCommands::test_zrank",
"tests/test_commands.py::TestRedisCommands::test_zrem",
"tests/test_commands.py::TestRedisCommands::test_zrem_multiple_keys",
"tests/test_commands.py::TestRedisCommands::test_zremrangebylex",
"tests/test_commands.py::TestRedisCommands::test_zremrangebyrank",
"tests/test_commands.py::TestRedisCommands::test_zremrangebyscore",
"tests/test_commands.py::TestRedisCommands::test_zrevrange",
"tests/test_commands.py::TestRedisCommands::test_zrevrangebyscore",
"tests/test_commands.py::TestRedisCommands::test_zrevrank",
"tests/test_commands.py::TestRedisCommands::test_zscore",
"tests/test_commands.py::TestRedisCommands::test_zunion",
"tests/test_commands.py::TestRedisCommands::test_zunionstore_sum",
"tests/test_commands.py::TestRedisCommands::test_zunionstore_max",
"tests/test_commands.py::TestRedisCommands::test_zunionstore_min",
"tests/test_commands.py::TestRedisCommands::test_zunionstore_with_weight",
"tests/test_commands.py::TestRedisCommands::test_zmscore",
"tests/test_commands.py::TestRedisCommands::test_pfadd",
"tests/test_commands.py::TestRedisCommands::test_pfcount",
"tests/test_commands.py::TestRedisCommands::test_pfmerge",
"tests/test_commands.py::TestRedisCommands::test_hget_and_hset",
"tests/test_commands.py::TestRedisCommands::test_hset_with_multi_key_values",
"tests/test_commands.py::TestRedisCommands::test_hset_with_key_values_passed_as_list",
"tests/test_commands.py::TestRedisCommands::test_hset_without_data",
"tests/test_commands.py::TestRedisCommands::test_hdel",
"tests/test_commands.py::TestRedisCommands::test_hexists",
"tests/test_commands.py::TestRedisCommands::test_hgetall",
"tests/test_commands.py::TestRedisCommands::test_hincrby",
"tests/test_commands.py::TestRedisCommands::test_hincrbyfloat",
"tests/test_commands.py::TestRedisCommands::test_hkeys",
"tests/test_commands.py::TestRedisCommands::test_hlen",
"tests/test_commands.py::TestRedisCommands::test_hmget",
"tests/test_commands.py::TestRedisCommands::test_hmset",
"tests/test_commands.py::TestRedisCommands::test_hsetnx",
"tests/test_commands.py::TestRedisCommands::test_hvals",
"tests/test_commands.py::TestRedisCommands::test_hstrlen",
"tests/test_commands.py::TestRedisCommands::test_sort_basic",
"tests/test_commands.py::TestRedisCommands::test_sort_limited",
"tests/test_commands.py::TestRedisCommands::test_sort_by",
"tests/test_commands.py::TestRedisCommands::test_sort_get",
"tests/test_commands.py::TestRedisCommands::test_sort_get_multi",
"tests/test_commands.py::TestRedisCommands::test_sort_get_groups_two",
"tests/test_commands.py::TestRedisCommands::test_sort_groups_string_get",
"tests/test_commands.py::TestRedisCommands::test_sort_groups_just_one_get",
"tests/test_commands.py::TestRedisCommands::test_sort_groups_no_get",
"tests/test_commands.py::TestRedisCommands::test_sort_groups_three_gets",
"tests/test_commands.py::TestRedisCommands::test_sort_desc",
"tests/test_commands.py::TestRedisCommands::test_sort_alpha",
"tests/test_commands.py::TestRedisCommands::test_sort_store",
"tests/test_commands.py::TestRedisCommands::test_sort_all_options",
"tests/test_commands.py::TestRedisCommands::test_sort_ro",
"tests/test_commands.py::TestRedisCommands::test_sort_issue_924",
"tests/test_commands.py::TestRedisCommands::test_cluster_addslots",
"tests/test_commands.py::TestRedisCommands::test_cluster_count_failure_reports",
"tests/test_commands.py::TestRedisCommands::test_cluster_countkeysinslot",
"tests/test_commands.py::TestRedisCommands::test_cluster_delslots",
"tests/test_commands.py::TestRedisCommands::test_cluster_failover",
"tests/test_commands.py::TestRedisCommands::test_cluster_forget",
"tests/test_commands.py::TestRedisCommands::test_cluster_info",
"tests/test_commands.py::TestRedisCommands::test_cluster_keyslot",
"tests/test_commands.py::TestRedisCommands::test_cluster_meet",
"tests/test_commands.py::TestRedisCommands::test_cluster_nodes",
"tests/test_commands.py::TestRedisCommands::test_cluster_replicate",
"tests/test_commands.py::TestRedisCommands::test_cluster_reset",
"tests/test_commands.py::TestRedisCommands::test_cluster_saveconfig",
"tests/test_commands.py::TestRedisCommands::test_cluster_setslot",
"tests/test_commands.py::TestRedisCommands::test_cluster_slaves",
"tests/test_commands.py::TestRedisCommands::test_readonly_invalid_cluster_state",
"tests/test_commands.py::TestRedisCommands::test_readonly",
"tests/test_commands.py::TestRedisCommands::test_geoadd",
"tests/test_commands.py::TestRedisCommands::test_geoadd_nx",
"tests/test_commands.py::TestRedisCommands::test_geoadd_xx",
"tests/test_commands.py::TestRedisCommands::test_geoadd_ch",
"tests/test_commands.py::TestRedisCommands::test_geoadd_invalid_params",
"tests/test_commands.py::TestRedisCommands::test_geodist",
"tests/test_commands.py::TestRedisCommands::test_geodist_units",
"tests/test_commands.py::TestRedisCommands::test_geodist_missing_one_member",
"tests/test_commands.py::TestRedisCommands::test_geodist_invalid_units",
"tests/test_commands.py::TestRedisCommands::test_geohash",
"tests/test_commands.py::TestRedisCommands::test_geopos",
"tests/test_commands.py::TestRedisCommands::test_geopos_no_value",
"tests/test_commands.py::TestRedisCommands::test_geosearch",
"tests/test_commands.py::TestRedisCommands::test_geosearch_member",
"tests/test_commands.py::TestRedisCommands::test_geosearch_sort",
"tests/test_commands.py::TestRedisCommands::test_geosearch_with",
"tests/test_commands.py::TestRedisCommands::test_geosearch_negative",
"tests/test_commands.py::TestRedisCommands::test_geosearchstore",
"tests/test_commands.py::TestRedisCommands::test_geosearchstore_dist",
"tests/test_commands.py::TestRedisCommands::test_georadius_Issue2609",
"tests/test_commands.py::TestRedisCommands::test_georadius",
"tests/test_commands.py::TestRedisCommands::test_georadius_no_values",
"tests/test_commands.py::TestRedisCommands::test_georadius_units",
"tests/test_commands.py::TestRedisCommands::test_georadius_with",
"tests/test_commands.py::TestRedisCommands::test_georadius_count",
"tests/test_commands.py::TestRedisCommands::test_georadius_sort",
"tests/test_commands.py::TestRedisCommands::test_georadius_store",
"tests/test_commands.py::TestRedisCommands::test_georadius_store_dist",
"tests/test_commands.py::TestRedisCommands::test_georadiusmember",
"tests/test_commands.py::TestRedisCommands::test_georadiusmember_count",
"tests/test_commands.py::TestRedisCommands::test_xack",
"tests/test_commands.py::TestRedisCommands::test_xadd",
"tests/test_commands.py::TestRedisCommands::test_xadd_nomkstream",
"tests/test_commands.py::TestRedisCommands::test_xadd_minlen_and_limit",
"tests/test_commands.py::TestRedisCommands::test_xadd_explicit_ms",
"tests/test_commands.py::TestRedisCommands::test_xautoclaim_negative",
"tests/test_commands.py::TestRedisCommands::test_xclaim",
"tests/test_commands.py::TestRedisCommands::test_xclaim_trimmed",
"tests/test_commands.py::TestRedisCommands::test_xdel",
"tests/test_commands.py::TestRedisCommands::test_xgroup_create",
"tests/test_commands.py::TestRedisCommands::test_xgroup_create_mkstream",
"tests/test_commands.py::TestRedisCommands::test_xgroup_create_entriesread",
"tests/test_commands.py::TestRedisCommands::test_xgroup_delconsumer",
"tests/test_commands.py::TestRedisCommands::test_xgroup_createconsumer",
"tests/test_commands.py::TestRedisCommands::test_xgroup_destroy",
"tests/test_commands.py::TestRedisCommands::test_xgroup_setid",
"tests/test_commands.py::TestRedisCommands::test_xinfo_stream",
"tests/test_commands.py::TestRedisCommands::test_xinfo_stream_full",
"tests/test_commands.py::TestRedisCommands::test_xlen",
"tests/test_commands.py::TestRedisCommands::test_xpending",
"tests/test_commands.py::TestRedisCommands::test_xpending_range",
"tests/test_commands.py::TestRedisCommands::test_xpending_range_idle",
"tests/test_commands.py::TestRedisCommands::test_xpending_range_negative",
"tests/test_commands.py::TestRedisCommands::test_xrange",
"tests/test_commands.py::TestRedisCommands::test_xread",
"tests/test_commands.py::TestRedisCommands::test_xreadgroup",
"tests/test_commands.py::TestRedisCommands::test_xrevrange",
"tests/test_commands.py::TestRedisCommands::test_xtrim",
"tests/test_commands.py::TestRedisCommands::test_xtrim_minlen_and_length_args",
"tests/test_commands.py::TestRedisCommands::test_bitfield_operations",
"tests/test_commands.py::TestRedisCommands::test_bitfield_ro",
"tests/test_commands.py::TestRedisCommands::test_memory_help",
"tests/test_commands.py::TestRedisCommands::test_memory_doctor",
"tests/test_commands.py::TestRedisCommands::test_memory_malloc_stats",
"tests/test_commands.py::TestRedisCommands::test_memory_stats",
"tests/test_commands.py::TestRedisCommands::test_memory_usage",
"tests/test_commands.py::TestRedisCommands::test_latency_histogram_not_implemented",
"tests/test_commands.py::TestRedisCommands::test_latency_graph_not_implemented",
"tests/test_commands.py::TestRedisCommands::test_latency_doctor_not_implemented",
"tests/test_commands.py::TestRedisCommands::test_latency_history",
"tests/test_commands.py::TestRedisCommands::test_latency_latest",
"tests/test_commands.py::TestRedisCommands::test_latency_reset",
"tests/test_commands.py::TestRedisCommands::test_module_list",
"tests/test_commands.py::TestRedisCommands::test_command_count",
"tests/test_commands.py::TestRedisCommands::test_command_docs",
"tests/test_commands.py::TestRedisCommands::test_command_list",
"tests/test_commands.py::TestRedisCommands::test_command_getkeys",
"tests/test_commands.py::TestRedisCommands::test_command",
"tests/test_commands.py::TestRedisCommands::test_command_getkeysandflags",
"tests/test_commands.py::TestRedisCommands::test_module",
"tests/test_commands.py::TestRedisCommands::test_module_loadex",
"tests/test_commands.py::TestRedisCommands::test_restore",
"tests/test_commands.py::TestRedisCommands::test_restore_idletime",
"tests/test_commands.py::TestRedisCommands::test_restore_frequency",
"tests/test_commands.py::TestRedisCommands::test_replicaof",
"tests/test_commands.py::TestRedisCommands::test_shutdown",
"tests/test_commands.py::TestRedisCommands::test_shutdown_with_params",
"tests/test_commands.py::TestRedisCommands::test_sync",
"tests/test_commands.py::TestRedisCommands::test_psync",
"tests/test_commands.py::TestBinarySave::test_binary_get_set",
"tests/test_commands.py::TestBinarySave::test_binary_lists",
"tests/test_commands.py::TestBinarySave::test_22_info",
"tests/test_commands.py::TestBinarySave::test_large_responses",
"tests/test_commands.py::TestBinarySave::test_floating_point_encoding"
] | [
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_client_no_touch[single-python-parser]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_client_no_touch[pool-python-parser]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_client_no_touch[single-hiredis]",
"tests/test_asyncio/test_commands.py::TestRedisCommands::test_client_no_touch[pool-hiredis]",
"tests/test_commands.py::TestRedisCommands::test_client_no_touch"
] | Python | [] | [] |
|
redis/redis-py | 2,752 | redis__redis-py-2752 | [
"2638"
] | cfdcfd87acdc10bedba6230b0cbe7dcf44b4652a | diff --git a/redis/asyncio/client.py b/redis/asyncio/client.py
--- a/redis/asyncio/client.py
+++ b/redis/asyncio/client.py
@@ -139,8 +139,12 @@ class initializer. In the case of conflicting arguments, querystring
arguments always win.
"""
+ single_connection_client = kwargs.pop("single_connection_client", False)
connection_pool = ConnectionPool.from_url(url, **kwargs)
- return cls(connection_pool=connection_pool)
+ return cls(
+ connection_pool=connection_pool,
+ single_connection_client=single_connection_client,
+ )
def __init__(
self,
diff --git a/redis/client.py b/redis/client.py
--- a/redis/client.py
+++ b/redis/client.py
@@ -899,8 +899,12 @@ class initializer. In the case of conflicting arguments, querystring
arguments always win.
"""
+ single_connection_client = kwargs.pop("single_connection_client", False)
connection_pool = ConnectionPool.from_url(url, **kwargs)
- return cls(connection_pool=connection_pool)
+ return cls(
+ connection_pool=connection_pool,
+ single_connection_client=single_connection_client,
+ )
def __init__(
self,
| diff --git a/tests/test_asyncio/test_connection.py b/tests/test_asyncio/test_connection.py
--- a/tests/test_asyncio/test_connection.py
+++ b/tests/test_asyncio/test_connection.py
@@ -271,3 +271,9 @@ async def open_connection(*args, **kwargs):
vals = await asyncio.gather(do_read(), do_close())
assert vals == [b"Hello, World!", None]
+
+
[email protected]
+def test_create_single_connection_client_from_url():
+ client = Redis.from_url("redis://localhost:6379/0?", single_connection_client=True)
+ assert client.single_connection_client is True
diff --git a/tests/test_connection.py b/tests/test_connection.py
--- a/tests/test_connection.py
+++ b/tests/test_connection.py
@@ -205,3 +205,11 @@ def test_pack_command(Class):
actual = Class().pack_command(*cmd)[0]
assert actual == expected, f"actual = {actual}, expected = {expected}"
+
+
[email protected]
+def test_create_single_connection_client_from_url():
+ client = redis.Redis.from_url(
+ "redis://localhost:6379/0?", single_connection_client=True
+ )
+ assert client.connection is not None
| Redis.from_url() is a misnomer
**Version**: 4.5.2
**Platform**: Python 3.11.2
**Description**: Redis.from_url is a convenience function, but the kwargs are passed into the ConnectionPool object and not the Redis instance. This formerly wasn't a problem, but it appears as though auto_close_connection_pool now defaults to true, so you can't reliably use Redis.from_url() in a singleton.
| "2023-05-08T08:29:13Z" | 4.5 | [
"tests/test_asyncio/test_connection.py::test_invalid_response[single-python-parser]",
"tests/test_asyncio/test_connection.py::test_invalid_response[pool-python-parser]",
"tests/test_asyncio/test_connection.py::test_invalid_response[single-hiredis]",
"tests/test_asyncio/test_connection.py::test_invalid_response[pool-hiredis]",
"tests/test_asyncio/test_connection.py::test_single_connection",
"tests/test_asyncio/test_connection.py::test_loading_external_modules[single-python-parser]",
"tests/test_asyncio/test_connection.py::test_loading_external_modules[pool-python-parser]",
"tests/test_asyncio/test_connection.py::test_loading_external_modules[single-hiredis]",
"tests/test_asyncio/test_connection.py::test_loading_external_modules[pool-hiredis]",
"tests/test_asyncio/test_connection.py::test_socket_param_regression[single-python-parser]",
"tests/test_asyncio/test_connection.py::test_socket_param_regression[pool-python-parser]",
"tests/test_asyncio/test_connection.py::test_socket_param_regression[single-hiredis]",
"tests/test_asyncio/test_connection.py::test_socket_param_regression[pool-hiredis]",
"tests/test_asyncio/test_connection.py::test_can_run_concurrent_commands[pool-python-parser]",
"tests/test_asyncio/test_connection.py::test_can_run_concurrent_commands[pool-hiredis]",
"tests/test_asyncio/test_connection.py::test_connect_retry_on_timeout_error",
"tests/test_asyncio/test_connection.py::test_connect_without_retry_on_os_error",
"tests/test_asyncio/test_connection.py::test_connect_timeout_error_without_retry",
"tests/test_asyncio/test_connection.py::test_connection_parse_response_resume[single-python-parser]",
"tests/test_asyncio/test_connection.py::test_connection_parse_response_resume[pool-python-parser]",
"tests/test_asyncio/test_connection.py::test_connection_parse_response_resume[single-hiredis]",
"tests/test_asyncio/test_connection.py::test_connection_parse_response_resume[pool-hiredis]",
"tests/test_asyncio/test_connection.py::test_connection_disconect_race[PythonParser]",
"tests/test_asyncio/test_connection.py::test_connection_disconect_race[HiredisParser]",
"tests/test_connection.py::test_loading_external_modules",
"tests/test_connection.py::TestConnection::test_disconnect",
"tests/test_connection.py::TestConnection::test_disconnect__shutdown_OSError",
"tests/test_connection.py::TestConnection::test_disconnect__close_OSError",
"tests/test_connection.py::TestConnection::test_retry_connect_on_timeout_error",
"tests/test_connection.py::TestConnection::test_connect_without_retry_on_os_error",
"tests/test_connection.py::TestConnection::test_connect_timeout_error_without_retry",
"tests/test_connection.py::test_connection_parse_response_resume[PythonParser]",
"tests/test_connection.py::test_connection_parse_response_resume[HiredisParser]",
"tests/test_connection.py::test_pack_command[Connection]",
"tests/test_connection.py::test_pack_command[SSLConnection]",
"tests/test_connection.py::test_pack_command[UnixDomainSocketConnection]"
] | [
"tests/test_asyncio/test_connection.py::test_create_single_connection_client_from_url",
"tests/test_connection.py::test_create_single_connection_client_from_url"
] | Python | [] | [] |
|
redis/redis-py | 2,913 | redis__redis-py-2913 | [
"2901"
] | 509c77c5263188fad239b07c80ecc5b0e8372647 | diff --git a/redis/asyncio/client.py b/redis/asyncio/client.py
--- a/redis/asyncio/client.py
+++ b/redis/asyncio/client.py
@@ -114,7 +114,7 @@ def from_url(
cls,
url: str,
single_connection_client: bool = False,
- auto_close_connection_pool: bool = True,
+ auto_close_connection_pool: Optional[bool] = None,
**kwargs,
):
"""
@@ -160,12 +160,39 @@ class initializer. In the case of conflicting arguments, querystring
"""
connection_pool = ConnectionPool.from_url(url, **kwargs)
- redis = cls(
+ client = cls(
connection_pool=connection_pool,
single_connection_client=single_connection_client,
)
- redis.auto_close_connection_pool = auto_close_connection_pool
- return redis
+ if auto_close_connection_pool is not None:
+ warnings.warn(
+ DeprecationWarning(
+ '"auto_close_connection_pool" is deprecated '
+ "since version 5.0.0. "
+ "Please create a ConnectionPool explicitly and "
+ "provide to the Redis() constructor instead."
+ )
+ )
+ else:
+ auto_close_connection_pool = True
+ client.auto_close_connection_pool = auto_close_connection_pool
+ return client
+
+ @classmethod
+ def from_pool(
+ cls: Type["Redis"],
+ connection_pool: ConnectionPool,
+ ) -> "Redis":
+ """
+ Return a Redis client from the given connection pool.
+ The Redis client will take ownership of the connection pool and
+ close it when the Redis client is closed.
+ """
+ client = cls(
+ connection_pool=connection_pool,
+ )
+ client.auto_close_connection_pool = True
+ return client
def __init__(
self,
@@ -200,7 +227,8 @@ def __init__(
lib_version: Optional[str] = get_lib_version(),
username: Optional[str] = None,
retry: Optional[Retry] = None,
- auto_close_connection_pool: bool = True,
+ # deprecated. create a pool and use connection_pool instead
+ auto_close_connection_pool: Optional[bool] = None,
redis_connect_func=None,
credential_provider: Optional[CredentialProvider] = None,
protocol: Optional[int] = 2,
@@ -213,14 +241,21 @@ def __init__(
To retry on TimeoutError, `retry_on_timeout` can also be set to `True`.
"""
kwargs: Dict[str, Any]
- # auto_close_connection_pool only has an effect if connection_pool is
- # None. This is a similar feature to the missing __del__ to resolve #1103,
- # but it accounts for whether a user wants to manually close the connection
- # pool, as a similar feature to ConnectionPool's __del__.
- self.auto_close_connection_pool = (
- auto_close_connection_pool if connection_pool is None else False
- )
+
+ if auto_close_connection_pool is not None:
+ warnings.warn(
+ DeprecationWarning(
+ '"auto_close_connection_pool" is deprecated '
+ "since version 5.0.0. "
+ "Please create a ConnectionPool explicitly and "
+ "provide to the Redis() constructor instead."
+ )
+ )
+ else:
+ auto_close_connection_pool = True
+
if not connection_pool:
+ # Create internal connection pool, expected to be closed by Redis instance
if not retry_on_error:
retry_on_error = []
if retry_on_timeout is True:
@@ -277,7 +312,13 @@ def __init__(
"ssl_check_hostname": ssl_check_hostname,
}
)
+ # This arg only used if no pool is passed in
+ self.auto_close_connection_pool = auto_close_connection_pool
connection_pool = ConnectionPool(**kwargs)
+ else:
+ # If a pool is passed in, do not close it
+ self.auto_close_connection_pool = False
+
self.connection_pool = connection_pool
self.single_connection_client = single_connection_client
self.connection: Optional[Connection] = None
diff --git a/redis/asyncio/connection.py b/redis/asyncio/connection.py
--- a/redis/asyncio/connection.py
+++ b/redis/asyncio/connection.py
@@ -1106,8 +1106,8 @@ class BlockingConnectionPool(ConnectionPool):
"""
A blocking connection pool::
- >>> from redis.asyncio.client import Redis
- >>> client = Redis(connection_pool=BlockingConnectionPool())
+ >>> from redis.asyncio import Redis, BlockingConnectionPool
+ >>> client = Redis.from_pool(BlockingConnectionPool())
It performs the same function as the default
:py:class:`~redis.asyncio.ConnectionPool` implementation, in that,
diff --git a/redis/asyncio/sentinel.py b/redis/asyncio/sentinel.py
--- a/redis/asyncio/sentinel.py
+++ b/redis/asyncio/sentinel.py
@@ -340,12 +340,7 @@ def master_for(
connection_pool = connection_pool_class(service_name, self, **connection_kwargs)
# The Redis object "owns" the pool
- auto_close_connection_pool = True
- client = redis_class(
- connection_pool=connection_pool,
- )
- client.auto_close_connection_pool = auto_close_connection_pool
- return client
+ return redis_class.from_pool(connection_pool)
def slave_for(
self,
@@ -377,9 +372,4 @@ def slave_for(
connection_pool = connection_pool_class(service_name, self, **connection_kwargs)
# The Redis object "owns" the pool
- auto_close_connection_pool = True
- client = redis_class(
- connection_pool=connection_pool,
- )
- client.auto_close_connection_pool = auto_close_connection_pool
- return client
+ return redis_class.from_pool(connection_pool)
diff --git a/redis/client.py b/redis/client.py
--- a/redis/client.py
+++ b/redis/client.py
@@ -4,7 +4,7 @@
import time
import warnings
from itertools import chain
-from typing import Optional
+from typing import Optional, Type
from redis._parsers.helpers import (
_RedisCallbacks,
@@ -136,10 +136,28 @@ class initializer. In the case of conflicting arguments, querystring
"""
single_connection_client = kwargs.pop("single_connection_client", False)
connection_pool = ConnectionPool.from_url(url, **kwargs)
- return cls(
+ client = cls(
connection_pool=connection_pool,
single_connection_client=single_connection_client,
)
+ client.auto_close_connection_pool = True
+ return client
+
+ @classmethod
+ def from_pool(
+ cls: Type["Redis"],
+ connection_pool: ConnectionPool,
+ ) -> "Redis":
+ """
+ Return a Redis client from the given connection pool.
+ The Redis client will take ownership of the connection pool and
+ close it when the Redis client is closed.
+ """
+ client = cls(
+ connection_pool=connection_pool,
+ )
+ client.auto_close_connection_pool = True
+ return client
def __init__(
self,
@@ -275,6 +293,10 @@ def __init__(
}
)
connection_pool = ConnectionPool(**kwargs)
+ self.auto_close_connection_pool = True
+ else:
+ self.auto_close_connection_pool = False
+
self.connection_pool = connection_pool
self.connection = None
if single_connection_client:
@@ -477,6 +499,9 @@ def close(self):
self.connection = None
self.connection_pool.release(conn)
+ if self.auto_close_connection_pool:
+ self.connection_pool.disconnect()
+
def _send_command_parse_response(self, conn, command_name, *args, **options):
"""
Send a command and parse the response
diff --git a/redis/sentinel.py b/redis/sentinel.py
--- a/redis/sentinel.py
+++ b/redis/sentinel.py
@@ -353,10 +353,8 @@ def master_for(
kwargs["is_master"] = True
connection_kwargs = dict(self.connection_kwargs)
connection_kwargs.update(kwargs)
- return redis_class(
- connection_pool=connection_pool_class(
- service_name, self, **connection_kwargs
- )
+ return redis_class.from_pool(
+ connection_pool_class(service_name, self, **connection_kwargs)
)
def slave_for(
@@ -386,8 +384,6 @@ def slave_for(
kwargs["is_master"] = False
connection_kwargs = dict(self.connection_kwargs)
connection_kwargs.update(kwargs)
- return redis_class(
- connection_pool=connection_pool_class(
- service_name, self, **connection_kwargs
- )
+ return redis_class.from_pool(
+ connection_pool_class(service_name, self, **connection_kwargs)
)
| diff --git a/tests/test_asyncio/test_connection.py b/tests/test_asyncio/test_connection.py
--- a/tests/test_asyncio/test_connection.py
+++ b/tests/test_asyncio/test_connection.py
@@ -11,7 +11,7 @@
_AsyncRESP3Parser,
_AsyncRESPBase,
)
-from redis.asyncio import Redis
+from redis.asyncio import ConnectionPool, Redis
from redis.asyncio.connection import Connection, UnixDomainSocketConnection, parse_url
from redis.asyncio.retry import Retry
from redis.backoff import NoBackoff
@@ -288,7 +288,7 @@ def test_create_single_connection_client_from_url():
assert client.single_connection_client is True
[email protected]("from_url", (True, False))
[email protected]("from_url", (True, False), ids=("from_url", "from_args"))
async def test_pool_auto_close(request, from_url):
"""Verify that basic Redis instances have auto_close_connection_pool set to True"""
@@ -305,20 +305,116 @@ async def get_redis_connection():
await r1.close()
[email protected]("from_url", (True, False))
-async def test_pool_auto_close_disable(request, from_url):
- """Verify that auto_close_connection_pool can be disabled"""
+async def test_pool_from_url_deprecation(request):
+ url: str = request.config.getoption("--redis-url")
+
+ with pytest.deprecated_call():
+ return Redis.from_url(url, auto_close_connection_pool=False)
+
+
+async def test_pool_auto_close_disable(request):
+ """Verify that auto_close_connection_pool can be disabled (deprecated)"""
url: str = request.config.getoption("--redis-url")
url_args = parse_url(url)
async def get_redis_connection():
- if from_url:
- return Redis.from_url(url, auto_close_connection_pool=False)
url_args["auto_close_connection_pool"] = False
- return Redis(**url_args)
+ with pytest.deprecated_call():
+ return Redis(**url_args)
r1 = await get_redis_connection()
assert r1.auto_close_connection_pool is False
await r1.connection_pool.disconnect()
await r1.close()
+
+
[email protected]("from_url", (True, False), ids=("from_url", "from_args"))
+async def test_redis_connection_pool(request, from_url):
+ """Verify that basic Redis instances using `connection_pool`
+ have auto_close_connection_pool set to False"""
+
+ url: str = request.config.getoption("--redis-url")
+ url_args = parse_url(url)
+
+ pool = None
+
+ async def get_redis_connection():
+ nonlocal pool
+ if from_url:
+ pool = ConnectionPool.from_url(url)
+ else:
+ pool = ConnectionPool(**url_args)
+ return Redis(connection_pool=pool)
+
+ called = 0
+
+ async def mock_disconnect(_):
+ nonlocal called
+ called += 1
+
+ with patch.object(ConnectionPool, "disconnect", mock_disconnect):
+ async with await get_redis_connection() as r1:
+ assert r1.auto_close_connection_pool is False
+
+ assert called == 0
+ await pool.disconnect()
+
+
[email protected]("from_url", (True, False), ids=("from_url", "from_args"))
+async def test_redis_from_pool(request, from_url):
+ """Verify that basic Redis instances created using `from_pool()`
+ have auto_close_connection_pool set to True"""
+
+ url: str = request.config.getoption("--redis-url")
+ url_args = parse_url(url)
+
+ pool = None
+
+ async def get_redis_connection():
+ nonlocal pool
+ if from_url:
+ pool = ConnectionPool.from_url(url)
+ else:
+ pool = ConnectionPool(**url_args)
+ return Redis.from_pool(pool)
+
+ called = 0
+
+ async def mock_disconnect(_):
+ nonlocal called
+ called += 1
+
+ with patch.object(ConnectionPool, "disconnect", mock_disconnect):
+ async with await get_redis_connection() as r1:
+ assert r1.auto_close_connection_pool is True
+
+ assert called == 1
+ await pool.disconnect()
+
+
[email protected]("auto_close", (True, False))
+async def test_redis_pool_auto_close_arg(request, auto_close):
+ """test that redis instance where pool is provided have
+ auto_close_connection_pool set to False, regardless of arg"""
+
+ url: str = request.config.getoption("--redis-url")
+ pool = ConnectionPool.from_url(url)
+
+ async def get_redis_connection():
+ with pytest.deprecated_call():
+ client = Redis(connection_pool=pool, auto_close_connection_pool=auto_close)
+ return client
+
+ called = 0
+
+ async def mock_disconnect(_):
+ nonlocal called
+ called += 1
+
+ with patch.object(ConnectionPool, "disconnect", mock_disconnect):
+ async with await get_redis_connection() as r1:
+ assert r1.auto_close_connection_pool is False
+
+ assert called == 0
+ await pool.disconnect()
diff --git a/tests/test_connection.py b/tests/test_connection.py
--- a/tests/test_connection.py
+++ b/tests/test_connection.py
@@ -5,9 +5,15 @@
import pytest
import redis
+from redis import ConnectionPool, Redis
from redis._parsers import _HiredisParser, _RESP2Parser, _RESP3Parser
from redis.backoff import NoBackoff
-from redis.connection import Connection, SSLConnection, UnixDomainSocketConnection
+from redis.connection import (
+ Connection,
+ SSLConnection,
+ UnixDomainSocketConnection,
+ parse_url,
+)
from redis.exceptions import ConnectionError, InvalidResponse, TimeoutError
from redis.retry import Retry
from redis.utils import HIREDIS_AVAILABLE
@@ -209,3 +215,84 @@ def test_create_single_connection_client_from_url():
"redis://localhost:6379/0?", single_connection_client=True
)
assert client.connection is not None
+
+
[email protected]("from_url", (True, False), ids=("from_url", "from_args"))
+def test_pool_auto_close(request, from_url):
+ """Verify that basic Redis instances have auto_close_connection_pool set to True"""
+
+ url: str = request.config.getoption("--redis-url")
+ url_args = parse_url(url)
+
+ def get_redis_connection():
+ if from_url:
+ return Redis.from_url(url)
+ return Redis(**url_args)
+
+ r1 = get_redis_connection()
+ assert r1.auto_close_connection_pool is True
+ r1.close()
+
+
[email protected]("from_url", (True, False), ids=("from_url", "from_args"))
+def test_redis_connection_pool(request, from_url):
+ """Verify that basic Redis instances using `connection_pool`
+ have auto_close_connection_pool set to False"""
+
+ url: str = request.config.getoption("--redis-url")
+ url_args = parse_url(url)
+
+ pool = None
+
+ def get_redis_connection():
+ nonlocal pool
+ if from_url:
+ pool = ConnectionPool.from_url(url)
+ else:
+ pool = ConnectionPool(**url_args)
+ return Redis(connection_pool=pool)
+
+ called = 0
+
+ def mock_disconnect(_):
+ nonlocal called
+ called += 1
+
+ with patch.object(ConnectionPool, "disconnect", mock_disconnect):
+ with get_redis_connection() as r1:
+ assert r1.auto_close_connection_pool is False
+
+ assert called == 0
+ pool.disconnect()
+
+
[email protected]("from_url", (True, False), ids=("from_url", "from_args"))
+def test_redis_from_pool(request, from_url):
+ """Verify that basic Redis instances created using `from_pool()`
+ have auto_close_connection_pool set to True"""
+
+ url: str = request.config.getoption("--redis-url")
+ url_args = parse_url(url)
+
+ pool = None
+
+ def get_redis_connection():
+ nonlocal pool
+ if from_url:
+ pool = ConnectionPool.from_url(url)
+ else:
+ pool = ConnectionPool(**url_args)
+ return Redis.from_pool(pool)
+
+ called = 0
+
+ def mock_disconnect(_):
+ nonlocal called
+ called += 1
+
+ with patch.object(ConnectionPool, "disconnect", mock_disconnect):
+ with get_redis_connection() as r1:
+ assert r1.auto_close_connection_pool is True
+
+ assert called == 1
+ pool.disconnect()
diff --git a/tests/test_sentinel.py b/tests/test_sentinel.py
--- a/tests/test_sentinel.py
+++ b/tests/test_sentinel.py
@@ -1,4 +1,5 @@
import socket
+from unittest import mock
import pytest
import redis.sentinel
@@ -240,3 +241,28 @@ def test_flushconfig(cluster, sentinel):
def test_reset(cluster, sentinel):
cluster.master["is_odown"] = True
assert sentinel.sentinel_reset("mymaster")
+
+
[email protected]
[email protected]("method_name", ["master_for", "slave_for"])
+def test_auto_close_pool(cluster, sentinel, method_name):
+ """
+ Check that the connection pool created by the sentinel client is
+ automatically closed
+ """
+
+ method = getattr(sentinel, method_name)
+ client = method("mymaster", db=9)
+ pool = client.connection_pool
+ assert client.auto_close_connection_pool is True
+ calls = 0
+
+ def mock_disconnect():
+ nonlocal calls
+ calls += 1
+
+ with mock.patch.object(pool, "disconnect", mock_disconnect):
+ client.close()
+
+ assert calls == 1
+ pool.disconnect()
| `auto_close_connection_pool` ignored
This defect pertains to issues discovered with #2831
In async mode, it is important that a `Redis` object which holds its own pool, can be told to close it when the `Redis` object
is closed, to free the user from such hassles. Examples in the code include
``` python
from redis.asyncio.client import Redis
client = Redis(connection_pool=BlockingConnectionPool())
```
when this client is closed, the internal connection pool remains open, i.e. its connections are not shut down.
The `Redis` class includes a `auto_close_connection_pool` argument. However, this is _ignored_ when a connection pool
is provided to the constructor. Presumably the thinking is that the caller will manage that pool himself.
But that makes the above kind of pattern, also used within the code, unusable.
```python
client = Redis(connection_pool=BlockingConnectionPool(), auto_close_connection_pool=True)
assert client.auto_close_connection_pool=False
```
Instead, it is necessary to have code like this, which is very poor practice:
```python
client = Redis(connection_pool=BlockingConnectionPool(), auto_close_connection_pool=True)
client.auto_close_connection_pool=True
```
I propose, that we either:
- Always honour the `auto_close_connection_pool` argument.
- add a `own_connection_pool:ConnectionPool` argument, to be used instead of `connection_pool`.
- rename `auto_close_connection_pool` to `own_pool`, or something less cumbersome.
- add a `construct_connection_pool` argument which takes a callable which returns a connection pool, one which Redis considers its own.
At any rate, it should be easy to create a connection pool, and hand it to the `Redis` constructor for it to "own" without
having to fudge an internal property afterwards.
In addition, I propose that the same mechanics be added to the non-`async` parts of the library. Currently the `redis.Redis` objects do not close connection pools, instead the library relies on the prompt execution of `__del__()` methods, or explicit `ConnectionPool.disconnect()` calls.
Relying on garbage collection to free resources is considered bad programming practice in Python, where resource management should in general be more explicit. It is particularly frowned upon for async code, since executing asynchronous code there is not a good idea(*). the synchronous code should receive whatever solution we choose for the above as well, so that pools owned by a `Redis` client object are also disconnected when those Redis objects are closed.
> (*) `__del__()` methods are not async. While it is possible to use something like `asyncio.run` within such a function, it will __block__ the rest of the program. Also, `__del__()` methods are often invoked during program cleanup when it is simply not possible to do anything and trying to do so will produce errors and confusion.
### Update
In pr #2913 I have updated a `Redis.from_pool()` method to use when handing over a `ConnectionPool` to be _owned_ by the `Redis` instance. I think this is a much nicer way than to heap on more constructor arguments to `Redis`
| "2023-08-24T16:02:09Z" | 5.0 | [
"tests/test_asyncio/test_connection.py::test_invalid_response[single-python-parser]",
"tests/test_asyncio/test_connection.py::test_invalid_response[pool-python-parser]",
"tests/test_asyncio/test_connection.py::test_invalid_response[single-hiredis]",
"tests/test_asyncio/test_connection.py::test_invalid_response[pool-hiredis]",
"tests/test_asyncio/test_connection.py::test_single_connection",
"tests/test_asyncio/test_connection.py::test_loading_external_modules[single-python-parser]",
"tests/test_asyncio/test_connection.py::test_loading_external_modules[pool-python-parser]",
"tests/test_asyncio/test_connection.py::test_loading_external_modules[single-hiredis]",
"tests/test_asyncio/test_connection.py::test_loading_external_modules[pool-hiredis]",
"tests/test_asyncio/test_connection.py::test_socket_param_regression[single-python-parser]",
"tests/test_asyncio/test_connection.py::test_socket_param_regression[pool-python-parser]",
"tests/test_asyncio/test_connection.py::test_socket_param_regression[single-hiredis]",
"tests/test_asyncio/test_connection.py::test_socket_param_regression[pool-hiredis]",
"tests/test_asyncio/test_connection.py::test_can_run_concurrent_commands[pool-python-parser]",
"tests/test_asyncio/test_connection.py::test_can_run_concurrent_commands[pool-hiredis]",
"tests/test_asyncio/test_connection.py::test_connect_retry_on_timeout_error",
"tests/test_asyncio/test_connection.py::test_connect_without_retry_on_os_error",
"tests/test_asyncio/test_connection.py::test_connect_timeout_error_without_retry",
"tests/test_asyncio/test_connection.py::test_connection_parse_response_resume[single-python-parser]",
"tests/test_asyncio/test_connection.py::test_connection_parse_response_resume[pool-python-parser]",
"tests/test_asyncio/test_connection.py::test_connection_parse_response_resume[single-hiredis]",
"tests/test_asyncio/test_connection.py::test_connection_parse_response_resume[pool-hiredis]",
"tests/test_asyncio/test_connection.py::test_connection_disconect_race[AsyncRESP2Parser]",
"tests/test_asyncio/test_connection.py::test_connection_disconect_race[AsyncRESP3Parser]",
"tests/test_asyncio/test_connection.py::test_connection_disconect_race[AsyncHiredisParser]",
"tests/test_asyncio/test_connection.py::test_create_single_connection_client_from_url",
"tests/test_asyncio/test_connection.py::test_pool_auto_close[from_url]",
"tests/test_asyncio/test_connection.py::test_pool_auto_close[from_args]",
"tests/test_asyncio/test_connection.py::test_redis_connection_pool[from_url]",
"tests/test_asyncio/test_connection.py::test_redis_connection_pool[from_args]",
"tests/test_connection.py::test_loading_external_modules",
"tests/test_connection.py::TestConnection::test_disconnect",
"tests/test_connection.py::TestConnection::test_disconnect__shutdown_OSError",
"tests/test_connection.py::TestConnection::test_disconnect__close_OSError",
"tests/test_connection.py::TestConnection::test_retry_connect_on_timeout_error",
"tests/test_connection.py::TestConnection::test_connect_without_retry_on_os_error",
"tests/test_connection.py::TestConnection::test_connect_timeout_error_without_retry",
"tests/test_connection.py::test_connection_parse_response_resume[RESP2Parser]",
"tests/test_connection.py::test_connection_parse_response_resume[RESP3Parser]",
"tests/test_connection.py::test_connection_parse_response_resume[HiredisParser]",
"tests/test_connection.py::test_pack_command[Connection]",
"tests/test_connection.py::test_pack_command[SSLConnection]",
"tests/test_connection.py::test_pack_command[UnixDomainSocketConnection]",
"tests/test_connection.py::test_create_single_connection_client_from_url",
"tests/test_sentinel.py::test_discover_master",
"tests/test_sentinel.py::test_discover_master_error",
"tests/test_sentinel.py::test_dead_pool",
"tests/test_sentinel.py::test_discover_master_sentinel_down",
"tests/test_sentinel.py::test_discover_master_sentinel_timeout",
"tests/test_sentinel.py::test_master_min_other_sentinels",
"tests/test_sentinel.py::test_master_odown",
"tests/test_sentinel.py::test_master_sdown",
"tests/test_sentinel.py::test_discover_slaves",
"tests/test_sentinel.py::test_master_for",
"tests/test_sentinel.py::test_slave_for",
"tests/test_sentinel.py::test_slave_for_slave_not_found_error",
"tests/test_sentinel.py::test_slave_round_robin",
"tests/test_sentinel.py::test_ckquorum",
"tests/test_sentinel.py::test_flushconfig",
"tests/test_sentinel.py::test_reset"
] | [
"tests/test_asyncio/test_connection.py::test_pool_from_url_deprecation",
"tests/test_asyncio/test_connection.py::test_pool_auto_close_disable",
"tests/test_asyncio/test_connection.py::test_redis_from_pool[from_url]",
"tests/test_asyncio/test_connection.py::test_redis_from_pool[from_args]",
"tests/test_asyncio/test_connection.py::test_redis_pool_auto_close_arg[True]",
"tests/test_asyncio/test_connection.py::test_redis_pool_auto_close_arg[False]",
"tests/test_connection.py::test_pool_auto_close[from_url]",
"tests/test_connection.py::test_pool_auto_close[from_args]",
"tests/test_connection.py::test_redis_connection_pool[from_url]",
"tests/test_connection.py::test_redis_connection_pool[from_args]",
"tests/test_connection.py::test_redis_from_pool[from_url]",
"tests/test_connection.py::test_redis_from_pool[from_args]",
"tests/test_sentinel.py::test_auto_close_pool[master_for]",
"tests/test_sentinel.py::test_auto_close_pool[slave_for]"
] | Python | [] | [] |
|
redis/redis-py | 2,984 | redis__redis-py-2984 | [
"2983"
] | 5391c5fc39a2c9aaa1b2e5acc799b93444270b88 | diff --git a/redis/asyncio/connection.py b/redis/asyncio/connection.py
--- a/redis/asyncio/connection.py
+++ b/redis/asyncio/connection.py
@@ -861,6 +861,7 @@ def to_bool(value) -> Optional[bool]:
"max_connections": int,
"health_check_interval": int,
"ssl_check_hostname": to_bool,
+ "timeout": float,
}
)
diff --git a/redis/connection.py b/redis/connection.py
--- a/redis/connection.py
+++ b/redis/connection.py
@@ -853,6 +853,7 @@ def to_bool(value):
"max_connections": int,
"health_check_interval": int,
"ssl_check_hostname": to_bool,
+ "timeout": float,
}
| diff --git a/tests/test_asyncio/test_connection_pool.py b/tests/test_asyncio/test_connection_pool.py
--- a/tests/test_asyncio/test_connection_pool.py
+++ b/tests/test_asyncio/test_connection_pool.py
@@ -454,6 +454,31 @@ def test_invalid_scheme_raises_error(self):
)
+class TestBlockingConnectionPoolURLParsing:
+ def test_extra_typed_querystring_options(self):
+ pool = redis.BlockingConnectionPool.from_url(
+ "redis://localhost/2?socket_timeout=20&socket_connect_timeout=10"
+ "&socket_keepalive=&retry_on_timeout=Yes&max_connections=10&timeout=13.37"
+ )
+
+ assert pool.connection_class == redis.Connection
+ assert pool.connection_kwargs == {
+ "host": "localhost",
+ "db": 2,
+ "socket_timeout": 20.0,
+ "socket_connect_timeout": 10.0,
+ "retry_on_timeout": True,
+ }
+ assert pool.max_connections == 10
+ assert pool.timeout == 13.37
+
+ def test_invalid_extra_typed_querystring_options(self):
+ with pytest.raises(ValueError):
+ redis.BlockingConnectionPool.from_url(
+ "redis://localhost/2?timeout=_not_a_float_"
+ )
+
+
class TestConnectionPoolUnixSocketURLParsing:
def test_defaults(self):
pool = redis.ConnectionPool.from_url("unix:///socket")
diff --git a/tests/test_connection_pool.py b/tests/test_connection_pool.py
--- a/tests/test_connection_pool.py
+++ b/tests/test_connection_pool.py
@@ -359,6 +359,31 @@ def test_invalid_scheme_raises_error_when_double_slash_missing(self):
)
+class TestBlockingConnectionPoolURLParsing:
+ def test_extra_typed_querystring_options(self):
+ pool = redis.BlockingConnectionPool.from_url(
+ "redis://localhost/2?socket_timeout=20&socket_connect_timeout=10"
+ "&socket_keepalive=&retry_on_timeout=Yes&max_connections=10&timeout=42"
+ )
+
+ assert pool.connection_class == redis.Connection
+ assert pool.connection_kwargs == {
+ "host": "localhost",
+ "db": 2,
+ "socket_timeout": 20.0,
+ "socket_connect_timeout": 10.0,
+ "retry_on_timeout": True,
+ }
+ assert pool.max_connections == 10
+ assert pool.timeout == 42.0
+
+ def test_invalid_extra_typed_querystring_options(self):
+ with pytest.raises(ValueError):
+ redis.BlockingConnectionPool.from_url(
+ "redis://localhost/2?timeout=_not_a_float_"
+ )
+
+
class TestConnectionPoolUnixSocketURLParsing:
def test_defaults(self):
pool = redis.ConnectionPool.from_url("unix:///socket")
| Instantiating BlockingConnectionPool.from_url with timeout in query args fails
**Version**: 5.0.1
**Platform**: All
**Description**: Missing cast in URL_QUERY_ARGUMENT_PARSERS for "timeout" parameter causes a runtime TypeError
When instantiating a BlockingConnectionPool from url with timeout query parameter, timeout is not cast to float, resulting in timeout to be set to string in BlockingConnectionPool.__init__.
Later on when attempting to use the BlockingConnectionPool, comparison of timeout (as a string) to an int causes a `TypeError '<' not supported between instances of 'str' and 'int'`
TestCase:
```python
>>> from redis.connection import BlockingConnectionPool
>>> pool = BlockingConnectionPool.from_url("redis://127.0.0.1:6379/1?timeout=42")
>>> pool.timeout
'42'
>>> pool.get_connection("PING")
Traceback (most recent call last):
File "<stdin>", line 1, in <module>
File "/home/r0ro/.virtualenvs/backoffice/lib/python3.10/site-packages/redis/connection.py", line 1262, in get_connection
connection = self.pool.get(block=True, timeout=self.timeout)
File "/usr/lib/python3.10/queue.py", line 172, in get
elif timeout < 0:
TypeError: '<' not supported between instances of 'str' and 'int'
```
| "2023-10-05T23:07:18Z" | 5.0 | [
"tests/test_asyncio/test_connection_pool.py::TestRedisAutoReleaseConnectionPool::test_auto_disconnect_redis_created_pool[single-python-parser]",
"tests/test_asyncio/test_connection_pool.py::TestRedisAutoReleaseConnectionPool::test_auto_disconnect_redis_created_pool[pool-python-parser]",
"tests/test_asyncio/test_connection_pool.py::TestRedisAutoReleaseConnectionPool::test_auto_disconnect_redis_created_pool[single-hiredis]",
"tests/test_asyncio/test_connection_pool.py::TestRedisAutoReleaseConnectionPool::test_auto_disconnect_redis_created_pool[pool-hiredis]",
"tests/test_asyncio/test_connection_pool.py::TestRedisAutoReleaseConnectionPool::test_do_not_auto_disconnect_redis_created_pool[single-python-parser]",
"tests/test_asyncio/test_connection_pool.py::TestRedisAutoReleaseConnectionPool::test_do_not_auto_disconnect_redis_created_pool[pool-python-parser]",
"tests/test_asyncio/test_connection_pool.py::TestRedisAutoReleaseConnectionPool::test_do_not_auto_disconnect_redis_created_pool[single-hiredis]",
"tests/test_asyncio/test_connection_pool.py::TestRedisAutoReleaseConnectionPool::test_do_not_auto_disconnect_redis_created_pool[pool-hiredis]",
"tests/test_asyncio/test_connection_pool.py::TestRedisAutoReleaseConnectionPool::test_auto_release_override_true_manual_created_pool[single-python-parser]",
"tests/test_asyncio/test_connection_pool.py::TestRedisAutoReleaseConnectionPool::test_auto_release_override_true_manual_created_pool[pool-python-parser]",
"tests/test_asyncio/test_connection_pool.py::TestRedisAutoReleaseConnectionPool::test_auto_release_override_true_manual_created_pool[single-hiredis]",
"tests/test_asyncio/test_connection_pool.py::TestRedisAutoReleaseConnectionPool::test_auto_release_override_true_manual_created_pool[pool-hiredis]",
"tests/test_asyncio/test_connection_pool.py::TestRedisAutoReleaseConnectionPool::test_close_override[single-python-parser-True]",
"tests/test_asyncio/test_connection_pool.py::TestRedisAutoReleaseConnectionPool::test_close_override[single-python-parser-False]",
"tests/test_asyncio/test_connection_pool.py::TestRedisAutoReleaseConnectionPool::test_close_override[pool-python-parser-True]",
"tests/test_asyncio/test_connection_pool.py::TestRedisAutoReleaseConnectionPool::test_close_override[pool-python-parser-False]",
"tests/test_asyncio/test_connection_pool.py::TestRedisAutoReleaseConnectionPool::test_close_override[single-hiredis-True]",
"tests/test_asyncio/test_connection_pool.py::TestRedisAutoReleaseConnectionPool::test_close_override[single-hiredis-False]",
"tests/test_asyncio/test_connection_pool.py::TestRedisAutoReleaseConnectionPool::test_close_override[pool-hiredis-True]",
"tests/test_asyncio/test_connection_pool.py::TestRedisAutoReleaseConnectionPool::test_close_override[pool-hiredis-False]",
"tests/test_asyncio/test_connection_pool.py::TestRedisAutoReleaseConnectionPool::test_negate_auto_close_client_pool[single-python-parser-True]",
"tests/test_asyncio/test_connection_pool.py::TestRedisAutoReleaseConnectionPool::test_negate_auto_close_client_pool[single-python-parser-False]",
"tests/test_asyncio/test_connection_pool.py::TestRedisAutoReleaseConnectionPool::test_negate_auto_close_client_pool[pool-python-parser-True]",
"tests/test_asyncio/test_connection_pool.py::TestRedisAutoReleaseConnectionPool::test_negate_auto_close_client_pool[pool-python-parser-False]",
"tests/test_asyncio/test_connection_pool.py::TestRedisAutoReleaseConnectionPool::test_negate_auto_close_client_pool[single-hiredis-True]",
"tests/test_asyncio/test_connection_pool.py::TestRedisAutoReleaseConnectionPool::test_negate_auto_close_client_pool[single-hiredis-False]",
"tests/test_asyncio/test_connection_pool.py::TestRedisAutoReleaseConnectionPool::test_negate_auto_close_client_pool[pool-hiredis-True]",
"tests/test_asyncio/test_connection_pool.py::TestRedisAutoReleaseConnectionPool::test_negate_auto_close_client_pool[pool-hiredis-False]",
"tests/test_asyncio/test_connection_pool.py::TestConnectionPool::test_connection_creation",
"tests/test_asyncio/test_connection_pool.py::TestConnectionPool::test_aclosing",
"tests/test_asyncio/test_connection_pool.py::TestConnectionPool::test_multiple_connections",
"tests/test_asyncio/test_connection_pool.py::TestConnectionPool::test_max_connections",
"tests/test_asyncio/test_connection_pool.py::TestConnectionPool::test_reuse_previously_released_connection",
"tests/test_asyncio/test_connection_pool.py::TestConnectionPool::test_repr_contains_db_info_tcp",
"tests/test_asyncio/test_connection_pool.py::TestConnectionPool::test_repr_contains_db_info_unix",
"tests/test_asyncio/test_connection_pool.py::TestBlockingConnectionPool::test_connection_creation",
"tests/test_asyncio/test_connection_pool.py::TestBlockingConnectionPool::test_disconnect",
"tests/test_asyncio/test_connection_pool.py::TestBlockingConnectionPool::test_multiple_connections",
"tests/test_asyncio/test_connection_pool.py::TestBlockingConnectionPool::test_connection_pool_blocks_until_timeout",
"tests/test_asyncio/test_connection_pool.py::TestBlockingConnectionPool::test_connection_pool_blocks_until_conn_available",
"tests/test_asyncio/test_connection_pool.py::TestBlockingConnectionPool::test_reuse_previously_released_connection",
"tests/test_asyncio/test_connection_pool.py::TestBlockingConnectionPool::test_repr_contains_db_info_tcp",
"tests/test_asyncio/test_connection_pool.py::TestBlockingConnectionPool::test_repr_contains_db_info_unix",
"tests/test_asyncio/test_connection_pool.py::TestConnectionPoolURLParsing::test_hostname",
"tests/test_asyncio/test_connection_pool.py::TestConnectionPoolURLParsing::test_quoted_hostname",
"tests/test_asyncio/test_connection_pool.py::TestConnectionPoolURLParsing::test_port",
"tests/test_asyncio/test_connection_pool.py::TestConnectionPoolURLParsing::test_username",
"tests/test_asyncio/test_connection_pool.py::TestConnectionPoolURLParsing::test_quoted_username",
"tests/test_asyncio/test_connection_pool.py::TestConnectionPoolURLParsing::test_password",
"tests/test_asyncio/test_connection_pool.py::TestConnectionPoolURLParsing::test_quoted_password",
"tests/test_asyncio/test_connection_pool.py::TestConnectionPoolURLParsing::test_username_and_password",
"tests/test_asyncio/test_connection_pool.py::TestConnectionPoolURLParsing::test_db_as_argument",
"tests/test_asyncio/test_connection_pool.py::TestConnectionPoolURLParsing::test_db_in_path",
"tests/test_asyncio/test_connection_pool.py::TestConnectionPoolURLParsing::test_db_in_querystring",
"tests/test_asyncio/test_connection_pool.py::TestConnectionPoolURLParsing::test_extra_typed_querystring_options",
"tests/test_asyncio/test_connection_pool.py::TestConnectionPoolURLParsing::test_boolean_parsing",
"tests/test_asyncio/test_connection_pool.py::TestConnectionPoolURLParsing::test_client_name_in_querystring",
"tests/test_asyncio/test_connection_pool.py::TestConnectionPoolURLParsing::test_invalid_extra_typed_querystring_options",
"tests/test_asyncio/test_connection_pool.py::TestConnectionPoolURLParsing::test_extra_querystring_options",
"tests/test_asyncio/test_connection_pool.py::TestConnectionPoolURLParsing::test_calling_from_subclass_returns_correct_instance",
"tests/test_asyncio/test_connection_pool.py::TestConnectionPoolURLParsing::test_client_creates_connection_pool",
"tests/test_asyncio/test_connection_pool.py::TestConnectionPoolURLParsing::test_invalid_scheme_raises_error",
"tests/test_asyncio/test_connection_pool.py::TestConnectionPoolUnixSocketURLParsing::test_defaults",
"tests/test_asyncio/test_connection_pool.py::TestConnectionPoolUnixSocketURLParsing::test_username",
"tests/test_asyncio/test_connection_pool.py::TestConnectionPoolUnixSocketURLParsing::test_quoted_username",
"tests/test_asyncio/test_connection_pool.py::TestConnectionPoolUnixSocketURLParsing::test_password",
"tests/test_asyncio/test_connection_pool.py::TestConnectionPoolUnixSocketURLParsing::test_quoted_password",
"tests/test_asyncio/test_connection_pool.py::TestConnectionPoolUnixSocketURLParsing::test_quoted_path",
"tests/test_asyncio/test_connection_pool.py::TestConnectionPoolUnixSocketURLParsing::test_db_as_argument",
"tests/test_asyncio/test_connection_pool.py::TestConnectionPoolUnixSocketURLParsing::test_db_in_querystring",
"tests/test_asyncio/test_connection_pool.py::TestConnectionPoolUnixSocketURLParsing::test_client_name_in_querystring",
"tests/test_asyncio/test_connection_pool.py::TestConnectionPoolUnixSocketURLParsing::test_extra_querystring_options",
"tests/test_asyncio/test_connection_pool.py::TestSSLConnectionURLParsing::test_host",
"tests/test_asyncio/test_connection_pool.py::TestSSLConnectionURLParsing::test_cert_reqs_options",
"tests/test_asyncio/test_connection_pool.py::TestConnection::test_on_connect_error",
"tests/test_asyncio/test_connection_pool.py::TestConnection::test_busy_loading_disconnects_socket[single-python-parser]",
"tests/test_asyncio/test_connection_pool.py::TestConnection::test_busy_loading_disconnects_socket[pool-python-parser]",
"tests/test_asyncio/test_connection_pool.py::TestConnection::test_busy_loading_disconnects_socket[single-hiredis]",
"tests/test_asyncio/test_connection_pool.py::TestConnection::test_busy_loading_disconnects_socket[pool-hiredis]",
"tests/test_asyncio/test_connection_pool.py::TestConnection::test_busy_loading_from_pipeline_immediate_command[single-python-parser]",
"tests/test_asyncio/test_connection_pool.py::TestConnection::test_busy_loading_from_pipeline_immediate_command[pool-python-parser]",
"tests/test_asyncio/test_connection_pool.py::TestConnection::test_busy_loading_from_pipeline_immediate_command[single-hiredis]",
"tests/test_asyncio/test_connection_pool.py::TestConnection::test_busy_loading_from_pipeline_immediate_command[pool-hiredis]",
"tests/test_asyncio/test_connection_pool.py::TestConnection::test_busy_loading_from_pipeline[single-python-parser]",
"tests/test_asyncio/test_connection_pool.py::TestConnection::test_busy_loading_from_pipeline[pool-python-parser]",
"tests/test_asyncio/test_connection_pool.py::TestConnection::test_busy_loading_from_pipeline[single-hiredis]",
"tests/test_asyncio/test_connection_pool.py::TestConnection::test_busy_loading_from_pipeline[pool-hiredis]",
"tests/test_asyncio/test_connection_pool.py::TestConnection::test_read_only_error[single-python-parser]",
"tests/test_asyncio/test_connection_pool.py::TestConnection::test_read_only_error[pool-python-parser]",
"tests/test_asyncio/test_connection_pool.py::TestConnection::test_read_only_error[single-hiredis]",
"tests/test_asyncio/test_connection_pool.py::TestConnection::test_read_only_error[pool-hiredis]",
"tests/test_asyncio/test_connection_pool.py::TestConnection::test_oom_error[single-python-parser]",
"tests/test_asyncio/test_connection_pool.py::TestConnection::test_oom_error[pool-python-parser]",
"tests/test_asyncio/test_connection_pool.py::TestConnection::test_oom_error[single-hiredis]",
"tests/test_asyncio/test_connection_pool.py::TestConnection::test_oom_error[pool-hiredis]",
"tests/test_asyncio/test_connection_pool.py::TestConnection::test_connect_from_url_tcp",
"tests/test_asyncio/test_connection_pool.py::TestConnection::test_connect_from_url_unix",
"tests/test_asyncio/test_connection_pool.py::TestConnection::test_connect_no_auth_supplied_when_required[single-python-parser]",
"tests/test_asyncio/test_connection_pool.py::TestConnection::test_connect_no_auth_supplied_when_required[pool-python-parser]",
"tests/test_asyncio/test_connection_pool.py::TestConnection::test_connect_no_auth_supplied_when_required[single-hiredis]",
"tests/test_asyncio/test_connection_pool.py::TestConnection::test_connect_no_auth_supplied_when_required[pool-hiredis]",
"tests/test_asyncio/test_connection_pool.py::TestConnection::test_connect_invalid_password_supplied[single-python-parser]",
"tests/test_asyncio/test_connection_pool.py::TestConnection::test_connect_invalid_password_supplied[pool-python-parser]",
"tests/test_asyncio/test_connection_pool.py::TestConnection::test_connect_invalid_password_supplied[single-hiredis]",
"tests/test_asyncio/test_connection_pool.py::TestConnection::test_connect_invalid_password_supplied[pool-hiredis]",
"tests/test_connection_pool.py::TestConnectionPool::test_connection_creation",
"tests/test_connection_pool.py::TestConnectionPool::test_closing",
"tests/test_connection_pool.py::TestConnectionPool::test_multiple_connections",
"tests/test_connection_pool.py::TestConnectionPool::test_max_connections",
"tests/test_connection_pool.py::TestConnectionPool::test_reuse_previously_released_connection",
"tests/test_connection_pool.py::TestConnectionPool::test_repr_contains_db_info_tcp",
"tests/test_connection_pool.py::TestConnectionPool::test_repr_contains_db_info_unix",
"tests/test_connection_pool.py::TestBlockingConnectionPool::test_connection_creation",
"tests/test_connection_pool.py::TestBlockingConnectionPool::test_multiple_connections",
"tests/test_connection_pool.py::TestBlockingConnectionPool::test_connection_pool_blocks_until_timeout",
"tests/test_connection_pool.py::TestBlockingConnectionPool::test_connection_pool_blocks_until_conn_available",
"tests/test_connection_pool.py::TestBlockingConnectionPool::test_reuse_previously_released_connection",
"tests/test_connection_pool.py::TestBlockingConnectionPool::test_repr_contains_db_info_tcp",
"tests/test_connection_pool.py::TestBlockingConnectionPool::test_repr_contains_db_info_unix",
"tests/test_connection_pool.py::TestConnectionPoolURLParsing::test_hostname",
"tests/test_connection_pool.py::TestConnectionPoolURLParsing::test_quoted_hostname",
"tests/test_connection_pool.py::TestConnectionPoolURLParsing::test_port",
"tests/test_connection_pool.py::TestConnectionPoolURLParsing::test_username",
"tests/test_connection_pool.py::TestConnectionPoolURLParsing::test_quoted_username",
"tests/test_connection_pool.py::TestConnectionPoolURLParsing::test_password",
"tests/test_connection_pool.py::TestConnectionPoolURLParsing::test_quoted_password",
"tests/test_connection_pool.py::TestConnectionPoolURLParsing::test_username_and_password",
"tests/test_connection_pool.py::TestConnectionPoolURLParsing::test_db_as_argument",
"tests/test_connection_pool.py::TestConnectionPoolURLParsing::test_db_in_path",
"tests/test_connection_pool.py::TestConnectionPoolURLParsing::test_db_in_querystring",
"tests/test_connection_pool.py::TestConnectionPoolURLParsing::test_extra_typed_querystring_options",
"tests/test_connection_pool.py::TestConnectionPoolURLParsing::test_boolean_parsing",
"tests/test_connection_pool.py::TestConnectionPoolURLParsing::test_client_name_in_querystring",
"tests/test_connection_pool.py::TestConnectionPoolURLParsing::test_invalid_extra_typed_querystring_options",
"tests/test_connection_pool.py::TestConnectionPoolURLParsing::test_extra_querystring_options",
"tests/test_connection_pool.py::TestConnectionPoolURLParsing::test_calling_from_subclass_returns_correct_instance",
"tests/test_connection_pool.py::TestConnectionPoolURLParsing::test_client_creates_connection_pool",
"tests/test_connection_pool.py::TestConnectionPoolURLParsing::test_invalid_scheme_raises_error",
"tests/test_connection_pool.py::TestConnectionPoolURLParsing::test_invalid_scheme_raises_error_when_double_slash_missing",
"tests/test_connection_pool.py::TestConnectionPoolUnixSocketURLParsing::test_defaults",
"tests/test_connection_pool.py::TestConnectionPoolUnixSocketURLParsing::test_username",
"tests/test_connection_pool.py::TestConnectionPoolUnixSocketURLParsing::test_quoted_username",
"tests/test_connection_pool.py::TestConnectionPoolUnixSocketURLParsing::test_password",
"tests/test_connection_pool.py::TestConnectionPoolUnixSocketURLParsing::test_quoted_password",
"tests/test_connection_pool.py::TestConnectionPoolUnixSocketURLParsing::test_quoted_path",
"tests/test_connection_pool.py::TestConnectionPoolUnixSocketURLParsing::test_db_as_argument",
"tests/test_connection_pool.py::TestConnectionPoolUnixSocketURLParsing::test_db_in_querystring",
"tests/test_connection_pool.py::TestConnectionPoolUnixSocketURLParsing::test_client_name_in_querystring",
"tests/test_connection_pool.py::TestConnectionPoolUnixSocketURLParsing::test_extra_querystring_options",
"tests/test_connection_pool.py::TestConnectionPoolUnixSocketURLParsing::test_connection_class_override",
"tests/test_connection_pool.py::TestSSLConnectionURLParsing::test_host",
"tests/test_connection_pool.py::TestSSLConnectionURLParsing::test_connection_class_override",
"tests/test_connection_pool.py::TestSSLConnectionURLParsing::test_cert_reqs_options",
"tests/test_connection_pool.py::TestConnection::test_on_connect_error",
"tests/test_connection_pool.py::TestConnection::test_busy_loading_disconnects_socket",
"tests/test_connection_pool.py::TestConnection::test_busy_loading_from_pipeline_immediate_command",
"tests/test_connection_pool.py::TestConnection::test_busy_loading_from_pipeline",
"tests/test_connection_pool.py::TestConnection::test_read_only_error",
"tests/test_connection_pool.py::TestConnection::test_oom_error",
"tests/test_connection_pool.py::TestConnection::test_connect_from_url_tcp",
"tests/test_connection_pool.py::TestConnection::test_connect_from_url_unix",
"tests/test_connection_pool.py::TestConnection::test_connect_no_auth_configured",
"tests/test_connection_pool.py::TestConnection::test_connect_invalid_auth_credentials_supplied",
"tests/test_connection_pool.py::TestMultiConnectionClient::test_multi_connection_command",
"tests/test_connection_pool.py::TestHealthCheck::test_health_check_runs",
"tests/test_connection_pool.py::TestHealthCheck::test_arbitrary_command_invokes_health_check",
"tests/test_connection_pool.py::TestHealthCheck::test_arbitrary_command_advances_next_health_check",
"tests/test_connection_pool.py::TestHealthCheck::test_health_check_not_invoked_within_interval",
"tests/test_connection_pool.py::TestHealthCheck::test_health_check_in_pipeline",
"tests/test_connection_pool.py::TestHealthCheck::test_health_check_in_transaction",
"tests/test_connection_pool.py::TestHealthCheck::test_health_check_in_watched_pipeline",
"tests/test_connection_pool.py::TestHealthCheck::test_health_check_in_pubsub_before_subscribe",
"tests/test_connection_pool.py::TestHealthCheck::test_health_check_in_pubsub_after_subscribed",
"tests/test_connection_pool.py::TestHealthCheck::test_health_check_in_pubsub_poll"
] | [
"tests/test_asyncio/test_connection_pool.py::TestBlockingConnectionPoolURLParsing::test_extra_typed_querystring_options",
"tests/test_asyncio/test_connection_pool.py::TestBlockingConnectionPoolURLParsing::test_invalid_extra_typed_querystring_options",
"tests/test_connection_pool.py::TestBlockingConnectionPoolURLParsing::test_extra_typed_querystring_options",
"tests/test_connection_pool.py::TestBlockingConnectionPoolURLParsing::test_invalid_extra_typed_querystring_options"
] | Python | [] | [] |
|
redis/redis-py | 3,062 | redis__redis-py-3062 | [
"3059"
] | e13356db9220de1196ca3b7d10a2de623cc15b69 | diff --git a/redis/commands/core.py b/redis/commands/core.py
--- a/redis/commands/core.py
+++ b/redis/commands/core.py
@@ -97,6 +97,7 @@ def acl_genpass(self, bits: Union[int, None] = None, **kwargs) -> ResponseT:
b = int(bits)
if b < 0 or b > 4096:
raise ValueError
+ pieces.append(b)
except ValueError:
raise DataError(
"genpass optionally accepts a bits argument, between 0 and 4096."
| diff --git a/tests/test_commands.py b/tests/test_commands.py
--- a/tests/test_commands.py
+++ b/tests/test_commands.py
@@ -201,8 +201,9 @@ def test_acl_genpass(self, r):
r.acl_genpass(-5)
r.acl_genpass(5555)
- r.acl_genpass(555)
+ password = r.acl_genpass(555)
assert isinstance(password, (str, bytes))
+ assert len(password) == 139
@skip_if_server_version_lt("7.0.0")
@skip_if_redis_enterprise()
| "bug" about acl_genpass method
Thanks for wanting to report an issue you've found in redis-py. Please delete this text and fill in the template below.
It is of course not always possible to reduce your code to a small test case, but it's highly appreciated to have as much data as possible. Thank you!
**Version**: 5.0.1, "bug" about acl_genpass method
**Platform**: win11
**Description**: Refer to the following demo
demo:
```python
from redis import Redis
url = "redis://localhost:6379/1"
rdb = Redis.from_url(url)
print(rdb.acl_genpass(32))
```
This script is similar to `ACL GENPASS [bits]`, we will get an 8-digit password. But what we got didn't meet expectations.
Your source code might get the desired result this way:
```python
def acl_genpass(self, bits: Union[int, None] = None, **kwargs) -> ResponseT:
"""Generate a random password value.
If ``bits`` is supplied then use this number of bits, rounded to
the next multiple of 4.
See: https://redis.io/commands/acl-genpass
"""
pieces = []
if bits is not None:
try:
b = int(bits)
if b < 0 or b > 4096:
raise ValueError
pieces.append(b)
except ValueError:
raise DataError(
"genpass optionally accepts a bits argument, between 0 and 4096."
)
return self.execute_command("ACL GENPASS", *pieces, **kwargs)
```
| "2023-12-03T01:28:27Z" | 5.1 | [
"tests/test_commands.py::TestResponseCallbacks::test_response_callbacks",
"tests/test_commands.py::TestResponseCallbacks::test_case_insensitive_command_names",
"tests/test_commands.py::TestRedisCommands::test_auth",
"tests/test_commands.py::TestRedisCommands::test_command_on_invalid_key_type",
"tests/test_commands.py::TestRedisCommands::test_acl_cat_no_category",
"tests/test_commands.py::TestRedisCommands::test_acl_cat_with_category",
"tests/test_commands.py::TestRedisCommands::test_acl_dryrun",
"tests/test_commands.py::TestRedisCommands::test_acl_deluser",
"tests/test_commands.py::TestRedisCommands::test_acl_getuser_setuser",
"tests/test_commands.py::TestRedisCommands::test_acl_help",
"tests/test_commands.py::TestRedisCommands::test_acl_list",
"tests/test_commands.py::TestRedisCommands::test_acl_log",
"tests/test_commands.py::TestRedisCommands::test_acl_setuser_categories_without_prefix_fails",
"tests/test_commands.py::TestRedisCommands::test_acl_setuser_commands_without_prefix_fails",
"tests/test_commands.py::TestRedisCommands::test_acl_setuser_add_passwords_and_nopass_fails",
"tests/test_commands.py::TestRedisCommands::test_acl_users",
"tests/test_commands.py::TestRedisCommands::test_acl_whoami",
"tests/test_commands.py::TestRedisCommands::test_client_list",
"tests/test_commands.py::TestRedisCommands::test_client_info",
"tests/test_commands.py::TestRedisCommands::test_client_list_types_not_replica",
"tests/test_commands.py::TestRedisCommands::test_client_list_replica",
"tests/test_commands.py::TestRedisCommands::test_client_list_client_id",
"tests/test_commands.py::TestRedisCommands::test_client_id",
"tests/test_commands.py::TestRedisCommands::test_client_trackinginfo",
"tests/test_commands.py::TestRedisCommands::test_client_tracking",
"tests/test_commands.py::TestRedisCommands::test_client_unblock",
"tests/test_commands.py::TestRedisCommands::test_client_getname",
"tests/test_commands.py::TestRedisCommands::test_client_setname",
"tests/test_commands.py::TestRedisCommands::test_client_setinfo",
"tests/test_commands.py::TestRedisCommands::test_client_kill",
"tests/test_commands.py::TestRedisCommands::test_client_kill_filter_invalid_params",
"tests/test_commands.py::TestRedisCommands::test_client_kill_filter_by_id",
"tests/test_commands.py::TestRedisCommands::test_client_kill_filter_by_addr",
"tests/test_commands.py::TestRedisCommands::test_client_list_after_client_setname",
"tests/test_commands.py::TestRedisCommands::test_client_kill_filter_by_laddr",
"tests/test_commands.py::TestRedisCommands::test_client_kill_filter_by_user",
"tests/test_commands.py::TestRedisCommands::test_client_pause",
"tests/test_commands.py::TestRedisCommands::test_client_pause_all",
"tests/test_commands.py::TestRedisCommands::test_client_unpause",
"tests/test_commands.py::TestRedisCommands::test_client_no_evict",
"tests/test_commands.py::TestRedisCommands::test_client_no_touch",
"tests/test_commands.py::TestRedisCommands::test_client_reply",
"tests/test_commands.py::TestRedisCommands::test_client_getredir",
"tests/test_commands.py::TestRedisCommands::test_hello_notI_implemented",
"tests/test_commands.py::TestRedisCommands::test_config_get",
"tests/test_commands.py::TestRedisCommands::test_config_get_multi_params",
"tests/test_commands.py::TestRedisCommands::test_config_resetstat",
"tests/test_commands.py::TestRedisCommands::test_config_set",
"tests/test_commands.py::TestRedisCommands::test_config_set_multi_params",
"tests/test_commands.py::TestRedisCommands::test_failover",
"tests/test_commands.py::TestRedisCommands::test_dbsize",
"tests/test_commands.py::TestRedisCommands::test_echo",
"tests/test_commands.py::TestRedisCommands::test_info",
"tests/test_commands.py::TestRedisCommands::test_info_multi_sections",
"tests/test_commands.py::TestRedisCommands::test_lastsave",
"tests/test_commands.py::TestRedisCommands::test_lolwut",
"tests/test_commands.py::TestRedisCommands::test_reset",
"tests/test_commands.py::TestRedisCommands::test_object",
"tests/test_commands.py::TestRedisCommands::test_ping",
"tests/test_commands.py::TestRedisCommands::test_quit",
"tests/test_commands.py::TestRedisCommands::test_role",
"tests/test_commands.py::TestRedisCommands::test_select",
"tests/test_commands.py::TestRedisCommands::test_slowlog_get",
"tests/test_commands.py::TestRedisCommands::test_slowlog_get_limit",
"tests/test_commands.py::TestRedisCommands::test_slowlog_length",
"tests/test_commands.py::TestRedisCommands::test_time",
"tests/test_commands.py::TestRedisCommands::test_bgsave",
"tests/test_commands.py::TestRedisCommands::test_never_decode_option",
"tests/test_commands.py::TestRedisCommands::test_empty_response_option",
"tests/test_commands.py::TestRedisCommands::test_append",
"tests/test_commands.py::TestRedisCommands::test_bitcount",
"tests/test_commands.py::TestRedisCommands::test_bitcount_mode",
"tests/test_commands.py::TestRedisCommands::test_bitop_not_empty_string",
"tests/test_commands.py::TestRedisCommands::test_bitop_not",
"tests/test_commands.py::TestRedisCommands::test_bitop_not_in_place",
"tests/test_commands.py::TestRedisCommands::test_bitop_single_string",
"tests/test_commands.py::TestRedisCommands::test_bitop_string_operands",
"tests/test_commands.py::TestRedisCommands::test_bitpos",
"tests/test_commands.py::TestRedisCommands::test_bitpos_wrong_arguments",
"tests/test_commands.py::TestRedisCommands::test_bitpos_mode",
"tests/test_commands.py::TestRedisCommands::test_copy",
"tests/test_commands.py::TestRedisCommands::test_copy_and_replace",
"tests/test_commands.py::TestRedisCommands::test_copy_to_another_database",
"tests/test_commands.py::TestRedisCommands::test_decr",
"tests/test_commands.py::TestRedisCommands::test_decrby",
"tests/test_commands.py::TestRedisCommands::test_delete",
"tests/test_commands.py::TestRedisCommands::test_delete_with_multiple_keys",
"tests/test_commands.py::TestRedisCommands::test_delitem",
"tests/test_commands.py::TestRedisCommands::test_unlink",
"tests/test_commands.py::TestRedisCommands::test_unlink_with_multiple_keys",
"tests/test_commands.py::TestRedisCommands::test_lcs",
"tests/test_commands.py::TestRedisCommands::test_dump_and_restore",
"tests/test_commands.py::TestRedisCommands::test_dump_and_restore_and_replace",
"tests/test_commands.py::TestRedisCommands::test_dump_and_restore_absttl",
"tests/test_commands.py::TestRedisCommands::test_exists",
"tests/test_commands.py::TestRedisCommands::test_exists_contains",
"tests/test_commands.py::TestRedisCommands::test_expire",
"tests/test_commands.py::TestRedisCommands::test_expire_option_nx",
"tests/test_commands.py::TestRedisCommands::test_expire_option_xx",
"tests/test_commands.py::TestRedisCommands::test_expire_option_gt",
"tests/test_commands.py::TestRedisCommands::test_expire_option_lt",
"tests/test_commands.py::TestRedisCommands::test_expireat_datetime",
"tests/test_commands.py::TestRedisCommands::test_expireat_no_key",
"tests/test_commands.py::TestRedisCommands::test_expireat_unixtime",
"tests/test_commands.py::TestRedisCommands::test_expiretime",
"tests/test_commands.py::TestRedisCommands::test_expireat_option_nx",
"tests/test_commands.py::TestRedisCommands::test_expireat_option_xx",
"tests/test_commands.py::TestRedisCommands::test_expireat_option_gt",
"tests/test_commands.py::TestRedisCommands::test_expireat_option_lt",
"tests/test_commands.py::TestRedisCommands::test_get_and_set",
"tests/test_commands.py::TestRedisCommands::test_getdel",
"tests/test_commands.py::TestRedisCommands::test_getex",
"tests/test_commands.py::TestRedisCommands::test_getitem_and_setitem",
"tests/test_commands.py::TestRedisCommands::test_getitem_raises_keyerror_for_missing_key",
"tests/test_commands.py::TestRedisCommands::test_getitem_does_not_raise_keyerror_for_empty_string",
"tests/test_commands.py::TestRedisCommands::test_get_set_bit",
"tests/test_commands.py::TestRedisCommands::test_getrange",
"tests/test_commands.py::TestRedisCommands::test_getset",
"tests/test_commands.py::TestRedisCommands::test_incr",
"tests/test_commands.py::TestRedisCommands::test_incrby",
"tests/test_commands.py::TestRedisCommands::test_incrbyfloat",
"tests/test_commands.py::TestRedisCommands::test_keys",
"tests/test_commands.py::TestRedisCommands::test_mget",
"tests/test_commands.py::TestRedisCommands::test_lmove",
"tests/test_commands.py::TestRedisCommands::test_blmove",
"tests/test_commands.py::TestRedisCommands::test_mset",
"tests/test_commands.py::TestRedisCommands::test_msetnx",
"tests/test_commands.py::TestRedisCommands::test_pexpire",
"tests/test_commands.py::TestRedisCommands::test_pexpire_option_nx",
"tests/test_commands.py::TestRedisCommands::test_pexpire_option_xx",
"tests/test_commands.py::TestRedisCommands::test_pexpire_option_gt",
"tests/test_commands.py::TestRedisCommands::test_pexpire_option_lt",
"tests/test_commands.py::TestRedisCommands::test_pexpireat_datetime",
"tests/test_commands.py::TestRedisCommands::test_pexpireat_no_key",
"tests/test_commands.py::TestRedisCommands::test_pexpireat_unixtime",
"tests/test_commands.py::TestRedisCommands::test_pexpireat_option_nx",
"tests/test_commands.py::TestRedisCommands::test_pexpireat_option_xx",
"tests/test_commands.py::TestRedisCommands::test_pexpireat_option_gt",
"tests/test_commands.py::TestRedisCommands::test_pexpireat_option_lt",
"tests/test_commands.py::TestRedisCommands::test_pexpiretime",
"tests/test_commands.py::TestRedisCommands::test_psetex",
"tests/test_commands.py::TestRedisCommands::test_psetex_timedelta",
"tests/test_commands.py::TestRedisCommands::test_pttl",
"tests/test_commands.py::TestRedisCommands::test_pttl_no_key",
"tests/test_commands.py::TestRedisCommands::test_hrandfield",
"tests/test_commands.py::TestRedisCommands::test_randomkey",
"tests/test_commands.py::TestRedisCommands::test_rename",
"tests/test_commands.py::TestRedisCommands::test_renamenx",
"tests/test_commands.py::TestRedisCommands::test_set_nx",
"tests/test_commands.py::TestRedisCommands::test_set_xx",
"tests/test_commands.py::TestRedisCommands::test_set_px",
"tests/test_commands.py::TestRedisCommands::test_set_px_timedelta",
"tests/test_commands.py::TestRedisCommands::test_set_ex",
"tests/test_commands.py::TestRedisCommands::test_set_ex_str",
"tests/test_commands.py::TestRedisCommands::test_set_ex_timedelta",
"tests/test_commands.py::TestRedisCommands::test_set_exat_timedelta",
"tests/test_commands.py::TestRedisCommands::test_set_pxat_timedelta",
"tests/test_commands.py::TestRedisCommands::test_set_multipleoptions",
"tests/test_commands.py::TestRedisCommands::test_set_keepttl",
"tests/test_commands.py::TestRedisCommands::test_set_get",
"tests/test_commands.py::TestRedisCommands::test_setex",
"tests/test_commands.py::TestRedisCommands::test_setnx",
"tests/test_commands.py::TestRedisCommands::test_setrange",
"tests/test_commands.py::TestRedisCommands::test_strlen",
"tests/test_commands.py::TestRedisCommands::test_substr",
"tests/test_commands.py::TestRedisCommands::test_ttl",
"tests/test_commands.py::TestRedisCommands::test_ttl_nokey",
"tests/test_commands.py::TestRedisCommands::test_type",
"tests/test_commands.py::TestRedisCommands::test_blpop",
"tests/test_commands.py::TestRedisCommands::test_brpop",
"tests/test_commands.py::TestRedisCommands::test_brpoplpush",
"tests/test_commands.py::TestRedisCommands::test_brpoplpush_empty_string",
"tests/test_commands.py::TestRedisCommands::test_blmpop",
"tests/test_commands.py::TestRedisCommands::test_lmpop",
"tests/test_commands.py::TestRedisCommands::test_lindex",
"tests/test_commands.py::TestRedisCommands::test_linsert",
"tests/test_commands.py::TestRedisCommands::test_llen",
"tests/test_commands.py::TestRedisCommands::test_lpop",
"tests/test_commands.py::TestRedisCommands::test_lpop_count",
"tests/test_commands.py::TestRedisCommands::test_lpush",
"tests/test_commands.py::TestRedisCommands::test_lpushx",
"tests/test_commands.py::TestRedisCommands::test_lpushx_with_list",
"tests/test_commands.py::TestRedisCommands::test_lrange",
"tests/test_commands.py::TestRedisCommands::test_lrem",
"tests/test_commands.py::TestRedisCommands::test_lset",
"tests/test_commands.py::TestRedisCommands::test_ltrim",
"tests/test_commands.py::TestRedisCommands::test_rpop",
"tests/test_commands.py::TestRedisCommands::test_rpop_count",
"tests/test_commands.py::TestRedisCommands::test_rpoplpush",
"tests/test_commands.py::TestRedisCommands::test_rpush",
"tests/test_commands.py::TestRedisCommands::test_lpos",
"tests/test_commands.py::TestRedisCommands::test_rpushx",
"tests/test_commands.py::TestRedisCommands::test_scan",
"tests/test_commands.py::TestRedisCommands::test_scan_type",
"tests/test_commands.py::TestRedisCommands::test_scan_iter",
"tests/test_commands.py::TestRedisCommands::test_sscan",
"tests/test_commands.py::TestRedisCommands::test_sscan_iter",
"tests/test_commands.py::TestRedisCommands::test_hscan",
"tests/test_commands.py::TestRedisCommands::test_hscan_iter",
"tests/test_commands.py::TestRedisCommands::test_zscan",
"tests/test_commands.py::TestRedisCommands::test_zscan_iter",
"tests/test_commands.py::TestRedisCommands::test_sadd",
"tests/test_commands.py::TestRedisCommands::test_scard",
"tests/test_commands.py::TestRedisCommands::test_sdiff",
"tests/test_commands.py::TestRedisCommands::test_sdiffstore",
"tests/test_commands.py::TestRedisCommands::test_sinter",
"tests/test_commands.py::TestRedisCommands::test_sintercard",
"tests/test_commands.py::TestRedisCommands::test_sinterstore",
"tests/test_commands.py::TestRedisCommands::test_sismember",
"tests/test_commands.py::TestRedisCommands::test_smembers",
"tests/test_commands.py::TestRedisCommands::test_smismember",
"tests/test_commands.py::TestRedisCommands::test_smove",
"tests/test_commands.py::TestRedisCommands::test_spop",
"tests/test_commands.py::TestRedisCommands::test_spop_multi_value",
"tests/test_commands.py::TestRedisCommands::test_srandmember",
"tests/test_commands.py::TestRedisCommands::test_srandmember_multi_value",
"tests/test_commands.py::TestRedisCommands::test_srem",
"tests/test_commands.py::TestRedisCommands::test_sunion",
"tests/test_commands.py::TestRedisCommands::test_sunionstore",
"tests/test_commands.py::TestRedisCommands::test_debug_segfault",
"tests/test_commands.py::TestRedisCommands::test_script_debug",
"tests/test_commands.py::TestRedisCommands::test_zadd",
"tests/test_commands.py::TestRedisCommands::test_zadd_nx",
"tests/test_commands.py::TestRedisCommands::test_zadd_xx",
"tests/test_commands.py::TestRedisCommands::test_zadd_ch",
"tests/test_commands.py::TestRedisCommands::test_zadd_incr",
"tests/test_commands.py::TestRedisCommands::test_zadd_incr_with_xx",
"tests/test_commands.py::TestRedisCommands::test_zadd_gt_lt",
"tests/test_commands.py::TestRedisCommands::test_zcard",
"tests/test_commands.py::TestRedisCommands::test_zcount",
"tests/test_commands.py::TestRedisCommands::test_zdiff",
"tests/test_commands.py::TestRedisCommands::test_zdiffstore",
"tests/test_commands.py::TestRedisCommands::test_zincrby",
"tests/test_commands.py::TestRedisCommands::test_zlexcount",
"tests/test_commands.py::TestRedisCommands::test_zinter",
"tests/test_commands.py::TestRedisCommands::test_zintercard",
"tests/test_commands.py::TestRedisCommands::test_zinterstore_sum",
"tests/test_commands.py::TestRedisCommands::test_zinterstore_max",
"tests/test_commands.py::TestRedisCommands::test_zinterstore_min",
"tests/test_commands.py::TestRedisCommands::test_zinterstore_with_weight",
"tests/test_commands.py::TestRedisCommands::test_zpopmax",
"tests/test_commands.py::TestRedisCommands::test_zpopmin",
"tests/test_commands.py::TestRedisCommands::test_zrandemember",
"tests/test_commands.py::TestRedisCommands::test_bzpopmax",
"tests/test_commands.py::TestRedisCommands::test_bzpopmin",
"tests/test_commands.py::TestRedisCommands::test_zmpop",
"tests/test_commands.py::TestRedisCommands::test_bzmpop",
"tests/test_commands.py::TestRedisCommands::test_zrange",
"tests/test_commands.py::TestRedisCommands::test_zrange_errors",
"tests/test_commands.py::TestRedisCommands::test_zrange_params",
"tests/test_commands.py::TestRedisCommands::test_zrangestore",
"tests/test_commands.py::TestRedisCommands::test_zrangebylex",
"tests/test_commands.py::TestRedisCommands::test_zrevrangebylex",
"tests/test_commands.py::TestRedisCommands::test_zrangebyscore",
"tests/test_commands.py::TestRedisCommands::test_zrank",
"tests/test_commands.py::TestRedisCommands::test_zrank_withscore",
"tests/test_commands.py::TestRedisCommands::test_zrem",
"tests/test_commands.py::TestRedisCommands::test_zrem_multiple_keys",
"tests/test_commands.py::TestRedisCommands::test_zremrangebylex",
"tests/test_commands.py::TestRedisCommands::test_zremrangebyrank",
"tests/test_commands.py::TestRedisCommands::test_zremrangebyscore",
"tests/test_commands.py::TestRedisCommands::test_zrevrange",
"tests/test_commands.py::TestRedisCommands::test_zrevrangebyscore",
"tests/test_commands.py::TestRedisCommands::test_zrevrank",
"tests/test_commands.py::TestRedisCommands::test_zrevrank_withscore",
"tests/test_commands.py::TestRedisCommands::test_zscore",
"tests/test_commands.py::TestRedisCommands::test_zunion",
"tests/test_commands.py::TestRedisCommands::test_zunionstore_sum",
"tests/test_commands.py::TestRedisCommands::test_zunionstore_max",
"tests/test_commands.py::TestRedisCommands::test_zunionstore_min",
"tests/test_commands.py::TestRedisCommands::test_zunionstore_with_weight",
"tests/test_commands.py::TestRedisCommands::test_zmscore",
"tests/test_commands.py::TestRedisCommands::test_pfadd",
"tests/test_commands.py::TestRedisCommands::test_pfcount",
"tests/test_commands.py::TestRedisCommands::test_pfmerge",
"tests/test_commands.py::TestRedisCommands::test_hget_and_hset",
"tests/test_commands.py::TestRedisCommands::test_hset_with_multi_key_values",
"tests/test_commands.py::TestRedisCommands::test_hset_with_key_values_passed_as_list",
"tests/test_commands.py::TestRedisCommands::test_hset_without_data",
"tests/test_commands.py::TestRedisCommands::test_hdel",
"tests/test_commands.py::TestRedisCommands::test_hexists",
"tests/test_commands.py::TestRedisCommands::test_hgetall",
"tests/test_commands.py::TestRedisCommands::test_hincrby",
"tests/test_commands.py::TestRedisCommands::test_hincrbyfloat",
"tests/test_commands.py::TestRedisCommands::test_hkeys",
"tests/test_commands.py::TestRedisCommands::test_hlen",
"tests/test_commands.py::TestRedisCommands::test_hmget",
"tests/test_commands.py::TestRedisCommands::test_hmset",
"tests/test_commands.py::TestRedisCommands::test_hsetnx",
"tests/test_commands.py::TestRedisCommands::test_hvals",
"tests/test_commands.py::TestRedisCommands::test_hstrlen",
"tests/test_commands.py::TestRedisCommands::test_sort_basic",
"tests/test_commands.py::TestRedisCommands::test_sort_limited",
"tests/test_commands.py::TestRedisCommands::test_sort_by",
"tests/test_commands.py::TestRedisCommands::test_sort_get",
"tests/test_commands.py::TestRedisCommands::test_sort_get_multi",
"tests/test_commands.py::TestRedisCommands::test_sort_get_groups_two",
"tests/test_commands.py::TestRedisCommands::test_sort_groups_string_get",
"tests/test_commands.py::TestRedisCommands::test_sort_groups_just_one_get",
"tests/test_commands.py::TestRedisCommands::test_sort_groups_no_get",
"tests/test_commands.py::TestRedisCommands::test_sort_groups_three_gets",
"tests/test_commands.py::TestRedisCommands::test_sort_desc",
"tests/test_commands.py::TestRedisCommands::test_sort_alpha",
"tests/test_commands.py::TestRedisCommands::test_sort_store",
"tests/test_commands.py::TestRedisCommands::test_sort_all_options",
"tests/test_commands.py::TestRedisCommands::test_sort_ro",
"tests/test_commands.py::TestRedisCommands::test_sort_issue_924",
"tests/test_commands.py::TestRedisCommands::test_cluster_addslots",
"tests/test_commands.py::TestRedisCommands::test_cluster_count_failure_reports",
"tests/test_commands.py::TestRedisCommands::test_cluster_countkeysinslot",
"tests/test_commands.py::TestRedisCommands::test_cluster_delslots",
"tests/test_commands.py::TestRedisCommands::test_cluster_failover",
"tests/test_commands.py::TestRedisCommands::test_cluster_forget",
"tests/test_commands.py::TestRedisCommands::test_cluster_info",
"tests/test_commands.py::TestRedisCommands::test_cluster_keyslot",
"tests/test_commands.py::TestRedisCommands::test_cluster_meet",
"tests/test_commands.py::TestRedisCommands::test_cluster_nodes",
"tests/test_commands.py::TestRedisCommands::test_cluster_replicate",
"tests/test_commands.py::TestRedisCommands::test_cluster_reset",
"tests/test_commands.py::TestRedisCommands::test_cluster_saveconfig",
"tests/test_commands.py::TestRedisCommands::test_cluster_setslot",
"tests/test_commands.py::TestRedisCommands::test_cluster_slaves",
"tests/test_commands.py::TestRedisCommands::test_readonly_invalid_cluster_state",
"tests/test_commands.py::TestRedisCommands::test_readonly",
"tests/test_commands.py::TestRedisCommands::test_geoadd",
"tests/test_commands.py::TestRedisCommands::test_geoadd_nx",
"tests/test_commands.py::TestRedisCommands::test_geoadd_xx",
"tests/test_commands.py::TestRedisCommands::test_geoadd_ch",
"tests/test_commands.py::TestRedisCommands::test_geoadd_invalid_params",
"tests/test_commands.py::TestRedisCommands::test_geodist",
"tests/test_commands.py::TestRedisCommands::test_geodist_units",
"tests/test_commands.py::TestRedisCommands::test_geodist_missing_one_member",
"tests/test_commands.py::TestRedisCommands::test_geodist_invalid_units",
"tests/test_commands.py::TestRedisCommands::test_geohash",
"tests/test_commands.py::TestRedisCommands::test_geopos",
"tests/test_commands.py::TestRedisCommands::test_geopos_no_value",
"tests/test_commands.py::TestRedisCommands::test_geosearch",
"tests/test_commands.py::TestRedisCommands::test_geosearch_member",
"tests/test_commands.py::TestRedisCommands::test_geosearch_sort",
"tests/test_commands.py::TestRedisCommands::test_geosearch_with",
"tests/test_commands.py::TestRedisCommands::test_geosearch_negative",
"tests/test_commands.py::TestRedisCommands::test_geosearchstore",
"tests/test_commands.py::TestRedisCommands::test_geosearchstore_dist",
"tests/test_commands.py::TestRedisCommands::test_georadius_Issue2609",
"tests/test_commands.py::TestRedisCommands::test_georadius",
"tests/test_commands.py::TestRedisCommands::test_georadius_no_values",
"tests/test_commands.py::TestRedisCommands::test_georadius_units",
"tests/test_commands.py::TestRedisCommands::test_georadius_with",
"tests/test_commands.py::TestRedisCommands::test_georadius_count",
"tests/test_commands.py::TestRedisCommands::test_georadius_sort",
"tests/test_commands.py::TestRedisCommands::test_georadius_store",
"tests/test_commands.py::TestRedisCommands::test_georadius_store_dist",
"tests/test_commands.py::TestRedisCommands::test_georadiusmember",
"tests/test_commands.py::TestRedisCommands::test_georadiusmember_count",
"tests/test_commands.py::TestRedisCommands::test_xack",
"tests/test_commands.py::TestRedisCommands::test_xadd",
"tests/test_commands.py::TestRedisCommands::test_xadd_nomkstream",
"tests/test_commands.py::TestRedisCommands::test_xadd_minlen_and_limit",
"tests/test_commands.py::TestRedisCommands::test_xadd_explicit_ms",
"tests/test_commands.py::TestRedisCommands::test_xautoclaim",
"tests/test_commands.py::TestRedisCommands::test_xautoclaim_negative",
"tests/test_commands.py::TestRedisCommands::test_xclaim",
"tests/test_commands.py::TestRedisCommands::test_xclaim_trimmed",
"tests/test_commands.py::TestRedisCommands::test_xdel",
"tests/test_commands.py::TestRedisCommands::test_xgroup_create",
"tests/test_commands.py::TestRedisCommands::test_xgroup_create_mkstream",
"tests/test_commands.py::TestRedisCommands::test_xgroup_create_entriesread",
"tests/test_commands.py::TestRedisCommands::test_xgroup_delconsumer",
"tests/test_commands.py::TestRedisCommands::test_xgroup_createconsumer",
"tests/test_commands.py::TestRedisCommands::test_xgroup_destroy",
"tests/test_commands.py::TestRedisCommands::test_xgroup_setid",
"tests/test_commands.py::TestRedisCommands::test_xinfo_consumers",
"tests/test_commands.py::TestRedisCommands::test_xinfo_stream",
"tests/test_commands.py::TestRedisCommands::test_xinfo_stream_full",
"tests/test_commands.py::TestRedisCommands::test_xlen",
"tests/test_commands.py::TestRedisCommands::test_xpending",
"tests/test_commands.py::TestRedisCommands::test_xpending_range",
"tests/test_commands.py::TestRedisCommands::test_xpending_range_idle",
"tests/test_commands.py::TestRedisCommands::test_xpending_range_negative",
"tests/test_commands.py::TestRedisCommands::test_xrange",
"tests/test_commands.py::TestRedisCommands::test_xread",
"tests/test_commands.py::TestRedisCommands::test_xreadgroup",
"tests/test_commands.py::TestRedisCommands::test_xrevrange",
"tests/test_commands.py::TestRedisCommands::test_xtrim",
"tests/test_commands.py::TestRedisCommands::test_xtrim_minlen_and_length_args",
"tests/test_commands.py::TestRedisCommands::test_bitfield_operations",
"tests/test_commands.py::TestRedisCommands::test_bitfield_ro",
"tests/test_commands.py::TestRedisCommands::test_memory_help",
"tests/test_commands.py::TestRedisCommands::test_memory_doctor",
"tests/test_commands.py::TestRedisCommands::test_memory_malloc_stats",
"tests/test_commands.py::TestRedisCommands::test_memory_stats",
"tests/test_commands.py::TestRedisCommands::test_memory_usage",
"tests/test_commands.py::TestRedisCommands::test_latency_histogram_not_implemented",
"tests/test_commands.py::TestRedisCommands::test_latency_graph_not_implemented",
"tests/test_commands.py::TestRedisCommands::test_latency_doctor_not_implemented",
"tests/test_commands.py::TestRedisCommands::test_latency_history",
"tests/test_commands.py::TestRedisCommands::test_latency_latest",
"tests/test_commands.py::TestRedisCommands::test_latency_reset",
"tests/test_commands.py::TestRedisCommands::test_module_list",
"tests/test_commands.py::TestRedisCommands::test_command_count",
"tests/test_commands.py::TestRedisCommands::test_command_docs",
"tests/test_commands.py::TestRedisCommands::test_command_list",
"tests/test_commands.py::TestRedisCommands::test_command_getkeys",
"tests/test_commands.py::TestRedisCommands::test_command",
"tests/test_commands.py::TestRedisCommands::test_command_getkeysandflags",
"tests/test_commands.py::TestRedisCommands::test_module",
"tests/test_commands.py::TestRedisCommands::test_module_loadex",
"tests/test_commands.py::TestRedisCommands::test_restore",
"tests/test_commands.py::TestRedisCommands::test_restore_idletime",
"tests/test_commands.py::TestRedisCommands::test_restore_frequency",
"tests/test_commands.py::TestRedisCommands::test_replicaof",
"tests/test_commands.py::TestRedisCommands::test_shutdown",
"tests/test_commands.py::TestRedisCommands::test_shutdown_with_params",
"tests/test_commands.py::TestRedisCommands::test_psync",
"tests/test_commands.py::TestRedisCommands::test_interrupted_command",
"tests/test_commands.py::TestBinarySave::test_binary_get_set",
"tests/test_commands.py::TestBinarySave::test_binary_lists",
"tests/test_commands.py::TestBinarySave::test_22_info",
"tests/test_commands.py::TestBinarySave::test_large_responses",
"tests/test_commands.py::TestBinarySave::test_floating_point_encoding"
] | [
"tests/test_commands.py::TestRedisCommands::test_acl_genpass"
] | Python | [] | [] |
|
redis/redis-py | 3,264 | redis__redis-py-3264 | [
"3262"
] | e71119de095bb01201665d57a1dc63230aa0606e | diff --git a/redis/_parsers/helpers.py b/redis/_parsers/helpers.py
--- a/redis/_parsers/helpers.py
+++ b/redis/_parsers/helpers.py
@@ -38,7 +38,7 @@ def parse_info(response):
response = str_if_bytes(response)
def get_value(value):
- if "," not in value or "=" not in value:
+ if "," not in value and "=" not in value:
try:
if "." in value:
return float(value)
| diff --git a/tests/test_parsers/test_helpers.py b/tests/test_parsers/test_helpers.py
new file mode 100644
--- /dev/null
+++ b/tests/test_parsers/test_helpers.py
@@ -0,0 +1,35 @@
+from redis._parsers.helpers import parse_info
+
+
+def test_parse_info():
+ info_output = """
+# Modules
+module:name=search,ver=999999,api=1,filters=0,usedby=[],using=[ReJSON],options=[handle-io-errors]
+
+# search_fields_statistics
+search_fields_text:Text=3
+search_fields_tag:Tag=2,Sortable=1
+
+# search_version
+search_version:99.99.99
+search_redis_version:7.2.2 - oss
+
+# search_runtime_configurations
+search_query_timeout_ms:500
+ """
+ info = parse_info(info_output)
+
+ assert isinstance(info["modules"], list)
+ assert isinstance(info["modules"][0], dict)
+ assert info["modules"][0]["name"] == "search"
+
+ assert isinstance(info["search_fields_text"], dict)
+ assert info["search_fields_text"]["Text"] == 3
+
+ assert isinstance(info["search_fields_tag"], dict)
+ assert info["search_fields_tag"]["Tag"] == 2
+ assert info["search_fields_tag"]["Sortable"] == 1
+
+ assert info["search_version"] == "99.99.99"
+ assert info["search_redis_version"] == "7.2.2 - oss"
+ assert info["search_query_timeout_ms"] == 500
| Discrepancy in `INFO` response parsing
**Version**: `5.0.0`
**Description**: The code for parsing the `INFO` response is creating a discrepancy in the new RediSearch index field types field, e.g., `search_fields_text`.
If there is more than one section to this field its corresponding value (e.g., `TEXT=1, SORTABLE=1`) is returned as a dictionary (as expected), while if there is only one value for it (e.g., `TEXT=1`) it is returned as a string!
This is bad since we later use this value assuming that it is a dictionary, and thus face failures in the case that it is not.
The wanted behavior: We always get a dictionary back, whether we have only one section for this value or more.
Example: We have one section for the `TEXT` field, and two for the `TAG` field, and this is what we get:

The source of the problem is in the `parse_info()` function, [here](https://github.com/redis/redis-py/blob/19b55c62389c890a96dd611e28aaaedba7506720/redis/_parsers/helpers.py#L35-L36).
Thanks!
| "2024-06-05T10:10:12Z" | 5.0 | [] | [
"tests/test_parsers/test_helpers.py::test_parse_info"
] | Python | [] | [] |
|
redis/redis-py | 3,265 | redis__redis-py-3265 | [
"3262"
] | 0d47d6527a10fb70f8fcfdf8df69ae3b11ec92ef | diff --git a/redis/_parsers/helpers.py b/redis/_parsers/helpers.py
--- a/redis/_parsers/helpers.py
+++ b/redis/_parsers/helpers.py
@@ -38,7 +38,7 @@ def parse_info(response):
response = str_if_bytes(response)
def get_value(value):
- if "," not in value or "=" not in value:
+ if "," not in value and "=" not in value:
try:
if "." in value:
return float(value)
| diff --git a/tests/test_parsers/test_helpers.py b/tests/test_parsers/test_helpers.py
new file mode 100644
--- /dev/null
+++ b/tests/test_parsers/test_helpers.py
@@ -0,0 +1,35 @@
+from redis._parsers.helpers import parse_info
+
+
+def test_parse_info():
+ info_output = """
+# Modules
+module:name=search,ver=999999,api=1,filters=0,usedby=[],using=[ReJSON],options=[handle-io-errors]
+
+# search_fields_statistics
+search_fields_text:Text=3
+search_fields_tag:Tag=2,Sortable=1
+
+# search_version
+search_version:99.99.99
+search_redis_version:7.2.2 - oss
+
+# search_runtime_configurations
+search_query_timeout_ms:500
+ """
+ info = parse_info(info_output)
+
+ assert isinstance(info["modules"], list)
+ assert isinstance(info["modules"][0], dict)
+ assert info["modules"][0]["name"] == "search"
+
+ assert isinstance(info["search_fields_text"], dict)
+ assert info["search_fields_text"]["Text"] == 3
+
+ assert isinstance(info["search_fields_tag"], dict)
+ assert info["search_fields_tag"]["Tag"] == 2
+ assert info["search_fields_tag"]["Sortable"] == 1
+
+ assert info["search_version"] == "99.99.99"
+ assert info["search_redis_version"] == "7.2.2 - oss"
+ assert info["search_query_timeout_ms"] == 500
| Discrepancy in `INFO` response parsing
**Version**: `5.0.0`
**Description**: The code for parsing the `INFO` response is creating a discrepancy in the new RediSearch index field types field, e.g., `search_fields_text`.
If there is more than one section to this field its corresponding value (e.g., `TEXT=1, SORTABLE=1`) is returned as a dictionary (as expected), while if there is only one value for it (e.g., `TEXT=1`) it is returned as a string!
This is bad since we later use this value assuming that it is a dictionary, and thus face failures in the case that it is not.
The wanted behavior: We always get a dictionary back, whether we have only one section for this value or more.
Example: We have one section for the `TEXT` field, and two for the `TAG` field, and this is what we get:

The source of the problem is in the `parse_info()` function, [here](https://github.com/redis/redis-py/blob/19b55c62389c890a96dd611e28aaaedba7506720/redis/_parsers/helpers.py#L35-L36).
Thanks!
| "2024-06-05T11:31:12Z" | 5.1 | [] | [
"tests/test_parsers/test_helpers.py::test_parse_info"
] | Python | [] | [] |
|
prettier/prettier | 10,714 | prettier__prettier-10714 | [
"10709"
] | f8fff81005881441ffd1f9982b2b525dea413ea9 | diff --git a/src/language-js/print/function-parameters.js b/src/language-js/print/function-parameters.js
--- a/src/language-js/print/function-parameters.js
+++ b/src/language-js/print/function-parameters.js
@@ -26,6 +26,8 @@ import { locEnd } from "../loc.js";
import { ArgExpansionBailout } from "../../common/errors.js";
import { printFunctionTypeParameters } from "./misc.js";
+/** @typedef {import("../../common/ast-path").default} AstPath */
+
function printFunctionParameters(
path,
print,
@@ -91,7 +93,7 @@ function printFunctionParameters(
// } b,
// ) ) => {
// })
- if (expandArg) {
+ if (expandArg && !isDecoratedFunction(path)) {
if (willBreak(typeParams) || willBreak(printed)) {
// Removing lines in this case leads to broken or ugly output
throw new ArgExpansionBailout();
@@ -226,6 +228,59 @@ function shouldGroupFunctionParameters(functionNode, returnTypeDoc) {
);
}
+/**
+ * The "decorated function" pattern.
+ * The arrow function should be kept hugged even if its signature breaks.
+ *
+ * ```
+ * const decoratedFn = decorator(param1, param2)((
+ * ...
+ * ) => {
+ * ...
+ * });
+ * ```
+ * @param {AstPath} path
+ */
+function isDecoratedFunction(path) {
+ return path.match(
+ (node) =>
+ node.type === "ArrowFunctionExpression" &&
+ node.body.type === "BlockStatement",
+ (node, name) => {
+ if (
+ node.type === "CallExpression" &&
+ name === "arguments" &&
+ node.arguments.length === 1 &&
+ node.callee.type === "CallExpression"
+ ) {
+ const decorator = node.callee.callee;
+ return (
+ decorator.type === "Identifier" ||
+ (decorator.type === "MemberExpression" &&
+ !decorator.computed &&
+ decorator.object.type === "Identifier" &&
+ decorator.property.type === "Identifier")
+ );
+ }
+ return false;
+ },
+ (node, name) =>
+ (node.type === "VariableDeclarator" && name === "init") ||
+ (node.type === "ExportDefaultDeclaration" && name === "declaration") ||
+ (node.type === "TSExportAssignment" && name === "expression") ||
+ (node.type === "AssignmentExpression" &&
+ name === "right" &&
+ node.left.type === "MemberExpression" &&
+ node.left.object.type === "Identifier" &&
+ node.left.object.name === "module" &&
+ node.left.property.type === "Identifier" &&
+ node.left.property.name === "exports"),
+ (node) =>
+ node.type !== "VariableDeclaration" ||
+ (node.kind === "const" && node.declarations.length === 1)
+ );
+}
+
export {
printFunctionParameters,
shouldHugFunctionParameters,
| diff --git a/tests/format/flow/last-argument-expansion/__snapshots__/jsfmt.spec.js.snap b/tests/format/flow/last-argument-expansion/__snapshots__/jsfmt.spec.js.snap
new file mode 100644
--- /dev/null
+++ b/tests/format/flow/last-argument-expansion/__snapshots__/jsfmt.spec.js.snap
@@ -0,0 +1,152 @@
+// Jest Snapshot v1, https://goo.gl/fbAQLP
+
+exports[`break.js format 1`] = `
+====================================options=====================================
+parsers: ["flow"]
+printWidth: 80
+ | printWidth
+=====================================input======================================
+export default class AddAssetHtmlPlugin {
+ apply(compiler: WebpackCompilerType) {
+ compiler.plugin('compilation', (compilation: WebpackCompilationType) => {
+ compilation.plugin('html-webpack-plugin-before-html', (callback: Callback<any>) => {
+ addAllAssetsToCompilation(this.assets, compilation, htmlPluginData, callback);
+ });
+ });
+ }
+}
+
+=====================================output=====================================
+export default class AddAssetHtmlPlugin {
+ apply(compiler: WebpackCompilerType) {
+ compiler.plugin("compilation", (compilation: WebpackCompilationType) => {
+ compilation.plugin(
+ "html-webpack-plugin-before-html",
+ (callback: Callback<any>) => {
+ addAllAssetsToCompilation(
+ this.assets,
+ compilation,
+ htmlPluginData,
+ callback,
+ );
+ },
+ );
+ });
+ }
+}
+
+================================================================================
+`;
+
+exports[`decorated-function.js format 1`] = `
+====================================options=====================================
+parsers: ["flow"]
+printWidth: 80
+ | printWidth
+=====================================input======================================
+const Counter = decorator("my-counter")(
+ (props: { initialCount?: number; label?: string }) => {
+ const p = useDefault(props, {
+ initialCount: 0,
+ label: "Counter",
+ });
+
+ const [s, set] = useState({ count: p.initialCount });
+ const onClick = () => set("count", (it) => it + 1);
+
+ return () => (
+ <button onclick={onClick}>
+ {p.label}: {s.count}
+ </button>
+ );
+ }
+);
+
+const Counter2 = decorators.decorator("my-counter")(
+ (props: { initialCount?: number; label?: string }) => {
+ return () => (
+ <button onclick={onClick}>
+ {p.label}: {s.count}
+ </button>
+ );
+ }
+);
+
+export default decorators.decorator("my-counter")(
+ (props: { initialCount?: number; label?: string }) => {
+ return foo;
+ }
+);
+
+=====================================output=====================================
+const Counter = decorator("my-counter")((props: {
+ initialCount?: number,
+ label?: string,
+}) => {
+ const p = useDefault(props, {
+ initialCount: 0,
+ label: "Counter",
+ });
+
+ const [s, set] = useState({ count: p.initialCount });
+ const onClick = () => set("count", (it) => it + 1);
+
+ return () => (
+ <button onclick={onClick}>
+ {p.label}: {s.count}
+ </button>
+ );
+});
+
+const Counter2 = decorators.decorator("my-counter")((props: {
+ initialCount?: number,
+ label?: string,
+}) => {
+ return () => (
+ <button onclick={onClick}>
+ {p.label}: {s.count}
+ </button>
+ );
+});
+
+export default decorators.decorator("my-counter")((props: {
+ initialCount?: number,
+ label?: string,
+}) => {
+ return foo;
+});
+
+================================================================================
+`;
+
+exports[`edge_case.js format 1`] = `
+====================================options=====================================
+parsers: ["flow"]
+printWidth: 80
+ | printWidth
+=====================================input======================================
+var listener = DOM.listen(
+ introCard,
+ 'click',
+ sigil,
+ (event: JavelinEvent): void =>
+ BanzaiLogger.log(
+ config,
+ {...logData, ...DataStore.get(event.getNode(sigil))},
+ ),
+);
+
+=====================================output=====================================
+var listener = DOM.listen(
+ introCard,
+ "click",
+ sigil,
+ (event: JavelinEvent): void =>
+ BanzaiLogger.log(config, {
+ ...logData,
+ ...DataStore.get(event.getNode(sigil)),
+ }),
+);
+
+================================================================================
+`;
diff --git a/tests/format/flow/last-argument-expansion/break.js b/tests/format/flow/last-argument-expansion/break.js
new file mode 100644
--- /dev/null
+++ b/tests/format/flow/last-argument-expansion/break.js
@@ -0,0 +1,9 @@
+export default class AddAssetHtmlPlugin {
+ apply(compiler: WebpackCompilerType) {
+ compiler.plugin('compilation', (compilation: WebpackCompilationType) => {
+ compilation.plugin('html-webpack-plugin-before-html', (callback: Callback<any>) => {
+ addAllAssetsToCompilation(this.assets, compilation, htmlPluginData, callback);
+ });
+ });
+ }
+}
diff --git a/tests/format/flow/last-argument-expansion/decorated-function.js b/tests/format/flow/last-argument-expansion/decorated-function.js
new file mode 100644
--- /dev/null
+++ b/tests/format/flow/last-argument-expansion/decorated-function.js
@@ -0,0 +1,33 @@
+const Counter = decorator("my-counter")(
+ (props: { initialCount?: number; label?: string }) => {
+ const p = useDefault(props, {
+ initialCount: 0,
+ label: "Counter",
+ });
+
+ const [s, set] = useState({ count: p.initialCount });
+ const onClick = () => set("count", (it) => it + 1);
+
+ return () => (
+ <button onclick={onClick}>
+ {p.label}: {s.count}
+ </button>
+ );
+ }
+);
+
+const Counter2 = decorators.decorator("my-counter")(
+ (props: { initialCount?: number; label?: string }) => {
+ return () => (
+ <button onclick={onClick}>
+ {p.label}: {s.count}
+ </button>
+ );
+ }
+);
+
+export default decorators.decorator("my-counter")(
+ (props: { initialCount?: number; label?: string }) => {
+ return foo;
+ }
+);
diff --git a/tests/format/flow/last-argument-expansion/edge_case.js b/tests/format/flow/last-argument-expansion/edge_case.js
new file mode 100644
--- /dev/null
+++ b/tests/format/flow/last-argument-expansion/edge_case.js
@@ -0,0 +1,10 @@
+var listener = DOM.listen(
+ introCard,
+ 'click',
+ sigil,
+ (event: JavelinEvent): void =>
+ BanzaiLogger.log(
+ config,
+ {...logData, ...DataStore.get(event.getNode(sigil))},
+ ),
+);
diff --git a/tests/format/flow/last-argument-expansion/jsfmt.spec.js b/tests/format/flow/last-argument-expansion/jsfmt.spec.js
new file mode 100644
--- /dev/null
+++ b/tests/format/flow/last-argument-expansion/jsfmt.spec.js
@@ -0,0 +1 @@
+run_spec(import.meta, ["flow"]);
diff --git a/tests/format/typescript/last-argument-expansion/__snapshots__/jsfmt.spec.js.snap b/tests/format/typescript/last-argument-expansion/__snapshots__/jsfmt.spec.js.snap
--- a/tests/format/typescript/last-argument-expansion/__snapshots__/jsfmt.spec.js.snap
+++ b/tests/format/typescript/last-argument-expansion/__snapshots__/jsfmt.spec.js.snap
@@ -2,7 +2,7 @@
exports[`break.ts format 1`] = `
====================================options=====================================
-parsers: ["typescript", "babel", "flow"]
+parsers: ["typescript"]
printWidth: 80
| printWidth
=====================================input======================================
@@ -38,9 +38,137 @@ export default class AddAssetHtmlPlugin {
================================================================================
`;
+exports[`decorated-function.ts format 1`] = `
+====================================options=====================================
+parsers: ["typescript"]
+printWidth: 80
+ | printWidth
+=====================================input======================================
+const Counter = decorator("my-counter")(
+ (props: { initialCount?: number; label?: string }) => {
+ const p = useDefault(props, {
+ initialCount: 0,
+ label: "Counter",
+ });
+
+ const [s, set] = useState({ count: p.initialCount });
+ const onClick = () => set("count", (it) => it + 1);
+
+ return () => (
+ <button onclick={onClick}>
+ {p.label}: {s.count}
+ </button>
+ );
+ }
+);
+
+const Counter2 = decorators.decorator("my-counter")(
+ (props: { initialCount?: number; label?: string }) => {
+ return () => (
+ <button onclick={onClick}>
+ {p.label}: {s.count}
+ </button>
+ );
+ }
+);
+
+export default decorators.decorator("my-counter")(
+ (props: { initialCount?: number; label?: string }) => {
+ return foo;
+ }
+);
+
+export = decorators.decorator("my-counter")(
+ (props: { initialCount?: number; label?: string }) => {
+ return foo;
+ }
+);
+
+module.exports = decorators.decorator("my-counter")(
+ (props: { initialCount?: number; label?: string }) => {
+ return foo;
+ }
+);
+
+const Counter =
+ decorator("foo")(
+ decorator("bar")(
+ (props: {
+ loremFoo1: Array<Promise<any>>,
+ ipsumBarr2: Promise<number>,
+ }) => {
+ return <div/>;
+ }));
+
+=====================================output=====================================
+const Counter = decorator("my-counter")((props: {
+ initialCount?: number;
+ label?: string;
+}) => {
+ const p = useDefault(props, {
+ initialCount: 0,
+ label: "Counter",
+ });
+
+ const [s, set] = useState({ count: p.initialCount });
+ const onClick = () => set("count", (it) => it + 1);
+
+ return () => (
+ <button onclick={onClick}>
+ {p.label}: {s.count}
+ </button>
+ );
+});
+
+const Counter2 = decorators.decorator("my-counter")((props: {
+ initialCount?: number;
+ label?: string;
+}) => {
+ return () => (
+ <button onclick={onClick}>
+ {p.label}: {s.count}
+ </button>
+ );
+});
+
+export default decorators.decorator("my-counter")((props: {
+ initialCount?: number;
+ label?: string;
+}) => {
+ return foo;
+});
+
+export = decorators.decorator("my-counter")((props: {
+ initialCount?: number;
+ label?: string;
+}) => {
+ return foo;
+});
+
+module.exports = decorators.decorator("my-counter")((props: {
+ initialCount?: number;
+ label?: string;
+}) => {
+ return foo;
+});
+
+const Counter = decorator("foo")(
+ decorator("bar")(
+ (props: {
+ loremFoo1: Array<Promise<any>>;
+ ipsumBarr2: Promise<number>;
+ }) => {
+ return <div />;
+ },
+ ),
+);
+
+================================================================================
+`;
+
exports[`edge_case.ts format 1`] = `
====================================options=====================================
-parsers: ["typescript", "babel", "flow"]
+parsers: ["typescript"]
printWidth: 80
| printWidth
=====================================input======================================
diff --git a/tests/format/typescript/last-argument-expansion/decorated-function.ts b/tests/format/typescript/last-argument-expansion/decorated-function.ts
new file mode 100644
--- /dev/null
+++ b/tests/format/typescript/last-argument-expansion/decorated-function.ts
@@ -0,0 +1,55 @@
+const Counter = decorator("my-counter")(
+ (props: { initialCount?: number; label?: string }) => {
+ const p = useDefault(props, {
+ initialCount: 0,
+ label: "Counter",
+ });
+
+ const [s, set] = useState({ count: p.initialCount });
+ const onClick = () => set("count", (it) => it + 1);
+
+ return () => (
+ <button onclick={onClick}>
+ {p.label}: {s.count}
+ </button>
+ );
+ }
+);
+
+const Counter2 = decorators.decorator("my-counter")(
+ (props: { initialCount?: number; label?: string }) => {
+ return () => (
+ <button onclick={onClick}>
+ {p.label}: {s.count}
+ </button>
+ );
+ }
+);
+
+export default decorators.decorator("my-counter")(
+ (props: { initialCount?: number; label?: string }) => {
+ return foo;
+ }
+);
+
+export = decorators.decorator("my-counter")(
+ (props: { initialCount?: number; label?: string }) => {
+ return foo;
+ }
+);
+
+module.exports = decorators.decorator("my-counter")(
+ (props: { initialCount?: number; label?: string }) => {
+ return foo;
+ }
+);
+
+const Counter =
+ decorator("foo")(
+ decorator("bar")(
+ (props: {
+ loremFoo1: Array<Promise<any>>,
+ ipsumBarr2: Promise<number>,
+ }) => {
+ return <div/>;
+ }));
diff --git a/tests/format/typescript/last-argument-expansion/jsfmt.spec.js b/tests/format/typescript/last-argument-expansion/jsfmt.spec.js
--- a/tests/format/typescript/last-argument-expansion/jsfmt.spec.js
+++ b/tests/format/typescript/last-argument-expansion/jsfmt.spec.js
@@ -1 +1 @@
-run_spec(import.meta, ["typescript", "babel", "flow"]);
+run_spec(import.meta, ["typescript"]);
| Suboptimal additional indentation for decorated functions
**Prettier 2.2.1**
[Playground link](https://prettier.io/playground/#N4Igxg9gdgLgprEAuEB6VACAvDjcAeAhgLYAOANnBgIzY70ONPMtMYA6U6GMAFlQDMIAJ2KEY8ACYYIAVxil5MgT14BLAM4yoVAO5ry5beQCeGchAgBrDAHNLkztzUrSwuBLVxhGAG4AmADog2k0MWQ04aQxCLTUYHndxLQFZKDAYNWgtPnEnTEJ3DCgSKI4QSTgBNR12EAxJFwFvBATcqBk+by1JWSoYCAwAI1lbDABiagAGABZ-fwwAWnyMSKpeCVINJHRbeN5ZIcDIYlQ3D0zvM-dPK80NPo1Uabn-Tk5IKA0EgGE5WG82AaVRqcAAFAByYgmRaQNLwYQQgA0GDBwE4GAwNXiakI5D+8KBUyRGPMhCGcCMWAwEIJAMRnAAvkgMHSEQAFYQQLYASmwAD4MOiOhhPt8MABtOGwFGRGBsgC6QIicAAyjBxODsZk8WyeaSxQloD9yGowDZqSqfnjyENCOawWC+VhBXK2WD4gKsQkANQ0HkoiUK-Ui0k3WTCDpg0mYgA8Iwk0G0YFN5qwwGNqasjP5McxQvI5MpzKF0pgjLzsdQCYGUFzIr5mKZIZW9DwRDIlAwC1Yvb7-Y4UBWi0FXRikkamSTNUqsHEWQ6NVUghEYgkZTkCnkK3zYXJGgg5HklLMUAgCV0hAB0n47ne6Wyv3+CKBJ1I0FakOhsOf3mRqOFTFtVxfFnyJEkRULCkqRpNk-yZFk4OETluQ0Z1BUA0VH0lMtZQ8RVlUidVNQ9KAcV1Z8Q0xQ1tBNM0LXCSJrUMO0HSdL03WfD0Ehdb0MD9agA0lYNSTDDwIyjSsayTaAU3o9NM3onM80xYAoOLFlgDLCsRTjat5FretMUbQdGRDEAkRAbkpy+ZBQEKLldHZQoEA0ZAQDxS8TDcyyhmEe0rA8VVSHtGpbGQGBhD6Sy4GICkJyiAAZK9bFkQhbDgAAxVdxEyKBwpQQh5AgCyQA2YhyAAdXUeANBCsA1VcnFfHiEx3LADQfJAGpImEGBOXSsRkAEPFIksgArDR8AAIX880gtKRLQWG0a4AmqbVTCygAEVZHPOAVvIMaQBC4RevcmATFIOANDAYQ1FIGBSrcGoYEqtRJD4ZAAA5iROrlIkq-zSHc85et8A7LIARz2+AUK2dzYkWHQoiiUr3BhtR3AG2whqQEajrWkBImINRDuOjQtrgXb9oiqKiY1IZ3s+3hkH8SzIsIAwwr+Yg8ZAM8dFKlUABV93JonfD6ABJKBZxgVU7oemAAEE5fVExKAlxlGSAA)
<!-- prettier-ignore -->
```sh
--parser typescript
--no-semi
--single-quote
--trailing-comma none
```
**Input:**
<!-- prettier-ignore -->
```tsx
// === example 1 ==========================================
// the formatted output of this one will only look good
// if prettier v2.2.1 is used as it treats functions that
// are named "define" different than others due to bug #10422 -
// see https://github.com/prettier/prettier/issues/10422
const Counter = define('my-counter', ({
initialCount = 0,
label = 'Counter'
}: CounterProps) => {
const [count, setCount] = useState(initialCount)
const onClick = useCallback(() => setCount(it => it + 1), [])
return (
<button onclick={onClick}>
{label}: {count}
</button>
)
})
// === example 2 ==========================================
// -> the addition indentation in the formatted output
// is absolutely not wanted here
const Counter = component('my-counter', ({
initialCount = 0,
label = 'Counter'
}: CounterProps) => {
const [count, setCount] = useState(initialCount)
const onClick = useCallback(() => setCount(it => it + 1), [])
return (
<button onclick={onClick}>
{label}: {count}
</button>
)
})
```
**Output:**
<!-- prettier-ignore -->
```tsx
// === example 1 ==========================================
// the formatted output of this one will only look good
// if prettier v2.2.1 is used as it treats functions that
// are named "define" different than others due to bug #10422 -
// see https://github.com/prettier/prettier/issues/10422
const Counter = define('my-counter', ({
initialCount = 0,
label = 'Counter'
}: CounterProps) => {
const [count, setCount] = useState(initialCount)
const onClick = useCallback(() => setCount((it) => it + 1), [])
return (
<button onclick={onClick}>
{label}: {count}
</button>
)
})
// === example 2 ==========================================
// -> the addition indentation in the formatted output
// is absolutely not wanted here
const Counter = component(
'my-counter',
({ initialCount = 0, label = 'Counter' }: CounterProps) => {
const [count, setCount] = useState(initialCount)
const onClick = useCallback(() => setCount((it) => it + 1), [])
return (
<button onclick={onClick}>
{label}: {count}
</button>
)
}
)
```
**Expected behavior:**
Would be perfect if the function indentation stays as is (no additional two space indentation) - the formatting of the argument list is not that important.
Please ignore that the examples above look a bit like React or Preact - it's not about those libraries, it's just about the pattern.
Very often I have the case that special functions have the purpose to transform other functions, often including a bit of meta information (like a string or whatever), like in the examples above, but then the formatting by prettier will be quite ugly.
You may say for the examples above just define first a named function and then call the `component` function afterwards with the function variable as argument ... but in general this may explicitly not wanted and be more complicated (for example if you have `const Counter = component('my-counter', counterOptions, func)` where the type of `func` depends heavily on the type of `counterOptions`). Of course with some aditional lines of code and some utility types this is doable, but will be syntactically ugly and less readable.
Frankly, I have no concrete suggestion what could be a good solution regarding what format should be applied in what concrete cases - but I hoped you may have some ideas.
[Edit] One possibility could be: If all arguments except for the last one are literals or variables and the last argument is a closure and the literals and variables match one line then format as suggested above otherwise format as usual.
| Closely related: https://github.com/prettier/prettier/issues/6921
I don't believe there is a solution. In situations like this, the developer is willing to sacrifice the readability of a call to get less indentation. How can an algorithm guess this intention?
For `it`, `describe`, and co this was solved by hardcoding well-known function names, but that obviously doesn't scale.
Moreover, I suspect many people from #6921 would say that the current formatting is good because this is the best formatting for function composition.
Let's keep the issue open for discussion, but don't really expect this to lead anywhere. | "2021-04-16T13:48:14Z" | 2.8 | [] | [
"tests/format/typescript/last-argument-expansion/jsfmt.spec.js",
"tests/format/flow/last-argument-expansion/jsfmt.spec.js"
] | JavaScript | [] | [
"https://prettier.io/playground/#N4Igxg9gdgLgprEAuEB6VACAvDjcAeAhgLYAOANnBgIzY70ONPMtMYA6U6GMAFlQDMIAJ2KEY8ACYYIAVxil5MgT14BLAM4yoVAO5ry5beQCeGchAgBrDAHNLkztzUrSwuBLVxhGAG4AmADog2k0MWQ04aQxCLTUYHndxLQFZKDAYNWgtPnEnTEJ3DCgSKI4QSTgBNR12EAxJFwFvBATcqBk+by1JWSoYCAwAI1lbDABiagAGABZ-fwwAWnyMSKpeCVINJHRbeN5ZIcDIYlQ3D0zvM-dPK80NPo1Uabn-Tk5IKA0EgGE5WG82AaVRqcAAFAByYgmRaQNLwYQQgA0GDBwE4GAwNXiakI5D+8KBUyRGPMhCGcCMWAwEIJAMRnAAvkgMHSEQAFYQQLYASmwAD4MOiOhhPt8MABtOGwFGRGBsgC6QIicAAyjBxODsZk8WyeaSxQloD9yGowDZqSqfnjyENCOawWC+VhBXK2WD4gKsQkANQ0HkoiUK-Ui0k3WTCDpg0mYgA8Iwk0G0YFN5qwwGNqasjP5McxQvI5MpzKF0pgjLzsdQCYGUFzIr5mKZIZW9DwRDIlAwC1Yvb7-Y4UBWi0FXRikkamSTNUqsHEWQ6NVUghEYgkZTkCnkK3zYXJGgg5HklLMUAgCV0hAB0n47ne6Wyv3+CKBJ1I0FakOhsOf3mRqOFTFtVxfFnyJEkRULCkqRpNk-yZFk4OETluQ0Z1BUA0VH0lMtZQ8RVlUidVNQ9KAcV1Z8Q0xQ1tBNM0LXCSJrUMO0HSdL03WfD0Ehdb0MD9agA0lYNSTDDwIyjSsayTaAU3o9NM3onM80xYAoOLFlgDLCsRTjat5FretMUbQdGRDEAkRAbkpy+ZBQEKLldHZQoEA0ZAQDxS8TDcyyhmEe0rA8VVSHtGpbGQGBhD6Sy4GICkJyiAAZK9bFkQhbDgAAxVdxEyKBwpQQh5AgCyQA2YhyAAdXUeANBCsA1VcnFfHiEx3LADQfJAGpImEGBOXSsRkAEPFIksgArDR8AAIX880gtKRLQWG0a4AmqbVTCygAEVZHPOAVvIMaQBC4RevcmATFIOANDAYQ1FIGBSrcGoYEqtRJD4ZAAA5iROrlIkq-zSHc85et8A7LIARz2+AUK2dzYkWHQoiiUr3BhtR3AG2whqQEajrWkBImINRDuOjQtrgXb9oiqKiY1IZ3s+3hkH8SzIsIAwwr+Yg8ZAM8dFKlUABV93JonfD6ABJKBZxgVU7oemAAEE5fVExKAlxlGSAA"
] |
prettier/prettier | 11,487 | prettier__prettier-11487 | [
"11482"
] | f63e15d1cd37167984dc329a34fc050343e3a91b | diff --git a/src/language-css/printer-postcss.js b/src/language-css/printer-postcss.js
--- a/src/language-css/printer-postcss.js
+++ b/src/language-css/printer-postcss.js
@@ -550,6 +550,19 @@ function genericPrint(path, options, print) {
continue;
}
+ // Ignore SCSS @forward wildcard suffix
+ if (
+ insideAtRuleNode(path, "forward") &&
+ iNode.type === "value-word" &&
+ iNode.value &&
+ iPrevNode.type === "value-word" &&
+ iPrevNode.value === "as" &&
+ iNextNode.type === "value-operator" &&
+ iNextNode.value === "*"
+ ) {
+ continue;
+ }
+
// Ignore after latest node (i.e. before semicolon)
if (!iNextNode) {
continue;
| diff --git a/tests/format/scss/quotes/__snapshots__/jsfmt.spec.js.snap b/tests/format/scss/quotes/__snapshots__/jsfmt.spec.js.snap
--- a/tests/format/scss/quotes/__snapshots__/jsfmt.spec.js.snap
+++ b/tests/format/scss/quotes/__snapshots__/jsfmt.spec.js.snap
@@ -29,6 +29,8 @@ singleQuote: true
@forward "library" as btn-*;
+@forward "library" as btn*;
+
=====================================output=====================================
@use 'library';
@@ -47,7 +49,9 @@ singleQuote: true
@forward 'library' hide gradient;
-@forward 'library' as btn- *;
+@forward 'library' as btn-*;
+
+@forward 'library' as btn*;
================================================================================
`;
@@ -80,6 +84,8 @@ printWidth: 80
@forward "library" as btn-*;
+@forward "library" as btn*;
+
=====================================output=====================================
@use "library";
@@ -98,7 +104,9 @@ printWidth: 80
@forward "library" hide gradient;
-@forward "library" as btn- *;
+@forward "library" as btn-*;
+
+@forward "library" as btn*;
================================================================================
`;
diff --git a/tests/format/scss/quotes/quotes.scss b/tests/format/scss/quotes/quotes.scss
--- a/tests/format/scss/quotes/quotes.scss
+++ b/tests/format/scss/quotes/quotes.scss
@@ -19,3 +19,5 @@
@forward "library" hide gradient;
@forward "library" as btn-*;
+
+@forward "library" as btn*;
| [scss] Wildcard syntax in `@forward` broken
Using the latest prettier wildcard syntax is broken when using `@forward` pattern.
It worked as desired in v2.3.2.
**Prettier 2.4.0**
[Playground link](https://prettier.io/playground/#N4Igxg9gdgLgprEAuEABAZhATgdwIZYAmABAOQB0A9AA54wAWpxeAzsbQwLQBUA3ADpQM2fETJVIAG2xNWxKdh4ChmXARIVKcSXAC2CGLLba9BpYJAAaEBGowAltBbJQBLBBwAFAgmco8kvgAns7WAEZYeGAA1nAwAMp4+gAy9lBwyOgBLHDhkTFx8bRgaQDmyDBYAK65IHphcISEjcl4UKVVeKVwAGLYunQO7cggeFUwEFYg9DC6kgDq9PbwLMVw8b7L9gBuy0EjYCyhIGk5WDCekaUDmdm1AFYsAB7xZToAilUQ8LeSOda0LBnEYsQ7HahYNIweb2QgMZAADgADAD3Dl5pFqCMIXAztsMtYAI5feCXWx+UYsTjpRqNKZYODE+wMy5dG5ILJ-Wo5XT2CrVblvOCfb4ZDl3awwPBhGFw+jIABMksi9kkZQAwhBdOy6iwAKxTKo5AAq0r8nP+IG2NQAklBmrB4mBIXYAIL2+IwII6X45AC+fqAA)
<!-- prettier-ignore -->
```sh
--parser scss
```
**Input:**
<!-- prettier-ignore -->
```scss
@forward './path' as path-*;
@forward './color' as color-*;
@forward './element' as element-*;
```
**Output:**
<!-- prettier-ignore -->
```scss
@forward "./path" as path- *;
@forward "./color" as color- *;
@forward "./element" as element- *;
```
**Expected behavior:**
Wildcard syntax should be preserved.
<!-- prettier-ignore -->
```scss
@forward "./path" as path-*;
@forward "./color" as color-*;
@forward "./element" as element-*;
```
| Regression, thanks
Due https://github.com/prettier/prettier/pull/11461
/cc @niksy | "2021-09-10T06:54:29Z" | 2.5 | [] | [
"tests/format/scss/quotes/jsfmt.spec.js"
] | JavaScript | [] | [
"https://prettier.io/playground/#N4Igxg9gdgLgprEAuEABAZhATgdwIZYAmABAOQB0A9AA54wAWpxeAzsbQwLQBUA3ADpQM2fETJVIAG2xNWxKdh4ChmXARIVKcSXAC2CGLLba9BpYJAAaEBGowAltBbJQBLBBwAFAgmco8kvgAns7WAEZYeGAA1nAwAMp4+gAy9lBwyOgBLHDhkTFx8bRgaQDmyDBYAK65IHphcISEjcl4UKVVeKVwAGLYunQO7cggeFUwEFYg9DC6kgDq9PbwLMVw8b7L9gBuy0EjYCyhIGk5WDCekaUDmdm1AFYsAB7xZToAilUQ8LeSOda0LBnEYsQ7HahYNIweb2QgMZAADgADAD3Dl5pFqCMIXAztsMtYAI5feCXWx+UYsTjpRqNKZYODE+wMy5dG5ILJ-Wo5XT2CrVblvOCfb4ZDl3awwPBhGFw+jIABMksi9kkZQAwhBdOy6iwAKxTKo5AAq0r8nP+IG2NQAklBmrB4mBIXYAIL2+IwII6X45AC+fqAA"
] |
prettier/prettier | 11,515 | prettier__prettier-11515 | [
"10848"
] | 9e1038c0eb080da5b049a8689f0b9c08830e8fe3 | diff --git a/src/document/doc-utils.js b/src/document/doc-utils.js
--- a/src/document/doc-utils.js
+++ b/src/document/doc-utils.js
@@ -401,6 +401,16 @@ function replaceTextEndOfLine(text, replacement = literalline) {
return join(replacement, text.split("\n")).parts;
}
+function canBreakFn(doc) {
+ if (doc.type === "line") {
+ return true;
+ }
+}
+
+function canBreak(doc) {
+ return findInDoc(doc, canBreakFn, false);
+}
+
module.exports = {
isConcat,
getDocParts,
@@ -416,4 +426,5 @@ module.exports = {
cleanDoc,
replaceTextEndOfLine,
replaceEndOfLine,
+ canBreak,
};
diff --git a/src/language-js/print/assignment.js b/src/language-js/print/assignment.js
--- a/src/language-js/print/assignment.js
+++ b/src/language-js/print/assignment.js
@@ -3,7 +3,7 @@
const { isNonEmptyArray, getStringWidth } = require("../../common/util.js");
const {
builders: { line, group, indent, indentIfBreak },
- utils: { cleanDoc, willBreak },
+ utils: { cleanDoc, willBreak, canBreak },
} = require("../../document/index.js");
const {
hasLeadingOwnLineComment,
@@ -140,7 +140,8 @@ function chooseLayout(path, options, print, leftDoc, rightPropertyName) {
if (
isComplexDestructuring(node) ||
isComplexTypeAliasParams(node) ||
- hasComplexTypeAnnotation(node)
+ hasComplexTypeAnnotation(node) ||
+ (isArrowFunctionVariableDeclarator(node) && canBreak(leftDoc))
) {
return "break-lhs";
}
@@ -295,6 +296,14 @@ function hasComplexTypeAnnotation(node) {
);
}
+function isArrowFunctionVariableDeclarator(node) {
+ return (
+ node.type === "VariableDeclarator" &&
+ node.init &&
+ node.init.type === "ArrowFunctionExpression"
+ );
+}
+
function getTypeParametersFromTypeReference(node) {
if (
isTypeReference(node) &&
@@ -457,4 +466,5 @@ module.exports = {
printVariableDeclarator,
printAssignmentExpression,
printAssignment,
+ isArrowFunctionVariableDeclarator,
};
diff --git a/src/language-js/print/type-parameters.js b/src/language-js/print/type-parameters.js
--- a/src/language-js/print/type-parameters.js
+++ b/src/language-js/print/type-parameters.js
@@ -11,9 +11,11 @@ const {
isTSXFile,
shouldPrintComma,
getFunctionParameters,
+ isObjectType,
} = require("../utils.js");
const { createGroupIdMapper } = require("../../common/util.js");
const { shouldHugType } = require("./type-annotation.js");
+const { isArrowFunctionVariableDeclarator } = require("./assignment.js");
const getTypeParametersGroupId = createGroupIdMapper("typeParameters");
@@ -32,12 +34,22 @@ function printTypeParameters(path, options, print, paramsKey) {
const grandparent = path.getNode(2);
const isParameterInTestCall = grandparent && isTestCall(grandparent);
+ const isArrowFunctionVariable = path.match(
+ (node) =>
+ !(node[paramsKey].length === 1 && isObjectType(node[paramsKey][0])),
+ undefined,
+ (node, name) => name === "typeAnnotation",
+ (node) => node.type === "Identifier",
+ isArrowFunctionVariableDeclarator
+ );
+
const shouldInline =
- isParameterInTestCall ||
- node[paramsKey].length === 0 ||
- (node[paramsKey].length === 1 &&
- (shouldHugType(node[paramsKey][0]) ||
- node[paramsKey][0].type === "NullableTypeAnnotation"));
+ !isArrowFunctionVariable &&
+ (isParameterInTestCall ||
+ node[paramsKey].length === 0 ||
+ (node[paramsKey].length === 1 &&
+ (node[paramsKey][0].type === "NullableTypeAnnotation" ||
+ shouldHugType(node[paramsKey][0]))));
if (shouldInline) {
return [
| diff --git a/tests/format/typescript/assignment/__snapshots__/jsfmt.spec.js.snap b/tests/format/typescript/assignment/__snapshots__/jsfmt.spec.js.snap
--- a/tests/format/typescript/assignment/__snapshots__/jsfmt.spec.js.snap
+++ b/tests/format/typescript/assignment/__snapshots__/jsfmt.spec.js.snap
@@ -263,6 +263,150 @@ const foo = call<
================================================================================
`;
+exports[`issue-10848.tsx format 1`] = `
+====================================options=====================================
+parsers: ["typescript"]
+printWidth: 80
+ | printWidth
+=====================================input======================================
+const MyComponent: React.VoidFunctionComponent<MyComponentProps> = ({ x }) => {
+ const a = useA()
+ return <div>x = {x}; a = {a}</div>
+}
+
+const MyComponent2: React.VoidFunctionComponent<MyComponent2Props> = ({ x, y }) => {
+ const a = useA()
+ return <div>x = {x}; y = {y}; a = {a}</div>
+}
+
+const MyComponentWithLongName1: React.VoidFunctionComponent<MyComponentWithLongNameProps> = ({ x, y }) => {
+ const a = useA()
+ return <div>x = {x}; y = {y}; a = {a}</div>
+}
+
+const MyComponentWithLongName2: React.VoidFunctionComponent<MyComponentWithLongNameProps> = ({ x, y, anotherPropWithLongName1, anotherPropWithLongName2, anotherPropWithLongName3, anotherPropWithLongName4 }) => {
+ const a = useA()
+ return <div>x = {x}; y = {y}; a = {a}</div>
+}
+
+const MyGenericComponent: React.VoidFunctionComponent<MyGenericComponentProps<number>> = ({ x, y }) => {
+ const a = useA()
+ return <div>x = {x}; y = {y}; a = {a}</div>
+}
+
+export const ExportToExcalidrawPlus: React.FC<{
+ elements: readonly NonDeletedExcalidrawElement[];
+ appState: AppState;
+ onError: (error: Error) => void;
+}> = ({ elements, appState, onError }) => {
+ return null;
+}
+
+const Query: FunctionComponent<QueryProps> = ({
+ children,
+ type,
+ resource,
+ payload,
+ // Provides an undefined onSuccess just so the key \`onSuccess\` is defined
+ // This is used to detect options in useDataProvider
+ options = { onSuccess: undefined },
+}) =>
+ children(
+ useQuery(
+ { type, resource, payload },
+ { ...options, withDeclarativeSideEffectsSupport: true }
+ )
+ );
+
+=====================================output=====================================
+const MyComponent: React.VoidFunctionComponent<MyComponentProps> = ({ x }) => {
+ const a = useA();
+ return (
+ <div>
+ x = {x}; a = {a}
+ </div>
+ );
+};
+
+const MyComponent2: React.VoidFunctionComponent<MyComponent2Props> = ({
+ x,
+ y,
+}) => {
+ const a = useA();
+ return (
+ <div>
+ x = {x}; y = {y}; a = {a}
+ </div>
+ );
+};
+
+const MyComponentWithLongName1: React.VoidFunctionComponent<
+ MyComponentWithLongNameProps
+> = ({ x, y }) => {
+ const a = useA();
+ return (
+ <div>
+ x = {x}; y = {y}; a = {a}
+ </div>
+ );
+};
+
+const MyComponentWithLongName2: React.VoidFunctionComponent<
+ MyComponentWithLongNameProps
+> = ({
+ x,
+ y,
+ anotherPropWithLongName1,
+ anotherPropWithLongName2,
+ anotherPropWithLongName3,
+ anotherPropWithLongName4,
+}) => {
+ const a = useA();
+ return (
+ <div>
+ x = {x}; y = {y}; a = {a}
+ </div>
+ );
+};
+
+const MyGenericComponent: React.VoidFunctionComponent<
+ MyGenericComponentProps<number>
+> = ({ x, y }) => {
+ const a = useA();
+ return (
+ <div>
+ x = {x}; y = {y}; a = {a}
+ </div>
+ );
+};
+
+export const ExportToExcalidrawPlus: React.FC<{
+ elements: readonly NonDeletedExcalidrawElement[];
+ appState: AppState;
+ onError: (error: Error) => void;
+}> = ({ elements, appState, onError }) => {
+ return null;
+};
+
+const Query: FunctionComponent<QueryProps> = ({
+ children,
+ type,
+ resource,
+ payload,
+ // Provides an undefined onSuccess just so the key \`onSuccess\` is defined
+ // This is used to detect options in useDataProvider
+ options = { onSuccess: undefined },
+}) =>
+ children(
+ useQuery(
+ { type, resource, payload },
+ { ...options, withDeclarativeSideEffectsSupport: true }
+ )
+ );
+
+================================================================================
+`;
+
exports[`issue-10850.ts format 1`] = `
====================================options=====================================
parsers: ["typescript"]
diff --git a/tests/format/typescript/assignment/issue-10848.tsx b/tests/format/typescript/assignment/issue-10848.tsx
new file mode 100644
--- /dev/null
+++ b/tests/format/typescript/assignment/issue-10848.tsx
@@ -0,0 +1,48 @@
+const MyComponent: React.VoidFunctionComponent<MyComponentProps> = ({ x }) => {
+ const a = useA()
+ return <div>x = {x}; a = {a}</div>
+}
+
+const MyComponent2: React.VoidFunctionComponent<MyComponent2Props> = ({ x, y }) => {
+ const a = useA()
+ return <div>x = {x}; y = {y}; a = {a}</div>
+}
+
+const MyComponentWithLongName1: React.VoidFunctionComponent<MyComponentWithLongNameProps> = ({ x, y }) => {
+ const a = useA()
+ return <div>x = {x}; y = {y}; a = {a}</div>
+}
+
+const MyComponentWithLongName2: React.VoidFunctionComponent<MyComponentWithLongNameProps> = ({ x, y, anotherPropWithLongName1, anotherPropWithLongName2, anotherPropWithLongName3, anotherPropWithLongName4 }) => {
+ const a = useA()
+ return <div>x = {x}; y = {y}; a = {a}</div>
+}
+
+const MyGenericComponent: React.VoidFunctionComponent<MyGenericComponentProps<number>> = ({ x, y }) => {
+ const a = useA()
+ return <div>x = {x}; y = {y}; a = {a}</div>
+}
+
+export const ExportToExcalidrawPlus: React.FC<{
+ elements: readonly NonDeletedExcalidrawElement[];
+ appState: AppState;
+ onError: (error: Error) => void;
+}> = ({ elements, appState, onError }) => {
+ return null;
+}
+
+const Query: FunctionComponent<QueryProps> = ({
+ children,
+ type,
+ resource,
+ payload,
+ // Provides an undefined onSuccess just so the key `onSuccess` is defined
+ // This is used to detect options in useDataProvider
+ options = { onSuccess: undefined },
+}) =>
+ children(
+ useQuery(
+ { type, resource, payload },
+ { ...options, withDeclarativeSideEffectsSupport: true }
+ )
+ );
| The offset for React component body depends on component name length in v2.3
**Prettier 2.3.0**
[Playground link](https://prettier.io/playground/#N4Igxg9gdgLgprEAuEBLWcBOAzAhmOAAgFkBPAYQgFsAHaBGABUwhoGdDgAdKQwgDySEoAVyoAjLDwC+PHpChsYJCtTpQGQgEpx8MAHQA1CKgAmAMRFQwMVNEq16sADxkH6hs1ZsAfIQC8hAAUwAKE0gCUAX7cvIQKSoS4AYQibHAAgkERPHyYcDAimLzOpqgAbj78KcD80gDcSTW40s4A9GWVMnJQ6PA4+ERuak4wAExe7Jy5AkKiElJxpHNikpjdUPLQicOOGrBj2ro2RiYWVjZ2UO6jrqp7DBMs7H6BIQIANISk4VH+MTMEspkoE0plsjN8oVioRShUqjU6o0foFgKQGk1US12p0fBseH0sHgCCobvsYAB1VAwAAWABloABzAByuCocEmHFifEEwlWiz4yz5C3WUFkm2s22Uuw8sCptIZUBZbLgRz0pzMlmstnsI3JdzJDHl9KZrPZnNewVC-C+P0i0WmcSBmNS6SyOTiUKKJVx1VRSO+NXRjRBnGxHXhMhAHxArB1imQoFwmBYAHdGMmEGxkCBcAAbVO4UjZmPiTD4ADWBQAyjR8OhGcgYJgRHAY3AFqZTHBTHTcEqRLhGXBzBBMFRcDBbEqc7gRDAINGQDSYFQ8xSadS4Gw6wRq1nqRVqaQc2A2CW0IosExy4yJ8g8Hn0jGAFZsfgAIXLYCrMGrKrpdA4AffNnxAN9+GrBs8zgABFEQIHgECnzbEA60wdJMBzGBSBobcwEwVAaBgJcaEIuUzFpZAAA4AAYYzIiB0gpcsaBzMjtywcpgJjABHBD4E5Wc2AAWg0HseyXfJ+NQfJmCHe8kEfMD0ioVAmxbVC2GguCBOApTQNQmBcHEKlTCopAxhjZtcFQPMGwcRSQG3ABWJcwQAFRM7MDJQmNylbABJKBu1gasCKImAMhC6scJg5D0mkaQgA)
<!-- prettier-ignore -->
```sh
--parser typescript
```
After switching from Prettier 2.2.1 to 2.3.0 in a large React + TS codebase, I've noticed an unexpectedly large diff. The offset for many React components has shifted.
It turns out that the length of the component name now affects the location of `({`. If these opening brackets are moved to the new line, the whole component body is shifted. This makes git diffs quite fragile.
Imagine renaming some component during a refactor. In Prettier 2.2.1, the diff would be just one line but in Prettier 2.3.0, `git blame` for the whole file would end up broken.
**Input:**
<!-- prettier-ignore -->
```tsx
interface MyComponentProps {
x: number
}
const MyComponent: React.VoidFunctionComponent<MyComponentProps> = ({ x }) => {
const a = useA()
return <div>x = {x}; a = {a}</div>
}
interface MyComponent2Props {
x: number
y: number
}
const MyComponent2: React.VoidFunctionComponent<MyComponent2Props> = ({ x, y }) => {
const a = useA()
return <div>x = {x}; y = {y}; a = {a}</div>
}
interface MyComponentWithLongNameProps {
x: number
y: number
}
const MyComponentWithLongName: React.VoidFunctionComponent<MyComponentWithLongNameProps> = ({ x, y }) => {
const a = useA()
return <div>x = {x}; y = {y}; a = {a}</div>
}
```
**Output:**
<!-- prettier-ignore -->
```tsx
interface MyComponentProps {
x: number;
}
const MyComponent: React.VoidFunctionComponent<MyComponentProps> = ({ x }) => {
const a = useA();
return (
<div>
x = {x}; a = {a}
</div>
);
};
interface MyComponent2Props {
x: number;
y: number;
}
const MyComponent2: React.VoidFunctionComponent<MyComponent2Props> = ({
x,
y,
}) => {
const a = useA();
return (
<div>
x = {x}; y = {y}; a = {a}
</div>
);
};
interface MyComponentWithLongNameProps {
x: number;
y: number;
}
const MyComponentWithLongName: React.VoidFunctionComponent<MyComponentWithLongNameProps> =
({ x, y }) => {
const a = useA();
return (
<div>
x = {x}; y = {y}; a = {a}
</div>
);
};
```
**Expected behavior:**
All three `useA()` and `return` statements have only two leading spaces, i.e. the offset does not depend on the component name length.
Our team will be staying on Prettier 2.2.1 in the meantime. Happy to help with a PR if we come up with some solution!
| Just checked if the issue is present in pure JavaScript and could reproduce it too: [Playgound](https://prettier.io/playground/#N4Igxg9gdgLgprEAuc0DOMAEBZAngYQgFsAHaBLAXkwAphMAPTAXwEpNKA+TYAHSkyZIUDJgCGHTAFc0cAII1W-QQCc4MKSoEAeACYBLAG6cm1YA2YBucZOBjm2gPQHj-Zv37DReQqXKwAdX0YAAsANTgVXAAZaABzADkxInk5NPSMzKzsnMzJOkYAGkxcFnYuHmUhdCwJahl5RSq1DS1MPSMTWwtrUrNcKxszeycXTjcPKC8sH2IyKAog0Iio2KhE5NTc7Z2d-PoGYtK2Dm4+AWqRWskGhSULls0dMdMeHpLbAes6nhHnTrcIEKIAgJBg+nQyFAYhUKggAHcAAowhBoZAgMQAG3hYlwaOBACMVGIwABrdQAZRIJP062QMBUUjgwLgRAJcF0ug50TE6ykYjicAAYhAVEQxDBwXSUGIpDAIECQCEYERMQEQsE4GhqWA4BTUcEjMFcOiwGh8SBabIVDBEcS4uLkAAzLGyYEAKzQDAAQsSyZTNtFaXBna7mSBPQwKbS4pi4ABFKQQeChzFukDUlTW9EEsTszGKkgqWkwIK6ULIAAcAAZgUWILIAsSSOii1rIoYQ8CAI5J+B20FomVoAC0Cw5HMVal7+jUdoFjqQLrT4dkRH09MZq5jccTyZDS7DwJgebLFaQACZj8T9JiY75FyAtQBWRUNAAqeaHy-ThiZAEkoC5WAKTAYswTkICKRgXA41TWRmGYIA).
Of course, the name of the component should be quite long to shift the fn body 😅 The TS example is much closer to the real world scenario.
Is `git blame` the main concern here? It has the `-w` mode, in which it ignores whitespace changes. Also it has the [`--ignore-revs-file` feature](https://git-scm.com/docs/git-blame#Documentation/git-blame.txt---ignore-revs-fileltfilegt). Don't these Git features solve this problem completely?
Said that, I agree that it's better to minimize indentation when possible. For type-annotated variables, the following formatting seems to be an interesting idea:
```ts
const MyComponentWithLongName: (
React.VoidFunctonComponent<MyComponentWithLongNameProps>
) = ({ x, y }) => {
const a = useA();
return (
<div>
x = {x}; y = {y}; a = {a}
</div>
);
};
```
But for variables without type annotations, I'm afraid the current (2.3) formatting is going to stay since it's difficult to think of an alternative.
Git blame is one of the two concerns I have. Just checked what [VSCode git lens](https://marketplace.visualstudio.com/items?itemName=eamodio.gitlens) shows for the updated lines and saw the commit with an offset shift rather than the original one. This makes code introspection more challenging than it used to. Even if it’s technically possible to look ‘through’ the whitespace-only diffs, it's not a default setting, so requires an extra action from the whole team.
Another concern is the ‘broken rhythm’. I’d say that it’s even bigger than `git blame`.
Human brain unconsciously gets used to the standard offsets in the React files, which helps certain cognitive tasks. Determining if a given line is inside a hook or in the plain 'render phase' is a typical example. If there's no ‘rule of thumb‘ for the whole component body offset, this mini-task requires an extra split second hundreds of times a day.
> `gitlens.blame.ignoreWhitespace` Specifies whether to ignore whitespace when comparing revisions during blame operations
-- https://marketplace.visualstudio.com/items?itemName=eamodio.gitlens#gutter-blame-settings-
If we're talking specifically about React, I might be missing something. Why are these type annotations needed? In my React projects, I annotate only the props parameter and let TS infer the type of the variable. (Also I use function declarations instead of `const`s because I think hoisting is convenient.) Anyway, I guess you have your reasons for doing it that way, so if you feel like implementing https://github.com/prettier/prettier/issues/10848#issuecomment-836828099, please go ahead. I think it'd have good chances of getting merged.
Thanks @thorn0 – I might give that PR a shot soon.
I think I understand what you mean by using `function` keyword and by typing props only. In that case we get something like:
```tsx
interface MyComponentWithLongNameProps {
x: number;
y: number;
}
function MyComponentWithLongName({ x, y }: MyComponentWithLongNameProps) {
const a = useA();
return (
<div>
x = {x}; y = {y}; a = {a}
</div>
);
}
```
This is a rather wide-spread approach, but we don’t use it for these two reasons:
- Unlike `function myVar`, `const myVar = ...` is universal. By using [`"func-style": "error"` in ESLint](https://eslint.org/docs/rules/func-style), we enforce the expression fn style and this saves the team from a number of pitfalls and avoidable discussions. The difference between the two styles includes pretty nasty traps for newcomers, so it's best to not have to pick between one style and the other.
Not having hoisting can be seen as a good thing. It helps developers stick with a coherent ‘rhythm’ and thus write code that can be read linearly.
- [`React.VoidFunctonComponent`](https://github.com/DefinitelyTyped/DefinitelyTyped/pull/46643) checks the return type, not just the args. So it's harder to return `undefined` or `void` by accident. When using `function` + `props: MyProps`, the return type will be also checked, but only when the component is mentioned in some Jsx. This works too, but makes things a bit more confusing for the devs.
If `undefined` is returned in a component library, the typing issue might be only spotted after the release.
`React.VoidFunctonComponent` is also ‘symmetric’ with `React.ForwardRefRenderFunction`, which is an extra bonus.
Overall, with `const` + `React.VoidFunctonComponent`, there are fewer places that need to look bespoke. Thus, the ‘list of rules’ to follow is shorter. Human brains like that 🙂
@thorn0 WDYT of
```ts
const MyComponentWithLongName: React.VoidFunctonComponent<MyComponentWithLongNameProps> = ({
x,
y,
}) => {
```
instead of
```ts
const MyComponentWithLongName: (
React.VoidFunctonComponent<MyComponentWithLongNameProps>
) = ({ x, y }) => {
```
?
So my third example would work as the second one. The benefit I see here is that `const MyComponent = React.VoidFunctonComponent<MyComponentProps>` will always be on one line. This will make it easier for the brain to pattern-match. Sticking with one line despite its length is what Prettier 2.2.1 does already.
This line is too long. The fact that Prettier doesn't break it is kind of a bug. Also Prettier's general strategy with regard to arrow functions is "prefer to keep the signature on one line if possible".
Sorry for the lack of updates @thorn0. I’m still planning to look into [your suggestion](https://github.com/prettier/prettier/issues/10848#issuecomment-836828099) – just haven’t found time yet. When 2.3.1 shows up on the horizon, please let me know and I’ll prioritise the work!
If anyone else wants to submit a PR, that’ll be amazing!
Now that TS 4.3 got released, we need to release support for it, so Prettier 2.3.1 is near.
Thanks for the headsup @thorn0! I'm planning to look into the fix now. If you have any hints / technical suggestions, it'd be great if you could share them!
First of all, you'll need to detect this situation in the code that prints assignment-like constructions (`print/assignment.js`) and switch the _layout_ to `break-lhs`. Then modify the code that prints type annotations in variable declarations. | "2021-09-13T01:18:16Z" | 2.5 | [] | [
"tests/format/typescript/assignment/jsfmt.spec.js"
] | JavaScript | [] | [
"https://prettier.io/playground/#N4Igxg9gdgLgprEAuEBLWcBOAzAhmOAAgFkBPAYQgFsAHaBGABUwhoGdDgAdKQwgDySEoAVyoAjLDwC+PHpChsYJCtTpQGQgEpx8MAHQA1CKgAmAMRFQwMVNEq16sADxkH6hs1ZsAfIQC8hAAUwAKE0gCUAX7cvIQKSoS4AYQibHAAgkERPHyYcDAimLzOpqgAbj78KcD80gDcSTW40s4A9GWVMnJQ6PA4+ERuak4wAExe7Jy5AkKiElJxpHNikpjdUPLQicOOGrBj2ro2RiYWVjZ2UO6jrqp7DBMs7H6BIQIANISk4VH+MTMEspkoE0plsjN8oVioRShUqjU6o0foFgKQGk1US12p0fBseH0sHgCCobvsYAB1VAwAAWABloABzAByuCocEmHFifEEwlWiz4yz5C3WUFkm2s22Uuw8sCptIZUBZbLgRz0pzMlmstnsI3JdzJDHl9KZrPZnNewVC-C+P0i0WmcSBmNS6SyOTiUKKJVx1VRSO+NXRjRBnGxHXhMhAHxArB1imQoFwmBYAHdGMmEGxkCBcAAbVO4UjZmPiTD4ADWBQAyjR8OhGcgYJgRHAY3AFqZTHBTHTcEqRLhGXBzBBMFRcDBbEqc7gRDAINGQDSYFQ8xSadS4Gw6wRq1nqRVqaQc2A2CW0IosExy4yJ8g8Hn0jGAFZsfgAIXLYCrMGrKrpdA4AffNnxAN9+GrBs8zgABFEQIHgECnzbEA60wdJMBzGBSBobcwEwVAaBgJcaEIuUzFpZAAA4AAYYzIiB0gpcsaBzMjtywcpgJjABHBD4E5Wc2AAWg0HseyXfJ+NQfJmCHe8kEfMD0ioVAmxbVC2GguCBOApTQNQmBcHEKlTCopAxhjZtcFQPMGwcRSQG3ABWJcwQAFRM7MDJQmNylbABJKBu1gasCKImAMhC6scJg5D0mkaQgA"
] |
prettier/prettier | 11,593 | prettier__prettier-11593 | [
"10955"
] | 20df210ddae98bf8a7631227d1fc0c8224f27f97 | diff --git a/src/language-js/print/function.js b/src/language-js/print/function.js
--- a/src/language-js/print/function.js
+++ b/src/language-js/print/function.js
@@ -252,13 +252,20 @@ function printArrowChain(
const isAssignmentRhs = Boolean(args && args.assignmentLayout);
const shouldPutBodyOnSeparateLine =
tailNode.body.type !== "BlockStatement" &&
- tailNode.body.type !== "ObjectExpression";
+ tailNode.body.type !== "ObjectExpression" &&
+ tailNode.body.type !== "SequenceExpression";
const shouldBreakBeforeChain =
(isCallee && shouldPutBodyOnSeparateLine) ||
(args && args.assignmentLayout === "chain-tail-arrow-chain");
const groupId = Symbol("arrow-chain");
+ // We handle sequence expressions as the body of arrows specially,
+ // so that the required parentheses end up on their own lines.
+ if (tailNode.body.type === "SequenceExpression") {
+ bodyDoc = group(["(", indent([softline, bodyDoc]), softline, ")"]);
+ }
+
return group([
group(
indent([
| diff --git a/tests/format/js/arrows/__snapshots__/jsfmt.spec.js.snap b/tests/format/js/arrows/__snapshots__/jsfmt.spec.js.snap
--- a/tests/format/js/arrows/__snapshots__/jsfmt.spec.js.snap
+++ b/tests/format/js/arrows/__snapshots__/jsfmt.spec.js.snap
@@ -3691,6 +3691,9 @@ foo(c, a => b)
foo(c, a => b, d)
foo(a => b, d)
+foo(a => (0, 1));
+foo(a => b => (0, 1));
+
=====================================output=====================================
promise.then(
(result) => result,
@@ -3716,6 +3719,9 @@ foo(c, (a) => b);
foo(c, (a) => b, d);
foo((a) => b, d);
+foo((a) => (0, 1));
+foo((a) => (b) => (0, 1));
+
================================================================================
`;
@@ -3742,6 +3748,9 @@ foo(c, a => b)
foo(c, a => b, d)
foo(a => b, d)
+foo(a => (0, 1));
+foo(a => b => (0, 1));
+
=====================================output=====================================
promise.then(
result => result,
@@ -3767,5 +3776,8 @@ foo(c, a => b);
foo(c, a => b, d);
foo(a => b, d);
+foo(a => (0, 1));
+foo(a => b => (0, 1));
+
================================================================================
`;
diff --git a/tests/format/js/arrows/parens.js b/tests/format/js/arrows/parens.js
--- a/tests/format/js/arrows/parens.js
+++ b/tests/format/js/arrows/parens.js
@@ -13,3 +13,6 @@ foo(a => { return b })
foo(c, a => b)
foo(c, a => b, d)
foo(a => b, d)
+
+foo(a => (0, 1));
+foo(a => b => (0, 1));
| Using compound expression with comma operator as return value of curried function
**Prettier 2.3.0**
[Playground link](https://prettier.io/playground/#N4Igxg9gdgLgprEAuc0DOMAEAzArlMTAXkwAoBDAJwHMBGAGkyuoCYBKYgPjI6O4poMmNdgB0oIeiAgAHGAEt0yUFUoQA7gAUqCNMhDkANuvIBPPVIBGlcmADWcGAGUZt+VGrIYlXHClwAW0s4ABMQ0IAZcg9ccmo4ADEISgDyGAUPfXJcGAhJEAALGADDAHUC+Xg0VzA4J11K+QA3StN9MDQLEHc0OEoYTRtqVORsI16pACs0AA8AIRt7RydyALgI9zhR8b8QaZmnd2pDOABFXAh4bcMJkFdKXsp9S3Jgw3yZSncYUvkQmAKyAAHAAGKSfCC9Uo2GT6T5wR5NLZSACOF3gg1kehQ5DQAFooHBQqF8pQ4Gj5GTBnERkgxjddr0AvIvD5GUcTudLls6TspDBXr9-oCkCx+TZ5IYjgBhCABWkgBEAVnyuF6ABVXtj6bcmr4AJJQcKwJxgL5yACCRqcMFMJ2uvQAvo6gA)
<!-- prettier-ignore -->
```sh
--parser babel
```
**Input:**
<!-- prettier-ignore -->
```js
const func = (arg1, arg2) => () => (arg1, arg2)
```
**Output:**
<!-- prettier-ignore -->
```js
const func = (arg1, arg2) => () => arg1, arg2;
```
**Expected behavior:**
Using compound expression with comma operator is not usual, but is valid syntax (and can be really useful).
https://developer.mozilla.org/en-US/docs/Web/JavaScript/Reference/Operators/Comma_Operator
It should be handled correctly by prettier in all cases, including this currying case.
Given input should result with no changes at all.
The current output is not valid JS syntax as the `arg2` variable is not declared, and this gives an error when executed.
| In the input I didn't write the ending semi-colon, and I didn't configure prettier about this, so a semi-colon was added.
This does not change anything on the issue. With or without semi-colon, the issue is the same.
I tested the same code with prettier **2.2.1**, and there was no problem with this previous version.
I wonder which PR breaks it.
Must break during function print.
Here says:
https://github.com/prettier/prettier/blob/81a1c463116f9dc8ec5e8059d70a9254675847e6/src/language-js/needs-parens.js#L351-L352
https://github.com/prettier/prettier/pull/9992 Seems the one, but I don't know how.
With updated playground (with help from its "second output" option under debug):
**Prettier 2.3.1**
[Playground link](https://prettier.io/playground/#N4Igxg9gdgLgprEAuc0DOMAEAzArlMTAXkwAoBDAJwHMBGAGkyuoCYBKYgPjI6O4poMmNdiHogIABxgBLdMlBVKEAO4AFKgjTIQ5ADYryAT23iARpXJgA1nBgBlSVZlRqyGJVxxxcALZm4ABNAoIAZcldccmo4ADEISl9yGFlXHXJcGAgxEAALGF89AHVcmXg0JzA4ey0ymQA3MqMdMDRTEBc0OEoYNUtqJORsfS7xACs0AA8AIUsbO3tyXzhQlzghke8QCcn7F2o9OABFXAh4Db1RkCdKLsodM3IAvRzJShcYIplAmFzkAA4AAziN4QLpFSySHRvOB3errcQAR1O8D6Um0KHIaAAtFA4EEgjlKHBkTJiX1ooMkMNLlsur4ZO5PHT9ocTmd1tTNuIYE8vj8-kgWDzLDI9PsAMIQXxUkCwgCsOVwXQAKk8MTSrvUvABJKAhWD2MDvaQAQX19hgRkOFy6AF87UA)
<!-- prettier-ignore -->
```sh
--parser babel
```
**Input:**
<!-- prettier-ignore -->
```jsx
const func = (arg1, arg2) => () => (arg1, arg2)
```
**Output:**
<!-- prettier-ignore -->
```jsx
const func = (arg1, arg2) => () => arg1, arg2;
```
**Second Output:**
<!-- prettier-ignore -->
```jsx
SyntaxError: 'Const declarations' require an initialization value. (1:46)
> 1 | const func = (arg1, arg2) => () => arg1, arg2;
| ^
2 |
```
---
with TypeScript:
**Prettier 2.3.1**
[Playground link](https://prettier.io/playground/#N4Igxg9gdgLgprEAuc0DOMAEAzArlMTAXkwAoBDAJwHMBGAGkyuoCYBKYgPjI6O4poMmNdiHogIABxgBLdMlBVKEAO4AFKgjTIQ5ADYryAT23iARpXJgA1nBgBlSVZlRqyGJVxxxcALZm4ABNAoIAZcldccmo4ADEISl9yGFlXHXJcGAgxEAALGF89AHVcmXg0JzA4ey0ymQA3MqMdMDRTEBc0OEoYNUtqJORsfS7xACs0AA8AIUsbO3tyXzhQlzghke8QCcn7F2o9OABFXAh4Db1RkCdKLsodGCNJODQwShlpHMl32CKZQJguWQAA4AAzib4QLpFSySHTfF7derrcQAR1O8D6Um0KHIaAAtFA4EEgjlKHB0TJyX1ooMkMNLlsur4ZO5PEz9ocTmd1vTNuIYOQzH8AUCkCwBZYZHp9gBhCC+OkgF4AVhyuC6ABUhTiGVd6l4AJJQEKwexvD4wACCJvsj0OFy6AF8nUA)
<!-- prettier-ignore -->
```sh
--parser typescript
```
**Input:**
<!-- prettier-ignore -->
```tsx
const func = (arg1, arg2) => () => (arg1, arg2)
```
**Output:**
<!-- prettier-ignore -->
```tsx
const func = (arg1, arg2) => () => arg1, arg2;
```
**Second Output:**
<!-- prettier-ignore -->
```tsx
const func = (arg1, arg2) => () => arg1,
arg2;
```
---
With Flow, the second output would be an error as well. | "2021-09-27T02:55:24Z" | 2.5 | [] | [
"tests/format/js/arrows/jsfmt.spec.js"
] | JavaScript | [] | [
"https://prettier.io/playground/#N4Igxg9gdgLgprEAuc0DOMAEAzArlMTAXkwAoBDAJwHMBGAGkyuoCYBKYgPjI6O4poMmNdgB0oIeiAgAHGAEt0yUFUoQA7gAUqCNMhDkANuvIBPPVIBGlcmADWcGAGUZt+VGrIYlXHClwAW0s4ABMQ0IAZcg9ccmo4ADEISgDyGAUPfXJcGAhJEAALGADDAHUC+Xg0VzA4J11K+QA3StN9MDQLEHc0OEoYTRtqVORsI16pACs0AA8AIRt7RydyALgI9zhR8b8QaZmnd2pDOABFXAh4bcMJkFdKXsp9S3Jgw3yZSncYUvkQmAKyAAHAAGKSfCC9Uo2GT6T5wR5NLZSACOF3gg1kehQ5DQAFooHBQqF8pQ4Gj5GTBnERkgxjddr0AvIvD5GUcTudLls6TspDBXr9-oCkCx+TZ5IYjgBhCABWkgBEAVnyuF6ABVXtj6bcmr4AJJQcKwJxgL5yACCRqcMFMJ2uvQAvo6gA"
] |
prettier/prettier | 11,637 | prettier__prettier-11637 | [
"11635"
] | 5909f5b3f191a0a32f759e1f4378477d3b90e28e | diff --git a/src/language-css/printer-postcss.js b/src/language-css/printer-postcss.js
--- a/src/language-css/printer-postcss.js
+++ b/src/language-css/printer-postcss.js
@@ -74,6 +74,7 @@ const {
isColorAdjusterFuncNode,
lastLineHasInlineComment,
isAtWordPlaceholderNode,
+ isConfigurationNode,
} = require("./utils.js");
const { locStart, locEnd } = require("./loc.js");
@@ -807,6 +808,19 @@ function genericPrint(path, options, print) {
continue;
}
+ if (
+ iNode.value === "with" &&
+ iNextNode &&
+ iNextNode.type === "value-paren_group" &&
+ iNextNode.open &&
+ iNextNode.open.value === "(" &&
+ iNextNode.close &&
+ iNextNode.close.value === ")"
+ ) {
+ parts.push(" ");
+ continue;
+ }
+
// Be default all values go through `line`
parts.push(line);
}
@@ -872,6 +886,10 @@ function genericPrint(path, options, print) {
const lastItem = getLast(node.groups);
const isLastItemComment = lastItem && lastItem.type === "value-comment";
const isKey = isKeyInValuePairNode(node, parentNode);
+ const isConfiguration = isConfigurationNode(node, parentNode);
+
+ const shouldBreak = isConfiguration || (isSCSSMapItem && !isKey);
+ const shouldDedent = isConfiguration || isKey;
const printed = group(
[
@@ -915,11 +933,11 @@ function genericPrint(path, options, print) {
node.close ? print("close") : "",
],
{
- shouldBreak: isSCSSMapItem && !isKey,
+ shouldBreak,
}
);
- return isKey ? dedent(printed) : printed;
+ return shouldDedent ? dedent(printed) : printed;
}
case "value-func": {
return [
diff --git a/src/language-css/utils.js b/src/language-css/utils.js
--- a/src/language-css/utils.js
+++ b/src/language-css/utils.js
@@ -484,6 +484,30 @@ function isModuleRuleName(name) {
return moduleRuleNames.has(name);
}
+function isConfigurationNode(node, parentNode) {
+ if (
+ !node.open ||
+ node.open.value !== "(" ||
+ !node.close ||
+ node.close.value !== ")" ||
+ node.groups.some((group) => group.type !== "value-comma_group")
+ ) {
+ return false;
+ }
+ if (parentNode.type === "value-comma_group") {
+ const prevIdx = parentNode.groups.indexOf(node) - 1;
+ const maybeWithNode = parentNode.groups[prevIdx];
+ if (
+ maybeWithNode &&
+ maybeWithNode.type === "value-word" &&
+ maybeWithNode.value === "with"
+ ) {
+ return true;
+ }
+ }
+ return false;
+}
+
module.exports = {
getAncestorCounter,
getAncestorNode,
@@ -539,4 +563,5 @@ module.exports = {
stringifyNode,
isAtWordPlaceholderNode,
isModuleRuleName,
+ isConfigurationNode,
};
| diff --git a/tests/format/scss/configuration/__snapshots__/jsfmt.spec.js.snap b/tests/format/scss/configuration/__snapshots__/jsfmt.spec.js.snap
new file mode 100644
--- /dev/null
+++ b/tests/format/scss/configuration/__snapshots__/jsfmt.spec.js.snap
@@ -0,0 +1,94 @@
+// Jest Snapshot v1, https://goo.gl/fbAQLP
+
+exports[`use.scss - {"trailingComma":"none"} format 1`] = `
+====================================options=====================================
+parsers: ["scss"]
+printWidth: 80
+trailingComma: "none"
+ | printWidth
+=====================================input======================================
+@use "@fylgja/base" with (
+ $family-main: (
+ Rubik,
+ Roboto,
+ system-ui,
+ sans-serif,
+ ),
+ $font-weight: 350,
+ $h1-font-weight: 500,
+ $h2-font-weight: 500,
+ $h3-font-weight: 500
+);
+
+@use 'library' with (
+ $black: #222,
+ $border-radius: 0.1rem
+);
+
+=====================================output=====================================
+@use "@fylgja/base" with (
+ $family-main: (
+ Rubik,
+ Roboto,
+ system-ui,
+ sans-serif
+ ),
+ $font-weight: 350,
+ $h1-font-weight: 500,
+ $h2-font-weight: 500,
+ $h3-font-weight: 500
+);
+
+@use "library" with (
+ $black: #222,
+ $border-radius: 0.1rem
+);
+
+================================================================================
+`;
+
+exports[`use.scss format 1`] = `
+====================================options=====================================
+parsers: ["scss"]
+printWidth: 80
+ | printWidth
+=====================================input======================================
+@use "@fylgja/base" with (
+ $family-main: (
+ Rubik,
+ Roboto,
+ system-ui,
+ sans-serif,
+ ),
+ $font-weight: 350,
+ $h1-font-weight: 500,
+ $h2-font-weight: 500,
+ $h3-font-weight: 500
+);
+
+@use 'library' with (
+ $black: #222,
+ $border-radius: 0.1rem
+);
+
+=====================================output=====================================
+@use "@fylgja/base" with (
+ $family-main: (
+ Rubik,
+ Roboto,
+ system-ui,
+ sans-serif,
+ ),
+ $font-weight: 350,
+ $h1-font-weight: 500,
+ $h2-font-weight: 500,
+ $h3-font-weight: 500
+);
+
+@use "library" with (
+ $black: #222,
+ $border-radius: 0.1rem
+);
+
+================================================================================
+`;
diff --git a/tests/format/scss/configuration/jsfmt.spec.js b/tests/format/scss/configuration/jsfmt.spec.js
new file mode 100644
--- /dev/null
+++ b/tests/format/scss/configuration/jsfmt.spec.js
@@ -0,0 +1,2 @@
+run_spec(__dirname, ["scss"], { trailingComma: "none" });
+run_spec(__dirname, ["scss"]);
diff --git a/tests/format/scss/configuration/use.scss b/tests/format/scss/configuration/use.scss
new file mode 100644
--- /dev/null
+++ b/tests/format/scss/configuration/use.scss
@@ -0,0 +1,17 @@
+@use "@fylgja/base" with (
+ $family-main: (
+ Rubik,
+ Roboto,
+ system-ui,
+ sans-serif,
+ ),
+ $font-weight: 350,
+ $h1-font-weight: 500,
+ $h2-font-weight: 500,
+ $h3-font-weight: 500
+);
+
+@use 'library' with (
+ $black: #222,
+ $border-radius: 0.1rem
+);
diff --git a/tests/format/scss/quotes/__snapshots__/jsfmt.spec.js.snap b/tests/format/scss/quotes/__snapshots__/jsfmt.spec.js.snap
--- a/tests/format/scss/quotes/__snapshots__/jsfmt.spec.js.snap
+++ b/tests/format/scss/quotes/__snapshots__/jsfmt.spec.js.snap
@@ -34,8 +34,10 @@ singleQuote: true
=====================================output=====================================
@use 'library';
-@use 'library' with
- ($black: #222, $border-radius: 0.1rem $font-family: 'Helvetica, sans-serif');
+@use 'library' with (
+ $black: #222,
+ $border-radius: 0.1rem $font-family: 'Helvetica, sans-serif'
+);
@use 'library' as *;
@@ -89,8 +91,10 @@ printWidth: 80
=====================================output=====================================
@use "library";
-@use "library" with
- ($black: #222, $border-radius: 0.1rem $font-family: "Helvetica, sans-serif");
+@use "library" with (
+ $black: #222,
+ $border-radius: 0.1rem $font-family: "Helvetica, sans-serif"
+);
@use "library" as *;
| Weird wrapping for SCSS @use rules
<!--
BEFORE SUBMITTING AN ISSUE:
1. Search for your issue on GitHub: https://github.com/prettier/prettier/issues
A large number of opened issues are duplicates of existing issues.
If someone has already opened an issue for what you are experiencing,
you do not need to open a new issue — please add a 👍 reaction to the
existing issue instead.
2. We get a lot of requests for adding options, but Prettier is
built on the principle of being opinionated about code formatting.
This means we add options only in the case of strict technical necessity.
Find out more: https://prettier.io/docs/en/option-philosophy.html
Don't fill the form below manually! Let a program create a report for you:
1. Go to https://prettier.io/playground
2. Paste your code and set options
3. Press the "Report issue" button in the lower right
-->
**Prettier 2.4.1**
[Playground link](https://prettier.io/playground/#N4Igxg9gdgLgprEAuEABArgZzgAgDpoBmAngDYDmAVgIYD0ARtdgTgO4CWMAFjgBR5QcQnABJC1ALbtSxALQTq7KEj4Dh6nACV09dgGsANGo1DNEehBgQjgkzkzFM8CbPTsbd+9SiZZ2AE7shB7CAJQhQmLQMLKscOzkXDAqAMwArAAMEaJcAIyyhNGx8YnJOJlZxpFcAEwFRXEJSSoV2SJcKfWwxU1lFQKhANwgBiAQAA4w7NCYyKDU-v4QrAAKCwizKNSkrNSOIyD0-tRgenAwAMqScAAySnDI4qTYo0cnZ5fjJ0rkyDD+6DgozgEnocAAJuCITdvOR0NRyHAAGIQfwKGBTKC-LboKwHJISUgAdS4nDgmC+YDgFw2nHYADdOMRkOBMLNRkoAjAVsdyApHtsXiBKJgAB4XH6kOAARXQlgeSCeQq+-gCLMwYDZB3GgVgRPY4O4yAAHFkQDqINgicdxiydeS4P56Q9RgBHOXwHkTTYgJiyKBwCEQg7+ODu9ihnkI-mKwVAkDYKR-AHxzCSmUehVK+Mwaj0fWGrjIGqjf6KUg-ADCEAkMZA5LSBywcAAKnnNtnRvTAQBJKBQ2AXMCBSYAQX7FxgZCzcYAvrOgA)
Not sure which version this was introduced.
Previously this did not happen.
But the current SCSS syntax using `@use` gets wrapped in a weird way.
The opening parentheses is wrapped with a indent, and also all of the code inside it.
**Input:**
```scss
@use "@fylgja/base" with (
$family-main: (
Rubik,
Roboto,
system-ui,
sans-serif,
),
$font-weight: 350,
$h1-font-weight: 500,
$h2-font-weight: 500,
$h3-font-weight: 500
);
```
**Output:**
```scss
@use "@fylgja/base" with
(
$family-main: (
Rubik,
Roboto,
system-ui,
sans-serif,
),
$font-weight: 350,
$h1-font-weight: 500,
$h2-font-weight: 500,
$h3-font-weight: 500
);
```
**Expected behavior:**
```scss
@use "@fylgja/base" with (
$family-main: (
Rubik,
Roboto,
system-ui,
sans-serif,
),
$font-weight: 350,
$h1-font-weight: 500,
$h2-font-weight: 500,
$h3-font-weight: 500
);
```
| hm, both looks good...
I think the indent should not be there @alexander-akait
But it is also inconsistent with the SCSS map wrapping which does not wrap the opening parentheses to the next line | "2021-10-08T14:58:13Z" | 2.5 | [] | [
"tests/format/scss/configuration/jsfmt.spec.js",
"tests/format/scss/quotes/jsfmt.spec.js"
] | JavaScript | [] | [
"https://prettier.io/playground/#N4Igxg9gdgLgprEAuEABArgZzgAgDpoBmAngDYDmAVgIYD0ARtdgTgO4CWMAFjgBR5QcQnABJC1ALbtSxALQTq7KEj4Dh6nACV09dgGsANGo1DNEehBgQjgkzkzFM8CbPTsbd+9SiZZ2AE7shB7CAJQhQmLQMLKscOzkXDAqAMwArAAMEaJcAIyyhNGx8YnJOJlZxpFcAEwFRXEJSSoV2SJcKfWwxU1lFQKhANwgBiAQAA4w7NCYyKDU-v4QrAAKCwizKNSkrNSOIyD0-tRgenAwAMqScAAySnDI4qTYo0cnZ5fjJ0rkyDD+6DgozgEnocAAJuCITdvOR0NRyHAAGIQfwKGBTKC-LboKwHJISUgAdS4nDgmC+YDgFw2nHYADdOMRkOBMLNRkoAjAVsdyApHtsXiBKJgAB4XH6kOAARXQlgeSCeQq+-gCLMwYDZB3GgVgRPY4O4yAAHFkQDqINgicdxiydeS4P56Q9RgBHOXwHkTTYgJiyKBwCEQg7+ODu9ihnkI-mKwVAkDYKR-AHxzCSmUehVK+Mwaj0fWGrjIGqjf6KUg-ADCEAkMZA5LSBywcAAKnnNtnRvTAQBJKBQ2AXMCBSYAQX7FxgZCzcYAvrOgA",
"https://prettier.io/playground"
] |
prettier/prettier | 11,685 | prettier__prettier-11685 | [
"11684"
] | 2e450529f2f0940f18a1bf3ad57c9313bec780c7 | diff --git a/src/language-markdown/embed.js b/src/language-markdown/embed.js
--- a/src/language-markdown/embed.js
+++ b/src/language-markdown/embed.js
@@ -21,9 +21,13 @@ function embed(path, print, textToDoc, options) {
const style = styleUnit.repeat(
Math.max(3, getMaxContinuousCount(node.value, styleUnit) + 1)
);
+ const newOptions = { parser };
+ if (node.lang === "tsx") {
+ newOptions.filepath = "dummy.tsx";
+ }
const doc = textToDoc(
getFencedCodeBlockValue(node, options.originalText),
- { parser },
+ newOptions,
{ stripTrailingHardline: true }
);
return markAsRoot([
| diff --git a/tests/format/markdown/code/__snapshots__/jsfmt.spec.js.snap b/tests/format/markdown/code/__snapshots__/jsfmt.spec.js.snap
--- a/tests/format/markdown/code/__snapshots__/jsfmt.spec.js.snap
+++ b/tests/format/markdown/code/__snapshots__/jsfmt.spec.js.snap
@@ -290,3 +290,22 @@ hello world
================================================================================
`;
+
+exports[`tsx-trailing-comma.md - {"proseWrap":"always"} format 1`] = `
+====================================options=====================================
+parsers: ["markdown"]
+printWidth: 80
+proseWrap: "always"
+ | printWidth
+=====================================input======================================
+\`\`\`tsx
+const test = <T,>(value: T) => {};
+\`\`\`
+
+=====================================output=====================================
+\`\`\`tsx
+const test = <T,>(value: T) => {};
+\`\`\`
+
+================================================================================
+`;
diff --git a/tests/format/markdown/code/tsx-trailing-comma.md b/tests/format/markdown/code/tsx-trailing-comma.md
new file mode 100644
--- /dev/null
+++ b/tests/format/markdown/code/tsx-trailing-comma.md
@@ -0,0 +1,3 @@
+```tsx
+const test = <T,>(value: T) => {};
+```
| Prettier removes trailing comma of generic arrow function in Markdown tsx codeblock
**Prettier 2.4.1**
[Playground link](https://prettier.io/playground/#N4Igxg9gdgLgprEAuEADdACGBnAHgHSkimxizlIwF4MAeAFQBoA+ACgDcBDAGwFc4kGegEpqzDMAC+AbkLpUhEIxAQADjACW0bMlCcATvogB3AAoGEOlD2OcAnjuUAjfZzABrODADKnALZwADIaUHDIAGY82HDOrh5e3qpuIQDmyDD6-Mpwfk5wACb5BYGcUCm8nClwAGIQ+n6cMJplyCCcvDAQSiAAFjB+3ADqPRrw2ElgcN6Woxrso3atYNiOICHR+jCmrikNEVExIABWeN6p3HAAirwQ8Pvc0cpJ+hutDfru+SZQ3ar6ITBBhp8jAesgABwABieRmig1cqlafwocH07DCygAjjd4Ns1FY2tgALShAoFbr6ODYjSU7aVPZISIPQ7RPwadKZFnnK44sKMg7KGCcJxAkFgpAAJkFrg03FSAGEIH4GSAKABWbq8aL0YVWJmPEDsfgASSgRVg3jA-3UAEEzd4YHYLvdopJJEA)
<!-- prettier-ignore -->
```sh
--parser markdown
```
**Input:**
<!-- prettier-ignore -->
````markdown
``` tsx
const test = <T,>(value: T) => {};
```
````
**Output:**
<!-- prettier-ignore -->
````markdown
```tsx
const test = <T>(value: T) => {};
```
````
**Expected behavior:**
Prettier should not remove the trailing comma because this results in invalid code. Expected output is:
<!-- prettier-ignore -->
````markdown
```tsx
const test = <T,>(value: T) => {};
```
````
Prettier handles trailing commas correctly in `.tsx` files. It does it by checking if the file name ends with `.tsx`. As this is false in markdown files (`.md` instead) it removes the comma. See https://github.com/prettier/prettier/blob/main/src/language-js/print/type-parameters.js#L54-L64
| "2021-10-16T14:47:57Z" | 2.5 | [] | [
"tests/format/markdown/code/jsfmt.spec.js"
] | JavaScript | [] | [
"https://prettier.io/playground/#N4Igxg9gdgLgprEAuEADdACGBnAHgHSkimxizlIwF4MAeAFQBoA+ACgDcBDAGwFc4kGegEpqzDMAC+AbkLpUhEIxAQADjACW0bMlCcATvogB3AAoGEOlD2OcAnjuUAjfZzABrODADKnALZwADIaUHDIAGY82HDOrh5e3qpuIQDmyDD6-Mpwfk5wACb5BYGcUCm8nClwAGIQ+n6cMJplyCCcvDAQSiAAFjB+3ADqPRrw2ElgcN6Woxrso3atYNiOICHR+jCmrikNEVExIABWeN6p3HAAirwQ8Pvc0cpJ+hutDfru+SZQ3ar6ITBBhp8jAesgABwABieRmig1cqlafwocH07DCygAjjd4Ns1FY2tgALShAoFbr6ODYjSU7aVPZISIPQ7RPwadKZFnnK44sKMg7KGCcJxAkFgpAAJkFrg03FSAGEIH4GSAKABWbq8aL0YVWJmPEDsfgASSgRVg3jA-3UAEEzd4YHYLvdopJJEA"
] |
|
prettier/prettier | 11,717 | prettier__prettier-11717 | [
"11716"
] | d3163259c7a1c0cfac5c78d6e35e28bf51fbd4ba | diff --git a/src/language-js/needs-parens.js b/src/language-js/needs-parens.js
--- a/src/language-js/needs-parens.js
+++ b/src/language-js/needs-parens.js
@@ -140,6 +140,10 @@ function needsParens(path, options) {
break;
case "Identifier":
return false;
+ case "TaggedTemplateExpression":
+ // babel-parser cannot parse
+ // @foo`bar`
+ return options.parser !== "typescript";
default:
return true;
}
| diff --git a/tests/format/misc/errors/babel-ts/__snapshots__/jsfmt.spec.js.snap b/tests/format/misc/errors/babel-ts/__snapshots__/jsfmt.spec.js.snap
--- a/tests/format/misc/errors/babel-ts/__snapshots__/jsfmt.spec.js.snap
+++ b/tests/format/misc/errors/babel-ts/__snapshots__/jsfmt.spec.js.snap
@@ -41,6 +41,16 @@ exports[`multiline-declaration-type.ts [babel-ts] format 1`] = `
3 |"
`;
+exports[`parenthesized-decorators-tagged-template.ts [babel-ts] format 1`] = `
+"Unexpected token (2:7)
+ 1 | class Test {
+> 2 | @foo\`bar\`
+ | ^
+ 3 | text: string = \\"text\\"
+ 4 | }
+ 5 |"
+`;
+
exports[`type-annotation-expr-statement.ts [babel-ts] format 1`] = `
"Did not expect a type annotation here. (1:3)
> 1 | (a: T);
diff --git a/tests/format/misc/errors/babel-ts/parenthesized-decorators-tagged-template.ts b/tests/format/misc/errors/babel-ts/parenthesized-decorators-tagged-template.ts
new file mode 100644
--- /dev/null
+++ b/tests/format/misc/errors/babel-ts/parenthesized-decorators-tagged-template.ts
@@ -0,0 +1,4 @@
+class Test {
+ @foo`bar`
+ text: string = "text"
+}
diff --git a/tests/format/misc/typescript-babel-only/__snapshots__/jsfmt.spec.js.snap b/tests/format/misc/typescript-babel-only/__snapshots__/jsfmt.spec.js.snap
--- a/tests/format/misc/typescript-babel-only/__snapshots__/jsfmt.spec.js.snap
+++ b/tests/format/misc/typescript-babel-only/__snapshots__/jsfmt.spec.js.snap
@@ -60,6 +60,26 @@ class Bar {
================================================================================
`;
+exports[`parenthesized-decorators-tagged-template.ts format 1`] = `
+====================================options=====================================
+parsers: ["babel-ts"]
+printWidth: 80
+ | printWidth
+=====================================input======================================
+class Test {
+ @(foo\`bar\`)
+ text: string = "text"
+}
+
+=====================================output=====================================
+class Test {
+ @(foo\`bar\`)
+ text: string = "text";
+}
+
+================================================================================
+`;
+
exports[`prettier-ignore-nested-unions.ts format 1`] = `
====================================options=====================================
parsers: ["babel-ts"]
diff --git a/tests/format/misc/typescript-babel-only/parenthesized-decorators-tagged-template.ts b/tests/format/misc/typescript-babel-only/parenthesized-decorators-tagged-template.ts
new file mode 100644
--- /dev/null
+++ b/tests/format/misc/typescript-babel-only/parenthesized-decorators-tagged-template.ts
@@ -0,0 +1,4 @@
+class Test {
+ @(foo`bar`)
+ text: string = "text"
+}
diff --git a/tests/format/misc/typescript-only/__snapshots__/jsfmt.spec.js.snap b/tests/format/misc/typescript-only/__snapshots__/jsfmt.spec.js.snap
--- a/tests/format/misc/typescript-only/__snapshots__/jsfmt.spec.js.snap
+++ b/tests/format/misc/typescript-only/__snapshots__/jsfmt.spec.js.snap
@@ -93,6 +93,26 @@ class Bar {
================================================================================
`;
+exports[`parenthesized-decorators-tagged-template.ts format 1`] = `
+====================================options=====================================
+parsers: ["typescript"]
+printWidth: 80
+ | printWidth
+=====================================input======================================
+class Test {
+ @foo\`bar\`
+ text: string = "text"
+}
+
+=====================================output=====================================
+class Test {
+ @foo\`bar\`
+ text: string = "text";
+}
+
+================================================================================
+`;
+
exports[`prettier-ignore-parenthesized-type.ts format 1`] = `
====================================options=====================================
parsers: ["typescript"]
diff --git a/tests/format/misc/typescript-only/parenthesized-decorators-tagged-template.ts b/tests/format/misc/typescript-only/parenthesized-decorators-tagged-template.ts
new file mode 100644
--- /dev/null
+++ b/tests/format/misc/typescript-only/parenthesized-decorators-tagged-template.ts
@@ -0,0 +1,4 @@
+class Test {
+ @foo`bar`
+ text: string = "text"
+}
| Typescript Issue: Template string based decorators are wrapped with parentheses
I've developed an experimental project called [Dogma](https://www.npmjs.com/package/@dogmajs/core) that implements a template string based decorator to annotate retrievable comments for properties.
**Prettier 2.4.1**
https://prettier.io/playground/#N4Igxg9gdgLgprEAucAbAhgZ0wAgCpyYw7AA6UOOAAgCIQDmAtugHSSOMLEAGAsgJ452nWN3KV4ADxhIcRAE4BLKPXIBfEABoQEAA4xF0TMlDp58iAHcACmYTGU6VJfT9j2gEbz0YANZwYAGV0TgAZZThkADMnTDhPbz8AwN0fZXpkGHkAV3iQOEYPOAATYpLQ9BVs9Ho4ADEIeWYYAxVkEHRsmAgtEAALGEZUAHU+xXhMVLA4QPtxxQA3cf52sGxe5Tj5GGtvJnRo2LyAK0xJQPTUOABFbIh4Q9Q47VT5LfaYfl1CMCV9Xt0Slgw0UxRgfWQAA4AAwvCxxYbeXTtQGEODyBaRbQARzu8F2egcHUwAFooHASiVevI4LjFDTdjVmI9niA4oxFJkcnlMJcbnjIkgYk88jB0B4QWCIUgAEzaLLoRSodIAYQgHAOKEIAFZetk4nhxQ5hayFrkAJJQMqwQK-RT6ACCVsCnyuLLgajUQA
**Input:**
```typescript
class Test {
@Dogma.comment `My comment`
text: string
}
```
**Output:**
```typescript
class Test {
@(Dogma.comment`My comment`)
text: string;
}
```
Typescript parser, different from Babel, doesn't require that template string based decorators have parentheses, but prettier doesn't comprehend that by adding parentheses that makes the decoration uglier and defeats the purpose of having the decorator as template string based.
| "2021-10-25T11:35:09Z" | 2.5 | [
"tests/format/misc/typescript-babel-only/jsfmt.spec.js",
"tests/format/misc/errors/babel-ts/jsfmt.spec.js"
] | [
"tests/format/misc/typescript-only/jsfmt.spec.js"
] | JavaScript | [] | [
"https://prettier.io/playground/#N4Igxg9gdgLgprEAucAbAhgZ0wAgCpyYw7AA6UOOAAgCIQDmAtugHSSOMLEAGAsgJ452nWN3KV4ADxhIcRAE4BLKPXIBfEABoQEAA4xF0TMlDp58iAHcACmYTGU6VJfT9j2gEbz0YANZwYAGV0TgAZZThkADMnTDhPbz8AwN0fZXpkGHkAV3iQOEYPOAATYpLQ9BVs9Ho4ADEIeWYYAxVkEHRsmAgtEAALGEZUAHU+xXhMVLA4QPtxxQA3cf52sGxe5Tj5GGtvJnRo2LyAK0xJQPTUOABFbIh4Q9Q47VT5LfaYfl1CMCV9Xt0Slgw0UxRgfWQAA4AAwvCxxYbeXTtQGEODyBaRbQARzu8F2egcHUwAFooHASiVevI4LjFDTdjVmI9niA4oxFJkcnlMJcbnjIkgYk88jB0B4QWCIUgAEzaLLoRSodIAYQgHAOKEIAFZetk4nhxQ5hayFrkAJJQMqwQK-RT6ACCVsCnyuLLgajUQA"
] |
|
prettier/prettier | 11,800 | prettier__prettier-11800 | [
"11797"
] | ffbd04ebe286f6cf65bad5b6a0a0ade65af29153 | diff --git a/src/language-css/printer-postcss.js b/src/language-css/printer-postcss.js
--- a/src/language-css/printer-postcss.js
+++ b/src/language-css/printer-postcss.js
@@ -75,6 +75,7 @@ const {
lastLineHasInlineComment,
isAtWordPlaceholderNode,
isConfigurationNode,
+ isParenGroupNode,
} = require("./utils.js");
const { locStart, locEnd } = require("./loc.js");
@@ -810,14 +811,15 @@ function genericPrint(path, options, print) {
}
if (
- iNode.value === "with" &&
- iNextNode &&
- iNextNode.type === "value-paren_group" &&
- iNextNode.open &&
- iNextNode.open.value === "(" &&
- iNextNode.close &&
- iNextNode.close.value === ")"
+ isAtWordPlaceholderNode(iNode) &&
+ isParenGroupNode(iNextNode) &&
+ locEnd(iNode) === locStart(iNextNode.open)
) {
+ parts.push(softline);
+ continue;
+ }
+
+ if (iNode.value === "with" && isParenGroupNode(iNextNode)) {
parts.push(" ");
continue;
}
diff --git a/src/language-css/utils.js b/src/language-css/utils.js
--- a/src/language-css/utils.js
+++ b/src/language-css/utils.js
@@ -508,6 +508,16 @@ function isConfigurationNode(node, parentNode) {
return false;
}
+function isParenGroupNode(node) {
+ return (
+ node.type === "value-paren_group" &&
+ node.open &&
+ node.open.value === "(" &&
+ node.close &&
+ node.close.value === ")"
+ );
+}
+
module.exports = {
getAncestorCounter,
getAncestorNode,
@@ -564,4 +574,5 @@ module.exports = {
isAtWordPlaceholderNode,
isModuleRuleName,
isConfigurationNode,
+ isParenGroupNode,
};
| diff --git a/tests/format/js/multiparser-css/__snapshots__/jsfmt.spec.js.snap b/tests/format/js/multiparser-css/__snapshots__/jsfmt.spec.js.snap
--- a/tests/format/js/multiparser-css/__snapshots__/jsfmt.spec.js.snap
+++ b/tests/format/js/multiparser-css/__snapshots__/jsfmt.spec.js.snap
@@ -401,6 +401,43 @@ const style3 = css\`
================================================================================
`;
+exports[`issue-11797.js format 1`] = `
+====================================options=====================================
+parsers: ["babel", "typescript", "flow"]
+printWidth: 80
+ | printWidth
+=====================================input======================================
+const paragraph1 = css\`
+ font-size: 12px;
+ transform: \${vert ? 'translateY' : 'translateX'}(\${translation + handleOffset}px);
+\`;
+
+const paragraph2 = css\`
+ transform: \${expr}(30px);
+\`;
+
+const paragraph3 = css\`
+ transform: \${expr} (30px);
+\`;
+
+=====================================output=====================================
+const paragraph1 = css\`
+ font-size: 12px;
+ transform: \${vert ? "translateY" : "translateX"}
+ (\${translation + handleOffset}px);
+\`;
+
+const paragraph2 = css\`
+ transform: \${expr}(30px);
+\`;
+
+const paragraph3 = css\`
+ transform: \${expr} (30px);
+\`;
+
+================================================================================
+`;
+
exports[`styled-components.js format 1`] = `
====================================options=====================================
parsers: ["babel", "typescript", "flow"]
diff --git a/tests/format/js/multiparser-css/issue-11797.js b/tests/format/js/multiparser-css/issue-11797.js
new file mode 100644
--- /dev/null
+++ b/tests/format/js/multiparser-css/issue-11797.js
@@ -0,0 +1,12 @@
+const paragraph1 = css`
+ font-size: 12px;
+ transform: ${vert ? 'translateY' : 'translateX'}(${translation + handleOffset}px);
+`;
+
+const paragraph2 = css`
+ transform: ${expr}(30px);
+`;
+
+const paragraph3 = css`
+ transform: ${expr} (30px);
+`;
| Emotion css broken by space added between templated function name and opening parenthesis
**Prettier 2.4.1**
[Playground link](https://prettier.io/playground/#N4Igxg9gdgLgprEAuc0DOMAEAHAhgJ1wHNDsALTAXkzDTQAMAdKTTAM2hgFo0BLALzhJMARgBM2AB4BuZs1YxCUNB3wBbYQBJgANzj4sAfkwByRbmUAbXPACaJzMLNK01+AA0TAXwAU281Y2vNCYANSYZBYAJpZwAPJsbGhwMF5SAJSyUPRZIAA0IBDYMMHKyKAE+BAA7gAKBAhoyCC4ltW4AJ5NBQBGhGAA1ikAyrhqcAAyvFBwyGytyb39QzDDeGDTRMiKAK5wBXBqPXBRUScTFkQ7xHAAYhDqNiVQWyi4OzAQ+SBkMGqWAHUyLx4Gh1nBho0QbwdCCOs1aN0QNNkgZaoQiGpcHMFvsQAArNCSYabWIARR2EHgOMsixAeHwqOaPVwx0s32w+GmMABvCiMDIyHEAAYCpyIMkAaRmpy4Ki9N8AI6U+DoopNN5oLgzE4nb74ODK3gG9HELE0unJNS8bb4PYFPgvckq2ZIea0vEwVm8-mCpBiArmXiWTYAYQganNKDlAFZvjtkgAVVka910nR7ACSUDOsGGYC5xQAgjnhjAOrELXAvF4gA)
<!-- prettier-ignore -->
```sh
--parser babel
--print-width 120
```
**Input:**
<!-- prettier-ignore -->
```jsx
const paragraph = css`
font-size: 12px;
transform: ${vert ? 'translateY' : 'translateX'}(${translation + handleOffset}px);
`;
```
**Output:**
<!-- prettier-ignore -->
```jsx
const paragraph = css`
font-size: 12px;
transform: ${vert ? "translateY" : "translateX"} (${translation + handleOffset}px);
`;
```
**Expected behavior:**
Do not add a space between the css function name and the opening parenthesis.
| "2021-11-12T07:48:21Z" | 2.5 | [] | [
"tests/format/js/multiparser-css/jsfmt.spec.js"
] | JavaScript | [] | [
"https://prettier.io/playground/#N4Igxg9gdgLgprEAuc0DOMAEAHAhgJ1wHNDsALTAXkzDTQAMAdKTTAM2hgFo0BLALzhJMARgBM2AB4BuZs1YxCUNB3wBbYQBJgANzj4sAfkwByRbmUAbXPACaJzMLNK01+AA0TAXwAU281Y2vNCYANSYZBYAJpZwAPJsbGhwMF5SAJSyUPRZIAA0IBDYMMHKyKAE+BAA7gAKBAhoyCC4ltW4AJ5NBQBGhGAA1ikAyrhqcAAyvFBwyGytyb39QzDDeGDTRMiKAK5wBXBqPXBRUScTFkQ7xHAAYhDqNiVQWyi4OzAQ+SBkMGqWAHUyLx4Gh1nBho0QbwdCCOs1aN0QNNkgZaoQiGpcHMFvsQAArNCSYabWIARR2EHgOMsixAeHwqOaPVwx0s32w+GmMABvCiMDIyHEAAYCpyIMkAaRmpy4Ki9N8AI6U+DoopNN5oLgzE4nb74ODK3gG9HELE0unJNS8bb4PYFPgvckq2ZIea0vEwVm8-mCpBiArmXiWTYAYQganNKDlAFZvjtkgAVVka910nR7ACSUDOsGGYC5xQAgjnhjAOrELXAvF4gA"
] |
|
prettier/prettier | 11,884 | prettier__prettier-11884 | [
"11878"
] | 62ede8b710731228dddc8e900fe99e5d3cc487a8 | diff --git a/src/language-js/print/type-annotation.js b/src/language-js/print/type-annotation.js
--- a/src/language-js/print/type-annotation.js
+++ b/src/language-js/print/type-annotation.js
@@ -4,7 +4,7 @@ const {
printComments,
printDanglingComments,
} = require("../../main/comments.js");
-const { getLast } = require("../../common/util.js");
+const { getLast, isNonEmptyArray } = require("../../common/util.js");
const {
builders: { group, join, line, softline, indent, align, ifBreak },
} = require("../../document/index.js");
@@ -287,15 +287,21 @@ function printFunctionType(path, options, print) {
function printTupleType(path, options, print) {
const node = path.getValue();
const typesField = node.type === "TSTupleType" ? "elementTypes" : "types";
- const hasRest =
- node[typesField].length > 0 &&
- getLast(node[typesField]).type === "TSRestType";
+ const types = node[typesField];
+ const isNonEmptyTuple = isNonEmptyArray(types);
+ const hasRest = isNonEmptyTuple && getLast(types).type === "TSRestType";
+ const bracketsDelimiterLine = isNonEmptyTuple ? softline : "";
return group([
"[",
- indent([softline, printArrayItems(path, options, typesField, print)]),
- ifBreak(shouldPrintComma(options, "all") && !hasRest ? "," : ""),
+ indent([
+ bracketsDelimiterLine,
+ printArrayItems(path, options, typesField, print),
+ ]),
+ ifBreak(
+ isNonEmptyTuple && shouldPrintComma(options, "all") && !hasRest ? "," : ""
+ ),
printDanglingComments(path, options, /* sameIndent */ true),
- softline,
+ bracketsDelimiterLine,
"]",
]);
}
| diff --git a/tests/format/typescript/tuple/__snapshots__/jsfmt.spec.js.snap b/tests/format/typescript/tuple/__snapshots__/jsfmt.spec.js.snap
--- a/tests/format/typescript/tuple/__snapshots__/jsfmt.spec.js.snap
+++ b/tests/format/typescript/tuple/__snapshots__/jsfmt.spec.js.snap
@@ -208,6 +208,71 @@ export interface ShopQueryResult {
================================================================================
`;
+exports[`trailing-comma-for-empty-tuples.ts - {"trailingComma":"all"} format 1`] = `
+====================================options=====================================
+parsers: ["typescript"]
+printWidth: 80
+trailingComma: "all"
+ | printWidth
+=====================================input======================================
+type Loooooooooooooooooooooooooooooooooooooooooooooooooooooooooooooooooooong = []
+
+type Foo = Foooooooooooooooooooooooooooooooooooooooooooooooooooooooooo extends [] ? Foo3 : Foo4;
+=====================================output=====================================
+type Loooooooooooooooooooooooooooooooooooooooooooooooooooooooooooooooooooong =
+ [];
+
+type Foo =
+ Foooooooooooooooooooooooooooooooooooooooooooooooooooooooooo extends []
+ ? Foo3
+ : Foo4;
+
+================================================================================
+`;
+
+exports[`trailing-comma-for-empty-tuples.ts - {"trailingComma":"none"} format 1`] = `
+====================================options=====================================
+parsers: ["typescript"]
+printWidth: 80
+trailingComma: "none"
+ | printWidth
+=====================================input======================================
+type Loooooooooooooooooooooooooooooooooooooooooooooooooooooooooooooooooooong = []
+
+type Foo = Foooooooooooooooooooooooooooooooooooooooooooooooooooooooooo extends [] ? Foo3 : Foo4;
+=====================================output=====================================
+type Loooooooooooooooooooooooooooooooooooooooooooooooooooooooooooooooooooong =
+ [];
+
+type Foo =
+ Foooooooooooooooooooooooooooooooooooooooooooooooooooooooooo extends []
+ ? Foo3
+ : Foo4;
+
+================================================================================
+`;
+
+exports[`trailing-comma-for-empty-tuples.ts format 1`] = `
+====================================options=====================================
+parsers: ["typescript"]
+printWidth: 80
+ | printWidth
+=====================================input======================================
+type Loooooooooooooooooooooooooooooooooooooooooooooooooooooooooooooooooooong = []
+
+type Foo = Foooooooooooooooooooooooooooooooooooooooooooooooooooooooooo extends [] ? Foo3 : Foo4;
+=====================================output=====================================
+type Loooooooooooooooooooooooooooooooooooooooooooooooooooooooooooooooooooong =
+ [];
+
+type Foo =
+ Foooooooooooooooooooooooooooooooooooooooooooooooooooooooooo extends []
+ ? Foo3
+ : Foo4;
+
+================================================================================
+`;
+
exports[`tuple.ts - {"trailingComma":"all"} format 1`] = `
====================================options=====================================
parsers: ["typescript"]
diff --git a/tests/format/typescript/tuple/trailing-comma-for-empty-tuples.ts b/tests/format/typescript/tuple/trailing-comma-for-empty-tuples.ts
new file mode 100644
--- /dev/null
+++ b/tests/format/typescript/tuple/trailing-comma-for-empty-tuples.ts
@@ -0,0 +1,3 @@
+type Loooooooooooooooooooooooooooooooooooooooooooooooooooooooooooooooooooong = []
+
+type Foo = Foooooooooooooooooooooooooooooooooooooooooooooooooooooooooo extends [] ? Foo3 : Foo4;
\ No newline at end of file
| When formatting TypeScript generics, a comma was incorrectly inserted in the empty array.
**Prettier 2.5.0**
[Playground link](https://prettier.io/playground/#N4Igxg9gdgLgprEAuEMCeAHOACAhrgIwLDABNSAeXAPmwF5sBtAXQB1ZMcBrAKwAsArqQCWAMy64ANgFswUURQgEe2OAA94UUgGdsAJTiQATpW0wjwqAHMANNgFQuUCAHco1O-nKjRVq6o0EHWwzC2sWejxCYjJKJR5qWgYvUh8-AM1glgB+bCg4ADc4IyQ8wuKQGxAIDBhhaG1kUFwjI1cABRaERpQpF1w0RqqCI1wwLjgYAGVcaTgAGUs4ZFEpbThh0fHJqYwxyytkcwENkDhpAjhyK-ncawFcKzgAMQgjaVwYOutkEFwBGAQSogPgwaSSADqfGE8G0ezAcCm3RhwgKMLQvzA2iGIEs6yMMHaoysHxWa1OPG0aimB0kcAAigIIPAyZJ1lU9kZ8b90FhtGALLVgRgwjAIcJSDA+MgAIwAJgADBy2usIaMML8RXB8UVgQBHJnwIk1Hp-bQAWnyVyuwKMcANwjtRMepKQqzZp3W0mERyMJyq2lpDMNyzd5KqMEI4sl0qQcojo2EkgOAGEINJXX9JJJgQJ1gAVQg9d3skAFE4ASS0CGmAuEtQAglopug6az1gBfDtAA)
<!-- prettier-ignore -->
```sh
--parser typescript
--print-width 120
--trailing-comma all
```
**Input:**
<!-- prettier-ignore -->
```tsx
type aabbccdd<a> = []
type kjhudifkalmcnf<obj extends Record<string, unknown>, aaddffgg extends string[] = aabbccdd<obj>> = aaddffgg extends []? never: never
```
**Output:**
<!-- prettier-ignore -->
```tsx
type aabbccdd<a> = [];
type kjhudifkalmcnf<obj extends Record<string, unknown>, aaddffgg extends string[] = aabbccdd<obj>> = aaddffgg extends [
,
]
? never
: never;
```
**Expected behavior:**
like:
```
type aabbccdd<a> = [];
type kjhudifkalmcnf<
obj extends Record<string, unknown>,
aaddffgg extends string[] = aabbccdd<obj>
> = aaddffgg extends [] ? never : never;
```
It seems to be related to the length.
This problem only occurs when the `print-width` is 120 and the length of the identifier is exactly this length.
In addition, it outputs on my computer as:

| Minimal reproduce:
**Prettier 2.5.0**
[Playground link](https://prettier.io/playground/#N4Igxg9gdgLgprEAuEMCeAHOACAYhCbAXmwG0BdAbhABoQIMYBLaAZ2VAEMAnbiAdwAKPBOxScANv05p2dAEbdOYANZwYAZU4BbOABkmUOMgBmk1nAVLV6jRmWGA5shjcArpZBxt8uABM-fz1OKEc3Tkc4fG5tThhmUOQQTjcYCFoQAAsYbQkAdUymeFZ7MDgNUSKmADcitCSwVjkQQwtuGEElR1jTc08AK1YADw0nCTgARTcIeF6JCzp7bjak9CxWMG4mRgyMPgs8pQwkvbg26uM6AEdp+E6GMWTWAFojf38M7jgbpi-OiJ6SDM808Fm0TBc7lBY0mt2MQL6dBgnHkeSYfhgmWQACYkUomBInABhCDaQHJCQSDJuCwAFRRYmBCxA1Q8AEkoIFYBpNtsYABBTkadDjObMvaGGBojFYpAARgAzABfJVAA)
<!-- prettier-ignore -->
```sh
--parser typescript
--print-width 13
--trailing-comma all
```
**Input:**
<!-- prettier-ignore -->
```tsx
type Foo = [];
```
**Output:**
<!-- prettier-ignore -->
```tsx
type Foo = [
,
];
``` | "2021-11-30T12:59:49Z" | 2.6 | [] | [
"tests/format/typescript/tuple/jsfmt.spec.js"
] | JavaScript | [] | [
"https://prettier.io/playground/#N4Igxg9gdgLgprEAuEMCeAHOACAhrgIwLDABNSAeXAPmwF5sBtAXQB1ZMcBrAKwAsArqQCWAMy64ANgFswUURQgEe2OAA94UUgGdsAJTiQATpW0wjwqAHMANNgFQuUCAHco1O-nKjRVq6o0EHWwzC2sWejxCYjJKJR5qWgYvUh8-AM1glgB+bCg4ADc4IyQ8wuKQGxAIDBhhaG1kUFwjI1cABRaERpQpF1w0RqqCI1wwLjgYAGVcaTgAGUs4ZFEpbThh0fHJqYwxyytkcwENkDhpAjhyK-ncawFcKzgAMQgjaVwYOutkEFwBGAQSogPgwaSSADqfGE8G0ezAcCm3RhwgKMLQvzA2iGIEs6yMMHaoysHxWa1OPG0aimB0kcAAigIIPAyZJ1lU9kZ8b90FhtGALLVgRgwjAIcJSDA+MgAIwAJgADBy2usIaMML8RXB8UVgQBHJnwIk1Hp-bQAWnyVyuwKMcANwjtRMepKQqzZp3W0mERyMJyq2lpDMNyzd5KqMEI4sl0qQcojo2EkgOAGEINJXX9JJJgQJ1gAVQg9d3skAFE4ASS0CGmAuEtQAglopug6az1gBfDtAA"
] |
prettier/prettier | 11,900 | prettier__prettier-11900 | [
"11899"
] | d09545952ef6cc37b1f0ad985ef0c1e3887b7e56 | diff --git a/src/language-handlebars/utils.js b/src/language-handlebars/utils.js
--- a/src/language-handlebars/utils.js
+++ b/src/language-handlebars/utils.js
@@ -32,6 +32,7 @@ function isGlimmerComponent(node) {
return (
isNodeOfSomeType(node, ["ElementNode"]) &&
typeof node.tag === "string" &&
+ node.tag[0] !== ":" &&
(isUppercase(node.tag[0]) || node.tag.includes("."))
);
}
| diff --git a/tests/format/handlebars/basics/__snapshots__/jsfmt.spec.js.snap b/tests/format/handlebars/basics/__snapshots__/jsfmt.spec.js.snap
--- a/tests/format/handlebars/basics/__snapshots__/jsfmt.spec.js.snap
+++ b/tests/format/handlebars/basics/__snapshots__/jsfmt.spec.js.snap
@@ -93,6 +93,30 @@ printWidth: 80
================================================================================
`;
+exports[`named-block.hbs format 1`] = `
+====================================options=====================================
+parsers: ["glimmer"]
+printWidth: 80
+ | printWidth
+=====================================input======================================
+<ComponentWithNamedBlocks>
+ <:first-named-block></:first-named-block>
+ <:second-named-block> </:second-named-block>
+ <:named-block-with-comment>{{! Do not convert to an empty element}}</:named-block-with-comment>
+ <:named-block-with-content>Hello</:named-block-with-content>
+</ComponentWithNamedBlocks>
+
+=====================================output=====================================
+<ComponentWithNamedBlocks>
+ <:first-named-block></:first-named-block>
+ <:second-named-block> </:second-named-block>
+ <:named-block-with-comment
+ >{{! Do not convert to an empty element}}</:named-block-with-comment>
+ <:named-block-with-content>Hello</:named-block-with-content>
+</ComponentWithNamedBlocks>
+================================================================================
+`;
+
exports[`nested-path.hbs format 1`] = `
====================================options=====================================
parsers: ["glimmer"]
diff --git a/tests/format/handlebars/basics/named-block.hbs b/tests/format/handlebars/basics/named-block.hbs
new file mode 100644
--- /dev/null
+++ b/tests/format/handlebars/basics/named-block.hbs
@@ -0,0 +1,6 @@
+<ComponentWithNamedBlocks>
+ <:first-named-block></:first-named-block>
+ <:second-named-block> </:second-named-block>
+ <:named-block-with-comment>{{! Do not convert to an empty element}}</:named-block-with-comment>
+ <:named-block-with-content>Hello</:named-block-with-content>
+</ComponentWithNamedBlocks>
| Glimmer: Named blocks without content are self-closed on format
**Prettier 2.5.0**
[Playground link](https://prettier.io/playground/#N4Igxg9gdgLgprEAuEAeAwhAtgB2gmAdQEsYALAOQEMs4ATAIQBsIwBrAZwD4AdKAAn6okAM2IAnDjAC0UGvWkAjFuy6oA9KIlTZ8ukpVteAoUg5xIUfXNr7lrI0M3nL1vQYfHBwmwvvtpAHdSMmlILFpYLmBgAEJ+ABEIfigIGH5LADc4cXSYZKoBOFwYAE9+OCZiggBfGo0kXztDIJCw7EiYL1MmjwDg8nbYAi4ACUqWBt7-NlbBy3govg1MXHxYEnJqW2YHbj4+GPjU8SwqJgqqzo5+QIhxNn4qG7gADxwLeDp+OuW6YkyanU-0BBygR34AEl0uZijd8vwRBAmCxAvxyHB+Bx5PwWABzYhgJ43cJ4KAEG7qfgABWeYHO6Ge9Eu1VgHAA-D8astVmSCJsyBAAK4wTDDKIrbB8jYhYWi6CLLrLMgTCBAlUotVgkAAGhAEBwMGI0A4yFAVHE4gggVp4gQppQ50CVFKpr1inEVHYcBgAGV5AAZYjk5Aic7md2e71+nBe4N40PhuB64qKeh0egBwp4oVUPFwABi9zOMCNUATjpFEF1IDIMCwTEIZFIcA4sbAcF99tIANIpWQ4A4bpAwfMuWpnrxZ0TTAjIAAVhxXr741UAIpCtJwGdz2OSHIDvFMYgRA96nDiYNEYh0cjIAAcAAZz1bzIRPTgB06XcOAI6b+AJwNB0QGeWQ4Hoegaztf8JDgCc82nUQkz1cwsGIZAYHEIVkxADhVzgDctx3XCYCoRQSFvMhkAAJj1LCqGIY9y1WJCQFbABWGshXMAAVciHTDWdcMyHDISsAhfTAS9DQAQSsX0yiqEi9UXV4GCjNgfX9WggxDZDhLqIA)
<!-- prettier-ignore -->
```sh
--parser glimmer
--no-bracket-spacing
--prose-wrap always
```
**Input:**
<!-- prettier-ignore -->
```hbs
<ComponentWithNamedBlocks>
<:first-named-block></:first-named-block>
<:second-named-block> </:second-named-block>
<:named-block-with-comment>{{! Do not convert to an empty element}}</:named-block-with-comment>
<:named-block-with-content>Hello</:named-block-with-content>
</ComponentWithNamedBlocks>
{{! normal elements work as expected }}
<div></div>
{{! It seems to follow the same logic as components / PascalCased elements? }}
<ComponentWithoutContent></ComponentWithoutContent>
<hello></hello>
```
**Output:**
<!-- prettier-ignore -->
```hbs
<ComponentWithNamedBlocks>
<:first-named-block />
<:second-named-block />
<:named-block-with-comment
>{{! Do not convert to an empty element}}</:named-block-with-comment>
<:named-block-with-content>Hello</:named-block-with-content>
</ComponentWithNamedBlocks>
{{! normal elements work as expected }}
<div></div>
{{! It seems to follow the same logic as components / PascalCased elements? }}
<ComponentWithoutContent />
<hello></hello>
```
**Expected behavior:**
```hbs
<ComponentWithNamedBlocks>
<:first-named-block></:first-named-block>
<:second-named-block> </:second-named-block>
<:named-block-with-comment
>{{! Do not convert to an empty element}}</:named-block-with-comment>
<:named-block-with-content>Hello</:named-block-with-content>
</ComponentWithNamedBlocks>
{{! normal elements work as expected }}
<div></div>
{{! It seems to follow the same logic as components / PascalCased elements? }}
<ComponentWithoutContent />
<hello></hello>
```
Ember 3.25 enabled the yieldable named blocks feature which is explained in [this RFC](https://emberjs.github.io/rfcs/0460-yieldable-named-blocks.html).
Prettier does support the syntax already, but there is one case that doesn't work as expected: If a named block doesn't have any content Prettier will convert it to its self-closing equivalent (which seems to mimic the behavior for components in the Angle Bracket Invocation). The problem is that the Glimmer template compiler doesn't support self-closing named blocks and throws an error.
`<:named-block-name/> is not a valid named block: named blocks cannot be self-closing`.
So I think the named blocks should be treated the same as normal HTML elements instead of as components. The easiest way around this is to add a comment to the named block so that it will be not formatted as a self-closing element.
| Hi @Windvis, thanks for the detailed report!
For reference, even though the RFC allegedly supports self-closing named blocks, it stopped supporting later (https://github.com/glimmerjs/glimmer-vm/commit/7f8b6384d4ff1ff0c14d1c64585fb86be47e2bd4). | "2021-12-01T16:01:30Z" | 2.6 | [] | [
"tests/format/handlebars/basics/jsfmt.spec.js"
] | JavaScript | [] | [
"https://prettier.io/playground/#N4Igxg9gdgLgprEAuEAeAwhAtgB2gmAdQEsYALAOQEMs4ATAIQBsIwBrAZwD4AdKAAn6okAM2IAnDjAC0UGvWkAjFuy6oA9KIlTZ8ukpVteAoUg5xIUfXNr7lrI0M3nL1vQYfHBwmwvvtpAHdSMmlILFpYLmBgAEJ+ABEIfigIGH5LADc4cXSYZKoBOFwYAE9+OCZiggBfGo0kXztDIJCw7EiYL1MmjwDg8nbYAi4ACUqWBt7-NlbBy3govg1MXHxYEnJqW2YHbj4+GPjU8SwqJgqqzo5+QIhxNn4qG7gADxwLeDp+OuW6YkyanU-0BBygR34AEl0uZijd8vwRBAmCxAvxyHB+Bx5PwWABzYhgJ43cJ4KAEG7qfgABWeYHO6Ge9Eu1VgHAA-D8astVmSCJsyBAAK4wTDDKIrbB8jYhYWi6CLLrLMgTCBAlUotVgkAAGhAEBwMGI0A4yFAVHE4gggVp4gQppQ50CVFKpr1inEVHYcBgAGV5AAZYjk5Aic7md2e71+nBe4N40PhuB64qKeh0egBwp4oVUPFwABi9zOMCNUATjpFEF1IDIMCwTEIZFIcA4sbAcF99tIANIpWQ4A4bpAwfMuWpnrxZ0TTAjIAAVhxXr741UAIpCtJwGdz2OSHIDvFMYgRA96nDiYNEYh0cjIAAcAAZz1bzIRPTgB06XcOAI6b+AJwNB0QGeWQ4Hoegaztf8JDgCc82nUQkz1cwsGIZAYHEIVkxADhVzgDctx3XCYCoRQSFvMhkAAJj1LCqGIY9y1WJCQFbABWGshXMAAVciHTDWdcMyHDISsAhfTAS9DQAQSsX0yiqEi9UXV4GCjNgfX9WggxDZDhLqIA"
] |
prettier/prettier | 11,941 | prettier__prettier-11941 | [
"11939"
] | db0604eddd203031328b1ff88044dfdd0caf3c60 | diff --git a/src/language-js/loc.js b/src/language-js/loc.js
--- a/src/language-js/loc.js
+++ b/src/language-js/loc.js
@@ -32,7 +32,8 @@ function locEnd(node) {
* @returns {boolean}
*/
function hasSameLocStart(nodeA, nodeB) {
- return locStart(nodeA) === locStart(nodeB);
+ const nodeAStart = locStart(nodeA);
+ return Number.isInteger(nodeAStart) && nodeAStart === locStart(nodeB);
}
/**
@@ -41,7 +42,8 @@ function hasSameLocStart(nodeA, nodeB) {
* @returns {boolean}
*/
function hasSameLocEnd(nodeA, nodeB) {
- return locEnd(nodeA) === locEnd(nodeB);
+ const nodeAEnd = locEnd(nodeA);
+ return Number.isInteger(nodeAEnd) && nodeAEnd === locEnd(nodeB);
}
/**
diff --git a/src/language-js/print/module.js b/src/language-js/print/module.js
--- a/src/language-js/print/module.js
+++ b/src/language-js/print/module.js
@@ -11,6 +11,8 @@ const {
CommentCheckFlags,
shouldPrintComma,
needsHardlineAfterDanglingComment,
+ isStringLiteral,
+ rawText,
} = require("../utils.js");
const { locStart, hasSameLoc } = require("../loc.js");
const {
@@ -294,6 +296,8 @@ function printModuleSpecifier(path, options, print) {
const isImport = type.startsWith("Import");
const leftSideProperty = isImport ? "imported" : "local";
const rightSideProperty = isImport ? "local" : "exported";
+ const leftSideNode = node[leftSideProperty];
+ const rightSideNode = node[rightSideProperty];
let left = "";
let right = "";
if (
@@ -301,16 +305,11 @@ function printModuleSpecifier(path, options, print) {
type === "ImportNamespaceSpecifier"
) {
left = "*";
- } else if (node[leftSideProperty]) {
+ } else if (leftSideNode) {
left = print(leftSideProperty);
}
- if (
- node[rightSideProperty] &&
- (!node[leftSideProperty] ||
- // import {a as a} from '.'
- !hasSameLoc(node[leftSideProperty], node[rightSideProperty]))
- ) {
+ if (rightSideNode && !isShorthandSpecifier(node)) {
right = print(rightSideProperty);
}
@@ -318,6 +317,43 @@ function printModuleSpecifier(path, options, print) {
return parts;
}
+function isShorthandSpecifier(specifier) {
+ if (
+ specifier.type !== "ImportSpecifier" &&
+ specifier.type !== "ExportSpecifier"
+ ) {
+ return false;
+ }
+
+ const {
+ local,
+ [specifier.type === "ImportSpecifier" ? "imported" : "exported"]:
+ importedOrExported,
+ } = specifier;
+
+ if (
+ local.type !== importedOrExported.type ||
+ !hasSameLoc(local, importedOrExported)
+ ) {
+ return false;
+ }
+
+ if (isStringLiteral(local)) {
+ return (
+ local.value === importedOrExported.value &&
+ rawText(local) === rawText(importedOrExported)
+ );
+ }
+
+ switch (local.type) {
+ case "Identifier":
+ return local.name === importedOrExported.name;
+ default:
+ /* istanbul ignore next */
+ return false;
+ }
+}
+
module.exports = {
printImportDeclaration,
printExportDeclaration,
| diff --git a/tests/integration/__tests__/__snapshots__/format-ast.js.snap b/tests/integration/__tests__/__snapshots__/format-ast.js.snap
new file mode 100644
--- /dev/null
+++ b/tests/integration/__tests__/__snapshots__/format-ast.js.snap
@@ -0,0 +1,21 @@
+// Jest Snapshot v1, https://goo.gl/fbAQLP
+
+exports[`formatAST Shorthand specifier 1`] = `
+"export { specifier1 as specifier2 };
+"
+`;
+
+exports[`formatAST Shorthand specifier 2 1`] = `
+"export { specifier1 as specifier2 };
+"
+`;
+
+exports[`formatAST Shorthand specifier 3 1`] = `
+"export { \\"specifier\\" as specifier };
+"
+`;
+
+exports[`formatAST Shorthand specifier 4 1`] = `
+"export { \\"specifier\\" as \\"specifier\\" };
+"
+`;
diff --git a/tests/integration/__tests__/format-ast.js b/tests/integration/__tests__/format-ast.js
new file mode 100644
--- /dev/null
+++ b/tests/integration/__tests__/format-ast.js
@@ -0,0 +1,97 @@
+"use strict";
+
+const {
+ __debug: { formatAST },
+} = require("prettier-local");
+
+describe("formatAST", () => {
+ const formatExportSpecifier = (specifier) => {
+ const { formatted } = formatAST(
+ {
+ type: "Program",
+ body: [
+ {
+ type: "ExportNamedDeclaration",
+ specifiers: [specifier],
+ },
+ ],
+ },
+ { parser: "meriyah" }
+ );
+
+ return formatted;
+ };
+
+ test("Shorthand specifier", () => {
+ expect(
+ formatExportSpecifier({
+ type: "ExportSpecifier",
+ local: {
+ type: "Identifier",
+ name: "specifier1",
+ },
+ exported: {
+ type: "Identifier",
+ name: "specifier2",
+ },
+ })
+ ).toMatchSnapshot();
+ });
+
+ test("Shorthand specifier 2", () => {
+ expect(
+ formatExportSpecifier({
+ type: "ExportSpecifier",
+ local: {
+ type: "Identifier",
+ name: "specifier1",
+ range: [0, 0],
+ },
+ exported: {
+ type: "Identifier",
+ name: "specifier2",
+ range: [0, 0],
+ },
+ })
+ ).toMatchSnapshot();
+ });
+
+ test("Shorthand specifier 3", () => {
+ expect(
+ formatExportSpecifier({
+ type: "ExportSpecifier",
+ local: {
+ type: "Literal",
+ value: "specifier",
+ raw: '"specifier"',
+ range: [0, 0],
+ },
+ exported: {
+ type: "Identifier",
+ name: "specifier",
+ range: [0, 0],
+ },
+ })
+ ).toMatchSnapshot();
+ });
+
+ test("Shorthand specifier 4", () => {
+ expect(
+ formatExportSpecifier({
+ type: "ExportSpecifier",
+ local: {
+ type: "Literal",
+ value: "specifier",
+ raw: '"specifier"',
+ range: [0, 0],
+ },
+ exported: {
+ type: "Literal",
+ value: "specifier",
+ raw: "'specifier'",
+ range: [0, 0],
+ },
+ })
+ ).toMatchSnapshot();
+ });
+});
| Invalid shorthand for ExportSpecifier
Sorry for not following the usual issue formatting but this issue is only available via the API:
```js
// Actual: export {specifier1};
// Expected: export {specifier1 as specifier2};
console.log(require("prettier").format(".", {
parser: () => ({
type: "Program",
body: [
{
type: "ExportNamedDeclaration",
declaration: null,
specifiers: [
{
type: "ExportSpecifier",
local: {
// start: 0, end: 0,
type: "Identifier",
name: "specifier1",
},
exported: {
// start: 1, end: 1,
type: "Identifier",
name: "specifier2",
},
},
],
source: null,
},
],
sourceType: "module",
}),
}));
```
Uncommenting the `start` and `end` properties solves the issue. I understand that `prettier` looks into the `start` and `end` properties to inspect the original formatting of the code. But I submit to you that these properties should only affect the formatting of the generated code and not its correctness. This can be motivated by the fact that the `start` and `end` properties are not part of the [estree specification](https://github.com/estree/estree).
| Location info is required to use Prettier.
But we can check specifier name first | "2021-12-09T12:29:50Z" | 2.6 | [] | [
"tests/integration/__tests__/format-ast.js"
] | JavaScript | [] | [] |
prettier/prettier | 11,992 | prettier__prettier-11992 | [
"11991"
] | a91465dfdef9e41995317756240c975c8acc8390 | diff --git a/src/language-js/utils.js b/src/language-js/utils.js
--- a/src/language-js/utils.js
+++ b/src/language-js/utils.js
@@ -308,7 +308,10 @@ function isTemplateLiteral(node) {
}
/**
- * Note: `inject` is used in AngularJS 1.x, `async` in Angular 2+
+ * Note: `inject` is used in AngularJS 1.x, `async` and `fakeAsync` in
+ * Angular 2+, although `async` is deprecated and replaced by `waitForAsync`
+ * since Angular 12.
+ *
* example: https://docs.angularjs.org/guide/unit-testing#using-beforeall-
*
* @param {CallExpression} node
@@ -318,9 +321,7 @@ function isAngularTestWrapper(node) {
return (
isCallExpression(node) &&
node.callee.type === "Identifier" &&
- (node.callee.name === "async" ||
- node.callee.name === "inject" ||
- node.callee.name === "fakeAsync")
+ ["async", "inject", "fakeAsync", "waitForAsync"].includes(node.callee.name)
);
}
| diff --git a/tests/format/js/test-declarations/__snapshots__/jsfmt.spec.js.snap b/tests/format/js/test-declarations/__snapshots__/jsfmt.spec.js.snap
--- a/tests/format/js/test-declarations/__snapshots__/jsfmt.spec.js.snap
+++ b/tests/format/js/test-declarations/__snapshots__/jsfmt.spec.js.snap
@@ -276,6 +276,133 @@ function x() {
================================================================================
`;
+exports[`angular_waitForAsync.js - {"arrowParens":"avoid"} format 1`] = `
+====================================options=====================================
+arrowParens: "avoid"
+parsers: ["babel", "flow", "typescript"]
+printWidth: 80
+ | printWidth
+=====================================input======================================
+beforeEach(waitForAsync(() => {
+ // code
+}));
+
+afterAll(waitForAsync(() => {
+ console.log('Hello');
+}));
+
+it('should create the app', waitForAsync(() => {
+ //code
+}));
+
+it("does something really long and complicated so I have to write a very long name for the test", waitForAsync(() => {
+ // code
+}));
+
+it("does something really long and complicated so I have to write a very long name for the test", waitForAsync(() => new SSSSSSSSSSSSSSSSSSSSSSSSSSSSSSSSSSSSSSSSSSSSSSSSSSSSSSSSSSSSSSSSSSSSSSSSSSSSSSSS));
+
+/*
+* isTestCall(parent) should only be called when parent exists
+* and parent.type is CallExpression. This test makes sure that
+* no errors are thrown when calling isTestCall(parent)
+*/
+function x() { waitForAsync(() => {}) }
+
+=====================================output=====================================
+beforeEach(waitForAsync(() => {
+ // code
+}));
+
+afterAll(waitForAsync(() => {
+ console.log("Hello");
+}));
+
+it("should create the app", waitForAsync(() => {
+ //code
+}));
+
+it("does something really long and complicated so I have to write a very long name for the test", waitForAsync(() => {
+ // code
+}));
+
+it("does something really long and complicated so I have to write a very long name for the test", waitForAsync(() =>
+ new SSSSSSSSSSSSSSSSSSSSSSSSSSSSSSSSSSSSSSSSSSSSSSSSSSSSSSSSSSSSSSSSSSSSSSSSSSSSSSSS()));
+
+/*
+ * isTestCall(parent) should only be called when parent exists
+ * and parent.type is CallExpression. This test makes sure that
+ * no errors are thrown when calling isTestCall(parent)
+ */
+function x() {
+ waitForAsync(() => {});
+}
+
+================================================================================
+`;
+
+exports[`angular_waitForAsync.js format 1`] = `
+====================================options=====================================
+parsers: ["babel", "flow", "typescript"]
+printWidth: 80
+ | printWidth
+=====================================input======================================
+beforeEach(waitForAsync(() => {
+ // code
+}));
+
+afterAll(waitForAsync(() => {
+ console.log('Hello');
+}));
+
+it('should create the app', waitForAsync(() => {
+ //code
+}));
+
+it("does something really long and complicated so I have to write a very long name for the test", waitForAsync(() => {
+ // code
+}));
+
+it("does something really long and complicated so I have to write a very long name for the test", waitForAsync(() => new SSSSSSSSSSSSSSSSSSSSSSSSSSSSSSSSSSSSSSSSSSSSSSSSSSSSSSSSSSSSSSSSSSSSSSSSSSSSSSSS));
+
+/*
+* isTestCall(parent) should only be called when parent exists
+* and parent.type is CallExpression. This test makes sure that
+* no errors are thrown when calling isTestCall(parent)
+*/
+function x() { waitForAsync(() => {}) }
+
+=====================================output=====================================
+beforeEach(waitForAsync(() => {
+ // code
+}));
+
+afterAll(waitForAsync(() => {
+ console.log("Hello");
+}));
+
+it("should create the app", waitForAsync(() => {
+ //code
+}));
+
+it("does something really long and complicated so I have to write a very long name for the test", waitForAsync(() => {
+ // code
+}));
+
+it("does something really long and complicated so I have to write a very long name for the test", waitForAsync(() =>
+ new SSSSSSSSSSSSSSSSSSSSSSSSSSSSSSSSSSSSSSSSSSSSSSSSSSSSSSSSSSSSSSSSSSSSSSSSSSSSSSSS()));
+
+/*
+ * isTestCall(parent) should only be called when parent exists
+ * and parent.type is CallExpression. This test makes sure that
+ * no errors are thrown when calling isTestCall(parent)
+ */
+function x() {
+ waitForAsync(() => {});
+}
+
+================================================================================
+`;
+
exports[`angularjs_inject.js - {"arrowParens":"avoid"} format 1`] = `
====================================options=====================================
arrowParens: "avoid"
diff --git a/tests/format/js/test-declarations/angular_waitForAsync.js b/tests/format/js/test-declarations/angular_waitForAsync.js
new file mode 100644
--- /dev/null
+++ b/tests/format/js/test-declarations/angular_waitForAsync.js
@@ -0,0 +1,24 @@
+beforeEach(waitForAsync(() => {
+ // code
+}));
+
+afterAll(waitForAsync(() => {
+ console.log('Hello');
+}));
+
+it('should create the app', waitForAsync(() => {
+ //code
+}));
+
+it("does something really long and complicated so I have to write a very long name for the test", waitForAsync(() => {
+ // code
+}));
+
+it("does something really long and complicated so I have to write a very long name for the test", waitForAsync(() => new SSSSSSSSSSSSSSSSSSSSSSSSSSSSSSSSSSSSSSSSSSSSSSSSSSSSSSSSSSSSSSSSSSSSSSSSSSSSSSSS));
+
+/*
+* isTestCall(parent) should only be called when parent exists
+* and parent.type is CallExpression. This test makes sure that
+* no errors are thrown when calling isTestCall(parent)
+*/
+function x() { waitForAsync(() => {}) }
| Prettier does not recognize test-statement when using "waitForAsync" instead of "async"
**Prettier 2.5.1**
[Playground link](https://prettier.io/playground/#N4Igxg9gdgLgprEAuE8DOMAUByAZhCAAgCMBDAJ2wBpCB3UgSxgDEJyBBNATyjE0wCUhALwA+QsAA6UQoUhQMhfEWGFJIMuXUBuabLgAPAA5wwWZQIB0MCAFEAjgFdSAG0zrN6gdIC+AgdogVCAQRjAM0GjIoBTkELQAChQIUSiu9FxRwcTkpGAA1nAwAMqkALZwADIMUHDIuK5ocNm5BUXFRnk1AObIMOSOzSBwZcRwACbjE5WkUN3O3XCs5GWkMOFzyCCkjjZBIAAWMGUuAOoHTHBonWBwxSlMDABuTFxbYGhZIDVN5DAJuW6q3qjSGACs0AZij0XHAAIqOCDwEEuJrBTrkX5bMhjFz7IzkGowU4McYwA7IAAcAAZ0XEmqdckYtgSrnByE86sEnEi4ADQqltmgALS1CYTfbkOBOBhSgGkIGkFFokBNMoMPoDIZoGHwxHIpANVFDGCkYgkskUpAAJmC-UYLh6AGEIGVgSgrgBWfaOJoAFTNqSNKqegwAklAprBimBCWF2JHijAuLDlXAfD4gA)
<!-- prettier-ignore -->
```sh
--parser babel
```
**Input:**
<!-- prettier-ignore -->
```jsx
test('foo bar', waitForAsync(() => {
const foo = "bar";
expect(foo).toEqual("bar")
}));
```
**Output:**
<!-- prettier-ignore -->
```jsx
test(
"foo bar",
waitForAsync(() => {
const foo = "bar";
expect(foo).toEqual("bar");
})
);
```
**Expected behavior:**
<!-- prettier-ignore -->
```jsx
test("foo bar", waitForAsync(() => {
const foo = "bar";
expect(foo).toEqual("bar");
}));
```
In this case, Prettier does not recognize this test-statement and so, incorrectly adds newlines before each argument. The issue here seems to be the `waitForAsync`. When using `async` it works as expected. This is not a realistic fix however, since async is being deprecated by Angular.
| Probably requires a simple addition to the method `isAngularTestWrapper` (in file `/src/language-js/utils.js`):
```
function isAngularTestWrapper(node) {
return (
isCallExpression(node) &&
node.callee.type === "Identifier" &&
(node.callee.name === "async" ||
node.callee.name === "inject" ||
node.callee.name === "fakeAsync")
);
}
```
to
```
function isAngularTestWrapper(node) {
return (
isCallExpression(node) &&
node.callee.type === "Identifier" &&
(node.callee.name === "async" ||
node.callee.name === "inject" ||
node.callee.name === "fakeAsync" ||
node.callee.name === "waitForAsync")
);
}
```
| "2021-12-20T14:20:35Z" | 2.6 | [] | [
"tests/format/js/test-declarations/jsfmt.spec.js"
] | JavaScript | [] | [
"https://prettier.io/playground/#N4Igxg9gdgLgprEAuE8DOMAUByAZhCAAgCMBDAJ2wBpCB3UgSxgDEJyBBNATyjE0wCUhALwA+QsAA6UQoUhQMhfEWGFJIMuXUBuabLgAPAA5wwWZQIB0MCAFEAjgFdSAG0zrN6gdIC+AgdogVCAQRjAM0GjIoBTkELQAChQIUSiu9FxRwcTkpGAA1nAwAMqkALZwADIMUHDIuK5ocNm5BUXFRnk1AObIMOSOzSBwZcRwACbjE5WkUN3O3XCs5GWkMOFzyCCkjjZBIAAWMGUuAOoHTHBonWBwxSlMDABuTFxbYGhZIDVN5DAJuW6q3qjSGACs0AZij0XHAAIqOCDwEEuJrBTrkX5bMhjFz7IzkGowU4McYwA7IAAcAAZ0XEmqdckYtgSrnByE86sEnEi4ADQqltmgALS1CYTfbkOBOBhSgGkIGkFFokBNMoMPoDIZoGHwxHIpANVFDGCkYgkskUpAAJmC-UYLh6AGEIGVgSgrgBWfaOJoAFTNqSNKqegwAklAprBimBCWF2JHijAuLDlXAfD4gA"
] |
prettier/prettier | 12,017 | prettier__prettier-12017 | [
"10250"
] | c9c6d3364f8cf5140c3c16b44b92943464be7873 | diff --git a/src/language-js/comments.js b/src/language-js/comments.js
--- a/src/language-js/comments.js
+++ b/src/language-js/comments.js
@@ -27,6 +27,7 @@ const {
isObjectProperty,
getComments,
CommentCheckFlags,
+ markerForIfWithoutBlockAndSameLineComment,
} = require("./utils.js");
const { locStart, locEnd } = require("./loc.js");
@@ -206,7 +207,24 @@ function handleIfStatementComments({
if (precedingNode.type === "BlockStatement") {
addTrailingComment(precedingNode, comment);
} else {
- addDanglingComment(enclosingNode, comment);
+ const isSingleLineComment =
+ comment.type === "SingleLine" ||
+ comment.loc.start.line === comment.loc.end.line;
+ const isSameLineComment =
+ comment.loc.start.line === precedingNode.loc.start.line;
+ if (isSingleLineComment && isSameLineComment) {
+ // example:
+ // if (cond1) expr1; // comment A
+ // else if (cond2) expr2; // comment A
+ // else expr3;
+ addDanglingComment(
+ precedingNode,
+ comment,
+ markerForIfWithoutBlockAndSameLineComment
+ );
+ } else {
+ addDanglingComment(enclosingNode, comment);
+ }
}
return true;
}
diff --git a/src/language-js/printer-estree.js b/src/language-js/printer-estree.js
--- a/src/language-js/printer-estree.js
+++ b/src/language-js/printer-estree.js
@@ -28,6 +28,7 @@ const {
hasIgnoreComment,
isCallExpression,
isMemberExpression,
+ markerForIfWithoutBlockAndSameLineComment,
} = require("./utils.js");
const { locStart, locEnd } = require("./loc.js");
@@ -180,7 +181,7 @@ function printPathNoParens(path, options, print, args) {
// Babel extension.
case "EmptyStatement":
return "";
- case "ExpressionStatement":
+ case "ExpressionStatement": {
// Detect Flow and TypeScript directives
if (node.directive) {
return [printDirective(node.expression, options), semi];
@@ -200,11 +201,20 @@ function printPathNoParens(path, options, print, args) {
}
}
+ const danglingComment = printDanglingComments(
+ path,
+ options,
+ /** sameIndent */ true,
+ ({ marker }) => marker === markerForIfWithoutBlockAndSameLineComment
+ );
+
// Do not append semicolon after the only JSX element in a program
return [
print("expression"),
isTheOnlyJsxElementInMarkdown(options, path) ? "" : semi,
+ danglingComment ? [" ", danglingComment] : "",
];
+ }
// Babel non-standard node. Used for Closure-style type casts. See postprocess.js.
case "ParenthesizedExpression": {
const shouldHug =
diff --git a/src/language-js/utils.js b/src/language-js/utils.js
--- a/src/language-js/utils.js
+++ b/src/language-js/utils.js
@@ -1315,6 +1315,13 @@ function isEnabledHackPipeline(options) {
return Boolean(options.__isUsingHackPipeline);
}
+/**
+ * This is used as a marker for dangling comments.
+ */
+const markerForIfWithoutBlockAndSameLineComment = Symbol(
+ "ifWithoutBlockAndSameLineComment"
+);
+
module.exports = {
getFunctionParameters,
iterateFunctionParametersPath,
@@ -1379,4 +1386,5 @@ module.exports = {
hasComment,
getComments,
CommentCheckFlags,
+ markerForIfWithoutBlockAndSameLineComment,
};
| diff --git a/tests/format/js/if/__snapshots__/jsfmt.spec.js.snap b/tests/format/js/if/__snapshots__/jsfmt.spec.js.snap
--- a/tests/format/js/if/__snapshots__/jsfmt.spec.js.snap
+++ b/tests/format/js/if/__snapshots__/jsfmt.spec.js.snap
@@ -87,6 +87,59 @@ function f() {
================================================================================
`;
+exports[`expr_and_same_line_comments.js format 1`] = `
+====================================options=====================================
+parsers: ["babel", "flow", "typescript"]
+printWidth: 80
+ | printWidth
+=====================================input======================================
+if (a === 0) doSomething(); // comment A1
+else if (a === 1) doSomethingElse(); // comment B1
+else if (a === 2) doSomethingElse(); // comment C1
+
+if (a === 0) doSomething(); /* comment A2 */
+else if (a === 1) doSomethingElse(); /* comment B2 */
+else if (a === 2) doSomethingElse(); /* comment C2 */
+
+if (a === 0) expr; // comment A3
+else if (a === 1) expr; // comment B3
+else if (a === 2) expr; // comment C3
+
+if (a === 0) expr; /* comment A4 */
+else if (a === 1) expr; /* comment B4 */
+else if (a === 2) expr; /* comment C4 */
+
+if (a === 0) looooooooooooooooooooooooooooooooooooooooooooooooooooooooooooong; // comment A5
+else if (a === 1) looooooooooooooooooooooooooooooooooooooooooooooooooooooooooooong; // comment B5
+else if (a === 2) looooooooooooooooooooooooooooooooooooooooooooooooooooooooooooong; // comment C5
+
+=====================================output=====================================
+if (a === 0) doSomething(); // comment A1
+else if (a === 1) doSomethingElse(); // comment B1
+else if (a === 2) doSomethingElse(); // comment C1
+
+if (a === 0) doSomething(); /* comment A2 */
+else if (a === 1) doSomethingElse(); /* comment B2 */
+else if (a === 2) doSomethingElse(); /* comment C2 */
+
+if (a === 0) expr; // comment A3
+else if (a === 1) expr; // comment B3
+else if (a === 2) expr; // comment C3
+
+if (a === 0) expr; /* comment A4 */
+else if (a === 1) expr; /* comment B4 */
+else if (a === 2) expr; /* comment C4 */
+
+if (a === 0)
+ looooooooooooooooooooooooooooooooooooooooooooooooooooooooooooong; // comment A5
+else if (a === 1)
+ looooooooooooooooooooooooooooooooooooooooooooooooooooooooooooong; // comment B5
+else if (a === 2)
+ looooooooooooooooooooooooooooooooooooooooooooooooooooooooooooong; // comment C5
+
+================================================================================
+`;
+
exports[`if_comments.js format 1`] = `
====================================options=====================================
parsers: ["babel", "flow", "typescript"]
diff --git a/tests/format/js/if/expr_and_same_line_comments.js b/tests/format/js/if/expr_and_same_line_comments.js
new file mode 100644
--- /dev/null
+++ b/tests/format/js/if/expr_and_same_line_comments.js
@@ -0,0 +1,19 @@
+if (a === 0) doSomething(); // comment A1
+else if (a === 1) doSomethingElse(); // comment B1
+else if (a === 2) doSomethingElse(); // comment C1
+
+if (a === 0) doSomething(); /* comment A2 */
+else if (a === 1) doSomethingElse(); /* comment B2 */
+else if (a === 2) doSomethingElse(); /* comment C2 */
+
+if (a === 0) expr; // comment A3
+else if (a === 1) expr; // comment B3
+else if (a === 2) expr; // comment C3
+
+if (a === 0) expr; /* comment A4 */
+else if (a === 1) expr; /* comment B4 */
+else if (a === 2) expr; /* comment C4 */
+
+if (a === 0) looooooooooooooooooooooooooooooooooooooooooooooooooooooooooooong; // comment A5
+else if (a === 1) looooooooooooooooooooooooooooooooooooooooooooooooooooooooooooong; // comment B5
+else if (a === 2) looooooooooooooooooooooooooooooooooooooooooooooooooooooooooooong; // comment C5
| why the if comment is line breaked?
Is there any way to prevent line break?
**Prettier 2.2.1**
[Playground link](https://prettier.io/playground/#N4Igxg9gdgLgprEAuc0DOMAEMCGaDWAgpgLyYAUAlKQHybAC+AOlJFBtnvgEKkXUk6jFmw64CAYT5Va9ZlBYB6AFTKWmTMswABAA44ATjgC29AEYQIAGzg4oDTAEs0mALTY4HfUePrNilgAzAFdWGEdoDwxyZ2pgP0dAmLRqcSIZRUUnFxJczAAVA2C4WXyuQj84KzQSxPIAQljOAm4MrOdSPIAxHGqSwQKubkq+5vwJNuzOslCAEzhAxyg4WdKuCRZ5EAAaEAhdcPRkUEMDCAB3AAVDBDRkEF7znABPO92zIzB8OBgAZX0wEsAObIGBFOC7ODGMwreazAAydiBwRwQLgXQgBmMOBg4SgIJQOGCMAgOxAAAsYMYrAB1cmOeBoAFwX63BmOABuDOe90cQKgmLgZKWNQMMEuRiB2OQgV6NV2ACs0AAPbifb5-ExweFLIVIWV9RUq37AmwARWCEHgMrlEJA3lF9zMOBhVjJugMSxgNMcsxg5OQAA4AAy7D0QGo0oy6e6PF5vEAAR0t8Al+zuKA9njgBg5Qt2BjgycchYlqOl+ttuxqxkcNsNIDQprgFqterBxV2uDMPr9AaQACYu0ZHFZgRIIMYKyBPABWMnBGplMwZg3ykAc4oASSg81gvzAnoOhF3vxgzxs9ZqDAYQA)
```sh
--html-whitespace-sensitivity ignore
--parser babel
--prose-wrap always
--quote-props preserve
--no-semi
--single-quote
```
**Input:**
```jsx
const taskA = () => {}
const taskB = () => {}
const taskC = () => {}
/**
* @param {boolean} is - test param
*/
function test(is) {
if(is) taskA() // is === True => TaskA
else if(!is) taskB() // is === False => TaskB
else taskC() // is === undefined => TaskC
}
```
**Output:**
```jsx
const taskA = () => {}
const taskB = () => {}
const taskC = () => {}
/**
* @param {boolean} is - test param
*/
function test(is) {
if (is) taskA()
// is === True => TaskA
else if (!is) taskB()
// is === False => TaskB
else taskC() // is === undefined => TaskC
}
```
**Expected behavior:**
```jsx
const taskA = () => {}
const taskB = () => {}
const taskC = () => {}
/**
* @param {boolean} is - test param
*/
function test(is) {
if (is) taskA() // is === True => TaskA
else if (!is) taskB() // is === False => TaskB
else taskC() // is === undefined => TaskC
}
```
| The intention was to support these often-requested cases:
```js
function test(is) {
if (is) {
taskA()
}
// case 1 "comment before else"
else if (!is) {
taskB()
} // case 2 "comment after }", for things like "// end if ..."
else taskC()
}
```
This issue might be an unintended side-effect of fixing that. | "2022-01-01T17:43:01Z" | 2.6 | [] | [
"tests/format/js/if/jsfmt.spec.js"
] | JavaScript | [] | [
"https://prettier.io/playground/#N4Igxg9gdgLgprEAuc0DOMAEMCGaDWAgpgLyYAUAlKQHybAC+AOlJFBtnvgEKkXUk6jFmw64CAYT5Va9ZlBYB6AFTKWmTMswABAA44ATjgC29AEYQIAGzg4oDTAEs0mALTY4HfUePrNilgAzAFdWGEdoDwxyZ2pgP0dAmLRqcSIZRUUnFxJczAAVA2C4WXyuQj84KzQSxPIAQljOAm4MrOdSPIAxHGqSwQKubkq+5vwJNuzOslCAEzhAxyg4WdKuCRZ5EAAaEAhdcPRkUEMDCAB3AAVDBDRkEF7znABPO92zIzB8OBgAZX0wEsAObIGBFOC7ODGMwreazAAydiBwRwQLgXQgBmMOBg4SgIJQOGCMAgOxAAAsYMYrAB1cmOeBoAFwX63BmOABuDOe90cQKgmLgZKWNQMMEuRiB2OQgV6NV2ACs0AAPbifb5-ExweFLIVIWV9RUq37AmwARWCEHgMrlEJA3lF9zMOBhVjJugMSxgNMcsxg5OQAA4AAy7D0QGo0oy6e6PF5vEAAR0t8Al+zuKA9njgBg5Qt2BjgycchYlqOl+ttuxqxkcNsNIDQprgFqterBxV2uDMPr9AaQACYu0ZHFZgRIIMYKyBPABWMnBGplMwZg3ykAc4oASSg81gvzAnoOhF3vxgzxs9ZqDAYQA"
] |
prettier/prettier | 12,075 | prettier__prettier-12075 | [
"12073"
] | dd3e1a49e04b7fd147dfbccb2489cc5967304491 | diff --git a/src/language-js/comments.js b/src/language-js/comments.js
--- a/src/language-js/comments.js
+++ b/src/language-js/comments.js
@@ -68,6 +68,7 @@ function handleOwnLineComment(context) {
handleAssignmentPatternComments,
handleMethodNameComments,
handleLabeledStatementComments,
+ handleBreakAndContinueStatementComments,
].some((fn) => fn(context));
}
@@ -91,6 +92,7 @@ function handleEndOfLineComment(context) {
handleOnlyComments,
handleTypeAliasComments,
handleVariableDeclaratorComments,
+ handleBreakAndContinueStatementComments,
].some((fn) => fn(context));
}
| diff --git a/tests/config/format-test.js b/tests/config/format-test.js
--- a/tests/config/format-test.js
+++ b/tests/config/format-test.js
@@ -39,6 +39,7 @@ const unstableTests = new Map(
["flow/no-semi/comments.js", (options) => options.semi === false],
"typescript/prettier-ignore/mapped-types.ts",
"js/comments/html-like/comment.js",
+ "js/for/continue-and-break-comment-without-blocks.js",
].map((fixture) => {
const [file, isUnstable = () => true] = Array.isArray(fixture)
? fixture
diff --git a/tests/format/js/for/__snapshots__/jsfmt.spec.js.snap b/tests/format/js/for/__snapshots__/jsfmt.spec.js.snap
--- a/tests/format/js/for/__snapshots__/jsfmt.spec.js.snap
+++ b/tests/format/js/for/__snapshots__/jsfmt.spec.js.snap
@@ -74,6 +74,709 @@ for (x of y);
================================================================================
`;
+exports[`continue-and-break-comment-1.js format 1`] = `
+====================================options=====================================
+parsers: ["babel", "flow", "typescript"]
+printWidth: 80
+ | printWidth
+=====================================input======================================
+for(;;) {
+ continue // comment
+ ;
+}
+
+for (;;) {
+ break // comment
+ ;
+}
+
+for (const f of []) {
+ continue // comment
+ ;
+}
+
+for (const f of []) {
+ break // comment
+ ;
+}
+
+for (const f in {}) {
+ continue // comment
+ ;
+}
+
+for (const f in {}) {
+ break // comment
+ ;
+}
+
+while(true) {
+ continue // comment
+ ;
+}
+
+while (true) {
+ break // comment
+ ;
+}
+
+do {
+ continue // comment
+ ;
+} while(true);
+
+
+do {
+ break // comment
+ ;
+} while(true);
+
+label1: for (;;) {
+ continue label1 // comment
+ ;
+}
+
+label2: {
+ break label2 // comment
+ ;
+};
+
+for(;;) {
+ continue /* comment */
+ ;
+}
+
+for (;;) {
+ break /* comment */
+ ;
+}
+
+for (const f of []) {
+ continue /* comment */
+ ;
+}
+
+for (const f of []) {
+ break /* comment */
+ ;
+}
+
+for (const f in {}) {
+ continue /* comment */
+ ;
+}
+
+for (const f in {}) {
+ break /* comment */
+ ;
+}
+
+while(true) {
+ continue /* comment */
+ ;
+}
+
+while (true) {
+ break /* comment */
+ ;
+}
+
+do {
+ continue /* comment */
+ ;
+} while(true);
+
+
+do {
+ break /* comment */
+ ;
+} while(true);
+
+label1: for (;;) {
+ continue label1 /* comment */
+ ;
+}
+
+label2: {
+ break label2 /* comment */
+ ;
+};
+
+=====================================output=====================================
+for (;;) {
+ continue; // comment
+}
+
+for (;;) {
+ break; // comment
+}
+
+for (const f of []) {
+ continue; // comment
+}
+
+for (const f of []) {
+ break; // comment
+}
+
+for (const f in {}) {
+ continue; // comment
+}
+
+for (const f in {}) {
+ break; // comment
+}
+
+while (true) {
+ continue; // comment
+}
+
+while (true) {
+ break; // comment
+}
+
+do {
+ continue; // comment
+} while (true);
+
+do {
+ break; // comment
+} while (true);
+
+label1: for (;;) {
+ continue label1; // comment
+}
+
+label2: {
+ break label2; // comment
+}
+
+for (;;) {
+ continue; /* comment */
+}
+
+for (;;) {
+ break; /* comment */
+}
+
+for (const f of []) {
+ continue; /* comment */
+}
+
+for (const f of []) {
+ break; /* comment */
+}
+
+for (const f in {}) {
+ continue; /* comment */
+}
+
+for (const f in {}) {
+ break; /* comment */
+}
+
+while (true) {
+ continue; /* comment */
+}
+
+while (true) {
+ break; /* comment */
+}
+
+do {
+ continue; /* comment */
+} while (true);
+
+do {
+ break; /* comment */
+} while (true);
+
+label1: for (;;) {
+ continue label1 /* comment */;
+}
+
+label2: {
+ break label2 /* comment */;
+}
+
+================================================================================
+`;
+
+exports[`continue-and-break-comment-2.js format 1`] = `
+====================================options=====================================
+parsers: ["babel", "flow", "typescript"]
+printWidth: 80
+ | printWidth
+=====================================input======================================
+for(;;) {
+ continue
+ // comment
+ ;
+}
+
+for (;;) {
+ break
+ // comment
+ ;
+}
+
+for (const f of []) {
+ continue
+ // comment
+ ;
+}
+
+for (const f of []) {
+ break
+ // comment
+ ;
+}
+
+for (const f in {}) {
+ continue
+ // comment
+ ;
+}
+
+for (const f in {}) {
+ break
+ // comment
+ ;
+}
+
+while(true) {
+ continue
+ // comment
+ ;
+}
+
+while (true) {
+ break
+ // comment
+ ;
+}
+
+do {
+ continue
+ // comment
+ ;
+} while(true);
+
+
+do {
+ break
+ // comment
+ ;
+} while(true);
+
+label1: for (;;) {
+ continue label1
+ // comment
+ ;
+}
+
+label2: {
+ break label2
+ // comment
+ ;
+};
+
+for(;;) {
+ continue
+ /* comment */
+ ;
+}
+
+for (;;) {
+ break
+ /* comment */
+ ;
+}
+
+for (const f of []) {
+ continue
+ /* comment */
+ ;
+}
+
+for (const f of []) {
+ break
+ /* comment */
+ ;
+}
+
+for (const f in {}) {
+ continue
+ /* comment */
+ ;
+}
+
+for (const f in {}) {
+ break
+ /* comment */
+ ;
+}
+
+while(true) {
+ continue
+ /* comment */
+ ;
+}
+
+while (true) {
+ break
+ /* comment */
+ ;
+}
+
+do {
+ continue
+ /* comment */
+ ;
+} while(true);
+
+
+do {
+ break
+ /* comment */
+ ;
+} while(true);
+
+label1: for (;;) {
+ continue label1
+ /* comment */
+ ;
+}
+
+label2: {
+ break label2
+ /* comment */
+ ;
+};
+
+=====================================output=====================================
+for (;;) {
+ continue;
+ // comment
+}
+
+for (;;) {
+ break;
+ // comment
+}
+
+for (const f of []) {
+ continue;
+ // comment
+}
+
+for (const f of []) {
+ break;
+ // comment
+}
+
+for (const f in {}) {
+ continue;
+ // comment
+}
+
+for (const f in {}) {
+ break;
+ // comment
+}
+
+while (true) {
+ continue;
+ // comment
+}
+
+while (true) {
+ break;
+ // comment
+}
+
+do {
+ continue;
+ // comment
+} while (true);
+
+do {
+ break;
+ // comment
+} while (true);
+
+label1: for (;;) {
+ continue label1;
+ // comment
+}
+
+label2: {
+ break label2;
+ // comment
+}
+
+for (;;) {
+ continue;
+ /* comment */
+}
+
+for (;;) {
+ break;
+ /* comment */
+}
+
+for (const f of []) {
+ continue;
+ /* comment */
+}
+
+for (const f of []) {
+ break;
+ /* comment */
+}
+
+for (const f in {}) {
+ continue;
+ /* comment */
+}
+
+for (const f in {}) {
+ break;
+ /* comment */
+}
+
+while (true) {
+ continue;
+ /* comment */
+}
+
+while (true) {
+ break;
+ /* comment */
+}
+
+do {
+ continue;
+ /* comment */
+} while (true);
+
+do {
+ break;
+ /* comment */
+} while (true);
+
+label1: for (;;) {
+ continue label1;
+ /* comment */
+}
+
+label2: {
+ break label2;
+ /* comment */
+}
+
+================================================================================
+`;
+
+exports[`continue-and-break-comment-without-blocks.js format 1`] = `
+====================================options=====================================
+parsers: ["babel", "flow", "typescript"]
+printWidth: 80
+ | printWidth
+=====================================input======================================
+for(;;) continue
+// comment
+;
+
+for (;;) break
+// comment
+;
+
+for (const f of []) continue
+// comment
+;
+
+for (const f of []) break
+// comment
+;
+
+for (const f in {}) continue
+// comment
+;
+
+for (const f in {}) break
+// comment
+;
+
+for(;;) continue // comment
+;
+
+for (;;) break // comment
+;
+
+for (const f of []) continue // comment
+;
+
+for (const f of []) break // comment
+;
+
+for (const f in {}) continue // comment
+;
+
+for (const f in {}) break // comment
+;
+
+for(;;) continue /* comment */
+;
+
+for (;;) break /* comment */
+;
+
+for (const f of []) continue /* comment */
+;
+
+for (const f of []) break /* comment */
+;
+
+for (const f in {}) continue /* comment */
+;
+
+for (const f in {}) break /* comment */
+;
+
+for(;;) continue
+/* comment */
+;
+
+for (;;) break
+/* comment */
+;
+
+for (const f of []) continue
+/* comment */
+;
+
+for (const f of []) break
+/* comment */
+;
+
+for (const f in {}) continue
+/* comment */
+;
+
+for (const f in {}) break
+/* comment */
+;
+
+label1: for (;;) continue label1 /* comment */
+;
+
+label1: for (;;) continue label1
+/* comment */
+;
+
+label1: for (;;) continue label1 // comment
+;
+
+label1: for (;;) continue label1
+// comment
+;
+
+=====================================output=====================================
+for (;;)
+ continue;
+ // comment
+
+for (;;)
+ break;
+ // comment
+
+for (const f of [])
+ continue;
+ // comment
+
+for (const f of [])
+ break;
+ // comment
+
+for (const f in {})
+ continue;
+ // comment
+
+for (const f in {})
+ break;
+ // comment
+
+for (;;)
+ continue; // comment
+
+for (;;)
+ break; // comment
+
+for (const f of [])
+ continue; // comment
+
+for (const f of [])
+ break; // comment
+
+for (const f in {})
+ continue; // comment
+
+for (const f in {})
+ break; // comment
+
+for (;;) continue; /* comment */
+
+for (;;) break; /* comment */
+
+for (const f of []) continue; /* comment */
+
+for (const f of []) break; /* comment */
+
+for (const f in {}) continue; /* comment */
+
+for (const f in {}) break; /* comment */
+
+for (;;)
+ continue;
+ /* comment */
+
+for (;;)
+ break;
+ /* comment */
+
+for (const f of [])
+ continue;
+ /* comment */
+
+for (const f of [])
+ break;
+ /* comment */
+
+for (const f in {})
+ continue;
+ /* comment */
+
+for (const f in {})
+ break;
+ /* comment */
+
+label1: for (;;) continue label1 /* comment */;
+
+label1: for (;;)
+ continue label1;
+ /* comment */
+
+label1: for (;;)
+ continue label1; // comment
+
+label1: for (;;)
+ continue label1;
+ // comment
+
+================================================================================
+`;
+
exports[`for.js format 1`] = `
====================================options=====================================
parsers: ["babel", "flow", "typescript"]
diff --git a/tests/format/js/for/continue-and-break-comment-1.js b/tests/format/js/for/continue-and-break-comment-1.js
new file mode 100644
--- /dev/null
+++ b/tests/format/js/for/continue-and-break-comment-1.js
@@ -0,0 +1,121 @@
+for(;;) {
+ continue // comment
+ ;
+}
+
+for (;;) {
+ break // comment
+ ;
+}
+
+for (const f of []) {
+ continue // comment
+ ;
+}
+
+for (const f of []) {
+ break // comment
+ ;
+}
+
+for (const f in {}) {
+ continue // comment
+ ;
+}
+
+for (const f in {}) {
+ break // comment
+ ;
+}
+
+while(true) {
+ continue // comment
+ ;
+}
+
+while (true) {
+ break // comment
+ ;
+}
+
+do {
+ continue // comment
+ ;
+} while(true);
+
+
+do {
+ break // comment
+ ;
+} while(true);
+
+label1: for (;;) {
+ continue label1 // comment
+ ;
+}
+
+label2: {
+ break label2 // comment
+ ;
+};
+
+for(;;) {
+ continue /* comment */
+ ;
+}
+
+for (;;) {
+ break /* comment */
+ ;
+}
+
+for (const f of []) {
+ continue /* comment */
+ ;
+}
+
+for (const f of []) {
+ break /* comment */
+ ;
+}
+
+for (const f in {}) {
+ continue /* comment */
+ ;
+}
+
+for (const f in {}) {
+ break /* comment */
+ ;
+}
+
+while(true) {
+ continue /* comment */
+ ;
+}
+
+while (true) {
+ break /* comment */
+ ;
+}
+
+do {
+ continue /* comment */
+ ;
+} while(true);
+
+
+do {
+ break /* comment */
+ ;
+} while(true);
+
+label1: for (;;) {
+ continue label1 /* comment */
+ ;
+}
+
+label2: {
+ break label2 /* comment */
+ ;
+};
diff --git a/tests/format/js/for/continue-and-break-comment-2.js b/tests/format/js/for/continue-and-break-comment-2.js
new file mode 100644
--- /dev/null
+++ b/tests/format/js/for/continue-and-break-comment-2.js
@@ -0,0 +1,145 @@
+for(;;) {
+ continue
+ // comment
+ ;
+}
+
+for (;;) {
+ break
+ // comment
+ ;
+}
+
+for (const f of []) {
+ continue
+ // comment
+ ;
+}
+
+for (const f of []) {
+ break
+ // comment
+ ;
+}
+
+for (const f in {}) {
+ continue
+ // comment
+ ;
+}
+
+for (const f in {}) {
+ break
+ // comment
+ ;
+}
+
+while(true) {
+ continue
+ // comment
+ ;
+}
+
+while (true) {
+ break
+ // comment
+ ;
+}
+
+do {
+ continue
+ // comment
+ ;
+} while(true);
+
+
+do {
+ break
+ // comment
+ ;
+} while(true);
+
+label1: for (;;) {
+ continue label1
+ // comment
+ ;
+}
+
+label2: {
+ break label2
+ // comment
+ ;
+};
+
+for(;;) {
+ continue
+ /* comment */
+ ;
+}
+
+for (;;) {
+ break
+ /* comment */
+ ;
+}
+
+for (const f of []) {
+ continue
+ /* comment */
+ ;
+}
+
+for (const f of []) {
+ break
+ /* comment */
+ ;
+}
+
+for (const f in {}) {
+ continue
+ /* comment */
+ ;
+}
+
+for (const f in {}) {
+ break
+ /* comment */
+ ;
+}
+
+while(true) {
+ continue
+ /* comment */
+ ;
+}
+
+while (true) {
+ break
+ /* comment */
+ ;
+}
+
+do {
+ continue
+ /* comment */
+ ;
+} while(true);
+
+
+do {
+ break
+ /* comment */
+ ;
+} while(true);
+
+label1: for (;;) {
+ continue label1
+ /* comment */
+ ;
+}
+
+label2: {
+ break label2
+ /* comment */
+ ;
+};
diff --git a/tests/format/js/for/continue-and-break-comment-without-blocks.js b/tests/format/js/for/continue-and-break-comment-without-blocks.js
new file mode 100644
--- /dev/null
+++ b/tests/format/js/for/continue-and-break-comment-without-blocks.js
@@ -0,0 +1,97 @@
+for(;;) continue
+// comment
+;
+
+for (;;) break
+// comment
+;
+
+for (const f of []) continue
+// comment
+;
+
+for (const f of []) break
+// comment
+;
+
+for (const f in {}) continue
+// comment
+;
+
+for (const f in {}) break
+// comment
+;
+
+for(;;) continue // comment
+;
+
+for (;;) break // comment
+;
+
+for (const f of []) continue // comment
+;
+
+for (const f of []) break // comment
+;
+
+for (const f in {}) continue // comment
+;
+
+for (const f in {}) break // comment
+;
+
+for(;;) continue /* comment */
+;
+
+for (;;) break /* comment */
+;
+
+for (const f of []) continue /* comment */
+;
+
+for (const f of []) break /* comment */
+;
+
+for (const f in {}) continue /* comment */
+;
+
+for (const f in {}) break /* comment */
+;
+
+for(;;) continue
+/* comment */
+;
+
+for (;;) break
+/* comment */
+;
+
+for (const f of []) continue
+/* comment */
+;
+
+for (const f of []) break
+/* comment */
+;
+
+for (const f in {}) continue
+/* comment */
+;
+
+for (const f in {}) break
+/* comment */
+;
+
+label1: for (;;) continue label1 /* comment */
+;
+
+label1: for (;;) continue label1
+/* comment */
+;
+
+label1: for (;;) continue label1 // comment
+;
+
+label1: for (;;) continue label1
+// comment
+;
diff --git a/tests/format/misc/errors/typescript/__snapshots__/jsfmt.spec.js.snap b/tests/format/misc/errors/typescript/__snapshots__/jsfmt.spec.js.snap
--- a/tests/format/misc/errors/typescript/__snapshots__/jsfmt.spec.js.snap
+++ b/tests/format/misc/errors/typescript/__snapshots__/jsfmt.spec.js.snap
@@ -23,8 +23,6 @@ exports[`invalid-jsx-1.ts [typescript] format 1`] = `
4 |"
`;
-exports[`issue-8773.ts [typescript] format 1`] = `"Comment \\"comment\\" was not printed. Please report this error!"`;
-
exports[`module-attributes-static.ts [typescript] format 1`] = `
"';' expected. (1:28)
> 1 | import foo from \\"foo.json\\" with type: \\"json\\";
diff --git a/tests/format/misc/errors/typescript/issue-8773.ts b/tests/format/misc/errors/typescript/issue-8773.ts
deleted file mode 100644
--- a/tests/format/misc/errors/typescript/issue-8773.ts
+++ /dev/null
@@ -1,5 +0,0 @@
-// prettier-ignore
-foo + foo;
-
-continue // comment
-;
| Prettier [error] -> Comment "' + comment.value.trim() + '" was not printed..."
[Playground Link](https://prettier.io/playground/#N4Igxg9gdgLgprEAuEBuABAWwgEwK4A2cAdHAB4AOEATjAM7F0AWcBBAomXGOgLzoAzPFDAwAltHTNWHLmAAUkTJgCGUHAEoAOlHTpg6AG4rq6AmKhw6fKSzak5iiMrU4ANMDpiisJDGp4cAC+GsQQeDAUEYwU5jDyAPRaOgnauugYBBAA5vIARhAEOE4u6hppehgCNOjyxqZi6BZmFlYVevotlnQA2mIAusT+Ypg6HRlNArUAhObdff0a6JCwFoHoCQlSANZiFOh5BGrbXVZjHZk58nNWC+16QeePUCBuIBAU4tB0yKAm1BAAO4ABRMCB+KBUBEBKgAnj83nlqCowNs4DAAMoqTBwAAyrWQAihdDgiORqPRGIoKIs2WQ-kCbzgmDycBwODZuLU2TwKmycAAYjRVDBxFA6ZCIhBXiAmDBMAQAOpMMTwOjUsBwDHg1ViQyq2HIcB0BEgCwk2jA5HZVSE4mkkAAKzoZAxtKIAEU8BB4HaCCS3tTqBajXkVKyCDKKNQLDBFWIcDAmMgABwABkDAJJiuRFCN0ascGohjgMoAjt74FaPhCQCo6ABaSxstky6hwCtidtWvm2pBE-0OkmYMT0gJD91wL0+0v9+1vGDh+OJ5NIABMC+R3lpAGFnH2QFYAKwyvAkgAq4YhA4DIEMgQAkuoEJiwDHPgBBdQYmCwoh+kkgiCIA)
**Input**
```
$ prettier someScript.js --check
```
`someScript.js`
```
; module.exports.shellExec = function shellExec(command)
{ var lines = shell.exec(command,{silent:true}).output.split(/\n/)
; log(bold(command))
; for (var i in lines)
{ lines[i].trim
; if (!lines[i]) continue // skip blank lines
; log(lines[i])
}
}
```
**Output**
```
Checking formatting...
someScript.js [error] someScript.js: Error: Comment "skip blank lines" was not printed. Please report this error!
[error] at Object.ensureAllCommentsPrinted (/usr/local/Cellar/prettier/2.5.1/libexec/lib/node_modules/prettier/index.js:13824:13)
[error] at coreFormat (/usr/local/Cellar/prettier/2.5.1/libexec/lib/node_modules/prettier/index.js:14538:14)
[error] at formatWithCursor$1 (/usr/local/Cellar/prettier/2.5.1/libexec/lib/node_modules/prettier/index.js:14765:14)
[error] at Object.formatWithCursor (/usr/local/Cellar/prettier/2.5.1/libexec/lib/node_modules/prettier/index.js:60959:12)
[error] at format$1 (/usr/local/Cellar/prettier/2.5.1/libexec/lib/node_modules/prettier/bin-prettier.js:18001:21)
[error] at Object.formatFiles$1 [as formatFiles] (/usr/local/Cellar/prettier/2.5.1/libexec/lib/node_modules/prettier/bin-prettier.js:18115:16)
[error] at async main (/usr/local/Cellar/prettier/2.5.1/libexec/lib/node_modules/prettier/bin-prettier.js:20216:5)
[error] at async Object.run (/usr/local/Cellar/prettier/2.5.1/libexec/lib/node_modules/prettier/bin-prettier.js:20159:5)
All matched files use Prettier code style!
```
**Expected Behavior**
```
[warn] someScript.js
[warn] Code style issues found in the above file(s). Forgot to run Prettier?
```
On prettier version 2.5.1.
This is my first issue in this repo, so please let me know if there's something missing from this bug report.
| Please follow the issue template, i.e. include a reproduction in the Prettier Playground. Here it is: [Playground link](https://prettier.io/playground/#N4Igxg9gdgLgprEAuEBuABAWwgEwK4A2cAdHAB4AOEATjAM7F0AWcBBAomXGOgLzoAzPFDAwAltHTNWHLmAAUkTJgCGUHAEoAOlHTpg6AG4rq6AmKhw6fKSzak5iiMrU4ANMDpiisJDGp4cAC+GsQQeDAUEYwU5jDyAPRaOgnauugYBBAA5vIARhAEOE4u6hppehgCNOjyxqZi6BZmFlYVevotlnQA2mIAusT+Ypg6HRlNArUAhObdff0a6JCwFoHoCQlSANZiFOh5BGrbXVZjHZk58nNWC+16QeePUCBuIBAU4tB0yKAm1BAAO4ABRMCB+KBUBEBKgAnj83nlqCowNs4DAAMoqTBwAAyrWQAihdDgiORqPRGIoKIs2WQ-kCbzgmDycBwODZuLU2TwKmycAAYjRVDBxFA6ZCIhBXiAmDBMAQAOpMMTwOjUsBwDHg1ViQyq2HIcB0BEgCwk2jA5HZVSE4mkkAAKzoZAxtKIAEU8BB4HaCCS3tTqBajXkVKyCDKKNQLDBFWIcDAmMgABwABkDAJJiuRFCN0ascGohjgMoAjt74FaPhCQCo6ABaSxstky6hwCtidtWvm2pBE-0OkmYMT0gJD91wL0+0v9+1vGDh+OJ5NIABMC+R3lpAGFnH2QFYAKwyvAkgAq4YhA4DIEMgQAkuoEJiwDHPgBBdQYmCwoh+kkgiCIA).
Replacing
```diff
- ; if (!lines[i]) continue // skip blank lines
+ ; if (!lines[i]) continue; // skip blank lines
```
fixes the error. [Playground link](https://prettier.io/playground/#N4Igxg9gdgLgprEAuEBuABAWwgEwK4A2cAdHAB4AOEATjAM7F0AWcBBAomXGOgLzoAzPFDAwAltHTNWHLmAAUkTJgCGUHAEoAOlHTpg6AG4rq6AmKhw6fKSzak5iiMrU4ANMDpiisJDGp4cAC+GsQQeDAUEYwU5jDyAPRaOgnauugYBBAA5vIARhAEOE4u6hppehgCNOjyxqZi6BZmFlYVevotlnQA2mIAusT+Ypg6HRlNArUAhObdff0a6JCwFoHoCQlSANZiFOh5BGrbXVZjHZk58nNWC+16QeePUCBuIBAU4tB0yKAm1BAAO4ABRMCB+KBUBEBKgAnj83nlqCowNs4DAAMoqTBwAAyrWQAihdDgiORqPRGIoKIs2WQ-kCbzgmDycBwODZuLU2TwKmycAAYjRVDBxFA6ZCIhBXiAmDBMAQAOpMMTwOjUsBwDHg1ViQyq2HIcB0BEgCwk2jA5HZVSE4mkkAAKzoZAxtKIAEU8BB4HaCCS3tTqBajXkVKyCDKKNQLDBFWIcDAmMgABwABkDAJJiuRFCN0ascGohjgMoAjt74FaPhCQCo6ABaSxstky6hwCtidtWvm2pBE-0OkmYMT0gJD91wL0+0v9+1vGDh+OJ5NIABMC+R3lpAGFnH2QFYAKwyvAkgAq4YhA4DIEMgQAkuoEJiwDHPgBBdQYmCwoh+kkgiCIA).
Thank you for the information. I was able to resolve the issue but just wanted to make sure the error was reported!
Thanks! Minimal repro ([Playground](https://prettier.io/playground/#N4Igxg9gdgLgprEAuEAzCAnABACgNx4CUWksAllAK5xYD0tJEAtkwjADpR4gA0IEABxhloAZ2SgAhhgwQA7gAVpCcSkkAbOZICe4vgCMMksAGs4MAMqTWAGQpxkqDaLgGjp8xYHGKAc2QwGNR8cEz6cAAmEZE2klC+lJK+cABimEySMMLxyCCSlDAQvCAAFjBM6gDqJWTwot5gcBYqtWQAbrXauWCieiAULhgwCka+GY7OriAAVqIAHhZ+6nAAipQQ8BPqLnzeGIO5+pLh6sUCGBQwlWQRMCXIABwADLuyLpVGArnncINtDnwAI7reAjQSqPKiAC0UDgkUixQwcGBZCRIyS4yQTm2UxcTDIASCuKWqxBDixkz4MGO11u9yQACYqUYyOo-ABhZiYkC-ACsxUoLgAKsdVNidiA2tQAJJQaKwCxgC5CACCcosMG0yy2LgAvrqgA)):
```js
for (;;) continue // comment
;
```
Parser = flow, babel*, typescript, espree
Looks like it’s to do with an empty statement following `continue // comment \n`. Removing `;` on the following line or replacing it with `a;` prevents the error. | "2022-01-11T18:13:22Z" | 2.6 | [
"tests/format/misc/errors/typescript/jsfmt.spec.js"
] | [
"tests/format/js/for/jsfmt.spec.js"
] | JavaScript | [] | [
"https://prettier.io/playground/#N4Igxg9gdgLgprEAuEBuABAWwgEwK4A2cAdHAB4AOEATjAM7F0AWcBBAomXGOgLzoAzPFDAwAltHTNWHLmAAUkTJgCGUHAEoAOlHTpg6AG4rq6AmKhw6fKSzak5iiMrU4ANMDpiisJDGp4cAC+GsQQeDAUEYwU5jDyAPRaOgnauugYBBAA5vIARhAEOE4u6hppehgCNOjyxqZi6BZmFlYVevotlnQA2mIAusT+Ypg6HRlNArUAhObdff0a6JCwFoHoCQlSANZiFOh5BGrbXVZjHZk58nNWC+16QeePUCBuIBAU4tB0yKAm1BAAO4ABRMCB+KBUBEBKgAnj83nlqCowNs4DAAMoqTBwAAyrWQAihdDgiORqPRGIoKIs2WQ-kCbzgmDycBwODZuLU2TwKmycAAYjRVDBxFA6ZCIhBXiAmDBMAQAOpMMTwOjUsBwDHg1ViQyq2HIcB0BEgCwk2jA5HZVSE4mkkAAKzoZAxtKIAEU8BB4HaCCS3tTqBajXkVKyCDKKNQLDBFWIcDAmMgABwABkDAJJiuRFCN0ascGohjgMoAjt74FaPhCQCo6ABaSxstky6hwCtidtWvm2pBE-0OkmYMT0gJD91wL0+0v9+1vGDh+OJ5NIABMC+R3lpAGFnH2QFYAKwyvAkgAq4YhA4DIEMgQAkuoEJiwDHPgBBdQYmCwoh+kkgiCIA"
] |
prettier/prettier | 12,113 | prettier__prettier-12113 | [
"12114"
] | 53da86c34dd1c1e1241e85c57469100f2467fcec | diff --git a/src/language-html/syntax-vue.js b/src/language-html/syntax-vue.js
--- a/src/language-html/syntax-vue.js
+++ b/src/language-html/syntax-vue.js
@@ -40,8 +40,13 @@ function parseVueFor(value) {
if (!inMatch) {
return;
}
+
const res = {};
res.for = inMatch[3].trim();
+ if (!res.for) {
+ return;
+ }
+
const alias = inMatch[1].trim().replace(stripParensRE, "");
const iteratorMatch = alias.match(forIteratorRE);
if (iteratorMatch) {
@@ -54,10 +59,18 @@ function parseVueFor(value) {
res.alias = alias;
}
+ const left = [res.alias, res.iterator1, res.iterator2];
+ if (
+ left.some(
+ (part, index) =>
+ !part && (index === 0 || left.slice(index + 1).some(Boolean))
+ )
+ ) {
+ return;
+ }
+
return {
- left: `${[res.alias, res.iterator1, res.iterator2]
- .filter(Boolean)
- .join(",")}`,
+ left: left.filter(Boolean).join(","),
operator: inMatch[2],
right: res.for,
};
| diff --git a/tests/format/vue/invalid/__snapshots__/jsfmt.spec.js.snap b/tests/format/vue/invalid/__snapshots__/jsfmt.spec.js.snap
new file mode 100644
--- /dev/null
+++ b/tests/format/vue/invalid/__snapshots__/jsfmt.spec.js.snap
@@ -0,0 +1,52 @@
+// Jest Snapshot v1, https://goo.gl/fbAQLP
+
+exports[`v-for.vue format 1`] = `
+====================================options=====================================
+parsers: ["vue"]
+printWidth: 80
+ | printWidth
+=====================================input======================================
+<template>
+ <div>
+ <div
+ v-for=" _ in "
+ v-for=" in _ "
+ v-for=" in "
+ v-for=" _, in a "
+ v-for=" ,_ in a "
+
+ v-for=" a, b, in a "
+ v-for=" a, , c in a "
+
+ v-for=" , b, c in a "
+ v-for=" a, b, in a "
+
+ v-for=" , b, c in a "
+ v-for=" a, , c in a "
+ v-for=" (,a,b) of 'abcd' "
+ ></div>
+ </div>
+</template>
+
+=====================================output=====================================
+<template>
+ <div>
+ <div
+ v-for=" _ in "
+ v-for=" in _ "
+ v-for=" in "
+ v-for="_ in a"
+ v-for=" ,_ in a "
+ v-for="(a, b) in a"
+ v-for=" a, , c in a "
+ v-for=" , b, c in a "
+ v-for="(a, b) in a"
+ v-for=" , b, c in a "
+ v-for=" a, , c in a "
+ v-for=" (,a,b) of 'abcd' "
+ ></div>
+ </div>
+</template>
+
+================================================================================
+`;
diff --git a/tests/format/vue/invalid/jsfmt.spec.js b/tests/format/vue/invalid/jsfmt.spec.js
new file mode 100644
--- /dev/null
+++ b/tests/format/vue/invalid/jsfmt.spec.js
@@ -0,0 +1 @@
+run_spec(__dirname, ["vue"]);
diff --git a/tests/format/vue/invalid/v-for.vue b/tests/format/vue/invalid/v-for.vue
new file mode 100644
--- /dev/null
+++ b/tests/format/vue/invalid/v-for.vue
@@ -0,0 +1,21 @@
+<template>
+ <div>
+ <div
+ v-for=" _ in "
+ v-for=" in _ "
+ v-for=" in "
+ v-for=" _, in a "
+ v-for=" ,_ in a "
+
+ v-for=" a, b, in a "
+ v-for=" a, , c in a "
+
+ v-for=" , b, c in a "
+ v-for=" a, b, in a "
+
+ v-for=" , b, c in a "
+ v-for=" a, , c in a "
+ v-for=" (,a,b) of 'abcd' "
+ ></div>
+ </div>
+</template>
| [vue] v-for incorrectly removing commas from left hand side
**Prettier 2.5.1**
[Playground link](https://prettier.io/playground/#N4Igxg9gdgLgprEAuEAeeBbADgGwIbwB8AOlAARmoAmAlgG5l0C0AZhAE4C8xIAFADR5+AIwCUZCCzJgehVAHpadElAWZcBOCpD8QELDBrQAzslB527CAHcAChYSmUeHNbwBPU7uHs8YANZwMADKeBhwADI0UHDILC7GcN6+AUHBWH7RAObIMOwArkkgcBjCcFRU5RF4UFn5eFlwAGIcGASGtcggePkwEDogABYwGDgA6oM08MYZYHDBjlP0U+5dYMZeINGJ7DC2vlltcQlFAFbGAB7B2ThwAIr5EPDHOIm6Gew7XXSFA1js0RgYxoVBgg2QAA4AAzvKyJMa+LBdf5wHZ0WK6ACOj3g+30Tm6xiYMXK5QG7Dg2JoFP2DSOSHiryKiQwNFyBWZN3uONiDJOuhgeGEwNB4KQACYBb4aDhsgBhCAYenFYwAVgG+USABUhU5GW8QD84ABJKCVWDBMAAgwAQTNwRg7luL0SAF9XUA)
**Input:**
<!-- prettier-ignore -->
```vue
<template>
<div v-for="(,a,b) of c"></div>
</template>
```
**Output:**
<!-- prettier-ignore -->
```vue
<template>
<div v-for="(a, b) of c"></div>
</template>
```
**Expected behavior:**
the `(,a,b)` is converted to `(a, b)` silently, which is wrong in semantics. It should be left untouched. Since the vue template compiler actually accepts it and correctly ignoring the `b`, while assigning `a` the index number.
| "2022-01-18T13:11:35Z" | 2.6 | [] | [
"tests/format/vue/invalid/jsfmt.spec.js"
] | JavaScript | [] | [
"https://prettier.io/playground/#N4Igxg9gdgLgprEAuEAeeBbADgGwIbwB8AOlAARmoAmAlgG5l0C0AZhAE4C8xIAFADR5+AIwCUZCCzJgehVAHpadElAWZcBOCpD8QELDBrQAzslB527CAHcAChYSmUeHNbwBPU7uHs8YANZwMADKeBhwADI0UHDILC7GcN6+AUHBWH7RAObIMOwArkkgcBjCcFRU5RF4UFn5eFlwAGIcGASGtcggePkwEDogABYwGDgA6oM08MYZYHDBjlP0U+5dYMZeINGJ7DC2vlltcQlFAFbGAB7B2ThwAIr5EPDHOIm6Gew7XXSFA1js0RgYxoVBgg2QAA4AAzvKyJMa+LBdf5wHZ0WK6ACOj3g+30Tm6xiYMXK5QG7Dg2JoFP2DSOSHiryKiQwNFyBWZN3uONiDJOuhgeGEwNB4KQACYBb4aDhsgBhCAYenFYwAVgG+USABUhU5GW8QD84ABJKCVWDBMAAgwAQTNwRg7luL0SAF9XUA"
] |
|
prettier/prettier | 12,177 | prettier__prettier-12177 | [
"12108"
] | 9106e7ea1353a2f7c03026f939d540206620f66f | diff --git a/src/language-js/comments.js b/src/language-js/comments.js
--- a/src/language-js/comments.js
+++ b/src/language-js/comments.js
@@ -24,6 +24,7 @@ const {
isCallExpression,
isMemberExpression,
isObjectProperty,
+ isLineComment,
getComments,
CommentCheckFlags,
markerForIfWithoutBlockAndSameLineComment,
@@ -93,6 +94,7 @@ function handleEndOfLineComment(context) {
handleTypeAliasComments,
handleVariableDeclaratorComments,
handleBreakAndContinueStatementComments,
+ handleSwitchDefaultCaseComments,
].some((fn) => fn(context));
}
@@ -897,6 +899,28 @@ function handleTSMappedTypeComments({
return false;
}
+function handleSwitchDefaultCaseComments({
+ comment,
+ enclosingNode,
+ followingNode,
+}) {
+ if (
+ !enclosingNode ||
+ enclosingNode.type !== "SwitchCase" ||
+ enclosingNode.test
+ ) {
+ return false;
+ }
+
+ if (followingNode.type === "BlockStatement" && isLineComment(comment)) {
+ addBlockStatementFirstComment(followingNode, comment);
+ } else {
+ addDanglingComment(enclosingNode, comment);
+ }
+
+ return true;
+}
+
/**
* @param {Node} node
* @returns {boolean}
diff --git a/src/language-js/printer-estree.js b/src/language-js/printer-estree.js
--- a/src/language-js/printer-estree.js
+++ b/src/language-js/printer-estree.js
@@ -696,6 +696,10 @@ function printPathNoParens(path, options, print, args) {
parts.push("default:");
}
+ if (hasComment(node, CommentCheckFlags.Dangling)) {
+ parts.push(" ", printDanglingComments(path, options, true));
+ }
+
const consequent = node.consequent.filter(
(node) => node.type !== "EmptyStatement"
);
| diff --git a/tests/format/js/switch/__snapshots__/jsfmt.spec.js.snap b/tests/format/js/switch/__snapshots__/jsfmt.spec.js.snap
--- a/tests/format/js/switch/__snapshots__/jsfmt.spec.js.snap
+++ b/tests/format/js/switch/__snapshots__/jsfmt.spec.js.snap
@@ -30,6 +30,41 @@ switch(x) {
}
}
+switch(x) {
+ default: // comment
+ break;
+}
+
+switch(x) {
+ default: // comment
+ {break;}
+}
+
+switch(x) {
+ default: {// comment
+ break;}
+}
+
+switch(x) {
+ default: /* comment */
+ break;
+}
+
+switch(x) {
+ default: /* comment */
+ {break;}
+}
+
+switch(x) {
+ default: {/* comment */
+ break;}
+}
+
+switch(x) {
+ default: /* comment */ {
+ break;}
+}
+
=====================================output=====================================
switch (true) {
case true:
@@ -55,6 +90,49 @@ switch (x) {
}
}
+switch (x) {
+ default: // comment
+ break;
+}
+
+switch (x) {
+ default: {
+ // comment
+ break;
+ }
+}
+
+switch (x) {
+ default: {
+ // comment
+ break;
+ }
+}
+
+switch (x) {
+ default: /* comment */
+ break;
+}
+
+switch (x) {
+ default: /* comment */ {
+ break;
+ }
+}
+
+switch (x) {
+ default: {
+ /* comment */
+ break;
+ }
+}
+
+switch (x) {
+ default: /* comment */ {
+ break;
+ }
+}
+
================================================================================
`;
diff --git a/tests/format/js/switch/comments.js b/tests/format/js/switch/comments.js
--- a/tests/format/js/switch/comments.js
+++ b/tests/format/js/switch/comments.js
@@ -21,3 +21,38 @@ switch(x) {
case y: {
}
}
+
+switch(x) {
+ default: // comment
+ break;
+}
+
+switch(x) {
+ default: // comment
+ {break;}
+}
+
+switch(x) {
+ default: {// comment
+ break;}
+}
+
+switch(x) {
+ default: /* comment */
+ break;
+}
+
+switch(x) {
+ default: /* comment */
+ {break;}
+}
+
+switch(x) {
+ default: {/* comment */
+ break;}
+}
+
+switch(x) {
+ default: /* comment */ {
+ break;}
+}
| `switch` formatting: `case: // comment` and `default: // comment` have different outcomes
**Prettier 2.3.2**
[Playground link](https://prettier.io/playground/#N4Igxg9gdgLgprEAuEAzArlMMCW0AEATnAIYAmA+gM4wnwC2CMAFAJT7AA6U++VA7jhhgAFvmYAHOHADWFGXACe-CITJt2XHr3xgSVOPgDSSlWoB0OehIA2SfAHoH+K7YA8AFQB8+AOKEIfhscKABzTx9UVXwAdQgbVA4AX24dHWIYdEIeAElrGwARODAbEkI6PChzYnI2AG5uVJ0yOFQSdBsYeyd8OAAPCWIqKkqG7TSMrJ4AUQGhkegAZVoGJmrSdVYx3hSoXZAAGhAICVxoKmRQMoD+AAUyhAuUEht+EkULo4AjcrAFGEWEhIYBCoWQMEI6DgRzg9C+cDILTIABkSGF0CRQnAAGKqeh0XBhZAgdowCCHEAiGD0GwxERCOBUIFgOCLR5CHAANyEimJYGGFJCBkIMFu5VC+OQbRsBiOACsqH0AEK-f6LEiMZEhOBSl6ykAKvqLUE2OAARXQEHgupl0JAQMIwuJXxI8JsFMGIRgMRwZBgImQAA4AAxHQYQAwxcoSYmDRlwQicnVHACOlvgYpOTxJVAAtFBpEiKcQ0zhiGLMZKkNL9QZ6DhwZC7SMwqaLVaddW9XbaF8fX6A0gAExHCEkHDBMIAYQg9CrIEZAFYKegDB5XU8a3bOVCclAWrBFmBCDhTgBBffLRSmm0GJJJIA)
<!-- prettier-ignore -->
```sh
--parser babel
```
**Input:**
<!-- prettier-ignore -->
```jsx
function read_statement() {
switch (peek_keyword()) {
case Keyword.impl: // impl<T> Growling<T> for Wolf {}
return ImplDeclaration.read();
default: // expression;
return ExpressionStatement.read();
}
}
```
**Output:**
<!-- prettier-ignore -->
```jsx
function read_statement() {
switch (peek_keyword()) {
case Keyword.impl: // impl<T> Growling<T> for Wolf {}
return ImplDeclaration.read();
default:
// expression;
return ExpressionStatement.read();
}
}
```
**Expected behavior:**
```ts
default: // expression;
```
<img width="468" alt="Code_2022-01-16_11-13-00" src="https://user-images.githubusercontent.com/30108880/149655786-0ca0c50e-30ac-4811-bbbb-9948f89f6cb3.png">
| "2022-01-27T20:56:18Z" | 2.6 | [] | [
"tests/format/js/switch/jsfmt.spec.js"
] | JavaScript | [
"https://user-images.githubusercontent.com/30108880/149655786-0ca0c50e-30ac-4811-bbbb-9948f89f6cb3.png"
] | [
"https://prettier.io/playground/#N4Igxg9gdgLgprEAuEAzArlMMCW0AEATnAIYAmA+gM4wnwC2CMAFAJT7AA6U++VA7jhhgAFvmYAHOHADWFGXACe-CITJt2XHr3xgSVOPgDSSlWoB0OehIA2SfAHoH+K7YA8AFQB8+AOKEIfhscKABzTx9UVXwAdQgbVA4AX24dHWIYdEIeAElrGwARODAbEkI6PChzYnI2AG5uVJ0yOFQSdBsYeyd8OAAPCWIqKkqG7TSMrJ4AUQGhkegAZVoGJmrSdVYx3hSoXZAAGhAICVxoKmRQMoD+AAUyhAuUEht+EkULo4AjcrAFGEWEhIYBCoWQMEI6DgRzg9C+cDILTIABkSGF0CRQnAAGKqeh0XBhZAgdowCCHEAiGD0GwxERCOBUIFgOCLR5CHAANyEimJYGGFJCBkIMFu5VC+OQbRsBiOACsqH0AEK-f6LEiMZEhOBSl6ykAKvqLUE2OAARXQEHgupl0JAQMIwuJXxI8JsFMGIRgMRwZBgImQAA4AAxHQYQAwxcoSYmDRlwQicnVHACOlvgYpOTxJVAAtFBpEiKcQ0zhiGLMZKkNL9QZ6DhwZC7SMwqaLVaddW9XbaF8fX6A0gAExHCEkHDBMIAYQg9CrIEZAFYKegDB5XU8a3bOVCclAWrBFmBCDhTgBBffLRSmm0GJJJIA"
] |
|
prettier/prettier | 12,185 | prettier__prettier-12185 | [
"11145"
] | e3efd11401e73b824e7da0cd407ada4fa928c0a0 | diff --git a/src/language-js/comments.js b/src/language-js/comments.js
--- a/src/language-js/comments.js
+++ b/src/language-js/comments.js
@@ -459,6 +459,7 @@ function handleMethodNameComments({
if (
enclosingNode &&
precedingNode &&
+ getNextNonSpaceNonCommentCharacter(text, comment, locEnd) === "(" &&
// "MethodDefinition" is handled in getCommentChildNodes
(enclosingNode.type === "Property" ||
enclosingNode.type === "TSDeclareMethod" ||
| diff --git a/tests/format/typescript/comments/__snapshots__/jsfmt.spec.js.snap b/tests/format/typescript/comments/__snapshots__/jsfmt.spec.js.snap
--- a/tests/format/typescript/comments/__snapshots__/jsfmt.spec.js.snap
+++ b/tests/format/typescript/comments/__snapshots__/jsfmt.spec.js.snap
@@ -28,6 +28,40 @@ abstract class AbstractRule {
================================================================================
`;
+exports[`abstract_methods.ts format 1`] = `
+====================================options=====================================
+parsers: ["typescript"]
+printWidth: 80
+ | printWidth
+=====================================input======================================
+abstract class AbstractFoo {
+ abstract method1(/* comment */ arg: string);
+ abstract method2(
+ /* comment */
+ arg: string
+ );
+ abstract method3(
+ // comment
+ arg: string
+ );
+}
+
+=====================================output=====================================
+abstract class AbstractFoo {
+ abstract method1(/* comment */ arg: string);
+ abstract method2(
+ /* comment */
+ arg: string
+ );
+ abstract method3(
+ // comment
+ arg: string
+ );
+}
+
+================================================================================
+`;
+
exports[`after_jsx_generic.ts format 1`] = `
====================================options=====================================
parsers: ["typescript"]
@@ -602,6 +636,8 @@ export class Point {
mismatchedIndentation() {}
inline /* foo*/ (/* bar */) /* baz */ {}
+
+ noBody(/* comment */ arg);
}
=====================================output=====================================
@@ -651,6 +687,8 @@ export class Point {
mismatchedIndentation() {}
inline /* foo*/(/* bar */) /* baz */ {}
+
+ noBody(/* comment */ arg);
}
================================================================================
diff --git a/tests/format/typescript/comments/abstract_methods.ts b/tests/format/typescript/comments/abstract_methods.ts
new file mode 100644
--- /dev/null
+++ b/tests/format/typescript/comments/abstract_methods.ts
@@ -0,0 +1,11 @@
+abstract class AbstractFoo {
+ abstract method1(/* comment */ arg: string);
+ abstract method2(
+ /* comment */
+ arg: string
+ );
+ abstract method3(
+ // comment
+ arg: string
+ );
+}
diff --git a/tests/format/typescript/comments/methods.ts b/tests/format/typescript/comments/methods.ts
--- a/tests/format/typescript/comments/methods.ts
+++ b/tests/format/typescript/comments/methods.ts
@@ -44,4 +44,6 @@ export class Point {
mismatchedIndentation() {}
inline /* foo*/ (/* bar */) /* baz */ {}
+
+ noBody(/* comment */ arg);
}
| Misplaced parameter comment in abstract method
**Prettier 2.3.0**
[Playground link](https://prettier.io/playground/#N4Igxg9gdgLgprEAuEBDARgZxgJ1WGAAjABtVNNCAxCCQ4AHSkMI2zwMPVRwAomWLAPQAqEcQgBbSQiJwAHgAcyASyhqA5oQCumODgCSAE0KKeqSYRFCBg3fuMAaWwEomAXxCOQERTBXQmMigPDgQAO4ACjwIQSioJOGoAJ5B3ugcANZwMADKZmCayLjacN5wkuhwRkbVADKoUBraqBpwNDiSqDD+Tcho2jAQXiAAFjCSJADqoyrwmAVwubFzKgBuc8n9YBQjano4MJF4Gl3IAGYJet4AVpjyAEJZObkWcHVqcBdXZSB38rlNCQ4ABFbQQeDfEjXEBmHAHfrcKokAC0MDSsJwahgUxURhgo2QAA4AAzeRRhPRTPCKfoUuAHNZfbwAR3B8GOvjiaEwKKgcGq1RGODgbJUIuOrTOSEu0N+ekkKmKOFK3kwQNB7K+Mp+3hgGFx+MJSAATHq8CoSJoAMJSaUgBkAVhG9gAKmwoTC1qUDFBarBcmAsX4AIJ+3IwZLAz1wdzuIA)
<!-- prettier-ignore -->
```sh
--parser babel-ts
```
**Input:**
<!-- prettier-ignore -->
```tsx
abstract class Foo {
abstract bar(
/** comment explaining userId param */
userId,
)
}
```
**Output:**
<!-- prettier-ignore -->
```tsx
abstract class Foo {
abstract bar(userId);
/** comment explaining userId param */
}
```
**Expected behavior:**
<!-- prettier-ignore -->
```tsx
abstract class Foo {
abstract bar(
/** comment explaining userId param */
userId
)
}
```
I expect the comment to remain directly above the `userId` argument. It only seems to repro when the method is abstract and has exactly one argument.
| Also it happens for a method that has no body.
**Prettier 2.3.2**
[Playground link](https://prettier.io/playground/#N4Igxg9gdgLgprEAucAbAhgZ0wAgGIQQ7AA6UOOARugE4AUZFFA9AFSs6QC2XCMOcAB4AHDAEsoEgOY4Arpjg0AkgBMcw2ui45WzRk3mLVAGn0BKMgF8QxkBGEwx0TMlC0aEAO4AFWghco6Kie6ACeLraUNOhgANZwMADKGmDSyDA0snC2cFyUcCoqBQAy6FBSsuhScAQ0XOgwjuXIIOiyMBA2IAAWMFyoAOrdYvCYKXCJ-iNiAG4joS1g2F0SCjQw3tFS9cgAZkEKtgBWmIIAQtFxCYlacMUScHsH2SAngonSqHAAirIQ8E9UIcQBoaGsWtR8qgALQwCIgmgSGADMQqGDdZAADgADLZhB4FANosIWvi4GsZo9bABHP7wTb2AKtTDQqBwAoFLo0OC0sTczZVHZIfZAl4KLhidKZMWfH50x7C562GDoSgotEYpAAJmV0TEqGkAGEIDx0C1yQBWLqGAAqqoCIuBMyySigRVgiTAiIcAEE3YkYKEvoCFJZLEA)
<!-- prettier-ignore -->
```sh
--parser babel-ts
```
**Input:**
<!-- prettier-ignore -->
```tsx
class Foo {
bar(
/** comment explaining userId param */
userId,
)
}
```
**Output:**
<!-- prettier-ignore -->
```tsx
class Foo {
bar(userId);
/** comment explaining userId param */
}
```
This is blocking us from adopting prettier 2.3. Would love to see some traction on this diff.
I investigated this issue a little.
```typescript
abstract class Foo {
abstract bar(
/** comment explaining userId param */
userId,
)
}
```
In the `babel-ts` parser, the above comment has become the trailing comment for `bar`.
This might be the reason why the comment is misplaced.
Thanks @t-mangoe . If you can get us unblocked we'd be extremely grateful. I've never worked on a tool like prettier before, so I've procrastinated biting the bullet and diving in. This one is really blocking us and we can't leverage new features like support for the override keyword.
After bisecting, I found that this issue was introduced in #9872
@fisker is this something you can take a look at?
Looks like the change in canAttachComment is to blame, but the fix may be more complicated.
May be we can fix this in attachComment?
Forgive me, but I've never contributed to this project before. I don't see an attachComment API. Is it maybe referring to this PR? https://github.com/prettier/prettier/pull/11525 | "2022-01-28T20:59:05Z" | 2.6 | [] | [
"tests/format/typescript/comments/jsfmt.spec.js"
] | JavaScript | [] | [
"https://prettier.io/playground/#N4Igxg9gdgLgprEAuEBDARgZxgJ1WGAAjABtVNNCAxCCQ4AHSkMI2zwMPVRwAomWLAPQAqEcQgBbSQiJwAHgAcyASyhqA5oQCumODgCSAE0KKeqSYRFCBg3fuMAaWwEomAXxCOQERTBXQmMigPDgQAO4ACjwIQSioJOGoAJ5B3ugcANZwMADKZmCayLjacN5wkuhwRkbVADKoUBraqBpwNDiSqDD+Tcho2jAQXiAAFjCSJADqoyrwmAVwubFzKgBuc8n9YBQjano4MJF4Gl3IAGYJet4AVpjyAEJZObkWcHVqcBdXZSB38rlNCQ4ABFbQQeDfEjXEBmHAHfrcKokAC0MDSsJwahgUxURhgo2QAA4AAzeRRhPRTPCKfoUuAHNZfbwAR3B8GOvjiaEwKKgcGq1RGODgbJUIuOrTOSEu0N+ekkKmKOFK3kwQNB7K+Mp+3hgGFx+MJSAATHq8CoSJoAMJSaUgBkAVhG9gAKmwoTC1qUDFBarBcmAsX4AIJ+3IwZLAz1wdzuIA"
] |
prettier/prettier | 12,213 | prettier__prettier-12213 | [
"10291"
] | e22c4911f8bb951932715a7223dc29a81dfdb982 | diff --git a/src/language-css/printer-postcss.js b/src/language-css/printer-postcss.js
--- a/src/language-css/printer-postcss.js
+++ b/src/language-css/printer-postcss.js
@@ -493,8 +493,8 @@ function genericPrint(path, options, print) {
grandParent.type === "value-func" &&
grandParent.value === "selector"
) {
- const start = locStart(parentNode.open) + 1;
- const end = locEnd(parentNode.close) - 1;
+ const start = locEnd(parentNode.open) + 1;
+ const end = locStart(parentNode.close);
const selector = options.originalText.slice(start, end).trim();
return lastLineHasInlineComment(selector)
| diff --git a/tests/format/css/atrule/__snapshots__/jsfmt.spec.js.snap b/tests/format/css/atrule/__snapshots__/jsfmt.spec.js.snap
--- a/tests/format/css/atrule/__snapshots__/jsfmt.spec.js.snap
+++ b/tests/format/css/atrule/__snapshots__/jsfmt.spec.js.snap
@@ -3482,6 +3482,8 @@ $background
@mixin button-variant($foo: " ... ") {}
@mixin sexy-border($color, $width, $foo: (color: red)) {}
+@mixin selector($param: "value") {}
+
=====================================output=====================================
@mixin clearfix {
}
@@ -3551,6 +3553,9 @@ $background
@mixin sexy-border($color, $width, $foo: (color: red)) {
}
+@mixin selector($param: "value") {
+}
+
================================================================================
`;
diff --git a/tests/format/css/atrule/mixin.css b/tests/format/css/atrule/mixin.css
--- a/tests/format/css/atrule/mixin.css
+++ b/tests/format/css/atrule/mixin.css
@@ -105,3 +105,5 @@ $background
@mixin button-variant($foo: " ... ") {}
@mixin button-variant($foo: " ... ") {}
@mixin sexy-border($color, $width, $foo: (color: red)) {}
+
+@mixin selector($param: "value") {}
| scss mixin breaks on format if name is "selector"
<!--
BEFORE SUBMITTING AN ISSUE:
1. Search for your issue on GitHub: https://github.com/prettier/prettier/issues
A large number of opened issues are duplicates of existing issues.
If someone has already opened an issue for what you are experiencing,
you do not need to open a new issue — please add a 👍 reaction to the
existing issue instead.
2. We get a lot of requests for adding options, but Prettier is
built on the principle of being opinionated about code formatting.
This means we have a very high bar for adding new options.
Find out more: https://prettier.io/docs/en/option-philosophy.html
Tip! Don't write this stuff manually.
1. Go to https://prettier.io/playground
2. Paste your code and set options
3. Press the "Report issue" button in the lower right
-->
If a SCSS mixin is named "selector" (maybe other keywords also) and has a default value for a parameter, each format removes the last character of the default value.
**Prettier 2.2.1**
```sh
# Options:
--single-quote
--trailing-comma all
--no-semi
--print-width 120
```
**Input:**
```scss
@mixin selector($param: 'value') {
}
```
**Output:**
```scss
@mixin selector($param: 'value) {
}
```
**Expected behavior:**
Don't remove the last character of the default value...
| Just FYI: There seems to be a workaround: Add a space between closing quote and closing parenthesis.
I started analyzing the bug with the following test:
```scss
@mixin selector($param: 'value') {
}
@mixin selector($param: 'value' ) {
}
@mixin selector($param: "value") {
}
@mixin selector( $param: "value" ) {
}
@mixin selector2($param: 'value') {
}
@mixin elector($param: 'value') {
}
```
Which resulted in the following transformations:
```scss
@mixin selector($param: 'value) {
}
@mixin selector($param: 'value') {
}
@mixin selector($param: "value) {
}
@mixin selector($param: "value") {
}
@mixin selector2($param: "value") {
}
@mixin elector($param: "value") {
}
```
Since I'm not used to this project, I couldn't find the proper location to fix the issue within reasonable time. So someone else has to fix this (or point me to the right direction).
Maybe here https://github.com/prettier/prettier/blob/7d84c0a5a419d7fa3e65407b03e0ea55f45c1182/src/language-css/printer-postcss.js#L491 | "2022-02-02T12:30:14Z" | 2.6 | [] | [
"tests/format/css/atrule/jsfmt.spec.js"
] | JavaScript | [] | [
"https://prettier.io/playground"
] |
prettier/prettier | 12,226 | prettier__prettier-12226 | [
"12220"
] | e73a7bc47ebcfc991104689df097b910ceb251c9 | diff --git a/src/language-js/needs-parens.js b/src/language-js/needs-parens.js
--- a/src/language-js/needs-parens.js
+++ b/src/language-js/needs-parens.js
@@ -95,7 +95,6 @@ function needsParens(path, options) {
node.type === "LogicalExpression" ||
node.type === "NewExpression" ||
node.type === "ObjectExpression" ||
- node.type === "ParenthesizedExpression" ||
node.type === "SequenceExpression" ||
node.type === "TaggedTemplateExpression" ||
node.type === "UnaryExpression" ||
| diff --git a/tests/format/js/comments-closure-typecast/__snapshots__/jsfmt.spec.js.snap b/tests/format/js/comments-closure-typecast/__snapshots__/jsfmt.spec.js.snap
--- a/tests/format/js/comments-closure-typecast/__snapshots__/jsfmt.spec.js.snap
+++ b/tests/format/js/comments-closure-typecast/__snapshots__/jsfmt.spec.js.snap
@@ -603,6 +603,20 @@ const OverlapWrapper =
================================================================================
`;
+exports[`superclass.js format 1`] = `
+====================================options=====================================
+parsers: ["babel"]
+printWidth: 80
+ | printWidth
+=====================================input======================================
+class Foo extends /** @type {string} */ (Bar) {}
+
+=====================================output=====================================
+class Foo extends /** @type {string} */ (Bar) {}
+
+================================================================================
+`;
+
exports[`ways-to-specify-type.js format 1`] = `
====================================options=====================================
parsers: ["babel"]
diff --git a/tests/format/js/comments-closure-typecast/superclass.js b/tests/format/js/comments-closure-typecast/superclass.js
new file mode 100644
--- /dev/null
+++ b/tests/format/js/comments-closure-typecast/superclass.js
@@ -0,0 +1 @@
+class Foo extends /** @type {string} */ (Bar) {}
| JSDoc super class type override becomes broken
**Prettier 2.3.2**
[Playground link](https://prettier.io/playground/#N4Igxg9gdgLgprEAucAbAhgZ0wAgCo5wAe8UAJrgPQBU1OAAjAJ4AOcOwAYhBADwBC6AE4A+AL45qlHAApuEAJQcxIADQgILGAEtomZKGFCIAdwAKwhPpTpUJ9E33qARkPRgA1nBgBlFu+0oAHNkGCEAVzh1OABbZzgyMgSAGXRg8PQguG4hGPQYHWDkEHRwmAg1EAALGBjUAHUq7XhMfzA4Hytm7QA3ZqZisGxKwMw4IRgzNyC85AAzWzH1ACtMIn43T28fdBi4ZMC4ecWokFWiH0Cg1DgARXCIeGPUJZB-ITGhYud0eNRKlhCQIweraMgwKrIAAcAAZ1ICIGN6m4WMVAXBPj0juoAI4PeBTTTWEqYAC0UDgCQSlSEcDx2lpU0ysyQCxepzGMW0oQiHKuN3ujyOrJO6hgv1B4MhSAATGK3NpUFcAMIQGIskAYgCslXCYzwv2sbNePUiAElyAhfGAgVoAILkHzMG7PMZiMRAA)
<!-- prettier-ignore -->
```sh
--parser babel
```
**Input:**
<!-- prettier-ignore -->
```jsx
class T extends /** @type {Foo<Bar>} */ (Foo) {}
```
**Output:**
<!-- prettier-ignore -->
```jsx
class T /** @type {Foo<Bar>} */ extends ((Foo)) {}
```
**Expected behavior:**
```jsx
class T extends /** @type {Foo<Bar>} */ (Foo) {}
```
The type override is needed to specify the generic type and should not be moved.
| @wmertens Yeah, Prettier shouldn't move this comment, it's a bug.
But unrelated to the bug, shouldn't the syntax be something like this?
```js
/** @extends {Foo<Bar>} */
class T extends Foo {}
```
I wish, it looks like the extends keyword can't use generics. i can't get it to work in any case
Is that using tsc? [This](https://www.typescriptlang.org/play?filetype=js#code/PQKhAIAEBcFMFsAOAbAhncAVcJgCgBjNAZ2PADEB7S8Abz3HFAkgCdZoBXVgOzNswBfHPkYBzDuABm1ABQBKOg0bh2XXkxbQAnolh0hI8D07JkAbmWC81vMyiwAHnB4ATMlUoAeYtFYBLHjEAPhFCEjIAQXAnF3cKajpbexhdfV8AoLCCSj5ocEdwAF5jWAB3cEiFADoZSks7MAdnWDcPai8TeAAjWFZQ3HDUUnAAIRiWtoSaWmSm1L1jTh6+7NzfcG1i0orRmrrzIA) works for me and in the playground | "2022-02-05T13:54:12Z" | 2.6 | [] | [
"tests/format/js/comments-closure-typecast/jsfmt.spec.js"
] | JavaScript | [] | [
"https://prettier.io/playground/#N4Igxg9gdgLgprEAucAbAhgZ0wAgCo5wAe8UAJrgPQBU1OAAjAJ4AOcOwAYhBADwBC6AE4A+AL45qlHAApuEAJQcxIADQgILGAEtomZKGFCIAdwAKwhPpTpUJ9E33qARkPRgA1nBgBlFu+0oAHNkGCEAVzh1OABbZzgyMgSAGXRg8PQguG4hGPQYHWDkEHRwmAg1EAALGBjUAHUq7XhMfzA4Hytm7QA3ZqZisGxKwMw4IRgzNyC85AAzWzH1ACtMIn43T28fdBi4ZMC4ecWokFWiH0Cg1DgARXCIeGPUJZB-ITGhYud0eNRKlhCQIweraMgwKrIAAcAAZ1ICIGN6m4WMVAXBPj0juoAI4PeBTTTWEqYAC0UDgCQSlSEcDx2lpU0ysyQCxepzGMW0oQiHKuN3ujyOrJO6hgv1B4MhSAATGK3NpUFcAMIQGIskAYgCslXCYzwv2sbNePUiAElyAhfGAgVoAILkHzMG7PMZiMRAA"
] |
prettier/prettier | 12,260 | prettier__prettier-12260 | [
"12257"
] | b1645073b897bfc4318186bc42936bf91e86538b | diff --git a/src/language-js/needs-parens.js b/src/language-js/needs-parens.js
--- a/src/language-js/needs-parens.js
+++ b/src/language-js/needs-parens.js
@@ -1,6 +1,7 @@
"use strict";
const getLast = require("../utils/get-last.js");
+const isNonEmptyArray = require("../utils/is-non-empty-array.js");
const {
getFunctionParameters,
getLeftSidePathName,
@@ -667,6 +668,10 @@ function needsParens(path, options) {
}
case "ClassExpression":
+ if (isNonEmptyArray(node.decorators)) {
+ return true;
+ }
+
switch (parent.type) {
case "NewExpression":
return name === "callee";
diff --git a/src/language-js/printer-estree.js b/src/language-js/printer-estree.js
--- a/src/language-js/printer-estree.js
+++ b/src/language-js/printer-estree.js
@@ -106,19 +106,44 @@ function genericPrint(path, options, print, args) {
return printed;
}
+ let parts = [printed];
+
const printedDecorators = printDecorators(path, options, print);
- // Nodes with decorators can't have parentheses and don't need leading semicolons
+ const isClassExpressionWithDecorators =
+ node.type === "ClassExpression" && printedDecorators;
+ // Nodes (except `ClassExpression`) with decorators can't have parentheses and don't need leading semicolons
if (printedDecorators) {
- return group([...printedDecorators, printed]);
+ parts = [...printedDecorators, printed];
+
+ if (!isClassExpressionWithDecorators) {
+ return group(parts);
+ }
}
const needsParens = pathNeedsParens(path, options);
if (!needsParens) {
- return args && args.needsSemi ? [";", printed] : printed;
+ if (args && args.needsSemi) {
+ parts.unshift(";");
+ }
+
+ // In member-chain print, it add `label` to the doc, if we return array here it will be broken
+ if (parts.length === 1 && parts[0] === printed) {
+ return printed;
+ }
+
+ return parts;
+ }
+
+ if (isClassExpressionWithDecorators) {
+ parts = [indent([line, ...parts])];
}
- const parts = [args && args.needsSemi ? ";(" : "(", printed];
+ parts.unshift("(");
+
+ if (args && args.needsSemi) {
+ parts.unshift(";");
+ }
if (hasFlowShorthandAnnotationComment(node)) {
const [comment] = node.trailingComments;
@@ -126,6 +151,10 @@ function genericPrint(path, options, print, args) {
comment.printed = true;
}
+ if (isClassExpressionWithDecorators) {
+ parts.push(line);
+ }
+
parts.push(")");
return parts;
| diff --git a/tests/format/js/decorators/__snapshots__/jsfmt.spec.js.snap b/tests/format/js/decorators/__snapshots__/jsfmt.spec.js.snap
--- a/tests/format/js/decorators/__snapshots__/jsfmt.spec.js.snap
+++ b/tests/format/js/decorators/__snapshots__/jsfmt.spec.js.snap
@@ -51,16 +51,20 @@ export class Bar {}
export default class Baz {}
const foo =
- @deco
- class {
- //
- };
+ (
+ @deco
+ class {
+ //
+ }
+ );
const bar =
- @deco
- class {
- //
- };
+ (
+ @deco
+ class {
+ //
+ }
+ );
================================================================================
`;
diff --git a/tests/format/js/decorators/class-expression/__snapshots__/jsfmt.spec.js.snap b/tests/format/js/decorators/class-expression/__snapshots__/jsfmt.spec.js.snap
new file mode 100644
--- /dev/null
+++ b/tests/format/js/decorators/class-expression/__snapshots__/jsfmt.spec.js.snap
@@ -0,0 +1,543 @@
+// Jest Snapshot v1, https://goo.gl/fbAQLP
+
+exports[`arguments.js [acorn] format 1`] = `
+"Unexpected character '@' (1:13)
+> 1 | console.log(@deco class Foo {})
+ | ^
+ 2 | console.log(@deco class {})
+ 3 |"
+`;
+
+exports[`arguments.js [espree] format 1`] = `
+"Unexpected character '@' (1:13)
+> 1 | console.log(@deco class Foo {})
+ | ^
+ 2 | console.log(@deco class {})
+ 3 |"
+`;
+
+exports[`arguments.js [flow] format 1`] = `
+"Unexpected token \`@\` (1:13)
+> 1 | console.log(@deco class Foo {})
+ | ^
+ 2 | console.log(@deco class {})
+ 3 |"
+`;
+
+exports[`arguments.js [typescript] format 1`] = `
+"Argument expression expected. (1:13)
+> 1 | console.log(@deco class Foo {})
+ | ^
+ 2 | console.log(@deco class {})
+ 3 |"
+`;
+
+exports[`arguments.js - {"semi":false} [acorn] format 1`] = `
+"Unexpected character '@' (1:13)
+> 1 | console.log(@deco class Foo {})
+ | ^
+ 2 | console.log(@deco class {})
+ 3 |"
+`;
+
+exports[`arguments.js - {"semi":false} [espree] format 1`] = `
+"Unexpected character '@' (1:13)
+> 1 | console.log(@deco class Foo {})
+ | ^
+ 2 | console.log(@deco class {})
+ 3 |"
+`;
+
+exports[`arguments.js - {"semi":false} [flow] format 1`] = `
+"Unexpected token \`@\` (1:13)
+> 1 | console.log(@deco class Foo {})
+ | ^
+ 2 | console.log(@deco class {})
+ 3 |"
+`;
+
+exports[`arguments.js - {"semi":false} [typescript] format 1`] = `
+"Argument expression expected. (1:13)
+> 1 | console.log(@deco class Foo {})
+ | ^
+ 2 | console.log(@deco class {})
+ 3 |"
+`;
+
+exports[`arguments.js - {"semi":false} format 1`] = `
+====================================options=====================================
+parsers: ["babel", "flow", "typescript"]
+printWidth: 80
+semi: false
+ | printWidth
+=====================================input======================================
+console.log(@deco class Foo {})
+console.log(@deco class {})
+
+=====================================output=====================================
+console.log(
+ (
+ @deco
+ class Foo {}
+ )
+)
+console.log(
+ (
+ @deco
+ class {}
+ )
+)
+
+================================================================================
+`;
+
+exports[`arguments.js format 1`] = `
+====================================options=====================================
+parsers: ["babel", "flow", "typescript"]
+printWidth: 80
+ | printWidth
+=====================================input======================================
+console.log(@deco class Foo {})
+console.log(@deco class {})
+
+=====================================output=====================================
+console.log(
+ (
+ @deco
+ class Foo {}
+ )
+);
+console.log(
+ (
+ @deco
+ class {}
+ )
+);
+
+================================================================================
+`;
+
+exports[`class-expression.js [acorn] format 1`] = `
+"Unexpected character '@' (1:13)
+> 1 | const a1 = (@deco class Foo {});
+ | ^
+ 2 | const a2 = (@deco class {});
+ 3 |
+ 4 | (@deco class Foo {});"
+`;
+
+exports[`class-expression.js [espree] format 1`] = `
+"Unexpected character '@' (1:13)
+> 1 | const a1 = (@deco class Foo {});
+ | ^
+ 2 | const a2 = (@deco class {});
+ 3 |
+ 4 | (@deco class Foo {});"
+`;
+
+exports[`class-expression.js [flow] format 1`] = `
+"Unexpected token \`@\` (1:13)
+> 1 | const a1 = (@deco class Foo {});
+ | ^
+ 2 | const a2 = (@deco class {});
+ 3 |
+ 4 | (@deco class Foo {});"
+`;
+
+exports[`class-expression.js [typescript] format 1`] = `
+"Expression expected. (1:13)
+> 1 | const a1 = (@deco class Foo {});
+ | ^
+ 2 | const a2 = (@deco class {});
+ 3 |
+ 4 | (@deco class Foo {});"
+`;
+
+exports[`class-expression.js - {"semi":false} [acorn] format 1`] = `
+"Unexpected character '@' (1:13)
+> 1 | const a1 = (@deco class Foo {});
+ | ^
+ 2 | const a2 = (@deco class {});
+ 3 |
+ 4 | (@deco class Foo {});"
+`;
+
+exports[`class-expression.js - {"semi":false} [espree] format 1`] = `
+"Unexpected character '@' (1:13)
+> 1 | const a1 = (@deco class Foo {});
+ | ^
+ 2 | const a2 = (@deco class {});
+ 3 |
+ 4 | (@deco class Foo {});"
+`;
+
+exports[`class-expression.js - {"semi":false} [flow] format 1`] = `
+"Unexpected token \`@\` (1:13)
+> 1 | const a1 = (@deco class Foo {});
+ | ^
+ 2 | const a2 = (@deco class {});
+ 3 |
+ 4 | (@deco class Foo {});"
+`;
+
+exports[`class-expression.js - {"semi":false} [typescript] format 1`] = `
+"Expression expected. (1:13)
+> 1 | const a1 = (@deco class Foo {});
+ | ^
+ 2 | const a2 = (@deco class {});
+ 3 |
+ 4 | (@deco class Foo {});"
+`;
+
+exports[`class-expression.js - {"semi":false} format 1`] = `
+====================================options=====================================
+parsers: ["babel", "flow", "typescript"]
+printWidth: 80
+semi: false
+ | printWidth
+=====================================input======================================
+const a1 = (@deco class Foo {});
+const a2 = (@deco class {});
+
+(@deco class Foo {});
+(@deco class {});
+
+const b1 = []
+;(@deco class Foo {})
+
+const b2 = []
+;(@deco class {})
+
+// This is not a \`ClassExpression\` but \`ClassDeclaration\`
+@deco class Foo {}
+
+=====================================output=====================================
+const a1 =
+ (
+ @deco
+ class Foo {}
+ )
+const a2 =
+ (
+ @deco
+ class {}
+ )
+
+;(
+ @deco
+ class Foo {}
+)
+;(
+ @deco
+ class {}
+)
+
+const b1 = []
+;(
+ @deco
+ class Foo {}
+)
+
+const b2 = []
+;(
+ @deco
+ class {}
+)
+
+// This is not a \`ClassExpression\` but \`ClassDeclaration\`
+@deco
+class Foo {}
+
+================================================================================
+`;
+
+exports[`class-expression.js format 1`] = `
+====================================options=====================================
+parsers: ["babel", "flow", "typescript"]
+printWidth: 80
+ | printWidth
+=====================================input======================================
+const a1 = (@deco class Foo {});
+const a2 = (@deco class {});
+
+(@deco class Foo {});
+(@deco class {});
+
+const b1 = []
+;(@deco class Foo {})
+
+const b2 = []
+;(@deco class {})
+
+// This is not a \`ClassExpression\` but \`ClassDeclaration\`
+@deco class Foo {}
+
+=====================================output=====================================
+const a1 =
+ (
+ @deco
+ class Foo {}
+ );
+const a2 =
+ (
+ @deco
+ class {}
+ );
+
+(
+ @deco
+ class Foo {}
+);
+(
+ @deco
+ class {}
+);
+
+const b1 = [];
+(
+ @deco
+ class Foo {}
+);
+
+const b2 = [];
+(
+ @deco
+ class {}
+);
+
+// This is not a \`ClassExpression\` but \`ClassDeclaration\`
+@deco
+class Foo {}
+
+================================================================================
+`;
+
+exports[`member-expression.js [acorn] format 1`] = `
+"Unexpected character '@' (1:2)
+> 1 | (@deco class Foo {}).name;
+ | ^
+ 2 | (@deco class {}).name;
+ 3 |"
+`;
+
+exports[`member-expression.js [espree] format 1`] = `
+"Unexpected character '@' (1:2)
+> 1 | (@deco class Foo {}).name;
+ | ^
+ 2 | (@deco class {}).name;
+ 3 |"
+`;
+
+exports[`member-expression.js [flow] format 1`] = `
+"Unexpected token \`@\` (1:2)
+> 1 | (@deco class Foo {}).name;
+ | ^
+ 2 | (@deco class {}).name;
+ 3 |"
+`;
+
+exports[`member-expression.js [typescript] format 1`] = `
+"Expression expected. (1:2)
+> 1 | (@deco class Foo {}).name;
+ | ^
+ 2 | (@deco class {}).name;
+ 3 |"
+`;
+
+exports[`member-expression.js - {"semi":false} [acorn] format 1`] = `
+"Unexpected character '@' (1:2)
+> 1 | (@deco class Foo {}).name;
+ | ^
+ 2 | (@deco class {}).name;
+ 3 |"
+`;
+
+exports[`member-expression.js - {"semi":false} [espree] format 1`] = `
+"Unexpected character '@' (1:2)
+> 1 | (@deco class Foo {}).name;
+ | ^
+ 2 | (@deco class {}).name;
+ 3 |"
+`;
+
+exports[`member-expression.js - {"semi":false} [flow] format 1`] = `
+"Unexpected token \`@\` (1:2)
+> 1 | (@deco class Foo {}).name;
+ | ^
+ 2 | (@deco class {}).name;
+ 3 |"
+`;
+
+exports[`member-expression.js - {"semi":false} [typescript] format 1`] = `
+"Expression expected. (1:2)
+> 1 | (@deco class Foo {}).name;
+ | ^
+ 2 | (@deco class {}).name;
+ 3 |"
+`;
+
+exports[`member-expression.js - {"semi":false} format 1`] = `
+====================================options=====================================
+parsers: ["babel", "flow", "typescript"]
+printWidth: 80
+semi: false
+ | printWidth
+=====================================input======================================
+(@deco class Foo {}).name;
+(@deco class {}).name;
+
+=====================================output=====================================
+;((
+ @deco
+ class Foo {}
+).name)
+;((
+ @deco
+ class {}
+).name)
+
+================================================================================
+`;
+
+exports[`member-expression.js format 1`] = `
+====================================options=====================================
+parsers: ["babel", "flow", "typescript"]
+printWidth: 80
+ | printWidth
+=====================================input======================================
+(@deco class Foo {}).name;
+(@deco class {}).name;
+
+=====================================output=====================================
+((
+ @deco
+ class Foo {}
+).name);
+((
+ @deco
+ class {}
+).name);
+
+================================================================================
+`;
+
+exports[`super-class.js [acorn] format 1`] = `
+"Unexpected character '@' (1:20)
+> 1 | class Foo extends (@deco class Foo {}){}
+ | ^
+ 2 |
+ 3 | class Foo extends (@deco class {}){}
+ 4 |"
+`;
+
+exports[`super-class.js [espree] format 1`] = `
+"Unexpected character '@' (1:20)
+> 1 | class Foo extends (@deco class Foo {}){}
+ | ^
+ 2 |
+ 3 | class Foo extends (@deco class {}){}
+ 4 |"
+`;
+
+exports[`super-class.js [flow] format 1`] = `
+"Unexpected token \`@\` (1:20)
+> 1 | class Foo extends (@deco class Foo {}){}
+ | ^
+ 2 |
+ 3 | class Foo extends (@deco class {}){}
+ 4 |"
+`;
+
+exports[`super-class.js [typescript] format 1`] = `
+"Expression expected. (1:20)
+> 1 | class Foo extends (@deco class Foo {}){}
+ | ^
+ 2 |
+ 3 | class Foo extends (@deco class {}){}
+ 4 |"
+`;
+
+exports[`super-class.js - {"semi":false} [acorn] format 1`] = `
+"Unexpected character '@' (1:20)
+> 1 | class Foo extends (@deco class Foo {}){}
+ | ^
+ 2 |
+ 3 | class Foo extends (@deco class {}){}
+ 4 |"
+`;
+
+exports[`super-class.js - {"semi":false} [espree] format 1`] = `
+"Unexpected character '@' (1:20)
+> 1 | class Foo extends (@deco class Foo {}){}
+ | ^
+ 2 |
+ 3 | class Foo extends (@deco class {}){}
+ 4 |"
+`;
+
+exports[`super-class.js - {"semi":false} [flow] format 1`] = `
+"Unexpected token \`@\` (1:20)
+> 1 | class Foo extends (@deco class Foo {}){}
+ | ^
+ 2 |
+ 3 | class Foo extends (@deco class {}){}
+ 4 |"
+`;
+
+exports[`super-class.js - {"semi":false} [typescript] format 1`] = `
+"Expression expected. (1:20)
+> 1 | class Foo extends (@deco class Foo {}){}
+ | ^
+ 2 |
+ 3 | class Foo extends (@deco class {}){}
+ 4 |"
+`;
+
+exports[`super-class.js - {"semi":false} format 1`] = `
+====================================options=====================================
+parsers: ["babel", "flow", "typescript"]
+printWidth: 80
+semi: false
+ | printWidth
+=====================================input======================================
+class Foo extends (@deco class Foo {}){}
+
+class Foo extends (@deco class {}){}
+
+=====================================output=====================================
+class Foo extends (
+ @deco
+ class Foo {}
+) {}
+
+class Foo extends (
+ @deco
+ class {}
+) {}
+
+================================================================================
+`;
+
+exports[`super-class.js format 1`] = `
+====================================options=====================================
+parsers: ["babel", "flow", "typescript"]
+printWidth: 80
+ | printWidth
+=====================================input======================================
+class Foo extends (@deco class Foo {}){}
+
+class Foo extends (@deco class {}){}
+
+=====================================output=====================================
+class Foo extends (
+ @deco
+ class Foo {}
+) {}
+
+class Foo extends (
+ @deco
+ class {}
+) {}
+
+================================================================================
+`;
diff --git a/tests/format/js/decorators/class-expression/arguments.js b/tests/format/js/decorators/class-expression/arguments.js
new file mode 100644
--- /dev/null
+++ b/tests/format/js/decorators/class-expression/arguments.js
@@ -0,0 +1,2 @@
+console.log(@deco class Foo {})
+console.log(@deco class {})
diff --git a/tests/format/js/decorators/class-expression/class-expression.js b/tests/format/js/decorators/class-expression/class-expression.js
new file mode 100644
--- /dev/null
+++ b/tests/format/js/decorators/class-expression/class-expression.js
@@ -0,0 +1,14 @@
+const a1 = (@deco class Foo {});
+const a2 = (@deco class {});
+
+(@deco class Foo {});
+(@deco class {});
+
+const b1 = []
+;(@deco class Foo {})
+
+const b2 = []
+;(@deco class {})
+
+// This is not a `ClassExpression` but `ClassDeclaration`
+@deco class Foo {}
diff --git a/tests/format/js/decorators/class-expression/jsfmt.spec.js b/tests/format/js/decorators/class-expression/jsfmt.spec.js
new file mode 100644
--- /dev/null
+++ b/tests/format/js/decorators/class-expression/jsfmt.spec.js
@@ -0,0 +1,9 @@
+const errors = {
+ flow: true,
+ typescript: true,
+ acorn: true,
+ espree: true,
+};
+
+run_spec(__dirname, ["babel", "flow", "typescript"], { errors });
+run_spec(__dirname, ["babel", "flow", "typescript"], { semi: false, errors });
diff --git a/tests/format/js/decorators/class-expression/member-expression.js b/tests/format/js/decorators/class-expression/member-expression.js
new file mode 100644
--- /dev/null
+++ b/tests/format/js/decorators/class-expression/member-expression.js
@@ -0,0 +1,2 @@
+(@deco class Foo {}).name;
+(@deco class {}).name;
diff --git a/tests/format/js/decorators/class-expression/super-class.js b/tests/format/js/decorators/class-expression/super-class.js
new file mode 100644
--- /dev/null
+++ b/tests/format/js/decorators/class-expression/super-class.js
@@ -0,0 +1,3 @@
+class Foo extends (@deco class Foo {}){}
+
+class Foo extends (@deco class {}){}
diff --git a/tests/format/typescript/type-arguments-bit-shift-left-like/4.ts b/tests/format/typescript/type-arguments-bit-shift-left-like/4.ts
new file mode 100644
--- /dev/null
+++ b/tests/format/typescript/type-arguments-bit-shift-left-like/4.ts
@@ -0,0 +1 @@
+(@f<<T>(v: T) => void>() class {});
diff --git a/tests/format/typescript/type-arguments-bit-shift-left-like/__snapshots__/jsfmt.spec.js.snap b/tests/format/typescript/type-arguments-bit-shift-left-like/__snapshots__/jsfmt.spec.js.snap
--- a/tests/format/typescript/type-arguments-bit-shift-left-like/__snapshots__/jsfmt.spec.js.snap
+++ b/tests/format/typescript/type-arguments-bit-shift-left-like/__snapshots__/jsfmt.spec.js.snap
@@ -49,6 +49,30 @@ exports[`3.ts [typescript] format 1`] = `
2 |"
`;
+exports[`4.ts [babel-ts] format 1`] = `
+====================================options=====================================
+parsers: ["typescript"]
+printWidth: 80
+ | printWidth
+=====================================input======================================
+(@f<<T>(v: T) => void>() class {});
+
+=====================================output=====================================
+(
+ @f<<T>(v: T) => void>()
+ class {}
+);
+
+================================================================================
+`;
+
+exports[`4.ts [typescript] format 1`] = `
+"Expression expected. (1:2)
+> 1 | (@f<<T>(v: T) => void>() class {});
+ | ^
+ 2 |"
+`;
+
exports[`5.tsx [babel-ts] format 1`] = `
====================================options=====================================
parsers: ["typescript"]
diff --git a/tests/format/typescript/type-arguments-bit-shift-left-like/jsfmt.spec.js b/tests/format/typescript/type-arguments-bit-shift-left-like/jsfmt.spec.js
--- a/tests/format/typescript/type-arguments-bit-shift-left-like/jsfmt.spec.js
+++ b/tests/format/typescript/type-arguments-bit-shift-left-like/jsfmt.spec.js
@@ -1,3 +1,3 @@
run_spec(__dirname, ["typescript"], {
- errors: { typescript: ["3.ts", "5.tsx"] },
+ errors: { typescript: ["3.ts", "4.ts", "5.tsx"] },
});
| Missing parens for `ClassExpression` with decorators
**Prettier 2.5.1**
[Playground link](https://prettier.io/playground/#N4Igxg9gdgLgprEAuEAKAAgM1QSgARgA2AhgM6l7AC+OA3CADQgQAOMAltKcqMQE58IAdwAK-BNxTFCQ4gE9uTAEZ9iYANZwYAZWIBbOABl2UOMkzTScZao1btLNSYDmyGHwCu1kHD1K4ACYBgYbEUM4exM5wAGIQfHrEMBzhyCDEHjAQjCAAFjB6hADquezwpI5gcNoSZewAbmVyaWDkOSZWfDAiqs6J5pbeAFakAB7aLoRwAIoeEPADhFZMjnydaUrE-oQ5LHwmMEXsATC5yAAcAAwrglZFqixpe3Cd9WZMAI5z8D2skumkAC0pkCgRyfDgX3YEJ6UX6SAsS28Vj07DcnmRkxm3zMCMGTBgWyOJzOSAATATVOxCC4AMIQPTwnykACsOQ8VgAKltJIjliB6l4AJJQYKwbRgfZsACCou0MDkU0WVioVCAA)
<!-- prettier-ignore -->
```sh
--parser babel
```
**Input:**
<!-- prettier-ignore -->
```jsx
(@f() class {});
```
**Output:**
<!-- prettier-ignore -->
```jsx
@f()
class {};
```
**Second Output:**
<!-- prettier-ignore -->
```jsx
SyntaxError: A class name is required. (2:7)
1 | @f()
> 2 | class {};
| ^
3 |
```
**Expected behavior:**
Should keep parens
----
Found this during #12241, when fixing this, should add [this test](https://github.com/prettier/prettier/pull/12241/commits/84d52514b3922912b24fe9f5826936543709c386) back.
| "2022-02-07T08:38:33Z" | 2.6 | [] | [
"tests/format/js/decorators/class-expression/jsfmt.spec.js",
"tests/format/js/decorators/jsfmt.spec.js",
"tests/format/typescript/type-arguments-bit-shift-left-like/jsfmt.spec.js"
] | JavaScript | [] | [
"https://prettier.io/playground/#N4Igxg9gdgLgprEAuEAKAAgM1QSgARgA2AhgM6l7AC+OA3CADQgQAOMAltKcqMQE58IAdwAK-BNxTFCQ4gE9uTAEZ9iYANZwYAZWIBbOABl2UOMkzTScZao1btLNSYDmyGHwCu1kHD1K4ACYBgYbEUM4exM5wAGIQfHrEMBzhyCDEHjAQjCAAFjB6hADquezwpI5gcNoSZewAbmVyaWDkOSZWfDAiqs6J5pbeAFakAB7aLoRwAIoeEPADhFZMjnydaUrE-oQ5LHwmMEXsATC5yAAcAAwrglZFqixpe3Cd9WZMAI5z8D2skumkAC0pkCgRyfDgX3YEJ6UX6SAsS28Vj07DcnmRkxm3zMCMGTBgWyOJzOSAATATVOxCC4AMIQPTwnykACsOQ8VgAKltJIjliB6l4AJJQYKwbRgfZsACCou0MDkU0WVioVCAA"
] |
|
prettier/prettier | 12,302 | prettier__prettier-12302 | [
"11401"
] | ceed26dee3392cb83b5b0df750d56393f2c422b4 | diff --git a/src/language-handlebars/parser-glimmer.js b/src/language-handlebars/parser-glimmer.js
--- a/src/language-handlebars/parser-glimmer.js
+++ b/src/language-handlebars/parser-glimmer.js
@@ -12,8 +12,21 @@ function addBackslash(/* options*/) {
return {
name: "addBackslash",
visitor: {
- TextNode(node) {
- node.chars = node.chars.replace(/\\/, "\\\\");
+ All(node) {
+ const childrenOrBody = node.children || node.body;
+ if (childrenOrBody) {
+ for (let i = 0; i < childrenOrBody.length - 1; i++) {
+ if (
+ childrenOrBody[i].type === "TextNode" &&
+ childrenOrBody[i + 1].type === "MustacheStatement"
+ ) {
+ childrenOrBody[i].chars = childrenOrBody[i].chars.replace(
+ /\\$/,
+ "\\\\"
+ );
+ }
+ }
+ }
},
},
};
| diff --git a/tests/format/handlebars/escape/__snapshots__/jsfmt.spec.js.snap b/tests/format/handlebars/escape/__snapshots__/jsfmt.spec.js.snap
--- a/tests/format/handlebars/escape/__snapshots__/jsfmt.spec.js.snap
+++ b/tests/format/handlebars/escape/__snapshots__/jsfmt.spec.js.snap
@@ -1,5 +1,37 @@
// Jest Snapshot v1, https://goo.gl/fbAQLP
+exports[`backslashes.hbs format 1`] = `
+====================================options=====================================
+parsers: ["glimmer"]
+printWidth: 80
+ | printWidth
+=====================================input======================================
+<p>\\</p>
+<p>\\\\</p>
+<p>\\\\\\</p>
+<p>\\\\\\ \\\\{{non-escaped-moustache}}</p>
+
+=====================================output=====================================
+<p>\\</p>
+<p>\\\\</p>
+<p>\\\\\\</p>
+<p>\\\\\\ \\\\{{non-escaped-moustache}}</p>
+================================================================================
+`;
+
+exports[`backslashes-in-attributes.hbs format 1`] = `
+====================================options=====================================
+parsers: ["glimmer"]
+printWidth: 80
+ | printWidth
+=====================================input======================================
+<p data-attr="backslash \\ in an attribute"></p>
+
+=====================================output=====================================
+<p data-attr="backslash \\ in an attribute"></p>
+================================================================================
+`;
+
exports[`html-entities.hbs format 1`] = `
====================================options=====================================
parsers: ["glimmer"]
diff --git a/tests/format/handlebars/escape/backslashes-in-attributes.hbs b/tests/format/handlebars/escape/backslashes-in-attributes.hbs
new file mode 100644
--- /dev/null
+++ b/tests/format/handlebars/escape/backslashes-in-attributes.hbs
@@ -0,0 +1 @@
+<p data-attr="backslash \ in an attribute"></p>
diff --git a/tests/format/handlebars/escape/backslashes.hbs b/tests/format/handlebars/escape/backslashes.hbs
new file mode 100644
--- /dev/null
+++ b/tests/format/handlebars/escape/backslashes.hbs
@@ -0,0 +1,4 @@
+<p>\</p>
+<p>\\</p>
+<p>\\\</p>
+<p>\\\ \\{{non-escaped-moustache}}</p>
| Prettier should not re-escape a backslash in a string literal
## Description
Formatting handlebars prettier is escaping a backslash with another backslash.
Currently this PR only adds a breaking test.
For this example
```hbs
<DateFormat @pathFormat="dateFormat:YYYY-MM-DD hh\:mm\:ss" />
```
Current output
```hbs
<DateFormat @pathFormat="dateFormat:YYYY-MM-DD hh\\:mm\\:ss" />
```
Expected output
```hbs
<DateFormat @pathFormat="dateFormat:YYYY-MM-DD hh\:mm\:ss" />
```
## Checklist
<!-- Please ensure you’ve done all of these things (if applicable). -->
<!-- You can replace the `[ ]` with `[x]` to mark each task as done. -->
- [x] I’ve added tests to confirm my change works.
- [ ] (If changing the API or CLI) I’ve documented the changes I’ve made (in the `docs/` directory).
- [ ] (If the change is user-facing) I’ve added my changes to `changelog_unreleased/*/XXXX.md` file following `changelog_unreleased/TEMPLATE.md`.
- [x] I’ve read the [contributing guidelines](https://github.com/prettier/prettier/blob/main/CONTRIBUTING.md).
<!-- Please DO NOT remove the playground link -->
**✨[Try the playground for this PR](https://prettier.io/playground-redirect)✨**
| Can you open an issue instead? Or do you want fix it?
I have tried to fix it. But I was in able to fix it.
@fisker Is it worth adding a failing test and a issue.
Have you try unescape [here](https://github.com/prettier/prettier/blob/2380e113624300bfee65cc9ea1cffae44e8208be/src/language-handlebars/printer-glimmer.js#L194)?
Or maybe it's bug in the glimmer parser?
This function seems to be responsible for duplicating the first backslash (`\`): https://github.com/prettier/prettier/blob/c9c6d3364f8cf5140c3c16b44b92943464be7873/src/language-handlebars/parser-glimmer.js#L7-L20
`node.chars` is as expected before but have the duplicated slash afterwards. Not a big surprise if looking at that code.
It is run as a plugin for Glimmer parser. https://github.com/prettier/prettier/blob/c9c6d3364f8cf5140c3c16b44b92943464be7873/src/language-handlebars/parser-glimmer.js#L43-L46
If I remove that plugin for the Glimmer parser the following tests start to fail:
```
FAIL tests/format/handlebars/mustache-statement/jsfmt.spec.js
● escaped.hbs › format
expect(received).toMatchSnapshot()
Snapshot name: `escaped.hbs format 1`
- Snapshot - 2
+ Received + 2
@@ -11,8 +11,8 @@
\\\{{my-component}}
=====================================output=====================================
an escaped mustache:
\{{my-component}} a non-escaped mustache:
- \\{{my-component}}
- another non-escaped mustache: \\\{{my-component}}
+ \{{my-component}}
+ another non-escaped mustache: \\{{my-component}}
================================================================================
283 | CURSOR_PLACEHOLDER,
284 | })
> 285 | ).toMatchSnapshot();
| ^
286 | });
287 |
288 | if (!FULL_TEST) {
at Object.<anonymous> (tests/config/format-test.js:285:7)
● escaped.hbs - {"singleQuote":true} › format
expect(received).toMatchSnapshot()
Snapshot name: `escaped.hbs - {"singleQuote":true} format 1`
- Snapshot - 2
+ Received + 2
@@ -12,8 +12,8 @@
\\\{{my-component}}
=====================================output=====================================
an escaped mustache:
\{{my-component}} a non-escaped mustache:
- \\{{my-component}}
- another non-escaped mustache: \\\{{my-component}}
+ \{{my-component}}
+ another non-escaped mustache: \\{{my-component}}
================================================================================
283 | CURSOR_PLACEHOLDER,
284 | })
> 285 | ).toMatchSnapshot();
| ^
286 | });
287 |
288 | if (!FULL_TEST) {
at Object.<anonymous> (tests/config/format-test.js:285:7)
› 2 snapshots failed.
```
So it seems to be still needed to work-a-round another bug in the Glimmer parser.
To be honest until today I have never seen that escape syntax. Not sure if it is even officially supported by Glimmer at all. | "2022-02-13T15:35:42Z" | 2.6 | [] | [
"tests/format/handlebars/escape/jsfmt.spec.js"
] | JavaScript | [] | [
"https://prettier.io/playground-redirect"
] |
prettier/prettier | 12,349 | prettier__prettier-12349 | [
"12270"
] | 2cde2dbf9d280260f8c499039d89419b21fa4dc0 | diff --git a/src/language-js/print/assignment.js b/src/language-js/print/assignment.js
--- a/src/language-js/print/assignment.js
+++ b/src/language-js/print/assignment.js
@@ -2,7 +2,7 @@
const { isNonEmptyArray, getStringWidth } = require("../../common/util.js");
const {
- builders: { line, group, indent, indentIfBreak },
+ builders: { line, group, indent, indentIfBreak, lineSuffixBoundary },
utils: { cleanDoc, willBreak, canBreak },
} = require("../../document/index.js");
const {
@@ -50,6 +50,7 @@ function printAssignment(
group(leftDoc),
operator,
group(indent(line), { id: groupId }),
+ lineSuffixBoundary,
indentIfBreak(rightDoc, { groupId }),
]);
}
| diff --git a/tests/format/js/assignment-comments/__snapshots__/jsfmt.spec.js.snap b/tests/format/js/assignment-comments/__snapshots__/jsfmt.spec.js.snap
--- a/tests/format/js/assignment-comments/__snapshots__/jsfmt.spec.js.snap
+++ b/tests/format/js/assignment-comments/__snapshots__/jsfmt.spec.js.snap
@@ -1,5 +1,47 @@
// Jest Snapshot v1, https://goo.gl/fbAQLP
+exports[`call.js format 1`] = `
+====================================options=====================================
+parsers: ["babel", "flow", "typescript"]
+printWidth: 80
+ | printWidth
+=====================================input======================================
+if (true)
+ if (true)
+ if (true)
+ if (true)
+ if (true)
+ longVariableName1 = // @ts-ignore
+ (variable01 + veryLongVariableNameNumber2).method();
+
+=====================================output=====================================
+if (true)
+ if (true)
+ if (true)
+ if (true)
+ if (true)
+ longVariableName1 = // @ts-ignore
+ (variable01 + veryLongVariableNameNumber2).method();
+
+================================================================================
+`;
+
+exports[`call2.js format 1`] = `
+====================================options=====================================
+parsers: ["babel", "flow", "typescript"]
+printWidth: 80
+ | printWidth
+=====================================input======================================
+const kochabCooieGameOnOboleUnweave = // ???
+ rhubarbRhubarb(annularCooeedSplicesWalksWayWay);
+
+=====================================output=====================================
+const kochabCooieGameOnOboleUnweave = // ???
+ rhubarbRhubarb(annularCooeedSplicesWalksWayWay);
+
+================================================================================
+`;
+
exports[`function.js format 1`] = `
====================================options=====================================
parsers: ["babel", "flow", "typescript"]
@@ -82,7 +124,8 @@ f3 = (
a = b //comment
) => {};
-f4 = () => {}; // Comment
+f4 = // Comment
+ () => {};
f5 =
// Comment
@@ -109,7 +152,8 @@ let f3 = (
a = b //comment
) => {};
-let f4 = () => {}; // Comment
+let f4 = // Comment
+ () => {};
let f5 =
// Comment
@@ -141,7 +185,8 @@ const bifornCringerMoshedPerplexSawder = // !!!
anodyneCondosMalateOverateRetinol;
=====================================output=====================================
-const kochabCooieGameOnOboleUnweave = annularCooeedSplicesWalksWayWay; // ???
+const kochabCooieGameOnOboleUnweave = // ???
+ annularCooeedSplicesWalksWayWay;
const bifornCringerMoshedPerplexSawder = // !!!
glimseGlyphsHazardNoopsTieTie +
diff --git a/tests/format/js/assignment-comments/call.js b/tests/format/js/assignment-comments/call.js
new file mode 100644
--- /dev/null
+++ b/tests/format/js/assignment-comments/call.js
@@ -0,0 +1,7 @@
+if (true)
+ if (true)
+ if (true)
+ if (true)
+ if (true)
+ longVariableName1 = // @ts-ignore
+ (variable01 + veryLongVariableNameNumber2).method();
diff --git a/tests/format/js/assignment-comments/call2.js b/tests/format/js/assignment-comments/call2.js
new file mode 100644
--- /dev/null
+++ b/tests/format/js/assignment-comments/call2.js
@@ -0,0 +1,2 @@
+const kochabCooieGameOnOboleUnweave = // ???
+ rhubarbRhubarb(annularCooeedSplicesWalksWayWay);
| Unstable formatting: variable assignment, expression in parentheses, method call
**Prettier 2.5.1**
[Playground link](https://prettier.io/playground/#N4Igxg9gdgLgprEAuEBLAZgAgBQwE4CucAlADpSaYY75FkWVVa6EnmOPUt3sefO02DPowA20AOYA1AIZ5UMgEai4AORkBbOAEZMAXkwB6Q5gACMAM4BaVBKgQ8cXiMrYAbnIXK4ABl0BqTDc4PABPABlJWXklFXUtVQINRRCAJmIAOi0YAAsIABNsYgBuchAAGhAIAAcYVGgLZFA5PAgAdwAFOQRGlBlRNplQxsrFPBkwAGs4GABlTThw1Cg4ZHR+izhR8amZ2eqJ5YlkQUq4ZLh8-MvwmSgJAhkJOAAxBw0ZGDr75BAZAhgEAqIByMA0ogA6jlUPALAcwHBZj0Yag3DDQr8wBYRmgoJs8DAOuMJB81hstiAAFYWAAesyOKgAigQIPAyaJNpUDnh8b8YKFqnALGB5LVgdV5LAIah8rlkAAOHxc1qbCHjaq-CVCkLBYEARxZ8CJNV6f2sK0ul2BjgNqEcRKepKQ6w5FM2GlQJ1YlQsDLgzNZq2d5MqMCU0tlOWQqVD41QoiOAGEIBonSAhQBWYEETYAFSUvRdnJAbiIAEkoNdYLMRahagBBSuzfkqdmbAC+7aAA)
<!-- prettier-ignore -->
```sh
--parser typescript
```
**Input:**
<!-- prettier-ignore -->
```tsx
if (true)
if (true)
if (true)
if (true)
if (true)
longVariableName1 = // @ts-ignore
(variable01 + veryLongVariableNameNumber2).method();
```
**Output:**
<!-- prettier-ignore -->
```tsx
if (true)
if (true)
if (true)
if (true)
if (true)
longVariableName1 = ( // @ts-ignore
variable01 + veryLongVariableNameNumber2
).method();
```
**Second Output:**
<!-- prettier-ignore -->
```tsx
if (true)
if (true)
if (true)
if (true)
if (true)
longVariableName1 = // @ts-ignore
(variable01 + veryLongVariableNameNumber2).method();
```
**Expected behavior:**
The formatter output should be stable, not loop between two different formats.
| Another manifestation:
**Prettier 2.5.1**
[Playground link](https://prettier.io/playground/#N4Igxg9gdgLgprEAuc0DOMAEBrCYAWAhgEYDCEEAlnAOKEC2cA8lE8RADZwCqUA7nEIA3OJgC8mAPSTMAfnkAdKJhWqATvgCuxQmuIAlLTr0AKQlCiaOu8hDhwAJgGUADh0pg4aAOqEO2H0IAT18ggEoAbiUlEAAaEAgXGEp0ZFBdNQg+AAVdBDRkED8+YIL44jVCMGw4GCcGOAAZSig4ZAAzPzQ4csrq2tcqloBzZBg1TR6QOHpiRwdHRvNhzUJhuAAxCDV6QhhkqFGUQk0YCDiQfBh6Dm98Sng0Fyq4J3yHyiEHoMKwNDKQC1umoYNlKsNdh0ulMAFZoAAeThGXAAipoIPAoRxuvFnmpgYUdHMOBcXGoWjBvJQHDB8MgABwABlxmW63kqLkKZK8cDUIguAEd0fAwYkCsc0ABaVqORwXNRwIWUBVgtaQpCdbFTbr0ShjCba5FwNEYtoa6HxGAkKk0ulIABMlsqlHch3I9HV0zQAFYLppugAVEjizU4kBCSYASSgC1gTjA5KSAEEY04YEEuFjugBfbNAA)
<!-- prettier-ignore -->
```sh
--parser babel
```
**Input:**
<!-- prettier-ignore -->
```jsx
const kochabCooieGameOnOboleUnweave = // ???
rhubarbRhubarb(annularCooeedSplicesWalksWayWay);
```
**Output:**
<!-- prettier-ignore -->
```jsx
const kochabCooieGameOnOboleUnweave = rhubarbRhubarb( // ???
annularCooeedSplicesWalksWayWay
);
```
**Second Output:**
<!-- prettier-ignore -->
```jsx
const kochabCooieGameOnOboleUnweave = rhubarbRhubarb(
// ???
annularCooeedSplicesWalksWayWay
);
```
though note that your output is stable after second pass. though ideally it shouldn't need that | "2022-02-22T15:45:45Z" | 2.6 | [] | [
"tests/format/js/assignment-comments/jsfmt.spec.js"
] | JavaScript | [] | [
"https://prettier.io/playground/#N4Igxg9gdgLgprEAuEBLAZgAgBQwE4CucAlADpSaYY75FkWVVa6EnmOPUt3sefO02DPowA20AOYA1AIZ5UMgEai4AORkBbOAEZMAXkwB6Q5gACMAM4BaVBKgQ8cXiMrYAbnIXK4ABl0BqTDc4PABPABlJWXklFXUtVQINRRCAJmIAOi0YAAsIABNsYgBuchAAGhAIAAcYVGgLZFA5PAgAdwAFOQRGlBlRNplQxsrFPBkwAGs4GABlTThw1Cg4ZHR+izhR8amZ2eqJ5YlkQUq4ZLh8-MvwmSgJAhkJOAAxBw0ZGDr75BAZAhgEAqIByMA0ogA6jlUPALAcwHBZj0Yag3DDQr8wBYRmgoJs8DAOuMJB81hstiAAFYWAAesyOKgAigQIPAyaJNpUDnh8b8YKFqnALGB5LVgdV5LAIah8rlkAAOHxc1qbCHjaq-CVCkLBYEARxZ8CJNV6f2sK0ul2BjgNqEcRKepKQ6w5FM2GlQJ1YlQsDLgzNZq2d5MqMCU0tlOWQqVD41QoiOAGEIBonSAhQBWYEETYAFSUvRdnJAbiIAEkoNdYLMRahagBBSuzfkqdmbAC+7aAA"
] |
prettier/prettier | 12,362 | prettier__prettier-12362 | [
"12352"
] | 896970a18518bc31f1f15233b5ec612394453624 | diff --git a/src/document/doc-printer.js b/src/document/doc-printer.js
--- a/src/document/doc-printer.js
+++ b/src/document/doc-printer.js
@@ -202,10 +202,6 @@ function fits(next, restCommands, width, options, hasLineSuffix, mustBeFlat) {
? getLast(doc.expandedStates)
: doc.contents,
]);
-
- if (doc.id) {
- groupModeMap[doc.id] = groupMode;
- }
break;
}
case "fill":
@@ -216,7 +212,9 @@ function fits(next, restCommands, width, options, hasLineSuffix, mustBeFlat) {
break;
case "if-break":
case "indent-if-break": {
- const groupMode = doc.groupId ? groupModeMap[doc.groupId] : mode;
+ const groupMode = doc.groupId
+ ? groupModeMap[doc.groupId] || MODE_FLAT
+ : mode;
if (groupMode === MODE_BREAK) {
const breakContents =
doc.type === "if-break"
| diff --git a/tests/format/js/arrays/__snapshots__/jsfmt.spec.js.snap b/tests/format/js/arrays/__snapshots__/jsfmt.spec.js.snap
--- a/tests/format/js/arrays/__snapshots__/jsfmt.spec.js.snap
+++ b/tests/format/js/arrays/__snapshots__/jsfmt.spec.js.snap
@@ -56,9 +56,7 @@ printWidth: 80
}
}
{
- for (const srcPath of [
- 123, 123_123_123, 123_123_123_1, 13_123_3123_31_432,
- ]) {
+ for (const srcPath of [123, 123_123_123, 123_123_123_1, 13_123_3123_31_432]) {
}
}
{
diff --git a/tests/format/typescript/interface/long-type-parameters/__snapshots__/jsfmt.spec.js.snap b/tests/format/typescript/interface/long-type-parameters/__snapshots__/jsfmt.spec.js.snap
new file mode 100644
--- /dev/null
+++ b/tests/format/typescript/interface/long-type-parameters/__snapshots__/jsfmt.spec.js.snap
@@ -0,0 +1,79 @@
+// Jest Snapshot v1, https://goo.gl/fbAQLP
+
+exports[`long-type-parameters.ts - {"printWidth":109} format 1`] = `
+====================================options=====================================
+parsers: ["typescript"]
+printWidth: 109
+ | printWidth
+=====================================input======================================
+// https://github.com/vega/vega-lite/blob/ae13aff7b480cf9c994031eca08a6b1720e01ab3/src/mark.ts#L602
+export interface MarkDef<
+ M extends string | Mark = Mark,
+ ES extends ExprRef | SignalRef = ExprRef | SignalRef
+> extends GenericMarkDef<M>,
+ Omit<
+ MarkConfig<ES> &
+ AreaConfig<ES> &
+ BarConfig<ES> & // always extends RectConfig
+ LineConfig<ES> &
+ TickConfig<ES>,
+ 'startAngle' | 'endAngle' | 'width' | 'height'
+ >,
+ MarkDefMixins<ES> {}
+
+=====================================output=====================================
+// https://github.com/vega/vega-lite/blob/ae13aff7b480cf9c994031eca08a6b1720e01ab3/src/mark.ts#L602
+export interface MarkDef<
+ M extends string | Mark = Mark,
+ ES extends ExprRef | SignalRef = ExprRef | SignalRef
+> extends GenericMarkDef<M>,
+ Omit<
+ MarkConfig<ES> &
+ AreaConfig<ES> &
+ BarConfig<ES> & // always extends RectConfig
+ LineConfig<ES> &
+ TickConfig<ES>,
+ "startAngle" | "endAngle" | "width" | "height"
+ >,
+ MarkDefMixins<ES> {}
+
+================================================================================
+`;
+
+exports[`long-type-parameters.ts - {"printWidth":110} format 1`] = `
+====================================options=====================================
+parsers: ["typescript"]
+printWidth: 110
+ | printWidth
+=====================================input======================================
+// https://github.com/vega/vega-lite/blob/ae13aff7b480cf9c994031eca08a6b1720e01ab3/src/mark.ts#L602
+export interface MarkDef<
+ M extends string | Mark = Mark,
+ ES extends ExprRef | SignalRef = ExprRef | SignalRef
+> extends GenericMarkDef<M>,
+ Omit<
+ MarkConfig<ES> &
+ AreaConfig<ES> &
+ BarConfig<ES> & // always extends RectConfig
+ LineConfig<ES> &
+ TickConfig<ES>,
+ 'startAngle' | 'endAngle' | 'width' | 'height'
+ >,
+ MarkDefMixins<ES> {}
+
+=====================================output=====================================
+// https://github.com/vega/vega-lite/blob/ae13aff7b480cf9c994031eca08a6b1720e01ab3/src/mark.ts#L602
+export interface MarkDef<M extends string | Mark = Mark, ES extends ExprRef | SignalRef = ExprRef | SignalRef>
+ extends GenericMarkDef<M>,
+ Omit<
+ MarkConfig<ES> &
+ AreaConfig<ES> &
+ BarConfig<ES> & // always extends RectConfig
+ LineConfig<ES> &
+ TickConfig<ES>,
+ "startAngle" | "endAngle" | "width" | "height"
+ >,
+ MarkDefMixins<ES> {}
+
+================================================================================
+`;
diff --git a/tests/format/typescript/interface/long-type-parameters/jsfmt.spec.js b/tests/format/typescript/interface/long-type-parameters/jsfmt.spec.js
new file mode 100644
--- /dev/null
+++ b/tests/format/typescript/interface/long-type-parameters/jsfmt.spec.js
@@ -0,0 +1,2 @@
+run_spec(__dirname, ["typescript"], { printWidth: 109 });
+run_spec(__dirname, ["typescript"], { printWidth: 110 });
diff --git a/tests/format/typescript/interface/long-type-parameters/long-type-parameters.ts b/tests/format/typescript/interface/long-type-parameters/long-type-parameters.ts
new file mode 100644
--- /dev/null
+++ b/tests/format/typescript/interface/long-type-parameters/long-type-parameters.ts
@@ -0,0 +1,14 @@
+// https://github.com/vega/vega-lite/blob/ae13aff7b480cf9c994031eca08a6b1720e01ab3/src/mark.ts#L602
+export interface MarkDef<
+ M extends string | Mark = Mark,
+ ES extends ExprRef | SignalRef = ExprRef | SignalRef
+> extends GenericMarkDef<M>,
+ Omit<
+ MarkConfig<ES> &
+ AreaConfig<ES> &
+ BarConfig<ES> & // always extends RectConfig
+ LineConfig<ES> &
+ TickConfig<ES>,
+ 'startAngle' | 'endAngle' | 'width' | 'height'
+ >,
+ MarkDefMixins<ES> {}
diff --git a/tests/integration/__tests__/doc-printer.js b/tests/integration/__tests__/doc-printer.js
new file mode 100644
--- /dev/null
+++ b/tests/integration/__tests__/doc-printer.js
@@ -0,0 +1,27 @@
+"use strict";
+
+const prettier = require("prettier-local");
+const { group, ifBreak } = prettier.doc.builders;
+const { printDocToString } = prettier.doc.printer;
+const docToString = (doc, options) =>
+ printDocToString(doc, { tabSize: 2, ...options }).formatted;
+
+// Extra group should not effect `ifBreak` inside
+test("`ifBreak` inside `group`", () => {
+ const groupId = Symbol("test-group-id");
+ const FLAT_TEXT = "flat";
+ const doc = group(ifBreak("break", FLAT_TEXT, { groupId }), {
+ id: groupId,
+ });
+ const docs = [
+ doc,
+ [group(""), doc],
+ [doc, group("")],
+ group(doc),
+ group(doc, { id: Symbol("wrapper-group-id") }),
+ ];
+
+ expect(
+ docs.map((doc) => docToString(doc, { printWidth: FLAT_TEXT.length }))
+ ).toStrictEqual(Array.from({ length: docs.length }, () => FLAT_TEXT));
+});
| Unnecessary line breaks caused by `ifBreak` with group id nested in `group` with the same id
**Prettier 2.5.1**
[Playground link](https://prettier.io/playground/#N4Igxg9gdgLgprEAuEwA6UAEmBmEBOmAFJFAM4yZn5gAKAhjABaYQ6YDaAjAEwDMAGky8+AfRHj+QiTP7jpYiXyVdRAFj48AugEpM6LJgC+GIyAEgIABxgBLaGWSh6+fBADuDfAkcp6AG3d6AE9HCwAjfHowAGs4GABlegBbOAAZWyg4ZBwAsjgIqNj4hKtozIBzZBh8AFcCkDhk8LgAE1a2tPooCtr6CrgAMQJkxjse5BB6WpgIcxAmGGT-AHUmW3gyMrA4BJ8N2wA3DeDJsDIwkEz8-BhaKIrRnLyGgCsyAA8Eyv84AEVahB4M9-PkLGV8DdJuF6C1-PMrPhMjAVrZWsxkAAOAAM4Lc+RWUSsk0RcBuh2yFgAjoD4PdrL4pmQALRZNptebeGm2bz3fpPJC5UENfLJWzVOoin7-WnZQUvCwwWGo9FMZA8RVRWz+SoAYQgyQFjTIAFZ5rV8gAVWG+IVgkCHeoASSgHVgCTASJsAEFXQkYMFfiD8kYjEA)
<!-- prettier-ignore -->
```sh
--parser babel
```
**Input:**
<!-- prettier-ignore -->
```jsx
{
for (const srcPath of [123, 123_123_123, 123_123_123_1, 13_123_3123_31_432]) {
}
}
```
**Output:**
<!-- prettier-ignore -->
```jsx
{
for (const srcPath of [
123, 123_123_123, 123_123_123_1, 13_123_3123_31_432,
]) {
}
}
```
**Expected behavior:**
Should format as input.
Found this when playing with the "doc", in this case, the input is exactly 80 width. I think there should be bug in the doc print.
The test code from https://github.com/prettier/prettier/blob/2cde2dbf9d280260f8c499039d89419b21fa4dc0/tests/format/js/arrays/issue-10159.js#L3
@thorn0 You may interest.
| It's known: #10159
I'll close that issue in favor of this one because you have a better description here.
The root cause is described here: https://github.com/prettier/prettier/pull/10160#issuecomment-768668404
Sorry, totally forgot about that.
After debugging with your doc [here](https://github.com/prettier/prettier/pull/10160#issuecomment-768668404), I think the bug is in `fits()`, maybe also related to how [`line` works in `fits()`](https://github.com/prettier/prettier/blob/896970a18518bc31f1f15233b5ec612394453624/src/document/doc-printer.js#L246)
| "2022-02-24T10:35:18Z" | 2.6 | [] | [
"tests/format/typescript/interface/long-type-parameters/jsfmt.spec.js",
"tests/format/js/arrays/jsfmt.spec.js"
] | JavaScript | [] | [
"https://prettier.io/playground/#N4Igxg9gdgLgprEAuEwA6UAEmBmEBOmAFJFAM4yZn5gAKAhjABaYQ6YDaAjAEwDMAGky8+AfRHj+QiTP7jpYiXyVdRAFj48AugEpM6LJgC+GIyAEgIABxgBLaGWSh6+fBADuDfAkcp6AG3d6AE9HCwAjfHowAGs4GABlegBbOAAZWyg4ZBwAsjgIqNj4hKtozIBzZBh8AFcCkDhk8LgAE1a2tPooCtr6CrgAMQJkxjse5BB6WpgIcxAmGGT-AHUmW3gyMrA4BJ8N2wA3DeDJsDIwkEz8-BhaKIrRnLyGgCsyAA8Eyv84AEVahB4M9-PkLGV8DdJuF6C1-PMrPhMjAVrZWsxkAAOAAM4Lc+RWUSsk0RcBuh2yFgAjoD4PdrL4pmQALRZNptebeGm2bz3fpPJC5UENfLJWzVOoin7-WnZQUvCwwWGo9FMZA8RVRWz+SoAYQgyQFjTIAFZ5rV8gAVWG+IVgkCHeoASSgHVgCTASJsAEFXQkYMFfiD8kYjEA"
] |
prettier/prettier | 12,390 | prettier__prettier-12390 | [
"12389"
] | 542549c37216e9a32d555276089ee889c15c9fca | diff --git a/src/language-js/print/type-annotation.js b/src/language-js/print/type-annotation.js
--- a/src/language-js/print/type-annotation.js
+++ b/src/language-js/print/type-annotation.js
@@ -4,7 +4,7 @@ const {
printComments,
printDanglingComments,
} = require("../../main/comments.js");
-const { getLast, isNonEmptyArray } = require("../../common/util.js");
+const { isNonEmptyArray } = require("../../common/util.js");
const {
builders: { group, join, line, softline, indent, align, ifBreak },
} = require("../../document/index.js");
@@ -289,7 +289,6 @@ function printTupleType(path, options, print) {
const typesField = node.type === "TSTupleType" ? "elementTypes" : "types";
const types = node[typesField];
const isNonEmptyTuple = isNonEmptyArray(types);
- const hasRest = isNonEmptyTuple && getLast(types).type === "TSRestType";
const bracketsDelimiterLine = isNonEmptyTuple ? softline : "";
return group([
"[",
@@ -297,9 +296,7 @@ function printTupleType(path, options, print) {
bracketsDelimiterLine,
printArrayItems(path, options, typesField, print),
]),
- ifBreak(
- isNonEmptyTuple && shouldPrintComma(options, "all") && !hasRest ? "," : ""
- ),
+ ifBreak(isNonEmptyTuple && shouldPrintComma(options, "all") ? "," : ""),
printDanglingComments(path, options, /* sameIndent */ true),
bracketsDelimiterLine,
"]",
| diff --git a/tests/format/typescript/tuple/__snapshots__/jsfmt.spec.js.snap b/tests/format/typescript/tuple/__snapshots__/jsfmt.spec.js.snap
--- a/tests/format/typescript/tuple/__snapshots__/jsfmt.spec.js.snap
+++ b/tests/format/typescript/tuple/__snapshots__/jsfmt.spec.js.snap
@@ -1,91 +1,5 @@
// Jest Snapshot v1, https://goo.gl/fbAQLP
-exports[`no-trailing-comma-after-rest.ts - {"trailingComma":"all"} format 1`] = `
-====================================options=====================================
-parsers: ["typescript"]
-printWidth: 80
-trailingComma: "all"
- | printWidth
-=====================================input======================================
-type ValidateArgs = [
- {
- [key: string]: any;
- },
- string,
- string,
- ...string[],
-];
-
-=====================================output=====================================
-type ValidateArgs = [
- {
- [key: string]: any;
- },
- string,
- string,
- ...string[]
-];
-
-================================================================================
-`;
-
-exports[`no-trailing-comma-after-rest.ts - {"trailingComma":"none"} format 1`] = `
-====================================options=====================================
-parsers: ["typescript"]
-printWidth: 80
-trailingComma: "none"
- | printWidth
-=====================================input======================================
-type ValidateArgs = [
- {
- [key: string]: any;
- },
- string,
- string,
- ...string[],
-];
-
-=====================================output=====================================
-type ValidateArgs = [
- {
- [key: string]: any;
- },
- string,
- string,
- ...string[]
-];
-
-================================================================================
-`;
-
-exports[`no-trailing-comma-after-rest.ts format 1`] = `
-====================================options=====================================
-parsers: ["typescript"]
-printWidth: 80
- | printWidth
-=====================================input======================================
-type ValidateArgs = [
- {
- [key: string]: any;
- },
- string,
- string,
- ...string[],
-];
-
-=====================================output=====================================
-type ValidateArgs = [
- {
- [key: string]: any;
- },
- string,
- string,
- ...string[]
-];
-
-================================================================================
-`;
-
exports[`trailing-comma.ts - {"trailingComma":"all"} format 1`] = `
====================================options=====================================
parsers: ["typescript"]
@@ -273,6 +187,92 @@ type Foo =
================================================================================
`;
+exports[`trailing-comma-trailing-rest.ts - {"trailingComma":"all"} format 1`] = `
+====================================options=====================================
+parsers: ["typescript"]
+printWidth: 80
+trailingComma: "all"
+ | printWidth
+=====================================input======================================
+type ValidateArgs = [
+ {
+ [key: string]: any;
+ },
+ string,
+ string,
+ ...string[],
+];
+
+=====================================output=====================================
+type ValidateArgs = [
+ {
+ [key: string]: any;
+ },
+ string,
+ string,
+ ...string[],
+];
+
+================================================================================
+`;
+
+exports[`trailing-comma-trailing-rest.ts - {"trailingComma":"none"} format 1`] = `
+====================================options=====================================
+parsers: ["typescript"]
+printWidth: 80
+trailingComma: "none"
+ | printWidth
+=====================================input======================================
+type ValidateArgs = [
+ {
+ [key: string]: any;
+ },
+ string,
+ string,
+ ...string[],
+];
+
+=====================================output=====================================
+type ValidateArgs = [
+ {
+ [key: string]: any;
+ },
+ string,
+ string,
+ ...string[]
+];
+
+================================================================================
+`;
+
+exports[`trailing-comma-trailing-rest.ts format 1`] = `
+====================================options=====================================
+parsers: ["typescript"]
+printWidth: 80
+ | printWidth
+=====================================input======================================
+type ValidateArgs = [
+ {
+ [key: string]: any;
+ },
+ string,
+ string,
+ ...string[],
+];
+
+=====================================output=====================================
+type ValidateArgs = [
+ {
+ [key: string]: any;
+ },
+ string,
+ string,
+ ...string[]
+];
+
+================================================================================
+`;
+
exports[`tuple.ts - {"trailingComma":"all"} format 1`] = `
====================================options=====================================
parsers: ["typescript"]
diff --git a/tests/format/typescript/tuple/no-trailing-comma-after-rest.ts b/tests/format/typescript/tuple/trailing-comma-trailing-rest.ts
similarity index 100%
rename from tests/format/typescript/tuple/no-trailing-comma-after-rest.ts
rename to tests/format/typescript/tuple/trailing-comma-trailing-rest.ts
| Prettier removes trailing comma from multiline type with `trailingComma: all`
**Prettier 2.5.1**
[Playground link](https://prettier.io/playground/#N4Igxg9gdgLgprEAuEMCeAHOACAYgSwCcBnGAFUzgHV8YALANTkLQBloBzAOQEMBbHAF5swADpRs2HkmxQArnwBGzANziAvuPHos2AMpxIUACYUsNekxbso3fkJHjJimfKWqNW2JTz4oPABszalpGZjZOXgFsYQBtJ18SckoLMOtI+wAaBIA6PIMjUxTQqwjbKLhYgF1sqCqVEEyQCAwYfGhiZFAeQkIIAHcABR6ETpRA-p40TqbFQh4wAGs4GD17Vj84ZAAzQOI4Wfmllb0MBb8OZBhCOQOQOHdjYzhjVh5bOR4OOFwIQj4eDA2rZkCAeHIYBBGiA6DA+AEqHRaHBiGcwHADFBiLR8AA3WhoUFgYgzEB+faEGCDeYcAE7PZ3ABWxAAHnoLgE4ABFOQQeD0gL7JpnEjMUE6FFgQj4VrQjDS2A0Yz0ZAADgADMK+vsqPMMKD5SjmLitk0AI68+DUlpjMHEAC0UDgLxe0MIcAtRDg1K+dKQu0Fd32fHwVxuQY53MtW39DKaMB4iiVKqQACZ4-N8AELgBhCB8P1ggIBaFyfZkRNjANCkC424ASRMCFWUplMAAgiY9OhOQL9up1EA)
```sh
# Options:
--parser typescript
--trailingComma all
```
**Input:**
```jsx
type FirstTypeWithVeryLongName = {
a: number;
}
type SecondTypeWithVeryLongName = {
b: number;
}
type FinalTypeWithVeryLongName = [
FirstTypeWithVeryLongName,
...SecondTypeWithVeryLongName[],
];
```
**Output:**
```jsx
type FirstTypeWithVeryLongName = {
a: number;
};
type SecondTypeWithVeryLongName = {
b: number;
};
type FinalTypeWithVeryLongName = [
FirstTypeWithVeryLongName,
...SecondTypeWithVeryLongName[]
];
```
**Expected behavior:**
Prettier doesn't remove trailing comma from `FinalTypeWithVeryLongName` after `...SecondTypeWithVeryLongName[]` and thus doesn't conflict with `@typescript-eslint/comma-dangle` rule.
| https://github.com/prettier/prettier/issues/11684
It's also reproduced
This is a remnant of the old TS spec of placing rest elements only at the end of a tuple. I think we can fix this.
We can put leading/middle rest type in tuples types since TypeScript 4.2 https://www.typescriptlang.org/docs/handbook/release-notes/typescript-4-2.html#leadingmiddle-rest-elements-in-tuple-types | "2022-03-04T00:52:40Z" | 2.6 | [] | [
"tests/format/typescript/tuple/jsfmt.spec.js"
] | JavaScript | [] | [
"https://prettier.io/playground/#N4Igxg9gdgLgprEAuEMCeAHOACAYgSwCcBnGAFUzgHV8YALANTkLQBloBzAOQEMBbHAF5swADpRs2HkmxQArnwBGzANziAvuPHos2AMpxIUACYUsNekxbso3fkJHjJimfKWqNW2JTz4oPABszalpGZjZOXgFsYQBtJ18SckoLMOtI+wAaBIA6PIMjUxTQqwjbKLhYgF1sqCqVEEyQCAwYfGhiZFAeQkIIAHcABR6ETpRA-p40TqbFQh4wAGs4GD17Vj84ZAAzQOI4Wfmllb0MBb8OZBhCOQOQOHdjYzhjVh5bOR4OOFwIQj4eDA2rZkCAeHIYBBGiA6DA+AEqHRaHBiGcwHADFBiLR8AA3WhoUFgYgzEB+faEGCDeYcAE7PZ3ABWxAAHnoLgE4ABFOQQeD0gL7JpnEjMUE6FFgQj4VrQjDS2A0Yz0ZAADgADMK+vsqPMMKD5SjmLitk0AI68+DUlpjMHEAC0UDgLxe0MIcAtRDg1K+dKQu0Fd32fHwVxuQY53MtW39DKaMB4iiVKqQACZ4-N8AELgBhCB8P1ggIBaFyfZkRNjANCkC424ASRMCFWUplMAAgiY9OhOQL9up1EA"
] |
prettier/prettier | 12,508 | prettier__prettier-12508 | [
"12413"
] | 5ef82125a9c2ba0d6d7d5f9711075a2527ab47ee | diff --git a/src/language-js/print/assignment.js b/src/language-js/print/assignment.js
--- a/src/language-js/print/assignment.js
+++ b/src/language-js/print/assignment.js
@@ -441,7 +441,9 @@ function isCallExpressionWithComplexTypeArguments(node, print) {
firstArg.type === "TSUnionType" ||
firstArg.type === "UnionTypeAnnotation" ||
firstArg.type === "TSIntersectionType" ||
- firstArg.type === "IntersectionTypeAnnotation"
+ firstArg.type === "IntersectionTypeAnnotation" ||
+ firstArg.type === "TSTypeLiteral" ||
+ firstArg.type === "ObjectTypeAnnotation"
) {
return true;
}
| diff --git a/tests/format/flow/assignments/__snapshots__/jsfmt.spec.js.snap b/tests/format/flow/assignments/__snapshots__/jsfmt.spec.js.snap
--- a/tests/format/flow/assignments/__snapshots__/jsfmt.spec.js.snap
+++ b/tests/format/flow/assignments/__snapshots__/jsfmt.spec.js.snap
@@ -110,3 +110,52 @@ const map: Map<Function, Foo<S>> = new Map();
================================================================================
`;
+
+exports[`issue-12413.js format 1`] = `
+====================================options=====================================
+parsers: ["babel-flow", "flow"]
+printWidth: 80
+ | printWidth
+=====================================input======================================
+let emit =
+ defineEmits<{ (event: "ready", canvas: HTMLCanvasElement): void; (event:"resize",canvas:HTMLCanvasElement):void; }>();
+
+let abc =
+ func<{a:2,b:3,d:78,e:9,f:8,g:7,h:6,i:5,j:4,k:3,l:2,m:1,n:0,o:9,p:8,q:7,r:6,s:5,t:4,u:3,v:2,w:1,x:0,y:9,z:8}>();
+
+=====================================output=====================================
+let emit = defineEmits<{
+ (event: "ready", canvas: HTMLCanvasElement): void,
+ (event: "resize", canvas: HTMLCanvasElement): void,
+}>();
+
+let abc = func<{
+ a: 2,
+ b: 3,
+ d: 78,
+ e: 9,
+ f: 8,
+ g: 7,
+ h: 6,
+ i: 5,
+ j: 4,
+ k: 3,
+ l: 2,
+ m: 1,
+ n: 0,
+ o: 9,
+ p: 8,
+ q: 7,
+ r: 6,
+ s: 5,
+ t: 4,
+ u: 3,
+ v: 2,
+ w: 1,
+ x: 0,
+ y: 9,
+ z: 8,
+}>();
+
+================================================================================
+`;
diff --git a/tests/format/flow/assignments/issue-12413.js b/tests/format/flow/assignments/issue-12413.js
new file mode 100644
--- /dev/null
+++ b/tests/format/flow/assignments/issue-12413.js
@@ -0,0 +1,5 @@
+let emit =
+ defineEmits<{ (event: "ready", canvas: HTMLCanvasElement): void; (event:"resize",canvas:HTMLCanvasElement):void; }>();
+
+let abc =
+ func<{a:2,b:3,d:78,e:9,f:8,g:7,h:6,i:5,j:4,k:3,l:2,m:1,n:0,o:9,p:8,q:7,r:6,s:5,t:4,u:3,v:2,w:1,x:0,y:9,z:8}>();
diff --git a/tests/format/typescript/assignment/__snapshots__/jsfmt.spec.js.snap b/tests/format/typescript/assignment/__snapshots__/jsfmt.spec.js.snap
--- a/tests/format/typescript/assignment/__snapshots__/jsfmt.spec.js.snap
+++ b/tests/format/typescript/assignment/__snapshots__/jsfmt.spec.js.snap
@@ -443,6 +443,55 @@ const map: Map<Function, Foo<S>> = new Map();
================================================================================
`;
+exports[`issue-12413.ts format 1`] = `
+====================================options=====================================
+parsers: ["typescript"]
+printWidth: 80
+ | printWidth
+=====================================input======================================
+let emit =
+ defineEmits<{ (event: "ready", canvas: HTMLCanvasElement): void; (event:"resize",canvas:HTMLCanvasElement):void; }>();
+
+let abc =
+ func<{a:2,b:3,d:78,e:9,f:8,g:7,h:6,i:5,j:4,k:3,l:2,m:1,n:0,o:9,p:8,q:7,r:6,s:5,t:4,u:3,v:2,w:1,x:0,y:9,z:8}>();
+
+=====================================output=====================================
+let emit = defineEmits<{
+ (event: "ready", canvas: HTMLCanvasElement): void;
+ (event: "resize", canvas: HTMLCanvasElement): void;
+}>();
+
+let abc = func<{
+ a: 2;
+ b: 3;
+ d: 78;
+ e: 9;
+ f: 8;
+ g: 7;
+ h: 6;
+ i: 5;
+ j: 4;
+ k: 3;
+ l: 2;
+ m: 1;
+ n: 0;
+ o: 9;
+ p: 8;
+ q: 7;
+ r: 6;
+ s: 5;
+ t: 4;
+ u: 3;
+ v: 2;
+ w: 1;
+ x: 0;
+ y: 9;
+ z: 8;
+}>();
+
+================================================================================
+`;
+
exports[`lone-arg.ts format 1`] = `
====================================options=====================================
parsers: ["typescript"]
diff --git a/tests/format/typescript/assignment/issue-12413.ts b/tests/format/typescript/assignment/issue-12413.ts
new file mode 100644
--- /dev/null
+++ b/tests/format/typescript/assignment/issue-12413.ts
@@ -0,0 +1,5 @@
+let emit =
+ defineEmits<{ (event: "ready", canvas: HTMLCanvasElement): void; (event:"resize",canvas:HTMLCanvasElement):void; }>();
+
+let abc =
+ func<{a:2,b:3,d:78,e:9,f:8,g:7,h:6,i:5,j:4,k:3,l:2,m:1,n:0,o:9,p:8,q:7,r:6,s:5,t:4,u:3,v:2,w:1,x:0,y:9,z:8}>();
| Long lines of code need to be formatted twice
**Prettier 2.5.1**
[Playground link](https://prettier.io/playground/#N4Igxg9gdgLgprEAuEAbOMAEcC2BLLAXgB0pNzMATOAMzyjgFF8YBnAHmEwAo4A3BDCSZiIAE5wAhpQCeogDSYwkqH0mthACQAqAWQAyAYRVrWjdDkEBKYXwh5KAbh79BSURNZ4AXnAXLVdSQdA2NAswtrJDsHZwBfAD5uK0cQeRAIAAcYPGhWZFBJMTEIAHcABSKEfJRJVFLJGXz0gCMxSTAAawwAZUlLfXo4ZBo61jhW9q7ezI76AHNkGDEAVwmQXBa4SmpKfRV5lcl5uAAxCDEcSRgcqEXalZgINJAACxgcVAB1V4I4VlmYDgPWqBDwfAIMmQ4FYzRA9HGYhg5Xa8yuIzG6wAVqwAB49BboACKKwg8AxqHG6VmYkR0JgMky-zAYjw2RemVZsC+Dhgr2QAA4AAzUkrjL7tTLQzn-OBiAQvACOpPgKKyNRA6gAtAxttsXhJlXgJCjjuikKNKetxvglqtrYS4CSycMLZj0jBJC0eZQ+cgAEwe9p4VALQwQHDmjasACsLxW420XpqlqpID4awAklBqLAeiy2TAAII5noM9AU8ZxOJAA), [second playground link](https://prettier.io/playground/#N4Igxg9gdgLgprEAuEAbOMAEcC2BLLAXgB0pNMATOAMzyjgFF8YBnAHmFPPIAo4A3BDCSZiIAE5wAhhQCeYgDSYwUqPyksRACQAqAWQAyAYVXqWDdDiEBKEfwh4KAbi7c+g2CLGSWeAF5wisqmGtr6xiHmljZ2Ds6uAL4AfDzWTiAKIBAADjB40CzIoFLi4hAA7gAKJQiFKFKo5VKyhZkARuJSYADWGADKUlYGdHDI1A0scO2dPf3ZXXQA5sgw4gCuUyC4bXAUVBQGqotrUotwAGIQ4jhSMHlQy-VrMBAZIAAWMDioAOrvBHAWPMwHA+rUCHh+ARZMhwCxWiA6JNxDBKp1FjcxhNNgArFgADz6S3QAEU1hB4FjUJNMvNxMjYTBZNlAWBxHhcm9suzYD9HDB3sgABwABlpZUmP062Vh3MBcHEgjeAEdyfA0Tk6iANABaei7XZvSSqvCSNGnTFIcbUzaTfArda24lwMkU0ZW7GZGBSNp8igC5AAJi9nTwqCWRggOEtWxYAFY3mtJjofXVrTSQPwNgBJKBUWB9NkcmAAQTzfSZ6CpkwSCSAA)
<!-- prettier-ignore -->
```sh
--parser typescript
```
**Input:**
<!-- prettier-ignore -->
```tsx
let emit =
defineEmits<{ (event: "ready", canvas: HTMLCanvasElement): void; (event:"resize",canvas:HTMLCanvasElement):void; }>();
```
**Output:**
<!-- prettier-ignore -->
```tsx
let emit =
defineEmits<{
(event: "ready", canvas: HTMLCanvasElement): void;
(event: "resize", canvas: HTMLCanvasElement): void;
}>();
```
**Output upon reformatting:**
<!-- prettier-ignore -->
```tsx
let emit = defineEmits<{
(event: "ready", canvas: HTMLCanvasElement): void;
(event: "resize", canvas: HTMLCanvasElement): void;
}>();
```
**Issue:**
Upon reformatting the code, Prettier gives a different response than the original.
**Expected behavior:**
Prettier should only require a single formatting to correctly format the code.
| I've noticed this is often an issue when combining TypeScript generics with object literals. Here's a second example for reference:
**Prettier 2.5.1**
[Playground link](https://prettier.io/playground/#N4Igxg9gdgLgprEAuEAzArlMMCW0AEGWAPACoB8AFAJT7AC+AOlMwDZwz4CGARmALzN8w4UTDFgXJACYANDyQBmWQBMkAdgAcsuEgCcs1Em0BzDbIAWSAGyycSAKyyAVkgAssgNZLZrGbIBbJABGWSgkAAZZCH1ZAAdjWQBHcwAnG1kAZ0dZGHdZdB8AN38AdxDZAA9I2QBPWIAvY3oqagBuEFkQCDjcaEzkUC5U1IhSgAVhhAGULlZSrlqBrp5UrjBPDgBlLgC4ABkcKDhkVDnMuBW1je249aOTZBhU9EuQOACeOBUVb-2uKAmdBcExwABiEFSAS4MFwgOQIC46BgEE6IAsMACrAA6hYcPBMncwHAttN8TgivjagiwJlliAjhdUjBxmsTNDTuc3s5MpUtg92ABFdAQeCc1gXLp3VJMhEwWpxOCZMCpHC9NFxVWwbE4FQwCzITRRECaiAXbFrOIIzVKuCpIonLpJEXwVk9GaIzIAWmO32+aNScGdOEDrJBHKQZwlbwuARwTxeMYFcGFopOka5XRgvB1eoN-hAzy4OFYDwAwhAAhH3pkHGj0BdSLwZlHJSAiq8AJJQX6wLYqtUwACCPa28vY4ou9HoQA), [Second playground link](https://prettier.io/playground/#N4Igxg9gdgLgprEAuEAzArlMMCW0AEGWAPACoB8AFAJT7AC+AOlMwDZwz4CGARmPgF5m+QpjDFgwkdyT4ATAG4pInrIDMSqNPwATWQHYAHJu1xZAThPTUs48vwBzA1ZEALWQDYX+HLICs3gBWsgAs3gDW6t6ssor2ALayAIzeULIADN4QFt4ADrbeAI7O9gBOnt4Azv7eMKHe6FH2AG6x3gDuyd4AHhneAJ459gBeBVL0VNSaIAA0IBC5uNCVyKBcpaUQ7QAK6wgrKFys7Vz9K3M8pVxg4RwAylzxcAAyOFBwyKhHlXAXVzf3XLXN4OZAwUroX4gODxHhwHQ6eHPLhQBzoLgOOAAMQgpXiXBguFRyBAXHQMAgsxArhg8VYAHVXDh4JUgWA4Hd9sycM1mf0SWBKucQG8fqUYNsrg58Z9vlDApVuncQewAIroCDwWWsH5zIGlMUkmD9XJwSpgUo4RZU3KW2D0nA6GCuZCGdJ6zY-elXXIk21muClZofOaFDXwSULA6kyoAWne8PhVNKcDDOBTkoxMqQXx1UJ+8RwYIh+ZVcHVmo+OblcxgvAdTpdSDktauOFYIIAwhB4tnoZU-FT0D9SLwDrndSBmpCAJJQRGwO4Wq0wACC87uxvY2p+9HoQA)
<!-- prettier-ignore -->
```sh
--parser typescript
```
**Input:**
<!-- prettier-ignore -->
```tsx
function func<T>() {}
let abc=
func<{a:2,b:3,d:78,e:9,f:8,g:7,h:6,i:5,j:4,k:3,l:2,m:1,n:0,o:9,p:8,q:7,r:6,s:5,t:4,u:3,v:2,w:1,x:0,y:9,z:8}>();
```
**Output:**
<!-- prettier-ignore -->
```tsx
function func<T>() {}
let abc =
func<{
a: 2;
b: 3;
d: 78;
e: 9;
f: 8;
g: 7;
h: 6;
i: 5;
j: 4;
k: 3;
l: 2;
m: 1;
n: 0;
o: 9;
p: 8;
q: 7;
r: 6;
s: 5;
t: 4;
u: 3;
v: 2;
w: 1;
x: 0;
y: 9;
z: 8;
}>();
```
**Output upon reformatting:**
<!-- prettier-ignore -->
```tsx
function func<T>() {}
let abc = func<{
a: 2;
b: 3;
d: 78;
e: 9;
f: 8;
g: 7;
h: 6;
i: 5;
j: 4;
k: 3;
l: 2;
m: 1;
n: 0;
o: 9;
p: 8;
q: 7;
r: 6;
s: 5;
t: 4;
u: 3;
v: 2;
w: 1;
x: 0;
y: 9;
z: 8;
}>();
```
**Expected behavior:**
This is another example where Prettier requires two passes to format the code correctly. | "2022-03-22T16:59:30Z" | 2.7 | [] | [
"tests/format/flow/assignments/jsfmt.spec.js",
"tests/format/typescript/assignment/jsfmt.spec.js"
] | JavaScript | [] | [
"https://prettier.io/playground/#N4Igxg9gdgLgprEAuEAbOMAEcC2BLLAXgB0pNMATOAMzyjgFF8YBnAHmFPPIAo4A3BDCSZiIAE5wAhhQCeYgDSYwUqPyksRACQAqAWQAyAYVXqWDdDiEBKEfwh4KAbi7c+g2CLGSWeAF5wisqmGtr6xiHmljZ2Ds6uAL4AfDzWTiAKIBAADjB40CzIoFLi4hAA7gAKJQiFKFKo5VKyhZkARuJSYADWGADKUlYGdHDI1A0scO2dPf3ZXXQA5sgw4gCuUyC4bXAUVBQGqotrUotwAGIQ4jhSMHlQy-VrMBAZIAAWMDioAOrvBHAWPMwHA+rUCHh+ARZMhwCxWiA6JNxDBKp1FjcxhNNgArFgADz6S3QAEU1hB4FjUJNMvNxMjYTBZNlAWBxHhcm9suzYD9HDB3sgABwABlpZUmP062Vh3MBcHEgjeAEdyfA0Tk6iANABaei7XZvSSqvCSNGnTFIcbUzaTfArda24lwMkU0ZW7GZGBSNp8igC5AAJi9nTwqCWRggOEtWxYAFY3mtJjofXVrTSQPwNgBJKBUWB9NkcmAAQTzfSZ6CpkwSCSAA",
"https://prettier.io/playground/#N4Igxg9gdgLgprEAuEAbOMAEcC2BLLAXgB0pNzMATOAMzyjgFF8YBnAHmEwAo4A3BDCSZiIAE5wAhpQCeogDSYwkqH0mthACQAqAWQAyAYRVrWjdDkEBKYXwh5KAbh79BSURNZ4AXnAXLVdSQdA2NAswtrJDsHZwBfAD5uK0cQeRAIAAcYPGhWZFBJMTEIAHcABSKEfJRJVFLJGXz0gCMxSTAAawwAZUlLfXo4ZBo61jhW9q7ezI76AHNkGDEAVwmQXBa4SmpKfRV5lcl5uAAxCDEcSRgcqEXalZgINJAACxgcVAB1V4I4VlmYDgPWqBDwfAIMmQ4FYzRA9HGYhg5Xa8yuIzG6wAVqwAB49BboACKKwg8AxqHG6VmYkR0JgMky-zAYjw2RemVZsC+Dhgr2QAA4AAzUkrjL7tTLQzn-OBiAQvACOpPgKKyNRA6gAtAxttsXhJlXgJCjjuikKNKetxvglqtrYS4CSycMLZj0jBJC0eZQ+cgAEwe9p4VALQwQHDmjasACsLxW420XpqlqpID4awAklBqLAeiy2TAAII5noM9AU8ZxOJAA"
] |
prettier/prettier | 12,584 | prettier__prettier-12584 | [
"12414"
] | c50f2bbe3075757840bbd7ed941647a9bde84266 | diff --git a/src/language-html/embed.js b/src/language-html/embed.js
--- a/src/language-html/embed.js
+++ b/src/language-html/embed.js
@@ -127,11 +127,14 @@ function printEmbeddedAttributeValue(node, htmlTextToDoc, options) {
if (isKeyMatched(vueEventBindingPatterns)) {
const value = getValue();
+ const parser = isVueEventBindingExpression(value)
+ ? "__js_expression"
+ : options.__should_parse_vue_template_with_ts
+ ? "__vue_ts_event_binding"
+ : "__vue_event_binding";
return printMaybeHug(
attributeTextToDoc(value, {
- parser: isVueEventBindingExpression(value)
- ? "__js_expression"
- : "__vue_event_binding",
+ parser,
})
);
}
@@ -318,7 +321,9 @@ function embed(path, print, textToDoc, options) {
textToDocOptions.parser = "__ng_interpolation";
textToDocOptions.trailingComma = "none";
} else if (options.parser === "vue") {
- textToDocOptions.parser = "__vue_expression";
+ textToDocOptions.parser = options.__should_parse_vue_template_with_ts
+ ? "__vue_ts_expression"
+ : "__vue_expression";
} else {
textToDocOptions.parser = "__js_expression";
}
diff --git a/src/language-html/print-preprocess.js b/src/language-html/print-preprocess.js
--- a/src/language-html/print-preprocess.js
+++ b/src/language-html/print-preprocess.js
@@ -14,6 +14,7 @@ const {
isLeadingSpaceSensitiveNode,
isTrailingSpaceSensitiveNode,
isWhitespaceSensitiveNode,
+ isVueScriptTag,
} = require("./utils/index.js");
const PREPROCESS_PIPELINE = [
@@ -27,6 +28,7 @@ const PREPROCESS_PIPELINE = [
addHasHtmComponentClosingTag,
addIsSpaceSensitive,
mergeSimpleElementIntoText,
+ markTsScript,
];
function preprocess(ast, options) {
@@ -408,4 +410,19 @@ function addIsSpaceSensitive(ast, options) {
});
}
+function markTsScript(ast, options) {
+ if (options.parser === "vue") {
+ const vueScriptTag = ast.children.find((child) =>
+ isVueScriptTag(child, options)
+ );
+ if (!vueScriptTag) {
+ return;
+ }
+ const { lang } = vueScriptTag.attrMap;
+ if (lang === "ts" || lang === "typescript") {
+ options.__should_parse_vue_template_with_ts = true;
+ }
+ }
+}
+
module.exports = preprocess;
diff --git a/src/language-html/utils/index.js b/src/language-html/utils/index.js
--- a/src/language-html/utils/index.js
+++ b/src/language-html/utils/index.js
@@ -638,6 +638,10 @@ function getTextValueParts(node, value = node.value) {
: getDocParts(join(line, splitByHtmlWhitespace(value)));
}
+function isVueScriptTag(node, options) {
+ return isVueSfcBlock(node, options) && node.name === "script";
+}
+
module.exports = {
htmlTrim,
htmlTrimPreserveIndentation,
@@ -657,6 +661,7 @@ module.exports = {
inferScriptParser,
isVueCustomBlock,
isVueNonHtmlBlock,
+ isVueScriptTag,
isVueSlotAttribute,
isVueSfcBindingsAttribute,
isDanglingSpaceSensitiveNode,
diff --git a/src/language-js/parse/babel.js b/src/language-js/parse/babel.js
--- a/src/language-js/parse/babel.js
+++ b/src/language-js/parse/babel.js
@@ -170,6 +170,11 @@ const parseEstree = createParse(
);
const parseExpression = createParse("parseExpression", appendPlugins(["jsx"]));
+const parseTSExpression = createParse(
+ "parseExpression",
+ appendPlugins(["typescript"])
+);
+
// Error codes are defined in
// - https://github.com/babel/babel/blob/v7.14.0/packages/babel-parser/src/parser/error-message.js
// - https://github.com/babel/babel/blob/v7.14.0/packages/babel-parser/src/plugins/typescript/index.js#L69-L153
@@ -228,21 +233,27 @@ const allowedMessageCodes = new Set([
]);
const babel = createParser(parse);
+const babelTs = createParser(parseTypeScript);
const babelExpression = createParser(parseExpression);
+const babelTSExpression = createParser(parseTSExpression);
// Export as a plugin so we can reuse the same bundle for UMD loading
module.exports = {
parsers: {
babel,
"babel-flow": createParser(parseFlow),
- "babel-ts": createParser(parseTypeScript),
+ "babel-ts": babelTs,
...jsonParsers,
/** @internal */
__js_expression: babelExpression,
/** for vue filter */
__vue_expression: babelExpression,
+ /** for vue filter written in TS */
+ __vue_ts_expression: babelTSExpression,
/** for vue event binding to handle semicolon */
__vue_event_binding: babel,
+ /** for vue event binding written in TS to handle semicolon */
+ __vue_ts_event_binding: babelTs,
/** verify that we can print this AST */
__babel_estree: createParser(parseEstree),
},
diff --git a/src/language-js/parse/parsers.js b/src/language-js/parse/parsers.js
--- a/src/language-js/parse/parsers.js
+++ b/src/language-js/parse/parsers.js
@@ -26,9 +26,15 @@ module.exports = {
get __vue_expression() {
return require("./babel.js").parsers.__vue_expression;
},
+ get __vue_ts_expression() {
+ return require("./babel.js").parsers.__vue_ts_expression;
+ },
get __vue_event_binding() {
return require("./babel.js").parsers.__vue_event_binding;
},
+ get __vue_ts_event_binding() {
+ return require("./babel.js").parsers.__vue_ts_event_binding;
+ },
// JS - Flow
get flow() {
return require("./flow.js").parsers.flow;
diff --git a/src/language-js/print-preprocess.js b/src/language-js/print-preprocess.js
--- a/src/language-js/print-preprocess.js
+++ b/src/language-js/print-preprocess.js
@@ -7,6 +7,7 @@ function preprocess(ast, options) {
case "json-stringify":
case "__js_expression":
case "__vue_expression":
+ case "__vue_ts_expression":
return {
...ast,
type: options.parser.startsWith("__") ? "JsExpressionRoot" : "JsonRoot",
diff --git a/src/language-js/printer-estree.js b/src/language-js/printer-estree.js
--- a/src/language-js/printer-estree.js
+++ b/src/language-js/printer-estree.js
@@ -218,7 +218,10 @@ function printPathNoParens(path, options, print, args) {
return [printDirective(node.expression, options), semi];
}
- if (options.parser === "__vue_event_binding") {
+ if (
+ options.parser === "__vue_event_binding" ||
+ options.parser === "__vue_ts_event_binding"
+ ) {
const parent = path.getParentNode();
if (
parent.type === "Program" &&
diff --git a/src/main/comments.js b/src/main/comments.js
--- a/src/main/comments.js
+++ b/src/main/comments.js
@@ -200,7 +200,8 @@ function attach(comments, ast, text, options) {
options.parser === "json" ||
options.parser === "json5" ||
options.parser === "__js_expression" ||
- options.parser === "__vue_expression"
+ options.parser === "__vue_expression" ||
+ options.parser === "__vue_ts_expression"
) {
if (locStart(comment) - locStart(ast) <= 0) {
addLeadingComment(ast, comment);
| diff --git a/tests/format/vue/ts-event-binding/__snapshots__/jsfmt.spec.js.snap b/tests/format/vue/ts-event-binding/__snapshots__/jsfmt.spec.js.snap
new file mode 100644
--- /dev/null
+++ b/tests/format/vue/ts-event-binding/__snapshots__/jsfmt.spec.js.snap
@@ -0,0 +1,43 @@
+// Jest Snapshot v1, https://goo.gl/fbAQLP
+
+exports[`basic.vue format 1`] = `
+====================================options=====================================
+parsers: ["vue"]
+printWidth: 80
+ | printWidth
+=====================================input======================================
+<script setup lang="ts">
+let x = 1;
+function log(...args) {
+ console.log(...args);
+}
+</script>
+
+<template>
+ <div @click="if (x === 1 as number) { log('hello') } else { log('nonhello') };">{{ x }}</div>
+</template>
+
+=====================================output=====================================
+<script setup lang="ts">
+let x = 1;
+function log(...args) {
+ console.log(...args);
+}
+</script>
+
+<template>
+ <div
+ @click="
+ if (x === (1 as number)) {
+ log('hello');
+ } else {
+ log('nonhello');
+ }
+ "
+ >
+ {{ x }}
+ </div>
+</template>
+
+================================================================================
+`;
diff --git a/tests/format/vue/ts-event-binding/basic.vue b/tests/format/vue/ts-event-binding/basic.vue
new file mode 100644
--- /dev/null
+++ b/tests/format/vue/ts-event-binding/basic.vue
@@ -0,0 +1,10 @@
+<script setup lang="ts">
+let x = 1;
+function log(...args) {
+ console.log(...args);
+}
+</script>
+
+<template>
+ <div @click="if (x === 1 as number) { log('hello') } else { log('nonhello') };">{{ x }}</div>
+</template>
diff --git a/tests/format/vue/ts-event-binding/jsfmt.spec.js b/tests/format/vue/ts-event-binding/jsfmt.spec.js
new file mode 100644
--- /dev/null
+++ b/tests/format/vue/ts-event-binding/jsfmt.spec.js
@@ -0,0 +1 @@
+run_spec(__dirname, ["vue"]);
diff --git a/tests/format/vue/ts-expression/__snapshots__/jsfmt.spec.js.snap b/tests/format/vue/ts-expression/__snapshots__/jsfmt.spec.js.snap
new file mode 100644
--- /dev/null
+++ b/tests/format/vue/ts-expression/__snapshots__/jsfmt.spec.js.snap
@@ -0,0 +1,45 @@
+// Jest Snapshot v1, https://goo.gl/fbAQLP
+
+exports[`basic.vue format 1`] = `
+====================================options=====================================
+parsers: ["vue"]
+printWidth: 80
+ | printWidth
+=====================================input======================================
+<template>
+ <p v-if="isFolder(file)">{{ ( file as mymodule.Folder ).deadline }}</p>
+</template>
+
+<script lang="ts"></script>
+
+=====================================output=====================================
+<template>
+ <p v-if="isFolder(file)">{{ (file as mymodule.Folder).deadline }}</p>
+</template>
+
+<script lang="ts"></script>
+
+================================================================================
+`;
+
+exports[`not-working-with-non-ts-script.vue format 1`] = `
+====================================options=====================================
+parsers: ["vue"]
+printWidth: 80
+ | printWidth
+=====================================input======================================
+<template>
+ <p v-if="isFolder(file)">{{ ( file as mymodule.Folder ).deadline }}</p>
+</template>
+
+<script></script>
+
+=====================================output=====================================
+<template>
+ <p v-if="isFolder(file)">{{ ( file as mymodule.Folder ).deadline }}</p>
+</template>
+
+<script></script>
+
+================================================================================
+`;
diff --git a/tests/format/vue/ts-expression/basic.vue b/tests/format/vue/ts-expression/basic.vue
new file mode 100644
--- /dev/null
+++ b/tests/format/vue/ts-expression/basic.vue
@@ -0,0 +1,5 @@
+<template>
+ <p v-if="isFolder(file)">{{ ( file as mymodule.Folder ).deadline }}</p>
+</template>
+
+<script lang="ts"></script>
diff --git a/tests/format/vue/ts-expression/jsfmt.spec.js b/tests/format/vue/ts-expression/jsfmt.spec.js
new file mode 100644
--- /dev/null
+++ b/tests/format/vue/ts-expression/jsfmt.spec.js
@@ -0,0 +1 @@
+run_spec(__dirname, ["vue"]);
diff --git a/tests/format/vue/ts-expression/not-working-with-non-ts-script.vue b/tests/format/vue/ts-expression/not-working-with-non-ts-script.vue
new file mode 100644
--- /dev/null
+++ b/tests/format/vue/ts-expression/not-working-with-non-ts-script.vue
@@ -0,0 +1,5 @@
+<template>
+ <p v-if="isFolder(file)">{{ ( file as mymodule.Folder ).deadline }}</p>
+</template>
+
+<script></script>
| "as" TypeScript keyword yields error within Vue template
<!--
BEFORE SUBMITTING AN ISSUE:
1. Search for your issue on GitHub: https://github.com/prettier/prettier/issues
A large number of opened issues are duplicates of existing issues.
If someone has already opened an issue for what you are experiencing,
you do not need to open a new issue — please add a 👍 reaction to the
existing issue instead.
2. We get a lot of requests for adding options, but Prettier is
built on the principle of being opinionated about code formatting.
This means we add options only in the case of strict technical necessity.
Find out more: https://prettier.io/docs/en/option-philosophy.html
Don't fill the form below manually! Let a program create a report for you:
1. Go to https://prettier.io/playground
2. Paste your code and set options
3. Press the "Report issue" button in the lower right
-->
**Prettier 2.5.1**
[Playground link](https://prettier.io/playground/#N4Igxg9gdgLgprEAuEAeeBbADgGwIbwB8AOlKlgAQBuAtAJYBmAvMSHQM4BiEOAJnACcAFAzo44ASlaFgwCiLFwKedhQwBPDBF4BXcQDpufQRP388vHHShKAvrdQB6LIQpv3pJ5lwE4JKJ7sYAJ0WDAU+FAA5iwgMOzSno5BIWH+gcGh4exwMDqUkTGs8YlkyZlppIEw6uIUQRBYcLwReNGxQewJIP5O7DXihCAANCCNMHTQ7MigeAICEADuAApzCNMoeDiLeOrTowBGAnhgANa5AMp4GHAAMtZwyAxbOYfHZ5dYJ9ZRyDACOjgozgGAOzX4vFubSiOjwUTg3AEGAIE2iyBAeB0MAgIxAAAsYBgcAB1PF0eDsL5gOAXdbkuhUcnqdFgLq46w5AQwZbHKLIp4vIEgABW7AAHhcfuIAIo6CDwAU4V4gL4CTnoqiA3FYEKwYl0XgwPHIACMACYAAyjHUQHLE45YdE6uCcqiPUYARzl8B5jQ2GPYNBszWauIEcC9dHDPLh-KQzyVQpyGDofwBSalcFl8seSH+gNGMDwB31huNSAALIXjmIfgBhCAYOMgF0AVlxOhyABVixsE8rNXAAJJQfiwC4VGAAQVHFwGuf7cHsQA)
```sh
# Options:
--parser vue
--embedded-language-formatting auto
--single-quote
--tab-width 4
--print-width 120
```
**Input:**
```vue
<template>
<p v-if="isFolder(file)">{{ (file as mymodule.Folder).deadline }}</p>
</template>
<script lang="ts">
</script>
<script setup lang="ts">
</script>
<style scoped lang="scss">
</style>
```
**Output:**
```vue
SyntaxError: Unexpected token, expected "," (1:8)
> 1 | (file as mymodule.Folder).deadline
| ^
```
**Expected behavior:**
`prettier` should recognise TypeScript patterns such as the `as` keyword within Vue templates. I believe this issue has to do with the `--embedded-language-formatting` system not being able to parse the keyword, as when this option is set off the error is not given; however, setting this option off is not a suitable solution as it is quite useful.
| Any reference that this is allowed in Vue SFC?
@fisker I don't know about any Vue doc covering this specific use case, so official support on this is unknown to me. However, AFAIK Vue 3 fully supports TypeScript and the `as` keyword is part of the language. Furthermore, my app runs just fine with a bunch of `as` keywords in the templates, including an identical example to the one given above (which is not the same as Vue officially supporting it, which is what you are asking, but still).
For more context, prettier not only throws an error with the `{{ }}` syntax, but also with things like:
```vue
<p v-if="isFolder(file as mymodule.Folder)">test</p>
```
@fisker never mind, it is officially supported. [Here](https://vuejs.org/guide/typescript/overview.html#typescript-in-templates) you have an example in the official docs.
Thank you.
To add a bit more context: using the `as` keyword within the `<script>` section does work fine, so the bug is only happening with embedded TS within templates.
> We need to update the vue expression parser to allow typescript syntax, luckily we have babel-ts, so we don't need use typescript parser.
Original posted https://github.com/prettier/plugin-pug/issues/365#issuecomment-1085622997
That's a related issue from plugin. | "2022-04-02T18:06:41Z" | 2.7 | [] | [
"tests/format/vue/ts-event-binding/jsfmt.spec.js",
"tests/format/vue/ts-expression/jsfmt.spec.js"
] | JavaScript | [] | [
"https://prettier.io/playground/#N4Igxg9gdgLgprEAuEAeeBbADgGwIbwB8AOlKlgAQBuAtAJYBmAvMSHQM4BiEOAJnACcAFAzo44ASlaFgwCiLFwKedhQwBPDBF4BXcQDpufQRP388vHHShKAvrdQB6LIQpv3pJ5lwE4JKJ7sYAJ0WDAU+FAA5iwgMOzSno5BIWH+gcGh4exwMDqUkTGs8YlkyZlppIEw6uIUQRBYcLwReNGxQewJIP5O7DXihCAANCCNMHTQ7MigeAICEADuAApzCNMoeDiLeOrTowBGAnhgANa5AMp4GHAAMtZwyAxbOYfHZ5dYJ9ZRyDACOjgozgGAOzX4vFubSiOjwUTg3AEGAIE2iyBAeB0MAgIxAAAsYBgcAB1PF0eDsL5gOAXdbkuhUcnqdFgLq46w5AQwZbHKLIp4vIEgABW7AAHhcfuIAIo6CDwAU4V4gL4CTnoqiA3FYEKwYl0XgwPHIACMACYAAyjHUQHLE45YdE6uCcqiPUYARzl8B5jQ2GPYNBszWauIEcC9dHDPLh-KQzyVQpyGDofwBSalcFl8seSH+gNGMDwB31huNSAALIXjmIfgBhCAYOMgF0AVlxOhyABVixsE8rNXAAJJQfiwC4VGAAQVHFwGuf7cHsQA",
"https://prettier.io/playground"
] |
prettier/prettier | 12,608 | prettier__prettier-12608 | [
"12607"
] | f98c197b66db7491021f5d1cb65d137aa5d7ac68 | diff --git a/src/language-graphql/printer-graphql.js b/src/language-graphql/printer-graphql.js
--- a/src/language-graphql/printer-graphql.js
+++ b/src/language-graphql/printer-graphql.js
@@ -125,13 +125,15 @@ function genericPrint(path, options, print) {
}
case "StringValue": {
if (node.block) {
- return [
- '"""',
- hardline,
- join(hardline, node.value.replace(/"""/g, "\\$&").split("\n")),
+ const lines = node.value.replace(/"""/g, "\\$&").split("\n");
+ if (lines.length === 1) {
+ lines[0] = lines[0].trim();
+ }
+
+ return join(
hardline,
- '"""',
- ];
+ ['"""', ...(lines.length > 0 ? lines : []), '"""'].filter(Boolean)
+ );
}
return [
'"',
@@ -572,7 +574,13 @@ function printInterfaces(path, options, print) {
return parts;
}
-function clean(/*node, newNode , parent*/) {}
+function clean(node, newNode /* , parent */) {
+ // We print single line `""" string """` as multiple line string,
+ // and the parser ignores space in multiple line string
+ if (node.kind === "StringValue" && node.block && !node.value.includes("\n")) {
+ newNode.value = newNode.value.trim();
+ }
+}
clean.ignoredProperties = new Set(["loc", "comments"]);
function hasPrettierIgnore(path) {
| diff --git a/tests/format/graphql/string/__snapshots__/jsfmt.spec.js.snap b/tests/format/graphql/string/__snapshots__/jsfmt.spec.js.snap
--- a/tests/format/graphql/string/__snapshots__/jsfmt.spec.js.snap
+++ b/tests/format/graphql/string/__snapshots__/jsfmt.spec.js.snap
@@ -1,5 +1,82 @@
// Jest Snapshot v1, https://goo.gl/fbAQLP
+exports[`description.graphql format 1`] = `
+====================================options=====================================
+parsers: ["graphql"]
+printWidth: 80
+ | printWidth
+=====================================input======================================
+""" Customer """
+type Person {name: String}
+
+""" Customer """
+type Person {name: String}
+
+"""
+
+ Customer
+
+
+"""
+type Person {name: String}
+
+""""""
+type Person {name: String}
+
+""" """
+type Person {name: String}
+
+"""
+ 1
+ 2
+"""
+type Person {name: String}
+
+=====================================output=====================================
+"""
+Customer
+"""
+type Person {
+ name: String
+}
+
+"""
+Customer
+"""
+type Person {
+ name: String
+}
+
+"""
+Customer
+"""
+type Person {
+ name: String
+}
+
+"""
+"""
+type Person {
+ name: String
+}
+
+"""
+"""
+type Person {
+ name: String
+}
+
+"""
+ 1
+2
+"""
+type Person {
+ name: String
+}
+
+================================================================================
+`;
+
exports[`string.graphql format 1`] = `
====================================options=====================================
parsers: ["graphql"]
@@ -59,6 +136,14 @@ one: string
foo(input: {multiline: "ab\\ncd"}) { id }
}
+{
+ foo(input: {multiline: """ foo """}) { id }
+}
+
+{
+ foo(input: {multiline: """ """}) { id }
+}
+
=====================================output=====================================
query X($a: Int) @relay(meta: "{\\"lowPri\\": true}") {
a
@@ -129,5 +214,28 @@ input Input {
}
}
+{
+ foo(
+ input: {
+ multiline: """
+ foo
+ """
+ }
+ ) {
+ id
+ }
+}
+
+{
+ foo(
+ input: {
+ multiline: """
+ """
+ }
+ ) {
+ id
+ }
+}
+
================================================================================
`;
diff --git a/tests/format/graphql/string/description.graphql b/tests/format/graphql/string/description.graphql
new file mode 100644
--- /dev/null
+++ b/tests/format/graphql/string/description.graphql
@@ -0,0 +1,25 @@
+""" Customer """
+type Person {name: String}
+
+""" Customer """
+type Person {name: String}
+
+"""
+
+ Customer
+
+
+"""
+type Person {name: String}
+
+""""""
+type Person {name: String}
+
+""" """
+type Person {name: String}
+
+"""
+ 1
+ 2
+"""
+type Person {name: String}
diff --git a/tests/format/graphql/string/string.graphql b/tests/format/graphql/string/string.graphql
--- a/tests/format/graphql/string/string.graphql
+++ b/tests/format/graphql/string/string.graphql
@@ -50,3 +50,11 @@ one: string
{
foo(input: {multiline: "ab\ncd"}) { id }
}
+
+{
+ foo(input: {multiline: """ foo """}) { id }
+}
+
+{
+ foo(input: {multiline: """ """}) { id }
+}
| Prettier GraphQL `description` leading space bug
**Prettier 2.6.2**
[Playground link](https://prettier.io/playground/#N4Igxg9gdgLgprEAuEAdNGAEApCALKTAEQjk3QrVgE8AHMgBTgCcBnaTYVQzKAQwC2cJJgDKMZgEsoAc24BfEABoQEWjEnRWyUH2bMIAdwZ6E2lHwA2hvtW0qARsz5gA1nBijBcADLS4yABmVqxwjs5uHqK0LtIyyBIArmEgcAIOcAAmmVk+fLKJfDJwAGIQzAJ8MBqyyCB8iTAQyiB4MAKWAOp4kvCsMWBwoma9kgBuvdR1YKz2INKhzDAMzjKVQSEpAFasAB6icZZwAIqJEPAblqEqMWwsdTLOtHgAjpYttFKwnZKZMHjIAAcAAYbgZQp0nnVPnBFmMAioXmd4Cs1OZ6qwALRQOBZLItZhwJGSQkrIrrJDBK4pUICSQJZjJFSsQ4nZEBSmbFQwPgOH5-AFIABM3OckkscQAwhABBTUqwAKwtRKhAAqvPMVOuIDGyQAklAcrBRGApOoAIKG8TUI6XULyeRAA)
<!-- prettier-ignore -->
```sh
--parser graphql
```
**Input:**
<!-- prettier-ignore -->
```graphql
""" Customer """
type Person {
name: String
}
```
**Output:**
<!-- prettier-ignore -->
```graphql
"""
Customer
"""
type Person {
name: String
}
```
**Second Output:**
<!-- prettier-ignore -->
```graphql
"""
Customer
"""
type Person {
name: String
}
```
**Expected behavior:**
```gql
"""
Customer
"""
type Person {
name: String
}
```
Seems like the leading space in the GraphQL `description` is not removed.
Running `prettier` twice (2x) will yield the expected result.
Also created a tiny repo with this bug: https://github.com/chimurai/prettier-graphql-comment-bug
| "2022-04-06T19:35:54Z" | 2.7 | [] | [
"tests/format/graphql/string/jsfmt.spec.js"
] | JavaScript | [] | [
"https://prettier.io/playground/#N4Igxg9gdgLgprEAuEAdNGAEApCALKTAEQjk3QrVgE8AHMgBTgCcBnaTYVQzKAQwC2cJJgDKMZgEsoAc24BfEABoQEWjEnRWyUH2bMIAdwZ6E2lHwA2hvtW0qARsz5gA1nBijBcADLS4yABmVqxwjs5uHqK0LtIyyBIArmEgcAIOcAAmmVk+fLKJfDJwAGIQzAJ8MBqyyCB8iTAQyiB4MAKWAOp4kvCsMWBwoma9kgBuvdR1YKz2INKhzDAMzjKVQSEpAFasAB6icZZwAIqJEPAblqEqMWwsdTLOtHgAjpYttFKwnZKZMHjIAAcAAYbgZQp0nnVPnBFmMAioXmd4Cs1OZ6qwALRQOBZLItZhwJGSQkrIrrJDBK4pUICSQJZjJFSsQ4nZEBSmbFQwPgOH5-AFIABM3OckkscQAwhABBTUqwAKwtRKhAAqvPMVOuIDGyQAklAcrBRGApOoAIKG8TUI6XULyeRAA"
] |
|
prettier/prettier | 12,746 | prettier__prettier-12746 | [
"12738"
] | e23b89b8c70da643eed9adcfc6b815c7f4432dc2 | diff --git a/src/language-js/comments.js b/src/language-js/comments.js
--- a/src/language-js/comments.js
+++ b/src/language-js/comments.js
@@ -65,7 +65,7 @@ function handleOwnLineComment(context) {
handleForComments,
handleUnionTypeComments,
handleOnlyComments,
- handleImportDeclarationComments,
+ handleModuleSpecifiersComments,
handleAssignmentPatternComments,
handleMethodNameComments,
handleLabeledStatementComments,
@@ -787,17 +787,20 @@ function handleForComments({ comment, enclosingNode }) {
return false;
}
-function handleImportDeclarationComments({
+function handleModuleSpecifiersComments({
comment,
precedingNode,
enclosingNode,
text,
}) {
+ const isImportDeclaration =
+ precedingNode?.type === "ImportSpecifier" &&
+ enclosingNode?.type === "ImportDeclaration";
+ const isExportDeclaration =
+ precedingNode?.type === "ExportSpecifier" &&
+ enclosingNode?.type === "ExportNamedDeclaration";
if (
- precedingNode &&
- precedingNode.type === "ImportSpecifier" &&
- enclosingNode &&
- enclosingNode.type === "ImportDeclaration" &&
+ (isImportDeclaration || isExportDeclaration) &&
hasNewline(text, locEnd(comment))
) {
addTrailingComment(precedingNode, comment);
| diff --git a/tests/format/js/export/__snapshots__/jsfmt.spec.js.snap b/tests/format/js/export/__snapshots__/jsfmt.spec.js.snap
--- a/tests/format/js/export/__snapshots__/jsfmt.spec.js.snap
+++ b/tests/format/js/export/__snapshots__/jsfmt.spec.js.snap
@@ -1,5 +1,114 @@
// Jest Snapshot v1, https://goo.gl/fbAQLP
+exports[`blank-line-between-specifiers.js - {"bracketSpacing":false} format 1`] = `
+====================================options=====================================
+bracketSpacing: false
+parsers: ["babel", "flow", "typescript"]
+printWidth: 80
+ | printWidth
+=====================================input======================================
+export {
+ // a
+ foo1,
+
+ // b
+ bar1,
+ baz1,
+} from "mod";
+
+const foo2 = 1;
+const bar2 = 1;
+const baz2 = 1;
+
+export {
+ // a
+ foo2,
+
+ // b
+ bar2,
+ baz2,
+};
+
+=====================================output=====================================
+export {
+ // a
+ foo1,
+
+ // b
+ bar1,
+ baz1,
+} from "mod";
+
+const foo2 = 1;
+const bar2 = 1;
+const baz2 = 1;
+
+export {
+ // a
+ foo2,
+
+ // b
+ bar2,
+ baz2,
+};
+
+================================================================================
+`;
+
+exports[`blank-line-between-specifiers.js format 1`] = `
+====================================options=====================================
+parsers: ["babel", "flow", "typescript"]
+printWidth: 80
+ | printWidth
+=====================================input======================================
+export {
+ // a
+ foo1,
+
+ // b
+ bar1,
+ baz1,
+} from "mod";
+
+const foo2 = 1;
+const bar2 = 1;
+const baz2 = 1;
+
+export {
+ // a
+ foo2,
+
+ // b
+ bar2,
+ baz2,
+};
+
+=====================================output=====================================
+export {
+ // a
+ foo1,
+
+ // b
+ bar1,
+ baz1,
+} from "mod";
+
+const foo2 = 1;
+const bar2 = 1;
+const baz2 = 1;
+
+export {
+ // a
+ foo2,
+
+ // b
+ bar2,
+ baz2,
+};
+
+================================================================================
+`;
+
exports[`bracket.js - {"bracketSpacing":false} format 1`] = `
====================================options=====================================
bracketSpacing: false
diff --git a/tests/format/js/export/blank-line-between-specifiers.js b/tests/format/js/export/blank-line-between-specifiers.js
new file mode 100644
--- /dev/null
+++ b/tests/format/js/export/blank-line-between-specifiers.js
@@ -0,0 +1,21 @@
+export {
+ // a
+ foo1,
+
+ // b
+ bar1,
+ baz1,
+} from "mod";
+
+const foo2 = 1;
+const bar2 = 1;
+const baz2 = 1;
+
+export {
+ // a
+ foo2,
+
+ // b
+ bar2,
+ baz2,
+};
| Blank line between export specifiers not preserved
**Prettier 2.6.2**
[Playground link](https://prettier.io/playground/#N4Igxg9gdgLgprEAuEcAeAHCAnGACYAHSjzwHoy8BDY0gMwggBpa9WK8AjVzq7FklyoAvAQF8A3CCYgIGGAEtoAZ2Sg+2CAHcACnwSqUVADZaqAT1UzO2KmADWcGAGUqAWzgAZBVDjI6Jspw1rYOTs4Ydj4A5sgw2ACuwahunHAAJukZnlRQ0QlU0XAAYjhuVDCKecggVAkwENIgABYwbsYA6s0K8MqRYHDOBj0KAG495jVgylYgPkG4OrbR5f6ByQBWymjOMcZwAIoJEPBrxkEykdgLNbxpxk0Y2D4wHQrpMM3IABwADJeaIIdWwYGpPOALUZ+GQAR2O8CWckMtWUAFpfBkMk1sHA4QocUtCqskAFzskgm4FHFEuS9od4X4SesZDAqJw3h8vkgAEws2wKYwxADCEDcxNQygArE0EkEACpswyki4gUZJACSUCysGcYGe8gAglrnDBzPszkExGIgA)
<!-- prettier-ignore -->
```sh
--parser babel
```
**Input:**
<!-- prettier-ignore -->
```jsx
export {
// a
foo,
// b
bar,
baz,
};
```
**Output:**
<!-- prettier-ignore -->
```jsx
export {
// a
foo,
// b
bar,
baz,
};
```
**Expected behavior:**
Keep blank line.
Should work as [import and other similar cases](https://prettier.io/playground/#N4Igxg9gdgLgprEAuEcAeAHCAnGACYAHSjzwHoy8BDY0gMwggBpa9WK8AjVzq7FklyoAvAQF88dbBAC2eAOQMI84sXRZcBdpRqClA0tq48+BoaOISpshUpVRiASxkb8RQR131GZo90G8-CYWUFbScoqM9sSQUADObkZekj6sfiZBASLieAC8KRAgTCAQGDCO0HHIoHzSAO4ACnwIVShUADZ1VACeVcWc2FRgANZwMADKVDJwADKOUHDIdB1xcP2DI2PjGEPzAObIMNgArmuoMpxwACZX1zNUUHvHVHtwAGI4MlQw5Y-IIFRjjBCsUABYwGTtADqoMc8DiOzAcHGLThjgAbnDuv8wHE+iB5qtcA1BnsvksVmcAFZxNDjfbtOAARWOEHgFPaq2KO2wRP+vEu7SKIAw2HmMChjiuMFByAAHAAGbnSVZQwYYf6iuBE9GLYoAR1Z8BJpVaALiAFoFtdrsLsHBDY57SSXuSkMtOWdVjJHIcTl6GcyjYt3ZTijAqJxJdLZUgAEzhwaOdr7ADCsjdqDiAFZhcdVgAVSOtD1ckDo04ASSgt1g4zAYrKAEEa+MYN1GRzVmIxEA)
| "2022-04-29T06:46:56Z" | 2.7 | [] | [
"tests/format/js/export/jsfmt.spec.js"
] | JavaScript | [] | [
"https://prettier.io/playground/#N4Igxg9gdgLgprEAuEcAeAHCAnGACYAHSjzwHoy8BDY0gMwggBpa9WK8AjVzq7FklyoAvAQF88dbBAC2eAOQMI84sXRZcBdpRqClA0tq48+BoaOISpshUpVRiASxkb8RQR131GZo90G8-CYWUFbScoqM9sSQUADObkZekj6sfiZBASLieAC8KRAgTCAQGDCO0HHIoHzSAO4ACnwIVShUADZ1VACeVcWc2FRgANZwMADKVDJwADKOUHDIdB1xcP2DI2PjGEPzAObIMNgArmuoMpxwACZX1zNUUHvHVHtwAGI4MlQw5Y-IIFRjjBCsUABYwGTtADqoMc8DiOzAcHGLThjgAbnDuv8wHE+iB5qtcA1BnsvksVmcAFZxNDjfbtOAARWOEHgFPaq2KO2wRP+vEu7SKIAw2HmMChjiuMFByAAHAAGbnSVZQwYYf6iuBE9GLYoAR1Z8BJpVaALiAFoFtdrsLsHBDY57SSXuSkMtOWdVjJHIcTl6GcyjYt3ZTijAqJxJdLZUgAEzhwaOdr7ADCsjdqDiAFZhcdVgAVSOtD1ckDo04ASSgt1g4zAYrKAEEa+MYN1GRzVmIxEA",
"https://prettier.io/playground/#N4Igxg9gdgLgprEAuEcAeAHCAnGACYAHSjzwHoy8BDY0gMwggBpa9WK8AjVzq7FklyoAvAQF8A3CCYgIGGAEtoAZ2Sg+2CAHcACnwSqUVADZaqAT1UzO2KmADWcGAGUqAWzgAZBVDjI6Jspw1rYOTs4Ydj4A5sgw2ACuwahunHAAJukZnlRQ0QlU0XAAYjhuVDCKecggVAkwENIgABYwbsYA6s0K8MqRYHDOBj0KAG495jVgylYgPkG4OrbR5f6ByQBWymjOMcZwAIoJEPBrxkEykdgLNbxpxk0Y2D4wHQrpMM3IABwADJeaIIdWwYGpPOALUZ+GQAR2O8CWckMtWUAFpfBkMk1sHA4QocUtCqskAFzskgm4FHFEuS9od4X4SesZDAqJw3h8vkgAEws2wKYwxADCEDcxNQygArE0EkEACpswyki4gUZJACSUCysGcYGe8gAglrnDBzPszkExGIgA"
] |
|
prettier/prettier | 12,860 | prettier__prettier-12860 | [
"12750"
] | 77e5589591d0d558ad3c75d10a8bdd554af3c550 | diff --git a/src/language-js/comments.js b/src/language-js/comments.js
--- a/src/language-js/comments.js
+++ b/src/language-js/comments.js
@@ -61,7 +61,6 @@ function handleOwnLineComment(context) {
handleWhileComments,
handleTryStatementComments,
handleClassComments,
- handleImportSpecifierComments,
handleForComments,
handleUnionTypeComments,
handleOnlyComments,
@@ -82,7 +81,7 @@ function handleEndOfLineComment(context) {
handleClosureTypeCastComments,
handleLastFunctionArgComments,
handleConditionalExpressionComments,
- handleImportSpecifierComments,
+ handleModuleSpecifiersComments,
handleIfStatementComments,
handleWhileComments,
handleTryStatementComments,
@@ -623,14 +622,6 @@ function handleLastFunctionArgComments({
return false;
}
-function handleImportSpecifierComments({ comment, enclosingNode }) {
- if (enclosingNode?.type === "ImportSpecifier") {
- addLeadingComment(enclosingNode, comment);
- return true;
- }
- return false;
-}
-
function handleLabeledStatementComments({ comment, enclosingNode }) {
if (enclosingNode?.type === "LabeledStatement") {
addLeadingComment(enclosingNode, comment);
@@ -768,6 +759,14 @@ function handleModuleSpecifiersComments({
enclosingNode,
text,
}) {
+ if (
+ enclosingNode?.type === "ImportSpecifier" ||
+ enclosingNode?.type === "ExportSpecifier"
+ ) {
+ addLeadingComment(enclosingNode, comment);
+ return true;
+ }
+
const isImportDeclaration =
precedingNode?.type === "ImportSpecifier" &&
enclosingNode?.type === "ImportDeclaration";
| diff --git a/tests/format/js/comments/__snapshots__/jsfmt.spec.js.snap b/tests/format/js/comments/__snapshots__/jsfmt.spec.js.snap
--- a/tests/format/js/comments/__snapshots__/jsfmt.spec.js.snap
+++ b/tests/format/js/comments/__snapshots__/jsfmt.spec.js.snap
@@ -1474,6 +1474,24 @@ export {
barr, // comment
}
+const foooo = ''
+const barrr = ''
+export {
+ foooo,
+
+ barrr as // comment
+ baz,
+} from 'foo'
+
+const fooooo = ''
+const barrrr = ''
+export {
+ fooooo,
+
+ barrrr as // comment
+ bazz,
+}
+
=====================================output=====================================
export //comment
{}
@@ -1498,6 +1516,22 @@ export {
barr, // comment
}
+const foooo = ""
+const barrr = ""
+export {
+ foooo,
+ // comment
+ barrr as baz,
+} from "foo"
+
+const fooooo = ""
+const barrrr = ""
+export {
+ fooooo,
+ // comment
+ barrrr as bazz,
+}
+
================================================================================
`;
@@ -1530,6 +1564,24 @@ export {
barr, // comment
}
+const foooo = ''
+const barrr = ''
+export {
+ foooo,
+
+ barrr as // comment
+ baz,
+} from 'foo'
+
+const fooooo = ''
+const barrrr = ''
+export {
+ fooooo,
+
+ barrrr as // comment
+ bazz,
+}
+
=====================================output=====================================
export //comment
{};
@@ -1554,6 +1606,141 @@ export {
barr, // comment
};
+const foooo = "";
+const barrr = "";
+export {
+ foooo,
+ // comment
+ barrr as baz,
+} from "foo";
+
+const fooooo = "";
+const barrrr = "";
+export {
+ fooooo,
+ // comment
+ barrrr as bazz,
+};
+
+================================================================================
+`;
+
+exports[`export-and-import.js - {"semi":false} format 1`] = `
+====================================options=====================================
+parsers: ["babel", "flow", "typescript"]
+printWidth: 80
+semi: false
+ | printWidth
+=====================================input======================================
+// These are tests to compare comment formats in \`export\` and \`import\`.
+
+export {
+ foo,
+
+ bar as // comment
+ baz,
+} from 'foo'
+
+const fooo = ""
+const barr = ""
+
+export {
+ fooo,
+
+ barr as // comment
+ bazz,
+}
+
+import {
+ foo,
+
+ bar as // comment
+ baz,
+} from 'foo'
+
+=====================================output=====================================
+// These are tests to compare comment formats in \`export\` and \`import\`.
+
+export {
+ foo,
+ // comment
+ bar as baz,
+} from "foo"
+
+const fooo = ""
+const barr = ""
+
+export {
+ fooo,
+ // comment
+ barr as bazz,
+}
+
+import {
+ foo,
+ // comment
+ bar as baz,
+} from "foo"
+
+================================================================================
+`;
+
+exports[`export-and-import.js format 1`] = `
+====================================options=====================================
+parsers: ["babel", "flow", "typescript"]
+printWidth: 80
+ | printWidth
+=====================================input======================================
+// These are tests to compare comment formats in \`export\` and \`import\`.
+
+export {
+ foo,
+
+ bar as // comment
+ baz,
+} from 'foo'
+
+const fooo = ""
+const barr = ""
+
+export {
+ fooo,
+
+ barr as // comment
+ bazz,
+}
+
+import {
+ foo,
+
+ bar as // comment
+ baz,
+} from 'foo'
+
+=====================================output=====================================
+// These are tests to compare comment formats in \`export\` and \`import\`.
+
+export {
+ foo,
+ // comment
+ bar as baz,
+} from "foo";
+
+const fooo = "";
+const barr = "";
+
+export {
+ fooo,
+ // comment
+ barr as bazz,
+};
+
+import {
+ foo,
+ // comment
+ bar as baz,
+} from "foo";
+
================================================================================
`;
diff --git a/tests/format/js/comments/export-and-import.js b/tests/format/js/comments/export-and-import.js
new file mode 100644
--- /dev/null
+++ b/tests/format/js/comments/export-and-import.js
@@ -0,0 +1,25 @@
+// These are tests to compare comment formats in `export` and `import`.
+
+export {
+ foo,
+
+ bar as // comment
+ baz,
+} from 'foo'
+
+const fooo = ""
+const barr = ""
+
+export {
+ fooo,
+
+ barr as // comment
+ bazz,
+}
+
+import {
+ foo,
+
+ bar as // comment
+ baz,
+} from 'foo'
diff --git a/tests/format/js/comments/export.js b/tests/format/js/comments/export.js
--- a/tests/format/js/comments/export.js
+++ b/tests/format/js/comments/export.js
@@ -20,3 +20,21 @@ export {
fooo, // comment
barr, // comment
}
+
+const foooo = ''
+const barrr = ''
+export {
+ foooo,
+
+ barrr as // comment
+ baz,
+} from 'foo'
+
+const fooooo = ''
+const barrrr = ''
+export {
+ fooooo,
+
+ barrrr as // comment
+ bazz,
+}
| Inconsistent between comments inside export and import specifiers
**Prettier 2.6.2**
[Playground link](https://prettier.io/playground/#N4Igxg9gdgLgprEAuEcAeAHCAnGACYAHSjzwDMIIAaY02vAIwENs8mBnUgei70gFt+CGMUIj8zAF40oAX3LYI-PAHIKEFaKjosuAvXUy6JRizac8PPkqGxR409OKytAS3678RE4fr1mrBzcvAK2IlBiYo4y8mSKymqUKiBUIBAYMK7Q7MigLIoA7gAKLAg5KEwANgVMAJ45qQzYTGAA1nAwAMpMQgAyrtrIZFXscI3NbR2dGC0DAObIMNgArmOo-AxwACZb271MUHPLTHNwAGI4-EwwmYfIIEzLMBApIAAWMPyVAOpvrvDsGZgOCdMr-VwAN3+tXuYHYDRAA1GuCKzTmVyGIzWACt2GhOvNKnAAIrLCDwTGVUapGbYZH3ZibSqvDDYAYwb6uLYwN7IAAcAAYaYpRt9mhh7qy4MiIXBXgBHMnwVHpcoPdgAWm0222r2wcEVrn1qJOGKQwypa1G-FcixWVsJJKVcvNWNSMCYDE53N5SAATO7mq5KvMAMI2Jj3aUAVley1GABVPeULdSQBDVgBJKC7WCdMBsjIAQRznRgtSJlNGslkQA)
<!-- prettier-ignore -->
```sh
--parser babel
```
**Input:**
<!-- prettier-ignore -->
```jsx
export {
foo,
bar as // comment
baz,
} from 'foo'
export {
foo,
bar as // comment
baz,
}
import {
foo,
bar as // comment
baz,
} from 'foo'
```
**Output:**
<!-- prettier-ignore -->
```jsx
export {
foo,
bar as baz, // comment
} from "foo";
export {
foo,
bar as baz, // comment
};
import {
foo,
// comment
bar as baz,
} from "foo";
```
**Expected behavior:**
This is edge case, but worth to investigate where the difference is.
[#12746](https://deploy-preview-12746--prettier.netlify.app/playground/#N4Igxg9gdgLgprEAuEcAeAHCAnGACYAHSjzwDMIIAaY02vAIwENs8mBnUgei70gFt+CGMUIj8zAF40oAX3LYI-PAHIKEFaKjosuAvXUy6JRizac8PPkqGxR409OKytAS3678RE4fr1mrBzcvAK2IlBiYo4y8mSKymqUKiBUIBAYMK7Q7MigLIoA7gAKLAg5KEwANgVMAJ45qQzYTGAA1nAwAMpMQgAyrtrIZFXscI3NbR2dGC0DAObIMNgArmOo-AxwACZb271MUHPLTHNwAGI4-EwwmYfIIEzLMBApIAAWMPyVAOpvrvDsGZgOCdMr-VwAN3+tXuYHYDRAA1GuCKzTmVyGIzWACt2GhOvNKnAAIrLCDwTGVUapGbYZH3ZibSqvDDYAYwb6uLYwN7IAAcAAYaYpRt9mhh7qy4MiIXBXgBHMnwVHpcoPdgAWm0222r2wcEVrn1qJOGKQwypa1G-FcixWVsJJKVcvNWNSMCYDE53N5SAATO7mq5KvMAMI2Jj3aUAVley1GABVPeULdSQBDVgBJKC7WCdMBsjIAQRznRgtSJlNGslkQA) didn't change output.
| "2022-05-11T05:07:44Z" | 2.7 | [] | [
"tests/format/js/comments/jsfmt.spec.js"
] | JavaScript | [] | [
"https://deploy-preview-12746--prettier.netlify.app/playground/#N4Igxg9gdgLgprEAuEcAeAHCAnGACYAHSjzwDMIIAaY02vAIwENs8mBnUgei70gFt+CGMUIj8zAF40oAX3LYI-PAHIKEFaKjosuAvXUy6JRizac8PPkqGxR409OKytAS3678RE4fr1mrBzcvAK2IlBiYo4y8mSKymqUKiBUIBAYMK7Q7MigLIoA7gAKLAg5KEwANgVMAJ45qQzYTGAA1nAwAMpMQgAyrtrIZFXscI3NbR2dGC0DAObIMNgArmOo-AxwACZb271MUHPLTHNwAGI4-EwwmYfIIEzLMBApIAAWMPyVAOpvrvDsGZgOCdMr-VwAN3+tXuYHYDRAA1GuCKzTmVyGIzWACt2GhOvNKnAAIrLCDwTGVUapGbYZH3ZibSqvDDYAYwb6uLYwN7IAAcAAYaYpRt9mhh7qy4MiIXBXgBHMnwVHpcoPdgAWm0222r2wcEVrn1qJOGKQwypa1G-FcixWVsJJKVcvNWNSMCYDE53N5SAATO7mq5KvMAMI2Jj3aUAVley1GABVPeULdSQBDVgBJKC7WCdMBsjIAQRznRgtSJlNGslkQA",
"https://prettier.io/playground/#N4Igxg9gdgLgprEAuEcAeAHCAnGACYAHSjzwDMIIAaY02vAIwENs8mBnUgei70gFt+CGMUIj8zAF40oAX3LYI-PAHIKEFaKjosuAvXUy6JRizac8PPkqGxR409OKytAS3678RE4fr1mrBzcvAK2IlBiYo4y8mSKymqUKiBUIBAYMK7Q7MigLIoA7gAKLAg5KEwANgVMAJ45qQzYTGAA1nAwAMpMQgAyrtrIZFXscI3NbR2dGC0DAObIMNgArmOo-AxwACZb271MUHPLTHNwAGI4-EwwmYfIIEzLMBApIAAWMPyVAOpvrvDsGZgOCdMr-VwAN3+tXuYHYDRAA1GuCKzTmVyGIzWACt2GhOvNKnAAIrLCDwTGVUapGbYZH3ZibSqvDDYAYwb6uLYwN7IAAcAAYaYpRt9mhh7qy4MiIXBXgBHMnwVHpcoPdgAWm0222r2wcEVrn1qJOGKQwypa1G-FcixWVsJJKVcvNWNSMCYDE53N5SAATO7mq5KvMAMI2Jj3aUAVley1GABVPeULdSQBDVgBJKC7WCdMBsjIAQRznRgtSJlNGslkQA"
] |
|
prettier/prettier | 12,895 | prettier__prettier-12895 | [
"12787"
] | 83ec3c36e34864470a6cb88889d837c46777ab7d | diff --git a/src/language-html/print/tag.js b/src/language-html/print/tag.js
--- a/src/language-html/print/tag.js
+++ b/src/language-html/print/tag.js
@@ -17,6 +17,7 @@ const {
isPreLikeNode,
hasPrettierIgnore,
shouldPreserveContent,
+ isVueSfcBlock,
} = require("../utils/index.js");
function printClosingTag(node, options) {
@@ -251,8 +252,11 @@ function printAttributes(path, options, print) {
node.attrs[0].fullName === "src" &&
node.children.length === 0;
- const attributeLine =
- options.singleAttributePerLine && node.attrs.length > 1 ? hardline : line;
+ const shouldPrintAttributePerLine =
+ options.singleAttributePerLine &&
+ node.attrs.length > 1 &&
+ !isVueSfcBlock(node, options);
+ const attributeLine = shouldPrintAttributePerLine ? hardline : line;
/** @type {Doc[]} */
const parts = [
diff --git a/src/language-html/utils/index.js b/src/language-html/utils/index.js
--- a/src/language-html/utils/index.js
+++ b/src/language-html/utils/index.js
@@ -674,6 +674,7 @@ module.exports = {
isVueScriptTag,
isVueSlotAttribute,
isVueSfcBindingsAttribute,
+ isVueSfcBlock,
isDanglingSpaceSensitiveNode,
isIndentationSensitiveNode,
isLeadingSpaceSensitiveNode,
| diff --git a/tests/format/vue/single-attribute-per-line/__snapshots__/jsfmt.spec.js.snap b/tests/format/vue/single-attribute-per-line/__snapshots__/jsfmt.spec.js.snap
--- a/tests/format/vue/single-attribute-per-line/__snapshots__/jsfmt.spec.js.snap
+++ b/tests/format/vue/single-attribute-per-line/__snapshots__/jsfmt.spec.js.snap
@@ -1,5 +1,152 @@
// Jest Snapshot v1, https://goo.gl/fbAQLP
+exports[`sfc-blocks.vue - {"singleAttributePerLine":true} format 1`] = `
+====================================options=====================================
+parsers: ["vue"]
+printWidth: 80
+singleAttributePerLine: true
+ | printWidth
+=====================================input======================================
+<script setup lang="ts">
+console.log("hello")
+</script>
+
+<style scoped lang="scss">
+p {
+ color: red;
+}
+</style>
+
+<unknown-block foo bar="bar" baz long_long_long_long_attribute></unknown-block>
+
+<script lang="ts" src="./long_long_long_long_long_long_file_path.ts">
+</script>
+
+<script lang="ts" src="./long_long_long_long_long_long_long_file_path.ts">
+</script>
+
+<script lang="ts" src="./short">
+</script>
+
+<template lang="pug" src="./long_long_long_long_long_long_file_path.pug">
+</template>
+
+<template lang="pug" src="./long_long_long_long_long_long_long_long_file_path.pug">
+</template>
+
+<template lang="pug" src="./short">
+</template>
+
+=====================================output=====================================
+<script setup lang="ts">
+console.log("hello");
+</script>
+
+<style scoped lang="scss">
+p {
+ color: red;
+}
+</style>
+
+<unknown-block foo bar="bar" baz long_long_long_long_attribute></unknown-block>
+
+<script lang="ts" src="./long_long_long_long_long_long_file_path.ts"></script>
+
+<script
+ lang="ts"
+ src="./long_long_long_long_long_long_long_file_path.ts"
+></script>
+
+<script lang="ts" src="./short"></script>
+
+<template lang="pug" src="./long_long_long_long_long_long_file_path.pug">
+</template>
+
+<template
+ lang="pug"
+ src="./long_long_long_long_long_long_long_long_file_path.pug"
+>
+</template>
+
+<template lang="pug" src="./short">
+</template>
+
+================================================================================
+`;
+
+exports[`sfc-blocks.vue format 1`] = `
+====================================options=====================================
+parsers: ["vue"]
+printWidth: 80
+ | printWidth
+=====================================input======================================
+<script setup lang="ts">
+console.log("hello")
+</script>
+
+<style scoped lang="scss">
+p {
+ color: red;
+}
+</style>
+
+<unknown-block foo bar="bar" baz long_long_long_long_attribute></unknown-block>
+
+<script lang="ts" src="./long_long_long_long_long_long_file_path.ts">
+</script>
+
+<script lang="ts" src="./long_long_long_long_long_long_long_file_path.ts">
+</script>
+
+<script lang="ts" src="./short">
+</script>
+
+<template lang="pug" src="./long_long_long_long_long_long_file_path.pug">
+</template>
+
+<template lang="pug" src="./long_long_long_long_long_long_long_long_file_path.pug">
+</template>
+
+<template lang="pug" src="./short">
+</template>
+
+=====================================output=====================================
+<script setup lang="ts">
+console.log("hello");
+</script>
+
+<style scoped lang="scss">
+p {
+ color: red;
+}
+</style>
+
+<unknown-block foo bar="bar" baz long_long_long_long_attribute></unknown-block>
+
+<script lang="ts" src="./long_long_long_long_long_long_file_path.ts"></script>
+
+<script
+ lang="ts"
+ src="./long_long_long_long_long_long_long_file_path.ts"
+></script>
+
+<script lang="ts" src="./short"></script>
+
+<template lang="pug" src="./long_long_long_long_long_long_file_path.pug">
+</template>
+
+<template
+ lang="pug"
+ src="./long_long_long_long_long_long_long_long_file_path.pug"
+>
+</template>
+
+<template lang="pug" src="./short">
+</template>
+
+================================================================================
+`;
+
exports[`single-attribute-per-line.vue - {"singleAttributePerLine":true} format 1`] = `
====================================options=====================================
parsers: ["vue"]
diff --git a/tests/format/vue/single-attribute-per-line/sfc-blocks.vue b/tests/format/vue/single-attribute-per-line/sfc-blocks.vue
new file mode 100644
--- /dev/null
+++ b/tests/format/vue/single-attribute-per-line/sfc-blocks.vue
@@ -0,0 +1,29 @@
+<script setup lang="ts">
+console.log("hello")
+</script>
+
+<style scoped lang="scss">
+p {
+ color: red;
+}
+</style>
+
+<unknown-block foo bar="bar" baz long_long_long_long_attribute></unknown-block>
+
+<script lang="ts" src="./long_long_long_long_long_long_file_path.ts">
+</script>
+
+<script lang="ts" src="./long_long_long_long_long_long_long_file_path.ts">
+</script>
+
+<script lang="ts" src="./short">
+</script>
+
+<template lang="pug" src="./long_long_long_long_long_long_file_path.pug">
+</template>
+
+<template lang="pug" src="./long_long_long_long_long_long_long_long_file_path.pug">
+</template>
+
+<template lang="pug" src="./short">
+</template>
| Avoid tags for the singleAttributePerLine rule
Is it possible to specify a list of tags to avoid for this rule? I'm working with Vue 3 and I would LOVE to keep both of this tags in one line:
```
<script
setup
lang="ts"
>
...
</script>
```
```
<style
scoped
lang="scss"
>
...
</style>
```
| Can we disable it for `script` and `style`?
me too,how resolve it
> We can disable this just like we did with indentation (`vueIndentScriptAndStyle`).
answer error
me too,how resolve it
> me too,how resolve it
i dont know. I've given up using prettier | "2022-05-20T03:26:24Z" | 2.7 | [] | [
"tests/format/vue/single-attribute-per-line/jsfmt.spec.js"
] | JavaScript | [] | [] |
prettier/prettier | 12,930 | prettier__prettier-12930 | [
"12926"
] | 3147244f55e6e0aafd2cc6fa5875f7c8cfa8e4e3 | diff --git a/src/language-js/print/typescript.js b/src/language-js/print/typescript.js
--- a/src/language-js/print/typescript.js
+++ b/src/language-js/print/typescript.js
@@ -413,7 +413,12 @@ function printTypescript(path, options, print) {
return parts;
case "TSEnumMember":
- parts.push(print("id"));
+ if (node.computed) {
+ parts.push("[", print("id"), "]");
+ } else {
+ parts.push(print("id"));
+ }
+
if (node.initializer) {
parts.push(" = ", print("initializer"));
}
| diff --git a/tests/format/typescript/enum/__snapshots__/jsfmt.spec.js.snap b/tests/format/typescript/enum/__snapshots__/jsfmt.spec.js.snap
--- a/tests/format/typescript/enum/__snapshots__/jsfmt.spec.js.snap
+++ b/tests/format/typescript/enum/__snapshots__/jsfmt.spec.js.snap
@@ -1,5 +1,53 @@
// Jest Snapshot v1, https://goo.gl/fbAQLP
+exports[`computed-members.ts [babel-ts] format 1`] = `
+"Unexpected token (2:3)
+ 1 | enum A {
+> 2 | [i++],
+ | ^
+ 3 | }
+ 4 |
+ 5 | const bar = "bar""
+`;
+
+exports[`computed-members.ts format 1`] = `
+====================================options=====================================
+parsers: ["typescript"]
+printWidth: 80
+ | printWidth
+=====================================input======================================
+enum A {
+ [i++],
+}
+
+const bar = "bar"
+enum B {
+ [bar] = 2,
+}
+
+const foo = () => "foo";
+enum C {
+ [foo()] = 2,
+}
+
+=====================================output=====================================
+enum A {
+ [i++],
+}
+
+const bar = "bar";
+enum B {
+ [bar] = 2,
+}
+
+const foo = () => "foo";
+enum C {
+ [foo()] = 2,
+}
+
+================================================================================
+`;
+
exports[`enum.ts format 1`] = `
====================================options=====================================
parsers: ["typescript"]
diff --git a/tests/format/typescript/enum/computed-members.ts b/tests/format/typescript/enum/computed-members.ts
new file mode 100644
--- /dev/null
+++ b/tests/format/typescript/enum/computed-members.ts
@@ -0,0 +1,13 @@
+enum A {
+ [i++],
+}
+
+const bar = "bar"
+enum B {
+ [bar] = 2,
+}
+
+const foo = () => "foo";
+enum C {
+ [foo()] = 2,
+}
diff --git a/tests/format/typescript/enum/jsfmt.spec.js b/tests/format/typescript/enum/jsfmt.spec.js
--- a/tests/format/typescript/enum/jsfmt.spec.js
+++ b/tests/format/typescript/enum/jsfmt.spec.js
@@ -1 +1,5 @@
-run_spec(__dirname, ["typescript"]);
+run_spec(__dirname, ["typescript"], {
+ errors: {
+ "babel-ts": ["computed-members.ts"],
+ },
+});
| Syntax error after formatting when using computed key in TypeScript enum
**Prettier 2.6.2**
[Playground link](https://prettier.io/playground/#N4Igxg9gdgLgprEAuECCuBbABAQS8AHSiywG0BLAakoF0iBfEAGhAgAcZzoBnZUAQwBOgiAHcACkIS8U-ADaj+AT14sARoP5gA1nBgBlfhjgAZclDjIAZvO5x1mnXv1st5gObIYgtPdQY1OAATIOCTfih3NH53OAAxCEEMfhhOSOQQfjQYCGYQAAsYDDkAdXzyeG5XMDh9aQryADcKpQywblUQcztBGHFNd2TrWz8AK24AD30POTgARTQIeGG5OxZXQR6MmCU2OG4wQXIOPLYj2BLyIJh85AAOAAZ1kTsSzTYMs-24QUbLFgAjot4P12DJMtwALQWYLBPKCOBA8gI-oxIZIGyrPx2DDkLw+bEzebAywYkYsGD8NSXa63JAAJgpmnIcg8AGEIBh0ahuABWPJoOwAFSpMkxaxAjV8AEkoKFYPpDscYDg5fodrMVnZ6PQgA)
<!-- prettier-ignore -->
```sh
--parser typescript
```
**Input 1:**
<!-- prettier-ignore -->
```tsx
enum A {
[i++]
}
```
**Output 1:**
<!-- prettier-ignore -->
```tsx
enum A {
i++,
}
```
**Input 2:**
<!-- prettier-ignore -->
```tsx
const a = "b"
enum A {
[a] = 2
}
```
**Output 2:**
<!-- prettier-ignore -->
```tsx
const a = "b";
enum A {
a = 2,
}
```
**Expected behavior:**
The output should be valid syntax. Although TypeScript will error during compiling that computed keys are not allowed in enums, they are valid syntax and parse correctly. Prettier should still support formatting this. Note that TypeScript still produces emit even when erroring, meaning the code would be able to run.
**Actual behavior:**
The output incorrectly removes the brackets, producing a syntax error on example 1, or modifying the runtime behaviour in example 2.
| "2022-05-27T08:36:59Z" | 2.7 | [] | [
"tests/format/typescript/enum/jsfmt.spec.js"
] | JavaScript | [] | [
"https://prettier.io/playground/#N4Igxg9gdgLgprEAuECCuBbABAQS8AHSiywG0BLAakoF0iBfEAGhAgAcZzoBnZUAQwBOgiAHcACkIS8U-ADaj+AT14sARoP5gA1nBgBlfhjgAZclDjIAZvO5x1mnXv1st5gObIYgtPdQY1OAATIOCTfih3NH53OAAxCEEMfhhOSOQQfjQYCGYQAAsYDDkAdXzyeG5XMDh9aQryADcKpQywblUQcztBGHFNd2TrWz8AK24AD30POTgARTQIeGG5OxZXQR6MmCU2OG4wQXIOPLYj2BLyIJh85AAOAAZ1kTsSzTYMs-24QUbLFgAjot4P12DJMtwALQWYLBPKCOBA8gI-oxIZIGyrPx2DDkLw+bEzebAywYkYsGD8NSXa63JAAJgpmnIcg8AGEIBh0ahuABWPJoOwAFSpMkxaxAjV8AEkoKFYPpDscYDg5fodrMVnZ6PQgA"
] |
|
prettier/prettier | 12,982 | prettier__prettier-12982 | [
"12963"
] | be9d63e543b6ac28aa6eee7ab8dc45849cd8f0b3 | diff --git a/src/language-js/parse/postprocess/index.js b/src/language-js/parse/postprocess/index.js
--- a/src/language-js/parse/postprocess/index.js
+++ b/src/language-js/parse/postprocess/index.js
@@ -6,6 +6,7 @@ const isTypeCastComment = require("../../utils/is-type-cast-comment.js");
const getLast = require("../../../utils/get-last.js");
const visitNode = require("./visit-node.js");
const { throwErrorForInvalidNodes } = require("./typescript.js");
+const throwSyntaxError = require("./throw-syntax-error.js");
function postprocess(ast, options) {
if (
@@ -102,6 +103,20 @@ function postprocess(ast, options) {
};
}
break;
+ case "ObjectExpression":
+ // #12963
+ if (options.parser === "typescript") {
+ const invalidProperty = node.properties.find(
+ (property) =>
+ property.type === "Property" &&
+ property.value.type === "TSEmptyBodyFunctionExpression"
+ );
+ if (invalidProperty) {
+ throwSyntaxError(invalidProperty.value, "Unexpected token.");
+ }
+ }
+ break;
+
case "SequenceExpression": {
// Babel (unlike other parsers) includes spaces and comments in the range. Let's unify this.
const lastExpression = getLast(node.expressions);
diff --git a/src/language-js/parse/postprocess/throw-syntax-error.js b/src/language-js/parse/postprocess/throw-syntax-error.js
new file mode 100644
--- /dev/null
+++ b/src/language-js/parse/postprocess/throw-syntax-error.js
@@ -0,0 +1,12 @@
+"use strict";
+const createError = require("../../../common/parser-create-error.js");
+
+function throwSyntaxError(node, message) {
+ const { start, end } = node.loc;
+ throw createError(message, {
+ start: { line: start.line, column: start.column + 1 },
+ end: { line: end.line, column: end.column + 1 },
+ });
+}
+
+module.exports = throwSyntaxError;
diff --git a/src/language-js/parse/postprocess/typescript.js b/src/language-js/parse/postprocess/typescript.js
--- a/src/language-js/parse/postprocess/typescript.js
+++ b/src/language-js/parse/postprocess/typescript.js
@@ -1,15 +1,7 @@
"use strict";
-const createError = require("../../../common/parser-create-error.js");
const visitNode = require("./visit-node.js");
-
-function throwSyntaxError(node, message) {
- const { start, end } = node.loc;
- throw createError(message, {
- start: { line: start.line, column: start.column + 1 },
- end: { line: end.line, column: end.column + 1 },
- });
-}
+const throwSyntaxError = require("./throw-syntax-error.js");
// Invalid decorators are removed since `@typescript-eslint/typescript-estree` v4
// https://github.com/typescript-eslint/typescript-eslint/pull/2375
@@ -73,6 +65,7 @@ function throwErrorForInvalidNodes(ast, options) {
if (esTreeNode !== node) {
return;
}
+
throwErrorForInvalidDecorator(tsNode, esTreeNode, tsNodeToESTreeNodeMap);
throwErrorForInvalidAbstractProperty(tsNode, esTreeNode);
});
| diff --git a/tests/format/misc/errors/invalid/__snapshots__/jsfmt.spec.js.snap b/tests/format/misc/errors/invalid/__snapshots__/jsfmt.spec.js.snap
--- a/tests/format/misc/errors/invalid/__snapshots__/jsfmt.spec.js.snap
+++ b/tests/format/misc/errors/invalid/__snapshots__/jsfmt.spec.js.snap
@@ -156,92 +156,236 @@ exports[`snippet: #1 [typescript] format 1`] = `
| ^"
`;
+exports[`snippet: #2 [acorn] format 1`] = `
+"Unexpected token (1:13)
+> 1 | ({ method() })
+ | ^"
+`;
+
exports[`snippet: #2 [babel] format 1`] = `
+"Unexpected token, expected "{" (1:13)
+> 1 | ({ method() })
+ | ^"
+`;
+
+exports[`snippet: #2 [babel] format 2`] = `
"Invalid parenthesized assignment pattern. (1:1)
> 1 | (a = 1) = 2
| ^"
`;
exports[`snippet: #2 [babel-flow] format 1`] = `
+"Unexpected token, expected "{" (1:13)
+> 1 | ({ method() })
+ | ^"
+`;
+
+exports[`snippet: #2 [babel-flow] format 2`] = `
"Invalid parenthesized assignment pattern. (1:1)
> 1 | (a = 1) = 2
| ^"
`;
exports[`snippet: #2 [babel-ts] format 1`] = `
+"Unexpected token, expected "{" (1:13)
+> 1 | ({ method() })
+ | ^"
+`;
+
+exports[`snippet: #2 [babel-ts] format 2`] = `
"Invalid parenthesized assignment pattern. (1:1)
> 1 | (a = 1) = 2
| ^"
`;
+exports[`snippet: #2 [espree] format 1`] = `
+"Unexpected token } (1:13)
+> 1 | ({ method() })
+ | ^"
+`;
+
exports[`snippet: #2 [flow] format 1`] = `
+"Unexpected token \`}\`, expected the token \`{\` (1:13)
+> 1 | ({ method() })
+ | ^"
+`;
+
+exports[`snippet: #2 [flow] format 2`] = `
"Invalid left-hand side in assignment (1:2)
> 1 | (a = 1) = 2
| ^^^^^"
`;
exports[`snippet: #2 [meriyah] format 1`] = `
+"Expected '{' (1:13)
+> 1 | ({ method() })
+ | ^"
+`;
+
+exports[`snippet: #2 [meriyah] format 2`] = `
"Invalid left-hand side in assignment (1:9)
> 1 | (a = 1) = 2
| ^"
`;
+exports[`snippet: #2 [typescript] format 1`] = `
+"Unexpected token. (1:10)
+> 1 | ({ method() })
+ | ^^"
+`;
+
+exports[`snippet: #3 [acorn] format 1`] = `
+"Unexpected token (1:15)
+> 1 | ({ method({}) })
+ | ^"
+`;
+
exports[`snippet: #3 [babel] format 1`] = `
+"Unexpected token, expected "{" (1:15)
+> 1 | ({ method({}) })
+ | ^"
+`;
+
+exports[`snippet: #3 [babel] format 2`] = `
"Invalid parenthesized assignment pattern. (1:1)
> 1 | (a += b) = 1
| ^"
`;
exports[`snippet: #3 [babel-flow] format 1`] = `
+"Unexpected token, expected "{" (1:15)
+> 1 | ({ method({}) })
+ | ^"
+`;
+
+exports[`snippet: #3 [babel-flow] format 2`] = `
"Invalid parenthesized assignment pattern. (1:1)
> 1 | (a += b) = 1
| ^"
`;
exports[`snippet: #3 [babel-ts] format 1`] = `
+"Unexpected token, expected "{" (1:15)
+> 1 | ({ method({}) })
+ | ^"
+`;
+
+exports[`snippet: #3 [babel-ts] format 2`] = `
"Invalid parenthesized assignment pattern. (1:1)
> 1 | (a += b) = 1
| ^"
`;
+exports[`snippet: #3 [espree] format 1`] = `
+"Unexpected token } (1:15)
+> 1 | ({ method({}) })
+ | ^"
+`;
+
exports[`snippet: #3 [flow] format 1`] = `
+"Unexpected token \`}\`, expected the token \`{\` (1:15)
+> 1 | ({ method({}) })
+ | ^"
+`;
+
+exports[`snippet: #3 [flow] format 2`] = `
"Invalid left-hand side in assignment (1:2)
> 1 | (a += b) = 1
| ^^^^^^"
`;
exports[`snippet: #3 [meriyah] format 1`] = `
+"Expected '{' (1:15)
+> 1 | ({ method({}) })
+ | ^"
+`;
+
+exports[`snippet: #3 [meriyah] format 2`] = `
"Invalid left-hand side in assignment (1:10)
> 1 | (a += b) = 1
| ^"
`;
+exports[`snippet: #3 [typescript] format 1`] = `
+"Unexpected token. (1:10)
+> 1 | ({ method({}) })
+ | ^^^^"
+`;
+
+exports[`snippet: #4 [acorn] format 1`] = `
+"Unexpected token (1:23)
+> 1 | ({ method(parameter,) })
+ | ^"
+`;
+
exports[`snippet: #4 [babel] format 1`] = `
+"Unexpected token, expected "{" (1:23)
+> 1 | ({ method(parameter,) })
+ | ^"
+`;
+
+exports[`snippet: #4 [babel] format 2`] = `
"Invalid left-hand side in parenthesized expression. (1:2)
> 1 | (a = b) += 1
| ^"
`;
exports[`snippet: #4 [babel-flow] format 1`] = `
+"Unexpected token, expected "{" (1:23)
+> 1 | ({ method(parameter,) })
+ | ^"
+`;
+
+exports[`snippet: #4 [babel-flow] format 2`] = `
"Invalid left-hand side in parenthesized expression. (1:2)
> 1 | (a = b) += 1
| ^"
`;
exports[`snippet: #4 [babel-ts] format 1`] = `
+"Unexpected token, expected "{" (1:23)
+> 1 | ({ method(parameter,) })
+ | ^"
+`;
+
+exports[`snippet: #4 [babel-ts] format 2`] = `
"Invalid left-hand side in parenthesized expression. (1:2)
> 1 | (a = b) += 1
| ^"
`;
+exports[`snippet: #4 [espree] format 1`] = `
+"Unexpected token } (1:23)
+> 1 | ({ method(parameter,) })
+ | ^"
+`;
+
exports[`snippet: #4 [flow] format 1`] = `
+"Unexpected token \`}\`, expected the token \`{\` (1:23)
+> 1 | ({ method(parameter,) })
+ | ^"
+`;
+
+exports[`snippet: #4 [flow] format 2`] = `
"Invalid left-hand side in assignment (1:2)
> 1 | (a = b) += 1
| ^^^^^"
`;
exports[`snippet: #4 [meriyah] format 1`] = `
+"Expected '{' (1:23)
+> 1 | ({ method(parameter,) })
+ | ^"
+`;
+
+exports[`snippet: #4 [meriyah] format 2`] = `
"Invalid left-hand side in assignment (1:10)
> 1 | (a = b) += 1
| ^"
`;
+
+exports[`snippet: #4 [typescript] format 1`] = `
+"Unexpected token. (1:10)
+> 1 | ({ method(parameter,) })
+ | ^^^^^^^^^^^^"
+`;
diff --git a/tests/format/misc/errors/invalid/jsfmt.spec.js b/tests/format/misc/errors/invalid/jsfmt.spec.js
--- a/tests/format/misc/errors/invalid/jsfmt.spec.js
+++ b/tests/format/misc/errors/invalid/jsfmt.spec.js
@@ -1,7 +1,13 @@
run_spec(
{
dirname: __dirname,
- snippets: ["for each (a in b) {}", "class switch() {}"],
+ snippets: [
+ "for each (a in b) {}",
+ "class switch() {}",
+ "({ method() })",
+ "({ method({}) })",
+ "({ method(parameter,) })",
+ ],
},
[
"babel",
| Prettier parses `({ methodName() })` incorrectly
**Prettier 2.6.1**
[Playground link](https://prettier.io/playground/#N4Igxg9gdgLgprEAuEAKYACAtnGALCAEwDkBDHVASgwF9KQAaECABxgEtoBnZUUgJ34QA7gAUBCHilIAbYaQCePJgCN+pMAGtcAZXJwAMuyhxkAM1lc4q9Vt0sNxgObIY-AK7WQcLCriFCfwNSKCd3Uic4ADEIfixSGA5Q5BBSdxgIRhA8GCwZAHU8dnguBzA4HUli9gA3YoUUsC5lEGMrfhhRdSd480svACsuAA8dZxk4AEV3CHg+mSsmB352lJgFFjguMH52NiyWXdh89kJ8ZAAOAAYloSt89RYUw624fhrTJgBHGfgu1ikqS4AFoTP5-Fl+HAfuwoV0Ir0kBYFl4rFh2K4PKjxlNfqYkf0mDBSCoTmc8MgAExE9TsGTOADCECwiO8XAArFl3FYACokqTIxYgGqeACSUECsB0Oz2MAAghKdOsJvMrDQaEA)
<!-- prettier-ignore -->
```sh
--parser typescript
```
**Input:**
<!-- prettier-ignore -->
```tsx
({ methodName() })
```
**Output:**
<!-- prettier-ignore -->
```tsx
({ methodName(); });
```
**Expected behavior:**
The code inputted is invalid and shouldn't be parsed. Prettier recognizes this as an object because it can be turned into a multiline object and Prettier still has invalid output.
**Prettier 2.6.1**
[Playground link](https://prettier.io/playground/#N4Igxg9gdgLgprEAuEAKYAdKACbBbOGACwgBMA5AQwNQEosBfWkAGhAgAcYBLaAZ2ShKAJ2EQA7gAURCASkoAbcZQCeAtgCNhlMAGtCAZWpwAMtyhxkAM0V84m7XsMcd5gObIYwgK72QcPA04UlJgk0ooN29KNzgAMQhhPEoYHkjkEEpvGAhWECIYPAUAdSJueD4XMDgDWXLuADdylQywPnUQczthGEltN2TrWz8AKz4ADwN3BTgARW8IeCGFOzYXYW6MmBUOOD4wYW4uPI5D2GLuUmJkAA4ABjWxO2LtDgzTvbhhBss2AEcFvA+pw5Jk+ABaCzBYJ5YRwAHcOF9GKDJA2FZ+Ox4bieHyY6ZzQGWNHDNgwSgaC5XIjIABMZO03AU7gAwhA8Kj-HwAKx5bx2AAqFLk6NWIAavgAklBQrADAcjjAAIIygzbGbLOwMBhAA)
<!-- prettier-ignore -->
```sh
--parser typescript
```
**Input:**
<!-- prettier-ignore -->
```tsx
({
methodName()
})
```
**Output:**
<!-- prettier-ignore -->
```tsx
({
methodName();,
});
```
| By the way, testing shows that this doesn't happen with the `babel`, `babel-ts`, `babel-flow`, `acorn`, `espree`, or `meriyah` parsers, so it could be an upstream bug with TypeScript's parser. This might be because of TypeScript's function overloading not working properly in object literals, but I'm not sure.
I think that the typescript parser properly rejects this as malformed, but the typescript-eslint parser emits a `TSEmptyBodyFunctionExpression` AST node.
Related issue: https://github.com/prettier/prettier/issues/8416 | "2022-06-06T15:27:56Z" | 2.7 | [] | [
"tests/format/misc/errors/invalid/jsfmt.spec.js"
] | JavaScript | [] | [
"https://prettier.io/playground/#N4Igxg9gdgLgprEAuEAKYACAtnGALCAEwDkBDHVASgwF9KQAaECABxgEtoBnZUUgJ34QA7gAUBCHilIAbYaQCePJgCN+pMAGtcAZXJwAMuyhxkAM1lc4q9Vt0sNxgObIY-AK7WQcLCriFCfwNSKCd3Uic4ADEIfixSGA5Q5BBSdxgIRhA8GCwZAHU8dnguBzA4HUli9gA3YoUUsC5lEGMrfhhRdSd480svACsuAA8dZxk4AEV3CHg+mSsmB352lJgFFjguMH52NiyWXdh89kJ8ZAAOAAYloSt89RYUw624fhrTJgBHGfgu1ikqS4AFoTP5-Fl+HAfuwoV0Ir0kBYFl4rFh2K4PKjxlNfqYkf0mDBSCoTmc8MgAExE9TsGTOADCECwiO8XAArFl3FYACokqTIxYgGqeACSUECsB0Oz2MAAghKdOsJvMrDQaEA",
"https://prettier.io/playground/#N4Igxg9gdgLgprEAuEAKYAdKACbBbOGACwgBMA5AQwNQEosBfWkAGhAgAcYBLaAZ2ShKAJ2EQA7gAURCASkoAbcZQCeAtgCNhlMAGtCAZWpwAMtyhxkAM0V84m7XsMcd5gObIYwgK72QcPA04UlJgk0ooN29KNzgAMQhhPEoYHkjkEEpvGAhWECIYPAUAdSJueD4XMDgDWXLuADdylQywPnUQczthGEltN2TrWz8AKz4ADwN3BTgARW8IeCGFOzYXYW6MmBUOOD4wYW4uPI5D2GLuUmJkAA4ABjWxO2LtDgzTvbhhBss2AEcFvA+pw5Jk+ABaCzBYJ5YRwAHcOF9GKDJA2FZ+Ox4bieHyY6ZzQGWNHDNgwSgaC5XIjIABMZO03AU7gAwhA8Kj-HwAKx5bx2AAqFLk6NWIAavgAklBQrADAcjjAAIIygzbGbLOwMBhAA"
] |
prettier/prettier | 13,115 | prettier__prettier-13115 | [
"13018"
] | 2c77149e6a3f6d5a74745a23c38e13f2ffebe28d | diff --git a/src/language-js/utils/index.js b/src/language-js/utils/index.js
--- a/src/language-js/utils/index.js
+++ b/src/language-js/utils/index.js
@@ -883,9 +883,26 @@ function isSimpleCallArgument(node, depth) {
);
}
+ const targetUnaryExpressionOperators = {
+ "!": true,
+ "-": true,
+ "+": true,
+ "~": true,
+ };
if (
node.type === "UnaryExpression" &&
- (node.operator === "!" || node.operator === "-")
+ targetUnaryExpressionOperators[node.operator]
+ ) {
+ return isSimpleCallArgument(node.argument, depth);
+ }
+
+ const targetUpdateExpressionOperators = {
+ "++": true,
+ "--": true,
+ };
+ if (
+ node.type === "UpdateExpression" &&
+ targetUpdateExpressionOperators[node.operator]
) {
return isSimpleCallArgument(node.argument, depth);
}
| diff --git a/tests/format/js/method-chain/13018.js b/tests/format/js/method-chain/13018.js
new file mode 100644
--- /dev/null
+++ b/tests/format/js/method-chain/13018.js
@@ -0,0 +1,8 @@
+foo(_a).bar().leet();
+foo(-a).bar().leet();
+foo(+a).bar().leet();
+foo(~a).bar().leet();
+foo(++a).bar().leet();
+foo(--a).bar().leet();
+foo(a++).bar().leet();
+foo(a--).bar().leet();
diff --git a/tests/format/js/method-chain/__snapshots__/jsfmt.spec.js.snap b/tests/format/js/method-chain/__snapshots__/jsfmt.spec.js.snap
--- a/tests/format/js/method-chain/__snapshots__/jsfmt.spec.js.snap
+++ b/tests/format/js/method-chain/__snapshots__/jsfmt.spec.js.snap
@@ -1,5 +1,33 @@
// Jest Snapshot v1, https://goo.gl/fbAQLP
+exports[`13018.js format 1`] = `
+====================================options=====================================
+parsers: ["babel", "flow", "typescript"]
+printWidth: 80
+ | printWidth
+=====================================input======================================
+foo(_a).bar().leet();
+foo(-a).bar().leet();
+foo(+a).bar().leet();
+foo(~a).bar().leet();
+foo(++a).bar().leet();
+foo(--a).bar().leet();
+foo(a++).bar().leet();
+foo(a--).bar().leet();
+
+=====================================output=====================================
+foo(_a).bar().leet();
+foo(-a).bar().leet();
+foo(+a).bar().leet();
+foo(~a).bar().leet();
+foo(++a).bar().leet();
+foo(--a).bar().leet();
+foo(a++).bar().leet();
+foo(a--).bar().leet();
+
+================================================================================
+`;
+
exports[`bracket_0.js format 1`] = `
====================================options=====================================
parsers: ["babel", "flow", "typescript"]
| Unary `+` parameter triggers overeager breaking of method chain
**Prettier 2.7.1**
[Playground link](https://prettier.io/playground/#N4Igxg9gdgLgprEAuEAzCEAUB9AhgSgDoAjXAJ0yIBs44ZKBuAHSnSwFoCTzLCa7GLNpgDUXUhWq16+BiAA0ICAAcYAS2gBnZKHJkIAdwAK5BNpS4qB3AE9ti4mVxgA1nQDKuALZwAMmqg4ZFRLTTgHJ1cPZWcAgHNkGDIAV3CQOC9iOAATbJzfXCg45Nw4uAAxCDIvXBh1IuQQXGSYCAUQAAsYLyoAdQ61eE0YsDh3M0G1ADdBm0awTXsQALCyGCMnOJrg0LSAK00AD3d4mgBFZIh4HaowxRiyVcbSLKp25TIAmF61bJgO5AADgADPd9GFek5lI0PnBVlMgooAI6XeAbFTmJqadiBHI5dpkOAotSEjalbZIEK3NJhLxqRIpGmnOAXK5BSm7RQwXDEH5-AFIABMXKcaio8QAwhAvBT0poAKztZJhAAqPPMVLuICmqQAklA8rB3GBPqoAIIG9wwGw0G5hAC+9qAA)
<!-- prettier-ignore -->
```sh
--parser babel
```
**Input:**
<!-- prettier-ignore -->
```jsx
foo(_a).bar().leet();
foo(-a).bar().leet();
foo(+a).bar().leet();
```
**Output:**
<!-- prettier-ignore -->
```jsx
foo(_a).bar().leet();
foo(-a).bar().leet();
foo(+a)
.bar()
.leet();
```
**Expected behavior:**
```jsx
foo(_a).bar().leet();
foo(-a).bar().leet();
foo(+a).bar().leet();
```
| I have looked into this issue.
It seems that the Doc created by prettier is different for `+` parameter and other cases.
This Doc is created at the end of `printMemberChain` function in `src/language-js/print/member-chain.js`
https://github.com/prettier/prettier/blob/a043ac0d733c4d53f980aa73807a63fc914f23bd/src/language-js/print/member-chain.js#L387-L405
In the above process, `isSimpleCallArgument` function is used in the if statement.
But, `+` unary expression does not appear to be properly processed in this function.
https://github.com/prettier/prettier/blob/a043ac0d733c4d53f980aa73807a63fc914f23bd/src/language-js/utils/index.js#L886-L891
I added `node.operator === "+"` in the above if state as a trial, and the output seemed to be expected.
(So, if you like, maybe I can send a PR about this issue.)
> So, if you like, maybe I can send a PR about this issue.
@t-mangoe Go ahead!
@sosukesuzuki
Thanks for your comment. I will send a PR. | "2022-07-16T01:21:53Z" | 2.8 | [] | [
"tests/format/js/method-chain/jsfmt.spec.js"
] | JavaScript | [] | [
"https://prettier.io/playground/#N4Igxg9gdgLgprEAuEAzCEAUB9AhgSgDoAjXAJ0yIBs44ZKBuAHSnSwFoCTzLCa7GLNpgDUXUhWq16+BiAA0ICAAcYAS2gBnZKHJkIAdwAK5BNpS4qB3AE9ti4mVxgA1nQDKuALZwAMmqg4ZFRLTTgHJ1cPZWcAgHNkGDIAV3CQOC9iOAATbJzfXCg45Nw4uAAxCDIvXBh1IuQQXGSYCAUQAAsYLyoAdQ61eE0YsDh3M0G1ADdBm0awTXsQALCyGCMnOJrg0LSAK00AD3d4mgBFZIh4HaowxRiyVcbSLKp25TIAmF61bJgO5AADgADPd9GFek5lI0PnBVlMgooAI6XeAbFTmJqadiBHI5dpkOAotSEjalbZIEK3NJhLxqRIpGmnOAXK5BSm7RQwXDEH5-AFIABMXKcaio8QAwhAvBT0poAKztZJhAAqPPMVLuICmqQAklA8rB3GBPqoAIIG9wwGw0G5hAC+9qAA"
] |
prettier/prettier | 13,173 | prettier__prettier-13173 | [
"12964"
] | b65cb6c1f67d95be8e8f7719f2a4174eaa5fe66c | diff --git a/src/main/range-util.js b/src/main/range-util.js
--- a/src/main/range-util.js
+++ b/src/main/range-util.js
@@ -64,6 +64,9 @@ function findSiblingAncestors(
} else {
break;
}
+ if (resultStartNode === resultEndNode) {
+ break;
+ }
}
return {
| diff --git a/tests/format/js/range/__snapshots__/jsfmt.spec.js.snap b/tests/format/js/range/__snapshots__/jsfmt.spec.js.snap
--- a/tests/format/js/range/__snapshots__/jsfmt.spec.js.snap
+++ b/tests/format/js/range/__snapshots__/jsfmt.spec.js.snap
@@ -182,6 +182,27 @@ rangeStart: 0
================================================================================
`;
+exports[`function-body.js format 1`] = `
+====================================options=====================================
+parsers: ["babel", "flow", "typescript"]
+printWidth: 80
+rangeEnd: 33
+rangeStart: 20
+ | | printWidth
+=====================================input======================================
+ 1 | let fn =a((x ) => {
+> 2 | quux (); //
+ | ^^^^^^^^^^^^^
+ 3 | });
+ 4 |
+=====================================output=====================================
+let fn =a((x ) => {
+ quux(); //
+});
+
+================================================================================
+`;
+
exports[`function-declaration.js format 1`] = `
====================================options=====================================
parsers: ["babel", "flow", "typescript"]
diff --git a/tests/format/js/range/function-body.js b/tests/format/js/range/function-body.js
new file mode 100644
--- /dev/null
+++ b/tests/format/js/range/function-body.js
@@ -0,0 +1,3 @@
+let fn =a((x ) => {
+<<<PRETTIER_RANGE_START>>> quux (); //<<<PRETTIER_RANGE_END>>>
+});
| Code-breaking semicolon added when formatting range in arrow function with comment
**Prettier 2.6.2**
[Playground link](https://prettier.io/playground/#N4Igxg9gdgLgprEAuEAbOMAEAzKmC8mAFEQJQEB8mwAOnpgE4YCuDUA3JgPRd0C+pdiAA0ICAAcYAS2gBnZKACGDBhADuABWUJ5KRajWKAnvNEAjBorABrDAGVFAWzgAZKVDjJs+2XHOWbe3ErdwBzZBgGZj8QOEczOAATRKSXRShQ5kVQuAAxCAZHRRhpDOQQRWYYCBEQAAsYR1QAdTqpeFlgsDg7HXapADd2o3KwWVMQd18GGA1LUKKvHxiAK1kADzsw9ABFZgh4JdRfUWCGafKzRQTUWvEGdxhmqUSYOuQADgAGU9VfZss4nK9zg0wGnlEAEd9vA5hJdBVZABaDxJJK1JjQqRMObZRZIbzHGK+RxSCJRYnbOB7A6eAnLUQwa7PV7vJAAJkZlikqDCAGEII58bFZABWWrMXwAFWuukJJxAA2iAEkoClYHYwA9JABBNV2GBGdBHBWWDI9JkzZAARg+ojNOQAomrkABma18PhAA)
<!-- prettier-ignore -->
```sh
--parser babel
--range-start 18
--range-end 31
```
**Input:**
<!-- prettier-ignore -->
```jsx
let fn = (() => {
return; //
});
```
**Output:**
<!-- prettier-ignore -->
```jsx
let fn = (() => {
return; //
};);
```
**Expected behavior:**
The semicolon after the arrow function is not added.
Note that this does NOT happen when the arrow function is replaced with a `function` expression, nor if the comment inside the arrow function is removed.
| I wonder if this is related to #12963, because a semicolon was added improperly in that issue as well.
> I wonder if this is related to #12963, because a semicolon was added improperly in that issue as well.
I don't think so. This happens with the Babel parser and the parse tree looks correct to me, unlike with #12963
Based on further experimentation, I think this happens WHENEVER formatting a range inside a braced arrow function expression where the range ends with a comment (either `//` or `/*..*/`) but does not start with a comment.
> Based on further experimentation, I think this happens WHENEVER formatting a range inside a braced arrow function expression where the range ends with a comment (either `//` or `/*..*/`) but does not start with a comment.
I can confirm this. I had commented out a line at the end of a modified range of lines and had the editor.formatOnSaveMode setting set to 'modifications' and this added a semicolon at the very end of my file (the closing part of an IIEF: `};)();`.
Once I uncommented the line in question (and removed the semicolon), a new one was not added on save.
My solution, in the meantime, was to just go back to 'file' for editor.formatOnSaveMode. This solves it, since I never end a file with a comment.
> My solution, in the meantime, was to just go back to 'file' for editor.formatOnSaveMode. This solves it, since I never end a file with a comment.
That's an unfortunate solution, if understandably pragmatic. This is a pretty nasty bug since it corrupts correct code.
Even a comment at the end of the file wouldn't trigger this. It's just about a comment at the end of the *edited range inside* an arrow function.
I noticed another curious thing about this bug - if you have `--no-semi` enabled, instead of a code-breaking semicolon added *after* the arrow function, it inserts a code-breaking semicolon *before* the arrow function!
We are seeing an issue here too. We have the noSemi option and it's adding a semi-colon before the code and breaking it.
We are working in react. Not sure if that is relevant but worth mentioning
any solution ? Otherwise, I have to disable prettier
same here v2.7.1
input
```
map((data) => {
// this can be anything, code or comment, if I remove this, then it works
return data.sort();
}),
```
output
```
map((data) => {
// this can be anything, code or comment, if I remove this, then it works
return data.sort();
});,
``` | "2022-07-24T19:18:04Z" | 2.8 | [] | [
"tests/format/js/range/jsfmt.spec.js"
] | JavaScript | [] | [
"https://prettier.io/playground/#N4Igxg9gdgLgprEAuEAbOMAEAzKmC8mAFEQJQEB8mwAOnpgE4YCuDUA3JgPRd0C+pdiAA0ICAAcYAS2gBnZKACGDBhADuABWUJ5KRajWKAnvNEAjBorABrDAGVFAWzgAZKVDjJs+2XHOWbe3ErdwBzZBgGZj8QOEczOAATRKSXRShQ5kVQuAAxCAZHRRhpDOQQRWYYCBEQAAsYR1QAdTqpeFlgsDg7HXapADd2o3KwWVMQd18GGA1LUKKvHxiAK1kADzsw9ABFZgh4JdRfUWCGafKzRQTUWvEGdxhmqUSYOuQADgAGU9VfZss4nK9zg0wGnlEAEd9vA5hJdBVZABaDxJJK1JjQqRMObZRZIbzHGK+RxSCJRYnbOB7A6eAnLUQwa7PV7vJAAJkZlikqDCAGEII58bFZABWWrMXwAFWuukJJxAA2iAEkoClYHYwA9JABBNV2GBGdBHBWWDI9JkzZAARg+ojNOQAomrkABma18PhAA"
] |
prettier/prettier | 13,315 | prettier__prettier-13315 | [
"16493"
] | fa94c3099c4199208abac8a2af0c5716f4cfbb61 | diff --git a/src/document/printer.js b/src/document/printer.js
--- a/src/document/printer.js
+++ b/src/document/printer.js
@@ -1,6 +1,6 @@
import { convertEndOfLineToChars } from "../common/end-of-line.js";
import getStringWidth from "../utils/get-string-width.js";
-import { fill, hardlineWithoutBreakParent, indent } from "./builders.js";
+import { hardlineWithoutBreakParent, indent } from "./builders.js";
import {
DOC_TYPE_ALIGN,
DOC_TYPE_ARRAY,
@@ -32,6 +32,8 @@ const MODE_FLAT = Symbol("MODE_FLAT");
const CURSOR_PLACEHOLDER = Symbol("cursor");
+const IS_MUTABLE_FILL = Symbol("IS_MUTABLE_FILL");
+
function rootIndent() {
return { value: "", length: 0, queue: [] };
}
@@ -503,16 +505,26 @@ function printDocToString(doc, options) {
break;
}
+ const secondContent = parts[2];
+
// At this point we've handled the first pair (context, separator)
- // and will create a new fill doc for the rest of the content.
- // Ideally we wouldn't mutate the array here but copying all the
- // elements to a new array would make this algorithm quadratic,
+ // and will create a new *mutable* fill doc for the rest of the content.
+ // Copying all the elements to a new array would make this algorithm quadratic,
// which is unusable for large arrays (e.g. large texts in JSX).
- parts.splice(0, 2);
- /** @type {Command} */
- const remainingCmd = { ind, mode, doc: fill(parts) };
+ // https://github.com/prettier/prettier/issues/3263#issuecomment-344275152
+ let remainingDoc = doc;
+ if (doc[IS_MUTABLE_FILL]) {
+ parts.splice(0, 2);
+ } else {
+ remainingDoc = {
+ ...doc,
+ parts: parts.slice(2),
+ [IS_MUTABLE_FILL]: true,
+ };
+ }
- const secondContent = parts[0];
+ /** @type {Command} */
+ const remainingCmd = { ind, mode, doc: remainingDoc };
/** @type {Command} */
const firstAndSecondContentFlatCmd = {
| diff --git a/tests/format/js/template-literals/__snapshots__/format.test.js.snap b/tests/format/js/template-literals/__snapshots__/format.test.js.snap
--- a/tests/format/js/template-literals/__snapshots__/format.test.js.snap
+++ b/tests/format/js/template-literals/__snapshots__/format.test.js.snap
@@ -164,6 +164,8 @@ descirbe('something', () => {
throw new Error(\`pretty-format: Option "theme" has a key "\${key}" whose value "\${value}" is undefined in ansi-styles.\`,)
+a = \`\${[[1, 2, 3], [4, 5, 6]]}\`
+
=====================================output=====================================
const long1 = \`long \${
a.b //comment
@@ -224,6 +226,11 @@ throw new Error(
\`pretty-format: Option "theme" has a key "\${key}" whose value "\${value}" is undefined in ansi-styles.\`,
);
+a = \`\${[
+ [1, 2, 3],
+ [4, 5, 6],
+]}\`;
+
================================================================================
`;
diff --git a/tests/format/js/template-literals/expressions.js b/tests/format/js/template-literals/expressions.js
--- a/tests/format/js/template-literals/expressions.js
+++ b/tests/format/js/template-literals/expressions.js
@@ -44,3 +44,5 @@ descirbe('something', () => {
})
throw new Error(`pretty-format: Option "theme" has a key "${key}" whose value "${value}" is undefined in ansi-styles.`,)
+
+a = `${[[1, 2, 3], [4, 5, 6]]}`
diff --git a/tests/unit/doc-printer.js b/tests/unit/doc-printer.js
new file mode 100644
--- /dev/null
+++ b/tests/unit/doc-printer.js
@@ -0,0 +1,45 @@
+// TODO: Find a better way to test the performance
+import { fill, join, line } from "../../src/document/builders.js";
+import { printDocToString } from "../../src/document/printer.js";
+
+test("`printDocToString` should not manipulate docs", () => {
+ const printOptions = { printWidth: 40, tabWidth: 2 };
+ const doc = fill(
+ join(
+ line,
+ Array.from({ length: 255 }, (_, index) => String(index + 1)),
+ ),
+ );
+
+ expect(doc.parts.length).toBe(255 + 254);
+
+ const { formatted: firstPrint } = printDocToString(doc, printOptions);
+
+ expect(doc.parts.length).toBe(255 + 254);
+
+ const { formatted: secondPrint } = printDocToString(doc, printOptions);
+
+ expect(firstPrint).toBe(secondPrint);
+
+ {
+ // About 1000 lines, #3263
+ const WORD = "word";
+ const hugeParts = join(
+ line,
+ Array.from(
+ { length: 1000 * Math.ceil(printOptions.printWidth / WORD.length) },
+ () => WORD,
+ ),
+ );
+ const orignalLength = hugeParts.length;
+
+ const startTime = performance.now();
+ const { formatted } = printDocToString(fill(hugeParts), printOptions);
+ const endTime = performance.now();
+ expect(hugeParts.length).toBe(orignalLength);
+
+ const lines = formatted.split("\n");
+ expect(lines.length).toBeGreaterThan(1000);
+ expect(endTime - startTime).toBeLessThan(1000);
+ }
+});
| Modification of nested arrays inside format string
<!--
BEFORE SUBMITTING AN ISSUE:
1. Search for your issue on GitHub: https://github.com/prettier/prettier/issues
A large number of opened issues are duplicates of existing issues.
If someone has already opened an issue for what you are experiencing,
you do not need to open a new issue — please add a 👍 reaction to the
existing issue instead.
2. We get a lot of requests for adding options, but Prettier is
built on the principle of being opinionated about code formatting.
This means we add options only in the case of strict technical necessity.
Find out more: https://prettier.io/docs/en/option-philosophy.html
Don't fill the form below manually! Let a program create a report for you:
1. Go to https://prettier.io/playground
2. Paste your code and set options
3. Press the "Report issue" button in the lower right
-->
**Prettier 3.3.3**
[Playground link](https://prettier.io/playground/#N4Igxg9gdgLgprEAuEAPABAXnQAwCTADahAjADToBMAuhYQMwUAs11AvjiGSBAA4wBLaAGdkoAIYAnSRADuABSkJRKcQBtZ4gJ6juAI0niwAazgwAyuIC2cADICocZADN1wuPsMmz53kYcA5sgwkgCuHiDuVgLBYRFwqLxwkgI2sOoAKslQUgJwKq5q7tzCgWpwAIqhEPAubhEAVsKo5mWV1bVIhcUgAI4dcPIyvCog4sIAtI5wACazXCAh4gJqgQDCEFZW4shjamoLpVAB5QCCMCECeqHw8sn2jnVFEQAWMFZqAOovAvDCfmA4OZlL8BAA3X5aXZgYS6EBg8IASSgc1g5jAKX4pxR5hgWnKTx6vBk7k+hl4u2J+WSYKc3Ac7kkMCG4gC20JET8kkZuz04j0cAO3GJDhgnwEMxgL2QAA4AAzcSRwfoCJUstk7Lr1bgwfniyXSpCUbihdwZfkFbUgOBWAUzOYzWziY6hVlwABiEEk2wugV24huEBAbDYQA)
```sh
# Options (if any):
None
```
**Input:**
```jsx
x = `${[[1, 2], [3, 4]]}`
````
**Output:**
```jsx
x = `${[
[2],
[4],
]}`;
```
**Expected output:**
```jsx
x = `${[
[1, 2],
[3, 4],
]}`;
```
**Why?**
Prettier is changing the actual array contents, which it should not. Seems like it always takes the last element of each nested array.
I can work around it by pulling the array out into a separate variable ([playground link](https://prettier.io/playground/#N4Igxg9gdgLgprEAuEBDATugBAXiwbXwEYAaLAJgF0z8BmMgFksoB0oAPXLAAwBJgM6AL7cQJEBAAOMAJbQAzslCCIAdwAKGBIpSoANqtQBPReIBG6VGADWcGAGVUAWzgAZGVDjIAZvvlxzSxs7e0krDwBzZBh0AFcAkH8nGWi4hLh2STh0GRdYfQAVbKgMGTgdXz1-cXlIvTgARViIeB8-BIAreXZ7Osbm1qRK6pAARwG4dXQpHTR5AFpPOAATFbEQGNQZPUiAYQgnJ1RkND09ddqoCPqAQRgYmTNY+HVs9082qoSACxgnPQA6t8ZPB5GEwHB7NoQTIAG4gownMDyUwgWHxACSUFWsHsYBy0hu2PsMCM9U+I0k038AMskhOVPK2VhXnEHn86BgU1QESOFISYXQHJOZlQZjg53EVI8MABMmWMG+yAAHAAGcToODjGSa7m845DdriGBiuUKpVIcjiWL+ApiipGkBwJzi5arZauVBXWI8uAAMQg6CO90iJ1QzwgICEQiAA)).
| "2022-08-19T08:46:18Z" | 3.4 | [] | [
"tests/format/js/template-literals/format.test.js"
] | JavaScript | [] | [
"https://prettier.io/playground/#N4Igxg9gdgLgprEAuEBDATugBAXiwbXwEYAaLAJgF0z8BmMgFksoB0oAPXLAAwBJgM6AL7cQJEBAAOMAJbQAzslCCIAdwAKGBIpSoANqtQBPReIBG6VGADWcGAGVUAWzgAZGVDjIAZvvlxzSxs7e0krDwBzZBh0AFcAkH8nGWi4hLh2STh0GRdYfQAVbKgMGTgdXz1-cXlIvTgARViIeB8-BIAreXZ7Osbm1qRK6pAARwG4dXQpHTR5AFpPOAATFbEQGNQZPUiAYQgnJ1RkND09ddqoCPqAQRgYmTNY+HVs9082qoSACxgnPQA6t8ZPB5GEwHB7NoQTIAG4gownMDyUwgWHxACSUFWsHsYBy0hu2PsMCM9U+I0k038AMskhOVPK2VhXnEHn86BgU1QESOFISYXQHJOZlQZjg53EVI8MABMmWMG+yAAHAAGcToODjGSa7m845DdriGBiuUKpVIcjiWL+ApiipGkBwJzi5arZauVBXWI8uAAMQg6CO90iJ1QzwgICEQiAA",
"https://prettier.io/playground/#N4Igxg9gdgLgprEAuEAPABAXnQAwCTADahAjADToBMAuhYQMwUAs11AvjiGSBAA4wBLaAGdkoAIYAnSRADuABSkJRKcQBtZ4gJ6juAI0niwAazgwAyuIC2cADICocZADN1wuPsMmz53kYcA5sgwkgCuHiDuVgLBYRFwqLxwkgI2sOoAKslQUgJwKq5q7tzCgWpwAIqhEPAubhEAVsKo5mWV1bVIhcUgAI4dcPIyvCog4sIAtI5wACazXCAh4gJqgQDCEFZW4shjamoLpVAB5QCCMCECeqHw8sn2jnVFEQAWMFZqAOovAvDCfmA4OZlL8BAA3X5aXZgYS6EBg8IASSgc1g5jAKX4pxR5hgWnKTx6vBk7k+hl4u2J+WSYKc3Ac7kkMCG4gC20JET8kkZuz04j0cAO3GJDhgnwEMxgL2QAA4AAzcSRwfoCJUstk7Lr1bgwfniyXSpCUbihdwZfkFbUgOBWAUzOYzWziY6hVlwABiEEk2wugV24huEBAbDYQA",
"https://prettier.io/playground"
] |
|
prettier/prettier | 13,391 | prettier__prettier-13391 | [
"12597"
] | b7cfea5d5f85bf701560bdce070c6b66c0ac4edc | diff --git a/src/language-js/print/function.js b/src/language-js/print/function.js
--- a/src/language-js/print/function.js
+++ b/src/language-js/print/function.js
@@ -12,6 +12,7 @@ import {
softline,
group,
indent,
+ dedent,
ifBreak,
hardline,
join,
@@ -254,6 +255,10 @@ function printArrowChain(
const groupId = Symbol("arrow-chain");
+ if ((isCallLikeExpression(parent) && !isCallee) || isBinaryish(parent)) {
+ signatures = [dedent(signatures[0]), ...signatures.slice(1)];
+ }
+
// We handle sequence expressions as the body of arrows specially,
// so that the required parentheses end up on their own lines.
if (tailNode.body.type === "SequenceExpression") {
| diff --git a/tests/format/js/arrows/__snapshots__/jsfmt.spec.js.snap b/tests/format/js/arrows/__snapshots__/jsfmt.spec.js.snap
--- a/tests/format/js/arrows/__snapshots__/jsfmt.spec.js.snap
+++ b/tests/format/js/arrows/__snapshots__/jsfmt.spec.js.snap
@@ -2062,6 +2062,240 @@ foo(
================================================================================
`;
+exports[`chain-as-arg.js - {"arrowParens":"always"} format 1`] = `
+====================================options=====================================
+arrowParens: "always"
+parsers: ["babel", "typescript"]
+printWidth: 80
+ | printWidth
+=====================================input======================================
+const w = a.b(
+ (
+ c = "abcdefghijklmnopqrstuvwxyzabcdefghijklmnopqrstuvwxyzabcdef",
+ d = "abcdefghijklmnopqrstuvwxyzabcdefghijklmnopqrstuvwxyzabcdef"
+ ) =>
+ (e) =>
+ 0
+);
+
+const x = a.b(
+ (
+ c = "abcdefghijklmnopqrstuvwxyzabcdefghijklmnopqrstuvwxyzabcdef",
+ d = "abcdefghijklmnopqrstuvwxyzabcdefghijklmnopqrstuvwxyzabcdef"
+ ) =>
+ (e) =>
+ 0
+)(x);
+
+const y = a.b(
+ 1,
+ (
+ c = "abcdefghijklmnopqrstuvwxyzabcdefghijklmnopqrstuvwxyzabcdef",
+ d = "abcdefghijklmnopqrstuvwxyzabcdefghijklmnopqrstuvwxyzabcdef"
+ ) =>
+ (e) =>
+ 0
+)(x);
+
+const z = a.b(
+ (
+ c = "abcdefghijklmnopqrstuvwxyzabcdefghijklmnopqrstuvwxyzabcdef",
+ d = "abcdefghijklmnopqrstuvwxyzabcdefghijklmnopqrstuvwxyzabcdef"
+ ) =>
+ (e) =>
+ 0,
+ 2
+)(x);
+
+
+=====================================output=====================================
+const w = a.b(
+ (
+ c = "abcdefghijklmnopqrstuvwxyzabcdefghijklmnopqrstuvwxyzabcdef",
+ d = "abcdefghijklmnopqrstuvwxyzabcdefghijklmnopqrstuvwxyzabcdef",
+ ) =>
+ (e) =>
+ 0,
+);
+
+const x = a.b(
+ (
+ c = "abcdefghijklmnopqrstuvwxyzabcdefghijklmnopqrstuvwxyzabcdef",
+ d = "abcdefghijklmnopqrstuvwxyzabcdefghijklmnopqrstuvwxyzabcdef",
+ ) =>
+ (e) =>
+ 0,
+)(x);
+
+const y = a.b(
+ 1,
+ (
+ c = "abcdefghijklmnopqrstuvwxyzabcdefghijklmnopqrstuvwxyzabcdef",
+ d = "abcdefghijklmnopqrstuvwxyzabcdefghijklmnopqrstuvwxyzabcdef",
+ ) =>
+ (e) =>
+ 0,
+)(x);
+
+const z = a.b(
+ (
+ c = "abcdefghijklmnopqrstuvwxyzabcdefghijklmnopqrstuvwxyzabcdef",
+ d = "abcdefghijklmnopqrstuvwxyzabcdefghijklmnopqrstuvwxyzabcdef",
+ ) =>
+ (e) =>
+ 0,
+ 2,
+)(x);
+
+================================================================================
+`;
+
+exports[`chain-as-arg.js - {"arrowParens":"avoid"} format 1`] = `
+====================================options=====================================
+arrowParens: "avoid"
+parsers: ["babel", "typescript"]
+printWidth: 80
+ | printWidth
+=====================================input======================================
+const w = a.b(
+ (
+ c = "abcdefghijklmnopqrstuvwxyzabcdefghijklmnopqrstuvwxyzabcdef",
+ d = "abcdefghijklmnopqrstuvwxyzabcdefghijklmnopqrstuvwxyzabcdef"
+ ) =>
+ (e) =>
+ 0
+);
+
+const x = a.b(
+ (
+ c = "abcdefghijklmnopqrstuvwxyzabcdefghijklmnopqrstuvwxyzabcdef",
+ d = "abcdefghijklmnopqrstuvwxyzabcdefghijklmnopqrstuvwxyzabcdef"
+ ) =>
+ (e) =>
+ 0
+)(x);
+
+const y = a.b(
+ 1,
+ (
+ c = "abcdefghijklmnopqrstuvwxyzabcdefghijklmnopqrstuvwxyzabcdef",
+ d = "abcdefghijklmnopqrstuvwxyzabcdefghijklmnopqrstuvwxyzabcdef"
+ ) =>
+ (e) =>
+ 0
+)(x);
+
+const z = a.b(
+ (
+ c = "abcdefghijklmnopqrstuvwxyzabcdefghijklmnopqrstuvwxyzabcdef",
+ d = "abcdefghijklmnopqrstuvwxyzabcdefghijklmnopqrstuvwxyzabcdef"
+ ) =>
+ (e) =>
+ 0,
+ 2
+)(x);
+
+
+=====================================output=====================================
+const w = a.b(
+ (
+ c = "abcdefghijklmnopqrstuvwxyzabcdefghijklmnopqrstuvwxyzabcdef",
+ d = "abcdefghijklmnopqrstuvwxyzabcdefghijklmnopqrstuvwxyzabcdef",
+ ) =>
+ e =>
+ 0,
+);
+
+const x = a.b(
+ (
+ c = "abcdefghijklmnopqrstuvwxyzabcdefghijklmnopqrstuvwxyzabcdef",
+ d = "abcdefghijklmnopqrstuvwxyzabcdefghijklmnopqrstuvwxyzabcdef",
+ ) =>
+ e =>
+ 0,
+)(x);
+
+const y = a.b(
+ 1,
+ (
+ c = "abcdefghijklmnopqrstuvwxyzabcdefghijklmnopqrstuvwxyzabcdef",
+ d = "abcdefghijklmnopqrstuvwxyzabcdefghijklmnopqrstuvwxyzabcdef",
+ ) =>
+ e =>
+ 0,
+)(x);
+
+const z = a.b(
+ (
+ c = "abcdefghijklmnopqrstuvwxyzabcdefghijklmnopqrstuvwxyzabcdef",
+ d = "abcdefghijklmnopqrstuvwxyzabcdefghijklmnopqrstuvwxyzabcdef",
+ ) =>
+ e =>
+ 0,
+ 2,
+)(x);
+
+================================================================================
+`;
+
+exports[`chain-in-logical-expression.js - {"arrowParens":"always"} format 1`] = `
+====================================options=====================================
+arrowParens: "always"
+parsers: ["babel", "typescript"]
+printWidth: 80
+ | printWidth
+=====================================input======================================
+const x = a.b ?? (
+ (
+ c = "abcdefghijklmnopqrstuvwxyzabcdefghijklmnopqrstuvwxyzabcdef",
+ d = "abcdefghijklmnopqrstuvwxyzabcdefghijklmnopqrstuvwxyzabcdef"
+ ) =>
+ (e) =>
+ 0
+);
+
+=====================================output=====================================
+const x =
+ a.b ??
+ ((
+ c = "abcdefghijklmnopqrstuvwxyzabcdefghijklmnopqrstuvwxyzabcdef",
+ d = "abcdefghijklmnopqrstuvwxyzabcdefghijklmnopqrstuvwxyzabcdef",
+ ) =>
+ (e) =>
+ 0);
+
+================================================================================
+`;
+
+exports[`chain-in-logical-expression.js - {"arrowParens":"avoid"} format 1`] = `
+====================================options=====================================
+arrowParens: "avoid"
+parsers: ["babel", "typescript"]
+printWidth: 80
+ | printWidth
+=====================================input======================================
+const x = a.b ?? (
+ (
+ c = "abcdefghijklmnopqrstuvwxyzabcdefghijklmnopqrstuvwxyzabcdef",
+ d = "abcdefghijklmnopqrstuvwxyzabcdefghijklmnopqrstuvwxyzabcdef"
+ ) =>
+ (e) =>
+ 0
+);
+
+=====================================output=====================================
+const x =
+ a.b ??
+ ((
+ c = "abcdefghijklmnopqrstuvwxyzabcdefghijklmnopqrstuvwxyzabcdef",
+ d = "abcdefghijklmnopqrstuvwxyzabcdefghijklmnopqrstuvwxyzabcdef",
+ ) =>
+ e =>
+ 0);
+
+================================================================================
+`;
+
exports[`comment.js - {"arrowParens":"always"} format 1`] = `
====================================options=====================================
arrowParens: "always"
diff --git a/tests/format/js/arrows/chain-as-arg.js b/tests/format/js/arrows/chain-as-arg.js
new file mode 100644
--- /dev/null
+++ b/tests/format/js/arrows/chain-as-arg.js
@@ -0,0 +1,38 @@
+const w = a.b(
+ (
+ c = "abcdefghijklmnopqrstuvwxyzabcdefghijklmnopqrstuvwxyzabcdef",
+ d = "abcdefghijklmnopqrstuvwxyzabcdefghijklmnopqrstuvwxyzabcdef"
+ ) =>
+ (e) =>
+ 0
+);
+
+const x = a.b(
+ (
+ c = "abcdefghijklmnopqrstuvwxyzabcdefghijklmnopqrstuvwxyzabcdef",
+ d = "abcdefghijklmnopqrstuvwxyzabcdefghijklmnopqrstuvwxyzabcdef"
+ ) =>
+ (e) =>
+ 0
+)(x);
+
+const y = a.b(
+ 1,
+ (
+ c = "abcdefghijklmnopqrstuvwxyzabcdefghijklmnopqrstuvwxyzabcdef",
+ d = "abcdefghijklmnopqrstuvwxyzabcdefghijklmnopqrstuvwxyzabcdef"
+ ) =>
+ (e) =>
+ 0
+)(x);
+
+const z = a.b(
+ (
+ c = "abcdefghijklmnopqrstuvwxyzabcdefghijklmnopqrstuvwxyzabcdef",
+ d = "abcdefghijklmnopqrstuvwxyzabcdefghijklmnopqrstuvwxyzabcdef"
+ ) =>
+ (e) =>
+ 0,
+ 2
+)(x);
+
diff --git a/tests/format/js/arrows/chain-in-logical-expression.js b/tests/format/js/arrows/chain-in-logical-expression.js
new file mode 100644
--- /dev/null
+++ b/tests/format/js/arrows/chain-in-logical-expression.js
@@ -0,0 +1,8 @@
+const x = a.b ?? (
+ (
+ c = "abcdefghijklmnopqrstuvwxyzabcdefghijklmnopqrstuvwxyzabcdef",
+ d = "abcdefghijklmnopqrstuvwxyzabcdefghijklmnopqrstuvwxyzabcdef"
+ ) =>
+ (e) =>
+ 0
+);
| Misaligned indent in curried arrow function
**Prettier 2.6.2**
[Playground link](https://prettier.io/playground/#N4Igxg9gdgLgprEAuEwA6UAEn1ezjffSKAZxkwA9MBeTAQQDoAjACkKP3b0+NszQgAhszAATOADMA5gAsAlgCsA1gBsAtlAgAHAI4AncgFcAbgHdKATwBeI8VLlK1mnQePmrt0RMmCANBy82GL8gnY+jioaWnqGMKYWNuEOClEuse6JXva+IIGcAJS0AHz5RKxwRTTFmAAMZZgFANz5AL4c7VCdIH4gOjDy0KTIoEL6+hBmAApjCMMoQqpmQpbDvcz6QmDKcDAAykLqcAAy8lBwyJKLpHDrm9u7e9pbZ9LIMPpGtyBw6sxwYgkYmOQig0iMQmkcAAYhB9OohDABmDkMIjDAID0QLIYOpVAB1BTwUjPMBwPZzeQDExUyyosCkNYgM43fQwKabaQIy7Xb6KUiUPavVRwACKRgg8B5qhuvWehjg+lRMEs2jgpDA+nk2hgWO0Wtg+PkYhgsmQAEYAEy1OUTG74zbaVH69WKkwXXq6CXwDk6ebCUgAWnOAIBWP0cC98gjHMh3KQVxl3xu6nk70+yeFYu9FwTvN6MBERpNZqQloLm3kqleAGEIOp4z9SABWLFGG4AFRE80TspAJi+AEkoBJYHtNdqYPQR3sVSLpTdWq0gA)
<!-- prettier-ignore -->
```sh
--parser typescript
--print-width 120
```
**Input:**
<!-- prettier-ignore -->
```tsx
{
{
{
const x = A.b(
(
c = "abcdefghijklmnopqrstuvwxyzabcdefghijklmnopqrstuvwxyzabcdef",
d = "abcdefghijklmnopqrstuvwxyzabcdefghijklmnopqrstuvwxyzabcdef"
) =>
(e) => 0
);
}
}
}
```
**Output:**
<!-- prettier-ignore -->
```tsx
{
{
{
const x = A.b(
(
c = "abcdefghijklmnopqrstuvwxyzabcdefghijklmnopqrstuvwxyzabcdef",
d = "abcdefghijklmnopqrstuvwxyzabcdefghijklmnopqrstuvwxyzabcdef"
) =>
(e) =>
0
);
}
}
}
```
**Expected behavior:**
Same as Input.
| "2022-08-28T15:28:50Z" | 3.0 | [] | [
"tests/format/js/arrows/jsfmt.spec.js"
] | JavaScript | [] | [
"https://prettier.io/playground/#N4Igxg9gdgLgprEAuEwA6UAEn1ezjffSKAZxkwA9MBeTAQQDoAjACkKP3b0+NszQgAhszAATOADMA5gAsAlgCsA1gBsAtlAgAHAI4AncgFcAbgHdKATwBeI8VLlK1mnQePmrt0RMmCANBy82GL8gnY+jioaWnqGMKYWNuEOClEuse6JXva+IIGcAJS0AHz5RKxwRTTFmAAMZZgFANz5AL4c7VCdIH4gOjDy0KTIoEL6+hBmAApjCMMoQqpmQpbDvcz6QmDKcDAAykLqcAAy8lBwyJKLpHDrm9u7e9pbZ9LIMPpGtyBw6sxwYgkYmOQig0iMQmkcAAYhB9OohDABmDkMIjDAID0QLIYOpVAB1BTwUjPMBwPZzeQDExUyyosCkNYgM43fQwKabaQIy7Xb6KUiUPavVRwACKRgg8B5qhuvWehjg+lRMEs2jgpDA+nk2hgWO0Wtg+PkYhgsmQAEYAEy1OUTG74zbaVH69WKkwXXq6CXwDk6ebCUgAWnOAIBWP0cC98gjHMh3KQVxl3xu6nk70+yeFYu9FwTvN6MBERpNZqQloLm3kqleAGEIOp4z9SABWLFGG4AFRE80TspAJi+AEkoBJYHtNdqYPQR3sVSLpTdWq0gA"
] |
|
prettier/prettier | 13,396 | prettier__prettier-13396 | [
"13395"
] | c8ff3870c321b2d6a99387801437273fc8ea40cf | diff --git a/src/language-js/print/function-parameters.js b/src/language-js/print/function-parameters.js
--- a/src/language-js/print/function-parameters.js
+++ b/src/language-js/print/function-parameters.js
@@ -62,7 +62,7 @@ function printFunctionParameters(
const parent = path.getParentNode();
const isParametersInTestCall = isTestCall(parent);
- const shouldHugParameters = shouldHugFunctionParameters(functionNode);
+ const shouldHugParameters = shouldHugTheOnlyFunctionParameter(functionNode);
const printed = [];
iterateFunctionParametersPath(path, (parameterPath, index) => {
const isLastParameter = index === parameters.length - 1;
@@ -157,7 +157,7 @@ function printFunctionParameters(
];
}
-function shouldHugFunctionParameters(node) {
+function shouldHugTheOnlyFunctionParameter(node) {
if (!node) {
return false;
}
@@ -176,7 +176,8 @@ function shouldHugFunctionParameters(node) {
parameter.typeAnnotation.type === "TSTypeAnnotation") &&
isObjectType(parameter.typeAnnotation.typeAnnotation)) ||
(parameter.type === "FunctionTypeParam" &&
- isObjectType(parameter.typeAnnotation)) ||
+ isObjectType(parameter.typeAnnotation) &&
+ parameter !== node.rest) ||
(parameter.type === "AssignmentPattern" &&
(parameter.left.type === "ObjectPattern" ||
parameter.left.type === "ArrayPattern") &&
@@ -283,6 +284,6 @@ function isDecoratedFunction(path) {
export {
printFunctionParameters,
- shouldHugFunctionParameters,
+ shouldHugTheOnlyFunctionParameter,
shouldGroupFunctionParameters,
};
diff --git a/src/language-js/print/object.js b/src/language-js/print/object.js
--- a/src/language-js/print/object.js
+++ b/src/language-js/print/object.js
@@ -19,12 +19,12 @@ import {
getComments,
CommentCheckFlags,
isNextLineEmpty,
+ isObjectType,
} from "../utils/index.js";
import { locStart, locEnd } from "../loc.js";
import { printOptionalToken, printTypeAnnotation } from "./misc.js";
-import { shouldHugFunctionParameters } from "./function-parameters.js";
-import { shouldHugType } from "./type-annotation.js";
+import { shouldHugTheOnlyFunctionParameter } from "./function-parameters.js";
import { printHardlineAfterHeritage } from "./class.js";
/** @typedef {import("../../document/builders.js").Doc} Doc */
@@ -201,26 +201,21 @@ function printObject(path, options, print) {
if (
path.match(
(node) => node.type === "ObjectPattern" && !node.decorators,
- (node, name, number) =>
- shouldHugFunctionParameters(node) &&
- (name === "params" ||
- name === "parameters" ||
- name === "this" ||
- name === "rest") &&
- number === 0
- ) ||
- path.match(
- shouldHugType,
- (node, name) => name === "typeAnnotation",
- (node, name) => name === "typeAnnotation",
- (node, name, number) =>
- shouldHugFunctionParameters(node) &&
- (name === "params" ||
- name === "parameters" ||
- name === "this" ||
- name === "rest") &&
- number === 0
+ shouldHugTheOnlyParameter
) ||
+ (isObjectType(node) &&
+ (path.match(
+ undefined,
+ (node, name) => name === "typeAnnotation",
+ (node, name) => name === "typeAnnotation",
+ shouldHugTheOnlyParameter
+ ) ||
+ path.match(
+ undefined,
+ (node, name) =>
+ node.type === "FunctionTypeParam" && name === "typeAnnotation",
+ shouldHugTheOnlyParameter
+ ))) ||
// Assignment printing logic (printAssignment) is responsible
// for adding a group if needed
(!shouldBreak &&
@@ -237,4 +232,14 @@ function printObject(path, options, print) {
return group(content, { shouldBreak });
}
+function shouldHugTheOnlyParameter(node, name) {
+ return (
+ (name === "params" ||
+ name === "parameters" ||
+ name === "this" ||
+ name === "rest") &&
+ shouldHugTheOnlyFunctionParameter(node)
+ );
+}
+
export { printObject };
| diff --git a/tests/format/flow/function-single-destructuring/__snapshots__/jsfmt.spec.js.snap b/tests/format/flow/function-single-destructuring/__snapshots__/jsfmt.spec.js.snap
--- a/tests/format/flow/function-single-destructuring/__snapshots__/jsfmt.spec.js.snap
+++ b/tests/format/flow/function-single-destructuring/__snapshots__/jsfmt.spec.js.snap
@@ -133,3 +133,45 @@ const X = (props: { a: boolean }) => <A />;
================================================================================
`;
+
+exports[`object-type-in-declare-function.js format 1`] = `
+====================================options=====================================
+parsers: ["flow", "babel"]
+printWidth: 80
+ | printWidth
+=====================================input======================================
+declare function foo(this: { a: boolean, b: string, c: number }):
+ Promise<Array<foo>>
+
+declare function bazFlip({ a: boolean, b: string, c: number }):
+ Promise<Array<foo>>
+
+declare function bar(...{ a: boolean, b: string, c: number }):
+ Promise<Array<foo>>
+
+declare function bar(...x: { a: boolean, b: string, c: number }):
+ Promise<Array<foo>>
+
+=====================================output=====================================
+declare function foo(this: {
+ a: boolean,
+ b: string,
+ c: number,
+}): Promise<Array<foo>>;
+
+declare function bazFlip({
+ a: boolean,
+ b: string,
+ c: number,
+}): Promise<Array<foo>>;
+
+declare function bar(
+ ...{ a: boolean, b: string, c: number }
+): Promise<Array<foo>>;
+
+declare function bar(
+ ...x: { a: boolean, b: string, c: number }
+): Promise<Array<foo>>;
+
+================================================================================
+`;
diff --git a/tests/format/flow/function-single-destructuring/object-type-in-declare-function.js b/tests/format/flow/function-single-destructuring/object-type-in-declare-function.js
new file mode 100644
--- /dev/null
+++ b/tests/format/flow/function-single-destructuring/object-type-in-declare-function.js
@@ -0,0 +1,11 @@
+declare function foo(this: { a: boolean, b: string, c: number }):
+ Promise<Array<foo>>
+
+declare function bazFlip({ a: boolean, b: string, c: number }):
+ Promise<Array<foo>>
+
+declare function bar(...{ a: boolean, b: string, c: number }):
+ Promise<Array<foo>>
+
+declare function bar(...x: { a: boolean, b: string, c: number }):
+ Promise<Array<foo>>
diff --git a/tests/format/typescript/declare/__snapshots__/jsfmt.spec.js.snap b/tests/format/typescript/declare/__snapshots__/jsfmt.spec.js.snap
--- a/tests/format/typescript/declare/__snapshots__/jsfmt.spec.js.snap
+++ b/tests/format/typescript/declare/__snapshots__/jsfmt.spec.js.snap
@@ -215,3 +215,45 @@ class C {
================================================================================
`;
+
+exports[`object-type-in-declare-function.ts format 1`] = `
+====================================options=====================================
+parsers: ["typescript"]
+printWidth: 80
+ | printWidth
+=====================================input======================================
+declare function foo(this: { a: boolean, b: string, c: number }):
+ Promise<Array<foo>>
+
+declare function bazFlip({ a: boolean, b: string, c: number }):
+ Promise<Array<foo>>
+
+declare function bar(...{ a: boolean, b: string, c: number }):
+ Promise<Array<foo>>
+
+declare function bar(...x: { a: boolean, b: string, c: number }):
+ Promise<Array<foo>>
+
+=====================================output=====================================
+declare function foo(this: {
+ a: boolean;
+ b: string;
+ c: number;
+}): Promise<Array<foo>>;
+
+declare function bazFlip({
+ a: boolean,
+ b: string,
+ c: number,
+}): Promise<Array<foo>>;
+
+declare function bar(
+ ...{ a: boolean, b: string, c: number }
+): Promise<Array<foo>>;
+
+declare function bar(
+ ...x: { a: boolean; b: string; c: number }
+): Promise<Array<foo>>;
+
+================================================================================
+`;
diff --git a/tests/format/typescript/declare/object-type-in-declare-function.ts b/tests/format/typescript/declare/object-type-in-declare-function.ts
new file mode 100644
--- /dev/null
+++ b/tests/format/typescript/declare/object-type-in-declare-function.ts
@@ -0,0 +1,11 @@
+declare function foo(this: { a: boolean, b: string, c: number }):
+ Promise<Array<foo>>
+
+declare function bazFlip({ a: boolean, b: string, c: number }):
+ Promise<Array<foo>>
+
+declare function bar(...{ a: boolean, b: string, c: number }):
+ Promise<Array<foo>>
+
+declare function bar(...x: { a: boolean, b: string, c: number }):
+ Promise<Array<foo>>
| `declare function` formatted differently for Flow and TypeScript
**Prettier 2.7.1**
[Playground link](https://prettier.io/playground/#N4Igxg9gdgLgprEAuEATOYA2BDATnAAgDMBXKMGAS2gICMcCAKAB1wmYGckDgDtvaECJjjYoAGjrcOMXJSgBzSWG5QSAW1pxcBAL4BKJAB0oBAgAU26yhzgAeAIK5c2AJ52iQgHxeQ4kOxU0BzIoHhsAO7meAghKNiYEW4h-rQuYADWcDAAytjqcAAy8nDIRAm2qelZuczYYPIKyLIkcP5wmnCo6KiFYgok2ApwAGIQuOrYMFSKyCDYJDAQfiAAFjDqmADqq5TwHHVgcDmxe5QAbnuuc2AcKSDytrgwlkOTZRVtIABWHAAeOUaIgAiiQIPAPphKiA6rgnnMYK5mHAOGA5MwYCtWPIYFtKKgYKtkAAOAAM-lYEFsWxczDmrBR2nOpX8AEcwfBLOw4vMOABaKBwLpdFb4dmUfCvBTvJDlKFfWzWZq4Vr+DhAuCg8GlWWffwwbC0PEEolIABM+pclEwjQAwhB1DKQCiAKwrEi2AAqhricuh51aAEkoOhYDk0ZQMQ4QzlESJIbZdLogA)
<!-- prettier-ignore -->
```sh
--parser typescript
```
**Input:**
<!-- prettier-ignore -->
```tsx
declare function bla (props: { a: boolean, b: string, c: number }):
Promise<Array<foo>>
```
**Output:**
<!-- prettier-ignore -->
```tsx
declare function bla(props: {
a: boolean;
b: string;
c: number;
}): Promise<Array<foo>>;
```
**Prettier 2.7.1**
[Playground link](https://prettier.io/playground/#N4Igxg9gdgLgprEAuEATOYA2BDATnAAgDMBXKMGAS2gICMcCAKAB1wmYGckDgDtvaECJjjYoAGjrcOMXJSgBzSWG5QSAW1pxcBAL4BKJAB0oBAgAU26yhzgAeAIK5c2AJ52iQgHxeQ4kOxU0BzIoHhsAO7meAghKNiYEW4h-rQuYADWcDAAytjqcAAy8nDIRAm2qelZuczYYPIKyLIkcP5wmnCo6KiFYgok2ApwAGIQuOrYMFSKyCDYJDAQfiAAFjDqmADqq5TwHHVgcDmxe5QAbnuuc2AcKSDytrgwlkOTZRVtIABWHAAeOUaIgAiiQIPAPphKiA6rgnnMiJgIBEVqx5DAtpRUDBVsgABwABn8rAgti2LmYc1YcCe51K-gAjmD4JZ2HF5hwALRQOBdLorfBMyj4V4Kd5IcpQr62azNXCtfwcIFwUHg0oSz7+GDYWiY7G4pAAJi1LkomEaAGEIOpxSAaQBWFYkWwAFR1cUl0POrQAklB0LAcmA5MwYA5-TkYK4RJDbLpdEA)
<!-- prettier-ignore -->
```sh
--parser flow
```
**Output:**
<!-- prettier-ignore -->
```jsx
declare function bla(props: { a: boolean, b: string, c: number }): Promise<
Array<foo>
>;
```
**Expected behavior:**
The formatting used for TS ("the object type breaks before the return type") should be use for Flow too.
Related: https://github.com/prettier/prettier/pull/13393#issuecomment-1229540128
| "2022-08-28T23:29:36Z" | 3.0 | [
"tests/format/typescript/declare/jsfmt.spec.js"
] | [
"tests/format/flow/function-single-destructuring/jsfmt.spec.js"
] | JavaScript | [] | [
"https://prettier.io/playground/#N4Igxg9gdgLgprEAuEATOYA2BDATnAAgDMBXKMGAS2gICMcCAKAB1wmYGckDgDtvaECJjjYoAGjrcOMXJSgBzSWG5QSAW1pxcBAL4BKJAB0oBAgAU26yhzgAeAIK5c2AJ52iQgHxeQ4kOxU0BzIoHhsAO7meAghKNiYEW4h-rQuYADWcDAAytjqcAAy8nDIRAm2qelZuczYYPIKyLIkcP5wmnCo6KiFYgok2ApwAGIQuOrYMFSKyCDYJDAQfiAAFjDqmADqq5TwHHVgcDmxe5QAbnuuc2AcKSDytrgwlkOTZRVtIABWHAAeOUaIgAiiQIPAPphKiA6rgnnMiJgIBEVqx5DAtpRUDBVsgABwABn8rAgti2LmYc1YcCe51K-gAjmD4JZ2HF5hwALRQOBdLorfBMyj4V4Kd5IcpQr62azNXCtfwcIFwUHg0oSz7+GDYWiY7G4pAAJi1LkomEaAGEIOpxSAaQBWFYkWwAFR1cUl0POrQAklB0LAcmA5MwYA5-TkYK4RJDbLpdEA",
"https://prettier.io/playground/#N4Igxg9gdgLgprEAuEATOYA2BDATnAAgDMBXKMGAS2gICMcCAKAB1wmYGckDgDtvaECJjjYoAGjrcOMXJSgBzSWG5QSAW1pxcBAL4BKJAB0oBAgAU26yhzgAeAIK5c2AJ52iQgHxeQ4kOxU0BzIoHhsAO7meAghKNiYEW4h-rQuYADWcDAAytjqcAAy8nDIRAm2qelZuczYYPIKyLIkcP5wmnCo6KiFYgok2ApwAGIQuOrYMFSKyCDYJDAQfiAAFjDqmADqq5TwHHVgcDmxe5QAbnuuc2AcKSDytrgwlkOTZRVtIABWHAAeOUaIgAiiQIPAPphKiA6rgnnMYK5mHAOGA5MwYCtWPIYFtKKgYKtkAAOAAM-lYEFsWxczDmrBR2nOpX8AEcwfBLOw4vMOABaKBwLpdFb4dmUfCvBTvJDlKFfWzWZq4Vr+DhAuCg8GlWWffwwbC0PEEolIABM+pclEwjQAwhB1DKQCiAKwrEi2AAqhricuh51aAEkoOhYDk0ZQMQ4QzlESJIbZdLogA"
] |
|
prettier/prettier | 13,402 | prettier__prettier-13402 | [
"13202"
] | 26ea4c42fd3718f4dc9ff600171f4a64ffd54802 | diff --git a/src/language-css/printer-postcss.js b/src/language-css/printer-postcss.js
--- a/src/language-css/printer-postcss.js
+++ b/src/language-css/printer-postcss.js
@@ -72,6 +72,7 @@ import {
isAtWordPlaceholderNode,
isConfigurationNode,
isParenGroupNode,
+ isVarFunctionNode,
} from "./utils/index.js";
import { locStart, locEnd } from "./loc.js";
import printUnit from "./utils/print-unit.js";
@@ -901,6 +902,7 @@ function genericPrint(path, options, print) {
const isLastItemComment = lastItem && lastItem.type === "value-comment";
const isKey = isKeyInValuePairNode(node, parentNode);
const isConfiguration = isConfigurationNode(node, parentNode);
+ const isVarFunction = isVarFunctionNode(parentNode);
const shouldBreak = isConfiguration || (isSCSSMapItem && !isKey);
const shouldDedent = isConfiguration || isKey;
@@ -915,7 +917,17 @@ function genericPrint(path, options, print) {
path.map((childPath, index) => {
const child = childPath.getValue();
const isLast = index === node.groups.length - 1;
- const printed = [print(), isLast ? "" : ","];
+ const hasComma = () =>
+ Boolean(
+ child.source &&
+ options.originalText
+ .slice(locStart(child), locStart(node.close))
+ .trimEnd()
+ .endsWith(",")
+ );
+ const shouldPrintComma =
+ !isLast || (isVarFunction && hasComma());
+ const printed = [print(), shouldPrintComma ? "," : ""];
// Key/Value pair in open paren already indented
if (
diff --git a/src/language-css/utils/index.js b/src/language-css/utils/index.js
--- a/src/language-css/utils/index.js
+++ b/src/language-css/utils/index.js
@@ -120,6 +120,10 @@ function isURLFunctionNode(node) {
return node.type === "value-func" && node.value.toLowerCase() === "url";
}
+function isVarFunctionNode(node) {
+ return node.type === "value-func" && node.value.toLowerCase() === "var";
+}
+
function isLastNode(path, node) {
const nodes = path.getParentNode()?.nodes;
return nodes && nodes.indexOf(node) === nodes.length - 1;
@@ -444,4 +448,5 @@ export {
isAtWordPlaceholderNode,
isConfigurationNode,
isParenGroupNode,
+ isVarFunctionNode,
};
| diff --git a/tests/format/css/trailing-comma/__snapshots__/jsfmt.spec.js.snap b/tests/format/css/trailing-comma/__snapshots__/jsfmt.spec.js.snap
new file mode 100644
--- /dev/null
+++ b/tests/format/css/trailing-comma/__snapshots__/jsfmt.spec.js.snap
@@ -0,0 +1,27 @@
+// Jest Snapshot v1, https://goo.gl/fbAQLP
+
+exports[`var-func.css format 1`] = `
+====================================options=====================================
+parsers: ["css"]
+printWidth: 80
+ | printWidth
+=====================================input======================================
+.foo {
+ --bar: var(--baz,);
+ --bar: var(--baz ,);
+ --bar: var(--baz , );
+ --bar: var(--baz,);
+ --bar: var( --baz1, --baz2 , );
+}
+
+=====================================output=====================================
+.foo {
+ --bar: var(--baz,);
+ --bar: var(--baz,);
+ --bar: var(--baz,);
+ --bar: var(--baz,);
+ --bar: var(--baz1, --baz2,);
+}
+
+================================================================================
+`;
diff --git a/tests/format/css/trailing-comma/jsfmt.spec.js b/tests/format/css/trailing-comma/jsfmt.spec.js
new file mode 100644
--- /dev/null
+++ b/tests/format/css/trailing-comma/jsfmt.spec.js
@@ -0,0 +1 @@
+run_spec(import.meta, ["css"]);
diff --git a/tests/format/css/trailing-comma/var-func.css b/tests/format/css/trailing-comma/var-func.css
new file mode 100644
--- /dev/null
+++ b/tests/format/css/trailing-comma/var-func.css
@@ -0,0 +1,7 @@
+.foo {
+ --bar: var(--baz,);
+ --bar: var(--baz ,);
+ --bar: var(--baz , );
+ --bar: var(--baz,);
+ --bar: var( --baz1, --baz2 , );
+}
| [CSS] Prettier removes trailing comma inside `var` function
**Prettier 2.7.1**
[Playground link](https://prettier.io/playground/#N4Igxg9gdgLgprEAuEA6AZhCACYAdKbbAWmICMBDAJyWwDdqAKUygLwBoBKAbgIF8Q7EBAAOMAJbQAzslDUqEAO4AFaghkoKAG0UUAnjKFkqFMAGs4MAMoUAtnAAy4qHGTptUuEZPnLVkabOAObIMFQArl4gcLZkcAAm8QkOFFBB4RRBcABiEFS2FDASacggFOEwEIIgABYwtloA6jXi8FIBYHBW6q3idK16pWBShiDOnlQwyiZBBW4eUQBWUgAeVsFacACK4RDw81qeQgFUE0Mj1SJUzjCN4vEwNcgAHAAMxwqejSYipVdwEzoriEAEddvBpqINGUpMQXAkEtUqHAweJkdNMnMkO5DlFPLZxKEIniNttwa5sQshDAKGQ7g8nkgAEzUkziLTBADCEFsWOiUgArNVwp4ACq0jQ4o4gOiRACSUCSsCsYGuYgAgoqrDA9JsDp4+HwgA)
<!-- prettier-ignore -->
```sh
--parser css
```
**Input:**
<!-- prettier-ignore -->
```css
.foo {
--bar: var(--baz,);
}
```
**Output:**
<!-- prettier-ignore -->
```css
.foo {
--bar: var(--baz);
}
```
**Expected behavior:**
The bare comma, which indicates "fallback to nothing," remains.
<!-- prettier-ignore -->
```css
.foo {
--bar: var(--baz,);
}
```
## Spec
https://www.w3.org/TR/css-variables-1/#using-variables
> In an exception to the usual [comma elision rules](https://www.w3.org/TR/css-values-4/#comb-comma), which require commas to be omitted when they’re not separating values, a bare comma, with nothing following it, must be treated as valid in [var()](https://www.w3.org/TR/css-variables-1/#funcdef-var), indicating an empty fallback value.
> Tests
>
> Note: That is, var(--a,) is a valid function, specifying that if the --a custom property is invalid or missing, the [var()](https://www.w3.org/TR/css-variables-1/#funcdef-var) should be replaced with nothing.
| "2022-08-30T14:33:51Z" | 3.0 | [] | [
"tests/format/css/trailing-comma/jsfmt.spec.js"
] | JavaScript | [] | [
"https://prettier.io/playground/#N4Igxg9gdgLgprEAuEA6AZhCACYAdKbbAWmICMBDAJyWwDdqAKUygLwBoBKAbgIF8Q7EBAAOMAJbQAzslDUqEAO4AFaghkoKAG0UUAnjKFkqFMAGs4MAMoUAtnAAy4qHGTptUuEZPnLVkabOAObIMFQArl4gcLZkcAAm8QkOFFBB4RRBcABiEFS2FDASacggFOEwEIIgABYwtloA6jXi8FIBYHBW6q3idK16pWBShiDOnlQwyiZBBW4eUQBWUgAeVsFacACK4RDw81qeQgFUE0Mj1SJUzjCN4vEwNcgAHAAMxwqejSYipVdwEzoriEAEddvBpqINGUpMQXAkEtUqHAweJkdNMnMkO5DlFPLZxKEIniNttwa5sQshDAKGQ7g8nkgAEzUkziLTBADCEFsWOiUgArNVwp4ACq0jQ4o4gOiRACSUCSsCsYGuYgAgoqrDA9JsDp4+HwgA"
] |
|
prettier/prettier | 13,410 | prettier__prettier-13410 | [
"11794"
] | 40ffe244a35274419aa9aa15458490c24293f426 | diff --git a/src/language-js/print/function.js b/src/language-js/print/function.js
--- a/src/language-js/print/function.js
+++ b/src/language-js/print/function.js
@@ -57,7 +57,13 @@ function printFunction(path, print, options, args) {
args?.expandLastArg
) {
const parent = path.getParentNode();
- if (isCallExpression(parent) && getCallArguments(parent).length > 1) {
+ if (
+ isCallExpression(parent) &&
+ (getCallArguments(parent).length > 1 ||
+ getFunctionParameters(node).every(
+ (param) => param.type === "Identifier" && !param.typeAnnotation
+ ))
+ ) {
expandArg = true;
}
}
| diff --git a/tests/format/typescript/last-argument-expansion/__snapshots__/jsfmt.spec.js.snap b/tests/format/typescript/last-argument-expansion/__snapshots__/jsfmt.spec.js.snap
--- a/tests/format/typescript/last-argument-expansion/__snapshots__/jsfmt.spec.js.snap
+++ b/tests/format/typescript/last-argument-expansion/__snapshots__/jsfmt.spec.js.snap
@@ -197,3 +197,69 @@ var listener = DOM.listen(
================================================================================
`;
+
+exports[`forward-ref.ts format 1`] = `
+====================================options=====================================
+parsers: ["typescript"]
+printWidth: 80
+ | printWidth
+=====================================input======================================
+export const Link = forwardRef<HTMLAnchorElement, LinkProps>(
+ function Link(props, ref) {
+ return <ThemeUILink ref={ref} variant="default" {...props} />;
+ }
+);
+
+export const LinkWithLongName = forwardRef<HTMLAnchorElement, LinkProps>(
+ function Link(props, ref) {
+ return <ThemeUILink ref={ref} variant="default" {...props} />;
+ }
+);
+
+export const Arrow = forwardRef<HTMLAnchorElement, LinkProps>((props, ref) => {
+ return <ThemeUILink ref={ref} variant="default" {...props} />;
+});
+
+export const ArrowWithLongName = forwardRef<HTMLAnchorElement, LinkProps>(
+ (props, ref) => {
+ return <ThemeUILink ref={ref} variant="default" {...props} />;
+ }
+);
+
+const Link = React.forwardRef<HTMLAnchorElement, LinkProps>(
+ function Link(props, ref) {
+ return <ThemeUILink ref={ref} variant="default" {...props} />;
+ },
+);
+
+=====================================output=====================================
+export const Link = forwardRef<HTMLAnchorElement, LinkProps>(
+ function Link(props, ref) {
+ return <ThemeUILink ref={ref} variant="default" {...props} />;
+ },
+);
+
+export const LinkWithLongName = forwardRef<HTMLAnchorElement, LinkProps>(
+ function Link(props, ref) {
+ return <ThemeUILink ref={ref} variant="default" {...props} />;
+ },
+);
+
+export const Arrow = forwardRef<HTMLAnchorElement, LinkProps>((props, ref) => {
+ return <ThemeUILink ref={ref} variant="default" {...props} />;
+});
+
+export const ArrowWithLongName = forwardRef<HTMLAnchorElement, LinkProps>(
+ (props, ref) => {
+ return <ThemeUILink ref={ref} variant="default" {...props} />;
+ },
+);
+
+const Link = React.forwardRef<HTMLAnchorElement, LinkProps>(
+ function Link(props, ref) {
+ return <ThemeUILink ref={ref} variant="default" {...props} />;
+ },
+);
+
+================================================================================
+`;
diff --git a/tests/format/typescript/last-argument-expansion/forward-ref.ts b/tests/format/typescript/last-argument-expansion/forward-ref.ts
new file mode 100644
--- /dev/null
+++ b/tests/format/typescript/last-argument-expansion/forward-ref.ts
@@ -0,0 +1,27 @@
+export const Link = forwardRef<HTMLAnchorElement, LinkProps>(
+ function Link(props, ref) {
+ return <ThemeUILink ref={ref} variant="default" {...props} />;
+ }
+);
+
+export const LinkWithLongName = forwardRef<HTMLAnchorElement, LinkProps>(
+ function Link(props, ref) {
+ return <ThemeUILink ref={ref} variant="default" {...props} />;
+ }
+);
+
+export const Arrow = forwardRef<HTMLAnchorElement, LinkProps>((props, ref) => {
+ return <ThemeUILink ref={ref} variant="default" {...props} />;
+});
+
+export const ArrowWithLongName = forwardRef<HTMLAnchorElement, LinkProps>(
+ (props, ref) => {
+ return <ThemeUILink ref={ref} variant="default" {...props} />;
+ }
+);
+
+const Link = React.forwardRef<HTMLAnchorElement, LinkProps>(
+ function Link(props, ref) {
+ return <ThemeUILink ref={ref} variant="default" {...props} />;
+ },
+);
| Improve React forwardRef with named function expression
**Prettier 2.4.1**
[Playground link](https://prettier.io/playground/#N4Igxg9gdgLgprEAuEBLAtgBwgJxgAmHwDNcB3AQxwBMAlOY-AXxJwnXwB0Qc4KwY3ANycoGbHnwwAnpjiF8AGVRQA1gAU2mAM74KugCoALOOjgBVAJLK1miDuat2XEDBNmAtAFdUw0eNwCIhtVPUN3C2sVUJZiNg5uN1M4b18QEShROAAPCQIZOSVouwcAXnwAeXRUGAAeY2SrEJLtABoXfW58AB8XI15ibgA+DKzcwPxIKG0CEPxy0hxKGnpiWoAJAwBZRQBBKDAjXABRABtk2HbmrW0hgApRfBIvA5hUaCK1O8wb9oGASkIjye+F4MC8OCg+HqESa0VBDFKwAGLAAblRUBRYKVuNQGBQvKdBCBCAA6ck-ezaFgAehGwKYon+oygOTyk2gM0+qgA6jUjIpoABzAByFDM8xI5CodAYG22ewORxwZwuMCuxRu92BxBeAneUJC31+COIgOAwKeYIhUJhjSialNSJR+HROEx2Nx+MJxLJFJutPpUOYTJZbImUy5uxwbDIksWy1la02O32hxO5zMl25LXuxqpfwYgNKQyBwetkOhDTMcMdA2dDDRGKxMBxIDxxAJRK6wHJpMpOkDGSYzNEY3ZkYI0djfLcgqgovF8gW0pWcpTivTKszCHVOa1D2D+Z0hbN81LFuDVrg4MrdprDtC9eRjddzc97e93ZJvf9VKHDKhqIICtCA9hvJyyCgFQsbqFQCDaMgIAUKclDSIhoEAEY4Pwqg3gAykuNhwMgnanNocBYThYB4TA+GYPwKhCsgMA4F4lEgKYmFwNQeLUIoWJCl4FBCnAABiuDoBQMBvAuSEEjAEAgSARgwOgpw8kYNRwNoDFgHA+EITUqCojU0hIWA2gYWg0xwHgmgiVJpEoRRoEAFbaNk+FMecACKXgQPAznkRxDE4BROBIZhFDcacHgwNZPwqDAfLUG4yAABwAAygZSFE8jhmBIT8Ol2aiJGgQAjgF8AtPJ2geKyPE8cpvDVagvAOUKTlIGRrkgBR1QsWxHHaD5cD+YFJG9S5HEwDFqXpUgABMoGsRQqCnExADC7A9Zx2gAKzKV4FEGDFiEzSFoGouxlhQHisD4WA7qYDA+zUPhMjnMF-UedkABC1G0YRZjEb9cBMEwQA)
```sh
# Options (if any):
--parser babel-ts
```
**Input:**
```jsx
export const Link = forwardRef<HTMLAnchorElement, LinkProps>(
function Link(props, ref) {
return <ThemeUILink ref={ref} variant="default" {...props} />;
}
);
```
**Output:**
```jsx
export const Link = forwardRef<HTMLAnchorElement, LinkProps>(function Link(
props,
ref
) {
return <ThemeUILink ref={ref} variant="default" {...props} />;
});
```
**Expected behavior:**
The input is much prettier than the output, and more readily reveals what the code is doing. In the output, it's very easy to miss the named function expression ([which is important because of React DevTools display names](https://reactjs.org/docs/forwarding-refs.html#displaying-a-custom-name-in-devtools)).
Compare to how Prettier formats these alternatives, which are all much better:
```jsx
export const LinkWithLongName = forwardRef<HTMLAnchorElement, LinkProps>(
function LinkWithLongName(props, ref) {
return <ThemeUILink ref={ref} variant="default" {...props} />;
}
);
export const Arrow = forwardRef<HTMLAnchorElement, LinkProps>((props, ref) => {
return <ThemeUILink ref={ref} variant="default" {...props} />;
});
export const ArrowWithLongName = forwardRef<HTMLAnchorElement, LinkProps>(
(props, ref) => {
return <ThemeUILink ref={ref} variant="default" {...props} />;
}
);
```
It also makes for some terrible diffs - renaming `Link` to `LinkWithLongName` actually _reduces_ total LOC:
```diff
-export const Link = forwardRef<HTMLAnchorElement, LinkProps>(function Link(
- props,
- ref
-) {
- return <ThemeLink ref={ref} variant="default" {...props} as={GatsbyLink} />;
-});
+export const LinkWithLongName = forwardRef<HTMLAnchorElement, LinkProps>(
+ function LinkWithLongName(props, ref) {
+ return <ThemeLink ref={ref} variant="default" {...props} as={GatsbyLink} />;
+ }
+);
```
| "2022-09-03T08:16:02Z" | 3.0 | [] | [
"tests/format/typescript/last-argument-expansion/jsfmt.spec.js"
] | JavaScript | [] | [
"https://prettier.io/playground/#N4Igxg9gdgLgprEAuEBLAtgBwgJxgAmHwDNcB3AQxwBMAlOY-AXxJwnXwB0Qc4KwY3ANycoGbHnwwAnpjiF8AGVRQA1gAU2mAM74KugCoALOOjgBVAJLK1miDuat2XEDBNmAtAFdUw0eNwCIhtVPUN3C2sVUJZiNg5uN1M4b18QEShROAAPCQIZOSVouwcAXnwAeXRUGAAeY2SrEJLtABoXfW58AB8XI15ibgA+DKzcwPxIKG0CEPxy0hxKGnpiWoAJAwBZRQBBKDAjXABRABtk2HbmrW0hgApRfBIvA5hUaCK1O8wb9oGASkIjye+F4MC8OCg+HqESa0VBDFKwAGLAAblRUBRYKVuNQGBQvKdBCBCAA6ck-ezaFgAehGwKYon+oygOTyk2gM0+qgA6jUjIpoABzAByFDM8xI5CodAYG22ewORxwZwuMCuxRu92BxBeAneUJC31+COIgOAwKeYIhUJhjSialNSJR+HROEx2Nx+MJxLJFJutPpUOYTJZbImUy5uxwbDIksWy1la02O32hxO5zMl25LXuxqpfwYgNKQyBwetkOhDTMcMdA2dDDRGKxMBxIDxxAJRK6wHJpMpOkDGSYzNEY3ZkYI0djfLcgqgovF8gW0pWcpTivTKszCHVOa1D2D+Z0hbN81LFuDVrg4MrdprDtC9eRjddzc97e93ZJvf9VKHDKhqIICtCA9hvJyyCgFQsbqFQCDaMgIAUKclDSIhoEAEY4Pwqg3gAykuNhwMgnanNocBYThYB4TA+GYPwKhCsgMA4F4lEgKYmFwNQeLUIoWJCl4FBCnAABiuDoBQMBvAuSEEjAEAgSARgwOgpw8kYNRwNoDFgHA+EITUqCojU0hIWA2gYWg0xwHgmgiVJpEoRRoEAFbaNk+FMecACKXgQPAznkRxDE4BROBIZhFDcacHgwNZPwqDAfLUG4yAABwAAygZSFE8jhmBIT8Ol2aiJGgQAjgF8AtPJ2geKyPE8cpvDVagvAOUKTlIGRrkgBR1QsWxHHaD5cD+YFJG9S5HEwDFqXpUgABMoGsRQqCnExADC7A9Zx2gAKzKV4FEGDFiEzSFoGouxlhQHisD4WA7qYDA+zUPhMjnMF-UedkABC1G0YRZjEb9cBMEwQA"
] |
|
prettier/prettier | 13,438 | prettier__prettier-13438 | [
"9959"
] | 5766433a62767bf3c9bc5b96aca4fd2495579c34 | diff --git a/src/language-js/print/comment.js b/src/language-js/print/comment.js
--- a/src/language-js/print/comment.js
+++ b/src/language-js/print/comment.js
@@ -1,4 +1,3 @@
-import { hasNewline } from "../../common/util.js";
import { join, hardline } from "../../document/builders.js";
import { replaceEndOfLine } from "../../document/utils.js";
@@ -18,19 +17,7 @@ function printComment(commentPath, options) {
if (isBlockComment(comment)) {
if (isIndentableBlockComment(comment)) {
- const printed = printIndentableBlockComment(comment);
- // We need to prevent an edge case of a previous trailing comment
- // printed as a `lineSuffix` which causes the comments to be
- // interleaved. See https://github.com/prettier/prettier/issues/4412
- if (
- comment.trailing &&
- !hasNewline(options.originalText, locStart(comment), {
- backwards: true,
- })
- ) {
- return [hardline, printed];
- }
- return printed;
+ return printIndentableBlockComment(comment);
}
const commentEnd = locEnd(comment);
diff --git a/src/main/comments.js b/src/main/comments.js
--- a/src/main/comments.js
+++ b/src/main/comments.js
@@ -461,14 +461,17 @@ function printLeadingComment(path, options) {
return parts;
}
-function printTrailingComment(path, options) {
+function printTrailingComment(path, options, previousComment) {
const comment = path.getValue();
const printed = printComment(path, options);
const { printer, originalText, locStart } = options;
- const isBlock = printer.isBlockComment && printer.isBlockComment(comment);
+ const isBlock = printer.isBlockComment?.(comment);
- if (hasNewline(originalText, locStart(comment), { backwards: true })) {
+ if (
+ (previousComment?.hasLineSuffix && !previousComment?.isBlock) ||
+ hasNewline(originalText, locStart(comment), { backwards: true })
+ ) {
// This allows comments at the end of nested structures:
// {
// x: 1,
@@ -487,17 +490,22 @@ function printTrailingComment(path, options) {
locStart
);
- return lineSuffix([hardline, isLineBeforeEmpty ? hardline : "", printed]);
+ return {
+ doc: lineSuffix([hardline, isLineBeforeEmpty ? hardline : "", printed]),
+ isBlock,
+ hasLineSuffix: true,
+ };
}
- let parts = [" ", printed];
-
- // Trailing block comments never need a newline
- if (!isBlock) {
- parts = [lineSuffix(parts), breakParent];
+ if (!isBlock || previousComment?.hasLineSuffix) {
+ return {
+ doc: [lineSuffix([" ", printed]), breakParent],
+ isBlock,
+ hasLineSuffix: true,
+ };
}
- return parts;
+ return { doc: [" ", printed], isBlock, hasLineSuffix: false };
}
function printDanglingComments(path, options, sameIndent, filter) {
@@ -544,6 +552,7 @@ function printCommentsSeparately(path, options, ignored) {
const leadingParts = [];
const trailingParts = [];
+ let printedTrailingComment;
path.each(() => {
const comment = path.getValue();
if (ignored && ignored.has(comment)) {
@@ -554,7 +563,12 @@ function printCommentsSeparately(path, options, ignored) {
if (leading) {
leadingParts.push(printLeadingComment(path, options));
} else if (trailing) {
- trailingParts.push(printTrailingComment(path, options));
+ printedTrailingComment = printTrailingComment(
+ path,
+ options,
+ printedTrailingComment
+ );
+ trailingParts.push(printedTrailingComment.doc);
}
}, "comments");
| diff --git a/tests/format/js/comments/__snapshots__/jsfmt.spec.js.snap b/tests/format/js/comments/__snapshots__/jsfmt.spec.js.snap
--- a/tests/format/js/comments/__snapshots__/jsfmt.spec.js.snap
+++ b/tests/format/js/comments/__snapshots__/jsfmt.spec.js.snap
@@ -1444,6 +1444,101 @@ const test = "💖";
================================================================================
`;
+exports[`empty-statements.js - {"semi":false} format 1`] = `
+====================================options=====================================
+parsers: ["babel", "flow", "typescript"]
+printWidth: 80
+semi: false
+ | printWidth
+=====================================input======================================
+a; /* a */ // b
+; /* c */
+
+foo; // first
+;// second
+;// third
+
+function x() {
+} // first
+; // second
+
+a = (
+ b // 1
+ + // 2
+ c // 3
+ + // 4
+ d // 5
+ + /* 6 */
+ e // 7
+);
+
+=====================================output=====================================
+a /* a */ // b
+/* c */
+foo // first
+// second
+// third
+function x() {} // first
+// second
+a =
+ b + // 1
+ // 2
+ c + // 3
+ // 4
+ d + // 5
+ /* 6 */
+ e // 7
+
+================================================================================
+`;
+
+exports[`empty-statements.js format 1`] = `
+====================================options=====================================
+parsers: ["babel", "flow", "typescript"]
+printWidth: 80
+ | printWidth
+=====================================input======================================
+a; /* a */ // b
+; /* c */
+
+foo; // first
+;// second
+;// third
+
+function x() {
+} // first
+; // second
+
+a = (
+ b // 1
+ + // 2
+ c // 3
+ + // 4
+ d // 5
+ + /* 6 */
+ e // 7
+);
+
+=====================================output=====================================
+a; /* a */ // b
+/* c */
+foo; // first
+// second
+// third
+function x() {} // first
+// second
+a =
+ b + // 1
+ // 2
+ c + // 3
+ // 4
+ d + // 5
+ /* 6 */
+ e; // 7
+
+================================================================================
+`;
+
exports[`export.js - {"semi":false} format 1`] = `
====================================options=====================================
parsers: ["babel", "flow", "typescript"]
@@ -3189,8 +3284,7 @@ onClick={() => {}}>
;<div>
{
- a
- /* comment
+ a /* comment
*/
}
</div>
@@ -3430,8 +3524,7 @@ onClick={() => {}}>
<div>
{
- a
- /* comment
+ a /* comment
*/
}
</div>;
@@ -3924,8 +4017,7 @@ a
b
a /*
-1*/ /*2*/
-/*3
+1*/ /*2*/ /*3
*/
b
@@ -4116,8 +4208,7 @@ a;
b;
a; /*
-1*/ /*2*/
-/*3
+1*/ /*2*/ /*3
*/
b;
@@ -5489,11 +5580,9 @@ const CONNECTION_STATUS = (exports.CONNECTION_STATUS = {
NOT_CONNECTED: Object.freeze({ kind: "NOT_CONNECTED" }),
})
-/* A comment */
-/**
+/* A comment */ /**
* A type that can be written to a buffer.
- */
-/**
+ */ /**
* Describes the connection status of a ReactiveSocket/DuplexConnection.
* - NOT_CONNECTED: no connection established or pending.
* - CONNECTING: when \`connect()\` has been called but a connection is not yet
@@ -5501,12 +5590,10 @@ const CONNECTION_STATUS = (exports.CONNECTION_STATUS = {
* - CONNECTED: when a connection is established.
* - CLOSED: when the connection has been explicitly closed via \`close()\`.
* - ERROR: when the connection has been closed for any other reason.
- */
-/**
+ */ /**
* A contract providing different interaction models per the [ReactiveSocket protocol]
* (https://github.com/ReactiveSocket/reactivesocket/blob/master/Protocol.md).
- */
-/**
+ */ /**
* A single unit of data exchanged between the peers of a \`ReactiveSocket\`.
*/
@@ -5550,11 +5637,9 @@ const CONNECTION_STATUS = (exports.CONNECTION_STATUS = {
NOT_CONNECTED: Object.freeze({ kind: "NOT_CONNECTED" }),
});
-/* A comment */
-/**
+/* A comment */ /**
* A type that can be written to a buffer.
- */
-/**
+ */ /**
* Describes the connection status of a ReactiveSocket/DuplexConnection.
* - NOT_CONNECTED: no connection established or pending.
* - CONNECTING: when \`connect()\` has been called but a connection is not yet
@@ -5562,12 +5647,10 @@ const CONNECTION_STATUS = (exports.CONNECTION_STATUS = {
* - CONNECTED: when a connection is established.
* - CLOSED: when the connection has been explicitly closed via \`close()\`.
* - ERROR: when the connection has been closed for any other reason.
- */
-/**
+ */ /**
* A contract providing different interaction models per the [ReactiveSocket protocol]
* (https://github.com/ReactiveSocket/reactivesocket/blob/master/Protocol.md).
- */
-/**
+ */ /**
* A single unit of data exchanged between the peers of a \`ReactiveSocket\`.
*/
diff --git a/tests/format/js/comments/empty-statements.js b/tests/format/js/comments/empty-statements.js
new file mode 100644
--- /dev/null
+++ b/tests/format/js/comments/empty-statements.js
@@ -0,0 +1,20 @@
+a; /* a */ // b
+; /* c */
+
+foo; // first
+;// second
+;// third
+
+function x() {
+} // first
+; // second
+
+a = (
+ b // 1
+ + // 2
+ c // 3
+ + // 4
+ d // 5
+ + /* 6 */
+ e // 7
+);
| Single line comments are merged on trailing trivia
**Prettier 2.1.2**
[Playground link](https://prettier.io/playground/#N4Igxg9gdgLgprEAuEAzArlMMCW0AEAHgBQCU+wAOlAL74D09+qOATgM4zUDcj+7cSFAAmIADQgIAB1zR2yUAENWrCAHcACsoTyUigDZrFAT3kSARq0VgA1nBgBlKdZxQA5shit0cCXAC25nDCwsEAMoru6IpucABiEKz+ijC47sggiugwEOIgABYw-voA6vk48OzOYHAOOhU4AG4Vxhlg7GYgrgKsMBpWbsnIqAYCEgBW7IQAQla29g6K-nBhrnDDo74gk4QOrm76cACK6BDwG-pjIM4ccKwZ5opB+nlSrK4wJTjCMPnIABwABgkbwgAhKVikGTecB6jXWEgAjqd4P1pLpMuwALRQODBYJ5VhwZFsOD9GJDJAjS5bAT+HCeby0-aHE5ndZUzYSGBPL4-P5IABM3KsOH0+wAwhB-JSQLCAKx5dACAAqT101KujR8AEkRAhHGB3jIAIIiBwwYyHC4CGg0IA)
```sh
--parser babel
```
**Input:**
```jsx
function x() {
} // first
; // second
```
**Output:**
```jsx
function x() {} // first // second
```
**Expected behavior:**
```jsx
function x() {} // first
// second
```
**Details:**
As you can see, the two comments (that should be on their own lines) are merged on a single line. I think this has to do with the `lineSuffix` command being used on two tokens on the same line. When that happens, both the suffixes are appended to the same line.
This is obviously very dangerous, and destroys the semantic meaning of the comment. Not only it makes it much harder to read and reason about (since comments cannot be broken), it also means that it will break any tools/parsers that read the comment contents and expect certain strings to be there/not there, like ignores and other directives.
| - | First | Second |
| --------------- | --------------- | --------------- |
| Before | ` first` | ` second` |
| After | ` first // second` | **removed** |
This does not just affect JavaScript, but it also affects all other languages, as they have no other means AFAICT of fixing this correctly using other available commands.
| @sosukesuzuki I tracked the issue to this piece of code:
https://github.com/prettier/prettier/blob/74d144989659f0a03922f91c28d1f109cf12a14a/src/document/doc-printer.js#L468-L470
Happy to work on a fix and add tests, but since I'm a first time contributor, some pointers would be appreciated. Can you please let me know:
* Is there a case where `lineSuffix` can be used multiple times on the same line? I cannot find an existing example in a language.
* If not, should an unconditional break always exist after line suffixes?
TIA!
~Actually, this is already fixed. Will post details.~ Sorry, it's not.
I was attempt to fix it in #9772, but didn't finish. #9736 was blocking it at that point.
> Is there a case where lineSuffix can be used multiple times on the same line?
The Ruby plugin uses `lineSuffix` to support heredoc strings (https://github.com/prettier/plugin-ruby/pull/681):
```ruby
a(b(c(<<~HERE, <<~DOC)))
this is the content of the first string
HERE
this is the content of the second string
DOC
```
@fisker thanks for pointing out.
Do you plan on rebasing/merging #9772 since #9736 is now merged?
Going over your fix, it seems that this will also fix it for other languages as well.
I'm a little busy recently, and seems #9772 breaking some cases.
You can work on it if you like.
@fisker pulled the fix down and started going through the code. IIUC, I don't believe we are talking about the same issue. Your PR handles **multiple multi-line comments that should be on the same line**. This issue describes **single line comments that should NOT be on the same line**.
Just ignore my PR. | "2022-09-06T22:25:15Z" | 3.0 | [] | [
"tests/format/js/comments/jsfmt.spec.js"
] | JavaScript | [] | [
"https://prettier.io/playground/#N4Igxg9gdgLgprEAuEAzArlMMCW0AEAHgBQCU+wAOlAL74D09+qOATgM4zUDcj+7cSFAAmIADQgIAB1zR2yUAENWrCAHcACsoTyUigDZrFAT3kSARq0VgA1nBgBlKdZxQA5shit0cCXAC25nDCwsEAMoru6IpucABiEKz+ijC47sggiugwEOIgABYw-voA6vk48OzOYHAOOhU4AG4Vxhlg7GYgrgKsMBpWbsnIqAYCEgBW7IQAQla29g6K-nBhrnDDo74gk4QOrm76cACK6BDwG-pjIM4ccKwZ5opB+nlSrK4wJTjCMPnIABwABgkbwgAhKVikGTecB6jXWEgAjqd4P1pLpMuwALRQODBYJ5VhwZFsOD9GJDJAjS5bAT+HCeby0-aHE5ndZUzYSGBPL4-P5IABM3KsOH0+wAwhB-JSQLCAKx5dACAAqT101KujR8AEkRAhHGB3jIAIIiBwwYyHC4CGg0IA"
] |
prettier/prettier | 13,445 | prettier__prettier-13445 | [
"12653"
] | 2dc687c613bb40872922bd111f43a8cbe0cee56f | diff --git a/.eslintrc.cjs b/.eslintrc.cjs
--- a/.eslintrc.cjs
+++ b/.eslintrc.cjs
@@ -321,6 +321,7 @@ module.exports = {
},
{
files: ["src/language-js/**/*.js"],
+ excludedFiles: ["src/language-js/parse/postprocess/*.js"],
rules: {
"prettier-internal-rules/no-node-comments": [
"error",
@@ -329,7 +330,6 @@ module.exports = {
functions: ["hasComment", "getComments"],
},
"src/language-js/pragma.js",
- "src/language-js/parse/postprocess/*.js",
"src/language-js/parse/babel.js",
"src/language-js/parse/meriyah.js",
"src/language-js/parse/json.js",
diff --git a/src/language-js/parse/postprocess/index.js b/src/language-js/parse/postprocess/index.js
--- a/src/language-js/parse/postprocess/index.js
+++ b/src/language-js/parse/postprocess/index.js
@@ -2,6 +2,9 @@ import { locStart, locEnd } from "../../loc.js";
import isTsKeywordType from "../../utils/is-ts-keyword-type.js";
import isTypeCastComment from "../../utils/is-type-cast-comment.js";
import getLast from "../../../utils/get-last.js";
+import isNonEmptyArray from "../../../utils/is-non-empty-array.js";
+import isBlockComment from "../../utils/is-block-comment.js";
+import isIndentableBlockComment from "../../utils/is-indentable-block-comment.js";
import visitNode from "./visit-node.js";
import { throwErrorForInvalidNodes } from "./typescript.js";
import throwSyntaxError from "./throw-ts-syntax-error.js";
@@ -154,6 +157,25 @@ function postprocess(ast, options) {
}
});
+ if (isNonEmptyArray(ast.comments)) {
+ let followingComment = getLast(ast.comments);
+ for (let i = ast.comments.length - 2; i >= 0; i--) {
+ const comment = ast.comments[i];
+ if (
+ locEnd(comment) === locStart(followingComment) &&
+ isBlockComment(comment) &&
+ isBlockComment(followingComment) &&
+ isIndentableBlockComment(comment) &&
+ isIndentableBlockComment(followingComment)
+ ) {
+ ast.comments.splice(i + 1, 1);
+ comment.value += "*//*" + followingComment.value;
+ comment.range = [locStart(comment), locEnd(followingComment)];
+ }
+ followingComment = comment;
+ }
+ }
+
return ast;
/**
diff --git a/src/language-js/print/comment.js b/src/language-js/print/comment.js
--- a/src/language-js/print/comment.js
+++ b/src/language-js/print/comment.js
@@ -4,6 +4,7 @@ import { replaceEndOfLine } from "../../document/utils.js";
import { isLineComment } from "../utils/index.js";
import { locStart, locEnd } from "../loc.js";
import isBlockComment from "../utils/is-block-comment.js";
+import isIndentableBlockComment from "../utils/is-indentable-block-comment.js";
function printComment(commentPath, options) {
const comment = commentPath.getValue();
@@ -34,15 +35,6 @@ function printComment(commentPath, options) {
throw new Error("Not a comment: " + JSON.stringify(comment));
}
-function isIndentableBlockComment(comment) {
- // If the comment has multiple lines and every line starts with a star
- // we can fix the indentation of each line. The stars in the `/*` and
- // `*/` delimiters are not included in the comment value, so add them
- // back first.
- const lines = `*${comment.value}*`.split("\n");
- return lines.length > 1 && lines.every((line) => line.trim()[0] === "*");
-}
-
function printIndentableBlockComment(comment) {
const lines = comment.value.split("\n");
@@ -60,4 +52,4 @@ function printIndentableBlockComment(comment) {
];
}
-export { printComment };
+export { printComment, isIndentableBlockComment };
diff --git a/src/language-js/utils/is-indentable-block-comment.js b/src/language-js/utils/is-indentable-block-comment.js
new file mode 100644
--- /dev/null
+++ b/src/language-js/utils/is-indentable-block-comment.js
@@ -0,0 +1,10 @@
+function isIndentableBlockComment(comment) {
+ // If the comment has multiple lines and every line starts with a star
+ // we can fix the indentation of each line. The stars in the `/*` and
+ // `*/` delimiters are not included in the comment value, so add them
+ // back first.
+ const lines = `*${comment.value}*`.split("\n");
+ return lines.length > 1 && lines.every((line) => line.trimStart()[0] === "*");
+}
+
+export default isIndentableBlockComment;
| diff --git a/tests/format/js/comments/__snapshots__/jsfmt.spec.js.snap b/tests/format/js/comments/__snapshots__/jsfmt.spec.js.snap
--- a/tests/format/js/comments/__snapshots__/jsfmt.spec.js.snap
+++ b/tests/format/js/comments/__snapshots__/jsfmt.spec.js.snap
@@ -3144,6 +3144,230 @@ function HelloWorld() {
================================================================================
`;
+exports[`jsdoc-nestled.js - {"semi":false} format 1`] = `
+====================================options=====================================
+parsers: ["babel", "flow", "typescript"]
+printWidth: 80
+semi: false
+ | printWidth
+=====================================input======================================
+const issues = {
+ see: "#7724 and #12653"
+ /** Trailing comment 1 (not nestled as both comments should be multiline for that) *//**
+ * Trailing comment 2
+ */
+};
+
+/**
+ * @template T
+ * @param {Type} type
+ * @param {T} value
+ * @return {Value}
+ *//**
+ * @param {Type} type
+ * @return {Value}
+ */
+function value(type, value) {
+ if (arguments.length === 2) {
+ return new ConcreteValue(type, value);
+ } else {
+ return new Value(type);
+ }
+}
+
+/** Trailing nestled comment 1
+ *//** Trailing nestled comment 2
+ *//** Trailing nestled comment 3
+ */
+
+=====================================output=====================================
+const issues = {
+ see: "#7724 and #12653",
+ /** Trailing comment 1 (not nestled as both comments should be multiline for that) */ /**
+ * Trailing comment 2
+ */
+}
+
+/**
+ * @template T
+ * @param {Type} type
+ * @param {T} value
+ * @return {Value}
+ *//**
+ * @param {Type} type
+ * @return {Value}
+ */
+function value(type, value) {
+ if (arguments.length === 2) {
+ return new ConcreteValue(type, value)
+ } else {
+ return new Value(type)
+ }
+}
+
+/** Trailing nestled comment 1
+ *//** Trailing nestled comment 2
+ *//** Trailing nestled comment 3
+ */
+
+================================================================================
+`;
+
+exports[`jsdoc-nestled.js format 1`] = `
+====================================options=====================================
+parsers: ["babel", "flow", "typescript"]
+printWidth: 80
+ | printWidth
+=====================================input======================================
+const issues = {
+ see: "#7724 and #12653"
+ /** Trailing comment 1 (not nestled as both comments should be multiline for that) *//**
+ * Trailing comment 2
+ */
+};
+
+/**
+ * @template T
+ * @param {Type} type
+ * @param {T} value
+ * @return {Value}
+ *//**
+ * @param {Type} type
+ * @return {Value}
+ */
+function value(type, value) {
+ if (arguments.length === 2) {
+ return new ConcreteValue(type, value);
+ } else {
+ return new Value(type);
+ }
+}
+
+/** Trailing nestled comment 1
+ *//** Trailing nestled comment 2
+ *//** Trailing nestled comment 3
+ */
+
+=====================================output=====================================
+const issues = {
+ see: "#7724 and #12653",
+ /** Trailing comment 1 (not nestled as both comments should be multiline for that) */ /**
+ * Trailing comment 2
+ */
+};
+
+/**
+ * @template T
+ * @param {Type} type
+ * @param {T} value
+ * @return {Value}
+ *//**
+ * @param {Type} type
+ * @return {Value}
+ */
+function value(type, value) {
+ if (arguments.length === 2) {
+ return new ConcreteValue(type, value);
+ } else {
+ return new Value(type);
+ }
+}
+
+/** Trailing nestled comment 1
+ *//** Trailing nestled comment 2
+ *//** Trailing nestled comment 3
+ */
+
+================================================================================
+`;
+
+exports[`jsdoc-nestled-dangling.js - {"semi":false} format 1`] = `
+====================================options=====================================
+parsers: ["babel", "flow", "typescript"]
+printWidth: 80
+semi: false
+ | printWidth
+=====================================input======================================
+{{{{{{{
+o={
+ /**
+ * A
+ *//**
+ * B
+ */
+
+}
+}}}}}}}
+
+=====================================output=====================================
+{
+ {
+ {
+ {
+ {
+ {
+ {
+ o = {
+ /**
+ * A
+ *//**
+ * B
+ */
+ }
+ }
+ }
+ }
+ }
+ }
+ }
+}
+
+================================================================================
+`;
+
+exports[`jsdoc-nestled-dangling.js format 1`] = `
+====================================options=====================================
+parsers: ["babel", "flow", "typescript"]
+printWidth: 80
+ | printWidth
+=====================================input======================================
+{{{{{{{
+o={
+ /**
+ * A
+ *//**
+ * B
+ */
+
+}
+}}}}}}}
+
+=====================================output=====================================
+{
+ {
+ {
+ {
+ {
+ {
+ {
+ o = {
+ /**
+ * A
+ *//**
+ * B
+ */
+ };
+ }
+ }
+ }
+ }
+ }
+ }
+}
+
+================================================================================
+`;
+
exports[`jsx.js - {"semi":false} format 1`] = `
====================================options=====================================
parsers: ["babel", "flow", "typescript"]
diff --git a/tests/format/js/comments/jsdoc-nestled-dangling.js b/tests/format/js/comments/jsdoc-nestled-dangling.js
new file mode 100644
--- /dev/null
+++ b/tests/format/js/comments/jsdoc-nestled-dangling.js
@@ -0,0 +1,10 @@
+{{{{{{{
+o={
+ /**
+ * A
+ *//**
+ * B
+ */
+
+}
+}}}}}}}
diff --git a/tests/format/js/comments/issue-7724.js b/tests/format/js/comments/jsdoc-nestled.js
similarity index 50%
rename from tests/format/js/comments/issue-7724.js
rename to tests/format/js/comments/jsdoc-nestled.js
--- a/tests/format/js/comments/issue-7724.js
+++ b/tests/format/js/comments/jsdoc-nestled.js
@@ -1,4 +1,9 @@
-const foo = "Bar";
+const issues = {
+ see: "#7724 and #12653"
+ /** Trailing comment 1 (not nestled as both comments should be multiline for that) *//**
+ * Trailing comment 2
+ */
+};
/**
* @template T
@@ -15,4 +20,9 @@ function value(type, value) {
} else {
return new Value(type);
}
-}
\ No newline at end of file
+}
+
+/** Trailing nestled comment 1
+ *//** Trailing nestled comment 2
+ *//** Trailing nestled comment 3
+ */
| Nestled JSDoc comments are still broken after #9672
**Prettier 2.6.2**
[Playground link](https://prettier.io/playground/#N4Igxg9gdgLgprEAuc0DOMAEAzCFMC8mAOiAEICGATqQNzFQMD0AVCw5i5gALwC2ABwA2FeJgAqHLtwHUKfTMHEBPAXAC+mGKrhSesqvMXjNANwpCArrqiceVODEtVbwAGoXr6qU1btb0gZGSjqa2mp63A5OLooeVho+DNiWUGAwAJbQmOYJABThcAA0OZ5wAJSKHJgZ2Jh51ADmlnwIMGgAdEIIjTAAFoQERABMlcDVmJjRzrZQcADumADC0GDRcPHWBToludbl9LaYmnBCaHBVR5PTsXOLm3DbagfV3lBvIEUgEAKZ6MigahUCDzAAK1AQaGQIAs8woyihXwARoYwABrRwAZXkcAAMhk5shsBZzsjURiYJjZGACY1kDAqNYvnA+Ei4AATdkc3EUKDNCiNOAAMQgVD4okyfOhFEsMAgnxAfRgfCEAHU+hl4GhqXBMZDNRlTJrlNCwGhESACecqDBQYZGuKiSTiiAAFZoAAemNp3QAipYIPAnWcXQZrdCkRQ2UIFQIqASYKqMuz+sgABwABi+cYg51VhgE0LjcGtpjgCoAjgH4HaflCUBQ0ABaOYcjkKhxVjIOO0Cx1IYkhr7nPgZemMl1oH1wf2B8sD51fGBRpMpvrIYZLwwZIS0lZ8fsgEsAVgVlnO4ij9cHpJApmsAEkoFzYJi1hlfgBBZ+Y7TdYPnOo6hAA)
<!-- prettier-ignore -->
```sh
--parser babel
```
**Input:**
<!-- prettier-ignore -->
```jsx
const foo = "Bar";
/**
* @template T
* @param {Type} type
* @param {T} value
* @return {Value}
*//**
* @param {Type} type
* @return {Value}
*/
function value(type, value) {
if (arguments.length === 2) {
return new ConcreteValue(type, value);
} else {
return new Value(type);
}
}
```
**Output:**
<!-- prettier-ignore -->
```jsx
const foo = "Bar";
/**
* @template T
* @param {Type} type
* @param {T} value
* @return {Value}
*/ /**
* @param {Type} type
* @return {Value}
*/
function value(type, value) {
if (arguments.length === 2) {
return new ConcreteValue(type, value);
} else {
return new Value(type);
}
}
```
**Expected behavior:**
As reported in #7724, JSDoc function overloads require two comment blocks "nestled" together. When that issue was reported, "nestled" comments would have a blank line inserted between them, breaking the JSDoc parser. That issue was closed via #9672. Now, instead of inserting a blank line, Prettier inserts a single space, turning `*//**` into `*/ /**`. This still breaks the JSDoc parser. The sequence must be exactly `*//**`, and it must not be modified by Prettier at all.
/cc @fisker, who wrote the linked PR, and @grassator who reported the previous issue.
| "2022-09-08T08:23:51Z" | 3.0 | [] | [
"tests/format/js/comments/jsfmt.spec.js"
] | JavaScript | [] | [
"https://prettier.io/playground/#N4Igxg9gdgLgprEAuc0DOMAEAzCFMC8mAOiAEICGATqQNzFQMD0AVCw5i5gALwC2ABwA2FeJgAqHLtwHUKfTMHEBPAXAC+mGKrhSesqvMXjNANwpCArrqiceVODEtVbwAGoXr6qU1btb0gZGSjqa2mp63A5OLooeVho+DNiWUGAwAJbQmOYJABThcAA0OZ5wAJSKHJgZ2Jh51ADmlnwIMGgAdEIIjTAAFoQERABMlcDVmJjRzrZQcADumADC0GDRcPHWBToludbl9LaYmnBCaHBVR5PTsXOLm3DbagfV3lBvIEUgEAKZ6MigahUCDzAAK1AQaGQIAs8woyihXwARoYwABrRwAZXkcAAMhk5shsBZzsjURiYJjZGACY1kDAqNYvnA+Ei4AATdkc3EUKDNCiNOAAMQgVD4okyfOhFEsMAgnxAfRgfCEAHU+hl4GhqXBMZDNRlTJrlNCwGhESACecqDBQYZGuKiSTiiAAFZoAAemNp3QAipYIPAnWcXQZrdCkRQ2UIFQIqASYKqMuz+sgABwABi+cYg51VhgE0LjcGtpjgCoAjgH4HaflCUBQ0ABaOYcjkKhxVjIOO0Cx1IYkhr7nPgZemMl1oH1wf2B8sD51fGBRpMpvrIYZLwwZIS0lZ8fsgEsAVgVlnO4ij9cHpJApmsAEkoFzYJi1hlfgBBZ+Y7TdYPnOo6hAA"
] |
|
prettier/prettier | 13,487 | prettier__prettier-13487 | [
"12889"
] | 5107a55d6dbe6113505fabfab201acbae4055ebf | diff --git a/src/language-css/printer-postcss.js b/src/language-css/printer-postcss.js
--- a/src/language-css/printer-postcss.js
+++ b/src/language-css/printer-postcss.js
@@ -621,6 +621,7 @@ function genericPrint(path, options, print) {
// Ignore escape `\`
if (
+ iNode.type !== "value-string" &&
iNode.value &&
iNode.value.includes("\\") &&
iNextNode &&
| diff --git a/tests/format/scss/quotes/__snapshots__/jsfmt.spec.js.snap b/tests/format/scss/quotes/__snapshots__/jsfmt.spec.js.snap
--- a/tests/format/scss/quotes/__snapshots__/jsfmt.spec.js.snap
+++ b/tests/format/scss/quotes/__snapshots__/jsfmt.spec.js.snap
@@ -1,5 +1,38 @@
// Jest Snapshot v1, https://goo.gl/fbAQLP
+exports[`escape-in-string.scss - {"singleQuote":true} format 1`] = `
+====================================options=====================================
+parsers: ["scss"]
+printWidth: 80
+singleQuote: true
+ | printWidth
+=====================================input======================================
+$description: "Lorem ipsum dolor sit \\"amet\\", consectetur adipiscing elit, " +
+ "sed do eiusmod tempor incididunt ut labore et dolore magna aliqua.";
+
+=====================================output=====================================
+$description: 'Lorem ipsum dolor sit "amet", consectetur adipiscing elit, ' +
+ 'sed do eiusmod tempor incididunt ut labore et dolore magna aliqua.';
+
+================================================================================
+`;
+
+exports[`escape-in-string.scss format 1`] = `
+====================================options=====================================
+parsers: ["scss"]
+printWidth: 80
+ | printWidth
+=====================================input======================================
+$description: "Lorem ipsum dolor sit \\"amet\\", consectetur adipiscing elit, " +
+ "sed do eiusmod tempor incididunt ut labore et dolore magna aliqua.";
+
+=====================================output=====================================
+$description: 'Lorem ipsum dolor sit "amet", consectetur adipiscing elit, ' +
+ "sed do eiusmod tempor incididunt ut labore et dolore magna aliqua.";
+
+================================================================================
+`;
+
exports[`forward-with.scss - {"singleQuote":true} format 1`] = `
====================================options=====================================
parsers: ["scss"]
diff --git a/tests/format/scss/quotes/escape-in-string.scss b/tests/format/scss/quotes/escape-in-string.scss
new file mode 100644
--- /dev/null
+++ b/tests/format/scss/quotes/escape-in-string.scss
@@ -0,0 +1,2 @@
+$description: "Lorem ipsum dolor sit \"amet\", consectetur adipiscing elit, " +
+ "sed do eiusmod tempor incididunt ut labore et dolore magna aliqua.";
| SCSS: Prettier doesn't wrap multiline strings with escaped characters
**Prettier 2.6.2**
[Playground link](https://prettier.io/playground/#N4Igxg9gdgLgprEAuEASAJnAzmATgSwAcZ9okACAHRABkJc4BbcorAV2fQgBt7yt8MKpWoBDRnBgjqAGnKQoWOGHgw2ucqPRF8OfFADm5ON0Fzq5ANSUo5KiCXpyXY-jZZGEJ-EaE++sHxtdDZYcjYhblEAI3o4YyEuXgZyRlEDKFFNUwBHNlEAOmoAbhsQGRAIYlJFZFBRXFwIAHcABQaELGQQUW5m0QBPLoro3FEwAGtJAGVxOBp9OGQAM16lEbHJmcJx-QNkGFw2OAqmaLh0THQaUUN8gzgAMXo0mBJDbtEIiHKQAAsYIxuAB1P6CbA7MBwaadQT4ABuggG3TAWGGIH0SlwMFaYwMaRWaxOIAAVlgAB7TPbcOAARTYEHghO46xAO1wWO6ODRv0IBFgwKCMD+yAAHAAGCp8iBKYFjQjdPnYOC4eFLCp5RlwXFVLooURYAC0UDgFwuvwYeXwDFx6QJSFWLOJSkY+AOR2d1LpDKZDqJFRgMUF6GFyAATAGxvhTIYAMIQRj2kDYACsv3ccAAKjE9Y7WfDjgBJKCYWDTPBEGAAQRL0xgAxpzKUAF9m0A)
<!-- prettier-ignore -->
```sh
--parser scss
```
**Input:**
<!-- prettier-ignore -->
```scss
$description: "Lorem ipsum dolor sit \"amet\", consectetur adipiscing elit, " +
"sed do eiusmod tempor incididunt ut labore et dolore magna aliqua.";
```
**Output:**
<!-- prettier-ignore -->
```scss
$description: 'Lorem ipsum dolor sit "amet", consectetur adipiscing elit, '+ "sed do eiusmod tempor incididunt ut labore et dolore magna aliqua.";
```
**Expected behavior:**
<!-- prettier-ignore -->
```scss
$description: 'Lorem ipsum dolor sit "amet", consectetur adipiscing elit, ' +
"sed do eiusmod tempor incididunt ut labore et dolore magna aliqua.";
```
| "2022-09-17T20:40:32Z" | 3.0 | [] | [
"tests/format/scss/quotes/jsfmt.spec.js"
] | JavaScript | [] | [
"https://prettier.io/playground/#N4Igxg9gdgLgprEAuEASAJnAzmATgSwAcZ9okACAHRABkJc4BbcorAV2fQgBt7yt8MKpWoBDRnBgjqAGnKQoWOGHgw2ucqPRF8OfFADm5ON0Fzq5ANSUo5KiCXpyXY-jZZGEJ-EaE++sHxtdDZYcjYhblEAI3o4YyEuXgZyRlEDKFFNUwBHNlEAOmoAbhsQGRAIYlJFZFBRXFwIAHcABQaELGQQUW5m0QBPLoro3FEwAGtJAGVxOBp9OGQAM16lEbHJmcJx-QNkGFw2OAqmaLh0THQaUUN8gzgAMXo0mBJDbtEIiHKQAAsYIxuAB1P6CbA7MBwaadQT4ABuggG3TAWGGIH0SlwMFaYwMaRWaxOIAAVlgAB7TPbcOAARTYEHghO46xAO1wWO6ODRv0IBFgwKCMD+yAAHAAGCp8iBKYFjQjdPnYOC4eFLCp5RlwXFVLooURYAC0UDgFwuvwYeXwDFx6QJSFWLOJSkY+AOR2d1LpDKZDqJFRgMUF6GFyAATAGxvhTIYAMIQRj2kDYACsv3ccAAKjE9Y7WfDjgBJKCYWDTPBEGAAQRL0xgAxpzKUAF9m0A"
] |
|
prettier/prettier | 13,608 | prettier__prettier-13608 | [
"13588"
] | f4f36b94ec1a0133005c736585002f5de8be938f | diff --git a/src/language-js/print/array.js b/src/language-js/print/array.js
--- a/src/language-js/print/array.js
+++ b/src/language-js/print/array.js
@@ -23,6 +23,19 @@ import { printOptionalToken, printTypeAnnotation } from "./misc.js";
/** @typedef {import("../../document/builders.js").Doc} Doc */
+function printEmptyArray(path, openBracket, closeBracket, options) {
+ const { node } = path;
+ if (!hasComment(node, CommentCheckFlags.Dangling)) {
+ return [openBracket, closeBracket];
+ }
+ return group([
+ openBracket,
+ printDanglingComments(path, options),
+ softline,
+ closeBracket,
+ ]);
+}
+
function printArray(path, options, print) {
const { node } = path;
/** @type{Doc[]} */
@@ -31,18 +44,7 @@ function printArray(path, options, print) {
const openBracket = node.type === "TupleExpression" ? "#[" : "[";
const closeBracket = "]";
if (node.elements.length === 0) {
- if (!hasComment(node, CommentCheckFlags.Dangling)) {
- parts.push(openBracket, closeBracket);
- } else {
- parts.push(
- group([
- openBracket,
- printDanglingComments(path, options),
- softline,
- closeBracket,
- ])
- );
- }
+ parts.push(printEmptyArray(path, openBracket, closeBracket, options));
} else {
const lastElem = node.elements.at(-1);
const canHaveTrailingComma = !(lastElem && lastElem.type === "RestElement");
@@ -187,4 +189,9 @@ function printArrayItemsConcisely(path, options, print, trailingComma) {
return fill(parts);
}
-export { printArray, printArrayItems, isConciselyPrintedArray };
+export {
+ printArray,
+ printArrayItems,
+ isConciselyPrintedArray,
+ printEmptyArray,
+};
diff --git a/src/language-js/print/type-annotation.js b/src/language-js/print/type-annotation.js
--- a/src/language-js/print/type-annotation.js
+++ b/src/language-js/print/type-annotation.js
@@ -1,5 +1,4 @@
-import { printComments, printDanglingComments } from "../../main/comments.js";
-import { isNonEmptyArray } from "../../common/util.js";
+import { printComments } from "../../main/comments.js";
import {
group,
join,
@@ -23,7 +22,7 @@ import {
printFunctionParameters,
shouldGroupFunctionParameters,
} from "./function-parameters.js";
-import { printArrayItems } from "./array.js";
+import { printArrayItems, printEmptyArray } from "./array.js";
function shouldHugType(node) {
if (isSimpleType(node) || isObjectType(node)) {
@@ -289,18 +288,18 @@ function printTupleType(path, options, print) {
const { node } = path;
const typesField = node.type === "TSTupleType" ? "elementTypes" : "types";
const types = node[typesField];
- const isNonEmptyTuple = isNonEmptyArray(types);
- const bracketsDelimiterLine = isNonEmptyTuple ? softline : "";
+ const isEmptyTuple = types.length === 0;
+ const openBracket = "[";
+ const closeBracket = "]";
+ if (isEmptyTuple) {
+ return printEmptyArray(path, openBracket, closeBracket, options);
+ }
return group([
- "[",
- indent([
- bracketsDelimiterLine,
- printArrayItems(path, options, typesField, print),
- ]),
- ifBreak(isNonEmptyTuple && shouldPrintComma(options, "all") ? "," : ""),
- printDanglingComments(path, options, /* sameIndent */ true),
- bracketsDelimiterLine,
- "]",
+ openBracket,
+ indent([softline, printArrayItems(path, options, typesField, print)]),
+ ifBreak(shouldPrintComma(options, "all") ? "," : ""),
+ softline,
+ closeBracket,
]);
}
| diff --git a/tests/format/typescript/tuple/__snapshots__/jsfmt.spec.js.snap b/tests/format/typescript/tuple/__snapshots__/jsfmt.spec.js.snap
--- a/tests/format/typescript/tuple/__snapshots__/jsfmt.spec.js.snap
+++ b/tests/format/typescript/tuple/__snapshots__/jsfmt.spec.js.snap
@@ -1,5 +1,203 @@
// Jest Snapshot v1, https://goo.gl/fbAQLP
+exports[`dangling-comments.ts - {"trailingComma":"all"} format 1`] = `
+====================================options=====================================
+parsers: ["typescript"]
+printWidth: 80
+trailingComma: "all"
+ | printWidth
+=====================================input======================================
+type Foo1 = [/* comment */];
+
+type Foo2 = [
+ // comment
+];
+
+type Foo3 = [
+ // comment1
+ // comment2
+];
+
+type Foo4 = [
+ // comment1
+
+ // comment2
+];
+
+type Foo5 = [
+ /* comment1 */
+];
+
+type Foo6 = [
+ /* comment1 */
+
+ /* comment2 */
+];
+
+
+=====================================output=====================================
+type Foo1 = [
+ /* comment */
+];
+
+type Foo2 = [
+ // comment
+];
+
+type Foo3 = [
+ // comment1
+ // comment2
+];
+
+type Foo4 = [
+ // comment1
+ // comment2
+];
+
+type Foo5 = [
+ /* comment1 */
+];
+
+type Foo6 = [
+ /* comment1 */
+ /* comment2 */
+];
+
+================================================================================
+`;
+
+exports[`dangling-comments.ts - {"trailingComma":"es5"} format 1`] = `
+====================================options=====================================
+parsers: ["typescript"]
+printWidth: 80
+trailingComma: "es5"
+ | printWidth
+=====================================input======================================
+type Foo1 = [/* comment */];
+
+type Foo2 = [
+ // comment
+];
+
+type Foo3 = [
+ // comment1
+ // comment2
+];
+
+type Foo4 = [
+ // comment1
+
+ // comment2
+];
+
+type Foo5 = [
+ /* comment1 */
+];
+
+type Foo6 = [
+ /* comment1 */
+
+ /* comment2 */
+];
+
+
+=====================================output=====================================
+type Foo1 = [
+ /* comment */
+];
+
+type Foo2 = [
+ // comment
+];
+
+type Foo3 = [
+ // comment1
+ // comment2
+];
+
+type Foo4 = [
+ // comment1
+ // comment2
+];
+
+type Foo5 = [
+ /* comment1 */
+];
+
+type Foo6 = [
+ /* comment1 */
+ /* comment2 */
+];
+
+================================================================================
+`;
+
+exports[`dangling-comments.ts - {"trailingComma":"none"} format 1`] = `
+====================================options=====================================
+parsers: ["typescript"]
+printWidth: 80
+trailingComma: "none"
+ | printWidth
+=====================================input======================================
+type Foo1 = [/* comment */];
+
+type Foo2 = [
+ // comment
+];
+
+type Foo3 = [
+ // comment1
+ // comment2
+];
+
+type Foo4 = [
+ // comment1
+
+ // comment2
+];
+
+type Foo5 = [
+ /* comment1 */
+];
+
+type Foo6 = [
+ /* comment1 */
+
+ /* comment2 */
+];
+
+
+=====================================output=====================================
+type Foo1 = [
+ /* comment */
+];
+
+type Foo2 = [
+ // comment
+];
+
+type Foo3 = [
+ // comment1
+ // comment2
+];
+
+type Foo4 = [
+ // comment1
+ // comment2
+];
+
+type Foo5 = [
+ /* comment1 */
+];
+
+type Foo6 = [
+ /* comment1 */
+ /* comment2 */
+];
+
+================================================================================
+`;
+
exports[`trailing-comma.ts - {"trailingComma":"all"} format 1`] = `
====================================options=====================================
parsers: ["typescript"]
diff --git a/tests/format/typescript/tuple/dangling-comments.ts b/tests/format/typescript/tuple/dangling-comments.ts
new file mode 100644
--- /dev/null
+++ b/tests/format/typescript/tuple/dangling-comments.ts
@@ -0,0 +1,27 @@
+type Foo1 = [/* comment */];
+
+type Foo2 = [
+ // comment
+];
+
+type Foo3 = [
+ // comment1
+ // comment2
+];
+
+type Foo4 = [
+ // comment1
+
+ // comment2
+];
+
+type Foo5 = [
+ /* comment1 */
+];
+
+type Foo6 = [
+ /* comment1 */
+
+ /* comment2 */
+];
+
| Comment in empty array type breaks code
**Prettier 2.7.1**
[Playground link](https://prettier.io/playground/#N4Igxg9gdgLgprEAuEBLWcBOAzAhmOAAgA1DgAdKQwgDyUIG1LrqB6V25wgXQG5KAviAA0ICAAcYqaAGdkoXJkwQA7gAVFCOSlwAbFbgCec0QCNM+ANZwYAZVwBbOABl0cZHl0y4Zi2Gt24vjoAObIMJgArj4gcA6mcAAmiUnOuFAhkbghcABiEJgOuDBSGcgguJEwECIgABYwDroA6nWo8DJBBLZa7agAbu2G5WAyJmhQ3pgwahYhRR563qIAVjI0tqG6cACKkRDwi14xQZhT5TCG4nAyYJiokrXi97DNqIkwdcgAHAAMos8IN5mhZxOVnjcsP13KIAI77eCzCTaCoyAC0UDgSSStUwcHhqDxs2yCyQnmWIG8DlQ4SiMRkW12CPcZKWMRguFMbw+XyQACZRBFcKhdKEAMIQByk2IyACstUi3gAKpztOSYv1ogBJKApWC2O4PGAAQV1tku2yO3gEAiAA)
<!-- prettier-ignore -->
```sh
--parser typescript
```
**Input:**
<!-- prettier-ignore -->
```tsx
interface X {
x: [
// x
];
}
```
**Output:**
<!-- prettier-ignore -->
```tsx
interface X {
x: [// x];
}
```
The code is broken and no longer parsable as correct typescript. This happens both with "typescript" and "babel-ts" parsers.
**Expected behavior:**
Output should stay the same as input.
This is similar to https://github.com/prettier/prettier/issues/9299 but there it breaks only if there's a colon before the comment but with typescript it breaks any empty array type.
| Related comment about refactoring of dangling comments: https://github.com/prettier/prettier/pull/13445#issuecomment-1240723341 | "2022-10-10T08:54:39Z" | 3.0 | [] | [
"tests/format/typescript/tuple/jsfmt.spec.js"
] | JavaScript | [] | [
"https://prettier.io/playground/#N4Igxg9gdgLgprEAuEBLWcBOAzAhmOAAgA1DgAdKQwgDyUIG1LrqB6V25wgXQG5KAviAA0ICAAcYqaAGdkoXJkwQA7gAVFCOSlwAbFbgCec0QCNM+ANZwYAZVwBbOABl0cZHl0y4Zi2Gt24vjoAObIMJgArj4gcA6mcAAmiUnOuFAhkbghcABiEJgOuDBSGcgguJEwECIgABYwDroA6nWo8DJBBLZa7agAbu2G5WAyJmhQ3pgwahYhRR563qIAVjI0tqG6cACKkRDwi14xQZhT5TCG4nAyYJiokrXi97DNqIkwdcgAHAAMos8IN5mhZxOVnjcsP13KIAI77eCzCTaCoyAC0UDgSSStUwcHhqDxs2yCyQnmWIG8DlQ4SiMRkW12CPcZKWMRguFMbw+XyQACZRBFcKhdKEAMIQByk2IyACstUi3gAKpztOSYv1ogBJKApWC2O4PGAAQV1tku2yO3gEAiAA"
] |
prettier/prettier | 13,621 | prettier__prettier-13621 | [
"13620"
] | 6d6e5177dbb9746e55706f4bece8666df578aceb | diff --git a/src/language-js/print/template-literal.js b/src/language-js/print/template-literal.js
--- a/src/language-js/print/template-literal.js
+++ b/src/language-js/print/template-literal.js
@@ -50,6 +50,7 @@ function printTemplateLiteral(path, print, options) {
parts.push(lineSuffixBoundary, "`");
+ let previousQuasiIndentSize = 0;
path.each(({ index, node: quasi }) => {
parts.push(print());
@@ -69,7 +70,11 @@ function printTemplateLiteral(path, print, options) {
// expression inside at the beginning of ${ instead of the beginning
// of the `.
const { tabWidth } = options;
- const indentSize = getIndentSize(quasi.value.raw, tabWidth);
+ const text = quasi.value.raw;
+ const indentSize = text.includes("\n")
+ ? getIndentSize(text, tabWidth)
+ : previousQuasiIndentSize;
+ previousQuasiIndentSize = indentSize;
let expressionDoc = expressionDocs[index];
@@ -90,7 +95,7 @@ function printTemplateLiteral(path, print, options) {
}
const aligned =
- indentSize === 0 && quasi.value.raw.endsWith("\n")
+ indentSize === 0 && text.endsWith("\n")
? align(Number.NEGATIVE_INFINITY, expressionDoc)
: addAlignmentToDoc(expressionDoc, indentSize, tabWidth);
| diff --git a/tests/format/js/template-literals/__snapshots__/jsfmt.spec.js.snap b/tests/format/js/template-literals/__snapshots__/jsfmt.spec.js.snap
--- a/tests/format/js/template-literals/__snapshots__/jsfmt.spec.js.snap
+++ b/tests/format/js/template-literals/__snapshots__/jsfmt.spec.js.snap
@@ -231,6 +231,190 @@ throw new Error(
================================================================================
`;
+exports[`indention.js format 1`] = `
+====================================options=====================================
+parsers: ["babel", "flow", "typescript"]
+printWidth: 80
+ | printWidth
+=====================================input======================================
+[
+ \`
+ 1. Go to "-{chalk.green.underline(
+ "https://www.example.com/drupedalKangarooTransformer"
+ )}" \${chalk.green.underline(
+ "https://www.example.com/drupedalKangarooTransformer"
+ )}
+ \`,
+ \`
+ 2. Go to "\${chalk.green.underline(
+ "https://www.example.com/drupedalKangarooTransformer"
+ )}" \${chalk.green.underline(
+ "https://www.example.com/drupedalKangarooTransformer"
+ )}
+ \`,
+ \`
+ 1. Go to "-{chalk.green.underline(
+ "https://www.example.com/drupedalKangarooTransformer"
+ )}" \${chalk.green.underline(
+ "https://www.example.com/drupedalKangarooTransformer"
+ )}
+ 2. Go to "\${chalk.green.underline(
+ "https://www.example.com/drupedalKangarooTransformer"
+ )}" \${chalk.green.underline(
+ "https://www.example.com/drupedalKangarooTransformer"
+ )}
+ \`,
+ \`
+ 2. Go to "\${chalk.green.underline(
+ "https://www.example.com/drupedalKangarooTransformer"
+ )}" \${chalk.green.underline(
+ "https://www.example.com/drupedalKangarooTransformer"
+ )}
+ 1. Go to "-{chalk.green.underline(
+ "https://www.example.com/drupedalKangarooTransformer"
+ )}" \${chalk.green.underline(
+ "https://www.example.com/drupedalKangarooTransformer"
+ )}
+ \`,
+ \`
+ 1. Go to "-{chalk.green.underline(
+ "https://www.example.com/drupedalKangarooTransformer"
+ )}" \${chalk.green.underline(
+ "https://www.example.com/drupedalKangarooTransformer"
+ )}
+ 2. Go to "\${chalk.green.underline(
+ "https://www.example.com/drupedalKangarooTransformer"
+ )}" \${chalk.green.underline(
+ "https://www.example.com/drupedalKangarooTransformer"
+ )}
+ \`,
+ \`
+ 1. Go to "-{chalk.green.underline(
+ "https://www.example.com/drupedalKangarooTransformer"
+ )}" \${chalk.green.underline(
+ "https://www.example.com/drupedalKangarooTransformer"
+ )}
+ 2. Go to "\${chalk.green.underline(
+ "https://www.example.com/drupedalKangarooTransformer"
+ )}" \${chalk.green.underline(
+ "https://www.example.com/drupedalKangarooTransformer"
+ )}
+ \`,
+\`
+# blabla \${a} \${chalk.green.underline("https://www.example.com/drupedalKangarooTransformer")}
+
+ 2. Go to "\${chalk.green.underline(
+ "https://www.example.com/drupedalKangarooTransformer",
+ )}"
+
+# blabla \${a} \${chalk.green.underline("https://www.example.com/drupedalKangarooTransformer")}
+\`,
+ \`
+ # blabla \${a} \${chalk.green.underline("https://www.example.com/drupedalKangarooTransformer")}
+
+ 2. Go to "\${chalk.green.underline(
+ "https://www.example.com/drupedalKangarooTransformer",
+ )}"
+
+ # blabla \${a} \${chalk.green.underline("https://www.example.com/drupedalKangarooTransformer")}
+ \`,
+]
+
+=====================================output=====================================
+[
+ \`
+ 1. Go to "-{chalk.green.underline(
+ "https://www.example.com/drupedalKangarooTransformer"
+ )}" \${chalk.green.underline(
+ "https://www.example.com/drupedalKangarooTransformer",
+ )}
+ \`,
+ \`
+ 2. Go to "\${chalk.green.underline(
+ "https://www.example.com/drupedalKangarooTransformer",
+ )}" \${chalk.green.underline(
+ "https://www.example.com/drupedalKangarooTransformer",
+ )}
+ \`,
+ \`
+ 1. Go to "-{chalk.green.underline(
+ "https://www.example.com/drupedalKangarooTransformer"
+ )}" \${chalk.green.underline(
+ "https://www.example.com/drupedalKangarooTransformer",
+ )}
+ 2. Go to "\${chalk.green.underline(
+ "https://www.example.com/drupedalKangarooTransformer",
+ )}" \${chalk.green.underline(
+ "https://www.example.com/drupedalKangarooTransformer",
+ )}
+ \`,
+ \`
+ 2. Go to "\${chalk.green.underline(
+ "https://www.example.com/drupedalKangarooTransformer",
+ )}" \${chalk.green.underline(
+ "https://www.example.com/drupedalKangarooTransformer",
+ )}
+ 1. Go to "-{chalk.green.underline(
+ "https://www.example.com/drupedalKangarooTransformer"
+ )}" \${chalk.green.underline(
+ "https://www.example.com/drupedalKangarooTransformer",
+ )}
+ \`,
+ \`
+ 1. Go to "-{chalk.green.underline(
+ "https://www.example.com/drupedalKangarooTransformer"
+ )}" \${chalk.green.underline(
+ "https://www.example.com/drupedalKangarooTransformer",
+ )}
+ 2. Go to "\${chalk.green.underline(
+ "https://www.example.com/drupedalKangarooTransformer",
+ )}" \${chalk.green.underline(
+ "https://www.example.com/drupedalKangarooTransformer",
+ )}
+ \`,
+ \`
+ 1. Go to "-{chalk.green.underline(
+ "https://www.example.com/drupedalKangarooTransformer"
+ )}" \${chalk.green.underline(
+ "https://www.example.com/drupedalKangarooTransformer",
+ )}
+ 2. Go to "\${chalk.green.underline(
+ "https://www.example.com/drupedalKangarooTransformer",
+ )}" \${chalk.green.underline(
+ "https://www.example.com/drupedalKangarooTransformer",
+ )}
+ \`,
+ \`
+# blabla \${a} \${chalk.green.underline(
+ "https://www.example.com/drupedalKangarooTransformer",
+ )}
+
+ 2. Go to "\${chalk.green.underline(
+ "https://www.example.com/drupedalKangarooTransformer",
+ )}"
+
+# blabla \${a} \${chalk.green.underline(
+ "https://www.example.com/drupedalKangarooTransformer",
+ )}
+\`,
+ \`
+ # blabla \${a} \${chalk.green.underline(
+ "https://www.example.com/drupedalKangarooTransformer",
+ )}
+
+ 2. Go to "\${chalk.green.underline(
+ "https://www.example.com/drupedalKangarooTransformer",
+ )}"
+
+ # blabla \${a} \${chalk.green.underline(
+ "https://www.example.com/drupedalKangarooTransformer",
+ )}
+ \`,
+];
+
+================================================================================
+`;
+
exports[`logical-expressions.js format 1`] = `
====================================options=====================================
parsers: ["babel", "flow", "typescript"]
diff --git a/tests/format/js/template-literals/indention.js b/tests/format/js/template-literals/indention.js
new file mode 100644
--- /dev/null
+++ b/tests/format/js/template-literals/indention.js
@@ -0,0 +1,82 @@
+[
+ `
+ 1. Go to "-{chalk.green.underline(
+ "https://www.example.com/drupedalKangarooTransformer"
+ )}" ${chalk.green.underline(
+ "https://www.example.com/drupedalKangarooTransformer"
+ )}
+ `,
+ `
+ 2. Go to "${chalk.green.underline(
+ "https://www.example.com/drupedalKangarooTransformer"
+ )}" ${chalk.green.underline(
+ "https://www.example.com/drupedalKangarooTransformer"
+ )}
+ `,
+ `
+ 1. Go to "-{chalk.green.underline(
+ "https://www.example.com/drupedalKangarooTransformer"
+ )}" ${chalk.green.underline(
+ "https://www.example.com/drupedalKangarooTransformer"
+ )}
+ 2. Go to "${chalk.green.underline(
+ "https://www.example.com/drupedalKangarooTransformer"
+ )}" ${chalk.green.underline(
+ "https://www.example.com/drupedalKangarooTransformer"
+ )}
+ `,
+ `
+ 2. Go to "${chalk.green.underline(
+ "https://www.example.com/drupedalKangarooTransformer"
+ )}" ${chalk.green.underline(
+ "https://www.example.com/drupedalKangarooTransformer"
+ )}
+ 1. Go to "-{chalk.green.underline(
+ "https://www.example.com/drupedalKangarooTransformer"
+ )}" ${chalk.green.underline(
+ "https://www.example.com/drupedalKangarooTransformer"
+ )}
+ `,
+ `
+ 1. Go to "-{chalk.green.underline(
+ "https://www.example.com/drupedalKangarooTransformer"
+ )}" ${chalk.green.underline(
+ "https://www.example.com/drupedalKangarooTransformer"
+ )}
+ 2. Go to "${chalk.green.underline(
+ "https://www.example.com/drupedalKangarooTransformer"
+ )}" ${chalk.green.underline(
+ "https://www.example.com/drupedalKangarooTransformer"
+ )}
+ `,
+ `
+ 1. Go to "-{chalk.green.underline(
+ "https://www.example.com/drupedalKangarooTransformer"
+ )}" ${chalk.green.underline(
+ "https://www.example.com/drupedalKangarooTransformer"
+ )}
+ 2. Go to "${chalk.green.underline(
+ "https://www.example.com/drupedalKangarooTransformer"
+ )}" ${chalk.green.underline(
+ "https://www.example.com/drupedalKangarooTransformer"
+ )}
+ `,
+`
+# blabla ${a} ${chalk.green.underline("https://www.example.com/drupedalKangarooTransformer")}
+
+ 2. Go to "${chalk.green.underline(
+ "https://www.example.com/drupedalKangarooTransformer",
+ )}"
+
+# blabla ${a} ${chalk.green.underline("https://www.example.com/drupedalKangarooTransformer")}
+`,
+ `
+ # blabla ${a} ${chalk.green.underline("https://www.example.com/drupedalKangarooTransformer")}
+
+ 2. Go to "${chalk.green.underline(
+ "https://www.example.com/drupedalKangarooTransformer",
+ )}"
+
+ # blabla ${a} ${chalk.green.underline("https://www.example.com/drupedalKangarooTransformer")}
+ `,
+]
| Strange indention in template literal
**Prettier 2.7.1**
[Playground link](https://prettier.io/playground/#N4Igxg9gdgLgprEAuEADAOlABDrBGAOiwHEIsYz0QBaagQ2qqwBJhNdcwALOgGwGsCAcwBOcBAQCuUACZwRvAJZQ4ACipcYMAA4BnJAHoDAd1MEo2gLYArXQUiWD2umH50hcJ2K2L5Bl2BwurpUAJTsuAC+ETgATESk5JQgrHTRICxs2BxY3HyCouJQUrLySirqIJo6+kamxuZWtvYQjs6u7p7a3jC+Iv5ggcFhMVjRUKggADQgENq90LrIoHQiIhDGAAqrCEsofMZ0AJ5LMwBGIi78cDAAynSWcAAyynDIAGZ8unDnl643t3ayiEyBgIkkPxAcEsZzgMjkMiedCgQkknQAYhARJY6D4UcgQHRJBRplUYJZeAB1LiKeC6dpwW67WmKAButKOBLAwVJym+Ihgm0uQhxHy+kNsAA9bsDeHAAIqSCDwMW8b4zZwifkEs50WG8UndZQwSmKGQwLjIAAcAAYNetvpTLtoCd0gvJWW8ZgBHJXwIVzPaE3TUFRwuGksS+xRiIXuUVIT5qyHfSyKUHglOyhV+t6J8UzGB603my1IWKFy6KcpCADCrQTUN0AFZSZJvgAVPV7JPqkCsiEASVKsFuYBEinmAEFZLcYEc5arvpFIkA)
<!-- prettier-ignore -->
```sh
--parser babel
```
**Input:**
<!-- prettier-ignore -->
```jsx
`
1. Go to "--a-" ${
chalk.green.underline("https://www.npmjs.com/package/prettier/access")
}
2. Go to "${a}" ${
chalk.green.underline("https://www.npmjs.com/package/prettier/access")
}
`
```
**Output:**
<!-- prettier-ignore -->
```jsx
`
1. Go to "--a-" ${chalk.green.underline(
"https://www.npmjs.com/package/prettier/access"
)}
2. Go to "${a}" ${chalk.green.underline(
"https://www.npmjs.com/package/prettier/access"
)}
`;
```
**Expected behavior:**
Those two function calls should format in a similar style.
| Looking at the output doc, change it to
```diff
+ dedentToRoot(align(0,indent(indent(
group([
"chalk",
".",
"green",
".",
"underline",
group([
"(",
indent([softline, '"https://www.npmjs.com/package/prettier/access"']),
ifBreak(""),
softline,
")",
]),
]),
+ )))),
```
will result the same.
[playground](https://prettier.io/playground/#N4Igxg9gdgLgprEAuEBtAOlABFgNgSyjgGUBXAM3PwA8AhCUqAEwEMAnATwBpMd0QABvx7Y8+eGxa4CRETgDkOHAEYAdFgDiELDG38AtPpb7+WeXKwBzNgwAOACgyi+IACTBhvJUzg-YAFQgAJQgIGHsvJSwpfEsoCOcorAAGCySsQj9wyPSM5gRsxNyrG1IHJ2L0-jAACykAa08iyv5VJsqk-ms4BHaOpVa+-qx+Rh82GTgh-us7Rxzh-giQNOG8rPnm4YBnCHIYSdW1s34amBhbbaQAemuAdwfVKFsAWwArbdVIF+vbFjB6ixLHBfmw4Od8HA2Nd-mA4NttvxzAt+gBdACUR36+HItDBLHqy34mJRHV2+0OpJaIHR0w6GKx6QxVJwtK2WDZ6U5ShJiUmZEoNHoY3Y3By-AAvn0GV4CBIpJTRIolAAmdRaHR6EDI0SzMqbKL8dzTHxZQIhML2GJxeypdYFeyZB38FjE9Hu1b8ihUOgMZii1aS6W8hSmHU4PXlcVuDwrFlYU0Fc2hcLW+KpJ2wR35LM5SMGzrgOq4Rpx5qDMtVEDdXqVwttOuGkBjKGTOklOYVXJLds4TPhVDkg6EOBcE4gM4XK63B53J6vD5fCA-P4AoEg2xgiFQmFgOEIpEy9k4vFwAlEmmMrBDxXFYnto9RR+Vd0enJewW+kWcQMgKV1585ShBURwsfghDrfgAG4+jqNgmEVVEVhACBbBgfBoG2ZBQHYGw7gABXYBAsJQKQ7hYDgsK4EAACNJABcFiBYF44AAGRHZByCkbZR1o+j6kY1dCEsZAYDYUheLgF4aN8U1WJYKBLFIdcADEIDYF4WAhRTkBAFhSF0ZCzheXAAHUanEeFVxIYjxHwAA3cQOF0sAEWQwgeLYGB8MkSxNM47jeI+ahiGE3A4AARVIMI4AC3AeOov42E83SmAgMB9DgahbFwdSoWQzdCBgUz8CYGAamQAAOVIQE3CAeNMyRbF0zd4ShezYuogBHaL4B81CSL07Z9CIXxfGQsEevwMEfKBfykC4+LeJ4l58FE8TlrCyLetihbAuomAWBokqyoqpAVQOyR8BkSwAGFl3mkB4QAVmQ0geP8I6SMWhKQHsiSAEkcxgYgwDYfA0IAQWYYgYA4cK4p4iUJSAA) | "2022-10-12T11:12:03Z" | 3.0 | [] | [
"tests/format/js/template-literals/jsfmt.spec.js"
] | JavaScript | [] | [
"https://prettier.io/playground/#N4Igxg9gdgLgprEAuEADAOlABDrBGAOiwHEIsYz0QBaagQ2qqwBJhNdcwALOgGwGsCAcwBOcBAQCuUACZwRvAJZQ4ACipcYMAA4BnJAHoDAd1MEo2gLYArXQUiWD2umH50hcJ2K2L5Bl2BwurpUAJTsuAC+ETgATESk5JQgrHTRICxs2BxY3HyCouJQUrLySirqIJo6+kamxuZWtvYQjs6u7p7a3jC+Iv5ggcFhMVjRUKggADQgENq90LrIoHQiIhDGAAqrCEsofMZ0AJ5LMwBGIi78cDAAynSWcAAyynDIAGZ8unDnl643t3ayiEyBgIkkPxAcEsZzgMjkMiedCgQkknQAYhARJY6D4UcgQHRJBRplUYJZeAB1LiKeC6dpwW67WmKAButKOBLAwVJym+Ihgm0uQhxHy+kNsAA9bsDeHAAIqSCDwMW8b4zZwifkEs50WG8UndZQwSmKGQwLjIAAcAAYNetvpTLtoCd0gvJWW8ZgBHJXwIVzPaE3TUFRwuGksS+xRiIXuUVIT5qyHfSyKUHglOyhV+t6J8UzGB603my1IWKFy6KcpCADCrQTUN0AFZSZJvgAVPV7JPqkCsiEASVKsFuYBEinmAEFZLcYEc5arvpFIkA"
] |
prettier/prettier | 13,877 | prettier__prettier-13877 | [
"9760"
] | c4f1a670d9c47144cc19c1d9ea91950abe1cf293 | diff --git a/src/language-js/parse/babel.js b/src/language-js/parse/babel.js
--- a/src/language-js/parse/babel.js
+++ b/src/language-js/parse/babel.js
@@ -207,8 +207,6 @@ const allowedMessageCodes = new Set([
"InvalidTupleMemberLabel",
"NonClassMethodPropertyHasAbstractModifer",
- "ClassMethodHasDeclare",
- "ClassMethodHasReadonly",
"DuplicateAccessibilityModifier",
"IndexSignatureHasDeclare",
diff --git a/src/language-js/parse/postprocess/ts-syntax-kind.evaluate.js b/src/language-js/parse/postprocess/ts-syntax-kind.evaluate.js
deleted file mode 100644
--- a/src/language-js/parse/postprocess/ts-syntax-kind.evaluate.js
+++ /dev/null
@@ -1,11 +0,0 @@
-import ts from "typescript";
-
-export const {
- AbstractKeyword,
- SourceFile,
- DeclareKeyword,
- PropertyDeclaration,
- InKeyword,
- OutKeyword,
- MethodSignature,
-} = ts.SyntaxKind;
diff --git a/src/language-js/parse/postprocess/typescript.js b/src/language-js/parse/postprocess/typescript.js
--- a/src/language-js/parse/postprocess/typescript.js
+++ b/src/language-js/parse/postprocess/typescript.js
@@ -1,10 +1,11 @@
import isNonEmptyArray from "../../../utils/is-non-empty-array.js";
import visitNode from "./visit-node.js";
import throwTsSyntaxError from "./throw-ts-syntax-error.js";
-import * as SyntaxKind from "./ts-syntax-kind.evaluate.js";
+
+let ts;
function getTsNodeLocation(nodeOrToken) {
- const sourceFile = getSourceFileOfNode(nodeOrToken);
+ const sourceFile = ts.getSourceFileOfNode(nodeOrToken);
const [start, end] = [nodeOrToken.pos, nodeOrToken.end].map((position) => {
const { line, character: column } =
sourceFile.getLineAndCharacterOfPosition(position);
@@ -14,12 +15,8 @@ function getTsNodeLocation(nodeOrToken) {
return { start, end };
}
-// Copied from https://unpkg.com/[email protected]/lib/typescript.js
-function getSourceFileOfNode(node) {
- while (node && node.kind !== SyntaxKind.SourceFile) {
- node = node.parent;
- }
- return node;
+function throwErrorOnTsNode(node, message) {
+ throwTsSyntaxError({ loc: getTsNodeLocation(node) }, message);
}
// Invalid decorators are removed since `@typescript-eslint/typescript-estree` v4
@@ -33,10 +30,7 @@ function throwErrorForInvalidDecorator(tsNode) {
const [{ expression }] = illegalDecorators;
- throwTsSyntaxError(
- { loc: getTsNodeLocation(expression) },
- "Decorators are not valid here."
- );
+ throwErrorOnTsNode(expression, "Decorators are not valid here.");
}
// Values of abstract property is removed since `@typescript-eslint/typescript-estree` v5
@@ -44,11 +38,11 @@ function throwErrorForInvalidDecorator(tsNode) {
function throwErrorForInvalidAbstractProperty(tsNode, esTreeNode) {
if (
!(
- tsNode.kind === SyntaxKind.PropertyDeclaration &&
+ tsNode.kind === ts.SyntaxKind.PropertyDeclaration &&
tsNode.initializer &&
esTreeNode.value === null &&
tsNode.modifiers?.some(
- (modifier) => modifier.kind === SyntaxKind.AbstractKeyword
+ (modifier) => modifier.kind === ts.SyntaxKind.AbstractKeyword
)
)
) {
@@ -61,55 +55,86 @@ function throwErrorForInvalidAbstractProperty(tsNode, esTreeNode) {
);
}
-function throwErrorForInvalidDeclare(tsNode, esTreeNode) {
- if (
- !(
- esTreeNode.type === "MethodDefinition" &&
- (esTreeNode.kind === "get" || esTreeNode.kind === "set")
- )
- ) {
+// Based on `checkGrammarModifiers` function in `typescript`
+function throwErrorForInvalidModifier(node) {
+ const { modifiers } = node;
+ if (!isNonEmptyArray(modifiers)) {
return;
}
- const declareKeyword = tsNode.modifiers?.find(
- (modifier) => modifier.kind === SyntaxKind.DeclareKeyword
- );
+ const { SyntaxKind } = ts;
- if (!declareKeyword) {
- return;
- }
+ for (const modifier of modifiers) {
+ if (ts.isDecorator(modifier)) {
+ continue;
+ }
- throwTsSyntaxError(
- { loc: getTsNodeLocation(declareKeyword) },
- /* cspell:disable-next-line */
- `'declare' is not allowed in ${esTreeNode.kind}ters.`
- );
-}
+ if (modifier.kind !== SyntaxKind.ReadonlyKeyword) {
+ if (
+ node.kind === SyntaxKind.PropertySignature ||
+ node.kind === SyntaxKind.MethodSignature
+ ) {
+ throwErrorOnTsNode(
+ modifier,
+ `'${ts.tokenToString(
+ modifier.kind
+ )}' modifier cannot appear on a type member`
+ );
+ }
+
+ if (
+ node.kind === SyntaxKind.IndexSignature &&
+ (modifier.kind !== SyntaxKind.StaticKeyword ||
+ !ts.isClassLike(node.parent))
+ ) {
+ throwErrorOnTsNode(
+ modifier,
+ `'${ts.tokenToString(
+ modifier.kind
+ )}' modifier cannot appear on an index signature`
+ );
+ }
+ }
-function throwErrorForInvalidModifierOnTypeParameter(tsNode, esTreeNode) {
- if (
- esTreeNode.type !== "TSMethodSignature" ||
- tsNode.kind !== SyntaxKind.MethodSignature
- ) {
- return;
- }
+ if (
+ modifier.kind !== SyntaxKind.InKeyword &&
+ modifier.kind !== SyntaxKind.OutKeyword &&
+ node.kind === SyntaxKind.TypeParameter
+ ) {
+ throwErrorOnTsNode(
+ modifier,
+ `'${ts.tokenToString(
+ modifier.kind
+ )}' modifier cannot appear on a type parameter`
+ );
+ }
- const invalidModifier = tsNode.modifiers?.find(
- (modifier) =>
- !(
- modifier.kind === SyntaxKind.InKeyword ||
- modifier.kind === SyntaxKind.OutKeyword
- )
- );
+ if (
+ modifier.kind === SyntaxKind.ReadonlyKeyword &&
+ node.kind !== SyntaxKind.PropertyDeclaration &&
+ node.kind !== SyntaxKind.PropertySignature &&
+ node.kind !== SyntaxKind.IndexSignature &&
+ node.kind !== SyntaxKind.Parameter
+ ) {
+ throwErrorOnTsNode(
+ modifier,
+ "'readonly' modifier can only appear on a property declaration or index signature."
+ );
+ }
- if (!invalidModifier) {
- return;
+ if (
+ modifier.kind === SyntaxKind.DeclareKeyword &&
+ ts.isClassLike(node.parent) &&
+ !ts.isPropertyDeclaration(node)
+ ) {
+ throwErrorOnTsNode(
+ modifier,
+ `'${ts.tokenToString(
+ modifier.kind
+ )}' modifier cannot appear on class elements of this kind.`
+ );
+ }
}
-
- throwTsSyntaxError(
- { loc: getTsNodeLocation(invalidModifier) },
- "This modifier cannot appear on a type member"
- );
}
function getTsNode(node, tsParseResult) {
@@ -127,27 +152,46 @@ function getTsNode(node, tsParseResult) {
return tsNode;
}
-function throwErrorForInvalidNodes(tsParseResult, options) {
+async function throwErrorForInvalidNodes(tsParseResult, options) {
if (
- // decorators
- // abstract properties
- // declare in accessor
- // modifiers on type parameter
- !/@|abstract|declare|interface/.test(options.originalText)
+ // decorators and modifiers
+ !new RegExp(
+ [
+ "@",
+ "abstract",
+ "accessor",
+ "async",
+ "const",
+ "declare",
+ "default",
+ "export",
+ "static",
+ "in",
+ "out",
+ "override",
+ "public",
+ "private",
+ "protected",
+ "readonly",
+ ].join("|")
+ ).test(options.originalText)
) {
return;
}
+ if (!ts) {
+ ({ default: ts } = await import("typescript"));
+ }
+
visitNode(tsParseResult.ast, (esTreeNode) => {
const tsNode = getTsNode(esTreeNode, tsParseResult);
if (!tsNode) {
return;
}
- throwErrorForInvalidDeclare(tsNode, esTreeNode);
throwErrorForInvalidDecorator(tsNode);
throwErrorForInvalidAbstractProperty(tsNode, esTreeNode);
- throwErrorForInvalidModifierOnTypeParameter(tsNode, esTreeNode);
+ throwErrorForInvalidModifier(tsNode);
});
}
diff --git a/src/language-js/parse/typescript.js b/src/language-js/parse/typescript.js
--- a/src/language-js/parse/typescript.js
+++ b/src/language-js/parse/typescript.js
@@ -57,7 +57,7 @@ async function parse(text, options = {}) {
options.originalText = text;
- throwErrorForInvalidNodes(result, options);
+ await throwErrorForInvalidNodes(result, options);
return postprocess(result.ast, options);
}
diff --git a/src/language-js/print/class.js b/src/language-js/print/class.js
--- a/src/language-js/print/class.js
+++ b/src/language-js/print/class.js
@@ -165,14 +165,6 @@ function printClassMethod(path, options, print) {
if (node.accessibility) {
parts.push(node.accessibility + " ");
}
- // "readonly" and "declare" are supported by only "babel-ts"
- // https://github.com/prettier/prettier/issues/9760
- if (node.readonly) {
- parts.push("readonly ");
- }
- if (node.declare) {
- parts.push("declare ");
- }
if (node.static) {
parts.push("static ");
| diff --git a/tests/format/misc/errors/typescript/__snapshots__/jsfmt.spec.js.snap b/tests/format/misc/errors/typescript/__snapshots__/jsfmt.spec.js.snap
--- a/tests/format/misc/errors/typescript/__snapshots__/jsfmt.spec.js.snap
+++ b/tests/format/misc/errors/typescript/__snapshots__/jsfmt.spec.js.snap
@@ -1,7 +1,7 @@
// Jest Snapshot v1, https://goo.gl/fbAQLP
exports[`declare-getter.ts [typescript] format 1`] = `
-"'declare' is not allowed in getters. (1:12)
+"'declare' modifier cannot appear on class elements of this kind. (1:12)
> 1 | class Foo {
| ^
> 2 | declare get foo()
@@ -11,7 +11,7 @@ exports[`declare-getter.ts [typescript] format 1`] = `
`;
exports[`declare-setter.ts [typescript] format 1`] = `
-"'declare' is not allowed in setters. (1:12)
+"'declare' modifier cannot appear on class elements of this kind. (1:12)
> 1 | class Foo {
| ^
> 2 | declare set foo(v)
@@ -94,78 +94,6 @@ exports[`snippet: #5 [typescript] format 1`] = `
| ^"
`;
-exports[`snippet: #6 [typescript] format 1`] = `
-"This modifier cannot appear on a type member (1:16)
-> 1 | interface Foo {
- | ^
-> 2 | abstract e();
- | ^^^^^^^^^^^
- 3 | }"
-`;
-
-exports[`snippet: #7 [typescript] format 1`] = `
-"This modifier cannot appear on a type member (1:16)
-> 1 | interface Foo {
- | ^
-> 2 | declare e();
- | ^^^^^^^^^^
- 3 | }"
-`;
-
-exports[`snippet: #8 [typescript] format 1`] = `
-"This modifier cannot appear on a type member (1:16)
-> 1 | interface Foo {
- | ^
-> 2 | export e();
- | ^^^^^^^^^
- 3 | }"
-`;
-
-exports[`snippet: #9 [typescript] format 1`] = `
-"This modifier cannot appear on a type member (1:16)
-> 1 | interface Foo {
- | ^
-> 2 | static e();
- | ^^^^^^^^^
- 3 | }"
-`;
-
-exports[`snippet: #10 [typescript] format 1`] = `
-"This modifier cannot appear on a type member (1:16)
-> 1 | interface Foo {
- | ^
-> 2 | private e();
- | ^^^^^^^^^^
- 3 | }"
-`;
-
-exports[`snippet: #11 [typescript] format 1`] = `
-"This modifier cannot appear on a type member (1:16)
-> 1 | interface Foo {
- | ^
-> 2 | protected e();
- | ^^^^^^^^^^^^
- 3 | }"
-`;
-
-exports[`snippet: #12 [typescript] format 1`] = `
-"This modifier cannot appear on a type member (1:16)
-> 1 | interface Foo {
- | ^
-> 2 | public e();
- | ^^^^^^^^^
- 3 | }"
-`;
-
-exports[`snippet: #13 [typescript] format 1`] = `
-"This modifier cannot appear on a type member (1:16)
-> 1 | interface Foo {
- | ^
-> 2 | readonly e();
- | ^^^^^^^^^^^
- 3 | }"
-`;
-
exports[`value-of-abstract-property.ts [typescript] format 1`] = `
"Abstract property cannot have an initializer (2:3)
1 | abstract class Foo {
diff --git a/tests/format/misc/errors/typescript/babel-ts/__snapshots__/jsfmt.spec.js.snap b/tests/format/misc/errors/typescript/babel-ts/__snapshots__/jsfmt.spec.js.snap
--- a/tests/format/misc/errors/typescript/babel-ts/__snapshots__/jsfmt.spec.js.snap
+++ b/tests/format/misc/errors/typescript/babel-ts/__snapshots__/jsfmt.spec.js.snap
@@ -43,67 +43,3 @@ exports[`snippet: #2 [babel-ts] format 1`] = `
> 1 | <string>foo = '100';
| ^"
`;
-
-exports[`snippet: #3 [babel-ts] format 1`] = `
-"'abstract' modifier cannot appear on a type member. (2:3)
- 1 | interface Foo {
-> 2 | abstract e();
- | ^
- 3 | }"
-`;
-
-exports[`snippet: #4 [babel-ts] format 1`] = `
-"'declare' modifier cannot appear on a type member. (2:3)
- 1 | interface Foo {
-> 2 | declare e();
- | ^
- 3 | }"
-`;
-
-exports[`snippet: #5 [babel-ts] format 1`] = `
-"Unexpected token, expected ";" (2:10)
- 1 | interface Foo {
-> 2 | export e();
- | ^
- 3 | }"
-`;
-
-exports[`snippet: #6 [babel-ts] format 1`] = `
-"'static' modifier cannot appear on a type member. (2:3)
- 1 | interface Foo {
-> 2 | static e();
- | ^
- 3 | }"
-`;
-
-exports[`snippet: #7 [babel-ts] format 1`] = `
-"'private' modifier cannot appear on a type member. (2:3)
- 1 | interface Foo {
-> 2 | private e();
- | ^
- 3 | }"
-`;
-
-exports[`snippet: #8 [babel-ts] format 1`] = `
-"'protected' modifier cannot appear on a type member. (2:3)
- 1 | interface Foo {
-> 2 | protected e();
- | ^
- 3 | }"
-`;
-
-exports[`snippet: #9 [babel-ts] format 1`] = `
-"'public' modifier cannot appear on a type member. (2:3)
- 1 | interface Foo {
-> 2 | public e();
- | ^
- 3 | }"
-`;
-
-exports[`snippet: #10 [babel-ts] format 1`] = `
-"'readonly' modifier can only appear on a property declaration or index signature. (2:3)
- 1 | interface Foo {
-> 2 | readonly e();
- | ^
- 3 | }"
-`;
diff --git a/tests/format/misc/errors/typescript/babel-ts/jsfmt.spec.js b/tests/format/misc/errors/typescript/babel-ts/jsfmt.spec.js
--- a/tests/format/misc/errors/typescript/babel-ts/jsfmt.spec.js
+++ b/tests/format/misc/errors/typescript/babel-ts/jsfmt.spec.js
@@ -1,5 +1,3 @@
-import { outdent } from "outdent";
-
run_spec(
{
importMeta: import.meta,
@@ -7,22 +5,6 @@ run_spec(
"foo as any = 10;",
"({ a: b as any = 2000 } = x);",
"<string>foo = '100';",
- ...[
- "abstract",
- "declare",
- "export",
- "static",
- "private",
- "protected",
- "public",
- "readonly",
- ].map(
- (modifier) => outdent`
- interface Foo {
- ${modifier} e();
- }
- `
- ),
],
},
["babel-ts"]
diff --git a/tests/format/misc/errors/typescript/jsfmt.spec.js b/tests/format/misc/errors/typescript/jsfmt.spec.js
--- a/tests/format/misc/errors/typescript/jsfmt.spec.js
+++ b/tests/format/misc/errors/typescript/jsfmt.spec.js
@@ -1,5 +1,3 @@
-import { outdent } from "outdent";
-
run_spec(
{
importMeta: import.meta,
@@ -10,22 +8,6 @@ run_spec(
'let x4 = <div>{"foo"}></div>;',
'let x5 = <div>}{"foo"}</div>;',
'let x6 = <div>>{"foo"}</div>;',
- ...[
- "abstract",
- "declare",
- "export",
- "static",
- "private",
- "protected",
- "public",
- "readonly",
- ].map(
- (modifier) => outdent`
- interface Foo {
- ${modifier} e();
- }
- `
- ),
],
},
["typescript"]
diff --git a/tests/format/misc/errors/typescript/modifiers/__snapshots__/jsfmt.spec.js.snap b/tests/format/misc/errors/typescript/modifiers/__snapshots__/jsfmt.spec.js.snap
new file mode 100644
--- /dev/null
+++ b/tests/format/misc/errors/typescript/modifiers/__snapshots__/jsfmt.spec.js.snap
@@ -0,0 +1,1186 @@
+// Jest Snapshot v1, https://goo.gl/fbAQLP
+
+exports[`snippet: #0 [babel-ts] format 1`] = `
+"'abstract' modifier cannot appear on a type member. (2:3)
+ 1 | interface Foo {
+> 2 | abstract method();
+ | ^
+ 3 | }"
+`;
+
+exports[`snippet: #0 [typescript] format 1`] = `
+"'abstract' modifier cannot appear on a type member (1:16)
+> 1 | interface Foo {
+ | ^
+> 2 | abstract method();
+ | ^^^^^^^^^^^
+ 3 | }"
+`;
+
+exports[`snippet: #1 [babel-ts] format 1`] = `
+"'abstract' modifier cannot appear on a type member. (2:3)
+ 1 | interface Foo {
+> 2 | abstract property;
+ | ^
+ 3 | }"
+`;
+
+exports[`snippet: #1 [typescript] format 1`] = `
+"'abstract' modifier cannot appear on a type member (1:16)
+> 1 | interface Foo {
+ | ^
+> 2 | abstract property;
+ | ^^^^^^^^^^^
+ 3 | }"
+`;
+
+exports[`snippet: #2 [babel-ts] format 1`] = `
+"'abstract' modifier cannot appear on a type member. (2:3)
+ 1 | interface Foo {
+> 2 | abstract [index: string]: number
+ | ^
+ 3 | }"
+`;
+
+exports[`snippet: #2 [typescript] format 1`] = `
+"'abstract' modifier cannot appear on an index signature (1:16)
+> 1 | interface Foo {
+ | ^
+> 2 | abstract [index: string]: number
+ | ^^^^^^^^^^^
+ 3 | }"
+`;
+
+exports[`snippet: #3 [babel-ts] format 1`] = `
+"'abstract' modifier cannot appear on a type member. (2:3)
+ 1 | const foo: {
+> 2 | abstract [index: string] : string
+ | ^
+ 3 | } = {};"
+`;
+
+exports[`snippet: #3 [typescript] format 1`] = `
+"'abstract' modifier cannot appear on an index signature (1:13)
+> 1 | const foo: {
+ | ^
+> 2 | abstract [index: string] : string
+ | ^^^^^^^^^^^
+ 3 | } = {};"
+`;
+
+exports[`snippet: #4 [babel-ts] format 1`] = `
+"Unexpected token, expected ";" (2:12)
+ 1 | interface Foo {
+> 2 | accessor method();
+ | ^
+ 3 | }"
+`;
+
+exports[`snippet: #4 [typescript] format 1`] = `
+"'accessor' modifier cannot appear on a type member (1:16)
+> 1 | interface Foo {
+ | ^
+> 2 | accessor method();
+ | ^^^^^^^^^^^
+ 3 | }"
+`;
+
+exports[`snippet: #5 [babel-ts] format 1`] = `
+"Unexpected token, expected ";" (2:12)
+ 1 | interface Foo {
+> 2 | accessor property;
+ | ^
+ 3 | }"
+`;
+
+exports[`snippet: #5 [typescript] format 1`] = `
+"'accessor' modifier cannot appear on a type member (1:16)
+> 1 | interface Foo {
+ | ^
+> 2 | accessor property;
+ | ^^^^^^^^^^^
+ 3 | }"
+`;
+
+exports[`snippet: #6 [babel-ts] format 1`] = `
+"Unexpected token, expected ";" (2:12)
+ 1 | interface Foo {
+> 2 | accessor [index: string]: number
+ | ^
+ 3 | }"
+`;
+
+exports[`snippet: #6 [typescript] format 1`] = `
+"'accessor' modifier cannot appear on an index signature (1:16)
+> 1 | interface Foo {
+ | ^
+> 2 | accessor [index: string]: number
+ | ^^^^^^^^^^^
+ 3 | }"
+`;
+
+exports[`snippet: #7 [babel-ts] format 1`] = `
+"Unexpected token, expected ";" (2:12)
+ 1 | const foo: {
+> 2 | accessor [index: string] : string
+ | ^
+ 3 | } = {};"
+`;
+
+exports[`snippet: #7 [typescript] format 1`] = `
+"'accessor' modifier cannot appear on an index signature (1:13)
+> 1 | const foo: {
+ | ^
+> 2 | accessor [index: string] : string
+ | ^^^^^^^^^^^
+ 3 | } = {};"
+`;
+
+exports[`snippet: #8 [babel-ts] format 1`] = `
+"Unexpected token, expected ";" (2:9)
+ 1 | interface Foo {
+> 2 | async method();
+ | ^
+ 3 | }"
+`;
+
+exports[`snippet: #8 [typescript] format 1`] = `
+"'async' modifier cannot appear on a type member (1:16)
+> 1 | interface Foo {
+ | ^
+> 2 | async method();
+ | ^^^^^^^^
+ 3 | }"
+`;
+
+exports[`snippet: #9 [babel-ts] format 1`] = `
+"Unexpected token, expected ";" (2:9)
+ 1 | interface Foo {
+> 2 | async property;
+ | ^
+ 3 | }"
+`;
+
+exports[`snippet: #9 [typescript] format 1`] = `
+"'async' modifier cannot appear on a type member (1:16)
+> 1 | interface Foo {
+ | ^
+> 2 | async property;
+ | ^^^^^^^^
+ 3 | }"
+`;
+
+exports[`snippet: #10 [babel-ts] format 1`] = `
+"Unexpected token, expected ";" (2:9)
+ 1 | interface Foo {
+> 2 | async [index: string]: number
+ | ^
+ 3 | }"
+`;
+
+exports[`snippet: #10 [typescript] format 1`] = `
+"'async' modifier cannot appear on an index signature (1:16)
+> 1 | interface Foo {
+ | ^
+> 2 | async [index: string]: number
+ | ^^^^^^^^
+ 3 | }"
+`;
+
+exports[`snippet: #11 [babel-ts] format 1`] = `
+"Unexpected token, expected ";" (2:9)
+ 1 | const foo: {
+> 2 | async [index: string] : string
+ | ^
+ 3 | } = {};"
+`;
+
+exports[`snippet: #11 [typescript] format 1`] = `
+"'async' modifier cannot appear on an index signature (1:13)
+> 1 | const foo: {
+ | ^
+> 2 | async [index: string] : string
+ | ^^^^^^^^
+ 3 | } = {};"
+`;
+
+exports[`snippet: #12 [babel-ts] format 1`] = `
+"Unexpected token, expected ";" (2:9)
+ 1 | interface Foo {
+> 2 | const method();
+ | ^
+ 3 | }"
+`;
+
+exports[`snippet: #12 [typescript] format 1`] = `
+"';' expected. (2:9)
+ 1 | interface Foo {
+> 2 | const method();
+ | ^
+ 3 | }"
+`;
+
+exports[`snippet: #13 [babel-ts] format 1`] = `
+"Unexpected token, expected ";" (2:9)
+ 1 | interface Foo {
+> 2 | const property;
+ | ^
+ 3 | }"
+`;
+
+exports[`snippet: #13 [typescript] format 1`] = `
+"';' expected. (2:9)
+ 1 | interface Foo {
+> 2 | const property;
+ | ^
+ 3 | }"
+`;
+
+exports[`snippet: #14 [babel-ts] format 1`] = `
+"Unexpected token, expected ";" (2:9)
+ 1 | interface Foo {
+> 2 | const [index: string]: number
+ | ^
+ 3 | }"
+`;
+
+exports[`snippet: #14 [typescript] format 1`] = `
+"';' expected. (2:9)
+ 1 | interface Foo {
+> 2 | const [index: string]: number
+ | ^
+ 3 | }"
+`;
+
+exports[`snippet: #15 [babel-ts] format 1`] = `
+"Unexpected token, expected ";" (2:9)
+ 1 | const foo: {
+> 2 | const [index: string] : string
+ | ^
+ 3 | } = {};"
+`;
+
+exports[`snippet: #15 [typescript] format 1`] = `
+"';' expected. (2:9)
+ 1 | const foo: {
+> 2 | const [index: string] : string
+ | ^
+ 3 | } = {};"
+`;
+
+exports[`snippet: #16 [babel-ts] format 1`] = `
+"'declare' modifier cannot appear on a type member. (2:3)
+ 1 | interface Foo {
+> 2 | declare method();
+ | ^
+ 3 | }"
+`;
+
+exports[`snippet: #16 [typescript] format 1`] = `
+"'declare' modifier cannot appear on a type member (1:16)
+> 1 | interface Foo {
+ | ^
+> 2 | declare method();
+ | ^^^^^^^^^^
+ 3 | }"
+`;
+
+exports[`snippet: #17 [babel-ts] format 1`] = `
+"'declare' modifier cannot appear on a type member. (2:3)
+ 1 | interface Foo {
+> 2 | declare property;
+ | ^
+ 3 | }"
+`;
+
+exports[`snippet: #17 [typescript] format 1`] = `
+"'declare' modifier cannot appear on a type member (1:16)
+> 1 | interface Foo {
+ | ^
+> 2 | declare property;
+ | ^^^^^^^^^^
+ 3 | }"
+`;
+
+exports[`snippet: #18 [babel-ts] format 1`] = `
+"'declare' modifier cannot appear on a type member. (2:3)
+ 1 | interface Foo {
+> 2 | declare [index: string]: number
+ | ^
+ 3 | }"
+`;
+
+exports[`snippet: #18 [typescript] format 1`] = `
+"'declare' modifier cannot appear on an index signature (1:16)
+> 1 | interface Foo {
+ | ^
+> 2 | declare [index: string]: number
+ | ^^^^^^^^^^
+ 3 | }"
+`;
+
+exports[`snippet: #19 [babel-ts] format 1`] = `
+"'declare' modifier cannot appear on a type member. (2:3)
+ 1 | const foo: {
+> 2 | declare [index: string] : string
+ | ^
+ 3 | } = {};"
+`;
+
+exports[`snippet: #19 [typescript] format 1`] = `
+"'declare' modifier cannot appear on an index signature (1:13)
+> 1 | const foo: {
+ | ^
+> 2 | declare [index: string] : string
+ | ^^^^^^^^^^
+ 3 | } = {};"
+`;
+
+exports[`snippet: #20 [babel-ts] format 1`] = `
+"Unexpected token, expected ";" (2:11)
+ 1 | interface Foo {
+> 2 | default method();
+ | ^
+ 3 | }"
+`;
+
+exports[`snippet: #20 [typescript] format 1`] = `
+"';' expected. (2:11)
+ 1 | interface Foo {
+> 2 | default method();
+ | ^
+ 3 | }"
+`;
+
+exports[`snippet: #21 [babel-ts] format 1`] = `
+"Unexpected token, expected ";" (2:11)
+ 1 | interface Foo {
+> 2 | default property;
+ | ^
+ 3 | }"
+`;
+
+exports[`snippet: #21 [typescript] format 1`] = `
+"';' expected. (2:11)
+ 1 | interface Foo {
+> 2 | default property;
+ | ^
+ 3 | }"
+`;
+
+exports[`snippet: #22 [babel-ts] format 1`] = `
+"Unexpected token, expected ";" (2:11)
+ 1 | interface Foo {
+> 2 | default [index: string]: number
+ | ^
+ 3 | }"
+`;
+
+exports[`snippet: #22 [typescript] format 1`] = `
+"';' expected. (2:11)
+ 1 | interface Foo {
+> 2 | default [index: string]: number
+ | ^
+ 3 | }"
+`;
+
+exports[`snippet: #23 [babel-ts] format 1`] = `
+"Unexpected token, expected ";" (2:11)
+ 1 | const foo: {
+> 2 | default [index: string] : string
+ | ^
+ 3 | } = {};"
+`;
+
+exports[`snippet: #23 [typescript] format 1`] = `
+"';' expected. (2:11)
+ 1 | const foo: {
+> 2 | default [index: string] : string
+ | ^
+ 3 | } = {};"
+`;
+
+exports[`snippet: #24 [babel-ts] format 1`] = `
+"Unexpected token, expected ";" (2:10)
+ 1 | interface Foo {
+> 2 | export method();
+ | ^
+ 3 | }"
+`;
+
+exports[`snippet: #24 [typescript] format 1`] = `
+"'export' modifier cannot appear on a type member (1:16)
+> 1 | interface Foo {
+ | ^
+> 2 | export method();
+ | ^^^^^^^^^
+ 3 | }"
+`;
+
+exports[`snippet: #25 [babel-ts] format 1`] = `
+"Unexpected token, expected ";" (2:10)
+ 1 | interface Foo {
+> 2 | export property;
+ | ^
+ 3 | }"
+`;
+
+exports[`snippet: #25 [typescript] format 1`] = `
+"'export' modifier cannot appear on a type member (1:16)
+> 1 | interface Foo {
+ | ^
+> 2 | export property;
+ | ^^^^^^^^^
+ 3 | }"
+`;
+
+exports[`snippet: #26 [babel-ts] format 1`] = `
+"Unexpected token, expected ";" (2:10)
+ 1 | interface Foo {
+> 2 | export [index: string]: number
+ | ^
+ 3 | }"
+`;
+
+exports[`snippet: #26 [typescript] format 1`] = `
+"'export' modifier cannot appear on an index signature (1:16)
+> 1 | interface Foo {
+ | ^
+> 2 | export [index: string]: number
+ | ^^^^^^^^^
+ 3 | }"
+`;
+
+exports[`snippet: #27 [babel-ts] format 1`] = `
+"Unexpected token, expected ";" (2:10)
+ 1 | const foo: {
+> 2 | export [index: string] : string
+ | ^
+ 3 | } = {};"
+`;
+
+exports[`snippet: #27 [typescript] format 1`] = `
+"'export' modifier cannot appear on an index signature (1:13)
+> 1 | const foo: {
+ | ^
+> 2 | export [index: string] : string
+ | ^^^^^^^^^
+ 3 | } = {};"
+`;
+
+exports[`snippet: #28 [babel-ts] format 1`] = `
+"'static' modifier cannot appear on a type member. (2:3)
+ 1 | interface Foo {
+> 2 | static method();
+ | ^
+ 3 | }"
+`;
+
+exports[`snippet: #28 [typescript] format 1`] = `
+"'static' modifier cannot appear on a type member (1:16)
+> 1 | interface Foo {
+ | ^
+> 2 | static method();
+ | ^^^^^^^^^
+ 3 | }"
+`;
+
+exports[`snippet: #29 [babel-ts] format 1`] = `
+"'static' modifier cannot appear on a type member. (2:3)
+ 1 | interface Foo {
+> 2 | static property;
+ | ^
+ 3 | }"
+`;
+
+exports[`snippet: #29 [typescript] format 1`] = `
+"'static' modifier cannot appear on a type member (1:16)
+> 1 | interface Foo {
+ | ^
+> 2 | static property;
+ | ^^^^^^^^^
+ 3 | }"
+`;
+
+exports[`snippet: #30 [babel-ts] format 1`] = `
+"'static' modifier cannot appear on a type member. (2:3)
+ 1 | interface Foo {
+> 2 | static [index: string]: number
+ | ^
+ 3 | }"
+`;
+
+exports[`snippet: #30 [typescript] format 1`] = `
+"'static' modifier cannot appear on an index signature (1:16)
+> 1 | interface Foo {
+ | ^
+> 2 | static [index: string]: number
+ | ^^^^^^^^^
+ 3 | }"
+`;
+
+exports[`snippet: #31 [babel-ts] format 1`] = `
+"'static' modifier cannot appear on a type member. (2:3)
+ 1 | const foo: {
+> 2 | static [index: string] : string
+ | ^
+ 3 | } = {};"
+`;
+
+exports[`snippet: #31 [typescript] format 1`] = `
+"'static' modifier cannot appear on an index signature (1:13)
+> 1 | const foo: {
+ | ^
+> 2 | static [index: string] : string
+ | ^^^^^^^^^
+ 3 | } = {};"
+`;
+
+exports[`snippet: #32 [babel-ts] format 1`] = `
+"Unexpected token, expected ";" (2:6)
+ 1 | interface Foo {
+> 2 | in method();
+ | ^
+ 3 | }"
+`;
+
+exports[`snippet: #32 [typescript] format 1`] = `
+"'in' modifier cannot appear on a type member (1:16)
+> 1 | interface Foo {
+ | ^
+> 2 | in method();
+ | ^^^^^
+ 3 | }"
+`;
+
+exports[`snippet: #33 [babel-ts] format 1`] = `
+"Unexpected token, expected ";" (2:6)
+ 1 | interface Foo {
+> 2 | in property;
+ | ^
+ 3 | }"
+`;
+
+exports[`snippet: #33 [typescript] format 1`] = `
+"'in' modifier cannot appear on a type member (1:16)
+> 1 | interface Foo {
+ | ^
+> 2 | in property;
+ | ^^^^^
+ 3 | }"
+`;
+
+exports[`snippet: #34 [babel-ts] format 1`] = `
+"Unexpected token, expected ";" (2:6)
+ 1 | interface Foo {
+> 2 | in [index: string]: number
+ | ^
+ 3 | }"
+`;
+
+exports[`snippet: #34 [typescript] format 1`] = `
+"'in' modifier cannot appear on an index signature (1:16)
+> 1 | interface Foo {
+ | ^
+> 2 | in [index: string]: number
+ | ^^^^^
+ 3 | }"
+`;
+
+exports[`snippet: #35 [babel-ts] format 1`] = `
+"Unexpected token, expected ";" (2:6)
+ 1 | const foo: {
+> 2 | in [index: string] : string
+ | ^
+ 3 | } = {};"
+`;
+
+exports[`snippet: #35 [typescript] format 1`] = `
+"'in' modifier cannot appear on an index signature (1:13)
+> 1 | const foo: {
+ | ^
+> 2 | in [index: string] : string
+ | ^^^^^
+ 3 | } = {};"
+`;
+
+exports[`snippet: #36 [babel-ts] format 1`] = `
+"Unexpected token, expected ";" (2:7)
+ 1 | interface Foo {
+> 2 | out method();
+ | ^
+ 3 | }"
+`;
+
+exports[`snippet: #36 [typescript] format 1`] = `
+"'out' modifier cannot appear on a type member (1:16)
+> 1 | interface Foo {
+ | ^
+> 2 | out method();
+ | ^^^^^^
+ 3 | }"
+`;
+
+exports[`snippet: #37 [babel-ts] format 1`] = `
+"Unexpected token, expected ";" (2:7)
+ 1 | interface Foo {
+> 2 | out property;
+ | ^
+ 3 | }"
+`;
+
+exports[`snippet: #37 [typescript] format 1`] = `
+"'out' modifier cannot appear on a type member (1:16)
+> 1 | interface Foo {
+ | ^
+> 2 | out property;
+ | ^^^^^^
+ 3 | }"
+`;
+
+exports[`snippet: #38 [babel-ts] format 1`] = `
+"Unexpected token, expected ";" (2:7)
+ 1 | interface Foo {
+> 2 | out [index: string]: number
+ | ^
+ 3 | }"
+`;
+
+exports[`snippet: #38 [typescript] format 1`] = `
+"'out' modifier cannot appear on an index signature (1:16)
+> 1 | interface Foo {
+ | ^
+> 2 | out [index: string]: number
+ | ^^^^^^
+ 3 | }"
+`;
+
+exports[`snippet: #39 [babel-ts] format 1`] = `
+"Unexpected token, expected ";" (2:7)
+ 1 | const foo: {
+> 2 | out [index: string] : string
+ | ^
+ 3 | } = {};"
+`;
+
+exports[`snippet: #39 [typescript] format 1`] = `
+"'out' modifier cannot appear on an index signature (1:13)
+> 1 | const foo: {
+ | ^
+> 2 | out [index: string] : string
+ | ^^^^^^
+ 3 | } = {};"
+`;
+
+exports[`snippet: #40 [babel-ts] format 1`] = `
+"'override' modifier cannot appear on a type member. (2:3)
+ 1 | interface Foo {
+> 2 | override method();
+ | ^
+ 3 | }"
+`;
+
+exports[`snippet: #40 [typescript] format 1`] = `
+"'override' modifier cannot appear on a type member (1:16)
+> 1 | interface Foo {
+ | ^
+> 2 | override method();
+ | ^^^^^^^^^^^
+ 3 | }"
+`;
+
+exports[`snippet: #41 [babel-ts] format 1`] = `
+"'override' modifier cannot appear on a type member. (2:3)
+ 1 | interface Foo {
+> 2 | override property;
+ | ^
+ 3 | }"
+`;
+
+exports[`snippet: #41 [typescript] format 1`] = `
+"'override' modifier cannot appear on a type member (1:16)
+> 1 | interface Foo {
+ | ^
+> 2 | override property;
+ | ^^^^^^^^^^^
+ 3 | }"
+`;
+
+exports[`snippet: #42 [babel-ts] format 1`] = `
+"'override' modifier cannot appear on a type member. (2:3)
+ 1 | interface Foo {
+> 2 | override [index: string]: number
+ | ^
+ 3 | }"
+`;
+
+exports[`snippet: #42 [typescript] format 1`] = `
+"'override' modifier cannot appear on an index signature (1:16)
+> 1 | interface Foo {
+ | ^
+> 2 | override [index: string]: number
+ | ^^^^^^^^^^^
+ 3 | }"
+`;
+
+exports[`snippet: #43 [babel-ts] format 1`] = `
+"'override' modifier cannot appear on a type member. (2:3)
+ 1 | const foo: {
+> 2 | override [index: string] : string
+ | ^
+ 3 | } = {};"
+`;
+
+exports[`snippet: #43 [typescript] format 1`] = `
+"'override' modifier cannot appear on an index signature (1:13)
+> 1 | const foo: {
+ | ^
+> 2 | override [index: string] : string
+ | ^^^^^^^^^^^
+ 3 | } = {};"
+`;
+
+exports[`snippet: #44 [babel-ts] format 1`] = `
+"'public' modifier cannot appear on a type member. (2:3)
+ 1 | interface Foo {
+> 2 | public method();
+ | ^
+ 3 | }"
+`;
+
+exports[`snippet: #44 [typescript] format 1`] = `
+"'public' modifier cannot appear on a type member (1:16)
+> 1 | interface Foo {
+ | ^
+> 2 | public method();
+ | ^^^^^^^^^
+ 3 | }"
+`;
+
+exports[`snippet: #45 [babel-ts] format 1`] = `
+"'public' modifier cannot appear on a type member. (2:3)
+ 1 | interface Foo {
+> 2 | public property;
+ | ^
+ 3 | }"
+`;
+
+exports[`snippet: #45 [typescript] format 1`] = `
+"'public' modifier cannot appear on a type member (1:16)
+> 1 | interface Foo {
+ | ^
+> 2 | public property;
+ | ^^^^^^^^^
+ 3 | }"
+`;
+
+exports[`snippet: #46 [babel-ts] format 1`] = `
+"'public' modifier cannot appear on a type member. (2:3)
+ 1 | interface Foo {
+> 2 | public [index: string]: number
+ | ^
+ 3 | }"
+`;
+
+exports[`snippet: #46 [typescript] format 1`] = `
+"'public' modifier cannot appear on an index signature (1:16)
+> 1 | interface Foo {
+ | ^
+> 2 | public [index: string]: number
+ | ^^^^^^^^^
+ 3 | }"
+`;
+
+exports[`snippet: #47 [babel-ts] format 1`] = `
+"'public' modifier cannot appear on a type member. (2:3)
+ 1 | const foo: {
+> 2 | public [index: string] : string
+ | ^
+ 3 | } = {};"
+`;
+
+exports[`snippet: #47 [typescript] format 1`] = `
+"'public' modifier cannot appear on an index signature (1:13)
+> 1 | const foo: {
+ | ^
+> 2 | public [index: string] : string
+ | ^^^^^^^^^
+ 3 | } = {};"
+`;
+
+exports[`snippet: #48 [babel-ts] format 1`] = `
+"'private' modifier cannot appear on a type member. (2:3)
+ 1 | interface Foo {
+> 2 | private method();
+ | ^
+ 3 | }"
+`;
+
+exports[`snippet: #48 [typescript] format 1`] = `
+"'private' modifier cannot appear on a type member (1:16)
+> 1 | interface Foo {
+ | ^
+> 2 | private method();
+ | ^^^^^^^^^^
+ 3 | }"
+`;
+
+exports[`snippet: #49 [babel-ts] format 1`] = `
+"'private' modifier cannot appear on a type member. (2:3)
+ 1 | interface Foo {
+> 2 | private property;
+ | ^
+ 3 | }"
+`;
+
+exports[`snippet: #49 [typescript] format 1`] = `
+"'private' modifier cannot appear on a type member (1:16)
+> 1 | interface Foo {
+ | ^
+> 2 | private property;
+ | ^^^^^^^^^^
+ 3 | }"
+`;
+
+exports[`snippet: #50 [babel-ts] format 1`] = `
+"'private' modifier cannot appear on a type member. (2:3)
+ 1 | interface Foo {
+> 2 | private [index: string]: number
+ | ^
+ 3 | }"
+`;
+
+exports[`snippet: #50 [typescript] format 1`] = `
+"'private' modifier cannot appear on an index signature (1:16)
+> 1 | interface Foo {
+ | ^
+> 2 | private [index: string]: number
+ | ^^^^^^^^^^
+ 3 | }"
+`;
+
+exports[`snippet: #51 [babel-ts] format 1`] = `
+"'private' modifier cannot appear on a type member. (2:3)
+ 1 | const foo: {
+> 2 | private [index: string] : string
+ | ^
+ 3 | } = {};"
+`;
+
+exports[`snippet: #51 [typescript] format 1`] = `
+"'private' modifier cannot appear on an index signature (1:13)
+> 1 | const foo: {
+ | ^
+> 2 | private [index: string] : string
+ | ^^^^^^^^^^
+ 3 | } = {};"
+`;
+
+exports[`snippet: #52 [babel-ts] format 1`] = `
+"'protected' modifier cannot appear on a type member. (2:3)
+ 1 | interface Foo {
+> 2 | protected method();
+ | ^
+ 3 | }"
+`;
+
+exports[`snippet: #52 [typescript] format 1`] = `
+"'protected' modifier cannot appear on a type member (1:16)
+> 1 | interface Foo {
+ | ^
+> 2 | protected method();
+ | ^^^^^^^^^^^^
+ 3 | }"
+`;
+
+exports[`snippet: #53 [babel-ts] format 1`] = `
+"'protected' modifier cannot appear on a type member. (2:3)
+ 1 | interface Foo {
+> 2 | protected property;
+ | ^
+ 3 | }"
+`;
+
+exports[`snippet: #53 [typescript] format 1`] = `
+"'protected' modifier cannot appear on a type member (1:16)
+> 1 | interface Foo {
+ | ^
+> 2 | protected property;
+ | ^^^^^^^^^^^^
+ 3 | }"
+`;
+
+exports[`snippet: #54 [babel-ts] format 1`] = `
+"'protected' modifier cannot appear on a type member. (2:3)
+ 1 | interface Foo {
+> 2 | protected [index: string]: number
+ | ^
+ 3 | }"
+`;
+
+exports[`snippet: #54 [typescript] format 1`] = `
+"'protected' modifier cannot appear on an index signature (1:16)
+> 1 | interface Foo {
+ | ^
+> 2 | protected [index: string]: number
+ | ^^^^^^^^^^^^
+ 3 | }"
+`;
+
+exports[`snippet: #55 [babel-ts] format 1`] = `
+"'protected' modifier cannot appear on a type member. (2:3)
+ 1 | const foo: {
+> 2 | protected [index: string] : string
+ | ^
+ 3 | } = {};"
+`;
+
+exports[`snippet: #55 [typescript] format 1`] = `
+"'protected' modifier cannot appear on an index signature (1:13)
+> 1 | const foo: {
+ | ^
+> 2 | protected [index: string] : string
+ | ^^^^^^^^^^^^
+ 3 | } = {};"
+`;
+
+exports[`snippet: #56 [babel-ts] format 1`] = `
+"'readonly' modifier can only appear on a property declaration or index signature. (2:3)
+ 1 | interface Foo {
+> 2 | readonly method();
+ | ^
+ 3 | }"
+`;
+
+exports[`snippet: #56 [typescript] format 1`] = `
+"'readonly' modifier can only appear on a property declaration or index signature. (1:16)
+> 1 | interface Foo {
+ | ^
+> 2 | readonly method();
+ | ^^^^^^^^^^^
+ 3 | }"
+`;
+
+exports[`snippet: #57 [babel-ts] format 1`] = `
+"'abstract' modifier cannot appear on a type parameter. (1:15)
+> 1 | interface Foo<abstract T> {}
+ | ^"
+`;
+
+exports[`snippet: #57 [typescript] format 1`] = `
+"'abstract' modifier cannot appear on a type parameter (1:15)
+> 1 | interface Foo<abstract T> {}
+ | ^^^^^^^^"
+`;
+
+exports[`snippet: #58 [babel-ts] format 1`] = `
+"Unexpected token, expected "," (1:24)
+> 1 | interface Foo<accessor T> {}
+ | ^"
+`;
+
+exports[`snippet: #58 [typescript] format 1`] = `
+"'accessor' modifier cannot appear on a type parameter (1:15)
+> 1 | interface Foo<accessor T> {}
+ | ^^^^^^^^"
+`;
+
+exports[`snippet: #59 [babel-ts] format 1`] = `
+"Unexpected token, expected "," (1:21)
+> 1 | interface Foo<async T> {}
+ | ^"
+`;
+
+exports[`snippet: #59 [typescript] format 1`] = `
+"'async' modifier cannot appear on a type parameter (1:15)
+> 1 | interface Foo<async T> {}
+ | ^^^^^"
+`;
+
+exports[`snippet: #60 [babel-ts] format 1`] = `
+"Unexpected token, expected "," (1:21)
+> 1 | interface Foo<const T> {}
+ | ^"
+`;
+
+exports[`snippet: #60 [typescript] format 1`] = `
+"Type parameter declaration expected. (1:15)
+> 1 | interface Foo<const T> {}
+ | ^"
+`;
+
+exports[`snippet: #61 [babel-ts] format 1`] = `
+"'declare' modifier cannot appear on a type parameter. (1:15)
+> 1 | interface Foo<declare T> {}
+ | ^"
+`;
+
+exports[`snippet: #61 [typescript] format 1`] = `
+"'declare' modifier cannot appear on a type parameter (1:15)
+> 1 | interface Foo<declare T> {}
+ | ^^^^^^^"
+`;
+
+exports[`snippet: #62 [babel-ts] format 1`] = `
+"Unexpected token, expected "," (1:23)
+> 1 | interface Foo<default T> {}
+ | ^"
+`;
+
+exports[`snippet: #62 [typescript] format 1`] = `
+"Type parameter declaration expected. (1:15)
+> 1 | interface Foo<default T> {}
+ | ^"
+`;
+
+exports[`snippet: #63 [babel-ts] format 1`] = `
+"Unexpected token, expected "," (1:22)
+> 1 | interface Foo<export T> {}
+ | ^"
+`;
+
+exports[`snippet: #63 [typescript] format 1`] = `
+"Type parameter declaration expected. (1:15)
+> 1 | interface Foo<export T> {}
+ | ^"
+`;
+
+exports[`snippet: #64 [babel-ts] format 1`] = `
+"Unexpected token, expected "," (1:22)
+> 1 | interface Foo<static T> {}
+ | ^"
+`;
+
+exports[`snippet: #64 [typescript] format 1`] = `
+"'static' modifier cannot appear on a type parameter (1:15)
+> 1 | interface Foo<static T> {}
+ | ^^^^^^"
+`;
+
+exports[`snippet: #65 [babel-ts] format 1`] = `
+"'override' modifier cannot appear on a type parameter. (1:15)
+> 1 | interface Foo<override T> {}
+ | ^"
+`;
+
+exports[`snippet: #65 [typescript] format 1`] = `
+"'override' modifier cannot appear on a type parameter (1:15)
+> 1 | interface Foo<override T> {}
+ | ^^^^^^^^"
+`;
+
+exports[`snippet: #66 [babel-ts] format 1`] = `
+"'public' modifier cannot appear on a type parameter. (1:15)
+> 1 | interface Foo<public T> {}
+ | ^"
+`;
+
+exports[`snippet: #66 [typescript] format 1`] = `
+"'public' modifier cannot appear on a type parameter (1:15)
+> 1 | interface Foo<public T> {}
+ | ^^^^^^"
+`;
+
+exports[`snippet: #67 [babel-ts] format 1`] = `
+"'private' modifier cannot appear on a type parameter. (1:15)
+> 1 | interface Foo<private T> {}
+ | ^"
+`;
+
+exports[`snippet: #67 [typescript] format 1`] = `
+"'private' modifier cannot appear on a type parameter (1:15)
+> 1 | interface Foo<private T> {}
+ | ^^^^^^^"
+`;
+
+exports[`snippet: #68 [babel-ts] format 1`] = `
+"'protected' modifier cannot appear on a type parameter. (1:15)
+> 1 | interface Foo<protected T> {}
+ | ^"
+`;
+
+exports[`snippet: #68 [typescript] format 1`] = `
+"'protected' modifier cannot appear on a type parameter (1:15)
+> 1 | interface Foo<protected T> {}
+ | ^^^^^^^^^"
+`;
+
+exports[`snippet: #69 [babel-ts] format 1`] = `
+"'readonly' modifier cannot appear on a type parameter. (1:15)
+> 1 | interface Foo<readonly T> {}
+ | ^"
+`;
+
+exports[`snippet: #69 [typescript] format 1`] = `
+"'readonly' modifier cannot appear on a type parameter (1:15)
+> 1 | interface Foo<readonly T> {}
+ | ^^^^^^^^"
+`;
+
+exports[`snippet: #70 [babel-ts] format 1`] = `
+"Class methods cannot have the 'declare' modifier. (2:3)
+ 1 | class Foo {
+> 2 | declare method() {}
+ | ^
+ 3 | }"
+`;
+
+exports[`snippet: #70 [typescript] format 1`] = `
+"'declare' modifier cannot appear on class elements of this kind. (1:12)
+> 1 | class Foo {
+ | ^
+> 2 | declare method() {}
+ | ^^^^^^^^^^
+ 3 | }"
+`;
+
+exports[`snippet: #71 [babel-ts] format 1`] = `
+"Class methods cannot have the 'readonly' modifier. (2:3)
+ 1 | class Foo {
+> 2 | readonly method() {}
+ | ^
+ 3 | }"
+`;
+
+exports[`snippet: #71 [typescript] format 1`] = `
+"'readonly' modifier can only appear on a property declaration or index signature. (1:12)
+> 1 | class Foo {
+ | ^
+> 2 | readonly method() {}
+ | ^^^^^^^^^^^
+ 3 | }"
+`;
+
+exports[`snippet: #72 [babel-ts] format 1`] = `
+"'declare' is not allowed in getters. (2:3)
+ 1 | class Foo {
+> 2 | declare get getter() {}
+ | ^
+ 3 | }"
+`;
+
+exports[`snippet: #72 [typescript] format 1`] = `
+"'declare' modifier cannot appear on class elements of this kind. (1:12)
+> 1 | class Foo {
+ | ^
+> 2 | declare get getter() {}
+ | ^^^^^^^^^^
+ 3 | }"
+`;
+
+exports[`snippet: #73 [babel-ts] format 1`] = `
+"'declare' is not allowed in setters. (2:3)
+ 1 | class Foo {
+> 2 | declare set setter(v) {}
+ | ^
+ 3 | }"
+`;
+
+exports[`snippet: #73 [typescript] format 1`] = `
+"'declare' modifier cannot appear on class elements of this kind. (1:12)
+> 1 | class Foo {
+ | ^
+> 2 | declare set setter(v) {}
+ | ^^^^^^^^^^
+ 3 | }"
+`;
diff --git a/tests/format/misc/errors/typescript/modifiers/jsfmt.spec.js b/tests/format/misc/errors/typescript/modifiers/jsfmt.spec.js
new file mode 100644
--- /dev/null
+++ b/tests/format/misc/errors/typescript/modifiers/jsfmt.spec.js
@@ -0,0 +1,93 @@
+import { outdent } from "outdent";
+
+const POSSIBLE_MODIFIERS = [
+ "abstract",
+ "accessor",
+ "async",
+ "const",
+ "declare",
+ "default",
+ "export",
+ "static",
+ "in",
+ "out",
+ "override",
+ "public",
+ "private",
+ "protected",
+ "readonly",
+];
+
+run_spec(
+ {
+ importMeta: import.meta,
+ snippets: [
+ // Only `readonly` allowed in some places
+ ...POSSIBLE_MODIFIERS.filter(
+ (modifier) => modifier !== "readonly"
+ ).flatMap((modifier) => [
+ outdent`
+ interface Foo {
+ ${modifier} method();
+ }
+ `,
+ outdent`
+ interface Foo {
+ ${modifier} property;
+ }
+ `,
+ // index signature
+ outdent`
+ interface Foo {
+ ${modifier} [index: string]: number
+ }
+ `,
+ outdent`
+ const foo: {
+ ${modifier} [index: string] : string
+ } = {};
+ `,
+ ]),
+ outdent`
+ interface Foo {
+ readonly method();
+ }
+ `,
+
+ // TODO[@fisker]: Fix these tests
+ // ...["abstract", "static", "private", "protected", "public"].map(
+ // (modifier) =>
+ // outdent`
+ // module Foo {
+ // ${modifier} module Bar {}
+ // }
+ // `
+ // ),
+
+ // Only `in` and `out` allowed in type parameter
+ ...POSSIBLE_MODIFIERS.filter(
+ (modifier) => modifier !== "in" && modifier !== "out"
+ ).map((modifier) => `interface Foo<${modifier} T> {}`),
+
+ ...["declare", "readonly"].map(
+ (modifier) =>
+ outdent`
+ class Foo {
+ ${modifier} method() {}
+ }
+ `
+ ),
+ outdent`
+ class Foo {
+ declare get getter() {}
+ }
+ `,
+ outdent`
+ class Foo {
+ declare set setter(v) {}
+ }
+ `,
+ ],
+ },
+ ["babel-ts", "typescript"]
+);
diff --git a/tests/format/misc/typescript-babel-only/__snapshots__/jsfmt.spec.js.snap b/tests/format/misc/typescript-babel-only/__snapshots__/jsfmt.spec.js.snap
--- a/tests/format/misc/typescript-babel-only/__snapshots__/jsfmt.spec.js.snap
+++ b/tests/format/misc/typescript-babel-only/__snapshots__/jsfmt.spec.js.snap
@@ -20,26 +20,6 @@ class C {
================================================================================
`;
-exports[`invalid-modifiers.ts format 1`] = `
-====================================options=====================================
-parsers: ["babel-ts"]
-printWidth: 80
- | printWidth
-=====================================input======================================
-class Bar {
- declare e() {};
- readonly g() {};
-}
-
-=====================================output=====================================
-class Bar {
- declare e() {}
- readonly g() {}
-}
-
-================================================================================
-`;
-
exports[`parenthesized-decorators-call-expression.ts format 1`] = `
====================================options=====================================
parsers: ["babel-ts"]
diff --git a/tests/format/misc/typescript-babel-only/invalid-modifiers.ts b/tests/format/misc/typescript-babel-only/invalid-modifiers.ts
deleted file mode 100644
--- a/tests/format/misc/typescript-babel-only/invalid-modifiers.ts
+++ /dev/null
@@ -1,4 +0,0 @@
-class Bar {
- declare e() {};
- readonly g() {};
-}
diff --git a/tests/format/misc/typescript-only/__snapshots__/jsfmt.spec.js.snap b/tests/format/misc/typescript-only/__snapshots__/jsfmt.spec.js.snap
--- a/tests/format/misc/typescript-only/__snapshots__/jsfmt.spec.js.snap
+++ b/tests/format/misc/typescript-only/__snapshots__/jsfmt.spec.js.snap
@@ -51,28 +51,6 @@ await 0;
================================================================================
`;
-exports[`invalid-modifiers.ts format 1`] = `
-====================================options=====================================
-parsers: ["typescript"]
-printWidth: 80
- | printWidth
-=====================================input======================================
-class Bar {
- declare e() {};
- abstract f() {};
- readonly g() {};
-}
-
-=====================================output=====================================
-class Bar {
- e() {}
- abstract f() {}
- g() {}
-}
-
-================================================================================
-`;
-
exports[`parenthesized-decorators-call-expression.ts format 1`] = `
====================================options=====================================
parsers: ["typescript"]
diff --git a/tests/format/misc/typescript-only/invalid-modifiers.ts b/tests/format/misc/typescript-only/invalid-modifiers.ts
deleted file mode 100644
--- a/tests/format/misc/typescript-only/invalid-modifiers.ts
+++ /dev/null
@@ -1,5 +0,0 @@
-class Bar {
- declare e() {};
- abstract f() {};
- readonly g() {};
-}
diff --git a/tests/format/typescript/keywords/__snapshots__/jsfmt.spec.js.snap b/tests/format/typescript/keywords/__snapshots__/jsfmt.spec.js.snap
--- a/tests/format/typescript/keywords/__snapshots__/jsfmt.spec.js.snap
+++ b/tests/format/typescript/keywords/__snapshots__/jsfmt.spec.js.snap
@@ -1,14 +1,14 @@
// Jest Snapshot v1, https://goo.gl/fbAQLP
exports[`keywords.ts [babel-ts] format 1`] = `
-"Unexpected token, expected "{" (9:12)
- 7 |
- 8 | // Apparently this parses :P
-> 9 | export private public protected static readonly abstract async enum X { }
- | ^
- 10 |
- 11 | interface x {
- 12 | export private static readonly [x: any]: any;"
+"Missing semicolon. (4:11)
+ 2 |
+ 3 | module Y4 {
+> 4 | public enum Color { Blue, Red }
+ | ^
+ 5 | }
+ 6 |
+ 7 | module YY3 {"
`;
exports[`keywords.ts format 1`] = `
@@ -19,19 +19,6 @@ printWidth: 80
=====================================input======================================
// All of these should be an error
-module Y3 {
- public module Module {
- class A { s: string }
- }
-
- // Apparently this parses :P
- export private public protected static readonly abstract async enum X { }
-
- interface x {
- export private static readonly [x: any]: any;
- }
-}
-
module Y4 {
public enum Color { Blue, Red }
}
@@ -59,21 +46,6 @@ module YYY4 {
=====================================output=====================================
// All of these should be an error
-module Y3 {
- public module Module {
- class A {
- s: string;
- }
- }
-
- // Apparently this parses :P
- export private public protected static readonly abstract async enum X {}
-
- interface x {
- export private static readonly [x: any]: any;
- }
-}
-
module Y4 {
public enum Color {
Blue,
@@ -195,17 +167,6 @@ class G {
================================================================================
`;
-exports[`module.ts [babel-ts] format 1`] = `
-"Unexpected token, expected "{" (7:10)
- 5 |
- 6 | // Apparently this parses :P
-> 7 | export private public protected static readonly abstract async enum X { }
- | ^
- 8 |
- 9 | interface x {
- 10 | export private static readonly [x: any]: any;"
-`;
-
exports[`module.ts format 1`] = `
====================================options=====================================
parsers: ["typescript"]
@@ -213,31 +174,29 @@ printWidth: 80
| printWidth
=====================================input======================================
module Y3 {
- public module Module {
+ module Module {
class A { s: string }
}
- // Apparently this parses :P
- export private public protected static readonly abstract async enum X { }
+ export enum X { }
interface x {
- export private static readonly [x: any]: any;
+ readonly [x: any]: any;
}
}
=====================================output=====================================
module Y3 {
- public module Module {
+ module Module {
class A {
s: string;
}
}
- // Apparently this parses :P
- export private public protected static readonly abstract async enum X {}
+ export enum X {}
interface x {
- export private static readonly [x: any]: any;
+ readonly [x: any]: any;
}
}
diff --git a/tests/format/typescript/keywords/jsfmt.spec.js b/tests/format/typescript/keywords/jsfmt.spec.js
--- a/tests/format/typescript/keywords/jsfmt.spec.js
+++ b/tests/format/typescript/keywords/jsfmt.spec.js
@@ -1,3 +1,3 @@
run_spec(import.meta, ["typescript"], {
- errors: { "babel-ts": ["module.ts", "keywords.ts"] },
+ errors: { "babel-ts": ["keywords.ts"] },
});
diff --git a/tests/format/typescript/keywords/keywords.ts b/tests/format/typescript/keywords/keywords.ts
--- a/tests/format/typescript/keywords/keywords.ts
+++ b/tests/format/typescript/keywords/keywords.ts
@@ -1,18 +1,5 @@
// All of these should be an error
-module Y3 {
- public module Module {
- class A { s: string }
- }
-
- // Apparently this parses :P
- export private public protected static readonly abstract async enum X { }
-
- interface x {
- export private static readonly [x: any]: any;
- }
-}
-
module Y4 {
public enum Color { Blue, Red }
}
diff --git a/tests/format/typescript/keywords/module.ts b/tests/format/typescript/keywords/module.ts
--- a/tests/format/typescript/keywords/module.ts
+++ b/tests/format/typescript/keywords/module.ts
@@ -1,12 +1,11 @@
module Y3 {
- public module Module {
+ module Module {
class A { s: string }
}
- // Apparently this parses :P
- export private public protected static readonly abstract async enum X { }
+ export enum X { }
interface x {
- export private static readonly [x: any]: any;
+ readonly [x: any]: any;
}
}
| Missing `readonly` and `declare` in `ClassMethod` and `TSMethodSignature`
**Prettier 2.2.1**
[Playground link](https://prettier.io/playground/#N4Igxg9gdgLgprEAucAbAhgZ0wAgMI7AA6UOOATnOgCbSoCeOAVHABQCUhAviWdXGAyVmAMw7cSPKCQCWsOORHowcHADEIEQrxz9B6YW3YBuHegBGmGOWUwcYk5JAAaEBAAOMGdEzJQB8ggAdwAFAwRfFHRUIPR6X1dzGzAAazgYAGV3ZTkAc2RrAFc4VzgAW3M4an5qABl0KFzC9Fy4DXIy9BgvRuQQdEKYCBcQAAsYMtQAdVGZeExslQyIuZkANzn6PrBsEblMBRgQm1zO5CVUA9cAK0wADwAhZLTM9DK4Wrk4c+irkFu7hk8qg4ABFQoQeA-S4lEDZcgHch9GD0dxwTBgcgyTwjdxY2BTGTUGCjZAADgADK48RADlMbO4+nj0Qo1t9XABHCHwY4eSL9TAAWigcCqVRGlC5MkoxxaZyQFz+BzKMgK5GKrkwwLB3O+Ct+sJgFkJxNJSAATK5rOgZKg8ngIGV5SB0QBWEaFA4AFQskUVsLWxQAklB+LAMpjsTAAIKhjIokHQg5cLhAA)
<!-- prettier-ignore -->
```sh
--parser typescript
```
**Input:**
<!-- prettier-ignore -->
```tsx
class C {
readonly *e() {}
declare *f() {}
}
interface Foo {
declare e();
abstract f();
}
```
**Output:**
<!-- prettier-ignore -->
```tsx
class C {
*e() {}
*f() {}
}
interface Foo {
e();
f();
}
```
**Expected behavior:**
```tsx
class C {
readonly *e() {}
declare *f() {}
}
interface Foo {
declare e();
abstract f();
}
```
---
Update
**Expected behavior:**
Throw SyntaxError.
| > [Playground link](https://prettier.io/playground/#N4Igxg9gdgLgprEAucAbAhgZ0wAgMI7AA6UOOATnOgCbSoCeOAVHABQCUhAviWdXGAyVmAMw7cSXEABoQEAA4wAltEzJQ6cuQgB3AAqaEalOlQ709NbIBG5dGADWcGAGV59pVADmyGOQCucLJwALbWcNT81AAy6N7+6F5wAGIQ5CHoMMreyCDo-jAQMiAAFjAhqADqJUrwmO5gcC5GtUoAbrX0uWDYxZ6YcOQwenZeGcgipgOyAFaYAB4AQnaOzi7oIXDRnnATU0Egc-Munl6ocACK-hDwe6jTIO7kA+S5MPTycJhg5EqKxfJfrBKkpqDASsgABwABlkgIgA0qdnkuUBX0GbV2sgAjtd4CMFMY8pgALRQOARCLFSi4pSUEaJcZISb3A4DEJKXwBNmnc5XG67Zn7WQwdDWEFgiFIABMIrsSlQpzwEBCTJAXwArMV-AMACpi4wsh5tQIASSg-FgLh+fxgAEELS53uc7gMuFwgA)
Same output comes from both `typescript` and `babel-ts` parsers (other JavaScript parsers signal a syntax error).
Background is in PR #9748 & <https://github.com/typescript-eslint/typescript-eslint/issues/2908>.
I gather that the testing with AST_COMPARE=1 does not show an issue because the attributes are not present in the AST coming either from` babel-ts` or `typescript` parser.
> I gather that the testing with AST_COMPARE=1 does not show an issue because the attributes are not present in the AST
https://github.com/prettier/prettier/pull/9768
Invalid `declare` on accessors not throwing, should be the same problem. `babel-ts` fixed this in #10750.
**Prettier 2.2.1**
[Playground link](https://prettier.io/playground/#N4Igxg9gdgLgprEAucAbAhgZ0wAgGIQQ7AA6UOOAJnGBgE5w4DmcMOAZoQBQCUZF1WugY5MrDtwBufKAF8QAGhAQADjACW0TMlDC6EAO4AFYQm0p0qA+gCe2pQCM66MAGtWAZRUv1UJshg6AFc4JTgAWwc4SmpKABl0PyD0FgI6cPQYDT9kEHQgmAhFEAALGHDUAHUS9XhMbzA4DzNa9Ulam1ywbGLfMToYI2cmDOR2SzElACtMAA8AIWc3T3RwuDjfODGJ0JAZ2Y9fJlQ4AEUgiHht1EmQbzp+3JgbFThMMDp1NWKVT9hK9SUGAlZAADgADEpfhAxJVnCpcr83nA6JItkoAI4XeBDVTmPKYAC0UDg0WixQYWPUDCGKVGSHGN12YnC6gCwWZRxO50uWwZOyUMHQDgBQJBSAATILnOpUEcAMIQcL0kBvACsxSCYgAKsLzIzbpIQgBJKDUWAeD5fGAAQTNHmeJ2uYlksiAA)
<!-- prettier-ignore -->
```sh
--parser typescript
```
**Input:**
<!-- prettier-ignore -->
```tsx
class Foo {
declare get foo()
declare set foo(v)
}
```
**Output:**
<!-- prettier-ignore -->
```tsx
class Foo {
get foo();
set foo(v);
}
``` | "2022-11-21T09:21:29Z" | 3.0 | [
"tests/format/misc/errors/typescript/babel-ts/jsfmt.spec.js",
"tests/format/typescript/keywords/jsfmt.spec.js",
"tests/format/misc/typescript-only/jsfmt.spec.js",
"tests/format/misc/typescript-babel-only/jsfmt.spec.js"
] | [
"tests/format/misc/errors/typescript/modifiers/jsfmt.spec.js",
"tests/format/misc/errors/typescript/jsfmt.spec.js"
] | JavaScript | [] | [
"https://prettier.io/playground/#N4Igxg9gdgLgprEAucAbAhgZ0wAgMI7AA6UOOATnOgCbSoCeOAVHABQCUhAviWdXGAyVmAMw7cSPKCQCWsOORHowcHADEIEQrxz9B6YW3YBuHegBGmGOWUwcYk5JAAaEBAAOMGdEzJQB8ggAdwAFAwRfFHRUIPR6X1dzGzAAazgYAGV3ZTkAc2RrAFc4VzgAW3M4an5qABl0KFzC9Fy4DXIy9BgvRuQQdEKYCBcQAAsYMtQAdVGZeExslQyIuZkANzn6PrBsEblMBRgQm1zO5CVUA9cAK0wADwAhZLTM9DK4Wrk4c+irkFu7hk8qg4ABFQoQeA-S4lEDZcgHch9GD0dxwTBgcgyTwjdxY2BTGTUGCjZAADgADK48RADlMbO4+nj0Qo1t9XABHCHwY4eSL9TAAWigcCqVRGlC5MkoxxaZyQFz+BzKMgK5GKrkwwLB3O+Ct+sJgFkJxNJSAATK5rOgZKg8ngIGV5SB0QBWEaFA4AFQskUVsLWxQAklB+LAMpjsTAAIKhjIokHQg5cLhAA"
] |
prettier/prettier | 14,007 | prettier__prettier-14007 | [
"11483"
] | b50dfd1974d33abb980e9b4df949913932eeb478 | diff --git a/src/language-css/printer-postcss.js b/src/language-css/printer-postcss.js
--- a/src/language-css/printer-postcss.js
+++ b/src/language-css/printer-postcss.js
@@ -101,7 +101,10 @@ function genericPrint(path, options, print) {
return [node.raw, hardline];
case "css-root": {
const nodes = printNodeSequence(path, options, print);
- const after = node.raws.after.trim();
+ let after = node.raws.after.trim();
+ if (after.startsWith(";")) {
+ after = after.slice(1).trim();
+ }
return [
nodes,
| diff --git a/tests/format/less/less/__snapshots__/jsfmt.spec.js.snap b/tests/format/less/less/__snapshots__/jsfmt.spec.js.snap
--- a/tests/format/less/less/__snapshots__/jsfmt.spec.js.snap
+++ b/tests/format/less/less/__snapshots__/jsfmt.spec.js.snap
@@ -83,6 +83,24 @@ a: b;
================================================================================
`;
+exports[`issue-11483--never-append-anything.less format 1`] = `
+====================================options=====================================
+parsers: ["less"]
+printWidth: 80
+ | printWidth
+=====================================input======================================
+@variable: {
+ field: something;
+};
+
+=====================================output=====================================
+@variable: {
+ field: something;
+};
+
+================================================================================
+`;
+
exports[`less.less format 1`] = `
====================================options=====================================
parsers: ["less"]
diff --git a/tests/format/less/less/issue-11483--never-append-anything.less b/tests/format/less/less/issue-11483--never-append-anything.less
new file mode 100644
--- /dev/null
+++ b/tests/format/less/less/issue-11483--never-append-anything.less
@@ -0,0 +1,3 @@
+@variable: {
+ field: something;
+};
| Prettier infinitely suggests additional " ;" to the end of less detached rulesets
**Prettier 2.3.2**
[Playground link](https://prettier.io/playground/#N4Igxg9gdgLgprEAuEABAbgQwE4EtMBGANnEgATAA6UZZAZrnEQCbkDOEAtnDABa5QA5tQC+AbhAAaEBAAOMXNDbJQObBADuABRwJlKTEQ2YAnsukFsmMAGseAZUzcAMgLjI6htnAtXbD2WsBQWQYbABXHxA4TgI4ZmZ450whcMxBOAAxCGxOTBgFIWQQTHCYCCkQXhhOIgB1fng2QLA4ez1cBXROk2KwNnMQAW9sGC0rQTyPLyiAKzYAD3tgkgBFcIh4aaJvaUDsEeKSAcrZPFg63GY+ZAAOAAY99W86q1lis7gR9HdpAEcNvBxnJ9CU2ABaKBweLxSrYOAA3Dw8bpKZITw7KLeTi4UIRLErODrTbudEzaQwQiXa68ZAAJgpVlwRGCAGEuGjomwAKyVcLeAAqhH0GN2IHQkQAklBErB7GA8PIAIIy+wwEwkbZi+YLABCfjsMEcLjcWrgIhEQA)
<!-- prettier-ignore -->
```sh
--parser less
```
**Input:**
<!-- prettier-ignore -->
```less
@variable: {
field: something;
};
```
**Output:**
<!-- prettier-ignore -->
```less
@variable: {
field: something;
}; ;
```
(If you test it out in the playground, another space + semicolon are always added, no matter how many are in the "input")
**Expected behavior:**
I would expect `prettier` to be satisfied with zero or one semicolon, not always suggest another. See [detached rulesets docs](https://lesscss.org/features/#detached-rulesets-feature).
| the problem is only with the last LESS ruleset: https://prettier.io/playground/#N4Igxg9gdgLgprEAuEABAHkgBMAOlAXwG58Ns9CSoyd9j8QAaECABxgEtoBnZUAQwBOgiAHcACkIS8U-ADaj+AT17MARoP5gA1nBgBlfgFs4AGQ5Q4yAGbzucdZp179rLRYDmyGIICuDkDgjNTgAE1Cw034oD19+DzgAMQhBI34YThjkEH5fGAgmEAALGCM5AHUijnhuNzA4fWlqjgA3aqVssG5VEAt7QRhxTQ80mzsAgCtudH1POTgARV8IeDG5e2Y3QX7s+e7C1kELGHKOUJgi5AAOAAZNkXtyzVZsw7h+lqtmAEdl+CG2DIctwALSWMJhQqCOC-DjQobxUZIWzrAL2Iwcbx+NFzOAAQQyRzUeTg4jggnMljWGxA3FxSxWVmR42YMH4alO50uSAATKzNBw5J4AMIQIxIwLcACshV89gAKuyZCiaS1-ABJKARWD6MBHdh4rX6GBKebUybTABCTl0BmMZgsTJVcAIBCAA
maybe caused by https://github.com/prettier/prettier/pull/5353
and https://github.com/prettier/prettier/issues/12540 slightly semicolon related issue for JS
I would appretiate any help as this issue is making the prettier output unstable and prettier impossible to be used CI.
~It seems the problem is in the `postcss` parser - https://github.com/postcss/postcss/issues/1806~
UPDATE: I did a research (and debugging on `node_modules/postcss/lib/parser.js`) and I belive the problem is here
here is a https://prettier.io/playground/#N4Igxg9gdgLgprEAuEABAbgQwE4EtMBGANnAIxIAEwAOlBRQGa5xEAmlAzhALZwwAWuKAHMA3LQC+4qLQw58xOACZKNOo2ZtOPPoJHSptEABoQEAA4xc0DslA5sEAO4AFHAlspMRJ5gCetqYE2JhgANZ8AMqYvAAyQnDIDN4ccEEh4VHmoULCyDDYAK5pIHDcBHCsrJWxmCKFmMJwAGIQ2NyYMFYiyCCYhTAQJiD8MNxEAOqC8BzZYHCRHrhW6Mt+vWAcgSBCqdgwLiHCHUkpJQBWHAAekbkkAIqFEPCnRKmm2dh7vSRbw+Z4WATXCsATIAAcAAYPo5UhMQuZegC4Ht0IlTABHJ7wQ4WTx9DgAWigcEqlWG2DgWNwlMOjROSGSbxKqW4uHyRRZdzgAEEungCAM4C44Nh4iTXu8QBxuY9nolGWdTDBCMDQfxkEplSFcERcgBhHgM0ocACsw0KqQAKoRPEypehigBJKDVWCRMB4Sw812RGB+EiSuASCRAA playground that helps to explain it
AST dump (to see the `raws` content, click `**` in the playground AST dump):
```
{
"raws": {
"semicolon": false,
"after": ";\n"
},
"type": "css-root",
"nodes": [
{
"raws": {
"before": "",
"between": " ",
"afterName": ":",
"semicolon": true,
"after": "\n",
"value": "",
"params": ":"
},
"type": "css-atrule",
"name": "variable1",
"source": {
"start": {
"line": 1,
"column": 1
},
"input": {
"css": "@variable1: {\n field: something;\n};\n\n@variable2: {\n field: something;\n};\n",
"hasBOM": false,
"id": "<input css 2>"
},
"end": {
"line": 3,
"column": 1
},
"startOffset": 0,
"endOffset": 35
},
"nodes": [
{
...
}
],
"variable": true,
"value": ""
},
{
"raws": {
"before": ";\n\n",
"between": " ",
"afterName": ":",
"semicolon": true,
"after": "\n",
"value": "",
"params": ":"
},
"type": "css-atrule",
"name": "variable2",
"source": {
"start": {
"line": 5,
"column": 1
},
"input": {
"css": "@variable1: {\n field: something;\n};\n\n@variable2: {\n field: something;\n};\n",
"hasBOM": false,
"id": "<input css 2>"
},
"end": {
"line": 7,
"column": 1
},
"startOffset": 38,
"endOffset": 73
},
"nodes": [
{
...
}
],
"variable": true,
"value": ""
}
],
"source": {
"input": {
"css": "@variable1: {\n field: something;\n};\n\n@variable2: {\n field: something;\n};\n",
"hasBOM": false,
"id": "<input css 2>"
},
"start": {
"line": 1,
"column": 1
},
"startOffset": 0,
"endOffset": 73
}
}
```
when `postless` parses `raws.semicolon: true`, it does not consume the `;` char but keeps in in `raws.after`, this is why prettier prints it twice | "2022-12-17T13:37:26Z" | 2.9 | [] | [
"tests/format/less/less/jsfmt.spec.js"
] | JavaScript | [] | [
"https://prettier.io/playground/#N4Igxg9gdgLgprEAuEABAbgQwE4EtMBGANnEgATAA6UZZAZrnEQCbkDOEAtnDABa5QA5tQC+AbhAAaEBAAOMXNDbJQObBADuABRwJlKTEQ2YAnsukFsmMAGseAZUzcAMgLjI6htnAtXbD2WsBQWQYbABXHxA4TgI4ZmZ450whcMxBOAAxCGxOTBgFIWQQTHCYCCkQXhhOIgB1fng2QLA4ez1cBXROk2KwNnMQAW9sGC0rQTyPLyiAKzYAD3tgkgBFcIh4aaJvaUDsEeKSAcrZPFg63GY+ZAAOAAY99W86q1lis7gR9HdpAEcNvBxnJ9CU2ABaKBweLxSrYOAA3Dw8bpKZITw7KLeTi4UIRLErODrTbudEzaQwQiXa68ZAAJgpVlwRGCAGEuGjomwAKyVcLeAAqhH0GN2IHQkQAklBErB7GA8PIAIIy+wwEwkbZi+YLABCfjsMEcLjcWrgIhEQA"
] |
prettier/prettier | 14,008 | prettier__prettier-14008 | [
"13998"
] | b50dfd1974d33abb980e9b4df949913932eeb478 | diff --git a/src/language-css/printer-postcss.js b/src/language-css/printer-postcss.js
--- a/src/language-css/printer-postcss.js
+++ b/src/language-css/printer-postcss.js
@@ -744,6 +744,18 @@ function genericPrint(path, options, print) {
continue;
}
+ // No space before unary minus followed by an opening parenthesis `-(`
+ if (
+ (options.parser === "scss" || options.parser === "less") &&
+ isMathOperator &&
+ iNode.value === "-" &&
+ isParenGroupNode(iNextNode) &&
+ locEnd(iNode) === locStart(iNextNode.open) &&
+ iNextNode.open.value === "("
+ ) {
+ continue;
+ }
+
// Add `hardline` after inline comment (i.e. `// comment\n foo: bar;`)
if (isInlineValueCommentNode(iNode)) {
if (parentNode.type === "value-paren_group") {
| diff --git a/tests/format/less/parens/__snapshots__/jsfmt.spec.js.snap b/tests/format/less/parens/__snapshots__/jsfmt.spec.js.snap
--- a/tests/format/less/parens/__snapshots__/jsfmt.spec.js.snap
+++ b/tests/format/less/parens/__snapshots__/jsfmt.spec.js.snap
@@ -302,6 +302,23 @@ a {
unicode-range: U+0025-00FF, U+4??; /* multiple values */
}
+// no space after unary minus when followed by opening parenthesis, #13998
+.unary_minus_single {
+ margin: -(@a);
+}
+
+.unary_minus_multi_1 {
+ margin: 0 -(@a);
+}
+
+.unary_minus_multi_2 {
+ margin: 0 -( @a + @b );
+}
+
+.binary_minus {
+ margin: 0 - (@a);
+}
+
=====================================output=====================================
a {
prop1: func(1px, 1px, 1px, func(1px, 1px, 1px, func(1px, 1px, 1px)));
@@ -365,7 +382,7 @@ a {
background: element(#css-source);
padding-top: var(--paddingC);
margin: 1 * 1 (1) * 1 1 * (1) (1) * (1);
- prop: -1 * -1 - (-1) * -1 -1 * -(-1) - (-1) * -(-1);
+ prop: -1 * -1 -(-1) * -1 -1 * -(-1) -(-1) * -(-1);
prop4: +1;
prop5: -1;
prop6: word + 1; /* word1 */
@@ -396,7 +413,7 @@ a {
prop41: --(1);
prop42: 1px+1px+1px+1px;
prop43: 1px + 1px + 1px + 1px;
- prop44: -1+-1 - (-1)+-1 -1+-(-1) - (-1)+-(-1);
+ prop44: -1+-1 -(-1)+-1 -1+-(-1) -(-1)+-(-1);
prop45: round(1.5) * 2 round(1.5) * 2 round(1.5) * 2 round(1.5) * 2;
prop46: 2 * round(1.5) 2 * round(1.5) 2 * round(1.5) 2 * round(1.5);
prop47: (round(1.5) * 2) (round(1.5) * 2) (round(1.5) * 2) (round(1.5) * 2);
@@ -472,5 +489,22 @@ a {
unicode-range: U+0025-00FF, U+4??; /* multiple values */
}
+// no space after unary minus when followed by opening parenthesis, #13998
+.unary_minus_single {
+ margin: -(@a);
+}
+
+.unary_minus_multi_1 {
+ margin: 0 -(@a);
+}
+
+.unary_minus_multi_2 {
+ margin: 0 -(@a + @b);
+}
+
+.binary_minus {
+ margin: 0 - (@a);
+}
+
================================================================================
`;
diff --git a/tests/format/less/parens/parens.less b/tests/format/less/parens/parens.less
--- a/tests/format/less/parens/parens.less
+++ b/tests/format/less/parens/parens.less
@@ -259,3 +259,20 @@ a {
unicode-range: U+4??; /* wildcard range */
unicode-range: U+0025-00FF, U+4??; /* multiple values */
}
+
+// no space after unary minus when followed by opening parenthesis, #13998
+.unary_minus_single {
+ margin: -(@a);
+}
+
+.unary_minus_multi_1 {
+ margin: 0 -(@a);
+}
+
+.unary_minus_multi_2 {
+ margin: 0 -( @a + @b );
+}
+
+.binary_minus {
+ margin: 0 - (@a);
+}
diff --git a/tests/format/scss/parens/__snapshots__/jsfmt.spec.js.snap b/tests/format/scss/parens/__snapshots__/jsfmt.spec.js.snap
--- a/tests/format/scss/parens/__snapshots__/jsfmt.spec.js.snap
+++ b/tests/format/scss/parens/__snapshots__/jsfmt.spec.js.snap
@@ -418,10 +418,10 @@ a {
);
padding-top: var(--paddingC);
margin: 1 * 1 (1) * 1 1 * (1) (1) * (1);
- prop: -1 * -1 - (-1) * -1 -1 * -(-1) - (-1) * -(-1);
+ prop: -1 * -1 -(-1) * -1 -1 * -(-1) -(-1) * -(-1);
prop1: #{($m) * (10)};
prop2: #{$m * 10};
- prop3: #{- (-$m) * -(-10)};
+ prop3: #{-(-$m) * -(-10)};
prop4: +1;
prop5: -1;
prop6: word + 1; /* word1 */
@@ -469,7 +469,7 @@ a {
prop41: --(1);
prop42: 1px+1px+1px+1px;
prop43: 1px + 1px + 1px + 1px;
- prop44: -1+-1 - (-1)+-1 -1+-(-1) - (-1)+-(-1);
+ prop44: -1+-1 -(-1)+-1 -1+-(-1) -(-1)+-(-1);
prop45: round(1.5) * 2 round(1.5) * 2 round(1.5) * 2 round(1.5) * 2;
prop46: 2 * round(1.5) 2 * round(1.5) 2 * round(1.5) 2 * round(1.5);
prop47: (round(1.5) * 2) (round(1.5) * 2) (round(1.5) * 2) (round(1.5) * 2);
| Unary minus must not be followed by a space in LESS
Current version of Prettier is adding a space after unary minus operator in LESS.
Config:
```
module.exports = {
tabWidth: 4,
overrides: [
{
files: ['*.less'],
options: {
parser: 'less',
},
},
],
};
```
Wrong autoformat:
```diff
.xxx {
- margin: 0 -(@arrowBorderWidth / 2) 0 0;
+ margin: 0 - (@arrowBorderWidth / 2) 0 0;
}
```
I would expect no fix/added space.
| minimal playground repro link: https://prettier.io/playground/#N4Igxg9gdgLgprEAuEACAdAD264AdKVI1AWwEMAnAcwEsolUAGVAWgAoABSiiAdwCEIFACZwKAdRrCYAC1QB6VACYAlEyYBuAqgC+IADQgIABxg1oAZ2ShufAAqUEVlGQA2vMgE8rhgEYUyMABrOBgAZTISOAAZOjhkADM3Czg-AODQsONAuipkGAoAV1SQOBJfOGFRYWiyKCpCsio4ADEhchgzeuQQMkKYCAMQGRgSV3EZGngLbLA4MKcpmgA3Kc8esAsfEDoUihg7AKpyROSSgCsLTDDc1zgARUKIeFPXFMNsij2eu62h4wodBgkmkMmQAA5GB8eClxAFjD0AXA9st4oYAI5PeCHEzOXoWFhQOCVSpDChwTE0cmHJonJBJN4lFIkGj5IpM25wACCnUBvn6cDsYliRNe7xAFk5j2e8XpZ0MMDIvhBsmQSgVARorlyAGEICQ6aULABWIaFFIAFSVzgZ4uWxQAklBRLAwmBAaYuc6wjBPHcxXAdDogA
Please follow the [issue template](https://github.com/prettier/prettier/blob/main/.github/ISSUE_TEMPLATE/formatting.md?plain=1) in your first comment. Related to https://github.com/prettier/prettier/issues/12613 and https://github.com/prettier/prettier/issues/4698 (duplicate?) | "2022-12-17T15:59:49Z" | 2.9 | [] | [
"tests/format/scss/parens/jsfmt.spec.js",
"tests/format/less/parens/jsfmt.spec.js"
] | JavaScript | [] | [] |
prettier/prettier | 14,038 | prettier__prettier-14038 | [
"14036"
] | 985b895c47d1b9da25cde0c0b56376606b9c1559 | diff --git a/src/language-js/comments.js b/src/language-js/comments.js
--- a/src/language-js/comments.js
+++ b/src/language-js/comments.js
@@ -453,6 +453,9 @@ const propertyLikeNodeTypes = new Set([
"TSAbstractMethodDefinition",
"TSDeclareMethod",
"MethodDefinition",
+ "ClassAccessorProperty",
+ "AccessorProperty",
+ "TSAbstractAccessorProperty",
]);
function handleMethodNameComments({
comment,
| diff --git a/tests/config/format-test.js b/tests/config/format-test.js
--- a/tests/config/format-test.js
+++ b/tests/config/format-test.js
@@ -72,6 +72,7 @@ const meriyahDisabledTests = new Set([
"static.js",
"with-semicolon-1.js",
"with-semicolon-2.js",
+ "comments.js",
].map((filename) =>
path.join(__dirname, "../format/js/decorator-auto-accessors", filename)
),
diff --git a/tests/format/js/decorator-auto-accessors/__snapshots__/jsfmt.spec.js.snap b/tests/format/js/decorator-auto-accessors/__snapshots__/jsfmt.spec.js.snap
--- a/tests/format/js/decorator-auto-accessors/__snapshots__/jsfmt.spec.js.snap
+++ b/tests/format/js/decorator-auto-accessors/__snapshots__/jsfmt.spec.js.snap
@@ -73,6 +73,91 @@ class Foo {
================================================================================
`;
+exports[`comments.js [acorn] format 1`] = `
+"Unexpected character '@' (2:3)
+ 1 | class A {
+> 2 | @dec()
+ | ^
+ 3 | // comment
+ 4 | accessor b;
+ 5 | }"
+`;
+
+exports[`comments.js [espree] format 1`] = `
+"Unexpected character '@' (2:3)
+ 1 | class A {
+> 2 | @dec()
+ | ^
+ 3 | // comment
+ 4 | accessor b;
+ 5 | }"
+`;
+
+exports[`comments.js - {"semi":false} [acorn] format 1`] = `
+"Unexpected character '@' (2:3)
+ 1 | class A {
+> 2 | @dec()
+ | ^
+ 3 | // comment
+ 4 | accessor b;
+ 5 | }"
+`;
+
+exports[`comments.js - {"semi":false} [espree] format 1`] = `
+"Unexpected character '@' (2:3)
+ 1 | class A {
+> 2 | @dec()
+ | ^
+ 3 | // comment
+ 4 | accessor b;
+ 5 | }"
+`;
+
+exports[`comments.js - {"semi":false} format 1`] = `
+====================================options=====================================
+parsers: ["babel", "typescript", "babel-flow"]
+printWidth: 80
+semi: false
+ | printWidth
+=====================================input======================================
+class A {
+ @dec()
+ // comment
+ accessor b;
+}
+
+=====================================output=====================================
+class A {
+ @dec()
+ // comment
+ accessor b
+}
+
+================================================================================
+`;
+
+exports[`comments.js format 1`] = `
+====================================options=====================================
+parsers: ["babel", "typescript", "babel-flow"]
+printWidth: 80
+ | printWidth
+=====================================input======================================
+class A {
+ @dec()
+ // comment
+ accessor b;
+}
+
+=====================================output=====================================
+class A {
+ @dec()
+ // comment
+ accessor b;
+}
+
+================================================================================
+`;
+
exports[`computed.js [acorn] format 1`] = `
"Unexpected token (2:12)
1 | class Foo {
diff --git a/tests/format/js/decorator-auto-accessors/comments.js b/tests/format/js/decorator-auto-accessors/comments.js
new file mode 100644
--- /dev/null
+++ b/tests/format/js/decorator-auto-accessors/comments.js
@@ -0,0 +1,5 @@
+class A {
+ @dec()
+ // comment
+ accessor b;
+}
diff --git a/tests/format/js/decorator-auto-accessors/jsfmt.spec.js b/tests/format/js/decorator-auto-accessors/jsfmt.spec.js
--- a/tests/format/js/decorator-auto-accessors/jsfmt.spec.js
+++ b/tests/format/js/decorator-auto-accessors/jsfmt.spec.js
@@ -9,6 +9,7 @@ const errors = {
"static.js",
"with-semicolon-1.js",
"with-semicolon-2.js",
+ "comments.js",
],
acorn: [
"basic.js",
@@ -19,6 +20,7 @@ const errors = {
"static.js",
"with-semicolon-1.js",
"with-semicolon-2.js",
+ "comments.js",
],
};
run_spec(__dirname, parsers, { errors });
| Incorrect formatting for auto-accessors with comments
<!--
BEFORE SUBMITTING AN ISSUE:
1. Search for your issue on GitHub: https://github.com/prettier/prettier/issues
A large number of opened issues are duplicates of existing issues.
If someone has already opened an issue for what you are experiencing,
you do not need to open a new issue — please add a 👍 reaction to the
existing issue instead.
2. We get a lot of requests for adding options, but Prettier is
built on the principle of being opinionated about code formatting.
This means we add options only in the case of strict technical necessity.
Find out more: https://prettier.io/docs/en/option-philosophy.html
Don't fill the form below manually! Let a program create a report for you:
1. Go to https://prettier.io/playground
2. Paste your code and set options
3. Press the "Report issue" button in the lower right
-->
**Prettier 2.8.1**
[Playground link](https://prettier.io/playground/#N4Igxg9gdgLgprEAucAbAhgZ0wAgII7AA6UOOAAgCZxgAUAlCWQPTM5yaoCWsAtJV0zoARqji8ocAB4xe3SRRgBPAA4cwAJy4rZHeTGbD0UXjEy9IAW0sIYTHK0XmuAcygQNce+jBgOmDxxhAG4SAF8QABoQCB0uaExkUHQNDQgAdwAFFIRElHRUdPQlROjhDR8AazgYAGV0GwAZHjhkADMCzDgyirBqupUfHhdkGA0AV26QOEthOEpqSkbjF3H0FzgAMQ9LdBgYYeQQdHGYCCiQAAsYS1QAdUuueExBv1rcp64ANyelI7BsBceF0NDBMhUXLt2p0pgArTBSWrDMQARXGEHg0NQXWigw0IKORjmqAuKi0sDuXEoMEuyAAHAAGXFpLp3CoqI5kjhwDRfVrRACO6Pg4NieWO5kk83mF08Qq4nnB6yhSA62KmXUsXFGEw1yLgeH2WmEpzgmR5zUkWJxIEw+rRGNaqph0RgIkp1NpSAATK6Klx5C4AMIQazoI4cACsF3GXQAKiI8mqbV9JgBJKDUWC1TTaGB4TO1ZRia1wMJhIA)
```sh
# Options (if any):
```
**Input:**
```js
class A {
@dec()
// eslint-disable-next-line @typescript-eslint/ban-ts-comment
// @ts-ignore
accessor b;
}
```
**Output:**
```js
class A {
@dec()
accessor // eslint-disable-next-line @typescript-eslint/ban-ts-comment
// @ts-ignore
b;
}
```
**Expected behavior:**
The comments should not be placed between the keyword `accessor` and the identifier `b`, which causes the `accessor` to be treated as a class field instead of a keyword. Expected:
```js
class A {
@dec()
// eslint-disable-next-line @typescript-eslint/ban-ts-comment
// @ts-ignore
accessor b;
}
```
| This is actually bug in TypeScript https://github.com/microsoft/TypeScript/issues/51707
But I think here the issue has nothing todo with that bug in TypeScript.
My input is `accessor b` with no line terminators. The prettier formatted that into separated lines, which according to the spec draft, is not matching with accessor syntax, but two separated field properties. | "2022-12-19T10:39:42Z" | 2.9 | [] | [
"tests/format/js/decorator-auto-accessors/jsfmt.spec.js"
] | JavaScript | [] | [
"https://prettier.io/playground/#N4Igxg9gdgLgprEAucAbAhgZ0wAgII7AA6UOOAAgCZxgAUAlCWQPTM5yaoCWsAtJV0zoARqji8ocAB4xe3SRRgBPAA4cwAJy4rZHeTGbD0UXjEy9IAW0sIYTHK0XmuAcygQNce+jBgOmDxxhAG4SAF8QABoQCB0uaExkUHQNDQgAdwAFFIRElHRUdPQlROjhDR8AazgYAGV0GwAZHjhkADMCzDgyirBqupUfHhdkGA0AV26QOEthOEpqSkbjF3H0FzgAMQ9LdBgYYeQQdHGYCCiQAAsYS1QAdUuueExBv1rcp64ANyelI7BsBceF0NDBMhUXLt2p0pgArTBSWrDMQARXGEHg0NQXWigw0IKORjmqAuKi0sDuXEoMEuyAAHAAGXFpLp3CoqI5kjhwDRfVrRACO6Pg4NieWO5kk83mF08Qq4nnB6yhSA62KmXUsXFGEw1yLgeH2WmEpzgmR5zUkWJxIEw+rRGNaqph0RgIkp1NpSAATK6Klx5C4AMIQazoI4cACsF3GXQAKiI8mqbV9JgBJKDUWC1TTaGB4TO1ZRia1wMJhIA",
"https://prettier.io/playground"
] |
prettier/prettier | 14,044 | prettier__prettier-14044 | [
"10828"
] | 20fad630fab9f62ad37220ffdc7fbff1faf0f2af | diff --git a/src/language-js/needs-parens.js b/src/language-js/needs-parens.js
--- a/src/language-js/needs-parens.js
+++ b/src/language-js/needs-parens.js
@@ -67,16 +67,32 @@ function needsParens(path, options) {
return true;
}
- // `for (async of []);` is invalid
+ // `for ((async) of []);` and `for ((let) of []);`
if (
name === "left" &&
- node.name === "async" &&
+ (node.name === "async" || node.name === "let") &&
parent.type === "ForOfStatement" &&
!parent.await
) {
return true;
}
+ // `for ((let.a) of []);`
+ if (node.name === "let") {
+ const expression = path.findAncestor(
+ (node) => node.type === "ForOfStatement"
+ )?.left;
+ if (
+ expression &&
+ startsWithNoLookaheadToken(
+ expression,
+ (leftmostNode) => leftmostNode === node
+ )
+ ) {
+ return true;
+ }
+ }
+
// `(let)[a] = 1`
if (
name === "object" &&
@@ -89,8 +105,7 @@ function needsParens(path, options) {
(node) =>
node.type === "ExpressionStatement" ||
node.type === "ForStatement" ||
- node.type === "ForInStatement" ||
- node.type === "ForOfStatement"
+ node.type === "ForInStatement"
);
const expression = !statement
? undefined
| diff --git a/tests/format/js/identifier/for-of/__snapshots__/jsfmt.spec.js.snap b/tests/format/js/identifier/for-of/__snapshots__/jsfmt.spec.js.snap
new file mode 100644
--- /dev/null
+++ b/tests/format/js/identifier/for-of/__snapshots__/jsfmt.spec.js.snap
@@ -0,0 +1,57 @@
+// Jest Snapshot v1, https://goo.gl/fbAQLP
+
+exports[`await.js format 1`] = `
+====================================options=====================================
+parsers: ["babel", "typescript"]
+printWidth: 80
+ | printWidth
+=====================================input======================================
+async function a() {
+ for await((let).a of foo);
+ for await((let)[a] of foo);
+ for await((let)()[a] of foo);
+}
+
+=====================================output=====================================
+async function a() {
+ for await ((let).a of foo);
+ for await ((let)[a] of foo);
+ for await ((let)()[a] of foo);
+}
+
+================================================================================
+`;
+
+exports[`let.js format 1`] = `
+====================================options=====================================
+parsers: ["babel", "typescript"]
+printWidth: 80
+ | printWidth
+=====================================input======================================
+for ((let) of foo);
+for (foo of let);
+for (foo of let.a);
+for (foo of let[a]);
+for ((let.a) of foo);
+for ((let[a]) of foo);
+for ((let)().a of foo);
+for (letFoo of foo);
+
+for ((let.a) in foo);
+for ((let[a]) in foo);
+
+=====================================output=====================================
+for ((let) of foo);
+for (foo of let);
+for (foo of let.a);
+for (foo of let[a]);
+for ((let).a of foo);
+for ((let)[a] of foo);
+for ((let)().a of foo);
+for (letFoo of foo);
+
+for (let.a in foo);
+for ((let)[a] in foo);
+
+================================================================================
+`;
diff --git a/tests/format/js/identifier/for-of/await.js b/tests/format/js/identifier/for-of/await.js
new file mode 100644
--- /dev/null
+++ b/tests/format/js/identifier/for-of/await.js
@@ -0,0 +1,5 @@
+async function a() {
+ for await((let).a of foo);
+ for await((let)[a] of foo);
+ for await((let)()[a] of foo);
+}
diff --git a/tests/format/js/identifier/for-of/jsfmt.spec.js b/tests/format/js/identifier/for-of/jsfmt.spec.js
new file mode 100644
--- /dev/null
+++ b/tests/format/js/identifier/for-of/jsfmt.spec.js
@@ -0,0 +1,5 @@
+run_spec(__dirname, [
+ "babel",
+ // "flow",
+ "typescript",
+]);
diff --git a/tests/format/js/identifier/for-of/let.js b/tests/format/js/identifier/for-of/let.js
new file mode 100644
--- /dev/null
+++ b/tests/format/js/identifier/for-of/let.js
@@ -0,0 +1,11 @@
+for ((let) of foo);
+for (foo of let);
+for (foo of let.a);
+for (foo of let[a]);
+for ((let.a) of foo);
+for ((let[a]) of foo);
+for ((let)().a of foo);
+for (letFoo of foo);
+
+for ((let.a) in foo);
+for ((let[a]) in foo);
| Missing parentheses `for ((let) of []);`
**Prettier 2.2.1**
[Playground link](https://prettier.io/playground/#N4Igxg9gdgLgprEAuEAzCAnABAChwGzhgEosJUsBtAXWIG4QAaECABxgEtoBnZUAQwwYIAdwAKghLxT98I-gE9ezAEYZ+YANZEAyqw0coAc2QwMAVzjM4AWxVwAJg8cAZfsfP8jcAGKYb-DCcxsgg-OYwEEwgABYwNvgA6jEc8Nz6YHA6UqkcAG6pCqFg3MoghtxwGDBi6kYByKiylcwAVtwAHgBC6lq6-DZwLoZwjc1WIO0dOoZGhACK5hDwY-gtIPoYlRihKvz2+NGsGIYwiRwOMDHIABwADMzHEJWJ6qyhx3DbeaPMAI5LeC1NjSMLcAC0UDgjkc0QwcABHHhtS8DSQTTWE0qNg4pgsWNmC0Bo3R42YMH250u1yQACZyeoOPhZgBhCA2NEgL4AVmi5kqABV9tIMes8pYAJJQZywHRgE7sACC0p0MAUhFWlQAvlqgA)
<!-- prettier-ignore -->
```sh
--parser babel
```
**Input:**
<!-- prettier-ignore -->
```jsx
for ((let) of []);
```
**Output:**
<!-- prettier-ignore -->
```jsx
for (let of []);
```
**Second Output:**
<!-- prettier-ignore -->
```jsx
SyntaxError: Unexpected token, expected ";" (1:13)
> 1 | for (let of []);
| ^
2 |
```
The parens are also needed in `for ((let) of []);` and `for ((let.foo.bar) of []);`.
See https://tc39.es/ecma262/#sec-for-in-and-for-of-statements
_Originally posted by @thorn0 in https://github.com/prettier/prettier/issues/10777#issuecomment-829191658_
| ## summary
according to spec
**for in:**
```js
for (expression1 in expression2) {}
```
If `expressin1` starts with `let[`, it should be wrapped by parens.
**for of:**
```js
for (expression1 of expression2) {}
```
If `expression1` starts with `let` or `async of`, it should be wrapped by parens.
**for await of:**
```js
for await (expression1 of expression2) {}
```
If `expression1` starts with `let`, it should be wrapped by parens. | "2022-12-22T02:17:04Z" | 2.9 | [] | [
"tests/format/js/identifier/for-of/jsfmt.spec.js"
] | JavaScript | [] | [
"https://prettier.io/playground/#N4Igxg9gdgLgprEAuEAzCAnABAChwGzhgEosJUsBtAXWIG4QAaECABxgEtoBnZUAQwwYIAdwAKghLxT98I-gE9ezAEYZ+YANZEAyqw0coAc2QwMAVzjM4AWxVwAJg8cAZfsfP8jcAGKYb-DCcxsgg-OYwEEwgABYwNvgA6jEc8Nz6YHA6UqkcAG6pCqFg3MoghtxwGDBi6kYByKiylcwAVtwAHgBC6lq6-DZwLoZwjc1WIO0dOoZGhACK5hDwY-gtIPoYlRihKvz2+NGsGIYwiRwOMDHIABwADMzHEJWJ6qyhx3DbeaPMAI5LeC1NjSMLcAC0UDgjkc0QwcABHHhtS8DSQTTWE0qNg4pgsWNmC0Bo3R42YMH250u1yQACZyeoOPhZgBhCA2NEgL4AVmi5kqABV9tIMes8pYAJJQZywHRgE7sACC0p0MAUhFWlQAvlqgA"
] |
prettier/prettier | 14,073 | prettier__prettier-14073 | [
"13817"
] | 948b3af232d9be1cf973a143382cad3ebe33725a | diff --git a/src/language-js/print/type-parameters.js b/src/language-js/print/type-parameters.js
--- a/src/language-js/print/type-parameters.js
+++ b/src/language-js/print/type-parameters.js
@@ -44,12 +44,12 @@ function printTypeParameters(path, options, print, paramsKey) {
);
const shouldInline =
- !isArrowFunctionVariable &&
- (isParameterInTestCall ||
- node[paramsKey].length === 0 ||
- (node[paramsKey].length === 1 &&
- (node[paramsKey][0].type === "NullableTypeAnnotation" ||
- shouldHugType(node[paramsKey][0]))));
+ node[paramsKey].length === 0 ||
+ (!isArrowFunctionVariable &&
+ (isParameterInTestCall ||
+ (node[paramsKey].length === 1 &&
+ (node[paramsKey][0].type === "NullableTypeAnnotation" ||
+ shouldHugType(node[paramsKey][0])))));
if (shouldInline) {
return [
| diff --git a/tests/format/typescript/typeparams/empty-parameters-with-arrow-function/__snapshots__/jsfmt.spec.js.snap b/tests/format/typescript/typeparams/empty-parameters-with-arrow-function/__snapshots__/jsfmt.spec.js.snap
new file mode 100644
--- /dev/null
+++ b/tests/format/typescript/typeparams/empty-parameters-with-arrow-function/__snapshots__/jsfmt.spec.js.snap
@@ -0,0 +1,35 @@
+// Jest Snapshot v1, https://goo.gl/fbAQLP
+
+exports[`issue-13817.ts - {"arrowParens":"avoid","trailingComma":"all"} format 1`] = `
+====================================options=====================================
+arrowParens: "avoid"
+parsers: ["typescript", "flow", "babel-flow"]
+printWidth: 80
+trailingComma: "all"
+ | printWidth
+=====================================input======================================
+const xxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxx: xxxxxxxxxxxxxxxxxxxxxx<> =
+ arg => null;
+
+const xxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxx: xxxxxxxxxxxxxxxxxxxxxx</* comment */> =
+ arg => null;
+
+
+const xxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxx: xxxxxxxxxxxxxxxxxxxxxx<
+ // comment
+> =
+ arg => null;
+
+=====================================output=====================================
+const xxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxx: xxxxxxxxxxxxxxxxxxxxxx<> =
+ arg => null;
+
+const xxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxx: xxxxxxxxxxxxxxxxxxxxxx</* comment */> =
+ arg => null;
+
+const xxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxx: xxxxxxxxxxxxxxxxxxxxxx<
+ // comment
+> = arg => null;
+
+================================================================================
+`;
diff --git a/tests/format/typescript/typeparams/empty-parameters-with-arrow-function/issue-13817.ts b/tests/format/typescript/typeparams/empty-parameters-with-arrow-function/issue-13817.ts
new file mode 100644
--- /dev/null
+++ b/tests/format/typescript/typeparams/empty-parameters-with-arrow-function/issue-13817.ts
@@ -0,0 +1,11 @@
+const xxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxx: xxxxxxxxxxxxxxxxxxxxxx<> =
+ arg => null;
+
+const xxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxx: xxxxxxxxxxxxxxxxxxxxxx</* comment */> =
+ arg => null;
+
+
+const xxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxx: xxxxxxxxxxxxxxxxxxxxxx<
+ // comment
+> =
+ arg => null;
diff --git a/tests/format/typescript/typeparams/empty-parameters-with-arrow-function/jsfmt.spec.js b/tests/format/typescript/typeparams/empty-parameters-with-arrow-function/jsfmt.spec.js
new file mode 100644
--- /dev/null
+++ b/tests/format/typescript/typeparams/empty-parameters-with-arrow-function/jsfmt.spec.js
@@ -0,0 +1,6 @@
+run_spec(
+ __dirname,
+ ["typescript", "flow", "babel-flow"],
+ // #13817 require those options to reproduce
+ { arrowParens: "avoid", trailingComma: "all" }
+);
| [flow] broken code emitted for type annotation with empty type parameters when the value is an arrow function and `arrow-parens: 'avoid'`
**Prettier 2.7.1**
[Playground link](https://prettier.io/playground/#N4Igxg9gdgLgprEAuc0DOMAEAPXf8GFHGFI4kW4A8AfJgLwA6UmmAhgE4DmDdUArgBtBAbhAAaEBAAOMAJbpkoThwgB3AAqcEaZCDYA3CHIAmEkACMObMAGs4MAMpsAtnAAycqHGQAzNoJocJJWNvZO0jZeXMgwHPzBIHAuFnAmJmnubFBc-GxccABiEBwubDDyOXps-DAQ5gAWMC6CAOoNcvBokWBwjjqdcgadAJ56YGi6kl5BHDAa1lxlfgFBkgBWaNiO0YJwAIr8EPArgYmRHLN6voLq5tIcXjCtpjANyAAcAAySDxBBrWs0j0DzgswMPkkAEcjvAFjJdCg2GgALTeNJpcwcOAwuTYhb5ZZIfxnSRBFxyWLxRJoXYHWE+JBxBKSGBsCwvExvZAAJlZ1jkgmiAGEIC4ifphOZ+EEACrsxEktYgAwJACSUAysEcYEesgAgprHDARntTkEAL4WoA)
<!-- prettier-ignore -->
```sh
--parser flow
--arrow-parens avoid
--trailing-comma all
```
**Input:**
<!-- prettier-ignore -->
```jsx
const xxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxx: xxxxxxxxxxxxxxxxxxxxxx<> =
arg => null;
```
**Output:**
<!-- prettier-ignore -->
```jsx
const xxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxx: xxxxxxxxxxxxxxxxxxxxxx<
,
> = arg => null;
```
**Expected behavior:**
Not-broken code. I'd suggest probably:
```jsx
const xxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxx: xxxxxxxxxxxxxxxxxxxxxx<> =
arg => null;
```
| It's worth noting that the same code emits correctly [for `typescript`](https://prettier.io/playground/#N4Igxg9gdgLgprEAuc0DOMAEAPXf8GFHGFI4kW4A8AfJgLwA6UmmAhgE4DmDdUArgBtBAbhAAaEBAAOMAJbpkoThwgB3AAqcEaZCDYA3CHIAmEkACMObMAGs4MAMpsAtnAAycqHGQAzNoJocJJWNvZO0jZeXMgwHPzBIHAuFnAmJmnubFBc-GxccABiEBwubDDyOXps-DAQ5gAWMC6CAOoNcvBokWBwjjqdcgadAJ56YGi6kl5BHDAa1lxlfgFBkgBWaNiO0YJwAIr8EPArgYmRHLN6MCPScGhgHHKy5tJPsK2mMA3IABwADJI3hAgq1rNI9G97nAOAYfJIAI5HeALGS6FBsNAAWm8aTS5g4cCRckJC3yyyQ-jOkiCLjksXiiTQuwOyJ8SDiCUkMDYFk+Jm+yAATNzrHJBNEAMIQFwU-TCcz8IIAFV56KpaxABgSAEkoBlYI5Hs8YABBfWOG57U5BAC+tqAA) and `babel-ts`, but not `babel`, `babel-flow`, or `flow`.
However the output for the TS parsers is pretty ugly - it includes an empty line!
```ts
const xxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxx: xxxxxxxxxxxxxxxxxxxxxx<
> = arg => null;
``` | "2022-12-28T11:59:30Z" | 2.9 | [] | [
"tests/format/typescript/typeparams/empty-parameters-with-arrow-function/jsfmt.spec.js"
] | JavaScript | [] | [
"https://prettier.io/playground/#N4Igxg9gdgLgprEAuc0DOMAEAPXf8GFHGFI4kW4A8AfJgLwA6UmmAhgE4DmDdUArgBtBAbhAAaEBAAOMAJbpkoThwgB3AAqcEaZCDYA3CHIAmEkACMObMAGs4MAMpsAtnAAycqHGQAzNoJocJJWNvZO0jZeXMgwHPzBIHAuFnAmJmnubFBc-GxccABiEBwubDDyOXps-DAQ5gAWMC6CAOoNcvBokWBwjjqdcgadAJ56YGi6kl5BHDAa1lxlfgFBkgBWaNiO0YJwAIr8EPArgYmRHLN6voLq5tIcXjCtpjANyAAcAAySDxBBrWs0j0DzgswMPkkAEcjvAFjJdCg2GgALTeNJpcwcOAwuTYhb5ZZIfxnSRBFxyWLxRJoXYHWE+JBxBKSGBsCwvExvZAAJlZ1jkgmiAGEIC4ifphOZ+EEACrsxEktYgAwJACSUAysEcYEesgAgprHDARntTkEAL4WoA"
] |
prettier/prettier | 14,081 | prettier__prettier-14081 | [
"14080"
] | ef707da0a2cafa56b46a94b865069a3cf401835d | diff --git a/src/language-js/print/literal.js b/src/language-js/print/literal.js
--- a/src/language-js/print/literal.js
+++ b/src/language-js/print/literal.js
@@ -1,6 +1,7 @@
"use strict";
const { printString, printNumber } = require("../../common/util.js");
const { replaceTextEndOfLine } = require("../../document/doc-utils.js");
+const { printDirective } = require("./misc.js");
function printLiteral(path, options /*, print*/) {
const node = path.getNode();
@@ -41,7 +42,9 @@ function printLiteral(path, options /*, print*/) {
}
if (typeof value === "string") {
- return replaceTextEndOfLine(printString(node.raw, options));
+ return isDirective(path)
+ ? printDirective(node.raw, options)
+ : replaceTextEndOfLine(printString(node.raw, options));
}
return String(value);
@@ -49,6 +52,15 @@ function printLiteral(path, options /*, print*/) {
}
}
+function isDirective(path) {
+ if (path.getName() !== "expression") {
+ return;
+ }
+
+ const parent = path.getParentNode();
+ return parent.type === "ExpressionStatement" && parent.directive;
+}
+
function printBigInt(raw) {
return raw.toLowerCase();
}
diff --git a/src/language-js/print/misc.js b/src/language-js/print/misc.js
--- a/src/language-js/print/misc.js
+++ b/src/language-js/print/misc.js
@@ -93,6 +93,24 @@ function printRestSpread(path, options, print) {
return ["...", print("argument"), printTypeAnnotation(path, options, print)];
}
+function printDirective(rawText, options) {
+ const rawContent = rawText.slice(1, -1);
+
+ // Check for the alternate quote, to determine if we're allowed to swap
+ // the quotes on a DirectiveLiteral.
+ if (rawContent.includes('"') || rawContent.includes("'")) {
+ return rawText;
+ }
+
+ const enclosingQuote = options.singleQuote ? "'" : '"';
+
+ // Directives are exact code unit sequences, which means that you can't
+ // change the escape sequences they use.
+ // See https://github.com/prettier/prettier/issues/1555
+ // and https://tc39.github.io/ecma262/#directive-prologue
+ return enclosingQuote + rawContent + enclosingQuote;
+}
+
module.exports = {
printOptionalToken,
printDefiniteToken,
@@ -102,4 +120,5 @@ module.exports = {
printTypeAnnotation,
printRestSpread,
adjustClause,
+ printDirective,
};
diff --git a/src/language-js/printer-estree.js b/src/language-js/printer-estree.js
--- a/src/language-js/printer-estree.js
+++ b/src/language-js/printer-estree.js
@@ -23,7 +23,6 @@ const {
isLineComment,
isNextLineEmpty,
needsHardlineAfterDanglingComment,
- rawText,
hasIgnoreComment,
isCallExpression,
isMemberExpression,
@@ -47,6 +46,7 @@ const {
adjustClause,
printRestSpread,
printDefiniteToken,
+ printDirective,
} = require("./print/misc.js");
const {
printImportDeclaration,
@@ -215,11 +215,6 @@ function printPathNoParens(path, options, print, args) {
case "EmptyStatement":
return "";
case "ExpressionStatement": {
- // Detect Flow and TypeScript directives
- if (node.directive) {
- return [printDirective(node.expression, options), semi];
- }
-
if (
options.parser === "__vue_event_binding" ||
options.parser === "__vue_ts_event_binding"
@@ -429,7 +424,7 @@ function printPathNoParens(path, options, print, args) {
case "Directive":
return [print("value"), semi]; // Babel 6
case "DirectiveLiteral":
- return printDirective(node, options);
+ return printDirective(node.extra.raw, options);
case "UnaryExpression":
parts.push(node.operator);
@@ -813,25 +808,6 @@ function printPathNoParens(path, options, print, args) {
}
}
-function printDirective(node, options) {
- const raw = rawText(node);
- const rawContent = raw.slice(1, -1);
-
- // Check for the alternate quote, to determine if we're allowed to swap
- // the quotes on a DirectiveLiteral.
- if (rawContent.includes('"') || rawContent.includes("'")) {
- return raw;
- }
-
- const enclosingQuote = options.singleQuote ? "'" : '"';
-
- // Directives are exact code unit sequences, which means that you can't
- // change the escape sequences they use.
- // See https://github.com/prettier/prettier/issues/1555
- // and https://tc39.github.io/ecma262/#directive-prologue
- return enclosingQuote + rawContent + enclosingQuote;
-}
-
function canAttachComment(node) {
return (
node.type &&
| diff --git a/tests/format/js/directives/comments/__snapshots__/jsfmt.spec.js.snap b/tests/format/js/directives/comments/__snapshots__/jsfmt.spec.js.snap
new file mode 100644
--- /dev/null
+++ b/tests/format/js/directives/comments/__snapshots__/jsfmt.spec.js.snap
@@ -0,0 +1,569 @@
+// Jest Snapshot v1, https://goo.gl/fbAQLP
+
+exports[`snippet: #0 - {"semi":false} format 1`] = `
+====================================options=====================================
+parsers: ["babel", "flow", "typescript"]
+printWidth: 80
+semi: false
+ | printWidth
+=====================================input======================================
+/* comment */ 'use strict';
+=====================================output=====================================
+/* comment */ "use strict"
+
+================================================================================
+`;
+
+exports[`snippet: #0 format 1`] = `
+====================================options=====================================
+parsers: ["babel", "flow", "typescript"]
+printWidth: 80
+ | printWidth
+=====================================input======================================
+/* comment */ 'use strict';
+=====================================output=====================================
+/* comment */ "use strict";
+
+================================================================================
+`;
+
+exports[`snippet: #1 - {"semi":false} format 1`] = `
+====================================options=====================================
+parsers: ["babel", "flow", "typescript"]
+printWidth: 80
+semi: false
+ | printWidth
+=====================================input======================================
+function foo() {
+ /* comment */ 'use strict';
+}
+=====================================output=====================================
+function foo() {
+ /* comment */ "use strict"
+}
+
+================================================================================
+`;
+
+exports[`snippet: #1 format 1`] = `
+====================================options=====================================
+parsers: ["babel", "flow", "typescript"]
+printWidth: 80
+ | printWidth
+=====================================input======================================
+function foo() {
+ /* comment */ 'use strict';
+}
+=====================================output=====================================
+function foo() {
+ /* comment */ "use strict";
+}
+
+================================================================================
+`;
+
+exports[`snippet: #2 - {"semi":false} format 1`] = `
+====================================options=====================================
+parsers: ["babel", "flow", "typescript"]
+printWidth: 80
+semi: false
+ | printWidth
+=====================================input======================================
+'use strict' /* comment */;
+=====================================output=====================================
+"use strict" /* comment */
+
+================================================================================
+`;
+
+exports[`snippet: #2 format 1`] = `
+====================================options=====================================
+parsers: ["babel", "flow", "typescript"]
+printWidth: 80
+ | printWidth
+=====================================input======================================
+'use strict' /* comment */;
+=====================================output=====================================
+"use strict" /* comment */;
+
+================================================================================
+`;
+
+exports[`snippet: #3 - {"semi":false} format 1`] = `
+====================================options=====================================
+parsers: ["babel", "flow", "typescript"]
+printWidth: 80
+semi: false
+ | printWidth
+=====================================input======================================
+function foo() {
+ 'use strict' /* comment */;
+}
+=====================================output=====================================
+function foo() {
+ "use strict" /* comment */
+}
+
+================================================================================
+`;
+
+exports[`snippet: #3 format 1`] = `
+====================================options=====================================
+parsers: ["babel", "flow", "typescript"]
+printWidth: 80
+ | printWidth
+=====================================input======================================
+function foo() {
+ 'use strict' /* comment */;
+}
+=====================================output=====================================
+function foo() {
+ "use strict" /* comment */;
+}
+
+================================================================================
+`;
+
+exports[`snippet: #4 - {"semi":false} format 1`] = `
+====================================options=====================================
+parsers: ["babel", "flow", "typescript"]
+printWidth: 80
+semi: false
+ | printWidth
+=====================================input======================================
+// comment
+'use strict';
+=====================================output=====================================
+// comment
+"use strict"
+
+================================================================================
+`;
+
+exports[`snippet: #4 format 1`] = `
+====================================options=====================================
+parsers: ["babel", "flow", "typescript"]
+printWidth: 80
+ | printWidth
+=====================================input======================================
+// comment
+'use strict';
+=====================================output=====================================
+// comment
+"use strict";
+
+================================================================================
+`;
+
+exports[`snippet: #5 - {"semi":false} format 1`] = `
+====================================options=====================================
+parsers: ["babel", "flow", "typescript"]
+printWidth: 80
+semi: false
+ | printWidth
+=====================================input======================================
+function foo() {
+ // comment
+ 'use strict';
+}
+=====================================output=====================================
+function foo() {
+ // comment
+ "use strict"
+}
+
+================================================================================
+`;
+
+exports[`snippet: #5 format 1`] = `
+====================================options=====================================
+parsers: ["babel", "flow", "typescript"]
+printWidth: 80
+ | printWidth
+=====================================input======================================
+function foo() {
+ // comment
+ 'use strict';
+}
+=====================================output=====================================
+function foo() {
+ // comment
+ "use strict";
+}
+
+================================================================================
+`;
+
+exports[`snippet: #6 - {"semi":false} format 1`] = `
+====================================options=====================================
+parsers: ["babel", "flow", "typescript"]
+printWidth: 80
+semi: false
+ | printWidth
+=====================================input======================================
+'use strict' // comment
+=====================================output=====================================
+"use strict" // comment
+
+================================================================================
+`;
+
+exports[`snippet: #6 format 1`] = `
+====================================options=====================================
+parsers: ["babel", "flow", "typescript"]
+printWidth: 80
+ | printWidth
+=====================================input======================================
+'use strict' // comment
+=====================================output=====================================
+"use strict"; // comment
+
+================================================================================
+`;
+
+exports[`snippet: #7 - {"semi":false} format 1`] = `
+====================================options=====================================
+parsers: ["babel", "flow", "typescript"]
+printWidth: 80
+semi: false
+ | printWidth
+=====================================input======================================
+function foo() {
+ 'use strict' // comment
+}
+=====================================output=====================================
+function foo() {
+ "use strict" // comment
+}
+
+================================================================================
+`;
+
+exports[`snippet: #7 format 1`] = `
+====================================options=====================================
+parsers: ["babel", "flow", "typescript"]
+printWidth: 80
+ | printWidth
+=====================================input======================================
+function foo() {
+ 'use strict' // comment
+}
+=====================================output=====================================
+function foo() {
+ "use strict"; // comment
+}
+
+================================================================================
+`;
+
+exports[`snippet: #8 - {"semi":false} format 1`] = `
+====================================options=====================================
+parsers: ["babel", "flow", "typescript"]
+printWidth: 80
+semi: false
+ | printWidth
+=====================================input======================================
+'use strict';
+/* comment */
+(function () {})();
+=====================================output=====================================
+"use strict"
+/* comment */
+;(function () {})()
+
+================================================================================
+`;
+
+exports[`snippet: #8 format 1`] = `
+====================================options=====================================
+parsers: ["babel", "flow", "typescript"]
+printWidth: 80
+ | printWidth
+=====================================input======================================
+'use strict';
+/* comment */
+(function () {})();
+=====================================output=====================================
+"use strict";
+/* comment */
+(function () {})();
+
+================================================================================
+`;
+
+exports[`snippet: #9 - {"semi":false} format 1`] = `
+====================================options=====================================
+parsers: ["babel", "flow", "typescript"]
+printWidth: 80
+semi: false
+ | printWidth
+=====================================input======================================
+function foo() {
+ 'use strict';
+ /* comment */
+ (function () {})();
+}
+=====================================output=====================================
+function foo() {
+ "use strict"
+ /* comment */
+ ;(function () {})()
+}
+
+================================================================================
+`;
+
+exports[`snippet: #9 format 1`] = `
+====================================options=====================================
+parsers: ["babel", "flow", "typescript"]
+printWidth: 80
+ | printWidth
+=====================================input======================================
+function foo() {
+ 'use strict';
+ /* comment */
+ (function () {})();
+}
+=====================================output=====================================
+function foo() {
+ "use strict";
+ /* comment */
+ (function () {})();
+}
+
+================================================================================
+`;
+
+exports[`snippet: #10 - {"semi":false} format 1`] = `
+====================================options=====================================
+parsers: ["babel", "flow", "typescript"]
+printWidth: 80
+semi: false
+ | printWidth
+=====================================input======================================
+/* comment */
+'use strict';
+(function () {})();
+=====================================output=====================================
+/* comment */
+"use strict"
+;(function () {})()
+
+================================================================================
+`;
+
+exports[`snippet: #10 format 1`] = `
+====================================options=====================================
+parsers: ["babel", "flow", "typescript"]
+printWidth: 80
+ | printWidth
+=====================================input======================================
+/* comment */
+'use strict';
+(function () {})();
+=====================================output=====================================
+/* comment */
+"use strict";
+(function () {})();
+
+================================================================================
+`;
+
+exports[`snippet: #11 - {"semi":false} format 1`] = `
+====================================options=====================================
+parsers: ["babel", "flow", "typescript"]
+printWidth: 80
+semi: false
+ | printWidth
+=====================================input======================================
+function foo() {
+ /* comment */
+ 'use strict';
+ (function () {})();
+}
+=====================================output=====================================
+function foo() {
+ /* comment */
+ "use strict"
+ ;(function () {})()
+}
+
+================================================================================
+`;
+
+exports[`snippet: #11 format 1`] = `
+====================================options=====================================
+parsers: ["babel", "flow", "typescript"]
+printWidth: 80
+ | printWidth
+=====================================input======================================
+function foo() {
+ /* comment */
+ 'use strict';
+ (function () {})();
+}
+=====================================output=====================================
+function foo() {
+ /* comment */
+ "use strict";
+ (function () {})();
+}
+
+================================================================================
+`;
+
+exports[`snippet: #12 - {"semi":false} format 1`] = `
+====================================options=====================================
+parsers: ["babel", "flow", "typescript"]
+printWidth: 80
+semi: false
+ | printWidth
+=====================================input======================================
+'use strict';
+// comment
+(function () {})();
+=====================================output=====================================
+"use strict"
+// comment
+;(function () {})()
+
+================================================================================
+`;
+
+exports[`snippet: #12 format 1`] = `
+====================================options=====================================
+parsers: ["babel", "flow", "typescript"]
+printWidth: 80
+ | printWidth
+=====================================input======================================
+'use strict';
+// comment
+(function () {})();
+=====================================output=====================================
+"use strict";
+// comment
+(function () {})();
+
+================================================================================
+`;
+
+exports[`snippet: #13 - {"semi":false} format 1`] = `
+====================================options=====================================
+parsers: ["babel", "flow", "typescript"]
+printWidth: 80
+semi: false
+ | printWidth
+=====================================input======================================
+function foo() {
+ 'use strict';
+ // comment
+ (function () {})();
+}
+=====================================output=====================================
+function foo() {
+ "use strict"
+ // comment
+ ;(function () {})()
+}
+
+================================================================================
+`;
+
+exports[`snippet: #13 format 1`] = `
+====================================options=====================================
+parsers: ["babel", "flow", "typescript"]
+printWidth: 80
+ | printWidth
+=====================================input======================================
+function foo() {
+ 'use strict';
+ // comment
+ (function () {})();
+}
+=====================================output=====================================
+function foo() {
+ "use strict";
+ // comment
+ (function () {})();
+}
+
+================================================================================
+`;
+
+exports[`snippet: #14 - {"semi":false} format 1`] = `
+====================================options=====================================
+parsers: ["babel", "flow", "typescript"]
+printWidth: 80
+semi: false
+ | printWidth
+=====================================input======================================
+// comment
+'use strict';
+(function () {})();
+=====================================output=====================================
+// comment
+"use strict"
+;(function () {})()
+
+================================================================================
+`;
+
+exports[`snippet: #14 format 1`] = `
+====================================options=====================================
+parsers: ["babel", "flow", "typescript"]
+printWidth: 80
+ | printWidth
+=====================================input======================================
+// comment
+'use strict';
+(function () {})();
+=====================================output=====================================
+// comment
+"use strict";
+(function () {})();
+
+================================================================================
+`;
+
+exports[`snippet: #15 - {"semi":false} format 1`] = `
+====================================options=====================================
+parsers: ["babel", "flow", "typescript"]
+printWidth: 80
+semi: false
+ | printWidth
+=====================================input======================================
+function foo() {
+ // comment
+ 'use strict';
+ (function () {})();
+}
+=====================================output=====================================
+function foo() {
+ // comment
+ "use strict"
+ ;(function () {})()
+}
+
+================================================================================
+`;
+
+exports[`snippet: #15 format 1`] = `
+====================================options=====================================
+parsers: ["babel", "flow", "typescript"]
+printWidth: 80
+ | printWidth
+=====================================input======================================
+function foo() {
+ // comment
+ 'use strict';
+ (function () {})();
+}
+=====================================output=====================================
+function foo() {
+ // comment
+ "use strict";
+ (function () {})();
+}
+
+================================================================================
+`;
diff --git a/tests/format/js/directives/comments/jsfmt.spec.js b/tests/format/js/directives/comments/jsfmt.spec.js
new file mode 100644
--- /dev/null
+++ b/tests/format/js/directives/comments/jsfmt.spec.js
@@ -0,0 +1,55 @@
+const { outdent } = require("outdent");
+const indent = (text) =>
+ text
+ .split("\n")
+ .map((line) => (line ? ` ${line}` : line))
+ .join("\n");
+// TODO: Remove this when we drop support for Node.js v10
+// eslint-disable-next-line unicorn/prefer-spread
+const flat = (array) => [].concat(...array);
+
+const snippets = flat(
+ [
+ "/* comment */ 'use strict';",
+ "'use strict' /* comment */;",
+ outdent`
+ // comment
+ 'use strict';
+ `,
+ outdent`
+ 'use strict' // comment
+ `,
+ outdent`
+ 'use strict';
+ /* comment */
+ (function () {})();
+ `,
+ outdent`
+ /* comment */
+ 'use strict';
+ (function () {})();
+ `,
+ outdent`
+ 'use strict';
+ // comment
+ (function () {})();
+ `,
+ outdent`
+ // comment
+ 'use strict';
+ (function () {})();
+ `,
+ ].map((code) => [
+ code,
+ outdent`
+ function foo() {
+ ${indent(code)}
+ }
+ `,
+ ])
+);
+
+run_spec({ dirname: __dirname, snippets }, ["babel", "flow", "typescript"]);
+run_spec({ dirname: __dirname, snippets }, ["babel", "flow", "typescript"], {
+ semi: false,
+});
diff --git a/tests/format/markdown/auto-link/jsfmt.spec.js b/tests/format/markdown/auto-link/jsfmt.spec.js
--- a/tests/format/markdown/auto-link/jsfmt.spec.js
+++ b/tests/format/markdown/auto-link/jsfmt.spec.js
@@ -3,6 +3,7 @@
* @param {Array<Array<T>>} array
* @returns {Array<T>}
*/
+// TODO: Remove this when we drop support for Node.js v10
// eslint-disable-next-line unicorn/prefer-spread
const flat = (array) => [].concat(...array);
| Can't print comment after directive
**Prettier 2.8.1**
[Playground link](https://prettier.io/playground/#N4Igxg9gdgLgprEAuEByArgZzgAkzAJwEswZUBuAHSmoHoAqHSAW2YRh3tuoG0BdAHQAzCAQCiAQzAALABQBKciAA0ICAAcYRaJmSgJBAhADuABQMJdKCQBtjEgJ67VAIwJSA1nBgBlCWwAZIig4ZCFbbFd3MC9fdSlggHNkQnQ4VThmFzgAExzcgIkoRPQJRLgAMVFmCRgtYuQQCXQYCBUQaRhmGwB1aSJ4THiwOB9LAaIANwGHRrBMZxBg7AIYU3dEmrCI9JAAK0wADx8kmzgARXQIeG2bSJB4ghXGqVEodvViWB6iHJhpZAADgADKpPhBsD13OpGp84CtJqFVABHK7wdYaKxNTAAWhCuVy7QIcFRRGJ6zKWyQ4Tuu2wzCIt3umFOcAAgnViC4WnBTHACEEQky6azLtdQtSdqoYBIXD8-gCkAAmaXuIg2JIAYQgrAkjXhAFZ2lg4AAVWVWGn3SZpACSUHysB8YGImjZDp8MAcZ2FAF9fUA)
<!-- prettier-ignore -->
```sh
--parser acorn
--no-semi
```
**Input:**
<!-- prettier-ignore -->
```jsx
'use strict';
/* comment */
[].forEach();
```
**Output:**
<!-- prettier-ignore -->
```jsx
"use strict"
/* comment */
;[].forEach()
```
**Second Output:**
<!-- prettier-ignore -->
```jsx
Error: Comment "comment" was not printed. Please report this error!
at Object.N [as ensureAllCommentsPrinted] (https://prettier.io/lib/standalone.js:41:7554)
at A (https://prettier.io/lib/standalone.js:41:15193)
at x (https://prettier.io/lib/standalone.js:45:576)
at Object.formatWithCursor (https://prettier.io/lib/standalone.js:116:7283)
at formatCode (https://prettier.io/worker.js:173:21)
at handleFormatMessage (https://prettier.io/worker.js:161:34)
at handleMessage (https://prettier.io/worker.js:77:14)
at self.onmessage (https://prettier.io/worker.js:53:14)
```
**Expected behavior:**
Should be able to print
---
Reproduce in semi mode
**Prettier 2.8.1**
[Playground link](https://prettier.io/playground/#N4Igxg9gdgLgprEAuEByArgZzgAkzAJwEswZUcB6AKh0gFs6EYcqKBuEAGhAgAcYi0TMlABDAgQgB3AAriEwlKIA2U0QE9h3AEYFRYANZwYAZVGMAMkShxkAMxXYdew8ZO991gObJC6ONxwdNpwACahYRaiUF7ool5wAGIQBHSiMAIxyCCi6DAQXCAAFjB0ygDqRUTwmB5gcCYK1UQAbtXq2WCYWiDW2AQwMnpeafaOASAAVpgAHibeynAAiugQ8GPKTiAeBP3Z+ilQhbzEsOVEoTBFyAAcAAzcJxDY5Xq82Sdw-S223ACOq3gQz4ihymAAtDYwmFCgQ4ACiHChvFRkgHJsJtg6ERfAR-NxMAs4ABBDLEbR5OAyOAEKw2DZbQkxRYrNa2NHjbgwUTac6Xa5IABMXL0RGU3gAwhAGKJsl8AKyFLBwAAqPMU6K2LX8AEkoBFYCYwMR+MT9SYYOpFgy4ABfW1AA)
<!-- prettier-ignore -->
```sh
--parser acorn
```
**Input:**
<!-- prettier-ignore -->
```jsx
'use strict' /* comment */;
```
**Output:**
<!-- prettier-ignore -->
```jsx
Error: Comment "comment" was not printed. Please report this error!
at Object.N [as ensureAllCommentsPrinted] (https://prettier.io/lib/standalone.js:41:7554)
at A (https://prettier.io/lib/standalone.js:41:15193)
at x (https://prettier.io/lib/standalone.js:45:576)
at Object.formatWithCursor (https://prettier.io/lib/standalone.js:116:7283)
at formatCode (https://prettier.io/worker.js:173:21)
at handleFormatMessage (https://prettier.io/worker.js:104:24)
at handleMessage (https://prettier.io/worker.js:77:14)
at self.onmessage (https://prettier.io/worker.js:53:14)
```
| "2022-12-29T10:43:25Z" | 2.9 | [] | [
"tests/format/js/directives/comments/jsfmt.spec.js"
] | JavaScript | [] | [
"https://prettier.io/playground/#N4Igxg9gdgLgprEAuEByArgZzgAkzAJwEswZUBuAHSmoHoAqHSAW2YRh3tuoG0BdAHQAzCAQCiAQzAALABQBKciAA0ICAAcYRaJmSgJBAhADuABQMJdKCQBtjEgJ67VAIwJSA1nBgBlCWwAZIig4ZCFbbFd3MC9fdSlggHNkQnQ4VThmFzgAExzcgIkoRPQJRLgAMVFmCRgtYuQQCXQYCBUQaRhmGwB1aSJ4THiwOB9LAaIANwGHRrBMZxBg7AIYU3dEmrCI9JAAK0wADx8kmzgARXQIeG2bSJB4ghXGqVEodvViWB6iHJhpZAADgADKpPhBsD13OpGp84CtJqFVABHK7wdYaKxNTAAWhCuVy7QIcFRRGJ6zKWyQ4Tuu2wzCIt3umFOcAAgnViC4WnBTHACEEQky6azLtdQtSdqoYBIXD8-gCkAAmaXuIg2JIAYQgrAkjXhAFZ2lg4AAVWVWGn3SZpACSUHysB8YGImjZDp8MAcZ2FAF9fUA",
"https://prettier.io/playground/#N4Igxg9gdgLgprEAuEByArgZzgAkzAJwEswZUcB6AKh0gFs6EYcqKBuEAGhAgAcYi0TMlABDAgQgB3AAriEwlKIA2U0QE9h3AEYFRYANZwYAZVGMAMkShxkAMxXYdew8ZO991gObJC6ONxwdNpwACahYRaiUF7ool5wAGIQBHSiMAIxyCCi6DAQXCAAFjB0ygDqRUTwmB5gcCYK1UQAbtXq2WCYWiDW2AQwMnpeafaOASAAVpgAHibeynAAiugQ8GPKTiAeBP3Z+ilQhbzEsOVEoTBFyAAcAAzcJxDY5Xq82Sdw-S223ACOq3gQz4ihymAAtDYwmFCgQ4ACiHChvFRkgHJsJtg6ERfAR-NxMAs4ABBDLEbR5OAyOAEKw2DZbQkxRYrNa2NHjbgwUTac6Xa5IABMXL0RGU3gAwhAGKJsl8AKyFLBwAAqPMU6K2LX8AEkoBFYCYwMR+MT9SYYOpFgy4ABfW1AA"
] |
|
prettier/prettier | 14,082 | prettier__prettier-14082 | [
"11242"
] | ef707da0a2cafa56b46a94b865069a3cf401835d | diff --git a/src/language-js/print/jsx.js b/src/language-js/print/jsx.js
--- a/src/language-js/print/jsx.js
+++ b/src/language-js/print/jsx.js
@@ -3,6 +3,7 @@
const {
printComments,
printDanglingComments,
+ printCommentsSeparately,
} = require("../../main/comments.js");
const {
builders: {
@@ -490,7 +491,11 @@ function printJsxAttribute(path, options, print) {
options.jsxSingleQuote ? "'" : '"'
);
final = final.replace(regex, escaped);
- res = [quote, final, quote];
+ const { leading, trailing } = path.call(
+ () => printCommentsSeparately(path, options),
+ "value"
+ );
+ res = [leading, quote, final, quote, trailing];
} else {
res = print("value");
}
@@ -766,7 +771,7 @@ function printJsx(path, options, print) {
return printJsxEmptyExpression(path, options /*, print*/);
case "JSXText":
/* istanbul ignore next */
- throw new Error("JSXTest should be handled by JSXElement");
+ throw new Error("JSXText should be handled by JSXElement");
default:
/* istanbul ignore next */
throw new Error(`Unknown JSX node type: ${JSON.stringify(node.type)}.`);
| diff --git a/tests/config/format-test.js b/tests/config/format-test.js
--- a/tests/config/format-test.js
+++ b/tests/config/format-test.js
@@ -42,6 +42,7 @@ const unstableTests = new Map(
"js/for/continue-and-break-comment-without-blocks.js",
"typescript/satisfies-operators/comments-unstable.ts",
["js/identifier/parentheses/let.js", (options) => options.semi === false],
+ "jsx/comments/in-attributes.js",
].map((fixture) => {
const [file, isUnstable = () => true] = Array.isArray(fixture)
? fixture
diff --git a/tests/format/jsx/comments/__snapshots__/jsfmt.spec.js.snap b/tests/format/jsx/comments/__snapshots__/jsfmt.spec.js.snap
--- a/tests/format/jsx/comments/__snapshots__/jsfmt.spec.js.snap
+++ b/tests/format/jsx/comments/__snapshots__/jsfmt.spec.js.snap
@@ -27,6 +27,62 @@ const render = (items) => (
================================================================================
`;
+exports[`in-attributes.js - {"bracketSameLine":true} format 1`] = `
+====================================options=====================================
+bracketSameLine: true
+parsers: ["flow", "babel", "typescript"]
+printWidth: 80
+ | printWidth
+=====================================input======================================
+<div
+ attr=/* comment */"foo"
+></div>;
+
+<div
+ attr=
+ /* comment */
+ "foo"
+></div>;
+
+<div
+ attr= /* comment */
+ "foo"
+></div>;
+
+<div
+ attr=
+ /* comment */ "foo"
+></div>;
+
+<div
+ attr=
+ // comment
+ "foo"
+></div>;
+
+<div
+ attr= // comment
+ "foo"
+></div>;
+
+=====================================output=====================================
+<div attr=/* comment */ "foo"></div>;
+
+<div attr=/* comment */
+"foo"></div>;
+
+<div attr /* comment */="foo"></div>;
+
+<div attr=/* comment */ "foo"></div>;
+
+<div attr=// comment
+"foo"></div>;
+
+<div attr="foo"></div>; // comment
+
+================================================================================
+`;
+
exports[`in-end-tag.js - {"bracketSameLine":true} [typescript] format 1`] = `
"Identifier expected. (2:6)
1 | /* =========== before slash =========== */
diff --git a/tests/format/jsx/comments/in-attributes.js b/tests/format/jsx/comments/in-attributes.js
new file mode 100644
--- /dev/null
+++ b/tests/format/jsx/comments/in-attributes.js
@@ -0,0 +1,30 @@
+<div
+ attr=/* comment */"foo"
+></div>;
+
+<div
+ attr=
+ /* comment */
+ "foo"
+></div>;
+
+<div
+ attr= /* comment */
+ "foo"
+></div>;
+
+<div
+ attr=
+ /* comment */ "foo"
+></div>;
+
+<div
+ attr=
+ // comment
+ "foo"
+></div>;
+
+<div
+ attr= // comment
+ "foo"
+></div>;
| Using inline comments (/* */) before an element attribute's value in JSX causes document to not be formatted
**Prettier 2.3.2**
[Playground link](https://prettier.io/playground/#N4Igxg9gdgLgprEAuEAzArlMMCW0AEAsgJ4Bim2eUAwhALYAO0CMAFAJT7AA6U3MAHgBG6GDAIAbAIZC4EgLwB6AFRxli7iDiaAfCLHQBi-eKg7eAXxAAaEBAa5oAZ2SgpAJ3cQA7gAUPCC4oUhLeUsQutkLuUmAA1nAwAMoMsThQAObIMO7ocLZwdLIAJsVwxQAyUpnoUhlwpBDudFJi6VnBohA2IAAWMHQSAOq9OPBOqWBwSYFjOABuY8TI4E6RIOlOcO4wvjEZLcioIVu2AFZOAB4AQjHxiUlSdHAV6XBHJ-kgF5dJ7RJwACK6Ag8A+ElOIFS7i27hWQhkch6DHc6RgQxwxRgvWQAA4AAy2FEQLZDGIMFYouCw+bvWwARxB8D29iCICkTgAtFA4OVyj13HBGThBXs6ockMcIV8tnQcNlcjL-kCme9JZ9bDAZBisTikAAmTUxHASdq0OgSrROACsPXQWwAKjIglLIfM8gBJKBlWBJMCohwAQW9SRgxAB4K2FgsQA)
<!-- prettier-ignore -->
```sh
--parser babel
```
**Input:**
<!-- prettier-ignore -->
```jsx
function MyFunctionComponent() {
<button label=/*old*/"new">button</button>
}
```
**Output:**
<!-- prettier-ignore -->
```jsx
Error: Comment "old" was not printed. Please report this error!
at Object.ensureAllCommentsPrinted (https://prettier.io/lib/standalone.js:36:119166)
at Sd (https://prettier.io/lib/standalone.js:36:125590)
at Nd (https://prettier.io/lib/standalone.js:36:128103)
at Object.formatWithCursor (https://prettier.io/lib/standalone.js:76:38121)
at formatCode (https://prettier.io/worker.js:141:21)
at handleMessage (https://prettier.io/worker.js:78:26)
at self.onmessage (https://prettier.io/worker.js:36:14)
```
**Expected behaviour:**
The document should be formatted as usual
| Workaround
**Prettier 2.3.2**
[Playground link](https://prettier.io/playground/#N4Igxg9gdgLgprEAuEAzArlMMCW0AEAsgJ4Bim2eUAwhALYAO0CMAFAJT7AA6U3MAHgBG6GDAIAbAIZC4EgLwB6AFRxliniDjcQAXwB8IsdAGKj4qPt66QAGhAQGuaAGdkoKQCdPEAO4AFLwQ3FCkJXyliN3shTykwAGs4GABlBnicKABzZBhPdDh7ODpZABNSuFKAGSls9CksuFIITzopMUyc0NEIOxAACxg6CQB1fpx4F3SwOBTgiZwANwniZHAXaJBMlzhPGH84rLbkVDCd+wArFwAPACE4xOSUqTo4Ksy4E7PCkCvrlM6EjgAEV0BB4F8JOcQOlPDtPGshDI5H0GJ5MjARjhSjB+sgABwABnsaIgOxGcQYazRcHhi0+9gAjmD4AdHCEQFIXABaKBwSqVPqeODMnDCg4NY5IU5Qn47Og4XL5OWAkEsz7S772GAyLE4vFIABM2riOAknVodClWhcAFY+ugdgAVGQhGXQxYFACSUAqsBSYHRTgAgr6UjBiEDITtdLogA)
<!-- prettier-ignore -->
```sh
--parser babel
```
**Input:**
<!-- prettier-ignore -->
```jsx
function MyFunctionComponent() {
<button label=/*e*/{"e"}>button</button>
}
```
**Output:**
<!-- prettier-ignore -->
```jsx
function MyFunctionComponent() {
<button label=/*e*/ {"e"}>button</button>;
}
``` | "2022-12-29T11:07:25Z" | 2.9 | [] | [
"tests/format/jsx/comments/jsfmt.spec.js"
] | JavaScript | [] | [
"https://prettier.io/playground/#N4Igxg9gdgLgprEAuEAzArlMMCW0AEAsgJ4Bim2eUAwhALYAO0CMAFAJT7AA6U3MAHgBG6GDAIAbAIZC4EgLwB6AFRxli7iDiaAfCLHQBi-eKg7eAXxAAaEBAa5oAZ2SgpAJ3cQA7gAUPCC4oUhLeUsQutkLuUmAA1nAwAMoMsThQAObIMO7ocLZwdLIAJsVwxQAyUpnoUhlwpBDudFJi6VnBohA2IAAWMHQSAOq9OPBOqWBwSYFjOABuY8TI4E6RIOlOcO4wvjEZLcioIVu2AFZOAB4AQjHxiUlSdHAV6XBHJ-kgF5dJ7RJwACK6Ag8A+ElOIFS7i27hWQhkch6DHc6RgQxwxRgvWQAA4AAy2FEQLZDGIMFYouCw+bvWwARxB8D29iCICkTgAtFA4OVyj13HBGThBXs6ockMcIV8tnQcNlcjL-kCme9JZ9bDAZBisTikAAmTUxHASdq0OgSrROACsPXQWwAKjIglLIfM8gBJKBlWBJMCohwAQW9SRgxAB4K2FgsQA"
] |
prettier/prettier | 14,085 | prettier__prettier-14085 | [
"3722",
"6198"
] | be84156d43805a281369c934ae28148065d6316e | diff --git a/src/language-js/print/type-parameters.js b/src/language-js/print/type-parameters.js
--- a/src/language-js/print/type-parameters.js
+++ b/src/language-js/print/type-parameters.js
@@ -79,7 +79,7 @@ function printTypeParameters(path, options, print, paramsKey) {
!node[paramsKey][0].constraint &&
path.parent.type === "ArrowFunctionExpression"
? ","
- : shouldPrintComma(options, "all")
+ : shouldPrintComma(options)
? ifBreak(",")
: "";
| diff --git a/tests/format/flow/function-parentheses/__snapshots__/jsfmt.spec.js.snap b/tests/format/flow/function-parentheses/__snapshots__/jsfmt.spec.js.snap
--- a/tests/format/flow/function-parentheses/__snapshots__/jsfmt.spec.js.snap
+++ b/tests/format/flow/function-parentheses/__snapshots__/jsfmt.spec.js.snap
@@ -69,7 +69,7 @@ const selectorByPath:
const selectorByPath: (Path) => SomethingSelector<
SomethingUEditorContextType,
SomethingUEditorContextType,
- SomethingBulkValue<string>
+ SomethingBulkValue<string>,
> = memoizeWithArgs(/* ... */);
================================================================================
diff --git a/tests/format/flow/generic/__snapshots__/jsfmt.spec.js.snap b/tests/format/flow/generic/__snapshots__/jsfmt.spec.js.snap
--- a/tests/format/flow/generic/__snapshots__/jsfmt.spec.js.snap
+++ b/tests/format/flow/generic/__snapshots__/jsfmt.spec.js.snap
@@ -63,7 +63,7 @@ var X = {
E,
F,
G,
- T: (a: A, b: B, c: C, d: D, e: E, f: F) => G // eslint-disable-line space-before-function-paren
+ T: (a: A, b: B, c: C, d: D, e: E, f: F) => G, // eslint-disable-line space-before-function-paren
>(method: T, scope: any, a: A, b: B, c: C, d: D, e: E, f: F): G {},
};
@@ -316,7 +316,7 @@ type State = {
type State = {
errors: Immutable.Map<
Ahohohhohohohohohohohohohohooh,
- Fbt | Immutable.Map<ErrorIndex, Fbt>
+ Fbt | Immutable.Map<ErrorIndex, Fbt>,
>,
shouldValidate: boolean,
};
@@ -398,7 +398,7 @@ type Foo = Promise<
=====================================output=====================================
type Foo = Promise<
| { ok: true, bar: string, baz: SomeOtherLongType }
- | { ok: false, bar: SomeOtherLongType }
+ | { ok: false, bar: SomeOtherLongType },
>;
================================================================================
diff --git a/tests/format/flow/interface-types/break/__snapshots__/jsfmt.spec.js.snap b/tests/format/flow/interface-types/break/__snapshots__/jsfmt.spec.js.snap
new file mode 100644
--- /dev/null
+++ b/tests/format/flow/interface-types/break/__snapshots__/jsfmt.spec.js.snap
@@ -0,0 +1,544 @@
+// Jest Snapshot v1, https://goo.gl/fbAQLP
+
+exports[`break.js - {"trailingComma":"all"} format 1`] = `
+====================================options=====================================
+parsers: ["flow"]
+printWidth: 80
+trailingComma: "all"
+ | printWidth
+=====================================input======================================
+export interface Environment1 extends GenericEnvironment<
+ SomeType,
+ AnotherType,
+ YetAnotherType,
+> {
+ m(): void;
+};
+export class Environment2 extends GenericEnvironment<
+ SomeType,
+ AnotherType,
+ YetAnotherType,
+ DifferentType1,
+ DifferentType2,
+ DifferentType3,
+ DifferentType4,
+> {
+ m() {};
+};
+
+// Declare Interface Break
+declare interface ExtendsOne extends ASingleInterface {
+ x: string;
+}
+
+declare interface ExtendsLarge extends ASingleInterfaceWithAReallyReallyReallyReallyLongName {
+ x: string;
+}
+
+declare interface ExtendsMany extends Interface1, Interface2, Interface3, Interface4, Interface5, Interface6, Interface7 {
+ x: string;
+}
+
+// Interface declaration break
+interface ExtendsOne extends ASingleInterface {
+ x: string;
+}
+
+interface ExtendsLarge extends ASingleInterfaceWithAReallyReallyReallyReallyLongName {
+ x: string;
+}
+
+interface ExtendsMany extends Interface1, Interface2, Interface3, Interface4, Interface5, Interface6, Interface7 {
+ s: string;
+}
+
+// Generic Types
+interface ExtendsOne extends ASingleInterface<string> {
+ x: string;
+}
+
+interface ExtendsLarge extends ASingleInterfaceWithAReallyReallyReallyReallyLongName<string> {
+ x: string;
+}
+
+interface ExtendsMany
+ extends ASingleGenericInterface<Interface1, Interface2, Interface3, Interface4, Interface5, Interface6, Interface7> {
+ x: string;
+}
+
+interface ExtendsManyWithGenerics
+ extends InterfaceOne, InterfaceTwo, ASingleGenericInterface<Interface1, Interface2, Interface3, Interface4, Interface5, Interface6, Interface7>, InterfaceThree {
+
+ x: string;
+ }
+
+export interface ExtendsLongOneWithGenerics extends Bar< SomeLongTypeSomeLongTypeSomeLongTypeSomeLongType, ToBreakLineToBreakLineToBreakLine> {}
+
+=====================================output=====================================
+export interface Environment1
+ extends GenericEnvironment<SomeType, AnotherType, YetAnotherType> {
+ m(): void;
+}
+export class Environment2 extends GenericEnvironment<
+ SomeType,
+ AnotherType,
+ YetAnotherType,
+ DifferentType1,
+ DifferentType2,
+ DifferentType3,
+ DifferentType4,
+> {
+ m() {}
+}
+
+// Declare Interface Break
+declare interface ExtendsOne extends ASingleInterface {
+ x: string;
+}
+
+declare interface ExtendsLarge
+ extends ASingleInterfaceWithAReallyReallyReallyReallyLongName {
+ x: string;
+}
+
+declare interface ExtendsMany
+ extends Interface1,
+ Interface2,
+ Interface3,
+ Interface4,
+ Interface5,
+ Interface6,
+ Interface7 {
+ x: string;
+}
+
+// Interface declaration break
+interface ExtendsOne extends ASingleInterface {
+ x: string;
+}
+
+interface ExtendsLarge
+ extends ASingleInterfaceWithAReallyReallyReallyReallyLongName {
+ x: string;
+}
+
+interface ExtendsMany
+ extends Interface1,
+ Interface2,
+ Interface3,
+ Interface4,
+ Interface5,
+ Interface6,
+ Interface7 {
+ s: string;
+}
+
+// Generic Types
+interface ExtendsOne extends ASingleInterface<string> {
+ x: string;
+}
+
+interface ExtendsLarge
+ extends ASingleInterfaceWithAReallyReallyReallyReallyLongName<string> {
+ x: string;
+}
+
+interface ExtendsMany
+ extends ASingleGenericInterface<
+ Interface1,
+ Interface2,
+ Interface3,
+ Interface4,
+ Interface5,
+ Interface6,
+ Interface7,
+ > {
+ x: string;
+}
+
+interface ExtendsManyWithGenerics
+ extends InterfaceOne,
+ InterfaceTwo,
+ ASingleGenericInterface<
+ Interface1,
+ Interface2,
+ Interface3,
+ Interface4,
+ Interface5,
+ Interface6,
+ Interface7,
+ >,
+ InterfaceThree {
+ x: string;
+}
+
+export interface ExtendsLongOneWithGenerics
+ extends Bar<
+ SomeLongTypeSomeLongTypeSomeLongTypeSomeLongType,
+ ToBreakLineToBreakLineToBreakLine,
+ > {}
+
+================================================================================
+`;
+
+exports[`break.js - {"trailingComma":"es5"} format 1`] = `
+====================================options=====================================
+parsers: ["flow"]
+printWidth: 80
+trailingComma: "es5"
+ | printWidth
+=====================================input======================================
+export interface Environment1 extends GenericEnvironment<
+ SomeType,
+ AnotherType,
+ YetAnotherType,
+> {
+ m(): void;
+};
+export class Environment2 extends GenericEnvironment<
+ SomeType,
+ AnotherType,
+ YetAnotherType,
+ DifferentType1,
+ DifferentType2,
+ DifferentType3,
+ DifferentType4,
+> {
+ m() {};
+};
+
+// Declare Interface Break
+declare interface ExtendsOne extends ASingleInterface {
+ x: string;
+}
+
+declare interface ExtendsLarge extends ASingleInterfaceWithAReallyReallyReallyReallyLongName {
+ x: string;
+}
+
+declare interface ExtendsMany extends Interface1, Interface2, Interface3, Interface4, Interface5, Interface6, Interface7 {
+ x: string;
+}
+
+// Interface declaration break
+interface ExtendsOne extends ASingleInterface {
+ x: string;
+}
+
+interface ExtendsLarge extends ASingleInterfaceWithAReallyReallyReallyReallyLongName {
+ x: string;
+}
+
+interface ExtendsMany extends Interface1, Interface2, Interface3, Interface4, Interface5, Interface6, Interface7 {
+ s: string;
+}
+
+// Generic Types
+interface ExtendsOne extends ASingleInterface<string> {
+ x: string;
+}
+
+interface ExtendsLarge extends ASingleInterfaceWithAReallyReallyReallyReallyLongName<string> {
+ x: string;
+}
+
+interface ExtendsMany
+ extends ASingleGenericInterface<Interface1, Interface2, Interface3, Interface4, Interface5, Interface6, Interface7> {
+ x: string;
+}
+
+interface ExtendsManyWithGenerics
+ extends InterfaceOne, InterfaceTwo, ASingleGenericInterface<Interface1, Interface2, Interface3, Interface4, Interface5, Interface6, Interface7>, InterfaceThree {
+
+ x: string;
+ }
+
+export interface ExtendsLongOneWithGenerics extends Bar< SomeLongTypeSomeLongTypeSomeLongTypeSomeLongType, ToBreakLineToBreakLineToBreakLine> {}
+
+=====================================output=====================================
+export interface Environment1
+ extends GenericEnvironment<SomeType, AnotherType, YetAnotherType> {
+ m(): void;
+}
+export class Environment2 extends GenericEnvironment<
+ SomeType,
+ AnotherType,
+ YetAnotherType,
+ DifferentType1,
+ DifferentType2,
+ DifferentType3,
+ DifferentType4,
+> {
+ m() {}
+}
+
+// Declare Interface Break
+declare interface ExtendsOne extends ASingleInterface {
+ x: string;
+}
+
+declare interface ExtendsLarge
+ extends ASingleInterfaceWithAReallyReallyReallyReallyLongName {
+ x: string;
+}
+
+declare interface ExtendsMany
+ extends Interface1,
+ Interface2,
+ Interface3,
+ Interface4,
+ Interface5,
+ Interface6,
+ Interface7 {
+ x: string;
+}
+
+// Interface declaration break
+interface ExtendsOne extends ASingleInterface {
+ x: string;
+}
+
+interface ExtendsLarge
+ extends ASingleInterfaceWithAReallyReallyReallyReallyLongName {
+ x: string;
+}
+
+interface ExtendsMany
+ extends Interface1,
+ Interface2,
+ Interface3,
+ Interface4,
+ Interface5,
+ Interface6,
+ Interface7 {
+ s: string;
+}
+
+// Generic Types
+interface ExtendsOne extends ASingleInterface<string> {
+ x: string;
+}
+
+interface ExtendsLarge
+ extends ASingleInterfaceWithAReallyReallyReallyReallyLongName<string> {
+ x: string;
+}
+
+interface ExtendsMany
+ extends ASingleGenericInterface<
+ Interface1,
+ Interface2,
+ Interface3,
+ Interface4,
+ Interface5,
+ Interface6,
+ Interface7,
+ > {
+ x: string;
+}
+
+interface ExtendsManyWithGenerics
+ extends InterfaceOne,
+ InterfaceTwo,
+ ASingleGenericInterface<
+ Interface1,
+ Interface2,
+ Interface3,
+ Interface4,
+ Interface5,
+ Interface6,
+ Interface7,
+ >,
+ InterfaceThree {
+ x: string;
+}
+
+export interface ExtendsLongOneWithGenerics
+ extends Bar<
+ SomeLongTypeSomeLongTypeSomeLongTypeSomeLongType,
+ ToBreakLineToBreakLineToBreakLine,
+ > {}
+
+================================================================================
+`;
+
+exports[`break.js - {"trailingComma":"none"} format 1`] = `
+====================================options=====================================
+parsers: ["flow"]
+printWidth: 80
+trailingComma: "none"
+ | printWidth
+=====================================input======================================
+export interface Environment1 extends GenericEnvironment<
+ SomeType,
+ AnotherType,
+ YetAnotherType,
+> {
+ m(): void;
+};
+export class Environment2 extends GenericEnvironment<
+ SomeType,
+ AnotherType,
+ YetAnotherType,
+ DifferentType1,
+ DifferentType2,
+ DifferentType3,
+ DifferentType4,
+> {
+ m() {};
+};
+
+// Declare Interface Break
+declare interface ExtendsOne extends ASingleInterface {
+ x: string;
+}
+
+declare interface ExtendsLarge extends ASingleInterfaceWithAReallyReallyReallyReallyLongName {
+ x: string;
+}
+
+declare interface ExtendsMany extends Interface1, Interface2, Interface3, Interface4, Interface5, Interface6, Interface7 {
+ x: string;
+}
+
+// Interface declaration break
+interface ExtendsOne extends ASingleInterface {
+ x: string;
+}
+
+interface ExtendsLarge extends ASingleInterfaceWithAReallyReallyReallyReallyLongName {
+ x: string;
+}
+
+interface ExtendsMany extends Interface1, Interface2, Interface3, Interface4, Interface5, Interface6, Interface7 {
+ s: string;
+}
+
+// Generic Types
+interface ExtendsOne extends ASingleInterface<string> {
+ x: string;
+}
+
+interface ExtendsLarge extends ASingleInterfaceWithAReallyReallyReallyReallyLongName<string> {
+ x: string;
+}
+
+interface ExtendsMany
+ extends ASingleGenericInterface<Interface1, Interface2, Interface3, Interface4, Interface5, Interface6, Interface7> {
+ x: string;
+}
+
+interface ExtendsManyWithGenerics
+ extends InterfaceOne, InterfaceTwo, ASingleGenericInterface<Interface1, Interface2, Interface3, Interface4, Interface5, Interface6, Interface7>, InterfaceThree {
+
+ x: string;
+ }
+
+export interface ExtendsLongOneWithGenerics extends Bar< SomeLongTypeSomeLongTypeSomeLongTypeSomeLongType, ToBreakLineToBreakLineToBreakLine> {}
+
+=====================================output=====================================
+export interface Environment1
+ extends GenericEnvironment<SomeType, AnotherType, YetAnotherType> {
+ m(): void;
+}
+export class Environment2 extends GenericEnvironment<
+ SomeType,
+ AnotherType,
+ YetAnotherType,
+ DifferentType1,
+ DifferentType2,
+ DifferentType3,
+ DifferentType4
+> {
+ m() {}
+}
+
+// Declare Interface Break
+declare interface ExtendsOne extends ASingleInterface {
+ x: string;
+}
+
+declare interface ExtendsLarge
+ extends ASingleInterfaceWithAReallyReallyReallyReallyLongName {
+ x: string;
+}
+
+declare interface ExtendsMany
+ extends Interface1,
+ Interface2,
+ Interface3,
+ Interface4,
+ Interface5,
+ Interface6,
+ Interface7 {
+ x: string;
+}
+
+// Interface declaration break
+interface ExtendsOne extends ASingleInterface {
+ x: string;
+}
+
+interface ExtendsLarge
+ extends ASingleInterfaceWithAReallyReallyReallyReallyLongName {
+ x: string;
+}
+
+interface ExtendsMany
+ extends Interface1,
+ Interface2,
+ Interface3,
+ Interface4,
+ Interface5,
+ Interface6,
+ Interface7 {
+ s: string;
+}
+
+// Generic Types
+interface ExtendsOne extends ASingleInterface<string> {
+ x: string;
+}
+
+interface ExtendsLarge
+ extends ASingleInterfaceWithAReallyReallyReallyReallyLongName<string> {
+ x: string;
+}
+
+interface ExtendsMany
+ extends ASingleGenericInterface<
+ Interface1,
+ Interface2,
+ Interface3,
+ Interface4,
+ Interface5,
+ Interface6,
+ Interface7
+ > {
+ x: string;
+}
+
+interface ExtendsManyWithGenerics
+ extends InterfaceOne,
+ InterfaceTwo,
+ ASingleGenericInterface<
+ Interface1,
+ Interface2,
+ Interface3,
+ Interface4,
+ Interface5,
+ Interface6,
+ Interface7
+ >,
+ InterfaceThree {
+ x: string;
+}
+
+export interface ExtendsLongOneWithGenerics
+ extends Bar<
+ SomeLongTypeSomeLongTypeSomeLongTypeSomeLongType,
+ ToBreakLineToBreakLineToBreakLine
+ > {}
+
+================================================================================
+`;
diff --git a/tests/format/typescript/interface2/break.ts b/tests/format/flow/interface-types/break/break.js
similarity index 100%
rename from tests/format/typescript/interface2/break.ts
rename to tests/format/flow/interface-types/break/break.js
diff --git a/tests/format/flow/interface-types/break/jsfmt.spec.js b/tests/format/flow/interface-types/break/jsfmt.spec.js
new file mode 100644
--- /dev/null
+++ b/tests/format/flow/interface-types/break/jsfmt.spec.js
@@ -0,0 +1,3 @@
+run_spec(import.meta, ["flow"], { trailingComma: "none" });
+run_spec(import.meta, ["flow"], { trailingComma: "es5" });
+run_spec(import.meta, ["flow"], { trailingComma: "all" });
diff --git a/tests/format/flow/type-parameters/trailing-comma/__snapshots__/jsfmt.spec.js.snap b/tests/format/flow/type-parameters/trailing-comma/__snapshots__/jsfmt.spec.js.snap
new file mode 100644
--- /dev/null
+++ b/tests/format/flow/type-parameters/trailing-comma/__snapshots__/jsfmt.spec.js.snap
@@ -0,0 +1,61 @@
+// Jest Snapshot v1, https://goo.gl/fbAQLP
+
+exports[`type-paramters.js - {"trailingComma":"all"} format 1`] = `
+====================================options=====================================
+parsers: ["flow"]
+printWidth: 80
+trailingComma: "all"
+ | printWidth
+=====================================input======================================
+type FooThisNameIsVeryLongSoThatItBreaksToTheNextLine = Something<FirstParam, SecondParam>;
+type ShortName = Something<FirstParam, SecondParam>;
+
+=====================================output=====================================
+type FooThisNameIsVeryLongSoThatItBreaksToTheNextLine = Something<
+ FirstParam,
+ SecondParam,
+>;
+type ShortName = Something<FirstParam, SecondParam>;
+
+================================================================================
+`;
+
+exports[`type-paramters.js - {"trailingComma":"es5"} format 1`] = `
+====================================options=====================================
+parsers: ["flow"]
+printWidth: 80
+trailingComma: "es5"
+ | printWidth
+=====================================input======================================
+type FooThisNameIsVeryLongSoThatItBreaksToTheNextLine = Something<FirstParam, SecondParam>;
+type ShortName = Something<FirstParam, SecondParam>;
+
+=====================================output=====================================
+type FooThisNameIsVeryLongSoThatItBreaksToTheNextLine = Something<
+ FirstParam,
+ SecondParam,
+>;
+type ShortName = Something<FirstParam, SecondParam>;
+
+================================================================================
+`;
+
+exports[`type-paramters.js - {"trailingComma":"none"} format 1`] = `
+====================================options=====================================
+parsers: ["flow"]
+printWidth: 80
+trailingComma: "none"
+ | printWidth
+=====================================input======================================
+type FooThisNameIsVeryLongSoThatItBreaksToTheNextLine = Something<FirstParam, SecondParam>;
+type ShortName = Something<FirstParam, SecondParam>;
+
+=====================================output=====================================
+type FooThisNameIsVeryLongSoThatItBreaksToTheNextLine = Something<
+ FirstParam,
+ SecondParam
+>;
+type ShortName = Something<FirstParam, SecondParam>;
+
+================================================================================
+`;
diff --git a/tests/format/flow/type-parameters/trailing-comma/jsfmt.spec.js b/tests/format/flow/type-parameters/trailing-comma/jsfmt.spec.js
new file mode 100644
--- /dev/null
+++ b/tests/format/flow/type-parameters/trailing-comma/jsfmt.spec.js
@@ -0,0 +1,3 @@
+run_spec(import.meta, ["flow"], { trailingComma: "none" });
+run_spec(import.meta, ["flow"], { trailingComma: "es5" });
+run_spec(import.meta, ["flow"], { trailingComma: "all" });
diff --git a/tests/format/flow/type-parameters/trailing-comma/type-paramters.js b/tests/format/flow/type-parameters/trailing-comma/type-paramters.js
new file mode 100644
--- /dev/null
+++ b/tests/format/flow/type-parameters/trailing-comma/type-paramters.js
@@ -0,0 +1,2 @@
+type FooThisNameIsVeryLongSoThatItBreaksToTheNextLine = Something<FirstParam, SecondParam>;
+type ShortName = Something<FirstParam, SecondParam>;
diff --git a/tests/format/typescript/interface2/__snapshots__/jsfmt.spec.js.snap b/tests/format/typescript/interface2/__snapshots__/jsfmt.spec.js.snap
--- a/tests/format/typescript/interface2/__snapshots__/jsfmt.spec.js.snap
+++ b/tests/format/typescript/interface2/__snapshots__/jsfmt.spec.js.snap
@@ -1,186 +1,5 @@
// Jest Snapshot v1, https://goo.gl/fbAQLP
-exports[`break.ts - {"trailingComma":"es5"} format 1`] = `
-====================================options=====================================
-parsers: ["typescript", "flow"]
-printWidth: 80
-trailingComma: "es5"
- | printWidth
-=====================================input======================================
-export interface Environment1 extends GenericEnvironment<
- SomeType,
- AnotherType,
- YetAnotherType,
-> {
- m(): void;
-};
-export class Environment2 extends GenericEnvironment<
- SomeType,
- AnotherType,
- YetAnotherType,
- DifferentType1,
- DifferentType2,
- DifferentType3,
- DifferentType4,
-> {
- m() {};
-};
-
-// Declare Interface Break
-declare interface ExtendsOne extends ASingleInterface {
- x: string;
-}
-
-declare interface ExtendsLarge extends ASingleInterfaceWithAReallyReallyReallyReallyLongName {
- x: string;
-}
-
-declare interface ExtendsMany extends Interface1, Interface2, Interface3, Interface4, Interface5, Interface6, Interface7 {
- x: string;
-}
-
-// Interface declaration break
-interface ExtendsOne extends ASingleInterface {
- x: string;
-}
-
-interface ExtendsLarge extends ASingleInterfaceWithAReallyReallyReallyReallyLongName {
- x: string;
-}
-
-interface ExtendsMany extends Interface1, Interface2, Interface3, Interface4, Interface5, Interface6, Interface7 {
- s: string;
-}
-
-// Generic Types
-interface ExtendsOne extends ASingleInterface<string> {
- x: string;
-}
-
-interface ExtendsLarge extends ASingleInterfaceWithAReallyReallyReallyReallyLongName<string> {
- x: string;
-}
-
-interface ExtendsMany
- extends ASingleGenericInterface<Interface1, Interface2, Interface3, Interface4, Interface5, Interface6, Interface7> {
- x: string;
-}
-
-interface ExtendsManyWithGenerics
- extends InterfaceOne, InterfaceTwo, ASingleGenericInterface<Interface1, Interface2, Interface3, Interface4, Interface5, Interface6, Interface7>, InterfaceThree {
-
- x: string;
- }
-
-export interface ExtendsLongOneWithGenerics extends Bar< SomeLongTypeSomeLongTypeSomeLongTypeSomeLongType, ToBreakLineToBreakLineToBreakLine> {}
-
-=====================================output=====================================
-export interface Environment1
- extends GenericEnvironment<SomeType, AnotherType, YetAnotherType> {
- m(): void;
-}
-export class Environment2 extends GenericEnvironment<
- SomeType,
- AnotherType,
- YetAnotherType,
- DifferentType1,
- DifferentType2,
- DifferentType3,
- DifferentType4
-> {
- m() {}
-}
-
-// Declare Interface Break
-declare interface ExtendsOne extends ASingleInterface {
- x: string;
-}
-
-declare interface ExtendsLarge
- extends ASingleInterfaceWithAReallyReallyReallyReallyLongName {
- x: string;
-}
-
-declare interface ExtendsMany
- extends Interface1,
- Interface2,
- Interface3,
- Interface4,
- Interface5,
- Interface6,
- Interface7 {
- x: string;
-}
-
-// Interface declaration break
-interface ExtendsOne extends ASingleInterface {
- x: string;
-}
-
-interface ExtendsLarge
- extends ASingleInterfaceWithAReallyReallyReallyReallyLongName {
- x: string;
-}
-
-interface ExtendsMany
- extends Interface1,
- Interface2,
- Interface3,
- Interface4,
- Interface5,
- Interface6,
- Interface7 {
- s: string;
-}
-
-// Generic Types
-interface ExtendsOne extends ASingleInterface<string> {
- x: string;
-}
-
-interface ExtendsLarge
- extends ASingleInterfaceWithAReallyReallyReallyReallyLongName<string> {
- x: string;
-}
-
-interface ExtendsMany
- extends ASingleGenericInterface<
- Interface1,
- Interface2,
- Interface3,
- Interface4,
- Interface5,
- Interface6,
- Interface7
- > {
- x: string;
-}
-
-interface ExtendsManyWithGenerics
- extends InterfaceOne,
- InterfaceTwo,
- ASingleGenericInterface<
- Interface1,
- Interface2,
- Interface3,
- Interface4,
- Interface5,
- Interface6,
- Interface7
- >,
- InterfaceThree {
- x: string;
-}
-
-export interface ExtendsLongOneWithGenerics
- extends Bar<
- SomeLongTypeSomeLongTypeSomeLongTypeSomeLongType,
- ToBreakLineToBreakLineToBreakLine
- > {}
-
-================================================================================
-`;
-
exports[`comments.ts - {"trailingComma":"es5"} format 1`] = `
====================================options=====================================
parsers: ["typescript", "flow"]
diff --git a/tests/format/typescript/interface2/break/__snapshots__/jsfmt.spec.js.snap b/tests/format/typescript/interface2/break/__snapshots__/jsfmt.spec.js.snap
new file mode 100644
--- /dev/null
+++ b/tests/format/typescript/interface2/break/__snapshots__/jsfmt.spec.js.snap
@@ -0,0 +1,544 @@
+// Jest Snapshot v1, https://goo.gl/fbAQLP
+
+exports[`break.ts - {"trailingComma":"all"} format 1`] = `
+====================================options=====================================
+parsers: ["typescript"]
+printWidth: 80
+trailingComma: "all"
+ | printWidth
+=====================================input======================================
+export interface Environment1 extends GenericEnvironment<
+ SomeType,
+ AnotherType,
+ YetAnotherType,
+> {
+ m(): void;
+};
+export class Environment2 extends GenericEnvironment<
+ SomeType,
+ AnotherType,
+ YetAnotherType,
+ DifferentType1,
+ DifferentType2,
+ DifferentType3,
+ DifferentType4,
+> {
+ m() {};
+};
+
+// Declare Interface Break
+declare interface ExtendsOne extends ASingleInterface {
+ x: string;
+}
+
+declare interface ExtendsLarge extends ASingleInterfaceWithAReallyReallyReallyReallyLongName {
+ x: string;
+}
+
+declare interface ExtendsMany extends Interface1, Interface2, Interface3, Interface4, Interface5, Interface6, Interface7 {
+ x: string;
+}
+
+// Interface declaration break
+interface ExtendsOne extends ASingleInterface {
+ x: string;
+}
+
+interface ExtendsLarge extends ASingleInterfaceWithAReallyReallyReallyReallyLongName {
+ x: string;
+}
+
+interface ExtendsMany extends Interface1, Interface2, Interface3, Interface4, Interface5, Interface6, Interface7 {
+ s: string;
+}
+
+// Generic Types
+interface ExtendsOne extends ASingleInterface<string> {
+ x: string;
+}
+
+interface ExtendsLarge extends ASingleInterfaceWithAReallyReallyReallyReallyLongName<string> {
+ x: string;
+}
+
+interface ExtendsMany
+ extends ASingleGenericInterface<Interface1, Interface2, Interface3, Interface4, Interface5, Interface6, Interface7> {
+ x: string;
+}
+
+interface ExtendsManyWithGenerics
+ extends InterfaceOne, InterfaceTwo, ASingleGenericInterface<Interface1, Interface2, Interface3, Interface4, Interface5, Interface6, Interface7>, InterfaceThree {
+
+ x: string;
+ }
+
+export interface ExtendsLongOneWithGenerics extends Bar< SomeLongTypeSomeLongTypeSomeLongTypeSomeLongType, ToBreakLineToBreakLineToBreakLine> {}
+
+=====================================output=====================================
+export interface Environment1
+ extends GenericEnvironment<SomeType, AnotherType, YetAnotherType> {
+ m(): void;
+}
+export class Environment2 extends GenericEnvironment<
+ SomeType,
+ AnotherType,
+ YetAnotherType,
+ DifferentType1,
+ DifferentType2,
+ DifferentType3,
+ DifferentType4
+> {
+ m() {}
+}
+
+// Declare Interface Break
+declare interface ExtendsOne extends ASingleInterface {
+ x: string;
+}
+
+declare interface ExtendsLarge
+ extends ASingleInterfaceWithAReallyReallyReallyReallyLongName {
+ x: string;
+}
+
+declare interface ExtendsMany
+ extends Interface1,
+ Interface2,
+ Interface3,
+ Interface4,
+ Interface5,
+ Interface6,
+ Interface7 {
+ x: string;
+}
+
+// Interface declaration break
+interface ExtendsOne extends ASingleInterface {
+ x: string;
+}
+
+interface ExtendsLarge
+ extends ASingleInterfaceWithAReallyReallyReallyReallyLongName {
+ x: string;
+}
+
+interface ExtendsMany
+ extends Interface1,
+ Interface2,
+ Interface3,
+ Interface4,
+ Interface5,
+ Interface6,
+ Interface7 {
+ s: string;
+}
+
+// Generic Types
+interface ExtendsOne extends ASingleInterface<string> {
+ x: string;
+}
+
+interface ExtendsLarge
+ extends ASingleInterfaceWithAReallyReallyReallyReallyLongName<string> {
+ x: string;
+}
+
+interface ExtendsMany
+ extends ASingleGenericInterface<
+ Interface1,
+ Interface2,
+ Interface3,
+ Interface4,
+ Interface5,
+ Interface6,
+ Interface7
+ > {
+ x: string;
+}
+
+interface ExtendsManyWithGenerics
+ extends InterfaceOne,
+ InterfaceTwo,
+ ASingleGenericInterface<
+ Interface1,
+ Interface2,
+ Interface3,
+ Interface4,
+ Interface5,
+ Interface6,
+ Interface7
+ >,
+ InterfaceThree {
+ x: string;
+}
+
+export interface ExtendsLongOneWithGenerics
+ extends Bar<
+ SomeLongTypeSomeLongTypeSomeLongTypeSomeLongType,
+ ToBreakLineToBreakLineToBreakLine
+ > {}
+
+================================================================================
+`;
+
+exports[`break.ts - {"trailingComma":"es5"} format 1`] = `
+====================================options=====================================
+parsers: ["typescript"]
+printWidth: 80
+trailingComma: "es5"
+ | printWidth
+=====================================input======================================
+export interface Environment1 extends GenericEnvironment<
+ SomeType,
+ AnotherType,
+ YetAnotherType,
+> {
+ m(): void;
+};
+export class Environment2 extends GenericEnvironment<
+ SomeType,
+ AnotherType,
+ YetAnotherType,
+ DifferentType1,
+ DifferentType2,
+ DifferentType3,
+ DifferentType4,
+> {
+ m() {};
+};
+
+// Declare Interface Break
+declare interface ExtendsOne extends ASingleInterface {
+ x: string;
+}
+
+declare interface ExtendsLarge extends ASingleInterfaceWithAReallyReallyReallyReallyLongName {
+ x: string;
+}
+
+declare interface ExtendsMany extends Interface1, Interface2, Interface3, Interface4, Interface5, Interface6, Interface7 {
+ x: string;
+}
+
+// Interface declaration break
+interface ExtendsOne extends ASingleInterface {
+ x: string;
+}
+
+interface ExtendsLarge extends ASingleInterfaceWithAReallyReallyReallyReallyLongName {
+ x: string;
+}
+
+interface ExtendsMany extends Interface1, Interface2, Interface3, Interface4, Interface5, Interface6, Interface7 {
+ s: string;
+}
+
+// Generic Types
+interface ExtendsOne extends ASingleInterface<string> {
+ x: string;
+}
+
+interface ExtendsLarge extends ASingleInterfaceWithAReallyReallyReallyReallyLongName<string> {
+ x: string;
+}
+
+interface ExtendsMany
+ extends ASingleGenericInterface<Interface1, Interface2, Interface3, Interface4, Interface5, Interface6, Interface7> {
+ x: string;
+}
+
+interface ExtendsManyWithGenerics
+ extends InterfaceOne, InterfaceTwo, ASingleGenericInterface<Interface1, Interface2, Interface3, Interface4, Interface5, Interface6, Interface7>, InterfaceThree {
+
+ x: string;
+ }
+
+export interface ExtendsLongOneWithGenerics extends Bar< SomeLongTypeSomeLongTypeSomeLongTypeSomeLongType, ToBreakLineToBreakLineToBreakLine> {}
+
+=====================================output=====================================
+export interface Environment1
+ extends GenericEnvironment<SomeType, AnotherType, YetAnotherType> {
+ m(): void;
+}
+export class Environment2 extends GenericEnvironment<
+ SomeType,
+ AnotherType,
+ YetAnotherType,
+ DifferentType1,
+ DifferentType2,
+ DifferentType3,
+ DifferentType4
+> {
+ m() {}
+}
+
+// Declare Interface Break
+declare interface ExtendsOne extends ASingleInterface {
+ x: string;
+}
+
+declare interface ExtendsLarge
+ extends ASingleInterfaceWithAReallyReallyReallyReallyLongName {
+ x: string;
+}
+
+declare interface ExtendsMany
+ extends Interface1,
+ Interface2,
+ Interface3,
+ Interface4,
+ Interface5,
+ Interface6,
+ Interface7 {
+ x: string;
+}
+
+// Interface declaration break
+interface ExtendsOne extends ASingleInterface {
+ x: string;
+}
+
+interface ExtendsLarge
+ extends ASingleInterfaceWithAReallyReallyReallyReallyLongName {
+ x: string;
+}
+
+interface ExtendsMany
+ extends Interface1,
+ Interface2,
+ Interface3,
+ Interface4,
+ Interface5,
+ Interface6,
+ Interface7 {
+ s: string;
+}
+
+// Generic Types
+interface ExtendsOne extends ASingleInterface<string> {
+ x: string;
+}
+
+interface ExtendsLarge
+ extends ASingleInterfaceWithAReallyReallyReallyReallyLongName<string> {
+ x: string;
+}
+
+interface ExtendsMany
+ extends ASingleGenericInterface<
+ Interface1,
+ Interface2,
+ Interface3,
+ Interface4,
+ Interface5,
+ Interface6,
+ Interface7
+ > {
+ x: string;
+}
+
+interface ExtendsManyWithGenerics
+ extends InterfaceOne,
+ InterfaceTwo,
+ ASingleGenericInterface<
+ Interface1,
+ Interface2,
+ Interface3,
+ Interface4,
+ Interface5,
+ Interface6,
+ Interface7
+ >,
+ InterfaceThree {
+ x: string;
+}
+
+export interface ExtendsLongOneWithGenerics
+ extends Bar<
+ SomeLongTypeSomeLongTypeSomeLongTypeSomeLongType,
+ ToBreakLineToBreakLineToBreakLine
+ > {}
+
+================================================================================
+`;
+
+exports[`break.ts - {"trailingComma":"none"} format 1`] = `
+====================================options=====================================
+parsers: ["typescript"]
+printWidth: 80
+trailingComma: "none"
+ | printWidth
+=====================================input======================================
+export interface Environment1 extends GenericEnvironment<
+ SomeType,
+ AnotherType,
+ YetAnotherType,
+> {
+ m(): void;
+};
+export class Environment2 extends GenericEnvironment<
+ SomeType,
+ AnotherType,
+ YetAnotherType,
+ DifferentType1,
+ DifferentType2,
+ DifferentType3,
+ DifferentType4,
+> {
+ m() {};
+};
+
+// Declare Interface Break
+declare interface ExtendsOne extends ASingleInterface {
+ x: string;
+}
+
+declare interface ExtendsLarge extends ASingleInterfaceWithAReallyReallyReallyReallyLongName {
+ x: string;
+}
+
+declare interface ExtendsMany extends Interface1, Interface2, Interface3, Interface4, Interface5, Interface6, Interface7 {
+ x: string;
+}
+
+// Interface declaration break
+interface ExtendsOne extends ASingleInterface {
+ x: string;
+}
+
+interface ExtendsLarge extends ASingleInterfaceWithAReallyReallyReallyReallyLongName {
+ x: string;
+}
+
+interface ExtendsMany extends Interface1, Interface2, Interface3, Interface4, Interface5, Interface6, Interface7 {
+ s: string;
+}
+
+// Generic Types
+interface ExtendsOne extends ASingleInterface<string> {
+ x: string;
+}
+
+interface ExtendsLarge extends ASingleInterfaceWithAReallyReallyReallyReallyLongName<string> {
+ x: string;
+}
+
+interface ExtendsMany
+ extends ASingleGenericInterface<Interface1, Interface2, Interface3, Interface4, Interface5, Interface6, Interface7> {
+ x: string;
+}
+
+interface ExtendsManyWithGenerics
+ extends InterfaceOne, InterfaceTwo, ASingleGenericInterface<Interface1, Interface2, Interface3, Interface4, Interface5, Interface6, Interface7>, InterfaceThree {
+
+ x: string;
+ }
+
+export interface ExtendsLongOneWithGenerics extends Bar< SomeLongTypeSomeLongTypeSomeLongTypeSomeLongType, ToBreakLineToBreakLineToBreakLine> {}
+
+=====================================output=====================================
+export interface Environment1
+ extends GenericEnvironment<SomeType, AnotherType, YetAnotherType> {
+ m(): void;
+}
+export class Environment2 extends GenericEnvironment<
+ SomeType,
+ AnotherType,
+ YetAnotherType,
+ DifferentType1,
+ DifferentType2,
+ DifferentType3,
+ DifferentType4
+> {
+ m() {}
+}
+
+// Declare Interface Break
+declare interface ExtendsOne extends ASingleInterface {
+ x: string;
+}
+
+declare interface ExtendsLarge
+ extends ASingleInterfaceWithAReallyReallyReallyReallyLongName {
+ x: string;
+}
+
+declare interface ExtendsMany
+ extends Interface1,
+ Interface2,
+ Interface3,
+ Interface4,
+ Interface5,
+ Interface6,
+ Interface7 {
+ x: string;
+}
+
+// Interface declaration break
+interface ExtendsOne extends ASingleInterface {
+ x: string;
+}
+
+interface ExtendsLarge
+ extends ASingleInterfaceWithAReallyReallyReallyReallyLongName {
+ x: string;
+}
+
+interface ExtendsMany
+ extends Interface1,
+ Interface2,
+ Interface3,
+ Interface4,
+ Interface5,
+ Interface6,
+ Interface7 {
+ s: string;
+}
+
+// Generic Types
+interface ExtendsOne extends ASingleInterface<string> {
+ x: string;
+}
+
+interface ExtendsLarge
+ extends ASingleInterfaceWithAReallyReallyReallyReallyLongName<string> {
+ x: string;
+}
+
+interface ExtendsMany
+ extends ASingleGenericInterface<
+ Interface1,
+ Interface2,
+ Interface3,
+ Interface4,
+ Interface5,
+ Interface6,
+ Interface7
+ > {
+ x: string;
+}
+
+interface ExtendsManyWithGenerics
+ extends InterfaceOne,
+ InterfaceTwo,
+ ASingleGenericInterface<
+ Interface1,
+ Interface2,
+ Interface3,
+ Interface4,
+ Interface5,
+ Interface6,
+ Interface7
+ >,
+ InterfaceThree {
+ x: string;
+}
+
+export interface ExtendsLongOneWithGenerics
+ extends Bar<
+ SomeLongTypeSomeLongTypeSomeLongTypeSomeLongType,
+ ToBreakLineToBreakLineToBreakLine
+ > {}
+
+================================================================================
+`;
diff --git a/tests/format/typescript/interface2/break/break.ts b/tests/format/typescript/interface2/break/break.ts
new file mode 100644
--- /dev/null
+++ b/tests/format/typescript/interface2/break/break.ts
@@ -0,0 +1,66 @@
+export interface Environment1 extends GenericEnvironment<
+ SomeType,
+ AnotherType,
+ YetAnotherType,
+> {
+ m(): void;
+};
+export class Environment2 extends GenericEnvironment<
+ SomeType,
+ AnotherType,
+ YetAnotherType,
+ DifferentType1,
+ DifferentType2,
+ DifferentType3,
+ DifferentType4,
+> {
+ m() {};
+};
+
+// Declare Interface Break
+declare interface ExtendsOne extends ASingleInterface {
+ x: string;
+}
+
+declare interface ExtendsLarge extends ASingleInterfaceWithAReallyReallyReallyReallyLongName {
+ x: string;
+}
+
+declare interface ExtendsMany extends Interface1, Interface2, Interface3, Interface4, Interface5, Interface6, Interface7 {
+ x: string;
+}
+
+// Interface declaration break
+interface ExtendsOne extends ASingleInterface {
+ x: string;
+}
+
+interface ExtendsLarge extends ASingleInterfaceWithAReallyReallyReallyReallyLongName {
+ x: string;
+}
+
+interface ExtendsMany extends Interface1, Interface2, Interface3, Interface4, Interface5, Interface6, Interface7 {
+ s: string;
+}
+
+// Generic Types
+interface ExtendsOne extends ASingleInterface<string> {
+ x: string;
+}
+
+interface ExtendsLarge extends ASingleInterfaceWithAReallyReallyReallyReallyLongName<string> {
+ x: string;
+}
+
+interface ExtendsMany
+ extends ASingleGenericInterface<Interface1, Interface2, Interface3, Interface4, Interface5, Interface6, Interface7> {
+ x: string;
+}
+
+interface ExtendsManyWithGenerics
+ extends InterfaceOne, InterfaceTwo, ASingleGenericInterface<Interface1, Interface2, Interface3, Interface4, Interface5, Interface6, Interface7>, InterfaceThree {
+
+ x: string;
+ }
+
+export interface ExtendsLongOneWithGenerics extends Bar< SomeLongTypeSomeLongTypeSomeLongTypeSomeLongType, ToBreakLineToBreakLineToBreakLine> {}
diff --git a/tests/format/typescript/interface2/break/jsfmt.spec.js b/tests/format/typescript/interface2/break/jsfmt.spec.js
new file mode 100644
--- /dev/null
+++ b/tests/format/typescript/interface2/break/jsfmt.spec.js
@@ -0,0 +1,3 @@
+run_spec(import.meta, ["typescript"], { trailingComma: "none" });
+run_spec(import.meta, ["typescript"], { trailingComma: "es5" });
+run_spec(import.meta, ["typescript"], { trailingComma: "all" });
diff --git a/tests/format/typescript/trailing-comma/__snapshots__/jsfmt.spec.js.snap b/tests/format/typescript/trailing-comma/__snapshots__/jsfmt.spec.js.snap
--- a/tests/format/typescript/trailing-comma/__snapshots__/jsfmt.spec.js.snap
+++ b/tests/format/typescript/trailing-comma/__snapshots__/jsfmt.spec.js.snap
@@ -133,7 +133,7 @@ const {
=====================================output=====================================
export class BaseSingleLevelProfileTargeting<
- T extends ValidSingleLevelProfileNode
+ T extends ValidSingleLevelProfileNode,
> {}
enum Enum {
diff --git a/tests/format/typescript/typeparams/trailing-comma/__snapshots__/jsfmt.spec.js.snap b/tests/format/typescript/typeparams/trailing-comma/__snapshots__/jsfmt.spec.js.snap
new file mode 100644
--- /dev/null
+++ b/tests/format/typescript/typeparams/trailing-comma/__snapshots__/jsfmt.spec.js.snap
@@ -0,0 +1,61 @@
+// Jest Snapshot v1, https://goo.gl/fbAQLP
+
+exports[`type-paramters.ts - {"trailingComma":"all"} format 1`] = `
+====================================options=====================================
+parsers: ["typescript"]
+printWidth: 80
+trailingComma: "all"
+ | printWidth
+=====================================input======================================
+type FooThisNameIsVeryLongSoThatItBreaksToTheNextLine = Something<FirstParam, SecondParam>;
+type ShortName = Something<FirstParam, SecondParam>;
+
+=====================================output=====================================
+type FooThisNameIsVeryLongSoThatItBreaksToTheNextLine = Something<
+ FirstParam,
+ SecondParam
+>;
+type ShortName = Something<FirstParam, SecondParam>;
+
+================================================================================
+`;
+
+exports[`type-paramters.ts - {"trailingComma":"es5"} format 1`] = `
+====================================options=====================================
+parsers: ["typescript"]
+printWidth: 80
+trailingComma: "es5"
+ | printWidth
+=====================================input======================================
+type FooThisNameIsVeryLongSoThatItBreaksToTheNextLine = Something<FirstParam, SecondParam>;
+type ShortName = Something<FirstParam, SecondParam>;
+
+=====================================output=====================================
+type FooThisNameIsVeryLongSoThatItBreaksToTheNextLine = Something<
+ FirstParam,
+ SecondParam
+>;
+type ShortName = Something<FirstParam, SecondParam>;
+
+================================================================================
+`;
+
+exports[`type-paramters.ts - {"trailingComma":"none"} format 1`] = `
+====================================options=====================================
+parsers: ["typescript"]
+printWidth: 80
+trailingComma: "none"
+ | printWidth
+=====================================input======================================
+type FooThisNameIsVeryLongSoThatItBreaksToTheNextLine = Something<FirstParam, SecondParam>;
+type ShortName = Something<FirstParam, SecondParam>;
+
+=====================================output=====================================
+type FooThisNameIsVeryLongSoThatItBreaksToTheNextLine = Something<
+ FirstParam,
+ SecondParam
+>;
+type ShortName = Something<FirstParam, SecondParam>;
+
+================================================================================
+`;
diff --git a/tests/format/typescript/typeparams/trailing-comma/jsfmt.spec.js b/tests/format/typescript/typeparams/trailing-comma/jsfmt.spec.js
new file mode 100644
--- /dev/null
+++ b/tests/format/typescript/typeparams/trailing-comma/jsfmt.spec.js
@@ -0,0 +1,3 @@
+run_spec(import.meta, ["typescript"], { trailingComma: "none" });
+run_spec(import.meta, ["typescript"], { trailingComma: "es5" });
+run_spec(import.meta, ["typescript"], { trailingComma: "all" });
diff --git a/tests/format/typescript/typeparams/trailing-comma/type-paramters.ts b/tests/format/typescript/typeparams/trailing-comma/type-paramters.ts
new file mode 100644
--- /dev/null
+++ b/tests/format/typescript/typeparams/trailing-comma/type-paramters.ts
@@ -0,0 +1,2 @@
+type FooThisNameIsVeryLongSoThatItBreaksToTheNextLine = Something<FirstParam, SecondParam>;
+type ShortName = Something<FirstParam, SecondParam>;
| Trailing commas for type parameters in Flow with --trailing-comma=es5
I'm not sure if this is the correct behavior or not, but I just moved from `trailingComma: "all"` to `trailingComma: "es5"` in our codebase at work, and was surprised to see these ones disappear.
**Prettier 1.9.2**
[Playground link](https://prettier.io/playground/#N4Igxg9gdgLgprEAuEMCeAHOACAYhCAFQAsBLAZwDkBDAWzgElyA1OAJzQBloBzAZSLFqMBjABCbONQDW5QoLiU4ADxidSUHAF5sA+jDJQeAHlyk25GAAVqbOgBpdcSFAAmNu7QB8AbhD2QCAwYUmhyZFBbNggAdw8EcJRqADcIUld-EGpLZBg2AFc4AIAjOzBpOBg+DGowDR5cgqKQVwgwZAAzagAbcmaNPrZrOx5aak6evoCAK3JlCVqKqro4dU0J3uaIfJgMHYAmDamQGot2ZBBi6mK0buhMjDYNGAB1dINkAA4ABgDHiD6LzsGAujzgg2ScEykgAjvlzHArCMxkdmn1aKRGoUAuR6t04ABFfIQeCogIwa5vVwfJD7cl2UjdeoAYQgtBRKHBAFZMvk+oRrokupsAL4ioA)
```sh
--trailing-comma es5
```
**Input:**
```jsx
type FooThisNameIsVeryLongSoThatItBreaksToTheNextLine = Something<FirstParam, SecondParam>;
```
**Output:**
```jsx
type FooThisNameIsVeryLongSoThatItBreaksToTheNextLine = Something<
FirstParam,
SecondParam
>;
```
**Prettier 1.9.2**
[Playground link](https://prettier.io/playground/#N4Igxg9gdgLgprEAuEMCeAHOACAYhCAFQAsBLAZwDkBDAWzgElyA1OAJzQBloBzAZSLFqMBjABCbONQDW5QoLiU4ADxidSUHAF5sA+jDJQeAHlyk25GAAVqbOgBpdcSFAAmNu7QB8AbhD2QCAwYUmhyZFBbNggAdw8EcJRqADcIUld-EGpLZBg2AFc4AIAjOzBpOBg+DGowDR5cgqKQVwgwZAAzagAbcmaNPrZrOx5aak6evoCAK3JlCVqKqro4dU0J3uaIfJgMHYAmDamQGot2ZBBi6mK0buhMjDYNGAB1dINkAA4ABgDHiD6LzsGAujzgg2ScEykgAjvlzHArCMxkdmn1aKRGoUAuR6t04ABFfIQeCogIwa5vVwfJD7cl2UjdeoAYQgtBRSW63Uy+T6hGuiS6mwAvsKgA)
```sh
--trailing-comma all
```
**Input:**
```jsx
type FooThisNameIsVeryLongSoThatItBreaksToTheNextLine = Something<FirstParam, SecondParam>;
```
**Output:**
```jsx
type FooThisNameIsVeryLongSoThatItBreaksToTheNextLine = Something<
FirstParam,
SecondParam,
>;
```
This affects both flow and typescript.
Seeing as type parameters aren't valid ES5 anyway, should we maybe include trailing commas there even when using `trailingComma: "es5"`?
Epic: Trailing commas in TS/Flow
This is an old problem and I thought I had an issue open to discuss this topic, but we should make a decision on this matter:
How do we handle trailing commas in different versions of Flow/TS?
Should we add `flowXXX` and `tsX.X` options?
Should we always support new trailing commas in `"all"` and treat `"es5"` as `"none"`?
cc @prettier/core
| Related: https://github.com/prettier/prettier/issues/3662 https://github.com/prettier/prettier/pull/3313
Following that logic we could consider that `"es5" == "all"` since it's valid in TS anyway, not ES :joy:
Yeah I guess that's kinda weird... I'm looking at it from the flow perspective, though.
@suchipi looks like an oversight to me. Feel free to fix it :)
From memory, older versions of TS don't support trailing commas here, I think it was intentional. Might want to double check
Issues raised on this topic: #6197 #3722 #3662 #3313
> Should we add flowXXX and tsX.X options?
I think yes, we planned same for php plugin, sometimes languages change syntax
> Should we always support new trailing commas in "all" and treat "es5" as "none"?
To avoid breaking code we should considered:
- `all` and `tsX.X`/`flowXXX` together (`all` + `ts3.3` !== `all` +`ts3.5` )
- with `none` no problems
- `es5` was bad idea, i don't know here solution, maybe apply trailing comma for lower level (i.e. apply commas only for lower ts/flow version like we use es5 and lower ts/flow version)
- https://github.com/prettier/plugin-php/issues/964#issuecomment-465558448
> - Personally, I think we should replace `--trailing-comma <none|es5|all>` with `--[no-]trailing-comma`/`--js-trailing-comma es5`, but unfortunately there's no backward-compatible way to do it in the CLI since string flags would suddenly become part of filenames.
> - PHP plugin: any option that wants to extend the existing one should have their own name, `--php-trailing-comma` for example. (Since we do support sub-language formatting (`embed`), it's not possible to use the same name with different option.)
- https://github.com/prettier/plugin-php/issues/964#issuecomment-465563804
> I meant the php-specific value (`php5`, `php7.2`) should have their own name (`--php-trailing-comma`) but the general value (`all`, `none`) can still be used as before (`--trailing-comma`). The `--php-trailing-comma` should take precedence over `--trailing-comma` in this plugin, something like:
>
> ```ts
> function getPhpTrailingCommaValue(options): "all" | "php5" | "php7.2" | "none" {
> if (options.phpTrailingComma) return options.phpTrailingComma;
> if (options.trailingComma === "es5") return "none";
> return options.trailingComma;
> }
> ```
Same thing can be applied to TS/Flow as well.
@ikatyang your solution sounds reasonable. I'll investigate that for 2.0
We can probably close this issue in favor of https://github.com/prettier/prettier/issues/11465
I just noticed #3722 is still open. Should that be included with Prettier 3? | "2022-12-30T09:14:16Z" | 3.0 | [
"tests/format/typescript/typeparams/trailing-comma/jsfmt.spec.js",
"tests/format/typescript/interface2/break/jsfmt.spec.js",
"tests/format/typescript/interface2/jsfmt.spec.js"
] | [
"tests/format/typescript/trailing-comma/jsfmt.spec.js",
"tests/format/flow/type-parameters/trailing-comma/jsfmt.spec.js",
"tests/format/flow/interface-types/break/jsfmt.spec.js",
"tests/format/flow/function-parentheses/jsfmt.spec.js",
"tests/format/flow/generic/jsfmt.spec.js"
] | JavaScript | [] | [
"https://prettier.io/playground/#N4Igxg9gdgLgprEAuEMCeAHOACAYhCAFQAsBLAZwDkBDAWzgElyA1OAJzQBloBzAZSLFqMBjABCbONQDW5QoLiU4ADxidSUHAF5sA+jDJQeAHlyk25GAAVqbOgBpdcSFAAmNu7QB8AbhD2QCAwYUmhyZFBbNggAdw8EcJRqADcIUld-EGpLZBg2AFc4AIAjOzBpOBg+DGowDR5cgqKQVwgwZAAzagAbcmaNPrZrOx5aak6evoCAK3JlCVqKqro4dU0J3uaIfJgMHYAmDamQGot2ZBBi6mK0buhMjDYNGAB1dINkAA4ABgDHiD6LzsGAujzgg2ScEykgAjvlzHArCMxkdmn1aKRGoUAuR6t04ABFfIQeCogIwa5vVwfJD7cl2UjdeoAYQgtBRKHBAFZMvk+oRrokupsAL4ioA",
"https://prettier.io/playground/#N4Igxg9gdgLgprEAuEMCeAHOACAYhCAFQAsBLAZwDkBDAWzgElyA1OAJzQBloBzAZSLFqMBjABCbONQDW5QoLiU4ADxidSUHAF5sA+jDJQeAHlyk25GAAVqbOgBpdcSFAAmNu7QB8AbhD2QCAwYUmhyZFBbNggAdw8EcJRqADcIUld-EGpLZBg2AFc4AIAjOzBpOBg+DGowDR5cgqKQVwgwZAAzagAbcmaNPrZrOx5aak6evoCAK3JlCVqKqro4dU0J3uaIfJgMHYAmDamQGot2ZBBi6mK0buhMjDYNGAB1dINkAA4ABgDHiD6LzsGAujzgg2ScEykgAjvlzHArCMxkdmn1aKRGoUAuR6t04ABFfIQeCogIwa5vVwfJD7cl2UjdeoAYQgtBRSW63Uy+T6hGuiS6mwAvsKgA"
] |
prettier/prettier | 14,089 | prettier__prettier-14089 | [
"14088",
"13995"
] | b77d912c0c1a5df85e3e9b5b192fc92523e411ee | diff --git a/src/language-css/parser-postcss.js b/src/language-css/parser-postcss.js
--- a/src/language-css/parser-postcss.js
+++ b/src/language-css/parser-postcss.js
@@ -122,23 +122,6 @@ function parseValueNode(valueNode, options) {
parenGroupStack.pop();
parenGroup = getLast(parenGroupStack);
} else if (node.type === "comma") {
- if (commaGroup.groups.length > 1) {
- for (const group of commaGroup.groups) {
- // if css interpolation
- if (
- group.value &&
- typeof group.value === "string" &&
- group.value.includes("#{")
- ) {
- commaGroup.groups = [
- stringifyNode({
- groups: commaGroup.groups,
- }).trim(),
- ];
- break;
- }
- }
- }
parenGroup.groups.push(commaGroup);
commaGroup = {
groups: [],
diff --git a/src/language-css/printer-postcss.js b/src/language-css/printer-postcss.js
--- a/src/language-css/printer-postcss.js
+++ b/src/language-css/printer-postcss.js
@@ -547,6 +547,7 @@ function genericPrint(path, options, print) {
let insideSCSSInterpolationInString = false;
let didBreak = false;
+
for (let i = 0; i < node.groups.length; ++i) {
parts.push(printed[i]);
@@ -594,20 +595,20 @@ function genericPrint(path, options, print) {
}
// Ignore spaces before/after string interpolation (i.e. `"#{my-fn("_")}"`)
- const isStartSCSSInterpolationInString =
- iNode.type === "value-string" && iNode.value.startsWith("#{");
- const isEndingSCSSInterpolationInString =
- insideSCSSInterpolationInString &&
- iNextNode.type === "value-string" &&
- iNextNode.value.endsWith("}");
-
- if (
- isStartSCSSInterpolationInString ||
- isEndingSCSSInterpolationInString
- ) {
- insideSCSSInterpolationInString = !insideSCSSInterpolationInString;
-
- continue;
+ if (iNode.type === "value-string" && iNode.quoted) {
+ const positionOfOpeningInterpolation = iNode.value.lastIndexOf("#{");
+ const positionOfClosingInterpolation = iNode.value.lastIndexOf("}");
+ if (
+ positionOfOpeningInterpolation !== -1 &&
+ positionOfClosingInterpolation !== -1
+ ) {
+ insideSCSSInterpolationInString =
+ positionOfOpeningInterpolation > positionOfClosingInterpolation;
+ } else if (positionOfOpeningInterpolation !== -1) {
+ insideSCSSInterpolationInString = true;
+ } else if (positionOfClosingInterpolation !== -1) {
+ insideSCSSInterpolationInString = false;
+ }
}
if (insideSCSSInterpolationInString) {
| diff --git a/tests/format/scss/map/function-argument/__snapshots__/jsfmt.spec.js.snap b/tests/format/scss/map/function-argument/__snapshots__/jsfmt.spec.js.snap
--- a/tests/format/scss/map/function-argument/__snapshots__/jsfmt.spec.js.snap
+++ b/tests/format/scss/map/function-argument/__snapshots__/jsfmt.spec.js.snap
@@ -1,5 +1,56 @@
// Jest Snapshot v1, https://goo.gl/fbAQLP
+exports[`function-argument-2.scss format 1`] = `
+====================================options=====================================
+parsers: ["scss"]
+printWidth: 80
+ | printWidth
+=====================================input======================================
+$display-breakpoints: map-deep-merge(
+ (
+ "sm-only": "only screen and (min-width: #{$map-get + $grid-breakpoints + "hogehoge"}) and (max-width: #{$a})",
+ "sm-only": "inside a long long long long long long long long long long long long long long string #{call("")}",
+ "sm-only": "inside a long long long long long long long long long long long long long long string #{$foo} and #{call("")}",
+ "sm-only": "inside a long long long long long long long long long long long long long long string #{call($a)}",
+ ),
+ $display-breakpoints
+);
+
+@each $name, $hue in $hues {
+ $map: map.merge(
+ $map,
+ (
+ '#{$prefix}-#{$name}': blend.set($base, $hue: $hue),
+ )
+ );
+}
+
+=====================================output=====================================
+$display-breakpoints: map-deep-merge(
+ (
+ "sm-only": "only screen and (min-width: #{$map-get + $grid-breakpoints + "hogehoge"}) and (max-width: #{$a})",
+ "sm-only":
+ "inside a long long long long long long long long long long long long long long string #{call("")}",
+ "sm-only":
+ "inside a long long long long long long long long long long long long long long string #{$foo} and #{call("")}",
+ "sm-only":
+ "inside a long long long long long long long long long long long long long long string #{call($a)}",
+ ),
+ $display-breakpoints
+);
+
+@each $name, $hue in $hues {
+ $map: map.merge(
+ $map,
+ (
+ "#{$prefix}-#{$name}": blend.set($base, $hue: $hue),
+ )
+ );
+}
+
+================================================================================
+`;
+
exports[`functional-argument.scss format 1`] = `
====================================options=====================================
parsers: ["scss"]
diff --git a/tests/format/scss/map/function-argument/function-argument-2.scss b/tests/format/scss/map/function-argument/function-argument-2.scss
new file mode 100644
--- /dev/null
+++ b/tests/format/scss/map/function-argument/function-argument-2.scss
@@ -0,0 +1,18 @@
+$display-breakpoints: map-deep-merge(
+ (
+ "sm-only": "only screen and (min-width: #{$map-get + $grid-breakpoints + "hogehoge"}) and (max-width: #{$a})",
+ "sm-only": "inside a long long long long long long long long long long long long long long string #{call("")}",
+ "sm-only": "inside a long long long long long long long long long long long long long long string #{$foo} and #{call("")}",
+ "sm-only": "inside a long long long long long long long long long long long long long long string #{call($a)}",
+ ),
+ $display-breakpoints
+);
+
+@each $name, $hue in $hues {
+ $map: map.merge(
+ $map,
+ (
+ '#{$prefix}-#{$name}': blend.set($base, $hue: $hue),
+ )
+ );
+}
| Idempotent issue for SCSS map
**Prettier 2.8.1**
[Playground link](https://prettier.io/playground/#N4Igxg9gdgLgprEAuEASAJgSwM4AcA2AhgJ4C0ARgE5yEDWuEms2SABALaG6npxzfs4lAOZwAFAB0orVpOkzWEkAA9spaPmJK2SjcVbYw1BK0JR0szstIB3TOhgALNgGJgnbqJhjUwyvYpqOgYmGGwAGkUQbHYlAEpSAEYAXzilcKkZOIz5DBwCEkCaekZmKTiAbhBwkAhcGExobGRQQkpKCBsABTaEZpRCfBsSZpqqQjBaOBgAZUJBABkmOGQAM0HsODHKCanZ3AmmYWQYSgBXLZA4dnI4dF50BbNhM8JRADEISk4YBqhjgZnGAQaogRwwdj4ADqjkw8DwEzgMz6cMwADc4cRkOBsKMQExNpQYF0dsJOGsNpcAFbYZQzI74OAARTOEHgFPwmxqB0ohOxhlxoNw-lgUPsTmQAA4AAzcjqbKE7XDY4VwQlolY1ACOrPgJLq-RAhDUUD4D1B1B1mGoJLe5KQ605l027EwJ3OzoZcAAgr9-OQgXAukIlqaOVzol6WWyVg7KTUYIRyGKHI5kAAmBM7TD4I4AYQg7HtV2wAFZQWdNgAVJP9R0RtEXACS5gQsyMmHq3vMMxgxEZ4bgyWSQA)
<!-- prettier-ignore -->
```sh
--parser scss
```
**Input:**
<!-- prettier-ignore -->
```scss
$display-breakpoints: map-deep-merge(
(
"xs-only": "only screen and (max-width: #{map-get($grid-breakpoints, "sm")-1})",
),
$display-breakpoints
);
```
**Output:**
<!-- prettier-ignore -->
```scss
$display-breakpoints: map-deep-merge(
(
"xs-only": "only screen and (max-width: #{map-get($grid-breakpoints, "sm")-1})"
),
$display-breakpoints
);
```
**Second Output:**
<!-- prettier-ignore -->
```scss
$display-breakpoints: map-deep-merge(
(
"xs-only": "only screen and (max-width: #{map-get($grid-breakpoints, " sm
")-1})",
),
$display-breakpoints
);
```
**Expected behavior:**
same as input
**Related:**
- https://github.com/prettier/prettier/issues/9128
- https://github.com/prettier/prettier/issues/13984
Fix function argument list in SCSS maps
## Description
<!-- Please provide a brief summary of your changes: -->
Fix #13984
## Checklist
<!-- Please ensure you’ve done all of these things (if applicable). -->
<!-- You can replace the `[ ]` with `[x]` to mark each task as done. -->
- [ ] I’ve added tests to confirm my change works.
- [ ] (If changing the API or CLI) I’ve documented the changes I’ve made (in the `docs/` directory).
- [ ] (If the change is user-facing) I’ve added my changes to `changelog_unreleased/*/XXXX.md` file following `changelog_unreleased/TEMPLATE.md`.
- [ ] I’ve read the [contributing guidelines](https://github.com/prettier/prettier/blob/main/CONTRIBUTING.md).
<!-- Please DO NOT remove the playground link -->
**✨[Try the playground for this PR](https://prettier.io/playground-redirect)✨**
| "2022-12-30T14:45:59Z" | 2.9 | [] | [
"tests/format/scss/map/function-argument/jsfmt.spec.js"
] | JavaScript | [] | [
"https://prettier.io/playground-redirect",
"https://prettier.io/playground/#N4Igxg9gdgLgprEAuEASAJgSwM4AcA2AhgJ4C0ARgE5yEDWuEms2SABALaG6npxzfs4lAOZwAFAB0orVpOkzWEkAA9spaPmJK2SjcVbYw1BK0JR0szstIB3TOhgALNgGJgnbqJhjUwyvYpqOgYmGGwAGkUQbHYlAEpSAEYAXzilcKkZOIz5DBwCEkCaekZmKTiAbhBwkAhcGExobGRQQkpKCBsABTaEZpRCfBsSZpqqQjBaOBgAZUJBABkmOGQAM0HsODHKCanZ3AmmYWQYSgBXLZA4dnI4dF50BbNhM8JRADEISk4YBqhjgZnGAQaogRwwdj4ADqjkw8DwEzgMz6cMwADc4cRkOBsKMQExNpQYF0dsJOGsNpcAFbYZQzI74OAARTOEHgFPwmxqB0ohOxhlxoNw-lgUPsTmQAA4AAzcjqbKE7XDY4VwQlolY1ACOrPgJLq-RAhDUUD4D1B1B1mGoJLe5KQ605l027EwJ3OzoZcAAgr9-OQgXAukIlqaOVzol6WWyVg7KTUYIRyGKHI5kAAmBM7TD4I4AYQg7HtV2wAFZQWdNgAVJP9R0RtEXACS5gQsyMmHq3vMMxgxEZ4bgyWSQA"
] |
|
prettier/prettier | 14,109 | prettier__prettier-14109 | [
"11340"
] | 2f72344944384d28c6d00523febf03f294108e2c | diff --git a/src/language-css/parser-postcss.js b/src/language-css/parser-postcss.js
--- a/src/language-css/parser-postcss.js
+++ b/src/language-css/parser-postcss.js
@@ -220,6 +220,11 @@ async function parseNestedValue(node, options) {
}
async function parseValue(value, options) {
+ // Inline javascript in Less
+ if (options.parser === "less" && value.startsWith("~`")) {
+ return { type: "value-unknown", value };
+ }
+
const Parser = await import("postcss-values-parser/lib/parser.js").then(
(m) => m.default
);
| diff --git a/tests/format/less/inline-javascript/__snapshots__/jsfmt.spec.js.snap b/tests/format/less/inline-javascript/__snapshots__/jsfmt.spec.js.snap
new file mode 100644
--- /dev/null
+++ b/tests/format/less/inline-javascript/__snapshots__/jsfmt.spec.js.snap
@@ -0,0 +1,35 @@
+// Jest Snapshot v1, https://goo.gl/fbAQLP
+
+exports[`inline-javascript.less format 1`] = `
+====================================options=====================================
+parsers: ["less"]
+printWidth: 80
+ | printWidth
+=====================================input======================================
+// Deprecated feature https://lesscss.org/usage/#less-options-enable-inline-javascript-deprecated-
+
+.calcPxMixin() {
+ @functions: ~\`(function() {
+ const designWidth = 3840
+ const actualWidth = 5760
+ this.calcPx = function(_) {
+ return _ * actualWidth / designWidth + 'px'
+ }
+ })()\`
+}
+
+=====================================output=====================================
+// Deprecated feature https://lesscss.org/usage/#less-options-enable-inline-javascript-deprecated-
+
+.calcPxMixin() {
+ @functions: ~\`(function() {
+ const designWidth = 3840
+ const actualWidth = 5760
+ this.calcPx = function(_) {
+ return _ * actualWidth / designWidth + 'px'
+ }
+ })()\`;
+}
+
+================================================================================
+`;
diff --git a/tests/format/less/inline-javascript/inline-javascript.less b/tests/format/less/inline-javascript/inline-javascript.less
new file mode 100644
--- /dev/null
+++ b/tests/format/less/inline-javascript/inline-javascript.less
@@ -0,0 +1,11 @@
+// Deprecated feature https://lesscss.org/usage/#less-options-enable-inline-javascript-deprecated-
+
+.calcPxMixin() {
+ @functions: ~`(function() {
+ const designWidth = 3840
+ const actualWidth = 5760
+ this.calcPx = function(_) {
+ return _ * actualWidth / designWidth + 'px'
+ }
+ })()`
+}
diff --git a/tests/format/less/inline-javascript/jsfmt.spec.js b/tests/format/less/inline-javascript/jsfmt.spec.js
new file mode 100644
--- /dev/null
+++ b/tests/format/less/inline-javascript/jsfmt.spec.js
@@ -0,0 +1 @@
+run_spec(import.meta, ["less"]);
| less format problem
**Prettier 2.3.2**
[Playground link](https://prettier.io/playground/#N4Igxg9gdgLgprEAuEA6MBDANmACgDwFkBLfYqACgEoACYAHShpoAEAzAVyjBmOgGckNAH4ADCp269o1Oo2bNIUfjBoATOP2IBzKAHViamAAsaAXhoBmABwAWAAzyFSlTQw8O2A0dMWArADsAGyOTAomxPzo2Hj45jSSPHyUAPq0DGEKNABOcDAc2UwpNABUbh5ehiY0APTqmjr6VaYA1DQA5AAO+O1OzAC+Tv1U1KKM-SAANCAQndLKyKAY2dkQAO64ywj8yCDYaxgAnjvTAEbZ7gDWeQDKne7k2sgw2Rxw03AAtqdwahpqABkMFBtJ5tHAAGIQbKfDAwXgg3YYDgwCBTEDGGCfLB6YzEeD8e5gOA3bb44gAN3xh12YH4JxA5H4cGyMFwF20sOQbGwzOmACt+PgAEIXMDXGA3DCfOAA8hwbm894gQX4G6PLBwACKHAg8EVWD5IHu2WZ2V2mvp6M62XIMG8JmQ1ns0xtEGZegunV2Ns0LIpCumAEddfB2bMdigMPwALRQOC-X7o3Ih4i5dkYTkYA1G5mfYjPV7KrQgzU6vUKpA8w3KmAYU4O4zIABM0xeGGIWEeAGEIJ8uShNH50RxmQAVeuR6tGilvACSUA0sBuYFtcwAgoubjBDpqc3B+v0gA)
<!-- prettier-ignore -->
```sh
--parser less
```
**Input:**
<!-- prettier-ignore -->
```less
.calcPxMixin() {
@functions: ~`(function() {
const designWidth = 3840
const actualWidth = 5760
this.calcPx = function(_) {
return _ * actualWidth / designWidth + 'px'
}
})()`
}
```
**Output:**
<!-- prettier-ignore -->
```less
SyntaxError: CssSyntaxError: Unknown word (6:7)
4 | const actualWidth = 5760
5 | this.calcPx = function(_) {
> 6 | return _ * actualWidth / designWidth + 'px'
| ^
7 | }
8 | })()`
9 | }
```
**Expected behavior:**
| The next branch seems fixed the parse error, but not formatting as JavaScript code.
**Prettier pr-9583**
[Playground link](https://deploy-preview-9583--prettier.netlify.app/playground/#N4Igxg9gdgLgprEAuEA6MBDANmACgDwFkBLfYqACgEoACYAHShpoAEAzAVyjBmOgGckNAH4ADCp269o1Oo2bNIUfjBoATOP2IBzKAHViamAAsaAXhoBmABwAWAAzyFSlTQw8O2A0dMWArADsAGyOTAomxPzo2Hj45jSSPHyUAPq0DGEKNABOcDAc2UwpNABUbh5ehiY0APTqmjr6VaYA1DQA5AAO+O1OzAC+Tv1U1KKM-SAANCAQndLKyKAY2dkQAO64ywj8yCDYaxgAnjvTAEbZ7gDWeQDKne7k2sgw2Rxw03AAtqdwahpqABkMFBtJ5tHAAGIQbKfDAwXgg3YYDgwCBTEDGGCfLB6YzEeD8e5gOA3bb44gAN3xh12YH4JxA5H4cGyMFwF20sOQbGwzOmACt+PgAEIXMDXGA3DCfOAA8hwbm894gQX4G6PLBwACKHAg8EVWD5IHu2WZ2V2mvp6M62XIMG8JmQ1ns0xtEGZegunV2Ns0LIpCumAEddfB2bMdigMPwALRQOC-X7o3Ih4i5dkYTkYA1G5mfYjPV7KrQgzU6vUKpA8w3KmAYU4O4zIABM0xeGGIWEeAGEIJ8uShNH50RxmQAVeuR6tGilvACSUA0sBuYFtcwAgoubjBDpqc3B+v0gA)
<!-- prettier-ignore -->
```sh
--parser less
```
**Input:**
<!-- prettier-ignore -->
```less
.calcPxMixin() {
@functions: ~`(function() {
const designWidth = 3840
const actualWidth = 5760
this.calcPx = function(_) {
return _ * actualWidth / designWidth + 'px'
}
})()`
}
```
**Output:**
<!-- prettier-ignore -->
```less
.calcPxMixin() {
@functions: ~`(
function() {const designWidth = 3840 const actualWidth = 5760 this.calcPx =
function(_) {return _ * actualWidth / designWidth + "px"}}
)
() `;
}
```
Maybe we should apply js printer for this case
Is this feature deprecated? https://lesscss.org/usage/#less-options-enable-inline-javascript-deprecated- | "2023-01-04T09:46:00Z" | 3.0 | [] | [
"tests/format/less/inline-javascript/jsfmt.spec.js"
] | JavaScript | [] | [
"https://prettier.io/playground/#N4Igxg9gdgLgprEAuEA6MBDANmACgDwFkBLfYqACgEoACYAHShpoAEAzAVyjBmOgGckNAH4ADCp269o1Oo2bNIUfjBoATOP2IBzKAHViamAAsaAXhoBmABwAWAAzyFSlTQw8O2A0dMWArADsAGyOTAomxPzo2Hj45jSSPHyUAPq0DGEKNABOcDAc2UwpNABUbh5ehiY0APTqmjr6VaYA1DQA5AAO+O1OzAC+Tv1U1KKM-SAANCAQndLKyKAY2dkQAO64ywj8yCDYaxgAnjvTAEbZ7gDWeQDKne7k2sgw2Rxw03AAtqdwahpqABkMFBtJ5tHAAGIQbKfDAwXgg3YYDgwCBTEDGGCfLB6YzEeD8e5gOA3bb44gAN3xh12YH4JxA5H4cGyMFwF20sOQbGwzOmACt+PgAEIXMDXGA3DCfOAA8hwbm894gQX4G6PLBwACKHAg8EVWD5IHu2WZ2V2mvp6M62XIMG8JmQ1ns0xtEGZegunV2Ns0LIpCumAEddfB2bMdigMPwALRQOC-X7o3Ih4i5dkYTkYA1G5mfYjPV7KrQgzU6vUKpA8w3KmAYU4O4zIABM0xeGGIWEeAGEIJ8uShNH50RxmQAVeuR6tGilvACSUA0sBuYFtcwAgoubjBDpqc3B+v0gA"
] |
prettier/prettier | 14,163 | prettier__prettier-14163 | [
"13986"
] | d0eb185980a7909cf00667218a6e4bb5450f16da | diff --git a/src/language-js/print/jsx.js b/src/language-js/print/jsx.js
--- a/src/language-js/print/jsx.js
+++ b/src/language-js/print/jsx.js
@@ -13,6 +13,7 @@ import {
ifBreak,
lineSuffixBoundary,
join,
+ cursor,
} from "../../document/builders.js";
import { willBreak, replaceEndOfLine } from "../../document/utils.js";
import UnexpectedNodeError from "../../utils/unexpected-node-error.js";
@@ -50,6 +51,7 @@ const isEmptyStringOrAnyLine = (doc) =>
* @typedef {import("../../common/ast-path.js").default} AstPath
* @typedef {import("../types/estree.js").Node} Node
* @typedef {import("../types/estree.js").JSXElement} JSXElement
+ * @typedef {import("../../document/builders.js").Doc} Doc
*/
// JSX expands children from the inside-out, instead of the outside-in.
@@ -236,10 +238,22 @@ function printJsxElementInternal(path, options, print) {
// If there is text we use `fill` to fit as much onto each line as possible.
// When there is no text (just tags and expressions) we use `group`
// to output each on a separate line.
- const content = containsText
+ /** @type {Doc} */
+ let content = containsText
? fill(multilineChildren)
: group(multilineChildren, { shouldBreak: true });
+ /*
+ `printJsxChildren` won't call `print` on `JSXText`
+ When the cursorNode is inside `cursor` won't get print.
+ */
+ if (
+ options.cursorNode?.type === "JSXText" &&
+ node.children.includes(options.cursorNode)
+ ) {
+ content = [cursor, content, cursor];
+ }
+
if (isMdxBlock) {
return content;
}
| diff --git a/tests/format/jsx/cursor/__snapshots__/jsfmt.spec.js.snap b/tests/format/jsx/cursor/__snapshots__/jsfmt.spec.js.snap
new file mode 100644
--- /dev/null
+++ b/tests/format/jsx/cursor/__snapshots__/jsfmt.spec.js.snap
@@ -0,0 +1,20 @@
+// Jest Snapshot v1, https://goo.gl/fbAQLP
+
+exports[`in-jsx-text.js format 1`] = `
+====================================options=====================================
+cursorOffset: 3
+parsers: ["babel", "typescript", "flow"]
+printWidth: 80
+ | printWidth
+=====================================input======================================
+<>a<|>
+ <div>hi</div>
+</>
+
+=====================================output=====================================
+<>
+ a<|><div>hi</div>
+</>;
+
+================================================================================
+`;
diff --git a/tests/format/jsx/cursor/in-jsx-text.js b/tests/format/jsx/cursor/in-jsx-text.js
new file mode 100644
--- /dev/null
+++ b/tests/format/jsx/cursor/in-jsx-text.js
@@ -0,0 +1,3 @@
+<>a<|>
+ <div>hi</div>
+</>
diff --git a/tests/format/jsx/cursor/jsfmt.spec.js b/tests/format/jsx/cursor/jsfmt.spec.js
new file mode 100644
--- /dev/null
+++ b/tests/format/jsx/cursor/jsfmt.spec.js
@@ -0,0 +1 @@
+run_spec(import.meta, ["babel", "typescript", "flow"]);
| Wrong cursorOffset with formatted JSX text
In a similar vein to #12491 (which I am still intending to fix), I found a misplaced cursorOffset issue with code like this
```jsx
<>a•
<div>hi</div>
</>
```
(where `•` denotes the given cursor position)
When passed into prettier we get:
```jsx
<>•
a<div>hi</div>
</>
```
Which is unexpected, because the a correctly moves down, but the cursor stays up.
Here is code for testing it:
```js
const prettier = require("prettier");
prettier.formatWithCursor(["<>a", " <div>hi</div>", "</>"].join("\n"), { cursorOffset: 3, parser: 'babel' });
```
and the runkit to see it in the browser:
https://runkit.com/gregoor/prettier-jsx-cursor-offset-bug
| "2023-01-12T10:15:59Z" | 3.0 | [] | [
"tests/format/jsx/cursor/jsfmt.spec.js"
] | JavaScript | [] | [] |
|
prettier/prettier | 14,170 | prettier__prettier-14170 | [
"14168"
] | c1b976572c7512dfa7d7407706891f511883c0ad | diff --git a/src/language-html/parser-html.js b/src/language-html/parser-html.js
--- a/src/language-html/parser-html.js
+++ b/src/language-html/parser-html.js
@@ -24,7 +24,7 @@ const { locStart, locEnd } = require("./loc.js");
* @typedef {import('angular-html-parser/lib/compiler/src/ml_parser/parser').ParseTreeResult} ParserTreeResult
* @typedef {Omit<import('angular-html-parser').ParseOptions, 'canSelfClose'> & {
* name?: 'html' | 'angular' | 'vue' | 'lwc';
- * recognizeSelfClosing?: boolean;
+ * canSelfClose?: boolean;
* normalizeTagName?: boolean;
* normalizeAttributeName?: boolean;
* }} ParserOptions
@@ -42,7 +42,7 @@ const { locStart, locEnd } = require("./loc.js");
function ngHtmlParser(
input,
{
- recognizeSelfClosing,
+ canSelfClose,
normalizeTagName,
normalizeAttributeName,
allowHtmComponentClosingTags,
@@ -64,7 +64,7 @@ function ngHtmlParser(
} = require("angular-html-parser/lib/compiler/src/ml_parser/html_tags");
let { rootNodes, errors } = parser.parse(input, {
- canSelfClose: recognizeSelfClosing,
+ canSelfClose,
allowHtmComponentClosingTags,
isTagNameCaseSensitive,
getTagContentType,
@@ -97,7 +97,7 @@ function ngHtmlParser(
let secondParseResult;
const doSecondParse = () =>
parser.parse(input, {
- canSelfClose: recognizeSelfClosing,
+ canSelfClose,
allowHtmComponentClosingTags,
isTagNameCaseSensitive,
});
@@ -135,13 +135,13 @@ function ngHtmlParser(
}
} else {
// If not Vue SFC, treat as html
- recognizeSelfClosing = true;
+ canSelfClose = true;
normalizeTagName = true;
normalizeAttributeName = true;
allowHtmComponentClosingTags = true;
isTagNameCaseSensitive = false;
const htmlParseResult = parser.parse(input, {
- canSelfClose: recognizeSelfClosing,
+ canSelfClose,
allowHtmComponentClosingTags,
isTagNameCaseSensitive,
});
@@ -373,7 +373,7 @@ function _parse(text, options, parserOptions, shouldParseFrontMatter = true) {
*/
function createParser({
name,
- recognizeSelfClosing = false,
+ canSelfClose = false,
normalizeTagName = false,
normalizeAttributeName = false,
allowHtmComponentClosingTags = false,
@@ -386,7 +386,7 @@ function createParser({
text,
{ parser: name, ...options },
{
- recognizeSelfClosing,
+ canSelfClose,
normalizeTagName,
normalizeAttributeName,
allowHtmComponentClosingTags,
@@ -405,15 +405,15 @@ module.exports = {
parsers: {
html: createParser({
name: "html",
- recognizeSelfClosing: true,
+ canSelfClose: true,
normalizeTagName: true,
normalizeAttributeName: true,
allowHtmComponentClosingTags: true,
}),
- angular: createParser({ name: "angular" }),
+ angular: createParser({ name: "angular", canSelfClose: true }),
vue: createParser({
name: "vue",
- recognizeSelfClosing: true,
+ canSelfClose: true,
isTagNameCaseSensitive: true,
getTagContentType: (tagName, prefix, hasParent, attrs) => {
if (
| diff --git a/tests/format/angular/self-closing/__snapshots__/jsfmt.spec.js.snap b/tests/format/angular/self-closing/__snapshots__/jsfmt.spec.js.snap
new file mode 100644
--- /dev/null
+++ b/tests/format/angular/self-closing/__snapshots__/jsfmt.spec.js.snap
@@ -0,0 +1,36 @@
+// Jest Snapshot v1, https://goo.gl/fbAQLP
+
+exports[`self-closing.component.html format 1`] = `
+====================================options=====================================
+parsers: ["angular"]
+printWidth: 80
+ | printWidth
+=====================================input======================================
+<app-test/>
+<app-test />
+<app-test
+/>
+<img>
+<img/>
+<img />
+<img
+/>
+<div/>
+<div />
+<div
+/>
+
+=====================================output=====================================
+<app-test />
+<app-test />
+<app-test />
+<img />
+<img />
+<img />
+<img />
+<div />
+<div />
+<div />
+
+================================================================================
+`;
diff --git a/tests/format/angular/self-closing/jsfmt.spec.js b/tests/format/angular/self-closing/jsfmt.spec.js
new file mode 100644
--- /dev/null
+++ b/tests/format/angular/self-closing/jsfmt.spec.js
@@ -0,0 +1 @@
+run_spec(__dirname, ["angular"]);
diff --git a/tests/format/angular/self-closing/self-closing.component.html b/tests/format/angular/self-closing/self-closing.component.html
new file mode 100644
--- /dev/null
+++ b/tests/format/angular/self-closing/self-closing.component.html
@@ -0,0 +1,13 @@
+<app-test/>
+<app-test />
+<app-test
+/>
+<img>
+<img/>
+<img />
+<img
+/>
+<div/>
+<div />
+<div
+/>
| Angular templates self closing tags
<!--
BEFORE SUBMITTING AN ISSUE:
1. Search for your issue on GitHub: https://github.com/prettier/prettier/issues
A large number of opened issues are duplicates of existing issues.
If someone has already opened an issue for what you are experiencing,
you do not need to open a new issue — please add a 👍 reaction to the
existing issue instead.
2. We get a lot of requests for adding options, but Prettier is
built on the principle of being opinionated about code formatting.
This means we add options only in the case of strict technical necessity.
Find out more: https://prettier.io/docs/en/option-philosophy.html
Don't fill the form below manually! Let a program create a report for you:
1. Go to https://prettier.io/playground
2. Paste your code and set options
3. Press the "Report issue" button in the lower right
-->
**Prettier 2.8.2**
[Playground link](https://prettier.io/playground/#N4Igxg9gdgLgprEAuEAeAhgB0wWngZxgHoA+AHShABoQJMYBLafZUdAJ3YgHcAFDhCxToANt3QBPFjQBG7dGADWcGAGV0AWzgAZBlDjIAZqPxxZ8pStWYFegObIY7AK5mQcDTLgATbz+3oUHbO6HZwAGIQ7BroMIxByCDozjAQ1CAAFjAaIgDqGQwENmBwqoKFDABuhRKJYPjSIHqm7DC88nYxRiZuAFb4AB6q9iJwAIrOEPDdIqY0NuwtiYHBIhzpmOx6MLkM3jAZyAAcAAzzXKa58piJm3AtlQY0AI6T8O10Qkn4OPo+Pul2HBXgwge1Ql0kMZZm5TBoGI4XLCRuM3gYoT0aDB0DJdvtDkgAExY+QMET2ADCEA0kPc+AArOlnKYACo4oTQuYgSquACSUD8sFUYC29AAggLVDAJKMZqYAL7yoA)
**Input:**
```jsx
<app-test/>
```
**Output:**
```jsx
SyntaxError: Only void and foreign elements can be self closed "app-test" (1:1)
> 1 | <app-test/>
| ^^^^^^^^^
2 |
```
**Expected behavior:**
In [Angular 15.1.0](https://github.com/angular/angular/releases/tag/15.1.0) developers are allowed to use self closed tags for custom elements https://github.com/angular/angular/pull/48535
Prettier should support this feature
| "2023-01-13T02:01:08Z" | 2.9 | [] | [
"tests/format/angular/self-closing/jsfmt.spec.js"
] | JavaScript | [] | [
"https://prettier.io/playground",
"https://prettier.io/playground/#N4Igxg9gdgLgprEAuEAeAhgB0wWngZxgHoA+AHShABoQJMYBLafZUdAJ3YgHcAFDhCxToANt3QBPFjQBG7dGADWcGAGV0AWzgAZBlDjIAZqPxxZ8pStWYFegObIY7AK5mQcDTLgATbz+3oUHbO6HZwAGIQ7BroMIxByCDozjAQ1CAAFjAaIgDqGQwENmBwqoKFDABuhRKJYPjSIHqm7DC88nYxRiZuAFb4AB6q9iJwAIrOEPDdIqY0NuwtiYHBIhzpmOx6MLkM3jAZyAAcAAzzXKa58piJm3AtlQY0AI6T8O10Qkn4OPo+Pul2HBXgwge1Ql0kMZZm5TBoGI4XLCRuM3gYoT0aDB0DJdvtDkgAExY+QMET2ADCEA0kPc+AArOlnKYACo4oTQuYgSquACSUD8sFUYC29AAggLVDAJKMZqYAL7yoA"
] |
|
prettier/prettier | 14,192 | prettier__prettier-14192 | [
"13980"
] | 9e855021075af184f2183114c7651d47b209f019 | diff --git a/src/language-js/printer-estree.js b/src/language-js/printer-estree.js
--- a/src/language-js/printer-estree.js
+++ b/src/language-js/printer-estree.js
@@ -29,6 +29,7 @@ import {
markerForIfWithoutBlockAndSameLineComment,
isArrayOrTupleExpression,
isObjectOrRecordExpression,
+ startsWithNoLookaheadToken,
} from "./utils/index.js";
import { locStart, locEnd } from "./loc.js";
import isBlockComment from "./utils/is-block-comment.js";
@@ -324,11 +325,18 @@ function printPathNoParens(path, options, print, args) {
(isMemberExpression(parent) && parent.object === node)
) {
parts = [indent([softline, ...parts]), softline];
+ // avoid printing `await (await` on one line
const parentAwaitOrBlock = path.findAncestor(
(node) =>
node.type === "AwaitExpression" || node.type === "BlockStatement"
);
- if (parentAwaitOrBlock?.type !== "AwaitExpression") {
+ if (
+ parentAwaitOrBlock?.type !== "AwaitExpression" ||
+ !startsWithNoLookaheadToken(
+ parentAwaitOrBlock.argument,
+ (leftmostNode) => leftmostNode === node
+ )
+ ) {
return group(parts);
}
}
| diff --git a/tests/format/js/async/__snapshots__/jsfmt.spec.js.snap b/tests/format/js/async/__snapshots__/jsfmt.spec.js.snap
--- a/tests/format/js/async/__snapshots__/jsfmt.spec.js.snap
+++ b/tests/format/js/async/__snapshots__/jsfmt.spec.js.snap
@@ -198,6 +198,31 @@ const getAccountCount = async () =>
================================================================================
`;
+exports[`nested2.js format 1`] = `
+====================================options=====================================
+parsers: ["babel", "flow", "typescript"]
+printWidth: 80
+ | printWidth
+=====================================input======================================
+async function f() {
+await Promise.all(
+ (await readdir("src")).map(async (path) => {
+ import(\`./\${path}\`);
+ })
+);}
+
+=====================================output=====================================
+async function f() {
+ await Promise.all(
+ (await readdir("src")).map(async (path) => {
+ import(\`./\${path}\`);
+ }),
+ );
+}
+
+================================================================================
+`;
+
exports[`parens.js format 1`] = `
====================================options=====================================
parsers: ["babel", "flow", "typescript"]
diff --git a/tests/format/js/async/nested2.js b/tests/format/js/async/nested2.js
new file mode 100644
--- /dev/null
+++ b/tests/format/js/async/nested2.js
@@ -0,0 +1,6 @@
+async function f() {
+await Promise.all(
+ (await readdir("src")).map(async (path) => {
+ import(`./${path}`);
+ })
+);}
| Unnecessary whitespace around `await` in multi-line chain passed to a function
**Prettier 2.8.1**
[Playground link](https://prettier.io/playground/#N4Igxg9gdgLgprEAuEBLAtgBwgJxgAmHxzgEMATc1HfAX3wDMcJ18AdEBgZwHpNn0qLnC4cA3GyiTSAd1KoCABQFC4AOlIAbTQApJ+fDtnyCJClRx6QXHGA4BKe2vSlMRrgE8oYQ5lIwAC3t8AF4APkJ9A3wMbDwdAAM1HgASYD9A2gT7CSgDWntJHMkQABoQCEwYVGguZFBSHGYZRUaEOpQtOQ868oAjHFIwAGs4GABlUnQ4ABlUKDhkBi1hfsGRsfG-MHmAc2QYHABXOHK4dD64SiuZ0ihdo9JduAAxXBcYavvkEFIjmAgZRAARg6E0AHUAgoRNs4ON2gpUAA3BQeH5gLi9NBQYR4ZRPFxLFanEAAKy4AA9xntNHAAIpHCDwImaVYgPw4XE-PqkS6aIH8eYwcGociBZAADgADOV+BBhODBpgfvwRHAcEjFuUAI6M+DKSodX5cAC0CyuVyBJF11Dg+N2hKQy1ZJOEggOx1dNLgAEFPjhUH1-nb1XMFiy2VxvQymYsncTyjBeSKxQFkAAmRODVCaPYAYRYjpAIgArECjsIACq8jrOtlIk4ASSg5AQEzAAaqPpb4xgHlpEbgtFoQA)
<!-- prettier-ignore -->
```sh
--parser babel
```
**Input:**
<!-- prettier-ignore -->
```jsx
import { readdir } from "fs/promises";
await Promise.all(
(await readdir("src")).map(async (path) => {
import(`./${path}`);
})
);
```
**Output:**
<!-- prettier-ignore -->
```jsx
import { readdir } from "fs/promises";
await Promise.all(
(
await readdir("src")
).map(async (path) => {
import(`./${path}`);
})
);
```
**Expected behavior:**
Preserve formatting to keep chained `await` on the first line of the chain. This is what is supposed to happen if you don't have a multi-line chain or don't pass it to a function.
**Note:**
It's important that Prettier preserves the parentheses around `await readdir("src")`, otherwise it would be parsed like `await (readdir("src").chain())`, which would break because `path` would be `Promise<string>` instead of `string`.
| "2023-01-16T20:24:27Z" | 3.0 | [] | [
"tests/format/js/async/jsfmt.spec.js"
] | JavaScript | [] | [
"https://prettier.io/playground/#N4Igxg9gdgLgprEAuEBLAtgBwgJxgAmHxzgEMATc1HfAX3wDMcJ18AdEBgZwHpNn0qLnC4cA3GyiTSAd1KoCABQFC4AOlIAbTQApJ+fDtnyCJClRx6QXHGA4BKe2vSlMRrgE8oYQ5lIwAC3t8AF4APkJ9A3wMbDwdAAM1HgASYD9A2gT7CSgDWntJHMkQABoQCEwYVGguZFBSHGYZRUaEOpQtOQ868oAjHFIwAGs4GABlUnQ4ABlUKDhkBi1hfsGRsfG-MHmAc2QYHABXOHK4dD64SiuZ0ihdo9JduAAxXBcYavvkEFIjmAgZRAARg6E0AHUAgoRNs4ON2gpUAA3BQeH5gLi9NBQYR4ZRPFxLFanEAAKy4AA9xntNHAAIpHCDwImaVYgPw4XE-PqkS6aIH8eYwcGociBZAADgADOV+BBhODBpgfvwRHAcEjFuUAI6M+DKSodX5cAC0CyuVyBJF11Dg+N2hKQy1ZJOEggOx1dNLgAEFPjhUH1-nb1XMFiy2VxvQymYsncTyjBeSKxQFkAAmRODVCaPYAYRYjpAIgArECjsIACq8jrOtlIk4ASSg5AQEzAAaqPpb4xgHlpEbgtFoQA"
] |
|
prettier/prettier | 14,208 | prettier__prettier-14208 | [
"6024",
"13260"
] | cf409fe6a458d080ed7f673a7347e00ec3c0b405 | diff --git a/src/language-css/printer-postcss.js b/src/language-css/printer-postcss.js
--- a/src/language-css/printer-postcss.js
+++ b/src/language-css/printer-postcss.js
@@ -1,5 +1,7 @@
"use strict";
+/** @typedef {import("../document").Doc} Doc */
+
const getLast = require("../utils/get-last.js");
const {
printNumber,
@@ -152,7 +154,16 @@ function genericPrint(path, options, print) {
? removeLines(print("value"))
: print("value");
- if (!isColon && lastLineHasInlineComment(trimmedBetween)) {
+ if (
+ !isColon &&
+ lastLineHasInlineComment(trimmedBetween) &&
+ !(
+ node.value.type === "value-root" &&
+ node.value.group.type === "value-value" &&
+ node.value.group.group.type === "value-paren_group" &&
+ path.call(() => shouldBreakList(path), "value", "group", "group")
+ )
+ ) {
value = indent([hardline, dedent(value)]);
}
@@ -924,17 +935,17 @@ function genericPrint(path, options, print) {
}
if (!node.open) {
- const printed = path.map(print, "groups");
- const res = [];
-
- for (let i = 0; i < printed.length; i++) {
- if (i !== 0) {
- res.push([",", line]);
- }
- res.push(printed[i]);
- }
-
- return group(indent(fill(res)));
+ const forceHardLine = shouldBreakList(path);
+ const parts = join(
+ [",", forceHardLine ? hardline : line],
+ path.map(print, "groups")
+ );
+ return indent(
+ forceHardLine
+ ? [hardline, parts]
+ : // TODO: Use `parts` when merge to `next` branch
+ group(fill(parts.parts))
+ );
}
const isSCSSMapItem = isSCSSMapItemNode(path);
@@ -1169,6 +1180,18 @@ function printCssNumber(rawNumber) {
);
}
+function shouldBreakList(path) {
+ const node = path.getNode();
+ const parentParentParentNode = path.getParentNode(2);
+ return (
+ !node.open &&
+ (parentParentParentNode.type === "css-decl" ||
+ (parentParentParentNode.type === "css-atrule" &&
+ parentParentParentNode.variable)) &&
+ node.groups.some((node) => node.type === "value-comma_group")
+ );
+}
+
module.exports = {
print: genericPrint,
embed,
| diff --git a/tests/format/css/atrule/__snapshots__/jsfmt.spec.js.snap b/tests/format/css/atrule/__snapshots__/jsfmt.spec.js.snap
--- a/tests/format/css/atrule/__snapshots__/jsfmt.spec.js.snap
+++ b/tests/format/css/atrule/__snapshots__/jsfmt.spec.js.snap
@@ -1580,33 +1580,39 @@ format(
=====================================output=====================================
@font-face {
font-family: "Open Sans";
- src: url("/fonts/OpenSans-Regular-webfont.woff2") format("woff2"),
+ src:
+ url("/fonts/OpenSans-Regular-webfont.woff2") format("woff2"),
url("/fonts/OpenSans-Regular-webfont.woff") format("woff");
}
@font-face {
font-family: "Open Sans";
- src: url("/fonts/OpenSans-Regular-webfont.woff2") format("woff2"),
+ src:
+ url("/fonts/OpenSans-Regular-webfont.woff2") format("woff2"),
url("/fonts/OpenSans-Regular-webfont.woff") format("woff");
}
@font-face {
font-family: "Open Sans";
- src: url("/fonts/OpenSans-Regular-webfont.woff2") format("woff2"),
+ src:
+ url("/fonts/OpenSans-Regular-webfont.woff2") format("woff2"),
url("/fonts/OpenSans-Regular-webfont.woff") format("woff");
}
@font-face {
font-family: "Open Sans";
- src: url("/fonts/OpenSans-Regular-webfont.woff2") format("woff2"),
+ src:
+ url("/fonts/OpenSans-Regular-webfont.woff2") format("woff2"),
url("/fonts/OpenSans-Regular-webfont.woff") format("woff");
}
@font-face {
font-family: "Open Sans";
- src: url("/fonts/OpenSans-Regular-webfont.woff2") format("woff2"),
+ src:
+ url("/fonts/OpenSans-Regular-webfont.woff2") format("woff2"),
url("/fonts/OpenSans-Regular-webfont.woff") format("woff");
}
@font-face {
font-family: "Open Sans";
- src: url("/fonts/OpenSans-Regular-webfont.woff2") format("woff2"),
+ src:
+ url("/fonts/OpenSans-Regular-webfont.woff2") format("woff2"),
url("/fonts/OpenSans-Regular-webfont.woff") format("woff");
}
diff --git a/tests/format/css/comments/__snapshots__/jsfmt.spec.js.snap b/tests/format/css/comments/__snapshots__/jsfmt.spec.js.snap
--- a/tests/format/css/comments/__snapshots__/jsfmt.spec.js.snap
+++ b/tests/format/css/comments/__snapshots__/jsfmt.spec.js.snap
@@ -397,7 +397,8 @@ a {
@font-face {
font-family: "Prettier";
- src: /* comment 16 */ local(/* comment 17 */ "Prettier" /* comment 18 */),
+ src: /* comment 16 */
+ local(/* comment 17 */ "Prettier" /* comment 18 */),
/* comment 19 */ /* comment 20 */ url("http://prettier.com/font.woff")
/* comment 21 */; /* comment 22 */
}
diff --git a/tests/format/css/fill-value/__snapshots__/jsfmt.spec.js.snap b/tests/format/css/fill-value/__snapshots__/jsfmt.spec.js.snap
--- a/tests/format/css/fill-value/__snapshots__/jsfmt.spec.js.snap
+++ b/tests/format/css/fill-value/__snapshots__/jsfmt.spec.js.snap
@@ -15,7 +15,12 @@ div {
div {
border-left: 1px solid
mix($warningBackgroundColors, $warningBorderColors, 50%);
- $fontFamily: "Lato", -apple-system, "Helvetica Neue", Helvetica, Arial,
+ $fontFamily:
+ "Lato",
+ -apple-system,
+ "Helvetica Neue",
+ Helvetica,
+ Arial,
sans-serif;
}
diff --git a/tests/format/css/indent/__snapshots__/jsfmt.spec.js.snap b/tests/format/css/indent/__snapshots__/jsfmt.spec.js.snap
--- a/tests/format/css/indent/__snapshots__/jsfmt.spec.js.snap
+++ b/tests/format/css/indent/__snapshots__/jsfmt.spec.js.snap
@@ -16,7 +16,8 @@ div {
div {
background: var(fig-light-02) url(/images/inset-shadow-east-ltr.png) 100% 0
repeat-y;
- box-shadow: 0 0 1px 2px rgba(88, 144, 255, 0.75),
+ box-shadow:
+ 0 0 1px 2px rgba(88, 144, 255, 0.75),
0 1px 1px rgba(0, 0, 0, 0.15);
padding-bottom: calc(
var(ads-help-tray-footer-with-support-link-height) +
diff --git a/tests/format/less/comments/__snapshots__/jsfmt.spec.js.snap b/tests/format/less/comments/__snapshots__/jsfmt.spec.js.snap
--- a/tests/format/less/comments/__snapshots__/jsfmt.spec.js.snap
+++ b/tests/format/less/comments/__snapshots__/jsfmt.spec.js.snap
@@ -455,7 +455,8 @@ printWidth: 80
#bbbbbb // then some B
#cccccc; // and round it out with C
-@test-comma-separated: #aaaaaa,
+@test-comma-separated:
+ #aaaaaa,
// Start with A
#bbbbbb,
// then some B
diff --git a/tests/format/less/less/__snapshots__/jsfmt.spec.js.snap b/tests/format/less/less/__snapshots__/jsfmt.spec.js.snap
--- a/tests/format/less/less/__snapshots__/jsfmt.spec.js.snap
+++ b/tests/format/less/less/__snapshots__/jsfmt.spec.js.snap
@@ -3198,7 +3198,9 @@ div {
}
.myclass {
- box-shadow: inset 0 0 10px #555, 0 0 20px black;
+ box-shadow:
+ inset 0 0 10px #555,
+ 0 0 20px black;
}
.mixin() {
diff --git a/tests/format/scss/comments/__snapshots__/jsfmt.spec.js.snap b/tests/format/scss/comments/__snapshots__/jsfmt.spec.js.snap
--- a/tests/format/scss/comments/__snapshots__/jsfmt.spec.js.snap
+++ b/tests/format/scss/comments/__snapshots__/jsfmt.spec.js.snap
@@ -224,16 +224,22 @@ selector {
// #8052
$font-family-rich:
// custom
- "Noto Sans TC", "Noto Sans SC", "Noto Sans JP",
+ "Noto Sans TC",
+ "Noto Sans SC",
+ "Noto Sans JP",
// Safari for OS X and iOS (San Francisco)
-apple-system,
BlinkMacSystemFont,
// fallback
Roboto,
- "Helvetica Neue", Helvetica, Arial, sans-serif,
+ "Helvetica Neue",
+ Helvetica,
+ Arial,
+ sans-serif,
// emoji
"Apple Color Emoji",
- "Segoe UI Emoji", "Segoe UI Symbol" !default;
+ "Segoe UI Emoji",
+ "Segoe UI Symbol" !default;
// #7109
.test {
@@ -394,7 +400,8 @@ $my-list2:
c;
=====================================output=====================================
-$my-list: "foo",
+$my-list:
+ "foo",
// Foo
"bar"; // Bar
diff --git a/tests/format/scss/scss/__snapshots__/jsfmt.spec.js.snap b/tests/format/scss/scss/__snapshots__/jsfmt.spec.js.snap
--- a/tests/format/scss/scss/__snapshots__/jsfmt.spec.js.snap
+++ b/tests/format/scss/scss/__snapshots__/jsfmt.spec.js.snap
@@ -1786,17 +1786,29 @@ $list-comma: "item-1", "item-2", "item-3";
$list-comma: "item-1", "item-2", "item-3";
$list-comma: "item-1", "item-2", "item-3";
$list-comma: "item-1", "item-2", "item-3";
-$list: "item-1.1" "item-1.2" "item-1.3", "item-2.1" "item-2.2" "item-2.3",
+$list:
+ "item-1.1" "item-1.2" "item-1.3",
+ "item-2.1" "item-2.2" "item-2.3",
"item-3.1" "item-3.2" "item-3.3";
-$list: "item-1.1" "item-1.2" "item-1.3", "item-2.1" "item-2.2" "item-2.3",
+$list:
+ "item-1.1" "item-1.2" "item-1.3",
+ "item-2.1" "item-2.2" "item-2.3",
"item-3.1" "item-3.2" "item-3.3";
-$list: "item-1.1" "item-1.2" "item-1.3", "item-2.1" "item-2.2" "item-2.3",
+$list:
+ "item-1.1" "item-1.2" "item-1.3",
+ "item-2.1" "item-2.2" "item-2.3",
"item-3.1" "item-3.2" "item-3.3";
-$list: "item-1.1" "item-1.2" "item-1.3", "item-2.1" "item-2.2" "item-2.3",
+$list:
+ "item-1.1" "item-1.2" "item-1.3",
+ "item-2.1" "item-2.2" "item-2.3",
"item-3.1" "item-3.2" "item-3.3";
-$list: "item-1.1" "item-1.2" "item-1.3", "item-2.1" "item-2.2" "item-2.3",
+$list:
+ "item-1.1" "item-1.2" "item-1.3",
+ "item-2.1" "item-2.2" "item-2.3",
"item-3.1" "item-3.2" "item-3.3";
-$list: "item-1.1" "item-1.2" "item-1.3", "item-2.1" "item-2.2" "item-2.3",
+$list:
+ "item-1.1" "item-1.2" "item-1.3",
+ "item-2.1" "item-2.2" "item-2.3",
"item-3.1" "item-3.2" "item-3.3";
$list: (
("item-1.1", "item-1.2", "item-1.3"),
@@ -1870,7 +1882,10 @@ $space-scale: (0, "0") (0.25, "0-25") (0.5, "0-5") (0.75, "0-75") (1, "1")
@error "Very long long long long long long long long long long long long long line Error (#{$message}).";
@error "Very long long long long long long long long long long long long long line Error (#{$message}).";
-$buttonConfig: "save" 50px, "cancel" 50px, "help" 100px;
+$buttonConfig:
+ "save" 50px,
+ "cancel" 50px,
+ "help" 100px;
$locale: "en_us";
html[lang="#{$locale}"] {
@@ -2204,7 +2219,10 @@ $foundation-dir: "foundation";
}
}
-$icons: wifi "\\600", wifi-hotspot "\\601", weather "\\602";
+$icons:
+ wifi "\\600",
+ wifi-hotspot "\\601",
+ weather "\\602";
@each $icon in $icons {
.icon-#{nth($icon, 1)},
@@ -2251,7 +2269,12 @@ $icons: wifi "\\600", wifi-hotspot "\\601", weather "\\602";
$last: nth($juggler, length($juggler));
$x: if($last%2==0, 1/2, 3/2);
$new: pow($last, $x);
-$sequence: 1, 1 1, 2 1, 1 2 1 1, 1 1 1 2 2 1;
+$sequence:
+ 1,
+ 1 1,
+ 2 1,
+ 1 2 1 1,
+ 1 1 1 2 2 1;
$new-entry: ();
$new-entry: ();
$new-entry: ();
@@ -2467,7 +2490,8 @@ a {
color: red; // Comment
}
-$my-list: "foo",
+$my-list:
+ "foo",
// Comment
"bar"; // Comment
@@ -3926,17 +3950,29 @@ $list-comma: "item-1", "item-2", "item-3";
$list-comma: "item-1", "item-2", "item-3";
$list-comma: "item-1", "item-2", "item-3";
$list-comma: "item-1", "item-2", "item-3";
-$list: "item-1.1" "item-1.2" "item-1.3", "item-2.1" "item-2.2" "item-2.3",
+$list:
+ "item-1.1" "item-1.2" "item-1.3",
+ "item-2.1" "item-2.2" "item-2.3",
"item-3.1" "item-3.2" "item-3.3";
-$list: "item-1.1" "item-1.2" "item-1.3", "item-2.1" "item-2.2" "item-2.3",
+$list:
+ "item-1.1" "item-1.2" "item-1.3",
+ "item-2.1" "item-2.2" "item-2.3",
"item-3.1" "item-3.2" "item-3.3";
-$list: "item-1.1" "item-1.2" "item-1.3", "item-2.1" "item-2.2" "item-2.3",
+$list:
+ "item-1.1" "item-1.2" "item-1.3",
+ "item-2.1" "item-2.2" "item-2.3",
"item-3.1" "item-3.2" "item-3.3";
-$list: "item-1.1" "item-1.2" "item-1.3", "item-2.1" "item-2.2" "item-2.3",
+$list:
+ "item-1.1" "item-1.2" "item-1.3",
+ "item-2.1" "item-2.2" "item-2.3",
"item-3.1" "item-3.2" "item-3.3";
-$list: "item-1.1" "item-1.2" "item-1.3", "item-2.1" "item-2.2" "item-2.3",
+$list:
+ "item-1.1" "item-1.2" "item-1.3",
+ "item-2.1" "item-2.2" "item-2.3",
"item-3.1" "item-3.2" "item-3.3";
-$list: "item-1.1" "item-1.2" "item-1.3", "item-2.1" "item-2.2" "item-2.3",
+$list:
+ "item-1.1" "item-1.2" "item-1.3",
+ "item-2.1" "item-2.2" "item-2.3",
"item-3.1" "item-3.2" "item-3.3";
$list: (
("item-1.1", "item-1.2", "item-1.3"),
@@ -4010,7 +4046,10 @@ $space-scale: (0, "0") (0.25, "0-25") (0.5, "0-5") (0.75, "0-75") (1, "1")
@error "Very long long long long long long long long long long long long long line Error (#{$message}).";
@error "Very long long long long long long long long long long long long long line Error (#{$message}).";
-$buttonConfig: "save" 50px, "cancel" 50px, "help" 100px;
+$buttonConfig:
+ "save" 50px,
+ "cancel" 50px,
+ "help" 100px;
$locale: "en_us";
html[lang="#{$locale}"] {
@@ -4344,7 +4383,10 @@ $foundation-dir: "foundation";
}
}
-$icons: wifi "\\600", wifi-hotspot "\\601", weather "\\602";
+$icons:
+ wifi "\\600",
+ wifi-hotspot "\\601",
+ weather "\\602";
@each $icon in $icons {
.icon-#{nth($icon, 1)},
@@ -4391,7 +4433,12 @@ $icons: wifi "\\600", wifi-hotspot "\\601", weather "\\602";
$last: nth($juggler, length($juggler));
$x: if($last%2==0, 1/2, 3/2);
$new: pow($last, $x);
-$sequence: 1, 1 1, 2 1, 1 2 1 1, 1 1 1 2 2 1;
+$sequence:
+ 1,
+ 1 1,
+ 2 1,
+ 1 2 1 1,
+ 1 1 1 2 2 1;
$new-entry: ();
$new-entry: ();
$new-entry: ();
@@ -4607,7 +4654,8 @@ a {
color: red; // Comment
}
-$my-list: "foo",
+$my-list:
+ "foo",
// Comment
"bar"; // Comment
diff --git a/tests/format/stylefmt-repo/font-face/__snapshots__/jsfmt.spec.js.snap b/tests/format/stylefmt-repo/font-face/__snapshots__/jsfmt.spec.js.snap
--- a/tests/format/stylefmt-repo/font-face/__snapshots__/jsfmt.spec.js.snap
+++ b/tests/format/stylefmt-repo/font-face/__snapshots__/jsfmt.spec.js.snap
@@ -12,7 +12,9 @@ printWidth: 80
@font-face {
font-family: "HelveticaNeueW02-45Ligh";
src: url("/fonts/pictos-web.eot");
- src: local("☺"), url("/fonts/pictos-web.woff") format("woff"),
+ src:
+ local("☺"),
+ url("/fonts/pictos-web.woff") format("woff"),
url("/fonts/pictos-web.ttf") format("truetype"),
url("/fonts/pictos-web.svg#webfontIyfZbseF") format("svg");
font-weight: normal;
diff --git a/tests/format/stylefmt-repo/font-shorthand/__snapshots__/jsfmt.spec.js.snap b/tests/format/stylefmt-repo/font-shorthand/__snapshots__/jsfmt.spec.js.snap
--- a/tests/format/stylefmt-repo/font-shorthand/__snapshots__/jsfmt.spec.js.snap
+++ b/tests/format/stylefmt-repo/font-shorthand/__snapshots__/jsfmt.spec.js.snap
@@ -15,7 +15,9 @@ printWidth: 80
.class {
font: normal normal 24px/1 "myfont";
font: normal normal normal 12px/20px myfont;
- font: normal 300 0.875em/1.3 "myfont", sans-serif;
+ font:
+ normal 300 0.875em/1.3 "myfont",
+ sans-serif;
}
================================================================================
| When box-shadow has multiple values (separated by comma) it'd be prettier to put each value on a separate line
**Prettier 1.16.4**
[Playground link](https://prettier.io/playground/#N4Igxg9gdgLgprEAuEAjCAPAtAZwBYCGAJhAO5IA6UABNQAwAOG1AjE-RwMQBmvANFVqNmAJnZ0ucKQG4qIPiAgMYAS2g5koAgCdtZAAo6EGlAQBuEFUXlptBMAGs4MAMoN7KqAHNkMbQFc4BTwYAFsAGwB1PBV4HHcwOBdjWJUzWIBPZHAcDQVPHDhtGH07L1CCZG4CcMKFACscDAAhO0dnFwJQuAAZTzgqmrqQRowXTy9wuABFfwh4QdqgkHdtQu1ssFybBm1PGEirGDxkAA46BV2IQsi7BmzduHWzAYVtOABHfxV30oJyypIapLBSFUIqXwBZY4CZTWbzAZAobLGAEVCHIjHZAiBR+AgqcITADCEFCFWyUGgrxA-kKABU0SZgYUAL4soA)
```sh
--parser css
```
**Input:**
```css
box-shadow:
0px 1px 0 0 #fff,
0px 2px 0 0 #eee;
```
**Output:**
```css
box-shadow: 0px 1px 0 0 #fff, 0px 2px 0 0 #eee;
```
**Expected behavior:**
```css
box-shadow:
0px 1px 0 0 #fff,
0px 2px 0 0 #eee;
```
When each value is on a separate line, the whole value feels significantly easier to read.
Always wrap certain CSS prop values (box-shadow, text-shadow, etc)
## Description
Always wrap specific CSS property values (box-shadow, transition, background-image, text-shadow, background). Fixes #6024
## Checklist
- [ ] I’ve added tests to confirm my change works.
- [ ] (If changing the API or CLI) I’ve documented the changes I’ve made (in the `docs/` directory).
- [ ] (If the change is user-facing) I’ve added my changes to `changelog_unreleased/*/XXXX.md` file following `changelog_unreleased/TEMPLATE.md`.
- [x] I’ve read the [contributing guidelines](https://github.com/prettier/prettier/blob/main/CONTRIBUTING.md).
**✨[Try the playground for this PR](https://prettier.io/playground-redirect)✨**
| I would love something like this!
I agree with this. `box-shadow` with multiple values looks better with each value on a separate line.
How about other properties like `background`, `background-image`, `text-shadow`, `@font-face#src`?
**background-image** (from [Lea Verou Patterns](http://projects.verou.me/css3patterns/#checkerboard))
```
background-image:
linear-gradient(45deg, black 25%, transparent 25%, transparent 75%, black 75%, black),
linear-gradient(45deg, black 25%, transparent 25%, transparent 75%, black 75%, black);
```
**text-shadow** (from [MDN docs](https://developer.mozilla.org/en-US/docs/Web/CSS/text-shadow))
```
text-shadow:
1px 1px 2px red,
0 0 1em blue,
0 0 0.2em blue;
```
**font-face** (from [MDN docs](https://developer.mozilla.org/en-US/docs/Web/CSS/@font-face))
```
@font-face {
font-family: "Open Sans";
src:
url("/fonts/OpenSans-Regular-webfont.woff2") format("woff2"),
url("/fonts/OpenSans-Regular-webfont.woff") format("woff");
}
```
I would argue that these all look prettier with separate lines when they are separated with commas, particularly when there are more than 2 values.
I think such a rule will need some consideration for which CSS properties are enhanced by splitting across lines and which ones are not. `font-family` for example, looks worse when split:
**font-family**
```
font-family:
'Helvetica Neue',
Helvetica,
system-ui,
sans-serif;
```
Could we implement this please as soon as possible. I think it's time to improve this ugly style behavior. It's one of the worst behavior in Prettier.
I prefer always multiline when multiple values.
**Bad**
```css
box-shadow: 0 0 10px black, 0 0 0 1px black;
```
**Good**
```css
box-shadow:
0 0 10px black,
0 0 0 1px black;
```
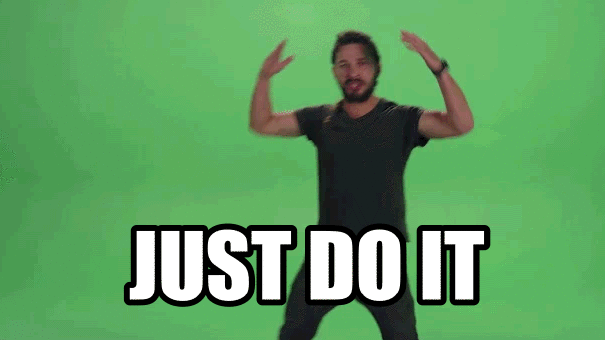
And variables:
```scss
$shadow:
0 0 0 1px #5b9dd9,
0 0 2px 1px rgba(30, 140, 190, 0.8);
```
From: https://github.com/stylelint-scss/stylelint-scss/blob/HEAD/src/rules/dollar-variable-colon-newline-after/README.md
I have a proof-of-concept PR that implements this here: https://github.com/prettier/prettier/pull/13260. It hard-codes specific properties to always wrap if they have more than one value. (Notably, I skipped font-family since as another commenter pointed out it doesn't look great wrapped.)
Note that since it looks at the property, it won't apply to SASS variables.
It seems like this might need some more discussion as to the preferred behavior - I've narrowed down the two primary decisions that would need to be made.
### 1. Always wrap lists
We can choose to always use the new wrapping behavior for lists with two or more elements, or only do it if the line would wrap anyways.
**1.a - Always wrapping lists:**
```css
.selector {
box-shadow:
0 0 0 1px rgba(0, 0, 0, 0.1),
0 1px 2px 0 rgba(0, 0, 0, 0.2);
box-shadow:
0 0 0 1px rgba(0, 0, 0, 0.1),
0 1px 2px 0 rgba(0, 0, 0, 0.2),
0 1px 2px 0 rgba(0, 0, 0, 0.2);
}
```
**1.b - Only use new wrapping if line needs to wrap:**
```css
.selector {
box-shadow: 0 0 0 1px rgba(0, 0, 0, 0.1), 0 1px 2px 0 rgba(0, 0, 0, 0.2);
box-shadow:
0 0 0 1px rgba(0, 0, 0, 0.1),
0 1px 2px 0 rgba(0, 0, 0, 0.2),
0 1px 2px 0 rgba(0, 0, 0, 0.2);
}
```
### 2. Only wrap certain properties
Orthogonal from the above, we can choose to apply this new wrapping behavior only for certain properties (like `box-shadow`, `background-image`, etc) while keeping the existing behavior for others (notably, `font-family`, but also `transition-property`). This makes sense if those properties often have many short elements, but makes the behavior inconsistent and possibly unexpected.
**2.a - Only apply new wrapping to certain properties:**
```css
.selector {
box-shadow:
0 0 0 1px rgba(0, 0, 0, 0.1),
0 1px 2px 0 rgba(0, 0, 0, 0.2),
0 1px 2px 0 rgba(0, 0, 0, 0.2);
font-family: -apple-system, BlinkMacSystemFont,
avenir next, avenir, segoe ui, helvetica neue, helvetica,
Cantarell, Ubuntu, roboto, noto, arial, sans-serif;
transition-property: opacity, color, transform, box-shadow,
margin, padding;
}
```
**2.b - Apply new wrapping behavior to every property:**
```css
.selector {
box-shadow:
0 0 0 1px rgba(0, 0, 0, 0.1),
0 1px 2px 0 rgba(0, 0, 0, 0.2),
0 1px 2px 0 rgba(0, 0, 0, 0.2);
font-family:
-apple-system,
BlinkMacSystemFont,
avenir next,
avenir,
segoe ui,
helvetica neue,
helvetica,
Cantarell,
Ubuntu,
roboto,
noto,
arial,
sans-serif;
transition-property:
opacity,
color,
transform,
box-shadow,
margin,
padding;
}
```
Personally, I prefer "always".
Another thing, where should we put `!important`?
> Personally, I prefer "always".
Thanks @fisker - can you clarify here? You mean option 1.a and 2.b?
> Another thing, where should we put `!important`?
Right now (in the PR), it goes on the same line as the last item in the property list.
1.a, only personal opinion, we need more discussion.
How about "wrap if any list item contains more than 2 spaces"?
That could work as well as an alternative for #2 where it doesn't require special-casing the property names.
Let's do it (https://github.com/prettier/prettier/issues/6024#issuecomment-1216191051) then, shall we?
👍 For this, we need more tests.
> For this, we need more tests.
Definitely - I also have a small thing to fix in the implementation first. Just wanted to make sure that this was a change that would be accepted before fixing up those additional things.
@fisker I think this PR needs a design decision before I can move forward - I'd be curious to hear your thoughts on https://github.com/prettier/prettier/issues/6024#issuecomment-1209580521.
This is much needed | "2023-01-20T13:00:08Z" | 2.9 | [] | [
"tests/format/scss/scss/jsfmt.spec.js",
"tests/format/scss/comments/jsfmt.spec.js",
"tests/format/less/less/jsfmt.spec.js",
"tests/format/less/comments/jsfmt.spec.js",
"tests/format/css/fill-value/jsfmt.spec.js",
"tests/format/css/indent/jsfmt.spec.js",
"tests/format/css/atrule/jsfmt.spec.js",
"tests/format/css/comments/jsfmt.spec.js",
"tests/format/stylefmt-repo/font-shorthand/jsfmt.spec.js",
"tests/format/stylefmt-repo/font-face/jsfmt.spec.js"
] | JavaScript | [] | [
"https://prettier.io/playground/#N4Igxg9gdgLgprEAuEAjCAPAtAZwBYCGAJhAO5IA6UABNQAwAOG1AjE-RwMQBmvANFVqNmAJnZ0ucKQG4qIPiAgMYAS2g5koAgCdtZAAo6EGlAQBuEFUXlptBMAGs4MAMoN7KqAHNkMbQFc4BTwYAFsAGwB1PBV4HHcwOBdjWJUzWIBPZHAcDQVPHDhtGH07L1CCZG4CcMKFACscDAAhO0dnFwJQuAAZTzgqmrqQRowXTy9wuABFfwh4QdqgkHdtQu1ssFybBm1PGEirGDxkAA46BV2IQsi7BmzduHWzAYVtOABHfxV30oJyypIapLBSFUIqXwBZY4CZTWbzAZAobLGAEVCHIjHZAiBR+AgqcITADCEFCFWyUGgrxA-kKABU0SZgYUAL4soA",
"https://prettier.io/playground-redirect"
] |
prettier/prettier | 14,262 | prettier__prettier-14262 | [
"14227"
] | 37fb53acf33a20379a93bfecea8242c03ce0a4fc | diff --git a/src/language-js/comments.js b/src/language-js/comments.js
--- a/src/language-js/comments.js
+++ b/src/language-js/comments.js
@@ -31,6 +31,7 @@ const {
} = require("./utils/index.js");
const { locStart, locEnd } = require("./loc.js");
const isBlockComment = require("./utils/is-block-comment.js");
+const isTypeCastComment = require("./utils/is-type-cast-comment.js");
/**
* @typedef {import("./types/estree").Node} Node
@@ -955,21 +956,6 @@ function getCommentChildNodes(node, options) {
}
}
-/**
- * @param {Comment} comment
- * @returns {boolean}
- */
-function isTypeCastComment(comment) {
- return (
- isBlockComment(comment) &&
- comment.value[0] === "*" &&
- // TypeScript expects the type to be enclosed in curly brackets, however
- // Closure Compiler accepts types in parens and even without any delimiters at all.
- // That's why we just search for "@type".
- /@type\b/.test(comment.value)
- );
-}
-
/**
* @param {AstPath} path
* @returns {boolean}
@@ -1005,7 +991,6 @@ module.exports = {
handleOwnLineComment,
handleEndOfLineComment,
handleRemainingComment,
- isTypeCastComment,
getCommentChildNodes,
willPrintOwnComments,
};
diff --git a/src/language-js/utils/is-type-cast-comment.js b/src/language-js/utils/is-type-cast-comment.js
--- a/src/language-js/utils/is-type-cast-comment.js
+++ b/src/language-js/utils/is-type-cast-comment.js
@@ -16,8 +16,8 @@ function isTypeCastComment(comment) {
comment.value[0] === "*" &&
// TypeScript expects the type to be enclosed in curly brackets, however
// Closure Compiler accepts types in parens and even without any delimiters at all.
- // That's why we just search for "@type".
- /@type\b/.test(comment.value)
+ // That's why we just search for "@type" and "@satisfies".
+ /@(?:type|satisfies)\b/.test(comment.value)
);
}
| diff --git a/tests/format/js/comments-closure-typecast/__snapshots__/jsfmt.spec.js.snap b/tests/format/js/comments-closure-typecast/__snapshots__/jsfmt.spec.js.snap
--- a/tests/format/js/comments-closure-typecast/__snapshots__/jsfmt.spec.js.snap
+++ b/tests/format/js/comments-closure-typecast/__snapshots__/jsfmt.spec.js.snap
@@ -571,6 +571,24 @@ const objectWithComment2 = /** @type MyType */ (
================================================================================
`;
+exports[`satisfies.js format 1`] = `
+====================================options=====================================
+parsers: ["babel"]
+printWidth: 80
+ | printWidth
+=====================================input======================================
+module.exports = /** @satisfies {Record<string, string>} */ ({
+ hello: 1337,
+});
+
+=====================================output=====================================
+module.exports = /** @satisfies {Record<string, string>} */ ({
+ hello: 1337,
+});
+
+================================================================================
+`;
+
exports[`styled-components.js format 1`] = `
====================================options=====================================
parsers: ["babel"]
diff --git a/tests/format/js/comments-closure-typecast/satisfies.js b/tests/format/js/comments-closure-typecast/satisfies.js
new file mode 100644
--- /dev/null
+++ b/tests/format/js/comments-closure-typecast/satisfies.js
@@ -0,0 +1,3 @@
+module.exports = /** @satisfies {Record<string, string>} */ ({
+ hello: 1337,
+});
| Allow parentheses when JSDoc to allow intellisense
**Prettier 2.8.3**
[Playground link](https://prettier.io/playground/#N4Igxg9gdgLgprEAuEBbCATArgGzgOjgA8AHCAJxgGcACAXhoHoAqZmgASoEMYBLKgGa84tYACU4kchgA8VGOV5QA5gBoa8xSoB8AXxrNGNABTAAOlBo0AFnBw4ISGgEYAzK4Dsqi7oCUAbhBVEAgSPmgqZFAucnIIAHcABRiESJQuHHiuAE9I4IAjci4wAGs4GABlLlQ4ABklOGQBDKo4AqLS8oqSYqVlZAUsNpA4VHy4DAwJ2q4VLC5lOAAxClQePhVkEC4sGAggkGsYVBwAdWteeCoesDgK1MveADdL7K2wKjyQJVbKRKLlGsmi1hgArKhECp9PAARSwEHgwJwrWCPXIvy2+S44xwBxIWhgp14GBg1mQAA4AAyouKtU5FEhbfEiODkJ6NYIAR3h8H+oTS2yoAFooHAJhMDuQ4NzeFL-gsgUhmsjhq1ULwBuQhsEqNC4ABBGAKXj5XZwRKs+qipEokC6lSwnmNJUg4IwbFEklkpAAJjdRV4OD6AGEIKhFSMqABWA5YVoAFWxaWVtqeQwAklAprAKmBFGF9VmKjBsngbXBdLogA)
<!-- prettier-ignore -->
```sh
--parser babel
```
**Input:**
<!-- prettier-ignore -->
```jsx
module.exports = /** @satisfies {Record<string, string>} */ ({
hello: 1337,
});
```
**Output:**
<!-- prettier-ignore -->
```jsx
module.exports = /** @satisfies {Record<string, string>} */ {
hello: 1337,
};
```
**Expected behavior:**
Keep those parentheses to not break intellisense.
```jsx
module.exports = /** @satisfies {Record<string, string>} */ ({
hello: 1337,
});
```
Here is an example of using `@satisfies` jsdoc. https://github.com/microsoft/TypeScript/pull/51753
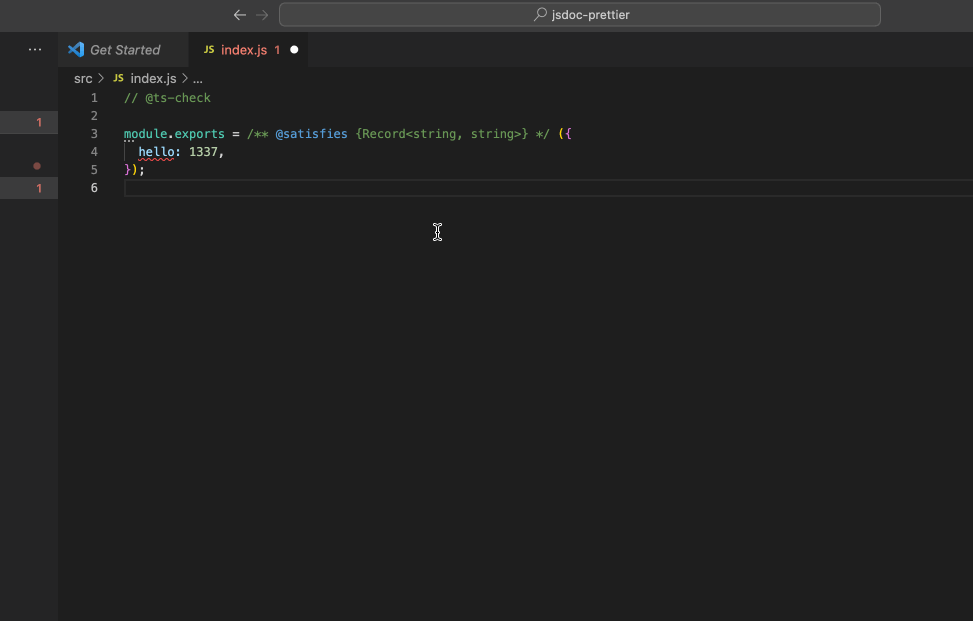
| It becomes more and more popular to include JSDoc for configs etc. It really improves developer experience to not have to jump into documentation pages for property names and also prevents typos.
Here is for instance Next.js official documentation.
https://nextjs.org/docs/api-reference/next.config.js/introduction
```js
/**
* @type {import('next').NextConfig}
*/
const nextConfig = {
/* config options here */
}
module.exports = nextConfig
```
At the moment I have to disable prettier because it removes those parentheses so I wasn't able to use `@satisfies` JSDoc when I had prettier running.
`@type` and `@satisfies` have different use cases. So it's sad if Prettier prevents us from using it.
How do we start a discussion or get some traction on this? What alternatives do we have?
I really want to continue use Prettier it's amazing! I also want to use best practices with JSDoc. Especially when it improves intellisense.
I have no experience with the Prettier team or this codebase. I only have love for what you guys created.
Is there something that I can help with? I don't know how your process work... :) | "2023-01-30T04:28:40Z" | 2.9 | [] | [
"tests/format/js/comments-closure-typecast/jsfmt.spec.js"
] | JavaScript | [] | [
"https://prettier.io/playground/#N4Igxg9gdgLgprEAuEBbCATArgGzgOjgA8AHCAJxgGcACAXhoHoAqZmgASoEMYBLKgGa84tYACU4kchgA8VGOV5QA5gBoa8xSoB8AXxrNGNABTAAOlBo0AFnBw4ISGgEYAzK4Dsqi7oCUAbhBVEAgSPmgqZFAucnIIAHcABRiESJQuHHiuAE9I4IAjci4wAGs4GABlLlQ4ABklOGQBDKo4AqLS8oqSYqVlZAUsNpA4VHy4DAwJ2q4VLC5lOAAxClQePhVkEC4sGAggkGsYVBwAdWteeCoesDgK1MveADdL7K2wKjyQJVbKRKLlGsmi1hgArKhECp9PAARSwEHgwJwrWCPXIvy2+S44xwBxIWhgp14GBg1mQAA4AAyouKtU5FEhbfEiODkJ6NYIAR3h8H+oTS2yoAFooHAJhMDuQ4NzeFL-gsgUhmsjhq1ULwBuQhsEqNC4ABBGAKXj5XZwRKs+qipEokC6lSwnmNJUg4IwbFEklkpAAJjdRV4OD6AGEIKhFSMqABWA5YVoAFWxaWVtqeQwAklAprAKmBFGF9VmKjBsngbXBdLogA"
] |
Subsets and Splits
No community queries yet
The top public SQL queries from the community will appear here once available.